PyTorch之Torch Script的简单使用
一、参考资料
TorchScript 简介
Torch Script
Loading a TorchScript Model in C++
TorchScript 解读(一):初识 TorchScript
libtorch教程(一)开发环境搭建:VS+libtorch和Qt+libtorch
二、Torch Script模型格式
1. Torch Script简介
Torch Script
是一种序列化和优化 PyTorch 模型的格式,在优化过程中,一个 torch.nn.Module
模型会被转换成 Torch Script 的 torch.jit.ScriptModule
模型。通常,TorchScript 被当成一种中间表示使用。
Torch Script 的主要用途是进行模型部署,需要记录生成一个便于推理优化的 IR,对计算图的编辑通常都是面向性能提升等等,不会给模型本身添加新的功能。
模型格式 | 支持语言 | 适用场景 |
---|---|---|
PyTorch model | Python | 模型训练 |
Torch Script | C++ | 模型推理,模型部署 |
2. 生成Torch Script模型
如何将PyTorch model格式转换为Torch Script,有两种方式: torch.jit.trace
、torch.jit.script
。
As its name suggests, the primary interface to PyTorch is the Python programming language. While Python is a suitable and preferred language for many scenarios requiring dynamism and ease of iteration, there are equally many situations where precisely these properties of Python are unfavorable. One environment in which the latter often applies is production – the land of low latencies and strict deployment requirements. For production scenarios, C++ is very often the language of choice, even if only to bind it into another language like Java, Rust or Go. The following paragraphs will outline the path PyTorch provides to go from an existing Python model to a serialized representation that can be loaded and executed purely from C++, with no dependency on Python.
A PyTorch model’s journey from Python to C++ is enabled by Torch Script, a representation of a PyTorch model that can be understood, compiled and serialized by the Torch Script compiler. If you are starting out from an existing PyTorch model written in the vanilla “eager” API, you must first convert your model to Torch Script.
There exist two ways of converting a PyTorch model to Torch Script. The first is known as tracing, a mechanism in which the structure of the model is captured by evaluating it once using example inputs, and recording the flow of those inputs through the model. This is suitable for models that make limited use of control flow. The second approach is to add explicit annotations to your model that inform the Torch Script compiler that it may directly parse and compile your model code, subject to the constraints imposed by the Torch Script language.
2.1 trace跟踪模式
Converting to Torch Script via Tracing
How to convert your PyTorch model to TorchScript
功能:将不带控制流的模型转换为
Torch Script
,并生成一个ScriptModule
对象。函数原型:
torch.jit.trace
所谓 trace 指的是进行一次模型推理,在推理的过程中记录所有经过的计算,将这些记录整合成计算图,即模型的静态图。
trace跟踪模式的缺点是:无法识别出模型中的控制流(如循环)。
To convert a PyTorch model to Torch Script via tracing, you must pass an instance of your model along with an example input to the
torch.jit.trace
function. This will produce atorch.jit.ScriptModule
object with the trace of your model evaluation embedded in the module’sforward
method.
import torch
import torchvision# An instance of your model.
model = torchvision.models.resnet18(pretrained=True)# Switch the model to eval model
model.eval()# An example input you would normally provide to your model's forward() method.
dummy_input = torch.rand(1, 3, 224, 224)# Use torch.jit.trace to generate a torch.jit.ScriptModule via tracing.
traced_script_module = torch.jit.trace(model, dummy_input)# Save the TorchScript model
traced_script_module.save("traced_resnet18_model.pt")output = traced_script_module(torch.ones(1, 3, 224, 224))# IR中间表示
print(traced_script_module.graph)
print(traced_script_module.code)# 调用traced_cell会产生与 Python 模块相同的结果
print(model(x, h))
print(traced_script_module(x, h))
2.2 script记录模式(带控制流)
功能:将带控制流的模型转换为
Torch Script
,并生成一个ScriptModule
对象。函数原型:
torch.jit.script
script记录模式,通过解析模型来正确记录所有的控制流。script记录模式直接解析网络定义的 python 代码,生成抽象语法树 AST。
Because the
forward
method of this module uses control flow that is dependent on the input, it is not suitable for tracing. Instead, we can convert it to aScriptModule
. In order to convert the module to theScriptModule
, one needs to compile the module withtorch.jit.script
as follows.
class MyModule(torch.nn.Module):def __init__(self, N, M):super(MyModule, self).__init__()self.weight = torch.nn.Parameter(torch.rand(N, M))def forward(self, input):if input.sum() > 0:output = self.weight.mv(input)else:output = self.weight + inputreturn outputmy_module = MyModule(10,20)
sm = torch.jit.script(my_module)# Save the ScriptModule
sm.save("my_module_model.pt")
2.3 trace格式转换
import torch
import torchvision
from unet import UNetmodel = UNet(3, 2)
model.load_state_dict(torch.load("best_weights.pth"))
model.eval()example = torch.rand(1, 3, 320, 480)
traced_script_module = torch.jit.trace(model, example)
traced_script_module.save("model.pt")
三、Loading a TorchScript Model in C++
1. C++加载 ScriptModule
创建一个简单的程序,目录结构如下所示:
example-app/CMakeLists.txtexample-app.cpp
1.1 example-app.cpp
#include <torch/script.h> // One-stop header.#include <iostream>
#include <memory>int main(int argc, const char* argv[]) {if (argc != 2) {std::cerr << "usage: example-app <path-to-exported-script-module>\n";return -1;}torch::jit::script::Module module;try {// Deserialize the ScriptModule from a file using torch::jit::load().module = torch::jit::load(argv[1]);}catch (const c10::Error& e) {std::cerr << "error loading the model\n";return -1;}std::cout << "ok\n";
}
The
<torch/script.h>
header encompasses all relevant includes from the LibTorch library necessary to run the example. Our application accepts the file path to a serialized PyTorchScriptModule
as its only command line argument and then proceeds to deserialize the module using thetorch::jit::load()
function, which takes this file path as input. In return we receive atorch::jit::script::Module
object.
1.2 CMakeLists.txt
cmake_minimum_required(VERSION 3.0 FATAL_ERROR)
project(custom_ops)find_package(Torch REQUIRED)add_executable(example-app example-app.cpp)
target_link_libraries(example-app "${TORCH_LIBRARIES}")
set_property(TARGET example-app PROPERTY CXX_STANDARD 14)
1.3 编译执行
mkdir build
cd build
cmake -DCMAKE_PREFIX_PATH=/path/to/libtorch ..
cmake --build . --config Release
make
输出结果
root@4b5a67132e81:/example-app# mkdir build
root@4b5a67132e81:/example-app# cd build
root@4b5a67132e81:/example-app/build# cmake -DCMAKE_PREFIX_PATH=/path/to/libtorch ..
-- The C compiler identification is GNU 5.4.0
-- The CXX compiler identification is GNU 5.4.0
-- Check for working C compiler: /usr/bin/cc
-- Check for working C compiler: /usr/bin/cc -- works
-- Detecting C compiler ABI info
-- Detecting C compiler ABI info - done
-- Detecting C compile features
-- Detecting C compile features - done
-- Check for working CXX compiler: /usr/bin/c++
-- Check for working CXX compiler: /usr/bin/c++ -- works
-- Detecting CXX compiler ABI info
-- Detecting CXX compiler ABI info - done
-- Detecting CXX compile features
-- Detecting CXX compile features - done
-- Looking for pthread.h
-- Looking for pthread.h - found
-- Looking for pthread_create
-- Looking for pthread_create - not found
-- Looking for pthread_create in pthreads
-- Looking for pthread_create in pthreads - not found
-- Looking for pthread_create in pthread
-- Looking for pthread_create in pthread - found
-- Found Threads: TRUE
-- Configuring done
-- Generating done
-- Build files have been written to: /example-app/build
root@4b5a67132e81:/example-app/build# make
Scanning dependencies of target example-app
[ 50%] Building CXX object CMakeFiles/example-app.dir/example-app.cpp.o
[100%] Linking CXX executable example-app
[100%] Built target example-app
1.4 执行结果
If we supply the path to the traced
ResNet18
modeltraced_resnet_model.pt
we created earlier to the resultingexample-app
binary, we should be rewarded with a friendly “ok”. Please note, if try to run this example withmy_module_model.pt
you will get an error saying that your input is of an incompatible shape.my_module_model.pt
expects 1D instead of 4D.
root@4b5a67132e81:/example-app/build# ./example-app <path_to_model>/traced_resnet_model.pt
ok
2. C++推理ScriptModule
在main()
函数中添加模型推理的代码:
// Create a vector of inputs.
std::vector<torch::jit::IValue> inputs;
inputs.push_back(torch::ones({1, 3, 224, 224}));// Execute the model and turn its output into a tensor.
at::Tensor output = module.forward(inputs).toTensor();
std::cout << output.slice(/*dim=*/1, /*start=*/0, /*end=*/5) << '\n';
编译执行
root@4b5a67132e81:/example-app/build# make
Scanning dependencies of target example-app
[ 50%] Building CXX object CMakeFiles/example-app.dir/example-app.cpp.o
[100%] Linking CXX executable example-app
[100%] Built target example-app
root@4b5a67132e81:/example-app/build# ./example-app traced_resnet_model.pt
-0.2698 -0.0381 0.4023 -0.3010 -0.0448
[ Variable[CPUFloatType]{1,5} ]
相关文章:

PyTorch之Torch Script的简单使用
一、参考资料 TorchScript 简介 Torch Script Loading a TorchScript Model in C TorchScript 解读(一):初识 TorchScript libtorch教程(一)开发环境搭建:VSlibtorch和Qtlibtorch 二、Torch Script模型格…...
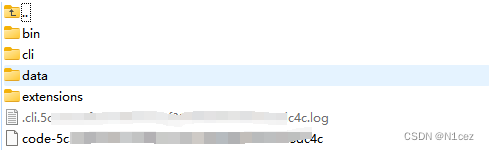
vscode 连接远程服务器 服务器无法上网 离线配置 .vscode-server
离线配置 vscode 连接远程服务器 .vscode-server 1. .vscode-server下载 使用vscode连接远程服务器时会自动下载配置.vscode-server文件夹,如果远程服务器无法联网,则需要手动下载 1)网址:https://update.code.visualstudio.com…...
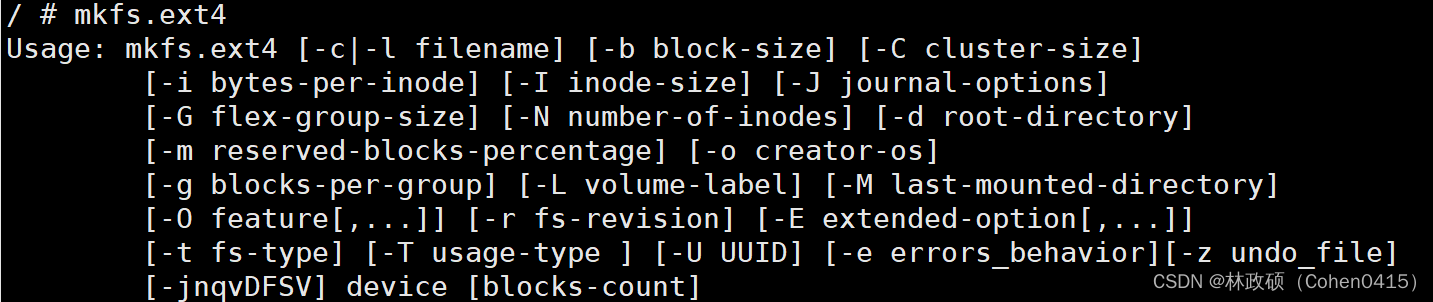
arm开发板移植工具mkfs.ext4
文章目录 一、前言二、手动安装e2fsprogs1、下载源码包2、解压源码3、配置4、编译5、安装 三、移植四、验证五、总结 一、前言 在buildroot菜单中,可以通过勾选e2fsprogs工具来安装mkfs.ext4工具: Target packages -> Filesystem and flash utilit…...
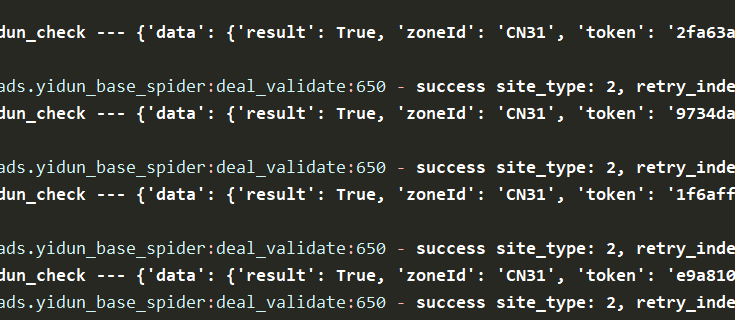
某盾滑块拼图验证码增强版
介绍 提示:文章仅供交流学习,严禁用于非法用途,如有不当可联系本人删除 最近某盾新推出了,滑块拼图验证码,如下图所示,这篇文章介绍怎么识别滑块距离相关。 参数attrs 通过GET请求获取的参数attrs, 决…...

