Lambda-常见的函数式接口
如果需要使用Lambda接口,就必须要有一个函数式接口
函数式接口是有且仅有一个抽象方法的接口, 对应的注解是@FunctionalInterface
Java中内置的常见函数式接口如下:
1.Runnable/ Callable
/*** The <code>Runnable</code> interface should be implemented by any* class whose instances are intended to be executed by a thread. The* class must define a method of no arguments called <code>run</code>.* <p>* This interface is designed to provide a common protocol for objects that* wish to execute code while they are active. For example,* <code>Runnable</code> is implemented by class <code>Thread</code>.* Being active simply means that a thread has been started and has not* yet been stopped.* <p>* In addition, <code>Runnable</code> provides the means for a class to be* active while not subclassing <code>Thread</code>. A class that implements* <code>Runnable</code> can run without subclassing <code>Thread</code>* by instantiating a <code>Thread</code> instance and passing itself in* as the target. In most cases, the <code>Runnable</code> interface should* be used if you are only planning to override the <code>run()</code>* method and no other <code>Thread</code> methods.* This is important because classes should not be subclassed* unless the programmer intends on modifying or enhancing the fundamental* behavior of the class.** @author Arthur van Hoff* @see java.lang.Thread* @see java.util.concurrent.Callable* @since JDK1.0*/
@FunctionalInterface
public interface Runnable {/*** When an object implementing interface <code>Runnable</code> is used* to create a thread, starting the thread causes the object's* <code>run</code> method to be called in that separately executing* thread.* <p>* The general contract of the method <code>run</code> is that it may* take any action whatsoever.** @see java.lang.Thread#run()*/public abstract void run();
}
分别用匿名内部类和Lambda实现Runnable
public class RunnableLambda {public static void main(String[] args) {new Thread(new Runnable() {@Overridepublic void run() {String name = Thread.currentThread().getName();System.out.println(name);}}).start();new Thread(()->{String name = Thread.currentThread().getName();System.out.println(name);}).start();}
}
Callable和Runnable类似
2.Supplier
特点:无方法参数, 返回值为定义的泛型
JDK中Supplier对应的定义:
package java.util.function;/*** Represents a supplier of results.** <p>There is no requirement that a new or distinct result be returned each* time the supplier is invoked.** <p>This is a <a href="package-summary.html">functional interface</a>* whose functional method is {@link #get()}.** @param <T> the type of results supplied by this supplier** @since 1.8*/
@FunctionalInterface
public interface Supplier<T> {/*** Gets a result.** @return a result*/T get();
}
Supplier的使用
public class SupplierLambda {public static void main(String[] args) {int[] arr = {2, 5, 8, 10, 500, 500, 50000};int max = getMax(() -> {int curMax = arr[0];for(int i = 1; i < arr.length; i++) {if(arr[i] > curMax) {curMax = arr[i];}}return curMax;});System.out.println(max);}public static int getMax(Supplier<Integer> supplier) {return supplier.get();}
}
3.Consumer
特点:一个输入参数 无输出 可以对一个入参进行多次使用(使用andThen)
JDK中Consumer的定义
package java.util.function;import java.util.Objects;/*** Represents an operation that accepts a single input argument and returns no* result. Unlike most other functional interfaces, {@code Consumer} is expected* to operate via side-effects.** <p>This is a <a href="package-summary.html">functional interface</a>* whose functional method is {@link #accept(Object)}.** @param <T> the type of the input to the operation** @since 1.8*/
@FunctionalInterface
public interface Consumer<T> {/*** Performs this operation on the given argument.** @param t the input argument*/void accept(T t);/*** Returns a composed {@code Consumer} that performs, in sequence, this* operation followed by the {@code after} operation. If performing either* operation throws an exception, it is relayed to the caller of the* composed operation. If performing this operation throws an exception,* the {@code after} operation will not be performed.** @param after the operation to perform after this operation* @return a composed {@code Consumer} that performs in sequence this* operation followed by the {@code after} operation* @throws NullPointerException if {@code after} is null*/default Consumer<T> andThen(Consumer<? super T> after) {Objects.requireNonNull(after);return (T t) -> { accept(t); after.accept(t); };}
}
Consumer的使用
import java.util.function.Consumer;public class ConsumerLambda {public static void main(String[] args) {consumerString(s-> System.out.println(s.toUpperCase()),s-> System.out.println(s.toLowerCase()));}static void consumerString(Consumer<String> comsumer) {comsumer.accept("Hello");}static void consumerString(Consumer<String> firstConsumer, Consumer<String> secondConsumer) {firstConsumer.andThen(secondConsumer).accept("Hello");}
}
4.Comparator
特点:两个参数(类型根据指定的泛型),返回值为int
JDK中关于Comparator的定义太长有500多行,我这里就不贴了,自己去看吧
使用如下:
import java.util.Arrays;
import java.util.Comparator;public class ComparatorLambda {public static void main(String[] args) {String[] strs = {"delay","a", "ab", "abc", "bcd"};Comparator<String> comparator = new Comparator<String>() {@Overridepublic int compare(String o1, String o2) {return o1.length() - o2.length();}};Arrays.sort(strs, comparator);System.out.println(Arrays.toString(strs));Arrays.sort(strs, (o1, o2)-> o2.length() - o1.length());System.out.println(Arrays.toString(strs));}
