lv3 嵌入式开发-11 Linux下GDB调试工具
目录
1 GDB简介
2 GDB基本命令
3 GDB调试程序
1 GDB简介
GDB是GNU开源组织发布的一个强大的Linux下的程序调试工具。 一般来说,GDB主要帮助你完成下面四个方面的功能:
- 1、启动你的程序,可以按照你的自定义的要求随心所欲的运行程序(按着自己的想法运行)。
- 2、可让被调试的程序在你所指定的调置的断点处停住。(断点可以是条件表达式)
- 3、当程序被停住时,可以检查此时你的程序中所发生的事。
- 4、你可以改变你的程序,将一个BUG产生的影响修正从而测试其他BUG。
2 GDB基本命令
Here are some of the most frequently needed GDB commands:break [file:]functionSet a breakpoint at function (in file).断点run [arglist]Start your program (with arglist, if specified).bt Backtrace: display the program stack.显示程序堆栈print exprDisplay the value of an expression.打印c Continue running your program (after stopping, e.g. at abreakpoint).继续nextExecute next program line (after stopping); step over any functioncalls in the line.下一句edit [file:]function 查看当前停止的程序行。look at the program line where it is presently stopped.list [file:]function 键入程序的文本当程序停止了的位置type the text of the program in the vicinity of where it ispresently stopped.step Execute next program line (after stopping); step into any functioncalls in the line. 执行下一行help [name]Show information about GDB command name, or general informationabout using GDB.quitExit from GDB.You can, instead, specify a process ID as a second argument or use option "-p", if you want to debug a running process:gdb program 1234gdb -p 1234
示例
linux@linux:~/Desktop$ ls
a.out gdb.c
linux@linux:~/Desktop$ gcc -g gdb.c
linux@linux:~/Desktop$ ./a.out
0
1
2
3
4
hello world
linux@linux:~/Desktop$ gdb a.out
GNU gdb (Ubuntu 7.7-0ubuntu3.1) 7.7
Copyright (C) 2014 Free Software Foundation, Inc.
License GPLv3+: GNU GPL version 3 or later <http://gnu.org/licenses/gpl.html>
This is free software: you are free to change and redistribute it.
There is NO WARRANTY, to the extent permitted by law. Type "show copying"
and "show warranty" for details.
This GDB was configured as "i686-linux-gnu".
Type "show configuration" for configuration details.
For bug reporting instructions, please see:
<http://www.gnu.org/software/gdb/bugs/>.
Find the GDB manual and other documentation resources online at:
<http://www.gnu.org/software/gdb/documentation/>.
For help, type "help".
Type "apropos word" to search for commands related to "word"...
Reading symbols from a.out...done.
(gdb) l
2
3 void print()
4 {
5 printf("hello world\n");
6 }
7 int main(int argc, const char *argv[])
8 {
9 int i;
10
11 for (i = 0; i < 5; i++)
(gdb) b main
Breakpoint 1 at 0x804846a: file gdb.c, line 11.
(gdb) r
Starting program: /home/linux/Desktop/a.out Breakpoint 1, main (argc=1, argv=0xbffff164) at gdb.c:11
11 for (i = 0; i < 5; i++)
(gdb) c
Continuing.
0
1
2
3
4
hello world
[Inferior 1 (process 5010) exited normally]
(gdb) b 10
Note: breakpoint 1 also set at pc 0x804846a.
Breakpoint 2 at 0x804846a: file gdb.c, line 10.
(gdb) r
Starting program: /home/linux/Desktop/a.out Breakpoint 1, main (argc=1, argv=0xbffff164) at gdb.c:11
11 for (i = 0; i < 5; i++)
(gdb) c
Continuing.
0
1
2
3
4
hello world
[Inferior 1 (process 5113) exited normally]
(gdb) r
Starting program: /home/linux/Desktop/a.out Breakpoint 1, main (argc=1, argv=0xbffff164) at gdb.c:11
11 for (i = 0; i < 5; i++)
(gdb) n
12 printf("%d\n",i);
(gdb) n
0
11 for (i = 0; i < 5; i++)
(gdb) n
12 printf("%d\n",i);
(gdb) n
1
11 for (i = 0; i < 5; i++)
(gdb) p &i
$1 = (int *) 0xbffff0bc
(gdb) p i
$2 = 1
(gdb) n
12 printf("%d\n",i);
(gdb) p i
$3 = 2
(gdb) n
2
11 for (i = 0; i < 5; i++)
(gdb) n
12 printf("%d\n",i);
(gdb) n
3
11 for (i = 0; i < 5; i++)
(gdb) n
12 printf("%d\n",i);
(gdb) p i
$4 = 4
(gdb) n
4
11 for (i = 0; i < 5; i++)
(gdb) n
14 print();
(gdb) s
print () at gdb.c:5
5 printf("hello world\n");
(gdb) n
hello world
6 }
(gdb) n
main (argc=1, argv=0xbffff164) at gdb.c:15
15 return 0;
(gdb)
3 GDB调试程序
示例:定位错误
代码
#include <stdio.h>#ifndef _CORE_void print()
{printf("hello world\n");
}
int main(int argc, const char *argv[])
{int i;for (i = 0; i < 5; i++)printf("%d\n",i);print();return 0;
}#else
int main(int argc,const char *argv[])
{int *temp = NULL;*temp = 10; //没有分配内存空间,直接会出错return 0;
}#endif
定位错误位置
linux@linux:~/Desktop$ ls
a.out gdb.c
linux@linux:~/Desktop$ gcc -g gdb.c -D _CORE_
linux@linux:~/Desktop$ ./a.out
Segmentation fault (core dumped)
linux@linux:~/Desktop$ ls
a.out core gdb.c
linux@linux:~/Desktop$ gdb a.out core
GNU gdb (Ubuntu 7.7-0ubuntu3.1) 7.7
Copyright (C) 2014 Free Software Foundation, Inc.
License GPLv3+: GNU GPL version 3 or later <http://gnu.org/licenses/gpl.html>
This is free software: you are free to change and redistribute it.
There is NO WARRANTY, to the extent permitted by law. Type "show copying"
and "show warranty" for details.
This GDB was configured as "i686-linux-gnu".
Type "show configuration" for configuration details.
For bug reporting instructions, please see:
<http://www.gnu.org/software/gdb/bugs/>.
Find the GDB manual and other documentation resources online at:
<http://www.gnu.org/software/gdb/documentation/>.
For help, type "help".
Type "apropos word" to search for commands related to "word"...
Reading symbols from a.out...done.
[New LWP 5904]
Core was generated by `./a.out'.
Program terminated with signal SIGSEGV, Segmentation fault.
#0 0x080483fd in main (argc=1, argv=0xbfea3544) at gdb.c:24
24 *temp = 10; //没有分配内存空间,直接会出错
(gdb)
如何调试正在运行的进程?
源码
linux@linux:~/Desktop$ cat gdb.c
#include <stdio.h>
#include <unistd.h>int main(int argc, const char *argv[])
{while(1){int i;i++;printf("%d\n",i);sleep(1);}return 0;
}linux@linux:~/Desktop$ gcc -g gdb.c
linux@linux:~/Desktop$ ./a.out
-1217503231
-1217503230
-1217503229
-1217503228...
再开一个终端
linux@linux:~$ ps aux | grep a.out
linux 6291 0.0 0.0 2028 280 pts/0 S+ 11:47 0:00 ./a.out
linux 6293 0.0 0.0 4680 832 pts/3 S+ 11:47 0:00 grep --color=auto a.out
linux@linux:~$ cd /home/linux/
.bakvim/ .gconf/ .sogouinput/
.cache/ .local/ Templates/
.config/ .mozilla/ tftpboot/
.dbus/ Music/ Videos/
Desktop/ Pictures/ .vim/
Documents/ .pki/ vmware-tools-distrib/
Downloads/ Public/
linux@linux:~$ cd /home/linux/Desktop/
linux@linux:~/Desktop$ ls
a.out core gdb.c
linux@linux:~/Desktop$ gdb a.out -p 4849
GNU gdb (Ubuntu 7.7-0ubuntu3.1) 7.7
Copyright (C) 2014 Free Software Foundation, Inc.
License GPLv3+: GNU GPL version 3 or later <http://gnu.org/licenses/gpl.html>
This is free software: you are free to change and redistribute it.
There is NO WARRANTY, to the extent permitted by law. Type "show copying"
and "show warranty" for details.
This GDB was configured as "i686-linux-gnu".
Type "show configuration" for configuration details.
For bug reporting instructions, please see:
<http://www.gnu.org/software/gdb/bugs/>.
Find the GDB manual and other documentation resources online at:
<http://www.gnu.org/software/gdb/documentation/>.
For help, type "help".
Type "apropos word" to search for commands related to "word"...
Reading symbols from a.out...done.
Attaching to program: /home/linux/Desktop/a.out, process 4849warning: unable to open /proc file '/proc/4849/status'warning: unable to open /proc file '/proc/4849/status'
ptrace: No such process.
(gdb) b main
Breakpoint 1 at 0x8048456: file gdb.c, line 9.
(gdb) n
The program is not being run.
