基于yolo v5与Deep Sort进行车辆以及速度检测与目标跟踪实战
项目实验结果展示:
基于yolo v5与Deep Sort进行车辆以及速度检测与目标跟踪实战——项目可以私聊
该项目可以作为毕业设计,以及企业级的项目开发,主要包含了车辆的目标检测、目标跟踪以及车辆的速度计算,同样可以进行二次开发。
这里附上主要的检测代码
import torch
import numpy as np
from models.experimental import attempt_load
from utils.general import non_max_suppression, scale_coords
from utils.torch_utils import select_device
from utils.datasets import letterbox
import cv2
from deep_sort.utils.parser import get_config
from deep_sort.deep_sort import DeepSort
from haversine import haversine, Unit
from sys import platform as _platformclass Detector:"""yolo目标检测"""def __init__(self):self.img_size = 1280self.conf_thres = 0.5self.iou_thres=0.5# 目标检测权重self.weights = 'weights/highway_m_300.pt'self.device = '0' if torch.cuda.is_available() else 'cpu'self.device = select_device(self.device)model = attempt_load(self.weights, map_location=self.device)model.to(self.device).eval()# 判断系统,支持MACOS 和 windowsif _platform == "darwin":# MAC OS Xmodel.float()else:# Windowsmodel.half()# self.m = modelself.names = model.module.names if hasattr(model, 'module') else model.names# 图片预处理def preprocess(self, img):img = letterbox(img, new_shape=self.img_size)[0]img = img[:, :, ::-1].transpose(2, 0, 1)img = np.ascontiguousarray(img)img = torch.from_numpy(img).to(self.device)if _platform == "darwin":# MAC OS Ximg = img.float() else:# Windowsimg = img.half()img /= 255.0 # 图像归一化if img.ndimension() == 3:img = img.unsqueeze(0)return img# 目标检测def yolo_detect(self, im):img = self.preprocess(im)pred = self.m(img, augment=False)[0]pred = pred.float()pred = non_max_suppression(pred, self.conf_thres, self.iou_thres )pred_boxes = []for det in pred:if det is not None and len(det):det[:, :4] = scale_coords(img.shape[2:], det[:, :4], im.shape).round()for *x, conf, cls_id in det:lbl = self.names[int(cls_id)]x1, y1 = int(x[0]), int(x[1])x2, y2 = int(x[2]), int(x[3])pred_boxes.append((x1, y1, x2, y2, lbl, conf))return pred_boxesclass Tracker:"""deepsort追踪"""def __init__(self):cfg = get_config()cfg.merge_from_file("deep_sort/configs/deep_sort.yaml")self.deepsort = DeepSort(cfg.DEEPSORT.REID_CKPT,max_dist=cfg.DEEPSORT.MAX_DIST, min_confidence=cfg.DEEPSORT.MIN_CONFIDENCE,nms_max_overlap=cfg.DEEPSORT.NMS_MAX_OVERLAP, max_iou_distance=cfg.DEEPSORT.MAX_IOU_DISTANCE,max_age=cfg.DEEPSORT.MAX_AGE, n_init=cfg.DEEPSORT.N_INIT, nn_budget=cfg.DEEPSORT.NN_BUDGET,use_cuda=True)def update_tracker(self,image, yolo_bboxes):bbox_xywh = []confs = []clss = []for x1, y1, x2, y2, cls_id, conf in yolo_bboxes:obj = [int((x1+x2)/2), int((y1+y2)/2),x2-x1, y2-y1]bbox_xywh.append(obj)confs.append(conf)clss.append(cls_id)xywhs = torch.Tensor(bbox_xywh)confss = torch.Tensor(confs)#更新追踪结果outputs = self.deepsort.update(xywhs, confss, clss, image)bboxes2draw = []for value in list(outputs):x1, y1, x2, y2, cls_, track_id = valuebboxes2draw.append((x1, y1, x2, y2, cls_, track_id))return bboxes2drawclass PixelMapper(object):"""Create an object for converting pixels to geographic coordinates,using four points with known locations which form a quadrilteral in both planesParameters----------pixel_array : (4,2) shape numpy arrayThe (x,y) pixel coordinates corresponding to the top left, top right, bottom right, bottom leftpixels of the known regionlonlat_array : (4,2) shape numpy arrayThe (lon, lat) coordinates corresponding to the top left, top right, bottom right, bottom leftpixels of the known region"""def __init__(self, pixel_array, lonlat_array):assert pixel_array.shape==(4,2), "Need (4,2) input array"assert lonlat_array.shape==(4,2), "Need (4,2) input array"self.M = cv2.getPerspectiveTransform(np.float32(pixel_array),np.float32(lonlat_array))self.invM = cv2.getPerspectiveTransform(np.float32(lonlat_array),np.float32(pixel_array))def pixel_to_lonlat(self, pixel):"""Convert a set of pixel coordinates to lon-lat coordinatesParameters----------pixel : (N,2) numpy array or (x,y) tupleThe (x,y) pixel coordinates to be convertedReturns-------(N,2) numpy arrayThe corresponding (lon, lat) coordinates"""if type(pixel) != np.ndarray:pixel = np.array(pixel).reshape(1,2)assert pixel.shape[1]==2, "Need (N,2) input array" pixel = np.concatenate([pixel, np.ones((pixel.shape[0],1))], axis=1)lonlat = np.dot(self.M,pixel.T)return (lonlat[:2,:]/lonlat[2,:]).Tdef lonlat_to_pixel(self, lonlat):"""Convert a set of lon-lat coordinates to pixel coordinatesParameters----------lonlat : (N,2) numpy array or (x,y) tupleThe (lon,lat) coordinates to be convertedReturns-------(N,2) numpy arrayThe corresponding (x, y) pixel coordinates"""if type(lonlat) != np.ndarray:lonlat = np.array(lonlat).reshape(1,2)assert lonlat.shape[1]==2, "Need (N,2) input array" lonlat = np.concatenate([lonlat, np.ones((lonlat.shape[0],1))], axis=1)pixel = np.dot(self.invM,lonlat.T)return (pixel[:2,:]/pixel[2,:]).Tclass SpeedEstimate:def __init__(self):# 配置相机画面与地图的映射点,需要根据自己镜头和地图上的点重新配置quad_coords = {"lonlat": np.array([[30.221866, 120.287402], # top left[30.221527,120.287632], # top right[30.222098,120.285806], # bottom left[30.221805,120.285748] # bottom right]),"pixel": np.array([[196,129],# top left[337,111], # top right[12,513], # bottom left[530,516] # bottom right])}self.pm = PixelMapper(quad_coords["pixel"], quad_coords["lonlat"])def pixel2lonlat(self,x,y):# 像素坐标转为经纬度return self.pm.pixel_to_lonlat((x,y))[0]def pixelDistance(self,pa_x,pa_y,pb_x,pb_y):# 相机画面两点在地图上实际的距离lonlat_a = self.pm.pixel_to_lonlat((pa_x,pa_y))lonlat_b = self.pm.pixel_to_lonlat((pb_x,pb_y))lonlat_a = tuple(lonlat_a[0])lonlat_b = tuple(lonlat_b[0])return haversine(lonlat_a, lonlat_b, unit='m')
项目需求+V: gldz_super
相关文章:

基于yolo v5与Deep Sort进行车辆以及速度检测与目标跟踪实战
项目实验结果展示: 基于yolo v5与Deep Sort进行车辆以及速度检测与目标跟踪实战——项目可以私聊 该项目可以作为毕业设计,以及企业级的项目开发,主要包含了车辆的目标检测、目标跟踪以及车辆的速度计算,同样可以进行二次开发。 …...