}
5.Predicate
特点:一个入参(类型由泛型指定),出参为boolean,内含条件判断方法,常用于判断
JDK中的定义如下:
package java.util.function;import java.util.Objects;/*** Represents a predicate (boolean-valued function) of one argument.** <p>This is a <a href="package-summary.html">functional interface</a>* whose functional method is {@link #test(Object)}.** @param <T> the type of the input to the predicate** @since 1.8*/
@FunctionalInterface
public interface Predicate<T> {/*** Evaluates this predicate on the given argument.** @param t the input argument* @return {@code true} if the input argument matches the predicate,* otherwise {@code false}*/boolean test(T t);/*** Returns a composed predicate that represents a short-circuiting logical* AND of this predicate and another. When evaluating the composed* predicate, if this predicate is {@code false}, then the {@code other}* predicate is not evaluated.** <p>Any exceptions thrown during evaluation of either predicate are relayed* to the caller; if evaluation of this predicate throws an exception, the* {@code other} predicate will not be evaluated.** @param other a predicate that will be logically-ANDed with this* predicate* @return a composed predicate that represents the short-circuiting logical* AND of this predicate and the {@code other} predicate* @throws NullPointerException if other is null*/default Predicate<T> and(Predicate<? super T> other) {Objects.requireNonNull(other);return (t) -> test(t) && other.test(t);}/*** Returns a predicate that represents the logical negation of this* predicate.** @return a predicate that represents the logical negation of this* predicate*/default Predicate<T> negate() {return (t) -> !test(t);}/*** Returns a composed predicate that represents a short-circuiting logical* OR of this predicate and another. When evaluating the composed* predicate, if this predicate is {@code true}, then the {@code other}* predicate is not evaluated.** <p>Any exceptions thrown during evaluation of either predicate are relayed* to the caller; if evaluation of this predicate throws an exception, the* {@code other} predicate will not be evaluated.** @param other a predicate that will be logically-ORed with this* predicate* @return a composed predicate that represents the short-circuiting logical* OR of this predicate and the {@code other} predicate* @throws NullPointerException if other is null*/default Predicate<T> or(Predicate<? super T> other) {Objects.requireNonNull(other);return (t) -> test(t) || other.test(t);}/*** Returns a predicate that tests if two arguments are equal according* to {@link Objects#equals(Object, Object)}.** @param <T> the type of arguments to the predicate* @param targetRef the object reference with which to compare for equality,* which may be {@code null}* @return a predicate that tests if two arguments are equal according* to {@link Objects#equals(Object, Object)}*/static <T> Predicate<T> isEqual(Object targetRef) {return (null == targetRef)? Objects::isNull: object -> targetRef.equals(object);}
}
简单用法
import java.util.function.Predicate;public class PredicateLambda {public static void main(String[] args) {andMethod(s->s.contains("W"),s-> s.contains("H"));orMethod(s->s.contains("W"),s-> s.contains("H"));negateMethod(s->s.length()>5);}static void andMethod(Predicate<String> first, Predicate<String> second) {boolean isValid = first.and(second).test("helloWorld");System.out.println("字符串符合要求吗:" + isValid);}static void orMethod(Predicate<String> first, Predicate<String> second) {boolean isValid = first.or(second).test("helloWorld");System.out.println("字符串符合要求吗:" + isValid);}static void negateMethod(Predicate<String> predicate) {boolean tooLong = predicate.negate().test("helloWorld");System.out.println("字符串特别长吗:" + tooLong);}
6.Function
特点:一个入参,有出参,入参和出参的类型都由泛型指定,可以多次处理(从第一个开始处理,然后把返回值作为第二个、第三个。。。的结果)
它的实例应该是具备某种功能的
简单使用如下:
import java.util.function.Function;public class FunctionLambda {public static void main(String[] args) {method(str-> Integer.parseInt(str) + 10,strInt -> strInt *= 10);String str = "zhangsan,80";int age = getAgeNum(str,s->s.split(",")[1],s->Integer.parseInt(s),i-> i -= 10);System.out.println("zhangsan十年前的年龄是:" + age);}static void method(Function<String, Integer> first, Function<Integer, Integer> second) {int num = first.andThen(second).apply("10");System.out.println(num);}static int getAgeNum(String str, Function<String, String> first,Function<String, Integer> second,Function<Integer, Integer> third) {return first.andThen(second).andThen(third).apply(str);}
}
相关文章:

Lambda-常见的函数式接口
如果需要使用Lambda接口,就必须要有一个函数式接口 函数式接口是有且仅有一个抽象方法的接口, 对应的注解是FunctionalInterface Java中内置的常见函数式接口如下: 1.Runnable/ Callable /*** The <code>Runnable</code> interface should be implem…...
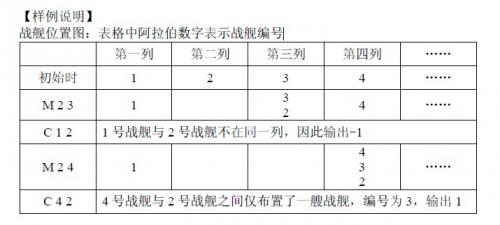
P1196 [NOI2002] 银河英雄传说 带权并查集
[NOI2002] 银河英雄传说 题目背景 公元 580158015801 年,地球居民迁至金牛座 α\alphaα 第二行星,在那里发表银河联邦创立宣言,同年改元为宇宙历元年,并开始向银河系深处拓展。 宇宙历 799799799 年,银河系的两大军…...

【项目实战】快来入门Groovy的基础语法吧
一、Groovy是什么? 1.1 与Java语言的关系 下一代的Java 语言,增强Java平台的唯一的脚本语言跟java一样,它也运行在 JVM 中。支持Java平台,无缝的集成了Java 的类和库;Groovy是一种运行在JVM上的动态语言,跑在JVM中的另一种语言编译后的.groovy也是以class的形式出现的。1…...
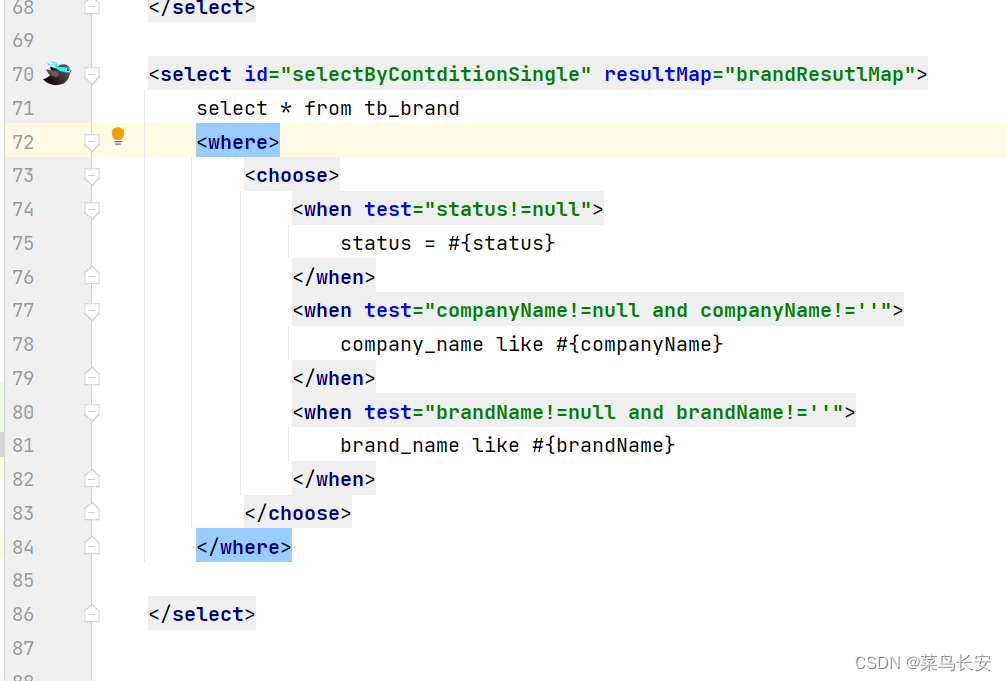
Mybatis中的动态SQL
Mybatis中的动态SQL 当存在多条件查询的SQL时,当用户某个条件的属性没有写时,就会存在问题,在test中则不能很好的运行 所以Mybatis提出了动态SQL。 即判断用户是否输入了某个属性 动态SQL中的一些问题 方法一 这个里的and是为了确保if条…...