(gdb) r
Starting program: /home/linux/Desktop/a.out Breakpoint 1, main (argc=1, argv=0xbffff0f4) at gdb.c:9
9 i++;
(gdb) n
10 printf("%d\n",i);
(gdb) n
-1208209407
11 sleep(1);
(gdb) n
12 }
(gdb) q
A debugging session is active.Inferior 1 [process 6317] will be killed.Quit anyway? (y or n) y
linux@linux:~/Desktop$
相关文章:
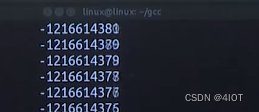
lv3 嵌入式开发-11 Linux下GDB调试工具
目录 1 GDB简介 2 GDB基本命令 3 GDB调试程序 1 GDB简介 GDB是GNU开源组织发布的一个强大的Linux下的程序调试工具。 一般来说,GDB主要帮助你完成下面四个方面的功能: 1、启动你的程序,可以按照你的自定义的要求随心所欲的运行程序&#…...
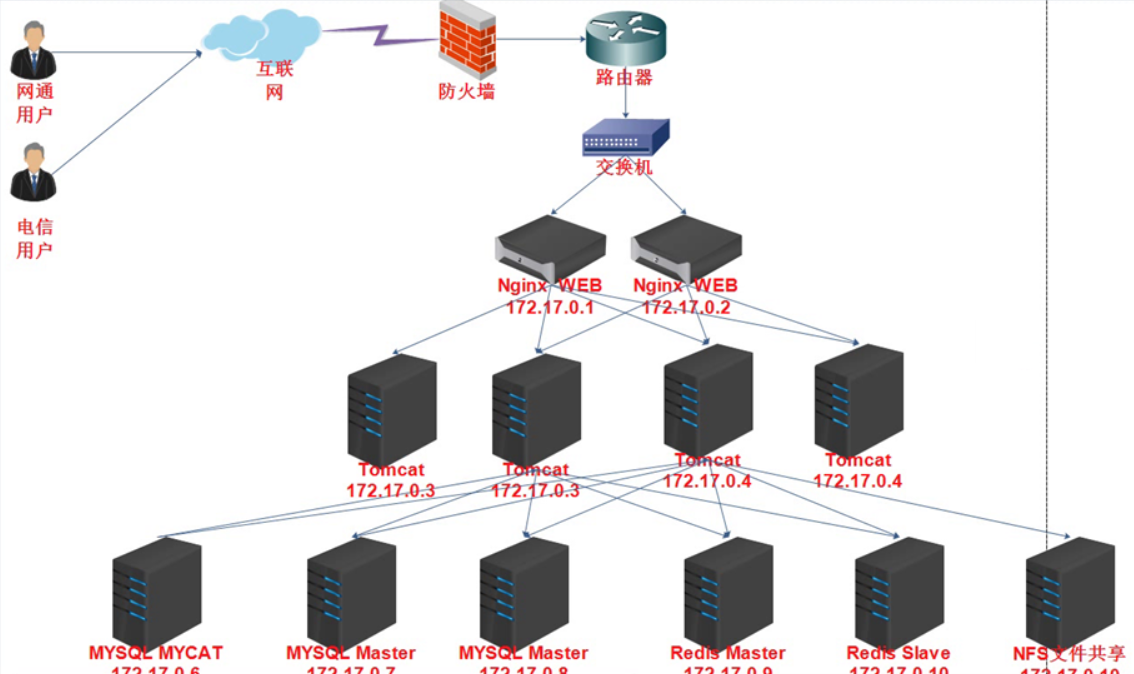
Zabbix监控平台概念
1.概念 Zabbix是一款开源的、免费的、分布式监控平台支持web管理,WEB界面可以方便管理员使用可以监控硬件服务器CPU温度、风扇转速、操作系统CPU、EME、DISK、I/O、流量宽带、负载、端口、进程等Zabbix是C/S架构,Client客户端和Server端组成 2.Zabbix可…...
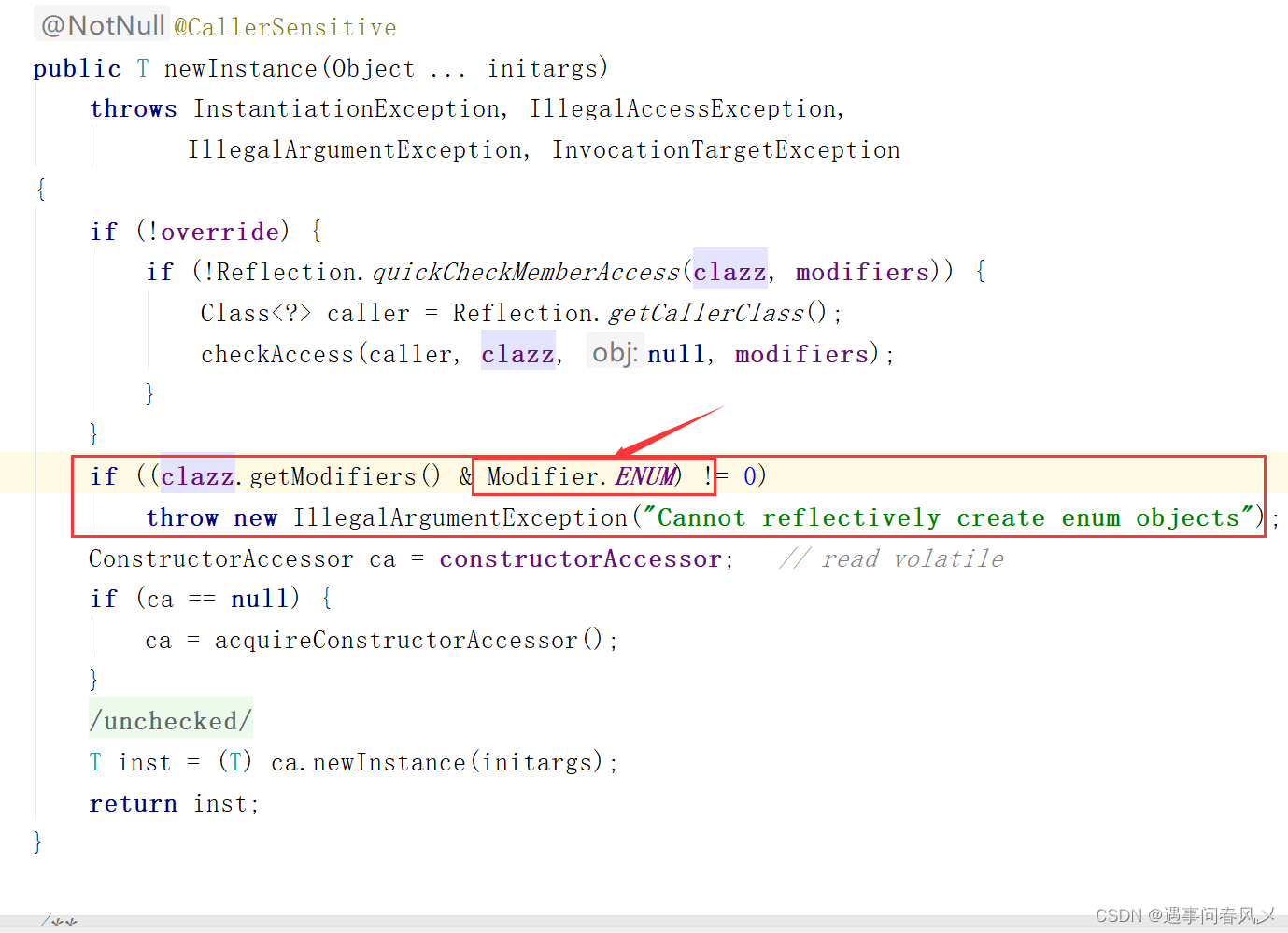
【javaSE】 枚举与枚举的使用
文章目录 🎄枚举的背景及定义⚾枚举特性总结: 🌲枚举的使用🚩switch语句🚩常用方法📌示例一📌示例二 🎍枚举优点缺点🌴枚举和反射🚩枚举是否可以通过反射&…...

NetSuite知识会汇编-管理员篇顾问篇2023
本月初,开学之际,我们发布了《NetSuite知识会汇编-用户篇 2023》,这次发布《NetSuite知识会汇编-管理员篇&顾问篇2023》。本篇挑选了近两年NetSuite知识会中的一些文章,涉及开发、权限、系统管理等较深的内容,共19…...

根号分治与多项式的巧妙结合:GYM-104386G
使用范围:序列上对于每种数的计数问题 考虑对每种数的出现次数进行根号分治 如果出现次数很少,直接平方暴力即可 如果很大考虑任意 ( i , j ) (i,j) (i,j),我们拆一下,再移一下,然后就变成了卷积形式...

通过FTP高速下载几百G数据
基因组下载 (FTP) 常见问题解答 基因组FTP站点有哪些亮点?下载多个基因组组装数据的最简单方法是什么?下载大型数据集的最佳协议是什么?为什么 NCBI 基因组 FTP 站点要重组?我如何及时了解 NCBI 基因组 FTP 站点的变化?...

cudnn-windows-x86_64-8.6.0.163_cuda11-archive 下载
网址不太好访问的话,请从下面我提供的分享下载 Download cuDNN v8.6.0 (October 3rd, 2022), for CUDA 11.x 此资源适配 cuda11.x 将bin和include文件夹里的文件,分别复制到C盘安装CUDA目录的对应文件夹里 安装cuda时自动设置了 CUDA_PATH_V11_8 及path C:\Progra…...
