Django学习笔记-实现联机对战
笔记内容转载自 AcWing 的 Django 框架课讲义,课程链接:AcWing Django 框架课。
CONTENTS
- 1. 统一长度单位
- 2. 增加联机对战模式
- 3. 配置Django Channels
1. 统一长度单位
多人模式中每个玩家所看到的地图相对来说应该是一样的,因此需要固定地图的长宽比,一般固定为16:9。我们需要在游戏窗口的长宽中取最小值,然后将地图渲染为16:9的大小。
我们在 AcGamePlayground
类中实现一个 resize
函数用于将长宽比调整为16:9并且达到最大:
class AcGamePlayground {constructor(root) {this.root = root;this.$playground = $(`<div class='ac_game_playground'></div>`);this.root.$ac_game.append(this.$playground);this.start();}get_random_color() {...}start() {this.hide(); // 初始化时需要先关闭playground界面let outer = this;$(window).resize(function() {outer.resize();}); // 用户改变窗口大小时改函数会触发}// 将长宽比调整为16:9resize() {this.width = this.$playground.width();this.height = this.$playground.height();let unit = Math.min(this.width / 16, this.height / 9);this.width = unit * 16;this.height = unit * 9;this.scale = this.height; // 当窗口大小改变时所有目标的相对大小和位置也要改变if (this.game_map) this.game_map.resize(); // 如果地图存在需要调用地图的resize函数}// 显示playground界面show() {this.$playground.show();// 将界面的宽高先存下来this.width = this.$playground.width();this.height = this.$playground.height();this.game_map = new GameMap(this); // 创建游戏画面this.resize(); // 界面打开后需要resize一次,需要将game_map也resize...}// 关闭playground界面hide() {this.$playground.hide();}
}
现在需要将窗口大小的修改效果作用到黑色背景上,因此我们在 GameMap
类中也实现一个 resize
函数用于修改背景大小:
class GameMap extends AcGameObject {constructor(playground) { // 需要将AcGamePlayground传进来super(); // 调用基类构造函数,相当于将自己添加到了AC_GAME_OBJECTS中this.playground = playground;this.$canvas = $(`<canvas></canvas>`); // 画布,用来渲染画面this.ctx = this.$canvas[0].getContext('2d'); // 二维画布this.ctx.canvas.width = this.playground.width; // 设置画布宽度this.ctx.canvas.height = this.playground.height; // 设置画布高度this.playground.$playground.append(this.$canvas); // 将画布添加到HTML中}start() {}resize() {this.ctx.canvas.width = this.playground.width;this.ctx.canvas.height = this.playground.height;this.ctx.fillStyle = 'rgba(0, 0, 0, 1)'; // 每次调整大小后直接涂一层不透明的背景this.ctx.fillRect(0, 0, this.ctx.canvas.width, this.ctx.canvas.height);}update() {this.render(); // 每一帧都要画一次}render() {this.ctx.fillStyle = 'rgba(0, 0, 0, 0.2)'; // 黑色背景// 左上角坐标(0, 0),右下角坐标(w, h)this.ctx.fillRect(0, 0, this.ctx.canvas.width, this.ctx.canvas.height);}
}
我们修改一下 game.css
文件,添加以下内容,实现将地图居中:
.ac_game_playground > canvas {position: relative;top: 50%;left: 50%;transform: translate(-50%, -50%);
}
现在我们还需要修改地图里面的目标,一共有三种分别是玩家、火球、被击中的粒子效果。
首先修改一下 AcGamePlayground
类中的玩家初始化代码:
class AcGamePlayground {constructor(root) {...}get_random_color() {...}start() {...}// 将长宽比调整为16:9resize() {...}// 显示playground界面show() {this.$playground.show();// 将界面的宽高先存下来this.width = this.$playground.width();this.height = this.$playground.height();this.game_map = new GameMap(this); // 创建游戏画面this.resize(); // 界面打开后需要resize一次,需要将game_map也resizethis.players = []; // 所有玩家this.players.push(new Player(this, this.width / 2 / this.scale, 0.5, 0.05, 'white', 0.15, true)); // 创建自己// 创建敌人for (let i = 0; i < 8; i++) {this.players.push(new Player(this, this.width / 2 / this.scale, 0.5, 0.05, this.get_random_color(), 0.15, false));}}// 关闭playground界面hide() {this.$playground.hide();}
}
然后我们修改 Player
类,将所有绝对变量替换为相对变量:
class Player extends AcGameObject {constructor(playground, x, y, radius, color, speed, is_me) {...this.eps = 0.01; // 误差小于0.01认为是0...}start() {if (this.is_me) {this.add_listening_events();} else {// Math.random()返回一个0~1之间的数,随机初始化AI的位置let tx = Math.random() * this.playground.width / this.playground.scale;let ty = Math.random() * this.playground.height / this.playground.scale;this.move_to(tx, ty);}}add_listening_events() {let outer = this;this.playground.game_map.$canvas.on('contextmenu', function() {return false;}); // 取消右键的菜单功能this.playground.game_map.$canvas.mousedown(function(e) {const rect = outer.ctx.canvas.getBoundingClientRect();if (e.which === 3) { // 1表示左键,2表示滚轮,3表示右键outer.move_to((e.clientX - rect.left) / outer.playground.scale, (e.clientY - rect.top) / outer.playground.scale); // e.clientX/Y为鼠标点 击坐标} else if (e.which === 1) {if (outer.cur_skill === 'fireball') {outer.shoot_fireball((e.clientX - rect.left) / outer.playground.scale, (e.clientY - rect.top) / outer.playground.scale);}outer.cur_skill = null; // 释放完一次技能后还原}});$(window).keydown(function(e) {if (e.which === 81) { // Q键outer.cur_skill = 'fireball';return false;}});}// 计算两点之间的欧几里得距离get_dist(x1, y1, x2, y2) {...}// 向(tx, ty)位置发射火球shoot_fireball(tx, ty) {...}move_to(tx, ty) {...}is_attacked(theta, damage) { // 被攻击到...