VUE常用API
1.$set数据变了,视图没变 this.$set(targe,key,value)2.$nextTick:返回参数[函数]。是一个异步的,功能获得更新后DOM$nextTick(callback){return Promise.resolve().then(()>{callback();}) }3.$refs获取dom4.$el获取当前组件根…...
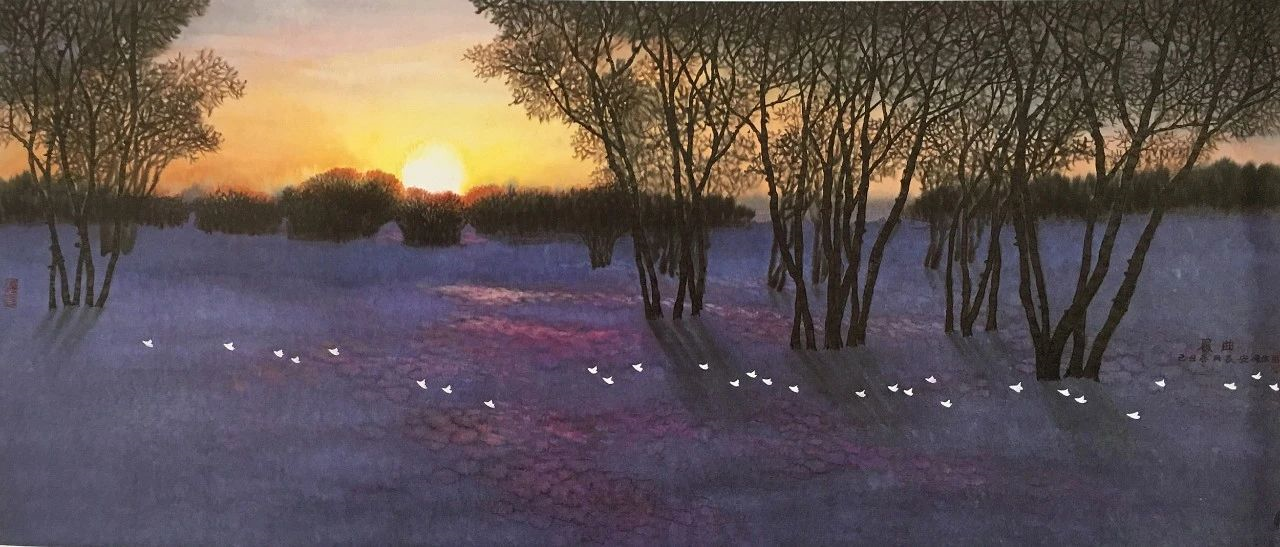
25 openEuler管理网络-使用nmcli命令配置ip
文章目录25 openEuler管理网络-使用nmcli命令配置ip25.1 nmcli介绍25.2 设备管理25.2.1 连接到设备25.2.2 断开设备连接25.3 设置网络连接25.3.1 配置动态IP连接25.3.1.1 配置IP25.3.1.2 激活连接并检查状态25.3.2 配置静态IP连接25.3.2.1 配置IP25.3.2.2 激活连接并检查状态25…...

如何安装和使用A-ops工具?
一、pip配置 1.配置信任域 pip3 config set global.trusted-host mirrors.tools.huawei.com2.配置pip源的url地址pip3 config set global.index-url http://mirrors.tools.huawei.com/pypi/simple 二、npm安装及配置 npm -v检测系统有无安装npm,如果没有的话需要配置ope…...
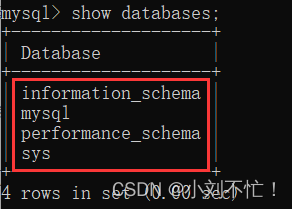
MySql数据库环境部署
MySql基础与Sql数据库概述基础环境的建立MYSQL数据库的连接方法MySql的默认数据库数据库端口号数据库概述 数据库(DataBase,DB)∶存储在磁带、磁盘、光盘或其他外存介质上、按定结构组织在一起的相关数据的集合。数据库管理系统〈DataBase Management S…...