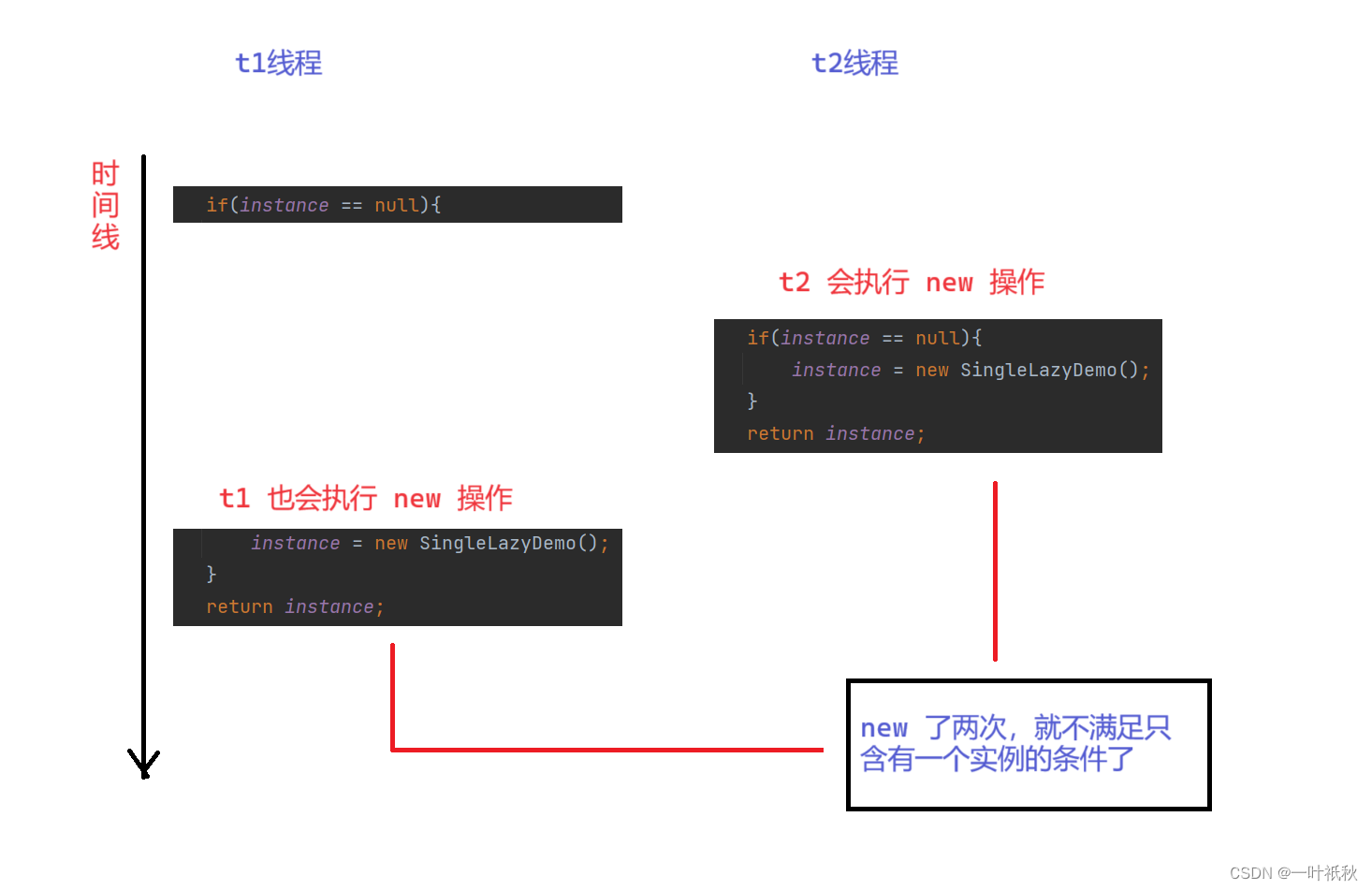
多线程案例(1) - 单例模式
目录 单例模式 饿汉模式 懒汉模式 前言 多线程中有许多非常经典的设计模式(这就类似于围棋的棋谱),这是用来解决我们在开发中遇到很多 "经典场景",简单来说,设计模式就是一份模板,可以套用。…...

Arduino驱动TCS34725传感器(颜色传感器篇)
目录 1、传感器特性 2、硬件原理图 3、控制器和传感器连线图 4、驱动程序 TCS34725是一款低成本,高性价比的RGB全彩颜色识别传感器,传感器通过光学感应来识别物体的表面颜色。...
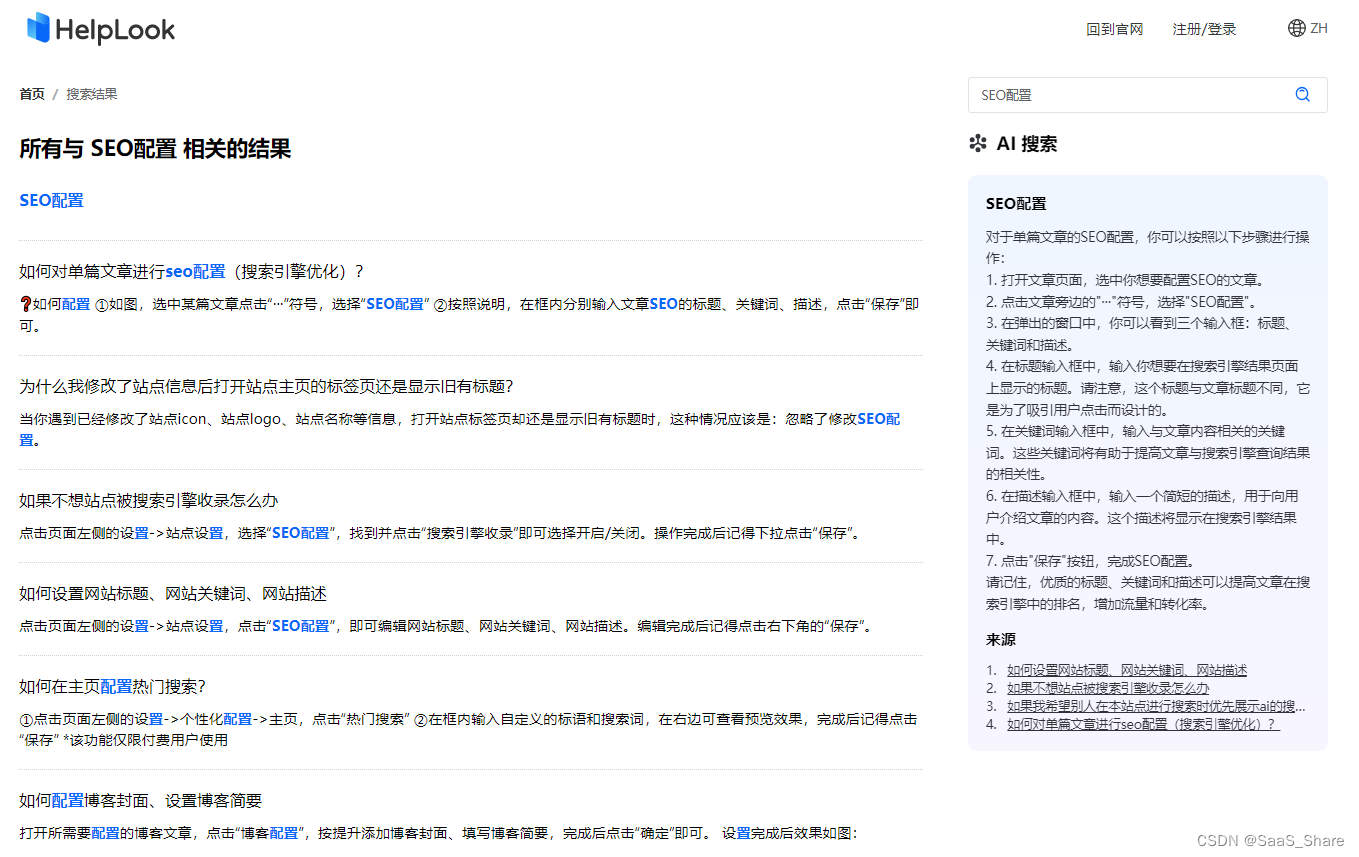
知识库网站如何搭建?需要注意这五个要点!
正因为知识库提供结构化知识库来记载信息和知识,便于团队沉淀经验、共享资源,形成完整的知识体系并持续进化,使得它成为当前企业发展新宠。 构建自己/团队的知识库是一个良好的习惯,可以提高工作和学习效率,以下是一…...
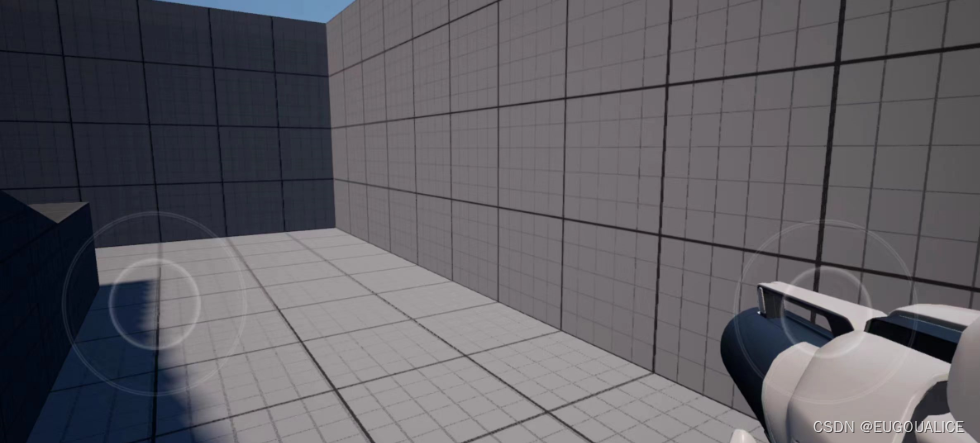
【UE虚幻引擎】UE源码版编译、Andorid配置、打包
首先是要下载源码版的UE,我这里下载的是5.2.1 首先要安装Git 在你准备放代码的文件夹下右键点击Git Bash Here 然后可以直接git clone https://github.com/EpicGames/UnrealEngine 不行的话可以直接去官方的Github上下载Zip压缩包后解压 运行里面的Setup.bat&a…...