}// 更新移动update_move() {this.spent_time += this.timedelta / 1000;// AI敌人随机向玩家射击,游戏刚开始前三秒AI不能射击if (this.spent_time > 3 && !this.is_me && Math.random() < 1 / 360.0) {let player = this.playground.players[0];this.shoot_fireball(player.x, player.y);}if (this.damage_speed > this.eps) { // 有击退效果时玩家无法移动this.vx = this.vy = 0;this.move_length = 0;this.x += this.damage_vx * this.damage_speed * this.timedelta / 1000;this.y += this.damage_vy * this.damage_speed * this.timedelta / 1000;this.damage_speed *= this.friction;} else {if (this.move_length < this.eps) {this.move_length = 0;this.vx = this.vy = 0;if (!this.is_me) { // AI敌人不能停下来let tx = Math.random() * this.playground.width / this.playground.scale;let ty = Math.random() * this.playground.height / this.playground.scale;this.move_to(tx, ty);}} else {// 计算真实移动距离,与一帧的移动距离取min防止移出界let true_move = Math.min(this.move_length, this.speed * this.timedelta / 1000);this.x += this.vx * true_move;this.y += this.vy * true_move;this.move_length -= true_move;}}}update() {this.update_move();this.render();}render() {let scale = this.playground.scale; // 要将相对值恢复成绝对值if (this.is_me) {this.ctx.save();this.ctx.beginPath();this.ctx.arc(this.x * scale, this.y * scale, this.radius * scale, 0, Math.PI * 2, false);this.ctx.stroke();this.ctx.clip();this.ctx.drawImage(this.img, (this.x - this.radius) * scale, (this.y - this.radius) * scale, this.radius * 2 * scale, this.radius * 2 * scale);this.ctx.restore();} else { // AIthis.ctx.beginPath();// 角度从0画到2PI,是否逆时针为falsethis.ctx.arc(this.x * scale, this.y * scale, this.radius * scale, 0, Math.PI * 2, false);this.ctx.fillStyle = this.color;this.ctx.fill();}}
}
然后修改 FireBall
类,只需要修改 eps
以及 render
函数即可:
class FireBall extends AcGameObject {// 火球需要标记是哪个玩家发射的,且射出后的速度方向与大小是固定的,射程为move_lengthconstructor(playground, player, x, y, radius, vx, vy, color, speed, move_length, damage) {...this.eps = 0.01;}start() {}update() {...}get_dist(x1, y1, x2, y2) {...}is_collision(player) {...}attack(player) {...}render() {let scale = this.playground.scale;this.ctx.beginPath();this.ctx.arc(this.x * scale, this.y * scale, this.radius * scale, 0, Math.PI * 2, false);this.ctx.fillStyle = this.color;this.ctx.fill();}
}
最后修改 Particle
类,同样也是只需要修改 eps
以及 render
函数即可:
class Particle extends AcGameObject {constructor(playground, x, y, radius, vx, vy, color, speed, move_length) {...this.eps = 0.01;this.friction = 0.9;}start() {}update() {...}render() {let scale = this.playground.scale;this.ctx.beginPath();this.ctx.arc(this.x * scale, this.y * scale, this.radius * scale, 0, Math.PI * 2, false);this.ctx.fillStyle = this.color;this.ctx.fill();}
}
2. 增加联机对战模式
我们先修改 AcGameMenu
类,实现多人模式按钮的逻辑:
class AcGameMenu {constructor(root) { // root用来传AcGame对象...}start() {this.hide();this.add_listening_events();}// 给按钮绑定监听函数add_listening_events() {let outer = this;// 注意在function中调用this指的是function本身,因此需要先将外面的this存起来this.$single.click(function() {outer.hide(); // 关闭menu界面outer.root.playground.show('single mode'); // 显示playground界面,加入参数用于区分});this.$multi.click(function() {outer.hide();outer.root.playground.show('multi mode'); // 多人模式});this.$settings.click(function() {outer.root.settings.logout_on_remote();});}// 显示menu界面show() {this.$menu.show();}// 关闭menu界面hide() {this.$menu.hide();}
}
然后修改 AcGamePlayground
类,区分两种模式,且需要进一步区分玩家类别,之前使用 True/False 表示是否是玩家本人,现在可以用字符串区分玩家本人、其他玩家以及人机:
class AcGamePlayground {constructor(root) {...}get_random_color() {...}start() {...}// 将长宽比调整为16:9resize() {...}// 显示playground界面show(mode) {this.$playground.show();// 将界面的宽高先存下来this.width = this.$playground.width();this.height = this.$playground.height();this.game_map = new GameMap(this); // 创建游戏画面this.resize(); // 界面打开后需要resize一次,需要将game_map也resizethis.players = []; // 所有玩家this.players.push(new Player(this, this.width / 2 / this.scale, 0.5, 0.05, 'white', 0.15, 'me', this.root.settings.username, this.root.settings.avatar)); // 创建自己,自己的用户名和头像从settings中获得// 单人模式下创建AI敌人if (mode === 'single mode'){for (let i = 0; i < 8; i++) {this.players.push(new Player(this, this.width / 2 / this.scale, 0.5, 0.05, this.get_random_color(), 0.15, 'robot'));}} else if (mode === 'multi mode') {}}// 关闭playground界面hide() {this.$playground.hide();}
}
然后还需要修改一下 Player
类,将原本的 this.is_me
判断进行修改:
class Player extends AcGameObject {constructor(playground, x, y, radius, color, speed, character, username, avatar) {...this.character = character;this.username = username;this.avatar = avatar;...if (this.character !== 'robot') { // 只有AI不用渲染图片this.img = new Image();this.img.src = this.avatar;}}start() {if (this.character === 'me') { // 只给自己添加监听函数this.add_listening_events();} else {...}}add_listening_events() {...}// 计算两点之间的欧几里得距离get_dist(x1, y1, x2, y2) {...}// 向(tx, ty)位置发射火球shoot_fireball(tx, ty) {...}move_to(tx, ty) {...}is_attacked(theta, damage) { // 被攻击到...}// 更新移动update_move() {this.spent_time += this.timedelta / 1000;// AI敌人随机向玩家射击,游戏刚开始前三秒AI不能射击if (this.character === 'robot' && this.spent_time > 3 && Math.random() < 1 / 360.0) {...}if (this.damage_speed > this.eps) { // 有击退效果时玩家无法移动...} else {if (this.move_length < this.eps) {...if (this.character === 'robot') { // AI敌人不能停下来...}} else {// 计算真实移动距离,与一帧的移动距离取min防止移出界...}}}update() {this.update_move();this.render();}render() {let scale = this.playground.scale; // 要将相对值恢复成绝对值if (this.character !== 'robot') {...} else { // AI...}}
}
3. 配置Django Channels
假设有三名玩家编号为1、2、3进行多人游戏,那么每个玩家都有自己的一个窗口,且窗口中都能看到三名玩家。如果当前玩家1、2在进行游戏,3加入了游戏,那么需要告诉1、2两名玩家3来了,且还要告诉3当前已经有玩家1、2了。
要实现这一点,可以通过一个中心服务器(可以就是自己租的云服务器),即3向服务器发送他来了,服务器给1、2发送消息,且服务器给3发送消息说之前已经有1、2两名玩家了。因此服务器中需要存储每个地图中的玩家信息,用于完成第一个同步事件:生成玩家事件。
我们之后一共需要实现四个同步函数:create_player
、move_to
、shoot_fireball
、attack
。前三个函数顾名思义,最后的 attack
函数是因为服务器存在延迟,比如3发射一个火球在本地看打中了1,但是由于延迟在1那边可能是没被打中的。
攻击判断是一个权衡问题,一般的游戏都是选择在本地进行攻击判断,而不是云服务器,即以发起攻击的玩家窗口进行判断,如果击中了则通过 attack
函数在服务器上广播信息。
在此之前我们使用的是 HTTP 协议,该协议为单向的,即客户端需要先向服务器请求信息后服务器才会返回信息,而服务器是不会主动向客户端发送信息的。
因此此处我们需要使用 WebSocket 协议(WS),同理该协议也有对应的加密协议 WSS,Django Channels 即为 Django 支持 WSS 协议的一种实现方式。
相关文章:

