C# 实现迷宫游戏
智能提示:
/// <summary>/// 迷宫/// </summary>internal class Maze : IDisposable{private MazeCell[,] cells;private readonly Stack<MazeCell> stack = new Stack<MazeCell>();private readonly Random rand = new Random();private int _width, _height;private Bitmap mazeBitmap; // 用于保存迷宫的位图private float cellWidth;private float cellHeight;private Point playerPosition;private float playerRadius;private bool _isMove = true;private int _canvasWidth;private int _canvasHeight;private MazeType _mazeType = MazeType.Default;public Maze(){}public Bitmap MazeBitmap => mazeBitmap;public bool IsMove => _isMove;public MazeType MazeType => _mazeType;public int CanvasWidth{get => _canvasWidth;set{_canvasWidth = value;}}public int CanvasHeight{get => _canvasHeight;set { _canvasHeight = value; }}private void GenerateMaze(MazeType mazeType){switch(mazeType){case MazeType.Default:GenerateMaze_RecursiveBacktracking();break;case MazeType.DFS:GenerateMaze_DFS();break;case MazeType.Prim:GenerateMaze_Prim();break;case MazeType.RecursiveDivision:GenerateMaze_RecursiveDivision();break;case MazeType.RecursiveBacktracking:GenerateMaze_RecursiveBacktracking();break;}}/// <summary>/// 获取方向/// </summary>/// <returns></returns>private IEnumerable<Tuple<int, int>> GetDirections(){yield return Tuple.Create(-1, 0);yield return Tuple.Create(1, 0);yield return Tuple.Create(0, -1);yield return Tuple.Create(0, 1);}#region 深度优先搜索算法private void GenerateMaze_DFS(){// 选择迷宫的左上角的点作为起始点int startX = 0;int startY = 0;// 使用DFS生成迷宫GenerateMaze(startX, startY);// 将起始点的左面的墙壁设为入口cells[startX, startY].LeftWall = false;// 找到迷宫的一个最远的边缘点,将它的边缘的墙壁设为出口int maxDist = 0;int endX = 0, endY = 0;bool isBottomEdge = false;for (int x = 0; x < _width; x++){for (int y = 0; y < _height; y++){int dist = Math.Abs(x - startX) + Math.Abs(y - startY);if (dist > maxDist && (x == 0 || y == 0 || x == _width - 1 || y == _height - 1)){maxDist = dist;endX = x;endY = y;isBottomEdge = (y == _height - 1);}}}if (isBottomEdge)cells[endX, endY].BottomWall = false;elsecells[endX, endY].RightWall = false;}private void GenerateMaze(int x, int y){// 标记当前点已被访问cells[x, y].Visited = true;var tempData = GetDirections().OrderBy(_ => rand.Next());// 随机访问四个方向foreach (var dir in tempData){int newX = x + dir.Item1, newY = y + dir.Item2;if (newX >= 0 && newX < _width && newY >= 0 && newY < _height && !cells[newX, newY].Visited){// 移除两个单元格之间的墙壁if (dir.Item1 == -1){cells[x, y].LeftWall = false;cells[newX, newY].RightWall = false;}else if (dir.Item1 == 1){cells[x, y].RightWall = false;cells[newX, newY].LeftWall = false;}else if (dir.Item2 == -1){cells[x, y].TopWall = false;cells[newX, newY].BottomWall = false;}else if (dir.Item2 == 1){cells[x, y].BottomWall = false;cells[newX, newY].TopWall = false;}// 递归访问下一个点GenerateMaze(newX, newY);}}}#endregion#region 普里姆算法private void GenerateMaze_Prim(){// 选择迷宫的一个随机点作为起始点int startX = rand.Next(_width);int startY = rand.Next(_height);cells[startX, startY].Visited = true;// 初始化边缘列表,包含起始点的所有邻居Queue<MazeCell> frontier = new Queue<MazeCell>();AddUnvisitedNeighborsToFrontier(cells[startX, startY], frontier);// 使用Prim算法生成迷宫while (frontier.Count > 0){// 从边缘列表中选择一个单元格,更倾向于选择最早添加的单元格var cell = frontier.Dequeue();// 找到与这个单元格相邻的已访问的单元格var neighbors = GetVisitedNeighbors(cell);if (neighbors.Count > 0){// 随机选择一个已访问的邻居var neighbor = neighbors[rand.Next(neighbors.Count)];// 移除两个单元格之间的墙壁if (cell.X > neighbor.Item2.X) // 如果邻居在当前单元格的左侧{cell.LeftWall = false;neighbor.Item2.RightWall = false;}else if (cell.X < neighbor.Item2.X) // 如果邻居在当前单元格的右侧{cell.RightWall = false;neighbor.Item2.LeftWall = false;}else if (cell.Y > neighbor.Item2.Y) // 如果邻居在当前单元格的上方{cell.TopWall = false;neighbor.Item2.BottomWall = false;}else if (cell.Y < neighbor.Item2.Y) // 如果邻居在当前单元格的下方{cell.BottomWall = false;neighbor.Item2.TopWall = false;}// 将这个单元格标记为已访问,并将它的所有未访问的邻居添加到边缘列表中cell.Visited = true;AddUnvisitedNeighborsToFrontier(cell, frontier);}}}private void AddUnvisitedNeighborsToFrontier(MazeCell cell, Queue<MazeCell> frontier){foreach (var dir in GetDirections()){int newX = cell.X + dir.Item1, newY = cell.Y + dir.Item2;if (newX >= 0 && newX < _width && newY >= 0 && newY < _height && !cells[newX, newY].Visited && !frontier.Contains(cells[newX, newY]))frontier.Enqueue(cells[newX, newY]);}}private List<Tuple<int, MazeCell>> GetVisitedNeighbors(MazeCell cell){var visitedNeighbors = new List<Tuple<int, MazeCell>>();foreach (var dir in GetDirections()){int newX = cell.X + dir.Item1, newY = cell.Y + dir.Item2;if (newX >= 0 && newX < _width && newY >= 0 && newY < _height && cells[newX, newY].Visited)visitedNeighbors.Add(Tuple.Create(dir.Item1, cells[newX, newY]));}return visitedNeighbors;}#endregion#region 递归除法算法private void GenerateMaze_RecursiveDivision(){// 初始化迷宫,所有的墙都被移除for (int x = 0; x < _width; ++x){for (int y = 0; y < _height; ++y){cells[x, y].TopWall = y == 0;cells[x, y].BottomWall = y == _height - 1;cells[x, y].LeftWall = x == 0;cells[x, y].RightWall = x == _width - 1;}}// 递归分割迷宫Divide(0, 0, _width - 1, _height - 1);}private void Divide(int x, int y, int width, int height){if (width < 3 || height < 3)return;bool horizontal = rand.Next(2) == 0;if (horizontal){// 横向分割int splitY = y + 2 + rand.Next(height - 3);int holeX = x + rand.Next(width);for (int i = x; i < x + width; ++i){if (i != holeX){cells[i, splitY].BottomWall = true;if (splitY + 1 < _height){cells[i, splitY + 1].TopWall = true;}}}Divide(x, y, width, splitY - y);Divide(x, splitY + 1, width, y + height - splitY - 1);}else{// 纵向分割int splitX = x + 2 + rand.Next(width - 3);int holeY = y + rand.Next(height);for (int i = y; i < y + height; ++i){if (i != holeY){cells[splitX, i].RightWall = true;if (splitX + 1 < _width){cells[splitX + 1, i].LeftWall = true;}}}Divide(x, y, splitX - x, height);Divide(splitX + 1, y, x + width - splitX - 1, height);}}#endregion#region 时间回溯算法private void GenerateMaze_RecursiveBacktracking(){// 初始化迷宫,所有的墙都存在for (int x = 0; x < _width; ++x){for (int y = 0; y < _height; ++y){cells[x, y].TopWall = true;cells[x, y].BottomWall = true;cells[x, y].LeftWall = true;cells[x, y].RightWall = true;}}// 递归生成迷宫VisitCell(rand.Next(_width), rand.Next(_height));}private void VisitCell(int x, int y){// 标记当前单元格为已访问cells[x, y].Visited = true;// 对邻居单元格的顺序进行随机排序foreach (var dir in GetDirections().OrderBy(d => rand.Next())){int nx = x + dir.Item1;int ny = y + dir.Item2;// 如果邻居单元格在迷宫内并且未被访问过,则移除墙并递归访问邻居单元格if (nx >= 0 && ny >= 0 && nx < _width && ny < _height && !cells[nx, ny].Visited){RemoveWall(x, y, dir);RemoveWall(nx, ny, Tuple.Create(-dir.Item1, -dir.Item2));VisitCell(nx, ny);}}}private void RemoveWall(int x, int y, Tuple<int, int> direction){if (direction.Equals(Tuple.Create(-1, 0))) // Left{cells[x, y].LeftWall = false;}else if (direction.Equals(Tuple.Create(1, 0))) // Right{cells[x, y].RightWall = false;}else if (direction.Equals(Tuple.Create(0, -1))) // Up{cells[x, y].TopWall = false;}else if (direction.Equals(Tuple.Create(0, 1))) // Down{cells[x, y].BottomWall = false;}}#endregionpublic void CreateMaze(int width, int height, int canvasWidth, int canvasHeight, MazeType mazeType= MazeType.Default,bool createOrUpdate=true){mazeBitmap?.Dispose();_isMove = true;if (createOrUpdate){playerPosition = new Point(0, 0); // 初始位置在迷宫的左上角stack.Clear();_width = width;_height = height;cells = new MazeCell[width, height];mazeBitmap = new Bitmap(width, height);_mazeType = mazeType;for (int x = 0; x < width; x++){for (int y = 0; y < height; y++){cells[x, y] = new MazeCell(x, y);}}}GenerateMaze(mazeType);// 生成迷宫后,将其绘制到位图上mazeBitmap = new Bitmap(canvasWidth, canvasHeight);using (var g = Graphics.FromImage(mazeBitmap)){DrawMaze(g, canvasWidth, canvasHeight);}}private void DrawMaze(Graphics g, int canvasWidth, int canvasHeight){int tempW = canvasWidth - 1;_canvasWidth = tempW;_canvasHeight = canvasHeight - 1;cellWidth = (float)_canvasWidth / _width;cellHeight = (float)_canvasHeight / _height;playerRadius = Math.Min(cellWidth, cellHeight) / 4;float lineWidth = 1f; // 线条的宽度float halfLineWidth = lineWidth / 2f; // 线条宽度的一半g.InterpolationMode = InterpolationMode.HighQualityBicubic;g.CompositingQuality = CompositingQuality.HighQuality;//g.SmoothingMode = SmoothingMode.AntiAlias;// 先绘制所有的垂直线for (int x = 0; x <= _width; x++){float left = x * cellWidth;for (int y = 0; y < _height; y++){var cell = cells[Math.Min(x, _width - 1), y];float top = y * cellHeight;float bottom = (y + 1) * cellHeight;if ((cell.LeftWall || x == _width) && !(x == _width && y == _height - 1))g.DrawLine(Pens.Black, left, top - halfLineWidth, left, bottom + halfLineWidth);}}// 再绘制所有的水平线for (int y = 0; y <= _height; y++){float top = y * cellHeight;for (int x = 0; x < _width; x++){var cell = cells[x, Math.Min(y, _height - 1)];float left = x * cellWidth;float right = (x + 1) * cellWidth;if ((cell.TopWall || y == _height) && !(x == _width - 1 && y == _height))g.DrawLine(Pens.Black, left - halfLineWidth, top, right + halfLineWidth, top);}}}public void Draw(Graphics g, int canvasWidth, int canvasHeight){if (cells == null)return;int tempW = canvasWidth - 1;if (tempW != _canvasWidth){CreateMaze(_width, _height, canvasWidth, canvasHeight, MazeType,false);}// 首先,绘制保存的迷宫位图g.DrawImage(mazeBitmap, 0, 0, canvasWidth, canvasHeight);// 在玩家位置处绘制一个小黑圆float playerX = (playerPosition.X + 0.5f) * cellWidth; // 玩家的X坐标float playerY = (playerPosition.Y + 0.5f) * cellHeight; // 玩家的Y坐标g.FillEllipse(Brushes.Black, playerX - playerRadius, playerY - playerRadius, 2 * playerRadius, 2 * playerRadius);// 在出口处写上"出口"//Font font = new Font("Arial", 16); // 设置字体和大小//float exitX = (_width - 2f) * cellWidth; // 出口的X坐标//float exitY = (_height - 1f) * cellHeight; // 出口的Y坐标//g.DrawString("出口", font, Brushes.Black, exitX, exitY);}public MoveResult Move(KeyEventArgs e){if (cells == null || !_isMove)return new MoveResult();Point newPosition = playerPosition;switch (e.KeyCode){case Keys.Up:newPosition.Y--;break;case Keys.Down:newPosition.Y++;break;case Keys.Left:newPosition.X--;break;case Keys.Right:newPosition.X++;break;}return Move(newPosition);}public MoveResult Move(Point newPosition){// 计算小黑点移动前后的矩形区域Rectangle oldRect = GetPlayerRect(playerPosition);bool status = false;if (newPosition.X < 0 || newPosition.Y < 0){goto Result;}int directionX = newPosition.X - playerPosition.X;if (directionX != 0){if (directionX > 0){if (newPosition.X < _width && !cells[playerPosition.X, playerPosition.Y].RightWall && !cells[newPosition.X, newPosition.Y].LeftWall){playerPosition = newPosition;status = true;goto Result;}}else{if (newPosition.X >= 0 && !cells[playerPosition.X, playerPosition.Y].LeftWall && !cells[newPosition.X, newPosition.Y].RightWall){playerPosition = newPosition;status = true;goto Result;}}}int directionY = newPosition.Y - playerPosition.Y;if (directionY != 0){if (directionY > 0){if (newPosition.Y < _height && !cells[playerPosition.X, playerPosition.Y].BottomWall && !cells[newPosition.X, newPosition.Y].TopWall){playerPosition = newPosition;status = true;goto Result;}}else{if (newPosition.Y >= 0 && !cells[playerPosition.X, playerPosition.Y].TopWall && !cells[newPosition.X, newPosition.Y].BottomWall){playerPosition = newPosition;status = true;goto Result;}}}// goto Result;Result:Rectangle newRect = GetPlayerRect(newPosition);bool isWin = playerPosition.X == _width - 1 && playerPosition.Y == _height - 1;_isMove = !isWin;return new MoveResult{IsInvalidate = status,IsWin = isWin,OldRect = oldRect,NewRect = newRect};}private Rectangle GetPlayerRect(Point position){int x = (int)Math.Round(position.X * cellWidth, 0);int y = (int)Math.Round(position.Y * cellHeight, 0);return new Rectangle(x, y, (int)Math.Round(cellWidth, 0), (int)Math.Round(cellHeight, 0));}public Bitmap DrawPath(Bitmap bitmap){if (mazeBitmap == null)return null;var path = FindPath();if (bitmap == null)bitmap = new Bitmap(_canvasWidth, _canvasHeight);// 创建一个Graphics对象using (Graphics g = Graphics.FromImage(bitmap)){// 绘制路径if (path != null){var pathPen = new Pen(Color.Red, 2); // 使用红色画笔来绘制路径for (int i = 0; i < path.Count - 1; i++){float x1 = (path[i].X + 0.5f) * cellWidth;float y1 = (path[i].Y + 0.5f) * cellHeight;float x2 = (path[i + 1].X + 0.5f) * cellWidth;float y2 = (path[i + 1].Y + 0.5f) * cellHeight;g.DrawLine(pathPen, x1, y1, x2, y2);}}}return bitmap;}public List<MazeCell> FindPath(){var start = cells[0, 0];var end = cells[_width - 1, _height - 1];var queue = new Queue<MazeCell>();var prev = new Dictionary<MazeCell, MazeCell>();queue.Enqueue(start);while (queue.Count > 0){var cell = queue.Dequeue();if (cell == end){var path = new List<MazeCell>();while (cell != start){path.Add(cell);cell = prev[cell];}path.Add(start);path.Reverse();return path;}foreach (var neighbor in GetNeighbors(cell)){if (prev.ContainsKey(neighbor))continue;prev[neighbor] = cell;queue.Enqueue(neighbor);}}return null; // 没有找到路径}private IEnumerable<MazeCell> GetNeighbors(MazeCell cell){var neighbors = new List<MazeCell>();if (cell.X > 0 && !cell.LeftWall)neighbors.Add(cells[cell.X - 1, cell.Y]);if (cell.X < _width - 1 && !cell.RightWall)neighbors.Add(cells[cell.X + 1, cell.Y]);if (cell.Y > 0 && !cell.TopWall)neighbors.Add(cells[cell.X, cell.Y - 1]);if (cell.Y < _height - 1 && !cell.BottomWall)neighbors.Add(cells[cell.X, cell.Y + 1]);return neighbors;}public void Dispose(){mazeBitmap?.Dispose();}~Maze(){Dispose();}}public class MazeCell{public int X { get; set; }public int Y { get; set; }public bool Visited { get; set; }public bool TopWall = true, BottomWall = true, LeftWall = true, RightWall = true;public MazeCell(int x, int y){X = x;Y = y;Visited = false;TopWall = BottomWall = LeftWall = RightWall = true;}}public class MoveResult{public bool IsInvalidate { get; set; }public Rectangle OldRect { get; set; }public Rectangle NewRect { get; set; }public bool IsWin { get; set; }}public enum MazeType{/// <summary>/// 默认RecursiveBacktracking/// </summary>Default,/// <summary>/// 深度优先搜索算法/// </summary>DFS,/// <summary>/// 普里姆算法/// </summary>Prim,/// <summary>/// 递归除法算法/// </summary>RecursiveDivision,/// <summary>/// 递归回溯算法/// </summary>RecursiveBacktracking}
