Vue3列表(List)
效果如下图:在线预览
APIs
List
参数 | 说明 | 类型 | 默认值 |
---|---|---|---|
bordered | 是否展示边框 | boolean | false |
vertical | 是否使用竖直样式 | boolean | false |
split | 是否展示分割线 | boolean | true |
size | 列表尺寸 | ‘small’ | ‘middle’ | ‘large’ | ‘middle’ |
loading | 是否加载中 | boolean | false |
hoverable | 是否显示悬浮样式 | boolean | false |
header | 列表头部 | string | slot | undefined |
footer | 列表底部 | string | slot | undefined |
spinProps | Spin 组件属性配置,参考 Spin Props,用于配置列表加载中样式 | object | {} |
emptyProps | Empty 组件属性配置,参考 Empty Props,用于配置暂无数据样式 | object | {} |
showPagination | 是否显示分页 | boolean | false |
pagination | Pagination 组件属性配置,参考 Pagination Props,用于配置分页功能 | object | {} |
ListItem
参数 | 说明 | 类型 | 默认值 |
---|---|---|---|
avatar | 列表元素的图标字符 | string | slot | undefined |
avatarProps | Avatar 组件属性配置,参考 Avatar Props,用于配置列表图标样式 | object | {} |
title | 列表元素的标题 | string | slot | undefined |
description | 列表元素的描述内容 | string | slot | undefined |
actions | 列表操作组 | slot | undefined |
extra | 额外内容,展示在列表右侧 | string | slot | undefined |
avatarStyle | 设置图标的样式 | CSSProperties | {} |
titleStyle | 设置标题的样式 | CSSProperties | {} |
descriptionStyle | 设置描述内容的样式 | CSSProperties | {} |
contentStyle | 设置内容的样式 | CSSProperties | {} |
actionsStyle | 设置操作区域的样式 | CSSProperties | {} |
extraStyle | 设置额外内容的样式 | CSSProperties | {} |
创建 List.vue 组件
其中引入使用了以下组件:
- [Vue3加载中(Spin)]((https://blog.csdn.net/Dandrose/article/details/129989730)
- Vue3空状态(Empty)
- Vue3分页(Pagination)
<script setup lang="ts">
import { computed, useSlots } from 'vue'
import Spin from '../spin'
import Empty from '../empty'
import Pagination from '../pagination'
interface Props {bordered?: boolean // 是否展示边框vertical?: boolean // 是否使用竖直样式split?: boolean // 是否展示分割线size?: 'small' | 'middle' | 'large' // 列表尺寸loading?: boolean // 是否加载中hoverable?: boolean // 是否显示悬浮样式header?: string // 列表头部 string | slotfooter?: string // 列表底部 string | slotspinProps?: object // Spin 组件属性配置,参考 Spin Props,用于配置列表加载中样式emptyProps?: object // Empty 组件属性配置,参考 Empty Props,用于配置暂无数据样式showPagination?: boolean // 是否显示分页pagination?: object // Pagination 组件属性配置,参考 Pagination Props,用于配置分页功能
}
const props = withDefaults(defineProps<Props>(), {bordered: false,vertical: false,split: true,size: 'middle',loading: false,hoverable: false,header: undefined,footer: undefined,spinProps: () => ({}),emptyProps: () => ({}),showPagination: false,pagination: () => ({})
})
const slots = useSlots()
const showHeader = computed(() => {const headerSlots = slots.header?.()return Boolean(headerSlots && headerSlots?.length) || props.header
})
const showDefault = computed(() => {const defaultSlots = slots.default?.()return Boolean(defaultSlots && defaultSlots?.length && defaultSlots[0].children?.length)
})
const showFooter = computed(() => {const footerSlots = slots.footer?.()return Boolean(footerSlots && footerSlots?.length) || props.footer
})
</script>
<template><Spin :spinning="loading" size="small" v-bind="spinProps"><divclass="m-list":class="{'list-bordered': bordered,'list-vertical': vertical,'list-split': split,'list-small': size === 'small','list-large': size === 'large','list-hoverable': hoverable}"><div class="m-list-header" v-if="showHeader"><slot name="header">{{ header }}</slot></div><slot v-if="showDefault"></slot><div class="m-list-empty" v-else><Empty image="outlined" v-bind="emptyProps" /></div><div class="m-list-footer" v-if="showFooter"><slot name="footer">{{ footer }}</slot></div><div class="m-list-pagination" v-if="showPagination"><Pagination placement="right" v-bind="pagination" /></div></div></Spin>
</template>
<style lang="less" scoped>
.m-list {margin: 0;position: relative;color: rgba(0, 0, 0, 0.88);font-size: 14px;line-height: 1.5714285714285714;.m-list-header,.m-list-footer {background: transparent;padding: 12px 0;transition: all 0.3s;}.m-list-empty {padding: 16px;}.m-list-pagination {margin-top: 24px;}
}
.list-bordered {border: 1px solid #d9d9d9;border-radius: 8px;.m-list-header,:deep(.m-list-item),.m-list-footer {padding-inline: 24px;}.m-list-pagination {margin: 16px 24px;}
}
.list-vertical {:deep(.m-list-item) {align-items: initial;.m-list-item-main {display: block;.m-list-item-meta {margin-bottom: 16px;.m-list-item-content {.list-item-title {margin-bottom: 12px;color: rgba(0, 0, 0, 0.88);font-size: 16px;font-weight: 700;line-height: 1.5;}}}.list-item-actions {margin-top: 16px;margin-left: auto;& > * {padding: 0 16px;&:first-child {padding-left: 0;}}}}}
}
.list-split {.m-list-header {border-bottom: 1px solid rgba(5, 5, 5, 0.06);}:deep(.m-list-item) {&:not(:last-child) {border-bottom: 1px solid rgba(5, 5, 5, 0.06);}}
}
.list-small {:deep(.m-list-item) {padding: 8px 16px;}
}
.list-bordered.list-small {.m-list-header,:deep(.m-list-item),.m-list-footer {padding: 8px 16px;}
}
.list-large {:deep(.m-list-item) {padding: 16px 24px;}
}
.list-bordered.list-large {.m-list-header,:deep(.m-list-item),.m-list-footer {padding: 16px 24px;}
}
.list-hoverable {:deep(.m-list-item) {&:hover {background-color: rgba(0, 0, 0, 0.02);}}
}
</style>
创建 ListItem.vue 组件
其中引入使用了以下组件:
- Vue3头像(Avatar)
<script setup lang="ts">
import { computed, useSlots } from 'vue'
import type { CSSProperties, Slot } from 'vue'
import Avatar from '../avatar'
interface Props {avatar?: string // 列表元素的图标字符 string | slotavatarProps?: object // Avatar 组件属性配置,参考 Avatar Props,用于配置列表图标样式title?: string // 列表元素的标题 string | slotdescription?: string // 列表元素的描述内容 string | slotactions?: Slot // 列表操作组 slotextra?: string // 额外内容,额外内容,展示在列表右侧 string | slotavatarStyle?: CSSProperties // 设置图标的样式titleStyle?: CSSProperties // 设置标题的样式descriptionStyle?: CSSProperties // 设置描述内容的样式contentStyle?: CSSProperties // 设置内容的样式actionsStyle?: CSSProperties // 设置操作区域的样式extraStyle?: CSSProperties // 设置额外内容的样式
}
const props = withDefaults(defineProps<Props>(), {avatar: undefined,avatarProps: () => ({}),title: undefined,description: undefined,actions: undefined,extra: undefined,avatarStyle: () => ({}),titleStyle: () => ({}),descriptionStyle: () => ({}),contentStyle: () => ({}),actionsStyle: () => ({}),extraStyle: () => ({})
})
const slots = useSlots()
const showAvatar = computed(() => {const avatarSlots = slots.avatar?.()return Boolean(avatarSlots && avatarSlots?.length) || props.avatar || JSON.stringify(props.avatarProps) !== '{}'
})
const showContent = computed(() => {const titleSlots = slots.title?.()const descriptionSlots = slots.description?.()let n = 0if (titleSlots && titleSlots?.length) {n++}if (descriptionSlots && descriptionSlots?.length) {n++}return Boolean(n) || props.title || props.description
})
const showDefault = computed(() => {const defaultSlots = slots.default?.()return Boolean(defaultSlots && defaultSlots?.length)
})
const showActions = computed(() => {const actionsSlots = slots.actions?.()return Boolean(actionsSlots && actionsSlots?.length)
})
const showExtra = computed(() => {const extraSlots = slots.extra?.()return Boolean(extraSlots && extraSlots?.length) || props.extra
})
</script>
<template><div class="m-list-item"><div class="m-list-item-main"><div class="m-list-item-meta" v-if="showAvatar || showContent"><div class="m-list-item-avatar" v-if="showAvatar" :style="avatarStyle"><slot name="avatar"><Avatar v-bind="avatarProps">{{ avatar }}</Avatar></slot></div><div class="m-list-item-content" v-if="showContent"><p class="list-item-title" :style="titleStyle"><slot name="title">{{ title }}</slot></p><div class="list-item-description" :style="descriptionStyle"><slot name="description">{{ description }}</slot></div></div></div><div v-if="showDefault" :style="contentStyle"><slot></slot></div><div class="list-item-actions" v-if="showActions" :style="actionsStyle"><slot name="actions"></slot></div></div><div class="list-item-extra" v-if="showExtra" :style="extraStyle"><slot name="extra">{{ extra }}</slot></div></div>
</template>
<style lang="less" scoped>
.m-list-item {display: flex;justify-content: space-between;align-items: center;padding: 12px 24px;color: rgba(0, 0, 0, 0.88);max-width: 100%;transition: all 0.3s;.m-list-item-main {display: flex;align-items: center;flex: 1;.m-list-item-meta {display: flex;flex: 1;align-items: flex-start;max-width: 100%;.m-list-item-avatar {margin-right: 16px;}.m-list-item-content {flex: 1 0;width: 0;color: rgba(0, 0, 0, 0.88);.list-item-title {margin-bottom: 4px;color: rgba(0, 0, 0, 0.88);font-size: 14px;font-weight: 700;line-height: 1.5714285714285714;:deep(a) {font-weight: 700;color: rgba(0, 0, 0, 0.88);transition: all 0.3s;&:hover {color: @themeColor;}}}.list-item-description {color: rgba(0, 0, 0, 0.45);font-size: 14px;line-height: 1.5714285714285714;}}}.list-item-actions {flex: 0 0 auto;margin-left: 48px;font-size: 0;& > * {// 选择所有直接子元素,不包括深层的后代position: relative;display: inline-flex;align-items: center;padding: 0 8px;color: rgba(0, 0, 0, 0.45);font-size: 14px;line-height: 1.5714285714285714;text-align: center;&:first-child {padding-left: 0;}&:not(:last-child) {&::after {position: absolute;top: 50%;right: 0;width: 1px;height: 14px;transform: translateY(-50%);background-color: rgba(5, 5, 5, 0.06);content: '';}}}& > :deep(a) {// 选择所有直接子元素且是 a 标签color: @themeColor;transition: color 0.3s;&:hover {color: #4096ff;}}}}.list-item-extra {margin-left: 24px;}
}
</style>
其中引入使用了以下组件:
- Vue3头像(Avatar)
- Vue3开关(Switch)
- Vue3单选框(Radio)
- Vue3弹性布局(Flex)
- Vue3栅格(Grid)
- Vue3间距(Space)
- Vue3输入框(Input)
在要使用的页面引入
<script setup lang="ts">
import List from './List.vue'
import ListItem from './ListItem.vue'
import { ref, reactive } from 'vue'
const listData = ref([{title: 'Vue Amazing UI Title 1',description: 'Vue Amazing UI, An Amazing Vue3 UI Components Library.',content: 'content'},{title: 'Vue Amazing UI Title 2',description: 'Vue Amazing UI, An Amazing Vue3 UI Components Library.',content: 'content'},{title: 'Vue Amazing UI Title 3',description: 'Vue Amazing UI, An Amazing Vue3 UI Components Library.',content: 'content'},{title: 'Vue Amazing UI Title 4',description: 'Vue Amazing UI, An Amazing Vue3 UI Components Library.',content: 'content'}
])
const bordered = ref(true)
const simpleListData = ref([{title: 'Vue Amazing UI Title 1',description: 'An Amazing Vue3 UI Components Library.',content: 'content'},{title: 'Vue Amazing UI Title 2',description: 'An Amazing Vue3 UI Components Library.',content: 'content'},{title: 'Vue Amazing UI Title 3',description: 'An Amazing Vue3 UI Components Library.',content: 'content'},{title: 'Vue Amazing UI Title 4',description: 'An Amazing Vue3 UI Components Library.',content: 'content'}
])
const simpleList = ref(['Vue Amazing UI is developed using TypeScript','An Amazing Vue3 UI Components Library','Streamline web development with Vue Amazing UI','Incredible Vue components for modern web design','Transform your Vue interface with Vue Amazing UI'
])
const sizeOptions = [{label: 'small',value: 'small'},{label: 'middle',value: 'middle'},{label: 'large',value: 'large'}
]
const size = ref('middle')
const loading = ref(true)
const allListData = ref<any[]>([])
for (let i = 1; i <= 8; i++) {allListData.value.push({href: 'https://themusecatcher.github.io/vue-amazing-ui/',title: `Vue Amazing UI part ${i}`,avatar: 'https://cdn.jsdelivr.net/gh/themusecatcher/resources@0.0.5/1.jpg',description: 'Vue Amazing UI, An Amazing Vue3 UI Components Library.',content:'Vue Amazing UI supplies streamline web development, incredible Vue components for modern web design and transform your Vue interface completely.'})
}
const paginationListData = ref<any[]>([])
paginationListData.value = allListData.value.slice(0, 3)
const pagination = {page: 1,pageSize: 3,total: 8,onChange: (page: number, pageSize: number) => {console.log('change page', page)console.log('change pageSize', pageSize)const start = (page - 1) * pageSize + 1const end = page * pageSize > 8 ? 8 : page * pageSizepaginationListData.value = allListData.value.slice(start - 1, end)}
}
const allConfigListData = ref<ang[]>([])