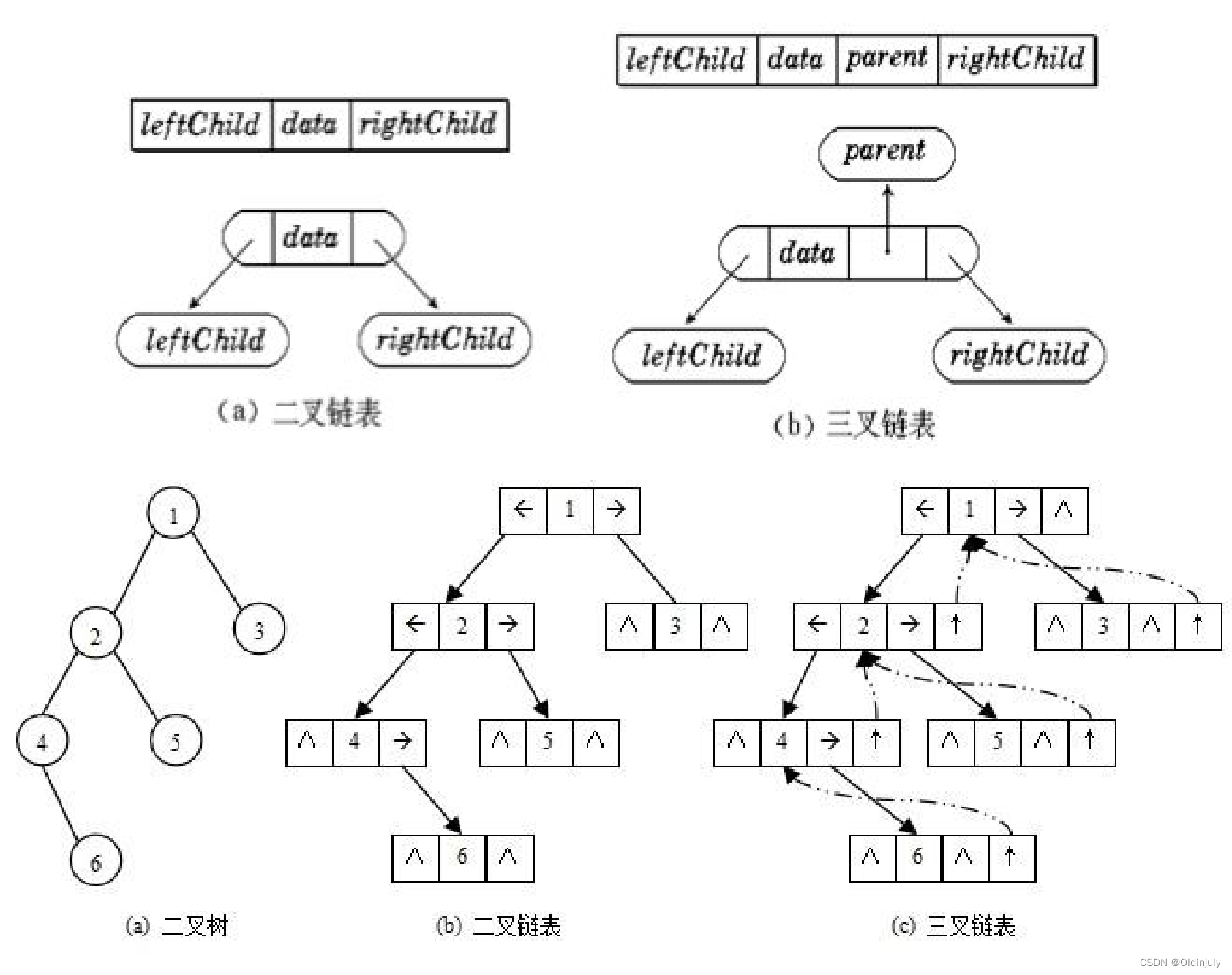
树和二叉树的相关概念及结构
目录 1.树的概念及结构 1.1 树的概念 1.2 树的相关概念 1.3 树的表示 1.3.1 孩子兄弟表示法 1.3.2 双亲表示法 1.4 树的实际应用 2.二叉树的概念及结构 2.1 二叉树的概念 2.2 特殊的二叉树 2.3 二叉树的性质 2.4 二叉树的存储 2.4.1 顺序存储 2.4.2 链式存储 1.树…...
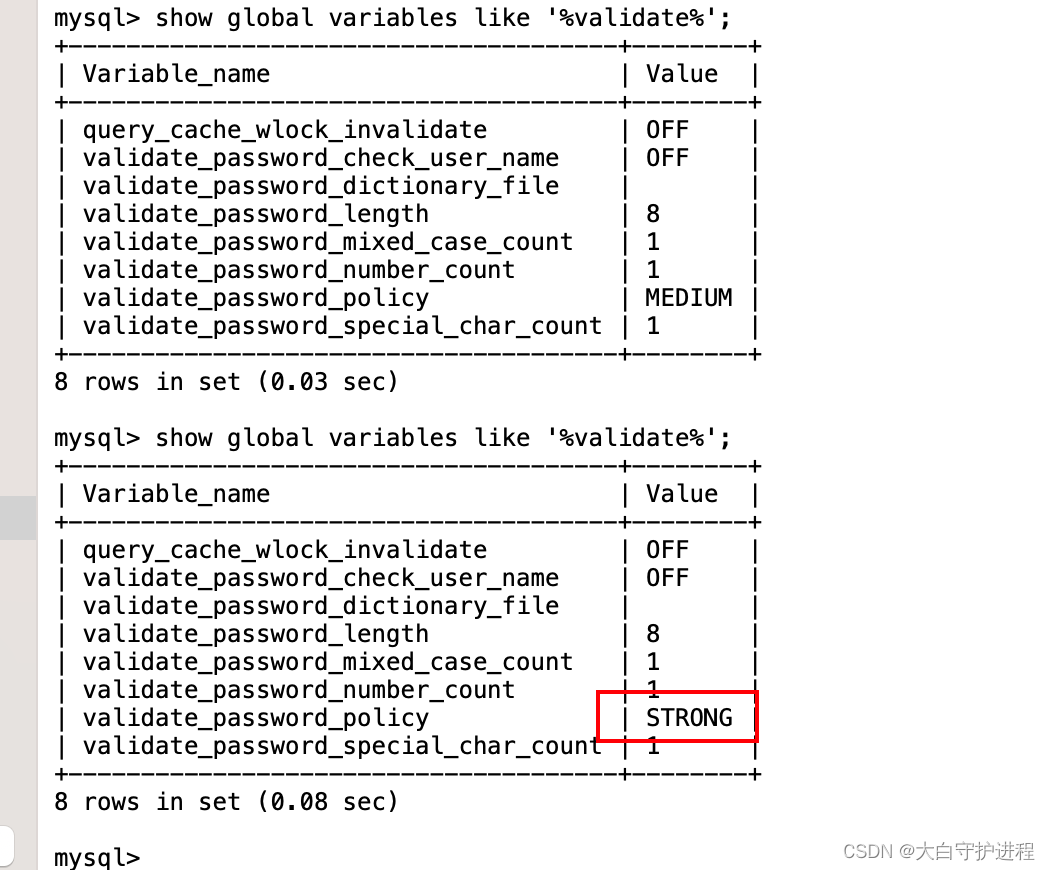
MySQL安装validate_password_policy插件
功能介绍 validate_password_policy 是插件用于验证密码强度的策略。该参数可以设定三种级别:0代表低,1代表中,2代表高。 validate_password_policy 主要影响密码的强度检查级别: 0/LOW:只检查密码长度。 1/MEDIUM&am…...

数据在内存中的存储——练习3
题目: 3.1 #include <stdio.h> int main() {char a -128;printf("%u\n",a);return 0; }3.2 #include <stdio.h> int main() {char a 128;printf("%u\n",a);return 0; }思路分析: 首先二者极其相似%u是无符号格式进行…...
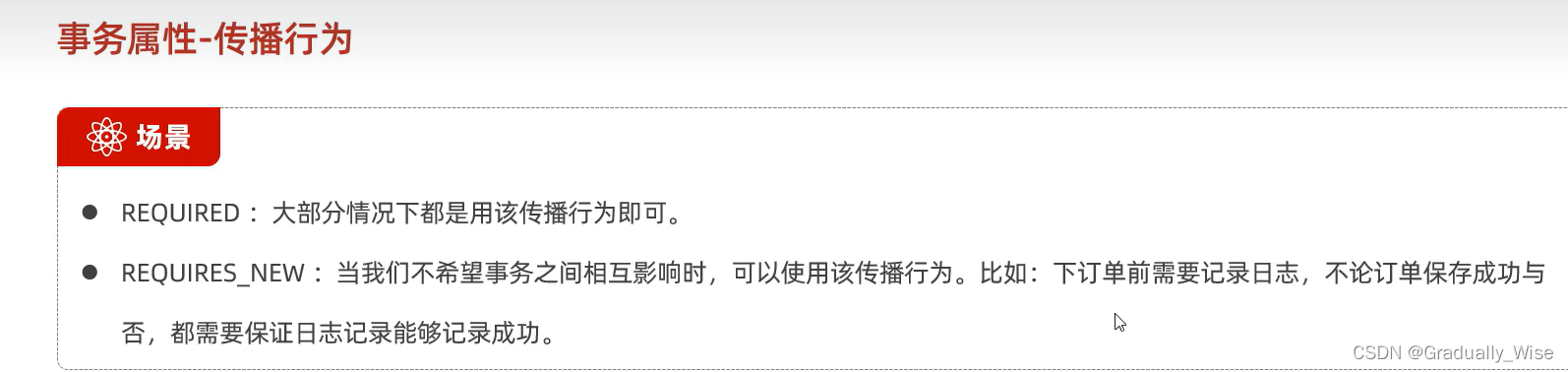
web-案例
分页插件 登录 事务...
