Inference with C# BERT NLP Deep Learning and ONNX Runtime
目录
效果
测试一
测试二
测试三
模型信息
项目
代码
下载
Inference with C# BERT NLP Deep Learning and ONNX Runtime
效果
测试一
Context :Bob is walking through the woods collecting blueberries and strawberries to make a pie.
Question :What is his name?
测试二
Context :Bob is walking through the woods collecting blueberries and strawberries to make a pie.
Question :What will he bring home?
测试三
Context :Bob is walking through the woods collecting blueberries and strawberries to make a pie.
Question :Where is Bob?
模型信息
Inputs
-------------------------
name:unique_ids_raw_output___9:0
tensor:Int64[-1]
name:segment_ids:0
tensor:Int64[-1, 256]
name:input_mask:0
tensor:Int64[-1, 256]
name:input_ids:0
tensor:Int64[-1, 256]
---------------------------------------------------------------
Outputs
-------------------------
name:unstack:1
tensor:Float[-1, 256]
name:unstack:0
tensor:Float[-1, 256]
name:unique_ids:0
tensor:Int64[-1]
---------------------------------------------------------------
项目
代码
using BERTTokenizers;
using Microsoft.ML.OnnxRuntime;
using System;
using System.Collections.Generic;
using System.Data;
using System.Diagnostics;
using System.Linq;
using System.Windows.Forms;
namespace Inference_with_C__BERT_NLP_Deep_Learning_and_ONNX_Runtime
{
public struct BertInput
{
public long[] InputIds { get; set; }
public long[] InputMask { get; set; }
public long[] SegmentIds { get; set; }
public long[] UniqueIds { get; set; }
}
public partial class Form1 : Form
{
public Form1()
{
InitializeComponent();
}
RunOptions runOptions;
InferenceSession session;
BertUncasedLargeTokenizer tokenizer;
Stopwatch stopWatch = new Stopwatch();
private void Form1_Load(object sender, EventArgs e)
{
string modelPath = "bertsquad-10.onnx";
runOptions = new RunOptions();
session = new InferenceSession(modelPath);
tokenizer = new BertUncasedLargeTokenizer();
}
int MaxAnswerLength = 30;
int bestN = 20;
private void button1_Click(object sender, EventArgs e)
{
txt_answer.Text = "";
Application.DoEvents();
string question = txt_question.Text.Trim();
string context = txt_context.Text.Trim();
// Get the sentence tokens.
var tokens = tokenizer.Tokenize(question, context);
// Encode the sentence and pass in the count of the tokens in the sentence.
var encoded = tokenizer.Encode(tokens.Count(), question, context);
var padding = Enumerable
.Repeat(0L, 256 - tokens.Count)
.ToList();
var bertInput = new BertInput()
{
InputIds = encoded.Select(t => t.InputIds).Concat(padding).ToArray(),
InputMask = encoded.Select(t => t.AttentionMask).Concat(padding).ToArray(),
SegmentIds = encoded.Select(t => t.TokenTypeIds).Concat(padding).ToArray(),
UniqueIds = new long[] { 0 }
};
// Create input tensors over the input data.
var inputIdsOrtValue = OrtValue.CreateTensorValueFromMemory(bertInput.InputIds,
new long[] { 1, bertInput.InputIds.Length });
var inputMaskOrtValue = OrtValue.CreateTensorValueFromMemory(bertInput.InputMask,
new long[] { 1, bertInput.InputMask.Length });
var segmentIdsOrtValue = OrtValue.CreateTensorValueFromMemory(bertInput.SegmentIds,
new long[] { 1, bertInput.SegmentIds.Length });
var uniqueIdsOrtValue = OrtValue.CreateTensorValueFromMemory(bertInput.UniqueIds,
new long[] { bertInput.UniqueIds.Length });
var inputs = new Dictionary<string, OrtValue>
{
{ "unique_ids_raw_output___9:0", uniqueIdsOrtValue },
{ "segment_ids:0", segmentIdsOrtValue},
{ "input_mask:0", inputMaskOrtValue },
{ "input_ids:0", inputIdsOrtValue }
};
stopWatch.Restart();
// Run session and send the input data in to get inference output.
var output = session.Run(runOptions, inputs, session.OutputNames);
stopWatch.Stop();
var startLogits = output[1].GetTensorDataAsSpan<float>();
var endLogits = output[0].GetTensorDataAsSpan<float>();
var uniqueIds = output[2].GetTensorDataAsSpan<long>();
var contextStart = tokens.FindIndex(o => o.Token == "[SEP]");
var bestStartLogits = startLogits.ToArray()
.Select((logit, index) => (Logit: logit, Index: index))
.OrderByDescending(o => o.Logit)
.Take(bestN);
var bestEndLogits = endLogits.ToArray()
.Select((logit, index) => (Logit: logit, Index: index))
.OrderByDescending(o => o.Logit)
.Take(bestN);
var bestResultsWithScore = bestStartLogits
.SelectMany(startLogit =>
bestEndLogits
.Select(endLogit =>
(
StartLogit: startLogit.Index,
EndLogit: endLogit.Index,
Score: startLogit.Logit + endLogit.Logit
)
)
)
.Where(entry => !(entry.EndLogit < entry.StartLogit || entry.EndLogit - entry.StartLogit > MaxAnswerLength || entry.StartLogit == 0 && entry.EndLogit == 0 || entry.StartLogit < contextStart))
.Take(bestN);
var (item, probability) = bestResultsWithScore
.Softmax(o => o.Score)
.OrderByDescending(o => o.Probability)
.FirstOrDefault();
int startIndex = item.StartLogit;
int endIndex = item.EndLogit;
var predictedTokens = tokens
.Skip(startIndex)
.Take(endIndex + 1 - startIndex)
.Select(o => tokenizer.IdToToken((int)o.VocabularyIndex))
.ToList();
// Print the result.
