Unity自定义数组在Inspector窗口的显示方式
了解
- 单行高度:EditorGUIUtility.singleLineHeight
- 获取 PropertyField 控件所需的高度:EditorGUI.GetPropertyHeight
- 属性是否在Inspector窗口展开:SerializedProperty.isExpanded
- 可重新排序列表类:ReorderableList
- 绘制纯色矩形:EditorGUI.DrawRect
示例
使用版本:2021.3.6f1c1
此版本的Unity已经实现数组元素添加,移除,移动功能。
自定义类
[System.Serializable]
public class Test
{public string name;public int age;
}
数据类
定义一个数组,数组元素为自定义类
using System.Collections.Generic;
using UnityEngine;
[CreateAssetMenu]
public class TestSO : ScriptableObject
{public List<Test> testList;
}
数据类文件默认显示如下
修改数据类显示
数据元素内容显示为一行
注意:不能使用自动布局api
using UnityEngine;
using UnityEditor;
[CustomPropertyDrawer(typeof(Test))]
public class TestDrawer : PropertyDrawer
{public override void OnGUI(Rect position, SerializedProperty property, GUIContent label){var spName = property.FindPropertyRelative("name");var spAge = property.FindPropertyRelative("age");var rect1 = position;var rect2 = position;var indentlevel = EditorGUI.indentLevel;var lableWidth = EditorGUIUtility.labelWidth;EditorGUI.indentLevel = 2;EditorGUIUtility.labelWidth = 60;rect1.width = position.width / 2;rect1.height = EditorGUIUtility.singleLineHeight;rect2.width = position.width / 2;rect2.height = EditorGUIUtility.singleLineHeight;rect2.x = position.width / 2+40;rect2.y = rect1.y;EditorGUI.PropertyField(rect1, spName, new GUIContent("名字"));EditorGUI.PropertyField(rect2, spAge, new GUIContent("年龄"));EditorGUI.indentLevel = indentlevel;EditorGUIUtility.labelWidth = lableWidth;//EditorGUI.DrawRect(rect1, Color.green);//EditorGUI.DrawRect(rect2, Color.gray);}//获取属性高度public override float GetPropertyHeight(SerializedProperty property, GUIContent label){return base.GetPropertyHeight(property, label);}
}
修改后显示效果如下图所示
TestList修改为指定名称;Element修改为指定名称
using UnityEngine;
using UnityEditor;
using UnityEditorInternal;
[CustomEditor(typeof(TestSO))]
public class TestSOEditor : Editor
{SerializedProperty sPTestList;ReorderableList arrayList;private void OnEnable(){sPTestList = serializedObject.FindProperty("testList");if (arrayList == null){arrayList = new ReorderableList(serializedObject, sPTestList, true, true, true, true); //绘制HeaderarrayList.drawHeaderCallback += DrawHeader;//绘制数组元素arrayList.drawElementCallback += DrawElement;//获取数组的高度arrayList.elementHeightCallback += DrawElementHeight;}}void DrawHeader(Rect rect){EditorGUI.LabelField(rect, "测试列表");}void DrawElement(Rect rect, int index, bool isActive, bool isFocused){var element = sPTestList.GetArrayElementAtIndex(index);var arrowRect = rect;int indentLevel = EditorGUI.indentLevel;EditorGUI.indentLevel = 1;arrowRect.height = EditorGUIUtility.singleLineHeight;element.isExpanded = EditorGUI.Foldout(arrowRect, element.isExpanded, new GUIContent("元素" + index));EditorGUI.indentLevel = indentLevel;//EditorGUI.DrawRect(rect, Color.red);//EditorGUI.DrawRect(arrowRect, Color.blue);if (element.isExpanded){rect.y += arrowRect.height;EditorGUI.PropertyField(rect, element);}}float DrawElementHeight(int index){var element = sPTestList.GetArrayElementAtIndex(index);var height = EditorGUIUtility.singleLineHeight;//折叠箭头的高度 if (element.isExpanded)//如果元素展开 获取展开内容的高度和箭头的高度之和height += EditorGUI.GetPropertyHeight(element);return height;}public override void OnInspectorGUI(){serializedObject.Update();arrayList.DoLayoutList();serializedObject.ApplyModifiedProperties();}
}
修改后如下图所示
示例二
高度计算放在PropertyDrawer的OnGUI中
自定义类
显示折叠箭头,数据元素内容显示为一行,Element修改为指定名称
using UnityEngine;
using UnityEditor;
[CustomPropertyDrawer(typeof(Test))]
public class TestDrawer : PropertyDrawer
{public override void OnGUI(Rect position, SerializedProperty property, GUIContent label){var spName = property.FindPropertyRelative("name");var spAge = property.FindPropertyRelative("age");var rect0 = position;rect0.height = EditorGUIUtility.singleLineHeight;var indentlevel = EditorGUI.indentLevel;EditorGUI.indentLevel = 1;property.isExpanded = EditorGUI.Foldout(rect0, property.isExpanded, label);EditorGUI.indentLevel = indentlevel;if (property.isExpanded){var rect1 = position;var rect2 = position;indentlevel = EditorGUI.indentLevel;var lableWidth = EditorGUIUtility.labelWidth;EditorGUI.indentLevel = 2;EditorGUIUtility.labelWidth = 60;rect1.width = position.width / 2;rect1.height = EditorGUIUtility.singleLineHeight;rect1.y += EditorGUIUtility.singleLineHeight;rect2.width = position.width / 2;rect2.height = EditorGUIUtility.singleLineHeight;rect2.x = position.width / 2 + 40;rect2.y = rect1.y;EditorGUI.PropertyField(rect1, spName, new GUIContent("名字"));EditorGUI.PropertyField(rect2, spAge, new GUIContent("年龄"));EditorGUI.indentLevel = indentlevel;EditorGUIUtility.labelWidth = lableWidth;//EditorGUI.DrawRect(rect1, Color.green);//EditorGUI.DrawRect(rect2, Color.gray);}}public override float GetPropertyHeight(SerializedProperty property, GUIContent label){if (property.isExpanded)return EditorGUIUtility.singleLineHeight * 2;elsereturn EditorGUIUtility.singleLineHeight;}
}
数据类
TestList修改为指定名称
using UnityEngine;
using UnityEditor;
using UnityEditorInternal;
[CustomEditor(typeof(TestSO))]
public class TestSOEditor : Editor
{SerializedProperty sPTestList;ReorderableList arrayList;private void OnEnable(){sPTestList = serializedObject.FindProperty("testList");if (arrayList == null){arrayList = new ReorderableList(serializedObject, sPTestList, true, true, true, true);//绘制HeaderarrayList.drawHeaderCallback += DrawHeader;//绘制数组元素arrayList.drawElementCallback += DrawElement;//绘制元素高度arrayList.elementHeightCallback += DrawHeight;void DrawHeader(Rect rect){EditorGUI.LabelField(rect, "测试列表");}void DrawElement(Rect rect, int index, bool isActive, bool isFocused){//参数会传递给PropertyDrawer的OnGUI EditorGUI.PropertyField(rect, sPTestList.GetArrayElementAtIndex(index), new GUIContent("元素 " + index));}float DrawHeight(int index){return EditorGUI.GetPropertyHeight(sPTestList.GetArrayElementAtIndex(index));}}}public override void OnInspectorGUI(){serializedObject.Update();arrayList.DoLayoutList();serializedObject.ApplyModifiedProperties();}
}
参考
看完后理解列表的高度如何计算
相关文章:
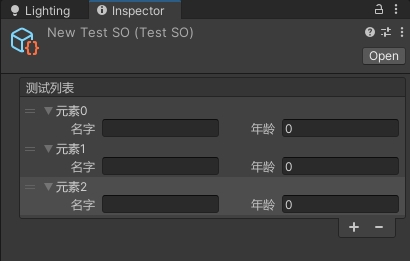
Unity自定义数组在Inspector窗口的显示方式
了解 单行高度:EditorGUIUtility.singleLineHeight获取 PropertyField 控件所需的高度:EditorGUI.GetPropertyHeight属性是否在Inspector窗口展开:SerializedProperty.isExpanded可重新排序列表类:ReorderableList绘制纯色矩形:EditorGUI.Dr…...

ERC论文阅读(03)--SPCL论文阅读笔记(2024-10-29)
SPCL论文阅读笔记 论文中心思想 这篇论文是研究ERC任务的论文,作者提出了监督原型对比学习的方法用于ERC任务。 论文 EMNLP2022 paper “Supervised Prototypical Contrastive Learning for Emotion Recognition in Conversation” 现存问题 现存的使用监督对…...

Straightforward Layer-wise Pruning for More Efficient Visual Adaptation
对于模型中冗余的参数,一个常见的方法是通过结构化剪枝方法减少参数容量。例如,基于幅度值和基于梯度的剪枝方法。尽管这些方法在传统训练上通用性,本文关注的PETL迁移有两个不可避免的问题: 显著增加了模型存储负担。由于不同的…...
