06 使用v-model实现双向数据绑定
概述
Vue achieves two-way data binding by creating a dedicated directive that watches a data property within your Vue component. The v-model directive triggers data updates when the target data property is modified on the UI.
Vue 通过创建一个专用指令来观察 Vue 组件中的数据属性,从而实现双向数据绑定。当用户界面上的目标数据属性被修改时,v-model 指令就会触发数据更新。
This directive is usually useful for HTML form elements that need to both display the data and modify it reactively – for example, input, textarea, radio buttons, and so on.
该指令通常适用于既需要显示数据又需要对数据进行反应式修改的 HTML 表单元素,例如输入、文本区域、单选按钮等。
We can enable two-way binding by adding the v-model directive to the target element and binding it to our desired data props:
我们可以在目标元素中添加 v-model 指令,并将其绑定到所需的数据道具,从而启用双向绑定:
<template><input v-model="name"/>
</template>
<script>
export default {data() {return {name: ''}}
}
</script>
In the next exercise, we are going to build a component using Vue’s twoway data binding and experiment with what it means to bind data in two ways.
在下一个练习中,我们将使用 Vue 的双向数据绑定构建一个组件,并尝试以两种方式绑定数据的含义。
练习:双向数据绑定
The context for this type of data model is usually forms or wherever you expect both input and output data. By the end of the exercise, we should be able to utilize the v-model attribute in the context of a form.
这类数据模型的上下文通常是表单或任何需要输入和输出数据的地方。在练习结束时,我们应该能够在表单中使用 v-model 属性。
Create a new Vue component file named Exercise1-04.vue in the src/components directory.
在 src/components 目录中新建一个名为 Exercise1-04.vue 的 Vue 组件文件。
在App.vue中引入该组件并渲染:
<script setup>
import Exercise from "./components/Exercise1-04.vue";
</script>
<template><Exercise/>
</template>
Inside Exercise1-04.vue, start by composing an HTML label and bind an input element to the name data prop using v-model inside the template area:
在 Exercise1-04.vue 中,首先创建一个 HTML 标签,然后在模板区域内使用 v-model 将输入元素与名称数据道具绑定:
<div class="form">
<label>Name<input type="text" v-model="name"/>
</label>
</div>
Complete the binding of the text input by returning a reactive data prop called name in the <script>
tag:
在 <script>
标记中返回名为 name 的反应式数据道具,从而完成文本输入的绑定:
<script>
export default {data() {return {name: '',}},
}
</script>
Next, compose a label and selectable HTML select tied to the language data prop using v-model inside of the template area:
接下来,在模板区域内使用 v 模型编写与语言数据道具绑定的标签和可选 HTML 选项:
<template><div class="form"><label>Name<input type="text" v-model="name"/></label><label>Preferred JavaScript style<select name="language" v-model="language"><option value="Javascript">JavaScript</option><option value="TypeScript">TypeScript</option><option value="CoffeeScript">CoffeeScript</option><option value="Dart">Dart</option></select></label></div>
</template>
Finish binding the select input by returning a reactive data prop called language in the <script>
tag:
在 <script>
标记中返回名为 language 的反应式数据道具,完成选择输入的绑定:
<script>
export default {data() {return {name: '',language: '',}},
}
</script>
Below the form fields, output the name and language inside of an unordered list structure (<ul>
and <li>
) by using curly braces such as {{ name }}. Your code should look as follows:
在表单字段下方,使用大括号(如 {{ name }})在无序列表结构(<ul>
和 <li>
)中输出名称和语言。您的代码应如下所示:
<template><section><div class="form"><label>Name<input type="text" v-model="name"/></label><label>Preferred JavaScript style<select name="language" v-model="language"><option value="JavaScript">JavaScript</option><option value="TypeScript">TypeScript</option><option value="CoffeeScript">CoffeeScript</option><option value="Dart">Dart</option></select></label></div><ul class="overview"><li><strong>Overview</strong></li><li>Name: {{ name }}</li><li>Preference: {{ language }}</li></ul></section>
</template>
Add styling inside the <style>
tag at the bottom of the component:
在组件底部的"<style>
"标签内添加样式:
<style>
.form {display: flex;justify-content: space-evenly;max-width: 800px;padding: 40px 20px;border-radius: 10px;margin: 0 auto;background: #ececec;
}.overview {display: flex;flex-direction: column;justify-content: space-evenly;max-width: 300px;margin: 40px auto;padding: 40px 20px;border-radius: 10px;border: 1px solid #ececec;
}.overview > li {list-style: none;
}.overview > li + li {margin-top: 20px;
}
</style>
When you update the data in the form, it should also update the overview area synchronously.
更新表单中的数据时,也应同步更新概览区域。
最终完整代码如下:
<template><section><div class="form"><label>Name<input type="text" v-model="name"/></label><label>Preferred JavaScript style<select name="language" v-model="language"><option value="JavaScript">JavaScript</option><option value="TypeScript">TypeScript</option><option value="CoffeeScript">CoffeeScript</option><option value="Dart">Dart</option></select></label></div><ul class="overview"><li><strong>Overview</strong></li><li>Name: {{ name }}</li><li>Preference: {{ language }}</li></ul></section>
</template>
<script>
export default {data() {return {name: '',language: '',}},
}
</script>
<style>
.form {display: flex;justify-content: space-evenly;max-width: 800px;padding: 40px 20px;border-radius: 10px;margin: 0 auto;background: #ececec;
}.overview {display: flex;flex-direction: column;justify-content: space-evenly;max-width: 300px;margin: 40px auto;padding: 40px 20px;border-radius: 10px;border: 1px solid #ececec;
}.overview > li {list-style: none;
}.overview > li + li {margin-top: 20px;
}
</style>
In this exercise, we used the v-model directive to bind the name and JavaScript-style drop-down selection to our local state’s data. When you modify the data, it will reactively update the DOM elements to which we output its value.
在本练习中,我们使用 v-model 指令将名称和 JavaScript 样式的下拉选择绑定到本地状态数据。当您修改数据时,它将反应式地更新我们输出其值的 DOM 元素。
Next, we will discuss our v-for directive further and different approaches to handling iterative data collection in Vue.