FrmMain主窗体:
public partial class FrmMain : Form{private readonly Maze _maze;private System.Windows.Forms.Timer _timer;public FrmMain(){InitializeComponent();this.SetStyle(ControlStyles.DoubleBuffer |ControlStyles.UserPaint |ControlStyles.AllPaintingInWmPaint,true);this.UpdateStyles();_maze = new Maze();}private void FrmGame_Load(object sender, EventArgs e){this.KeyPreview = true;BindType(typeof(MazeType), this.cbMazeType, "Default");}private void BindType(Type type, ComboBox comboBox, string defaultValue){var enumValues = Enum.GetValues(type);var list = new List<IdValues>();int index = 0, curIndex = 0;foreach (Enum value in enumValues){int hc = value.GetHashCode();list.Add(new IdValues{Id = hc.ToString(),Value = value.ToString(),Standby = hc});if (value.ToString() == defaultValue)index = curIndex;curIndex++;}comboBox.ValueMember = "Id";comboBox.DisplayMember = "Value";comboBox.DataSource = list;comboBox.SelectedIndex = index;}private void FrmGame_FormClosing(object sender, FormClosingEventArgs e){_maze.Dispose();this.Dispose();}private bool _isPlayGame = false;public bool IsPlayGame{get => _isPlayGame;set{if (_isPlayGame == value)return;_isPlayGame = value;if (value){btnPlayGame.ExecBeginInvoke(() =>{btnPlayGame.Text = "重新开始";});}else{btnPlayGame.ExecBeginInvoke(() =>{btnPlayGame.Text = "开启游戏";});}}}private void btnPlayGame_Click(object sender, EventArgs e){if (IsPlayGame){if (MessageBox.Show("正在游戏中,确认重新开始吗?", "迷宫游戏提示", MessageBoxButtons.OKCancel, MessageBoxIcon.Question) == DialogResult.Cancel){plGame.Focus();return;}}if (_timer != null){_timer.Stop();_timer.Dispose();_timer = null;}_isAutoMove = false;IsPlayGame = true;int w = 10, h = 8;if (rbEasy.Checked){w = 30;h = 21;}else if (rbMedium.Checked){w = 66;h = 45;}else{w = 100;h = 67;}using var g = plGame.CreateGraphics();MazeType mazeType = (MazeType)(this.cbMazeType.Items[cbMazeType.SelectedIndex] as IdValues).Standby;_maze.CreateMaze(w, h, plGame.Width, plGame.Height, mazeType);plGame.Controls.Clear();g.Clear(plGame.BackColor);_maze.Draw(g, plGame.Width, plGame.Height);_timer = new System.Windows.Forms.Timer();_timer.Interval = 1000;time = 0;_timer.Tick += timer_Tick;_timer.Start();plGame.Focus();}long time = 0;private void timer_Tick(object? sender, EventArgs e){lblTime.ExecBeginInvoke(() =>{lblTime.Text = Compute(++time);});}public string Compute(long time){if (time < 60)return $"00:{ChangeString(time)}";long minute = time / 60;if (minute < 60)return $"{ChangeString(minute)}:{ChangeString(time % 60)}";long hour = minute / 60;return $"{ChangeString(hour)}:{Compute(time - hour * 3600)}";}private string ChangeString(long val){return val.ToString("D2");}private void plGame_Paint(object sender, PaintEventArgs e){plGame.Controls.Clear();e.Graphics.Clear(plGame.BackColor);_maze.Draw(e.Graphics, plGame.Width, plGame.Height);}protected override void OnKeyDown(KeyEventArgs e){if (_isAutoMove)return;base.OnKeyDown(e);var result = _maze.Move(e);RefreshResult(result);}private void RefreshResult(MoveResult result){if (result.IsInvalidate){plGame.ExecInvoke(() =>{// 重绘迷宫plGame.Invalidate(result.OldRect);plGame.Invalidate(result.NewRect);});if (result.IsWin){IsPlayGame = false;if (_timer != null){_timer.Stop();_timer.Dispose();_timer = null;}MessageBox.Show("通过", "迷宫通过提示");}}}private void FrmMain_Activated(object sender, EventArgs e){plGame.Focus();}/// <summary>/// 提示/// </summary>/// <param name="sender"></param>/// <param name="e"></param>private void btnPrompt_Click(object sender, EventArgs e){if (_maze.MazeBitmap == null){return;}Bitmap bmp = new Bitmap(plGame.Width, plGame.Height);plGame.DrawToBitmap(bmp, new Rectangle(0, 0, bmp.Width, bmp.Height));int size = 0;if (rbEasy.Checked)size = 0;else if (rbMedium.Checked)size = 1;elsesize = 2;FrmPrompt frmPrompt = new FrmPrompt(_maze.DrawPath(bmp), size);frmPrompt.Show();plGame.Focus();}private bool _isAutoMove = false;/// <summary>/// 一键通过/// </summary>/// <param name="sender"></param>/// <param name="e"></param>private void btnPass_Click(object sender, EventArgs e){if (!_maze.IsMove)return;_isAutoMove = true;Task.Run(() =>{var path = _maze.FindPath();if (path != null){Point point = new Point(0, 0);foreach (var item in path){if (!_isAutoMove)break;point.X = item.X;point.Y = item.Y;var result = _maze.Move(point);RefreshResult(result);plGame.ExecInvoke(() =>{plGame.Update();});Thread.Sleep(50);}}_isAutoMove = false;});}private void plGame_Resize(object sender, EventArgs e){}}public class IdValues{public string Id { get; set; }public string Value { get; set; }public string Value2 { get; set; }public string Value3 { get; set; }public string Value4 { get; set; }public string Value5 { get; set; }public int Standby { get; set; }public static bool operator ==(IdValues idValues, IdValues idValues2){return idValues.Equals(idValues2);}public static bool operator !=(IdValues idValues, IdValues idValues2){return !idValues.Equals(idValues2);}public override int GetHashCode(){var code = (Id, Value, Value2, Value3, Value4, Value5, Standby).GetHashCode();return code;}public override bool Equals(object? obj){return obj?.GetHashCode() == GetHashCode();}const int TARGET = 0x1F;/// <summary>/// 将连续字段的哈希代码左移两位或更多位来加权各个哈希代码(最佳情况下,超出位 31 的位应环绕,而不是被丢弃)/// </summary>/// <param name="value"></param>/// <param name="positions"></param>/// <returns></returns>public int ShiftAndWrap(int value, int positions = 3){positions &= TARGET;uint number = BitConverter.ToUInt32(BitConverter.GetBytes(value), 0);uint wrapped = number >> (32 - positions);return BitConverter.ToInt32(BitConverter.GetBytes((number << positions) | wrapped), 0);}}
相关文章:
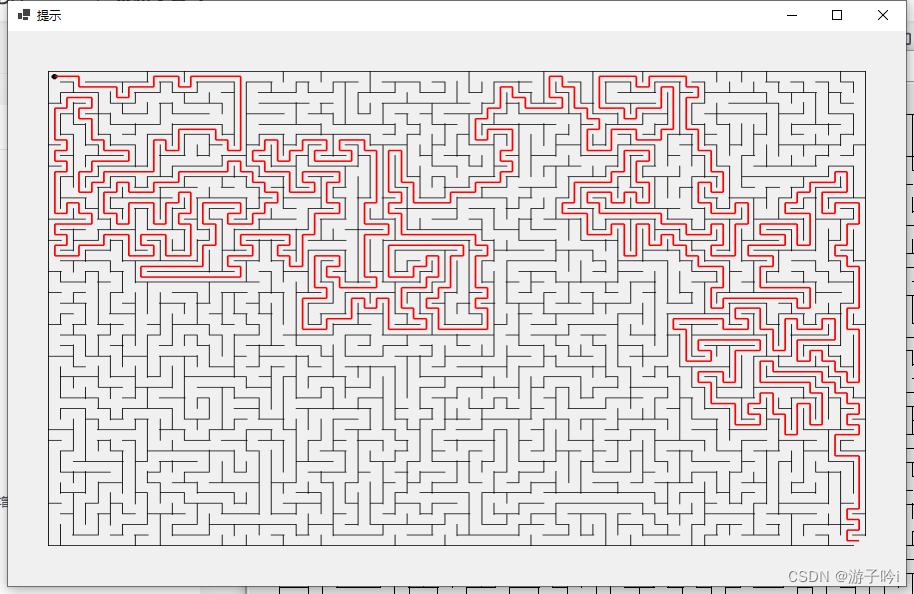
C# 实现迷宫游戏
智能提示: /// <summary>/// 迷宫/// </summary>internal class Maze : IDisposable{private MazeCell[,] cells;private readonly Stack<MazeCell> stack new Stack<MazeCell>();private readonly Random rand new Random();private int…...
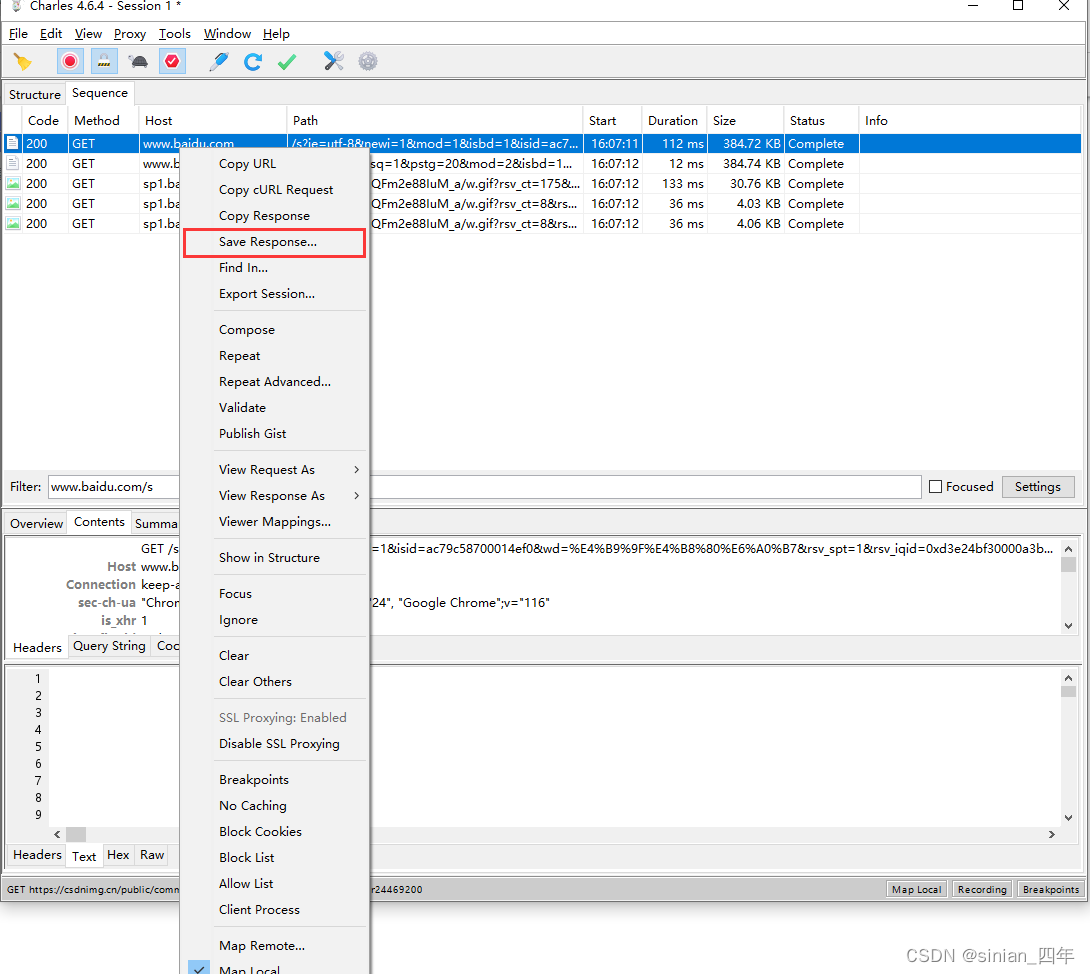
chales 重写/断点/映射/手机代理/其他主机代理
1 chales 安装和代理配置/手机代理配置/电脑代理配置 chales 安装和代理配置/手机代理配置/电脑代理配置 2 转载:Charles Rewrite重写(详解!必懂系列) Charles Rewrite重写(详解!必懂系列) 1.打开charles,点击菜单栏的Tools选中Rewrite2.…...
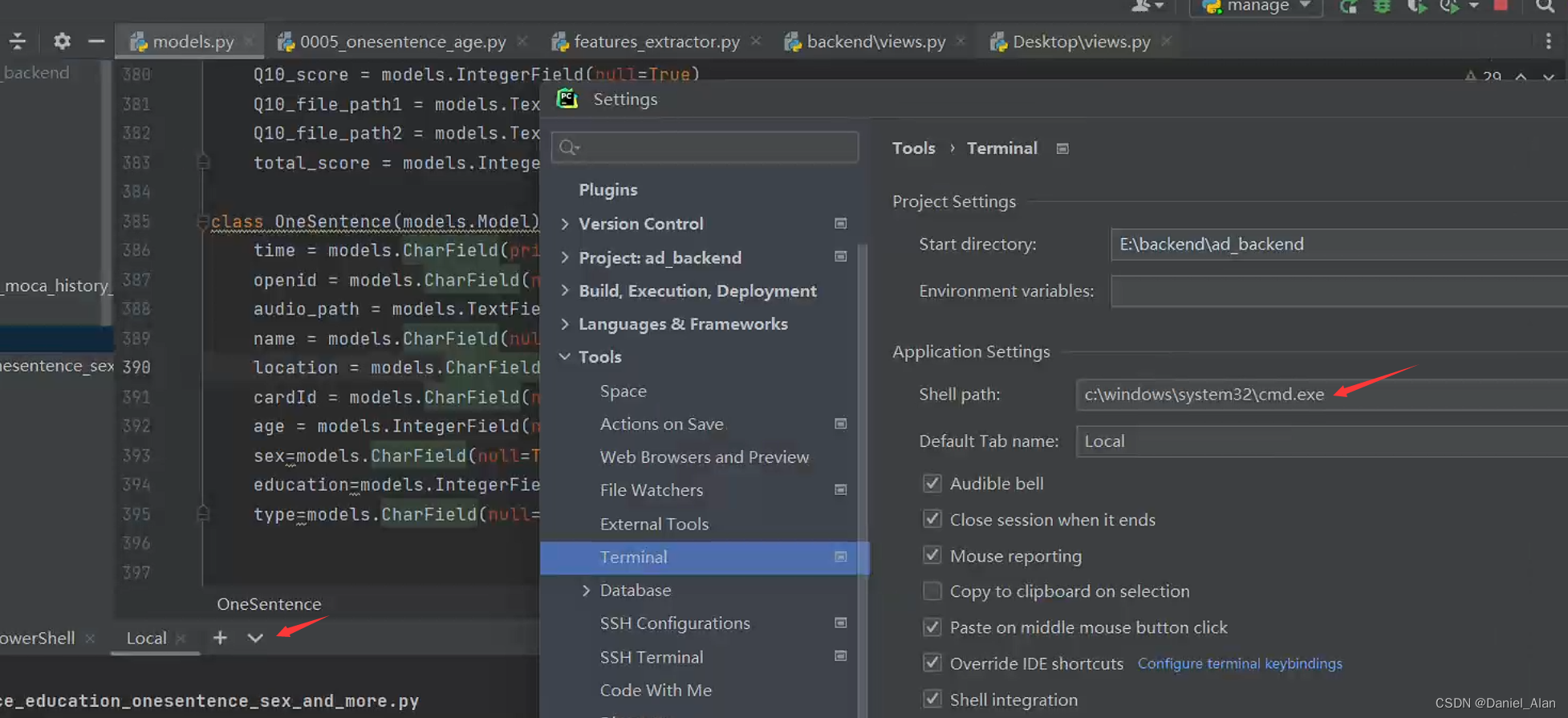
django添加数据库字段进行数据迁移
1.修改view.py里面的变量 2.在model.py新增字段 3.打开terminal并将环境切到项目所在环境,切换方式为 4.执行命令 python manage.py makemigrations backend python manage.py migrate...

