【Karapathy大神build-nanogpt】Take Away Notes
B站翻译LINK
Personal Note
Andrej rebuild gpt2 in pytorch.
Take Away Points
- Before entereing serious training, he use Shakespear’s work as a small debugging datset to see if a model can overfit. Overfitging is a should thing.
- If we use TF32 or BF32, (by default is FP32 in pytorch it takes more memory), gpu can do hundred times faster. Becuase the core is computing really fast, most time (more than 40%) it is waiting for the memory allocation/transfer… Every computation is done in a manner like breaking down to 4x4 matrix multiplication.
- when time a gpu programing, remember torch.cuda.synchronize()
- watch gpu: watch -n 0.1 nvidia-smi
- torch.set_float32_matmul_precision(‘high’) easily activate tf32 mode
- default is highest -> float 32
- High-> if avaible tensorflow32 (depends on GPU)
- simply use torch.set_float32_matmul_precision(‘high’) theoraticlaly should make us have 8x speed. However we only achieve 3x. Becuse we are still memory bound, moving data around still cost a lot.
- This can only be used in Amphere:
use torch.autocast(device_type=device,dtype=torch.bfloat16) to wrap the forward process. In this wrap, some CUDA ops can autocast to BF16, many other stays in float32. Matrix Multiplication will be BF16. - One debug technique: import code; code.interact(local=locals())
- torch.compile! Model = torch.compile(model)
- Flash Attention. Flash Attention2. Online softmax.
Use F.scale_dot_product_attention(q,k,v,is_causal=True) instead - Look for ugly numbers, make it to beautiful numbers. Any ugly numbers->increase it to have as much as 2 (Although flops will increase, time will decrease)
- ALL ABOVE CHANGES MAKE PRAGRAM TRAINING 10x FASTER!!
- Linear_warmup + cosine learning rate with minimum learning rate, see GPT3 paper for more details
- First stage of the training, the model is not differing each tokens, they are just learning which tokens can show up which are not and driving them probability to zero. It is the reason that why in the early training stage, a small batchsize will be OK, as the gradients will not behave different if you use full batchsize.
- parameters that should be weight decayed and should not be. WD: all weight tensors + embeddings (p.dim()>=2), NWD: all biaes, layernorms (p.dim()<2)
- AdamW’s use_fused configuration (accelarate training process)
- Model size up, lr down, batchsize up.
- Grad accumulation: Remember to normalize: loss /= grad_accum_steps
- when evaluation, use torch.Generator to create object used in torch.multinomial(xx,xx,generator=.), so that the generating process do not impact the global random number generator used for training.
- However, torch.compile must be banned, so that you can sample in the training process.
CODES FOR DDP (SAMPLE)
# torchrun --stand_alone --nproc_per_node=<num_gpu_per_node> <your_training_script.py> <script_arguments>
# Above only applies for single node training.# SETTINGS FOR EACH DIFFERENT RANK
ddp = int(os.environ.get('RANK',-1))!=-1
if ddp:assert torch.cuda.is_available()init_process_group(backend='nccl')ddp_rank = int(os.environ['RANK']) # It is a global rank, for each process it has a unique ddp_rankddp_local_rank = int(os.environ['LOCAL_RANK']) # It is a local rank in the local machine (node)ddp_world_size = int(os.environ['WORLD_SIZE']) # How many gpus (processes) in totaldevice = f'cuda:{ddp_local_rank}'torch.cuda.set_device(device)master_process = ddp_rank == 0
else:ddp_rank = 0ddp_local_rank = 0ddp_world_size = 1master_process = Truedevice = "cpu"if torhc.cuda.is_available():device = "cuda"elif hasattr(torch.backends,"mps") and torch.bakends.mps.is_available():device = "mps"print(f"using device:{device}")# IF YOU USE GRAD ACCUMULATION
total_batch_size = 524288 # batch size measured in token numbers
B = 16 # micro batch for each process
T = 1024 # sequence length
assert total_batch%(B * T * ddp_world_size) == 0
grad_accum_steps = total_batch_size // (B * T * ddp_world_size)# SET DATALOADER
Dataloader = DataLoader(*args, ddp_world_size, ddp_rank) # MUST! make each process deal with different part of datset# CREATE MODEL
model = createmodel()
model.to(device)
model = torch.compile(model)
if ddp:model = DDP(model,device_ids=[ddp_local_rank]) # this must be ddp_local_rank not ddp_rank
raw_model = model.module if ddp else model# FIX SEED
seed = 'YOUR LUCKY NUMBER'
torch.mannual_seed(seed)
if torch.cuda.is_available():torch.cuda.manual_seed(seed)# TRAIN
for step in range(max_steps):t0 = time.time() model.train()optimizer.zero_grad()loss_accum = 0.0for micro_step in range(grad_accum_steps):x,y = Dataloader.next_batch()x,y = x.to(device),y.to(device)with torch.autocast(device_type=device,dtype=torch.bfloat16):logits, loss = model(x,y)loss = loss / grad_accum_stepsloss_accum += loss.detach()if ddp:model.require_backward_grad_sync = (micro_step == grad_accum_steps - 1) # The ddp sync if applied to every micro step will be wasting time. So only the last backward in one accum cycle should be synchronized. See ddp.no_sync() contextmanager for official advice. Or use it in this way shown here.loss.backward() if ddp:torch.distributed.all_reduce(loss_accum,op=torch.distributed.ReduceOp.AVG)
norm = torch.nn.utils.clip_grad_norm_(model.parameters(),1.0)if step%100 == 0:# start evaluationmodel.eval()with torch.no_grad():# SOME EVALUATION CODE
if ddp:destroy_process_group()
相关文章:
【Karapathy大神build-nanogpt】Take Away Notes
B站翻译LINK Personal Note Andrej rebuild gpt2 in pytorch. Take Away Points Before entereing serious training, he use Shakespear’s work as a small debugging datset to see if a model can overfit. Overfitging is a should thing.If we use TF32 or BF32, (by…...

