文档在线预览word、pdf、excel文件转html以实现文档在线预览
目录
- 一、前言
- 1、aspose
- 2 、poi + pdfbox
- 3 spire
- 二、将文件转换成html字符串
- 1、将word文件转成html字符串
- 1.1 使用aspose
- 1.2 使用poi
- 1.3 使用spire
- 2、将pdf文件转成html字符串
- 2.1 使用aspose
- 2.2 使用 poi + pbfbox
- 2.3 使用spire
- 3、将excel文件转成html字符串
- 3.1 使用aspose
- 3.2 使用poi + pdfbox
- 3.3 使用spire
- 三、将文件转换成html,并生成html文件
- FileUtils类将html字符串生成html文件示例:
- 1、将word文件转换成html文件
- 1.1 使用aspose
- 1.2 使用poi + pdfbox
- 1.3 使用spire
- 2、将pdf文件转换成html文件
- 2.1 使用aspose
- 2.2 使用poi + pdfbox
- 2.3 使用spire
- 3、将excel文件转换成html文件
- 3.1 使用aspose
- 3.2 使用poi
- 3.3 使用spire
- 四、总结
- 1、将word文件转成html字符串
一、前言
以下代码分别提供基于aspose、pdfbox、spire来实现来实现txt、word、pdf、ppt、word等文件转图片的需求。
1、aspose
Aspose 是一家致力于.Net ,Java,SharePoint,JasperReports和SSRS组件的提供商,数十个国家的数千机构都有用过aspose组件,创建、编辑、转换或渲染 Office、OpenOffice、PDF、图像、ZIP、CAD、XPS、EPS、PSD 和更多文件格式。注意aspose是商用组件,未经授权导出文件里面都是是水印(尊重版权,远离破解版)。
需要在项目的pom文件里添加如下依赖
<dependency><groupId>com.aspose</groupId><artifactId>aspose-words</artifactId><version>23.1</version></dependency><dependency><groupId>com.aspose</groupId><artifactId>aspose-pdf</artifactId><version>23.1</version></dependency><dependency><groupId>com.aspose</groupId><artifactId>aspose-cells</artifactId><version>23.1</version></dependency><dependency><groupId>com.aspose</groupId><artifactId>aspose-slides</artifactId><version>23.1</version></dependency>
2 、poi + pdfbox
因为aspose和spire虽然好用,但是都是是商用组件,所以这里也提供使用开源库操作的方式的方式。
POI是Apache软件基金会用Java编写的免费开源的跨平台的 Java API,Apache POI提供API给Java程序对Microsoft Office格式档案读和写的功能。
Apache PDFBox是一个开源Java库,支持PDF文档的开发和转换。 使用此库,您可以开发用于创建,转换和操作PDF文档的Java程序。
需要在项目的pom文件里添加如下依赖
<dependency><groupId>org.apache.pdfbox</groupId><artifactId>pdfbox</artifactId><version>2.0.4</version></dependency><dependency><groupId>org.apache.poi</groupId><artifactId>poi</artifactId><version>5.2.0</version></dependency><dependency><groupId>org.apache.poi</groupId><artifactId>poi-ooxml</artifactId><version>5.2.0</version></dependency><dependency><groupId>org.apache.poi</groupId><artifactId>poi-scratchpad</artifactId><version>5.2.0</version></dependency><dependency><groupId>org.apache.poi</groupId><artifactId>poi-excelant</artifactId><version>5.2.0</version></dependency>
3 spire
spire一款专业的Office编程组件,涵盖了对Word、Excel、PPT、PDF等文件的读写、编辑、查看功能。spire提供免费版本,但是存在只能导出前3页以及只能导出前500行的限制,只要达到其一就会触发限制。需要超出前3页以及只能导出前500行的限制的这需要购买付费版(尊重版权,远离破解版)。这里使用免费版进行演示。
spire在添加pom之前还得先添加maven仓库来源
<repository><id>com.e-iceblue</id><name>e-iceblue</name><url>https://repo.e-iceblue.cn/repository/maven-public/</url></repository>
接着在项目的pom文件里添加如下依赖
免费版:
<dependency><groupId>e-iceblue</groupId><artifactId>spire.office.free</artifactId><version>5.3.1</version></dependency>
付费版版:
<dependency><groupId>e-iceblue</groupId><artifactId>spire.office</artifactId><version>5.3.1</version></dependency>
二、将文件转换成html字符串
1、将word文件转成html字符串
1.1 使用aspose
public static String wordToHtmlStr(String wordPath) {try {Document doc = new Document(wordPath); // Address是将要被转化的word文档String htmlStr = doc.toString();return htmlStr;} catch (Exception e) {e.printStackTrace();}return null;}
验证结果:
1.2 使用poi
public String wordToHtmlStr(String wordPath) throws TransformerException, IOException, ParserConfigurationException {String htmlStr = null;String ext = wordPath.substring(wordPath.lastIndexOf("."));if (ext.equals(".docx")) {htmlStr = word2007ToHtmlStr(wordPath);} else if (ext.equals(".doc")){htmlStr = word2003ToHtmlStr(wordPath);} else {throw new RuntimeException("文件格式不正确");}return htmlStr;}public String word2007ToHtmlStr(String wordPath) throws IOException {// 使用内存输出流try(ByteArrayOutputStream out = new ByteArrayOutputStream()){word2007ToHtmlOutputStream(wordPath, out);return out.toString();}}private void word2007ToHtmlOutputStream(String wordPath,OutputStream out) throws IOException {ZipSecureFile.setMinInflateRatio(-1.0d);InputStream in = Files.newInputStream(Paths.get(wordPath));XWPFDocument document = new XWPFDocument(in);XHTMLOptions options = XHTMLOptions.create().setIgnoreStylesIfUnused(false).setImageManager(new Base64EmbedImgManager());// 使用内存输出流XHTMLConverter.getInstance().convert(document, out, options);}private String word2003ToHtmlStr(String wordPath) throws TransformerException, IOException, ParserConfigurationException {org.w3c.dom.Document htmlDocument = word2003ToHtmlDocument(wordPath);// Transform document to stringStringWriter writer = new StringWriter();TransformerFactory tf = TransformerFactory.newInstance();Transformer transformer = tf.newTransformer();transformer.setOutputProperty(OutputKeys.OMIT_XML_DECLARATION, "no");transformer.setOutputProperty(OutputKeys.METHOD, "html");transformer.setOutputProperty(OutputKeys.INDENT, "yes");transformer.setOutputProperty(OutputKeys.ENCODING, "UTF-8");transformer.transform(new DOMSource(htmlDocument), new StreamResult(writer));return writer.toString();}private org.w3c.dom.Document word2003ToHtmlDocument(String wordPath) throws IOException, ParserConfigurationException {InputStream input = Files.newInputStream(Paths.get(wordPath));HWPFDocument wordDocument = new HWPFDocument(input);WordToHtmlConverter wordToHtmlConverter = new WordToHtmlConverter(DocumentBuilderFactory.newInstance().newDocumentBuilder().newDocument());wordToHtmlConverter.setPicturesManager((content, pictureType, suggestedName, widthInches, heightInches) -> {System.out.println(pictureType);if (PictureType.UNKNOWN.equals(pictureType)) {return null;}BufferedImage bufferedImage = ImgUtil.toImage(content);String base64Img = ImgUtil.toBase64(bufferedImage, pictureType.getExtension());// 带图片的word,则将图片转为base64编码,保存在一个页面中StringBuilder sb = (new StringBuilder(base64Img.length() + "data:;base64,".length()).append("data:;base64,").append(base64Img));return sb.toString();});// 解析word文档wordToHtmlConverter.processDocument(wordDocument);return wordToHtmlConverter.getDocument();}
1.3 使用spire
public String wordToHtmlStr(String wordPath) throws IOException {try(ByteArrayOutputStream outputStream = new ByteArrayOutputStream()) {Document document = new Document();document.loadFromFile(wordPath);document.saveToFile(outputStream, FileFormat.Html);return outputStream.toString();}}
2、将pdf文件转成html字符串
2.1 使用aspose
public static String pdfToHtmlStr(String pdfPath) throws IOException, ParserConfigurationException {PDDocument document = PDDocument.load(new File(pdfPath));Writer writer = new StringWriter();new PDFDomTree().writeText(document, writer);writer.close();document.close();return writer.toString();}
验证结果:
2.2 使用 poi + pbfbox
public String pdfToHtmlStr(String pdfPath) throws IOException, ParserConfigurationException {PDDocument document = PDDocument.load(new File(pdfPath));Writer writer = new StringWriter();new PDFDomTree().writeText(document, writer);writer.close();document.close();return writer.toString();}
2.3 使用spire
public String pdfToHtmlStr(String pdfPath) throws IOException, ParserConfigurationException {try(ByteArrayOutputStream outputStream = new ByteArrayOutputStream()) {PdfDocument pdf = new PdfDocument();pdf.loadFromFile(pdfPath);return outputStream.toString();}}
