一个通用的EXCEL生成下载方法
Excel是一个Java开发中必须会用到的东西,之前博主也发过一篇关于使用Excel的文章,但是最近工作中,发现了一个更好的使用方法,所以,就对之前的博客进行总结,然后就有了这篇新的,万能通用的方法说明书了
好了,闲话不多说,直接上干货了
控制器代码如下
@Operation(summary = "Excel导入", description = "Excel导入")@GetMapping("/commPointManagement/importExcel")public ResultBody importExcel(@RequestParam MultipartFile excel,@RequestParam(value = "creatorId") Long creatorId,HttpServletResponse response){try {return commPointManagementService.importExcel(excel,creatorId,response);} catch (Exception e) {return ResultBody.failed("导入失败"+e.getMessage());}}/*** excel导出* @param dto*/@Operation(summary = "Excel导出", description = "Excel导出")@PostMapping("/commPointManagement/downloadInfoExcel")public void downloadInfoExcel(@RequestBody CommPointManagementDTO dto, HttpServletResponse response){commPointManagementService.downloadInfoExcel(dto,response);}/*** 下载模板*/@GetMapping("/commPointManagement/downloadExcelTemplate")@Operation(summary = "下载模板excel模板", description = "下载模板excel模板")public void exportExcelFormat(HttpServletResponse response) {commPointManagementService.exportExcelFormat(response);}
上面总结了三个方法,都是不使用模板的,代码也有注释,大家可根据注释获取自己需要的方法
service接口就不粘贴了,直接上实现类了
public void exportExcelFormat(HttpServletResponse response) {List<List<String>> customTitle = new ArrayList<>();List<String> titles = Lists.newArrayList();titles.add("字段1");titles.add("字段2");titles.add("字段3");customTitle.add(titles);try {EasyPoiUtil.exportExcel("模板名称.xlsx", null, null, Lists.newArrayList(), CommPointManagementVO.class, customTitle, Lists.newArrayList(), response);} catch (Exception e) {throw new RuntimeException(e);}}@Overridepublic ResultBody importExcel(MultipartFile excel,Long creatorId, HttpServletResponse response) throws Exception {//获取Excel中的数据集合ExcelImportResult<CommPointManagementBean> importExcel = EasyPoiUtil.importExcel(excel, 1, 0, 0, false, CommPointManagementBean.class);List<CommPointManagementBean> importList = importExcel.getList();int failCount = 0;int insertCount = 0;if (CollUtil.isEmpty(importList)) {return ResultBody.ok().msg("导入excel成功");}Timestamp time = new Timestamp(new Date().getTime());for (int i = 0; i < importList.size(); i++) {CommPointManagementBean date = importList.get(i);}return ResultBody.ok().data().msg("导入excel成功");}@Overridepublic void downloadInfoExcel(CommPointManagementDTO dto, HttpServletResponse response) {//获取数据库数据IPage<CommPointManagementVO> page = commPointManagementMapper.findPage(new PageParams(dto.getPage(), dto.getLimit()), dto);List<CommPointManagementVO> records = page.getRecords();List<List<String>> customTitle = new ArrayList<>();List<String> titleList = new ArrayList<>();titleList.add("字段1");titleList.add("字段2");titleList.add("字段3");customTitle.add(titleList);try {EasyPoiUtil.exportExcel("表名.xlsx", "表头", "Excel中的页名", records,CommPointManagementVO.class, customTitle, new ArrayList<>(), response);} catch (Exception e) {throw new RuntimeException(e);}}
上面方法中的CommPointManagementVO.class是接口返回的实体类,虽然没有注解,但是细心一点看还是能看出来的,里面还有一个工具类EasyPoiUtil,这个就是咱们今天的主角了,主角介绍如下:
package com.secondcloud.system.server.util;import cn.afterturn.easypoi.excel.ExcelExportUtil;
import cn.afterturn.easypoi.excel.ExcelImportUtil;
import cn.afterturn.easypoi.excel.annotation.ExcelEntity;
import cn.afterturn.easypoi.excel.annotation.ExcelTarget;
import cn.afterturn.easypoi.excel.entity.ExportParams;
import cn.afterturn.easypoi.excel.entity.ImportParams;
import cn.afterturn.easypoi.excel.entity.enmus.ExcelType;
import cn.afterturn.easypoi.excel.entity.params.ExcelExportEntity;
import cn.afterturn.easypoi.excel.entity.result.ExcelImportResult;
import cn.afterturn.easypoi.excel.export.ExcelExportService;
import cn.afterturn.easypoi.util.PoiPublicUtil;
import cn.hutool.core.collection.CollectionUtil;
import cn.hutool.core.convert.Convert;
import cn.hutool.core.util.StrUtil;
import org.apache.poi.ss.usermodel.*;
import org.apache.poi.ss.util.CellRangeAddress;
import org.springframework.web.multipart.MultipartFile;import javax.servlet.http.HttpServletResponse;
import java.io.File;
import java.io.OutputStream;
import java.lang.reflect.Field;
import java.net.URLEncoder;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;/*** easyPoi excel通用导入导出*/
public class EasyPoiUtil {private static final String SUFFIX = ".xlsx";/*** exportExcel 通用导出方法(bean加注解)** @param fileName 文件名* @param title 标题* @param sheetName 表单名* @param list 数据* @param pojoClass 数据类* @param customTitle 自定义表头* @param mergeList 自定义合并* @param response 响应* @throws Exception*/public static void exportExcel(String fileName, String title, String sheetName, List<?> list, Class<?> pojoClass,List<List<String>> customTitle, List<CellRangeAddress> mergeList, HttpServletResponse response)throws Exception {defaultExport(list, pojoClass, fileName, response, simpleParams(title, sheetName, fileName), customTitle,mergeList, null);}/*** exportExcelEntity 通用导出方法(自定义ExcelExportEntity)** @param fileName 文件名* @param title 标题* @param sheetName 表单名* @param list 数据* @param entityList 数据配置* @param customTitle 自定义表头* @param mergeList 自定义合并* @param response 响应* @throws Exception*/public static void exportExcelEntity(String fileName, String title, String sheetName, List<?