05 Vue中常用的指令
概述
All Vue-based directives start with a v-* prefix as a Vue-specific attribute.
所有基于 Vue 的指令都以 v-* 前缀作为 Vue 特有的属性。
v-text
The v-text directive has the same reactivity as with interpolation. Interpolation with {{ }} is more performant than the v-text directive. However, you may find yourself in situations where you have pre-rendered text from a server and want to override it once your Vue application has finished loading. For example, you can pre-define a static placeholder text while waiting for the Vue engine to eventually replace it with the dynamic value received from vtext, as shown in the following code block:
v-text 指令的反应性与插值相同。与 v-text 指令相比,使用 {{ }} 进行插值的性能更高。不过,您可能会遇到这样的情况,即您已从服务器预渲染了文本,但希望在 Vue 应用程序加载完成后覆盖它。例如,您可以预先定义一个静态占位符文本,同时等待 Vue 引擎最终将其替换为从 vtext 接收的动态值,如下代码块所示:
<template><div v-text="msg">My placeholder</div>
</template>
<script setup>
const msg = "My message"
</script>
v-once
When used, it indicates the starting point of static content. The Vue engine will render the component with this attribute and its children exactly once. It also ignores all data updates for this component or element after the initial render. This attribute is handy for scenarios with no reactivity needed for certain parts. You can combine v-once with v-text, interpolation, and any Vue directive.
使用时,它表示静态内容的起点。Vue 引擎将对带有此属性的组件及其子组件进行一次精确的呈现。在首次呈现后,它还会忽略该组件或元素的所有数据更新。对于某些部分不需要反应性的场景,该属性非常方便。您可以将 v-once 与 v-text、插值和任何 Vue 指令结合使用。
v-html
Vue will parse the value passed to this directive and render your text data as a valid HTML code into the target element. We don’t recommend using this directive, especially on the client side, due to its performance impact and the potential security leak. The script tag can be embedded and triggered using this directive.
Vue 会解析传递给此指令的值,并将文本数据作为有效的 HTML 代码呈现到目标元素中。我们不建议使用此指令,尤其是在客户端,因为它会影响性能并可能造成安全漏洞。可使用此指令嵌入并触发脚本标签。
v-bind
This directive is one of the most popular Vue features. You can use this directive to enable one-way binding for a data variable or an expression to an HTML attribute, as shown in the following example:
该指令是最受欢迎的 Vue 功能之一。您可以使用该指令将数据变量或表达式单向绑定到 HTML 属性,如下例所示:
<template><img v-bind:src="logo" />
</template>
<script setup>
const logo = '../assets/logo.png';
</script>
The preceding code demonstrates how to bind the logo data variable to image’s src. The img component now takes the source value from the logo variable and renders the image accordingly.
前面的代码演示了如何将徽标数据变量与图像的 src 绑定。现在,img 组件从徽标变量中获取源值,并相应地渲染图像。
You can also use it to pass a local data variable as props to another component. A shorter way is using the :attr syntax instead of v-bind:attr. Take the preceding example, for instance. We can rewrite the template as follows:
您还可以使用它将本地数据变量作为道具传递给另一个组件。更简便的方法是使用 :attr 语法而不是 v-bind:attr。以前面的例子为例。我们可以将模板重写如下:
<template><img :src="logo" />
</template>
v-if
This is a powerful directive you can use to conditionally control how elements render inside a component. This directive operates like the if…else and if…else if… conditions.
这是一个功能强大的指令,可用于有条件地控制组件内元素的呈现方式。该指令的操作类似于 if…else 和 if…else if… 条件。
It comes with supporting directives, such as v-else, standing for the else case, and velse-if, standing for the else if case. For example, we want to render different text when count is 2, 4, and 6. The following code will demonstrate how to do so:
它带有支持指令,如 v-else(表示 else 情况)和 velse-if(表示 else if 情况)。例如,我们想在计数为 2、4 和 6 时显示不同的文本。下面的代码将演示如何做到这一点:
<template><div v-if="count === 2">Two</div><div v-else-if="count === 4">Four</div><div v-else-if="count === 6">Six</div><div v-else>Others</div>
</template>
<script setup>
const count = 6
</script>
v-show
You can also control the visible state of HTML elements by using v-show.
您还可以使用 v-show 来控制 HTML 元素的可见状态。
Unlike v-if, with v-show, the Vue engine still mounts the element to the DOM tree but hides it using the display: none CSS style.
与 v-if 不同,使用 v-show 时,Vue 引擎仍会将元素挂载到 DOM 树中,但会使用 display: none
CSS 样式将其隐藏。
You can still see the content of the hidden element visible in the DOM tree upon inspecting it, but it is not visible on the UI to end users.
在检查隐藏元素时,您仍然可以在 DOM 树中看到隐藏元素的内容,但最终用户无法在用户界面上看到它。
This directive does not work with v-else or v-else-if.
该指令不适用于 v-else 或 v-else-if。
If v-show results in a true Boolean, it will leave the DOM element as is. If it resolves as false, it will apply the display:none style to the element.
如果 v-show 的布尔值为 true,它将保持 DOM 元素的原样。如果解析为 false,则会对元素应用 display:none 样式。
v-for
We use the v-for directive to accomplish the goal of list rendering based on a data source. The data source is an iterative data collection, such as an array or object. We will dive deeper into different use cases for this directive in a separate section within this chapter.
我们使用 v-for 指令来实现基于数据源的列表渲染目标。数据源是一个迭代数据集合,如数组或对象。我们将在本章的另一节中深入探讨该指令的不同用例。
综合练习
We have gone over the most common directives in Vue. Let’s review and experiment with how to use these directives with the following exercise.
我们已经介绍了 Vue 中最常用的指令。让我们通过下面的练习来回顾并尝试如何使用这些指令。
More complicated components will use multiple directives to achieve the desired outcome. In this exercise, we will construct a component that uses several directives to bind, manipulate, and output data to a template view.
更复杂的组件将使用多个指令来实现所需的结果。在本练习中,我们将构建一个使用多个指令绑定、操作和输出数据到模板视图的组件。
Create a new Vue component file named Exercise1-03.vue in the src/components directory.