这个世界万物存在只有一种关系:博弈
$上证指数(SH000001)$ 我能给各位最大的帮助可能就是第一个从红警游戏引入了情绪周期视角的概念,而这个概念可以帮助很多人理解市场成为一种可能性,如果不理解可以重新回归游戏进行反复体验,你体验的足够多,思考的足够多ÿ…...

c#让不同的工厂生产不同的“鸭肉”
任务目标 实现对周黑鸭工厂的产品生产统一管理,主要产品包括鸭脖和鸭翅。武汉工厂能生生产鸭脖和鸭翅,南京工厂只能生产鸭翅,长沙工厂只能生产鸭脖。 分析任务 我们需要有武汉工厂、南京工厂、长沙工厂的类,类中需要实现生产鸭…...
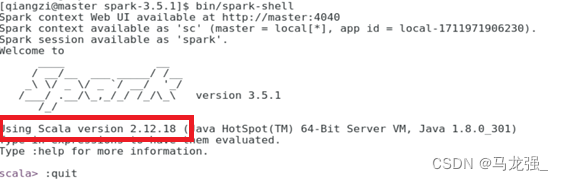
大数据分析与内存计算——Spark安装以及Hadoop操作——注意事项
一、Spark安装 1.相关链接 Spark安装和编程实践(Spark3.4.0)_厦大数据库实验室博客 (xmu.edu.cn) 2.安装Spark(Local模式) 按照文章中的步骤安装即可 遇到问题:xshell以及xftp不能使用 解决办法: 在…...
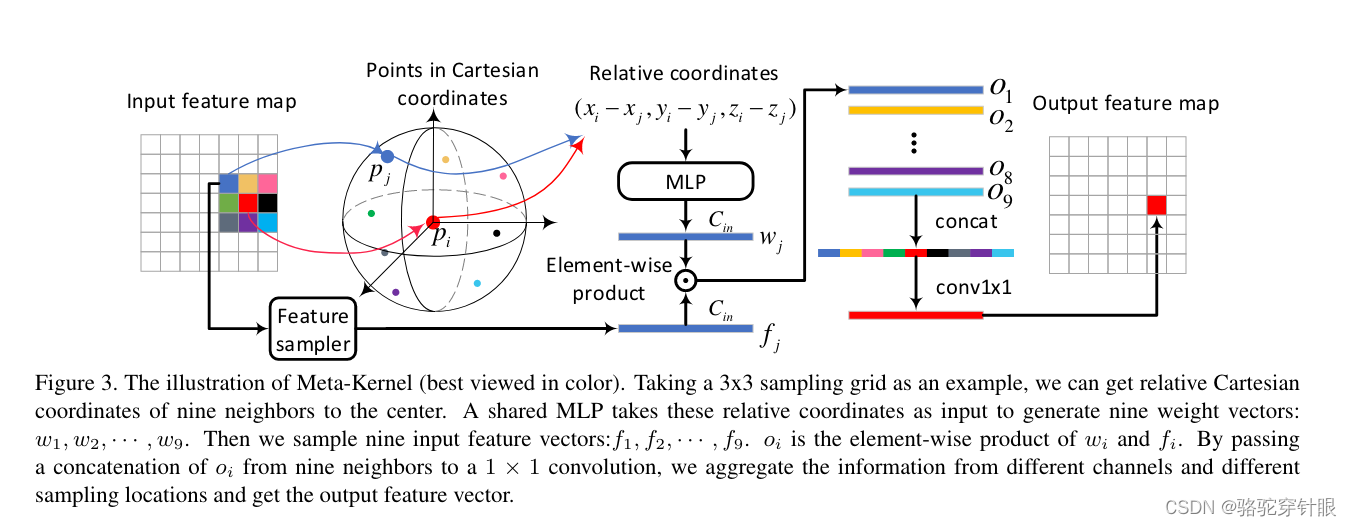
论文阅读RangeDet: In Defense of Range View for LiDAR-based 3D Object Detection
文章目录 RangeDet: In Defense of Range View for LiDAR-based 3D Object Detection问题笛卡尔坐标结构图Meta-Kernel Convolution RangeDet: In Defense of Range View for LiDAR-based 3D Object Detection 论文:https://arxiv.org/pdf/2103.10039.pdf 代码&…...

3D模型格式转换工具HOOPS Exchange如何将3D文件加载到PRC数据结构中?
HOOPS Exchange是一款高效的数据访问工具,专为开发人员设计,用于在不同的CAD(计算机辅助设计)系统之间进行高保真的数据转换和交换。由Tech Soft 3D公司开发,它支持广泛的CAD文件格式,包括但不限于AutoCAD的…...
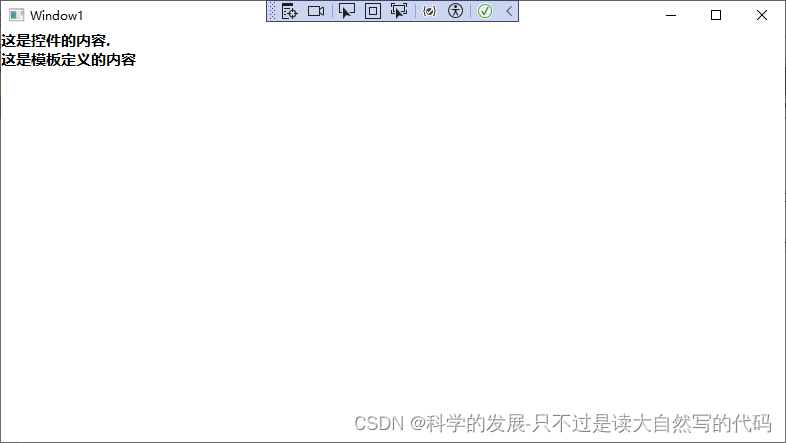
c# wpf Template ContentTemplate
1.概要 1.1 定义内容的外观 2.2 要点分析 2.代码 <Window x:Class"WpfApp2.Window1"xmlns"http://schemas.microsoft.com/winfx/2006/xaml/presentation"xmlns:x"http://schemas.microsoft.com/winfx/2006/xaml"xmlns:d"http://schem…...