for (let i = 1; i <= 30; i++) {allConfigListData.value.push({href: 'https://themusecatcher.github.io/vue-amazing-ui/',title: `Vue Amazing UI Title ${i}`,description: 'Vue Amazing UI, An Amazing Vue3 UI Components Library.',content: 'Incredible Vue components for modern web design'})
}
const configListData = ref<any[]>([])
configListData.value = allConfigListData.value.slice(0, 5)
const state = reactive({bordered: true,vertical: false,split: true,size: 'middle',loading: false,hoverable: true,header: 'list header',footer: 'list footer',extra: 'extra',showPagination: true,pagination: {page: 1,pageSize: 5,total: 30,showTotal: (total: number, range: number[]) => `${range[0]}-${range[1]} of ${total} items`,showSizeChanger: true,showQuickJumper: true,onChange: (page: number, pageSize: number) => {console.log('change page', page)console.log('change pageSize', pageSize)const start = (page - 1) * pageSize + 1const end = page * pageSize > state.pagination.total ? state.pagination.total : page * pageSizeconfigListData.value = allConfigListData.value.slice(start - 1, end)}}
})
</script>
<template><div><h1>{{ $route.name }} {{ $route.meta.title }}</h1><h2 class="mt30 mb10">基本使用</h2><List><ListItem v-for="(data, index) in listData" :key="index" :title="data.title"><template #avatar><Avatar src="https://cdn.jsdelivr.net/gh/themusecatcher/resources@0.0.5/1.jpg" /></template><template #description>{{ data.description }}</template></ListItem></List><h2 class="mt30 mb10">隐藏分割线</h2><List :split="false"><ListItem v-for="(data, index) in listData" :key="index" :title="data.title"><template #avatar><Avatar src="https://cdn.jsdelivr.net/gh/themusecatcher/resources@0.0.5/1.jpg" /></template><template #description>{{ data.description }}</template></ListItem></List><h2 class="mt30 mb10">带边框列表</h2><Flex vertical><Space align="center"> bordered:<Switch v-model="bordered" /> </Space><List :bordered="bordered"><template #header><div>Header</div></template><ListItem v-for="(data, index) in listData" :key="index" :title="data.title"><template #avatar><Avatar src="https://cdn.jsdelivr.net/gh/themusecatcher/resources@0.0.5/1.jpg" /></template><template #description>{{ data.description }}</template></ListItem><template #footer><div>Footer</div></template></List></Flex><h2 class="mt30 mb10">三种尺寸</h2><Flex vertical><Radio :options="sizeOptions" v-model:value="size" button button-style="solid" /><Row :gutter="32"><Col :span="12"><List bordered :size="size"><ListItem v-for="(data, index) in simpleListData" :key="index"><template #avatar><Avatar src="https://cdn.jsdelivr.net/gh/themusecatcher/resources@0.0.5/1.jpg" /></template><template #title><a href="https://themusecatcher.github.io/vue-amazing-ui/">{{ data.title }}</a></template><template #description>{{ data.description }}</template></ListItem></List></Col><Col :span="12"><List bordered :size="size"><template #header><div>Header</div></template><ListItem v-for="(data, index) in simpleList" :key="index">{{ data }}</ListItem><template #footer><div>Footer</div></template></List></Col></Row></Flex><h2 class="mt30 mb10">加载中</h2><Flex vertical><Space align="center"> Loading state:<Switch v-model="loading" /> </Space><Row :gutter="32"><Col :span="12"><List bordered :loading="loading"><ListItem v-for="(data, index) in simpleListData" :key="index" :title="data.title"><template #avatar><Avatar src="https://cdn.jsdelivr.net/gh/themusecatcher/resources@0.0.5/1.jpg" /></template><template #description>{{ data.description }}</template></ListItem></List></Col><Col :span="12"><List bordered :loading="loading" :spin-props="{ indicator: 'dynamic-circle' }"><template #header><div>Header</div></template><ListItem v-for="(data, index) in simpleList" :key="index">{{ data }}</ListItem><template #footer><div>Footer</div></template></List></Col></Row></Flex><h2 class="mt30 mb10">暂无数据</h2><List><ListItem v-for="(data, index) in []" :key="index"></ListItem></List><h2 class="mt30 mb10">显示悬浮样式</h2><Row :gutter="32"><Col :span="12"><List bordered hoverable><ListItem v-for="(data, index) in simpleListData" :key="index" :title="data.title"><template #avatar><Avatar src="https://cdn.jsdelivr.net/gh/themusecatcher/resources@0.0.5/1.jpg" /></template><template #description>{{ data.description }}</template></ListItem></List></Col><Col :span="12"><List bordered hoverable><template #header><div>Header</div></template><ListItem v-for="(data, index) in simpleList" :key="index">{{ data }}</ListItem><template #footer><div>Footer</div></template></List></Col></Row><h2 class="mt30 mb10">列表添加操作项</h2><List><ListItem v-for="(data, index) in listData" :key="index" :title="data.title"><template #avatar><Avatar src="https://cdn.jsdelivr.net/gh/themusecatcher/resources@0.0.5/1.jpg" /></template><template #description>{{ data.description }}</template>{{ data.content }}<template #actions><a>edit</a><a>more</a></template></ListItem></List><h2 class="mt30 mb10">自定义样式</h2><List><ListItem:avatar-props="{src: 'https://cdn.jsdelivr.net/gh/themusecatcher/resources@0.0.5/1.jpg',size: 56}":avatar-style="{ alignSelf: 'center' }":title-style="{ fontSize: '20px' }":description-style="{ fontSize: '16px' }":content-style="{ fontSize: '18px', color: '#f50', marginLeft: '16px' }":extra-style="{ overflow: 'hidden', borderRadius: '12px' }"v-for="(data, index) in listData":key="index":title="data.title"><template #description>{{ data.description }}</template>{{ data.content }}<template #actions><a>edit</a><a>more</a></template><template #extra><imgclass="u-img"width="200"alt="extra"src="https://cdn.jsdelivr.net/gh/themusecatcher/resources@0.0.5/2.jpg"/></template></ListItem></List><h2 class="mt30 mb10">竖排分页列表</h2><List vertical size="large" show-pagination :pagination="pagination"><ListItem v-for="(data, index) in paginationListData" :key="index"><template #title><a :href="data.href" target="_blank">{{ data.title }}</a></template><template #avatar><Avatar src="https://cdn.jsdelivr.net/gh/themusecatcher/resources@0.0.5/1.jpg" /></template><template #description>{{ data.description }}</template>{{ data.content }}<template #actions><span><svgclass="u-svg"focusable="false"data-icon="star"width="1em"height="1em"fill="currentColor"aria-hidden="true"viewBox="64 64 896 896"><pathd="M908.1 353.1l-253.9-36.9L540.7 86.1c-3.1-6.3-8.2-11.4-14.5-14.5-15.8-7.8-35-1.3-42.9 14.5L369.8 316.2l-253.9 36.9c-7 1-13.4 4.3-18.3 9.3a32.05 32.05 0 00.6 45.3l183.7 179.1-43.4 252.9a31.95 31.95 0 0046.4 33.7L512 754l227.1 119.4c6.2 3.3 13.4 4.4 20.3 3.2 17.4-3 29.1-19.5 26.1-36.9l-43.4-252.9 183.7-179.1c5-4.9 8.3-11.3 9.3-18.3 2.7-17.5-9.5-33.7-27-36.3zM664.8 561.6l36.1 210.3L512 672.7 323.1 772l36.1-210.3-152.8-149L417.6 382 512 190.7 606.4 382l211.2 30.7-152.8 148.9z"></path></svg>156</span><span><svgclass="u-svg"focusable="false"data-icon="like"width="1em"height="1em"fill="currentColor"aria-hidden="true"viewBox="64 64 896 896"><pathd="M885.9 533.7c16.8-22.2 26.1-49.4 26.1-77.7 0-44.9-25.1-87.4-65.5-111.1a67.67 67.67 0 00-34.3-9.3H572.4l6-122.9c1.4-29.7-9.1-57.9-29.5-79.4A106.62 106.62 0 00471 99.9c-52 0-98 35-111.8 85.1l-85.9 311H144c-17.7 0-32 14.3-32 32v364c0 17.7 14.3 32 32 32h601.3c9.2 0 18.2-1.8 26.5-5.4 47.6-20.3 78.3-66.8 78.3-118.4 0-12.6-1.8-25-5.4-37 16.8-22.2 26.1-49.4 26.1-77.7 0-12.6-1.8-25-5.4-37 16.8-22.2 26.1-49.4 26.1-77.7-.2-12.6-2-25.1-5.6-37.1zM184 852V568h81v284h-81zm636.4-353l-21.9 19 13.9 25.4a56.2 56.2 0 016.9 27.3c0 16.5-7.2 32.2-19.6 43l-21.9 19 13.9 25.4a56.2 56.2 0 016.9 27.3c0 16.5-7.2 32.2-19.6 43l-21.9 19 13.9 25.4a56.2 56.2 0 016.9 27.3c0 22.4-13.2 42.6-33.6 51.8H329V564.8l99.5-360.5a44.1 44.1 0 0142.2-32.3c7.6 0 15.1 2.2 21.1 6.7 9.9 7.4 15.2 18.6 14.6 30.5l-9.6 198.4h314.4C829 418.5 840 436.9 840 456c0 16.5-7.2 32.1-19.6 43z"></path></svg>156</span><span><svgclass="u-svg"focusable="false"data-icon="message"width="1em"height="1em"fill="currentColor"aria-hidden="true"viewBox="64 64 896 896"><pathd="M464 512a48 48 0 1096 0 48 48 0 10-96 0zm200 0a48 48 0 1096 0 48 48 0 10-96 0zm-400 0a48 48 0 1096 0 48 48 0 10-96 0zm661.2-173.6c-22.6-53.7-55-101.9-96.3-143.3a444.35 444.35 0 00-143.3-96.3C630.6 75.7 572.2 64 512 64h-2c-60.6.3-119.3 12.3-174.5 35.9a445.35 445.35 0 00-142 96.5c-40.9 41.3-73 89.3-95.2 142.8-23 55.4-34.6 114.3-34.3 174.9A449.4 449.4 0 00112 714v152a46 46 0 0046 46h152.1A449.4 449.4 0 00510 960h2.1c59.9 0 118-11.6 172.7-34.3a444.48 444.48 0 00142.8-95.2c41.3-40.9 73.8-88.7 96.5-142 23.6-55.2 35.6-113.9 35.9-174.5.3-60.9-11.5-120-34.8-175.6zm-151.1 438C704 845.8 611 884 512 884h-1.7c-60.3-.3-120.2-15.3-173.1-43.5l-8.4-4.5H188V695.2l-4.5-8.4C155.3 633.9 140.3 574 140 513.7c-.4-99.7 37.7-193.3 107.6-263.8 69.8-70.5 163.1-109.5 262.8-109.9h1.7c50 0 98.5 9.7 144.2 28.9 44.6 18.7 84.6 45.6 119 80 34.3 34.3 61.3 74.4 80 119 19.4 46.2 29.1 95.2 28.9 145.8-.6 99.6-39.7 192.9-110.1 262.7z"></path></svg>6</span></template><template #extra><imgclass="u-img"width="272"alt="extra"src="https://gw.alipayobjects.com/zos/rmsportal/mqaQswcyDLcXyDKnZfES.png"/></template></ListItem><template #footer><div><b>Vue Amazing UI</b>footer part</div></template></List><h2 class="mt30 mb10">列表配置器</h2><Flex gap="large" vertical><Row :gutter="[24, 12]"><Col :span="6"><Space gap="small" vertical> bordered:<Switch v-model="state.bordered" /> </Space></Col><Col :span="6"><Space gap="small" vertical> vertical:<Switch v-model="state.vertical" /> </Space></Col><Col :span="6"><Space gap="small" vertical> split:<Switch v-model="state.split" /> </Space></Col><Col :span="6"><Space gap="small" vertical>size:<Radio :options="sizeOptions" v-model:value="state.size" button button-style="solid" /></Space></Col><Col :span="6"><Space gap="small" vertical> loading:<Switch v-model="state.loading" /> </Space></Col><Col :span="6"><Space gap="small" vertical> hoverable:<Switch v-model="state.hoverable" /> </Space></Col><Col :span="6"><Flex gap="small" vertical> header:<Input v-model:value="state.header" placeholder="header" /> </Flex></Col><Col :span="6"><Flex gap="small" vertical> footer:<Input v-model:value="state.footer" placeholder="footer" /> </Flex></Col><Col :span="6"><Flex gap="small" vertical> extra:<Input v-model:value="state.extra" placeholder="extra" /> </Flex></Col><Col :span="6"><Space gap="small" vertical> showPagination:<Switch v-model="state.showPagination" /> </Space></Col><Col :span="6"><Space gap="small" vertical> showSizeChanger:<Switch v-model="state.pagination.showSizeChanger" /> </Space></Col><Col :span="6"><Space gap="small" vertical> showQuickJumper:<Switch v-model="state.pagination.showQuickJumper" /> </Space></Col></Row><List:bordered="state.bordered":vertical="state.vertical":split="state.split":size="state.size":loading="state.loading":hoverable="state.hoverable":header="state.header":footer="state.footer":showPagination="state.showPagination":pagination="state.pagination"><ListItem v-for="(data, index) in configListData" :key="index" :extra="state.extra"><template #title><a :href="data.href" target="_blank">{{ data.title }}</a></template><template #avatar><Avatar src="https://cdn.jsdelivr.net/gh/themusecatcher/resources@0.0.5/1.jpg" /></template><template #description>{{ data.description }}</template>{{ data.content }}</ListItem></List></Flex></div>
</template>
<style lang="less" scoped>
.u-img {display: inline-block;vertical-align: bottom;
}
.u-svg {margin-right: 8px;fill: rgba(0, 0, 0, 0.45);
}
</style>
相关文章:
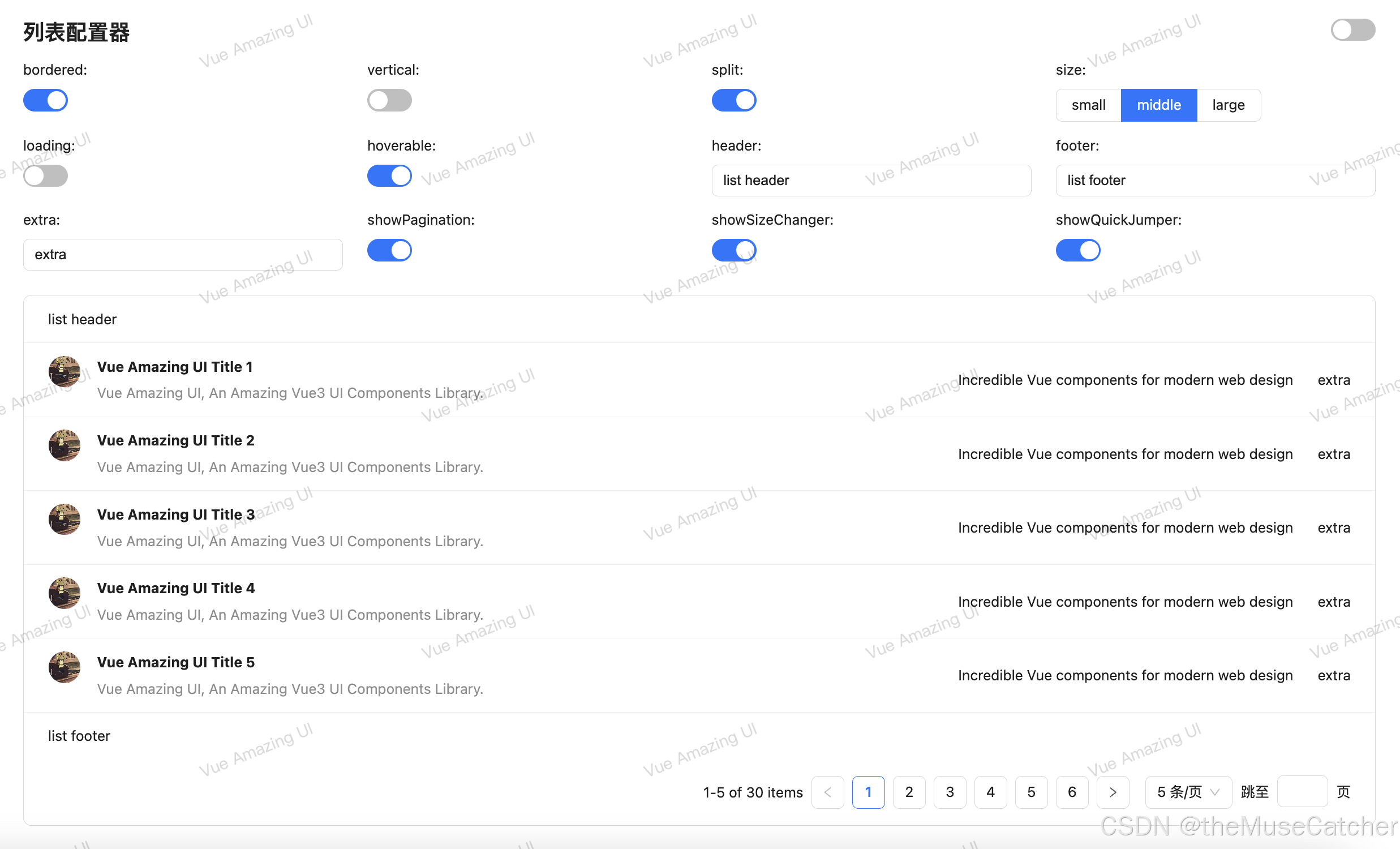
Vue3列表(List)
效果如下图:在线预览 APIs List 参数说明类型默认值bordered是否展示边框booleanfalsevertical是否使用竖直样式booleanfalsesplit是否展示分割线booleantruesize列表尺寸‘small’ | ‘middle’ | ‘large’‘middle’loading是否加载中booleanfalsehoverable是否…...
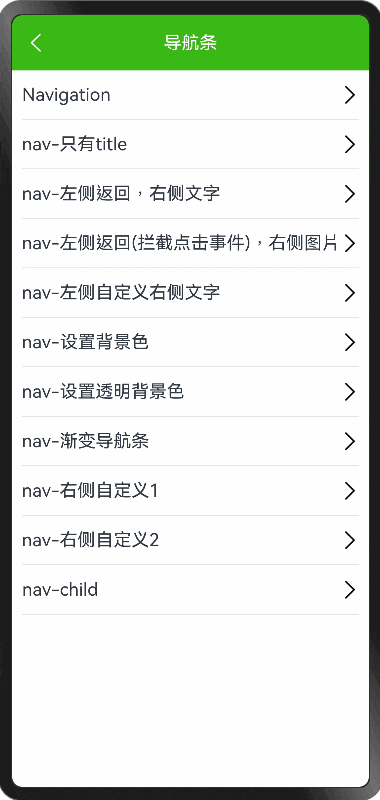
HarmonyOS NEXT - Navigation组件封装BaseNavigation
demo 地址: https://github.com/iotjin/JhHarmonyDemo 代码不定时更新,请前往github查看最新代码 在demo中这些组件和工具类都通过module实现了,具体可以参考HarmonyOS NEXT - 通过 module 模块化引用公共组件和utils 官方介绍 组件导航 (Navigation)(推…...
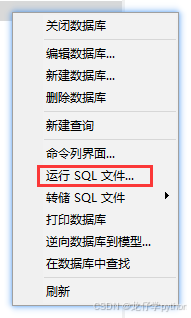
浅看MySQL数据库
有这么一句话:“一个不会数据库的程序员不是合格的程序员”。有点夸张,但是确是如此。透彻学习数据库是要学习好多知识,需要学的东西也是偏难的。我们今天来看数据库MySQL的一些简单基础东西,跟着小编一起来看一下吧。 什么是数据…...

