Mybatis的三种映射关系以及联表查询
目录
一、概念
二、一对一
1、配置generatorConfig.xml
2、Vo包的编写
3、xml的sql编写
4、编写对应接口及实现类
5、测试
三、一对多
1、Vo包类的编写
2、xml的sql编写
3、编写对应接口及实现类
4、测试
四、多对多
1、Vo类
2、xml的sql配置
3、接口及接口实现类
4、测试
一、概念
1、MyBatis中表之间的关系是如何映射的处理的?
resultType:使用多表查询我们经常会resultType="java.utils.Map" ,我们不推荐这样写,但是这样写对自己比较有利。
好处:resultType 是直接将查询结果映射到 Java 对象,可以使用简单的类型(如 int、String)或复杂的自定义类型。它的好处是简单直观,易于使用。
弊端:对于复杂的关系映射,resultType 可能会变得冗长,并且无法处理一对多或多对多的关系映射。
resultMap:resultMap 允许我们定义复杂的映射规则,将结果集中的多个字段映射到一个对象中。
好处:可以处理复杂的关系映射,支持一对多或多对多的关系映射。我们可以在 resultMap 中定义映射规则,指定字段与属性间的映射关系,并通过嵌套 resultMap 处理表之间的关系。
弊端:相对于 resultType,resultMap 的配置较为繁琐。
二、一对一
1、配置generatorConfig.xml
在我们的配置文件里面配置我们需要的几个表,自动生成所需文件
<table schema="" tableName="t_hibernate_book" domainObjectName="HBook"enableCountByExample="false" enableDeleteByExample="false"enableSelectByExample="false" enableUpdateByExample="false"></table><table schema="" tableName="t_hibernate_book_category" domainObjectName="HBookCategory"enableCountByExample="false" enableDeleteByExample="false"enableSelectByExample="false" enableUpdateByExample="false"></table><table schema="" tableName="t_hibernate_category" domainObjectName="HCategory"enableCountByExample="false" enableDeleteByExample="false"enableSelectByExample="false" enableUpdateByExample="false"></table><table schema="" tableName="t_hibernate_order" domainObjectName="HOrder"enableCountByExample="false" enableDeleteByExample="false"enableSelectByExample="false" enableUpdateByExample="false"></table><table schema="" tableName="t_hibernate_order_item" domainObjectName="HOrderItem"enableCountByExample="false" enableDeleteByExample="false"enableSelectByExample="false" enableUpdateByExample="false"></table>
然后生成我们想要的model和xml映射文件
2、Vo包的编写
当然我们要先建立这个包里面的类才能更好的下一步。
我们现在示例的是一对一的,所以根据前面以此类推我们建立一个HOrderItemVo类
package com.liwen.vo;import com.liwen.model.HOrder; import com.liwen.model.HOrderItem;/*** @软件包名 com.liwen.vo* @用户 liwen* @create 2023-08-26 下午4:37* @注释说明:*/ public class HOrderItemVo extends HOrderItem {private HOrder hOrder;public HOrder gethOrder() {return hOrder;}public void sethOrder(HOrder hOrder) {this.hOrder = hOrder;} }
3、xml的sql编写
在我们的里面添加一个sql的方法编写
<resultMap id="HOrderItemVoMap" type="com.liwen.vo.HOrderItemVo"><result column="order_itemId" property="orderItemId"/><result column="product_id" property="productId"/><result column="quantity" property="quantity"/><result column="oid" property="oid"/><!--association是一对一的关系--><association property="hOrder" javaType="com.liwen.model.HOrder"><result column="order_id" property="orderId"/><result column="order_no" property="orderNo"/></association></resultMap><select id="selectByHOrderId" resultMap="HOrderItemVoMap" parameterType="java.lang.Integer">select *from t_hibernate_order o,t_hibernate_order_item oiwhere o.order_id = oi.oidand oi.order_item_id = #{oiid}</select>
4、编写对应接口及实现类
在上面我们已经写好了sql,我们生成对应的接口及接口实现方法。
在我们生成的HOrderItemMapper 接口里面编写
package com.liwen.mapper;import com.liwen.model.HOrderItem; import com.liwen.vo.HOrderItemVo; import org.apache.ibatis.annotations.Param; import org.springframework.stereotype.Repository;@Repository public interface HOrderItemMapper {int deleteByPrimaryKey(Integer orderItemId);int insert(HOrderItem record);int insertSelective(HOrderItem record);HOrderItem selectByPrimaryKey(Integer orderItemId);int updateByPrimaryKeySelective(HOrderItem record);int updateByPrimaryKey(HOrderItem record);HOrderItemVo selectByHOrderId(@Param("oiid") Integer oiid); }
创建一个biz的包,里面编写一个HOrderItemBiz接口类并且编写接口方法
package com.liwen.biz;import com.liwen.vo.HOrderItemVo;/*** @软件包名 com.liwen.biz* @用户 liwen* @create 2023-08-26 下午4:48* @注释说明:*/ public interface HOrderItemBiz {HOrderItemVo selectByHOrderId(Integer oiid); }
在这个biz里面新建一个impl包,里面创建一个HOrderItemBizImpl 接口实现类,继承HOrderItemBiz
package com.liwen.biz.impl;import com.liwen.biz.HOrderItemBiz; import com.liwen.mapper.HOrderItemMapper; import com.liwen.vo.HOrderItemVo; import org.springframework.beans.factory.annotation.Autowired; import org.springframework.stereotype.Service;/*** @软件包名 com.liwen.biz.impl* @用户 liwen* @create 2023-08-26 下午4:48* @注释说明:*/ @Service public class HOrderItemBizImpl implements HOrderItemBiz {@Autowiredprivate HOrderItemMapper hOrderItemMapper;@Overridepublic HOrderItemVo selectByHOrderId(Integer oiid) {return hOrderItemMapper.selectByHOrderId(oiid);} }
5、测试
package com.liwen.biz.impl;import com.liwen.biz.HOrderItemBiz;
import com.liwen.vo.HOrderItemVo;
import org.junit.Test;
import org.junit.runner.RunWith;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.test.context.ContextConfiguration;
import org.springframework.test.context.junit4.SpringJUnit4ClassRunner;/*** @软件包名 com.liwen.biz.impl* @用户 liwen* @create 2023-08-26 下午4:58* @注释说明:*/
@RunWith(SpringJUnit4ClassRunner.class)
@ContextConfiguration(locations = {"classpath:spring-context.xml"})
public class HOrderItemBizImplTest {@Autowiredprivate HOrderItemBiz hOrderItemBiz;@Testpublic void selectByHOrderId() {HOrderItemVo hOrderItemVo = hOrderItemBiz.selectByHOrderId(27);System.out.println(hOrderItemVo);}
}
三、一对多
1、Vo包类的编写
因为我们是一对多的所以我们再编写vo类的时候,里面是使用list集合
package com.liwen.vo;import com.liwen.model.HOrder;
import com.liwen.model.HOrderItem;import java.util.ArrayList;