3、将excel文件转成html字符串
3.1 使用aspose
public static String excelToHtmlStr(String excelPath) throws Exception {FileInputStream fileInputStream = new FileInputStream(excelPath);Workbook workbook = new XSSFWorkbook(fileInputStream);DataFormatter dataFormatter = new DataFormatter();FormulaEvaluator formulaEvaluator = workbook.getCreationHelper().createFormulaEvaluator();Sheet sheet = workbook.getSheetAt(0);StringBuilder htmlStringBuilder = new StringBuilder();htmlStringBuilder.append("<html><head><title>Excel to HTML using Java and POI library</title>");htmlStringBuilder.append("<style>table, th, td { border: 1px solid black; }</style>");htmlStringBuilder.append("</head><body><table>");for (Row row : sheet) {htmlStringBuilder.append("<tr>");for (Cell cell : row) {CellType cellType = cell.getCellType();if (cellType == CellType.FORMULA) {formulaEvaluator.evaluateFormulaCell(cell);cellType = cell.getCachedFormulaResultType();}String cellValue = dataFormatter.formatCellValue(cell, formulaEvaluator);htmlStringBuilder.append("<td>").append(cellValue).append("</td>");}htmlStringBuilder.append("</tr>");}htmlStringBuilder.append("</table></body></html>");return htmlStringBuilder.toString();}
返回的html字符串:
<html><head><title>Excel to HTML using Java and POI library</title><style>table, th, td { border: 1px solid black; }</style></head><body><table><tr><td>序号</td><td>姓名</td><td>性别</td><td>联系方式</td><td>地址</td></tr><tr><td>1</td><td>张晓玲</td><td>女</td><td>11111111111</td><td>上海市浦东新区xx路xx弄xx号</td></tr><tr><td>2</td><td>王小二</td><td>男</td><td>1222222</td><td>上海市浦东新区xx路xx弄xx号</td></tr><tr><td>1</td><td>张晓玲</td><td>女</td><td>11111111111</td><td>上海市浦东新区xx路xx弄xx号</td></tr><tr><td>2</td><td>王小二</td><td>男</td><td>1222222</td><td>上海市浦东新区xx路xx弄xx号</td></tr><tr><td>1</td><td>张晓玲</td><td>女</td><td>11111111111</td><td>上海市浦东新区xx路xx弄xx号</td></tr><tr><td>2</td><td>王小二</td><td>男</td><td>1222222</td><td>上海市浦东新区xx路xx弄xx号</td></tr><tr><td>1</td><td>张晓玲</td><td>女</td><td>11111111111</td><td>上海市浦东新区xx路xx弄xx号</td></tr><tr><td>2</td><td>王小二</td><td>男</td><td>1222222</td><td>上海市浦东新区xx路xx弄xx号</td></tr><tr><td>1</td><td>张晓玲</td><td>女</td><td>11111111111</td><td>上海市浦东新区xx路xx弄xx号</td></tr><tr><td>2</td><td>王小二</td><td>男</td><td>1222222</td><td>上海市浦东新区xx路xx弄xx号</td></tr><tr><td>1</td><td>张晓玲</td><td>女</td><td>11111111111</td><td>上海市浦东新区xx路xx弄xx号</td></tr><tr><td>2</td><td>王小二</td><td>男</td><td>1222222</td><td>上海市浦东新区xx路xx弄xx号</td></tr><tr><td>1</td><td>张晓玲</td><td>女</td><td>11111111111</td><td>上海市浦东新区xx路xx弄xx号</td></tr><tr><td>2</td><td>王小二</td><td>男</td><td>1222222</td><td>上海市浦东新区xx路xx弄xx号</td></tr></table></body></html>
3.2 使用poi + pdfbox
public String excelToHtmlStr(String excelPath) throws Exception {FileInputStream fileInputStream = new FileInputStream(excelPath);try (Workbook workbook = WorkbookFactory.create(new File(excelPath))){DataFormatter dataFormatter = new DataFormatter();FormulaEvaluator formulaEvaluator = workbook.getCreationHelper().createFormulaEvaluator();org.apache.poi.ss.usermodel.Sheet sheet = workbook.getSheetAt(0);StringBuilder htmlStringBuilder = new StringBuilder();htmlStringBuilder.append("<html><head><title>Excel to HTML using Java and POI library</title>");htmlStringBuilder.append("<style>table, th, td { border: 1px solid black; }</style>");htmlStringBuilder.append("</head><body><table>");for (Row row : sheet) {htmlStringBuilder.append("<tr>");for (Cell cell : row) {CellType cellType = cell.getCellType();if (cellType == CellType.FORMULA) {formulaEvaluator.evaluateFormulaCell(cell);cellType = cell.getCachedFormulaResultType();}String cellValue = dataFormatter.formatCellValue(cell, formulaEvaluator);htmlStringBuilder.append("<td>").append(cellValue).append("</td>");}htmlStringBuilder.append("</tr>");}htmlStringBuilder.append("</table></body></html>");return htmlStringBuilder.toString();}}
3.3 使用spire
public String excelToHtmlStr(String excelPath) throws Exception {try(ByteArrayOutputStream outputStream = new ByteArrayOutputStream()) {Workbook workbook = new Workbook();workbook.loadFromFile(excelPath);workbook.saveToStream(outputStream, com.spire.xls.FileFormat.HTML);return outputStream.toString();}}
三、将文件转换成html,并生成html文件
有时我们是需要的不仅仅返回html字符串,而是需要生成一个html文件这时应该怎么做呢?一个改动量小的做法就是使用org.apache.commons.io包下的FileUtils工具类写入目标地址:
FileUtils类将html字符串生成html文件示例:
首先需要引入pom:
<dependency><groupId>commons-io</groupId><artifactId>commons-io</artifactId><version>2.8.0</version></dependency>
相关代码:
String htmlStr = FileConvertUtil.pdfToHtmlStr("D:\\书籍\\电子书\\小说\\历史小说\\最后的可汗.doc");
FileUtils.write(new File("D:\\test\\doc.html"), htmlStr, "utf-8");
除此之外,还可以对上面的代码进行一些调整,已实现生成html文件,代码调整如下:
1、将word文件转换成html文件
word原文件效果:
1.1 使用aspose
public static void wordToHtml(String wordPath, String htmlPath) {try {File sourceFile = new File(wordPath);String path = htmlPath + File.separator + sourceFile.getName().substring(0, sourceFile.getName().lastIndexOf(".")) + ".html";File file = new File(path); // 新建一个空白pdf文档FileOutputStream os = new FileOutputStream(file);Document doc = new Document(wordPath); // Address是将要被转化的word文档HtmlSaveOptions options = new HtmlSaveOptions();options.setExportImagesAsBase64(true);options.setExportRelativeFontSize(true);doc.save(os, options);} catch (Exception e) {e.printStackTrace();}}
转换成html的效果:
1.2 使用poi + pdfbox
public void wordToHtml(String wordPath, String htmlPath) throws TransformerException, IOException, ParserConfigurationException {htmlPath = FileUtil.getNewFileFullPath(wordPath, htmlPath, "html");String ext = wordPath.substring(wordPath.lastIndexOf("."));if (ext.equals(".docx")) {word2007ToHtml(wordPath, htmlPath);} else if (ext.equals(".doc")){word2003ToHtml(wordPath, htmlPath);} else {throw new RuntimeException("文件格式不正确");}}public void word2007ToHtml(String wordPath, String htmlPath) throws TransformerException, IOException, ParserConfigurationException {//try(OutputStream out = Files.newOutputStream(Paths.get(path))){try(FileOutputStream out = new FileOutputStream(htmlPath)){word2007ToHtmlOutputStream(wordPath, out);}}private void word2007ToHtmlOutputStream(String wordPath,OutputStream out) throws IOException {ZipSecureFile.setMinInflateRatio(-1.0d);InputStream in = Files.newInputStream(Paths.get(wordPath));XWPFDocument document = new XWPFDocument(in);XHTMLOptions options = XHTMLOptions.create().setIgnoreStylesIfUnused(false).setImageManager(new Base64EmbedImgManager());// 使用内存输出流XHTMLConverter.getInstance().convert(document, out, options);}public void word2003ToHtml(String wordPath, String htmlPath) throws TransformerException, IOException, ParserConfigurationException {org.w3c.dom.Document htmlDocument = word2003ToHtmlDocument(wordPath);// 生成html文件地址try(OutputStream outStream = Files.newOutputStream(Paths.get(htmlPath))){DOMSource domSource = new DOMSource(htmlDocument);StreamResult streamResult = new StreamResult(outStream);TransformerFactory factory = TransformerFactory.newInstance();Transformer serializer = factory.newTransformer();serializer.setOutputProperty(OutputKeys.ENCODING, "utf-8");serializer.setOutputProperty(OutputKeys.INDENT, "yes");serializer.setOutputProperty(OutputKeys.METHOD, "html");serializer.transform(domSource, streamResult);}}private org.w3c.dom.Document word2003ToHtmlDocument(String wordPath) throws IOException, ParserConfigurationException {InputStream input = Files.newInputStream(Paths.get(wordPath));HWPFDocument wordDocument = new HWPFDocument(input);WordToHtmlConverter wordToHtmlConverter = new WordToHtmlConverter(DocumentBuilderFactory.newInstance().newDocumentBuilder().newDocument());wordToHtmlConverter.setPicturesManager((content, pictureType, suggestedName, widthInches, heightInches) -> {System.out.println(pictureType);if (PictureType.UNKNOWN.equals(pictureType)) {return null;}BufferedImage bufferedImage = ImgUtil.toImage(content);String base64Img = ImgUtil.toBase64(bufferedImage, pictureType.getExtension());// 带图片的word,则将图片转为base64编码,保存在一个页面中StringBuilder sb = (new StringBuilder(base64Img.length() + "data:;base64,".length()).append("data:;base64,").append(base64Img));return sb.toString();});// 解析word文档wordToHtmlConverter.processDocument(wordDocument);return wordToHtmlConverter.getDocument();}
转换成html的效果:
1.3 使用spire
public void wordToHtml(String wordPath, String htmlPath) {htmlPath = FileUtil.getNewFileFullPath(wordPath, htmlPath, "html");Document document = new Document();document.loadFromFile(wordPath);document.saveToFile(htmlPath, FileFormat.Html);}
转换成html的效果:
因为使用的是免费版,存在页数和字数限制,需要完整功能的的可以选择付费版本。PS:这回76页的文档居然转成功了前50页。
2、将pdf文件转换成html文件
图片版pdf原文件效果:
文字版pdf原文件效果:
2.1 使用aspose
public static void pdfToHtml(String pdfPath, String htmlPath) throws IOException, ParserConfigurationException {File file = new File(pdfPath);String path = htmlPath + File.separator + file.getName().substring(0, file.getName().lastIndexOf(".")) + ".html";PDDocument document = PDDocument.load(new File(pdfPath));Writer writer = new PrintWriter(path, "UTF-8");new PDFDomTree().writeText(document, writer);writer.close();document.close();}
图片版PDF文件验证结果:
文字版PDF文件验证结果:
2.2 使用poi + pdfbox
public void pdfToHtml(String pdfPath, String htmlPath) throws IOException, ParserConfigurationException {String path = FileUtil.getNewFileFullPath(pdfPath, htmlPath, "html");PDDocument document = PDDocument.load(new File(pdfPath));Writer writer = new PrintWriter(path, "UTF-8");new PDFDomTree().writeText(document, writer);writer.close();document.close();}
图片版PDF文件验证结果:
文字版PDF原文件效果:
2.3 使用spire
public void pdfToHtml(String pdfPath, String htmlPath) throws IOException, ParserConfigurationException {htmlPath = FileUtil.getNewFileFullPath(pdfPath, htmlPath, "html");PdfDocument pdf = new PdfDocument();pdf.loadFromFile(pdfPath);pdf.saveToFile(htmlPath, com.spire.pdf.FileFormat.HTML);}
图片版PDF文件验证结果:
因为使用的是免费版,所以只有前三页是正常的。。。有超过三页需求的可以选择付费版本。
文字版PDF原文件效果:
报错了无法转换。。。
java.lang.NullPointerExceptionat com.spire.pdf.PdfPageWidget.spr┢⅛(Unknown Source)at com.spire.pdf.PdfPageWidget.getSize(Unknown Source)at com.spire.pdf.PdfPageBase.spr†™—(Unknown Source)at com.spire.pdf.PdfPageBase.getActualSize(Unknown Source)at com.spire.pdf.PdfPageBase.getSection(Unknown Source)at com.spire.pdf.general.PdfDestination.spr︻┎—(Unknown Source)at com.spire.pdf.general.PdfDestination.spr┻┑—(Unknown Source)at com.spire.pdf.general.PdfDestination.getElement(Unknown Source)at com.spire.pdf.primitives.PdfDictionary.setProperty(Unknown Source)at com.spire.pdf.bookmarks.PdfBookmark.setDestination(Unknown Source)at com.spire.pdf.bookmarks.PdfBookmarkWidget.spr┭┘—(Unknown Source)at com.spire.pdf.bookmarks.PdfBookmarkWidget.getDestination(Unknown Source)at com.spire.pdf.PdfDocumentBase.spr╻⅝(Unknown Source)at com.spire.pdf.widget.PdfPageCollection.spr┦⅝(Unknown Source)at com.spire.pdf.widget.PdfPageCollection.removeAt(Unknown Source)at com.spire.pdf.PdfDocumentBase.spr┞⅝(Unknown Source)at com.spire.pdf.PdfDocument.loadFromFile(Unknown Source)
3、将excel文件转换成html文件
excel原文件效果:
3.1 使用aspose
public void excelToHtml(String excelPath, String htmlPath) throws Exception {htmlPath = FileUtil.getNewFileFullPath(excelPath, htmlPath, "html");Workbook workbook = new Workbook(excelPath);com.aspose.cells.HtmlSaveOptions options = new com.aspose.cells.HtmlSaveOptions();workbook.save(htmlPath, options);}
转换成html的效果:
3.2 使用poi
public void excelToHtml(String excelPath, String htmlPath) throws Exception {String path = FileUtil.getNewFileFullPath(excelPath, htmlPath, "html");try(FileOutputStream fileOutputStream = new FileOutputStream(path)){String htmlStr = excelToHtmlStr(excelPath);byte[] bytes = htmlStr.getBytes();fileOutputStream.write(bytes);}}public String excelToHtmlStr(String excelPath) throws Exception {FileInputStream fileInputStream = new FileInputStream(excelPath);try (Workbook workbook = WorkbookFactory.create(new File(excelPath))){DataFormatter dataFormatter = new DataFormatter();FormulaEvaluator formulaEvaluator = workbook.getCreationHelper().createFormulaEvaluator();org.apache.poi.ss.usermodel.Sheet sheet = workbook.getSheetAt(0);StringBuilder htmlStringBuilder = new StringBuilder();htmlStringBuilder.append("<html><head><title>Excel to HTML using Java and POI library</title>");htmlStringBuilder.append("<style>table, th, td { border: 1px solid black; }</style>");htmlStringBuilder.append("</head><body><table>");for (Row row : sheet) {htmlStringBuilder.append("<tr>");for (Cell cell : row) {CellType cellType = cell.getCellType();if (cellType == CellType.FORMULA) {formulaEvaluator.evaluateFormulaCell(cell);cellType = cell.getCachedFormulaResultType();}String cellValue = dataFormatter.formatCellValue(cell, formulaEvaluator);htmlStringBuilder.append("<td>").append(cellValue).append("</td>");}htmlStringBuilder.append("</tr>");}htmlStringBuilder.append("</table></body></html>");return htmlStringBuilder.toString();}}
转换成html的效果:
3.3 使用spire
public void excelToHtml(String excelPath, String htmlPath) throws Exception {htmlPath = FileUtil.getNewFileFullPath(excelPath, htmlPath, "html");Workbook workbook = new Workbook();workbook.loadFromFile(excelPath);workbook.saveToFile(htmlPath, com.spire.xls.FileFormat.HTML);}
转换成html的效果:
四、总结
从上述的效果展示我们可以发现其实转成html效果不是太理想,很多细节样式没有还原,这其实是因为这类转换往往都是追求目标是通过使用文档中的语义信息并忽略其他细节来生成简单干净的 HTML,所以在转换过程中复杂样式被忽略,比如居中、首行缩进、字体,文本大小,颜色。举个例子在转换是 会将应用标题 1 样式的任何段落转换为 h1 元素,而不是尝试完全复制标题的样式。所以转成html的显示效果往往和原文档不太一样。这意味着对于较复杂的文档而言,这种转换不太可能是完美的。但如果都是只使用简单样式文档或者对文档样式不太关心的这种方式也不妨一试。
PS:如果想要展示效果好的话,其实可以将上篇文章《文档在线预览(一)通过将txt、word、pdf转成图片实现在线预览功能》说的内容和本文结合起来使用,即将文档里的内容都生成成图片(很可能是多张图片),然后将生成的图片全都放到一个html页面里 ,用html+css来保持样式并实现多张图片展示,再将html返回。开源组件kkfilevie就是用的就是这种做法。
kkfileview展示效果如下:
下图是kkfileview返回的html代码,从html代码我们可以看到kkfileview其实是将文件(txt文件除外)每页的内容都转成了图片,然后将这些图片都嵌入到一个html里,再返回给用户一个html页面。
相关文章:
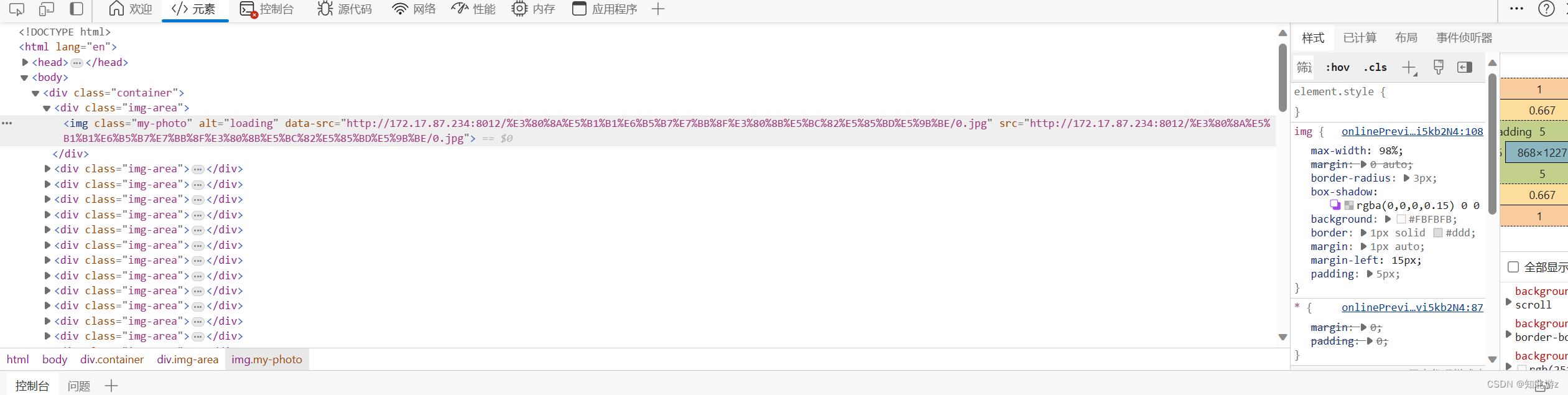
文档在线预览word、pdf、excel文件转html以实现文档在线预览
目录 一、前言 1、aspose2 、poi pdfbox3 spire二、将文件转换成html字符串 1、将word文件转成html字符串 1.1 使用aspose1.2 使用poi1.3 使用spire2、将pdf文件转成html字符串 2.1 使用aspose2.2 使用 poi pbfbox2.3 使用spire3、将excel文件转成html字符串 3.1 使用aspose…...