MySQL学习记录 —— 이십이 MySQL服务器日志
文章目录 1、日志介绍2、一般、慢查询日志1、一般查询日志2、慢查询日志FILE格式TABLE格式 3、错误日志4、二进制日志5、日志维护 1、日志介绍 中继服务器的数据来源于集群中的主服务。每次做一些操作时,把操作保存到重做日志,这样崩溃时就可以从重做日志…...
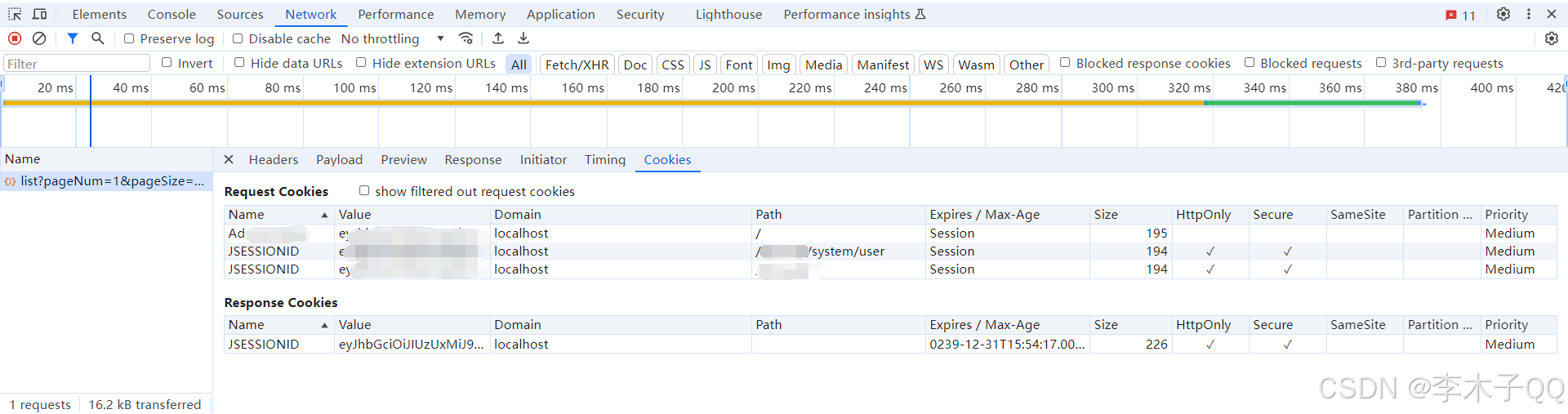
HTTPS请求头缺少HttpOnly和Secure属性解决方案
问题描述: 建立Filter拦截器类 package com.ruoyi.framework.security.filter;import com.ruoyi.common.core.domain.model.LoginUser; import com.ruoyi.common.utils.SecurityUtils; import com.ruoyi.common.utils.StringUtils; import com.ruoyi.framework.…...
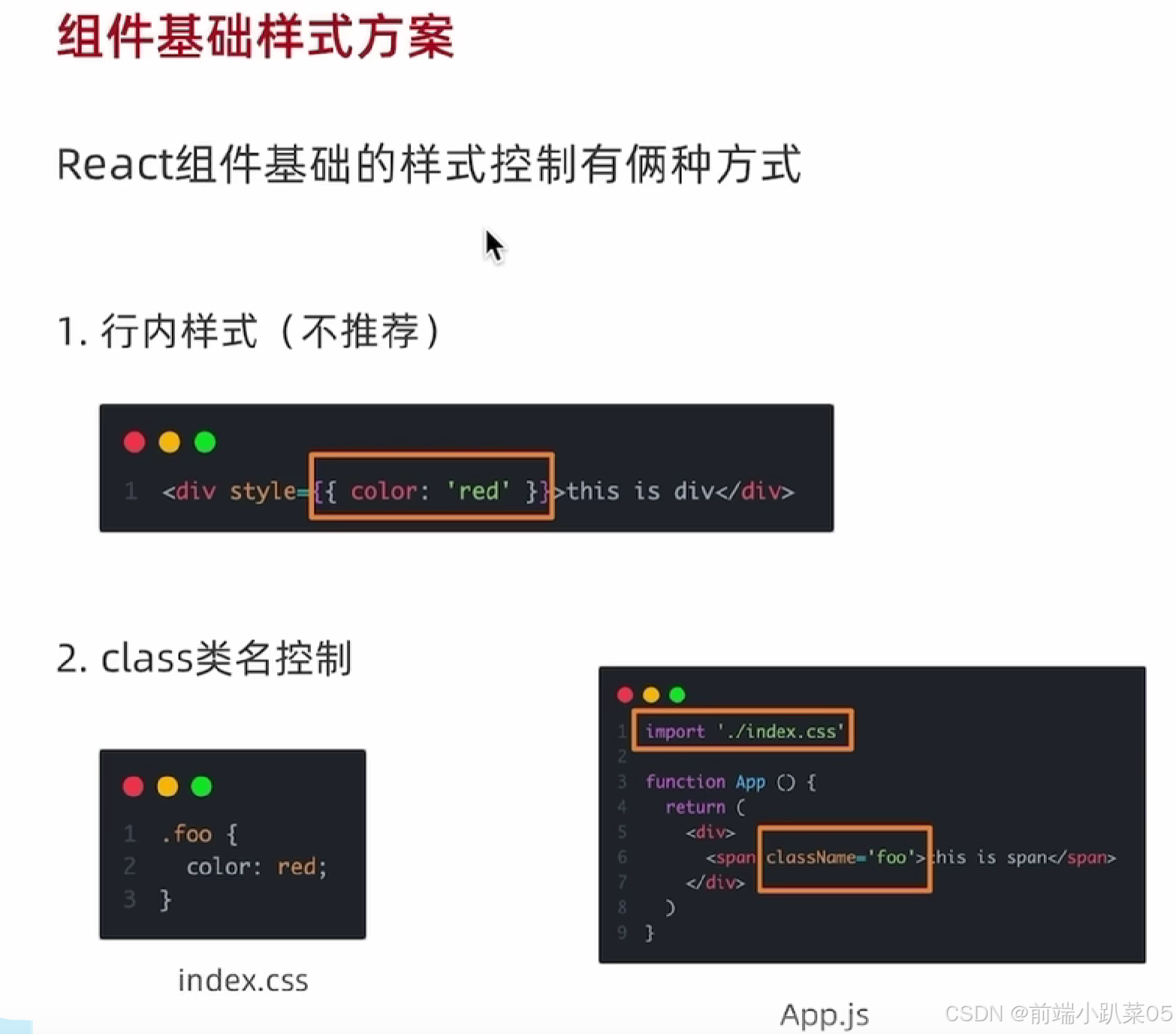
react基础样式控制
行内样式 <div style{{width:500px, height:300px,background:#ccc,margin:200px auto}}>文本</div> class类名 注意:在react中使用class类名必须使用className 在外部src下新建index.css文件写入你的样式 .fontcolor{color:red } 在用到的页面引入…...