import java.util.List;/*** @软件包名 com.liwen.vo* @用户 liwen* @create 2023-08-26 下午3:55* @注释说明:*/
public class HOrderVo extends HOrder {// 一个订单存在多个订单项private List<HOrderItem> hOrderItems = new ArrayList<>();public List<HOrderItem> gethOrderItems() {return hOrderItems;}public void sethOrderItems(List<HOrderItem> hOrderItems) {this.hOrderItems = hOrderItems;}
}
2、xml的sql编写
在原本的基础的sql上我们增加一个一对多的sql
<!-- resultType="com.liwen.vo.HOrderVo" 在多表的字段是无法使用的--><!-- 我们要写一个resultMap映射--><resultMap id="HOrderVoMap" type="com.liwen.vo.HOrderVo"><!-- 每个订单对应的属性,column:数据库属性名;property:实体类属性名 --><result column="order_id" property="orderId"/><result column="order_no" property="orderNo"/><!-- 我们设置hOrderItems数组里面的属性 --><!-- collection是一对多的关系 --><collection property="hOrderItems" ofType="com.liwen.model.HOrderItem"><result column="order_itemId" property="orderItemId"/><result column="product_id" property="productId"/><result column="quantity" property="quantity"/><result column="oid" property="oid"/></collection></resultMap><select id="byOid" resultMap="HOrderVoMap" parameterType="java.lang.Integer">select *from t_hibernate_order o,t_hibernate_order_item oiwhere o.order_id = oi.oidand o.order_id = #{oid}</select>
3、编写对应接口及实现类
根据sql生成的对应的HOrderMapper 类里面生成已经编写好的sql方法
package com.liwen.mapper;import com.liwen.model.HOrder;
import com.liwen.vo.HOrderVo;
import org.apache.ibatis.annotations.Param;
import org.springframework.stereotype.Repository;@Repository
public interface HOrderMapper {int deleteByPrimaryKey(Integer orderId);int insert(HOrder record);int insertSelective(HOrder record);HOrder selectByPrimaryKey(Integer orderId);int updateByPrimaryKeySelective(HOrder record);int updateByPrimaryKey(HOrder record);//============================================HOrderVo byOid(@Param("oid") Integer oid);
}
在biz包里面新建一个接口HOrderBiz
package com.liwen.biz;import com.liwen.vo.HOrderVo;/*** @软件包名 com.liwen.biz* @用户 liwen* @create 2023-08-26 下午4:15* @注释说明:*/
public interface HOrderBiz {HOrderVo byOid(Integer oid);
}
在biz包里面的impl里面新建一个Java类实现HOrderBiz 接口
package com.liwen.biz.impl;import com.liwen.biz.HOrderBiz;
import com.liwen.mapper.HOrderMapper;
import com.liwen.vo.HOrderVo;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Service;/*** @软件包名 com.liwen.biz.impl* @用户 liwen* @create 2023-08-26 下午4:16* @注释说明:*/
@Service
public class HOrderBizImpl implements HOrderBiz {@Autowiredprivate HOrderMapper hOrderMapper;@Overridepublic HOrderVo byOid(Integer oid) {return hOrderMapper.byOid(oid);}
}
4、测试
package com.liwen.biz.impl;import com.liwen.biz.HOrderBiz;
import com.liwen.vo.HOrderVo;
import org.junit.After;
import org.junit.Before;
import org.junit.Test;
import org.junit.runner.RunWith;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.test.context.ContextConfiguration;
import org.springframework.test.context.junit4.SpringJUnit4ClassRunner;import static org.junit.Assert.*;/*** @软件包名 com.liwen.biz.impl* @用户 liwen* @create 2023-08-26 下午4:22* @注释说明:*/
@RunWith(SpringJUnit4ClassRunner.class)
@ContextConfiguration(locations = {"classpath:spring-context.xml"})
public class HOrderBizImplTest {@Autowiredprivate HOrderBiz hOrderBiz;@Testpublic void byOid() {HOrderVo hOrderVo = hOrderBiz.byOid(7);System.out.println(hOrderVo);}}
四、多对多
1、Vo类
package com.liwen.vo;import com.liwen.model.HBook;
import com.liwen.model.HCategory;import java.util.List;/*** @软件包名 com.liwen.vo* @用户 liwen* @create 2023-08-27 下午10:29* @注释说明:*/
public class HBookVo extends HBook {private List<HCategory> hCategoryList;public List<HCategory> gethCategoryList() {return hCategoryList;}public void sethCategoryList(List<HCategory> hCategoryList) {this.hCategoryList = hCategoryList;}
}
package com.liwen.vo;import com.liwen.model.HBook;
import com.liwen.model.HCategory;import java.util.ArrayList;
import java.util.List;/*** @软件包名 com.liwen.vo* @用户 liwen* @create 2023-08-27 下午11:03* @注释说明:*/
public class HCategoryVo extends HCategory {private List<HBook> hBooks = new ArrayList<>();public List<HBook> gethBooks() {return hBooks;}public void sethBooks(List<HBook> hBooks) {this.hBooks = hBooks;}
}
2、xml的sql配置
分别在不同的xml配置文件里面配置
<resultMap id="HBookVo" type="com.liwen.vo.HBookVo"><result column="book_id" property="bookId"/><result column="book_name" property="bookName"/><result column="price" property="price"/><collection property="hCategoryList" ofType="com.liwen.model.HCategory"><result column="category_id" property="categoryId"/><result column="category_name" property="categoryName"/></collection></resultMap><!-- 根据书籍id查询出书籍信息及所属类别--><select id="selectByBId" resultMap="HBookVo" parameterType="java.lang.Integer">select *from t_hibernate_book b,t_hibernate_book_category bc,t_hibernate_category cwhere b.book_id = bc.bidand bc.cid = c.category_idand b.book_id = #{bid}</select>
<resultMap id="HCategoryVo" type="com.liwen.vo.HCategoryVo"><result column="category_id" property="categoryId"/><result column="category_name" property="categoryName"/><collection property="hBooks" ofType="com.liwen.model.HBook"><result column="book_id" property="bookId"/><result column="book_name" property="bookName"/><result column="price" property="price"/></collection></resultMap><select id="selectByCId" resultMap="HCategoryVo" parameterType="java.lang.Integer">select *from t_hibernate_book b,t_hibernate_book_category bc,t_hibernate_category cwhere b.book_id = bc.bidand bc.cid = c.category_idand c.category_id = #{cid}</select>