在 src/components 目录中新建一个名为 Exercise1-03.vue 的 Vue 组件文件。
Inside Exercise1-03.vue, compose the following code to display the text content:
在 Exercise1-03.vue 中,编写以下代码以显示文本内容:
<template><div><h1>{{ text }}</h1></div>
</template>
<script setup>
const text = 'Directive text';
</script>
修改App.vue,引入组件并渲染:
<script setup>
import Exercise from "./components/Exercise1-03.vue";
</script>
<template><Exercise/>
</template>
Replace the {{}} interpolation with the v-text attribute. The output should not change:
用 v-text 属性替换 {{}} 插值。输出结果不会改变:
<template><div><h1 v-text="text"></h1></div>
</template>
<script setup>
const text = 'Directive text';
</script>
Add the v-once directive to the same element. This will force this DOM element to only load the v-text data once:
在同一元素中添加 v-once 指令。这将迫使 DOM 元素只加载一次 v-text 数据:
<template><div><h1 v-once v-text="text"></h1></div>
</template>
Underneath the h1 element, include a new h2 element that uses the v-html attribute. Add a new local data called html that contains a string with HTML formatting in it, as shown in the following code block:
在 h1 元素下方,加入一个使用 v-html 属性的新 h2 元素。添加名为 html 的新本地数据,该数据包含一个带有 HTML 格式的字符串,如以下代码块所示:
<template><div><h1 v-once v-text="text"></h1><h2 v-html="html" /></div>
</template>
<script setup>
const text = 'Directive text';
const html = 'Stylise</br>HTML in<br/><b>your data</b>'
</script>
Add a new local link object that contains a bunch of information such as the URL, target, title, and tab index. Inside the template, add a new anchor HTML element and bind the link object to the HTML element using the v-bind short syntax – for example, :href=“link.url”:
添加一个新的本地链接对象,其中包含大量信息,如 URL、目标、标题和标签索引。在模板中添加一个新的锚 HTML 元素,并使用 v-bind 简语法将链接对象绑定到 HTML 元素,例如 :href=“link.url”:
<template><div><h1 v-once v-text="text"></h1><h2 v-html="html"/><a:href="link.url":target="link.target":tabindex="link.tabindex">{{ link.title }}</a></div>
</template>
<script setup>
const text = 'Directive text';
const html = 'Stylise</br>HTML in<br/><b>your data</b>'
const link = {title: "Go to Google",url: "https://google.com",tabindex: 1,target: '_blank',
};
</script>
Apply v-if=“false” to the h1 element, v-else-if=“false” to h2, and v-else to the a tag like this:
在 h1 元素上应用 v-if=“false”,在 h2 上应用 v-else-if=“false”,在 a 标签上应用 v-else,就像这样:
<template><div><h1 v-if="false" v-once v-text="text"></h1><h2 v-else-if="false" v-html="html"/><av-else:href="link.url":target="link.target":tabindex="link.tabindex">{{ link.title }}</a></div>
</template>
<script setup>
const text = 'Directive text';
const html = 'Stylise</br>HTML in<br/><b>your data</b>'
const link = {title: "Go to Google",url: "https://google.com",tabindex: 1,target: '_blank',
};
</script>
You should only see the <a>
tag on the page since we have set the main conditional statements to false.
由于我们已将主要条件语句设置为 false,因此在页面上应该只能看到 <a>
标记。
Change the template to use v-show instead of the v-if statements, remove v-else from the <a>
element, and change the value of v-show in h1 to true:
更改模板,使用 v-show 代替 v-if 语句,删除 <a>
元素中的 v-else,并将 h1 中 v-show 的值改为 true:
<template><div><h1 v-show="true" v-once v-text="text"></h1><h2 v-show="false" v-html="html"/><a:href="link.url":target="link.target":tabindex="link.tabindex">{{ link.title }}</a></div>
</template>
<script setup>
const text = 'Directive text';
const html = 'Stylise</br>HTML in<br/><b>your data</b>'
const link = {title: "Go to Google",url: "https://google.com",tabindex: 1,target: '_blank',
};
</script>
In this exercise, we learned about the core Vue directives to control, bind, show, and hide HTML template elements without requiring any JavaScript outside of adding new data objects to your local state.
在本练习中,我们学习了 Vue 的核心指令,这些指令用于控制、绑定、显示和隐藏 HTML 模板元素,而无需任何 JavaScript,只需在本地状态中添加新的数据对象即可。
In the next section, we will learn how to achieve two-way binding with the help of Vue’s v-model.
在下一节中,我们将学习如何借助 Vue 的 v 模型实现双向绑定。
相关文章:
05 Vue中常用的指令
概述 All Vue-based directives start with a v-* prefix as a Vue-specific attribute. 所有基于 Vue 的指令都以 v-* 前缀作为 Vue 特有的属性。 v-text The v-text directive has the same reactivity as with interpolation. Interpolation with {{ }} is more perform…...
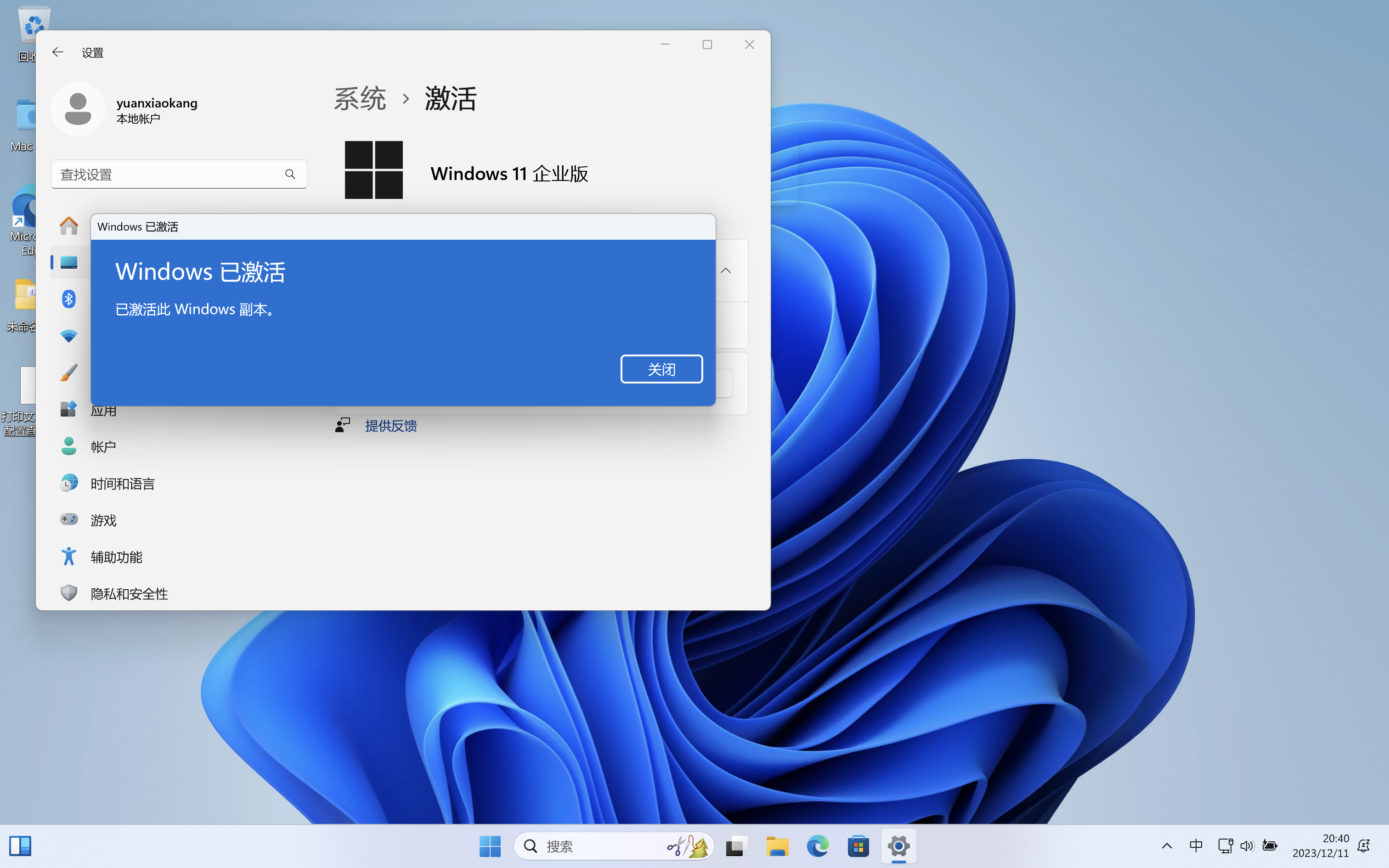
Mr. Cappuccino的第67杯咖啡——MacOS通过PD安装Win11
MacOS通过PD安装Win11 下载ParallelsDesktop安装ParallelsDesktop激活ParallelsDesktop下载Windows11安装Windows11激活Windows11 下载ParallelsDesktop ParallelsDesktop下载地址 安装ParallelsDesktop 关闭上面的窗口,继续操作 激活ParallelsDesktop 关闭上面的…...