Pytorch常用训练套路框架(CPU)
文章目录 1. 数据准备示例:加载 CIFAR-10 数据集 2. 模型定义示例:定义一个简单的卷积神经网络 3. 损失函数和优化器示例:定义损失函数和优化器 4. 训练循环示例:训练循环 5. 评估和测试示例:评估模型 6. 保存和加载模…...
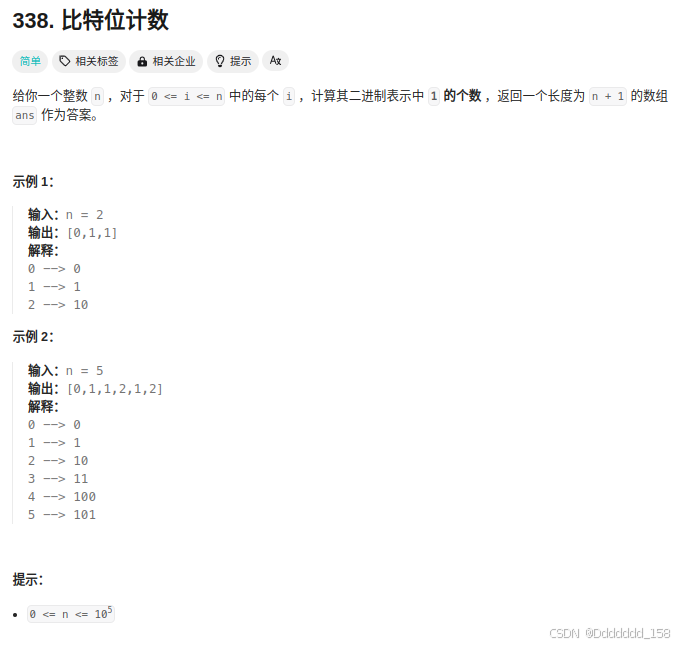
C++ | Leetcode C++题解之第338题比特位计数
题目: 题解: class Solution { public:vector<int> countBits(int n) {vector<int> bits(n 1);for (int i 1; i < n; i) {bits[i] bits[i & (i - 1)] 1;}return bits;} };...
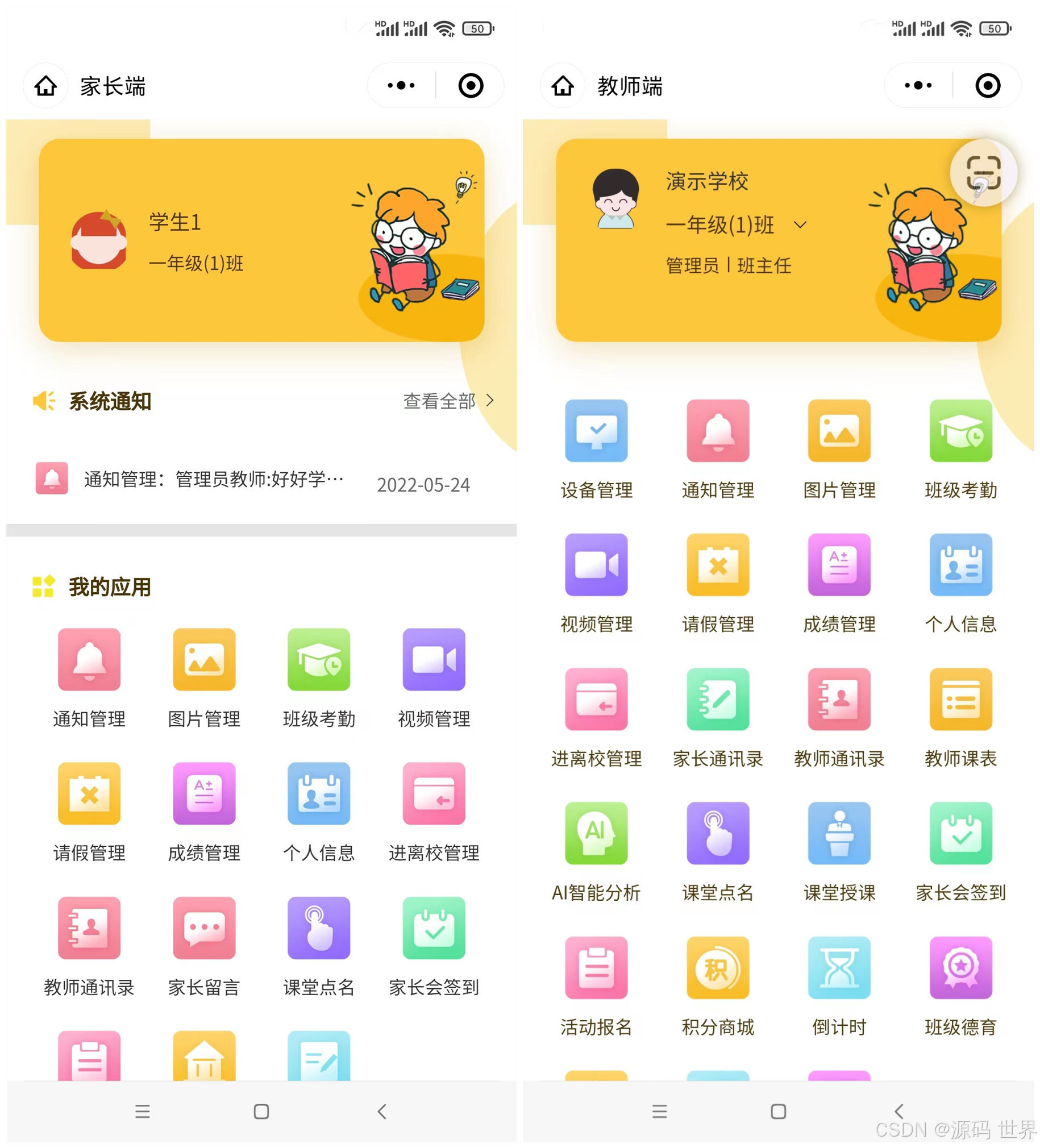
智慧校园云平台电子班牌系统源码,智慧教育一体化云解决方案
智慧校园云平台电子班牌系统,利用先进的云计算技术,将教育信息化资源和教学管理系统进行有效整合,实现生态基础数据共享、应用生态统一管理,为智慧教育建设的统一性,稳定性,可扩展性,互通性提供…...