flink1.15.0消费kafka 报错 The coordinator is not available.
报错 You should retry committing the latest consumed offsets. Caused by: org.apache.kafka.common.errors.CoordinatorNotAvailableException: The coordinator is not available. 但是任务还在正常跑. 开源bug [FLINK-28060] Kafka Commit on checkpointing fails re…...

2023华为杯研究生数学建模F题思路模型代码(9.22早第一时间更新)
目录 1.F题思路模型:9.7晚上比赛开始后,第一时间更新,获取见文末名片 3 全国大学生数学建模竞赛常见数模问题常见模型分类 3.1 分类问题 3.2 优化问题 详细思路见此名片,开赛第一时间更新 1.F题思路模型:9.7晚上比…...

[k8s] pod的创建过程
pod的创建过程 定义 Pod 的规范: apiVersion: v1 kind: Pod metadata:name: my-pod spec:containers:- name: my-containerimage: nginx:latest创建 Pod 对象: 使用 kubectl 命令行工具或其他客户端工具创建 Pod 对象: kubectl create -f…...
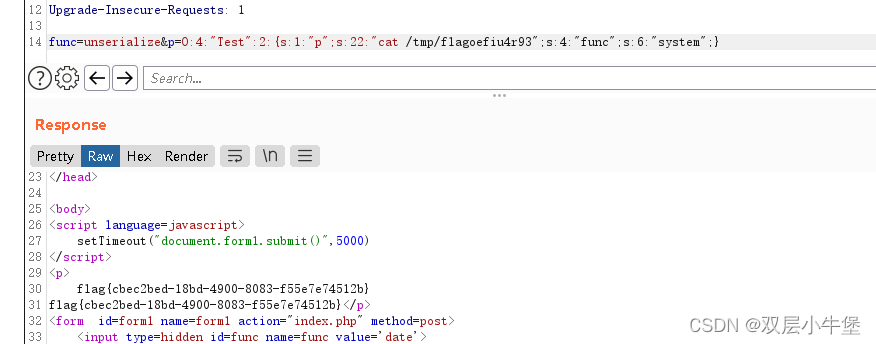
[网鼎杯 2020 朱雀组]phpweb call_user_func()
时间一跳一跳的 抓个包 很奇怪 结合上面的 date() 认为第一个是函数 我们随便输一个看看 发现过滤了 随便输一个 linux指令 发现报错了 call_user_func() 看看是啥 很容易理解 第一个参数是函数名 后面是 参数 那么这里就是 func 函数 p 数值 所以我们看看有什么办法可以…...
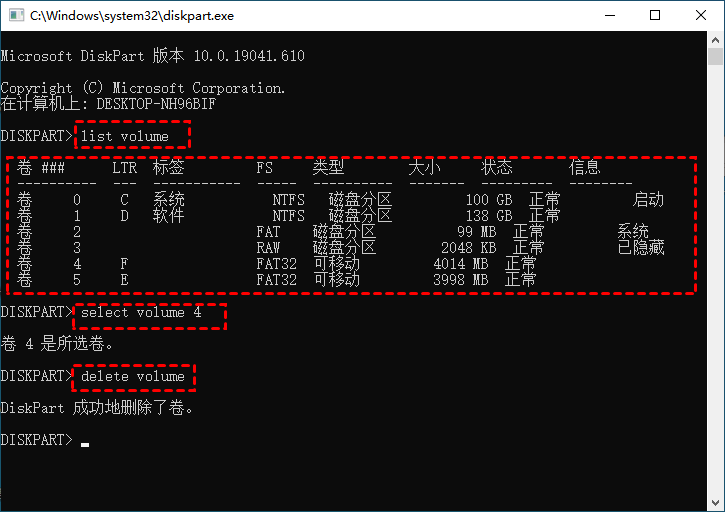
电脑怎么取消磁盘分区?
有时候,我们的电脑会出现一个磁盘爆满,但另一个却空着,这时我们可以通过取消磁盘分区来进行调整,那么,这该怎么操作呢?下面我们就来了解一下。 磁盘管理取消磁盘分区 磁盘管理是Windows自带的磁盘管理工具…...
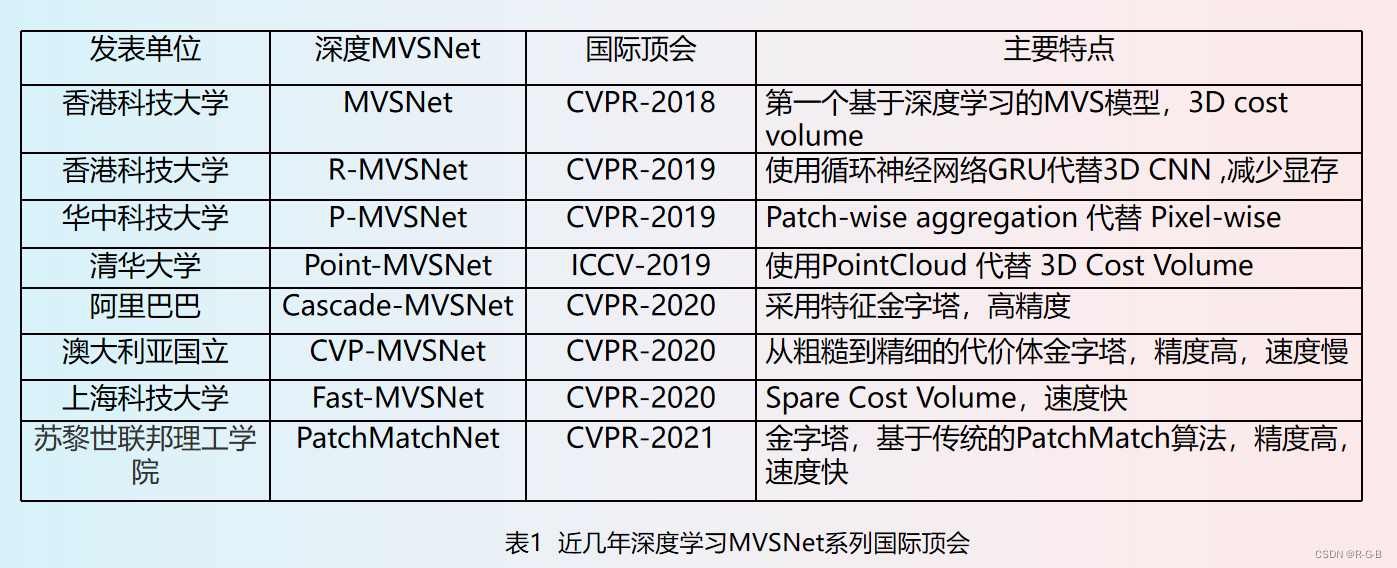
Cascade-MVSNet CVPR-2020 学习笔记总结 译文 深度学习三维重建
文章目录 4 Cascade-MVSNet CVPR-20204.0 主要特点4.1 背景介绍4.2 代价体构造回顾4.3 Cascade-MVSNet4.4 Loss的设置4.5 Cascade-MVSNet实战操作4.6 总结MVSNet系列最新顶刊 对比总结4 Cascade-MVSNet CVPR-2020 深度学习三维重建 cascade-MVSNet-CVPR-202(源码、原文、译文 …...
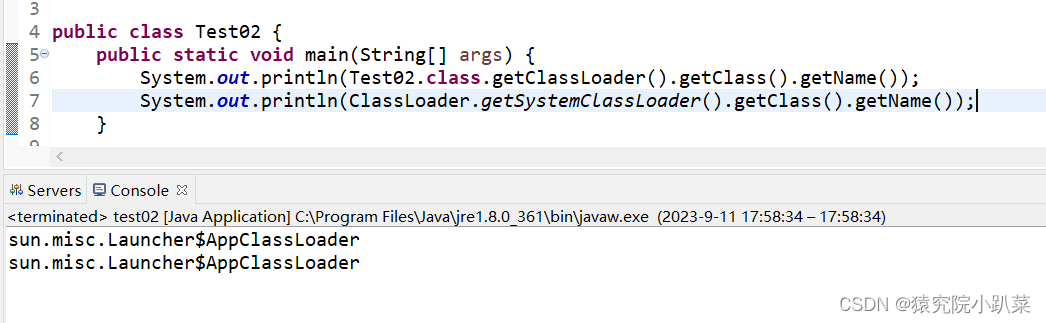
【JVM】Java类的加载机制!
一、类的生命周期 类加载过程包含:加载、验证、准备、解析和初始化 ,一共包括5 个阶段。 (1)加载: 简单来说就是将java类的字节码文件加载到机器内存中。在加载类时,Java虚拟机必须完成以下3件事情&…...
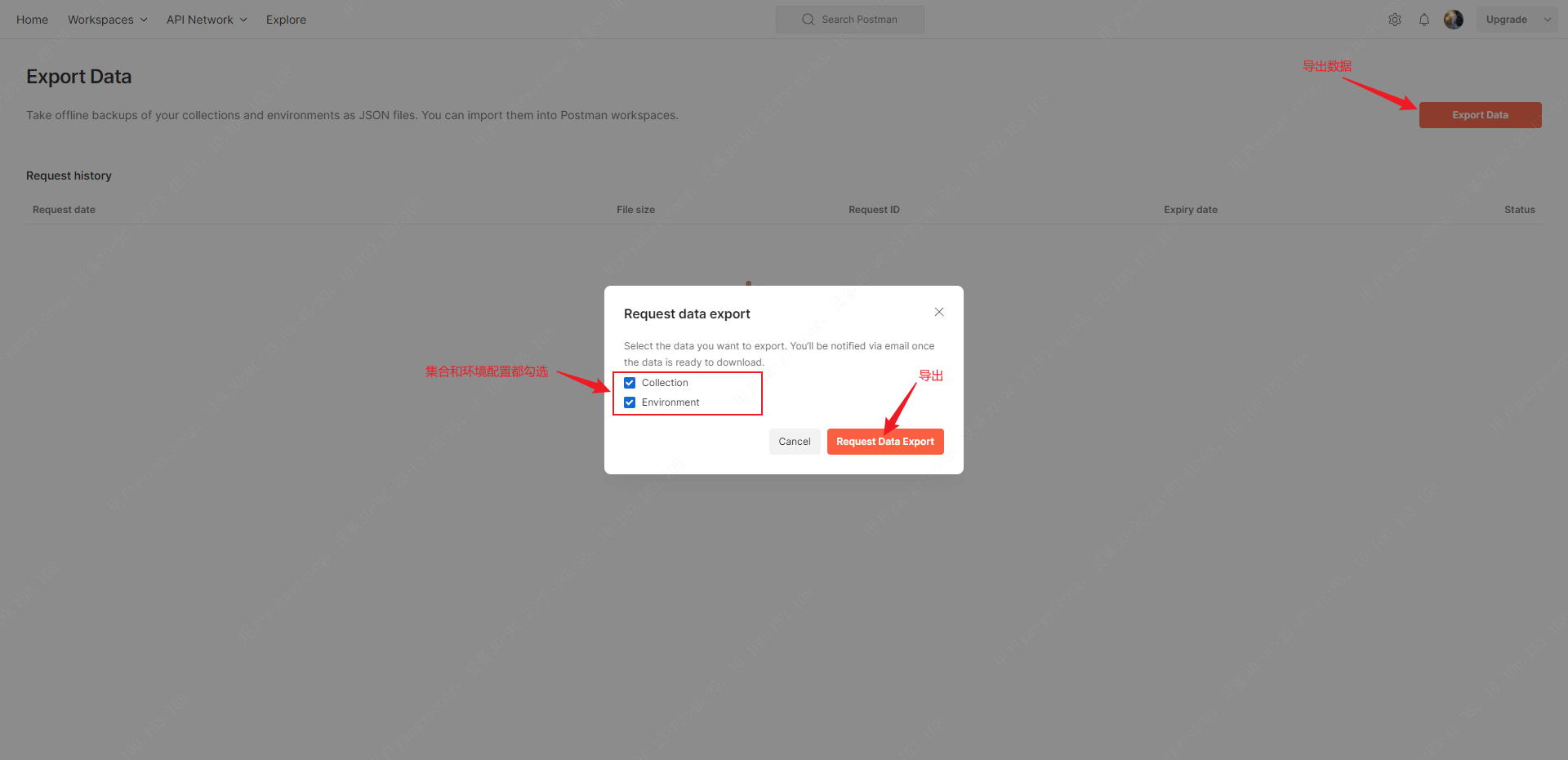
Postman使用_接口导入导出
文章目录 Postman导入数据Collections导出数据Environments导出数据Postman导出所有数据 Postman导入数据 可以导入collections(接口集)、Environments(环境配置)通过分享的链接或导出的JSON文件导入数据(还可以从第三…...