接下来,我们将进一步讨论 v-for 指令以及在 Vue 中处理迭代数据收集的不同方法。
相关文章:

06 使用v-model实现双向数据绑定
概述 Vue achieves two-way data binding by creating a dedicated directive that watches a data property within your Vue component. The v-model directive triggers data updates when the target data property is modified on the UI. Vue 通过创建一个专用指令来观…...
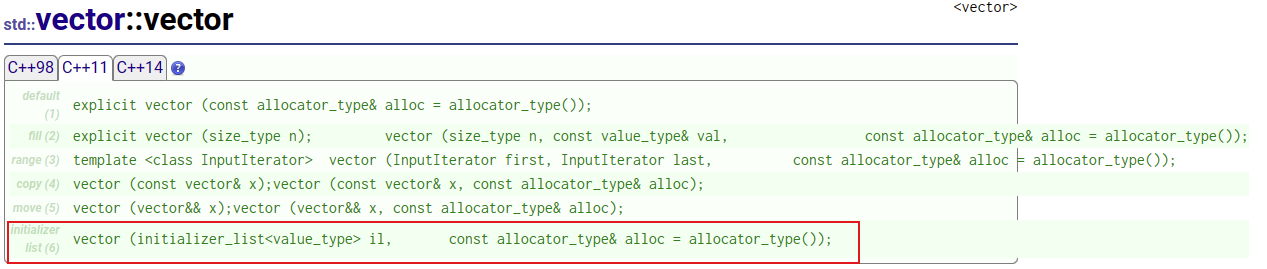
【C++11特性篇】C++11中新增的initializer_list——初始化的小利器(2)
前言 大家好吖,欢迎来到 YY 滴C11系列 ,热烈欢迎! 本章主要内容面向接触过C的老铁 主要内容含: 欢迎订阅 YY滴C专栏!更多干货持续更新!以下是传送门! 目录 一.探究std::initializer_list是什么…...
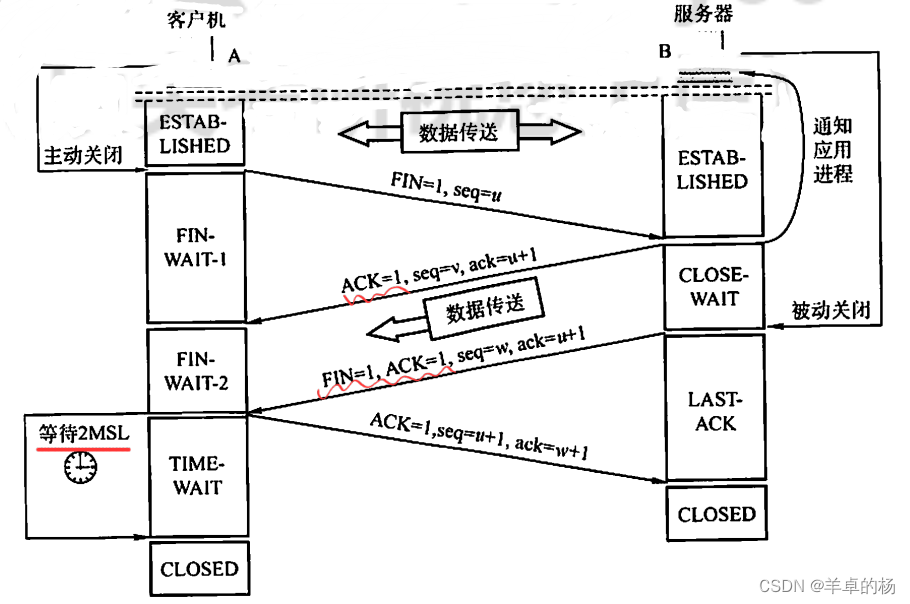
计算机网络传输层(期末、考研)
计算机网络总复习链接🔗 目录 传输层的功能端口UDP协议UDP数据报UDP的首部格式UDP校验 TCP协议(必考)TCP报文段TCP连接的建立TCP连接的释放TCP的可靠传输TCP的流量控制零窗口探测报文段 TCP的拥塞控制慢开始和拥塞控制快重传和快恢复 TCP和U…...
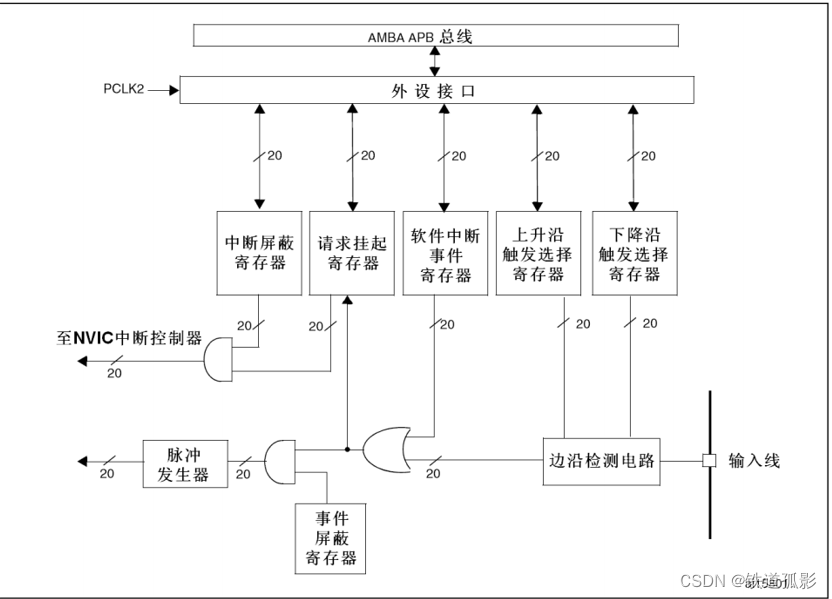
【STM32入门】4.1中断基本知识
1.中断概览 在开展红外传感器遮挡计次的实验之前,有必要系统性的了解“中断”的基本知识. 中断是指:在主程序运行过程中,出现了特定的中断触发条件(中断源),使得CPU暂停当前正在运行的程序,转…...