空和null是两回事
文章目录 前言 StringUtils1. 空(empty):字符串:集合: 2. null:引用类型变量:基本类型变量: 3. isBlank总结: 前言 StringUtils 提示:这里可以添加本文要记录…...
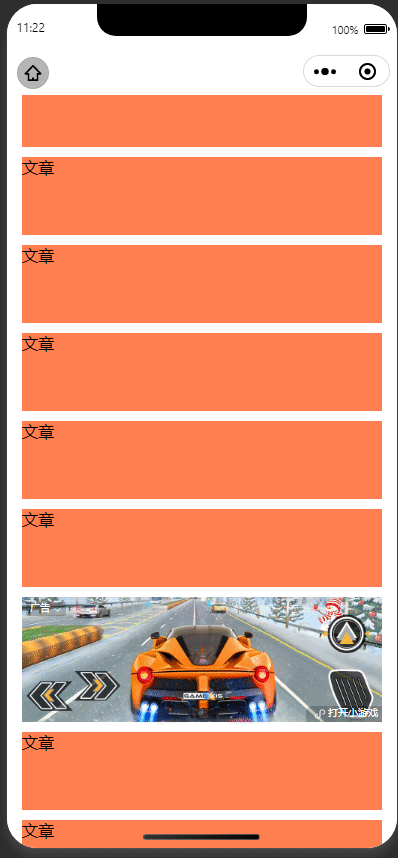
UNIAPP(小程序)每十个文章中间一个广告
三十秒刷新一次广告 ad-intervals"30" <template><view style"margin: 30rpx;"><view class"" v-for"(item,index) in 100"><!-- 广告 --><view style"margin-bottom: 20rpx;" v-if"(inde…...

pip包安装用国内镜像源
一:临时用国内源 可以在使用pip的时候加参数-i https://pypi.tuna.tsinghua.edu.cn/simple 例如:pip install -i https://pypi.tuna.tsinghua.edu.cn/simple pyspider,这样就会从清华这边的镜像去安装pyspider库 清华:https://py…...

uniapp:小程序腾讯地图程序文件qqmap-wx-jssdk.js 文件一直找不到无法导入
先看问题: 在使用腾讯地图api时无法导入到qqmap-wx-jssdk.js文件 解决方法:1、打开qqmap-wx-jssdk.js最后一行 然后导入:这里是我的路径位置,可以根据自己的路径位置进行更改导入 最后在生命周期函数中输出: 运行效果…...

如何物理控制另一台电脑以及无网络用作副屏(现成设备和使用)
初级代码游戏的专栏介绍与文章目录-CSDN博客 我的github:codetoys,所有代码都将会位于ctfc库中。已经放入库中我会指出在库中的位置。 这些代码大部分以Linux为目标但部分代码是纯C的,可以在任何平台上使用。 控制另一台电脑有很多方法&…...
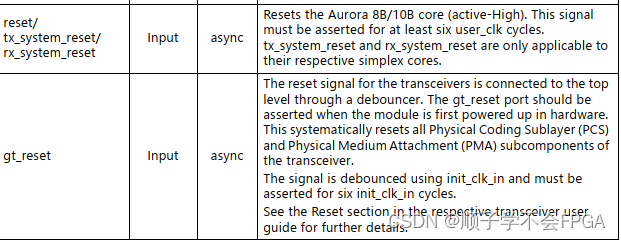
Aurora8b10b(1)IP核介绍并基于IP核进行设计
文章目录 前言一、IP核设置二、基于IP核进行设计2.1、设计框图2.2、aurora_8b10b_0模块2.3、aurora_8b10b_0_CLOCK_MODULE2.4、aurora_8b10b_0_SUPPORT_RESET_LOGIC2.5、aurora8b10b_channel模块2.6、IBUFDS_GTE2模块2.7、aurora_8b10b_0_gt_common_wrapper模块2.8、aurora8b10…...
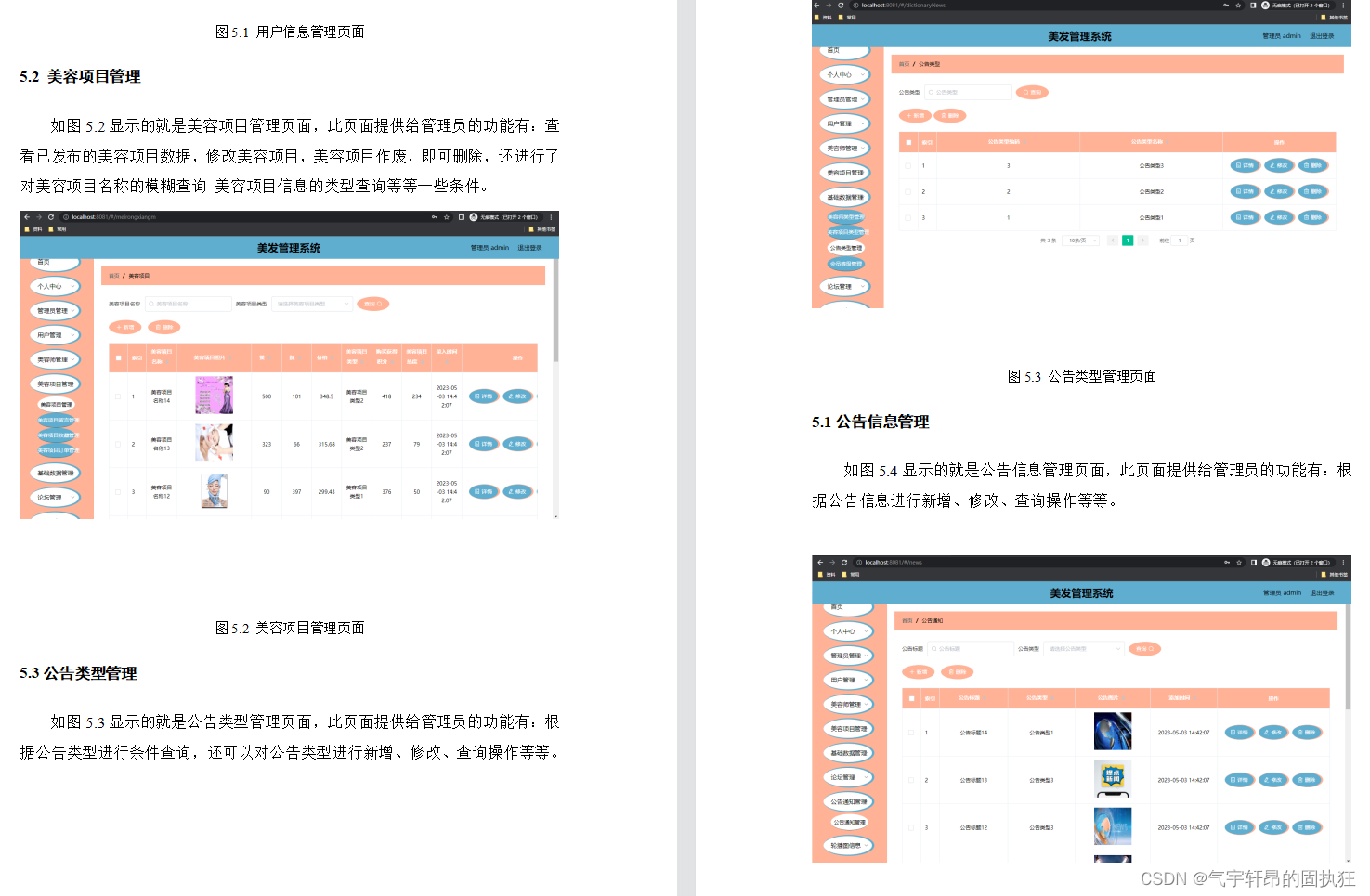
基于Springboot的美发管理系统(有报告)。Javaee项目,springboot项目。
演示视频: 基于Springboot的美发管理系统(有报告)。Javaee项目,springboot项目。 项目介绍: 采用M(model)V(view)C(controller)三层体系结构&…...