linux下centos7升级python版本
由于项目需要使用爬虫,爬虫框架支撑3.8以上版本。而linux自带的python版本是2.7.*,所以需要升级python版本至3.8 键脚本安装Python3.6-Python3.10 bash <(curl -sSL https://raw.githubusercontent.com/midoks/choose-linux-python/main/install.sh…...

Python空值None的意义
在 Python 中,有一个特殊的常量 None(N 必须大写)。和 False 不同,它不表示 0,也不表示空字符串,而表示没有值,也就是空值。 这里的空值并不代表空对象,即 None 和 [] 以及 "&q…...

什么是无线传输技术,如Wi-Fi、蓝牙和NFC的特点和应用场景
1、什么是无线传输技术,如Wi-Fi、蓝牙和NFC的特点和应用场景。 无线传输技术是指通过无线电波进行数据传输的技术。Wi-Fi、蓝牙和NFC都是常见的无线传输技术,它们的特点和应用场景如下: Wi-Fi:Wi-Fi是一种基于802.11协议的无线传…...

RUST 每日一省:全局变量
Rust中允许存在全局变量。它们一般有两种:常数和静态值。 常量 我们使用关键字 const 来创建常量。由于常量未使用关键字 let 声明,因此在创建它们时必须指定类型。常量只能进行简单赋值,并且没有固定的内存地址,无论它们在何处使…...

