MyBatis-Plus深入 —— 条件构造器与插件管理
前言
在前面的文章中,荔枝梳理了一个MyBatis-Plus的基本使用、配置和通用Service接口,我们发现在MyBatis-Plus的辅助增强下我们不再需要通过配置xml文件中的sql语句来实现基本的sql操作了,不愧是最佳搭档!在这篇文章中,荔枝会着重梳理有关MyBatis-Plus的两个知识点:条件构造器、分页插件和乐观锁插件,希望对有需要的小伙伴有帮助~~~
文章目录
前言
一、条件构造器
1.1 组装查询条件
1.2 组装排序条件
1.3 组装删除条件
1.4 使用QueryWrapper实现修改功能
1.5 条件优先级
1.6 子查询
1.7 使用UpdateWrapper实现修改功能
1.8 使用Condition组装条件
1.9 LambdaQueryWrapper
1.10 LambdaUpdateWrapper
二、分页插件
2.1 基本使用
2.2 自定义分页插件
三、乐观锁插件
3.1 乐观锁和悲观锁
3.2 乐观锁插件
总结
一、条件构造器
条件构造器,顾名思义就是用来封装当前我们用来查询的条件的,条件构造器的最顶层的接口是Mapper,被AbstractWrapper继承,其下由三个子类分别是:AbstractLambdaWrapper、UpdateWrapper和QueryWrapper。
1.1 组装查询条件
//条件构造器组装查询条件@Testpublic void testWrapper(){QueryWrapper<User> queryWrapper = new QueryWrapper<>();queryWrapper.like("user_name","crj").between("age",20,30).isNotNull("email");List<User> list = userMapper.selectList(queryWrapper);list.forEach(System.out::println);}
1.2 组装排序条件
//组装排序条件@Testpublic void test1(){//查询用户信息按照年龄的降序排序,若年龄相同则按照id升序排序QueryWrapper<User> queryWrapper = new QueryWrapper<>();queryWrapper.orderByDesc("age").orderByAsc("uid");List<User> list = userMapper.selectList(queryWrapper);list.forEach(System.out::println);}
1.3 组装删除条件
//组装删除条件@Testpublic void test2(){QueryWrapper<User> queryWrapper = new QueryWrapper<>();queryWrapper.isNull("email");int result = userMapper.delete(queryWrapper);System.out.println("受影响函数"+result);}
1.4 使用QueryWrapper实现修改功能
//实现修改功能@Testpublic void test3(){QueryWrapper<User> queryWrapper = new QueryWrapper<>();//把年龄大于20且姓名为crj或者是邮箱为null的用户信息进行修改queryWrapper.gt("age",20).like("user_name","crj").or().isNull("email");User user = new User();user.setName("CRJ");user.setEmail("123456@123.com");int result = userMapper.update(user,queryWrapper);System.out.println(result);}
1.5 条件优先级
在and()和or()中通过Lambda表达式实现优先级操作,其中Lambda表达式中的条件优先执行。
//条件优先级@Testpublic void test4(){//将用户名中含有crj并且(年龄大于20或邮箱为null)的用户信息修改//lambda中的条件优先执行QueryWrapper<User> queryWrapper = new QueryWrapper<>();queryWrapper.like("user_name","crj").and(i->i.gt("age",20).or().isNull("email"));User user = new User();user.setName("CRJ");user.setEmail("123456@123.com");int result = userMapper.update(user,queryWrapper);System.out.println(result);}
1.6 子查询
//子查询@Testpublic void test5(){//查询id小于等于100的用户信息QueryWrapper<User> queryWrapper = new QueryWrapper<>();queryWrapper.inSql("uid","select uid from t_user where uid<=100");List<User> list = userMapper.selectList(queryWrapper);}
1.7 使用UpdateWrapper实现修改功能
//使用UpdateWrapper实现修改功能
//将用户名中含有crj并且(年龄大于20或邮箱为null)的用户信息修改@Testpublic void test6(){UpdateWrapper<User> updateWrapper = new UpdateWrapper<>();updateWrapper.like("user_name","crj").and(i->i.gt("age",20).or().isNull("email"));updateWrapper.set("user_name","CRJ");userMapper.update(null,updateWrapper);}
1.8 使用Condition组装条件
1.9 LambdaQueryWrapper
@Testpublic void test8(){String username = "a";Integer ageBegin = null;Integer ageEnd = 30;LambdaQueryWrapper<User> queryWrapper = new LambdaQueryWrapper<>();queryWrapper.like(StringUtils.isNotBlank(username),User::getName,username).ge(ageBegin!=null,User::getAge,ageBegin).le(ageEnd!=null,User::getAge,ageEnd);List<User> list = userMapper.selectList(queryWrapper);list.forEach(System.out::println);}
1.10 LambdaUpdateWrapper
@Testpublic void test9(){LambdaUpdateWrapper<User> updateWrapper = new LambdaUpdateWrapper<>();updateWrapper.like(User::getName,"crj").and(i->i.gt(User::getAge,20).or().isNull(User::getEmail));updateWrapper.set(User::getName,"CRJ");userMapper.update(null,updateWrapper);}
二、分页插件
2.1 基本使用
分页插件的配置类
package com.crj.mybatisplus_test.config;import com.baomidou.mybatisplus.annotation.DbType;
import com.baomidou.mybatisplus.extension.plugins.MybatisPlusInterceptor;
import com.baomidou.mybatisplus.extension.plugins.inner.PaginationInnerInterceptor;
import org.mybatis.spring.annotation.MapperScan;
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;//配置类,配置MyBatisPlus的插件功能
@Configuration
@MapperScan("com.crj.mybatisplus_test.mapper")
public class MyBatisPlusConfig {@Beanpublic MybatisPlusInterceptor mybatisPlusInterceptor(){MybatisPlusInterceptor interceptor = new MybatisPlusInterceptor();interceptor.addInnerInterceptor(new PaginationInnerInterceptor(DbType.MYSQL));return interceptor;}
}
测试类
package com.crj.mybatisplus_test;import com.baomidou.mybatisplus.extension.plugins.pagination.Page;
import com.crj.mybatisplus_test.mapper.UserMapper;
import com.crj.mybatisplus_test.pojo.User;
import org.junit.jupiter.api.Test;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.boot.test.context.SpringBootTest;@SpringBootTest
public class MyBatisPlusPluginsTest {@Autowiredprivate UserMapper userMapper;@Testpublic void test1(){Page<User> page = new Page<>(1,3);userMapper.selectPage(page,null);System.out.println(page);}
}
page对象的几个方法:
- page.getRecords(): 获取当前页数据
- page.getCurrent():获取当前页的页码
- page.getSize():获取每页显示的条数
- page.getPages(): 获取总页数
- page.getTotal(): 获取总记录数
- page.hasNext(): 查看有没有下一页
- page.hasPrevious():查看有没有上一页
配置类型别名:
mybatis-plus:
type-aliases-package:全路径
2.2 自定义分页插件
之前借助条件构造器来实现分页的操作,通过查看源码知晓,selectPage要求两个参数,返回值和第一个参数都是IPage类型的,而IPage类型的接口是被Page类对象实现的,因此第一个参数一定是page对象。我们需要在userMapper接口中手写一个方法替代原来的selectPage,同时分页插件的配置文件保持不变,配置好MyBatisPlus的插件功能
UserMapper.java
package com.crj.mybatisplus_test.mapper;import com.baomidou.mybatisplus.core.mapper.BaseMapper;
import com.baomidou.mybatisplus.extension.plugins.pagination.Page;