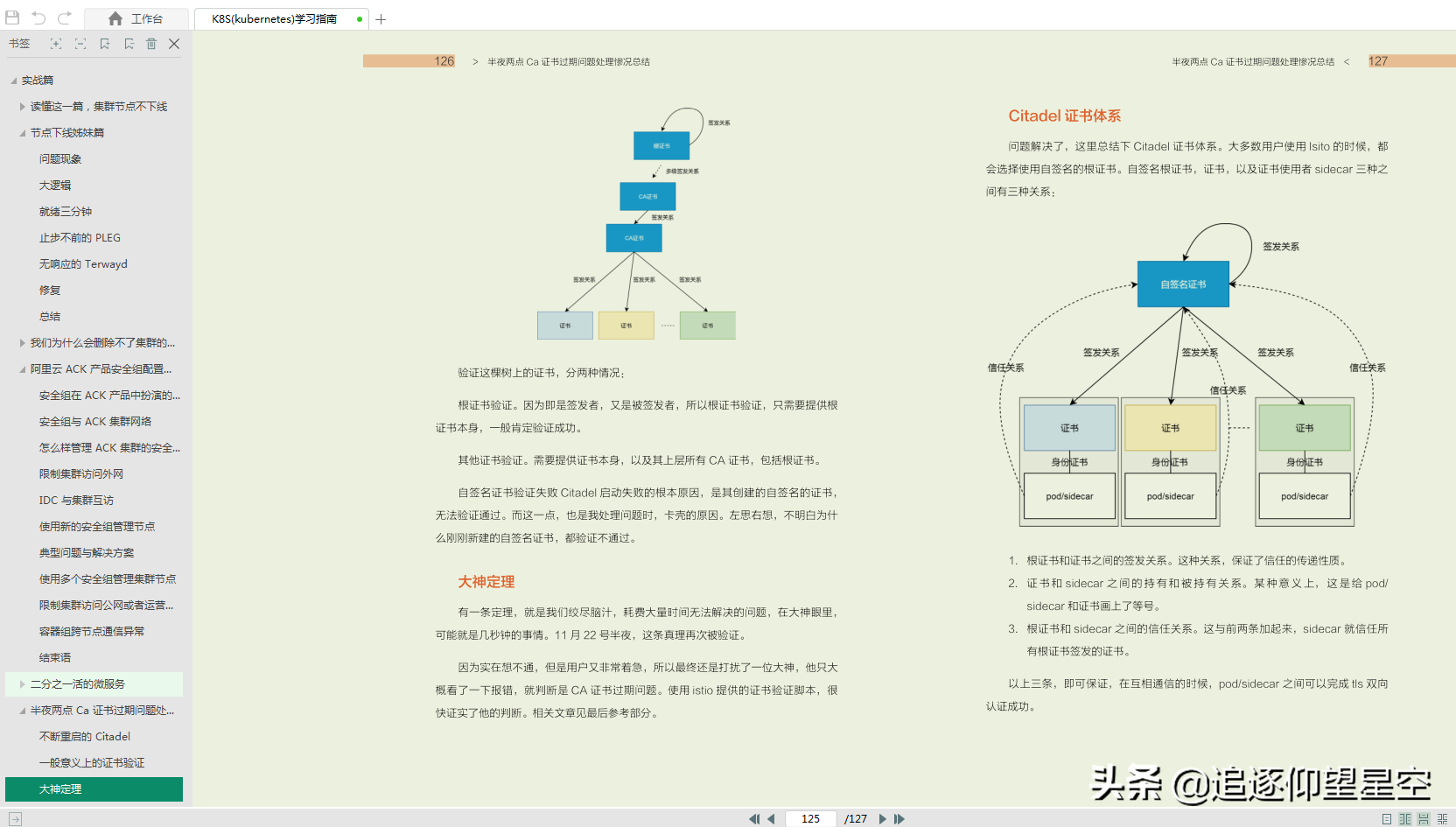
极品笔记,阿里P7爆款《K8s+Jenkins》技术笔记,职场必备
前些日子从阿里的朋友那里取得这两份K8sJenkins的爆款技术笔记:《K8S(kubernetes)学习指南》《Jenkins持续集成从入门到精通》,非常高质量的干货,我立马收藏! 而今天咱们文章的主角就是这非常之干货的技术笔记:K8SJenk…...
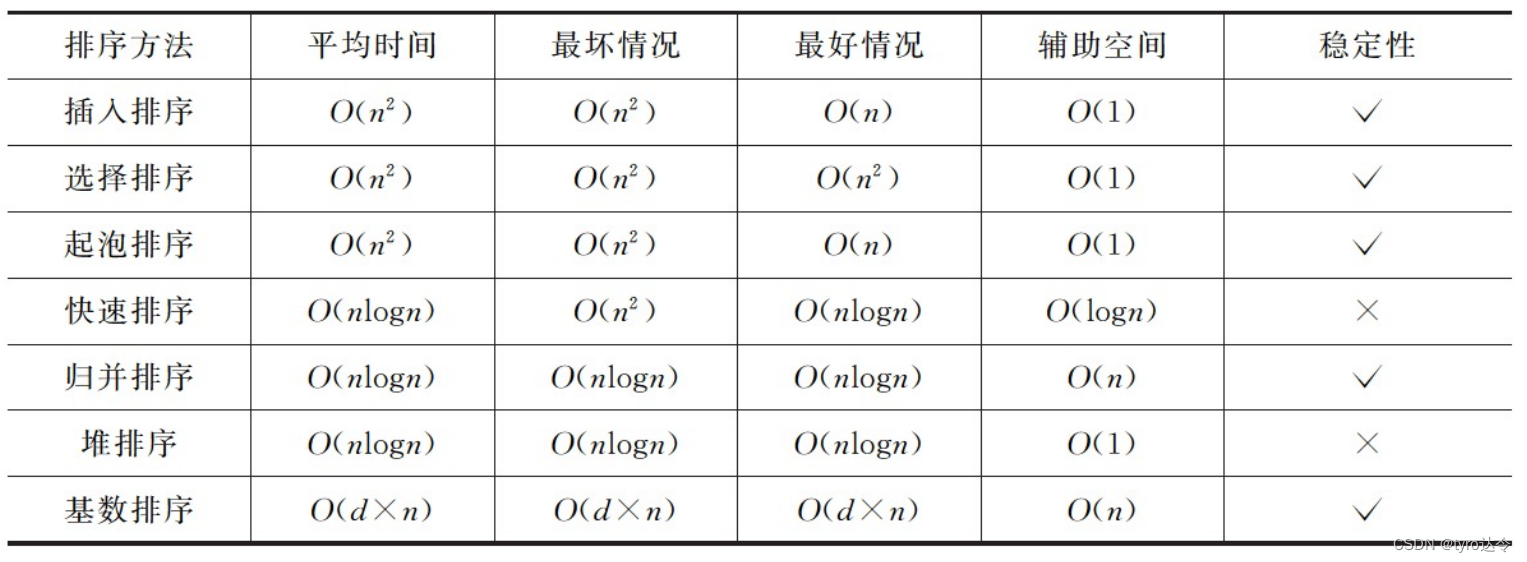
数据结构:各种排序方法的综合比较
排序方法的选用应视具体场合而定。一般情况下考虑的原则有:(1)待排序的记录个数 n;(2)记录本身的大小;(3)关键字的分布情况:(4)对排序稳定性的要求等。 1.时间性能 (1) 按平均的时间性能来分,有三类排序方法: 时间复杂度为 O(nlogn)的方法有:快速排序、堆排序和归并排序,其中…...