Arduino与Proteus仿真-WiFi网络仿真环境搭建
Arduino与Proteus网络(WiFi)仿真环境搭建 文章目录 Arduino与Proteus网络(WiFi)仿真环境搭建1、软件准备2、硬件准备3、仿真电路原理图4、仿真代码实现5、仿真结果本文将详细介绍如何在Proteus搭建Arduino的WiFi仿真环境。 1、软件准备 1)Arduino IDE或 VSCode + PlatformIO …...
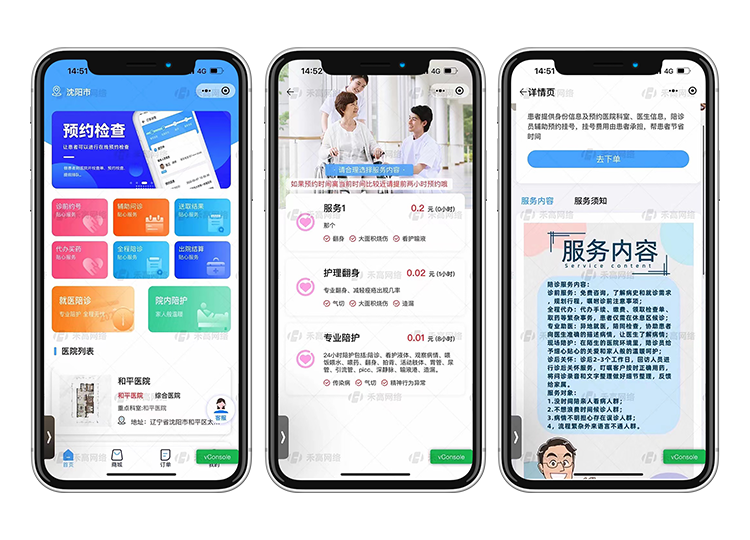
陪诊系统|陪诊软件革新医疗体验
随着科技的不断发展,陪诊小程序逐渐成为医疗行业中一股强大的力量,为患者和医护人员带来了前所未有的便捷和效益。作为一种创新的医疗服务工具,陪诊小程序在提升患者体验、优化医疗流程方面发挥着重要的作用。让我们一起来了解一下陪诊小程序…...
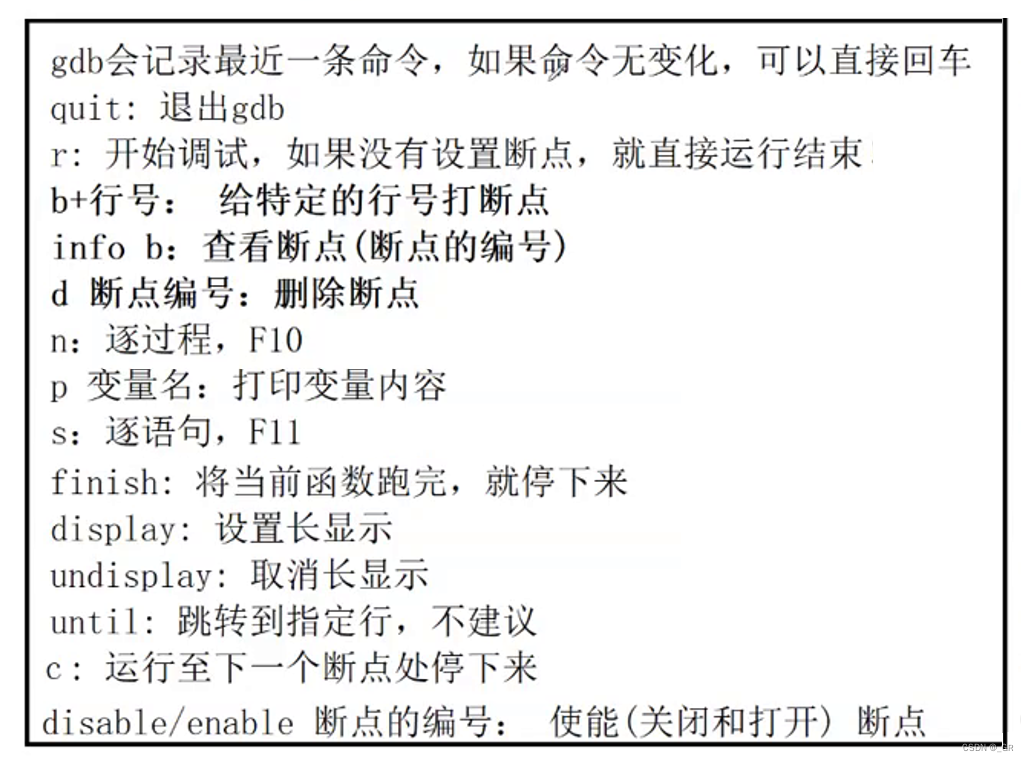
零基础Linux_5(开发工具_上)yum和vim和gcc/g++和gdb
目录 1. 软件包管理器 yum 1.1 安装软件的方式 1.2 yum 指令 2. vim(编辑器) 2.1 vim 的简单操作 2.1.1 方向键(HJKL) 2.1.2 退出 vim 2.2 vim 文本批量化操作(命令模式) 2.2.1 复制.粘贴.删除.剪贴.撤销 2.2.2 光标跳转…...

mysql建表的时候设置默认值为null会对存储和索引有影响吗?
在MySQL中,将字段的默认值设置为NULL在建表时对存储和索引可能会产生一些影响,具体取决于数据类型、索引设计和查询模式等因素。以下是可能的影响: 存储空间:如果将默认值设置为NULL,并且该字段允许存储NULL值…...
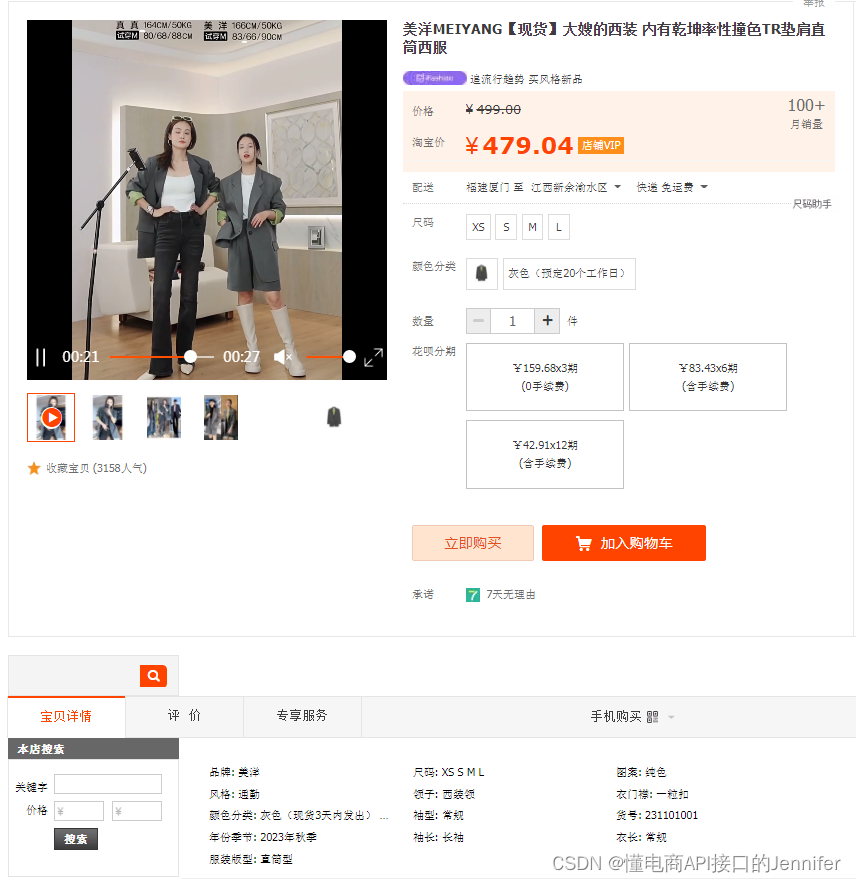
通过API爬取到的淘宝商品详情数据展示(api测试入口)
API名称:item_get 响应数据 item: { num_iid: "698291711589", title: "美洋MEIYANG【现货】大嫂的西装 内有乾坤率性撞色TR垫肩直筒西服", desc_short: "", price: 439.12, total_price: 0, suggestive_price: 0, orginal_price: …...