import com.crj.mybatisplus_test.pojo.User;
import org.apache.ibatis.annotations.Param;
import org.springframework.stereotype.Repository;@Repository
//继承MyBatis-Plus的BaseMapper接口
public interface UserMapper extends BaseMapper<User> {/*** 根据年龄查询用户信息并分页* @param page mybatis-plus提供的分页对象,必须放在第一个参数中* @param age* @return*/Page<User> selectPageVo(@Param("page") Page<User> page,@Param("age") Integer age);
}
UserMapper.xml
<?xml version="1.0" encoding="UTF-8" ?>
<!DOCTYPE mapperPUBLIC "-//mybatis.org//DTD Mapper 3.0//EN""http://mybatis.org/dtd/mybatis-3-mapper.dtd"><mapper namespace="com.crj.mybatisplus_test.mapper.UserMapper"><!-- Page<User> selectPageVo(@Param("page") Page<User> page,@Param("age") Integer age);--><select id="selectPageVo" resultType="User">select uid,name,age from t_user where age > #{age}</select>
</mapper>
测试类
@Testpublic void testPageVo(){Page<User> page = new Page<>(1,3);userMapper.selectPageVo(page,20);}
三、乐观锁插件
3.1 乐观锁和悲观锁
说到乐观锁和悲观锁,我们经常通过一个场景来理解:我们需要对一个值为100的数进行+10操作再进行-30操作,这两步使用多线程执行。A和B线程同时取一个值为100的数C,A对C进行+10操作,B对取出来的值进行-30的操作,如果没有加锁控制,那么A处理的值D不能被B拿到且会被B覆盖。对于加锁这里简单归纳两种:乐观锁和悲观锁,悲观锁会格外注重线程安全,只有等A操作完后才能由B取值;而乐观锁则是通过版本控制的方式来检测是否C被修改了。
未加锁的场景模拟
实体类
package com.crj.mybatisplus_test.pojo;import lombok.Data;@Data
public class Product {private Long id;private String name;private Integer price;private Integer version;}
mapper接口
package com.crj.mybatisplus_test.mapper;import com.baomidou.mybatisplus.core.mapper.BaseMapper;
import com.crj.mybatisplus_test.pojo.Product;
import org.springframework.stereotype.Service;@Service
public interface ProductMapper extends BaseMapper<Product> {}
测试类
package com.crj.mybatisplus_test;import com.crj.mybatisplus_test.mapper.ProductMapper;
import com.crj.mybatisplus_test.pojo.Product;
import org.junit.jupiter.api.Test;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.boot.test.context.SpringBootTest;//乐观锁插件使用
@SpringBootTest
public class MyBatisLockTest {@Autowiredprivate ProductMapper productMapper;//模拟线程场景@Testpublic void test1(){Product productA = productMapper.selectById(1);System.out.println("A查询的商品价格"+productA.getPrice());Product productB = productMapper.selectById(1);System.out.println("B查询的商品价格"+productB.getPrice());productA.setPrice(productA.getPrice()+10);productMapper.updateById(productA);productB.setPrice(productB.getPrice()-30);productMapper.updateById(productB);//最后结果Product productC = productMapper.selectById(1);System.out.println("A查询的商品价格"+productC.getPrice());}
}
3.2 乐观锁插件
前面知道乐观锁实现需要加上版本号来控制,因此实体类需要进行通过@Version来设置版本号。
实体类
package com.crj.mybatisplus_test.pojo;import com.baomidou.mybatisplus.annotation.Version;
import lombok.Data;@Data
public class Product {private Long id;private String name;private Integer price;@Version //标识乐观锁版本号字段private Integer version;}
MyBatis-Plus插件配置类
需要在配置类中配置好乐观锁插件方法OptimisticLockerInnerInterceptor()
package com.crj.mybatisplus_test.config;import com.baomidou.mybatisplus.annotation.DbType;
import com.baomidou.mybatisplus.extension.plugins.MybatisPlusInterceptor;
import com.baomidou.mybatisplus.extension.plugins.inner.OptimisticLockerInnerInterceptor;
import com.baomidou.mybatisplus.extension.plugins.inner.PaginationInnerInterceptor;
import org.mybatis.spring.annotation.MapperScan;
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;//配置类,配置MyBatisPlus的插件功能
@Configuration
@MapperScan("com.crj.mybatisplus_test.mapper")
public class MyBatisPlusConfig {@Beanpublic MybatisPlusInterceptor mybatisPlusInterceptor(){MybatisPlusInterceptor interceptor = new MybatisPlusInterceptor();//配置分页插件interceptor.addInnerInterceptor(new PaginationInnerInterceptor(DbType.MYSQL));//配置乐观锁插件interceptor.addInnerInterceptor(new OptimisticLockerInnerInterceptor());return interceptor;}
}
测试类
需要注意的是B修改数据失败后需要重试即可完成任务需求。
package com.crj.mybatisplus_test;import com.crj.mybatisplus_test.mapper.ProductMapper;
import com.crj.mybatisplus_test.pojo.Product;
import org.junit.jupiter.api.Test;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.boot.test.context.SpringBootTest;//乐观锁插件使用
@SpringBootTest
public class MyBatisLockTest {@Autowiredprivate ProductMapper productMapper;//模拟线程场景@Testpublic void test1(){Product productA = productMapper.selectById(1);System.out.println("A查询的商品价格"+productA.getPrice());Product productB = productMapper.selectById(1);System.out.println("B查询的商品价格"+productB.getPrice());productA.setPrice(productA.getPrice()+10);productMapper.updateById(productA);productB.setPrice(productB.getPrice()-30);int result = productMapper.updateById(productB);//由于加入了版本号控制,因此需要对修改失败的操作进行重试if(result==0){//失败重试Product productNew = productMapper.selectById(1);productNew.setPrice(productNew.getPrice()-30);productMapper.updateById(productNew);}//最后结果Product productC = productMapper.selectById(1);System.out.println("A查询的商品价格"+productC.getPrice());}
}
总结
通过条件构造器的几种基本用法使用示例,荔枝对wrapper类的使用有了一个比较直观的理解,同时荔枝觉得更需要注意的是两种插件的使用。接下来的文章中荔枝会对MyBatis-Plus的相关基础知识收尾,同时尝试整合到学习的项目中,跟荔枝一起期待一波吧哈哈哈哈哈~~~
今朝已然成为过去,明日依然向往未来!我是小荔枝,在技术成长的路上与你相伴,码文不易,麻烦举起小爪爪点个赞吧哈哈哈~~~ 比心心♥~~~
相关文章:

MyBatis-Plus深入 —— 条件构造器与插件管理
前言 在前面的文章中,荔枝梳理了一个MyBatis-Plus的基本使用、配置和通用Service接口,我们发现在MyBatis-Plus的辅助增强下我们不再需要通过配置xml文件中的sql语句来实现基本的sql操作了,不愧是最佳搭档!在这篇文章中,…...
C语言结构体的初始化方式
逐个初始化字段:这是最直接的方式,你可以逐个为结构体的每个字段进行初始化。 struct Student { char name[50]; int age; float marks; }; struct Student student1 {"Alice", 20, 89.5}; 2.使用结构体字面值初始化:这种方…...