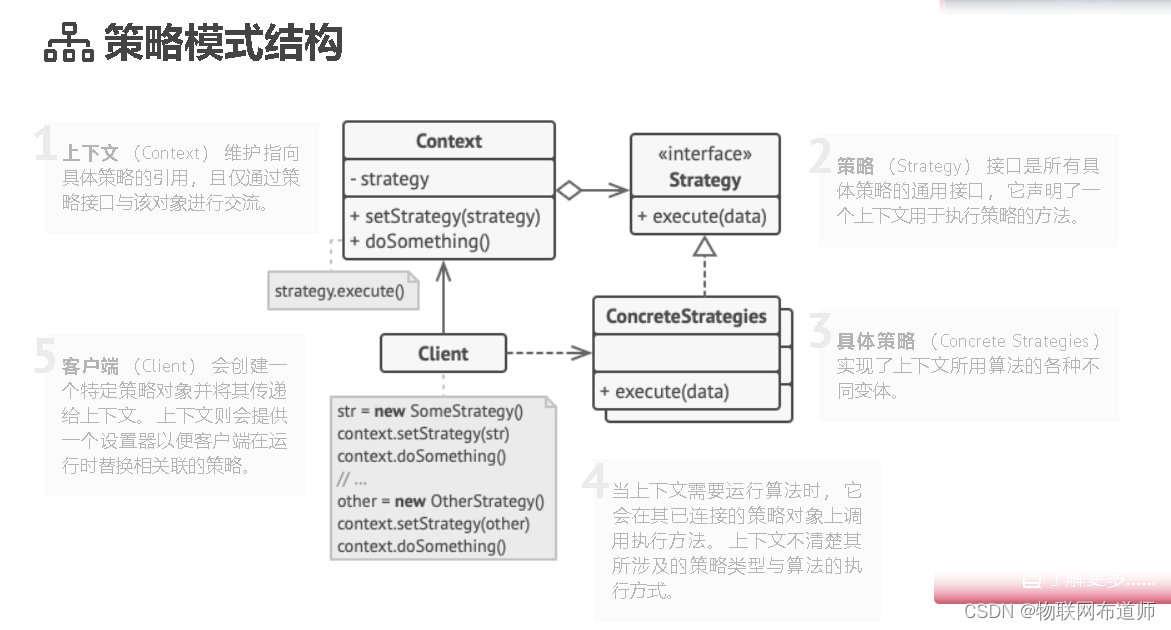
【设计模式】 策略模式介绍及C代码实现
【设计模式】 策略模式介绍及C代码实现 背景 在软件构建过程中,某些对象使用的算法可能多种多样,经常改变,如果将这些算法都编码到对象中,将会使对象变得异常复杂,而且有时候支持不使用的算法也是一个性能负担。 如何…...

【数据库】第二章 关系数据库
第二章 关系数据库 2.1关系数据结构及形式化定义 关系 域(domain) :域是一组具有相同数据类型的值的集合,可以取值的个数叫基数 笛卡尔积 :一个记录叫做一个元组(tuple),元组中每一个属性值,叫一个分量 基数&…...

oracle和mysql的分页
oracle的分页:rownum 注意:: 对 ROWNUM 只能使用 < 或 <, 用 、 >、 > 都不能返回任何数据。 rownum是对结果集的编序排列,始终是从1开始,所以rownum直接使用时不允许使用>、> 所以当查询中间部分的信息时&…...

深拷贝与浅拷贝的理解
浅拷贝的理解浅拷贝的话只会拷贝基本数据类型,例如像string、Number等这些,类似:Object、Array 这类的话拷贝的就是对象的一个指针(通俗来讲就是拷贝一个引用地址,指向的是一个内存同一份数据),也就是说当拷贝的对象数…...

Shell变量
一、变量分类 根据作用域分三种 (一)只在函数内有效,叫局部变量 (二)只在当前shell进程中有效,叫做全局变量 (三)在当前shell进程与子进程中都有效,叫做环境变量 shell进…...
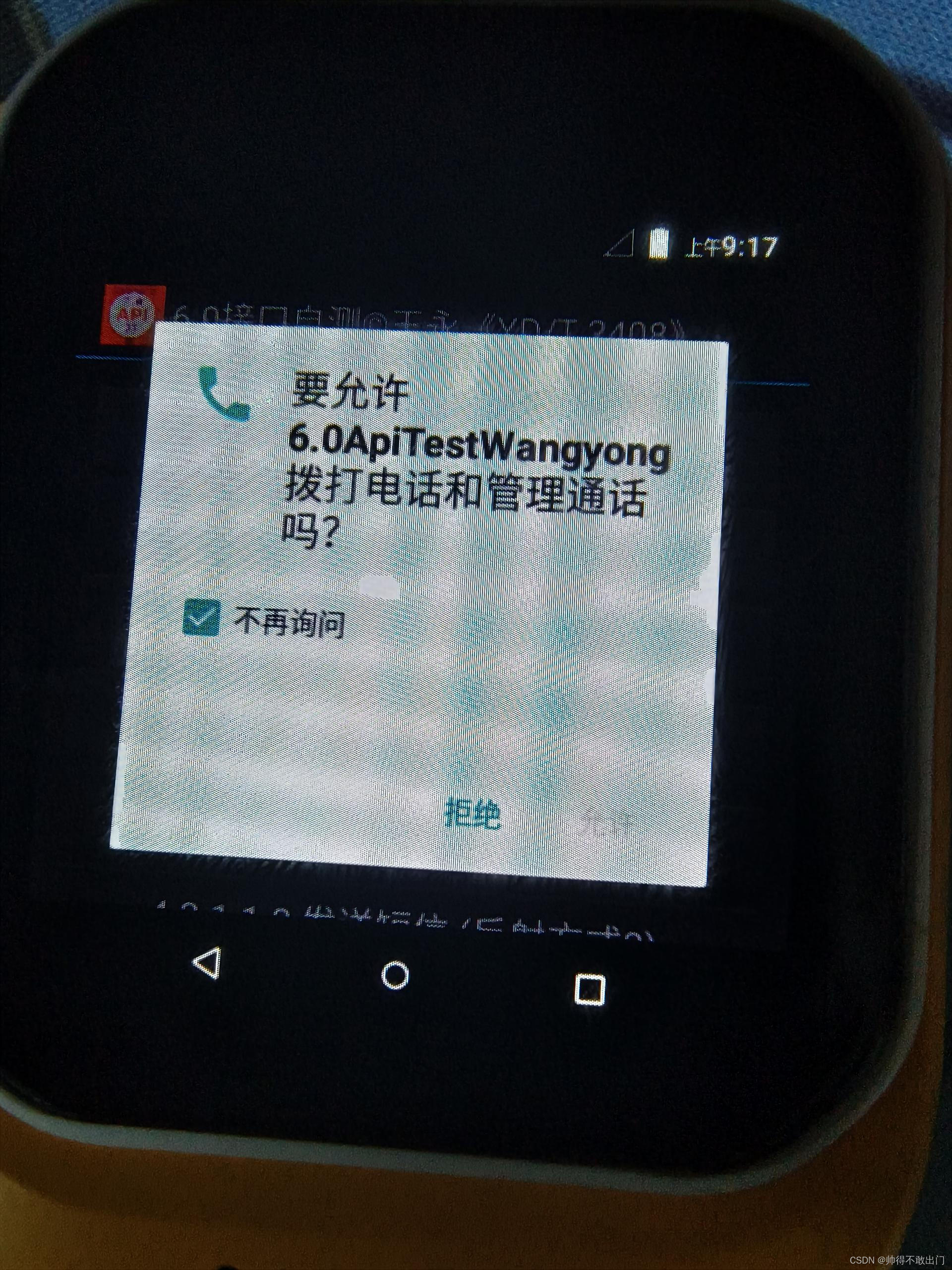
Android 8请求权限时弹窗BUG
弹窗BUG 应用使用requestPermissions申请权限时,系统会弹出一个选择窗口,可进行允许或拒绝, 此窗口中有一个”不再询问“的选择框, ”拒绝”及“允许”的按钮。 遇到一个Bug,单点击“不再询问”,“允许”这个按钮会变…...