【云原生kubernets】Service 的功能与应用
一、Service介绍 在kubernetes中,pod是应用程序的载体,我们可以通过pod的ip来访问应用程序,但是pod的ip地址不是固定的,这也就意味着不方便直接采用pod的ip对服务进行访问。为了解决这个问题,kubernetes提供了Service资…...
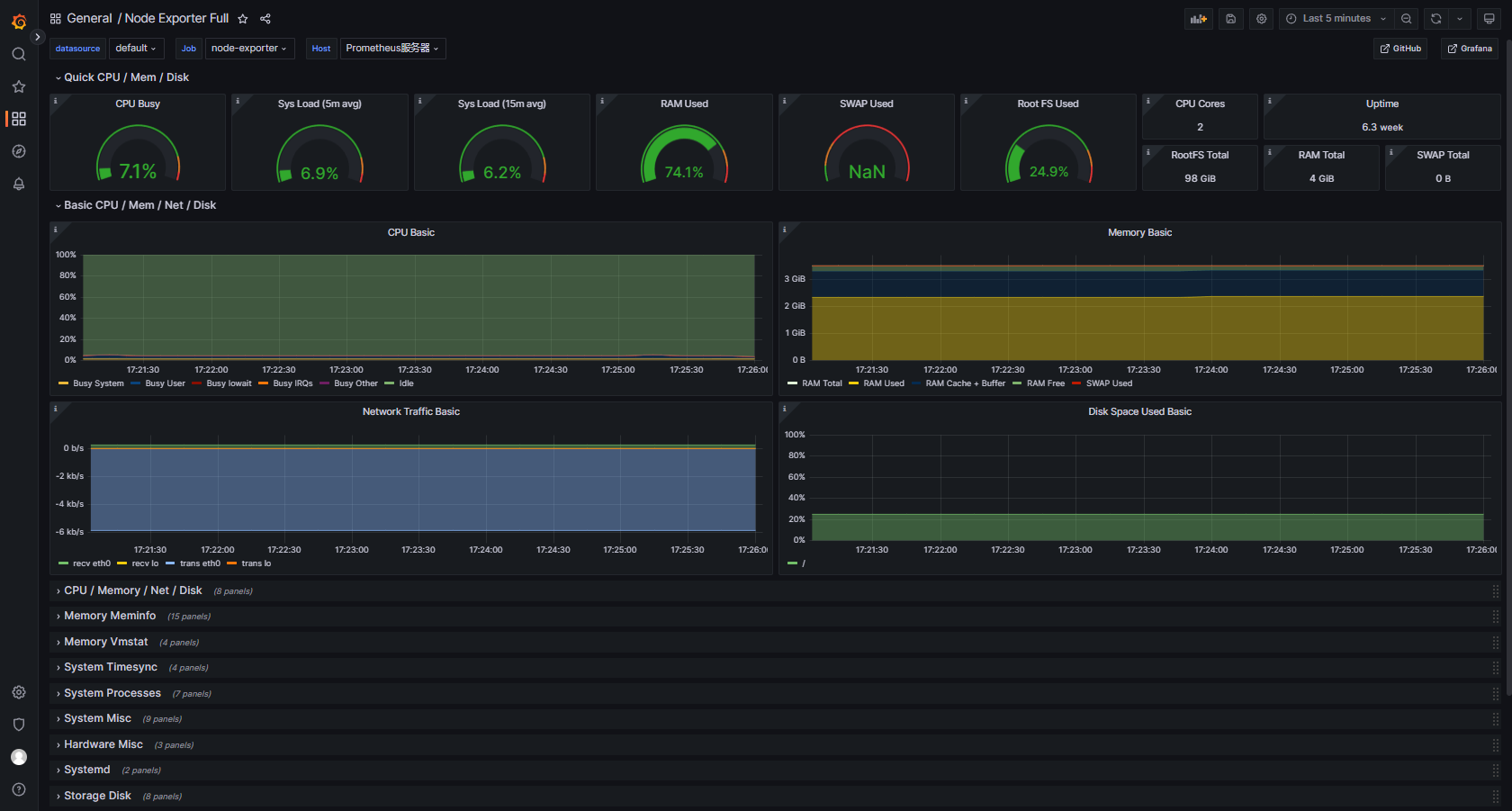
docker安装Prometheus
docker安装Prometheus Docker搭建Prometheus监控系统 环境准备(这里的环境和版本是经过测试没有问题,并不是必须这个版本) 主机名IP配置系统说明localhost随意2核4gCentOS7或者Ubuntu20.0.4docker版本23.0.1或者24.0.5,docker-compose版本1.29 安装Docker Ubuntu20.0.4版本…...