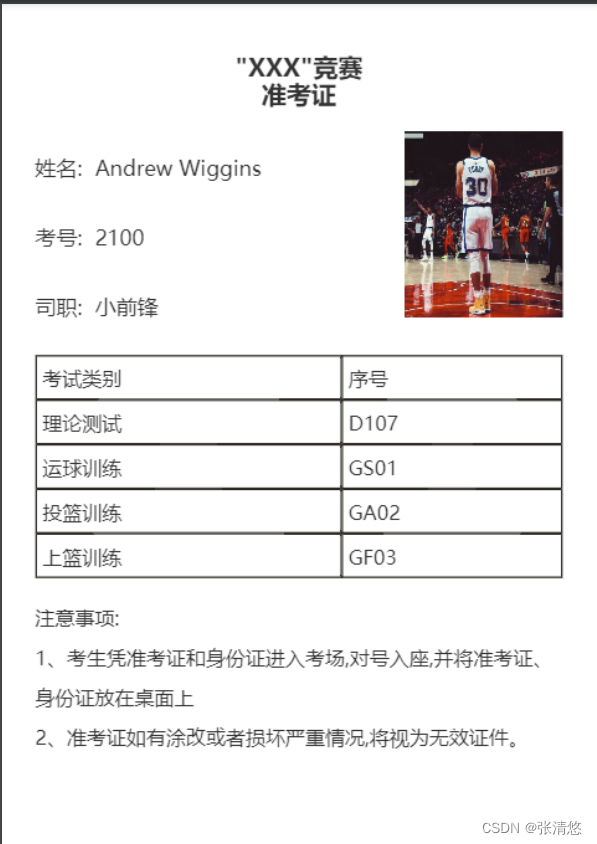
Vue生成多文件pdf准考证
这是渲染的数据 这是生成的pdf文件,直接可以打印 需要安装和npm依赖和引入封装的pdf.js文件 npm install --save html2canvas // 页面转图片 npm install jspdf --save // 图片转pdfpdf.js文件 import html2canvas from "html2canvas"; import jsPDF …...
Rust的derive思考
这几天在Yew的学习实践中,发现derive中的参数中包含了yew自己的东西,比如yew::Properties。习惯使用#[derive(Clone, Debug, PartialEq)]之后,发现还有新的成员,这让我好奇起来。 首先让我们来回顾一下derive是什么。 #[derive(…...
Python常用模块
文章目录 1. time:时间2. calendar:日历3. datetime:可以运算的时间4. sys:系统5. os:操作系统6. random:随机数7. json:序列化8. pickle:序列化9. logging 模块9.1 什么是logging模…...
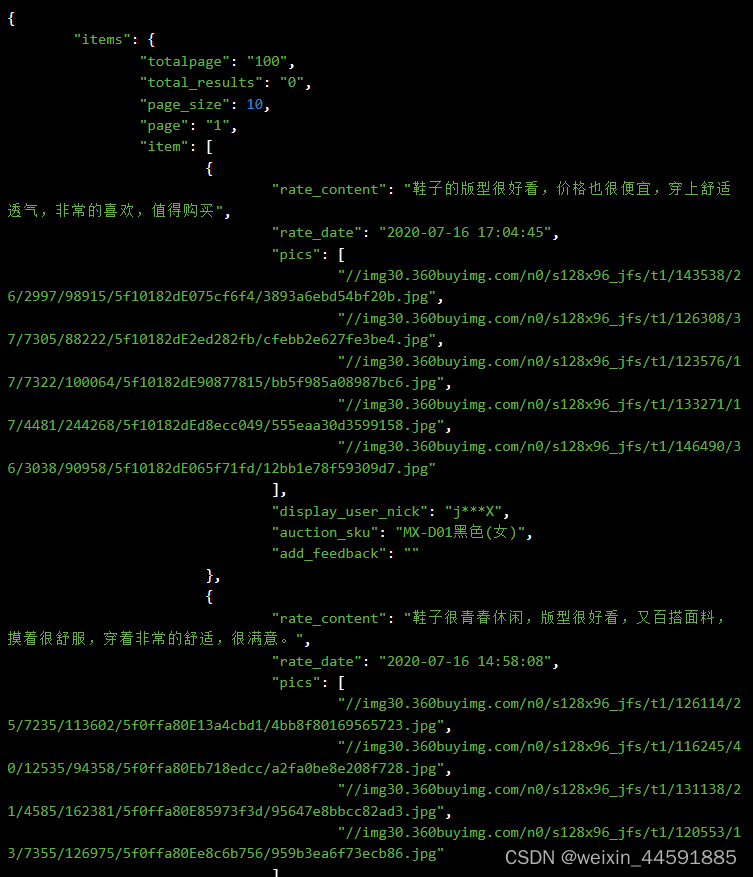
Java“牵手”京东商品评论数据接口方法,京东商品评论接口,京东商品评价接口,行业数据监测,京东API实现批量商品评论内容数据抓取示例
京东平台商品评论数据接口是开放平台提供的一种API接口,通过调用API接口,开发者可以获取京东商品的标题、价格、库存、月销量、总销量、库存、详情描述、图片、评论内容、评论日期、评论图片、追评内容等详细信息 。 获取商品评论接口API是一种用于获取…...
算法leetcode|75. 颜色分类(rust重拳出击)
文章目录 75. 颜色分类:样例 1:样例 2:提示: 分析:题解:rust:go:c:python:java: 75. 颜色分类: 给定一个包含红色、白色和蓝色、共 n…...
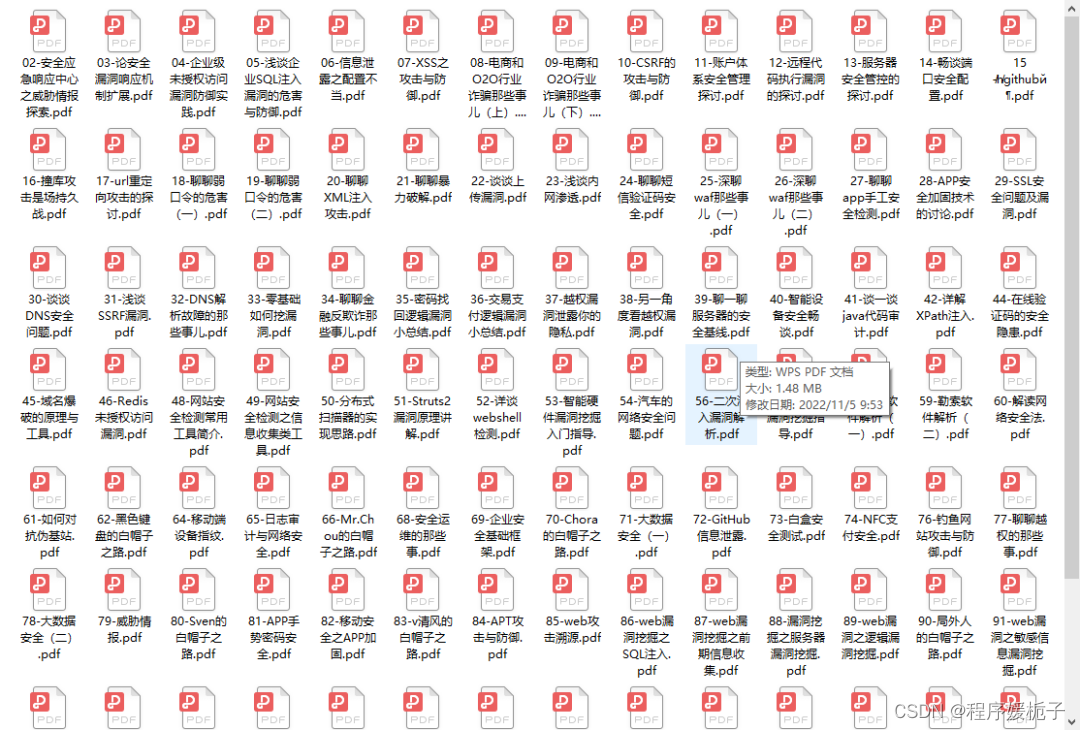
网络安全(黑客)自学笔记学习路线
谈起黑客,可能各位都会想到:盗号,其实不尽然;黑客是一群喜爱研究技术的群体,在黑客圈中,一般分为三大圈:娱乐圈 技术圈 职业圈。 娱乐圈:主要是初中生和高中生较多,玩网恋…...