FFmpeg视音频分离器----向雷神学习
雷神博客地址:https://blog.csdn.net/leixiaohua1020/article/details/39767055 本程序可以将封装格式中的视频码流数据和音频码流数据分离出来。 在该例子中, 将FLV的文件分离得到H.264视频码流文件和MP3 音频码流文件。 注意: 这个是简化版…...

CentOS 8开启bbr
CentOS 8 默认内核版本为 4.18.x,内核版本高于4.9 就可以直接开启 BBR,所以CentOS 8 启用BBR非常简单不需要再去升级内核。 开启bbr echo "net.core.default_qdiscfq" >> /etc/sysctl.conf echo "net.ipv4.tcp_congestion_contro…...
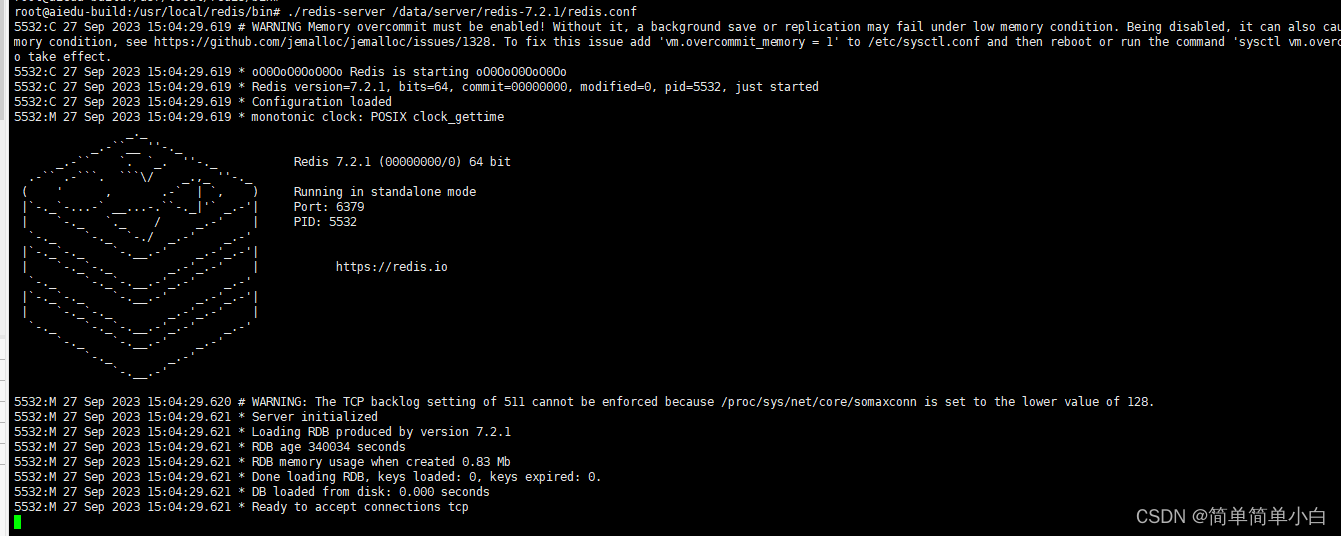
Redis的安装与基本使用
文章目录 Linux 环境下安装Redis下载Redis 安装包解压安装包安装Redis进入redis安装包下编译并且安装到指定目录下 启动redis配置远程访问找到Redis.config文件 Windows 环境下安装Redis说明官方提供方式安装或启用WSL2在WSL(Ubuntu)上安装Redis启动Redi…...
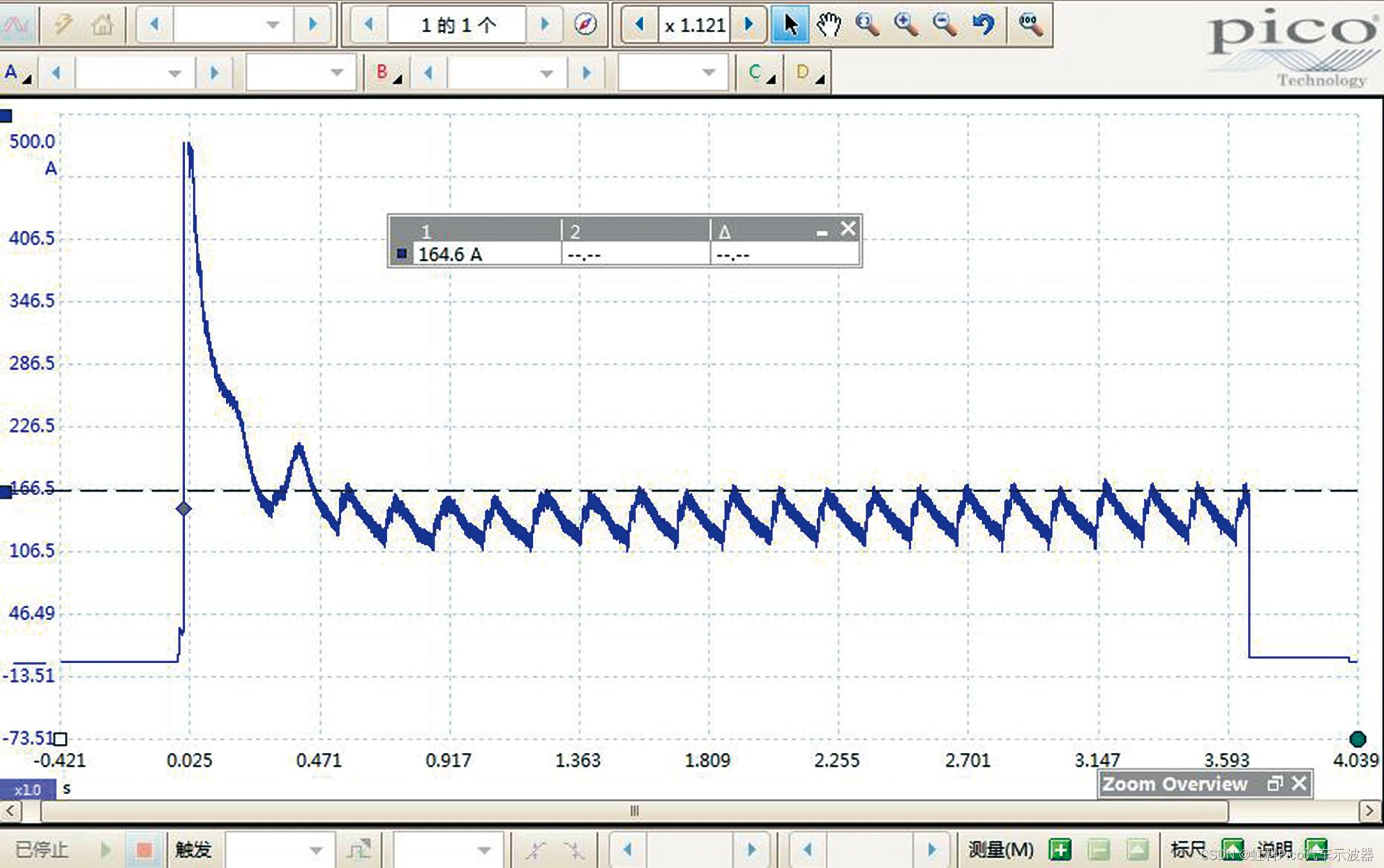
2014 款金旅牌小型客车 发动机怠速抖动、加速无力
故障现象 一辆2014款金旅牌小型客车,搭载JM491Q-ME发动机,累计行驶里程约为20万km。车主反映,最近该车发动机怠速抖动、加速无力,且经常缺少冷却液。 故障诊断 根据车主描述的故障现象,初步判断该车气缸垫损坏&#…...
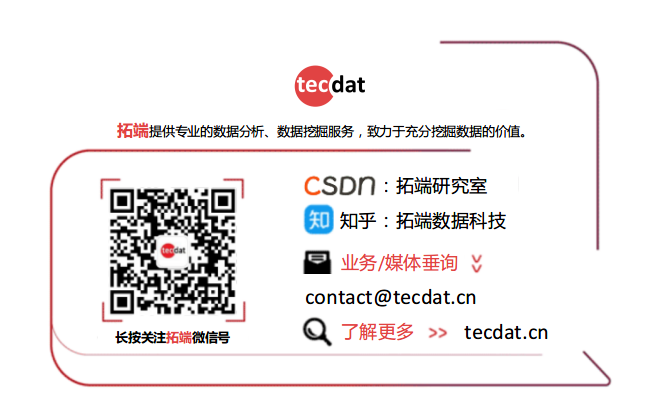
R语言逻辑回归、决策树、随机森林、神经网络预测患者心脏病数据混淆矩阵可视化...
全文链接:https://tecdat.cn/?p33760 众所周知,心脏疾病是目前全球最主要的死因。开发一个能够预测患者心脏疾病存在的计算系统将显著降低死亡率并大幅降低医疗保健成本。机器学习在全球许多领域中被广泛应用,尤其在医疗行业中越来越受欢迎。机器学习可…...