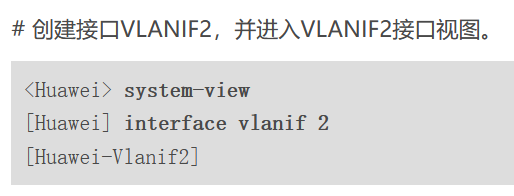
HCIA-H12-811题目解析(3)
1、【单选题】 以下关于路由器的描述,说法错误的是? 2、【单选题】某网络工程师在输入命令行时提示如下信息:Error:Unrecognized command foun at position.对于该提示信息说法正确的是? 3、【单选题】如下图所示的网络…...

【异步绘制】UIView刷新原理 与 异步绘制
快捷目录 壹、 iOS界面刷新机制贰、浅谈UIView的刷新与绘制概述一.UIView 与 CALayer1. UIView 与 CALayer的关系2. CALayer的一些常用属性contents属性contentGravity属性contentsScale属性maskToBounds属性contentsRect属性 二.View的布局与显示1.图像显示原理2.布局layoutSu…...

[ERROR] ocp-server-ce-py_script_start_check-4.2.1 RuntimeError: ‘tenant_name‘
Oceanbase 安装成功后关闭OCP,在重新启动时报错 使用OBD 启动OCP报如下错误 [adminobd ~]$ obd cluster start ocp Get local repositories ok Search plugins ok Open ssh connection ok Load cluster param plugin ok Check before start ocp-server x [ERROR] …...
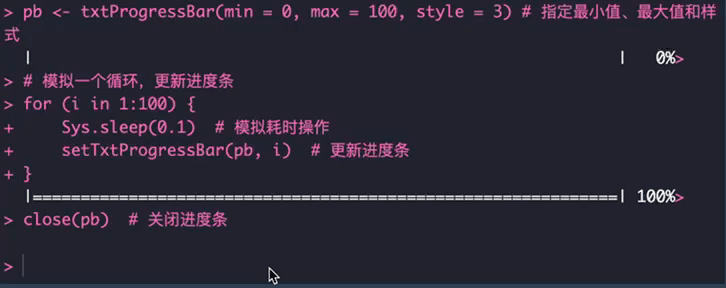
模拟实验中经常遇到的问题和常用技巧
简介 最近在进行新文章的数值模拟阶段。上一次已经跟读者们分享了模拟实验的大致流程,见:数值模拟流程记录和分享 。 本文是在前提下,汇总了小编在模拟实验中经常遇到的问题和常用技巧。 文章目录 简介1. 隐藏输出结果自动创建文件夹保存多…...

微信小程序(二) ——模版语法1
文章目录 wxml模板语法拼接字符数据绑定 wxml模板语法 拼接字符 <image src"{{test1src}}" mode""/>数据绑定 在data中定义数据,吧数据定义到data对象中在wxml中使用数据不论是绑定内容还是属性都是用 {{}} 语法 动态绑定内容 *声明…...
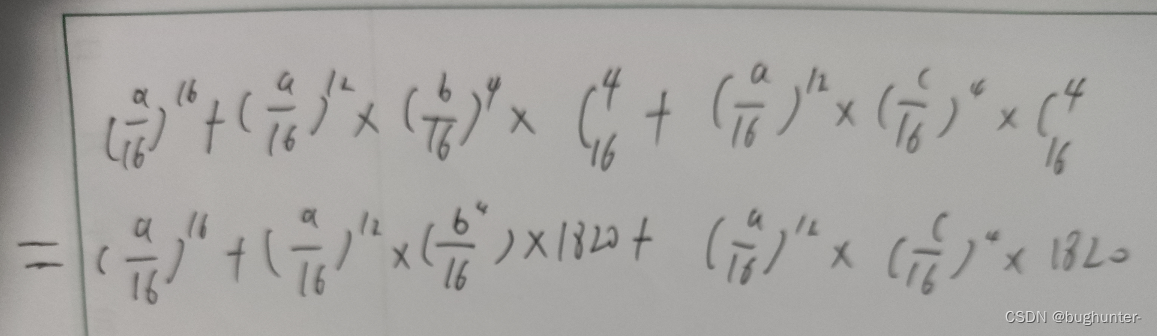
牛客小白月赛83 解题报告
题目链接: https://ac.nowcoder.com/acm/contest/72041#question A题 解题思路 签到 代码 #include <bits/stdc.h> using namespace std;int main() {int a, b, c, d, e;cin >> a >> b >> c >> d >> e;int A, B, C, D…...
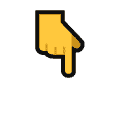
蓝桥杯专题-真题版含答案-【三角螺旋阵】【干支记年法】【异或加密法】【金字塔】
Unity3D特效百例案例项目实战源码Android-Unity实战问题汇总游戏脚本-辅助自动化Android控件全解手册再战Android系列Scratch编程案例软考全系列Unity3D学习专栏蓝桥系列ChatGPT和AIGC 👉关于作者 专注于Android/Unity和各种游戏开发技巧,以及各种资源分…...

鸿蒙篇——初次使用鸿蒙原生编译器DevEcoStudio创建一个鸿蒙原生应用遇到的坑--汇总(持续更新)
前言:欢迎各位鸿蒙初学者、开发者来本帖交流讨论,包含各位遇到的问题、鸿蒙的bug、解决方法等等,我会收集有效的内容更新到本文章中。 背景:2023年12月13日,使用DevEcoStudio 4.0.0.600版本,项目的compileS…...

细胞培养之一二三:哺乳动物细胞培养污染问题和解决方案
一、哺乳动物细胞污染是什么[1]? 污染通常是指在细胞培养基中存在不需要的微生物、不需要的哺乳动物细胞和各种生化或化学物质,从而影响所需哺乳动物细胞的生理和生长。由于微生物在包括人体特定部位在内的环境中无处不在,而且它们的繁殖速度…...