NoSQL:非关系型数据库分类
NoSQL,全称 Not Only SQL,意为不仅仅是 SQL,泛指非关系型数据库。NoSQL 是基于键值对的,而且不需要经过 SQL 层的解析,数据之间没有耦合性,性能非常高。 非关系型数据库又可细分如下: 键值存储…...
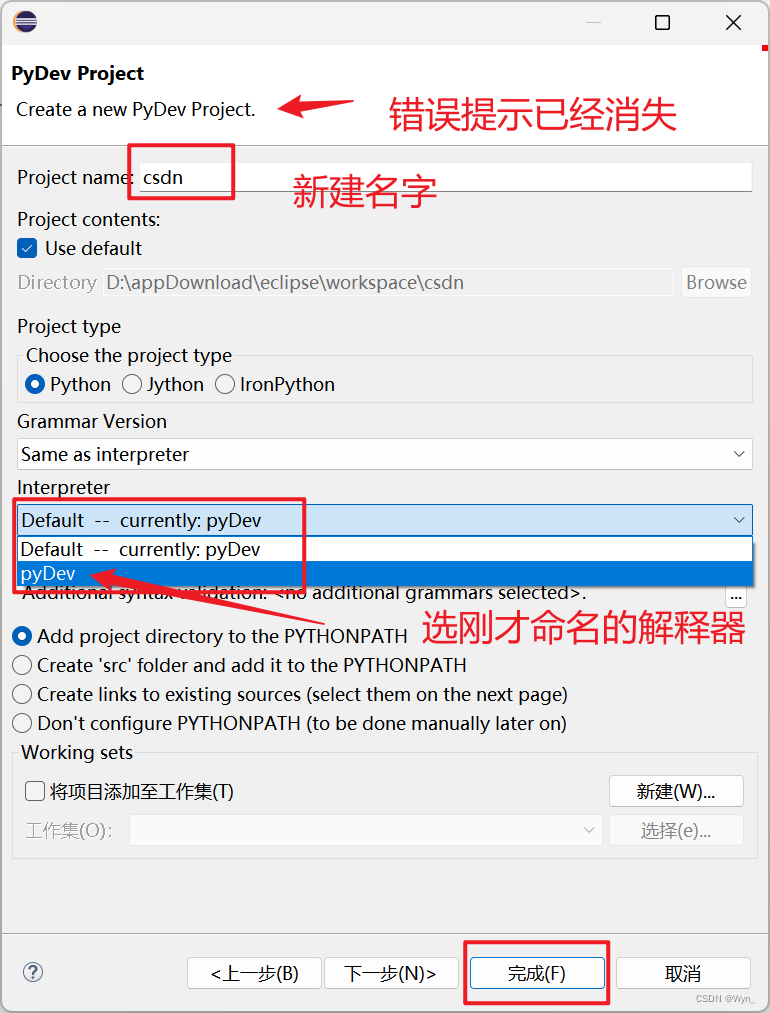
【Eclipse】Project interpreter not specified 新建项目时,错误提示,已解决
目录 0.环境 1)问题截图: 2)错误发生原因: 1.解决思路 2.具体步骤 0.环境 windows 11 64位,Eclipse 2021-06 1)问题截图: 2)错误发生原因: 由于我手欠,将…...
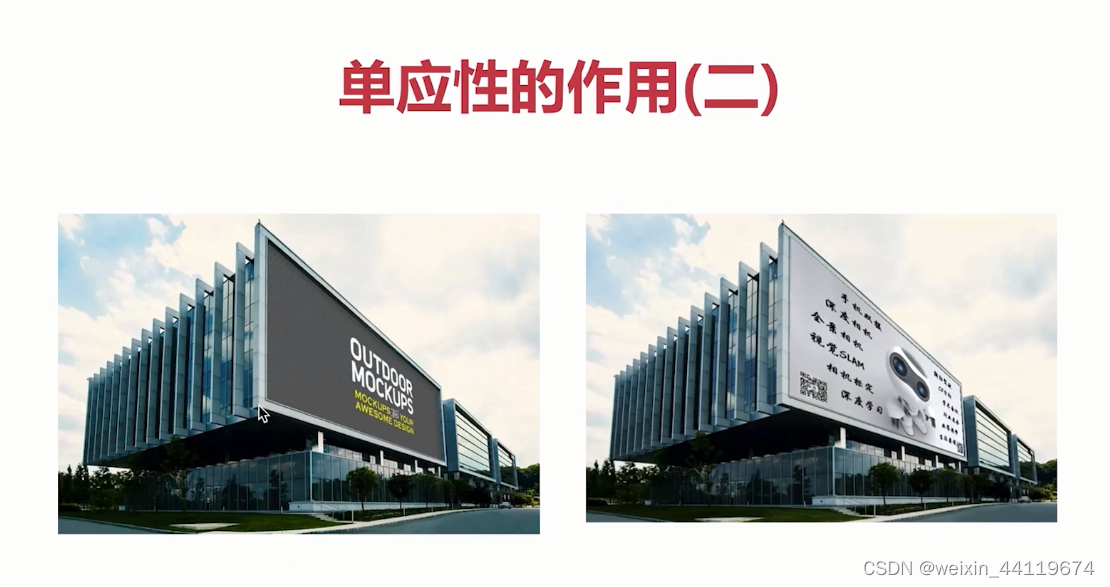
OPENCV实现图像查找
特征匹配+单应性矩阵 # -*- coding:utf-8 -*- """ 作者:794919561 日期:2023/9/4 """ import cv2 import numpy as np# 读图像 img1 = cv2.imread(F:\\learnOpenCV\\openCVLearning\\pictures\\chess...