string answer = "answer:" + String.Join(" ", StitchSentenceBackTogether(predictedTokens))
+ "\r\nprobability:" + probability
+ $"\r\n推理耗时:{stopWatch.ElapsedMilliseconds}毫秒";
txt_answer.Text = answer;
Console.WriteLine(answer);
}
private List<string> StitchSentenceBackTogether(List<string> tokens)
{
var currentToken = string.Empty;
tokens.Reverse();
var tokensStitched = new List<string>();
foreach (var token in tokens)
{
if (!token.StartsWith("##"))
{
currentToken = token + currentToken;
tokensStitched.Add(currentToken);
currentToken = string.Empty;
}
else
{
currentToken = token.Replace("##", "") + currentToken;
}
}
tokensStitched.Reverse();
return tokensStitched;
}
}
}
using BERTTokenizers;
using Microsoft.ML.OnnxRuntime;
using System;
using System.Collections.Generic;
using System.Data;
using System.Diagnostics;
using System.Linq;
using System.Windows.Forms;namespace Inference_with_C__BERT_NLP_Deep_Learning_and_ONNX_Runtime
{public struct BertInput{public long[] InputIds { get; set; }public long[] InputMask { get; set; }public long[] SegmentIds { get; set; }public long[] UniqueIds { get; set; }}public partial class Form1 : Form{public Form1(){InitializeComponent();}RunOptions runOptions;InferenceSession session;BertUncasedLargeTokenizer tokenizer;Stopwatch stopWatch = new Stopwatch();private void Form1_Load(object sender, EventArgs e){string modelPath = "bertsquad-10.onnx";runOptions = new RunOptions();session = new InferenceSession(modelPath);tokenizer = new BertUncasedLargeTokenizer();}int MaxAnswerLength = 30;int bestN = 20;private void button1_Click(object sender, EventArgs e){txt_answer.Text = "";Application.DoEvents();string question = txt_question.Text.Trim();string context = txt_context.Text.Trim();// Get the sentence tokens.var tokens = tokenizer.Tokenize(question, context);// Encode the sentence and pass in the count of the tokens in the sentence.var encoded = tokenizer.Encode(tokens.Count(), question, context);var padding = Enumerable.Repeat(0L, 256 - tokens.Count).ToList();var bertInput = new BertInput(){InputIds = encoded.Select(t => t.InputIds).Concat(padding).ToArray(),InputMask = encoded.Select(t => t.AttentionMask).Concat(padding).ToArray(),SegmentIds = encoded.Select(t => t.TokenTypeIds).Concat(padding).ToArray(),UniqueIds = new long[] { 0 }};// Create input tensors over the input data.var inputIdsOrtValue = OrtValue.CreateTensorValueFromMemory(bertInput.InputIds,new long[] { 1, bertInput.InputIds.Length });var inputMaskOrtValue = OrtValue.CreateTensorValueFromMemory(bertInput.InputMask,new long[] { 1, bertInput.InputMask.Length });var segmentIdsOrtValue = OrtValue.CreateTensorValueFromMemory(bertInput.SegmentIds,new long[] { 1, bertInput.SegmentIds.Length });var uniqueIdsOrtValue = OrtValue.CreateTensorValueFromMemory(bertInput.UniqueIds,new long[] { bertInput.UniqueIds.Length });var inputs = new Dictionary<string, OrtValue>{{ "unique_ids_raw_output___9:0", uniqueIdsOrtValue },{ "segment_ids:0", segmentIdsOrtValue},{ "input_mask:0", inputMaskOrtValue },{ "input_ids:0", inputIdsOrtValue }};stopWatch.Restart();// Run session and send the input data in to get inference output. var output = session.Run(runOptions, inputs, session.OutputNames);stopWatch.Stop();var startLogits = output[1].GetTensorDataAsSpan<float>();var endLogits = output[0].GetTensorDataAsSpan<float>();var uniqueIds = output[2].GetTensorDataAsSpan<long>();var contextStart = tokens.FindIndex(o => o.Token == "[SEP]");var bestStartLogits = startLogits.ToArray().Select((logit, index) => (Logit: logit, Index: index)).OrderByDescending(o => o.Logit).Take(bestN);var bestEndLogits = endLogits.ToArray().Select((logit, index) => (Logit: logit, Index: index)).OrderByDescending(o => o.Logit).Take(bestN);var bestResultsWithScore = bestStartLogits.SelectMany(startLogit =>bestEndLogits.Select(endLogit =>(StartLogit: startLogit.Index,EndLogit: endLogit.Index,Score: startLogit.Logit + endLogit.Logit))).Where(entry => !(entry.EndLogit < entry.StartLogit || entry.EndLogit - entry.StartLogit > MaxAnswerLength || entry.StartLogit == 0 && entry.EndLogit == 0 || entry.StartLogit < contextStart)).Take(bestN);var (item, probability) = bestResultsWithScore.Softmax(o => o.Score).OrderByDescending(o => o.Probability).FirstOrDefault();int startIndex = item.StartLogit;int endIndex = item.EndLogit;var predictedTokens = tokens.Skip(startIndex).Take(endIndex + 1 - startIndex).Select(o => tokenizer.IdToToken((int)o.VocabularyIndex)).ToList();// Print the result.string answer = "answer:" + String.Join(" ", StitchSentenceBackTogether(predictedTokens))+ "\r\nprobability:" + probability+ $"\r\n推理耗时:{stopWatch.ElapsedMilliseconds}毫秒";txt_answer.Text = answer;Console.WriteLine(answer);}private List<string> StitchSentenceBackTogether(List<string> tokens){var currentToken = string.Empty;tokens.Reverse();var tokensStitched = new List<string>();foreach (var token in tokens){if (!token.StartsWith("##")){currentToken = token + currentToken;tokensStitched.Add(currentToken);currentToken = string.Empty;}else{currentToken = token.Replace("##", "") + currentToken;}}tokensStitched.Reverse();return tokensStitched;}}
}
下载
源码下载
相关文章:
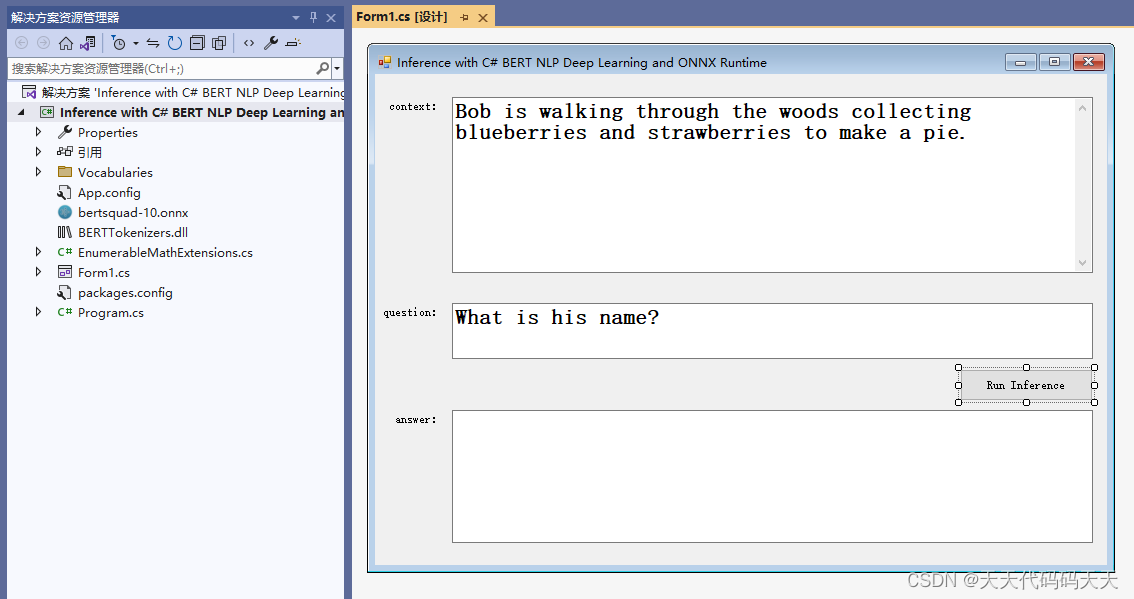
Inference with C# BERT NLP Deep Learning and ONNX Runtime
目录 效果 测试一 测试二 测试三 模型信息 项目 代码 下载 Inference with C# BERT NLP Deep Learning and ONNX Runtime 效果 测试一 Context :Bob is walking through the woods collecting blueberries and strawberries to make a pie. Question …...

6、原型模式(Prototype Pattern,不常用)
原型模式指通过调用原型实例的Clone方法或其他手段来创建对象。 原型模式属于创建型设计模式,它以当前对象为原型(蓝本)来创建另一个新的对象,而无须知道创建的细节。原型模式在Java中通常使用Clone技术实现,在JavaSc…...
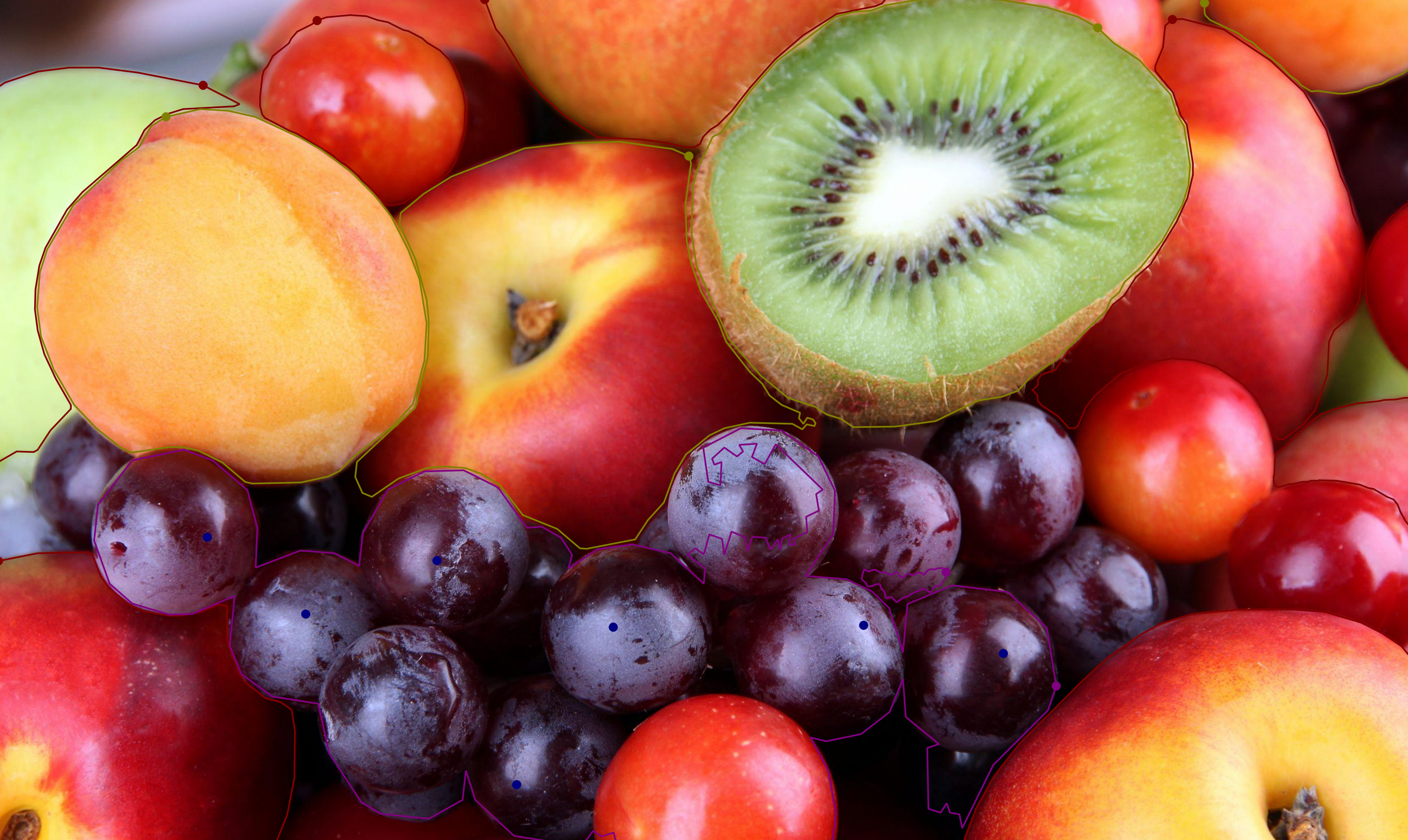
图像万物分割——Segment Anything算法解析与模型推理
一、概述 在视觉任务中,图像分割任务是一个很广泛的领域,应用于交互式分割,边缘检测,超像素化,感兴趣目标生成,前景分割,语义分割,实例分割,泛视分割等。 交互式分割&am…...