《Linux C编程实战》笔记:文件属性操作函数
获取文件属性 stat函数 在shell下直接使用ls就可以获得文件属性,但是在程序里应该怎么获得呢? #include<sys/types.h> #include <sys/stat.h> #include <unistd.h> int stat(const char *file_name,struct stat *buf); int fstat(i…...

linux中的网络知识
网络 认识基本网络网络划分计算机网络分为LAN、MAN、WAN公网ip和私网ip 传输介质单位换算客户端和服务端 OSI模型osi七层模型TCP/IP:传输控制协议簇HTTP协议简介UDP协议介绍物理地址:mac地址,全球唯一,mac由6段16进制数组成,每段有…...

tp中的调试模式
ThinkPHP有专门为开发过程而设置的调试模式,开启调试模式后,会牺牲一定的执行效率,但带来的方便和除错功能非常值得。 我们强烈建议ThinkPHP开发人员在开发阶段始终开启调试模式(直到正式部署后关闭调试模式)…...
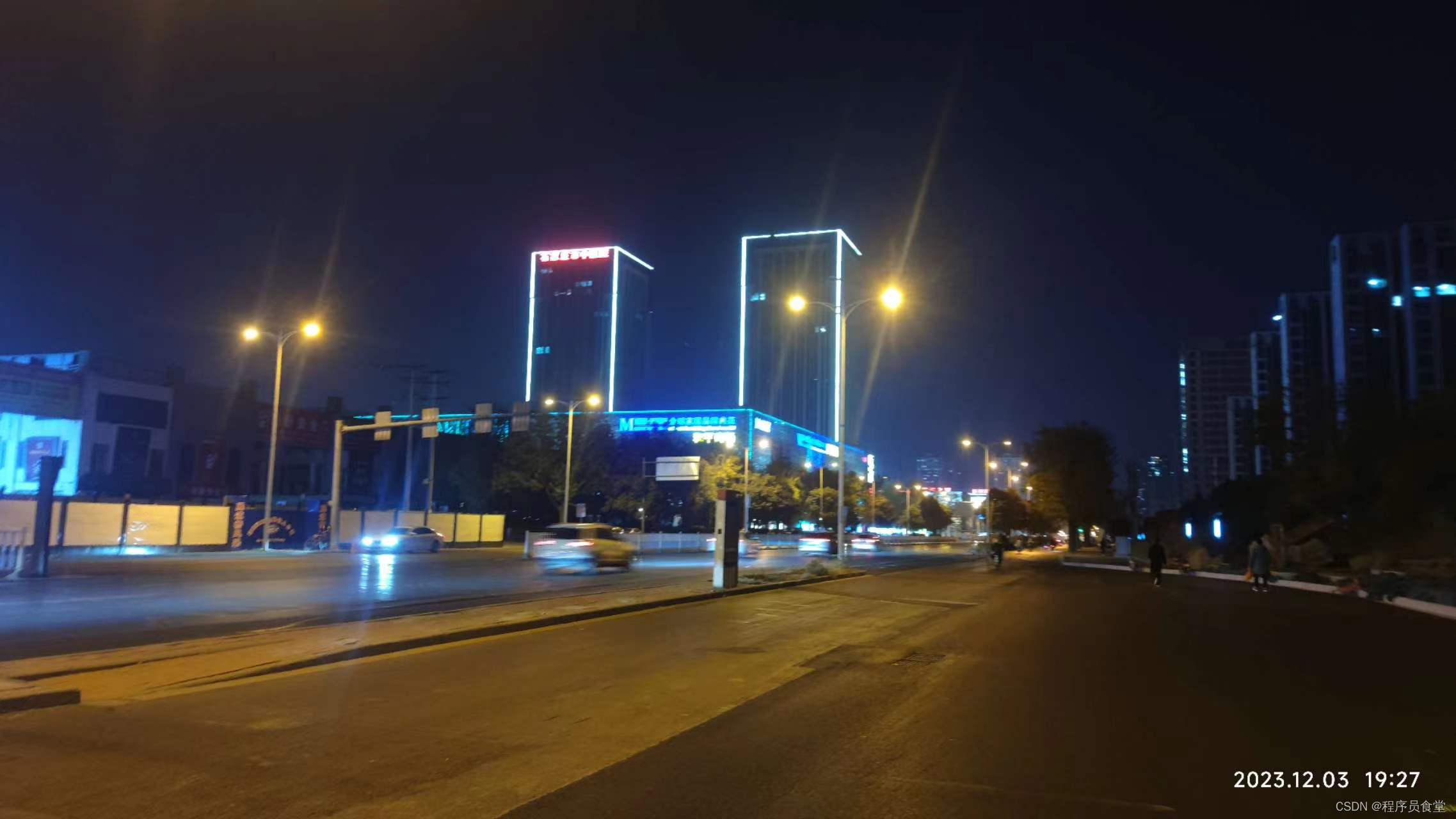
【docker 】基于Dockerfile创建镜像
Dockerfile文档 Dockerfile文档地址 Dockerfile 是一个用来构建镜像的文本文件,文本内容包含了一条条构建镜像所需的指令和说明。 DockerFile 可以说是一种可以被 Docker 程序解释的脚本,DockerFile 是由一条条的命令组成的,每条命令对应 …...
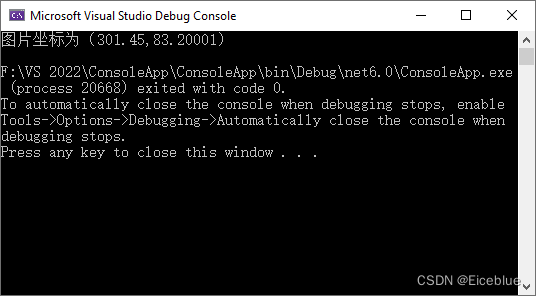
C# 提取PDF中指定文本、图片的坐标
获取PDF文件中文字或图片的坐标可以实现精确定位,这对于快速提取指定区域的元素,以及在PDF中添加注释、标记或自动盖章等操作非常有用。本文将详解如何使用国产PDF库通过C# 提取PDF中指定文本或图片的坐标位置(X, Y轴)。 ✍ 用于…...