网站被劫持了怎么办
网站被劫持了怎么办 建议新建一个index.html文件,文件中只写几个数字,上传到网站根目录,然后访问网站域名,看看是不是正常,从而可以确定是程序问题还是域名被劫持的问题。 如果是域名被劫持,你可以登录你的…...

【面试题精讲】Java包装类缓存机制
有的时候博客内容会有变动,首发博客是最新的,其他博客地址可能会未同步,认准https://blog.zysicyj.top 首发博客地址[1] 面试题手册[2] 系列文章地址[3] 1. 什么是 Java 包装类缓存机制? Java 中的包装类(Wrapper Class)是为了将…...

网络相关知识
0 socket SOCK_DGRAM #无连接UDP SOCK_STREAM #面向连接TCP 1 UDP 1.1 检测UDP yum install -y nc 使用netcat测试连通性 服务器端启动 UDP 30003 端口 nc -l -u 30003 客户端连接服务器的30003端口(假设服务的IP地址是119.23.67.12) nc -u 119…...

商品冷启动推荐综述
About Me: LuckBoyPhd/Resume (github.com) (1)一种基于三部图网络的协同过滤算法 推荐系统是电子商务领域最重要的技术之一,而协同过滤算法又是推荐系统用得最广泛的.提出了一种基于加权三部图网络的协同过滤算法,用户、产品及标签都被考虑到算法中,并且研究了标签结点的度对…...
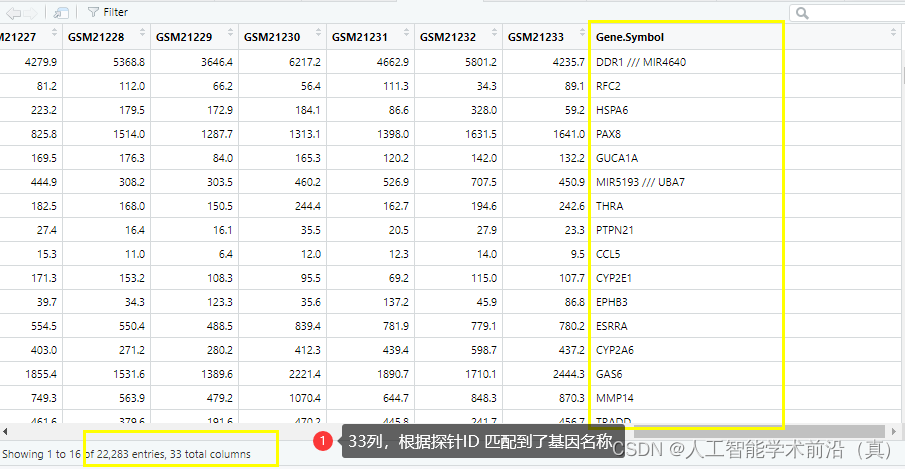
GEO生信数据挖掘(二)下载基因芯片平台文件及注释
检索到目标数据集后,开始数据挖掘,本文以阿尔兹海默症数据集GSE1297为例 目录 下载平台文件 1.AnnotGPL参数改为TRUE,联网下载芯片平台的soft文件。(国内网速奇慢经常中断) 2.手工去GEO官网下载 转换芯片探针ID为gene name 拓…...
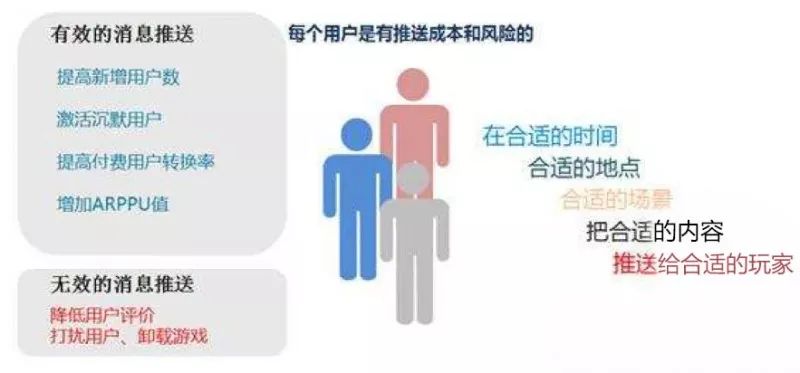
淘宝电商必备的大数据应用
在日常生活中,大家总能听到“大数据”“人工智能”的说法。现在的大数据技术应用,从大到巨大科学研究、社会信息审查、搜索引擎,小到社交联结、餐厅推荐等等,已经渗透到我们生活中的方方面面。到底大数据在电商行业可以怎么用&…...

Docker版部署RocketMQ开启ACL验证
一、拉取镜像 docker pull apache/rocketmq:latest 二、准备挂载目录 mkdir /usr/local/rocketmq/data mkdir /usr/local/rocketmq/conf 三、运行 docker run \ -d \ -p 9876:9876 \ -v /usr/local/rocketmq/data/logs:/home/rocketmq/logs \ -v /usr/local/rocketmq/data…...
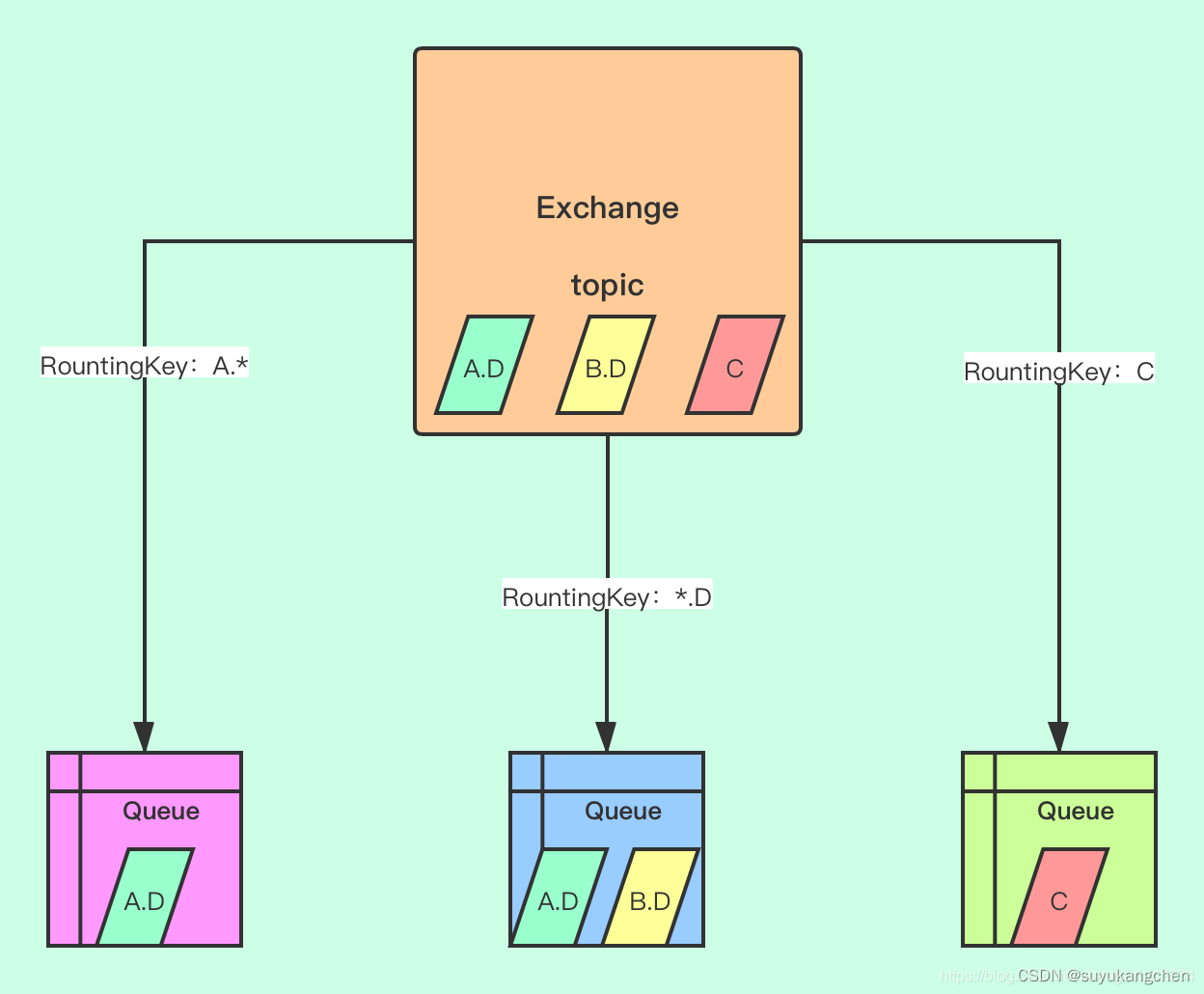
【RabbitMQ实战】04 RabbitMQ的基本概念:Exchange,Queue,Channel等
一、简介 Message Queue的需求由来已久,80年代最早在金融交易中,高盛等公司采用Teknekron公司的产品,当时的Message queuing软件叫做:the information bus(TIB)。 TIB被电信和通讯公司采用,路透…...