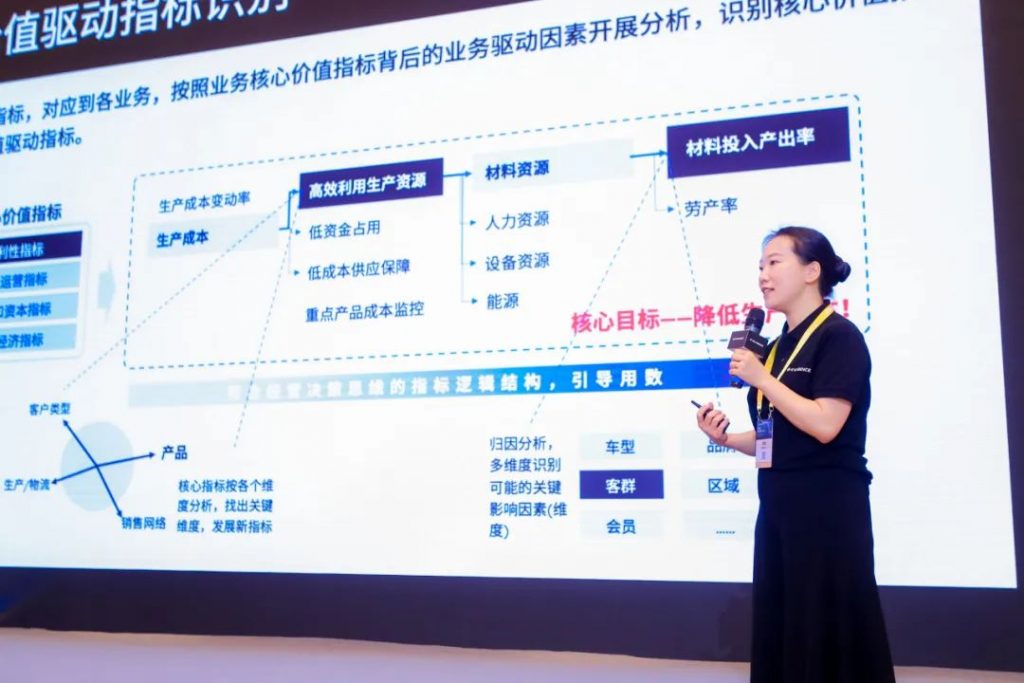
以指标驱动,保险、零售、制造企业开启精益敏捷运营的新范式
近日,以“释放数智生产力”为主题的 Kyligence 用户大会在上海前滩香格里拉大酒店成功举行。大会包含上午的主论坛和下午的 4 场平行论坛,并举办了闭门会议、Open Day 等活动。来自金融、零售、制造、医药等行业的客户及合作伙伴带来了超过 23 场主题演讲…...
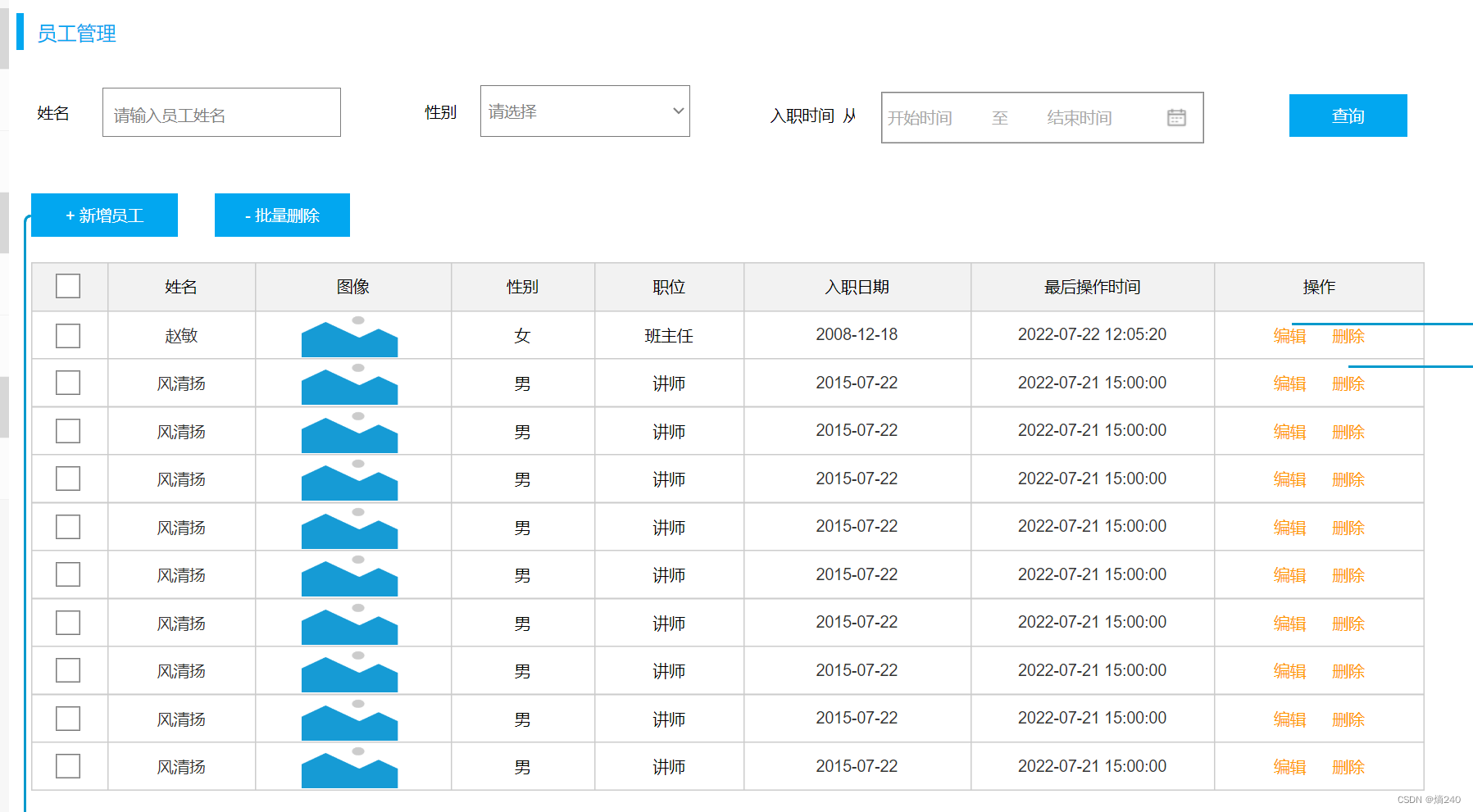
MyBatis-动态SQL-foreach
目录 标签有以下常用属性: 小结 <froeach> <foreach>标签有以下常用属性: collection:指定要迭代的集合或数组的参数名(遍历的对象)。item:指定在迭代过程中的每个元素的别名(遍历…...
