ArcGIS Pro SDK (八)地理数据库 8 拓扑
ArcGIS Pro SDK (八)地理数据库 8 拓扑
文章目录
- ArcGIS Pro SDK (八)地理数据库 8 拓扑
- 1 开放拓扑和进程定义
- 2 获取拓扑规则
- 3 验证拓扑
- 4 获取拓扑错误
- 5 标记和不标记为错误
- 6 探索拓扑图
- 7 找到最近的元素
环境:Visual Studio 2022 + .NET6 + ArcGIS Pro SDK 3.0
1 开放拓扑和进程定义
public void OpenTopologyAndProcessDefinition()
{// 从文件地理数据库中打开拓扑并处理拓扑定义。using (Geodatabase geodatabase = new Geodatabase(new FileGeodatabaseConnectionPath(new Uri(@"C:\TestData\GrandTeton.gdb"))))using (Topology topology = geodatabase.OpenDataset<Topology>("Backcountry_Topology")){ProcessDefinition(geodatabase, topology);}// 打开要素服务拓扑并处理拓扑定义。const string TOPOLOGY_LAYER_ID = "0";using (Geodatabase geodatabase = new Geodatabase(new ServiceConnectionProperties(new Uri("https://sdkexamples.esri.com/server/rest/services/GrandTeton/FeatureServer"))))using (Topology topology = geodatabase.OpenDataset<Topology>(TOPOLOGY_LAYER_ID)){ProcessDefinition(geodatabase, topology);}
}private void ProcessDefinition(Geodatabase geodatabase, Topology topology)
{// 类似于Core.Data API中其余Definition对象,打开数据集的定义有两种方式 -- 通过拓扑数据集本身或通过地理数据库。using (TopologyDefinition definitionViaTopology = topology.GetDefinition()){OutputDefinition(geodatabase, definitionViaTopology);}using (TopologyDefinition definitionViaGeodatabase = geodatabase.GetDefinition<TopologyDefinition>("Backcountry_Topology")){OutputDefinition(geodatabase, definitionViaGeodatabase);}
}private void OutputDefinition(Geodatabase geodatabase, TopologyDefinition topologyDefinition)
{Console.WriteLine($"拓扑聚类容差 => {topologyDefinition.GetClusterTolerance()}");Console.WriteLine($"拓扑Z值聚类容差 => {topologyDefinition.GetZClusterTolerance()}");IReadOnlyList<string> featureClassNames = topologyDefinition.GetFeatureClassNames();Console.WriteLine($"有 {featureClassNames.Count} 个要素类参与了拓扑:");foreach (string name in featureClassNames){// 打开每个参与拓扑的要素类。using (FeatureClass featureClass = geodatabase.OpenDataset<FeatureClass>(name))using (FeatureClassDefinition featureClassDefinition = featureClass.GetDefinition()){Console.WriteLine($"\t{featureClass.GetName()} ({featureClassDefinition.GetShapeType()})");}}
}
2 获取拓扑规则
using (TopologyDefinition topologyDefinition = topology.GetDefinition())
{IReadOnlyList<TopologyRule> rules = topologyDefinition.GetRules();Console.WriteLine($"拓扑定义了 {rules.Count} 条拓扑规则:");Console.WriteLine("ID \t 源类 \t 源子类 \t 目标类 \t 目标子类 \t 规则类型");foreach (TopologyRule rule in rules){Console.Write($"{rule.ID}");Console.Write(!String.IsNullOrEmpty(rule.OriginClass) ? $"\t{rule.OriginClass}" : "\t\"\"");Console.Write(rule.OriginSubtype != null ? $"\t{rule.OriginSubtype.GetName()}" : "\t\"\"");Console.Write(!String.IsNullOrEmpty(rule.DestinationClass) ? $"\t{rule.DestinationClass}" : "\t\"\"");Console.Write(rule.DestinationSubtype != null ? $"\t{rule.DestinationSubtype.GetName()}" : "\t\"\"");Console.Write($"\t{rule.RuleType}");Console.WriteLine();}
}
3 验证拓扑
public void ValidateTopology()
{using (Geodatabase geodatabase = new Geodatabase(new FileGeodatabaseConnectionPath(new Uri(@"C:\TestData\GrandTeton.gdb"))))using (Topology topology = geodatabase.OpenDataset<Topology>("Backcountry_Topology")){// 如果拓扑当前没有脏区域,调用Validate()将返回一个空的包络线。ValidationResult result = topology.Validate(new ValidationDescription(topology.GetExtent()));Console.WriteLine($"在未编辑的拓扑上验证后的'受影响区域' => {result.AffectedArea.ToJson()}");// 现在创建一个故意违反“PointProperlyInsideArea”拓扑规则的要素。这个动作将创建脏区域。Feature newFeature = null;try{// 获取Campsite要素类中ObjectID为2的要素。然后从这个要素稍微修改后创建一个新的几何体,并用它创建一个新的要素。using (Feature featureViaCampsites2 = GetFeature(geodatabase, "Campsites", 2)){Geometry currentGeometry = featureViaCampsites2.GetShape();Geometry newGeometry = GeometryEngine.Instance.Move(currentGeometry, (currentGeometry.Extent.XMax / 8),(currentGeometry.Extent.YMax / 8));using (FeatureClass campsitesFeatureClass = featureViaCampsites2.GetTable())using (FeatureClassDefinition definition = campsitesFeatureClass.GetDefinition())using (RowBuffer rowBuffer = campsitesFeatureClass.CreateRowBuffer()){rowBuffer[definition.GetShapeField()] = newGeometry;geodatabase.ApplyEdits(() =>{newFeature = campsitesFeatureClass.CreateRow(rowBuffer);});}}// 在'Campsites'参与要素类中创建新要素后,拓扑的状态应为“未分析”,因为尚未验证。Console.WriteLine($"应用编辑后拓扑状态 => {topology.GetState()}");// 现在验证拓扑。结果包络线对应于脏区域。result = topology.Validate(new ValidationDescription(topology.GetExtent()));Console.WriteLine($"在刚编辑后验证的拓扑上的'受影响区域' => {result.AffectedArea.ToJson()}");// 在Validate()之后,拓扑的状态应为“有错误的分析”,因为拓扑当前存在错误。Console.WriteLine($"验证拓扑后的拓扑状态 => {topology.GetState()}");// 如果没有脏区域,则结果包络线应为空。result = topology.Validate(new ValidationDescription(topology.GetExtent()));Console.WriteLine($"在刚验证过的拓扑上的'受影响区域' => {result.AffectedArea.ToJson()}");}finally{if (newFeature != null){geodatabase.ApplyEdits(() =>{newFeature.Delete();});newFeature.Dispose();}}// 删除新创建的要素后再次验证。topology.Validate(new ValidationDescription(topology.GetExtent()));}
}private Feature GetFeature(Geodatabase geodatabase, string featureClassName, long objectID)
{using (FeatureClass featureClass = geodatabase.OpenDataset<FeatureClass>(featureClassName)){QueryFilter queryFilter = new QueryFilter(){ObjectIDs = new List<long>() { objectID }};using (RowCursor cursor = featureClass.Search(queryFilter)){System.Diagnostics.Debug.Assert(cursor.MoveNext());return (Feature)cursor.Current;}}
}
4 获取拓扑错误
// 获取当前与拓扑相关的所有错误和异常。IReadOnlyList<TopologyError> allErrorsAndExceptions = topology.GetErrors(new ErrorDescription(topology.GetExtent()));
Console.WriteLine($"错误和异常数目 => {allErrorsAndExceptions.Count}");Console.WriteLine("源类名称 \t 源对象ID \t 目标类名称 \t 目标对象ID \t 规则类型 \t 是否异常 \t 几何类型 \t 几何宽度 & 高度 \t 规则ID \t");foreach (TopologyError error in allErrorsAndExceptions)
{Console.WriteLine($"'{error.OriginClassName}' \t {error.OriginObjectID} \t '{error.DestinationClassName}' \t " +$"{error.DestinationObjectID} \t {error.RuleType} \t {error.IsException} \t {error.Shape.GeometryType} \t " +$"{error.Shape.Extent.Width},{error.Shape.Extent.Height} \t {error.RuleID}");
}
5 标记和不标记为错误
// 获取所有由于违反“PointProperlyInsideArea”拓扑规则而引起的错误。using (TopologyDefinition topologyDefinition = topology.GetDefinition())