最新测试技术
在软件测试领域,随着技术的不断进步和行业需求的变化,新的测试技术和方法不断涌现。以下是一些最新的测试技术,它们正在塑造着软件测试的未来: 人工智能和机器学习(AI/ML)在测试中的应用 人工智能和机器学习正在被集成到软件测试中,以提高测试的自动化水平和效率。AI可…...

【算法】初识算法
尽量不说废话 算法 一、数据结构二、排序算法三、检索算法四、字符算类型算法五、递归算法六、贪心算法七、动态规划八、归一化算法后记 我们这里指的算法,是作为程序员在计算机编程时运用到的算法。 算法是一个庞大的体系,主要包括以下内容:…...

HomeBrew 安装与应用
目录 前言一、安装 HomeBrew二、使用 HomeBrew1、使用 brew 查看已安装的软件包2、使用 brew 安装软件包3、使用 brew 升级已安装的软件包4、brew 还有哪些命令呢? 前言 在 macOS(或Linux)系统里,默认是没有软件包的管理器的&…...
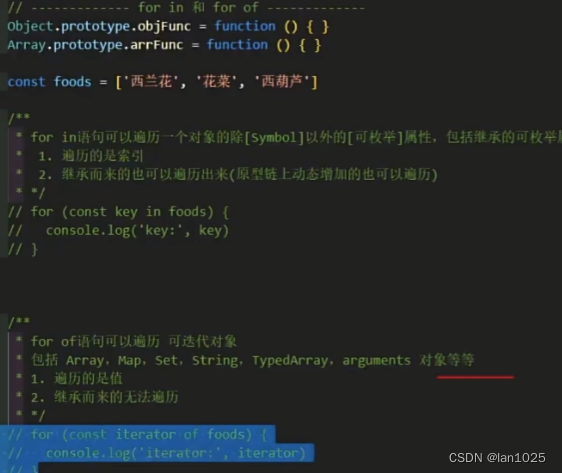
JS详解-设计模式
工厂模式: 单例模式: // 1、定义一个类class SingleTon{// 2、添加私有静态属性static #instance// 3、添加静态方法static getInstance(){// 4、判断实例是否存在if(!this.#instance){// 5、实例不存在,创建实例this.#instance new Single…...
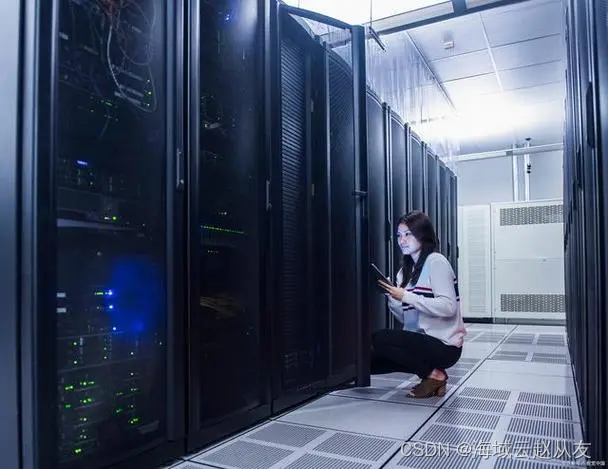
探寻马来西亚服务器托管的优势与魅力
随着全球跨境业务的不断增加,境外服务器成为越来越受欢迎的选择。在这其中,马来西亚服务器备受关注,其机房通常位于马来西亚首都吉隆坡。对于客户群体主要分布在东南亚、澳大利亚和新西兰等地区的用户来说,马来西亚服务器是一个理…...
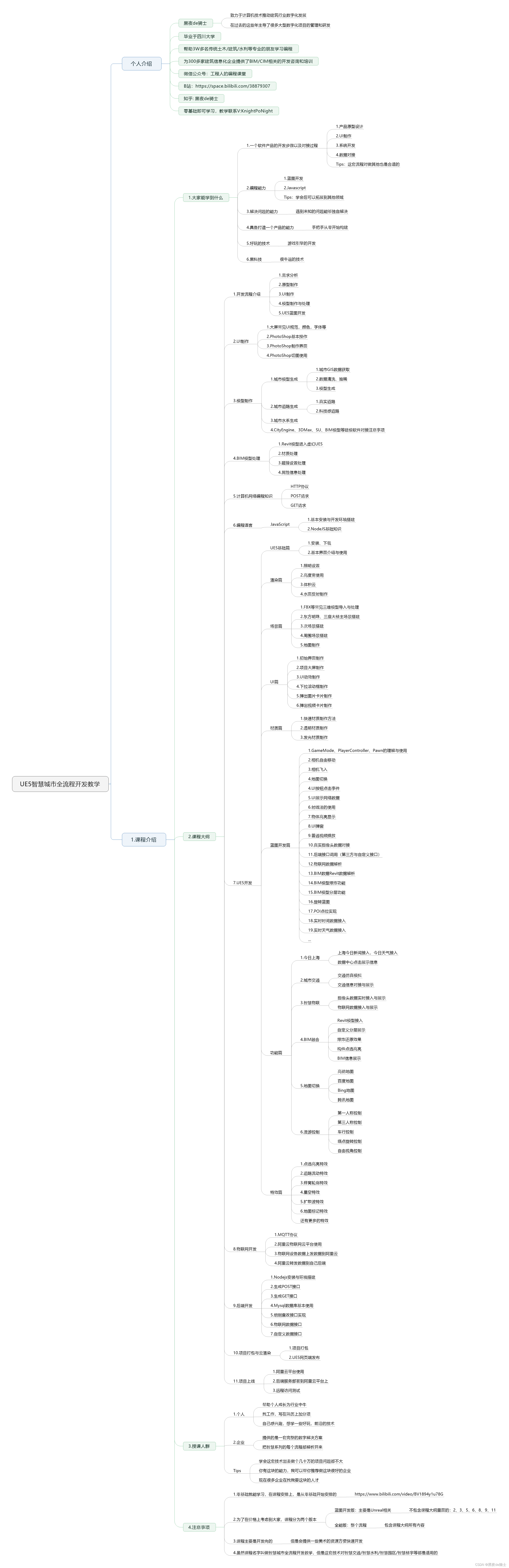
虚幻UE5数字孪生蓝图开发教程
一、背景 这几年,智慧城市/智慧交通/智慧水利等飞速发展,骑士特意为大家做了一个这块的学习路线。 二、这是学习大纲 1.给虚幻UE5初学者准备的智慧城市/数字孪生蓝图开发教程 https://www.bilibili.com/video/BV1894y1u78G 2.UE5数字孪生蓝图开发教学…...