数据库系统 第17节 数据仓库 案例赏析
下面我将通过几个具体的案例来说明数据仓库如何在不同的行业中发挥作用,并解决实际业务问题。 案例 1: 零售业 背景: 一家大型零售商希望改进其库存管理和市场营销策略,以提高销售额和顾客满意度。 解决方案: 数据仓库: 构建一个数据仓库࿰…...
硬件面试经典 100 题(71~90 题)
71、请问下图电路的作用是什么? 该电路实现 IIC 信号的电平转换(3.3V 和 5V 电平转换),并且是双向通信的。 上下两路是一样的,只分析 SDA 一路: 1) 从左到右通信(SDA2 为输入状态&…...

【git】代理相关
问题: 开启了翻墙代理工具,拉取代码时报错:fatal: 无法访问 xxxx : Failed to connect to github.com port 443: 连接超时 解决: 0,取消代理仍然无法拉取 1,查看控制面板-网络与Internet-代理ÿ…...

golang gin框架中创建自定义中间件的2种方式总结 - func(*gin.Context)方式和闭包函数方式定义gin中间件
在gin框架中,我们可以通过2种方式创建自定义中间件: 1. 直接定义一个类型为 func(*gin.Context)的函数或者方法 这种方式是我们常用的方式,也就是定义一个参数为*gin.Context的函数或者方法。定义的方法就是创建一个 参数类型为 gin.Handler…...

Linux高级编程 8.13 文件IO
一、文件IO 操作系统为了方便用户使用系统功能而对外提供的一组系统函数。称之为 系统调用(unistd.h) 其中有个 文件IO,一般都是对设备文件操作,当然也可以对普通文件进行操作。 这是一个基于Linux内核的没有缓存的IO机制 文件IO特性&…...

【k8s】ubuntu18.04 containerd 手动从1.7.15 换为1.7.20
ubutnu18.04之前手动安装了1.7.15现在下载1.7.20containerd-1.7.20-linux-amd64.tar.gz root@k8s-worker-i58265u:/home/zhangbin# root@k8s-worker-i58265u:/home/zhangbin# https://github.com/containerd/containerd/releases/download/v1.7.20/containerd-1.7.20-linux-am…...

常用浮动方式
目录 一、标准流 二、float浮动 三、 flex浮动 3.1flex组成 3.2 主轴对齐方式 3.3侧轴对齐方式 3.4修改主轴方向 3.5弹性盒子换行 3.6行对齐方式 一、标准流 标签在网页中的默认排布规则 例如: 块元素独占一行、行内元素可以一行显示多个 二、float浮动 让块…...

设计模式反模式:UML常见误用案例分析
文章目录 设计模式反模式:UML常见误用案例分析1. 反模式概述2. 反模式的 UML 图示误用2.1 God Object 反模式2.2 Spaghetti Code 反模式2.3 Golden Hammer 反模式2.4 Poltergeist 反模式 3. 总结 设计模式反模式:UML常见误用案例分析 在软件工程领域&am…...
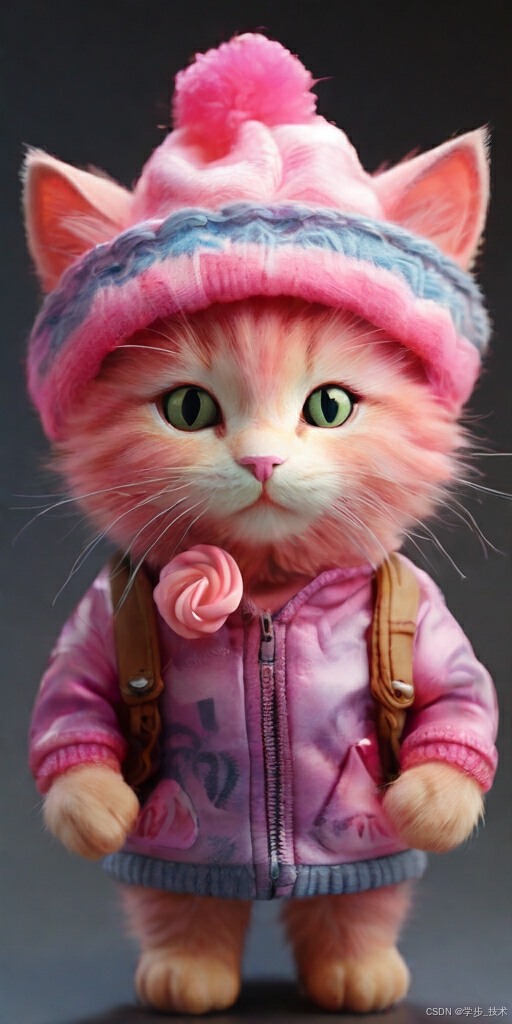
Python编码系列—Python SQL与NoSQL数据库交互:深入探索与实战应用
🌟🌟 欢迎来到我的技术小筑,一个专为技术探索者打造的交流空间。在这里,我们不仅分享代码的智慧,还探讨技术的深度与广度。无论您是资深开发者还是技术新手,这里都有一片属于您的天空。让我们在知识的海洋中…...

