Spring源码分析 事务 实现原理
文章目录
- 什么是事务
- Spring事务管理
- Spring事务实现原理
- 事务管理器
- 事务定义
- 事务的开启
- 事务核心方法
- 业务代码使用事务
- TransactionInterceptor
什么是事务
一般所指的事务是数据库事务,是指一批不可分割的数据库操作序列,也是数据库并发控制的基本单位。其执行的结果必须使数据库从一种一致性状态到另一种一致性状态。事务必须满足4个基本特性,即ACID
(原子性、一致性、隔离性、持久性)。
Spring事务管理
Spring并不直接支持事务,只有当数据库支持事务时,Spring才支持。他只是通过AOP简化了开发人员使用事务的步骤。
Spring可通过xml和注解配置和一些关键类,确保bean中涉及数据库操作的方法执行符合事务的基本特性。
例如:一个业务调用了2个Service,他们分别操作了不同的数据表,必须确保这些数据一起成功或者一起失败。
Spring事务实现原理
首先介绍Spring中和事务实现密切相关的重要类。
事务管理器
事务管理器的顶层接口是TransactionManager。以下是他的类图。
PlatformTransactionManager:平台事务管理器
ReactiveTransactionManager:响应式编程的事务管理器
Spring中事务关注的重点是:PlatformTransactionManager,以及有他的实现类
JtaTransactionManager:支持分布式事务(单个服务中的多数据源,默认只有weblogic和websphere2个实现)
DataSourceTransactionManager:单数据源事务管理器。平时用的最多的就是这个,是分析的重点。
public interface PlatformTransactionManager extends TransactionManager {/*** 获取事务*/TransactionStatus getTransaction(@Nullable TransactionDefinition definition)throws TransactionException;/*** 提交数据*/void commit(TransactionStatus status) throws TransactionException;/*** 回滚数据*/void rollback(TransactionStatus status) throws TransactionException;}
事务定义
事务定义类为TransactionDefinition
,在事务管理器获取事务时作为参数传入。它定义了事务的 传播属性,隔离级别,超时时间,是否只读等属性。
public interface TransactionDefinition {/*** 支持当前事务,若当前没有事务就创建一个事务*/int PROPAGATION_REQUIRED = 0;/*** 如果当前存在事务,则加入该事务;如果当前没有事务,则以非事务的方式运行 */int PROPAGATION_SUPPORTS = 1;/*** 如果当前存在事务,则加入该事务;如果当前没有事务,则抛出异常*/int PROPAGATION_MANDATORY = 2;/*** 创建一个新的事务,如果当前存在事务,则把当前事务挂起*/int PROPAGATION_REQUIRES_NEW = 3;/*** 以非事务方式运行,如果当前存在事务,则把当前事务挂起*/int PROPAGATION_NOT_SUPPORTED = 4;/*** 以非事务方式运行,如果当前存在事务,则抛出异常*/int PROPAGATION_NEVER = 5;/*** 如果外层存在事务,就以嵌套事务运行,被嵌套的事务可以独立于外层事务进行提交或者回滚(保存点),* 如果外层不存在事务,行为跟PROPAGATION_REQUIRES_NEW*/int PROPAGATION_NESTED = 6;/*** 使用数据库默认的隔离级别*/int ISOLATION_DEFAULT = -1;/*** 读未提交*/int ISOLATION_READ_UNCOMMITTED = 1; // same as java.sql.Connection.TRANSACTION_READ_UNCOMMITTED;/*** 读已提交*/int ISOLATION_READ_COMMITTED = 2; // same as java.sql.Connection.TRANSACTION_READ_COMMITTED;/*** 可重复读*/int ISOLATION_REPEATABLE_READ = 4; // same as java.sql.Connection.TRANSACTION_REPEATABLE_READ;/*** 可串行化*/int ISOLATION_SERIALIZABLE = 8; // same as java.sql.Connection.TRANSACTION_SERIALIZABLE;/*** 使用默认的超时时间*/int TIMEOUT_DEFAULT = -1;/*** 获取事务的传播行为*/default int getPropagationBehavior() {return PROPAGATION_REQUIRED;}/*** 获取事务的隔离级别*/default int getIsolationLevel() {return ISOLATION_DEFAULT;}/*** 获取事务的超时时间*/default int getTimeout() {return TIMEOUT_DEFAULT;}/*** 是否为只读事务*/default boolean isReadOnly() {return false;}/*** 获取当前事务的名称*/@Nullabledefault String getName() {return null;}static TransactionDefinition withDefaults() {return StaticTransactionDefinition.INSTANCE;}}
TransactionDefinition的体系结构
DefaultTransactionDefinition:是事务定义的默认实现
DefaultTransactionAttribute:扩展了TransactionAttribute中的属性的实现