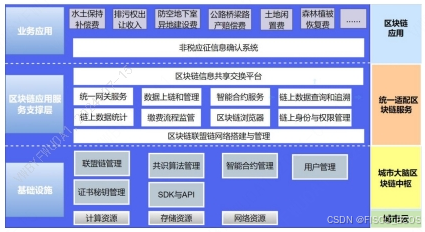
【区块链 + 智慧政务】涉税行政事业性收费“e 链通”项目 | FISCO BCOS应用案例
国内很多城市目前划转至税务部门征收的非税收入项目已达 17 项,其征管方式为行政主管部门核定后交由税务 部门征收。涉税行政事业性收费受限于传统的管理模式,缴费人、业务主管部门、税务部门、财政部门四方处于 相对孤立的状态,信息的传递靠…...
Socket、WebSocket 和 MQTT 的区别
Socket 协议 定义:操作系统提供的网络通信接口,抽象了TCP/IP协议,支持TCP和UDP。特点: 通用性:不限于Web应用,适用于各种网络通信。协议级别:直接使用TCP/UDP,需要手动管理连接和数…...
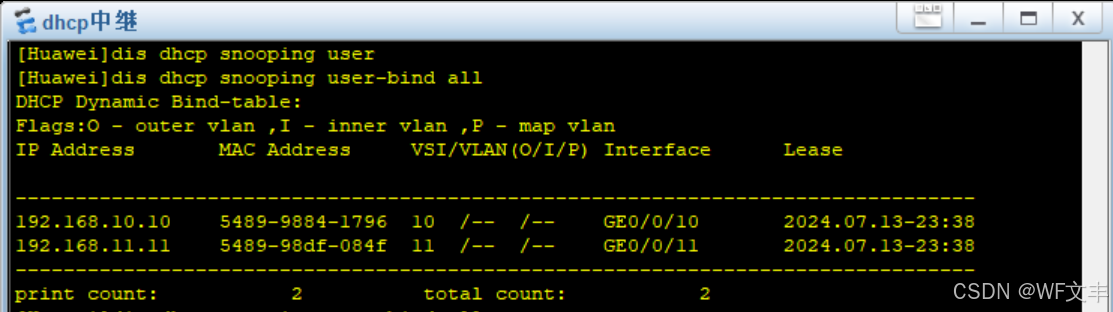
企业网络实验(vmware虚拟机充当DHCP服务器)所有IP全部保留,只为已知mac分配固定IP
文章目录 需求实验修改dhcp虚拟机配置文件测试PC获取IP查看user-bind 需求 (vmware虚拟机充当DHCP服务器)所有IP全部保留,只为已知mac分配固定IP 实验 前期配置: https://blog.csdn.net/xzzteach/article/details/140406092 后续配置均在以上配置的前…...