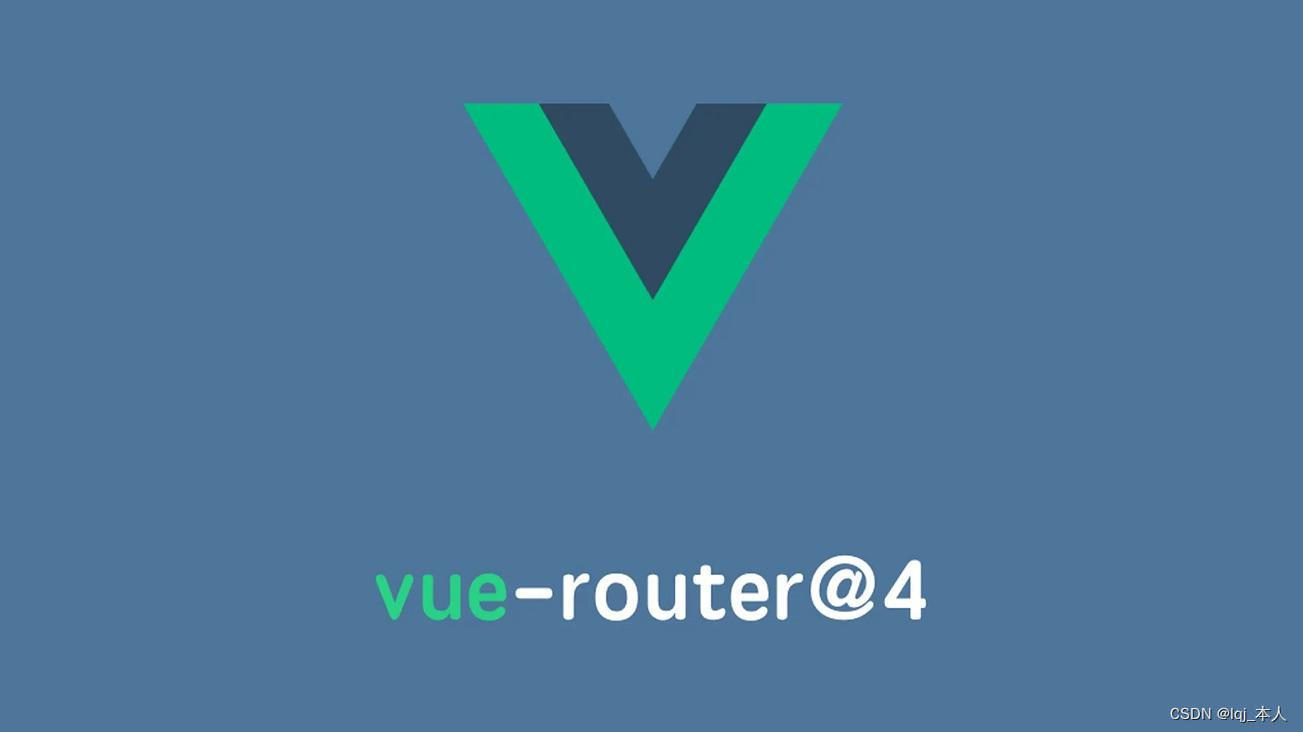
VUE框架:vue2转vue3全面细节总结(3)路由组件传参
大家好,我是csdn的博主:lqj_本人 这是我的个人博客主页: lqj_本人_python人工智能视觉(opencv)从入门到实战,前端,微信小程序-CSDN博客 最新的uniapp毕业设计专栏也放在下方了: https://blog.csdn.net/lbcy…...
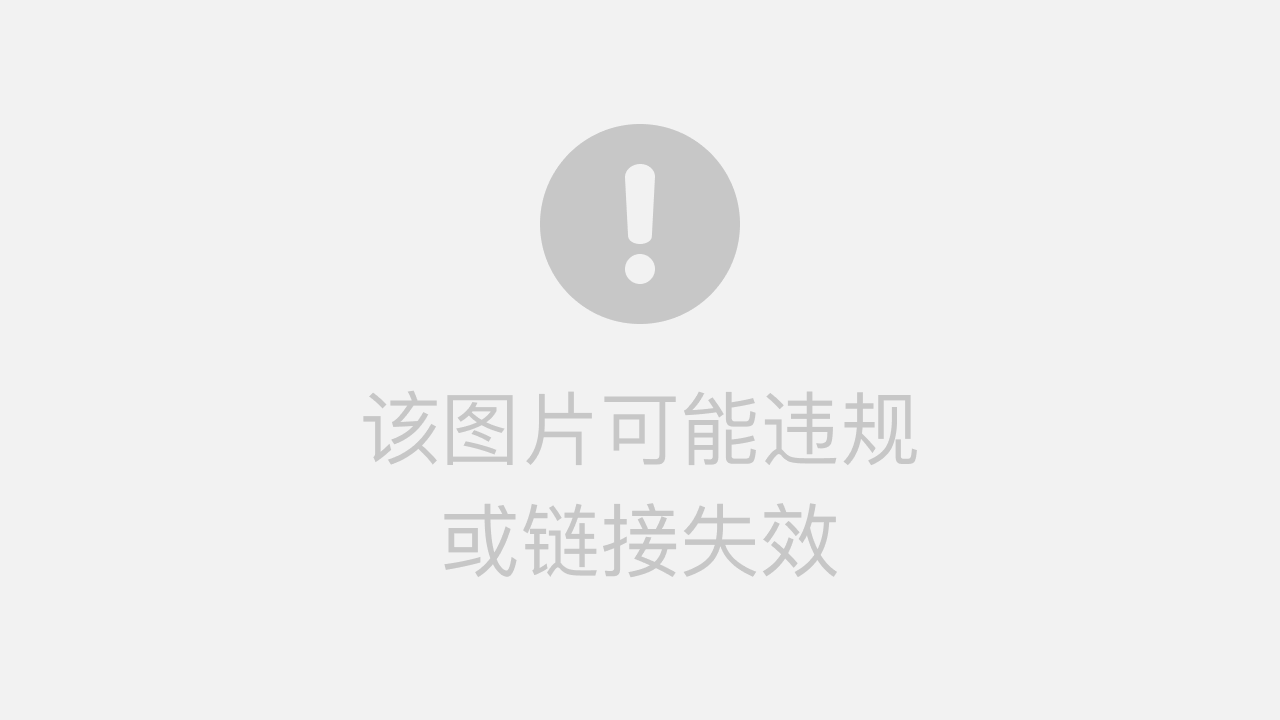
音视频技术开发周刊 | 305
每周一期,纵览音视频技术领域的干货。 新闻投稿:contributelivevideostack.com。 大神回归学界:何恺明宣布加入 MIT 「作为一位 FAIR 研究科学家,我将于 2024 年加入麻省理工学院(MIT)电气工程与计算机科学…...