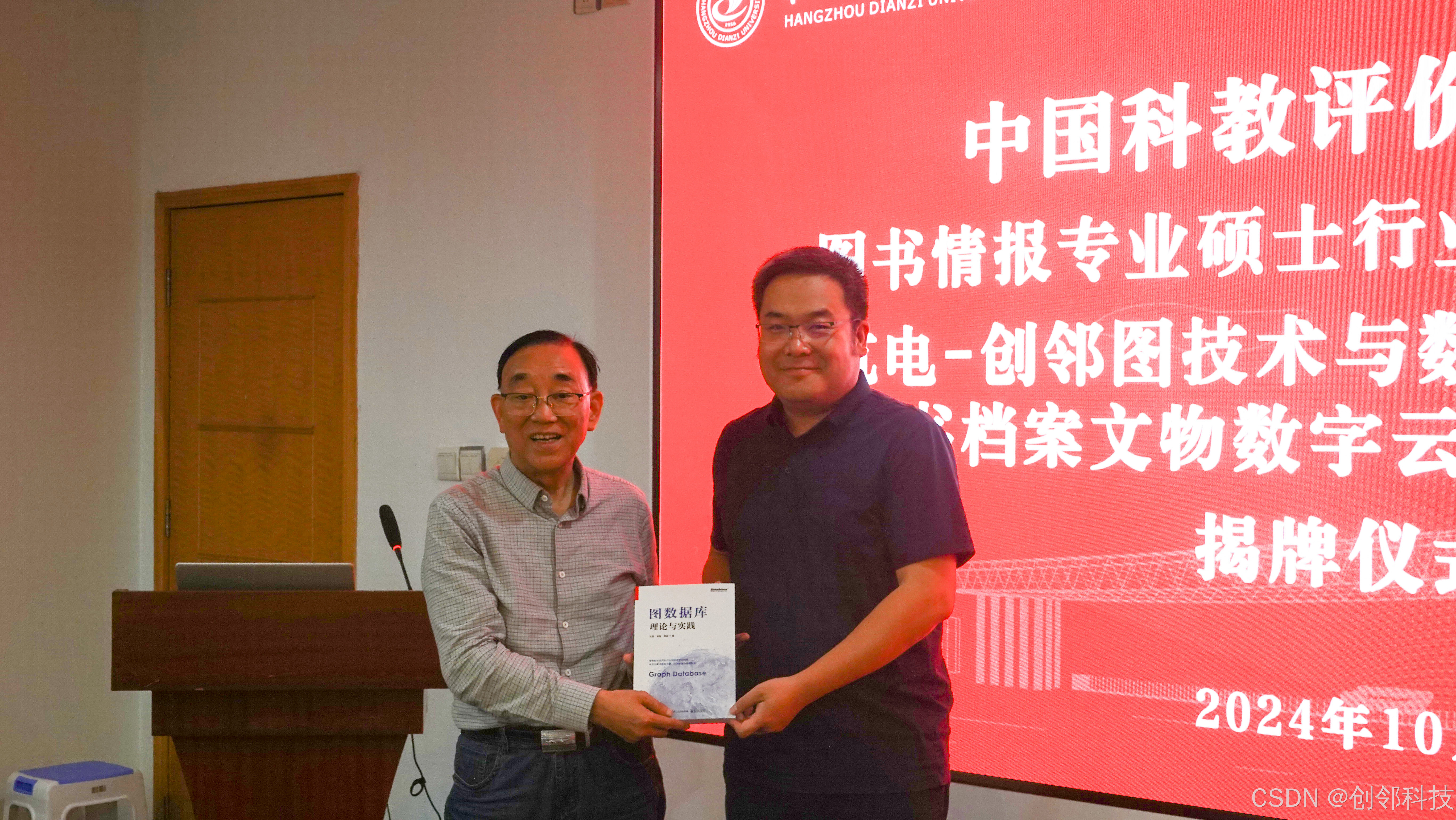
喜讯 | 创邻科技杭州电子科技大学联合实验室揭牌成立!
近日,杭州电子科技大学图书情报专业硕士行业导师聘任仪式暨杭电-创邻图技术与数字化联合实验室(图书档案文物数字云联合研发中心)揭牌仪式在杭州电子科技大学隆重举行。杭州电子科技大学原副校长吕金海、研究生院副院长潘建江,科研…...
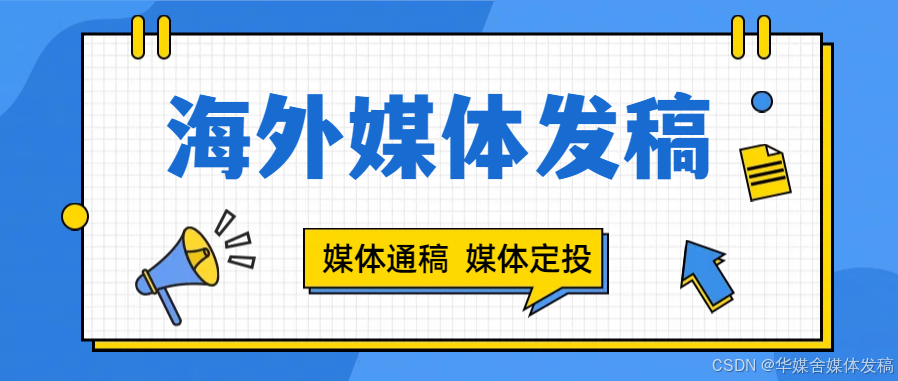
海外媒体发稿:如何打造媒体发稿策略
新闻媒体的发稿推广策略对于提升品牌知名度、吸引流量以及增加收入非常重要。本文将介绍一套在21天内打造爆款新闻媒体发稿推广策略的方法。 第一天至第七天:明确目标和定位 在这个阶段,你需要明确你的目标和定位,以便为你的新闻媒体建立一个…...

PyTorch模型保存与加载
1.保存与加载的概念(序列化与反序列化) 模型训练完毕之后,肯定想要把它保存下来,供以后使用,不需要再次去训练。 那么在pytorch中如何把训练好的模型,保存,保存之后又如何加载呢? 这就用需要序列化与反序列化,序列化与反序列化的概念如下图所示: 因为在内…...
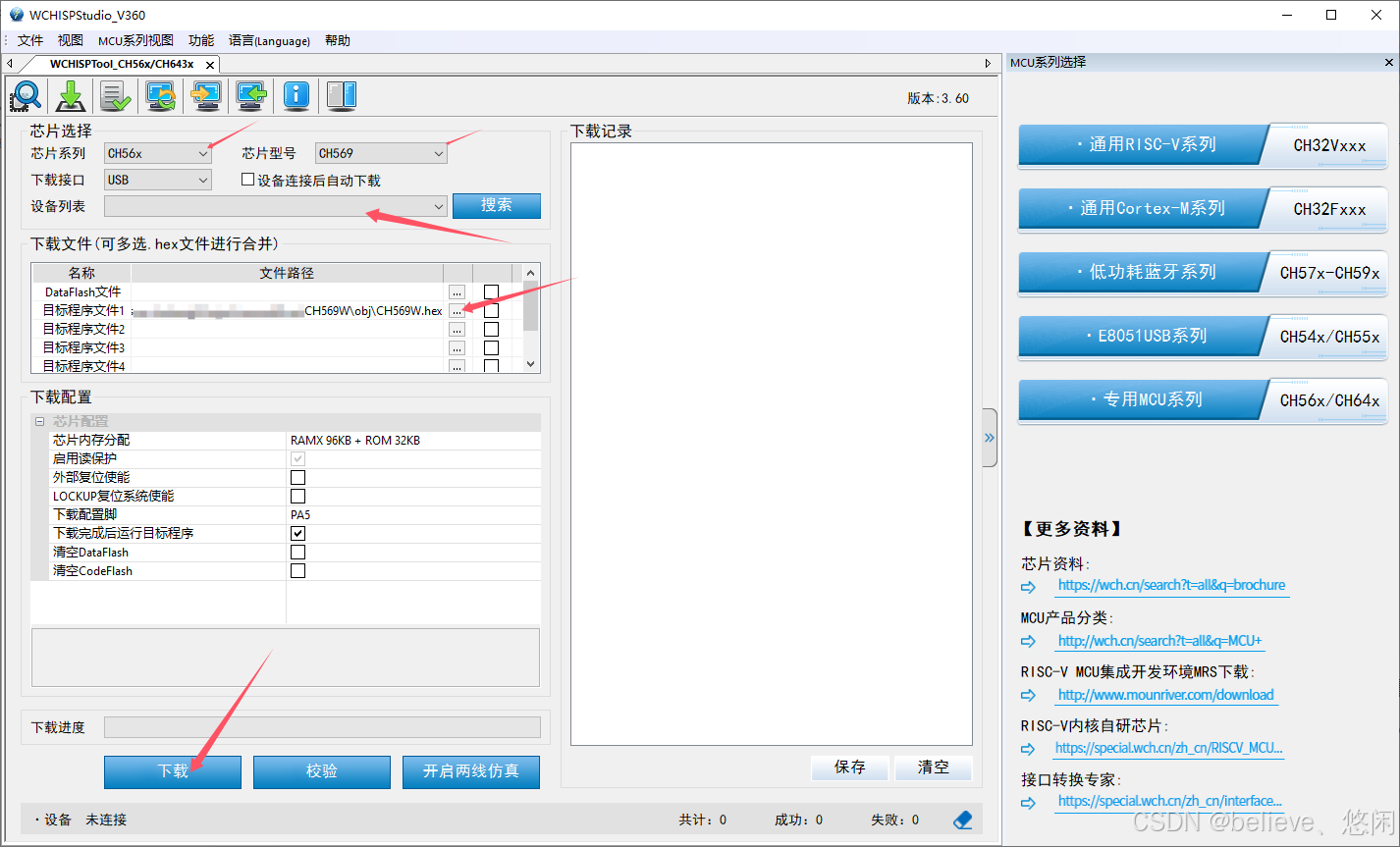
CH569开发前的测试
为了玩转准备Ch569的开发工作 ,准备了如下硬件和软件: 硬件 1.官方的 Ch569 开发板,官方买到的是两块插接在一起的;除了HSPI接口那里的电阻,这两块可以说是一样的。也意味着两块板子的开发也需要烧录两次;…...