APACHE NIFI学习之—RouteOnAttribute
RouteOnAttribute 描述: 使用属性表达式语言根据其属性路由数据流,每个表达式必须返回Boolean类型的值(true或false)。 标签: attributes, routing, Attribute Expression Language, regexp, regex, Regular Expression, Expression Language, 属性, 路由, 表达式, 正则…...
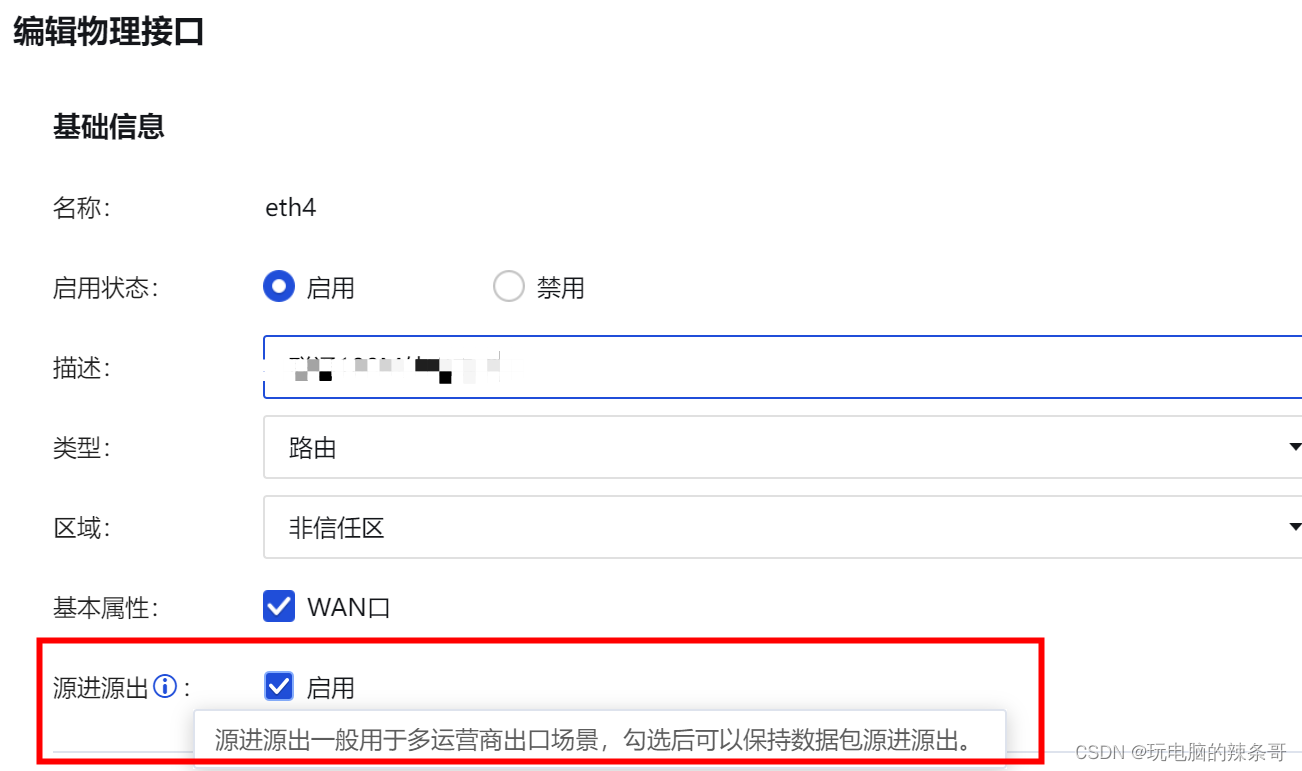
防火墙网络接口下面多个外网地址,只有第一地址可以访问通其他不通
环境: 主备防火墙 8.0.75 AF-2000-FH2130B-SC 问题描述: 两台防火墙双击热备,高可用防火墙虚拟网络接口Eth4下面有多个外网地址,只有第一地址可以访问通其他不通 解决方案: 1.检查防火墙路由设置(未解决…...
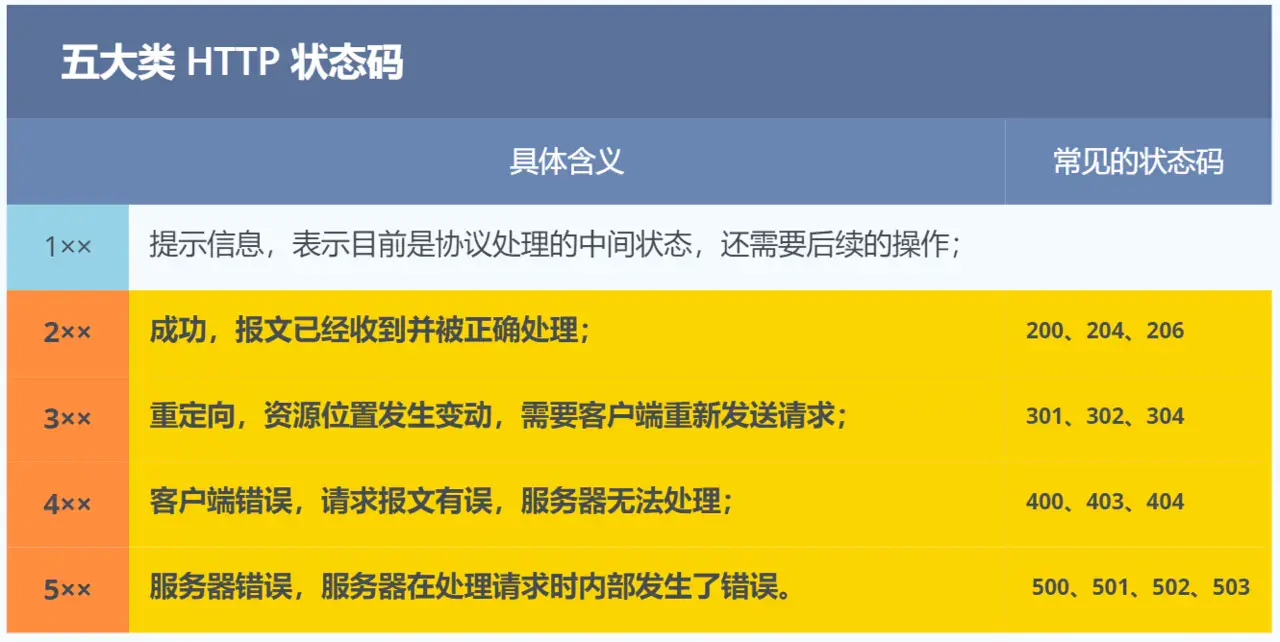
【HTTP】URL结构、HTTP请求和响应的报文格式、HTTP请求的方法、常见的状态码、GET和POST有什么区别、Cookie、Session等重点知识汇总
目录 URL格式 HTTP请求和响应报文的字段? HTTP请求方法 常见的状态码 GET 和 POST 的区别 Cookie 和 Session URL格式 ?:是用来分割URL的主体部分(通常是路径)和查询字符串(query string)…...
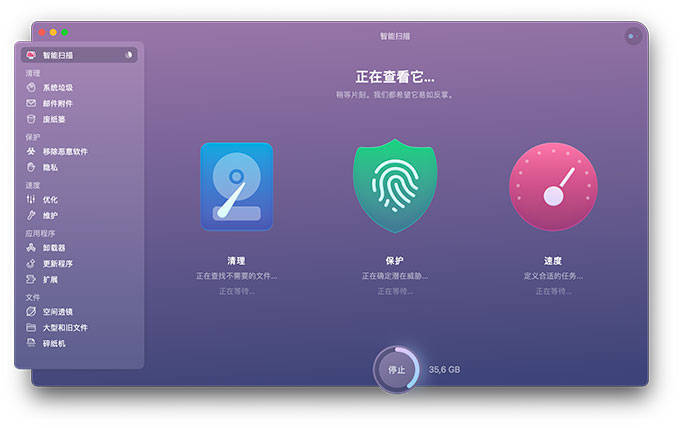
苹果mac电脑显示内存不足如何解决?
忍痛删应用、删文档、删照片视频等等一系列操作都是众多Mac用户清理内存空间的方法之一,悲催的是一顿“猛如虎的操作”下,释放出来的内存空间却少的可怜,原因很简单,这样释放内存空间是无效的。如何合理有效的清理内存空间&#x…...