路漫漫:网络空间的监管趋势
网络空间是“以相互依存的网络基础设施为基本架构,以代码、信息与数据的流动为环境,人类利用信息通讯技术与应用开展活动,并与其他空间高度融合与互动的空间”。随着信息化技术的发展,网络空间日益演绎成为与现实人类生存空间并存…...

洛谷 P1208 [USACO1.3]混合牛奶 Mixing Milk
最后水一篇水题题解(实在太水了) # [USACO1.3]混合牛奶 Mixing Milk ## 题目描述 由于乳制品产业利润很低,所以降低原材料(牛奶)价格就变得十分重要。帮助 Marry 乳业找到最优的牛奶采购方案。 Marry 乳业从一些奶农手…...
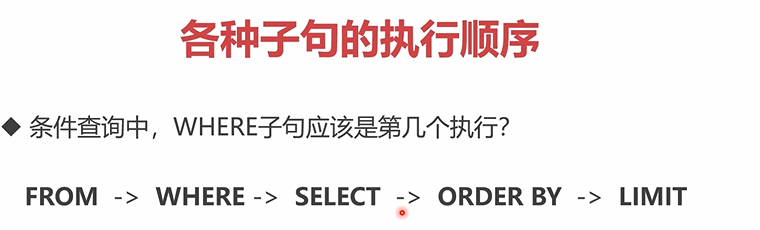
数据库的基本查询
注意:LIMIT的两个参数,第一个是起始位置,第二个是一次查询到多少页。注意:什么类型的数字都是可以排序的。日期的降序是从现在到以前,MySQL ENUM值如何排序?在MYSQL中,我们知道每个ENUM值都与一…...
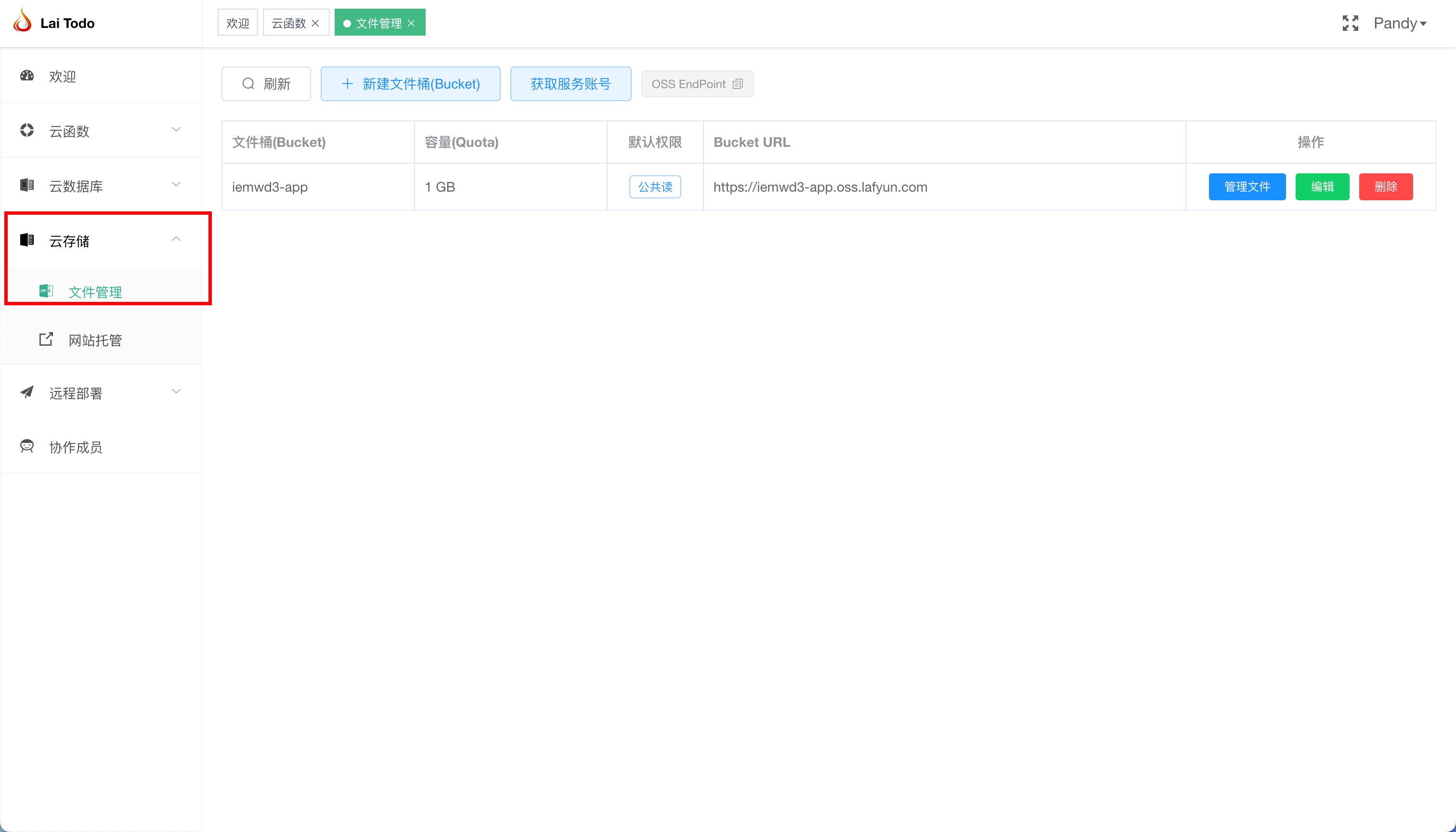
10 分钟把你的 Web 应用转为桌面端应用
在桌面端应用上,Electron 也早已做大做强,GitHub桌面端、VSCode、Figma、Notion、飞书、剪映、得物都基于此。但最近后起之秀的 Tauri 也引人注目,它解决了 Electron 一个大的痛点——打包产物特别大。 我们知道 Electron 基于谷歌内核 Chro…...