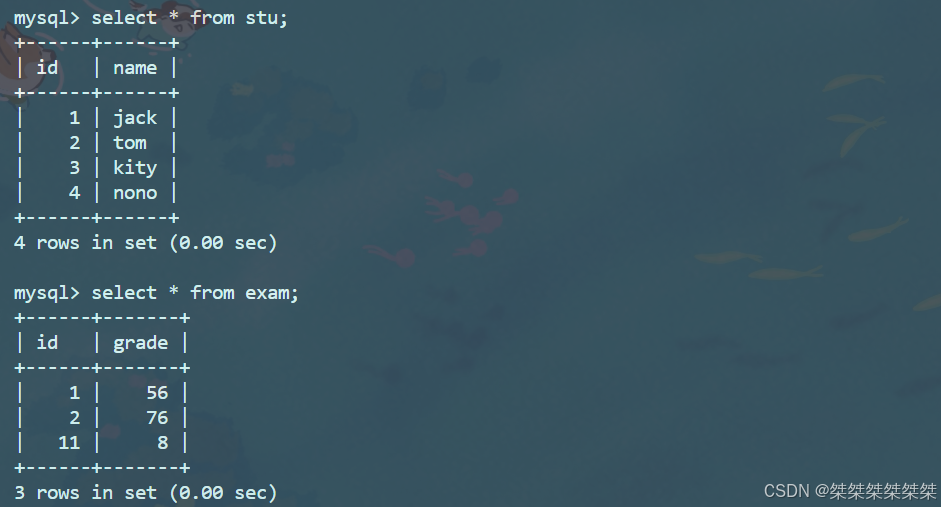
MySQL中表的外连接和内连接
内连接和外连接 表的连接分为内连接和外连接,内连接就是将需要连接的表形成笛卡尔积筛选;外连接分为左外连接和右外连接,左外连接为左侧的表需要完全显示,右外连接为右侧的表现需要完全显示。 文章目录 内连接和外连接内连接外…...
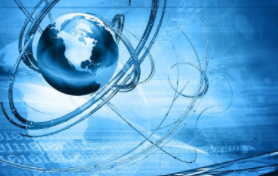
Ubuntu 上安装 Redmine 5.1 指南
文章目录 官网安装文档:命令步骤相关介绍GemRubyRailsBundler 安装 Redmine更新系统包列表和软件包:安装必要的依赖:安装 Ruby:安装 bundler下载 Redmine 源代码:安装 MySQL配置 Redmine 的数据库配置文件:…...
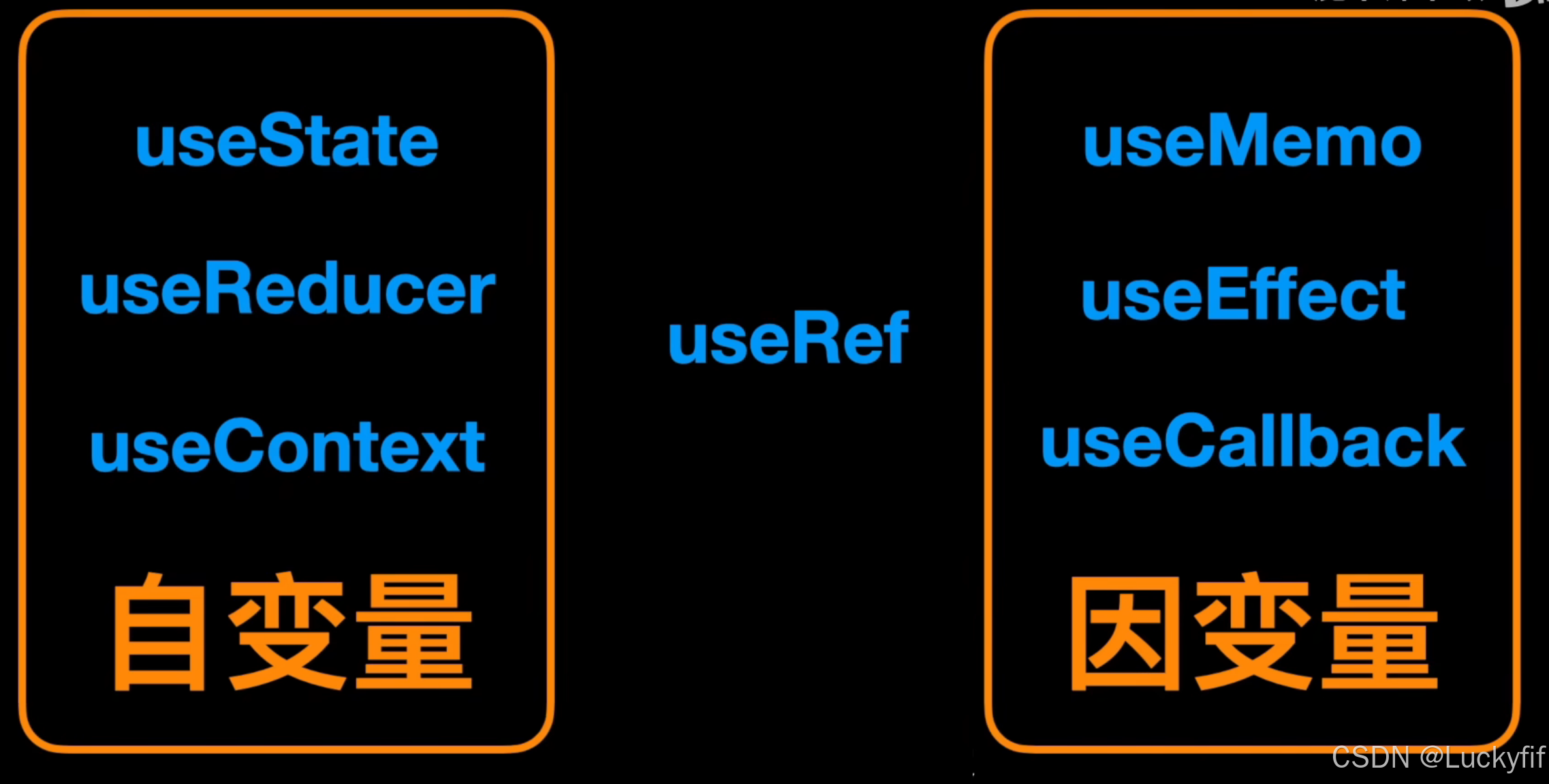
从变量的角度理解 Hooks , 变得更简单了
从变量角度理解Hooks 在React的世界里,Hooks的引入为函数式组件带来了前所未有的灵活性和能力。它们让我们得以完全摆脱class式的写法,在函数式组件中完成生命周期管理、状态管理、逻辑复用等几乎全部组件开发工作。这次,我们就从变量的角度…...
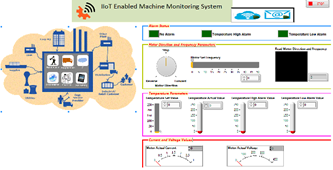
LabVIEW Modbus通讯稳定性提升
在LabVIEW开发Modbus通讯程序时,通讯不稳定是一个常见问题,可能导致数据丢失、延迟或错误。为了确保通讯的可靠性,可以从多个角度进行优化,以下是一些有效的解决方案,结合实际案例进行分析。 1. 优化通讯参数设置 通讯…...