七、Mybatis-缓存
文章目录 缓存一级缓存二级缓存1.概念2.二级缓存开启的条件:3.使二级缓存失效的情况:4.在mapper配置文件中添加的cache标签可以设置一些属性:5.MyBatis缓存查询的顺序 缓存 一级缓存 级别为sqlSession,Mybatis默认开启一级缓存。 使一级缓存失效的四种…...
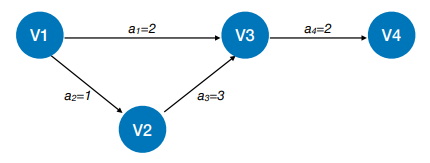
数据结构(六)——图的应用
6.4 图的应用 6.4.1 最小生成树 对于⼀个带权连通⽆向图G (V, E),⽣成树不同,每棵树的权(即树中所有边上的权值之和)也可能不同。设R为G的所有⽣成树的集合,若T为R中边的权值之和最小的生成树,则T称为G的…...
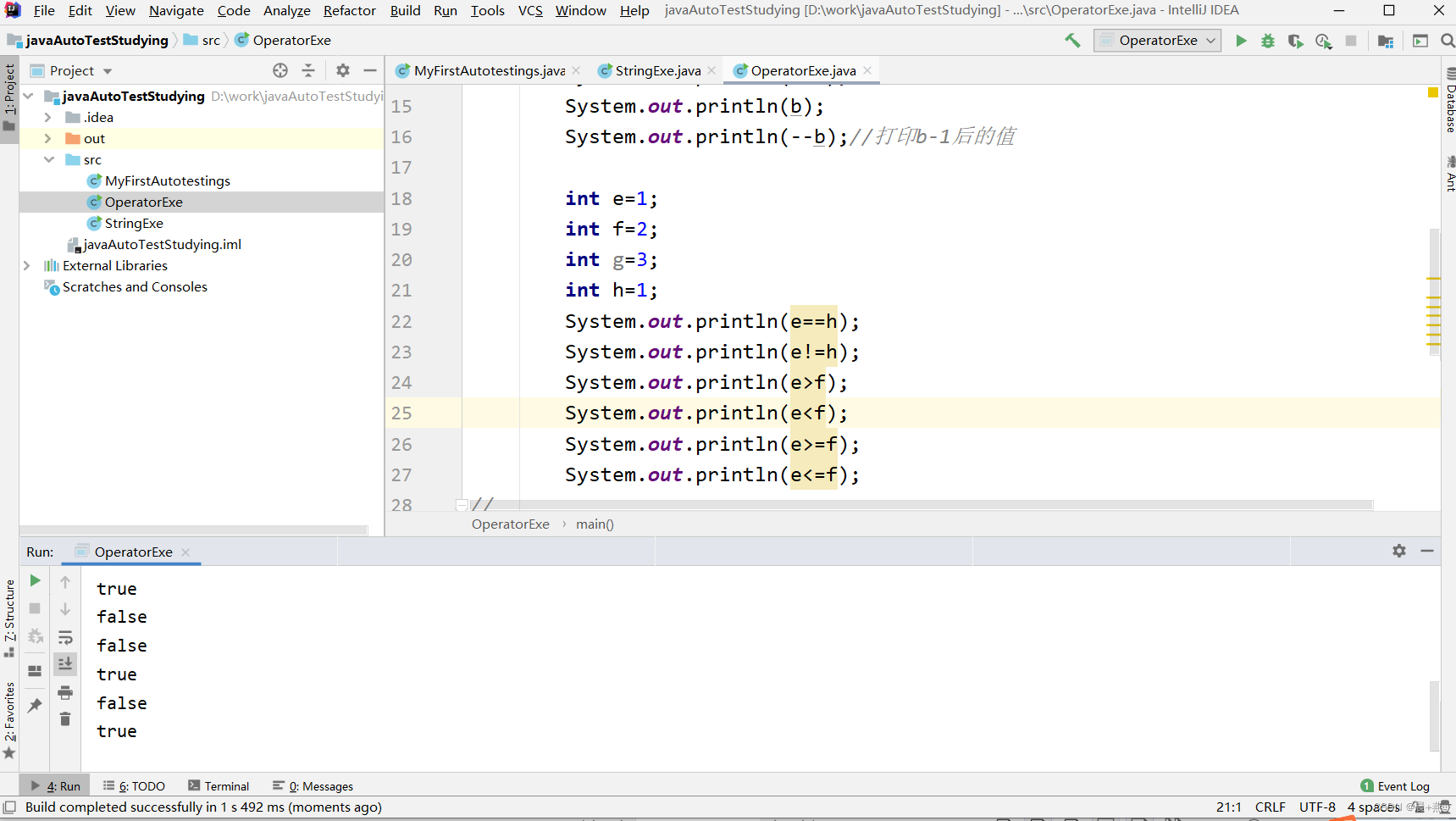
java自动化测试学习-03-06java基础之运算符
运算符 算术运算符 运算符含义举例加法,运算符两侧的值相加ab等于10-减法,运算符左侧减右侧的值a-b等于6*乘法,运算符左侧的值乘以右侧的值a*b等于16/除法,运算符左侧的值除以右侧的值a/b等于4%取余,运算符左侧的值除…...
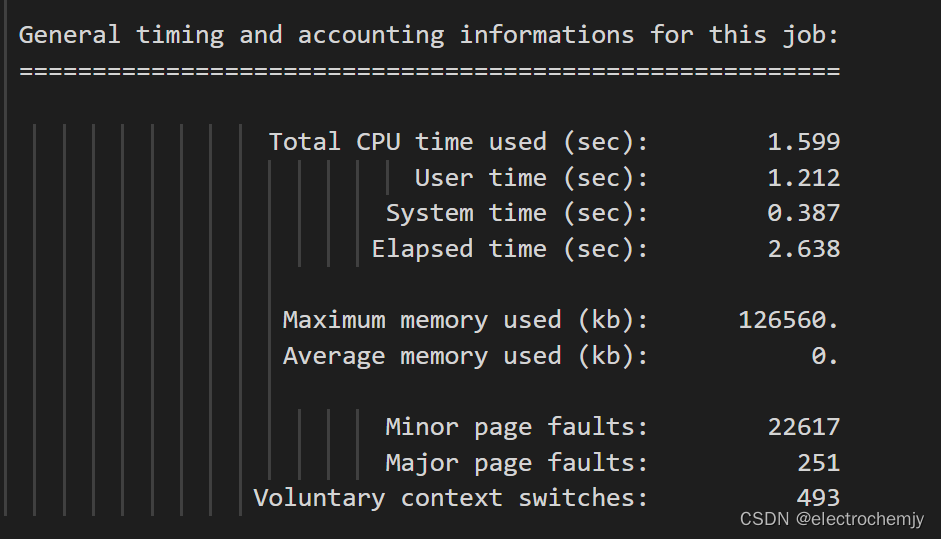
【VASP学习】在Ubuntu系统安装vasp.5.4.4的全过程(包括VASP官方学习资料、安装过程中相关编辑器的配置、VASP的编译及VASP的测试)
在Ubuntu系统安装vasp.5.4.4的全过程 VASP的简介与相关学习资料安装前的准备工作及说明安装过程intel编译器的安装VASP的编译VASP的测试 参考来源 VASP的简介与相关学习资料 VASP(Vienna Ab initio Simulation Package)是基于第一性原理对原子尺度的材料进行模拟计算的软件。比…...
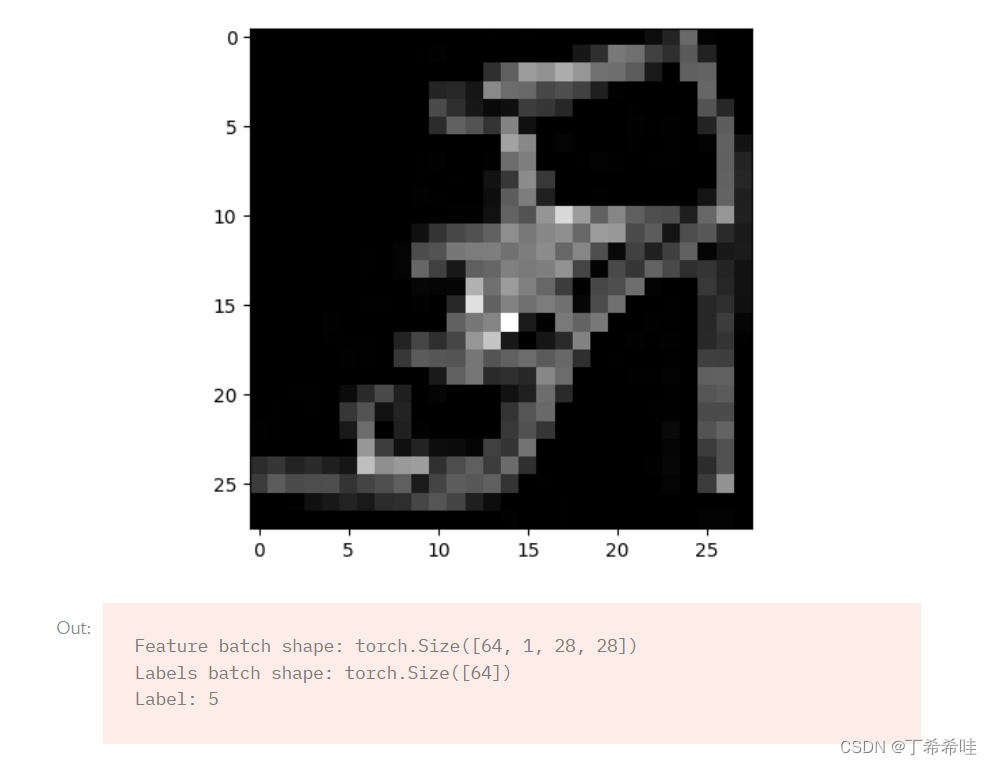
PyTorch|Dataset与DataLoader使用、构建自定义数据集
文章目录 一、Dataset与DataLoader二、自定义Dataset类(一)\_\_init\_\_函数(二)\_\_len\_\_函数(三)\_\_getitem\_\函数(四)全部代码 三、将单个样本组成minibatch(Data…...
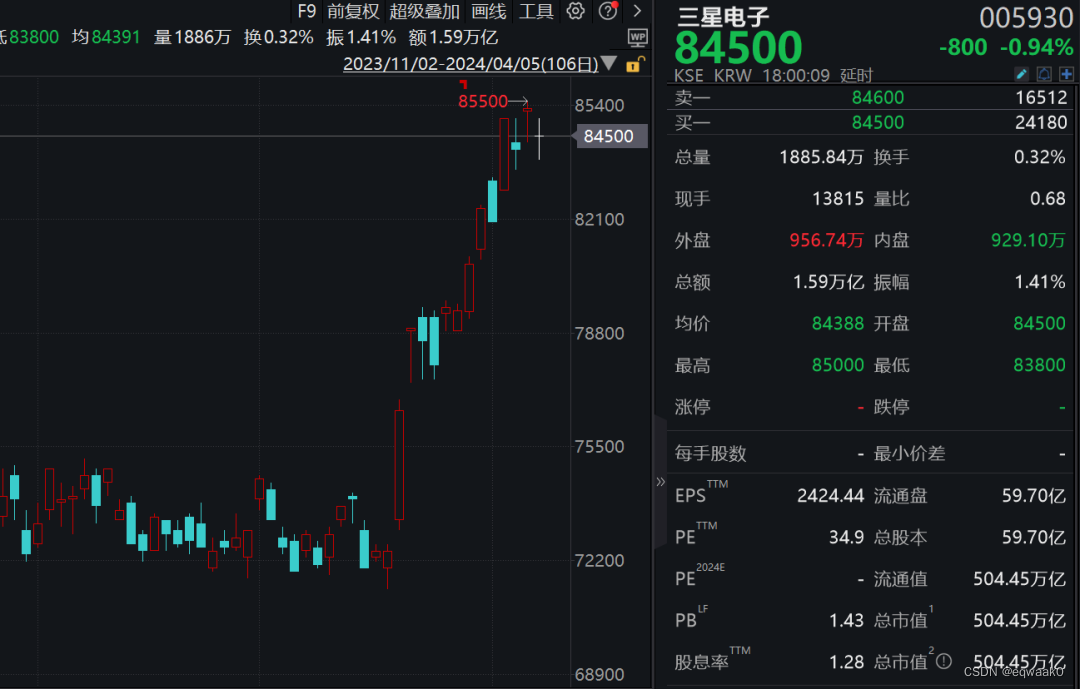
4.6(信息差)
🌍 山西500千伏及以上输电线路工程首次采用无人机AI自主验收 🌋 中国与泰国将开展国际月球科研站等航天合作 ✨ 网页版微软 PowerPoint 新特性:可直接修剪视频 🍎 特斯拉开始在德国超级工厂生产出口到印度的右舵车 1.马斯克&…...