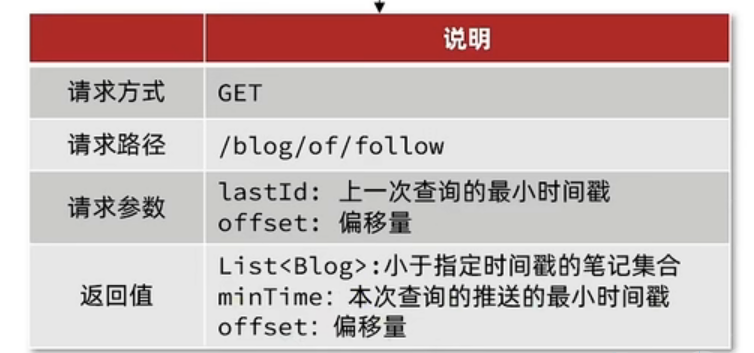
Redis实战篇笔记(最终篇)
Redis实战篇笔记(七) 文章目录 Redis实战篇笔记(七)前言达人探店发布和查看探店笔记点赞点赞排行榜 好友关注关注和取关共同关注关注推送关注推荐的实现 总结 前言 本系列文章是Redis实战篇笔记的最后一篇,那么到这里…...

游戏配置表的导入使用
游戏配置表是游戏策划的标配,如下图: 那么程序怎么把这张配置表导入使用? 1.首先,利用命令行把Excel格式的文件转化成Json格式: json-excel\json-excel json Tables\ Data\copy Data\CharacterDefine.txt ..\Clien…...
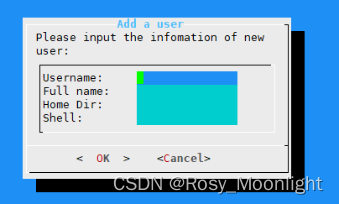
❀dialog命令运用于linux❀
目录 ❀dialog命令运用于linux❀ msgbox部件(消息框) yesno部件(yesno框) inputbox部件(输入文本框) textbox部件(文本框) menu部件(菜单框) fselect部…...
【算法】蓝桥杯2013国C 横向打印二叉树 题解
文章目录 题目链接题目描述输入格式输出格式样例自己的样例输入自己的样例输出 思路整体思路存储二叉搜索树中序遍历并存储计算目标数的行号dfs遍历并写入数组初始化和处理输入输出初始化处理输入处理输出 完整的代码如下 结束语更新初始化的修改存储二叉搜索树的修改中序遍历和…...
XunSearch 讯搜 error: storage size of ‘methods_bufferevent’ isn’t known
报错: error: storage size of ‘methods_bufferevent’ isn’t known CentOS8.0安装迅搜(XunSearch)引擎报错的解决办法 比较完整的文档 http://www.xunsearch.com/download/xs_quickstart.pdf 官方安装文档 http://www.xunsearch.com/doc/php/guide/start.in…...
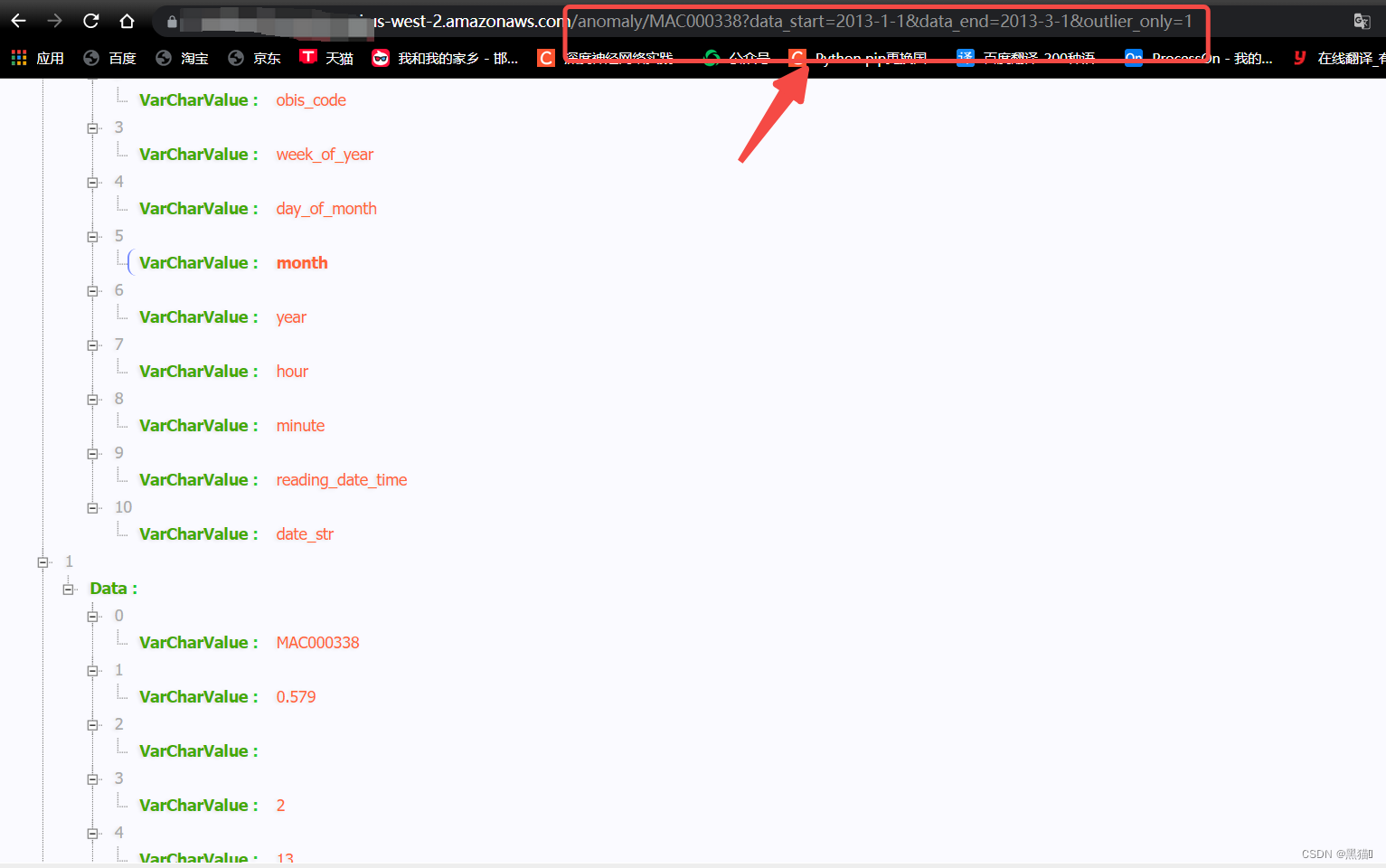
基于AWS Serverless的Glue服务进行ETL(提取、转换和加载)数据分析(三)——serverless数据分析
3 serverless数据分析 大纲 3 serverless数据分析3.1 创建Lambda3.2 创建API Gateway3.3 结果3.4 总结 3.1 创建Lambda 在Lambda中,我们将使用python3作为代码语言。 步骤图例1、入口2、创建(我们选择使用python3.7)3、IAM权限(…...