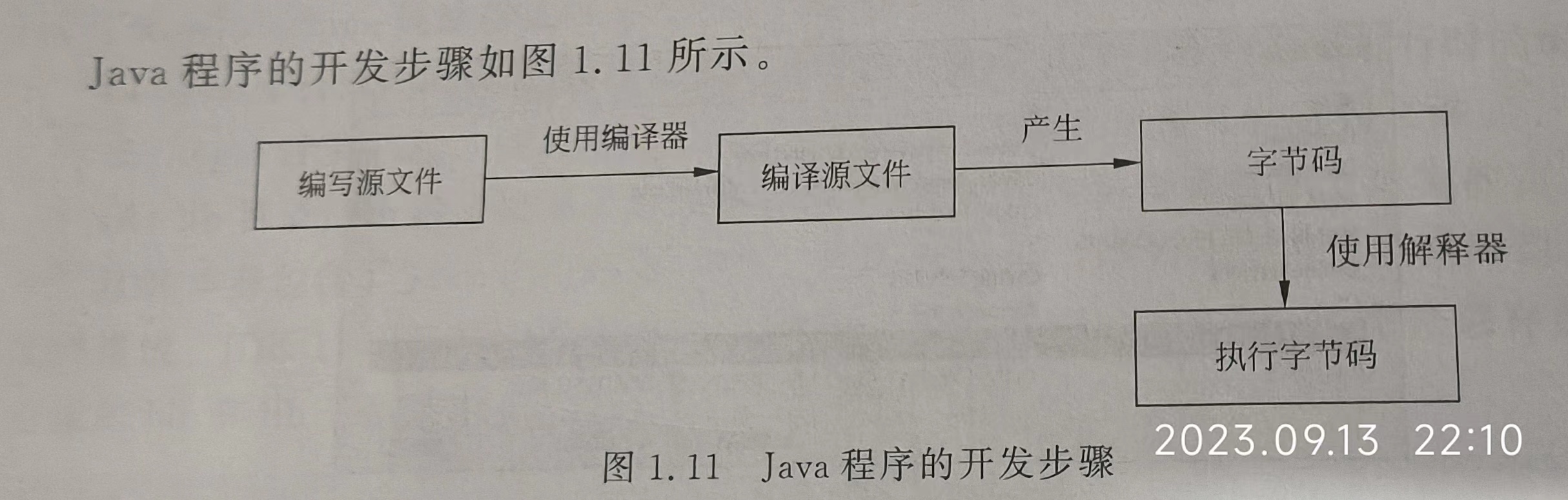
第一章 JAVA入门
文章目录 1.2 Java 的特点1.2.1 简单1.2.2 面向对象1.2.3 与平台无关① 平台与机器指令② C/C程序依赖平台③ Java 虚拟机与字节码1.2.4 多线程1.2.5 动态1.30安装 JDK1.3.1 平台简介0 Java SE②Java EE1.4 Java 程序的开发步骤②保存源文件1.5.2 编译1.8 Java之父-James Gosli…...
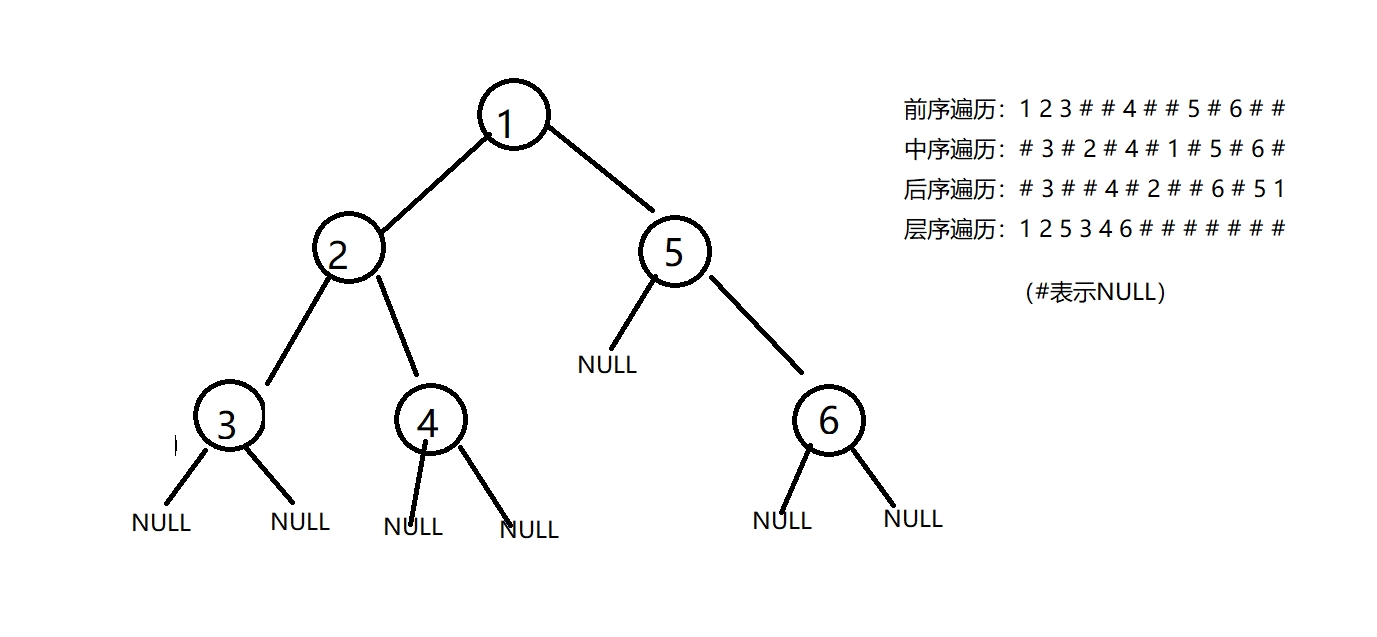
二叉树详解(求二叉树的结点个数、深度、第k层的个数、遍历等)
二叉树,是一种特殊的树,特点是树的度小于等于2(树的度是整个树的结点的度的最大值),由于该特性,构建二叉树的结点只有三个成员,结点的值和指向结点左、右子树的指针。 typedef int DateType; t…...
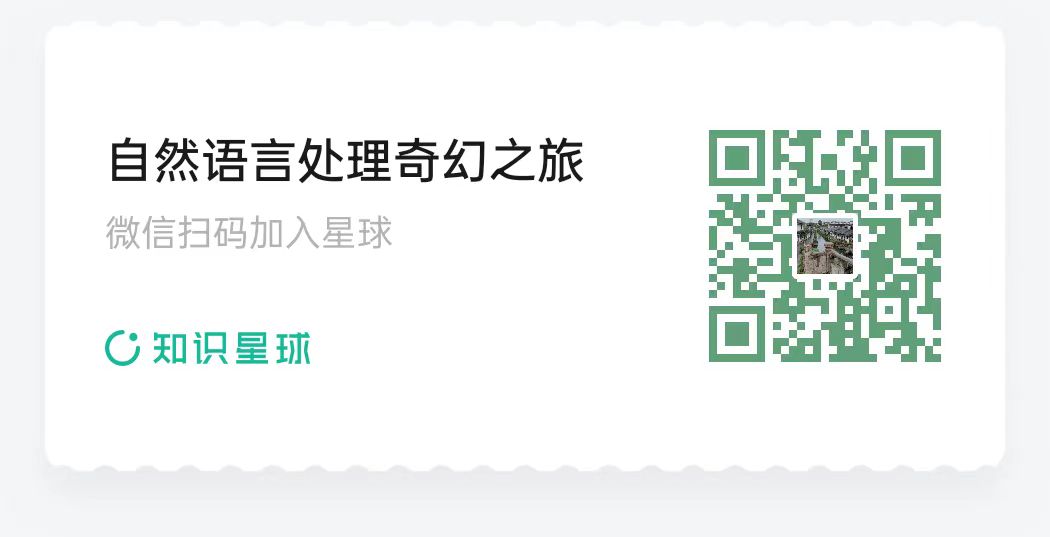
Apollo配置中心及Python连接
本文将会介绍如何启动Apollo,在Apollo中配置参数,以及如何使用Python连接Apollo. Apollo介绍 在文章Python之读取配置文件和文章Python之配置文件处理中,笔者分别介绍了如何使用Python来处理ini, yaml, conf等配置文件。这种配置方式比较方便…...