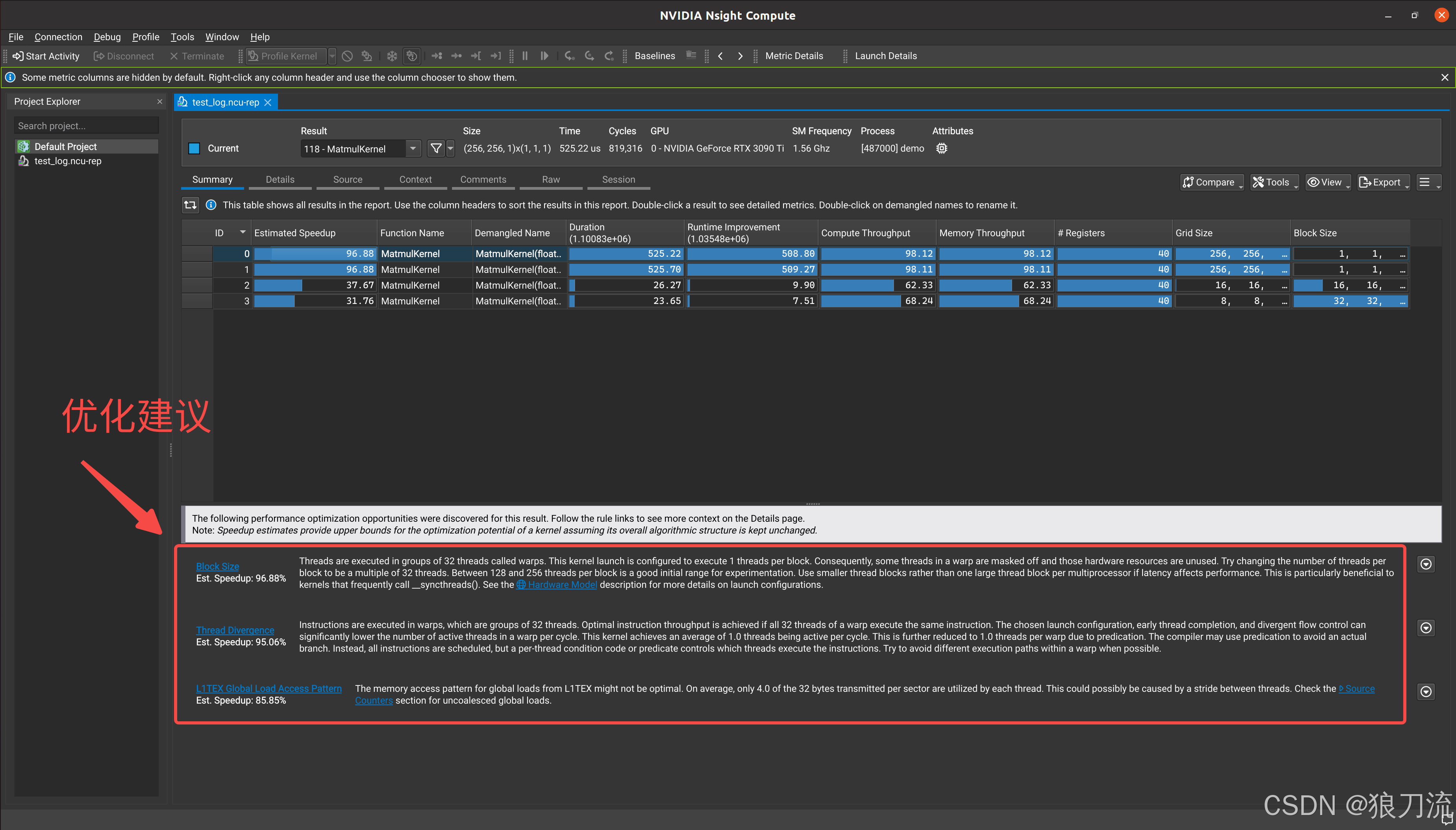
(8) cuda分析工具
文章目录 Nvidia GPU性能分析工具Nsight SystemNvidia GPU性能分析工具Nsight System Nvidia GPU性能分析工具Nsight System NVIDIA Nsight Systems是一个系统级的性能分析工具,用于分析和优化整个CUDA应用程序或系统的性能。它可以提供对应用程序整体性能的全面见…...
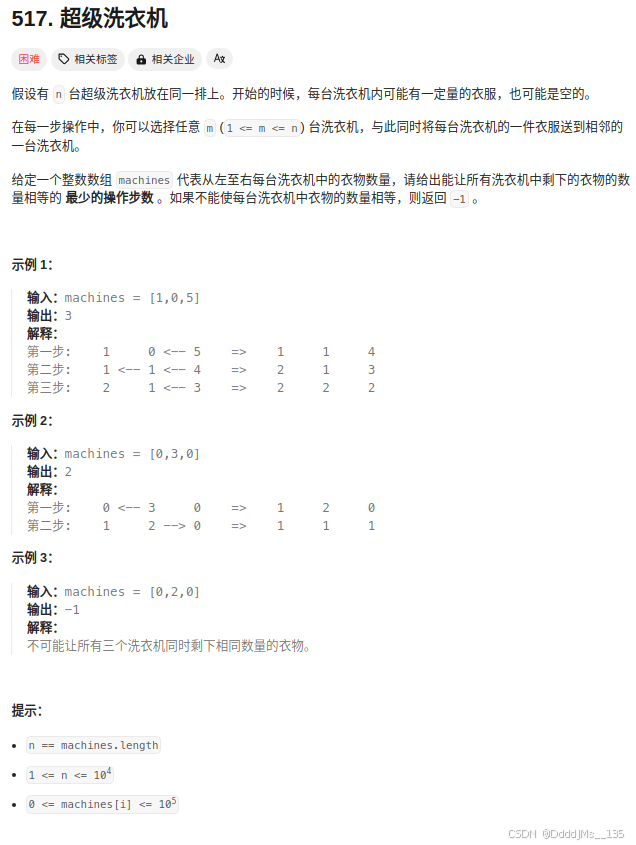
C语言 | Leetcode C语言题解之第517题超级洗衣机
题目: 题解: int findMinMoves(int* machines, int machinesSize){int sum0;for(int i0;i<machinesSize;i){summachines[i];}if(sum%machinesSize!0){return -1;}int psum/machinesSize;int ans0;int cur0;for(int i0;i<machinesSize;i){cur(mac…...
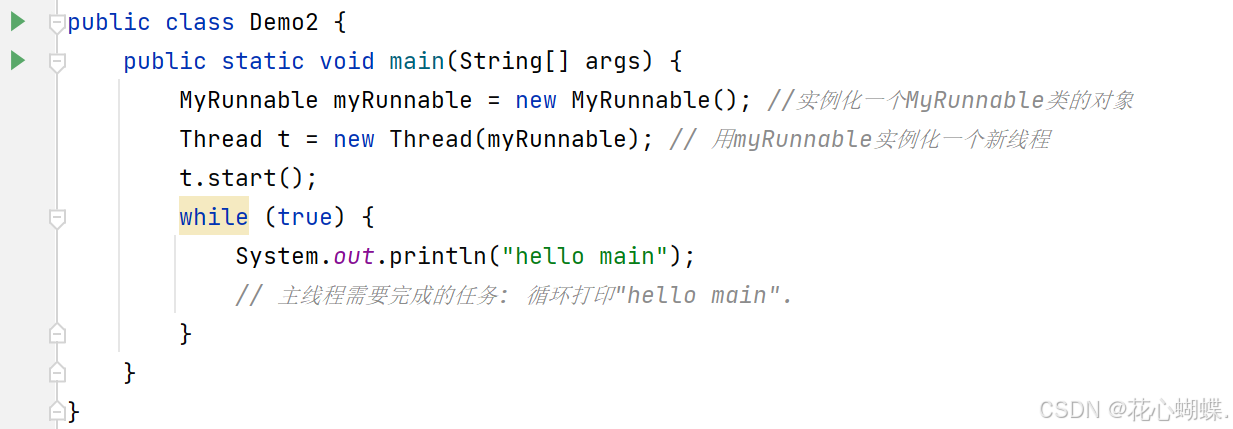
Java多线程编程基础
目录 编写第一个多线程程序 1. 方式一 : 继承Thread类, 重写run方法 2. 方式二: 实现Runnable接口, 重写run方法 3. 方式三: 使用Lambda表达式 [匿名内部类] [Lambda表达式] 在上个文章中, 我们了解了进程和线程的相关概念. 那么, 在Java中, 我们如何进行多线程编程呢? …...