贪心算法---跳跃游戏
题目: 给你一个非负整数数组 nums ,你最初位于数组的 第一个下标 。数组中的每个元素代表你在该位置可以跳跃的最大长度。 判断你是否能够到达最后一个下标,如果可以,返回 true ;否则,返回 false 。 思路…...
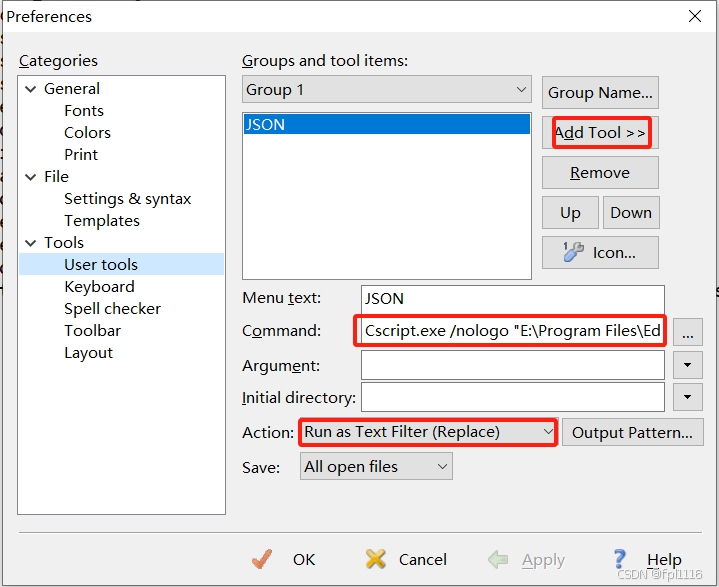
利用EditPlus进行Json数据格式化
利用EditPlus进行Json数据格式化 git下载地址:https://github.com/michael-deve/CommonData-EditPlusTools.git (安装过editplus的直接将里面的json.js文件复制走就行) 命令:Cscript.exe /nologo “D:\Program Files (x86)\EditPlus 3\json.js” D:\P…...
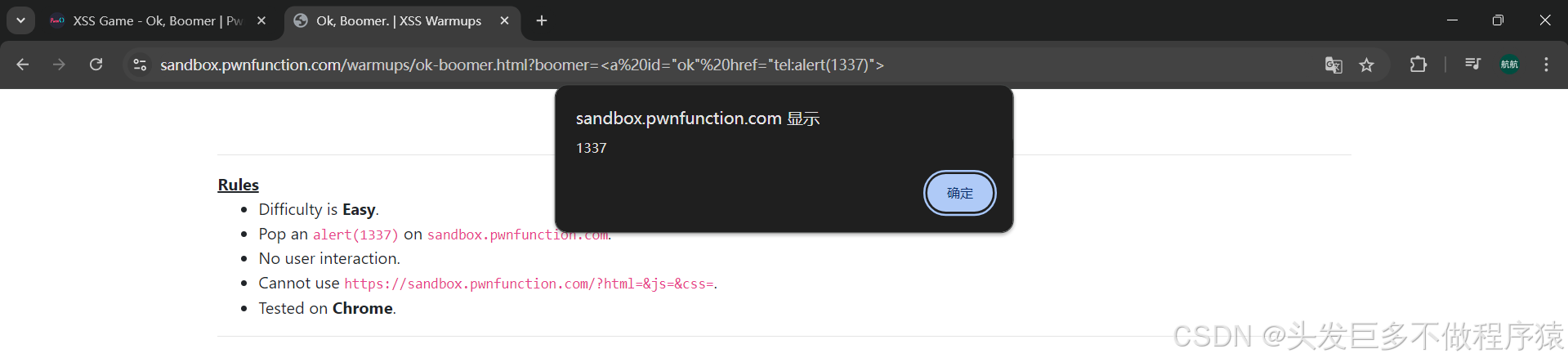
xss.function靶场(easy)
文章目录 第一关Ma Spaghet!第二关Jefff第三关Ugandan Knuckles第四关Ricardo Milos第五关Ah Thats Hawt第六关Ligma第七关Mafia第八关Ok, Boomer 网址:https://xss.pwnfunction.com/ 第一关Ma Spaghet! 源码 <!-- Challenge --> <h2 id"spaghet&qu…...
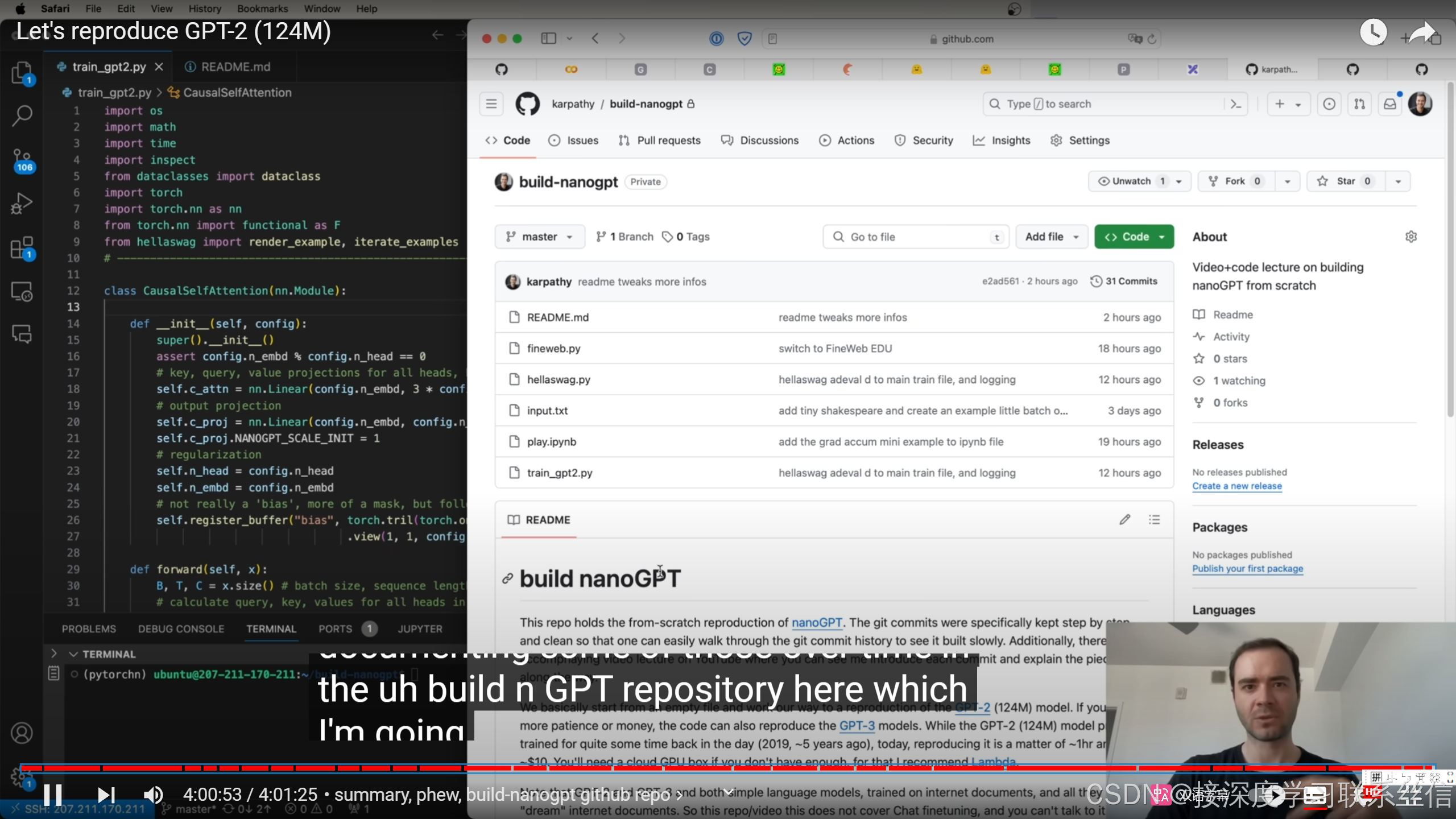
【LLM入门】Let‘s reproduce GPT-2 (124M)【完结,重新回顾一下,伟大!】
文章目录 03:43:05 SECTION 4: results in the morning! GPT-2, GPT-3 repro03:56:21 shoutout to llm.c, equivalent but faster code in raw C/CUDA【太牛了ba】03:59:39 summary, phew, build-nanogpt github repo 03:43:05 SECTION 4: results in the morning! GPT-2, GPT-…...

c语言----取反用什么符号
目录 前言 一、逻辑取反 二、按位取反 三、应用场景 前言 在C编程语言中,取反使用符号!表示逻辑取反,而使用~表示按位取反。 其中,逻辑取反!是将表达式的真值(非0值)转换为假(0),…...
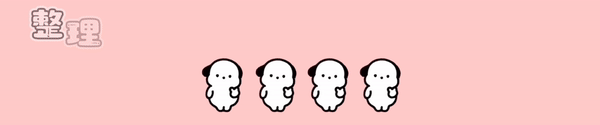
【html+css 绚丽Loading】 - 000003 乾坤阴阳轮
前言:哈喽,大家好,今天给大家分享htmlcss 绚丽Loading!并提供具体代码帮助大家深入理解,彻底掌握!创作不易,如果能帮助到大家或者给大家一些灵感和启发,欢迎收藏关注哦 💕…...
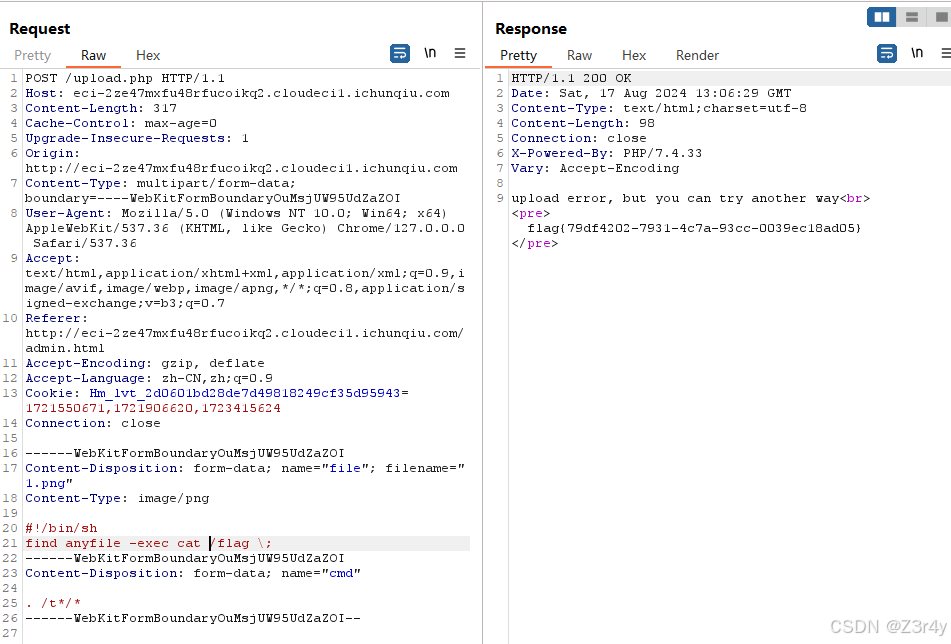
【Web】巅峰极客2024 部分题解
目录 EncirclingGame GoldenHornKing php_online admin_Test EncirclingGame 玩赢游戏就行 GoldenHornKing 利用点在传入的app 可以打python内存马 /calc?calc_reqconfig.__init__.__globals__[__builtins__][exec](app.add_api_route("/flag",lambda:__i…...
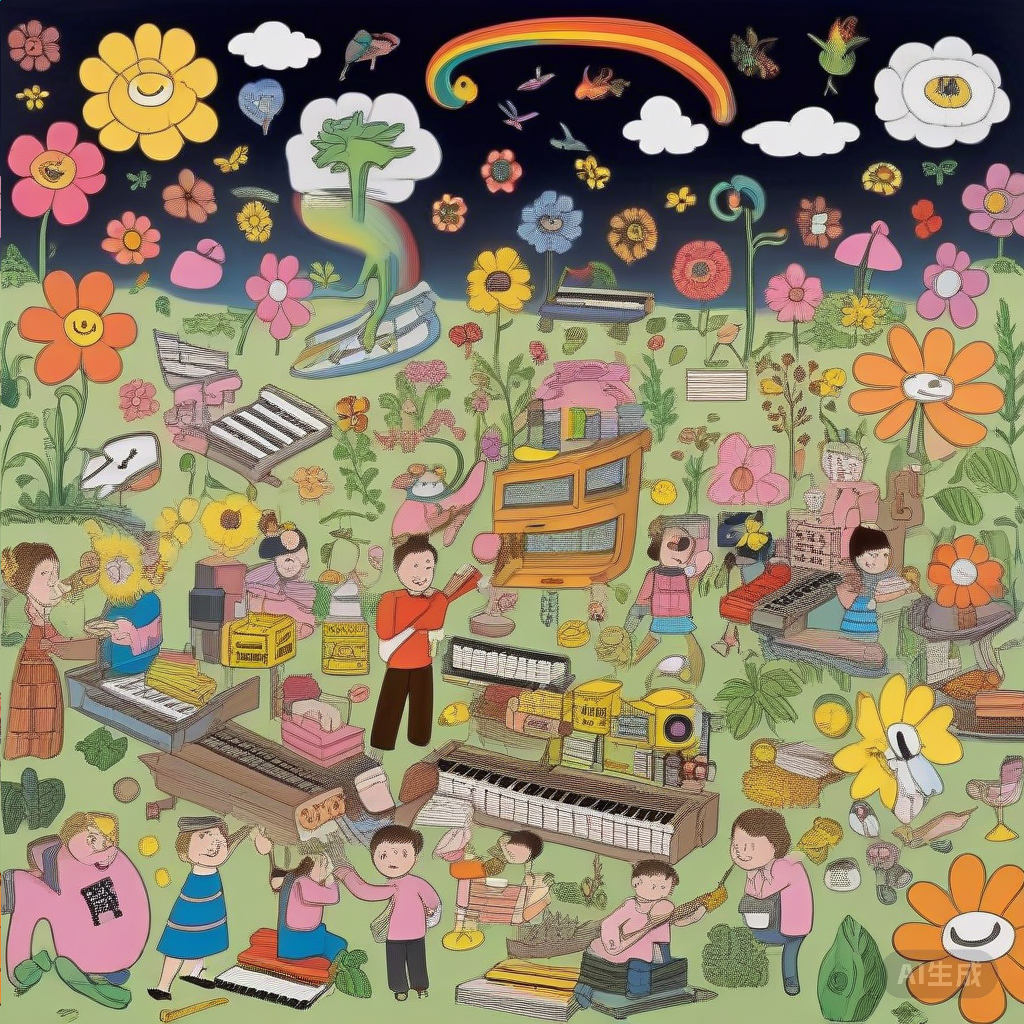
在AMD GPU上进行Grok-1模型的推理
Inferencing with Grok-1 on AMD GPUs — ROCm Blogs 我们展示了如何通过利用ROCm软件平台,能在AMD MI300X GPU加速器上无缝运行xAI公司的Grok-1模型。 介绍 xAI公司在2023年11月发布了Grok-1模型,允许任何人使用、实验和基于它构建。Grok-1的不同之处…...
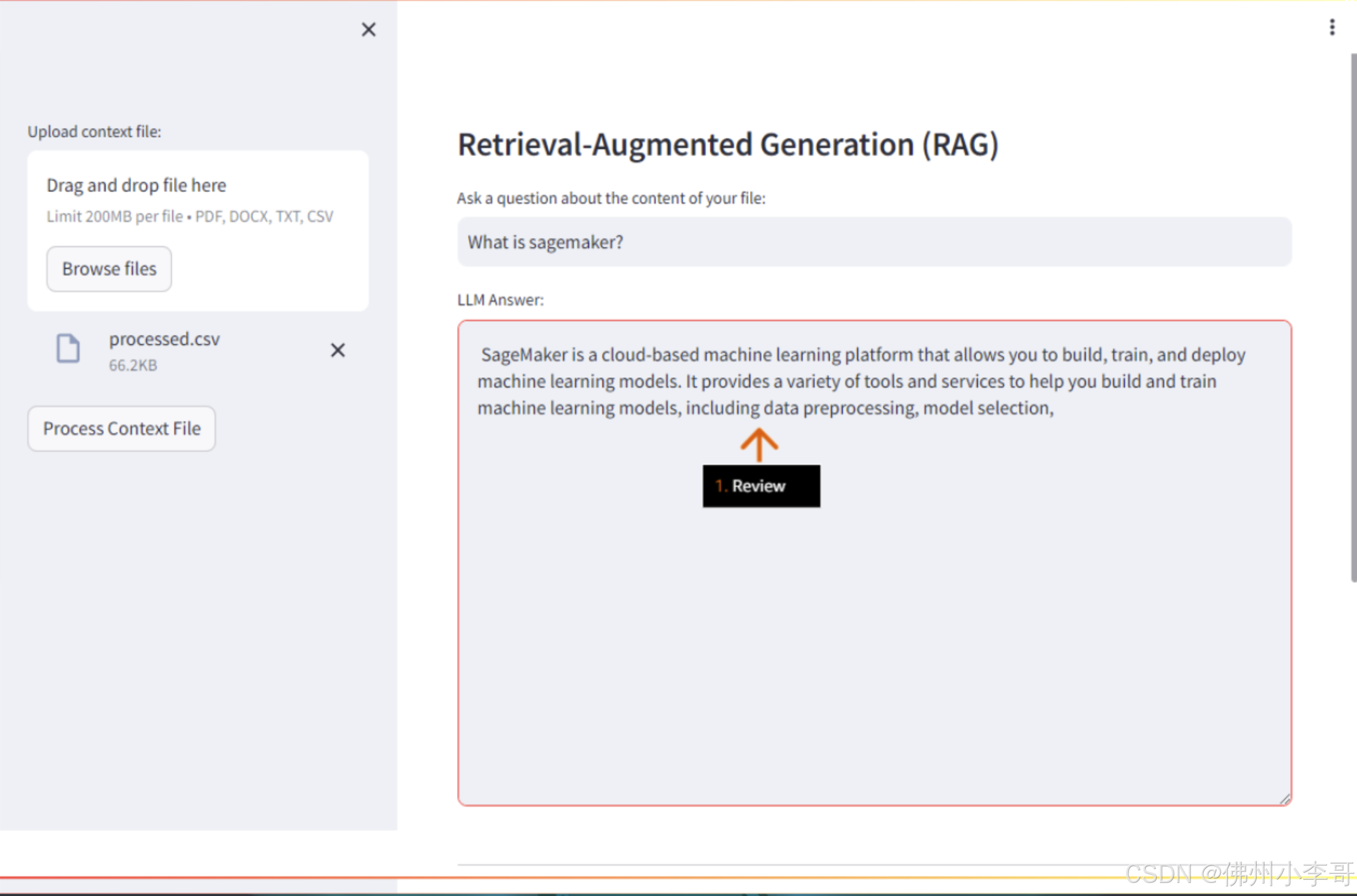
在亚马逊云科技上部署开源大模型并利用RAG和LangChain开发生成式AI应用
项目简介: 小李哥将继续每天介绍一个基于亚马逊云科技AWS云计算平台的全球前沿AI技术解决方案,帮助大家快速了解国际上最热门的云计算平台亚马逊云科技AWS AI最佳实践,并应用到自己的日常工作里。 本次介绍的是如何在亚马逊云科技上利用Sag…...