08、分析测试执行时间及获取pytest帮助
官方用例 # content of test_slow_func.py import pytest from time import sleeppytest.mark.parametrize(delay,(1.1,1.2,1.3,1.4,1.5,1.6,1.7,1.8,1.9,1.0,0.1,0.2,0,3)) def test_slow_func(delay):print("test_slow_func {}".format(delay))sleep(delay)assert…...
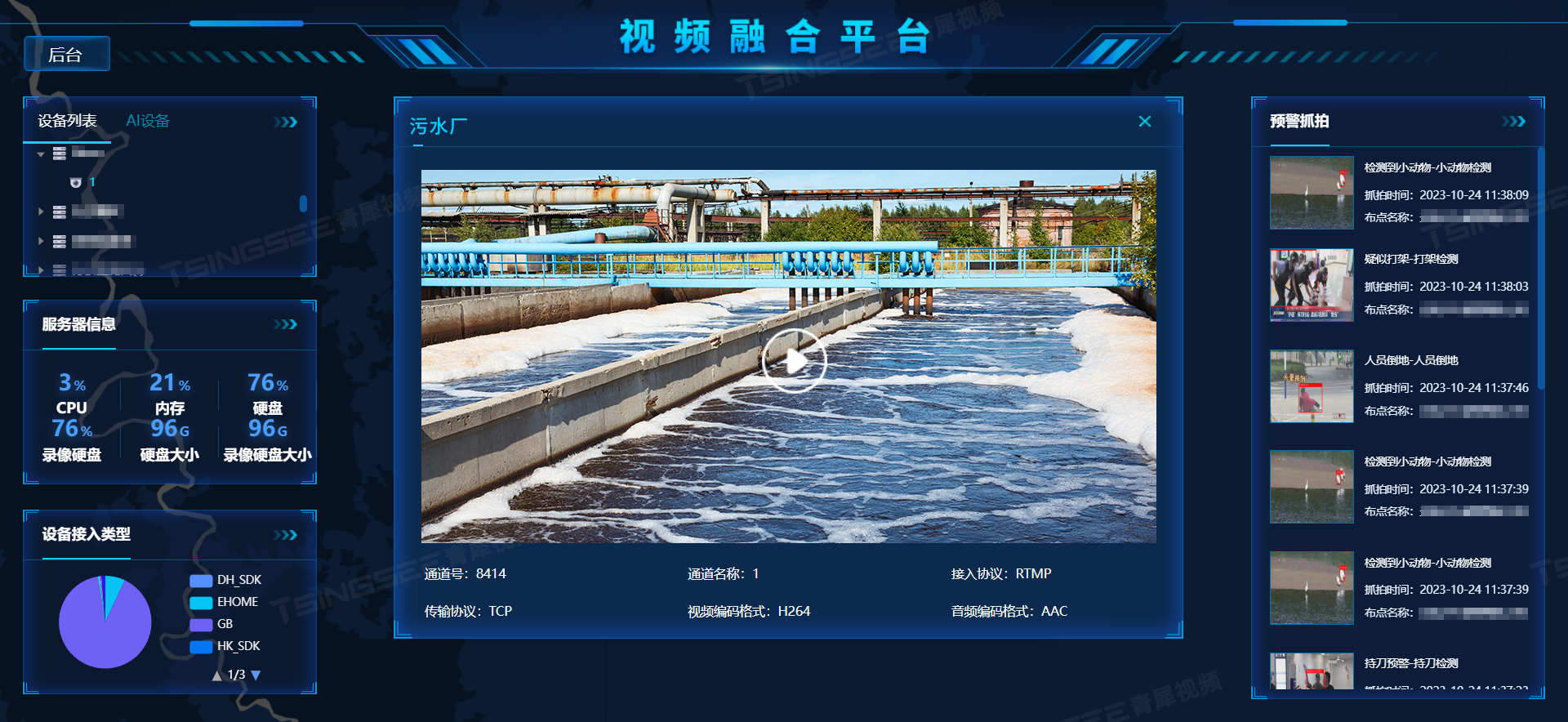
视频集中存储/智能分析融合云平台EasyCVR平台接入rtsp,突然断流是什么原因?
安防视频监控/视频集中存储/云存储/磁盘阵列EasyCVR平台可拓展性强、视频能力灵活、部署轻快,可支持的主流标准协议有国标GB28181、RTSP/Onvif、RTMP等,以及支持厂家私有协议与SDK接入,包括海康Ehome、海大宇等设备的SDK等。平台既具备传统安…...
JavaScript 复杂的<三元运算符和比较操作>的组合--案例(一)
在逆向的时候,碰上有些复杂的js代码,逻辑弄得人有点混; 因此本帖用来记录一些棘手的代码,方便自己记忆,也让大家拓展认识~ ----前言 内容: function(e, t, n) {try {1 (e "{" e[0] ? JSON.parse(e) : JSON.parse(webInstace.shell(e))).Status || 200 e.Code…...
uniapp搭建内网映射测试https域名
搭建Https域名服务器 使用github的frp搭建,使用宝塔申请免费https证书,需要先关闭宝塔nginx的反向代理,申请完域名后再开启反向代理即可。 教程 新版frp搭建教程 启动命令 服务器端 sudo systemctl start frps本地 cd D:\软件安装包\f…...