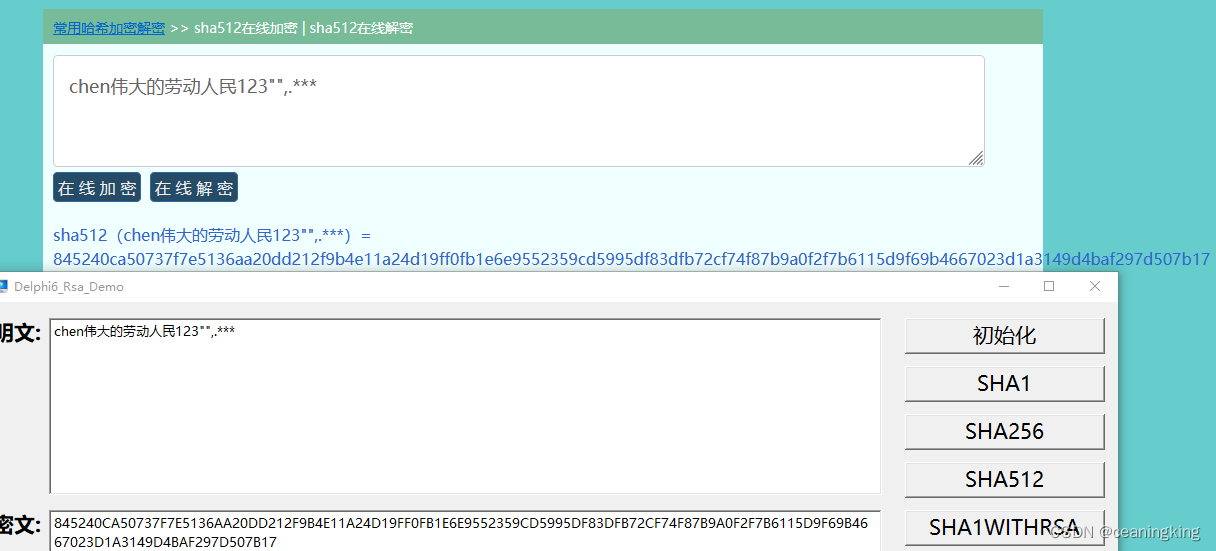
Delphi RSA加解密(二)
dll开发环境: Delphi XE 10.1 Berlin exe开发环境: Delphi 6 前提文章: Delphi RSA加解密(一) 目录 1. 概述 2. 准备工作 2.1 下载DEMO程序 2.2 字符编码说明 3. Cryption.dll封装 3.1 接口概况 3.2 uPub.pas单元代码 3.3 uInterface.pas单元代码 3.4 特别注意 4. 主程序…...

pytorch 深度学习早停设置
当你设置早停的时候你需要注意的是你可能得在几个epoch后才开始判断早停。 早停参数设置 早停(Early Stopping)是一种常用的防止深度学习模型过拟合的方法。早停的设置需要根据具体情况进行调整,常见的做法是在模型训练过程中使用验证集&am…...
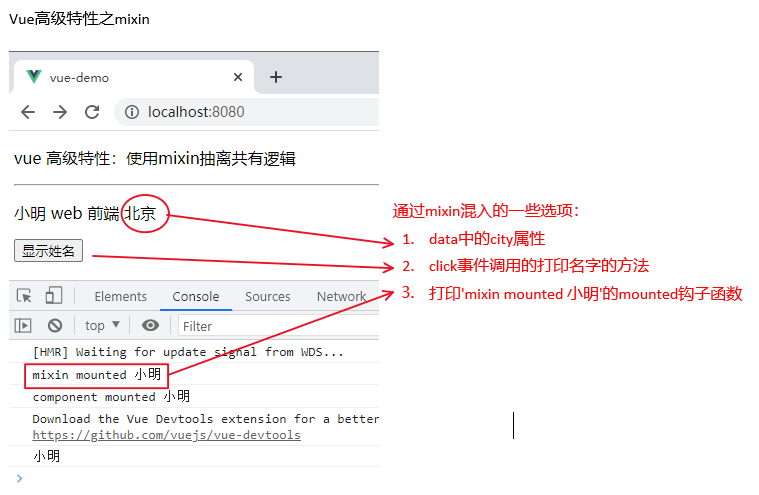
【Vue学习】Vue高级特性
1. 自定义v-model Vue中的自定义v-model指的是在自定义组件中使用v-model语法糖来实现双向绑定。在Vue中,通过v-model指令可以将表单元素的值与组件实例的数据进行双向绑定。但是对于自定义组件,如果要实现v-model的双向绑定,就需要自定义v-…...

Android 12.0 系统Settings去掉开发者模式功能
1.概述 在12.0的系统rom产品定制化开发中,在系统Settings中的关于手机的选项中,系统默认点击版本号5次会自动打开开发者模式,但是在某些产品开发过程中,禁止打开开发者模式,需要去掉开发者模式的功能,所以需要在系统Settings中查看开发者模式的相关流程代码,然后禁用掉开…...