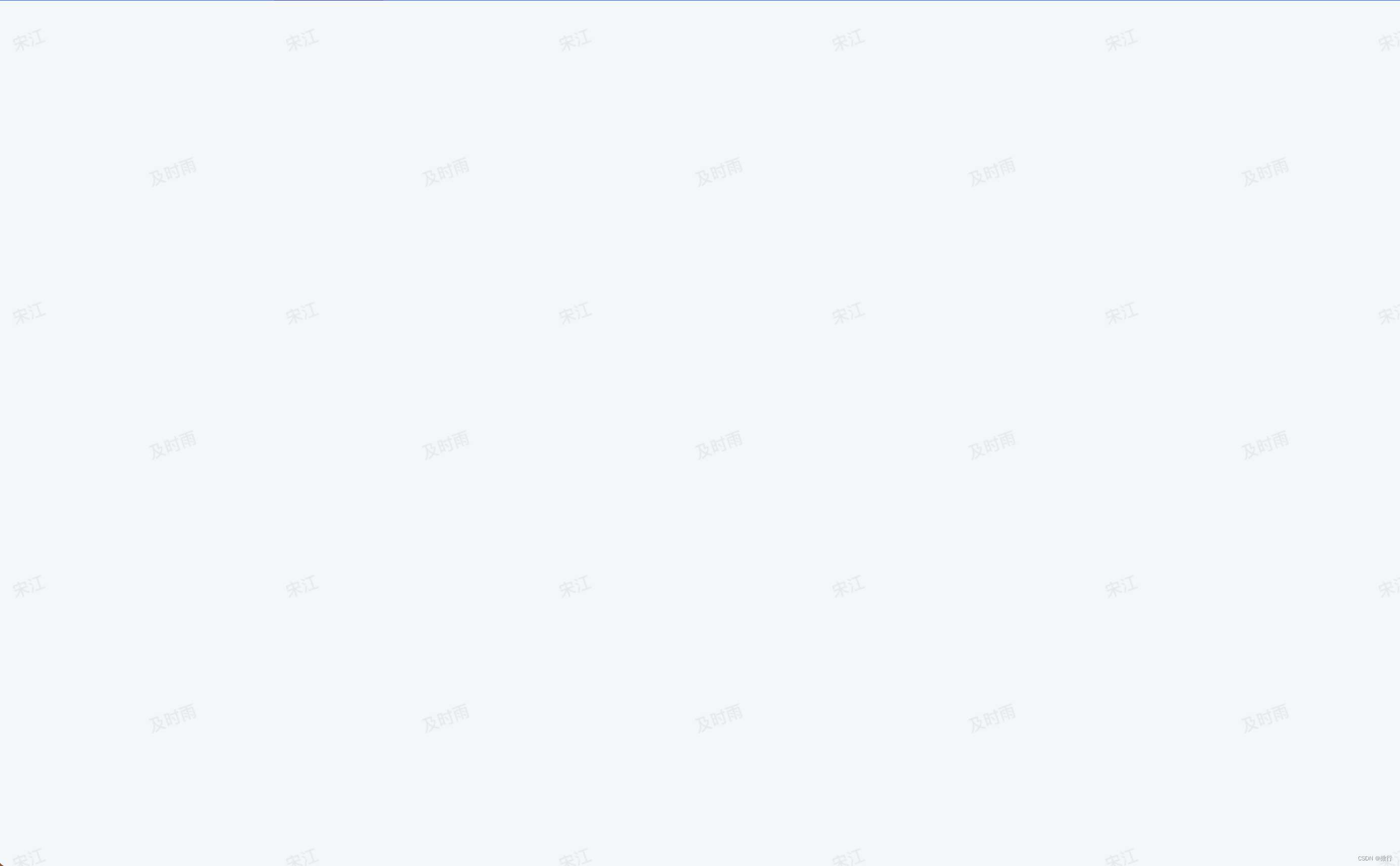
vue仿企微文档给页面加水印(水印内容可自定义,超简单)
1.在src下得到utils里新建一个文件watermark.js /** 水印添加方法 */let setWatermark (str1, str2) > {let id 1.23452384164.123412415if (document.getElementById(id) ! null) {document.body.removeChild(document.getElementById(id))}let can document.createE…...
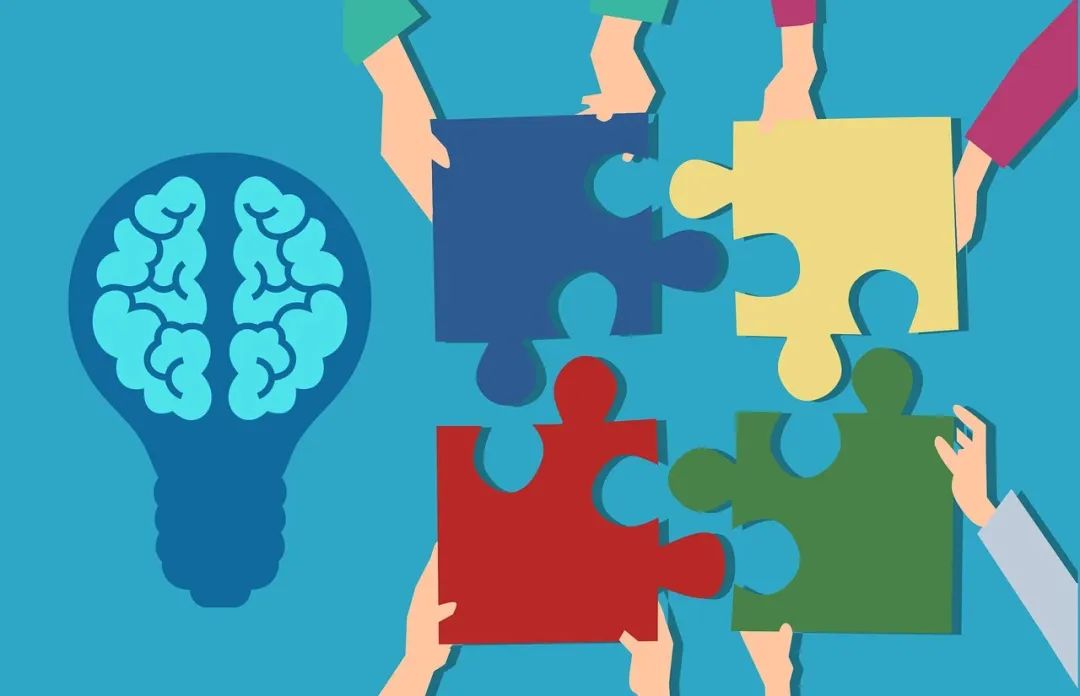
“金融级”数字底座:从时代的“源启”,到“源启”的时代
今年初《数字中国建设整体布局规划》正式发布,这代表着数字中国建设迈向了实质的落地阶段,其背后的驱动就是遍及各行各业的数字化转型。 千姿百态、复杂多样的应用场景,可以看作是遍布数字中国的“点”;千行百业、各种类型的行业…...
zabbix自动发现linux系统挂载的nas盘,并实现读写故障的监控告警
一.准备好被监控机器上面执行脚本,以备服务端发现和监控 脚本的内容: ZABBI安装路径可执行文件及配置文件根据实际部署的路径更改 #!/bin/bash >/zabbixconfpath/zbx_nas.conf >/zabbixscriptspath/findnas.sh >/zabbixscriptspath/checknas.sh >/zabbixscripts…...
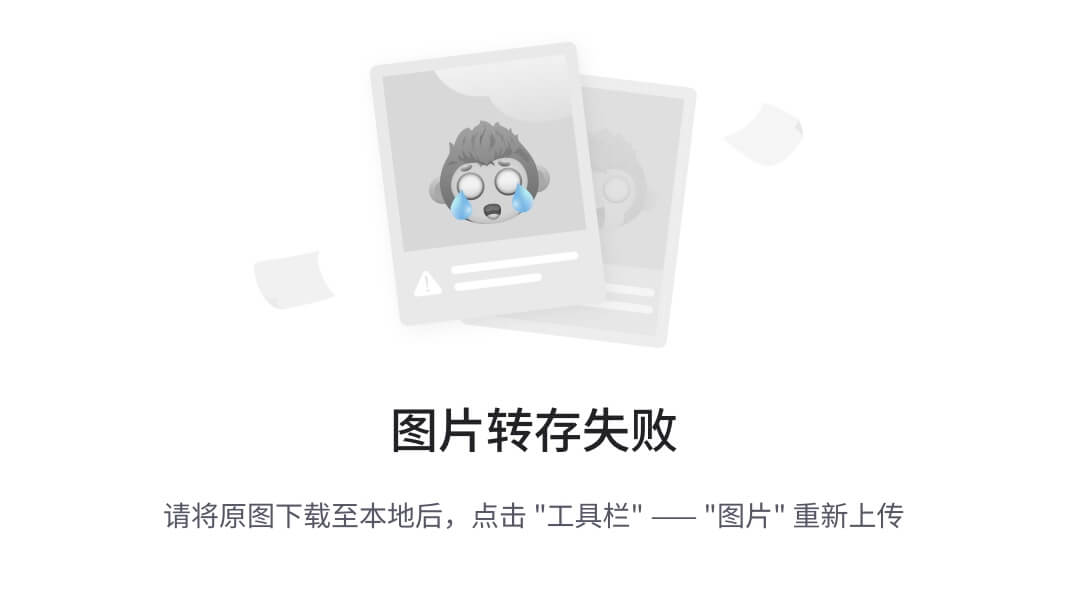
无涯教程-JavaScript - DAYS函数
描述 DAYS函数返回两个日期之间的天数。 语法 DAYS (end_date, start_date)争论 Argument描述Required/OptionalEnd_dateStart_date and End_date are the two dates between which you want to know the number of days.RequiredStart_dateStart_date and End_date are th…...