@Transactional:该组件会被解析加载为对应的 TransactionDefinition
对象。
事务的开启
在PlatformTransactionManager
中获取事务的时候返回的是TransactionStatus
对象。我们来看看这个对象。
子类中扩展了
事务核心方法
事务核心方法: AbstractPlatformTransactionManager.getTransaction()
/*** This implementation handles propagation behavior. Delegates to* {@code doGetTransaction}, {@code isExistingTransaction}* and {@code doBegin}.* @see #doGetTransaction* @see #isExistingTransaction* @see #doBegin*/@Overridepublic final TransactionStatus getTransaction(@Nullable TransactionDefinition definition)throws TransactionException {// Use defaults if no transaction definition given.// 如果没有事务定义信息则使用默认的事务管理器定义信息TransactionDefinition def = (definition != null ? definition : TransactionDefinition.withDefaults());// 获取事务Object transaction = doGetTransaction();boolean debugEnabled = logger.isDebugEnabled();// 判断当前线程是否存在事务,判断依据为当前线程记录的连接不为空且连接中的transactionActive属性不为空if (isExistingTransaction(transaction)) {// Existing transaction found -> check propagation behavior to find out how to behave.// 当前线程已经存在事务return handleExistingTransaction(def, transaction, debugEnabled);}// Check definition settings for new transaction.// 事务超时设置验证if (def.getTimeout() < TransactionDefinition.TIMEOUT_DEFAULT) {throw new InvalidTimeoutException("Invalid transaction timeout", def.getTimeout());}// No existing transaction found -> check propagation behavior to find out how to proceed.// 如果当前线程不存在事务,但是PropagationBehavior却被声明为PROPAGATION_MANDATORY抛出异常if (def.getPropagationBehavior() == TransactionDefinition.PROPAGATION_MANDATORY) {throw new IllegalTransactionStateException("No existing transaction found for transaction marked with propagation 'mandatory'");}// PROPAGATION_REQUIRED,PROPAGATION_REQUIRES_NEW,PROPAGATION_NESTED都需要新建事务else if (def.getPropagationBehavior() == TransactionDefinition.PROPAGATION_REQUIRED ||def.getPropagationBehavior() == TransactionDefinition.PROPAGATION_REQUIRES_NEW ||def.getPropagationBehavior() == TransactionDefinition.PROPAGATION_NESTED) {//没有当前事务的话,REQUIRED,REQUIRES_NEW,NESTED挂起的是空事务,然后创建一个新事务SuspendedResourcesHolder suspendedResources = suspend(null);if (debugEnabled) {logger.debug("Creating new transaction with name [" + def.getName() + "]: " + def);}try {return startTransaction(def, transaction, debugEnabled, suspendedResources);}catch (RuntimeException | Error ex) {// 恢复挂起的事务resume(null, suspendedResources);throw ex;}}else {// Create "empty" transaction: no actual transaction, but potentially synchronization.// 创建一个空的事务if (def.getIsolationLevel() != TransactionDefinition.ISOLATION_DEFAULT && logger.isWarnEnabled()) {logger.warn("Custom isolation level specified but no actual transaction initiated; " +"isolation level will effectively be ignored: " + def);}boolean newSynchronization = (getTransactionSynchronization() == SYNCHRONIZATION_ALWAYS);return prepareTransactionStatus(def, null, true, newSynchronization, debugEnabled, null);}}