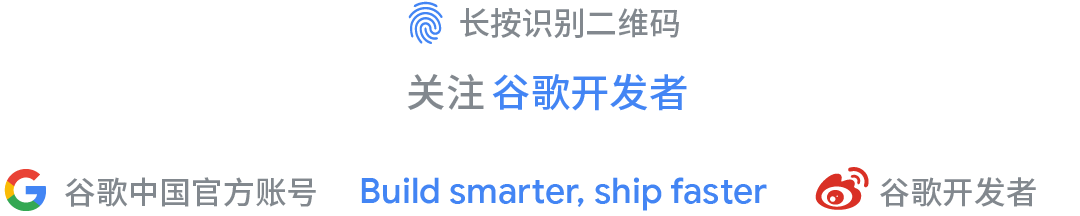
了解 Flutter 3.16 功能更新
作者 / Kevin Chisholm 我们在季度 Flutter 稳定版发布会上带来了 Flutter 3.16,此版本包含诸多更新: Material 3 成为新的默认主题、为 Android 带来 Impeller 的预览版、允许添加适用于 DevTools 的扩展程序等等,以及同步推出 Flutter 休闲游戏工具包重…...
python之画动态图 gif效果图
import pandas as pd import matplotlib import matplotlib.pyplot as plt import os# set up matplotlib is_ipython inline in matplotlib.get_backend() if is_ipython:from IPython import displayplt.ion()def find_csv_files(directory):csv_files [] # 用于存储找到的…...
【JavaWeb】用注解代替配置文件
WebServlet("/query") public class QueryServlet extends HttpServlet {...}在Servlet类上写WebServlet("query"),就相当于在配置文件里写了↓ <servlet><servlet-name>query</servlet-name><servlet-class>QueryServlet</se…...
SpringBoot 3.0 升级之 Swagger 升级
文章目录 SpringFox3.0.0openapi3Swagger 注解迁移ApiApiOperationApiImplicitParamApiModelApiModelProperty 最近想尝试一下最新的 SpringBoot 项目,于是将自己的开源项目进行了一些升级。 JDK 版本从 JDK8 升级至 JDK17。SpringBoot 版本从 SpringBoot 2.7.3 升…...
AR游戏开发
增强现实(Augmented Reality,AR)游戏是一种整合了虚拟和现实元素的游戏体验。玩家通过使用AR设备(如智能手机、AR眼镜或平板电脑)来与真实世界互动,游戏中的数字内容与真实环境相结合。以下是一些关于AR游戏…...
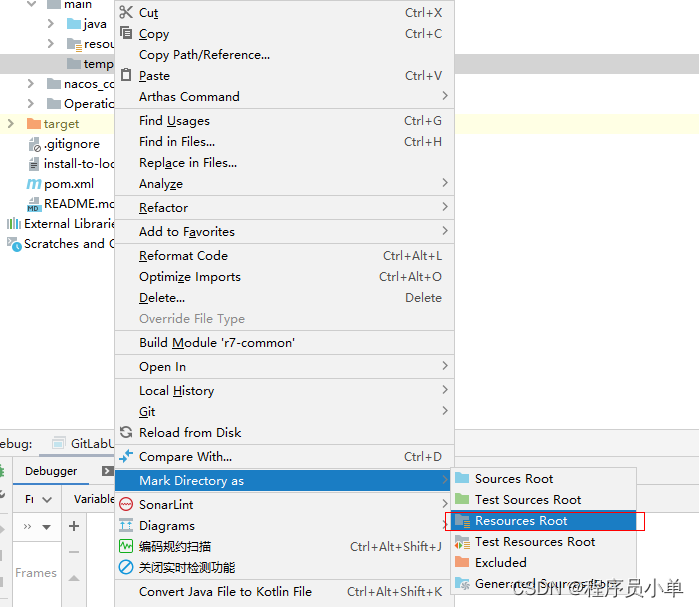
Easy Excel生成复杂下Excel模板(下拉框)给用户下载
引言 文件的下载是一个非常常见的功能,也有一些非常好的框架可以使用,这里我们就介绍一种比较常见的场景,下载Excel模版,导入功能通常会配有一个模版下载的功能,根据下载的模版,填充数据然后再上传。 需求…...
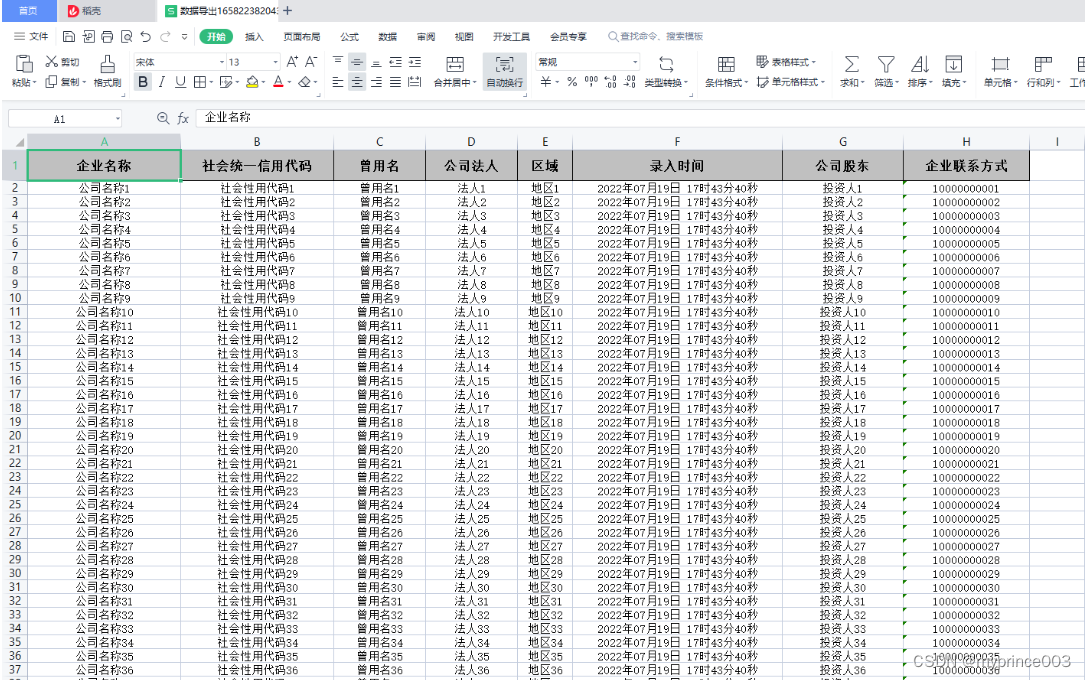
基于EasyExcel的数据导入导出
前言: 代码复制粘贴即可用,主要包含的功能有Excel模板下载、基于Excel数据导入、Excel数据导出。 根据实际情况修改一些细节即可,最后有结果展示,可以先看下结果,是否是您想要的。 台上一分钟,台下60秒&a…...