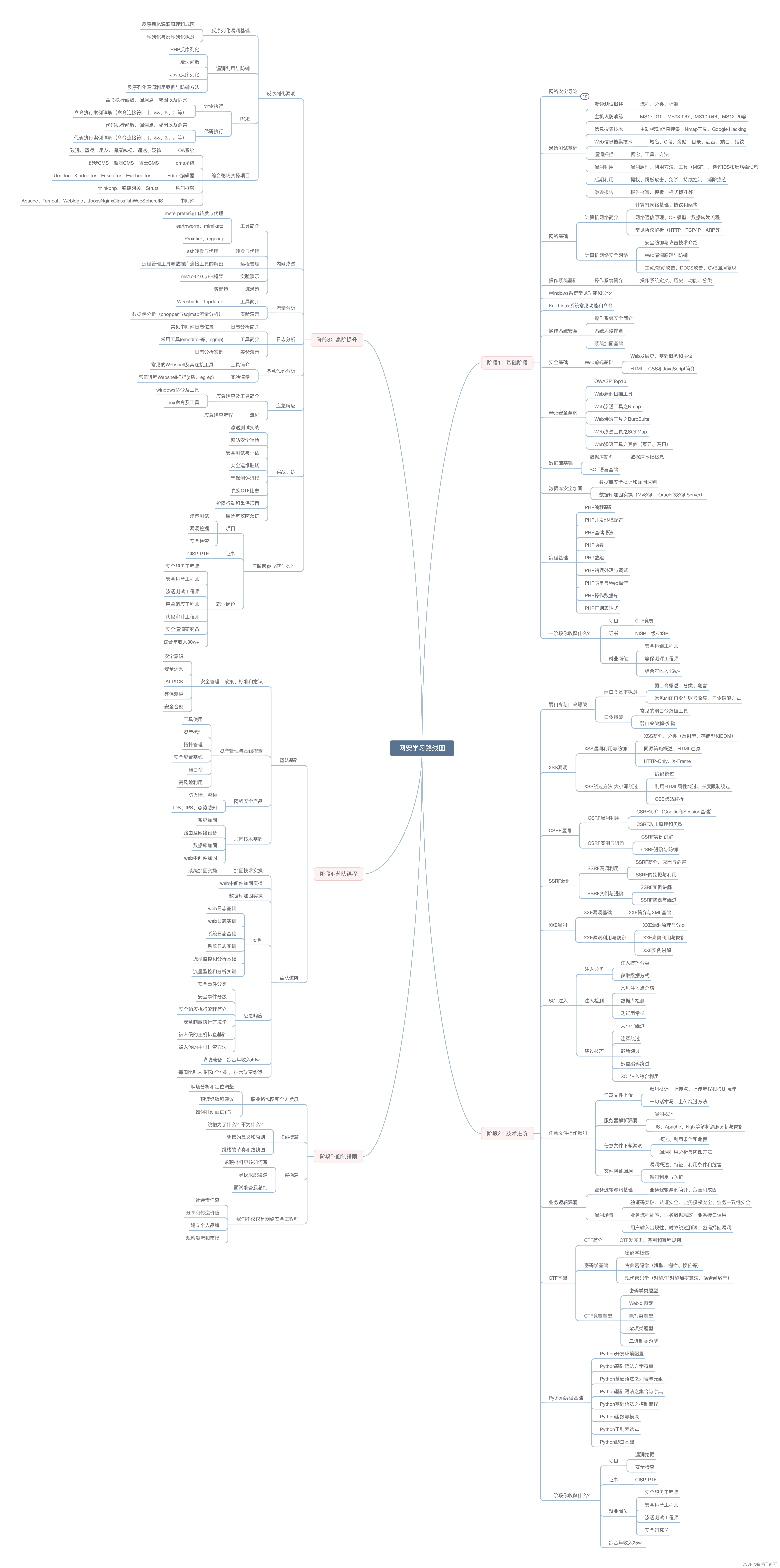
CTF网络安全大赛是干什么的?发展史、赛制、赛程介绍,参赛需要学什么?
CTF(Capture The Flag)是一种网络安全竞赛,它模拟了各种信息安全场景,旨在提升参与者的网络安全技能。CTF 赛事通常包含多种类型的挑战,如密码学、逆向工程、网络攻防、Web 安全、二进制利用等。 发展史 CTF 的概念…...

阿里云SMC迁移RedHat/CentOS 5 内核升级
阿里云SMC迁移RedHat/CentOS 5 内核升级 1. 起因 服务器需要迁移上阿里云,有几台服务器用的是Redhat 5.x,在使用SMC进行迁移时出现以下报错. [2023-12-13 09:50:55] [Error] Check System Info Failed, codeS16_111, msgGet OS Info Failed: [error] grub is too old for C…...