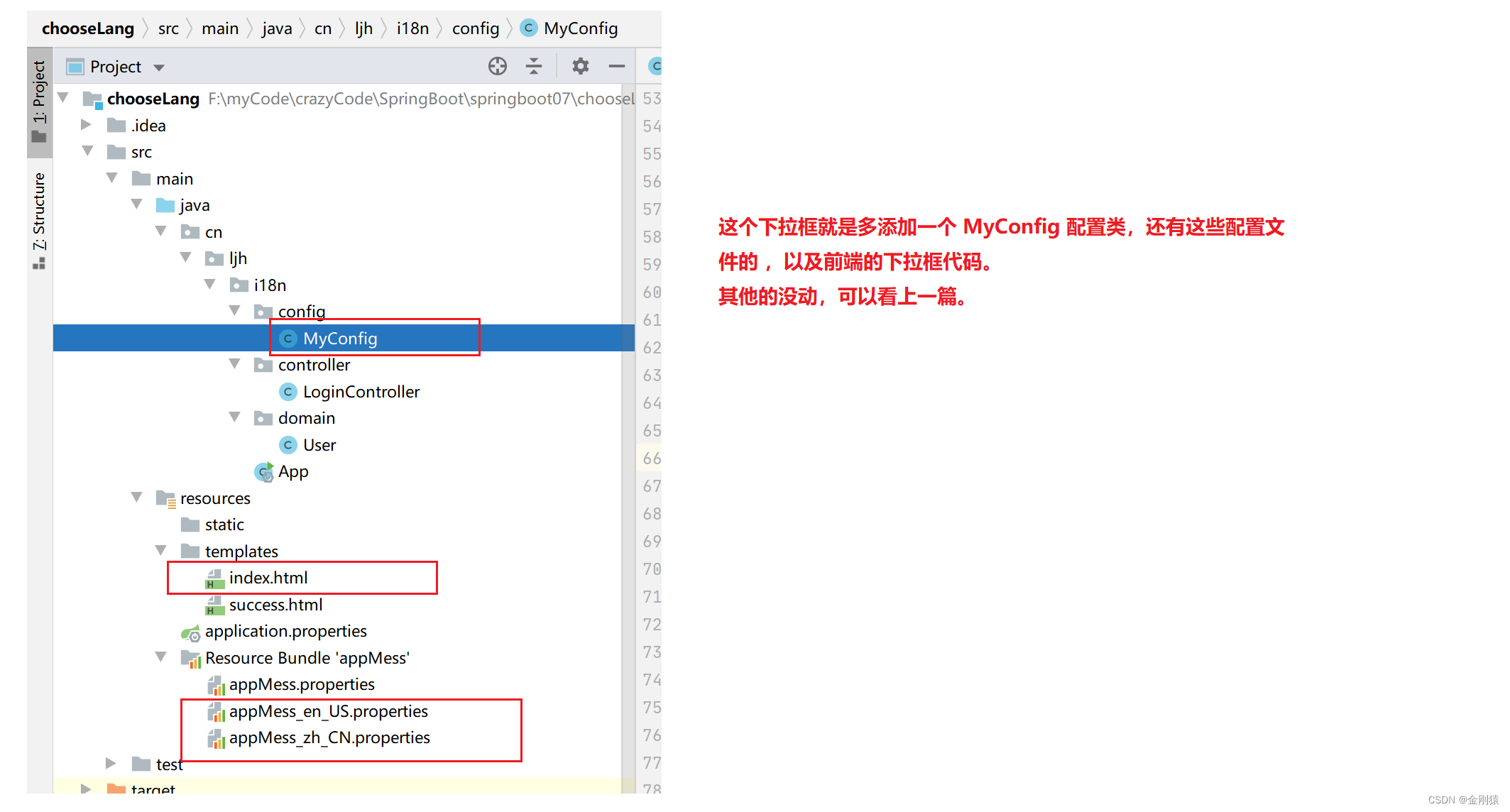
48、springboot 的国际化之让用户在程序界面上弄个下拉框,进行动态选择语言
上一篇是直接改浏览器的支持语言。 在浏览器上面直接改国际化语言 这次要实现的功能是直接在程序界面动态选择语言。 Locale 代表语言、国家。 ★ 在界面上动态改变语言 应用之所以能动态呈现不同的语言界面,其实关键在于如何确定客户端的Locale(代…...
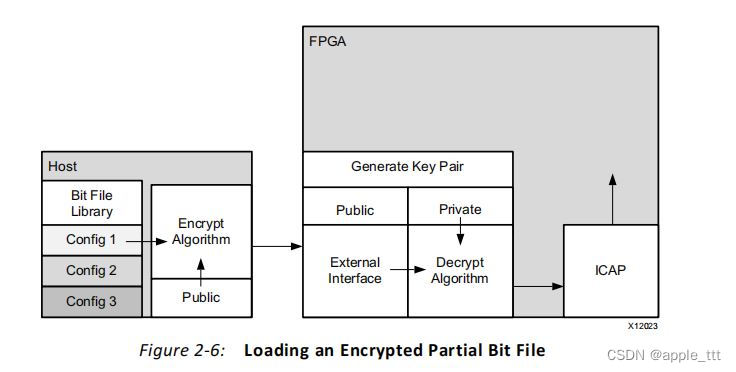
FPGA可重配置原理及实现(1)——导论
一、概述 可重配置技术是Xilinx提供的用来高效利用FPGA设计资源实现FPGA资源可重复利用的最新的FPGA设计技术,这种技术的发展为FPGA应用提供了更加广阔的前景。 术语“重构”是指FPGA已经配置后的重新编程。FPGA的重构有两种类型:完全的和部分的。完全重…...