国防科技大博士招生入学考试【50+论文主观题】
目录 回答模板大意创新和学术价值启发 论文分类(根据问题/场景分类)数学问题Efficient Multiset Synchronization(高效的多集同步【简单集合/可逆计数Bloom过滤器】)大意创新和学术价值启发 An empirical study of Bayesian netwo…...
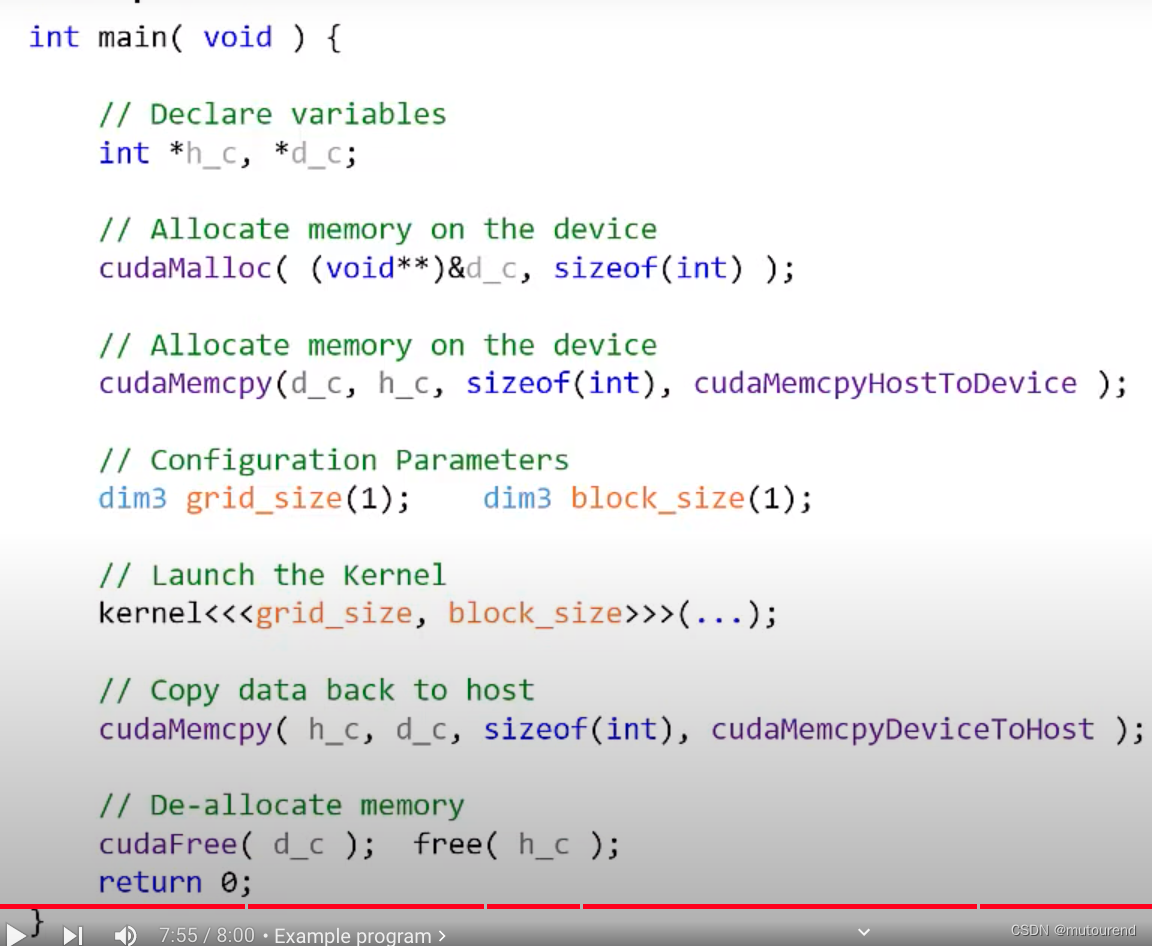
CUDA简介——编程模式
1. 引言 前序博客: CUDA简介——基本概念 CPU是用于控制的。即,host控制整个程序流程: 1)程序以Host代码main函数开始,然后顺序执行。 Host代码是顺序执行的,并执行在CPU之上。Host代码会负责Launch ke…...
Linux 软件安装
目录 一、Linux 1、Linux异常解决 1、JDK安装 1、Linux卸载JDK 2、Linux安装JDK 2、Redis安装 一、Linux 1、Linux异常解决 1、Another app is currently holding the yum lock; waiting for it to exit... 解决办法: rm -f /var/run/yum.pid1、杀死这个应用程序 ps a…...

flask之邮件发送
一、安装Flask-Mail扩展 pip install Flask-Mail二、配置Flask-Mail 格式:app.config[参数]值 三、实现方法 3.1、Mail类 常用类方法 3.2、Message类,它封装了一封电子邮件。构造函数参数如下: flask-mail.Message(subject, recipient…...
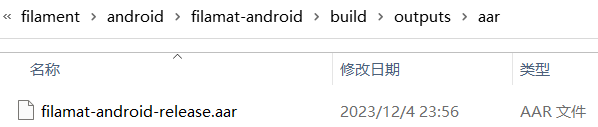
【Filament】Filament环境搭建
1 前言 Filament 是一个实时物理渲染引擎,用于 Android、iOS、Linux、macOS、Windows 和 WebGL 平台。该引擎旨在提供高效、实时的图形渲染,并被设计为在 Android 平台上尽可能小而尽可能高效。Filament 支持基于物理的渲染(PBR)&…...