如何在Windows 10上安装Go并搭建本地编程环境
引言 Go是在谷歌的挫折中诞生的编程语言。开发人员不得不不断地选择一种执行效率高但需要长时间编译的语言,或者选择一种易于编程但在生产环境中运行效率低的语言。Go被设计为同时提供这三种功能:快速编译、易于编程和在生产中高效执行。 虽然Go是一种通用的编程语…...
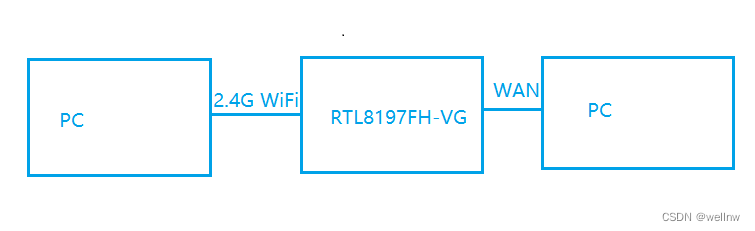
[Realtek sdk-3.4.14b]RTL8197FH-VG 2.4G to WAN吞吐量低于60%的问题分析及解决方案
问题描述 RTL8197FH-VG 2.4G wifi to WAN吞吐量低于65%的标准,正常2T2R的wifi 300Mbps x 65% = 195Mbps,但是实际只能跑到160Mbps,这个时候CPU的idl已经为0,sirq占用率达到98%左右 网络拓扑 一台PC通过2.4G WiFi连接到RTL8197FH-VG,另外一台PC直接通过WAN口连接到RTL8197…...
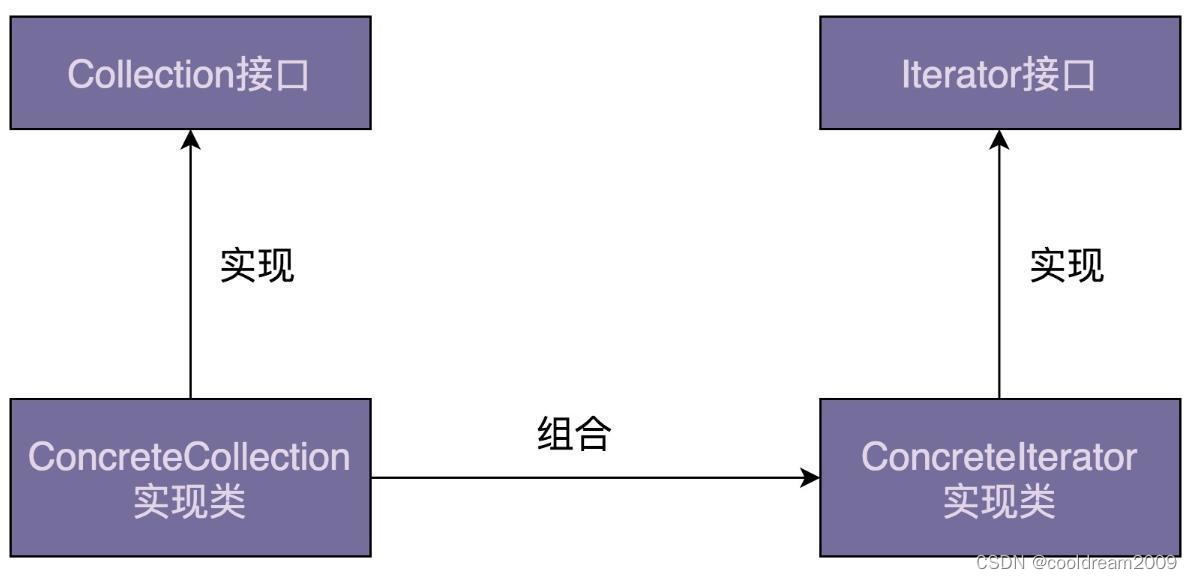
软件设计模式系列之十八——迭代器模式
1 模式的定义 迭代器模式是一种行为型设计模式,它允许客户端逐个访问一个聚合对象中的元素,而不暴露该对象的内部表示。迭代器模式提供了一种统一的方式来遍历不同类型的集合,使客户端代码更加简洁和可复用。 2 举例说明 为了更好地理解迭…...
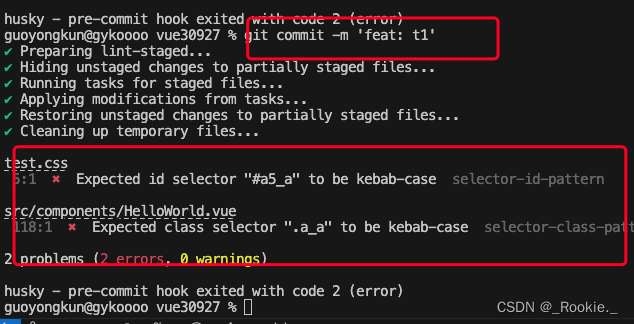
前端项目配置 prettier、eslint、lint-stages、husky、commitlint 、style-lint设置代码书写和提交规范
prettier中文网:Options Prettier 中文网 eslint中文网 :ESLint 中文网 github husky : https://github.com/typicode/husky commitlint.js 官网:commitlint - Lint commit messages 、github:GitHub - conventiona…...

如何开始着手一篇Meta分析 | Meta分析的流程及方法
Meta分析是针对某一科研问题,根据明确的搜索策略、选择筛选文献标准、采用严格的评价方法,对来源不同的研究成果进行收集、合并及定量统计分析的方法,最早出现于“循证医学”,现已广泛应用于农林生态,资源环境等方面。…...

【PID控制技术】
PID控制技术 简介控制原理相关术语调参技巧相互作用 相似算法与PWM对比 应用范围优缺点硬件支持 简介 PID控制是一种在工业过程控制中广泛应用的控制策略,其全称是比例-积分-微分(Proportional Integral Derivative)控制。它的基本原理是根据…...

docker openjdk:8-jdk-alpine 修改时区、添加字体
新建Dockerfile文件,制作新镜像 FROM openjdk:8-jdk-alpine 1、解决字体问题 RUN apk add --update ttf-dejavu fontconfig && rm -rf /var/cache/apk/* 2、解决时差问题 # 解决时差8小时问题ENV TZAsia/ShanghaiRUN ln -snf /usr/share/zoneinfo/$TZ /et…...
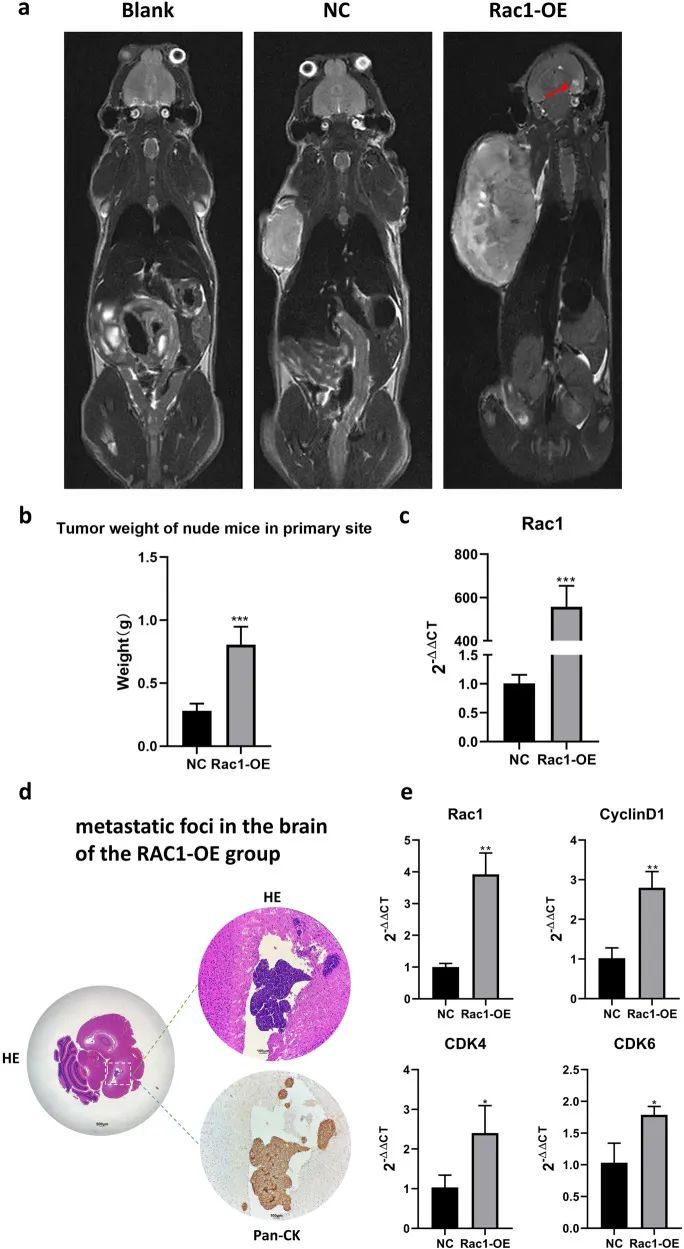
9+单细胞+实验验证,探讨单基因对癌细胞转移作用的思路方向
今天给同学们分享一篇单细胞实验的生信文章“Identification of RAC1 in promoting brain metastasis of lung adenocarcinoma using single-cell transcriptome sequencing”,这篇文章于2023年5月18日发表在Cell Death Dis期刊上,影响因子为9。 本研究旨…...
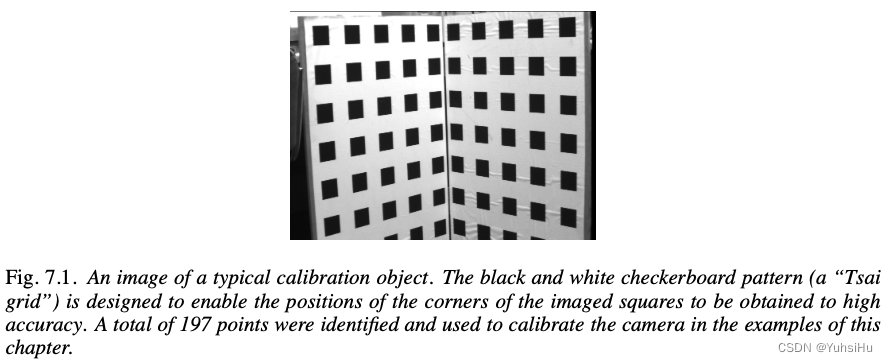
《计算机视觉中的多视图几何》笔记(7)
7 Computation of the Camera Matrix P P P 这章讲的是摄像机参数估计。摄像机标定,本质上就是求摄像机矩阵 P P P,当我们知道足够多的 X ↔ x X \leftrightarrow x X↔x,我们该如何计算 P P P?如果知道3D和2D点的对应ÿ…...
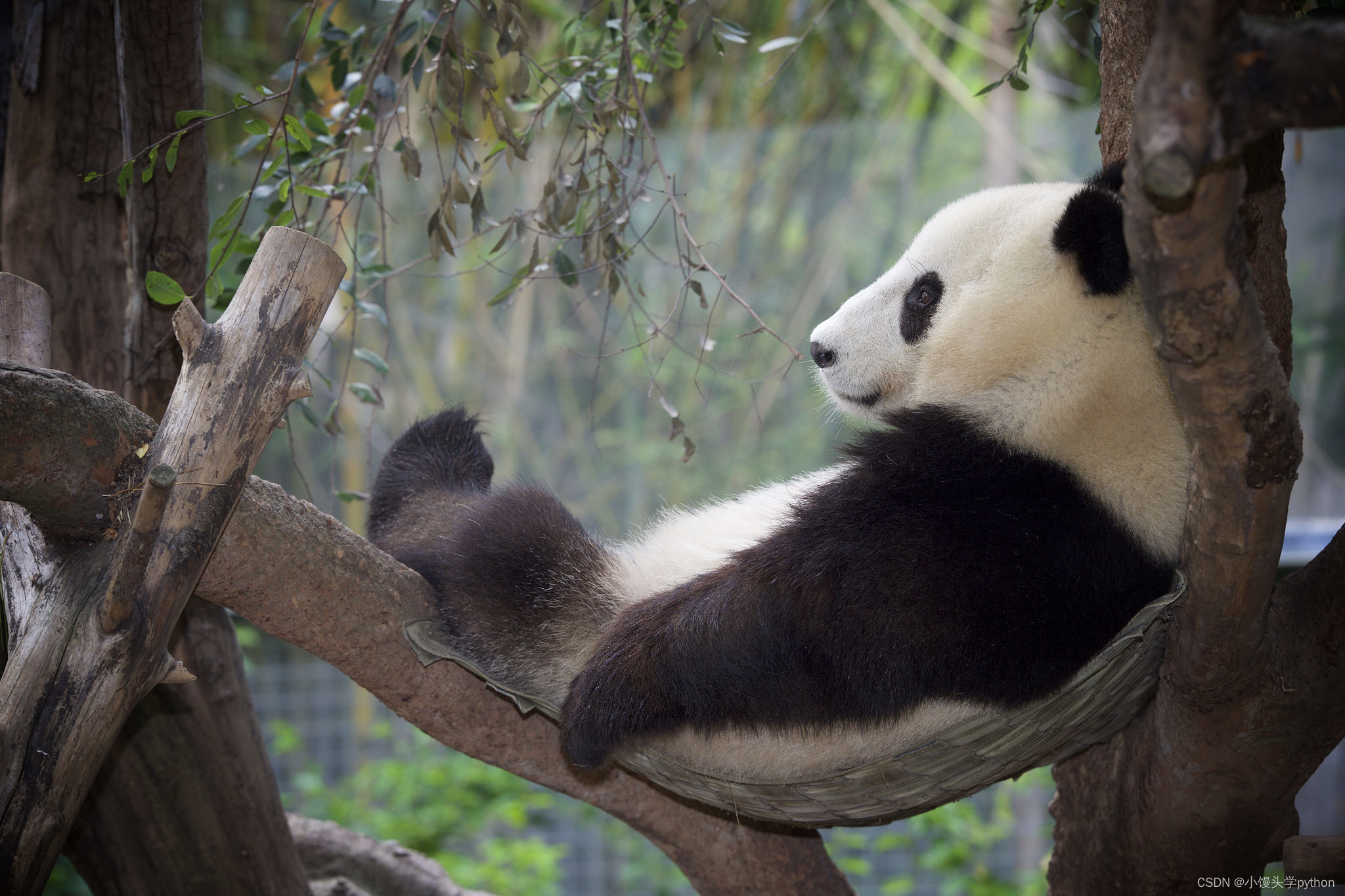
Python经典练习题(四)
文章目录 🍀第一题🍀第二题🍀第三题 🍀第一题 题目:打印出如下图案(菱形): 我们首先分析一下,本题实现的步骤主要有两个,分别是前四行和后三行 前四行:第一…...