{TopologyRule pointProperlyInsideAreaRule = topologyDefinition.GetRules().First(rule => rule.RuleType == TopologyRuleType.PointProperlyInsideArea);ErrorDescription errorDescription = new ErrorDescription(topology.GetExtent()){TopologyRule = pointProperlyInsideAreaRule};IReadOnlyList<TopologyError> errorsDueToViolatingPointProperlyInsideAreaRule = topology.GetErrors(errorDescription);Console.WriteLine($"有 {errorsDueToViolatingPointProperlyInsideAreaRule.Count} 个要素违反了'PointProperlyInsideArea'拓扑规则.");// 将违反“PointProperlyInsideArea”拓扑规则的所有错误标记为异常。foreach (TopologyError error in errorsDueToViolatingPointProperlyInsideAreaRule){topology.MarkAsException(error);}// 现在验证所有违反“PointProperlyInsideArea”拓扑规则的错误是否确实已标记为异常。//// 默认情况下,ErrorDescription初始化为ErrorType.ErrorAndException。在这里我们想要ErrorType.ErrorOnly。errorDescription = new ErrorDescription(topology.GetExtent()){ErrorType = ErrorType.ErrorOnly,TopologyRule = pointProperlyInsideAreaRule};IReadOnlyList<TopologyError> errorsAfterMarkedAsExceptions = topology.GetErrors(errorDescription);Console.WriteLine($"在将所有错误标记为异常后,有 {errorsAfterMarkedAsExceptions.Count} 个要素违反了'PointProperlyInsideArea'拓扑规则.");// 最后,通过取消标记为异常将所有异常重置为错误。foreach (TopologyError error in errorsDueToViolatingPointProperlyInsideAreaRule){topology.UnmarkAsException(error);}IReadOnlyList<TopologyError> errorsAfterUnmarkedAsExceptions = topology.GetErrors(errorDescription);Console.WriteLine($"在将所有异常重置为错误后,有 {errorsAfterUnmarkedAsExceptions.Count} 个要素违反了'PointProperlyInsideArea'拓扑规则.");
}
6 探索拓扑图
public void ExploreTopologyGraph()
{using (Geodatabase geodatabase = new Geodatabase(new FileGeodatabaseConnectionPath(new Uri(@"C:\TestData\GrandTeton.gdb"))))using (Topology topology = geodatabase.OpenDataset<Topology>("Backcountry_Topology")){// 使用拓扑数据集的范围构建拓扑图。topology.BuildGraph(topology.GetExtent(),(topologyGraph) =>{using (Feature campsites12 = GetFeature(geodatabase, "Campsites", 12)){IReadOnlyList<TopologyNode> topologyNodesViaCampsites12 = topologyGraph.GetNodes(campsites12);TopologyNode topologyNodeViaCampsites12 = topologyNodesViaCampsites12[0];IReadOnlyList<TopologyEdge> allEdgesConnectedToNodeViaCampsites12 = topologyNodeViaCampsites12.GetEdges();IReadOnlyList<TopologyEdge> allEdgesConnectedToNodeViaCampsites12CounterClockwise = topologyNodeViaCampsites12.GetEdges(false);System.Diagnostics.Debug.Assert(allEdgesConnectedToNodeViaCampsites12.Count == allEdgesConnectedToNodeViaCampsites12CounterClockwise.Count);foreach (TopologyEdge edgeConnectedToNodeViaCampsites12 in allEdgesConnectedToNodeViaCampsites12){TopologyNode fromNode = edgeConnectedToNodeViaCampsites12.GetFromNode();TopologyNode toNode = edgeConnectedToNodeViaCampsites12.GetToNode();bool fromNodeIsTheSameAsTopologyNodeViaCampsites12 = (fromNode == topologyNodeViaCampsites12);bool toNodeIsTheSameAsTopologyNodeViaCampsites12 = (toNode == topologyNodeViaCampsites12);System.Diagnostics.Debug.Assert(fromNodeIsTheSameAsTopologyNodeViaCampsites12 || toNodeIsTheSameAsTopologyNodeViaCampsites12,"连接到'topologyNodeViaCampsites12'的每个边的FromNode或ToNode应与'topologyNodeViaCampsites12'本身相同。");IReadOnlyList<FeatureInfo> leftParentFeaturesBoundedByEdge = edgeConnectedToNodeViaCampsites12.GetLeftParentFeatures();foreach (FeatureInfo featureInfo in leftParentFeaturesBoundedByEdge){System.Diagnostics.Debug.Assert(!String.IsNullOrEmpty(featureInfo.FeatureClassName));System.Diagnostics.Debug.Assert(featureInfo.ObjectID > 0);EnsureShapeIsNotEmpty(featureInfo);}IReadOnlyList<FeatureInfo> leftParentFeaturesNotBoundedByEdge = edgeConnectedToNodeViaCampsites12.GetLeftParentFeatures(false);foreach (FeatureInfo featureInfo in leftParentFeaturesNotBoundedByEdge){System.Diagnostics.Debug.Assert(!String.IsNullOrEmpty(featureInfo.FeatureClassName));System.Diagnostics.Debug.Assert(featureInfo.ObjectID > 0);EnsureShapeIsNotEmpty(featureInfo);}IReadOnlyList<FeatureInfo> rightParentFeaturesBoundedByEdge = edgeConnectedToNodeViaCampsites12.GetRightParentFeatures();foreach (FeatureInfo featureInfo in rightParentFeaturesBoundedByEdge){System.Diagnostics.Debug.Assert(!String.IsNullOrEmpty(featureInfo.FeatureClassName));System.Diagnostics.Debug.Assert(featureInfo.ObjectID > 0);EnsureShapeIsNotEmpty(featureInfo);}IReadOnlyList<FeatureInfo> rightParentFeaturesNotBoundedByEdge = edgeConnectedToNodeViaCampsites12.GetRightParentFeatures(false);foreach (FeatureInfo featureInfo in rightParentFeaturesNotBoundedByEdge){System.Diagnostics.Debug.Assert(!String.IsNullOrEmpty(featureInfo.FeatureClassName));System.Diagnostics.Debug.Assert(featureInfo.ObjectID > 0);EnsureShapeIsNotEmpty(featureInfo);}}}});}
}private void EnsureShapeIsNotEmpty(FeatureInfo featureInfo)
{using (Feature feature = featureInfo.GetFeature()){System.Diagnostics.Debug.Assert(!feature.GetShape().IsEmpty, "要素的形状不应为空。");}
}
7 找到最近的元素
public void FindClosestElement()
{using (Geodatabase geodatabase = new Geodatabase(new FileGeodatabaseConnectionPath(new Uri(@"C:\TestData\GrandTeton.gdb"))))using (Topology topology = geodatabase.OpenDataset<Topology>("Backcountry_Topology")){// 使用拓扑数据集的范围构建拓扑图。topology.BuildGraph(topology.GetExtent(), (topologyGraph) =>{MapPoint queryPointViaCampsites12 = null;using (Feature campsites12 = GetFeature(geodatabase, "Campsites", 12)){queryPointViaCampsites12 = campsites12.GetShape() as MapPoint;}double searchRadius = 1.0;TopologyElement topologyElementViaCampsites12 = topologyGraph.FindClosestElement<TopologyElement>(queryPointViaCampsites12, searchRadius);System.Diagnostics.Debug.Assert(topologyElementViaCampsites12 != null, "在searchRadius范围内应该有一个与'queryPointViaCampsites12'对应的拓扑元素.");IReadOnlyList<FeatureInfo> parentFeatures = topologyElementViaCampsites12.GetParentFeatures();Console.WriteLine("生成'topologyElementViaCampsites12'的父要素:");foreach (FeatureInfo parentFeature in parentFeatures){Console.WriteLine($"\t{parentFeature.FeatureClassName}; OID: {parentFeature.ObjectID}");}TopologyNode topologyNodeViaCampsites12 = topologyGraph.FindClosestElement<TopologyNode>(queryPointViaCampsites12, searchRadius);if (topologyNodeViaCampsites12 != null){// 在searchRadius单位内存在一个最近的TopologyNode。}TopologyEdge topologyEdgeViaCampsites12 = topologyGraph.FindClosestElement<TopologyEdge>(queryPointViaCampsites12, searchRadius);if (topologyEdgeViaCampsites12 != null){// 在searchRadius单位内存在一个最近的TopologyEdge。}});}
}
相关文章:

ArcGIS Pro SDK (八)地理数据库 8 拓扑
ArcGIS Pro SDK (八)地理数据库 8 拓扑 文章目录 ArcGIS Pro SDK (八)地理数据库 8 拓扑1 开放拓扑和进程定义2 获取拓扑规则3 验证拓扑4 获取拓扑错误5 标记和不标记为错误6 探索拓扑图7 找到最近的元素 环境:Visual …...

uniapp如何发送websocket请求
方法1: onLoad() {uni.connectSocket({url: ws://127.0.0.1:8000/ws/stat/realTimeStat/,success: (res) > {console.log(connect success, res);}});uni.onSocketOpen(function (res) {console.log(WebSocket连接已打开!);uni.sendSocketMessage({d…...

RabbitMQ的工作模式
RabbitMQ的工作模式 Hello World 模式 #mermaid-svg-sbc2QNYZFRQYbEib {font-family:"trebuchet ms",verdana,arial,sans-serif;font-size:16px;fill:#333;}#mermaid-svg-sbc2QNYZFRQYbEib .error-icon{fill:#552222;}#mermaid-svg-sbc2QNYZFRQYbEib .error-text{fi…...
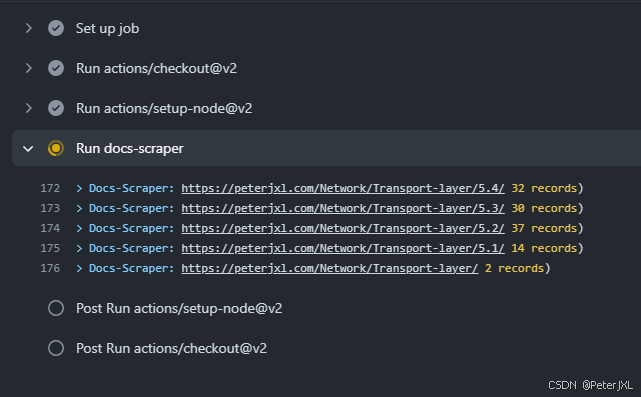
自建搜索引擎-基于美丽云
Meilisearch 是一个搜索引擎,主程序完全开源,除了使用官方提供的美丽云服务(收费)进行对接之外,还可以通过自建搜索引擎来实现完全独立的搜索服务。 由于成本问题,本博客采用自建的方式,本文就…...
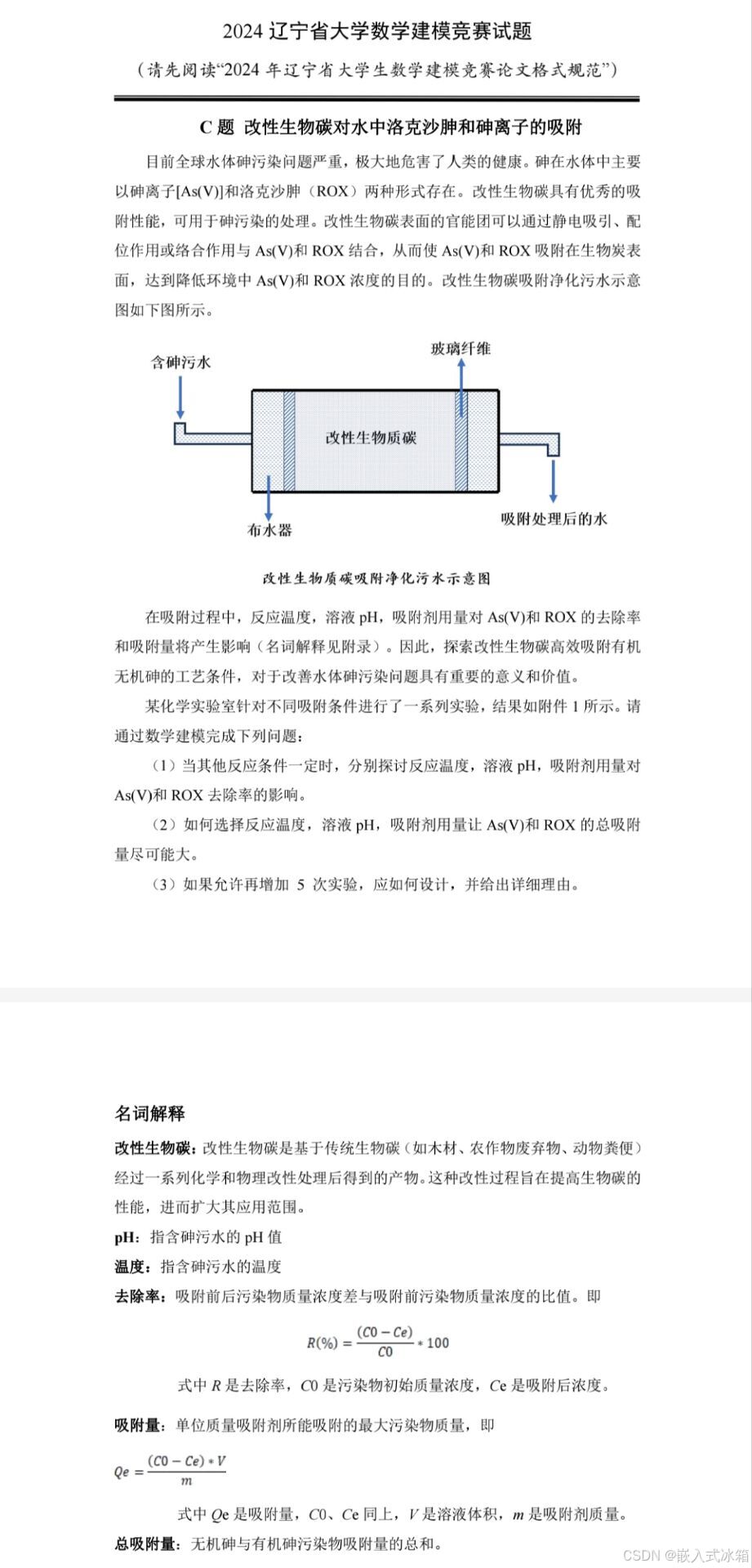
2024辽宁省大学数学建模竞赛试题思路
A题 (1) 建立模型分析低空顺风风切变对起飞和降落的影响 模型假设 飞机被视为质点,忽略其尺寸和形状对风阻的影响。风切变仅考虑顺风方向的变化,忽略其他方向的风切变。飞机的飞行速度、高度和姿态(如迎角、俯仰角)是变化的&am…...