vue 图片base64转化
import html2canvas from ‘html2canvas’ html2canvas(canvasDom, options).then(canvas > { //此时的图片是base64格式的,我们将图片格式转换一下 let type ‘png’; let imgData canvas.toDataURL(type); // 照片格式处理 let _fixType function(type) { …...

TS学习03-类
类 calss A {name: stringconstructor(name:string) {this.name name}greet() {return hello, this.name} } let people new A(RenNing)继承 子类是一个派生类,他派生自父类(基类),通过 extends关键字 派生类通常被称作 子类…...
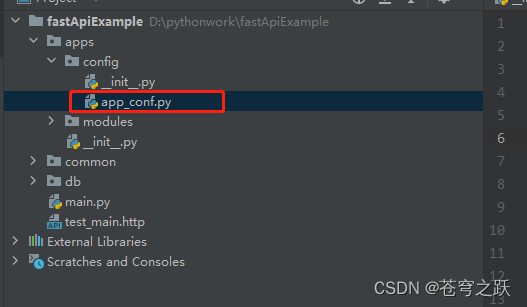
FastAPI(七)应用配置
目录 一、在apps下新建文件夹config 二、新建配置文件app_conf.py 一、在apps下新建文件夹config 二、新建配置文件app_conf.py from functools import lru_cachefrom pydantic.v1 import BaseSettingsclass AppConfig(BaseSettings):app_name: str "Windows10 插件&qu…...
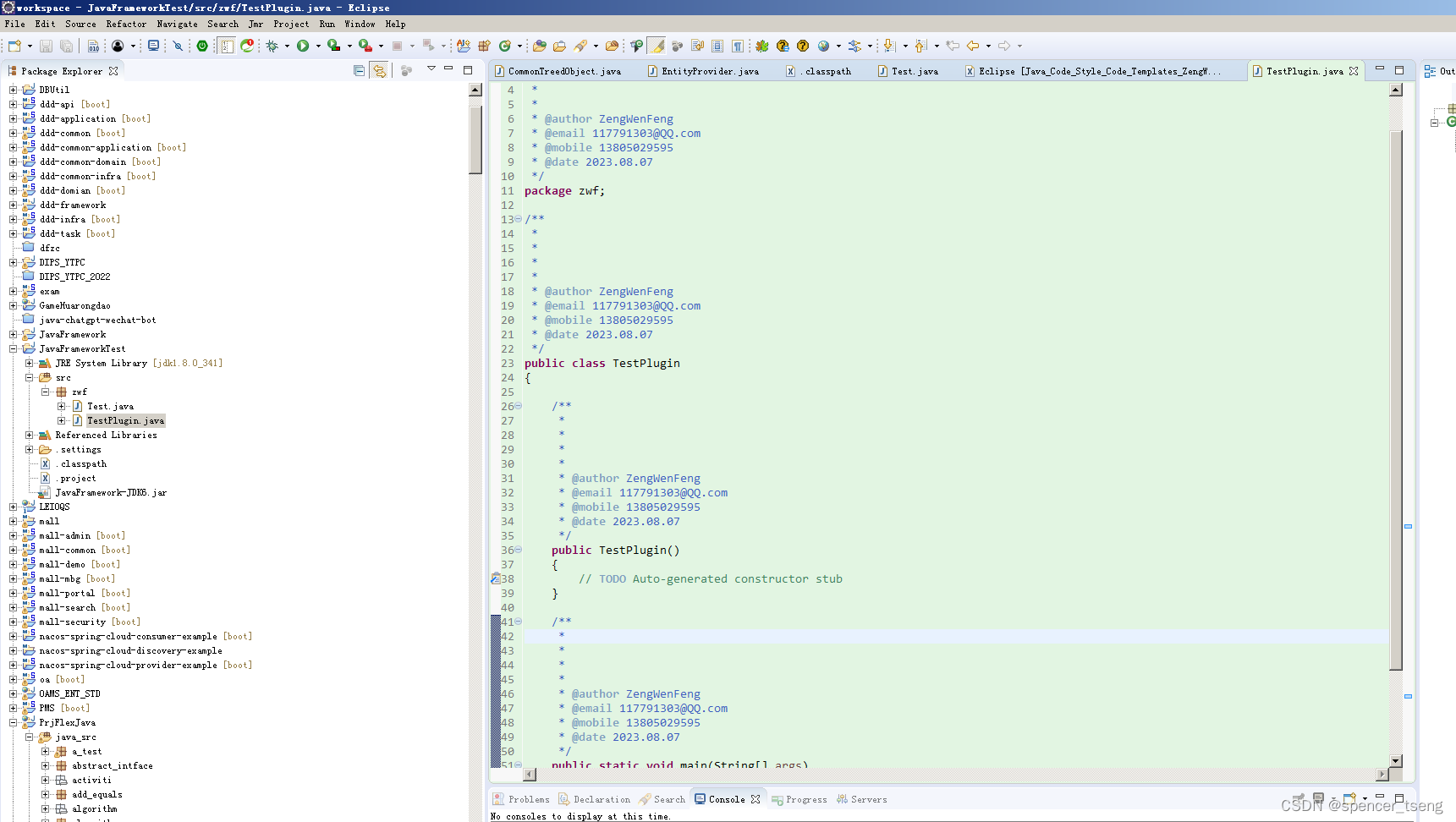
eclipse Java Code_Style Code_Templates
Preferences - Java - Code Style - Code Templates Eclipse [Java_Code_Style_Code_Templates_ZengWenFeng] 2023.08.07.xml 创建一个新的工程,不然有时候不生效,旧项目可能要重新导入eclipse 创建一个测试类试一试 所有的设置都生效了...
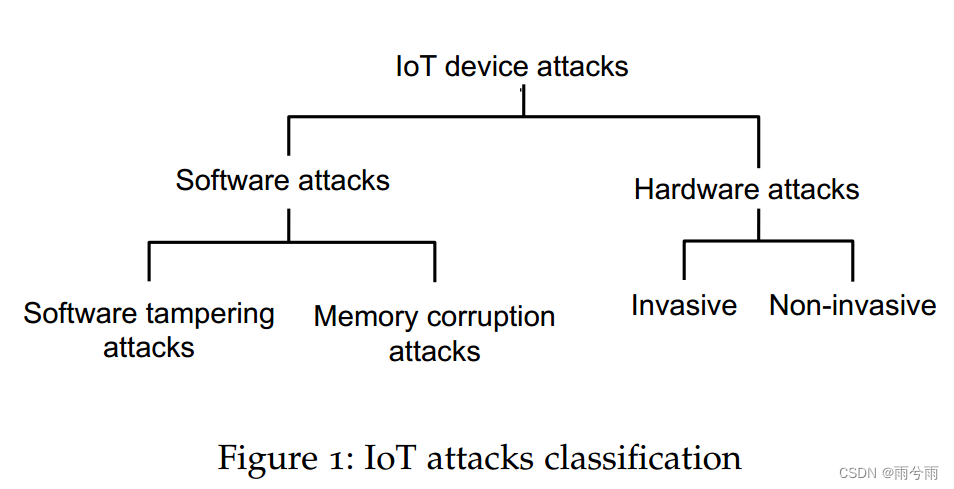
01《Detecting Software Attacks on Embedded IoT Devices》随笔
2023.08.05 今天读的是一篇博士论文 论文传送门:Detecting Software Attacks on Embedded IoT Devices 看了很长时间,发现有一百多页,没看完,没看到怎么实现的。 摘要 联网设备的增加使得嵌入式设备成为各种网络攻击的诱人目标&…...