Spring——Bean的生命周期
Bean的生命周期牵扯到Bean的实例化、属性赋值、初始化、销毁 其中Bean的实例化有四种方法、构造器实例化、静态工厂、实例工厂、实现FactoryBean接口 对于Bean的生命周期我们可以在Bean初始化之后、销毁之前对Bean进行控制 两种方法: 一、配置 1、在Bean的对象…...
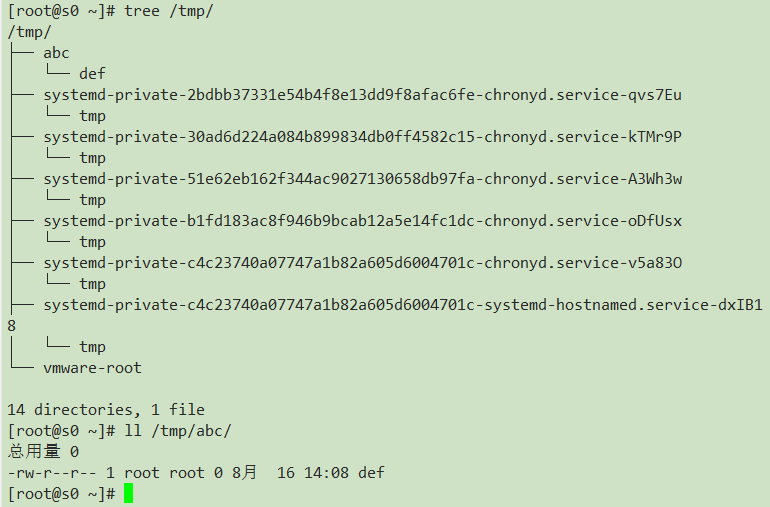
云计算实训30——自动化运维(ansible)
自动化运维 ansible----自动化运维工具 特点: 部署简单,使用ssh管理 管理端与被管理端不需要启动服务 配置简单、功能强大,扩展性强 一、ansible环境搭建 准备四台机器 安装步骤 mo服务器: #下载epel [rootmo ~]# yum -y i…...

网络性能优化:从问题诊断到解决方案
网络性能优化是确保网络高效、稳定运行的关键过程,它通过改进网络设备、协议和配置,以提高网络吞吐量、降低延迟并提升用户体验。在网络性能优化的全过程中,从问题诊断到解决方案的实施,需要经过一系列详细的步骤和策略。本文将从…...