3、接口及接口实现类
在生成的接口类里面编写对应的接口方法
package com.liwen.mapper;import com.liwen.model.HBook;
import com.liwen.vo.HBookVo;
import org.apache.ibatis.annotations.Param;public interface HBookMapper {int deleteByPrimaryKey(Integer bookId);int insert(HBook record);int insertSelective(HBook record);HBook selectByPrimaryKey(Integer bookId);int updateByPrimaryKeySelective(HBook record);int updateByPrimaryKey(HBook record);HBookVo selectByBId(@Param("bid") Integer bid);
}
package com.liwen.mapper;import com.liwen.model.HCategory;
import com.liwen.vo.HCategoryVo;
import org.apache.ibatis.annotations.Param;
import org.springframework.stereotype.Repository;@Repository
public interface HCategoryMapper {int deleteByPrimaryKey(Integer categoryId);int insert(HCategory record);int insertSelective(HCategory record);HCategory selectByPrimaryKey(Integer categoryId);int updateByPrimaryKeySelective(HCategory record);int updateByPrimaryKey(HCategory record);HCategoryVo selectByCId(@Param("cid") Integer cid);
}
在biz包里面新建一个HBookBiz接口类
package com.liwen.biz;import com.liwen.vo.HBookVo;/*** @软件包名 com.liwen.biz* @用户 liwen* @create 2023-08-27 下午10:50* @注释说明:*/
public interface HBookBiz {HBookVo selectByBId(Integer bid);
}
package com.liwen.biz;import com.liwen.vo.HCategoryVo;public interface HCategoryBiz {HCategoryVo selectByCId(Integer cid);
}
在Biz里面的impl包里面新
package com.liwen.biz.impl;import com.liwen.biz.HBookBiz;
import com.liwen.mapper.HBookMapper;
import com.liwen.vo.HBookVo;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Service;/*** @软件包名 com.liwen.biz.impl* @用户 liwen* @create 2023-08-27 下午10:53* @注释说明:*/
@Service
public class HBookBizImpl implements HBookBiz {@Autowiredprivate HBookMapper hBookMapper;@Overridepublic HBookVo selectByBId(Integer bid) {return hBookMapper.selectByBId(bid);}
}
建HBookBizImpl 接口实现HBookBiz接口类
package com.liwen.biz.impl;import com.liwen.biz.HBookBiz;
import com.liwen.mapper.HBookMapper;
import com.liwen.vo.HBookVo;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Service;/*** @软件包名 com.liwen.biz.impl* @用户 liwen* @create 2023-08-27 下午10:53* @注释说明:*/
@Service
public class HBookBizImpl implements HBookBiz {@Autowiredprivate HBookMapper hBookMapper;@Overridepublic HBookVo selectByBId(Integer bid) {return hBookMapper.selectByBId(bid);}
}
package com.liwen.biz.impl;import com.liwen.biz.HCategoryBiz;
import com.liwen.mapper.HCategoryMapper;
import com.liwen.vo.HCategoryVo;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Service;/*** @软件包名 com.liwen.biz.impl* @用户 liwen* @create 2023-08-27 下午11:12* @注释说明:*/
@Service
public class HCategoryBizImpl implements HCategoryBiz {@Autowiredprivate HCategoryMapper hCategoryMapper;@Overridepublic HCategoryVo selectByCId(Integer cid) {return hCategoryMapper.selectByCId(cid);}
}
4、测试
package com.liwen.biz.impl;import com.liwen.biz.HBookBiz;
import com.liwen.vo.HBookVo;
import org.junit.Test;
import org.junit.runner.RunWith;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.test.context.ContextConfiguration;
import org.springframework.test.context.junit4.SpringJUnit4ClassRunner;import static org.junit.Assert.*;/*** @软件包名 com.liwen.biz.impl* @用户 liwen* @create 2023-08-27 下午10:59* @注释说明:*/
@RunWith(SpringJUnit4ClassRunner.class)
@ContextConfiguration(locations = {"classpath:spring-context.xml"})
public class HBookBizImplTest {@Autowiredprivate HBookBiz hBookBiz;@Testpublic void selectByBId() {HBookVo hBookVo = this.hBookBiz.selectByBId(8);System.out.println(hBookVo);}
}
package com.liwen.biz.impl;import com.liwen.biz.HCategoryBiz;
import com.liwen.vo.HCategoryVo;
import org.junit.Test;
import org.junit.runner.RunWith;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.test.context.ContextConfiguration;
import org.springframework.test.context.junit4.SpringJUnit4ClassRunner;import static org.junit.Assert.*;/*** @软件包名 com.liwen.biz.impl* @用户 liwen* @create 2023-08-27 下午11:14* @注释说明:*/
@RunWith(SpringJUnit4ClassRunner.class)
@ContextConfiguration(locations = {"classpath:spring-context.xml"})
public class HCategoryBizImplTest {@Autowiredprivate HCategoryBiz hCategoryBiz;@Testpublic void selectByCId(){HCategoryVo hCategoryVo = hCategoryBiz.selectByCId(8);System.out.println(hCategoryVo);hCategoryVo.gethBooks().forEach(System.out::println);}}
相关文章:
Mybatis的三种映射关系以及联表查询
目录 一、概念 二、一对一 1、配置generatorConfig.xml 2、Vo包的编写 3、xml的sql编写 4、编写对应接口及实现类 5、测试 三、一对多 1、Vo包类的编写 2、xml的sql编写 3、编写对应接口及实现类 4、测试 四、多对多 1、Vo类 2、xml的sql配置 3、接口及接口实现…...
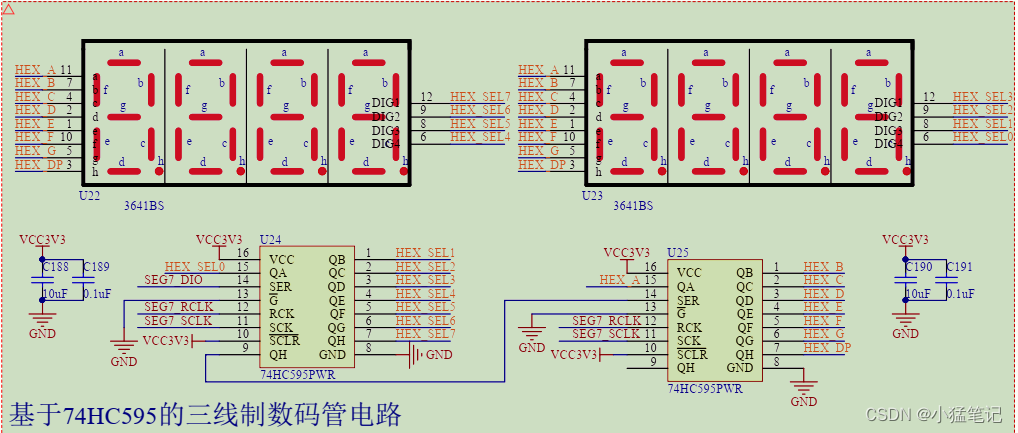
基于串口校时的数字钟设计
文章目录 设计目标硬件设计数码管串口 软件设计顶层模块串口接收模块数据处理模块时钟模块串口发送模块 总结 设计目标 环境:ACX720开发板 实现功能: 数码管能够显示时分秒能够接收串口数据修改时间能够将当前时间以1s一次速率发送到电脑 硬件设计 数…...
支持向量机(二)
文章目录 前言具体内容 前言 总算要对稍微有点难度的地方动手了,前面介绍的线性可分或者线性不可分的情况,都是使用平面作为分割面的,现在我们采用另一种分割面的设计方法,也就是核方法。 核方法涉及的分割面不再是 w x b 0 wx…...
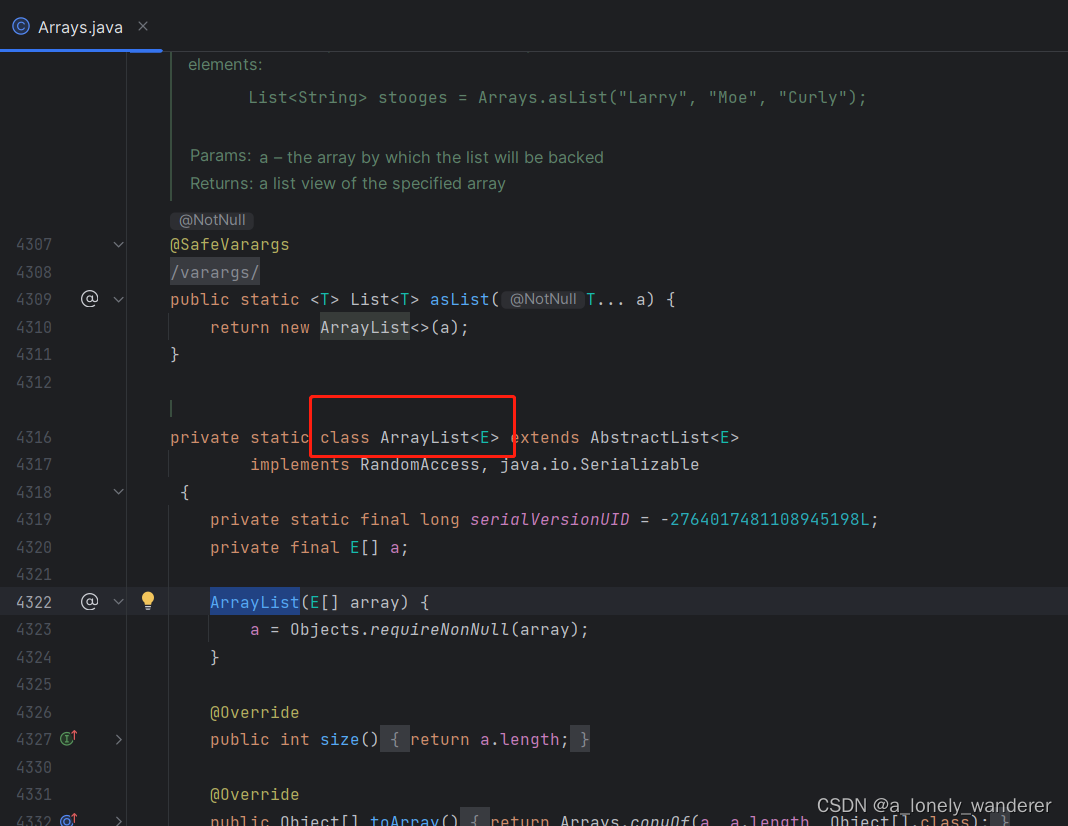
Arrays.asList 和 null 类型
一、Arrays.asList 类型简析 Arrays.asList() 返回的List 是它的内部类,不能使用 retainAll() 取交集,导致元素的删除,会报错。 List<String> list Arrays.asList(value.split(",")); 替换为> List<String> list…...