Django学习笔记-实现联机对战
笔记内容转载自 AcWing 的 Django 框架课讲义,课程链接:AcWing Django 框架课。 CONTENTS 1. 统一长度单位2. 增加联机对战模式3. 配置Django Channels 1. 统一长度单位 多人模式中每个玩家所看到的地图相对来说应该是一样的,因此需要固定地…...
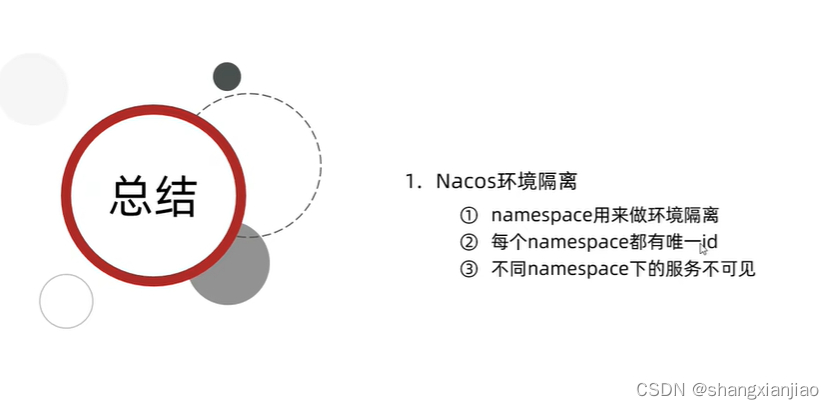
nacos总结1
5.Nacos注册中心 国内公司一般都推崇阿里巴巴的技术,比如注册中心,SpringCloudAlibaba也推出了一个名为Nacos的注册中心。 5.1.认识和安装Nacos Nacos是阿里巴巴的产品,现在是SpringCloud中的一个组件。相比Eureka功能更加丰富,…...
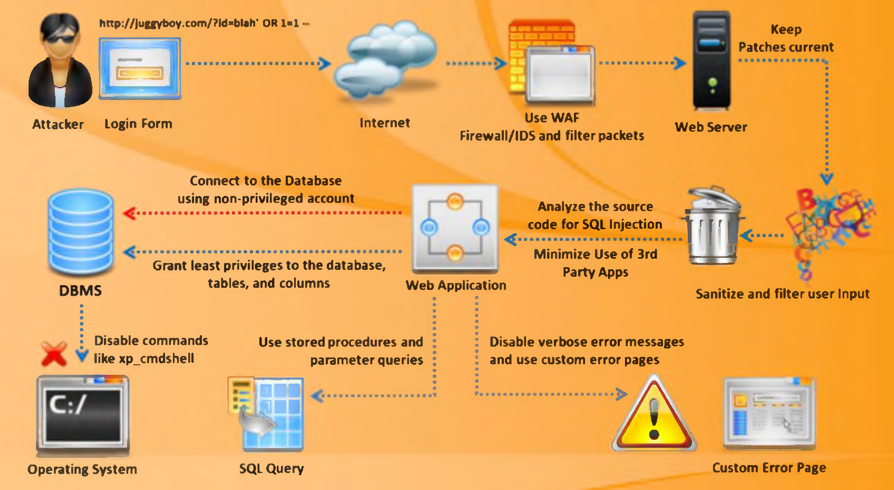
Web安全测试(三):SQL注入漏洞
一、前言 结合内部资料,与安全渗透部门同事合力整理的安全测试相关资料教程,全方位涵盖电商、支付、金融、网络、数据库等领域的安全测试,覆盖Web、APP、中间件、内外网、Linux、Windows多个平台。学完后一定能成为安全大佬! 全部…...