深度学习10--强化学习
强化学习(增强学习、再励学习、评价学习简称RL)是近年来机器学习领域最热门的方向之一,是实现通用人工智能的重要方法之一。本章将通俗易懂地讲一下强化学习中的两个重要的模型DQN 和DDPG。 马尔可夫决策过程(Markov Decison Process,MDP)包括两个对象ÿ…...
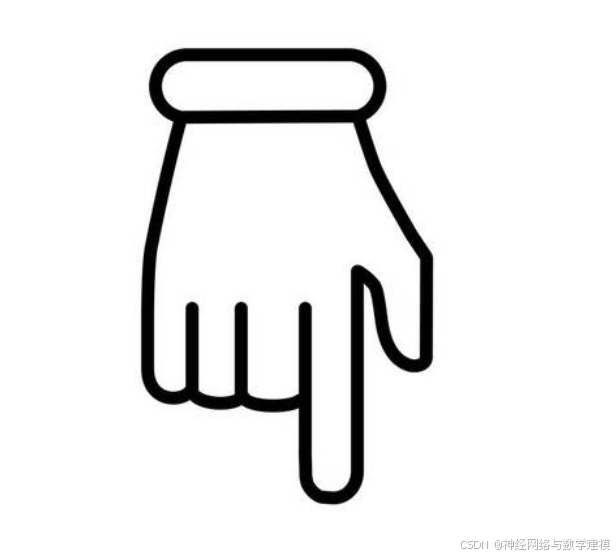
SSA-SVM多变量回归预测|樽海鞘群优化算法-支持向量机|Matalb
目录 一、程序及算法内容介绍: 基本内容: 亮点与优势: 二、实际运行效果: 三、算法介绍: 四、完整程序下载: 一、程序及算法内容介绍: 基本内容: 本代码基于Matlab平台编译&a…...
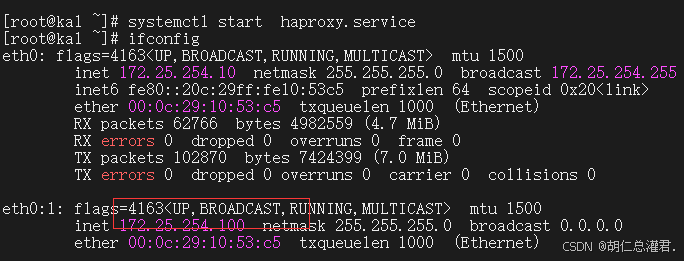
KEEPALIVED高可用集群知识大全
目录 一、KEEPALIVED高可用集群简介 1、Keepalived 高可用集群的工作原理 2、Keepalived 高可用集群的作用 二、KEEPALIVED部署 1、网络配置 2、软件安装与启动 3、配置虚拟路由器 4、效果实现 三、启用keepalived日志功能 四、KEEPALIVED的几种工作模式 1、KEEPALI…...
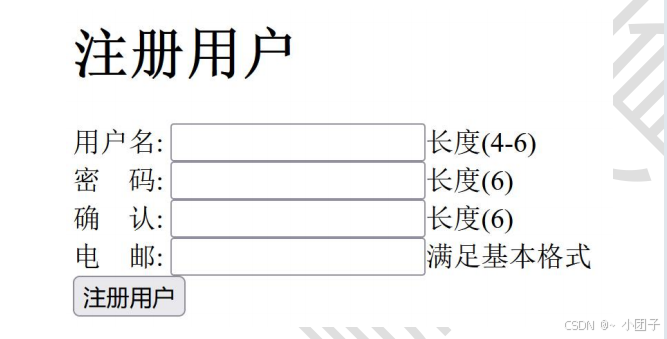
JavaWeb系列三: JavaScript学习 下
JavaScript学习 数组学习数组定义数组使用和遍历 js函数快速入门函数定义方式方式1: function关键字定义函数方式2: 将函数赋给变量 js函数注意事项和细节js函数练习 js自定义对象方式1: Object形式方式2: {}形式 事件基本介绍事件分类onload加载完成事件onclick单击事件onblur…...
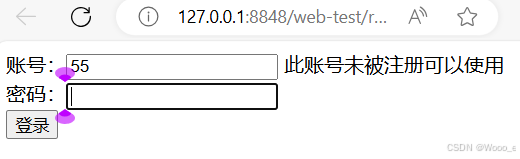
web开发,过滤器,前后端交互
目录 web开发概述 web开发环境搭建 Servlet概述 Servlet的作用: Servlet创建和使用 Servlet生命周期 http请求 过滤器 过滤器的使用场景: 通过Filter接口来实现: 前后端项目之间的交互: 1、同步请求 2、异步请求 优化…...
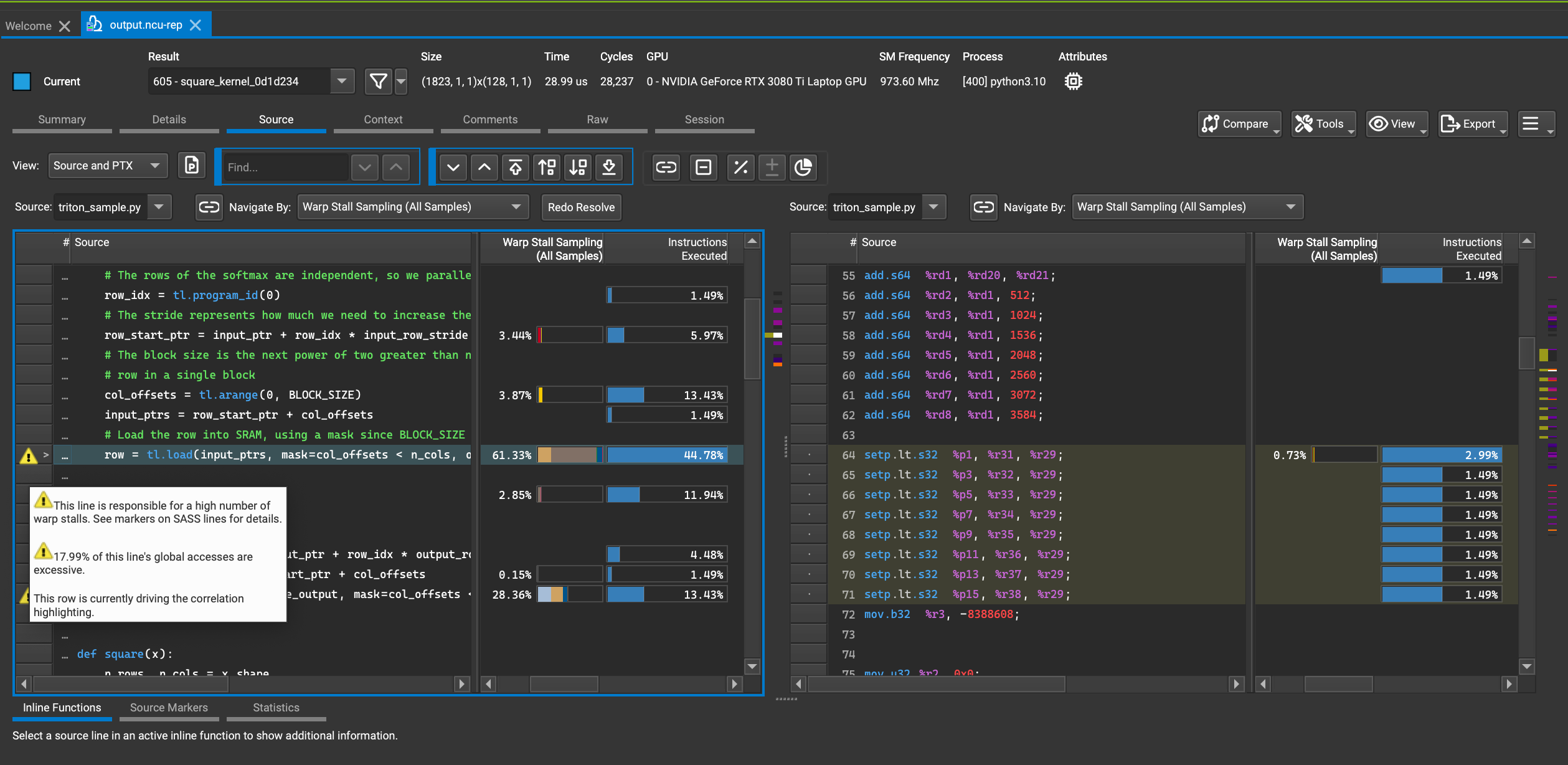
CUDA-MODE 第一课课后实战(下)
我的课程笔记,欢迎关注:https://github.com/BBuf/how-to-optim-algorithm-in-cuda/tree/master/cuda-mode CUDA-MODE 第一课课后实战(下) Nsight Compute Profile结果分析 继续对Nsight Compute的Profile结果进行分析࿰…...
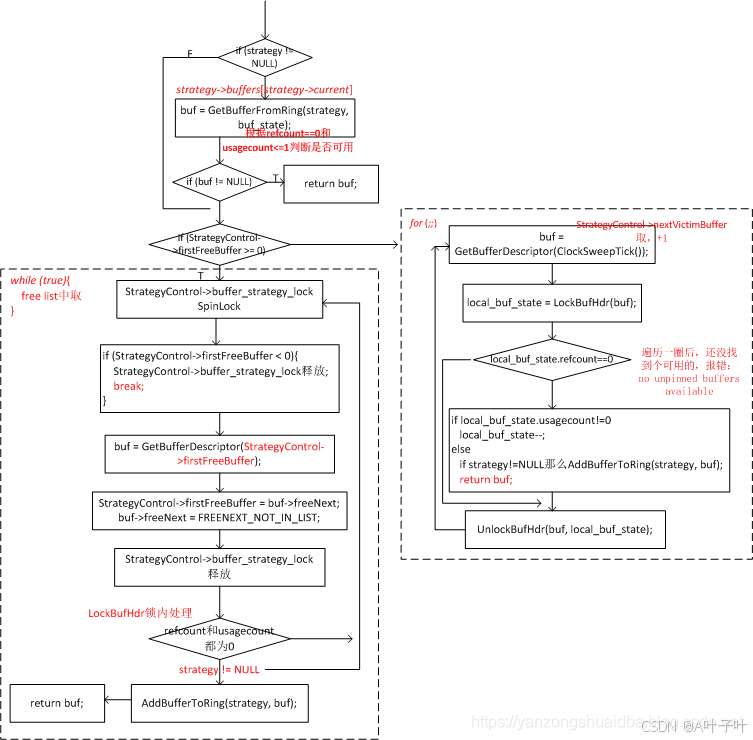
PostgreSQL数据库内核(三):缓冲区管理器
文章目录 共享缓冲区基础知识逻辑读和物理读LRU算法和CLOCK时钟算法 共享缓冲区管理器结构共享缓冲表层共享缓冲区描述符层共享缓冲页层 共享缓冲区管理器工作流程初始化缓冲区读缓冲区淘汰策略共享缓冲区锁 共享缓冲区基础知识 通常数据库系统都会在内存中预留buffer缓冲空间…...
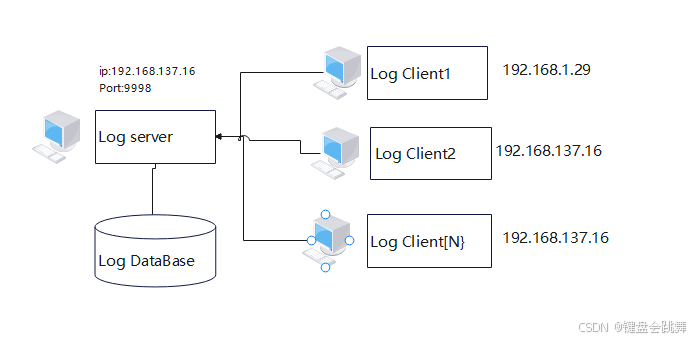
[log4cplus]: 快速搭建分布式日志系统
关键词: 日志系统 、日志分类、自动分文件夹、按时间(月/周/日/小时/分)轮替 一、引言 这里我默认看此文的我的朋友们都已经具备一定的基础,所以,我们本篇不打算讲关于log4cplus的基础内容,文中如果涉及到没有吃透的点,需要朋友们动动自己聪明的脑袋和发财的手指,进一…...
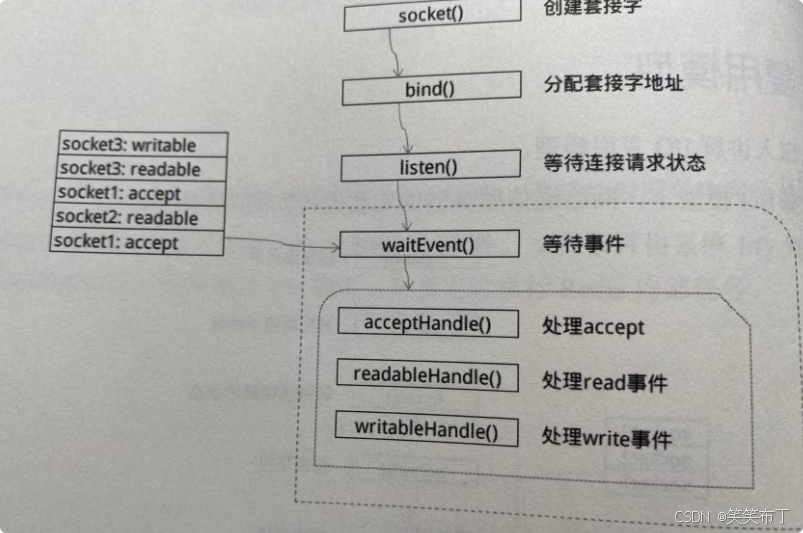
redis I/O复用机制
I/O复用模型 传统阻塞I/O模型 串行化处理,就是要等,假如进行到accept操作,cpu需要等待客户端发送的数据到tcp接收缓冲区才能进行read操作,而在此期间cpu不能执行任何操作。 I/O复用 用一个进程监听大量连接,当某个连…...

Adobe PhotoShop - 制图操作
1. 排布照片 菜单 - 视图 - 对齐:打开后图层将会根据鼠标的移动智能对齐 菜单 - 视图 - 标尺:打开后在页面出现横纵标尺,方便图层的对齐与排列 2. 自动生成全景照 在日常处理中,我们常常想要将几张图片进行拼接获得一张全景图&…...