《论文阅读》用提示和释义模拟对话情绪识别的思维过程 IJCAI 2023
《论文阅读》用提示和复述模拟对话情绪识别的思维过程 IJCAI 2023 前言简介相关知识prompt engineeringparaphrasing模型架构第一阶段第二阶段History-oriented promptExperience-oriented Prompt ConstructionLabel Paraphrasing损失函数前言 你是否也对于理解论文存在困惑?…...
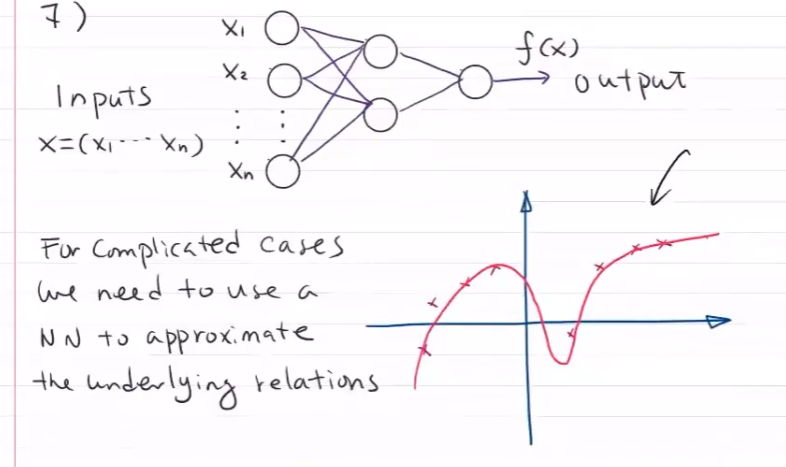
【AI】机器学习——绪论
文章目录 1.1 机器学习概念1.1.1 定义统计机器学习与数据挖掘区别机器学习前提 1.1.2 术语1.1.3 特点以数据为研究对象目标方法——基于数据构建模型SML三要素SML步骤 1.2 分类1.2.1 参数化/非参数化方法1.2.2 按算法分类1.2.3 按模型分类概率模型非概率模型逻辑斯蒂回归 1.2.4…...
linux 查看端口占用
查看端口占用 使用lsof 可以使用lsof -i:端口号 来查看端口占用情况 lsof -i:8010COMMAND PID USER FD TYPE DEVICE SIZE/OFF NODE NAMEnginx 35653 zhanghe 10u IPv4 0xcac2e413ddf9c5b9 0t0 TCP *:8010 (LISTEN)nginx 35654 zhanghe 10u…...
modernC++手撸任意层神经网络22前向传播反向传播梯度下降等23代码补全的例子0901b
以下神经网络代码,请添加输入:{{1,0},{1,1}},输出{1,0};添加反向传播,梯度下降等训练! 以下神经网络代码,请添加输入:{{1,0},{1,1}},输出{1,0};添加反向传播,梯度下降等训练! #include <iostream> #include<vector> #include<Eigen/Dense> #include<rando…...
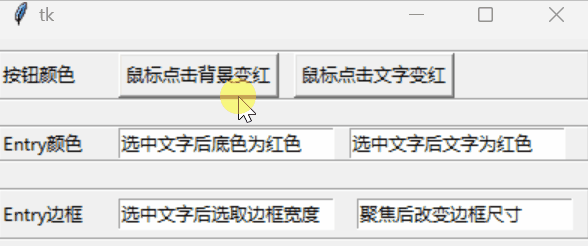
tkinter控件样式
文章目录 以按钮为例共有参数动态属性 tkinter系列: GUI初步💎布局💎绑定变量💎绑定事件💎消息框💎文件对话框💎控件样式扫雷小游戏💎强行表白神器 以按钮为例 tkinter对控件的诸…...
【linux命令讲解大全】042. 深入了解 which 命令:查找和显示命令的绝对路径
文章目录 which补充说明语法选项参数实例 从零学 python which 查找并显示给定命令的绝对路径 补充说明 which 命令用于查找并显示给定命令的绝对路径,环境变量 PATH 中保存了查找命令时需要遍历的目录。which 指令会在环境变量 $PATH 设置的目录里查找符合条件的…...
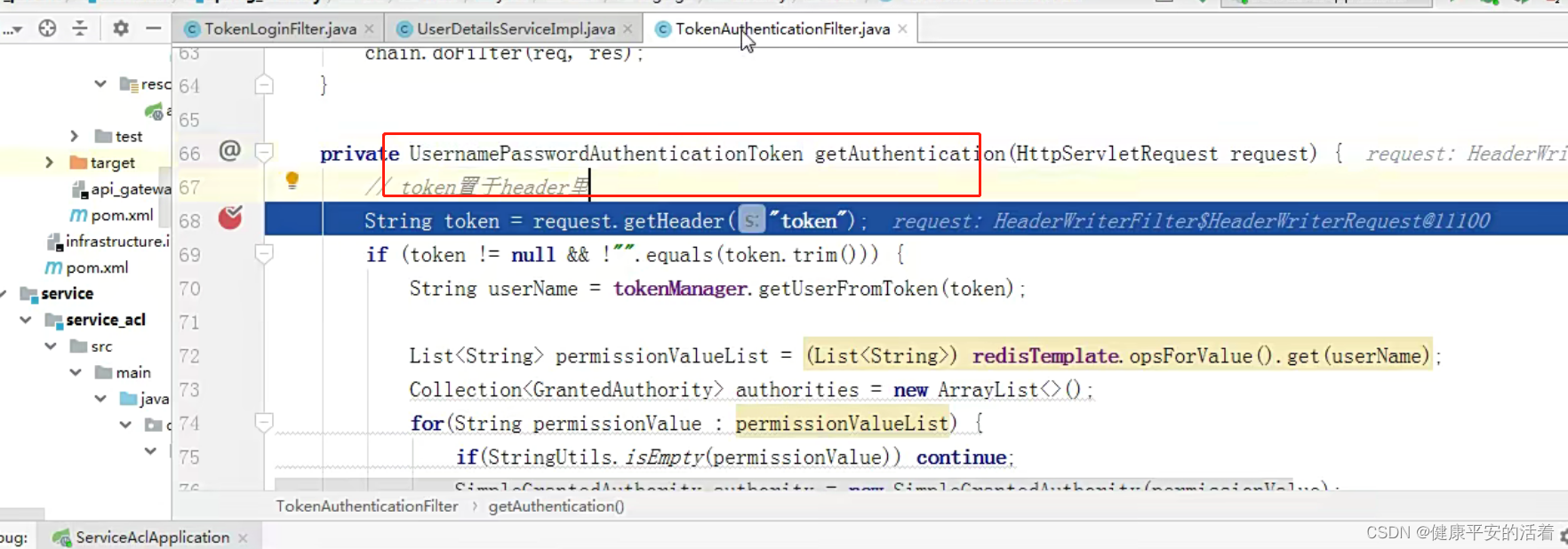
实战项目 在线学院之集成springsecurity的配置以及执行流程
一 后端操作配置 1.0 工程结构 1.1 在common下创建spring_security模块 1.2 pom文件中依赖的注入 1.3 在service_acl模块服务中引入spring-security权限认证模块 1.3.1 service_acl引入spring-security 1.3.2 在service_acl编写查询数据库信息 定义userDetailServiceImpl 查…...