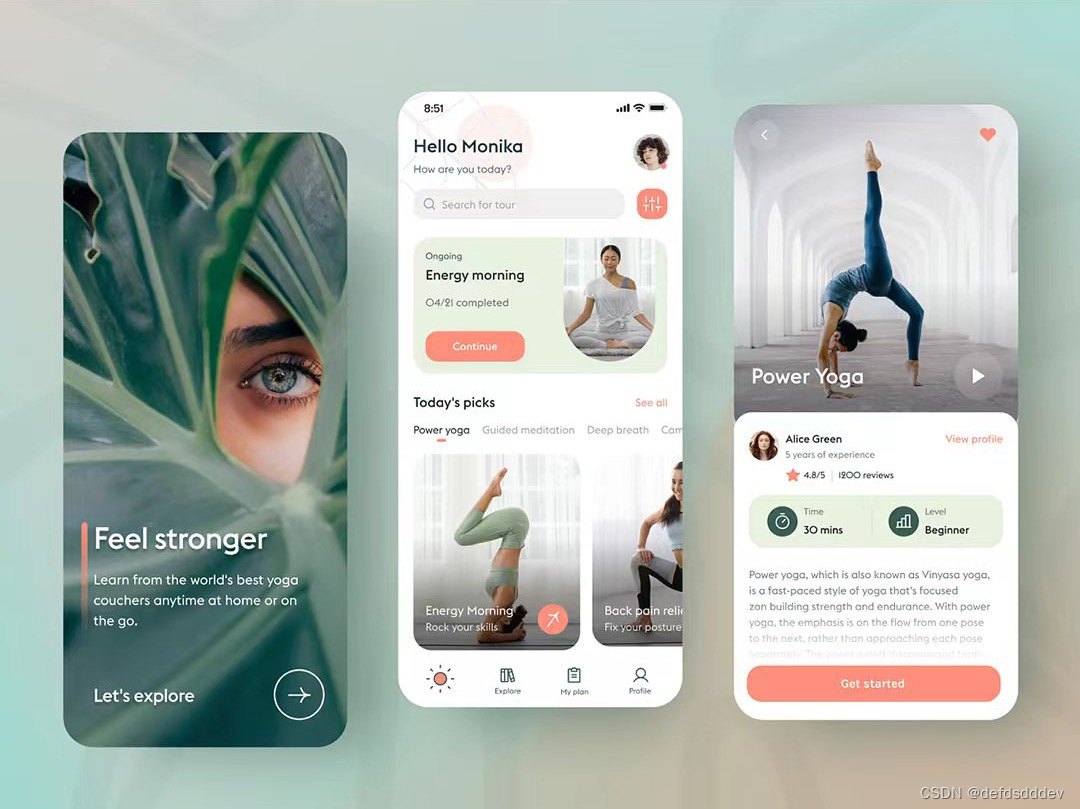
APP外包开发的学习流程
学习iOS App的开发是一项有趣和富有挑战性的任务,是一个不断学习和不断进步的过程。掌握基础知识后,不断实践和尝试新的项目将使您的技能不断提升。下面和大家分享一些建议,可以帮助您开始学习iOS App的开发。北京木奇移动技术有限公司&#…...

第0章 环境搭建汇总
mini商城第0章 环境搭建汇总 本文是整个mini商城的前置文档,所有用到的技术安装都在本篇文档中有详细描述。所有软件安装不分先后顺序,只是作为一个参考文档,需要用到什么技术软件,就按照文档安装什么软件,切不可一上来全部安装一遍。 文章中有些截图中服务器地址是192.16…...
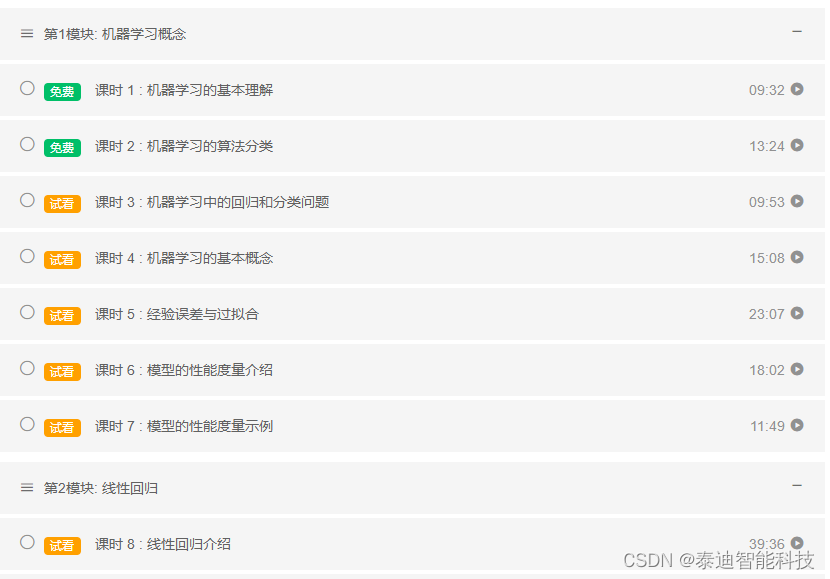
大数据培训课程-《机器学习从入门到精通》上新啦
《机器学习从入门到精通》课程是一门专业课程,面向人工智能技术服务,课程系统地介绍了Python编程库、分类、回归、无监督学习和模型使用技巧以及算法和案例充分融合。 《机器学习从入门到精通》课程亮点: 课程以任务为导向,逐步学…...
暗黑版GPT流窜暗网 降低犯罪门槛
随着AIGC应用的普及,不法分子利用AI技术犯罪的手段越来越高明,欺骗、敲诈、勒索也开始与人工智能沾边。 近期,专为网络犯罪设计的“暗黑版GPT”持续浮出水面,它们不仅没有任何道德界限,更没有使用门槛,没有…...

数电与Verilog基础知识之同步和异步、同步复位与异步复位
同步和异步是两种不同的处理方式,它们的区别主要在于是否需要等待结果。同步是指一个任务在执行过程中,必须等待上一个任务完成后才能继续执行下一个任务;异步是指一个任务在执行过程中,不需要等待上一个任务完成,可以…...