蓝桥杯2023年第十四届省赛真题-买瓜--题解
目录 蓝桥杯2023年第十四届省赛真题-买瓜 题目描述 输入格式 输出格式 样例输入 样例输出 提示 【思路解析】 【代码实现】 蓝桥杯2023年第十四届省赛真题-买瓜 时间限制: 3s 内存限制: 320MB 提交: 796 解决: 69 题目描述 小蓝正在一个瓜摊上买瓜。瓜摊上共有 n 个…...
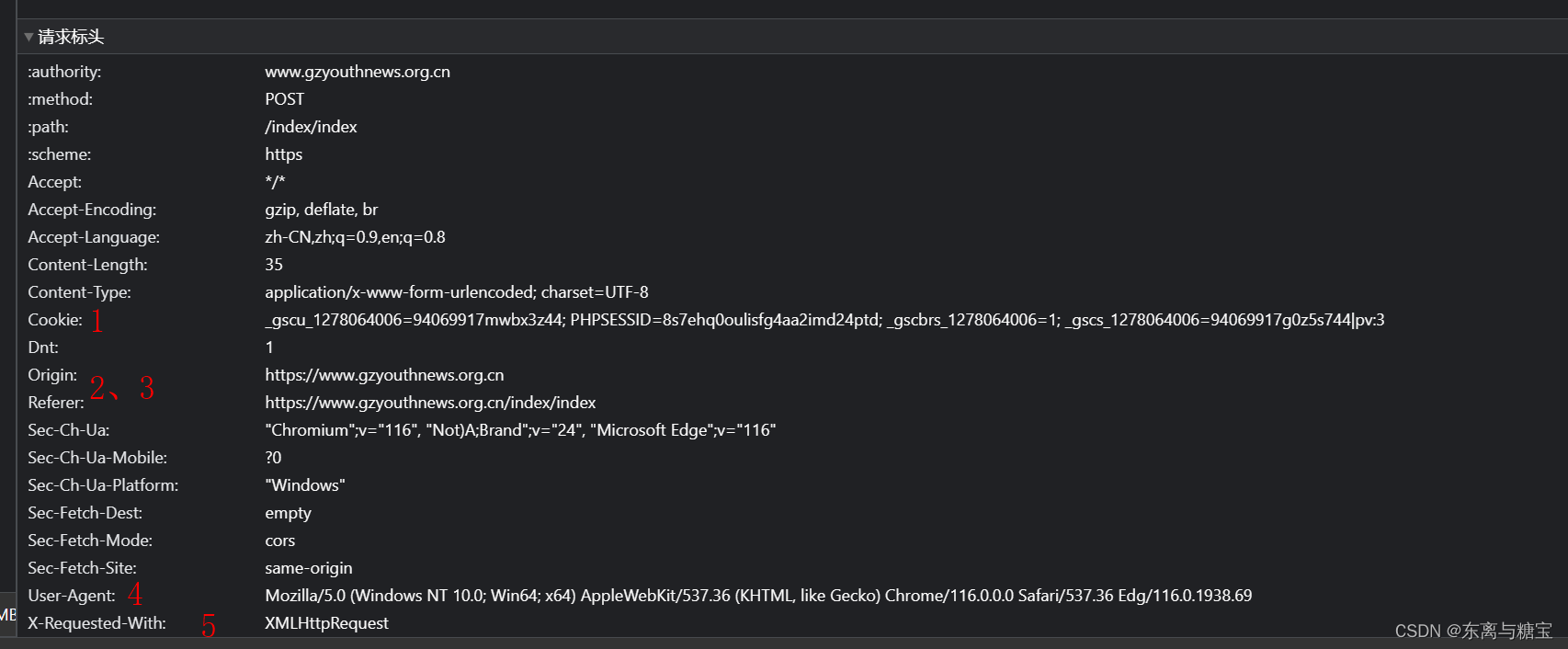
python萌新爬虫学习笔记【建议收藏】
文章目录 1. 如何何请求解析url2. 如何获取标签里面的文本3. 如何解析JSON格式4. 如何添加常用的header5. 如何合并两个div6. 如何删除html dom的部分结构7. 如何一次性获取所有div标签里的文本8. python爬虫如何改变响应文本字符集编码9. 如何进行字符集转码11. response.text…...
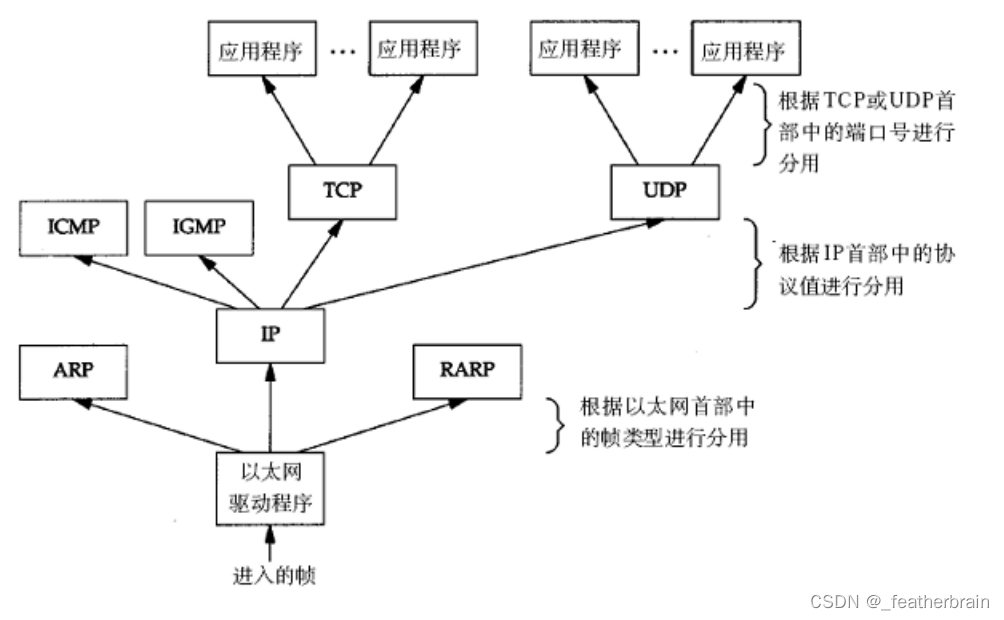
网络编程——基础知识
全文目录 网络发展协议OSI七层模型TCP/IP五层(或四层)模型 网络传输网络地址IP地址MAC地址 网络通信的本质 网络发展 网络没有出来之前计算机都是相互独立的: 网络就是将独立的计算机连接在一起,局域网和广域网的区别只是范围上的大小: 局域…...
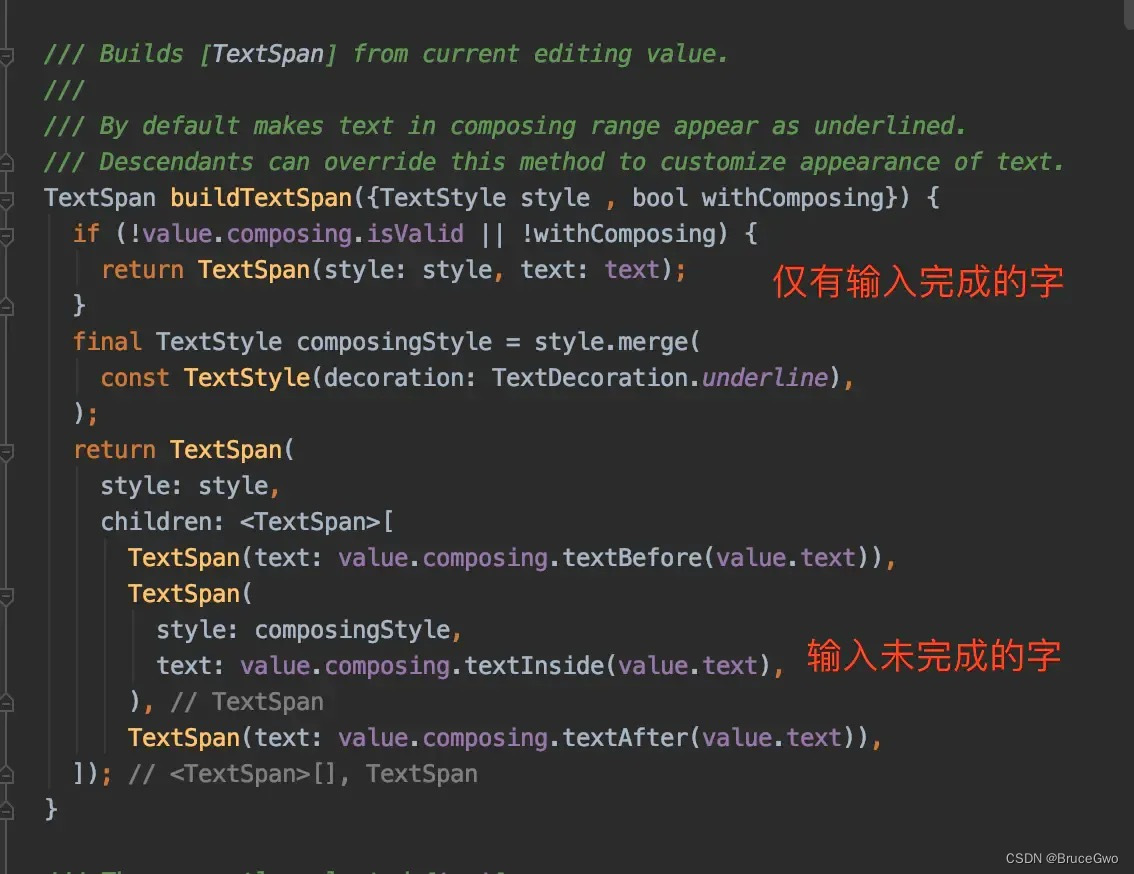
flutter聊天界面-TextField输入框实现@功能等匹配正则表达式展示高亮功能
flutter聊天界面-TextField输入框实现功能等匹配正则表达式展示高亮功能 一、简要描述 描述: 最近有位朋友讨论的时候,提到了输入框的高亮展示。在flutter TextField中需要插入特殊样式的标签,比如:“请 张三 回答一下”&#x…...

【C语言】指针的进阶(二)—— 回调函数的讲解以及qsort函数的使用方式
目录 1、函数指针数组 1.1、函数指针数组是什么? 1.2、函数指针数组的用途:转移表 2、扩展:指向函数指针的数组的指针 3、回调函数 3.1、回调函数介绍 3.2、回调函数的案例:qsort函数 3.2.1、回顾冒泡排序 3.2.1、什么是qso…...
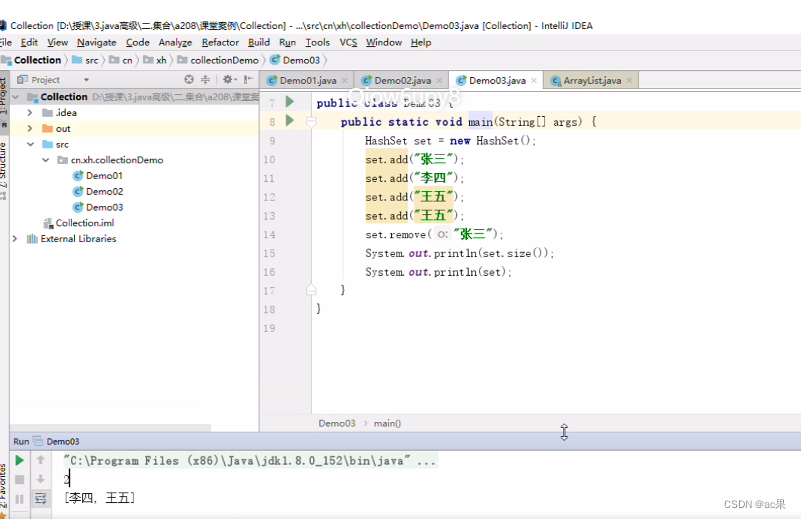
Java集合之HashSet接口
Set Set接口、HashSet类、TreeSet类 Set(组、集):表示无序,元素不能重复的集合,组中的元素必须唯一 Set接口 Set接口定义了组/集/集合(Set)。他扩展了Collection接口,并声明了不允…...
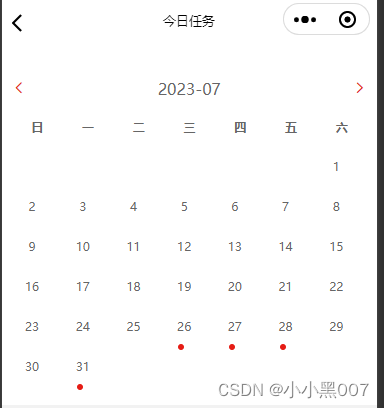
uniapp----微信小程序 日历组件(周日历 月日历)【Vue3+ts+uView】
uniapp----微信小程序 日历组件(周日历&& 月日历)【Vue3tsuView】 用Vue3tsuView来编写日历组件;存在周日历和月日历两种显示方式;高亮显示当天日期,红点渲染有数据的日期,点击显示数据 1. calenda…...
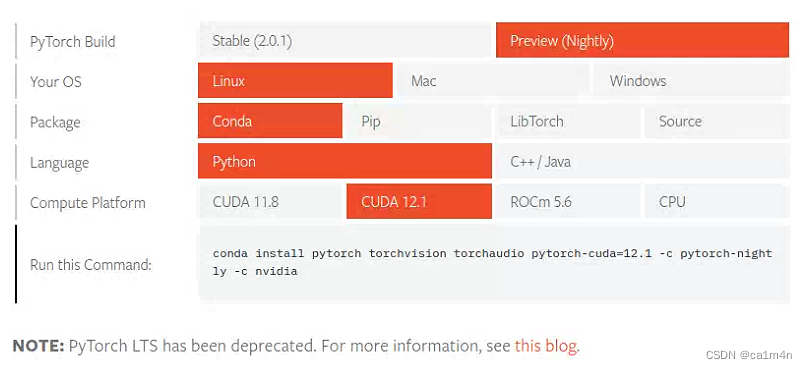
【记录】深度学习环境配置(pytorch版)
1080面对Transformer连勉强也算不上了,还是要去用小组的卡 完整记一个环境配置,方便后面自用✍️ 目前要简单许多,因为显卡驱动已经装好,后安装的库版本与其对应即可。 nvidia-smi查看GPU信息 ** CUDA版本12.2 conda -V查询conda…...