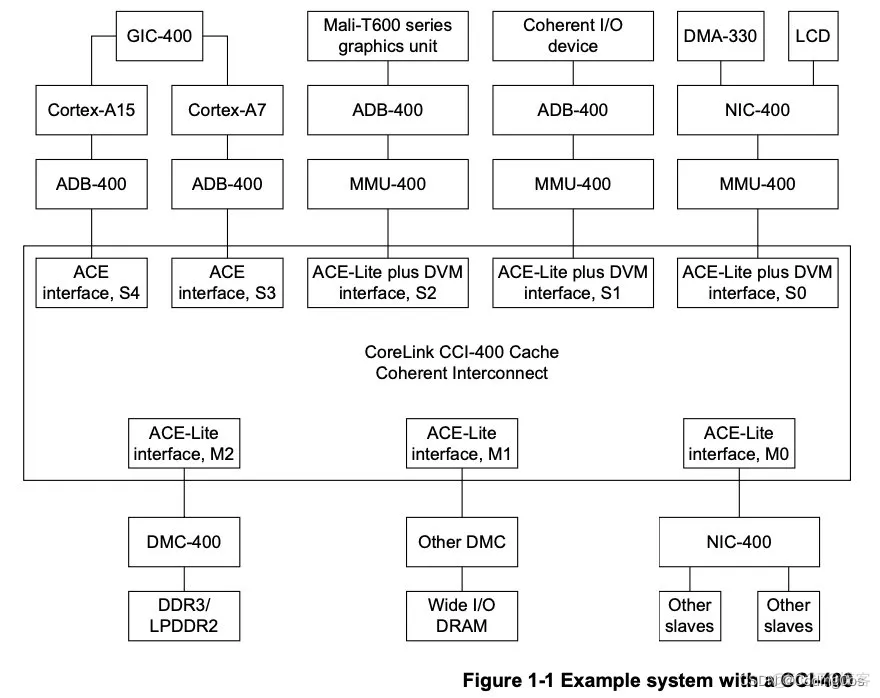
【ARM CoreLink CCI-400 控制器简介】
文章目录 CCI-400 介绍 CCI-400 介绍 CCI(Cache Coherent Interconnect)是ARM 中 的Cache一致性控制器。 CCI-400 将 Interconnect 和coherency 功能结合到一个模块中。它支持多达两个ACE master 点的interface,例如: Cortex-A…...
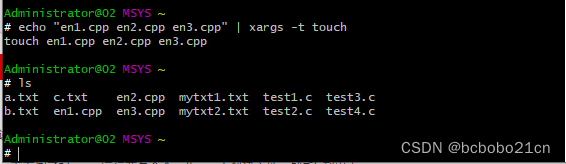
Linux xargs命令继续学习
之前学习过Linux xargs,对此非常的不熟悉,下面继续学习一下; xargs 可以将管道或标准输入(stdin)数据转换成命令行参数,也能够从文件的输出中读取数据; xargs也可以给命令传递参数;…...
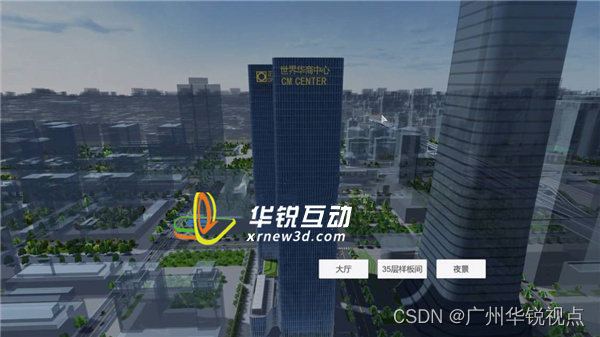
【广州华锐互动】数字孪生智慧楼宇3D可视化系统:掌握实时运行状态,优化运营管理
在过去的几年中,科技的发展极大地改变了我们的生活和工作方式。其中,三维数据可视化技术的出现,为我们提供了全新的理解和观察世界的方式。特别是在建筑行业,数字孪生智慧楼宇3D可视化系统的出现,让我们有机会重新定义…...
20230904工作心得:集合应该如何优雅判空?
1 集合判空 List<String> newlist null;//空指针if( !newlist.isEmpty()){newlist.forEach(System.out::println);}//空指针if(newlist.size()>0 && newlist!null){newlist.forEach(System.out::println);}//可行if(newlist!null && newlist.size()&…...
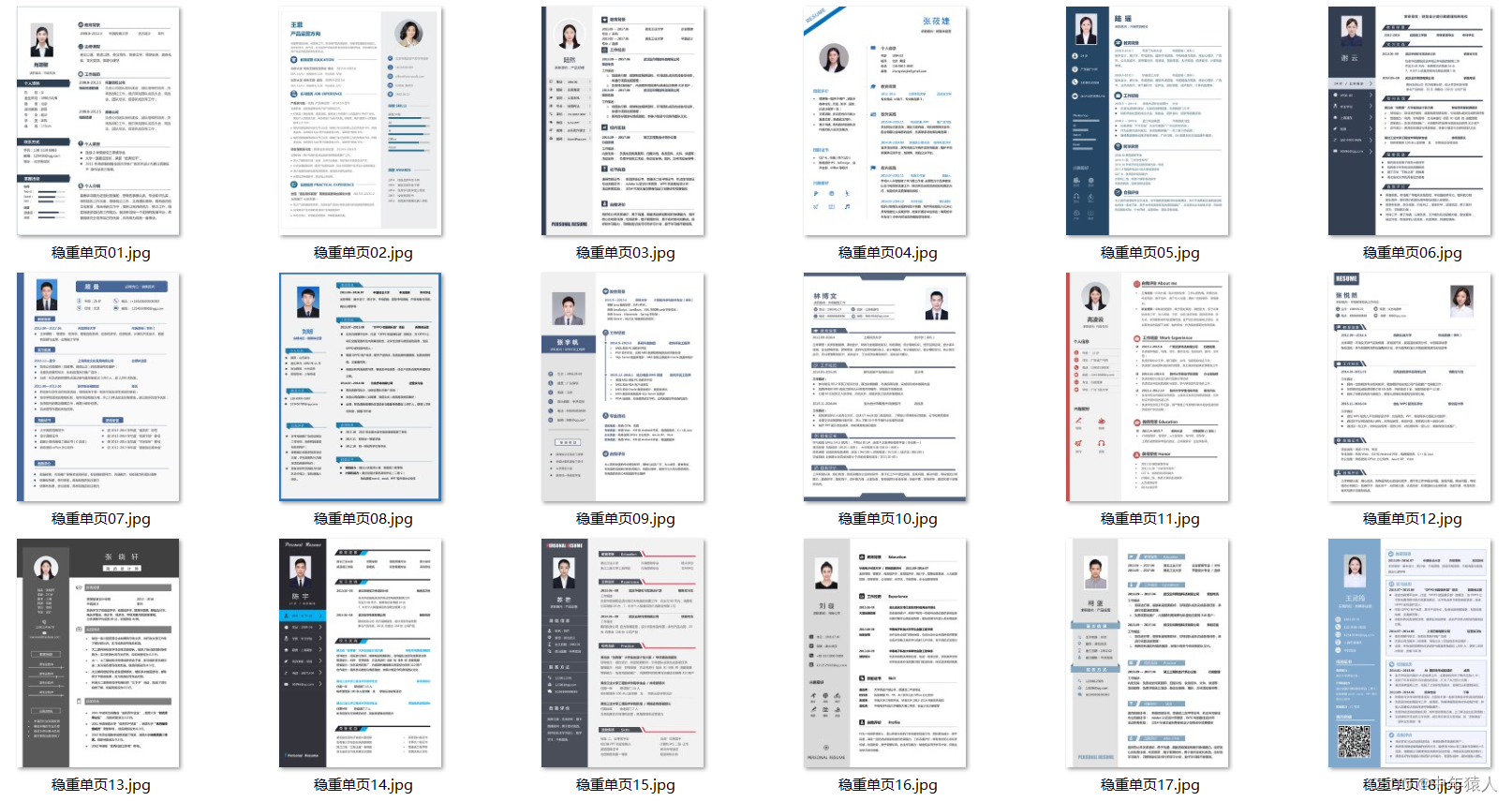
使用Python进行健身手表数据分析
健身手表(Fitness Watch)数据分析涉及分析健身可穿戴设备或智能手表收集的数据,以深入了解用户的健康和活动模式。这些设备可以跟踪所走的步数、消耗的能量、步行速度等指标。本文将带您完成使用Python进行Fitness Watch数据分析的任务。 Fitness Watch数据分析是健…...