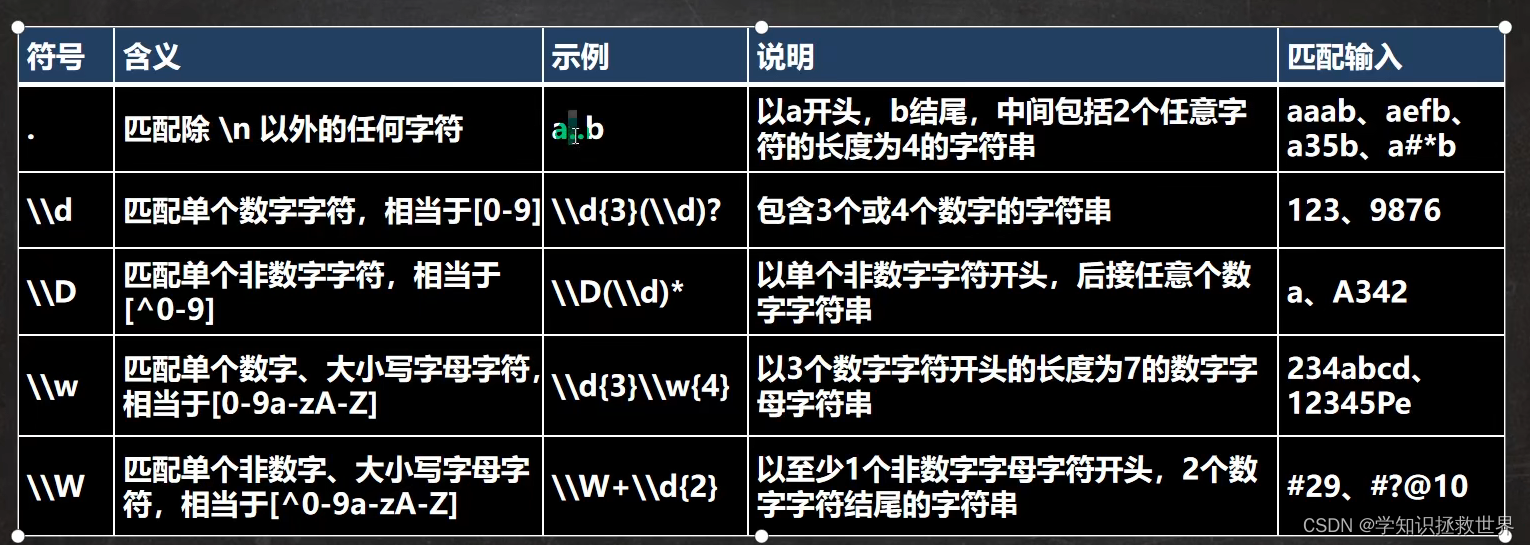
JAVA Android 正则表达式
正则表达式 正则表达式是对字符串执行模式匹配的技术。 正则表达式匹配流程 private void RegTheory() {// 正则表达式String content "1998年12月8日,第二代Java平台的企业版J2EE发布。1999年6月,Sun公司发布了第二代Java平台(简称为Java2) &qu…...

【MFC】07.MFC第三大机制:消息映射-笔记
本专栏上两篇文章分别介绍了【MFC】05.MFC第一大机制:程序启动机制和【MFC】06.MFC第二大机制:窗口创建机制,这篇文章来为大家介绍MFC的第三大机制:消息映射 typfd要实现消息映射,必须满足的三个条件: 类必…...

【jvm】jvm的生命周期
目录 一、启动二、执行三、退出 一、启动 1.java虚拟机的启动是通过引导类加载器bootstrap class loader创建一个初始类(initial class)来完成的,这个类是由虚拟机的具体实现指定的(根据具体虚拟机的类型) 二、执行 1.一个运行中的java虚拟机…...
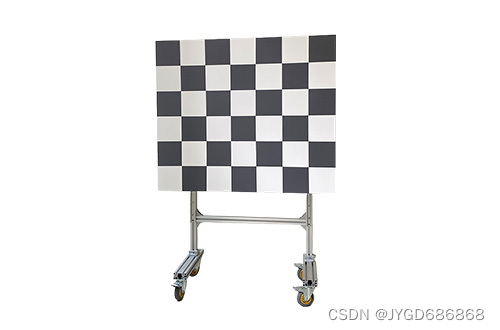
激光雷达测距和摄像头联合棋盘格反射率标定板
目前,激光雷达不仅在军事上起到了重要的作用,而且发挥其测程大、精度高、反应速度快、可靠性高等优点,在商业领域应用越来越广,发展越来越迅速,具有较高的实用价值和商业价值。车载三维成像激光雷达采用脉冲式测量原理…...
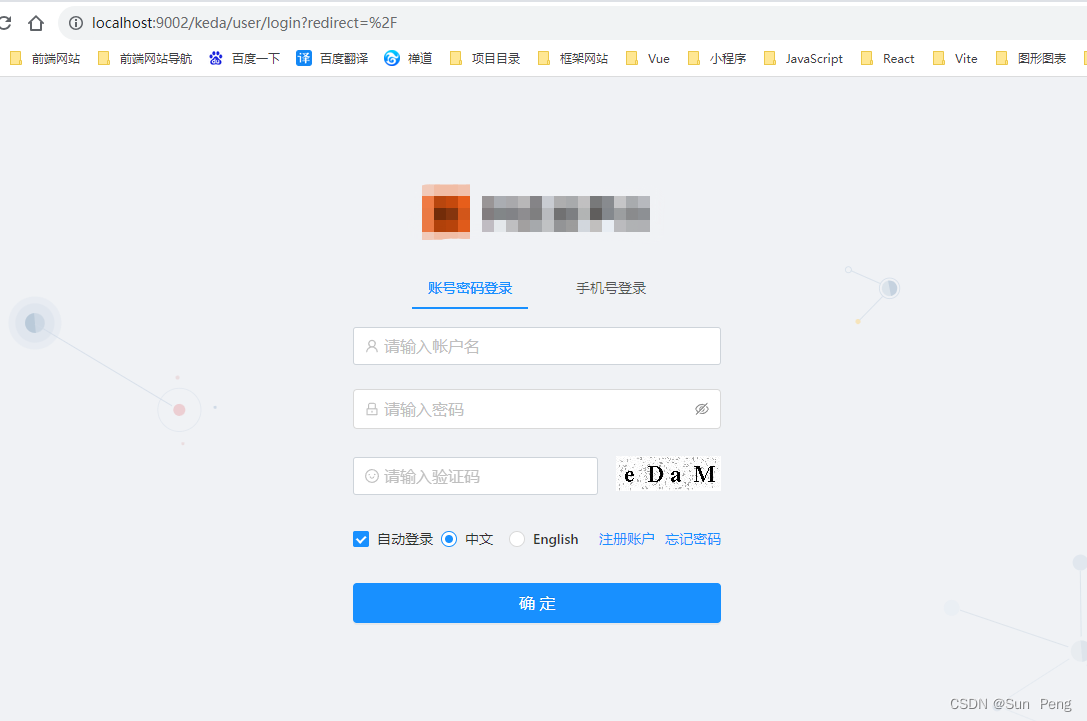
【Docker】docker镜像+nginx部署vue项目:
文章目录 一、文档:二、打包vue项目:三、配置nginx:四、配置Dockerfile:五、构建镜像:六、运行容器:七、最终效果: 一、文档: 【1】菜鸟教程:https://www.runoob.com/do…...