LuatOS-SOC接口文档(air780E)--audio - 多媒体音频
常量 常量 类型 解释 audio.PCM number PCM格式,即原始ADC数据 audio.MORE_DATA number audio.on回调函数传入参数的值,表示底层播放完一段数据,可以传入更多数据 audio.DONE number audio.on回调函数传入参数的值,表示…...
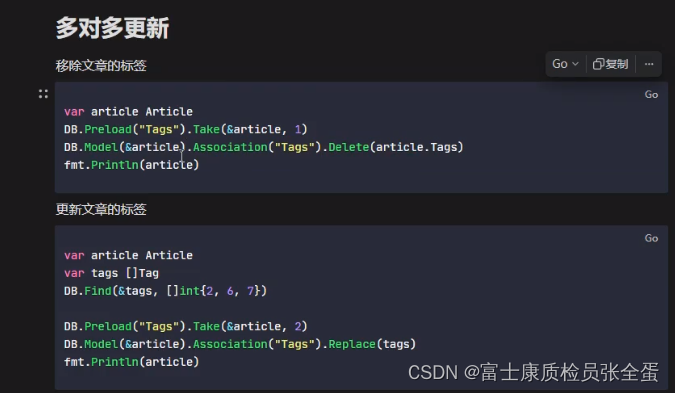
Golang gorm manytomany 多对多 更新、删除、替换
Delete 移除 只删除中间表的数据 删除原有的 var a Article1db.Preload("Tag1s").Take(&a, 1)fmt.Printf("%v", a) {1 k8s [{1 cloud []} {2 linux []}]}mysql> select * from article1; ------------ | id | title | ------------ | 1 | k8s …...

FPGA-结合协议时序实现UART收发器(四):串口驱动模块uart_drive、例化uart_rx、uart_tx
FPGA-结合协议时序实现UART收发器(四):串口驱动模块uart_drive、例化uart_rx、uart_tx 串口驱动模块uart_drive、例化uart_rx、uart_tx,功能实现 文章目录 FPGA-结合协议时序实现UART收发器(四)࿱…...
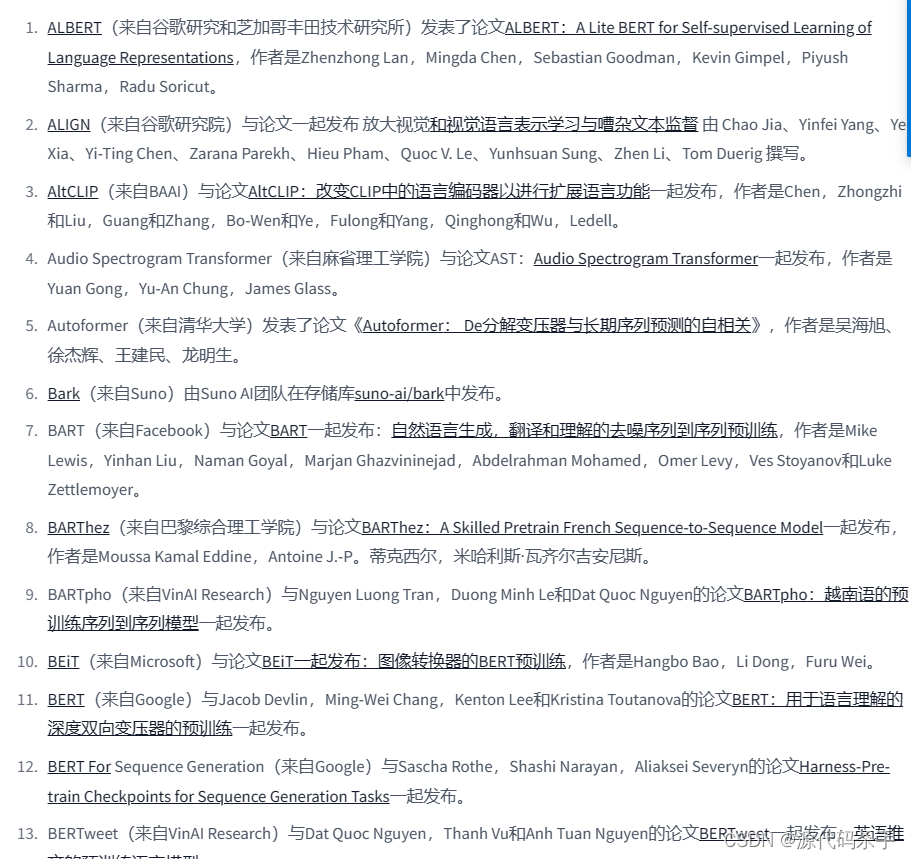
Transformers-Bert家族系列算法汇总
🤗 Transformers 提供 API 和工具,可轻松下载和训练最先进的预训练模型。使用预训练模型可以降低计算成本、碳足迹,并节省从头开始训练模型所需的时间和资源。这些模型支持不同形式的常见任务,例如: 📝 自…...
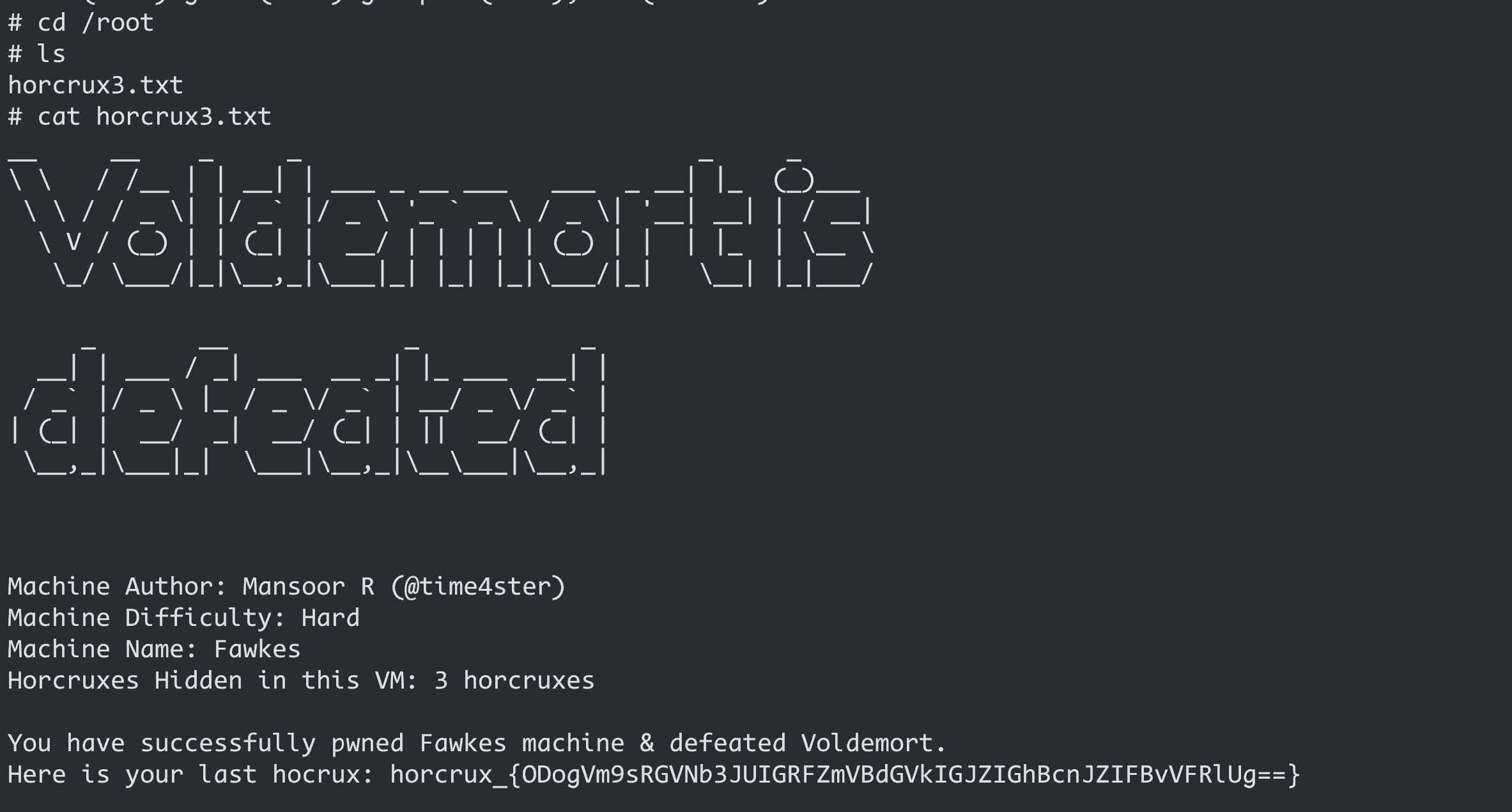
Vulnhub系列靶机---HarryPotter-Fawkes-哈利波特系列靶机-3
文章目录 信息收集主机发现端口扫描dirsearch扫描gobuster扫描 漏洞利用缓冲区溢出edb-debugger工具msf-pattern工具 docker容器内提权tcpdump流量分析容器外- sudo漏洞提权 靶机文档:HarryPotter: Fawkes 下载地址:Download (Mirror) 难易程度ÿ…...