Webstorm 入门级玩转uni-app 项目-微信小程序+移动端项目方案
1. Webstorm uni-app语法插件 : Uniapp Support Uniapp Support - IntelliJ IDEs Plugin | Marketplace 第一个是不收费,第二个收费 我选择了第二个Uniapp Support ,有试用30天,安装重启webstorm之后,可以提高生产率…...

从零开始的Hadoop学习(三)| 集群分发脚本xsync
1. Hadoop目录结构 bin目录:存放对Hadoop相关服务(hdfs,yarn,mapred)进行操作的脚本etc目录:Hadoop的配置文件目录,存放Hadoop的配置文件lib目录:存放Hadoop的本地库(对…...

golang http transport源码分析
golang http transport源码分析 前言 Golang http库在日常开发中使用会很多。这里通过一个demo例子出发,从源码角度梳理golang http库底层的数据结构以及大致的调用流程 例子 package mainimport ("fmt""net/http""net/url""…...
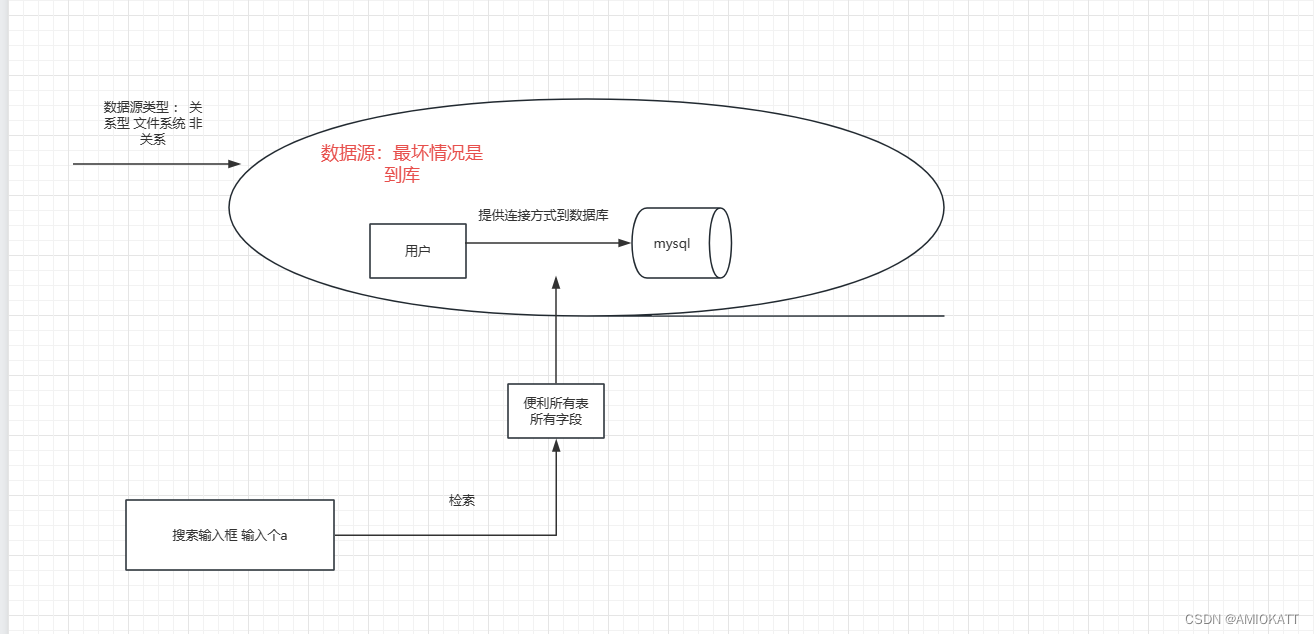
spring boot 项目整合 websocket
1.业务背景 负责的项目有一个搜索功能,搜索的范围几乎是全表扫,且数据源类型贼多。目前对搜索的数据量量级未知,但肯定不会太少,不仅需要搜索还得点击下载文件。 关于搜索这块类型 众多,未了避免有个别极大数据源影响整…...

统计学补充概念-17-线性决策边界
概念 线性决策边界是一个用于分类问题的线性超平面,可以将不同类别的样本分开。在二维空间中,线性决策边界是一条直线,将两个不同类别的样本分隔开来。对于更高维的数据,决策边界可能是一个超平面。 线性决策边界的一般形式可以表…...

指针变量、指针常量与常量指针的区别
指针变量、指针常量与常量指针 一、指针变量 定义:指针变量是指存放地址的变量,其值是地址。 一般格式:基类型 指针变量名;(int p) 关键点: 1、int * 表示一种指针类型(此处指int 类型),p(变量…...
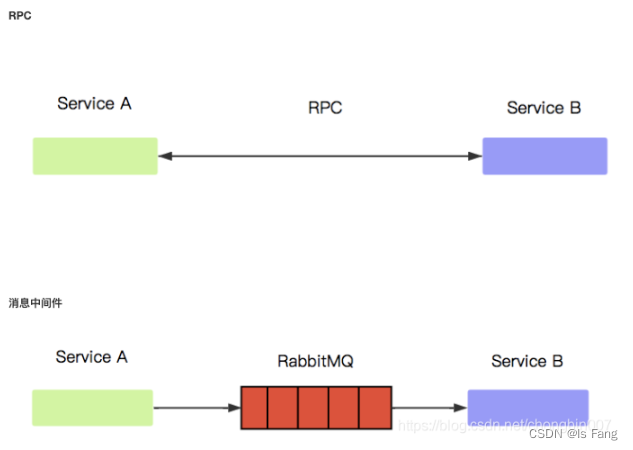
mq与mqtt的关系
文章目录 mqtt 与 mq的区别mqtt 与 mq的详细区别传统消息队列RocketMQ和微消息队列MQTT对比:MQ与RPC的区别 mqtt 与 mq的区别 mqtt:一种通信协议,规范 MQ:一种通信通道(方式),也叫消息队列 MQ…...

代码大全阅读随笔 (二)
软件设计 设计就是把需求分析和编码调试连在一起的活动。 设计不是在谁的头脑中直接跳出来了,他是不断的设计评估,非正式讨论,写实验代码以及修改实验代码中演化和完善。 作为软件开发人员,我们不应该试着在同一时间把整个程序都塞…...

vue 项目的屏幕自适应方案
方案一:使用 scale-box 组件 属性: width 宽度 默认 1920height 高度 默认 1080bgc 背景颜色 默认 "transparent"delay自适应缩放防抖延迟时间(ms) 默认 100 vue2版本:vue2大屏适配缩放组件(vu…...

23软件测试高频率面试题汇总
一、 你们的测试流程是怎么样的? 答:1.项目开始阶段,BA(需求分析师)从用户方收集需求并将需求转化为规格说明书,接 下来在项目组领导会组织需求评审。 2.需求评审通过后,BA 会组织项目经理…...
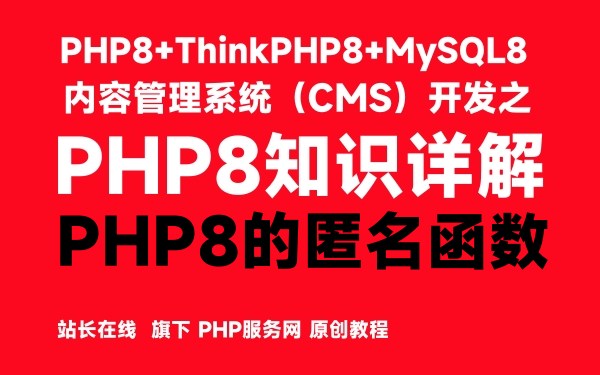
PHP8的匿名函数-PHP8知识详解
php 8引入了匿名函数(Anonymous Functions),它是一种创建短生命周期的函数,不需要命名,并且可以在其作用域内直接使用。以下是在PHP 8中使用匿名函数的知识要点: 1、创建匿名函数,语法格式如下&…...
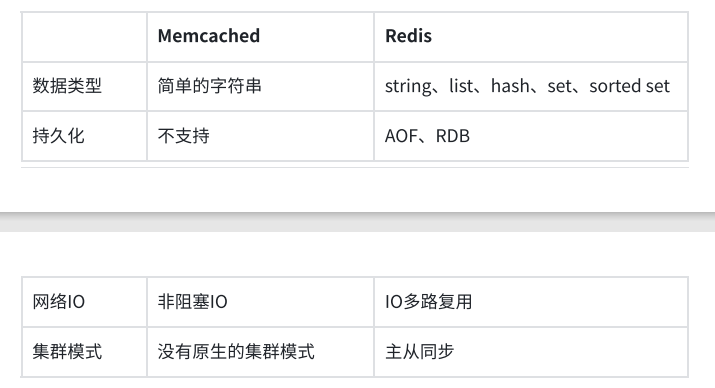
Redis—Redis介绍(是什么/为什么快/为什么做MySQL缓存等)
一、Redis是什么 Redis 是一种基于内存的数据库,对数据的读写操作都是在内存中完成,因此读写速度非常快,常用于缓存,消息队列、分布式锁等场景。 Redis 提供了多种数据类型来支持不同的业务场景,比如 String(字符串)、…...
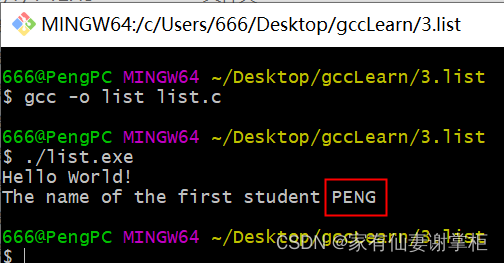
C语言链表梳理-2
链表头使用结构体:struct Class 链表中的每一项使用结构体:struct Student#include <stdio.h>struct Student {char * StudentName;int StudentAge;int StudentSex;struct Student * NextStudent; };struct Class {char *ClassName;struct Stude…...
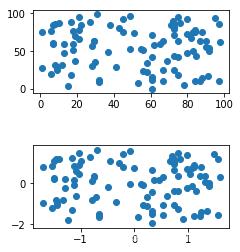
【深度学习】实验03 特征处理
文章目录 特征处理标准化归一化正则化 特征处理 标准化 # 导入标准化库 from sklearn.preprocessing import StandardScalerfrom matplotlib import gridspec import numpy as np import matplotlib.pyplot as plt import warnings warnings.filterwarnings("ignore&quo…...