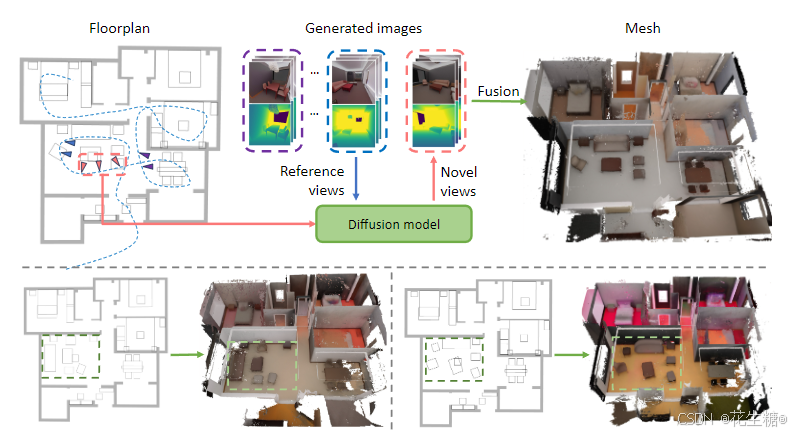
HouseCrafter:平面草稿至3D室内场景的革新之旅
在室内设计、房地产展示和影视布景设计等领域,将平面草稿图快速转换为立体的3D场景一直是一个迫切的需求。HouseCrafter,一个创新的AI室内设计方案,正致力于解决这一挑战。本文将探索HouseCrafter如何将这一过程自动化并提升至新的高度。 一、定位:AI室内设计的革新者 Ho…...
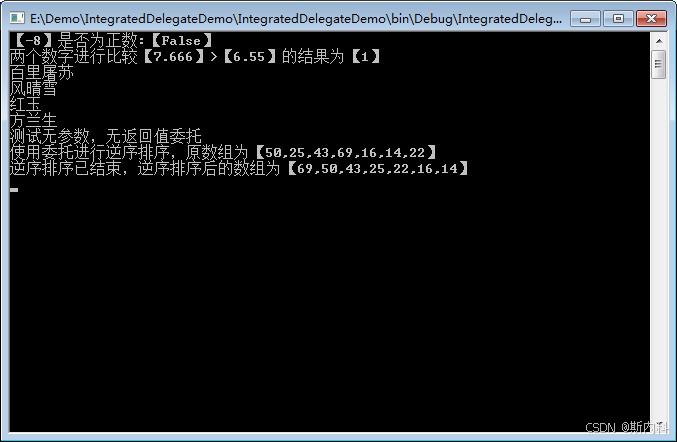
C#统一委托Func与Action
C#在System命名空间下提供两个委托Action和Func,这两个委托最多提供16个参数,基本上可以满足所有自定义事件所需的委托类型。几乎所有的 事件 都可以使用这两个内置的委托Action和Func进行处理。 Action委托: Action定义提供0~16个参数&…...
MongoDB 基本查询语句
基本查询 查询所有文档: db.collection.find()示例: db.users.find()按条件查询文档: db.collection.find({ key: value })示例: db.users.find({ age: 25 })查询并格式化输出: db.collection.find().pretty()示例&…...
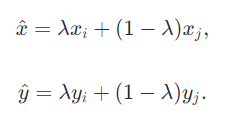
28_EfficientNetV2网络详解
V1:https://blog.csdn.net/qq_51605551/article/details/140487051?spm1001.2014.3001.5502 1.1 简介 EfficientNetV2是Google研究人员Mingxing Tan和Quoc V. Le等人在2021年提出的一种深度学习模型,它是EfficientNet系列的最新迭代,旨在提…...

PyCharm查看文件或代码变更记录
背景: Mac笔记本上有一个截图的定时任务在运行,本地Python使用的是PyCharm IDE,负责的同事休假,然后定时任务运行的结果不符合预期,一下子不知道问题出现在哪里。 定位思路: 1、先确认网络、账号等基本的…...
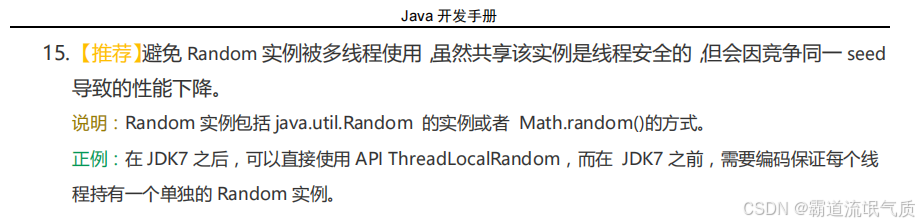
Java开发手册中-避免Random实例被多线程使用、多线程下Random与ThreadLoacalRandom性能对比
场景 Java中使用JMH(Java Microbenchmark Harness 微基准测试框架)进行性能测试和优化: Java中使用JMH(Java Microbenchmark Harness 微基准测试框架)进行性能测试和优化_java热点函数-CSDN博客 参考以上性能测试工具的使用。 Java开发手册中有这样一条…...