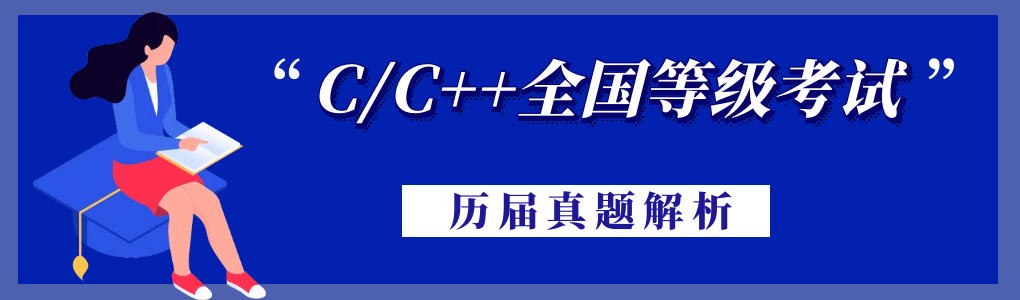
电子学会C/C++编程等级考试2021年06月(六级)真题解析
C/C++等级考试(1~8级)全部真题・点这里 第1题:逆波兰表达式 逆波兰表达式是一种把运算符前置的算术表达式,例如普通的表达式2 + 3的逆波兰表示法为+ 2 3。逆波兰表达式的优点是运算符之间不必有优先级关系,也不必用括号改变运算次序,例如(2 + 3) * 4的逆波兰表示法为* +…...
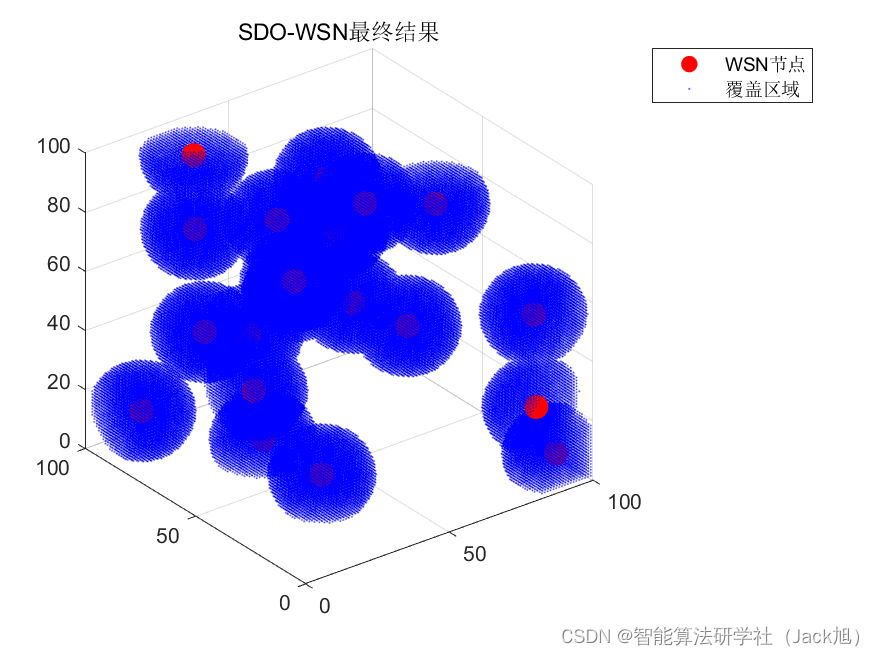
智能优化算法应用:基于供需算法3D无线传感器网络(WSN)覆盖优化 - 附代码
智能优化算法应用:基于供需算法3D无线传感器网络(WSN)覆盖优化 - 附代码 文章目录 智能优化算法应用:基于供需算法3D无线传感器网络(WSN)覆盖优化 - 附代码1.无线传感网络节点模型2.覆盖数学模型及分析3.供需算法4.实验参数设定5.算法结果6.参考文献7.MA…...
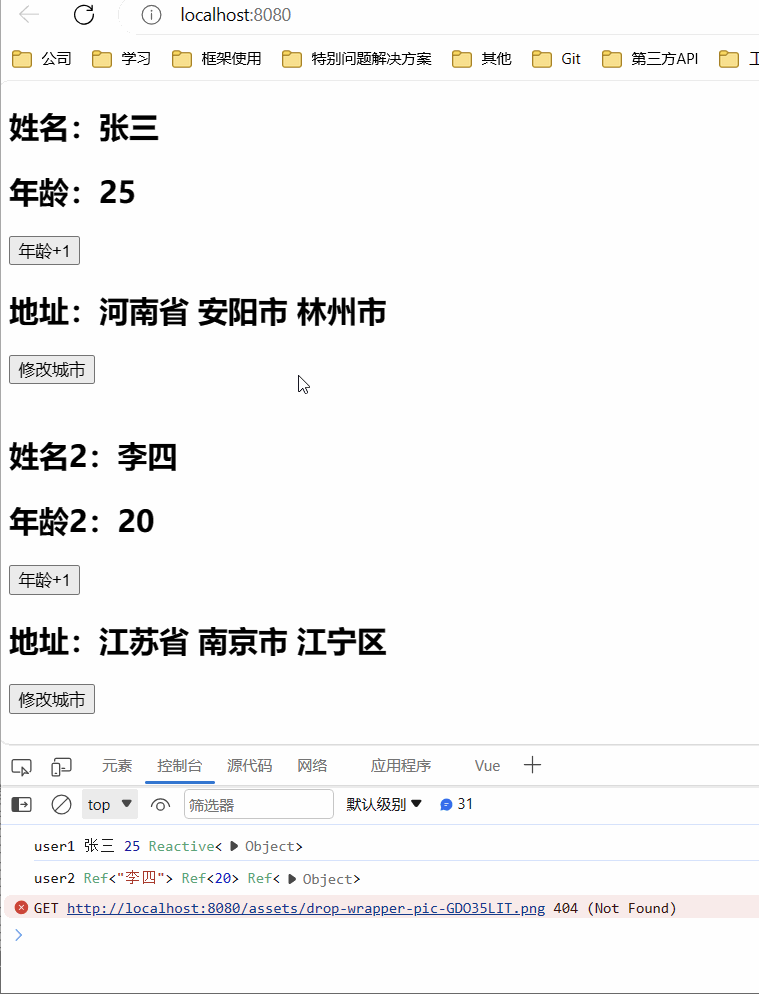
vue3 setup语法糖写法基本教程
前言 官网地址:Vue.js - 渐进式 JavaScript 框架 | Vue.js (vuejs.org)下面只讲Vue3与Vue2有差异的地方,一些相同的地方我会忽略或者一笔带过与Vue3一同出来的还有Vite,但是现在不使用它,等以后会有单独的教程使用。目前仍旧使用v…...
利用两个指针的差值求字符串长度
指针和指针也可以相加减,例如定义一个一维数组arr[10];再定义一个指针(int *p)指向数组首元素的地址,定义一个指针(int* q)指向数组最后一个元素的地址,那么q-p的结果就是整个数组的…...
ping命令的工作原理
ping,Packet Internet Groper,是一种因特网包探索器,用于测试网络连接量的程序。Ping 是工作在 TCP/IP 网络体系结构中应用层的一个服务命令, 主要是向特定的目的主机发送 ICMP(Internet Control Message Protocol 因特…...