Mysql 中的Undo日志
在 MySQL 的 InnoDB 存储引擎中,Undo Log 是用于实现数据库事务的回滚功能的一种日志。Undo Log 记录了对数据的修改,以便在事务出现问题时可以恢复到之前的状态。下面将介绍 Undo Log 的结构和样本数据。 Undo Log 的基本概念 目的: Undo Log 的主要目…...
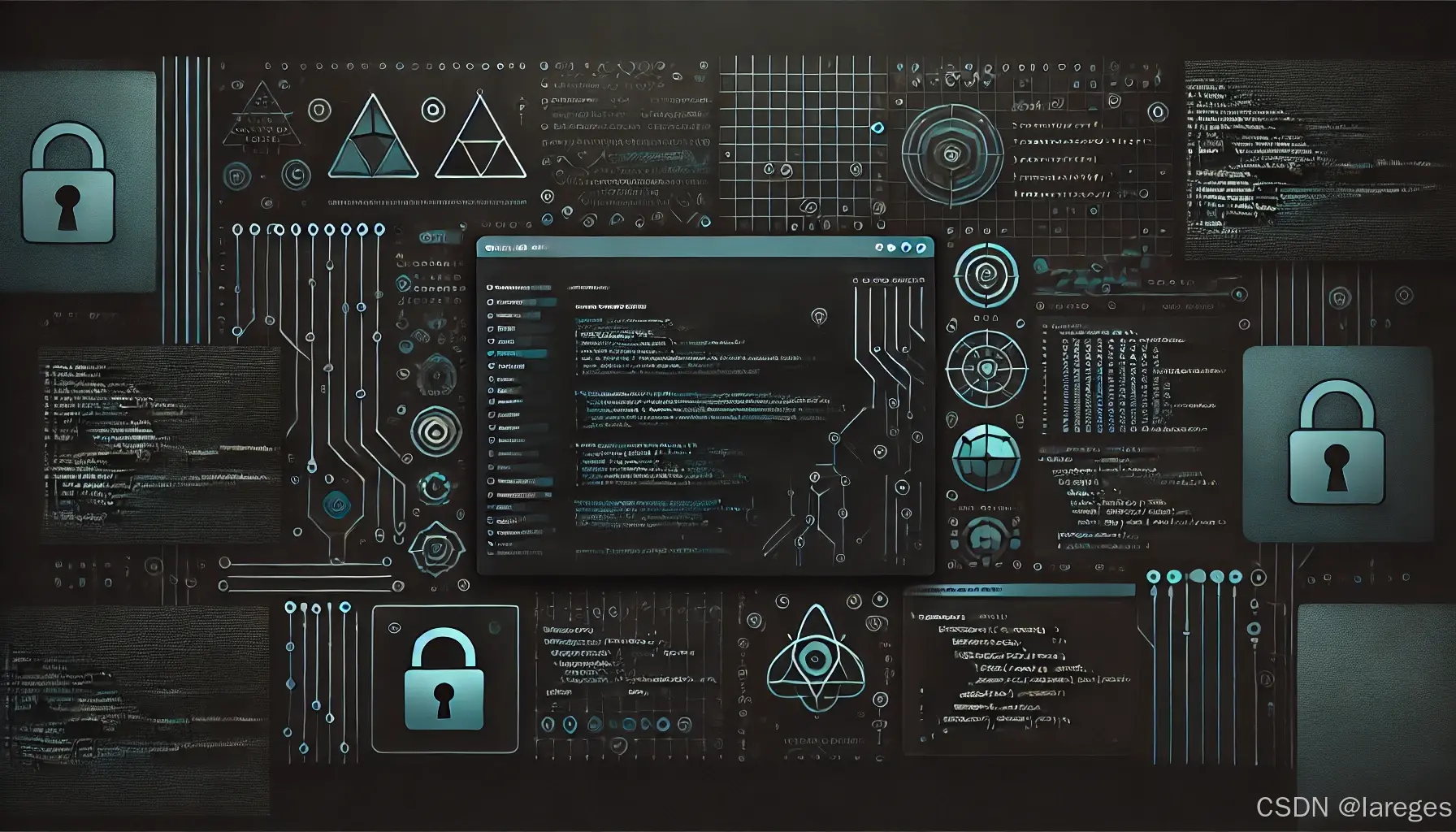
虹软科技25届校招笔试算法 A卷
目录 1. 第一题2. 第二题3. 论述题 ⏰ 时间:2024/08/18 🔄 输入输出:ACM格式 ⏳ 时长:2h 本试卷分为不定项选择,编程题,必做论述题和选做论述题,这里只展示编程题和必做论述题,一共三…...
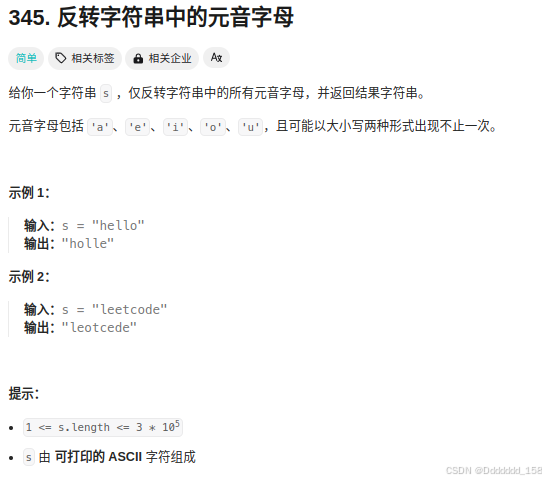
C++ | Leetcode C++题解之第345题反转字符串中的元音字母
题目: 题解: class Solution { public:string reverseVowels(string s) {auto isVowel [vowels "aeiouAEIOU"s](char ch) {return vowels.find(ch) ! string::npos;};int n s.size();int i 0, j n - 1;while (i < j) {while (i < …...
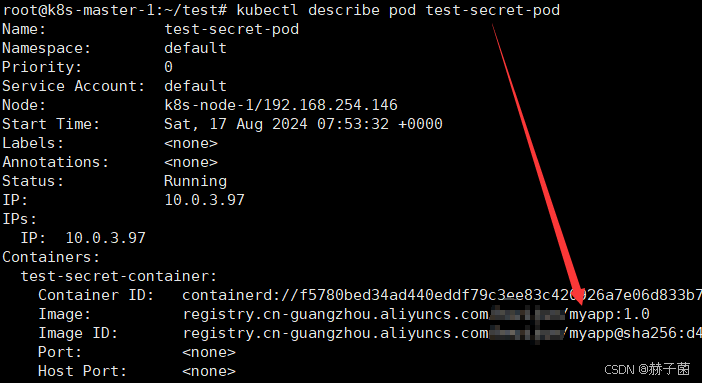
Kubernetes拉取阿里云的私人镜像
前提条件 登录到阿里云控制台 拥有阿里云的ACR服务 创建一个命名空间 获取仓库的访问凭证(可以设置固定密码) 例如 sudo docker login --usernameyourAliyunAccount registry.cn-guangzhou.aliyuncs.com 在K8s集群中创建一个secret 使用kubectl命令行…...
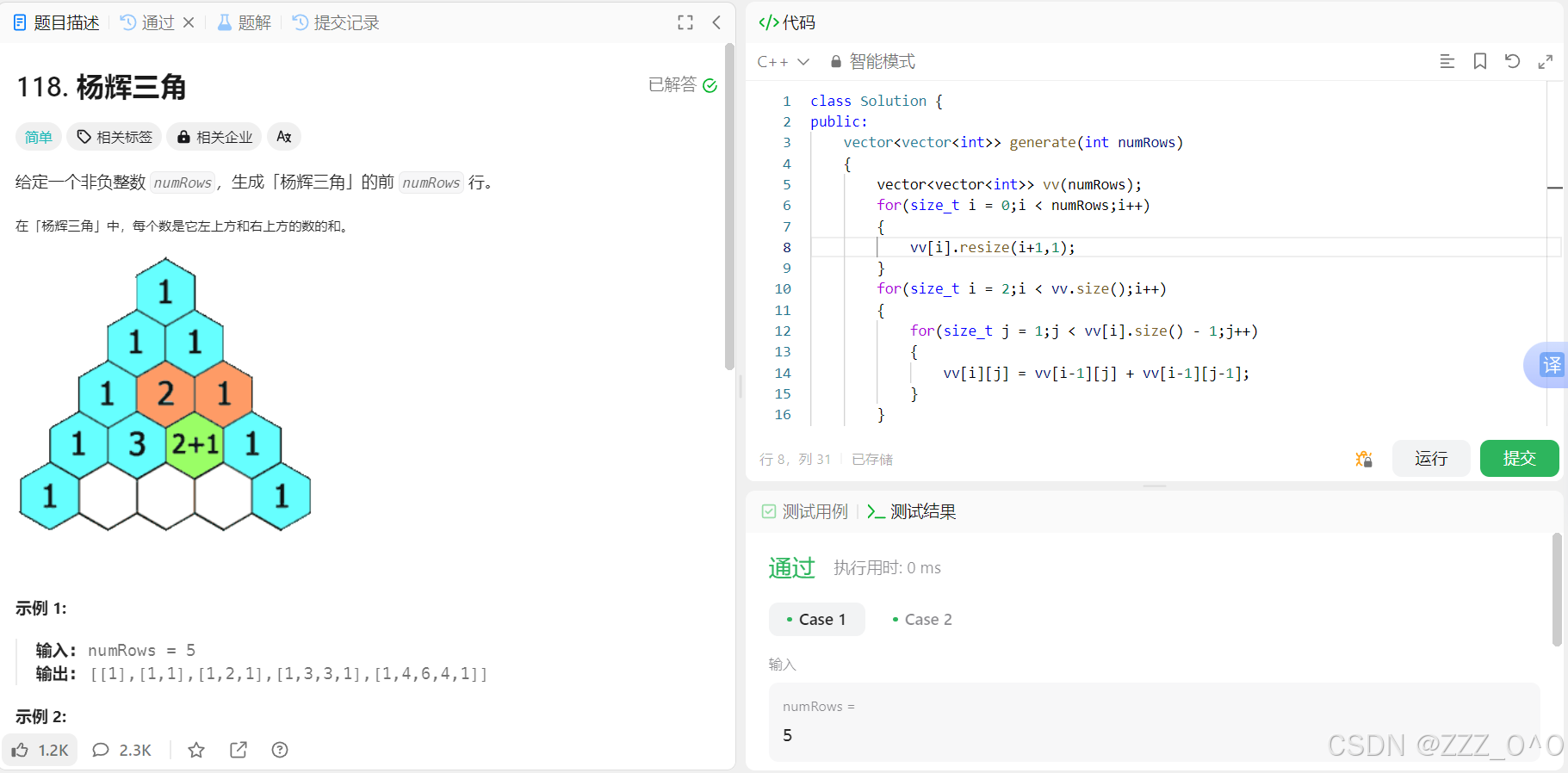
Leetcode每日刷题之118.杨辉三角
1.题目解析 杨辉三角作为一个经典的数学模型,其基本原理相信大家已经耳熟能详,这里主要是在学习了vector之后,对于本题有了新的解法,更加简便。关于vector的基本使用详见 面向对象程序设计(C)之 vector(初阶࿰…...

【ARM 芯片 安全与攻击 5.2 -- 芯片中侧信道攻击与防御方法介绍】
文章目录 什么是 Speculation Barriers?如何使用 Speculation Barriers?什么是 PAN?如何启用 PAN?使用 PAN 保护操作系统Spectre 攻击防御示例Meltdown 攻击防御示例Summary什么是 Speculation Barriers? Speculation Barriers,是一种防止处理器在投机执行中泄漏敏感信息…...
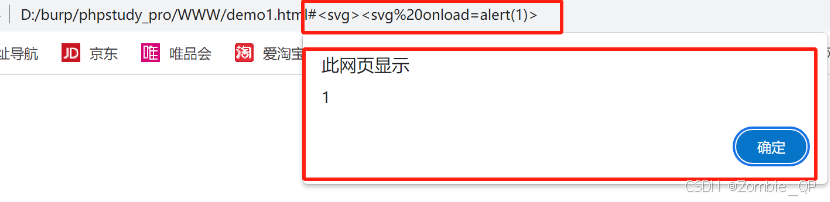
XSS-games
XSS 1.XSS 漏洞简介2.XSS的原理3.XSS的攻击方式4.XSS-GAMESMa SpaghetJefffUgandan KnucklesRicardo MilosAh Thats HawtLigmaMafiaOk, BoomerWW3svg 1.XSS 漏洞简介 XSS又叫CSS(Cross Site Script)跨站脚本攻击是指恶意攻击者往Web页面里插入恶意Sc…...
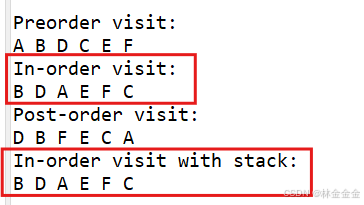
日撸Java三百行(day25:栈实现二叉树深度遍历之中序遍历)
目录 一、栈实现二叉树遍历的可行性 二、由递归推出栈如何实现中序遍历 1.左子树入栈 2.根结点出栈 3.右子树入栈 4.实例说明 三、代码实现 总结 一、栈实现二叉树遍历的可行性 在日撸Java三百行(day16:递归)中,我们讲过…...
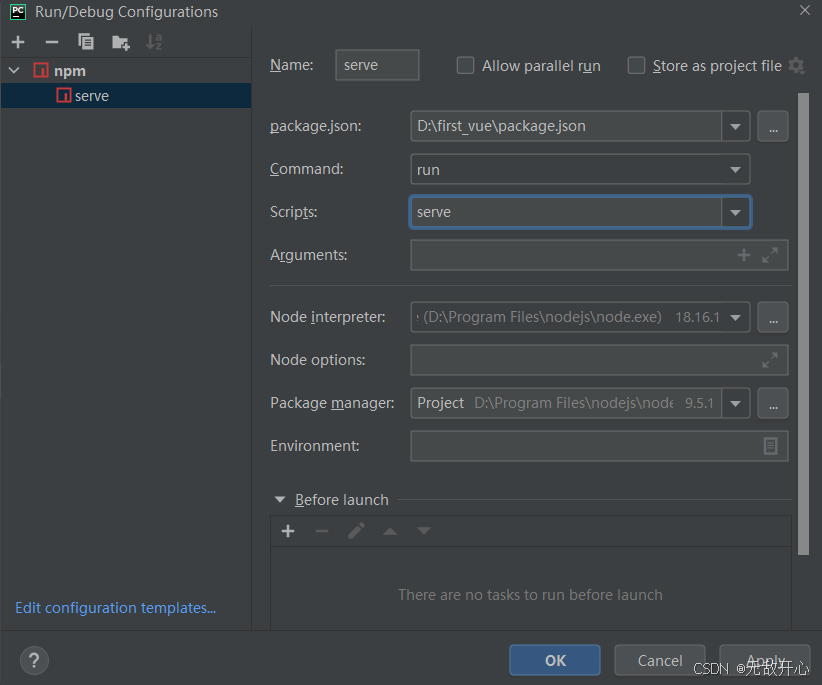
【vue讲解:ref属性、动态组件、插槽、vue-cli创建项目、vue项目目录介绍、vue项目开发规范、es6导入导出语法】
0 ref属性(组件间通信) # 1 ref属性放在普通标签上<input type"text" v-model"name" ref"myinput">通过 this.$refs[myinput] 拿到的是 原生dom对象操作dom对象:改值,换属性。。。# 2 ref属…...

ubuntu:最新安装使用docker
前言 系统:ubuntu 22.04 desktop 目的:安装使用docker 安装小猫猫 没有安装包的,可以自己去瞅瞅,这里不提供下载方式 sudo dpkg -i ./cat-verge_1.7.5_amd64.deb 在应用里,打开这个软件,并开启系统猫猫 配…...

Linux ssh 免密失效
sudo chmod -R 777 /home/xxx sudo chown -R xxx:xxx /home/xxx 为什么我输入这两条指令后,ssh免密失效了? 当你使用 sudo chmod -R 777 /home/xxx 和 sudo chown -R xxx:xxx /home/xxx 这两条指令后,可能会导致 SSH 免密登录失效的原因有以…...

k8s上部署ingress-controller
一、安装helm仓库 # helm pull ingress-nginx/ingress-nginx 二、修改 三、运行 # kubectl label nodes node01.110111.cn ingresstrue# kubectl label nodes node02.110112.cn ingresstrue# helm upgrade --install ingress-nginx -n ingress-nginx . -f values.yaml 四、检…...

Android 13 about launcher3 (1)
Android 13 Launcher3 android13#launcher3#分屏相关 Launcher3修改 wm density界面布局不改变 /packages/apps/Launcher3/src/com/android/launcher3/InvariantDeviceProfile.java Launcher的默认配置加载类,通过InvariantDeviceProfile方法可以看出,…...