文件编辑(vi/vim)
在所有的命令执行前,先按Esc 1、vi的基本概念 基本上vi可以分为三种状态,分别是命令模式(command mode)、插入模式(Insert mode)和底行模式(last line mode),各模式…...

1007 Maximum Subsequence Sum (PAT甲级)
惭愧,知道该用DP做,但是又翻了参考书才想起来。dp[i]表示以i项为结尾的最大子列。 #include <cstdio> #include <vector>int K, maxx, u, pu, pv; std::vector<int> vec, dp;int main(){scanf("%d", &K);vec.resize(K)…...
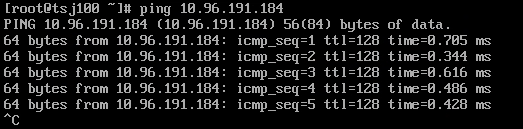
虚拟机centos7配置网络
虚拟机centos7配置网络 centos7克隆之后需要配置网络才能联网。 实验环境: VMware Workstation Pro 16CentOS 7系统虚拟机主机Windows 11系统 1.VMware网络模式设置为NAT模式 虚拟机–设置–网络适配器– 2.查看虚拟机 子网IP和网关IP 编辑–虚拟网…...
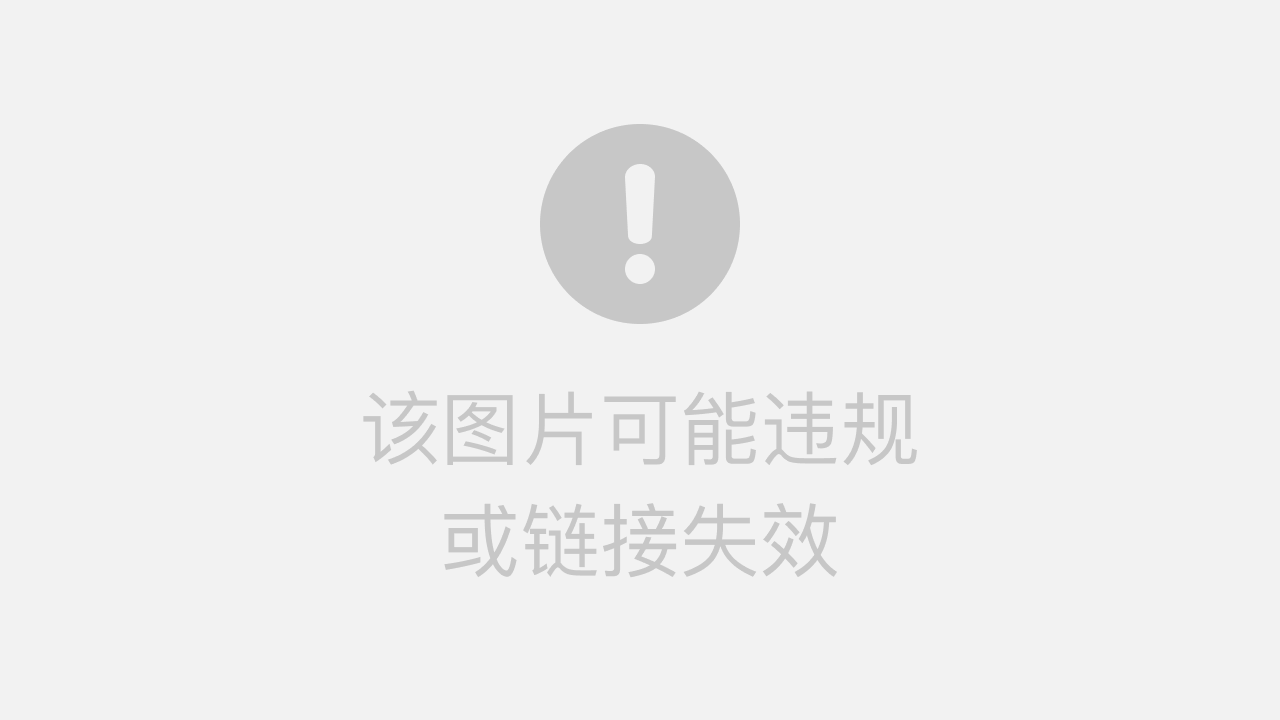
ChatGPT实战:创业咨询,少走弯路,少踩坑
用九死一生形容创业再适合不过,不过一旦成功回报也很诱人,这也是为什么那么多人下场创业。纸上得来终觉浅,绝知此事要躬行,创过业的人都知道其中的心酸,而他们也建议你去创业,因为那真不是一般人能干的事。…...
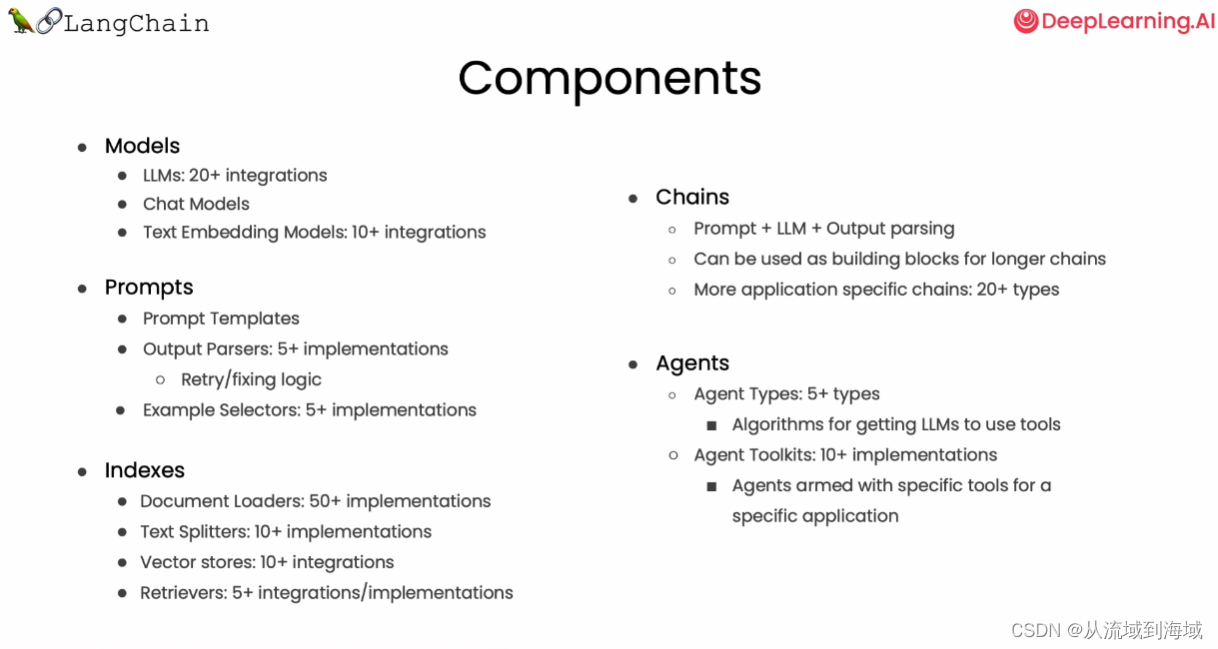
LangChain手记 Overview
整理并翻译自DeepLearning.AILangChain的官方课程:Overview 综述(Overview) LangChain是为大模型应用开发设计的开源框架 LangChain目前提供Python和JavaScript(TypeScript)两种语言的包 LangChain的主攻方向是聚合和…...