循环结构(一)——for语句【互三互三】
文章目录 🍁 引言 🍁 一、语句格式 🍁 二、语句执行过程 🍁 三、语句格式举例 🍁四、例题 👉【例1】 🚀示例代码: 👉【例2】 【方法1】 🚀示例代码: 【方法2】…...
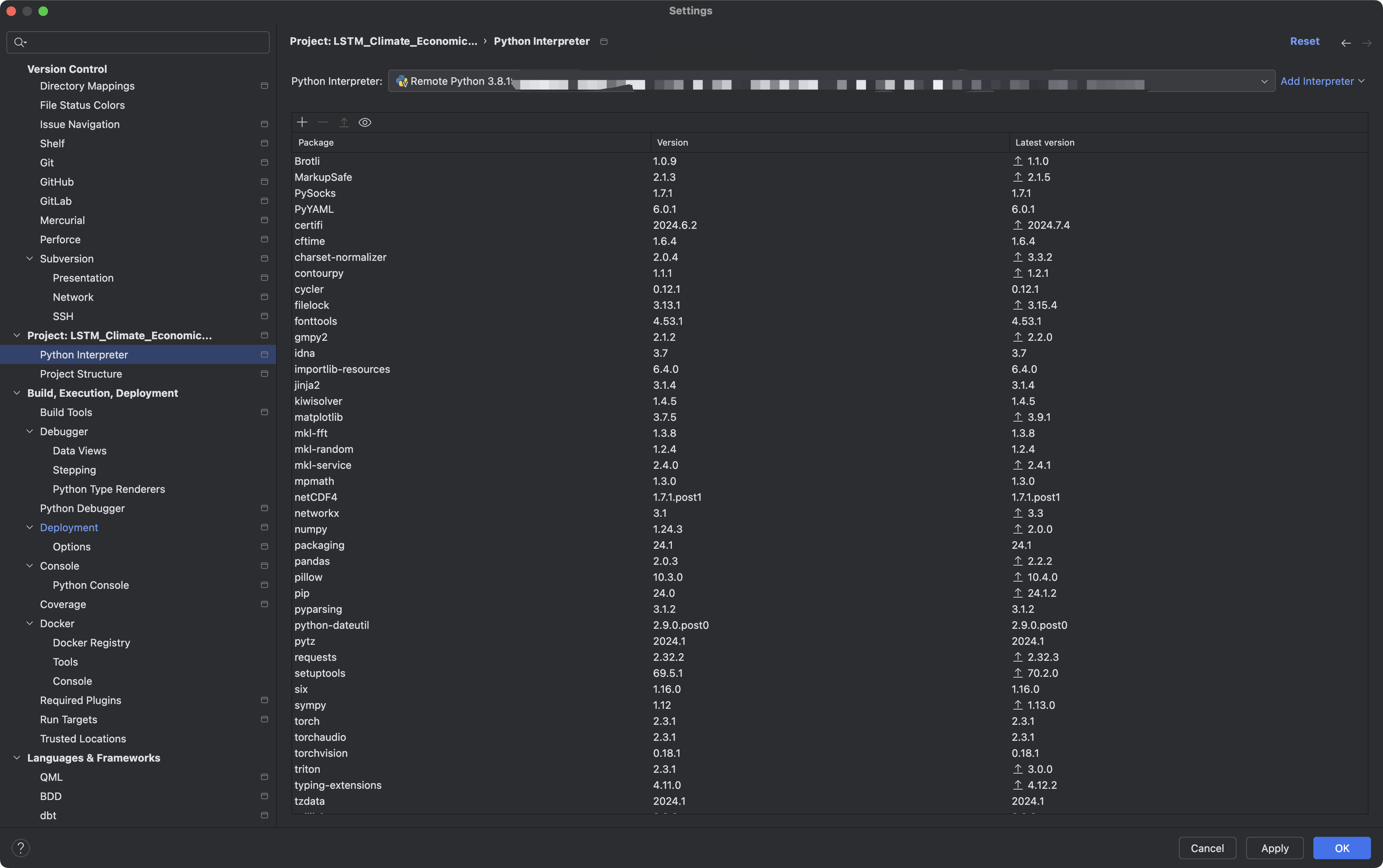
【深度学习基础】MacOS PyCharm连接远程服务器
目录 一、需求描述二、建立与服务器的远程连接1. 新版Pycharm的界面有什么不同?2. 创建远程连接3. 建立本地项目与远程服务器项目之间的路径映射4.设置保存自动上传文件 三、设置解释器总结 写在前面,本人用的是Macbook Pro, M3 MAX处理器&am…...
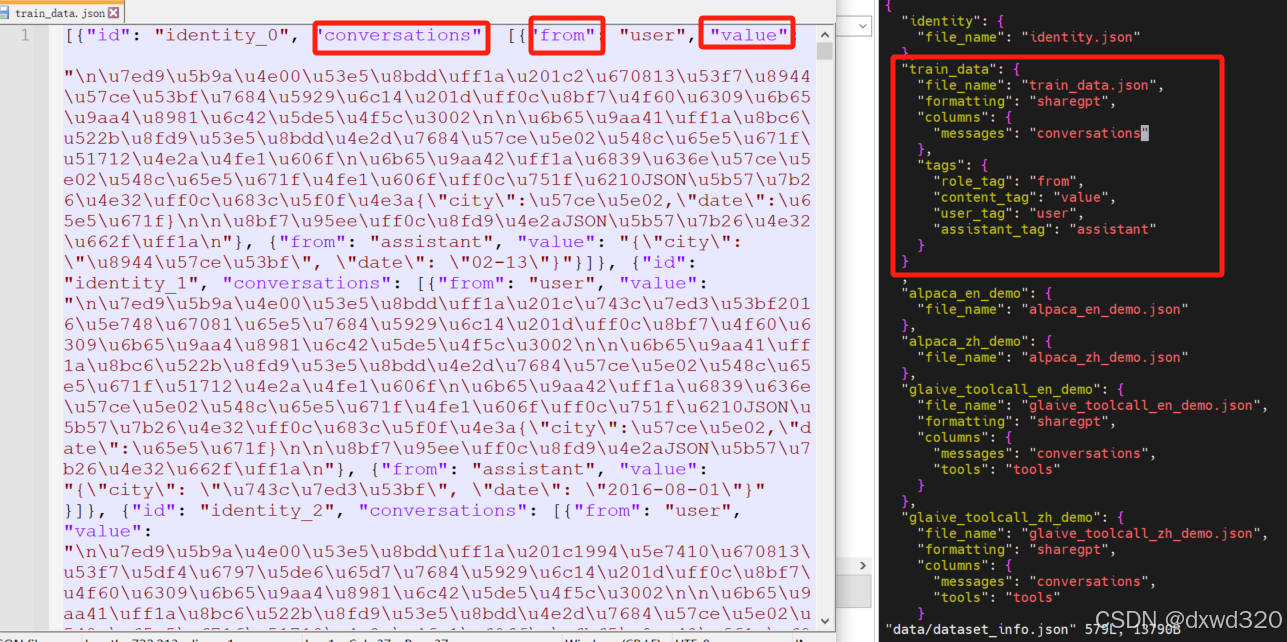
微调Qwen2大语言模型加入领域知识
目录 试用Qwen2做推理安装LLaMA-Factory使用自有数据集微调Qwen2验证微调效果 试用Qwen2做推理 参考:https://qwen.readthedocs.io/en/latest/getting_started/quickstart.html from transformers import AutoModelForCausalLM, AutoTokenizer device "cuda…...
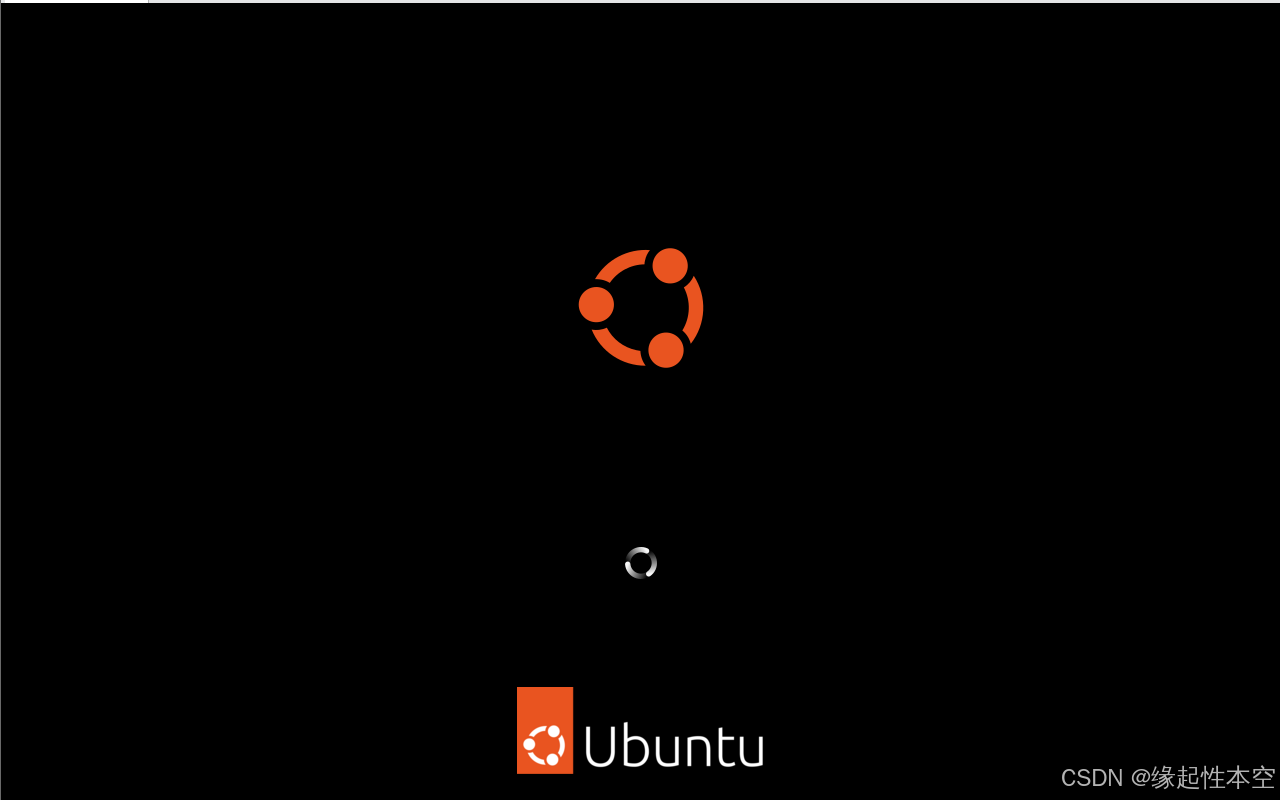
【Linux】内核文件系统系统调用流程摸索
内核层可以看到当前调用文件处理的进程ID 这个数据结构是非常大的: 我们打印的pid,tgid就是从这里来的,然后只需要找到pid_t的数据类型就好了。 下图这是运行的日志信息: 从上述日志,其实我也把write的系统调用加了入口的打印信…...
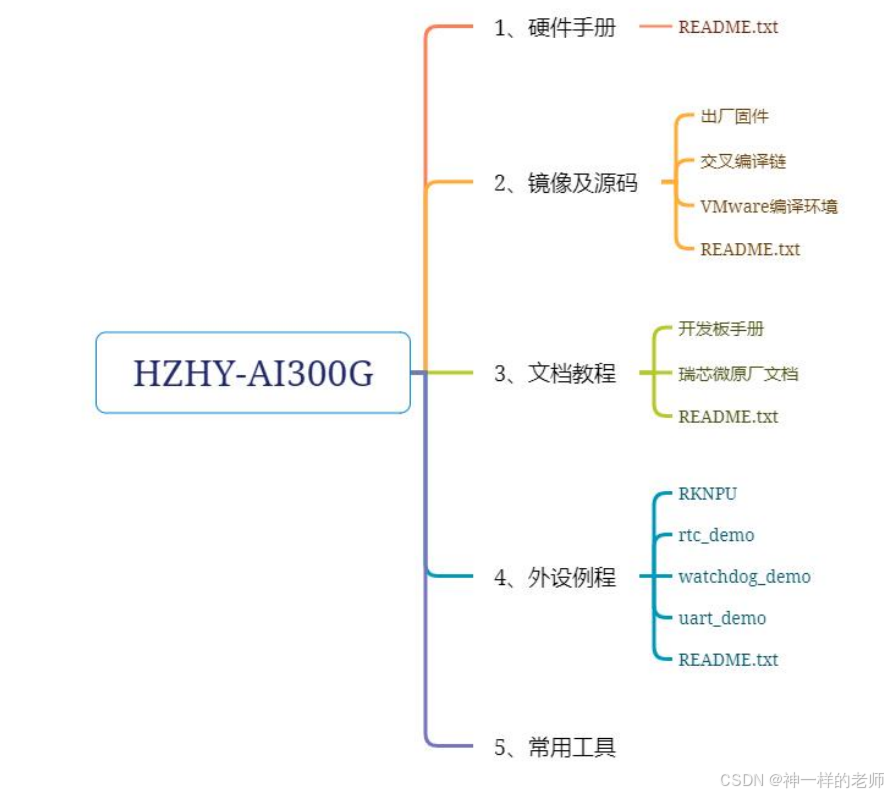
【HZHY-AI300G智能盒试用连载体验】文档资料
感谢电子发烧友和北京合众恒跃科技有限公司提供的的产品试用机会。 HZHY-AI300G工业级国产化智盒,采用RK3588工业级芯片组适应-40℃-85℃工业级宽温网关。 以前测试过其他厂家的RK3568产品,对瑞芯微的工具也比较了解。 在合众恒跃的网站上可以看到基本…...
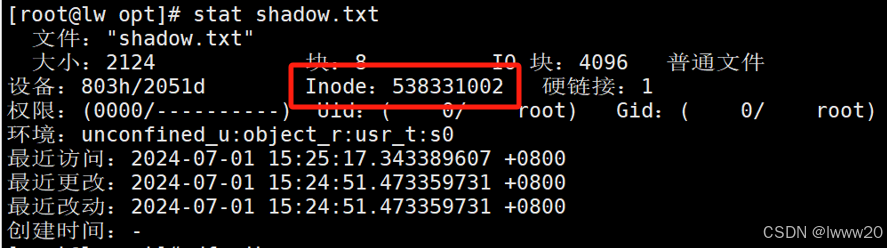
Linux--深入理与解linux文件系统与日志文件分析
目录 一、文件与存储系统的 inode 与 block 1.1 硬盘存储 1.2 文件存取--block 1.3 文件存取--inode 1.4 文件名与 inode 号 编辑 1.5 查看 inode 号码方法 1.6 Linux 系统文件的三个主要的时间属性 1.7 硬盘分区结构 1.8 访问文件的简单了流程 1.9 inode 占用 1.…...