如何将项目推送到GitHub中
将项目推送到 GitHub 仓库并管理相关操作,遵循以下步骤: 创建 GitHub 账户:如果您没有 GitHub 账户,首先需要在 GitHub 官网 上创建一个账户。 创建新仓库:在 GitHub 页面上,点击右上角的加号图标…...
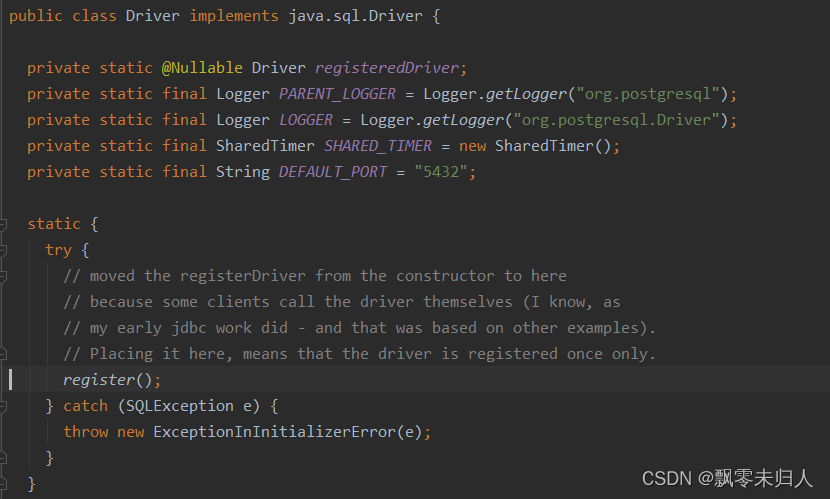
数据库直连提示 No suitable driver found for jdbc:postgresql
背景:我在代码里使用直连的方式在数据库中创建数据库等,由于需要适配各个数据库服务所以我分别兼容了mysql、postgresql、oracal等。但是在使用过程中会出现错误: No suitable driver found for jdbc:postgresql 但是我再使用mysql的直连方式…...
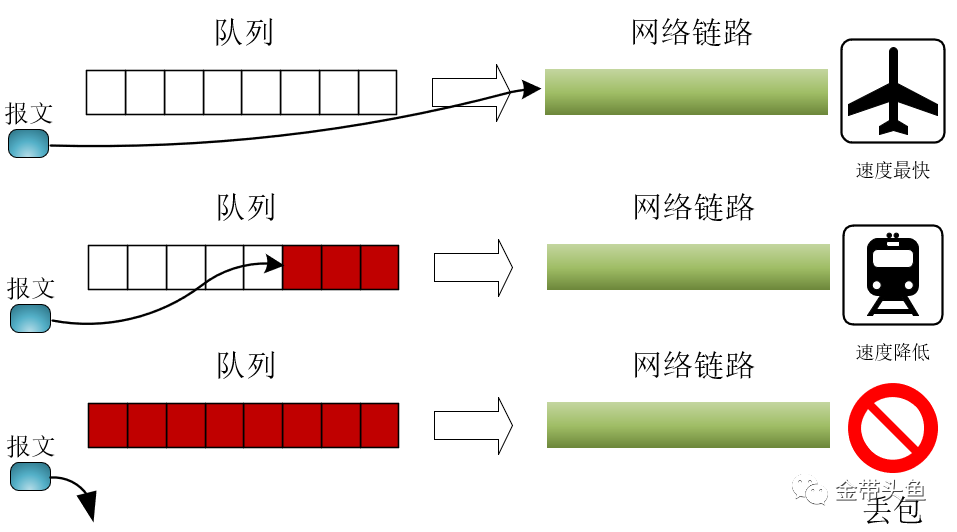
做网站的设计尺寸/百度智能建站平台
关于缓冲区 滑动窗口主要是为了处理能力不足时限制发送方的速度linux下 每一个tcp连接 都会占用4k左右的内存 -- 和缓冲区设置相关, 在 buff/cache当中.tcp连接由内核维护. 内核为每个连接建立内存缓冲区tcp需要保证每个报文都能达到对方server. 没有收到对应ack的时候需要重…...

招聘网站续费怎么做分录/领硕网站seo优化
以这个审批设置为例 里面是个vue项目,没有直接放在a.xxx.com下面,而是放在了/espace/下面 修改 1.router.js 2.vue.config.js 3.nginx配置 server {listen 8001;location / {root /opt/espace; #示例 /root/lc/dist #注意:用‘/’inde…...

wordpress 默认登陆/刷移动关键词优化
日常跑数的文件存放于FTP服务器需要定期删除;写一个删除FTP指定路径指定日期之前文件的工具类,后续或可应用于定时任务调度。使用到的jar包:commons-net-3.8.0.jar入参:FTP指定文件夹路径;指定日期时间代码:…...

凡科网站怎么样/公司品牌推广方案范文
index() 用于返回序列中指定元素的索引值,字符串 、元组 、列表都属于序列 In [1]: str "hello world"In [2]: str.index(e) # 返回元素e在序列中的索引值 Out[2]: 1In [3]: str.index(l) # 如果有相同元素,则默认返回第一个出现的元素…...
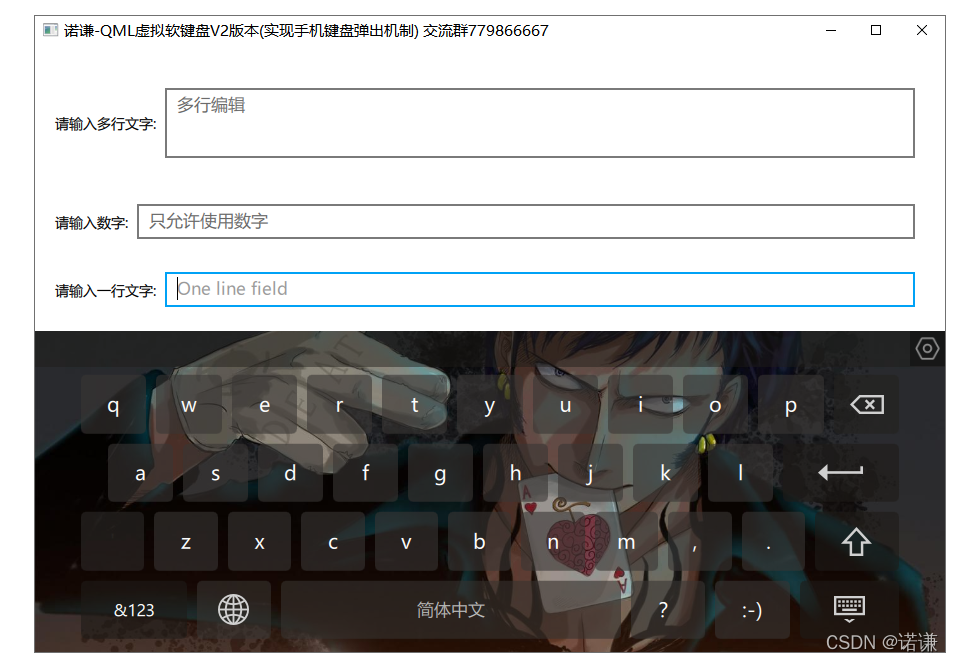
网站推广运作怎么做/杭州seo网站推广排名
在上章我们学习了45.qt quick-qml虚拟软键盘详解(一)_诺谦的博客-CSDN博客46.qt quick-自定义非常好看的qml虚拟软键盘-支持换肤、动态加载移除语言(二)_心中无女人 拔刀自然神 剑谱第一页 忘掉心上人-CSDN博客但是发现在嵌入式触摸屏上并没有手机那样方便、所以本章来实现手机…...

web网站建设/百度网站管理员工具
因为语言上的设计错误,arguments可以被当成一个数组。function zero () {console.log(arguments[0]);}也会有function zero () {for(var i0;iconsole.log(arguments[i]);}}它利用了Javascript的一个事实,即Javasc而这里的arguments变量给实参提供了一个类…...