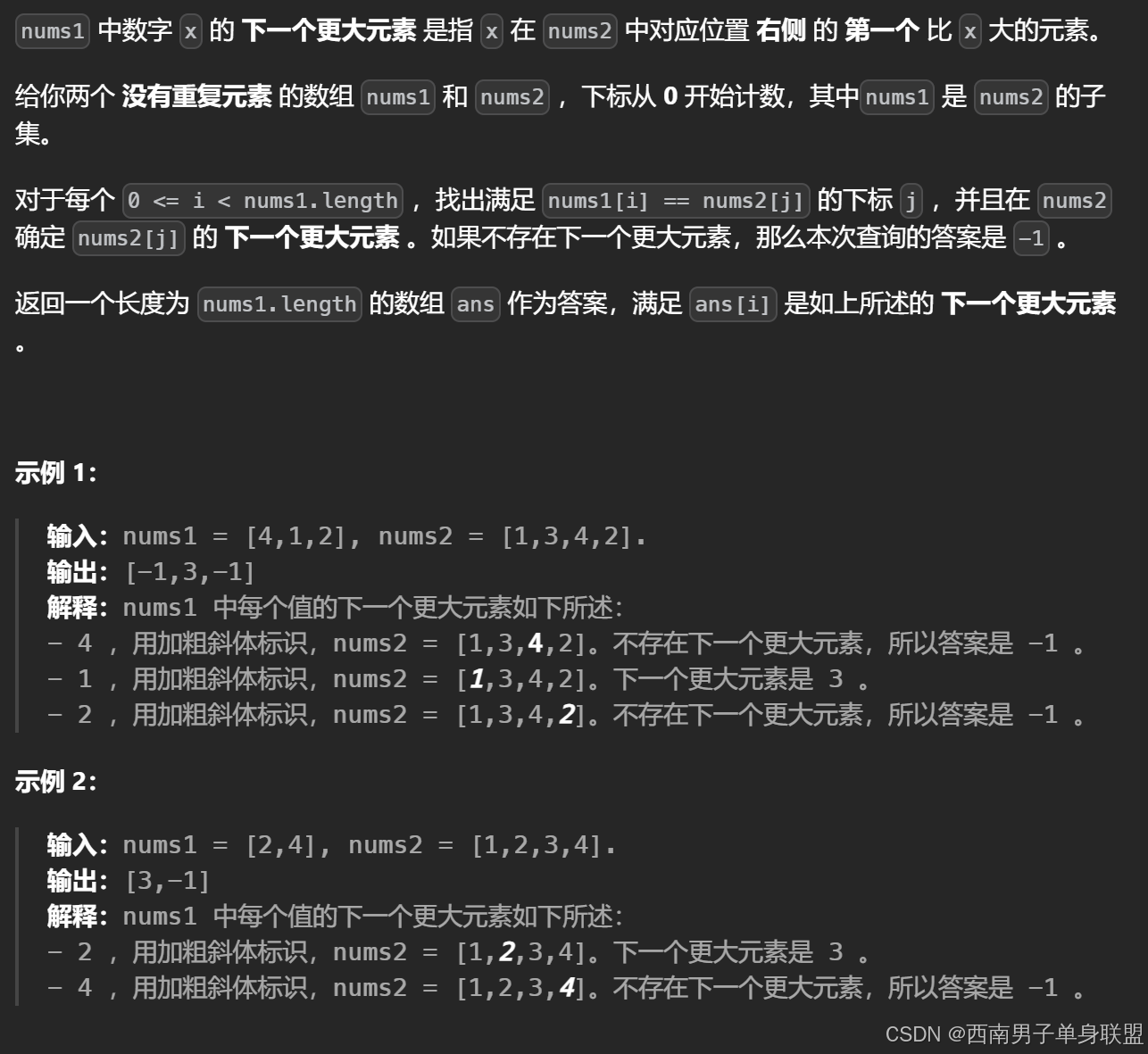
刷代随有感(134):单调栈——下一个更大元素I(难点涉及哈希表与单调栈的结合)
单调栈处理的是下标! 题干: 代码: class Solution { public:vector<int> nextGreaterElement(vector<int>& nums1, vector<int>& nums2) {stack<int>ddst;unordered_map<int,int>umap;vector<int…...
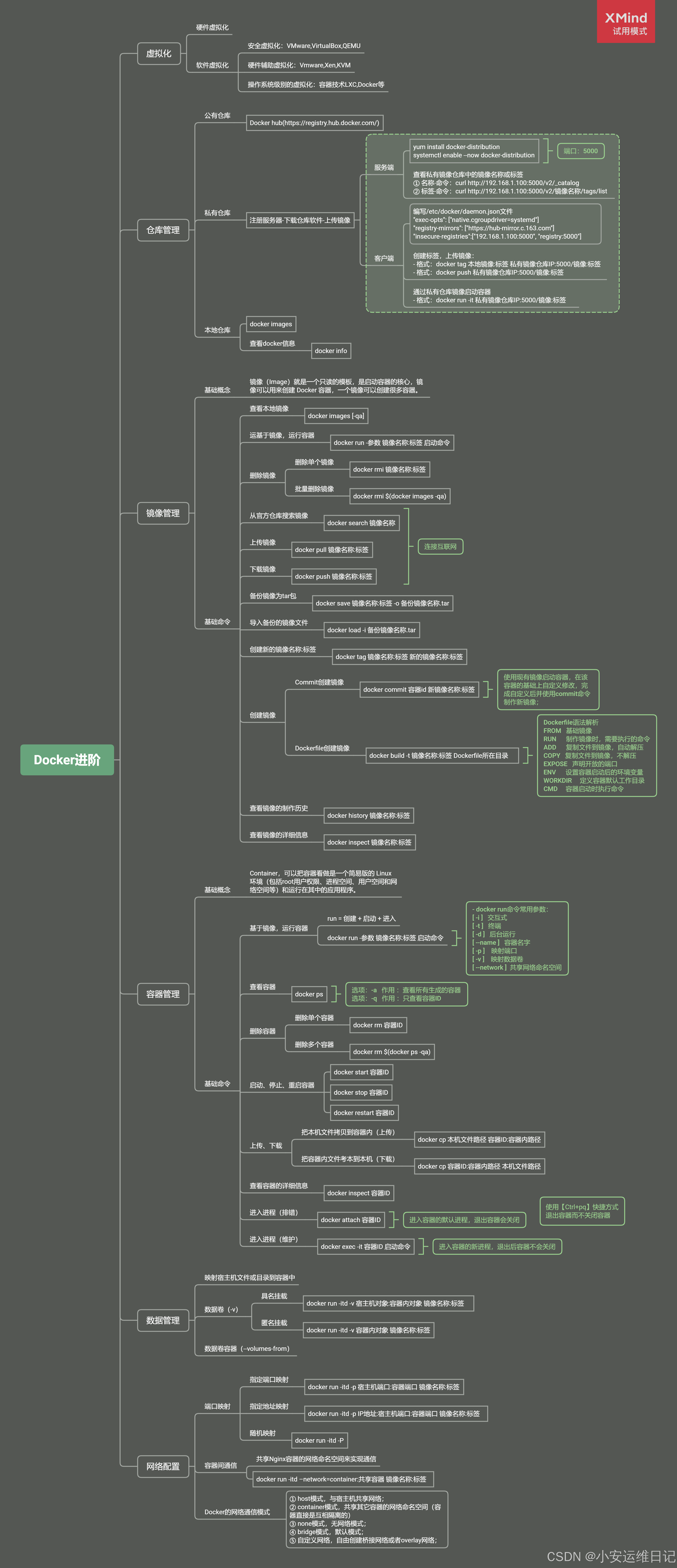
Linux云计算 |【第五阶段】CLOUD-DAY5
主要内容: 容器的镜像编排,commit简单镜像创建,Dockerfile制作服务镜像(语法、创建镜像)、创建复杂镜像(Docker微服务架构、示例:NGINXPHP)、私有仓库 一、简单镜像创建 1、自定义…...

被上传文件于后端的命名策略
上一篇博客我们了解了前端上传的文件资源应该存放在后端项目中的什么位置,那么随之而来的另一个问题——我们应该如何为上传的文件命名呢?往往直接采用原文件名并不稳妥,会导致命名冲突、文件冲突、数据库管理冲突等多种问题,下面…...

哈希表 算法专题
哈希表简介 是什么 存储数据的容器有啥用? "快速"查找某个元素什么时候用哈希表 频繁地查找某个数(有序用二分)怎么用哈希表 容器用数组模拟 字符串中的字符 范围比较小的数 一. 两数之和 两数之和 class Solution {public int[] twoSum(int[] nums, int targe…...

unity3d————[HideInInspector]
在Unity3D中,[HideInInspector]是一个属性修饰符,它的主要作用是在Unity的Inspector窗口中隐藏变量或属性。以下是关于[HideInInspector]的详细解释和作用: 作用 隐藏变量或属性:当你在脚本中使用[HideInInspector]修饰符时&…...

Soanrquber集成Gitlab 之 导入Gitlab项目
集成Gitlab 之 导入Gitlab项目 说明: Sonarquber里面的项目,顺便设置,只要在集成CI的时候,使用这个项目的项目标识即可。 当然项目名称一一对应是最好的了,所以这里讲导入Gitlab的项目,项目名称一一对应&…...

论区块链技术及应用
引言 区块链技术作为一种革命性的创新,近年来在全球范围内得到了广泛关注和应用。其去中心化、透明性和不可篡改的特性,使其在多个领域展现出了巨大的潜力。从金融到物联网,从医疗管理到政务监管,区块链正在改变我们处理信息和进…...