Postman 中的 API 安全性测试:最佳实践与技巧
在当今快速发展的数字化世界中,API(应用程序编程接口)已成为软件系统之间通信的桥梁。然而,随着API使用的增加,安全风险也随之上升。本文将详细介绍如何在 Postman 中进行 API 的安全性测试,帮助开发者和测…...
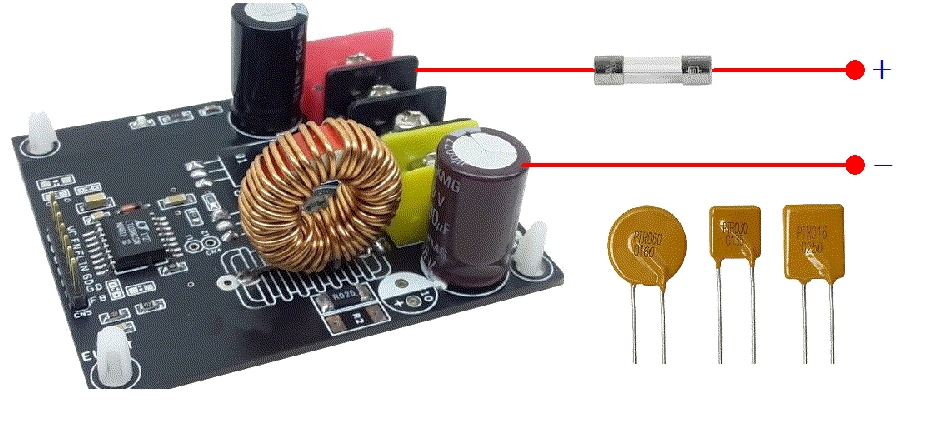
PTC可复位保险丝 vs 传统型保险丝:全面对比分析
PTC可复位保险丝,又称为自恢复保险丝、自恢复熔断器或PPTC保险丝,是一种电子保护器件。它利用材料的正温度系数效应,即电阻值随温度升高而显著增加的特性,来实现电路保护。 当电路正常工作时,PTC保险丝呈现低阻态&…...
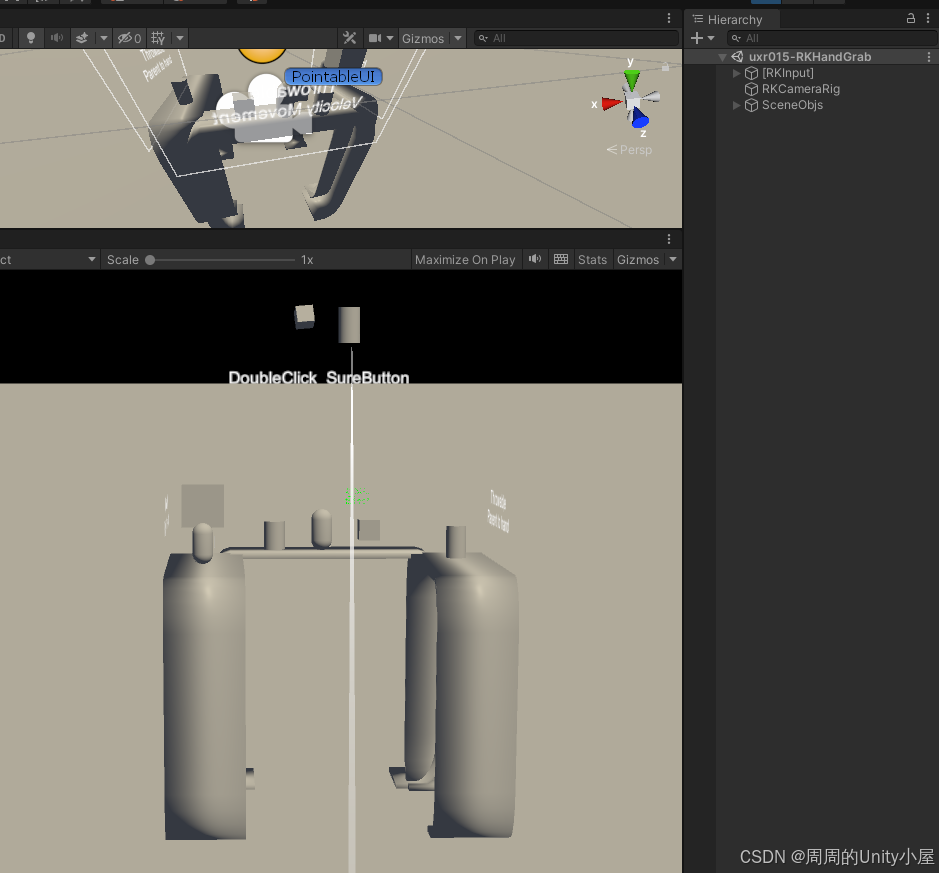
深入了解Rokid UXR2.0 SDK内置的Unity AR Glass开发组件
本文将了解到Rokid AR开发组件 一、RKCameraRig组件1.脚本属性说明2.如何使用 二、PointableUI组件1.脚本属性说明2.如何使用 三、PointableUICurve组件1.脚本属性说明2.如何使用 四、RKInput组件1.脚本属性说明2.如何使用 五、RKHand组件1.脚本属性说明2.如何使用3.如何禁用手…...
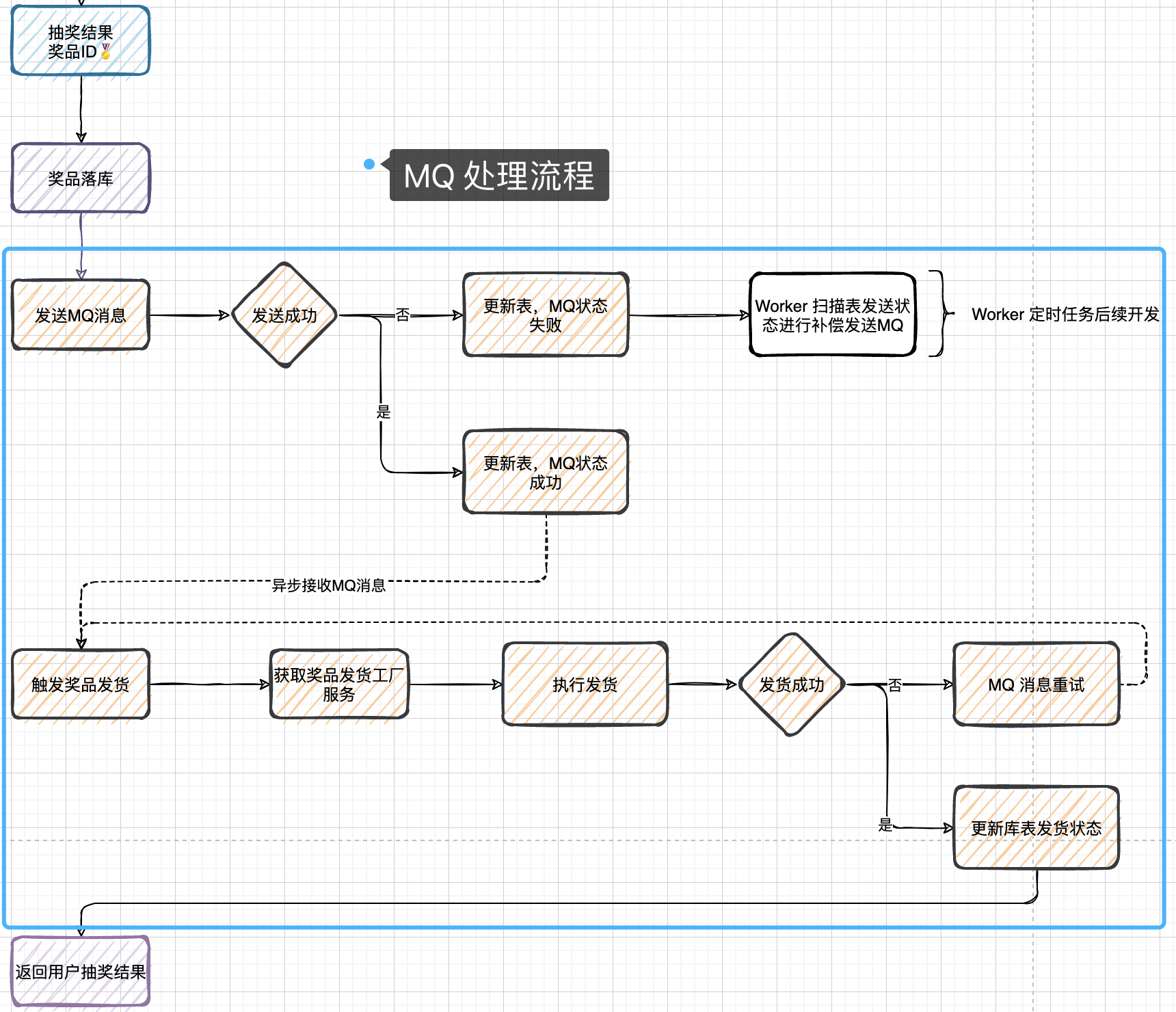
Lottery 分布式抽奖(个人向记录总结)
1.搭建(DDDRPC)架构 DDD——微服务架构(微服务是对系统拆分的方式) (Domain-Driven Design 领域驱动设计) DDD与MVC同属微服务架构 是由Eric Evans最先提出,目的是对软件所涉及到的领域进行建…...
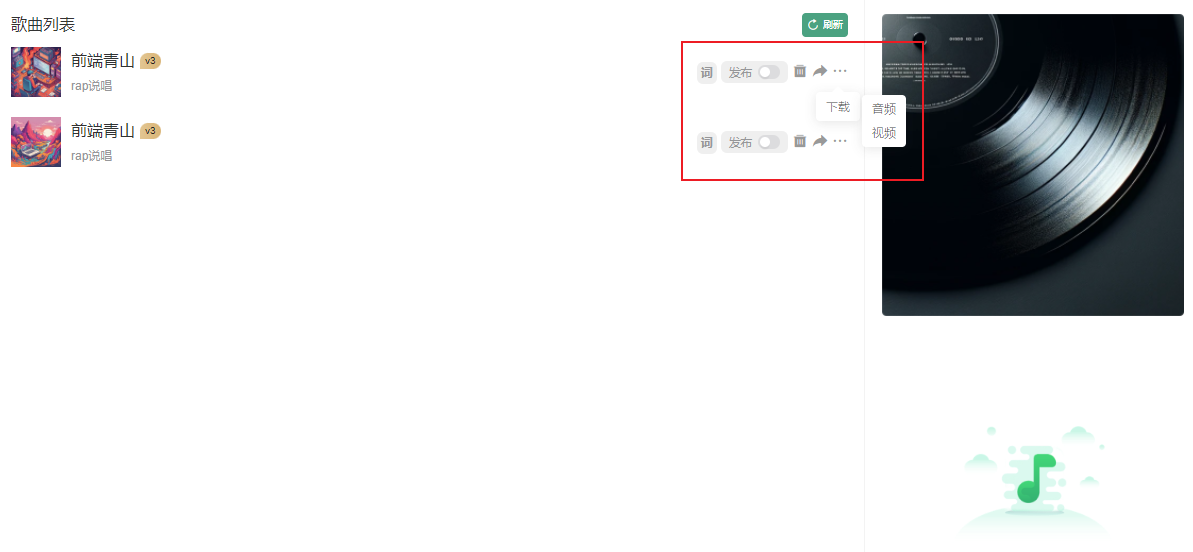
我的AI音乐梦:ChatGPT帮我做专辑
🌈个人主页:前端青山 🔥系列专栏:AI篇 🔖人终将被年少不可得之物困其一生 依旧青山,本期给大家带来ChatGPT帮我做音乐专辑 嘿,朋友们! 想象一下,如果有个超级聪明的机器人能帮你写…...
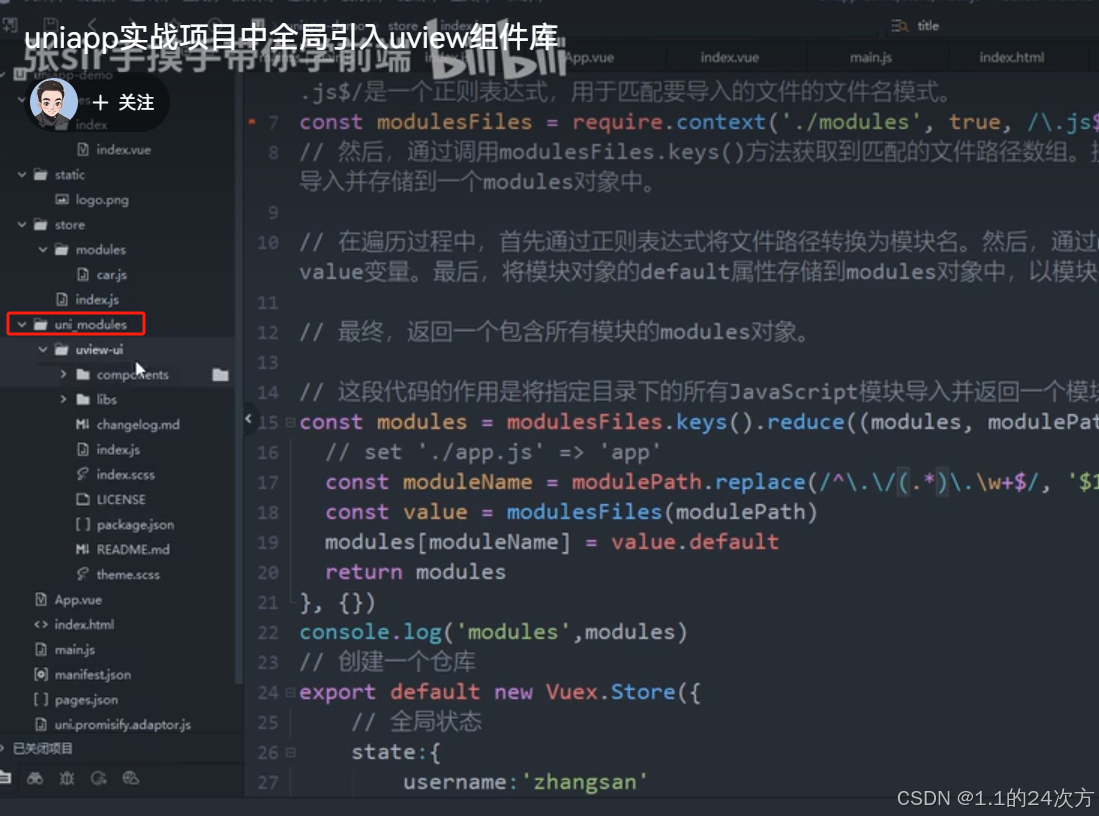
新手-前端生态
文章目录 新手的前端生态一、概念的理解1、脚手架2、组件 二、基础知识1、HTML2、css3、JavaScript 三、主流框架vue3框架 四、 工具(特定框架)1、uinapp 五、组件库()1、uView如何在哪项目中导入uView 六、应用(各种应…...