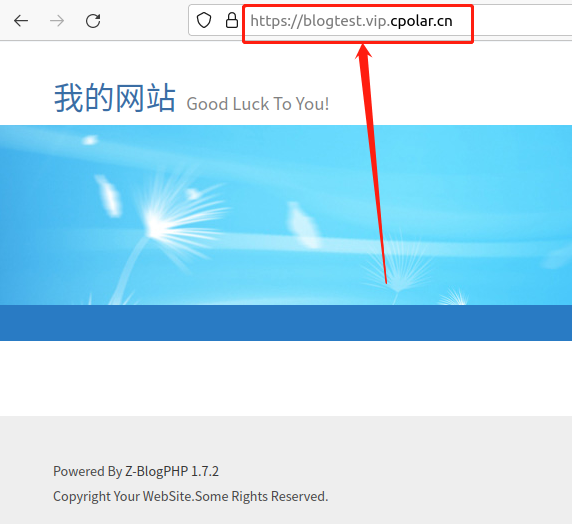
Ubuntu系统下使用宝塔面板实现一键搭建Z-Blog个人博客的方法和流程
文章目录 1.前言2.网站搭建2.1. 网页下载和安装2.2.网页测试2.3.cpolar的安装和注册 3.本地网页发布3.1.Cpolar临时数据隧道3.2.Cpolar稳定隧道(云端设置)3.3.Cpolar稳定隧道(本地设置) 4.公网访问测试5.结语 1.前言 Ubuntu系统作…...
数据结构 | 第一章 绪论
问题求解与程序设计 这一节都是介绍性的内容,但是哥尼斯堡的七桥问题值得写写。 #include <stdio.h>int Euler(int mat[4][4], int n) {int count 0;for (int i 0; i < n; i) {int degree 0;for (int j 0; j < n; j) {degree mat[i][j];}if (degr…...
python爬虫入门教程(非常详细):如何快速入门Python爬虫?
示例示例Python爬虫入门教程什么是爬虫爬虫(又称网络爬虫)是一种按照一定的规则,自动地抓取万维网信息的程序或者脚本。它可以自动地抓取网页内容,并从中提取有用的数据,存储到本地文件或数据库中。 Python爬虫入门教…...
Vue记事本应用实现教程
文章目录 1. 项目介绍2. 开发环境准备3. 设计应用界面4. 创建Vue实例和数据模型5. 实现记事本功能5.1 添加新记事项5.2 删除记事项5.3 清空所有记事 6. 添加样式7. 功能扩展:显示创建时间8. 功能扩展:记事项搜索9. 完整代码10. Vue知识点解析10.1 数据绑…...
Qt Widget类解析与代码注释
#include "widget.h" #include "ui_widget.h"Widget::Widget(QWidget *parent): QWidget(parent), ui(new Ui::Widget) {ui->setupUi(this); }Widget::~Widget() {delete ui; }//解释这串代码,写上注释 当然可以!这段代码是 Qt …...
【ROS】Nav2源码之nav2_behavior_tree-行为树节点列表
1、行为树节点分类 在 Nav2(Navigation2)的行为树框架中,行为树节点插件按照功能分为 Action(动作节点)、Condition(条件节点)、Control(控制节点) 和 Decorator(装饰节点) 四类。 1.1 动作节点 Action 执行具体的机器人操作或任务,直接与硬件、传感器或外部系统…...
Spring Boot面试题精选汇总
🤟致敬读者 🟩感谢阅读🟦笑口常开🟪生日快乐⬛早点睡觉 📘博主相关 🟧博主信息🟨博客首页🟫专栏推荐🟥活动信息 文章目录 Spring Boot面试题精选汇总⚙️ **一、核心概…...
C++中string流知识详解和示例
一、概览与类体系 C 提供三种基于内存字符串的流,定义在 <sstream> 中: std::istringstream:输入流,从已有字符串中读取并解析。std::ostringstream:输出流,向内部缓冲区写入内容,最终取…...
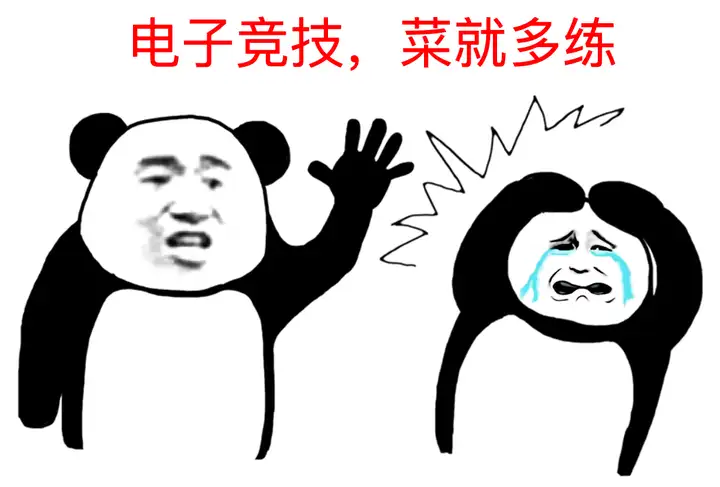
如何在最短时间内提升打ctf(web)的水平?
刚刚刷完2遍 bugku 的 web 题,前来答题。 每个人对刷题理解是不同,有的人是看了writeup就等于刷了,有的人是收藏了writeup就等于刷了,有的人是跟着writeup做了一遍就等于刷了,还有的人是独立思考做了一遍就等于刷了。…...
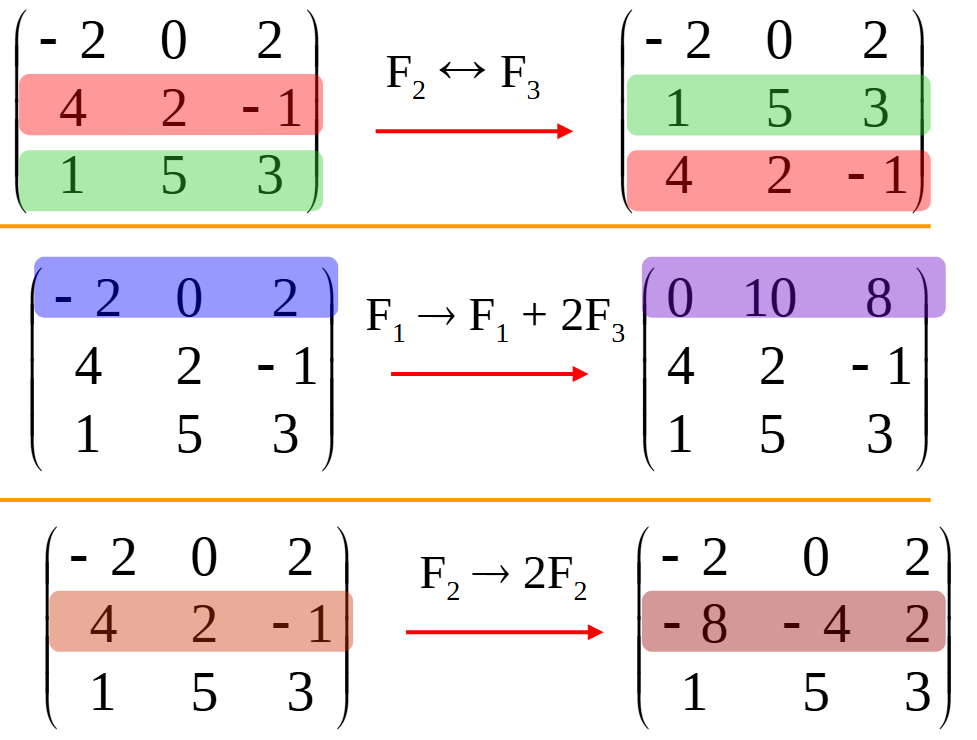
使用 SymPy 进行向量和矩阵的高级操作
在科学计算和工程领域,向量和矩阵操作是解决问题的核心技能之一。Python 的 SymPy 库提供了强大的符号计算功能,能够高效地处理向量和矩阵的各种操作。本文将深入探讨如何使用 SymPy 进行向量和矩阵的创建、合并以及维度拓展等操作,并通过具体…...