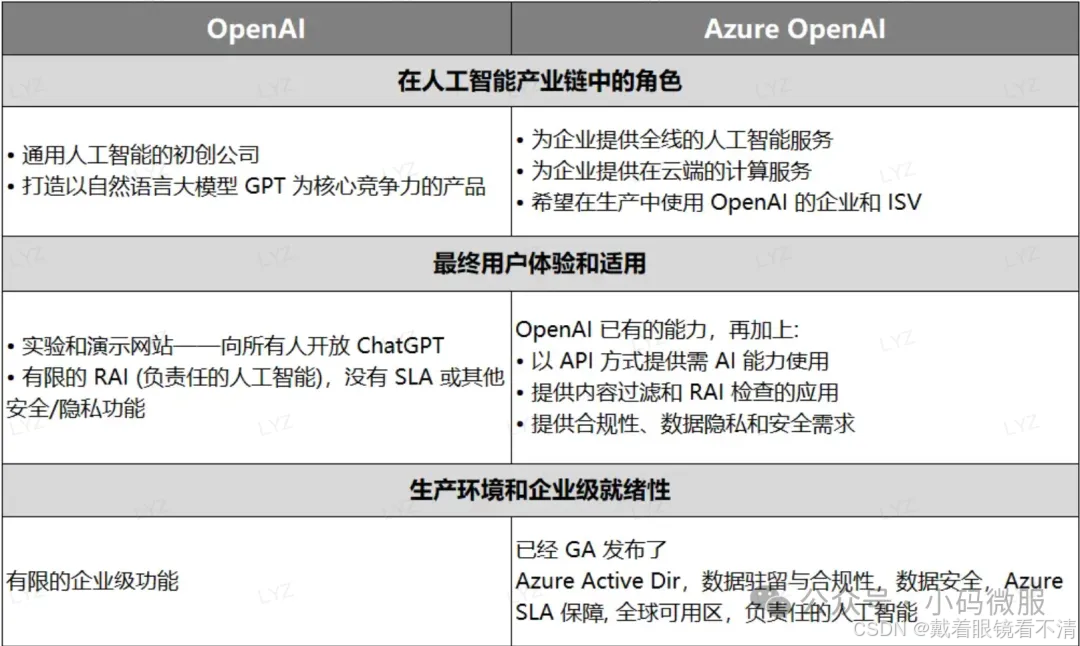
GPT避坑指南:如何辨别逆向、AZ、OpenAI官转
市面上有些说自己是官转,一刀只需要1块甚至几毛钱,并声称官方倍率的,很大可能就是使用的是 逆向或Azure。 如何鉴别逆向 逆向的种类很多,主要分为3类 逆向不知名A| 镜像站或偷的 key。成本约等于0,调用聊天数据可能在…...
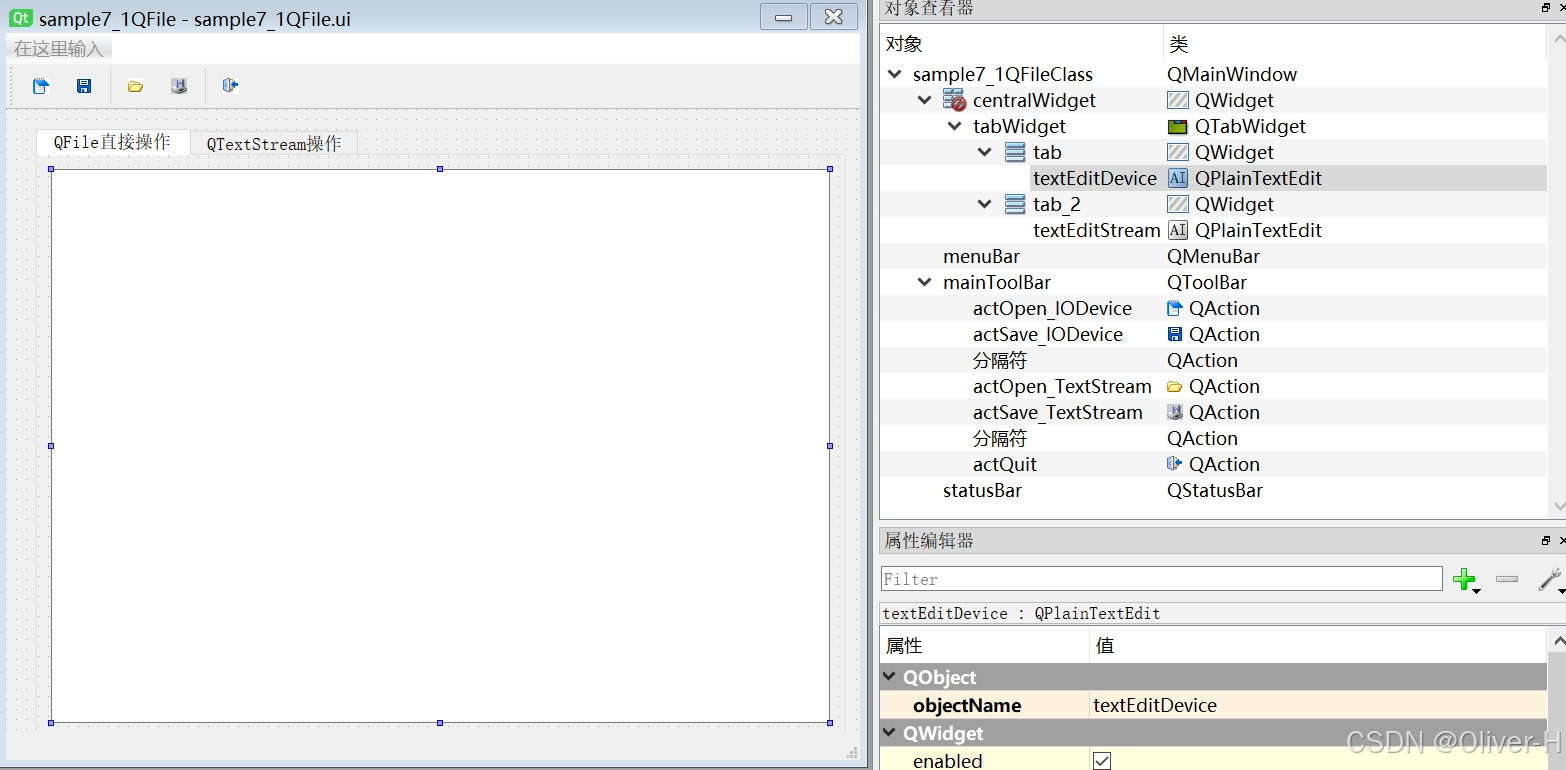
Qt 文本文件读写与保存
Qt 文本文件读写与保存 开发工具:VS2013 QT5.8 设计UI界面,如下图所示 sample7_1QFile.h 头文件: #pragma once#include <QtWidgets/QMainWindow> #include "ui_sample7_1QFile.h"class sample7_1QFile : public QMainWin…...
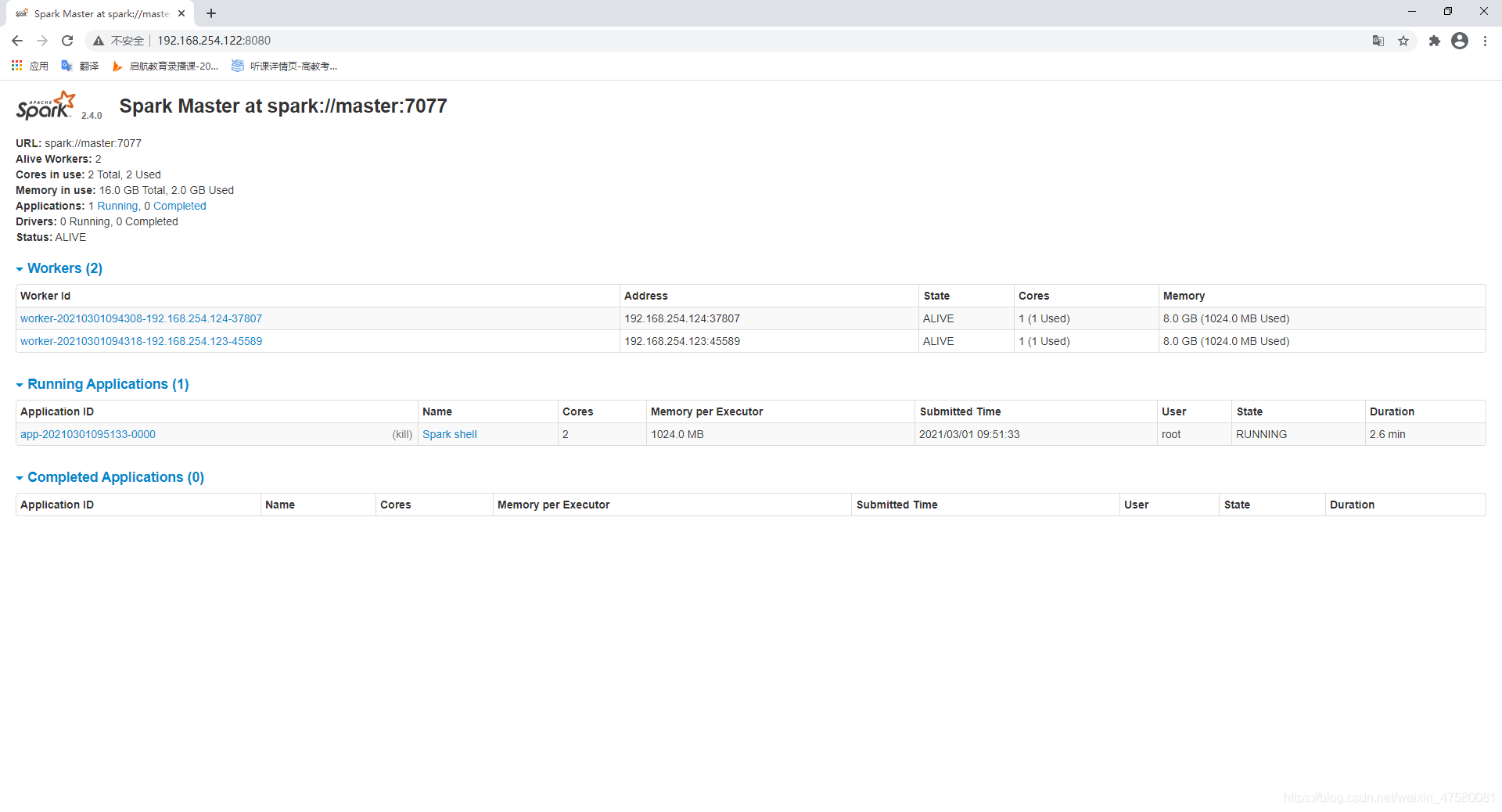
Linux基础环境搭建(CentOS7)- 安装Scala和Spark
#Linux基础环境搭建(CentOS7)- 安装Scala和Spark Linux基础环境搭建(CentOS7)- 安装Scala和Spark 大家注意以下的环境搭建版本号,如果版本不匹配有可能出现问题!(spark不要下2.4版本的 会报错…...