> list, List<ExcelExportEntity> entityList,List<List<String>> customTitle, List<CellRangeAddress> mergeList, HttpServletResponse response)throws Exception {defaultExport(list, null, fileName, response, simpleParams(title, sheetName, fileName), customTitle,mergeList, entityList);}/*** exportExcel 多表单导出方法(bean加注解)** @param fileName 文件名* @param configList title:标题 data:数据 entity:数据类* @param response 响应* @throws Exception*/public static void exportExcelMultiSheet(String fileName, List<Map<String, Object>> configList,HttpServletResponse response) throws Exception {// 根据fileName后缀确定excel文件是xls还是xlsxExcelType type = ExcelType.HSSF;if (StrUtil.endWith(fileName, SUFFIX)) {type = ExcelType.XSSF;}Workbook workbook = ExcelExportUtil.exportExcel(configList, type);if (workbook != null) {try {if (CollectionUtil.isNotEmpty(configList)) {int sheetIndex = 0;for (Map<String, Object> map : configList) {List<List<String>> customTitle = (List<List<String>>) map.get("customTitle");List<CellRangeAddress> mergeList = (List<CellRangeAddress>) map.get("mergeList");String sheetTitle = Convert.toStr(map.get("sheetTitle"));Sheet sheet = workbook.getSheetAt(sheetIndex);if (sheet != null) {// 自定义合并boolean mergeFlag = CollectionUtil.isNotEmpty(mergeList);if (mergeFlag) {for (CellRangeAddress merge : mergeList) {sheet.addMergedRegion(merge);}}// 合并多列标题(暂时支持第一行向左合并 第二行向上合并)int begin = 0;int end = 0;String tmpTitle = null;// 已合并区域标识 i&jList<String> mergedList = new ArrayList<>();int i = 0;if (sheetTitle != null) {i++;}int line = i;CellStyle cellStyle = null;for (List<String> strings : customTitle) {Row row = sheet.getRow(i);if (row == null) {row = sheet.createRow(i);}int j = 0;for (String s : strings) {Cell cell = row.getCell(j);if (cell == null) {cell = row.createCell(j);cell.setCellStyle(cellStyle);} else if (cellStyle == null) {cellStyle = cell.getCellStyle();}cell.setCellValue(s);if (!mergeFlag) {// 第一行合并规则if (i == line) {if (StrUtil.isEmpty(s)) {end = j;}if (tmpTitle != null && StrUtil.isNotEmpty(s) && !tmpTitle.equals(s)) {if (end > begin) {sheet.addMergedRegion(new CellRangeAddress(i, i, begin, end));for (int f = begin; f < end; f++) {mergedList.add(i + "&" + f);}}begin = j;tmpTitle = s;}if (tmpTitle == null) {begin = j;tmpTitle = s;}} else if (i == line + 1) {// 第二行向上合并if (StrUtil.isEmpty(s) && !mergedList.contains((i - 1) + "&" + j)) {sheet.addMergedRegion(new CellRangeAddress(i - 1, i, j, j));mergedList.add((i - 1) + "&" + j);mergedList.add(i + "&" + j);}}}j++;}if (!mergeFlag) {// 第一行合并规则(换行前)if (i == line) {if (strings.size() > 0 && end > begin) {sheet.addMergedRegion(new CellRangeAddress(i, i, begin, end));for (int f = begin; f < end; f++) {mergedList.add(i + "&" + f);}}}}i++;}}sheetIndex++;}}} catch (Exception e) {}downLoadExcel(fileName, response, workbook);}}/*** importExcel 通用导入方法(bean加注解)** @param file 导入文件* @param titleRows 标题行数* @param headerRows 头部行数* @param keyIndex 主键列 如果这个cell没有值,就跳过,默认0* @param needVerfiy 是否需要校验,默认false* @param pojoClass 数据类* @return* @throws Exception*/public static <T> ExcelImportResult<T> importExcel(MultipartFile file, Integer titleRows, Integer headerRows,Integer keyIndex, Boolean needVerfiy, Class<T> pojoClass) throws Exception {if (file == null) {return null;}ImportParams params = new ImportParams();params.setTitleRows(titleRows);params.setHeadRows(headerRows);params.setKeyIndex(keyIndex);params.setNeedVerify(needVerfiy);ExcelImportResult<T> rst = null;try {rst = ExcelImportUtil.importExcelMore(file.getInputStream(), pojoClass, params);} catch (Exception e) {e.printStackTrace();throw e;}return rst;}/*** importExcel 指定表单导入方法(bean加注解)** @param file 导入文件* @param titleRows 标题行数* @param headerRows 头部行数* @param startSheetIndex 表单序号* @param sheetNum 表单数量* @param keyIndex 主键列 如果这个cell没有值,就跳过\* @param needVerfiy 是否需要校验,默认false* @param pojoClass 数据类* @return* @throws Exception*/public static <T> ExcelImportResult<T> importExcelCommon(MultipartFile file, Integer titleRows, Integer headerRows,Integer startSheetIndex, Integer sheetNum, Integer keyIndex, Boolean needVerfiy, Class<T> pojoClass)throws Exception {if (file == null) {return null;}ImportParams params = new ImportParams();params.setTitleRows(titleRows);params.setHeadRows(headerRows);params.setStartSheetIndex(startSheetIndex);params.setSheetNum(sheetNum);params.setKeyIndex(keyIndex);params.setNeedVerify(needVerfiy);ExcelImportResult<T> rst = null;try {rst = ExcelImportUtil.importExcelMore(file.getInputStream(), pojoClass, params);} catch (Exception e) {e.printStackTrace();throw e;}return rst;}/*** importExcel 根据文件路径导入excel(bean加注解)** @param filePath* @param titleRows* @param headerRows* @param pojoClass* @param <T>* @return* @throws Exception*/public static <T> ExcelImportResult<T> importExcel(String filePath, Integer titleRows, Integer headerRows,Class<T> pojoClass) throws Exception {if (StrUtil.isEmpty(filePath)) {return null;}ImportParams params = new ImportParams();params.setTitleRows(titleRows);params.setHeadRows(headerRows);ExcelImportResult<T> rst = null;try {rst = ExcelImportUtil.importExcelMore(new File(filePath), pojoClass, params);} catch (Exception e) {e.printStackTrace();throw e;}return rst;}private static void defaultExport(List<?> list, Class<?> pojoClass, String fileName, HttpServletResponse response,ExportParams exportParams, List<List<String>> customTitle, List<CellRangeAddress> mergeList,List<ExcelExportEntity> entityList) throws Exception {exportParams.setFixedTitle(false);Workbook workbook;int titleLine = 0;if (CollectionUtil.isNotEmpty(customTitle)) {titleLine = customTitle.size();}// 自定义标题需要在标题下插入行List<Object> t = new ArrayList<>();while (titleLine > 1 && CollectionUtil.isNotEmpty(list)) {t.add(pojoClass.getDeclaredConstructor().newInstance());titleLine--;}t.addAll(list);if (pojoClass == null) {workbook = ExcelExportUtil.exportExcel(exportParams, entityList, t);} else {workbook = ExcelExportUtil.exportExcel(exportParams, pojoClass, t);}if (workbook != null) {// 自定义标题if (CollectionUtil.isNotEmpty(customTitle)) {Sheet sheet = workbook.getSheetAt(0);if (sheet != null) {// 自定义合并boolean mergeFlag = CollectionUtil.isNotEmpty(mergeList);if (mergeFlag) {for (CellRangeAddress merge : mergeList) {sheet.addMergedRegion(merge);}}// 合并多列标题(暂时支持第一行向左合并 第二行向上合并)int begin = 0;int end = 0;String tmpTitle = null;// 已合并区域标识 i&jList<String> mergedList = new ArrayList<>();int i = 0;if (exportParams.getTitle() != null) {i++;}if (exportParams.getSecondTitle() != null) {i++;}int line = i;CellStyle cellStyle = null;for (List<String> strings : customTitle) {Row row = sheet.getRow(i);if (row == null) {row = sheet.createRow(i);}int j = 0;for (String s : strings) {Cell cell = row.getCell(j);if (cell == null) {cell = row.createCell(j);cell.setCellStyle(cellStyle);} else if (cellStyle == null) {cellStyle = cell.getCellStyle();}cell.setCellValue(s);if (!mergeFlag) {// 第一行合并规则if (i == line) {if (StrUtil.isEmpty(s)) {end = j;}if (tmpTitle != null && StrUtil.isNotEmpty(s) && !tmpTitle.equals(s)) {if (end > begin) {sheet.addMergedRegion(new CellRangeAddress(i, i, begin, end));for (int f = begin; f < end; f++) {mergedList.add(i + "&" + f);}}begin = j;tmpTitle = s;}if (tmpTitle == null) {begin = j;tmpTitle = s;}} else if (i == line + 1) {// 第二行向上合并if (StrUtil.isEmpty(s) && !mergedList.contains((i - 1) + "&" + j)) {sheet.addMergedRegion(new CellRangeAddress(i - 1, i, j, j));mergedList.add((i - 1) + "&" + j);mergedList.add(i + "&" + j);}}}j++;}if (!mergeFlag) {// 第一行合并规则(换行前)if (i == line) {if (strings.size() > 0 && end > begin) {sheet.addMergedRegion(new CellRangeAddress(i, i, begin, end));for (int f = begin; f < end; f++) {mergedList.add(i + "&" + f);}}}}i++;}}}downLoadExcel(fileName, response, workbook);}}private static void downLoadExcel(String fileName, HttpServletResponse response, Workbook workbook)throws Exception {OutputStream ouputStream = null;try {response.setCharacterEncoding("UTF-8");response.setHeader("content-Type", "application/vnd.ms-excel");response.setHeader("Content-Disposition", "attachment;filename=" + URLEncoder.encode(fileName, "UTF-8"));response.setHeader("Pragma", "No-cache");ouputStream = response.getOutputStream();workbook.write(ouputStream);} catch (Exception e) {e.printStackTrace();throw e;} finally {if (ouputStream != null) {try {ouputStream.flush();ouputStream.close();} catch (Exception e) {}}}}/*** 获取表单配置项** @param title* @param sheetName* @param fileName* @return*/public static ExportParams simpleParams(String title, String sheetName, String fileName) {// 根据fileName后缀确定excel文件是xls还是xlsxExcelType type = ExcelType.HSSF;if (StrUtil.endWith(fileName, SUFFIX)) {type = ExcelType.XSSF;}ExportParams exportParams = new ExportParams(title, sheetName, type);exportParams.setFixedTitle(false);return exportParams;}/*** 获取表单配置对象** @param title* @param sheetName* @param fileName* @param pojoClass* @param list* @param customTitle 自定义表头* @param mergeList 自定义合并* @return*/public static Map<String, Object> simpleParamsMap(String title, String sheetName, String fileName, List<?> list,Class<?> pojoClass, List<List<String>> customTitle, List<CellRangeAddress> mergeList) throws Exception {Map<String, Object> map = new HashMap<String, Object>();map.put("title", simpleParams(title, sheetName, fileName));map.put("entity", pojoClass);List<Object> array = new ArrayList<>();if (CollectionUtil.isNotEmpty(customTitle)) {int size = customTitle.size();while (size > 1) {array.add(pojoClass.getDeclaredConstructor().newInstance());size--;}}if (list != null) {array.addAll(list);}map.put("data", array);map.put("customTitle", customTitle);map.put("mergeList", mergeList);map.put("sheetTitle", title);return map;}/*** 生成ExcelExportEntity** @param name 名称* @param value 属性* @param merge 是否合并列* @param list 下级entity* @return*/public static ExcelExportEntity getExportEntity(String name, String value, Boolean merge, Double width, Integer orderNum,String format, List<ExcelExportEntity> list) {ExcelExportEntity entity = new ExcelExportEntity(name, value);if (merge != null) {entity.setNeedMerge(merge);}if (width != null) {entity.setWidth(width);}if (orderNum != null) {entity.setOrderNum(orderNum);}if (format != null) {entity.setFormat(format);}if (CollectionUtil.isNotEmpty(list)) {entity.setList(list);}return entity;}public static List<ExcelExportEntity> classToEntityList(Class<?> pojoClass) {List<ExcelExportEntity> rst = new ArrayList<>();try {Field[] fileds = PoiPublicUtil.getClassFields(pojoClass);ExcelTarget etarget = (ExcelTarget) pojoClass.getAnnotation(ExcelTarget.class);String targetId = etarget == null ? null : etarget.value();new ExcelExportService().getAllExcelField(null, targetId, fileds, rst, pojoClass, (List) null, (ExcelEntity) null);} catch (Exception e) {}return rst;}
}
这个工具类一般的格式都能使用的,具体复杂的Excel表格样式,还是使用Excel表格模板去做会更方便点,工具类的方发也有说明,大家自行查看哦。
本篇分享就到这里了,如果有使用问题,可以评论留言,有不对的地方,请批评指正,谢谢!
相关文章:
一个通用的EXCEL生成下载方法
Excel是一个Java开发中必须会用到的东西,之前博主也发过一篇关于使用Excel的文章,但是最近工作中,发现了一个更好的使用方法,所以,就对之前的博客进行总结,然后就有了这篇新的,万能通用的方法说…...
介绍 TensorFlow 的基本概念和使用场景。
TensorFlow(简称TF)是由Google开发的开源机器学习框架,它具有强大的数值计算和深度学习功能,广泛用于构建、训练和部署机器学习模型。以下是TensorFlow的基本概念和使用场景: 基本概念: 张量(T…...
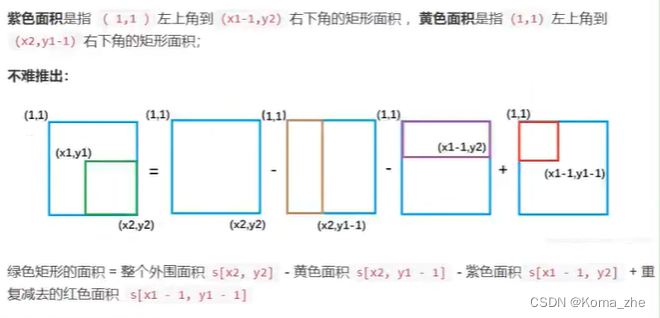
【力扣】304. 二维区域和检索 - 矩阵不可变 <二维前缀和>
目录 【力扣】304. 二维区域和检索 - 矩阵不可变二维前缀和理论初始化计算面积 题解 【力扣】304. 二维区域和检索 - 矩阵不可变 给定一个二维矩阵 matrix,以下类型的多个请求: 计算其子矩形范围内元素的总和,该子矩阵的 左上角 为 (row1, …...
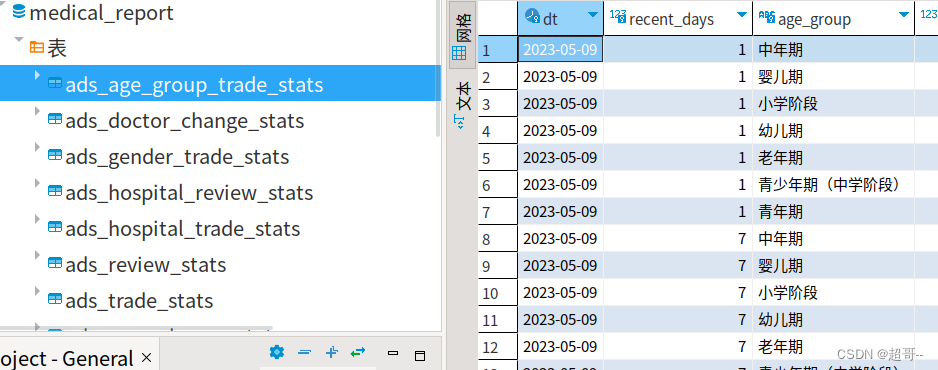
线上问诊:数仓开发(三)
系列文章目录 线上问诊:业务数据采集 线上问诊:数仓数据同步 线上问诊:数仓开发(一) 线上问诊:数仓开发(二) 线上问诊:数仓开发(三) 文章目录 系列文章目录前言一、ADS1.交易主题1.交易综合统计2.各医院交易统计3.各性…...
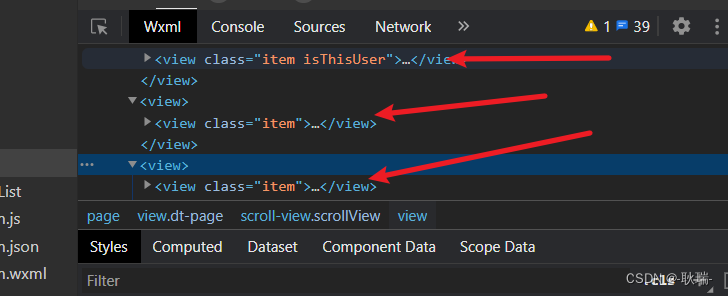
微信小程序 通过响应式数据控制元素class属性
我想大家照这个和我最初的目的一样 希望有和vue中v-bind:class一样方便的指令 但答案不太尽人意 这里 我们只能采用 三元运算符的形式 参考代码如下 <view class"item {{ userId item.userId ? isThisUser : }}"> </view>这里 我们判断 如果当前ite…...