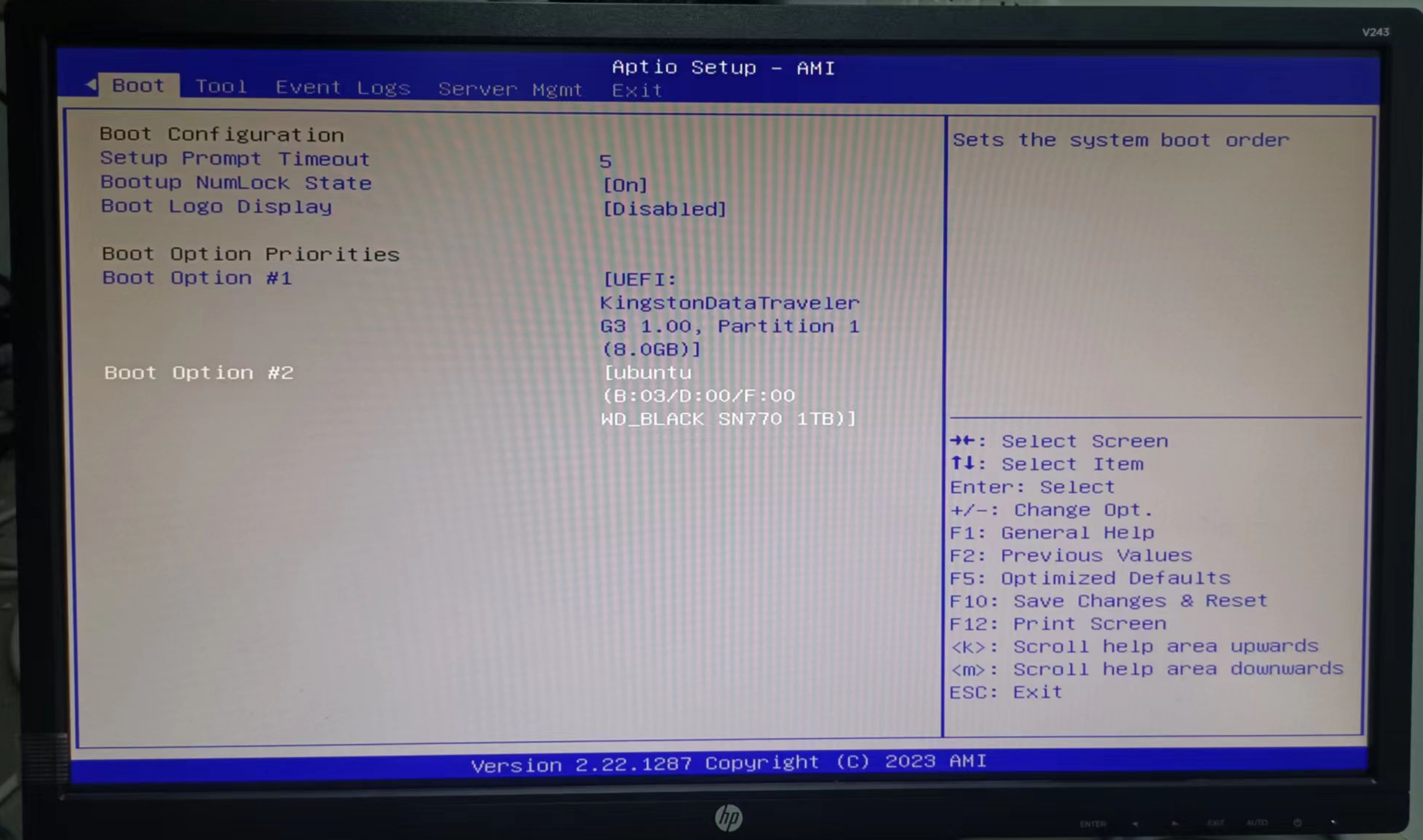
【服务器】ASUS ESC4000-E11 安装系统
ASUS ESC4000-E11说明书 没找到 ASUS ESC4000-E11的说明书,下面是ESC4000A-E11的说明书: https://manualzz.com/doc/65032674/asus-esc4000a-e11-servers-and-workstation-user-manual 下载地址: https://www.manualslib.com/manual/231379…...
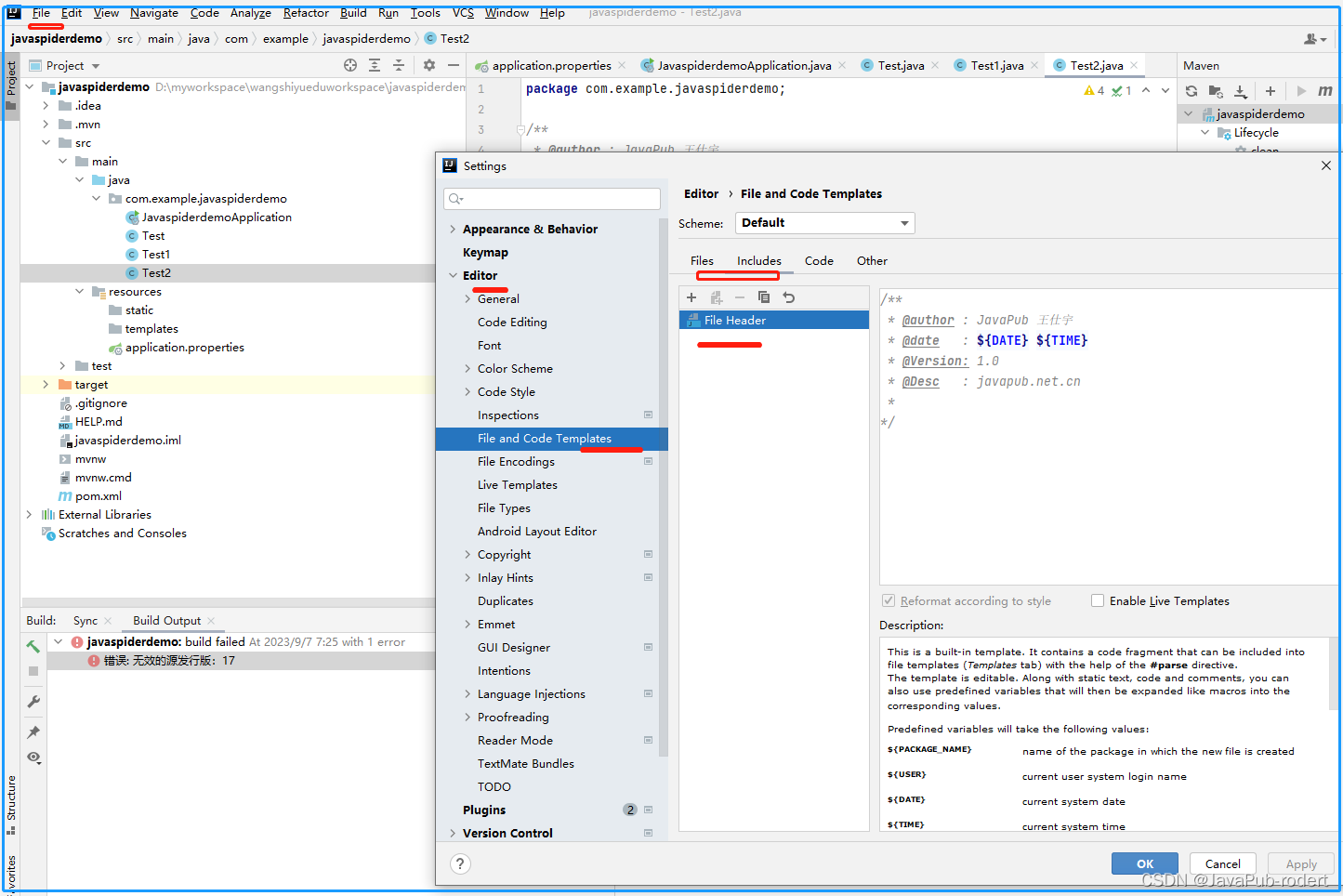
创建java文件 自动添加作者、时间等信息 – IDEA 技巧
2023 09 亲测 文章目录 效果修改位置配置信息 效果 每次创建文件的时候,自动加上作者、时间等信息 修改位置 打开:File —> Settings —> Editor —> File and Code Templates —> includes —> FileHeader 配置信息 /*** author : Java…...
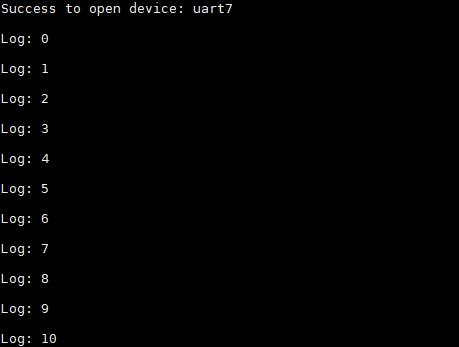
第27章_瑞萨MCU零基础入门系列教程之freeRTOS实验
本教程基于韦东山百问网出的 DShanMCU-RA6M5开发板 进行编写,需要的同学可以在这里获取: https://item.taobao.com/item.htm?id728461040949 配套资料获取:https://renesas-docs.100ask.net 瑞萨MCU零基础入门系列教程汇总: ht…...