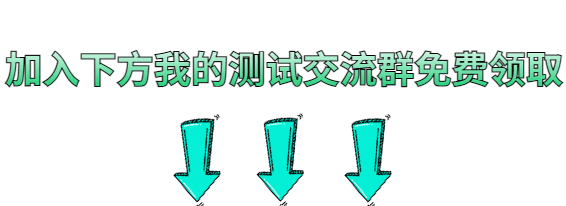
外包干了2个月,技术倒退2年。。。。。
先说一下自己的情况,本科生,20年通过校招进入深圳某软件公司,干了接近4年的功能测试,今年国庆,感觉自己不能够在这样下去了,长时间呆在一个舒适的环境会让一个人堕落!而我已经在一个企业干了四年的功能测试…...
使用 python ffmpeg 批量检查 音频文件 是否损坏或不完整
自用工具,检查下载的音乐是否有损坏 或 下载不完整 使用方法,把 in_dir r’D:\158首无损珍藏版’ 改成你自己的音乐文件夹路径 如果发现文件有损坏,则会在命令行打印错误文件的路径 注意,要求 ffmpeg 命令可以直接在命令行调用…...
【位运算】消失的两个数字(hard)
消失的两个数字(hard) 题⽬描述:解法(位运算):Java 算法代码:更简便代码 题⽬链接:⾯试题 17.19. 消失的两个数字 题⽬描述: 给定⼀个数组,包含从 1 到 N 所有…...
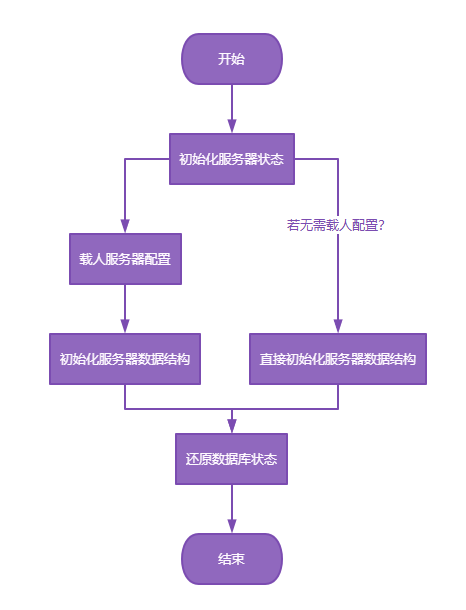
【Redis技术进阶之路】「原理分析系列开篇」分析客户端和服务端网络诵信交互实现(服务端执行命令请求的过程 - 初始化服务器)
服务端执行命令请求的过程 【专栏简介】【技术大纲】【专栏目标】【目标人群】1. Redis爱好者与社区成员2. 后端开发和系统架构师3. 计算机专业的本科生及研究生 初始化服务器1. 初始化服务器状态结构初始化RedisServer变量 2. 加载相关系统配置和用户配置参数定制化配置参数案…...
Auto-Coder使用GPT-4o完成:在用TabPFN这个模型构建一个预测未来3天涨跌的分类任务
通过akshare库,获取股票数据,并生成TabPFN这个模型 可以识别、处理的格式,写一个完整的预处理示例,并构建一个预测未来 3 天股价涨跌的分类任务 用TabPFN这个模型构建一个预测未来 3 天股价涨跌的分类任务,进行预测并输…...
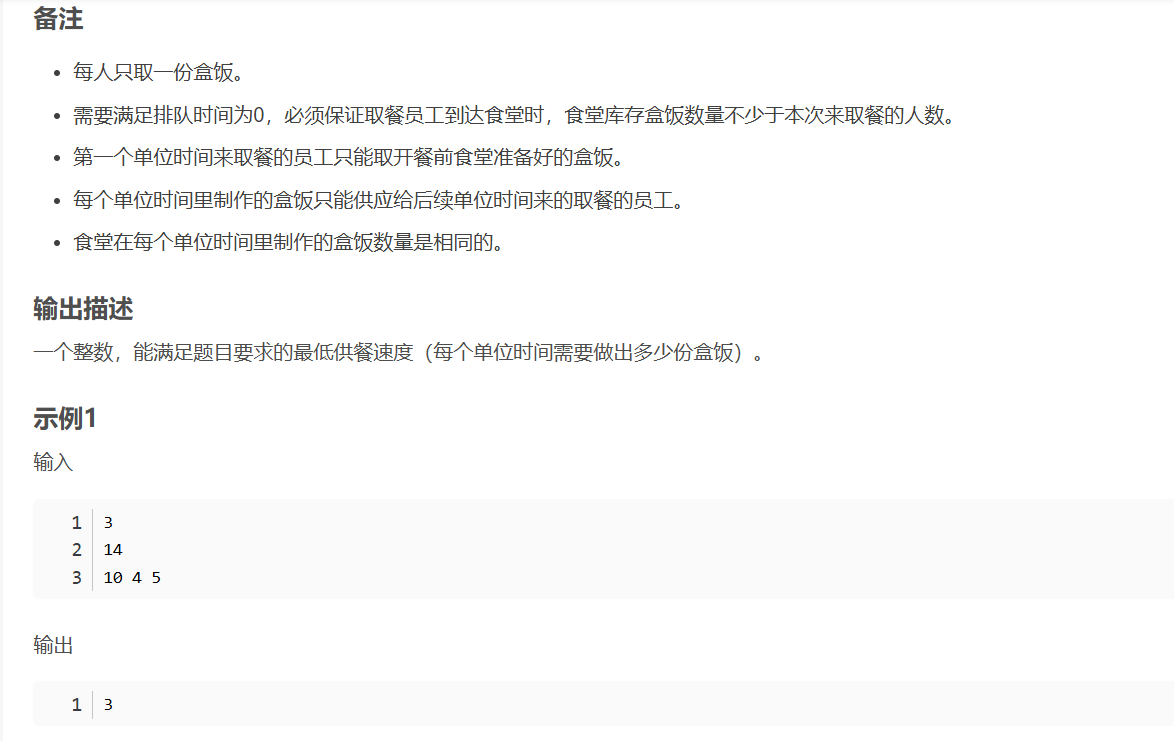
华为OD机试-食堂供餐-二分法
import java.util.Arrays; import java.util.Scanner;public class DemoTest3 {public static void main(String[] args) {Scanner in new Scanner(System.in);// 注意 hasNext 和 hasNextLine 的区别while (in.hasNextLine()) { // 注意 while 处理多个 caseint a in.nextIn…...