Vue_02:详细语法以及代码示例 + 知识点练习 + 综合案例(第二期)
2023年8月4日15:25:01 Vue_02_note 在Vue中,非相应式数据,直接往实例上面挂载就可以了。 01_Vue 指令修饰符 什么是指令修饰符呢? 答: 通过 " . " 指明一些指令后缀,不同 后缀 封装了不同的处理操作 —…...

[腾讯云 Cloud studio 实战训练营] 制作Scrapy Demo爬取起点网月票榜小说数据
首语 最近接触到了一个关于云开发的IDE,什么意思呢? 就是我们通常开发不是在电脑上吗,既要下载编译器,还要下载合适的编辑器,有的时候甚至还需要配置开发环境,有些繁琐。而这个云开发的IDE就是只需要一台…...

使用paddle进行酒店评论的情感分类5——batch准备
把原始语料中的每个句子通过截断和填充,转换成一个固定长度的句子,并将所有数据整理成mini-batch,用于训练模型,下面代码参照paddle官方 # 库文件导入 # encodingutf8 import re import random import requests import numpy as n…...
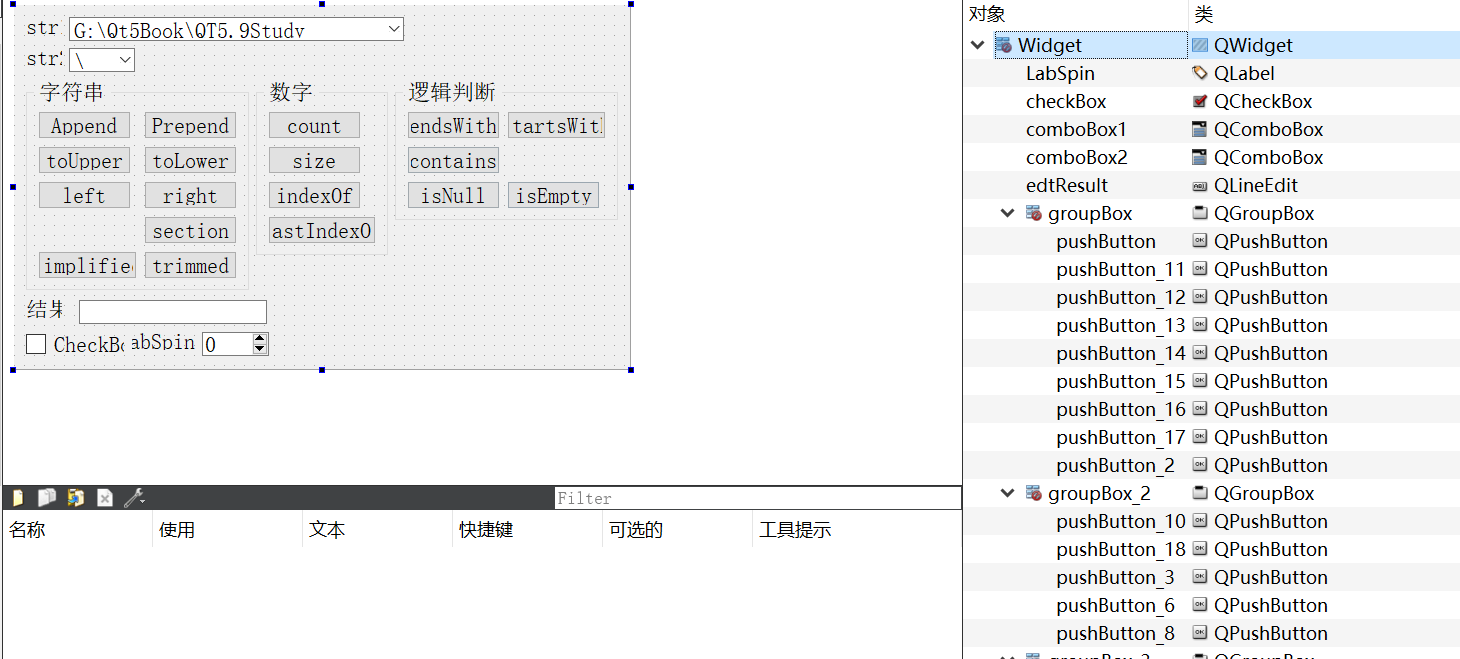
04-1_Qt 5.9 C++开发指南_常用界面设计组件_字符串QString
本章主要介绍Qt中的常用界面设计组件,因为更多的是涉及如何使用,因此会强调使用,也就是更多针对实例,而对于一些细节问题,需要参考《Qt5.9 c开发指南》进行学习。 文章目录 1. 字符串与普通转换、进制转换1.1 可视化U…...
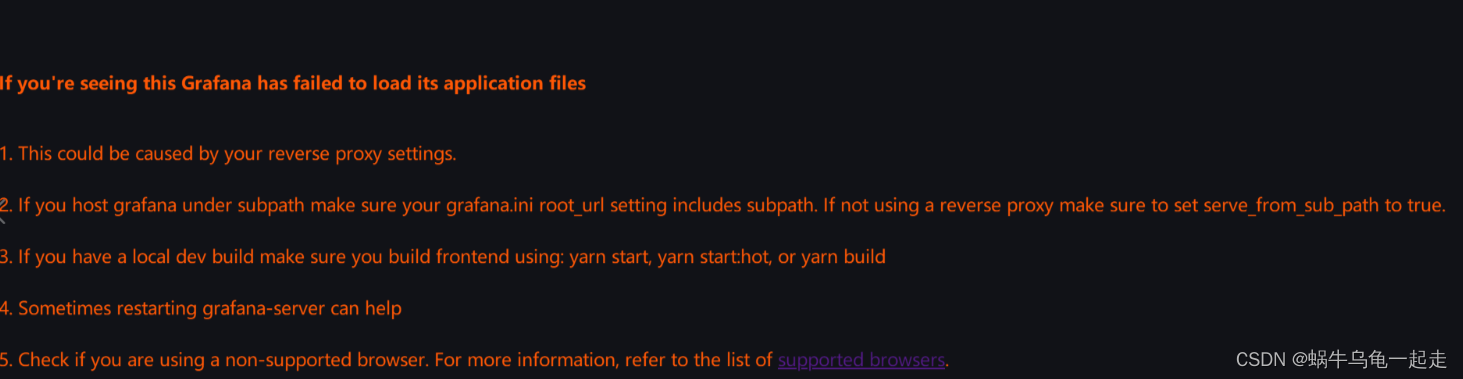
Centos 从0搭建grafana和Prometheus 服务以及问题解决
下载 虚拟机下载 https://customerconnect.vmware.com/en/downloads/info/slug/desktop_end_user_computing/vmware_workstation_player/17_0 cenos 镜像下载 https://www.centos.org/download/ grafana 服务下载 https://grafana.com/grafana/download/7.4.0?platformlinux …...