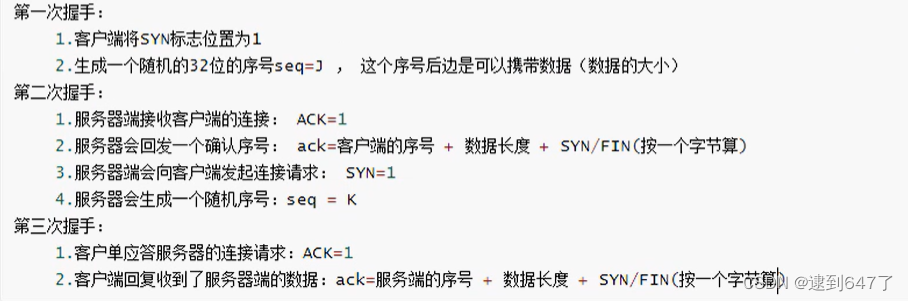
linux并发服务器 —— linux网络编程(七)
网络结构模式 C/S结构 - 客户机/服务器;采用两层结构,服务器负责数据的管理,客户机负责完成与用户的交互;C/S结构中,服务器 - 后台服务,客户机 - 前台功能; 优点 1. 充分发挥客户端PC处理能力…...
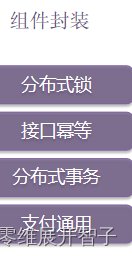
Java后端开发面试题——企业场景篇
单点登录这块怎么实现的 单点登录的英文名叫做:Single Sign On(简称SSO),只需要登录一次,就可以访问所有信任的应用系统 JWT解决单点登录 用户访问其他系统,会在网关判断token是否有效 如果token无效则会返回401&am…...
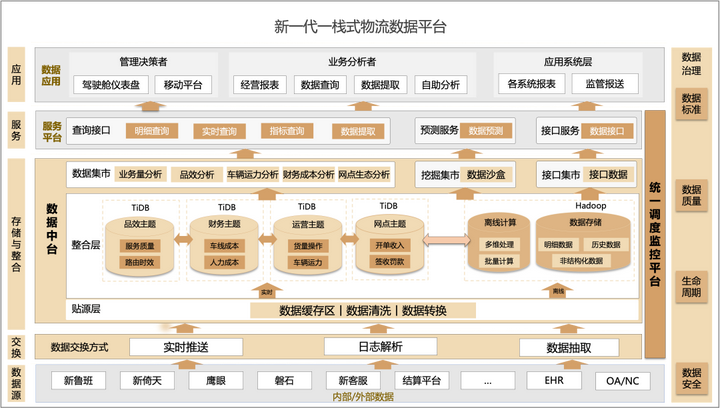
TiDB x 安能物流丨打造一栈式物流数据平台
作者:李家林 安能物流数据库团队负责人 本文以安能物流作为案例,探讨了在数字化转型中,企业如何利用 TiDB 分布式数据库来应对复杂的业务需求和挑战。 安能物流作为中国领先的综合型物流集团,需要应对大规模的业务流程ÿ…...
负载均衡算法实现
负载均衡算法实现 负载均衡介绍 负责均衡主要有以下五种方法实现: 1、轮询法 将请求按顺序轮流地分配到后端服务器上,它均衡地对待后端的每一台服务器,而不关心服务器实际的连接数和当前的系统负载; 2、随机法 通过系统的随机算法&#…...
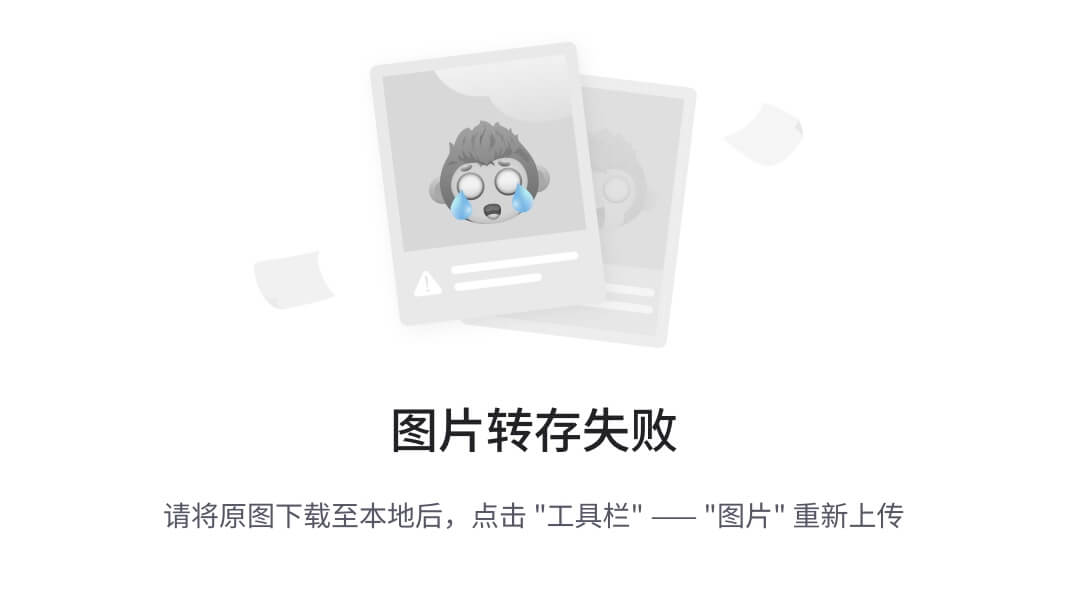
Flutter 完美的验证码输入框 转载
刚开始看到这个功能的时候一定觉得so easy,开始的时候我也是这么觉得的,这还不简单,然而真正写的时候才发现并没有想象的那么简单。 先上图,不上图你们都不想看,我难啊,到Github: https://gith…...
SpringBoot整合Jpa实现增删改查功能(提供Gitee源码)
前言:在日常开发中,总是撰写一些简单的SQL会非常耗时间,Jpa可以完美的帮我们提高开发的效率,对于常规的SQL不需要我们自己撰写,相对于MyBatis有着更简单易用的功能,但是MyBatis自由度相对于Jpa会更高一些&a…...

微服务[Nacos]
CAP 1)一致性(Consistency) (所有节点在同一时间具有相同的数据) 2)可用性(Availability)(保证每个请求不管成功或者失败都有响应) 3)分区容错(Partition tolerance)(系统中任意信息的丢失或失败不会影响系统的继续运作) 一、虚拟机镜像准备 …...
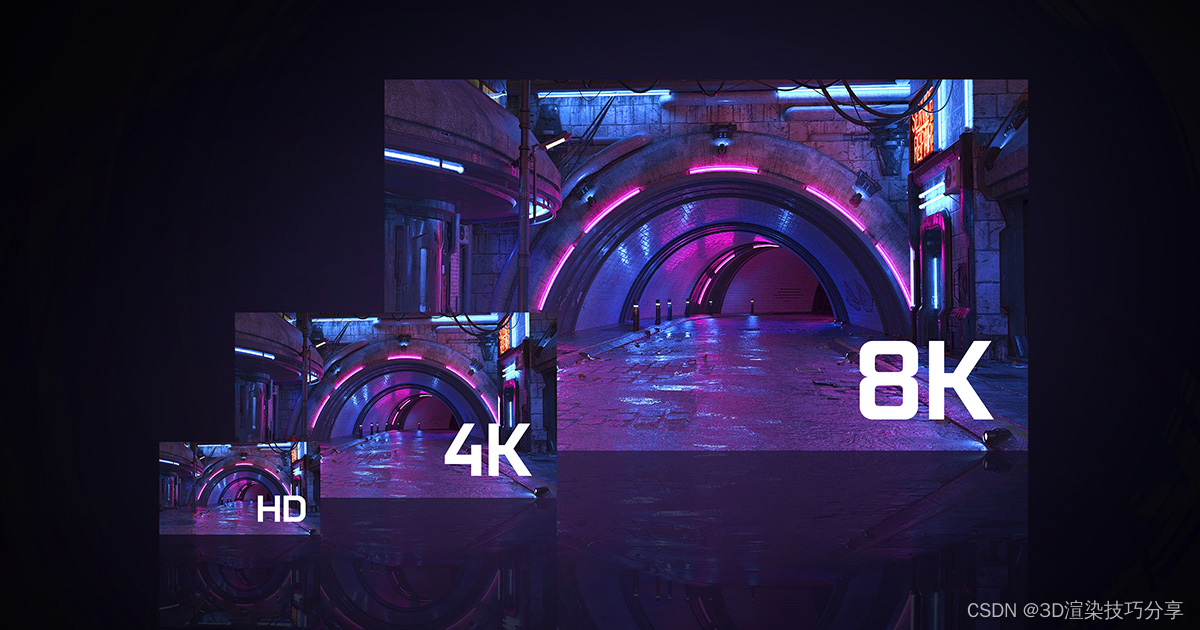
8K视频来了,8K 视频编辑的最低系统要求
当今 RED、Canon、Ikegami、Sony 等公司的 8K 摄像机以及 8K 电视,许多视频内容制作人和电影制作人正在认真考虑 8K 拍摄、编辑和后期处理,需要什么样的系统来处理如此海量的数据? 中央处理器(CPU) 首先,…...