C#中的类
声明类 public class MyClass{ } 注意 类里面 的属性可以输入prop之后再按Tab键 然后再按Tab进行修改属性的名称等等 Random rnd new Random(); int arnd.Next(3); 范围是0-3的整数 但是不包含3 Random rnd new Random(); int arnd.Next(2,3); 只包含2一个数 int?[]…...
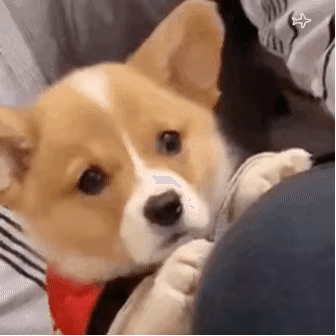
探索数据库编程:基础与进阶之存储函数
引言❤️❤️ 数据库存储过程是一组为了执行特定功能的SQL语句集合,它被存储在数据库中,可以通过指定存储过程的名称并给出相应的参数来调用。使用存储过程可以提高数据库操作的效率,减少网络传输量,并且可以封装复杂的逻辑。 编…...
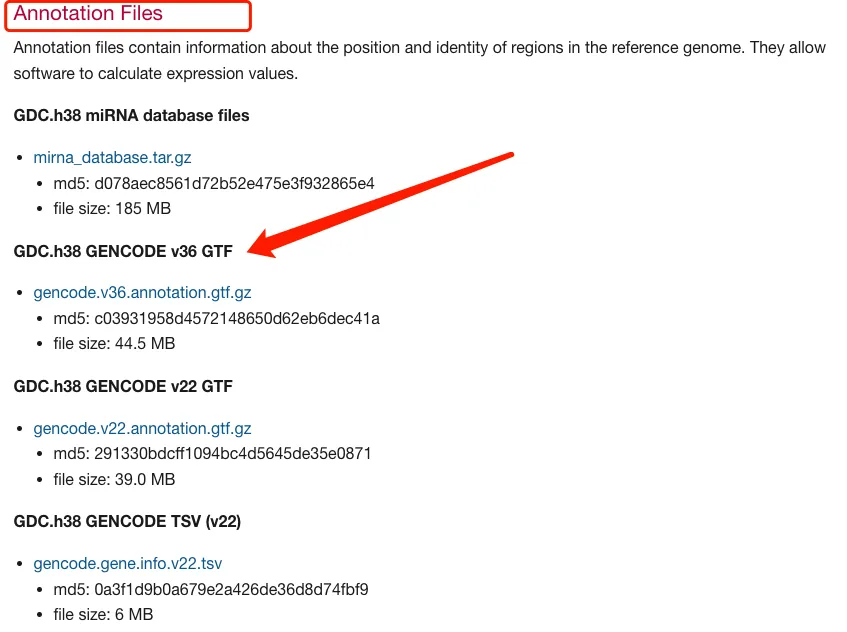
Count数据转换为TPM数据方法整理-常规方法、DGEobj.utils和IOBR包
在正式分析之前,对于数据的处理是至关重要的,这种重要性是体现在很多方面,其中有一点是要求分析者采用正确的数据类型。 对于芯片数据,原始数据进行log2处理之后可以进行很多常见的分析,比如差异分析、热图、箱线图、…...
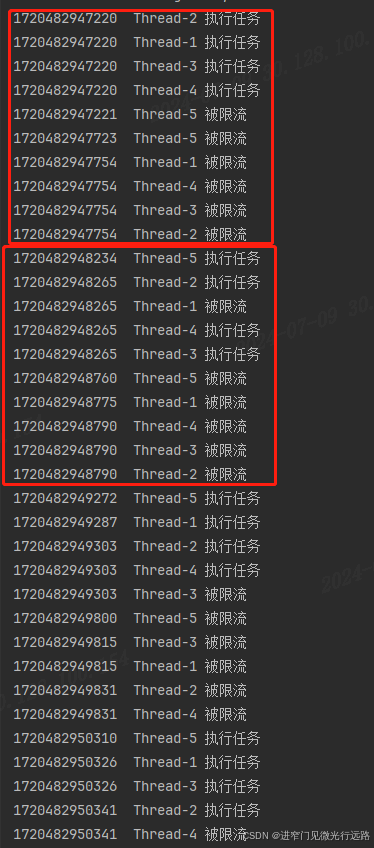
简易限流实现
需求描述 写一个1秒两个的限流工具类,2r/s 使用semaphore 代码实现-类似令牌桶算法 public class LimitHelper {private int maxLimit;private Semaphore semaphore;private int timeoutSeconds;public LimitHelper(int maxLimit, int timeoutSeconds) {this.max…...
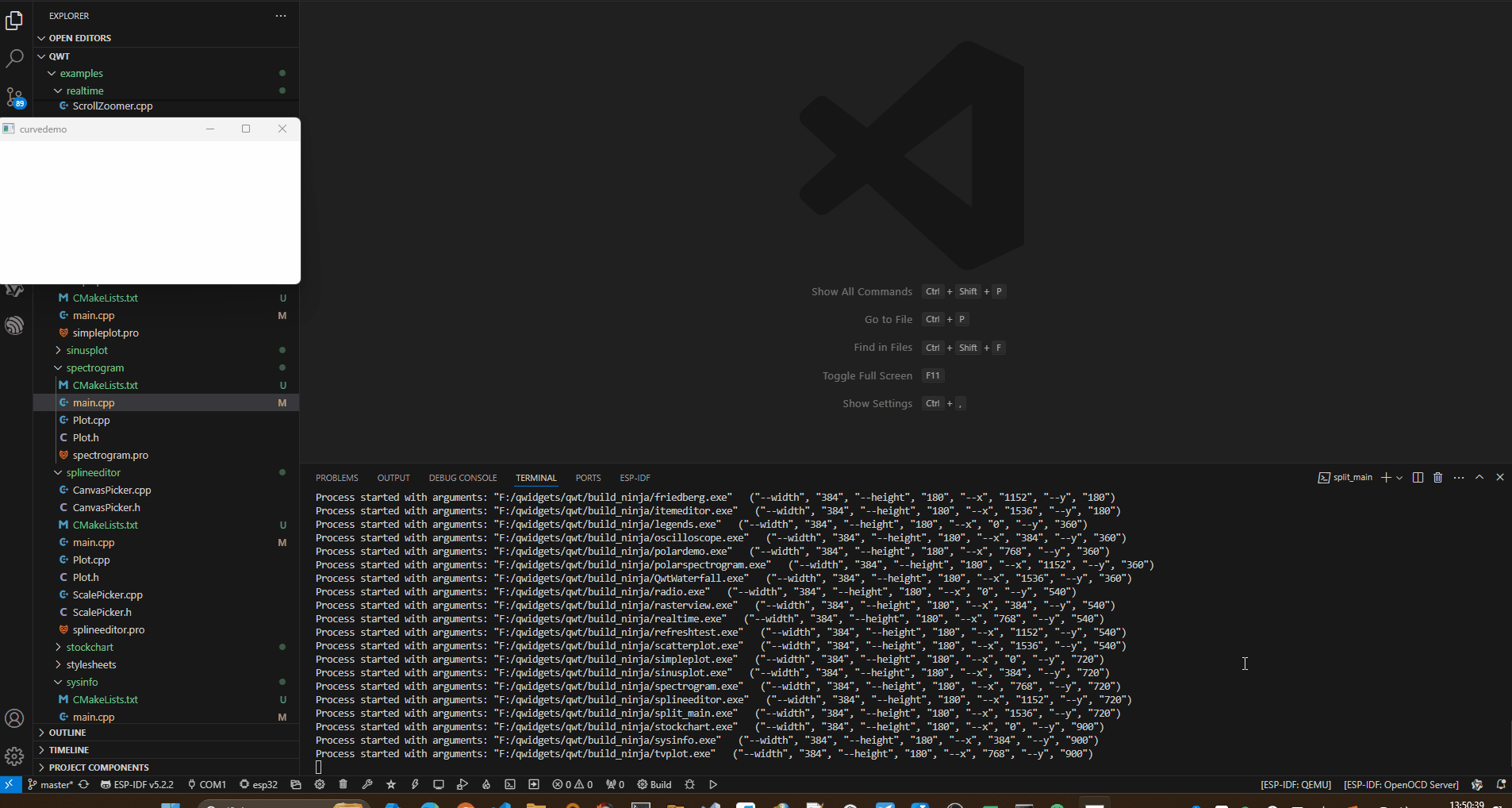
用Qwt进行图表和数据可视化开发
目录 Qwt介绍 示例应用场景 典型QWT开发流程 举一些Qwt的例子,多绘制几种类型的图像 1. 绘制折线图 (Line Plot) 2. 绘制散点图 (Scatter Plot) 3. 绘制柱状图 (Bar Plot) 4. 绘制直方图 (Histogram) Qwt介绍 QWT开发主要涉及使用QWT库进行图表和数据可视化…...