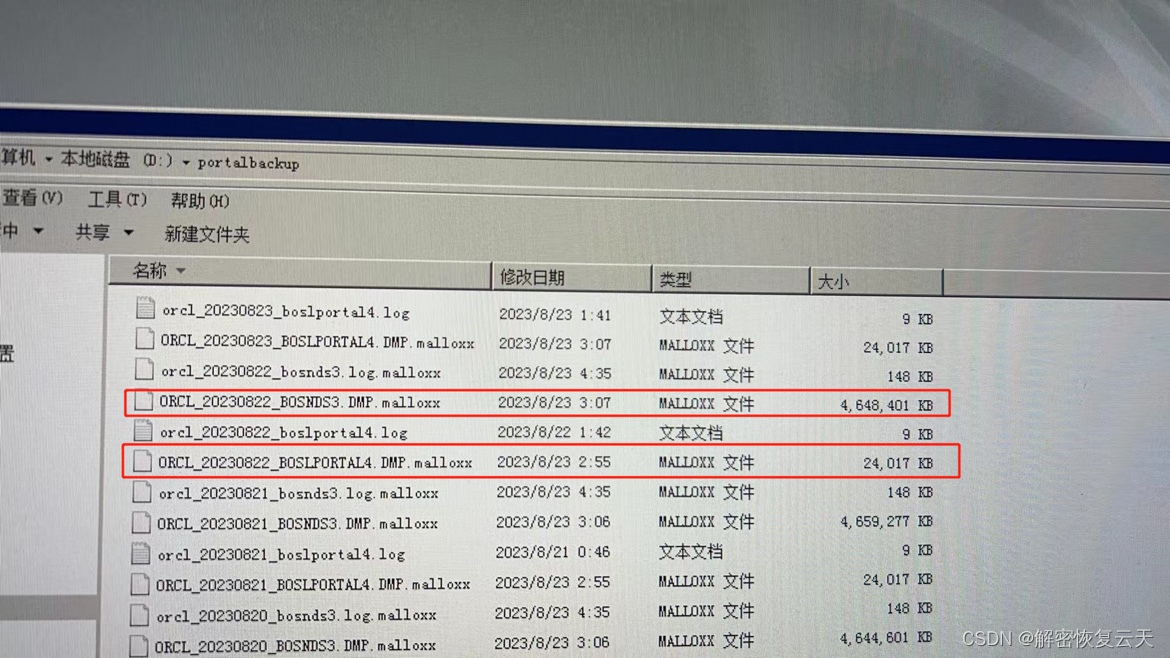
什么是malloxx勒索病毒,服务器中malloxx勒索病毒了怎么办?
Malloxx勒索病毒是一种新型的电脑病毒,它通过加密用户电脑中的重要文件数据来威胁用户,并以此勒索钱财。这种病毒并不是让用户的电脑瘫痪,而是以非常独特的方式进行攻击。在感染了Malloxx勒索病毒后,它会加密用户服务器中的数据&a…...
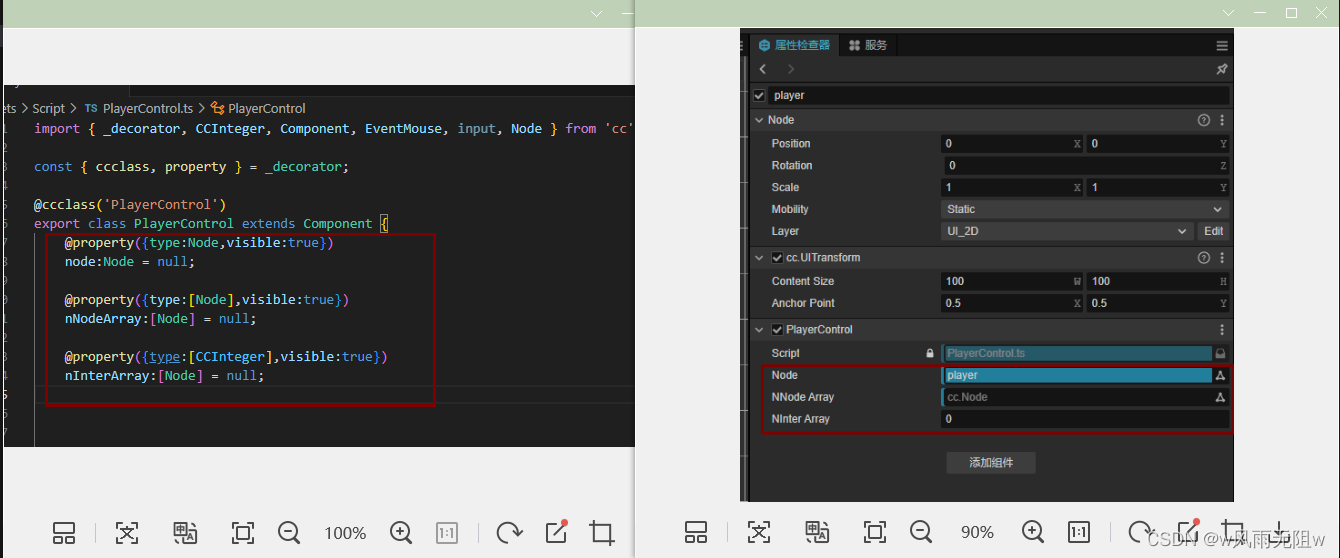
CocosCreator3.8研究笔记(六)CocosCreator 脚本装饰器的理解
一、什么是装饰器? 装饰器是TypeScript脚本语言中的概念。 TypeScript的解释:在一些场景下,我们需要额外的特性来支持标注或修改类及其成员。装饰器(Decorators)为我们在类的声明及成员上通过元编程语法添加标注提供了…...
docker login harbor http login登录
前言 搭建的 harbor 仓库为 http 协议,在本地登录时出现如下报错: docker login http://192.168.xx.xx Username: admin Password: Error response from daemon: Get "https://192.168.xx.xx/v2/": dialing 192.168.xx.xx:443 matches static …...