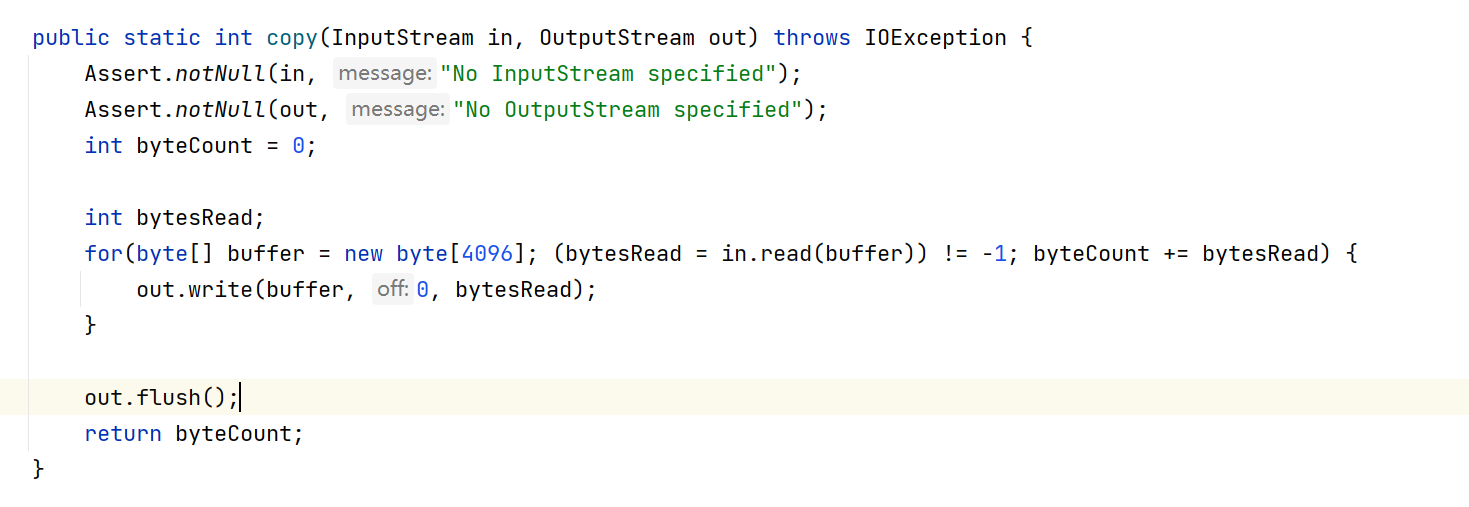
SpringBoot 下的Excel文件损坏与内容乱码问题
序言 随着打包部署的方式的改变,原本正常运行的代码可能带来一些新的问题,比如我们现在使用SpringBoot 的方式生成Jar包直接运行,就会对我们再在Resource下的Excel文件产生影响,导入与预期不符的情况发生cuiyaonan2000163.com 比…...
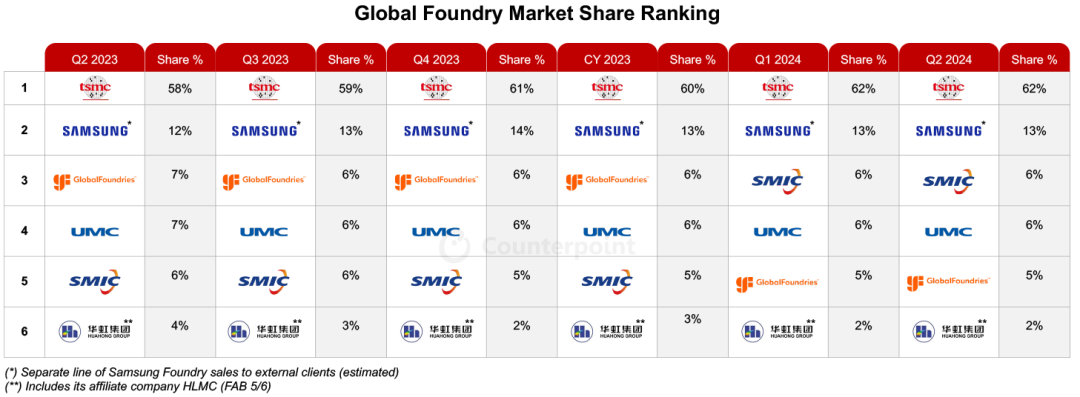
官宣下代GPU存在缺陷,50系显卡或将迎来涨价
如果说 AMD 在 Ryzen 3000 系列还是和 intel 在 CPU 方面棋差一着的话,Ryzen 5000 系列就是打了个漂亮的翻身仗了。 凭借先进的 7nm 工艺制程和全新架构,让后来 intel 急忙推出「14nm」的 11 代酷睿也难以望其项背。 直到 intel 12 代发布的时候…...
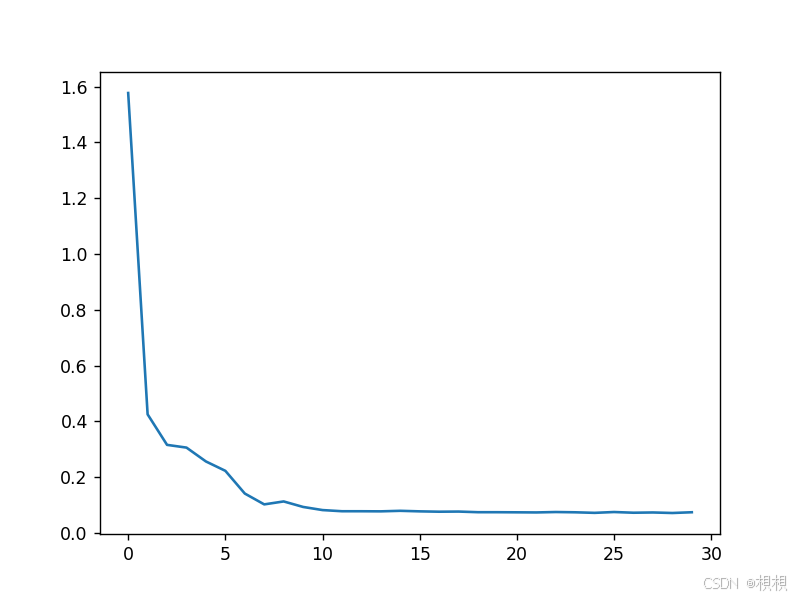
使用pytorch实现LSTM预测交通流
原始数据: 免费可下载原始参考数据 预测结果图: 根据测试数据test_data的真实值real_flow,与模型根据测试数据得到的输出结果pre_flow 完整源码: #!/usr/bin/env python # _*_ coding: utf-8 _*_import pandas as pd import nu…...
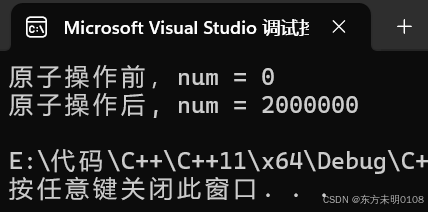
C/C++(八)C++11
目录 一、C11的简介 二、万能引用与完美转发 1、万能引用:模板中的 && 引用 2、完美转发:保持万能引用左右值属性的解决方案 三、可变参数模板 1、可变参数模板的基本使用 2、push 系列和 emplace 系列的区别 四、lambda表达式…...
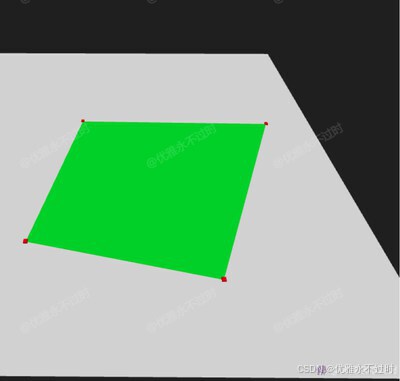
使用three.js 实现 自定义绘制平面的效果
使用three.js 实现 自定义绘制平面的效果 预览 import * as THREE from three import { OrbitControls } from three/examples/jsm/controls/OrbitControls.jsconst box document.getElementById(box)const scene new THREE.Scene()const camera new THREE.PerspectiveCam…...