蓝桥杯 冶炼金属
原题目链接 🔧 冶炼金属转换率推测题解 📜 原题描述 小蓝有一个神奇的炉子用于将普通金属 O O O 冶炼成为一种特殊金属 X X X。这个炉子有一个属性叫转换率 V V V,是一个正整数,表示每 V V V 个普通金属 O O O 可以冶炼出 …...
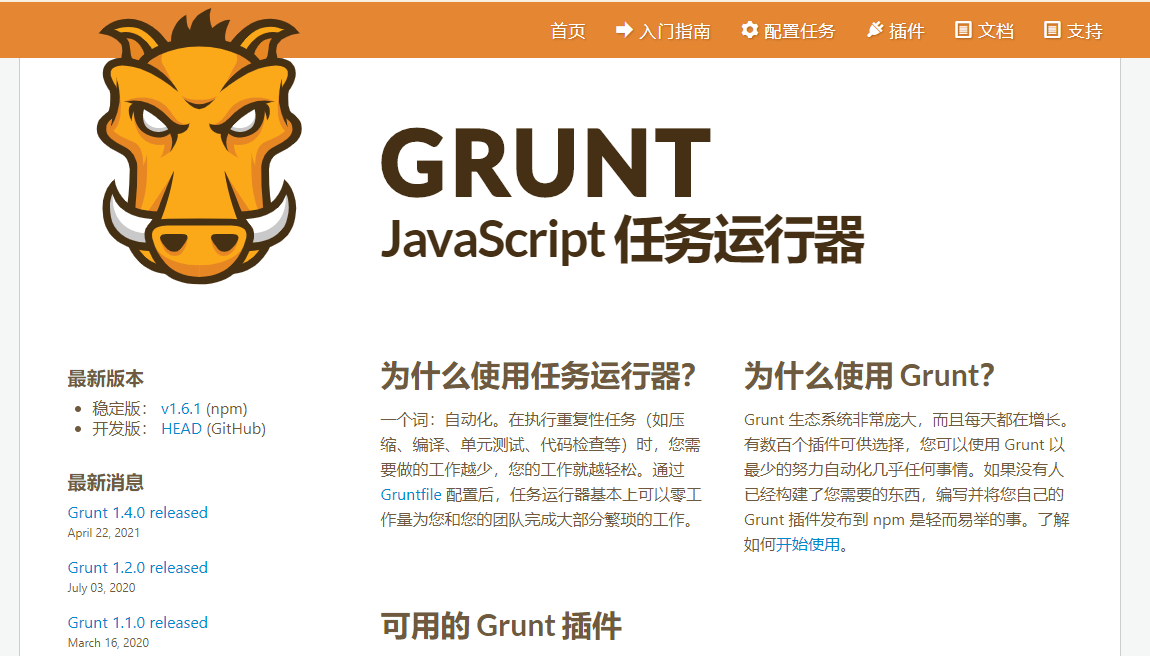
GruntJS-前端自动化任务运行器从入门到实战
Grunt 完全指南:从入门到实战 一、Grunt 是什么? Grunt是一个基于 Node.js 的前端自动化任务运行器,主要用于自动化执行项目开发中重复性高的任务,例如文件压缩、代码编译、语法检查、单元测试、文件合并等。通过配置简洁的任务…...
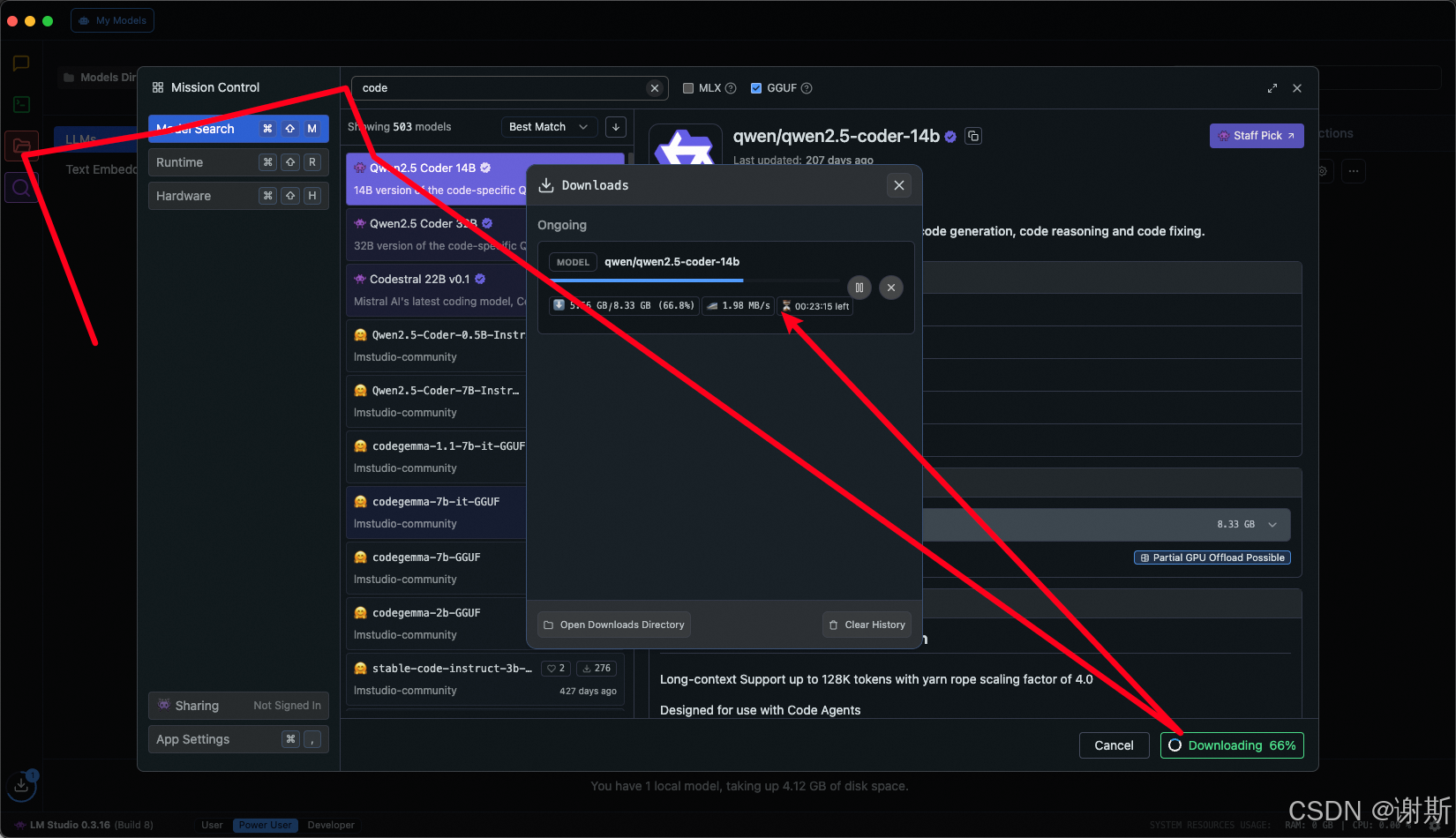
[大语言模型]在个人电脑上部署ollama 并进行管理,最后配置AI程序开发助手.
ollama官网: 下载 https://ollama.com/ 安装 查看可以使用的模型 https://ollama.com/search 例如 https://ollama.com/library/deepseek-r1/tags # deepseek-r1:7bollama pull deepseek-r1:7b改token数量为409622 16384 ollama命令说明 ollama serve #:…...