基于Dpabi的功能连接
1.预处理 这里预处理用Gretna软件进行,共分为以下几步: (1)DICOM转NIfTI格式 (2)去除前10个时间点(Remove first 10 times points):由于机器刚启动、被试刚躺进去也还需适应环境,导致刚开始扫描的数据很…...

在React项目是如何捕获错误的?
文章目录 react中的错误介绍解决方案后言 react中的错误介绍 错误在我们日常编写代码是非常常见的 举个例子,在react项目中去编写组件内JavaScript代码错误会导致 React 的内部状态被破坏,导致整个应用崩溃,这是不应该出现的现象 作为一个框架…...
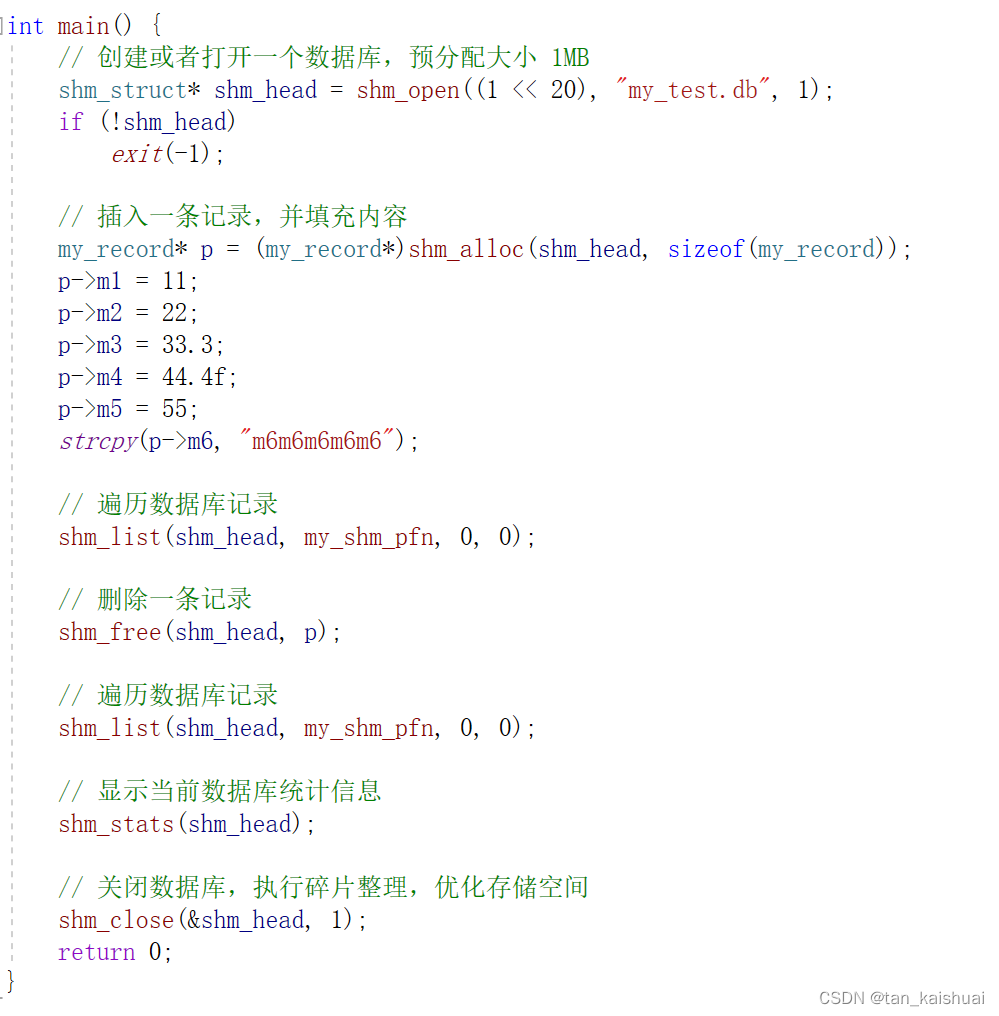
基于内存池的 简单高效的数据库 SDK简介
基于内存池的 简单高效的数据库 SDK简介 下载地址: https://gitee.com/tankaishuai/powerful_sdks/tree/master/shm_alloc_db_heap shm_alloc_db_heap 是一个基于内存池实现的简单高效的文件型数据存储引擎,利用它可以轻松地像访问内存块一样读、写、增…...
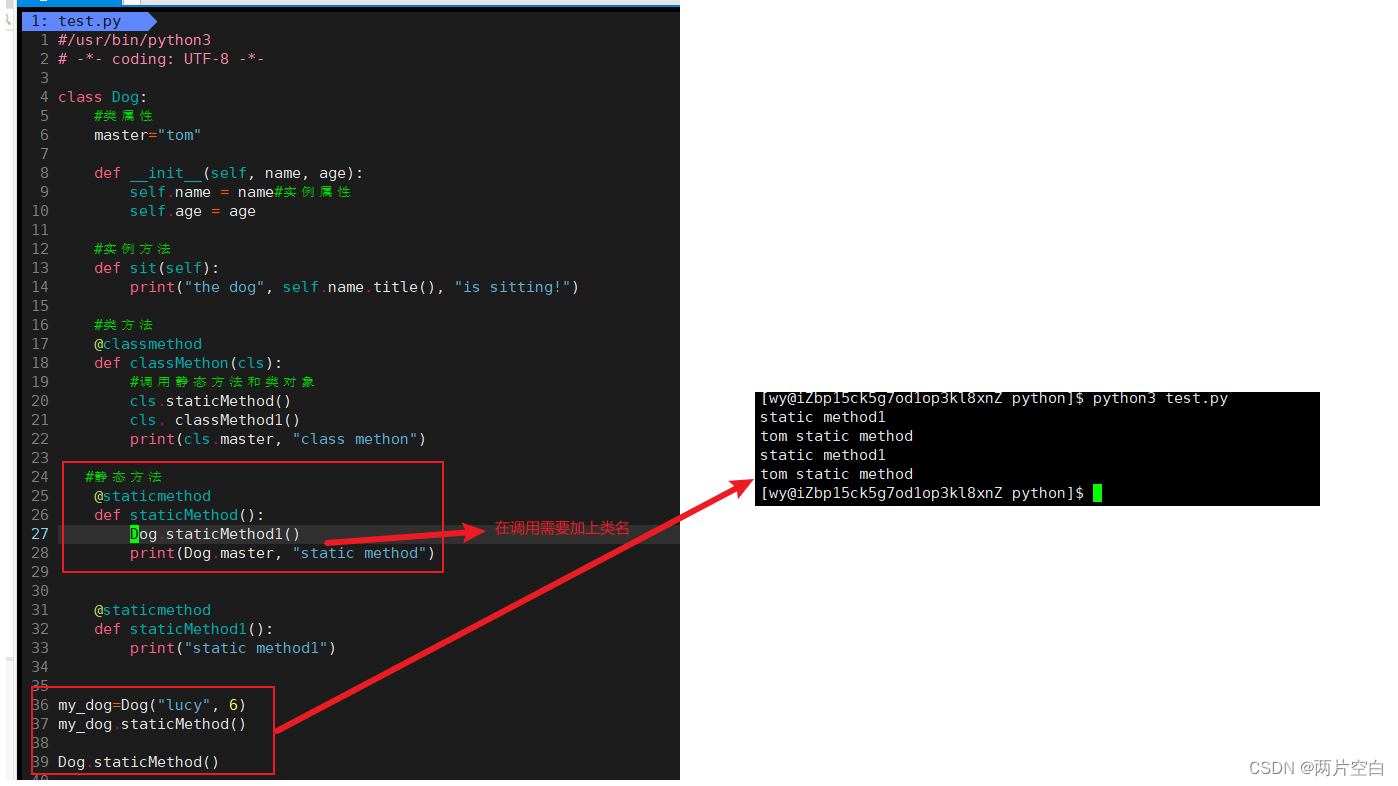
python实例方法,类方法和静态方法区别
为python中的装饰器 实例方法 实例方法时直接定义在类中的函数,不需要任何修饰。只能通过类的实例化对象来调用。不能通过类名来调用。 类方法 类方法,是类中使用classmethod修饰的函数。类方法在定义的时候需要有表示类对象的参数(一般命名为cls&#…...