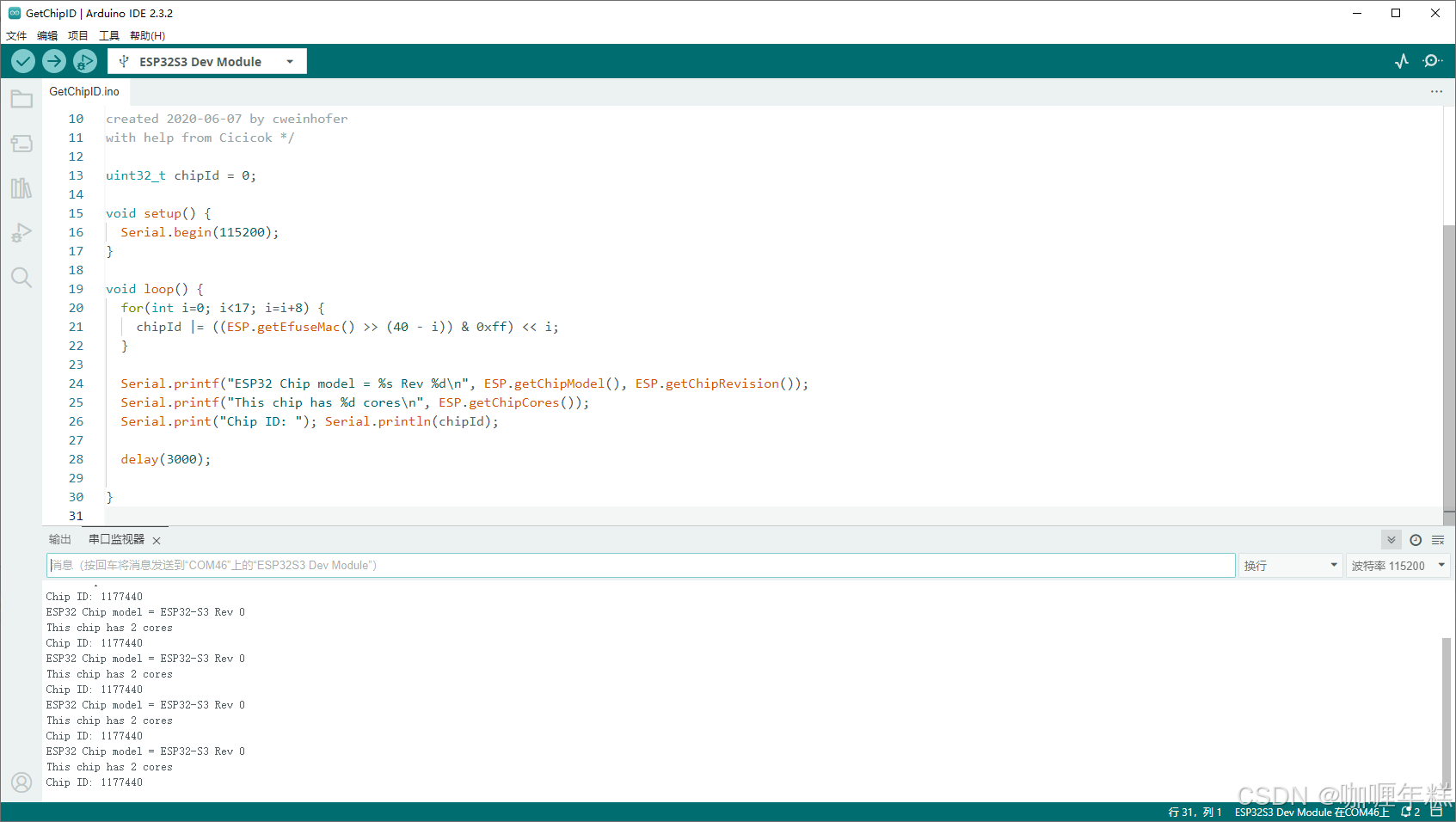
【Arduino IDE】安装及开发环境、ESP32库
一、Arduino IDE下载 二、Arduino IDE安装 三、ESP32库 四、Arduino-ESP32库配置 五、新建ESP32-S3N15R8工程文件 乐鑫官网 Arduino官方下载地址 Arduino官方社区 Arduino中文社区 一、Arduino IDE下载 ESP-IDF、MicroPython和Arduino是三种不同的开发框架,各自适…...
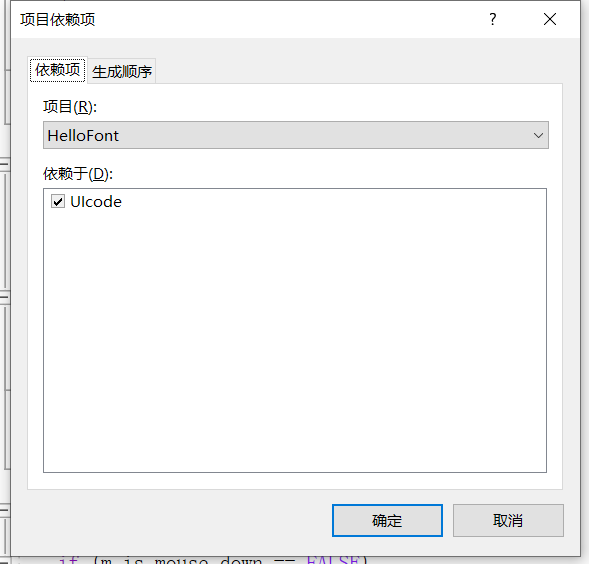
【C++开源】GuiLite:超轻量UI框架-入门
开发环境说明 使用visual Studio 2022进行开发 下载源码 从如下的网址进行源码和示例代码的下载: GitHub源码网址为:idea4good/GuiLite示例代码路径为:idea4good/GuiLiteExample使用方法 GuiLite是一个仅有头文件的一个库,使用的时候直接include到自己的UIcode.cpp文件…...
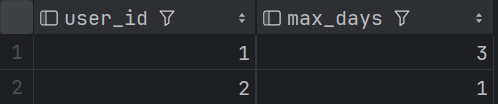
SQL面试题练习 —— 查询每个用户最大连续登录天数
目录 1 题目2 建表语句3 题解 1 题目 查询每个用户最大连续登录天数 样例数据如下 login_log: 2 建表语句 --建表语句 create table if not exists login_log (user_id int comment 用户id,login_time date comment 登录时间 ); --数据插入 INSERT overwrit…...