sqlalchemy使用with_entities返回指定数据列
sqlalchemy使用with_entities返回指定数据列 在 SQLAlchemy 中,with_entities 方法用于指定查询语句返回的实体(Entity)或列(Column)。它允许你限制查询的返回结果,只包含你感兴趣的特定字段或实体 使用方法 假设有一个名为 User 的 SQLAlchemy 模型类,包含以下字段:…...
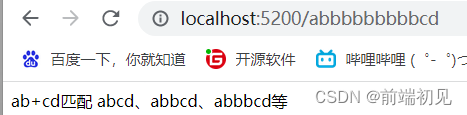
express
文章目录 🟢 Express⭐️ 1.初始Express✨安装✨使用Express 搭建一台服务器⭐️2.Express-基本路由✨1.使用字符串模式的路由路径示例:✨2.使用正则表达式的路由路径示例:✨3.中间件浅试(demo)⭐️3.Express-中间件✨1.应用级中间件✨2.路由级中间件✨3.错误处理中间件✨4…...
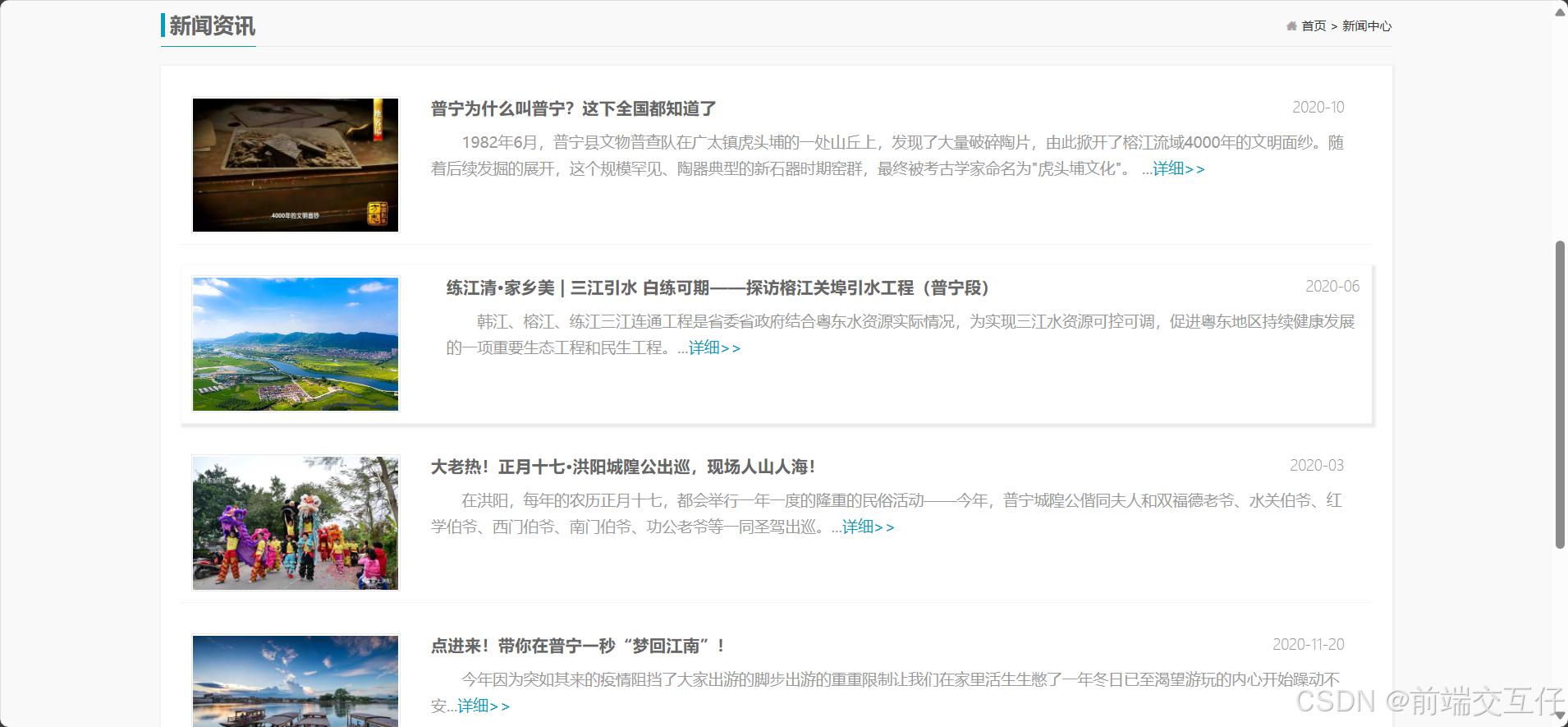
HTML网页大设计-家乡普宁德安里
代码地址: https://pan.quark.cn/s/57e48c3b3292...