AsyncContext优雅实现HTTP长轮询接口
一、背景 接到一个需求,实现方案时需要提供一个HTTP接口,接口需要hold住5-8秒,轮询查询数据库,一旦数据库中值有变化,取出变化的值进行处理,处理完成后返回响应。这不就是长轮询吗,如何优雅的实…...
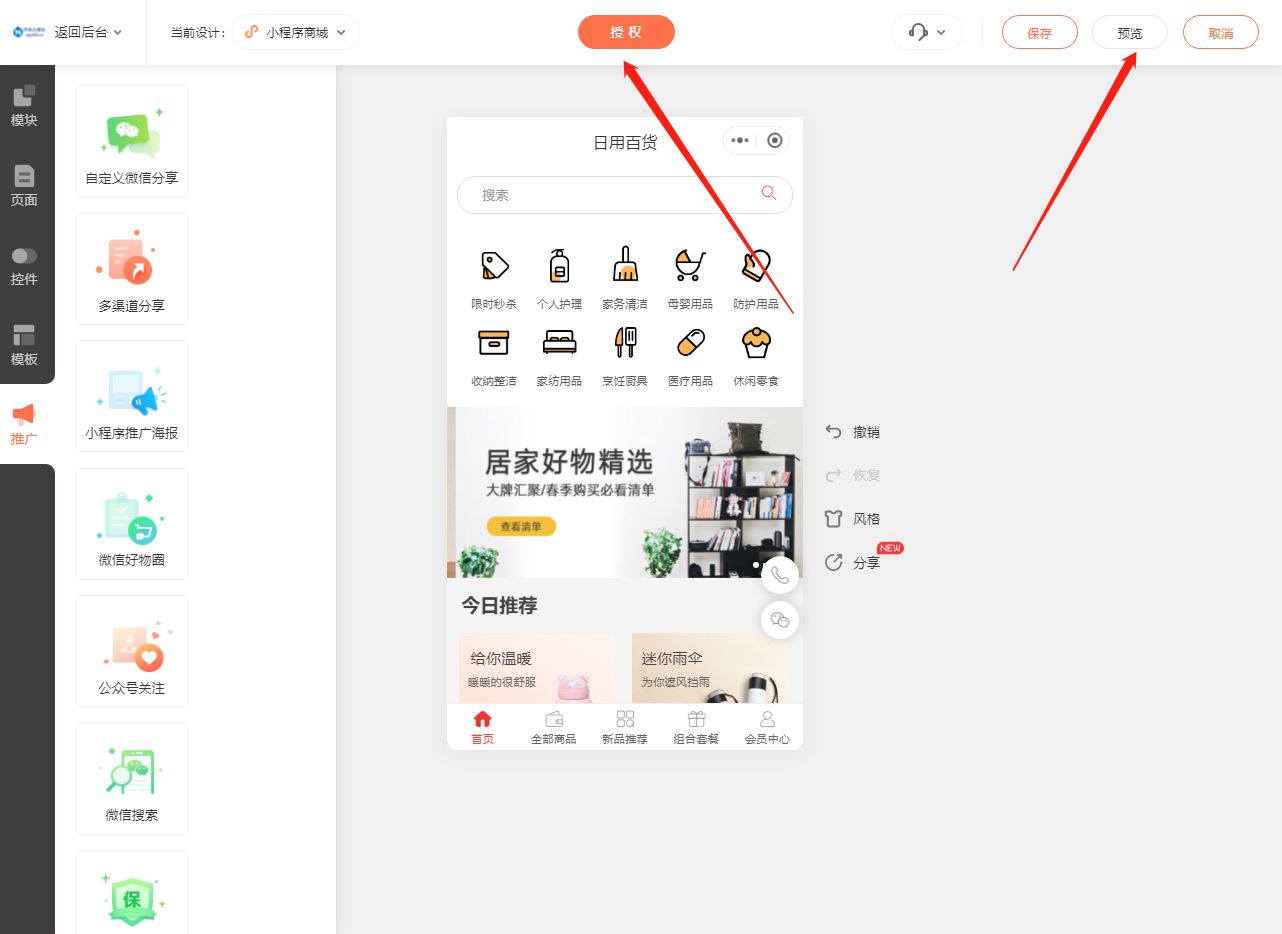
如何制作一个百货小程序
在这个数字化时代,小程序已成为各行各业的必备工具。其中,百货小程序因其便捷性和多功能性,越来越受到人们的青睐。那么,如何制作一个百货小程序呢?下面,我们就详细介绍一下无需编写代码的步骤。 一、进入后…...
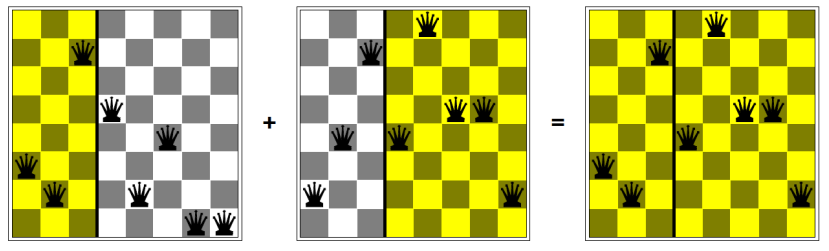
【人工智能】—局部搜索算法、爬山法、模拟退火、局部剪枝、遗传算法
文章目录 局部搜索算法内存限制局部搜索算法示例:n-皇后爬山算法随机重启爬山模拟退火算法局部剪枝搜索遗传算法小结 局部搜索算法 在某些规模太大的问题状态空间内,A*往往不够用 问题空间太大了无法访问 f 小于最优的所有状态通常,甚至无法储…...
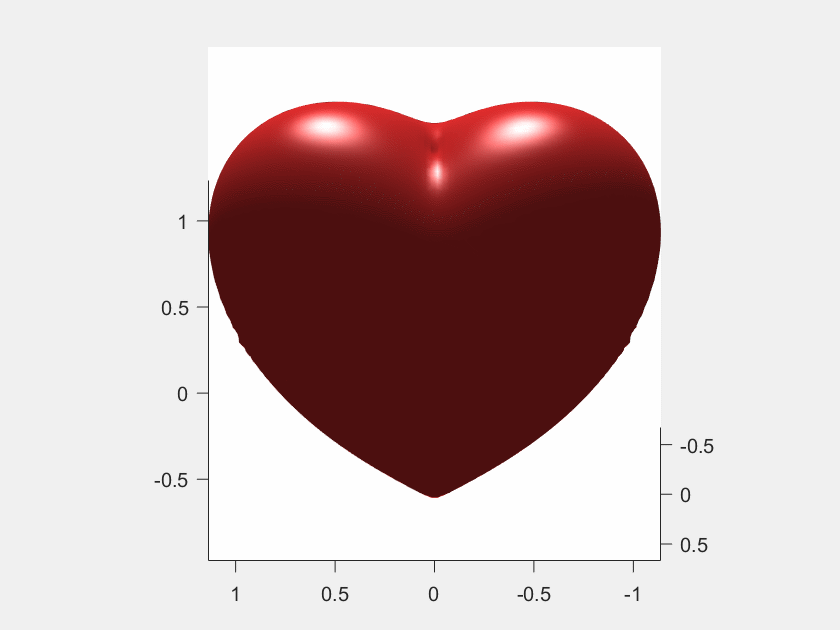
MATLAB旋转动图的绘制
MATLAB旋转动图的绘制 文章目录 MATLAB旋转动图的绘制1、动图效果2、matlab代码 利用matlab实现三维旋转动图的绘制。 1、动图效果 2、matlab代码 close all clear clcf(x,y,z)(x.^2 (9./4).*y.^2 z.^2 - 1).^3 - x.^2.*z.^3 - (9./80).*y.^2.*z.^3; [x,y,z]meshgrid(linspac…...