Pyecharts教程(四):使用pyecharts绘制3D折线图
Pyecharts教程(四):使用pyecharts绘制3D折线图 作者:安静到无声 个人主页 目录 Pyecharts教程(四):使用pyecharts绘制3D折线图准备工作数据准备绘制3D折线图推荐专栏在这篇文章中,我们将学习如何使用pyecharts库来绘制一个3D折线图。pyecharts是一个用于生成Echarts图表的…...
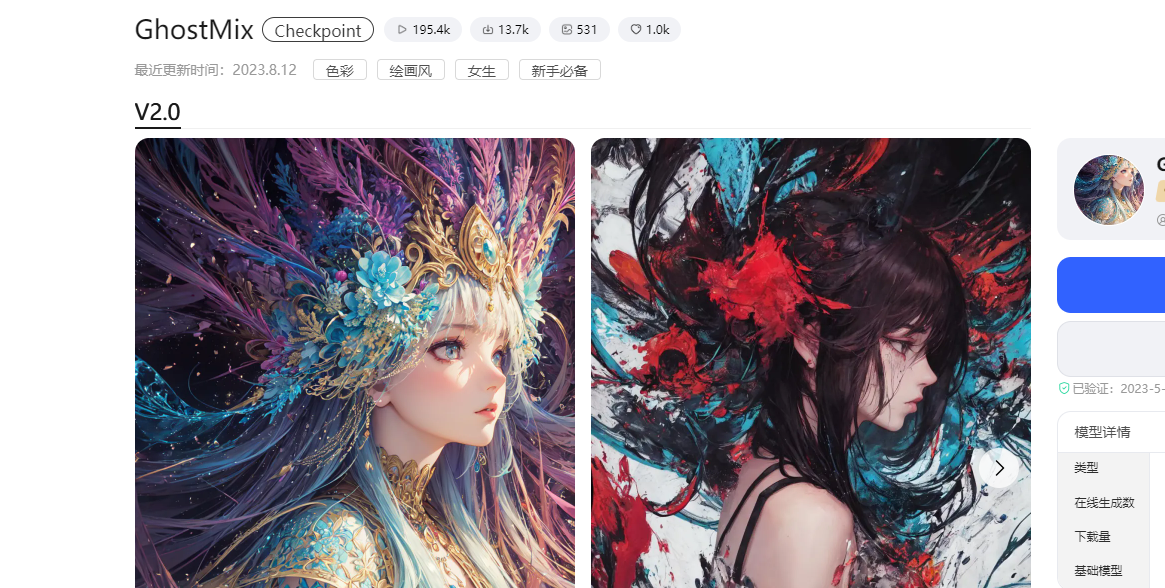
【stable-diffusion使用扩展+插件和模型资源(下)】
插件模型魔法图片等资源:https://tianfeng.space/1240.html 书接上文:(上) 插件推荐 1.lobe theme lobe theme是一款主题插件,直接可以在扩展安装 界面进行了重新布局,做了一些优化,有兴趣的…...