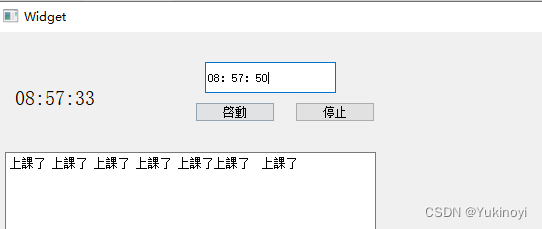
day5 qt
#include "widget.h" #include "ui_widget.h"Widget::Widget(QWidget *parent): QWidget(parent), ui(new Ui::Widget) {ui->setupUi(this);timer_idthis->startTimer(100);//啓動一個定時器 每100ms發送一次信號ui->Edit1->setPlaceholderTex…...
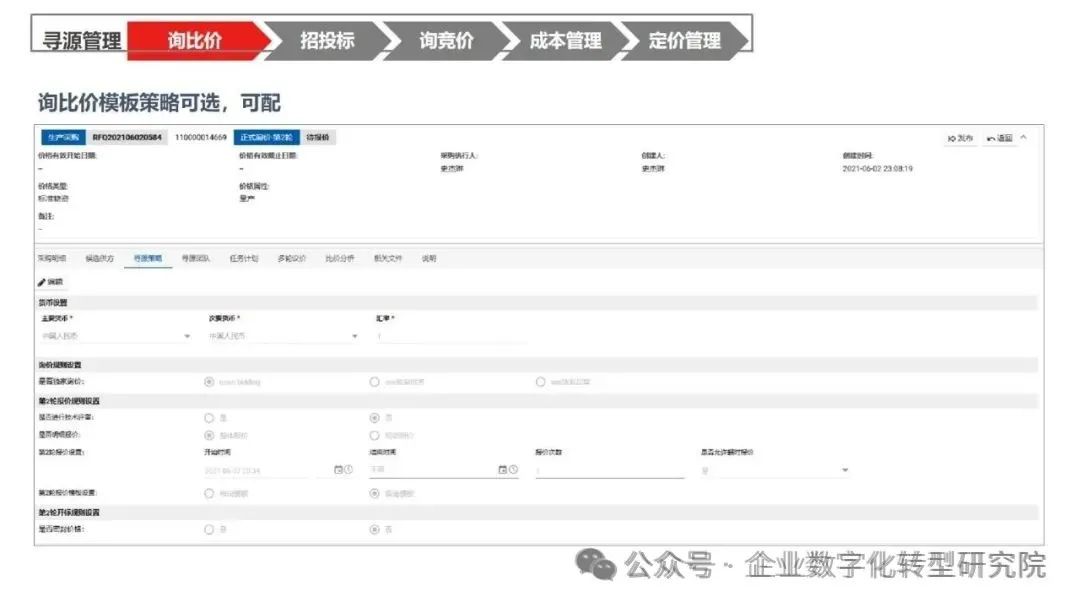
PPT|230页| 制造集团企业供应链端到端的数字化解决方案:从需求到结算的全链路业务闭环构建
制造业采购供应链管理是企业运营的核心环节,供应链协同管理在供应链上下游企业之间建立紧密的合作关系,通过信息共享、资源整合、业务协同等方式,实现供应链的全面管理和优化,提高供应链的效率和透明度,降低供应链的成…...
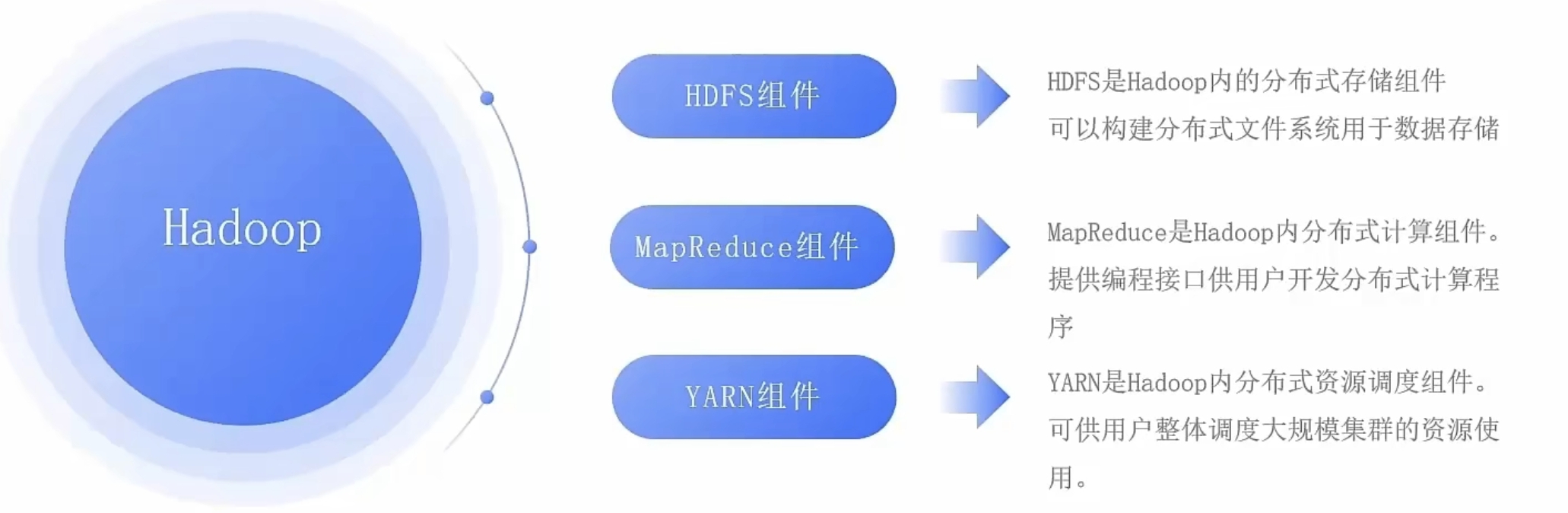
大数据零基础学习day1之环境准备和大数据初步理解
学习大数据会使用到多台Linux服务器。 一、环境准备 1、VMware 基于VMware构建Linux虚拟机 是大数据从业者或者IT从业者的必备技能之一也是成本低廉的方案 所以VMware虚拟机方案是必须要学习的。 (1)设置网关 打开VMware虚拟机,点击编辑…...
生成 Git SSH 证书
🔑 1. 生成 SSH 密钥对 在终端(Windows 使用 Git Bash,Mac/Linux 使用 Terminal)执行命令: ssh-keygen -t rsa -b 4096 -C "your_emailexample.com" 参数说明: -t rsa&#x…...
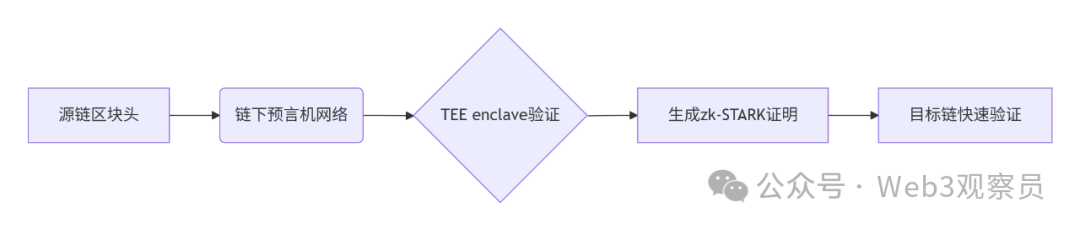
跨链模式:多链互操作架构与性能扩展方案
跨链模式:多链互操作架构与性能扩展方案 ——构建下一代区块链互联网的技术基石 一、跨链架构的核心范式演进 1. 分层协议栈:模块化解耦设计 现代跨链系统采用分层协议栈实现灵活扩展(H2Cross架构): 适配层…...
sqlserver 根据指定字符 解析拼接字符串
DECLARE LotNo NVARCHAR(50)A,B,C DECLARE xml XML ( SELECT <x> REPLACE(LotNo, ,, </x><x>) </x> ) DECLARE ErrorCode NVARCHAR(50) -- 提取 XML 中的值 SELECT value x.value(., VARCHAR(MAX))…...