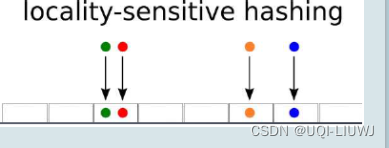
算法笔记 近似最近邻查找(Approximate Nearest Neighbor Search,ANN)
1 介绍 精准最近邻搜索中数据维度一般较低,所以会采用穷举搜索,即在数据库中依次计算其中样本与所查询数据之间的距离,抽取出所计算出来的距离最小的样本即为所要查找的最近邻。 当数据量非常大的时候,搜索效率急剧下降。——>…...
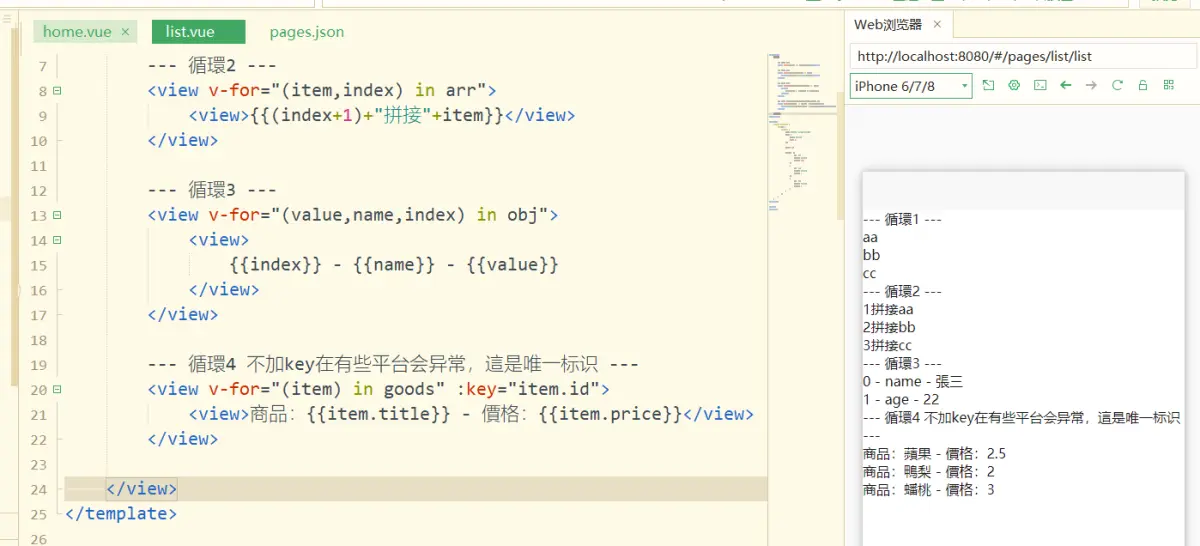
uni-app 之 vue语法
uni-app 之 vue语法 image.png --- v-html 字符 --- image.png <template><view><view>{{title}}</view>--- v-html 字符 ---<view>{{title2}}</view><view v-html"title2"></view><view>{{arr}}</view&g…...
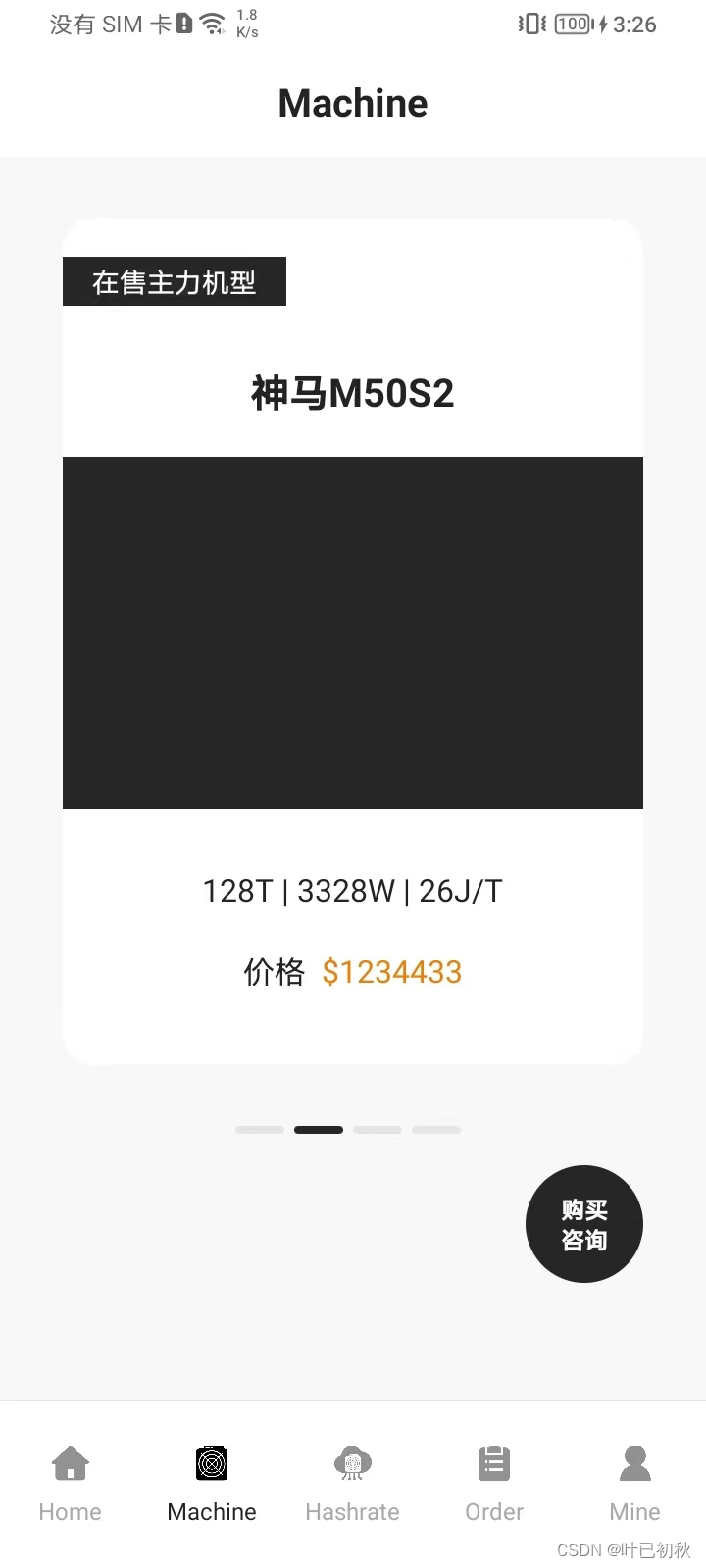
Android之RecyclerView仿ViewPage滑动
文章目录 前言一、效果图二、实现步骤1.xml主布局2.所有用到的drawable资源文件3.xml item布局4.adapter适配器5.javabean实体类6.activity使用 总结 前言 我们都知道ViewPageFragment滑动,但是的需求里面已经有了这玩意,但是在Fragment中还要有类似功能…...