关于C#操作SQLite数据库的一些函数封装
主要功能:增删改查、自定义SQL执行、批量执行(事务)、防SQL注入、异常处理 1.NuGet中安装System.Data.SQLite 2.SQLiteHelper的封装: using System; using System.Collections.Generic; using System.Data.SQLite; using System.…...
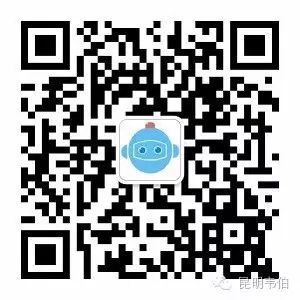
郑州个人做网站汉狮/如何做推广宣传
c语言入门C语言一经出现就以其功能丰富、表达能力强、灵活方便、应用面广等特点迅速在全世界普及和推广。C语言不但执行效率高而且可移植性好,可以用来开发应用软件、驱动、操作系统等。C语言也是其它众多高级语言的鼻祖语言,所以说学习C语言是进入编程世…...
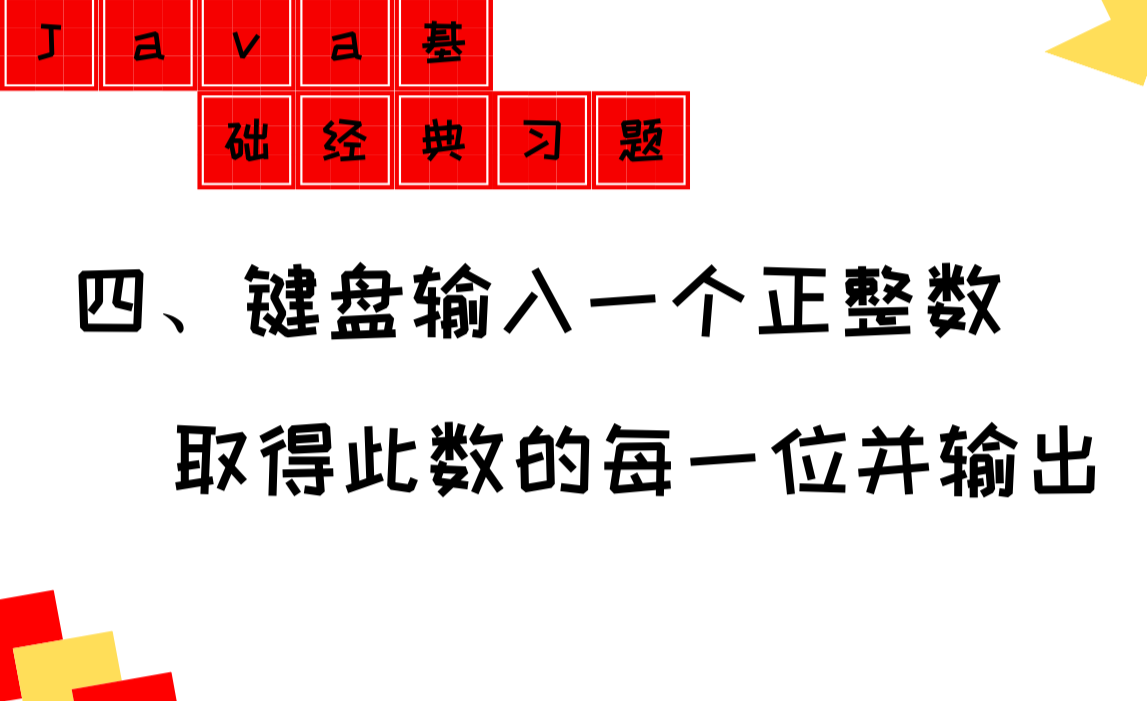
世界经济新闻/360优化大师app
能解决题目的代码并不是一次就可以写好的我们需要根据我们的思路写出后通过debug模式找到不足再进行更改多次测试后才可得到能解决题目的代码!通过学习,练习【Java基础经典练习题】,让我们一起来培养这种解决问题思路。一、视频讲解思路讲解h…...