一文了解SpringBoot中的Aop
目录 1.什么是Aop 2.相关概念 3.相关注解 4.为什么要用Aop 5.Aop使用案例 1.什么是Aop AOP:Aspect Oriented Programming,面向切面,是Spring三大思想之一,另外两个是 IOC-控制反转 DI-依赖注入 (Autowired、Qualifier、Re…...
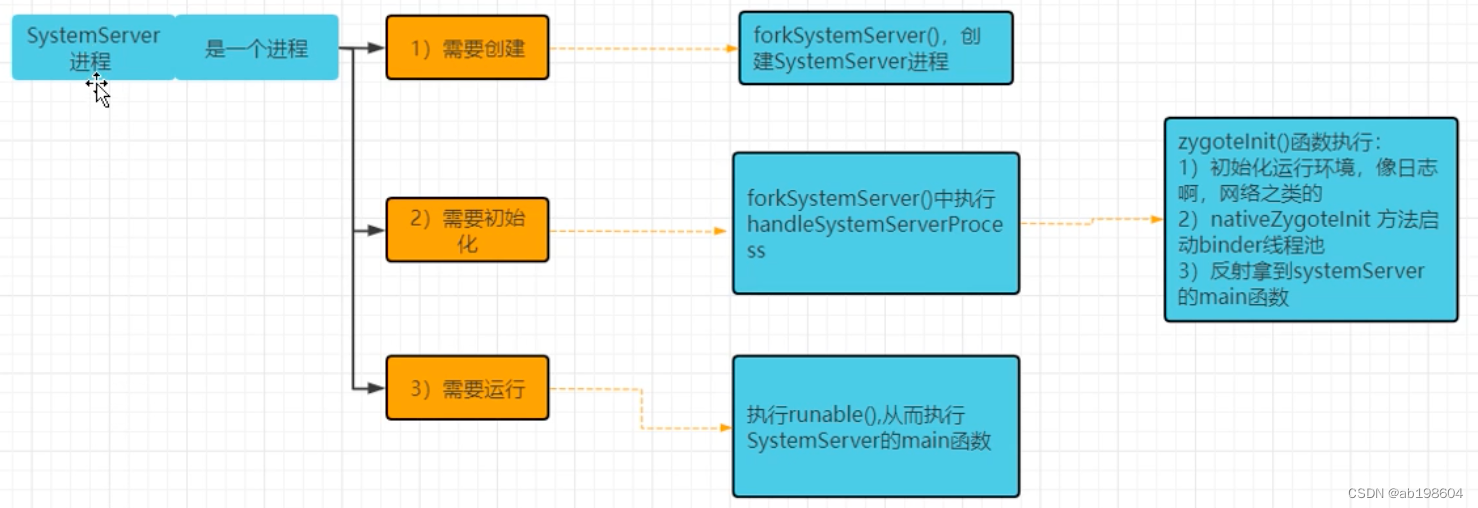
android系统启动流程之zygote如何创建SystemServer进程
SystemServer:是独立的进程,主要工作是管理服务的,它将启动大约90种服务Services. 它主要承担的职责是为APP的运行提供各种服务,像AMS,WMS这些服务并不是一个独立的进程, 它们其实都是SystemServer进程中需要管理的的众多服务之一…...
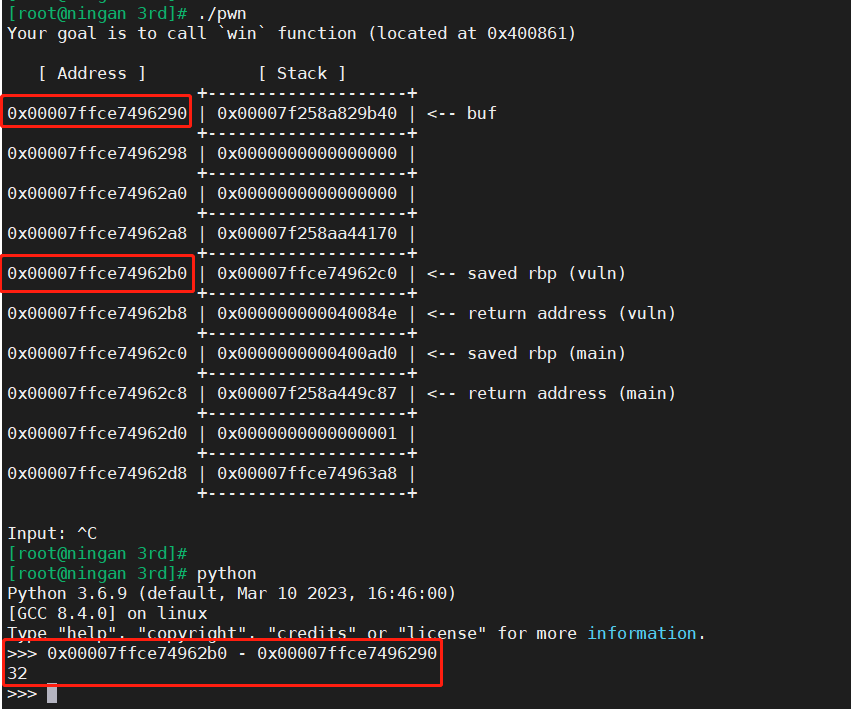
【awd系列】Bugku S3 AWD排位赛-9 pwn类型
文章目录 二进制下载检查分析运行二进制ida分析解题思路exp 二进制下载 下载地址:传送门 检查分析 [rootningan 3rd]# file pwn pwn: ELF 64-bit LSB executable, x86-64, version 1 (SYSV), dynamically linked, interpreter /lib64/ld-linux-x86-64.so.2, for …...
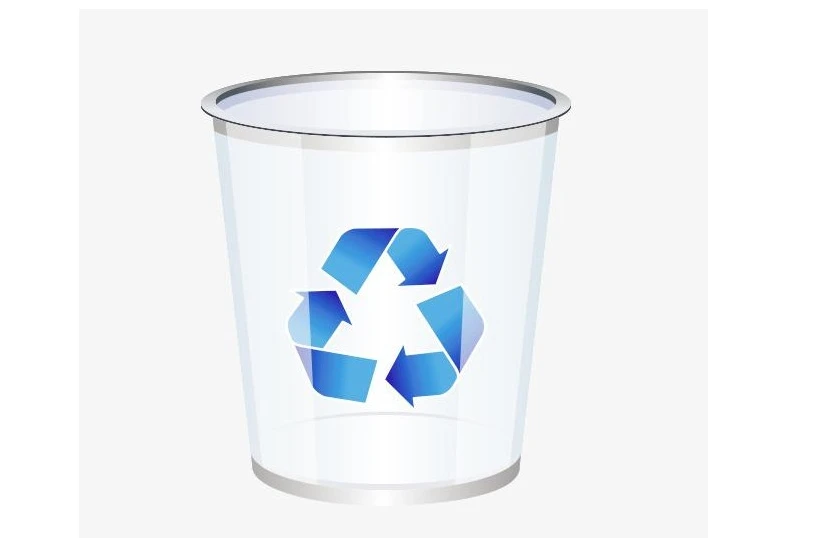
vcomp140.dll丢失的修复方法分享,电脑提示vcomp140.dll丢失修复方法
今天,我的电脑出现了一个奇怪的问题,打开某些程序时总是提示“找不到vcomp140.dll文件”。这个问题让我非常头疼,因为我无法正常使用电脑上的一些重要软件。为了解决这个问题,我在网上查找了很多资料,并尝试了多种方法…...
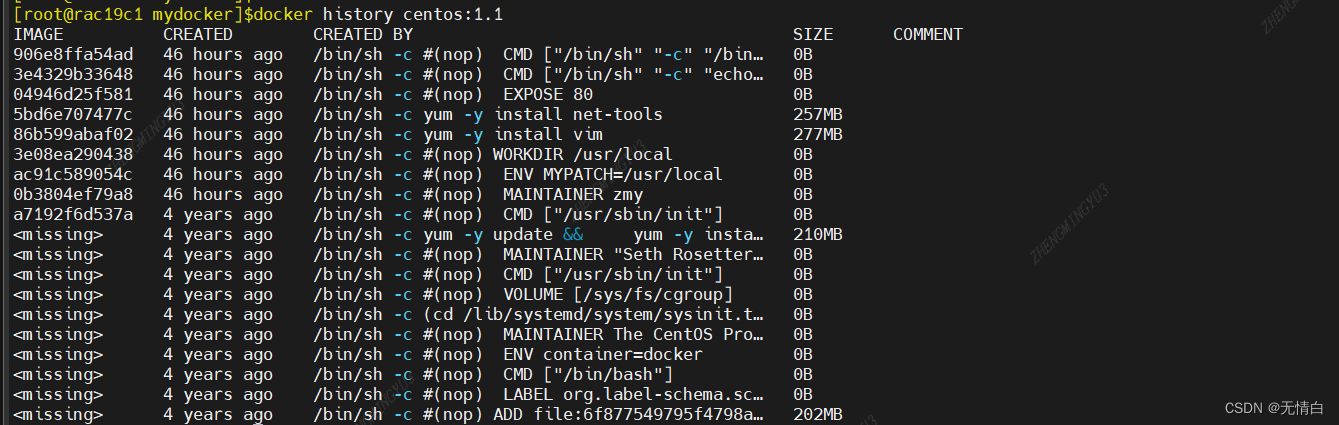
Docker file解析
文章目录 简介构建的三步骤Docker执行Dockerfile的大致流程DockerFile常用保留字指令创建第一个Dockerfile镜像的缓存特性 Docker file 解析 简介 Dockerfile是用来构建Docker镜像的文本文件,是由一条条构建镜像所需的指令和参数构成的脚本,记录了镜像构…...