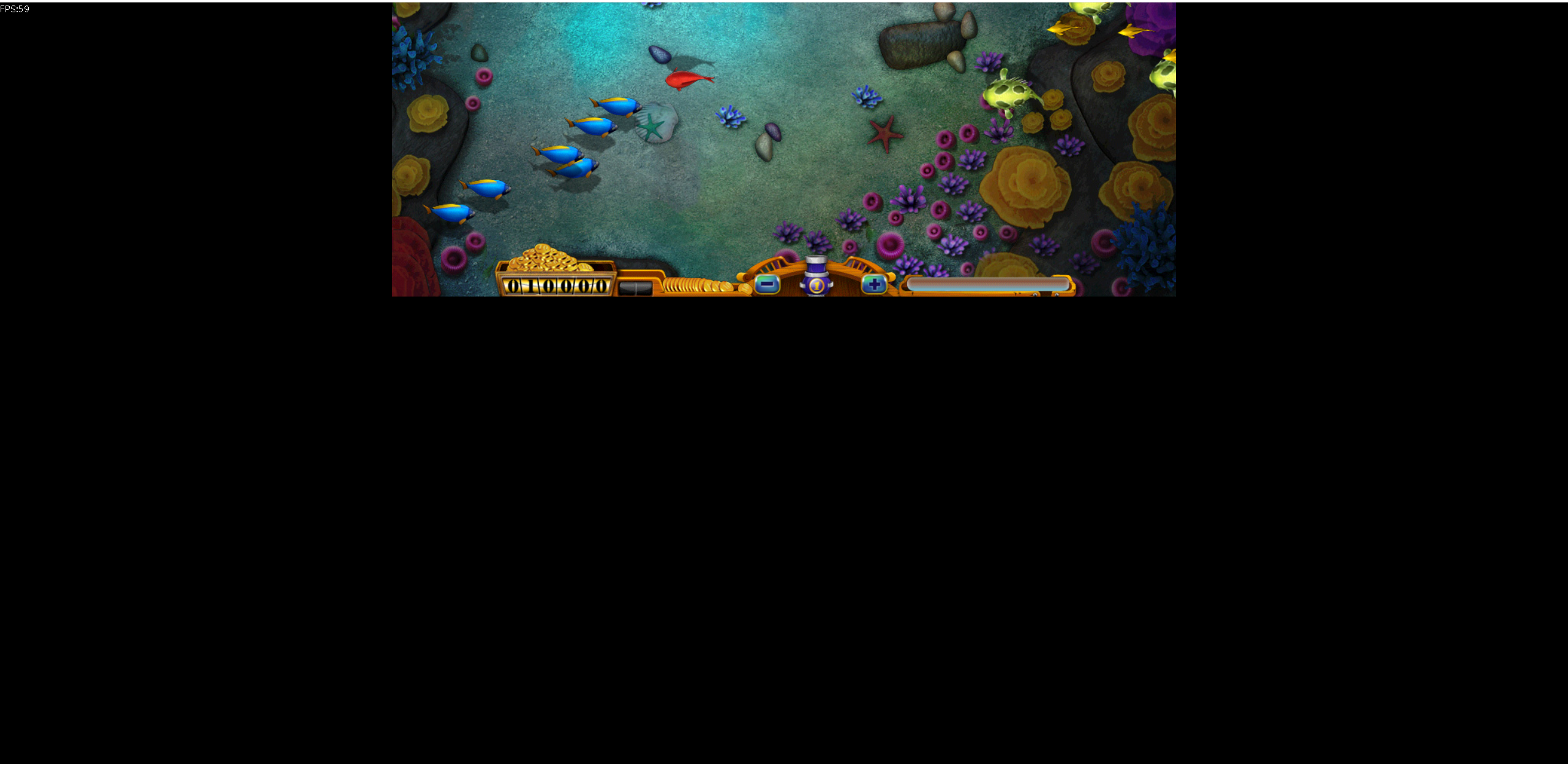
玩转Docker | 使用Docker部署捕鱼网页小游戏
玩转Docker | 使用Docker部署捕鱼网页小游戏 一、项目介绍项目简介项目预览二、系统要求环境要求环境检查Docker版本检查检查操作系统版本三、部署捕鱼网页小游戏下载镜像创建容器检查容器状态下载项目内容查看服务监听端口安全设置四、访问捕鱼网页小游戏五、总结一、项目介绍…...

网站建设制作一个网站的费用/如何写软文推广产品
字符串与字节数组的转换 String str "helloworld"; byte data[] str.getBytes(); for(int x 0 ; x < data.length ; x) { data[x]- 32 ; System.out.print(datd[x] ,); } System.out.println(new String(data)); 通过程序可以发现,字节并不适合处…...
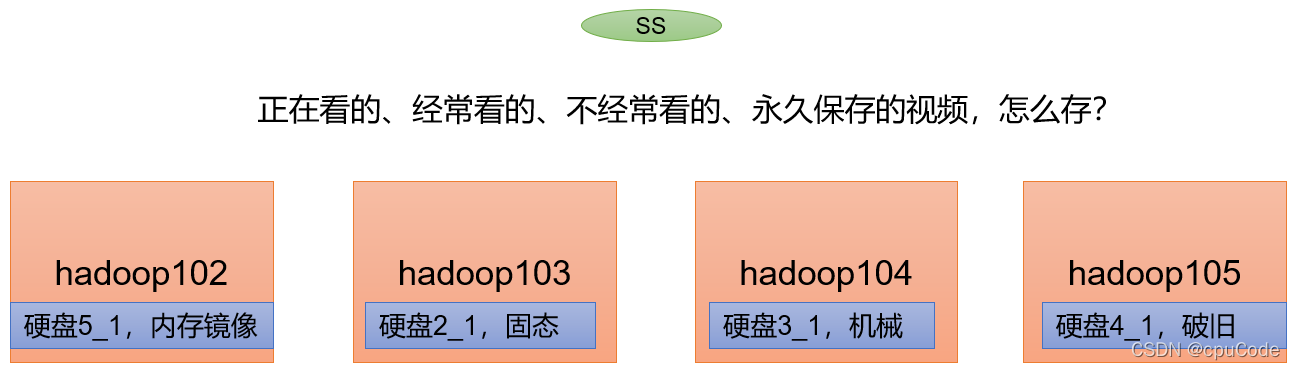
wordpress 小手枪图标/石家庄seo关键词
Hadoop 生产调优HDFS—核心参数NameNode 内存生产配置NameNode 内存计算Hadoop2.x 系列, 配置 NameNode 内存Hadoop3.x 系列,配置 NameNode 内存NameNode 心跳并发配置开启回收站配置回收站工作机制开启回收站功能参数说明查看回收站HDFS—集群压测测试 …...
焦作交友网站开发公司/知名网页设计公司
本文转自:https://blog.csdn.net/whm18322394724/article/details/80177950 版权声明:本文为博主原创文章,未经博主允许不得转载。 https://blog.csdn.net/whm18322394724/article/details/80177950正文小菜继续学习积累中,今天做…...
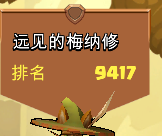
网站优化合同/南宁网站建设服务公司
在游戏中,我们都喜欢加一些描边效果,来凸显人物的边缘,提高识别度。美术一般都喜欢加。描边方式一般有两种,一种的模型边缘描边,一种的人物的转折点描边(这种需要用到卷轴) 在游戏中比较常用的…...
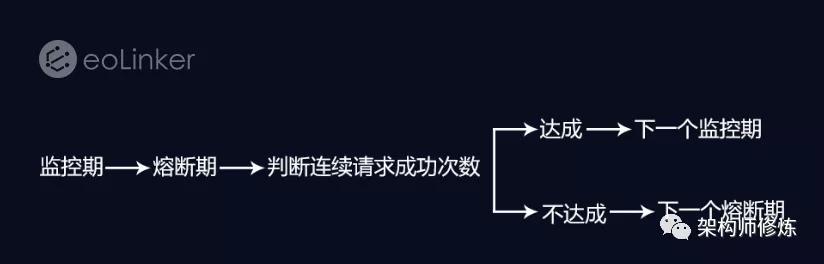
寿光市建设局网站/合肥网站维护公司
各位志同道合的朋友们大家好,我是一个一直在一线互联网踩坑十余年的编码爱好者,现在将我们的各种经验以及架构实战分享出来,如果大家喜欢,就关注我,一起将技术学深学透,我会每一篇分享结束都会预告下一专题…...

建立网站准备工作/郑州手机网站建设
看到个好文章,翻译一遍分享一下。 此为https://go101.org/article/channel-closing.html的翻译,侵删。 文章目录怎么优雅地关闭通道通道的关闭原则简单粗暴的方案礼貌的方案优雅的方案更多场景?结论怎么优雅地关闭通道 许多天前,…...