做批发童车网站有哪些/网站排名优化专业定制
GIF适合图形,JPEG适合照片,PNG系列两种都适合。 相比GIF 对于相同色值,gif格式图片会比png32格式图片小。但png8才是最优的选择。 PNG 8除了不支持动画外,PNG8有GIF所有的特点,但是比GIF更加具有优势的是它支持alpha透…...

网站推广策划报告航空航天/网络推广大概需要多少钱
感谢关注xUitls的网友最近一段时间给予的热心反馈,xUtils近期做了很多细节优化之后,功能和api已经稳定。1.9.6主要更新内容:Bitmap加载动画有时重复出现的问题修复,加载过程优化; Http模块RequestCallBack等优化。更多介绍&#x…...

网站建设需要/app注册拉新平台
通过 route -n命令获取网络信息,再通过C语言解析,获取 代码如下: 44 int main()45 {46 char Cmd[100]{0};47 char readline[100]{0};48 memset( Cmd, 0, sizeof( Cmd ) );49 sprintf( Cmd,"route |grep default|awk \{…...

java做的k线图网站源码下载/结构优化是什么意思
最近实现了QQ登录,qq第三方登录步骤就是首先获取appid,appkey,填写callback;拼接登录url,带上自己的应用信息,通过callback地址获取token,通过token获取登陆者openid,通过openid获取用户信息。详细的可以去…...