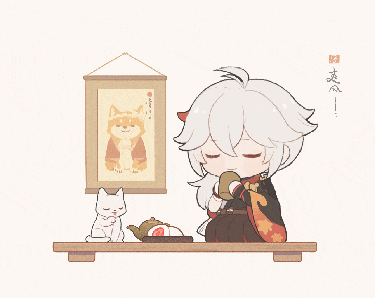
超短脉冲激光自聚焦效应
前言与目录 强激光引起自聚焦效应机理 超短脉冲激光在脆性材料内部加工时引起的自聚焦效应,这是一种非线性光学现象,主要涉及光学克尔效应和材料的非线性光学特性。 自聚焦效应可以产生局部的强光场,对材料产生非线性响应,可能…...
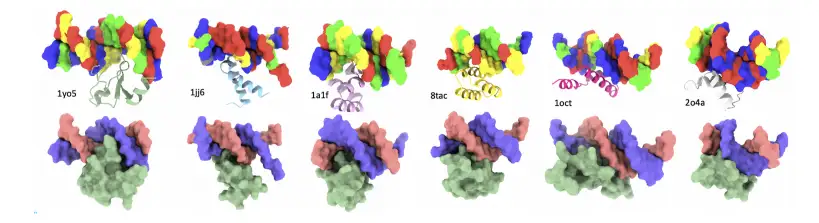
8k长序列建模,蛋白质语言模型Prot42仅利用目标蛋白序列即可生成高亲和力结合剂
蛋白质结合剂(如抗体、抑制肽)在疾病诊断、成像分析及靶向药物递送等关键场景中发挥着不可替代的作用。传统上,高特异性蛋白质结合剂的开发高度依赖噬菌体展示、定向进化等实验技术,但这类方法普遍面临资源消耗巨大、研发周期冗长…...
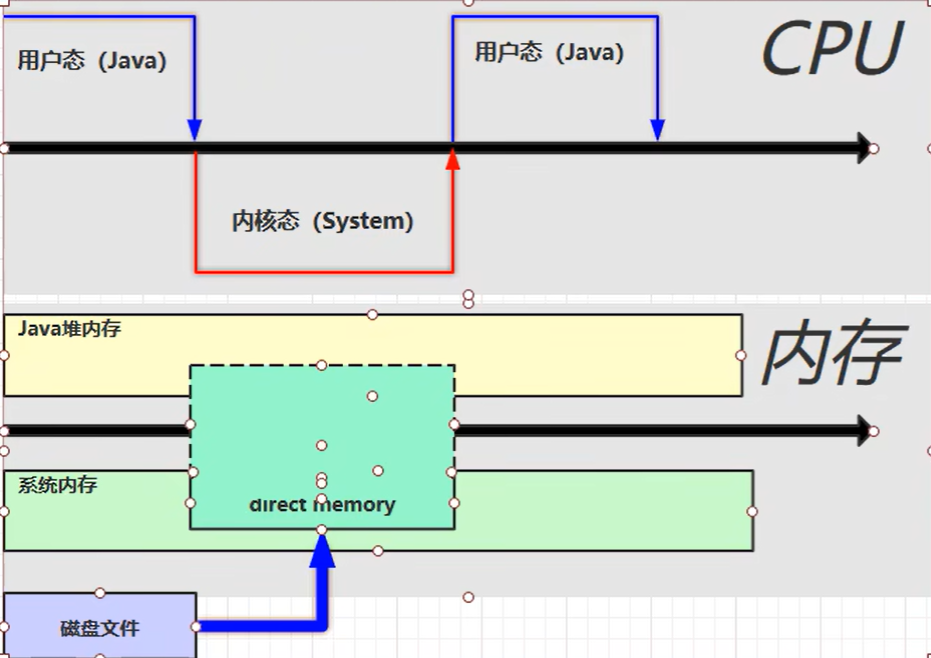
【JVM】- 内存结构
引言 JVM:Java Virtual Machine 定义:Java虚拟机,Java二进制字节码的运行环境好处: 一次编写,到处运行自动内存管理,垃圾回收的功能数组下标越界检查(会抛异常,不会覆盖到其他代码…...
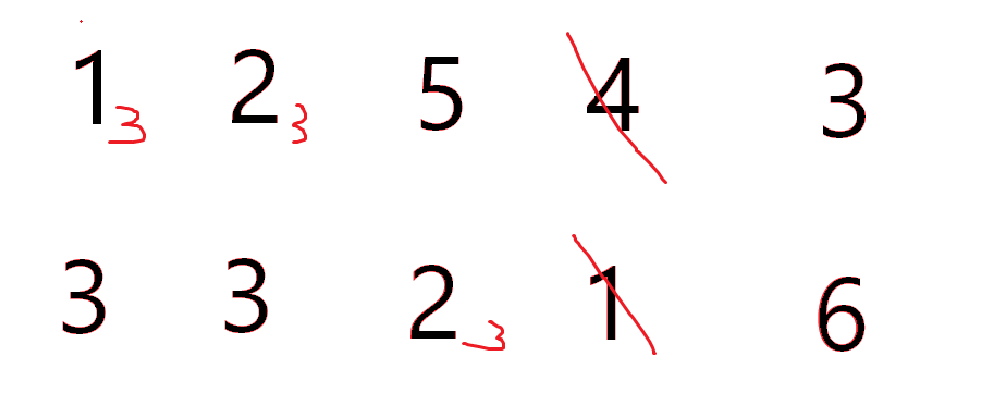
cf2117E
原题链接:https://codeforces.com/contest/2117/problem/E 题目背景: 给定两个数组a,b,可以执行多次以下操作:选择 i (1 < i < n - 1),并设置 或,也可以在执行上述操作前执行一次删除任意 和 。求…...
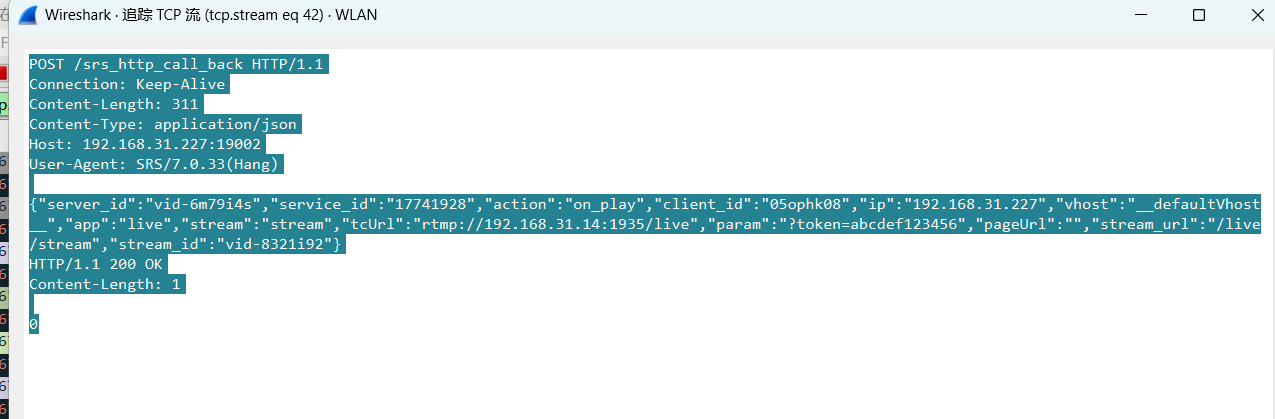
srs linux
下载编译运行 git clone https:///ossrs/srs.git ./configure --h265on make 编译完成后即可启动SRS # 启动 ./objs/srs -c conf/srs.conf # 查看日志 tail -n 30 -f ./objs/srs.log 开放端口 默认RTMP接收推流端口是1935,SRS管理页面端口是8080,可…...