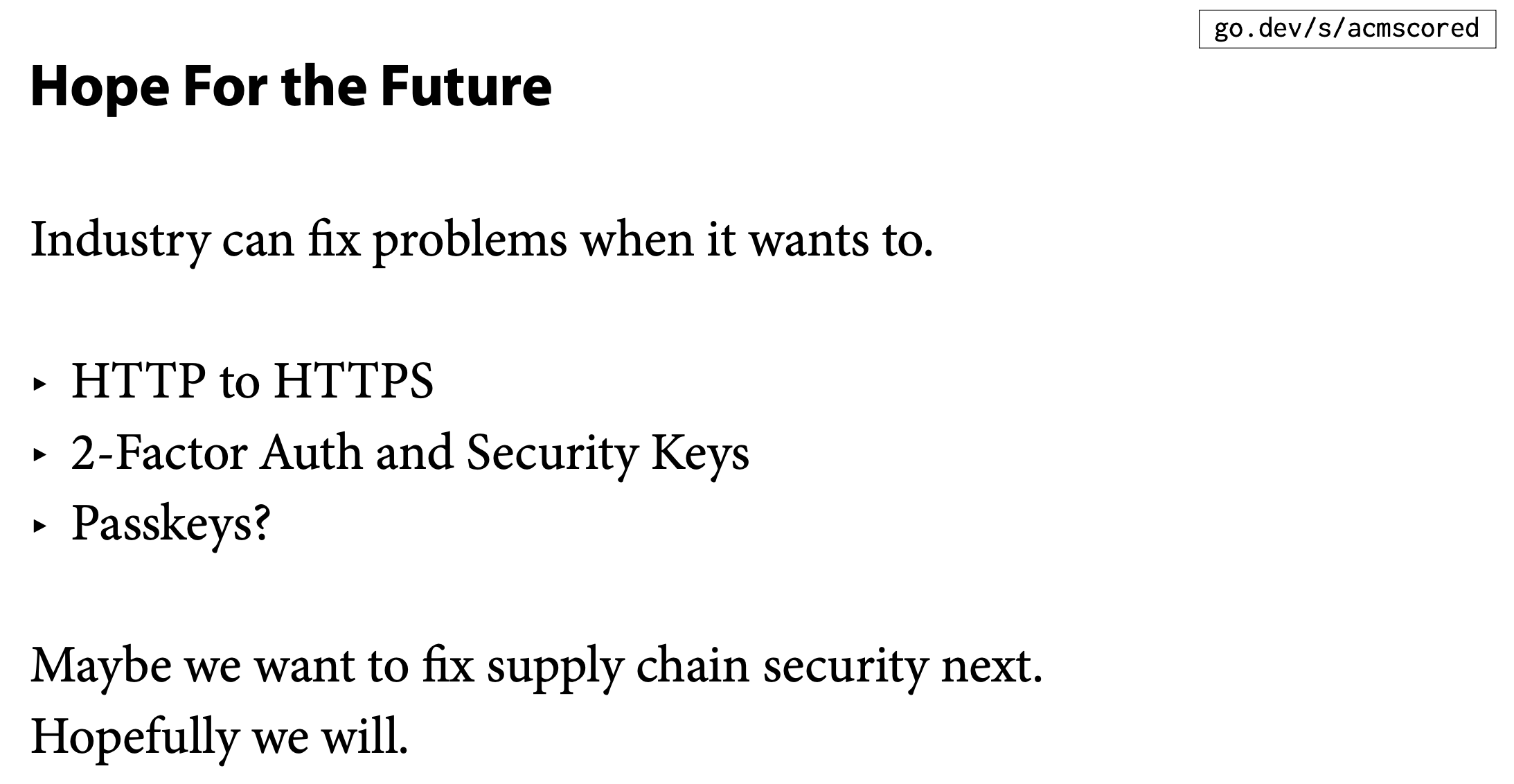
谷歌的开源供应链安全
本内容是对Go项目负责人Russ Cox 在 ACM SCORED 活动上演讲内容[1]的摘录与整理。 SCORED 是Software Supply Chain Offensive Research and Ecosystem Defenses的简称, SCORED 23[2]于2023年11月30日在丹麦哥本哈根及远程参会形式举行。 摘要 💡 谷歌在开源软件供应…...
分发饼干(贪心算法)
假设你是一位很棒的家长,想要给你的孩子们一些小饼干。但是,每个孩子最多只能给一块饼干。 对每个孩子 i,都有一个胃口值 g[i],这是能让孩子们满足胃口的饼干的最小尺寸;并且每块饼干 j,都有一个尺寸 s[j]…...
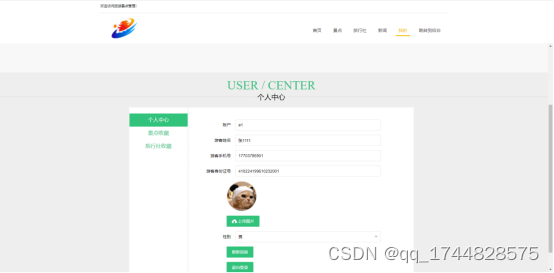
基于ssm旅游景点管理系统设计论文
摘 要 现代经济快节奏发展以及不断完善升级的信息化技术,让传统数据信息的管理升级为软件存储,归纳,集中处理数据信息的管理方式。本旅游景点管理系统就是在这样的大环境下诞生,其可以帮助管理者在短时间内处理完毕庞大的数据信息…...
用go封装一下封禁功能
思路 封禁业务也是在一般项目中比较常见的业务。我们也将它封装在库中作为功能之一。 我们同样使用adapter作为底层的存储结构,将封禁标示作为k-v结构存储。 把id和服务名称service作为key,把封禁的级别level作为value,以此我们能实现一些比…...