深度学习:从数据采集到模型测试的全面指南
摘要 随着人工智能和大数据技术的迅猛发展,深度学习已成为解决复杂问题的有力工具。然而,从项目启动到模型部署,包含了数据处理和模型研发的多个环节,每个环节的细致和严谨性直接决定了最终模型的性能和可靠性。本论文详细探讨了…...

Excel第29享:基于sum嵌套sumifs的多条件求和
1、需求描述 如下图所示,现要统计12.17-12.23这一周各个人员的“上班工时(a1)”。 下图为系统直接导出的工时数据明细样例。 2、解决思路 首先,确定逻辑:“对多个条件(日期、人员)进行“工时”…...
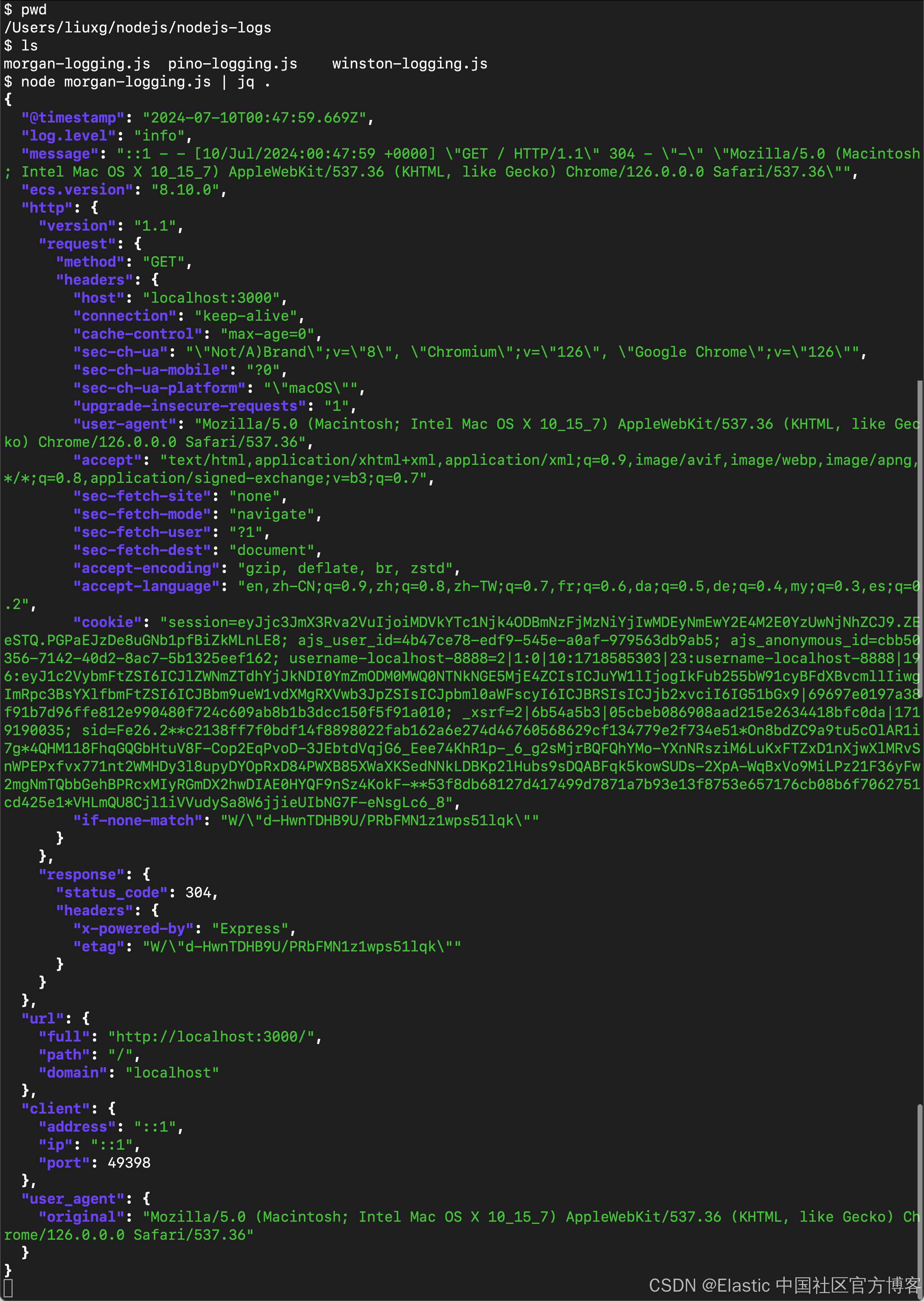
Elasticsearch:Node.js ECS 日志记录 - Morgan
这是之前系列文章: Elasticsearch:Node.js ECS 日志记录 - Pino Elasticsearch:Node.js ECS 日志记录 - Winston 中的第三篇文章。在今天的文章中,我将描述如何使用 Morgan 包针对 Node.js 应用进行日子记录。此 Morgan Node.j…...

ChatGPT对话:Python程序自动模拟操作网页,无法弹出下拉列表框
【编者按】需要编写Python程序自动模拟操作网页。编者有编程经验,但没有前端编程经验,完全不知道如何编写这种程序。通过与ChatGPT讨论,1天完成了任务。因为没有这类程序的编程经验,需要边学习,边编程,遇到…...

Unity 之 抖音小游戏集成排行榜功能详解
Unity 之 抖音小游戏集成排行榜功能详解 一,前言1.1 为游戏设计利于传播的元素2.2 多人竞技、社交传播二,集成说明2.1 功能介绍2.2 完整代码2.3 效果展示三,发现的问题和迭代计划一,前言 对于 Unity 开发者而言,在开发抖音小游戏时集成排行榜功能是提升游戏社交性和玩…...

网站建设后怎么/注册网址
1.HashSet存储字符串并遍历 * 特点:无序、无索引、无重复 HashSet存储字符串并遍历HashSet<String> hs new HashSet<>();hs.add("a");hs.add("b");hs.add("a");hs.add("c");hs.add("c");hs.add(&qu…...

公司年前做网站好处/竞价系统
nginx日志格式log_format access $remote_addr - [$time_local] "$request" $status $body_bytes_sent $request_time "$http_referer" "$http_user_agent" - $http_x_forwarded_for;以下是nginx的日志正则提取格式log_pattern r^(?P.*?) - \…...
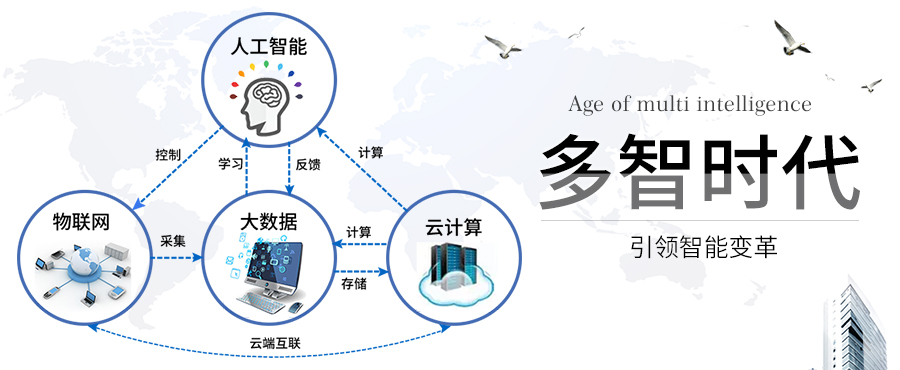
找网站开发项目/友链大全
如何理解“分布式”? 经常听到”分布式系统“,”分布式计算“,”分布式算法“。分布式的具体含义是什么?狭义的分布是指,指多台PC在地理位置上分布在不同的地方。 分布式——一个高大上的名词,是计算机软件…...

成都网站建设scwbo/陕西网站设计
前: New Relic的上市使得IT和资本界开始重新重视APM,当然跟传统APM相比,New Relic还是有相当的创新,另外还有一点是目前的创业潮导致的企业级需求增大。 In recent years, IT projects seem to have stopped asking “which APM s…...

武汉网络科技有限公司排名/福州seo按天收费
话说使用Redis已经有好一段时间,趁有点时间,结合Guang.com 使用经验,总结一下Redis 在社会化电商网站的实际应用场景。文笔较差,各位看官,凑合着看下吧。 1. 各种计数,商品维度计数和用户维度计数 说起电商…...
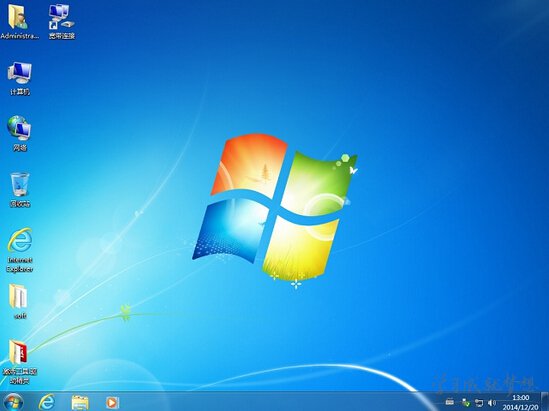
邳州做网站的公司/b2b网站免费推广
于戴尔笔记本怎么重装系统呢?其实关于戴尔笔记本怎么重装系统的方法小编也是说过的了,但是还是有不少的用户不知道戴尔笔记本电脑怎么重装系统,接下来是小编为大家收集的dell2900如何重装系统,欢迎大家阅读。dell2900如何重装系统第一步、设…...