工作与身体健康之间的平衡
大厂裁员,称35岁以后体能下滑,无法继续高效率地完成工作;体重上涨,因为35岁以后新陈代谢开始变慢;甚至坐久了会腰疼、睡眠困扰开始加重,在众多的归因中,仿佛35岁的到来,会为一切的焦…...

算法和数据结构
STL 【C】蓝桥杯必备 算法竞赛常用STL万字总结_蓝桥杯算法竞赛_Cpt1024的博客-CSDN博客 day1 1:正确 力扣(LeetCode)官网 - 全球极客挚爱的技术成长平台 // 中序遍历一遍二叉树,并统计节点数目 class Solution { public:int c…...

南昌做网站公司哪家好/搜索引擎优化答案
这是一款证件照制作的微信小程序,里面也支持直接微信公众号版本生成安装 支持多种尺寸制作 支持相册上传于直接相机拍摄 支持多种类型的证件制作如,职业证件,公务员证件,身份证等各种类型 支持电子照存档等等 拥有小程序推荐功能,可以给其它的小程序实现引流 另外还支持换…...

邯郸做企业网站设计的公司/google广告
功能1.支持行情图左右滑动2.支持行情图的惯性滑动3.支持行情图的方法和缩小4.支持 BOLL 和 MACD 技术指标(后面会继续丰富指标)5.支持主图副图动态添加,尺寸修改等6.支持长按滑动和长按弹框等效果图项目关键类行情图容器:MarketFigureChart行情图主图&am…...
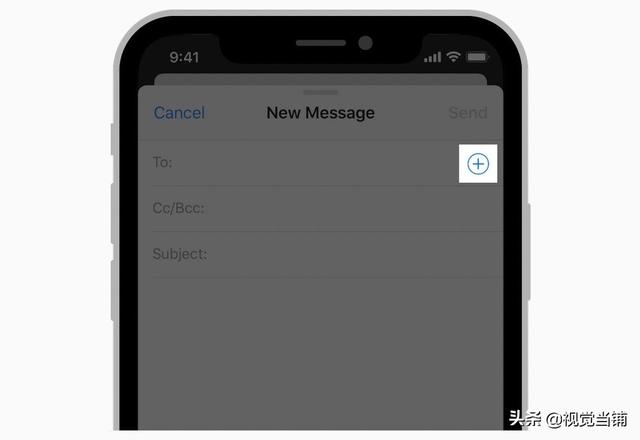
一个wordpress程序搭建多个网站/seo平台是什么
最近仔细研读了苹果官网最新的设计规范。网上没有找到很满意的翻译版本,于是自己老老实实的啃了几遍官方文档,顺便把学习笔记输出给大家分享一下。这里有几点要提醒一下大家:这是简明笔记,只选了重点内容,不是通篇逐字…...
天河区住房和建设水务局官方网站/关联词有哪些小学
整体架构图 Okhttp可以分为上层应用接口层,协议层,连接层,缓存层,I/O层,拦截器层。接口层就是我们上层开发人员调用的一些接口和API。连接层是核心,连接池以及网络请求优化都在这里面了。拦截器和缓存层是…...

通化公司做网站/搜索引擎排名竞价
第1篇:六年级上册数学圆的知识点整理一、认识圆1、圆的定义:圆是由曲线围成的一种平面图形。2、圆心:将一张圆形纸片对折两次,折痕相交于圆中心的一点,这一点叫做圆心。一般用字母o表示。它到圆上任意一点的距离都相等…...

网上虚拟银行注册网站/营销活动有哪些
对于刚接触linux系统的学员来说,确实是一件比较困难的事情,造成这种局面主要原因之一是windows的设计考虑到用户的体验效果,提供了更好的用户操作效果。以至于用户接触的最多的系统,所以刚接触linux的时候会感觉很不适应ÿ…...