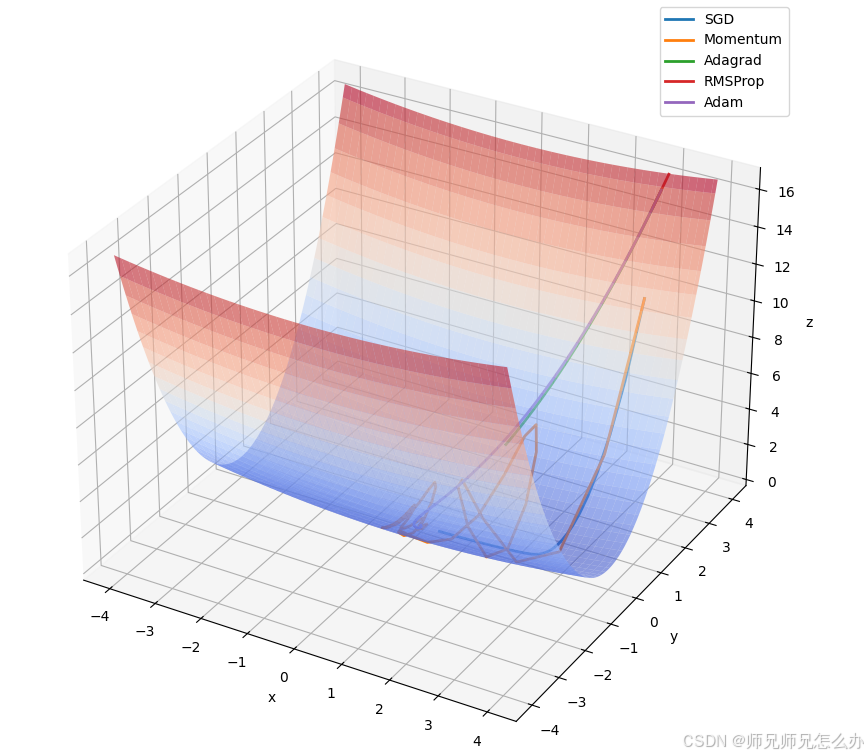
【人工智能】神经网络的优化器optimizer(二):Adagrad自适应学习率优化器
一.自适应梯度算法Adagrad概述 Adagrad(Adaptive Gradient Algorithm)是一种自适应学习率的优化算法,由Duchi等人在2011年提出。其核心思想是针对不同参数自动调整学习率,适合处理稀疏数据和不同参数梯度差异较大的场景。Adagrad通…...
测试markdown--肇兴
day1: 1、去程:7:04 --11:32高铁 高铁右转上售票大厅2楼,穿过候车厅下一楼,上大巴车 ¥10/人 **2、到达:**12点多到达寨子,买门票,美团/抖音:¥78人 3、中饭&a…...
Java多线程实现之Callable接口深度解析
Java多线程实现之Callable接口深度解析 一、Callable接口概述1.1 接口定义1.2 与Runnable接口的对比1.3 Future接口与FutureTask类 二、Callable接口的基本使用方法2.1 传统方式实现Callable接口2.2 使用Lambda表达式简化Callable实现2.3 使用FutureTask类执行Callable任务 三、…...
基于Java Swing的电子通讯录设计与实现:附系统托盘功能代码详解
JAVASQL电子通讯录带系统托盘 一、系统概述 本电子通讯录系统采用Java Swing开发桌面应用,结合SQLite数据库实现联系人管理功能,并集成系统托盘功能提升用户体验。系统支持联系人的增删改查、分组管理、搜索过滤等功能,同时可以最小化到系统…...
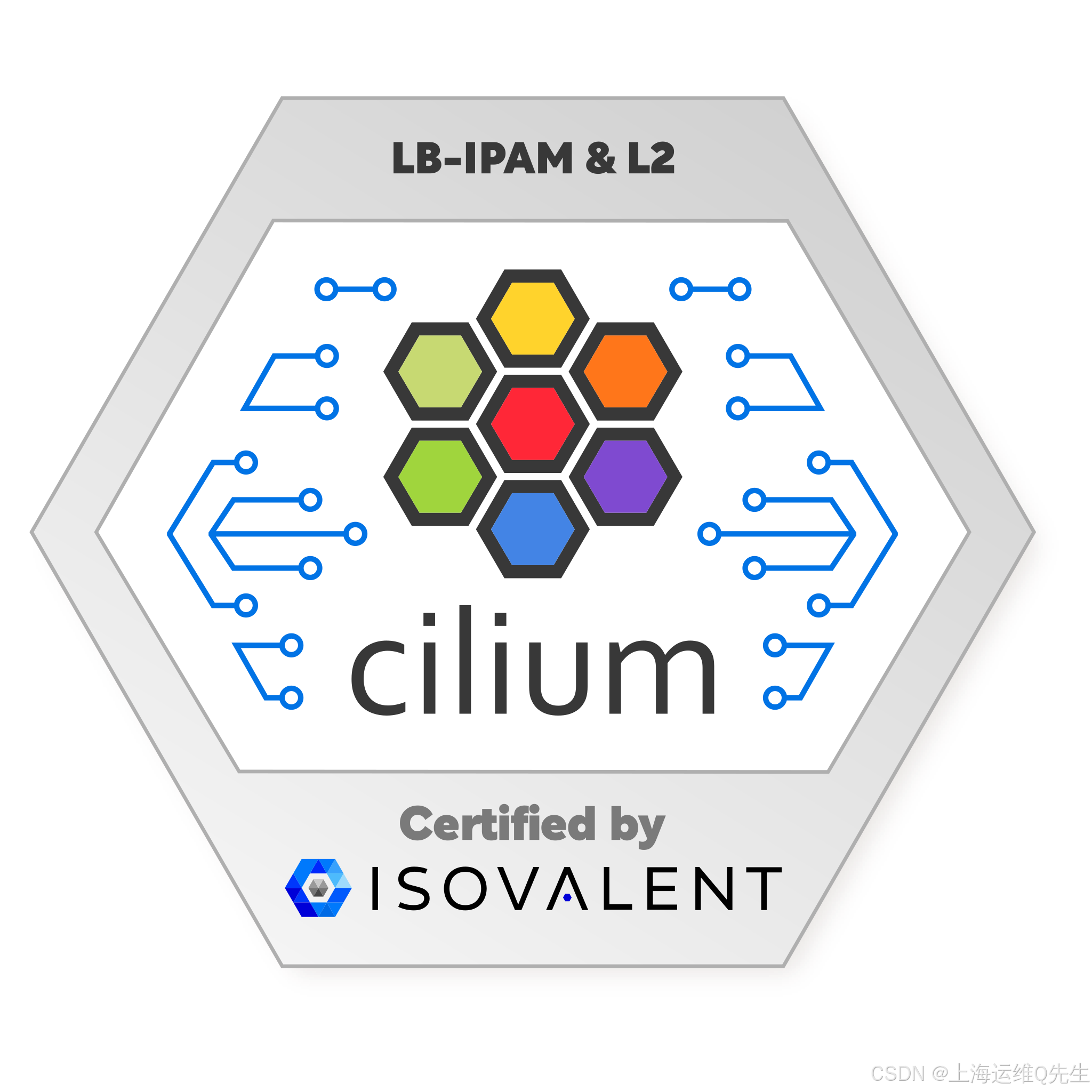
Cilium动手实验室: 精通之旅---13.Cilium LoadBalancer IPAM and L2 Service Announcement
Cilium动手实验室: 精通之旅---13.Cilium LoadBalancer IPAM and L2 Service Announcement 1. LAB环境2. L2公告策略2.1 部署Death Star2.2 访问服务2.3 部署L2公告策略2.4 服务宣告 3. 可视化 ARP 流量3.1 部署新服务3.2 准备可视化3.3 再次请求 4. 自动IPAM4.1 IPAM Pool4.2 …...