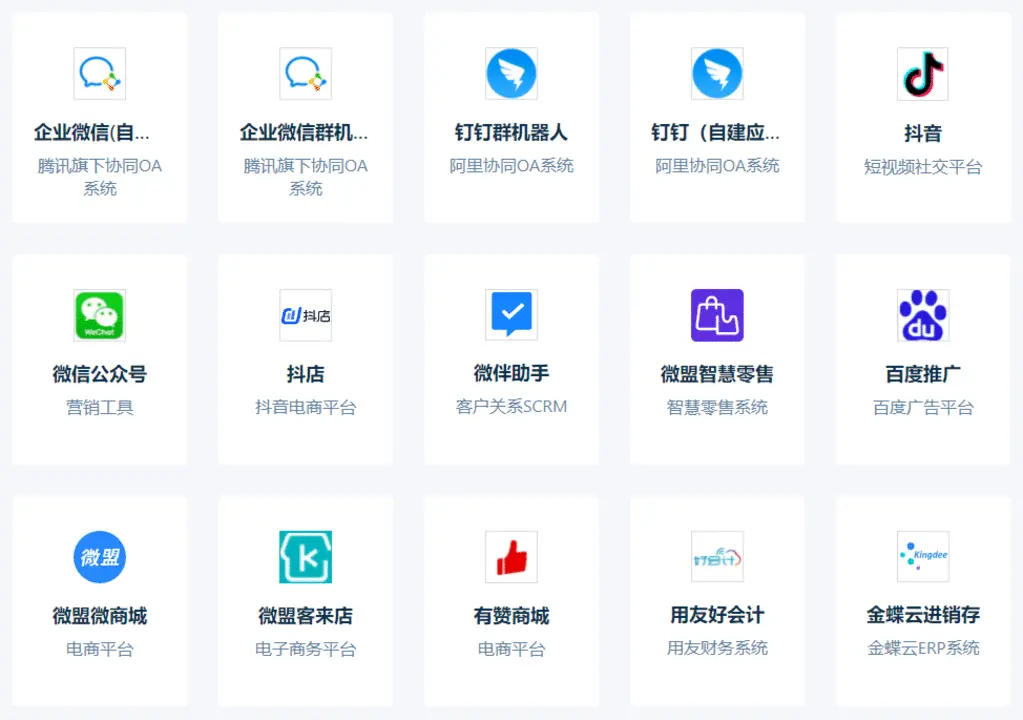
无代码开发让合利宝支付与CRM无缝API集成,提升电商用户运营效率
合利宝支付API的高效集成 在当今快速发展的电子商务领域,电商平台正寻求通过高效的支付系统集成来提升用户体验和业务处理效率。合利宝支付,作为中国领先的支付解决方案提供者,为电商平台提供了一个高效的API连接方案。这种方案允许无代码开…...
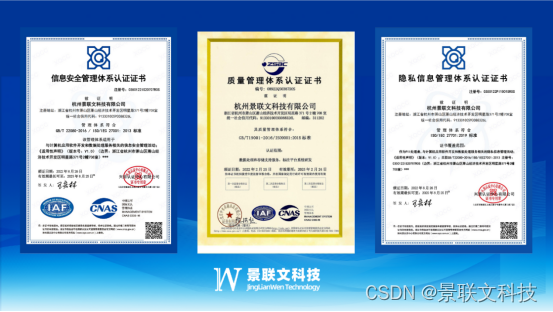
数据标注公司如何确保数据安全?景联文科技多维度提供保障
数据标注公司通常拥有大量的AI数据和用户数据,保护数据安全是数据标注公司的重要任务。 数据标注公司确保标注数据的安全可以从制度、人员、工具等多个方面入手,建立完善的安全管理体系和审计机制,加强应急预案和备份机制的建立,以…...

(C语言)精确计算程序运行时间的方法
一、先计算每秒多少个计数 typedef __int64 s64;s64 tps; /* timestamp counter per second */s64 get_tps(void) {s64 t0 rdtsc();Sleep(100);return (rdtsc() - t0) * 10; } 这段代码定义了一个函数 get_tps,该函数用于测量处理器的时间戳计数器(RD…...
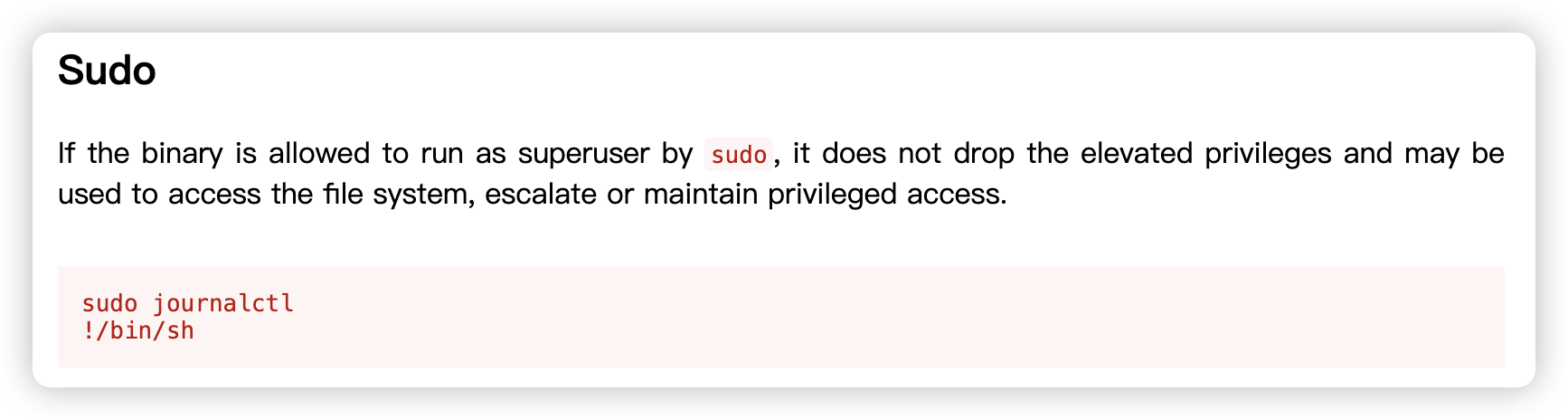
【Vulnhub 靶场】【VulnCMS: 1】【简单】【20210613】
1、环境介绍 靶场介绍:https://www.vulnhub.com/entry/vulncms-1,710/ 靶场下载:https://download.vulnhub.com/vulncms/VulnCMS.ova 靶场难度:简单 发布日期:2021年06月13日 文件大小:1.4 GB 靶场作者:to…...