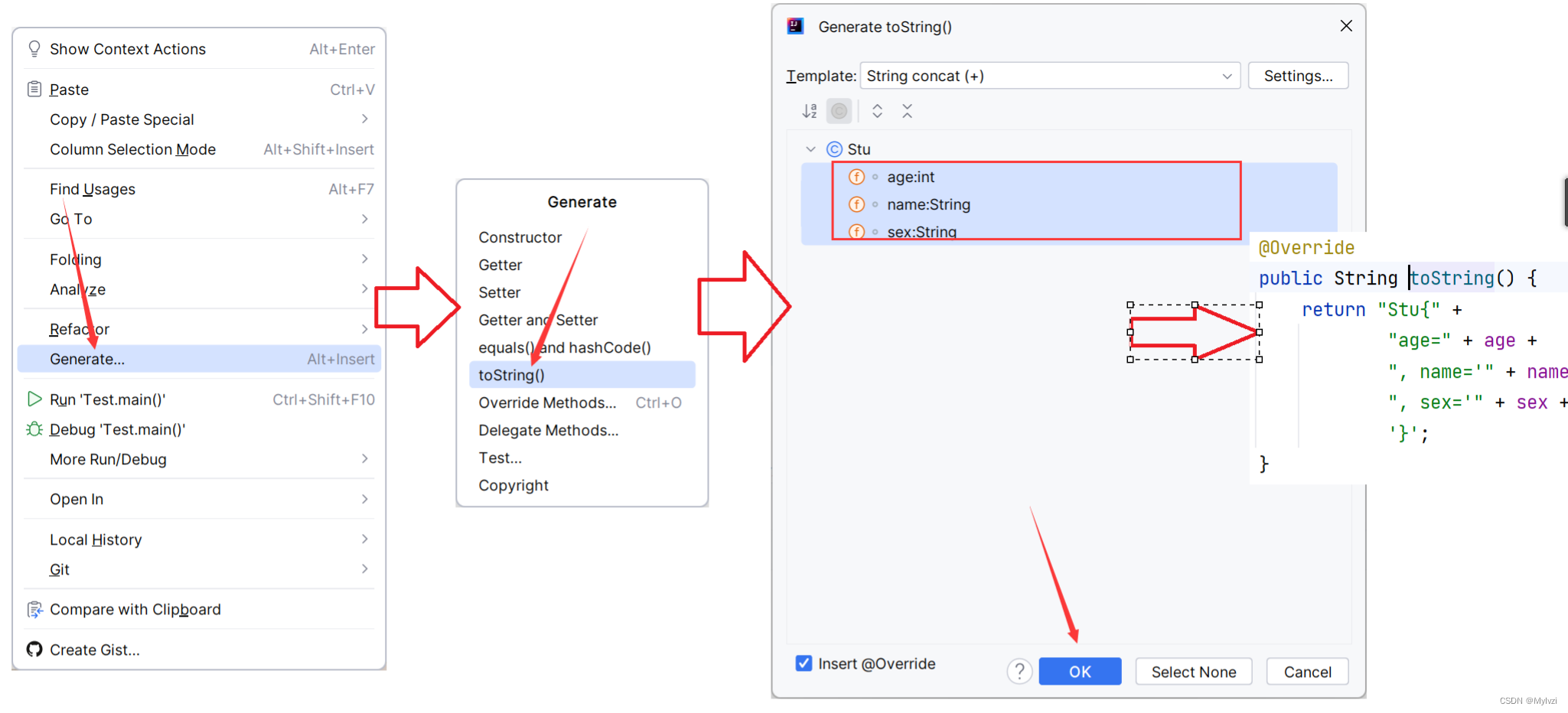
Java学习之--类和对象
💕粗缯大布裹生涯,腹有诗书气自华💕 作者:Mylvzi 文章主要内容:Java学习之--类和对象 类和对象 类的实例化: 1.什么叫做类的实例化 利用类创建一个具体的对象就叫做类的实例化! 当我们创建了…...
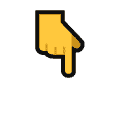
Unity技术手册-UGUI零基础详细教程-Canvas详解
点击跳转专栏>Unity3D特效百例点击跳转专栏>案例项目实战源码点击跳转专栏>游戏脚本-辅助自动化点击跳转专栏>Android控件全解手册点击跳转专栏>Scratch编程案例点击跳转>软考全系列点击跳转>蓝桥系列 👉关于作者 专注于Android/Unity和各种游…...
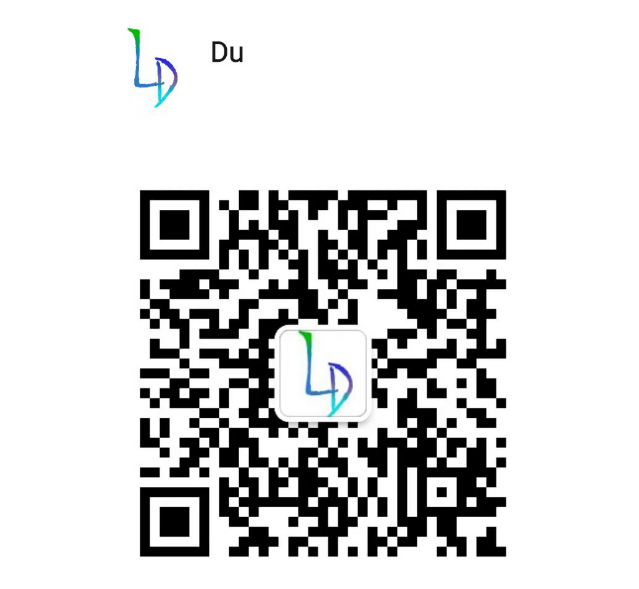
破天荒呀!小杜微信有名额了
写在前面 小杜粉,众所周知前面加小杜微信为好友的基本都是由名额限制的。一般都是付费进入社群且进行备注,小杜才会长期保留微信好友。主要由于,添加的人数太多了,微信账号人数名额有限。因此,小杜过一段时间…...
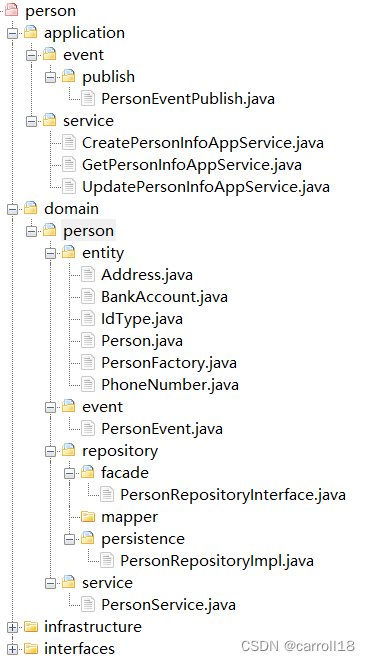
领域驱动设计:领域模型与代码模型的一致性
文章目录 领域对象的整理从领域模型到微服务的设计领域层的领域对象应用层的领域对象 领域对象与微服务代码对象的映射典型的领域模型非典型领域模型 DDD 强调先构建领域模型然后设计微服务,以保证领域模型和微服务的一体性,因此我们不能脱离领域模型来谈…...

内蒙古网站建设流程/关键词有哪些关联词
Paul Jaquays是一个多面手 Paul Jaquays是一个多面手,他在id Software里为QuakeⅡ设计关卡,并为QuakeⅢ做游戏和关卡设计。现在,还有谁不愿用他的东西呢?当Jaquays了解到Saqes的秘决后,他想出版一个关卡设计规则方面的…...
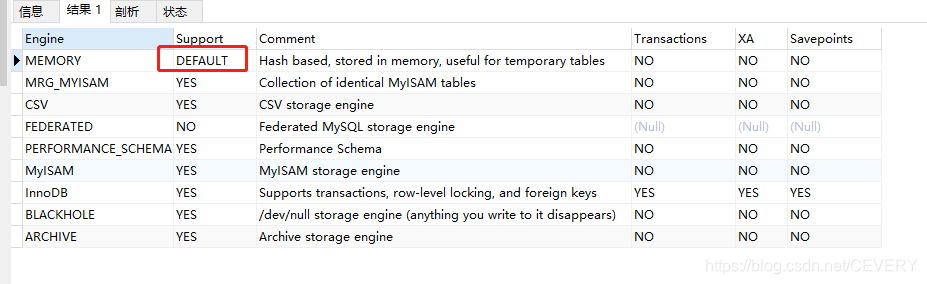
时尚杂志排版设计/seo实战密码第四版pdf
一、常用命令 1.1 查看当前默认存储引擎 show VARIABLES like "%storage_engine%";1.2 查看所有存储引擎 SHOW engines;1.3 临时修改存储引擎 SET default_storage_engineMEMORY;show ENGINES;二、介绍理解 2.1 InnoDB存储引擎 InnoDB是事务型数据库的首选引擎…...

html5做手机网站/有了域名如何建立网站
给你两个二进制字符串,返回它们的和(用二进制表示)。 输入为 非空 字符串且只包含数字 1 和 0。 示例 1: 输入: a "11", b "1" 输出: "100"示例 2: 输入: a "1010", b "1011" 输出…...

wordpress 主题 对比/网络营销方案范文
ELK 5.X 环境搭建与常用插件安装 环境介绍: ip: 192.168.250.131 os: CentOS 7.1.1503 (Core) 内存不要给的太低,至少4G吧,否则elasticsearch启动会报错。 软件及其版本 这里软件包都解压在了/opt下,注意! logstash-5.…...

做网站好的书/企业网站推广外包
文章转自:https://blog.csdn.net/github_38885296/article/details/85272964 众所周知, 软件开发时遵守一个规范的设计模式非常重要, 学习行业内主流的design pattern往往能够为你节省大部分时间. 根据我2年的全栈经验, 在Web应用程序领域最流行的, 并且若干年内不…...

卡密wordpress插件/网页推广平台
使用ajax跳转方法时,页面ctrlshifti调试报告了一个404错误,说找不到方法。页面地址栏直接指向方法的地址跳转也是404。目标方法是新增的,于是使用复制黏贴,确定各处方法名称一致之后,重启server。 调试时再次报错&…...