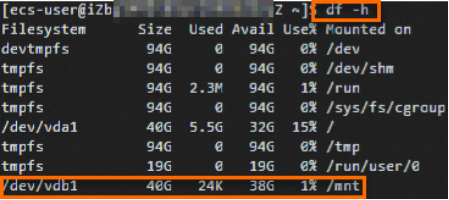
服务器系统盘存储不够,添加数据盘并挂载(阿里云)
目录 1.获取数据盘设备名称 2.为数据盘创建分区 3.为分区创建文件系统 4.配置开机自动挂载分区 阿里云数据盘挂载说明链接:在Linux系统中初始化小于等于2 TiB的数据盘_云服务器 ECS(ECS)-阿里云帮助中心 1.获取数据盘设备名称 sudo fdisk -lu 运行结果如下所示…...
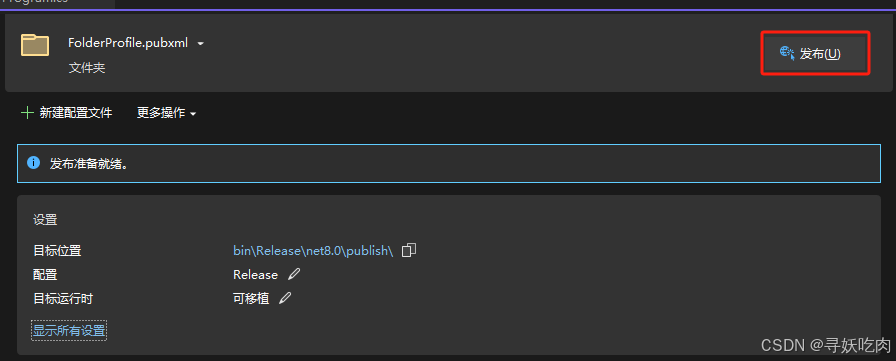
Visual Studio2022中使用.Net 8 在 Windows 下使用 Worker Service 创建守护进程
Visual Studio2022中使用.Net 8 在 Windows 下创建 Worker Service 1 什么是 .NET Core Worker Service1.1 确认Visual Studio中安装了 ASP.NET和Web开发2 创建 WorkerService项目2.1 新建一个WorkerService项目2.2 项目结构说明3 将应用转换成 Windows 服务3.1 安装Microsoft.…...
HTML5应用的安全防护策略与实践
随着HTML5及其相关技术(如CSS3和JavaScript)的普及,Web应用变得越来越强大和复杂,同时也成为黑客攻击的目标。本文将探讨HTML5应用面临的常见安全威胁,以及如何通过最佳实践和代码示例来增强应用的安全性。 HTML5安全…...
堆叠和集群
堆叠和集群 堆叠/集群:把多条/两台设备通过线缆进行连接,逻辑上组成一台设备,作为应该整体来管 理和转发流量 堆叠和集群的区别 1. 何时设备支持对贴,框式设备支持集群 2. 堆叠可以支持多台,框式只能支持两台 堆…...
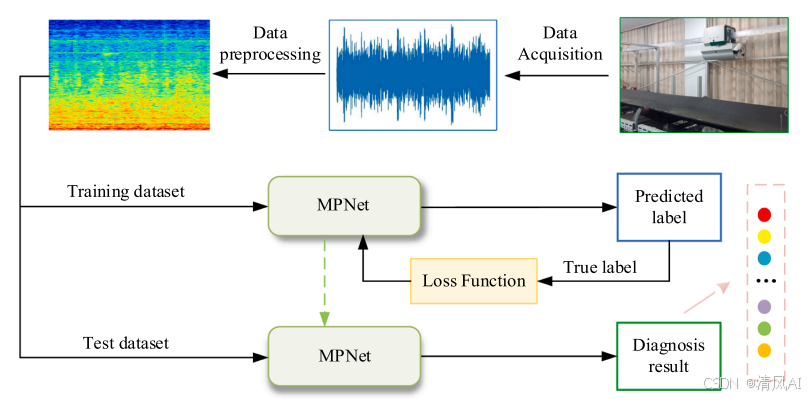
MPNet:旋转机械轻量化故障诊断模型详解python代码复现
目录 一、问题背景与挑战 二、MPNet核心架构 2.1 多分支特征融合模块(MBFM) 2.2 残差注意力金字塔模块(RAPM) 2.2.1 空间金字塔注意力(SPA) 2.2.2 金字塔残差块(PRBlock) 2.3 分类器设计 三、关键技术突破 3.1 多尺度特征融合 3.2 轻量化设计策略 3.3 抗噪声…...

调用支付宝接口响应40004 SYSTEM_ERROR问题排查
在对接支付宝API的时候,遇到了一些问题,记录一下排查过程。 Body:{"datadigital_fincloud_generalsaas_face_certify_initialize_response":{"msg":"Business Failed","code":"40004","sub_msg…...
进程地址空间(比特课总结)
一、进程地址空间 1. 环境变量 1 )⽤户级环境变量与系统级环境变量 全局属性:环境变量具有全局属性,会被⼦进程继承。例如当bash启动⼦进程时,环 境变量会⾃动传递给⼦进程。 本地变量限制:本地变量只在当前进程(ba…...