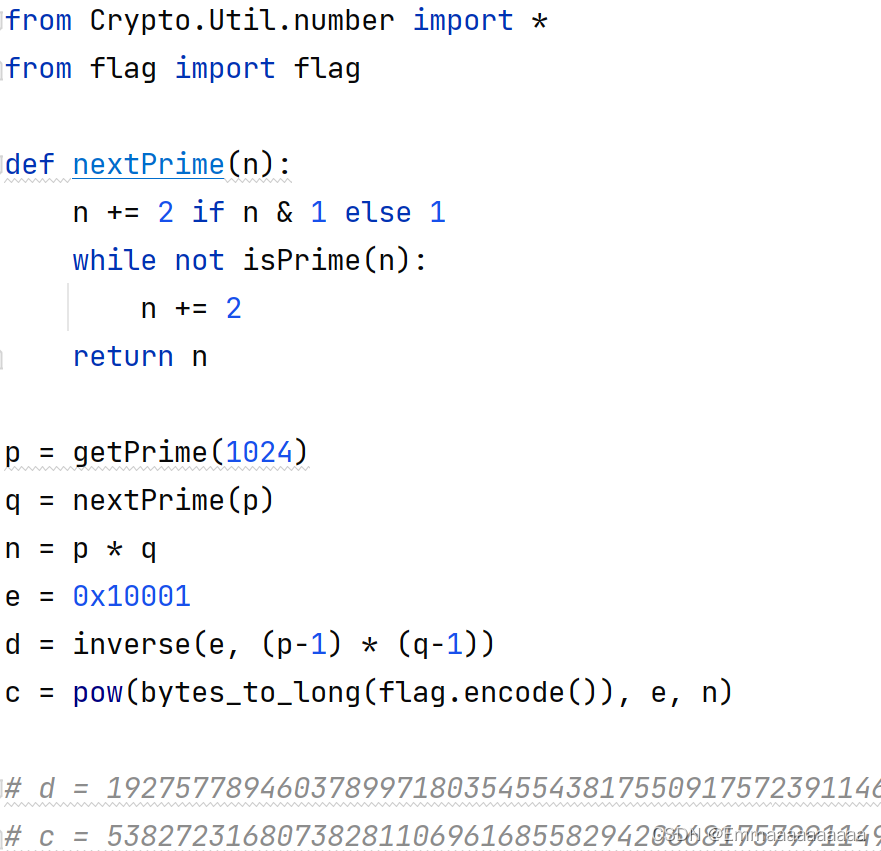
buu [NCTF2019]babyRSA 1
题目描述: 题目分析: 首先明确两个公式: e*d 1 mod (p-1)(q-1) ed1 e*d - 1 k(p-1)(q-1)想要解出此题,我们必须知道n,而要知道n,我们要知道p和q的值通过 e*d 的计算,我们知道其长度为2066位,而生成p的…...
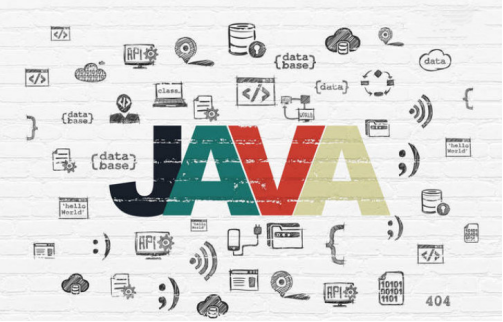
Java:如何选择一个Java API框架
Java编程语言是一种高级的、面向对象的语言,它使开发人员能够创建健壮的、可重用的代码。Java以其可移植性和平台独立性而闻名,这意味着Java代码可以在任何支持Java运行时环境(JRE)的系统上运行。Java和Node js一样,是一种功能强大的通用编程…...

mt6735 MIC 音量的调整及原理介绍
[DESCRIPTION] MIC 音量的调整及原理介绍[SOLUTION] audio_ver1_volume_custom_default.h#define VER1_AUD_VOLUME_MIC \ 64,112,192,144,192,192,184,184,184,184,184,0,0,0,0,\ 255,192,192,180,192,192,196,184,184,184,184,0,0,0,0,\ 255,208,208,180,255,208,196,0,0,0,0,…...
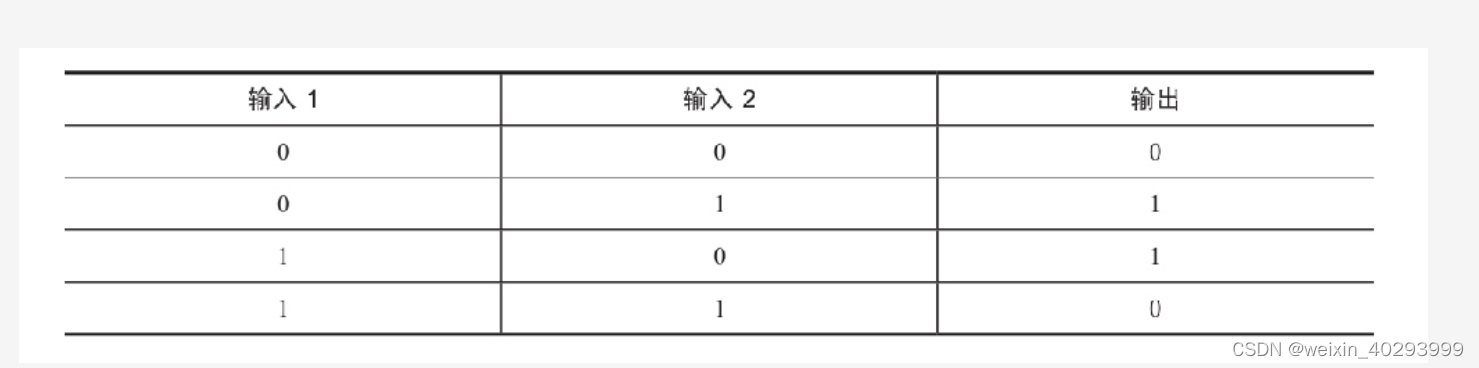
【深度学习】什么是线性回归逻辑回归单层神经元的缺陷
提示:文章写完后,目录可以自动生成,如何生成可参考右边的帮助文档 文章目录逻辑回归&线性回归单层神经元的缺陷单层神经元的缺陷逻辑回归&线性回归 线性回归预测的是一个连续值, 逻辑回归给出的”是”和“否”的回答. 等…...
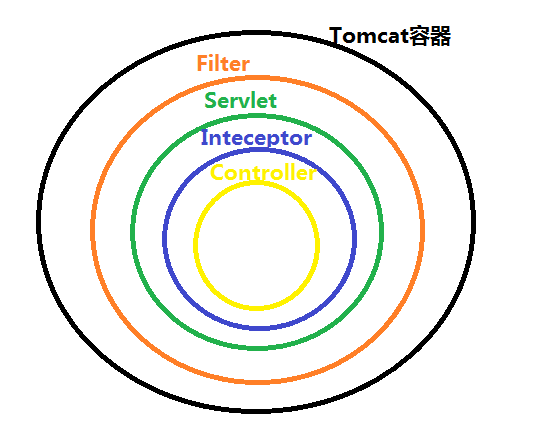
Spring拦截器
SpringMVC提供了拦截器机制,允许运行目标方法之前进行一些拦截工作或者目标方法运行之后进行一下其他相关的处理。自定义的拦截器必须实现HandlerInterceptor接口。preHandle():这个方法在业务处理器处理请求之前被调用,在该方法中对用户请求…...

8个可能降低网站搜索引擎信任度的错误
如果觉得文章对你有用请点赞与关注,每一份支持都是我坚持更新更优质内容的动力!!!例如,发布一段质量差的网站内容不会完全破坏您的排名机会,只要您的内容策略的其余部分井井有条。但是本地SEO中存在一些错误…...