Docker拉取MySQL后数据库连接失败的解决方案
在使用Docker部署MySQL时,拉取并启动容器后,有时可能会遇到数据库连接失败的问题。这种问题可能由多种原因导致,包括配置错误、网络设置问题、权限问题等。本文将分析可能的原因,并提供解决方案。 一、确认MySQL容器的运行状态 …...
StarRocks 全面向量化执行引擎深度解析
StarRocks 全面向量化执行引擎深度解析 StarRocks 的向量化执行引擎是其高性能的核心设计,相比传统行式处理引擎(如MySQL),性能可提升 5-10倍。以下是分层拆解: 1. 向量化 vs 传统行式处理 维度行式处理向量化处理数…...
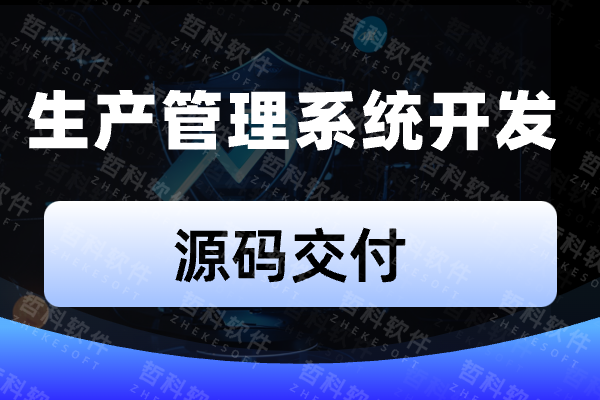
生产管理系统开发:专业软件开发公司的实践与思考
生产管理系统开发的关键点 在当前制造业智能化升级的转型背景下,生产管理系统开发正逐步成为企业优化生产流程的重要技术手段。不同行业、不同规模的企业在推进生产管理数字化转型过程中,面临的挑战存在显著差异。本文结合具体实践案例,分析…...
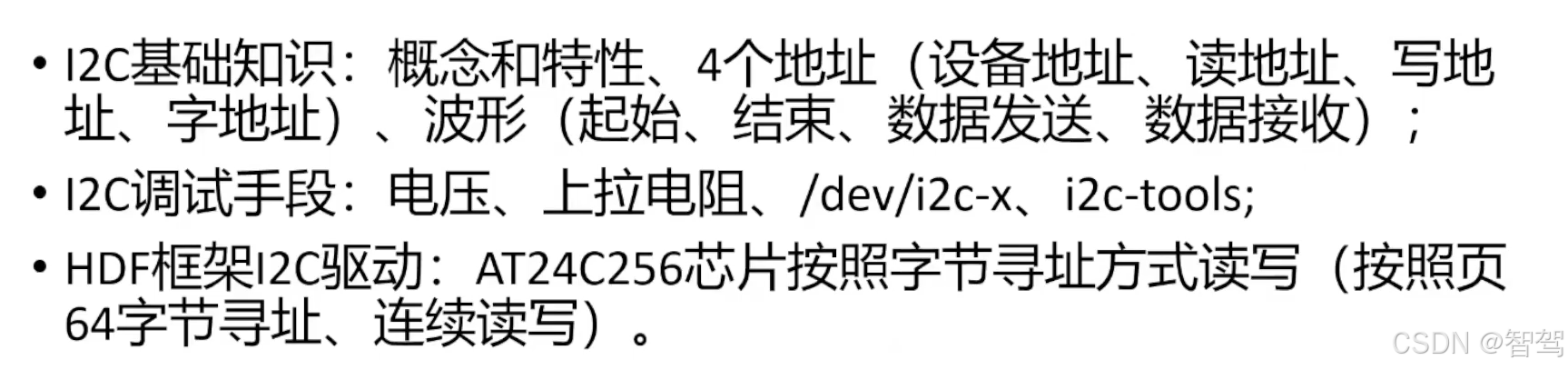
OpenHarmony标准系统-HDF框架之I2C驱动开发
文章目录 引言I2C基础知识概念和特性协议,四种信号组合 I2C调试手段硬件软件 HDF框架下的I2C设备驱动案例描述驱动Dispatch驱动读写 总结 引言 I2C基础知识 概念和特性 集成电路总线,由串网12C(1C、12C、Inter-Integrated Circuit BUS)行数据线SDA和串…...
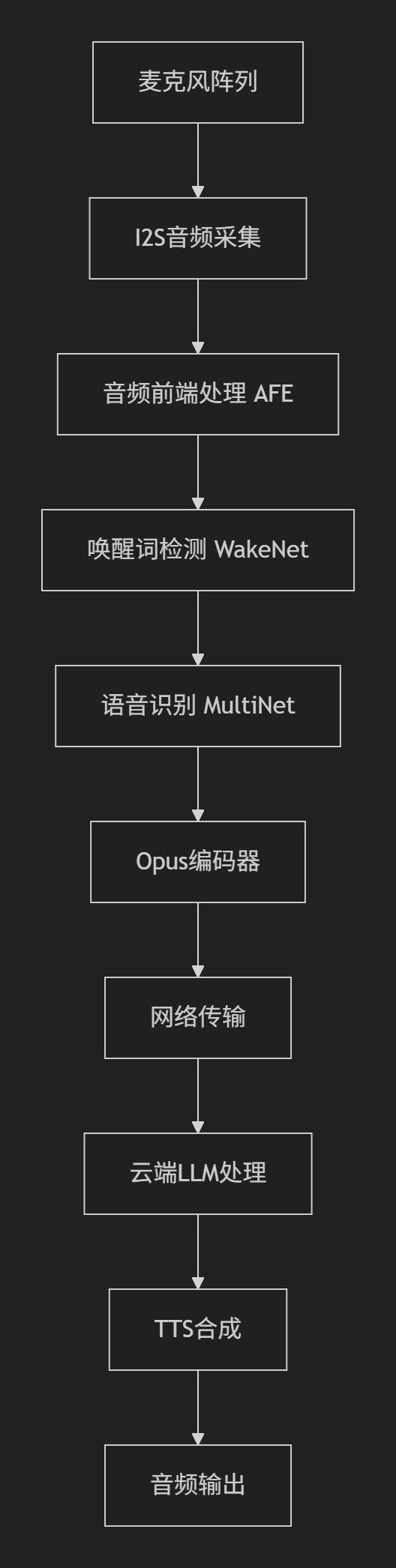
SDU棋界精灵——硬件程序ESP32实现opus编码
一、 音频处理框架 该项目基于Espressif的音频处理框架构建,核心组件包括 ESP-ADF 和 ESP-SR,以下是完整的音频处理框架实现细节: 1.核心组件 (1) 音频前端处理 (AFE - Audio Front-End) main/components/audio_pipeline/afe_processor.c功能: 声学回声…...