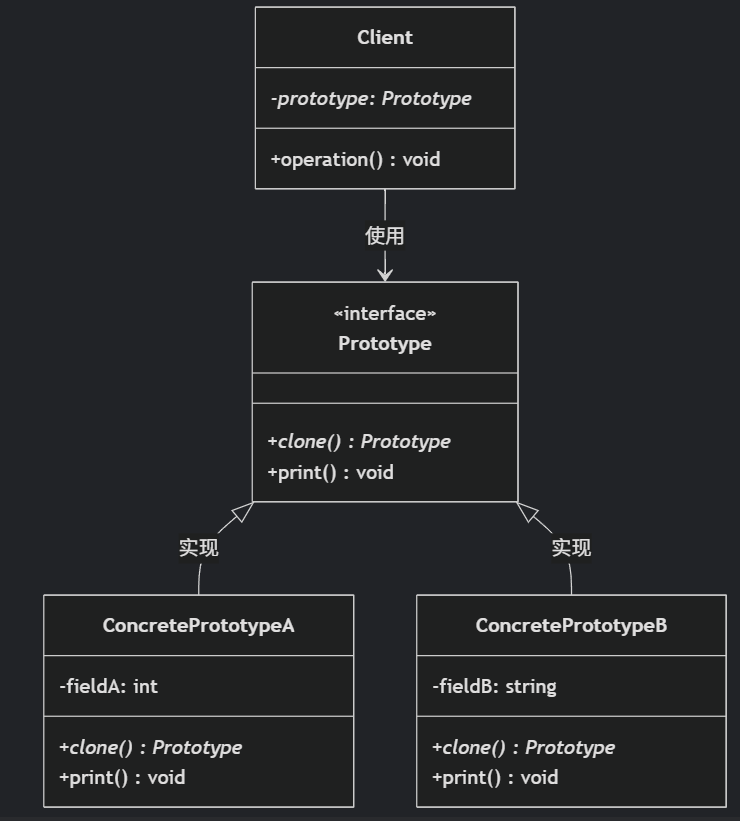
(二)原型模式
原型的功能是将一个已经存在的对象作为源目标,其余对象都是通过这个源目标创建。发挥复制的作用就是原型模式的核心思想。 一、源型模式的定义 原型模式是指第二次创建对象可以通过复制已经存在的原型对象来实现,忽略对象创建过程中的其它细节。 📌 核心特点: 避免重复初…...
基于matlab策略迭代和值迭代法的动态规划
经典的基于策略迭代和值迭代法的动态规划matlab代码,实现机器人的最优运输 Dynamic-Programming-master/Environment.pdf , 104724 Dynamic-Programming-master/README.md , 506 Dynamic-Programming-master/generalizedPolicyIteration.m , 1970 Dynamic-Programm…...
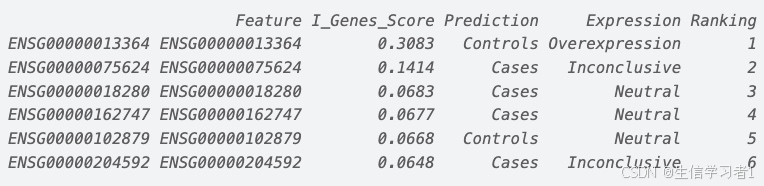
【数据分析】R版IntelliGenes用于生物标志物发现的可解释机器学习
禁止商业或二改转载,仅供自学使用,侵权必究,如需截取部分内容请后台联系作者! 文章目录 介绍流程步骤1. 输入数据2. 特征选择3. 模型训练4. I-Genes 评分计算5. 输出结果 IntelliGenesR 安装包1. 特征选择2. 模型训练和评估3. I-Genes 评分计…...
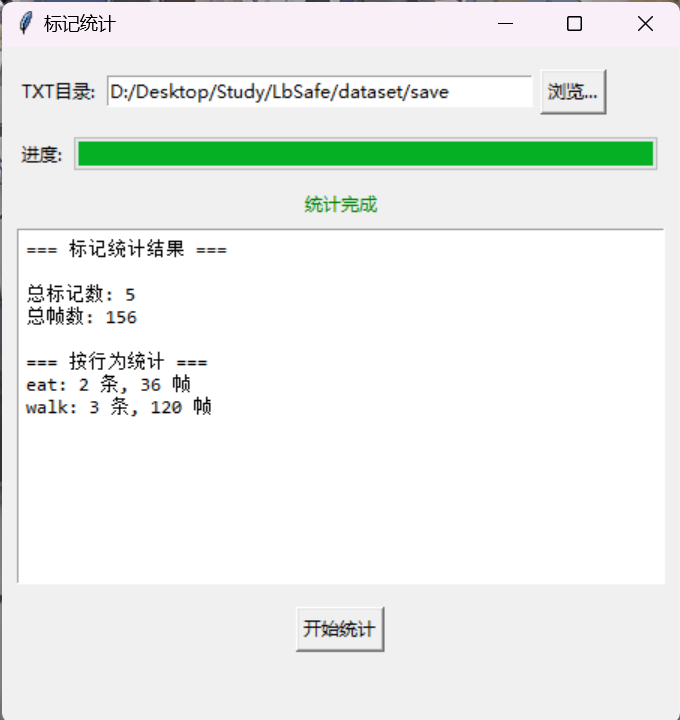
视频行为标注工具BehaviLabel(源码+使用介绍+Windows.Exe版本)
前言: 最近在做行为检测相关的模型,用的是时空图卷积网络(STGCN),但原有kinetic-400数据集数据质量较低,需要进行细粒度的标注,同时粗略搜了下已有开源工具基本都集中于图像分割这块,…...