高效线程安全的单例模式:Python 中的懒加载与自定义初始化参数
高效线程安全的单例模式:Python 中的懒加载与自定义初始化参数 在软件开发中,单例模式(Singleton Pattern)是一种常见的设计模式,确保一个类仅有一个实例,并提供一个全局访问点。在多线程环境下,实现单例模式时需要注意线程安全问题,以防止多个线程同时创建实例,导致…...
tomcat指定使用的jdk版本
说明 有时候需要对tomcat配置指定的jdk版本号,此时,我们可以通过以下方式进行配置 设置方式 找到tomcat的bin目录中的setclasspath.bat。如果是linux系统则是setclasspath.sh set JAVA_HOMEC:\Program Files\Java\jdk8 set JRE_HOMEC:\Program Files…...
go 里面的指针
指针 在 Go 中,指针(pointer)是一个变量的内存地址,就像 C 语言那样: a : 10 p : &a // p 是一个指向 a 的指针 fmt.Println(*p) // 输出 10,通过指针解引用• &a 表示获取变量 a 的地址 p 表示…...
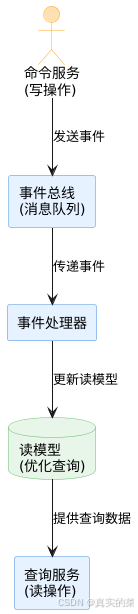
消息队列系统设计与实践全解析
文章目录 🚀 消息队列系统设计与实践全解析🔍 一、消息队列选型1.1 业务场景匹配矩阵1.2 吞吐量/延迟/可靠性权衡💡 权衡决策框架 1.3 运维复杂度评估🔧 运维成本降低策略 🏗️ 二、典型架构设计2.1 分布式事务最终一致…...
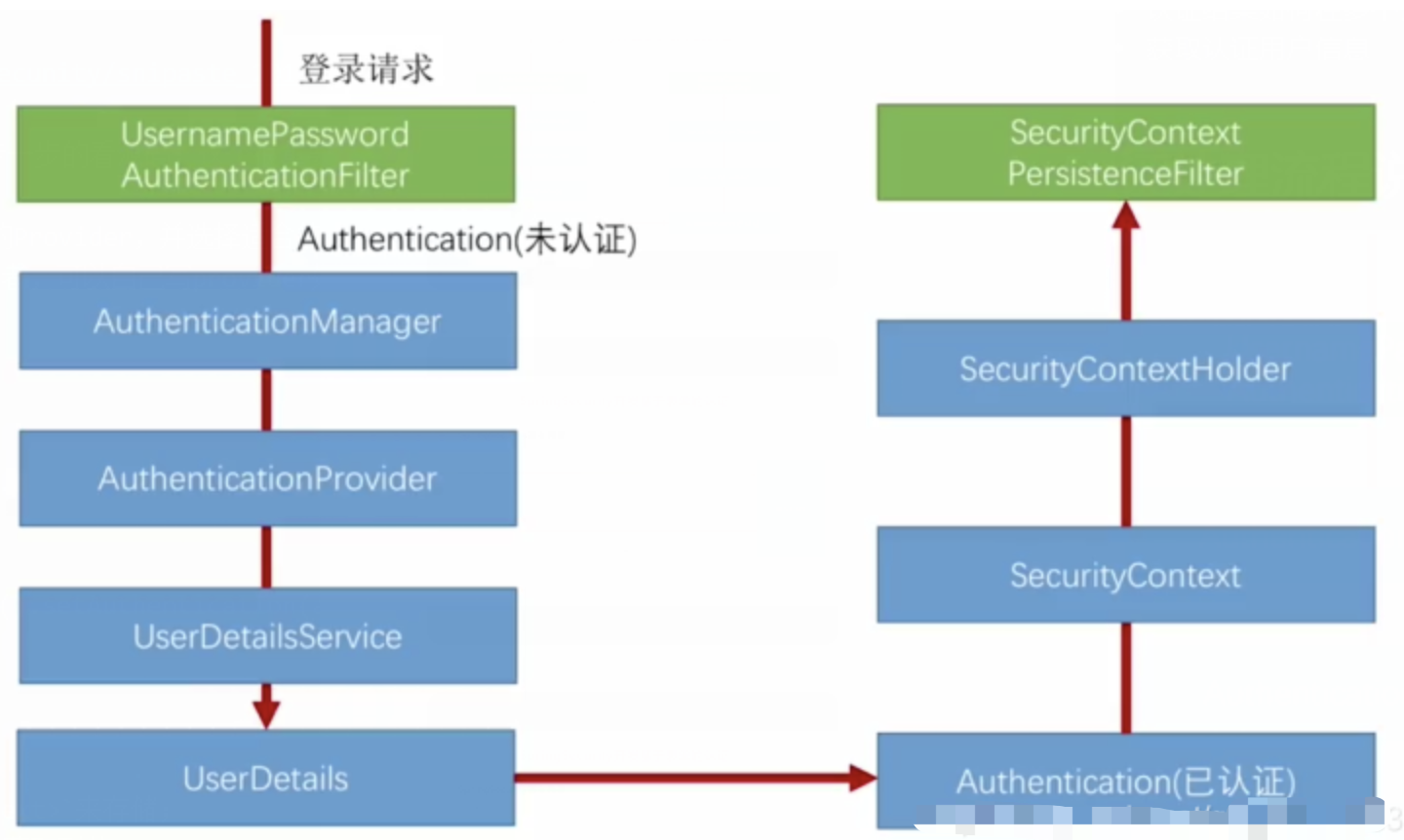
spring Security对RBAC及其ABAC的支持使用
RBAC (基于角色的访问控制) RBAC (Role-Based Access Control) 是 Spring Security 中最常用的权限模型,它将权限分配给角色,再将角色分配给用户。 RBAC 核心实现 1. 数据库设计 users roles permissions ------- ------…...

CVE-2023-25194源码分析与漏洞复现(Kafka JNDI注入)
漏洞概述 漏洞名称:Apache Kafka Connect JNDI注入导致的远程代码执行漏洞 CVE编号:CVE-2023-25194 CVSS评分:8.8 影响版本:Apache Kafka 2.3.0 - 3.3.2 修复版本:≥ 3.4.0 漏洞类型:反序列化导致的远程代…...