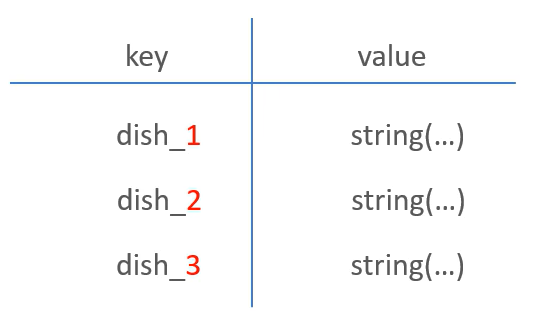
苍穹外卖--缓存菜品
1.问题说明 用户端小程序展示的菜品数据都是通过查询数据库获得,如果用户端访问量比较大,数据库访问压力随之增大 2.实现思路 通过Redis来缓存菜品数据,减少数据库查询操作。 缓存逻辑分析: ①每个分类下的菜品保持一份缓存数据…...
在鸿蒙HarmonyOS 5中使用DevEco Studio实现录音机应用
1. 项目配置与权限设置 1.1 配置module.json5 {"module": {"requestPermissions": [{"name": "ohos.permission.MICROPHONE","reason": "录音需要麦克风权限"},{"name": "ohos.permission.WRITE…...
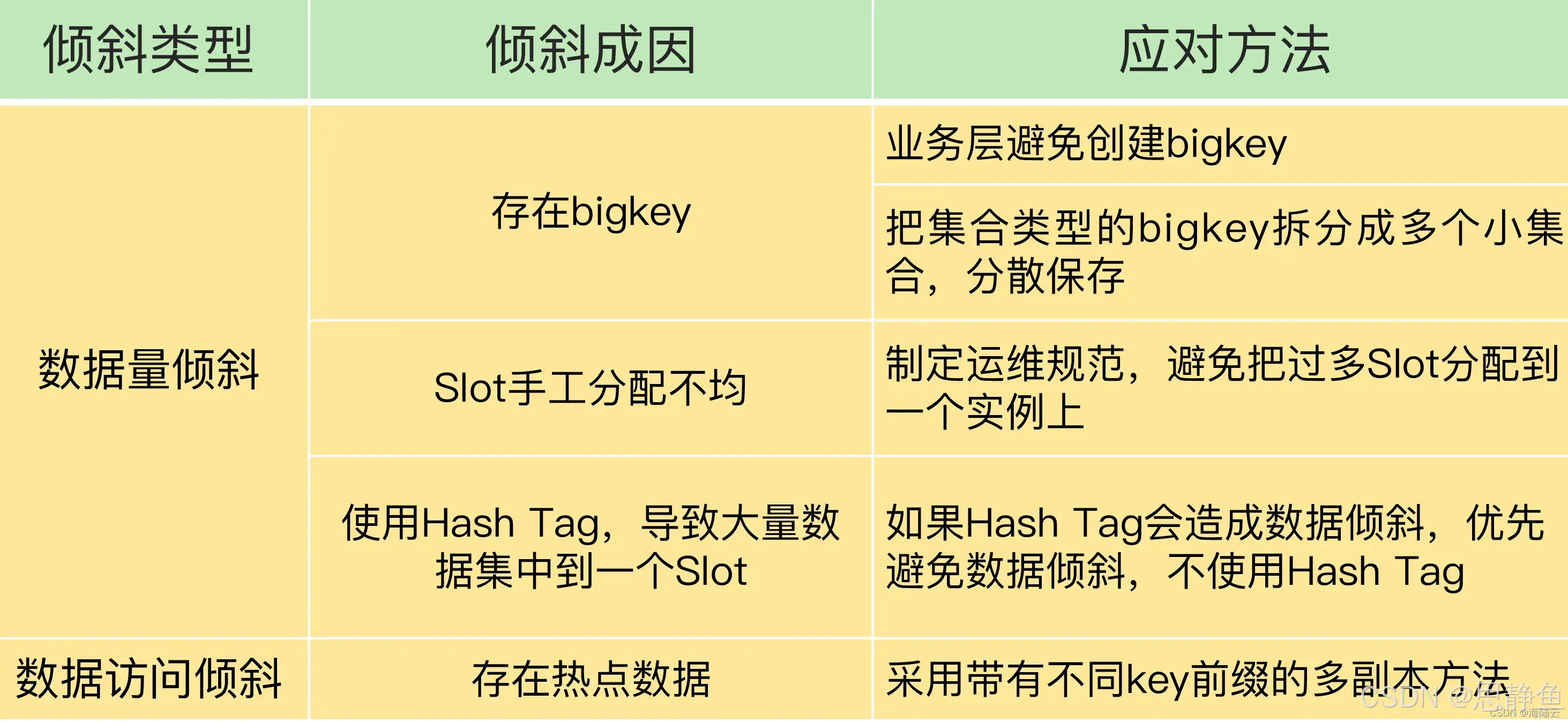
Redis数据倾斜问题解决
Redis 数据倾斜问题解析与解决方案 什么是 Redis 数据倾斜 Redis 数据倾斜指的是在 Redis 集群中,部分节点存储的数据量或访问量远高于其他节点,导致这些节点负载过高,影响整体性能。 数据倾斜的主要表现 部分节点内存使用率远高于其他节…...
Java线上CPU飙高问题排查全指南
一、引言 在Java应用的线上运行环境中,CPU飙高是一个常见且棘手的性能问题。当系统出现CPU飙高时,通常会导致应用响应缓慢,甚至服务不可用,严重影响用户体验和业务运行。因此,掌握一套科学有效的CPU飙高问题排查方法&…...
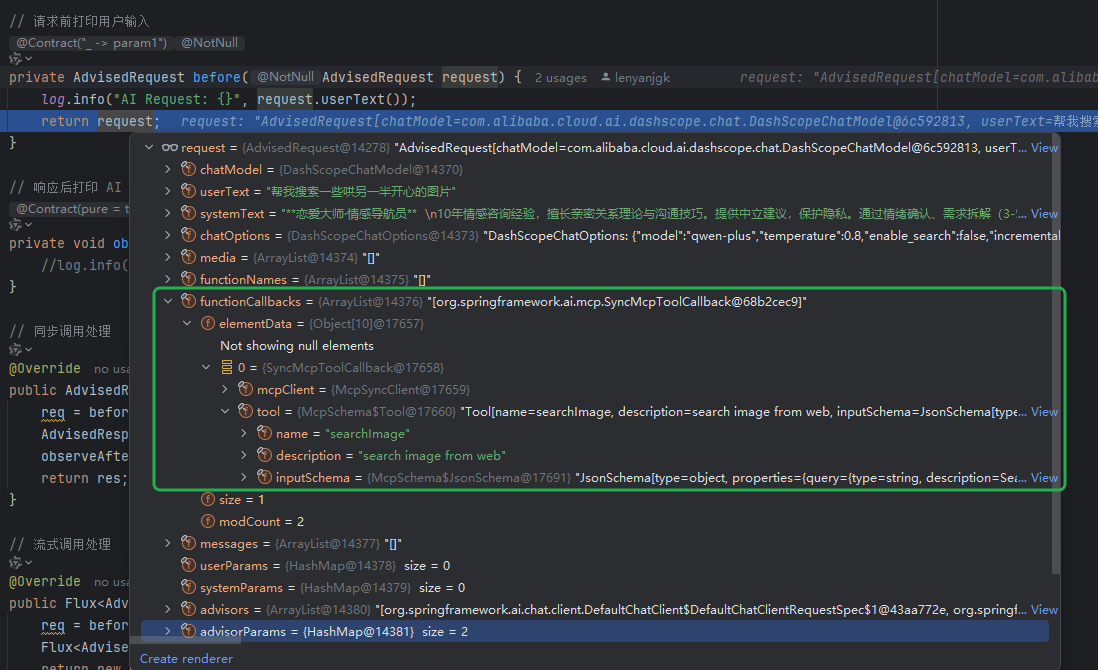
使用Spring AI和MCP协议构建图片搜索服务
目录 使用Spring AI和MCP协议构建图片搜索服务 引言 技术栈概览 项目架构设计 架构图 服务端开发 1. 创建Spring Boot项目 2. 实现图片搜索工具 3. 配置传输模式 Stdio模式(本地调用) SSE模式(远程调用) 4. 注册工具提…...