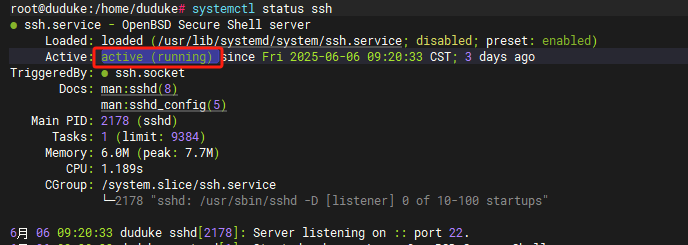
VM虚拟机网络配置(ubuntu24桥接模式):配置静态IP
编辑-虚拟网络编辑器-更改设置 选择桥接模式,然后找到相应的网卡(可以查看自己本机的网络连接) windows连接的网络点击查看属性 编辑虚拟机设置更改网络配置,选择刚才配置的桥接模式 静态ip设置: 我用的ubuntu24桌…...
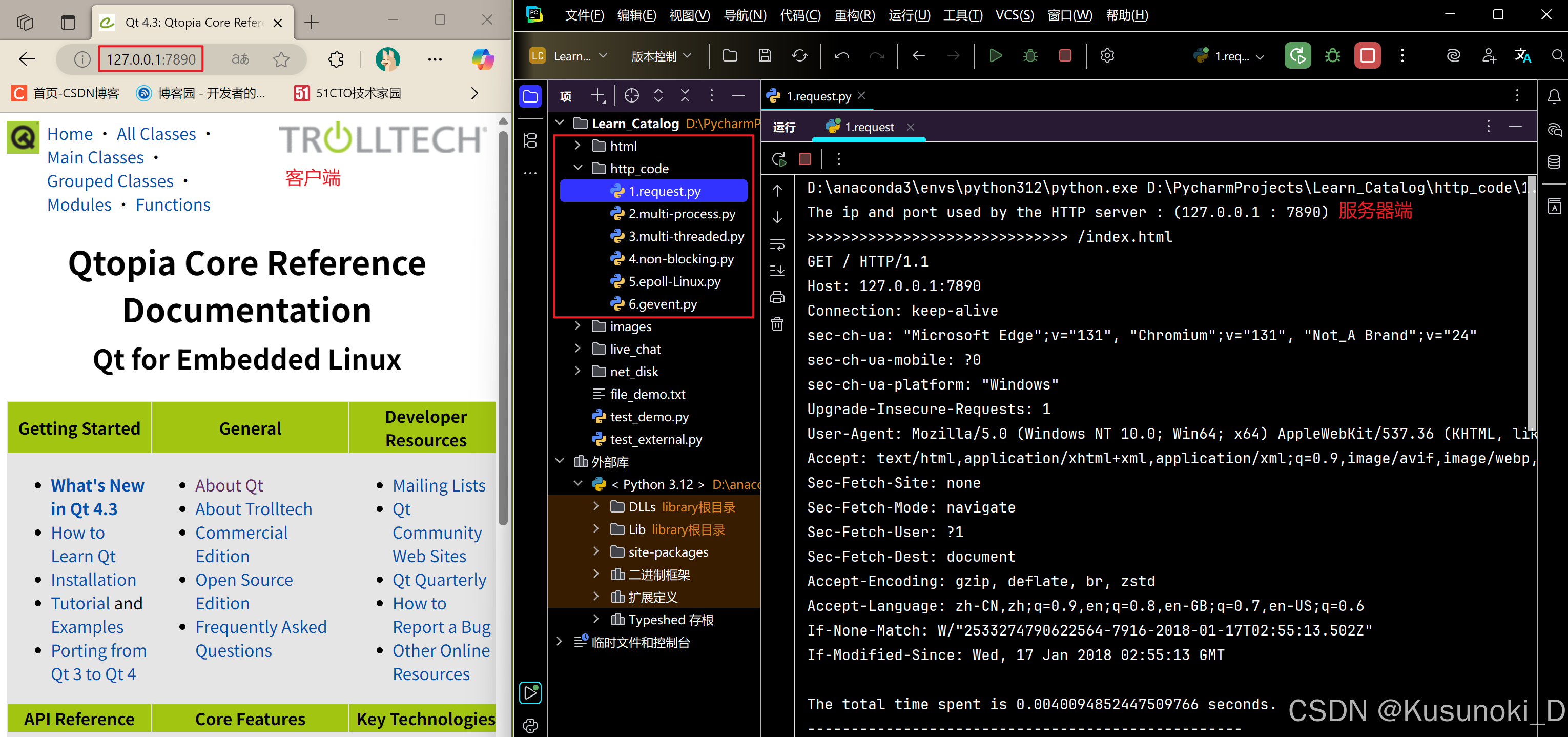
Python 实现 Web 静态服务器(HTTP 协议)
目录 一、在本地启动 HTTP 服务器1. Windows 下安装 node.js1)下载安装包2)配置环境变量3)安装镜像4)node.js 的常用命令 2. 安装 http-server 服务3. 使用 http-server 开启服务1)使用 http-server2)详解 …...
学习一下用鸿蒙DevEco Studio HarmonyOS5实现百度地图
在鸿蒙(HarmonyOS5)中集成百度地图,可以通过以下步骤和技术方案实现。结合鸿蒙的分布式能力和百度地图的API,可以构建跨设备的定位、导航和地图展示功能。 1. 鸿蒙环境准备 开发工具:下载安装 De…...