Mac Pro在重装系统时提示“未能与恢复服务器取得联系”
检查网络连接: 确保你的Mac Pro连接到稳定的网络。尝试更换其他网络,例如切换到不同的Wi-Fi或使用有线连接。 系统时间校正: 错误的系统时间有时会导致与恢复服务器的连接问题。在恢复模式下打开终端(在实用工具菜单中选择终端&a…...

【C/C++】指针常量、常量指针、指向常量的常指针
目录 1.概念2. const pointer3. pointer to a constant3.1 (pointer to a constant)-constant3.2 poiner-constant3.3 (pointer to a constant)-variable3.4 poiner-variable3.5 多层级关系时的兼容3.6 用处 4. a constant pointer to a constant 1.概念 首先明确这几个术语的…...

无锡网站优化推广/百度爱采购
##中位数是有序列表中间的数。如果列表长度是偶数,中位数则是中间两个数的平均值。 例如, [2,3,4] 的中位数是 3 [2,3] 的中位数是 (2 3) / 2 2.5 设计一个支持以下两种操作的数据结构: void addNum(int num) - 从数据流中添加一个整数…...

如何建设学校网站/网站seo的内容是什么
统计Xcode项目 代码行数 1、打开终端。 2、cd到你项目的路径。 3、输入下面的指令: grep -d recurse "\n" classes | wc -l classes,如果你不是默认的classes路径,改之。效果:yifei-yumatoMacBook-Pro:QJSG V0.3.1.1 yuyifei2000$ …...
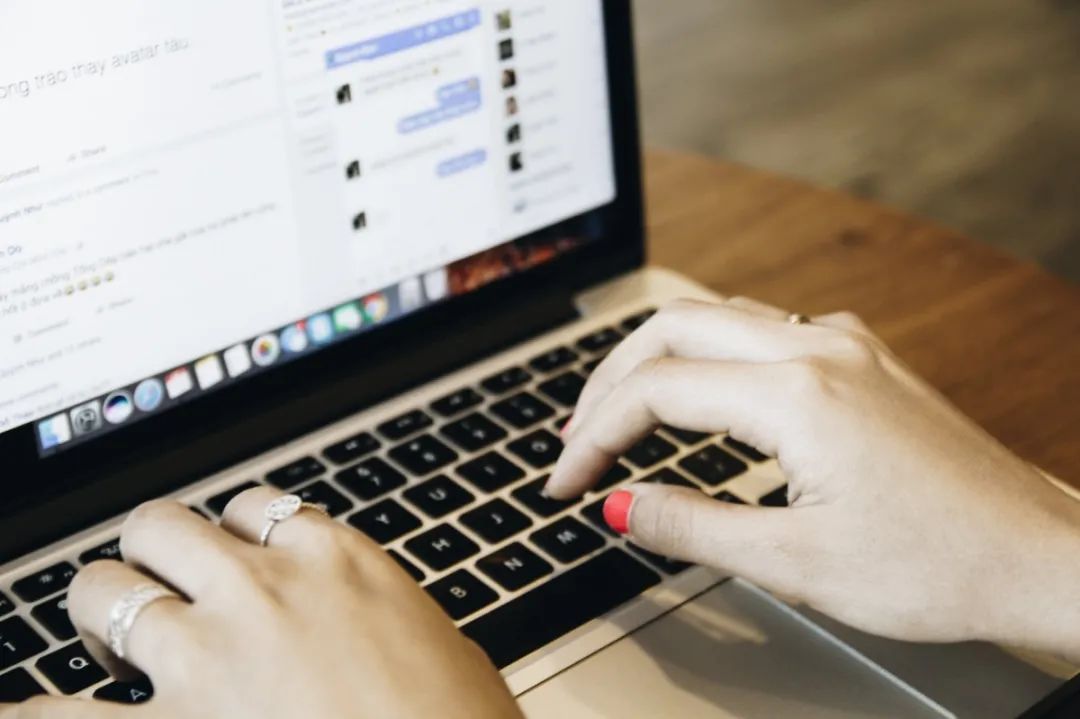
中小企业网站建设公司/百度做个人简介多少钱
作者 | 银川回民二小点文末“在看”,推荐给朋友导语:当大规模的在线教育铺开,区域教育的决策者急需对当下的实施情况进行把脉,以便做出更加科学的应对措施。一份成熟问卷设计,在此时显得尤为关键,为此&…...
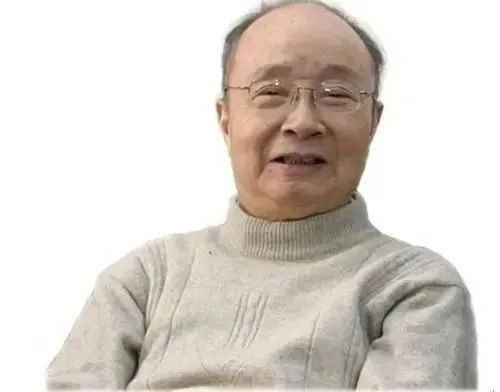
正规网站建设费用/谷歌seo零基础教程
来源 | 晓艳的科技坊本文仅用于学术分享,若有侵权,请联系删除一、中国核科学奠基人——王淦昌科学地位:中国核科学的奠基人和开拓者之一、中国科学院院士、两弹一星功勋奖章"获得者。世界激光惯性约束核聚变理论和研究的创始人之一。 突…...
网站服务器有什么区别/培训班该如何建站
JsoupJsoup 是一款轻量高效的 html 文档解析工具,可类比 xml 中的 dom4j 、jdomJsoup中的基本类DocumentHtml 整个文档在内容中的数据结构,继承 ElementElement标记元素的数据结构 ,继承 NodeElementsElement 的集合,继承 ArrayLi…...
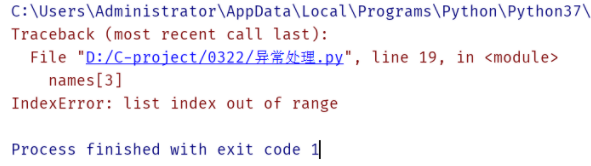
深圳宝安网站建设/宁波seo外包推广
Python异常处理中try与except用法的案例发布时间:2020-11-03 09:44:36来源:亿速云阅读:85作者:小新小编给大家分享一下Python异常处理中try与except用法的案例,希望大家阅读完这篇文章后大所收获,下面让我们…...