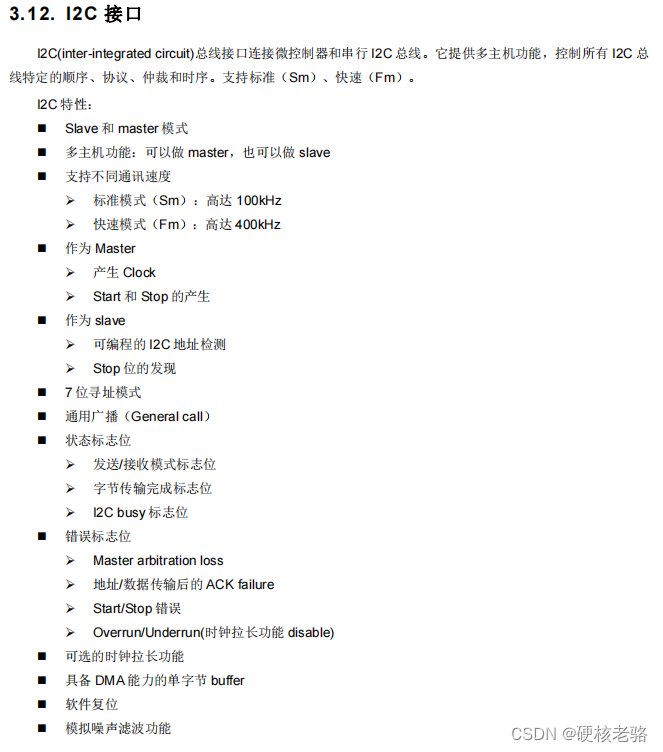
普冉(PUYA)单片机开发笔记(10): I2C通信-配置从机
概述 I2C 常用在某些型号的传感器和 MCU 的连接,速率要求不高,距离很短,使用简便。 I2C的通信基础知识请参见《基础通信协议之 IIC详细讲解 - 知乎》。 PY32F003 可以复用出一个 I2C 接口(PA3:SCL,PA2&a…...
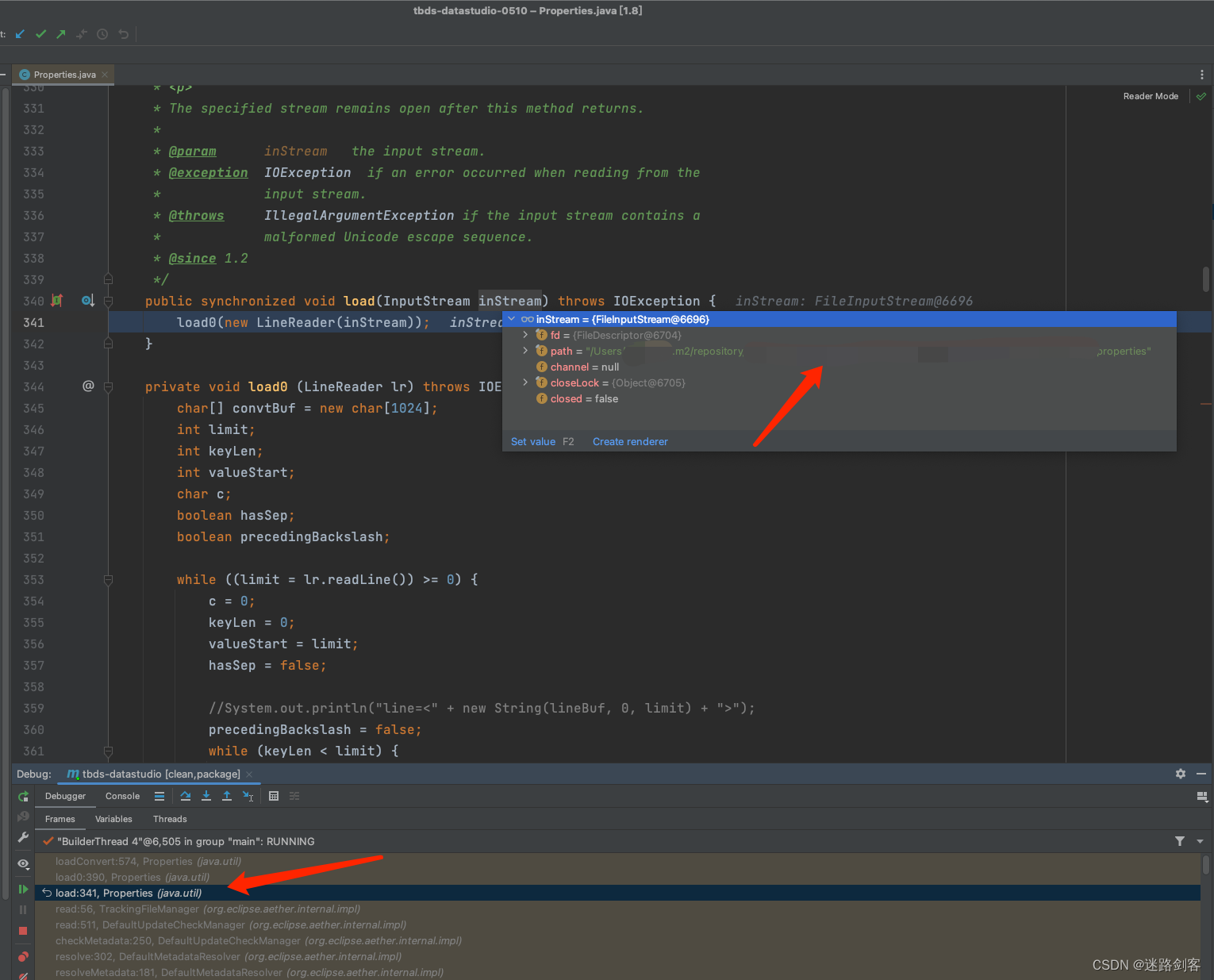
Idea maven打包时 报错 illegalArgumentException: Malformed \uxxxx encoding 解决方法
1 改变打包命令重新打包 在maven打包命令上加入 -e -X 2 找到报错类和方法 可以看到是 java.util.Properties#loadConvert类方法中有个throw new IllegalArgumentException( "Malformed \\uxxxx encoding."),在此打断点 3 以Debug方式重新运行maven…...

Qt中槽函数在那个线程执行的探索和思考
信号和槽是Qt的核心机制之一,通过该机制大大简化了开发者的开发难度。信号和槽属于观察者模式(本质上是回调函数的应用)。是函数就需要考虑其是在那个线程中执行,本文讨论的就是槽函数在那个线程中执行的问题。 目录 1. connect…...