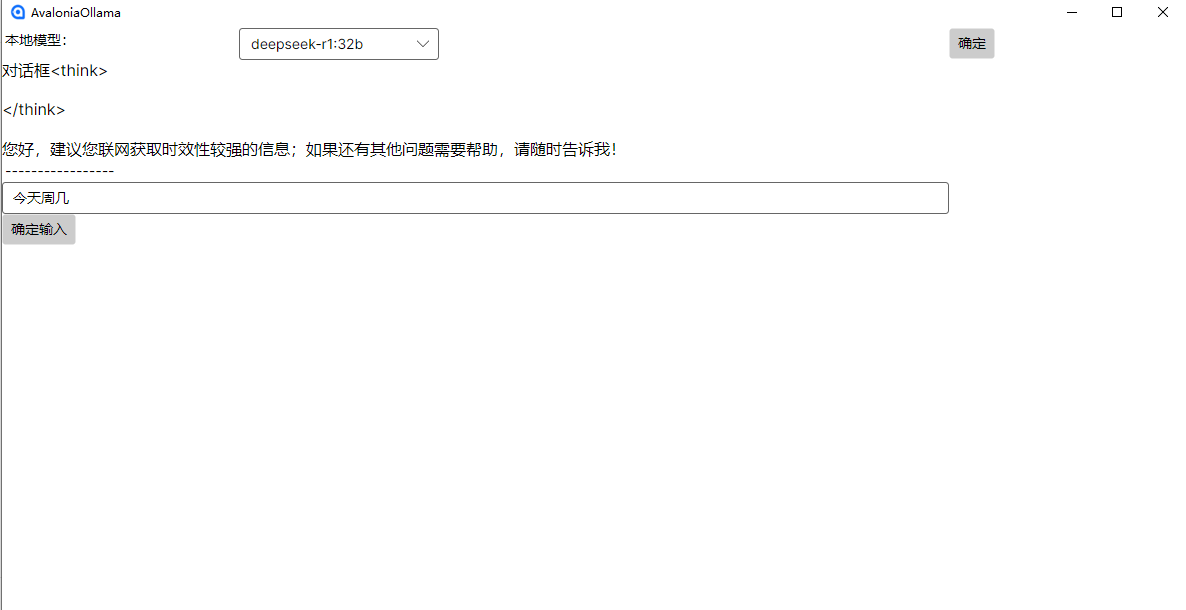
c#开发AI模型对话
AI模型 前面已经介绍了一般AI模型本地部署,直接调用现成的模型数据。这里主要讲述讲接口集成到我们自己的程序中使用方式。 微软提供了ML.NET来开发和使用AI模型,但是目前国内可能使用不多,至少实践例子很少看见。开发训练模型就不介绍了&am…...
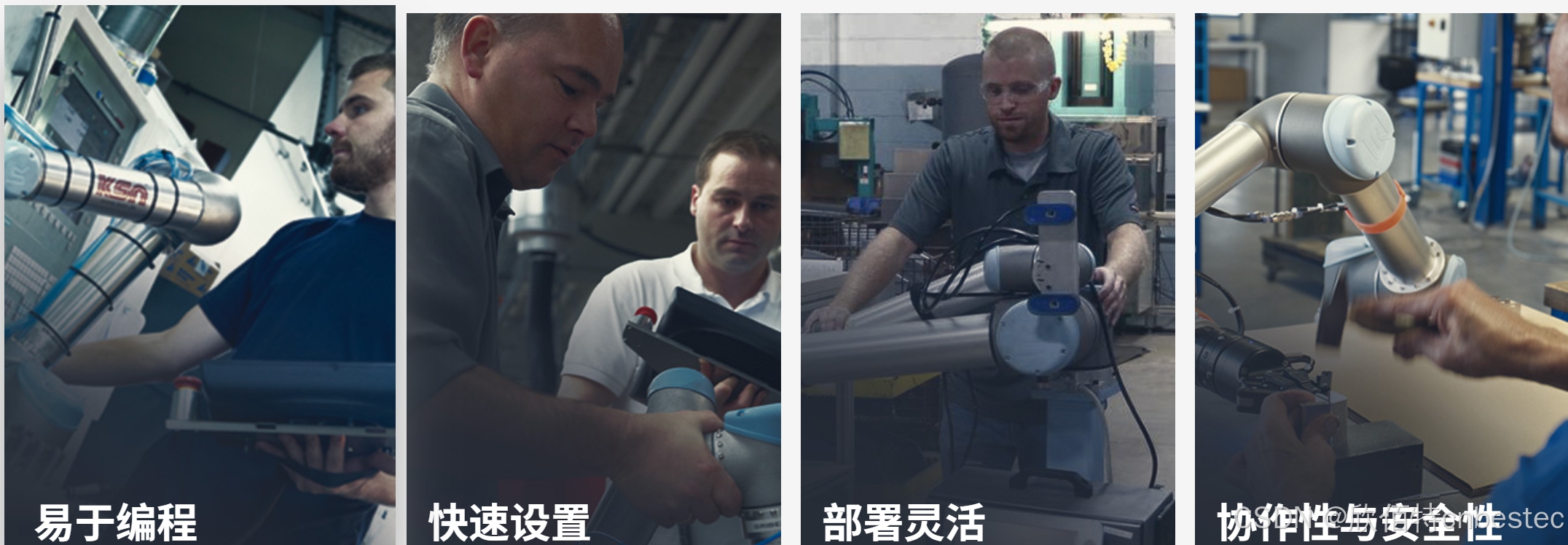
UR 协作机器人「三剑客」:精密轻量担当(UR7e)、全能协作主力(UR12e)、重型任务专家(UR15)
UR协作机器人正以其卓越性能在现代制造业自动化中扮演重要角色。UR7e、UR12e和UR15通过创新技术和精准设计满足了不同行业的多样化需求。其中,UR15以其速度、精度及人工智能准备能力成为自动化领域的重要突破。UR7e和UR12e则在负载规格和市场定位上不断优化…...
[Java恶补day16] 238.除自身以外数组的乘积
给你一个整数数组 nums,返回 数组 answer ,其中 answer[i] 等于 nums 中除 nums[i] 之外其余各元素的乘积 。 题目数据 保证 数组 nums之中任意元素的全部前缀元素和后缀的乘积都在 32 位 整数范围内。 请 不要使用除法,且在 O(n) 时间复杂度…...
今日学习:Spring线程池|并发修改异常|链路丢失|登录续期|VIP过期策略|数值类缓存
文章目录 优雅版线程池ThreadPoolTaskExecutor和ThreadPoolTaskExecutor的装饰器并发修改异常并发修改异常简介实现机制设计原因及意义 使用线程池造成的链路丢失问题线程池导致的链路丢失问题发生原因 常见解决方法更好的解决方法设计精妙之处 登录续期登录续期常见实现方式特…...

Reasoning over Uncertain Text by Generative Large Language Models
https://ojs.aaai.org/index.php/AAAI/article/view/34674/36829https://ojs.aaai.org/index.php/AAAI/article/view/34674/36829 1. 概述 文本中的不确定性在许多语境中传达,从日常对话到特定领域的文档(例如医学文档)(Heritage 2013;Landmark、Gulbrandsen 和 Svenevei…...