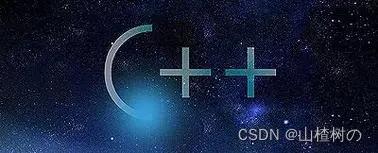
C++ 类模板
目录 前言 类模板语法 类模板和函数模板的区别 类模板没有自动类型推导的使用方式 类模板在模板参数列表中可以有默认参数 类模板中成员函数创建时机 类模板对象做函数参数 指定传入的类型 参数模板化 整个类模板化 类模板与继承 类模板成员函数类外实现 类模板分…...
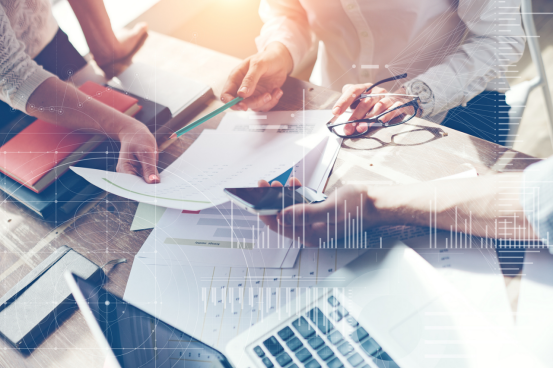
边缘计算系统设计与实践
随着科技的飞速发展,物联网和人工智能两大领域的不断突破,我们看到了一种新型的计算模型——边缘计算的崛起。这种计算模型在处理大规模数据、实现实时响应和降低延迟需求方面,展现出了巨大的潜力。本文将深入探讨边缘计算系统的设计原理和实…...
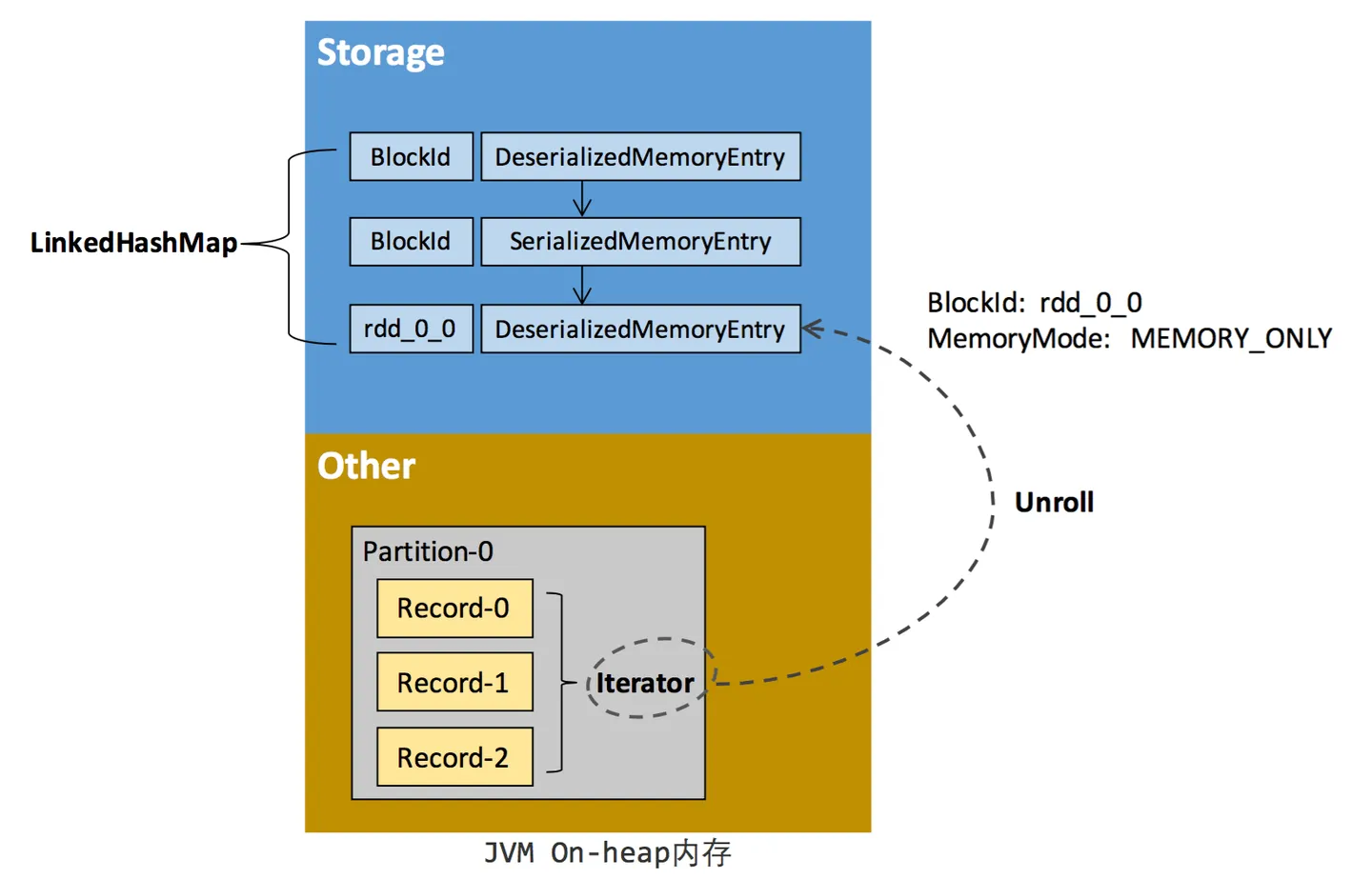
【Spark精讲】Spark存储原理
目录 类比HDFS的存储架构 Spark的存储架构 存储级别 RDD的持久化机制 RDD缓存的过程 Block淘汰和落盘 类比HDFS的存储架构 HDFS集群有两类节点以管理节点-工作节点模式运行,即一个NameNode(管理节点)和多个DataNode(工作节点)。 Namenode管理文件系统的命名空…...