其中关键的方法:doGetTransaction()方法,有很多不同的实现类。我们来看下DataSourceTransactionManager的
/*** 创建一个DataSourceTransactionObject当作事务,设置是否允许保存点,然后获取连接持有器ConnectionHolder* 里面会存放JDBC的连接,设置给DataSourceTransactionObject,当然第一次是空的** @return*/@Overrideprotected Object doGetTransaction() {// 创建一个数据源事务对象DataSourceTransactionObject txObject = new DataSourceTransactionObject();// 是否允许当前事务设置保持点txObject.setSavepointAllowed(isNestedTransactionAllowed());/*** TransactionSynchronizationManager 事务同步管理器对象(该类中都是局部线程变量)* 用来保存当前事务的信息,我们第一次从这里去线程变量中获取 事务连接持有器对象 通过数据源为key去获取* 由于第一次进来开始事务 我们的事务同步管理器中没有被存放.所以此时获取出来的conHolder为null*/ConnectionHolder conHolder =(ConnectionHolder) TransactionSynchronizationManager.getResource(obtainDataSource());// 非新创建连接则写falsetxObject.setConnectionHolder(conHolder, false);// 返回事务对象return txObject;}
然后事务管理的代码
/*** Create a TransactionStatus for an existing transaction.*/private TransactionStatus handleExistingTransaction(TransactionDefinition definition, Object transaction, boolean debugEnabled)throws TransactionException {/*** 判断当前的事务行为是不是PROPAGATION_NEVER的* 表示为不支持事务,但是当前又存在一个事务,所以抛出异常*/if (definition.getPropagationBehavior() == TransactionDefinition.PROPAGATION_NEVER) {throw new IllegalTransactionStateException("Existing transaction found for transaction marked with propagation 'never'");}/*** 判断当前的事务属性不支持事务,PROPAGATION_NOT_SUPPORTED,所以需要先挂起已经存在的事务*/if (definition.getPropagationBehavior() == TransactionDefinition.PROPAGATION_NOT_SUPPORTED) {if (debugEnabled) {logger.debug("Suspending current transaction");}// 挂起当前事务Object suspendedResources = suspend(transaction);boolean newSynchronization = (getTransactionSynchronization() == SYNCHRONIZATION_ALWAYS);// 创建一个新的非事务状态(保存了上一个存在事务状态的属性)return prepareTransactionStatus(definition, null, false, newSynchronization, debugEnabled, suspendedResources);}/*** 当前的事务属性状态是PROPAGATION_REQUIRES_NEW表示需要新开启一个事务状态*/if (definition.getPropagationBehavior() == TransactionDefinition.PROPAGATION_REQUIRES_NEW) {if (debugEnabled) {logger.debug("Suspending current transaction, creating new transaction with name [" +definition.getName() + "]");}// 挂起当前事务并返回挂起的资源持有器SuspendedResourcesHolder suspendedResources = suspend(transaction);try {// 创建一个新的非事务状态(保存了上一个存在事务状态的属性)return startTransaction(definition, transaction, debugEnabled, suspendedResources);}catch (RuntimeException | Error beginEx) {resumeAfterBeginException(transaction, suspendedResources, beginEx);throw beginEx;}}// 嵌套事务if (definition.getPropagationBehavior() == TransactionDefinition.PROPAGATION_NESTED) {// 不允许就报异常if (!isNestedTransactionAllowed()) {throw new NestedTransactionNotSupportedException("Transaction manager does not allow nested transactions by default - " +"specify 'nestedTransactionAllowed' property with value 'true'");}if (debugEnabled) {logger.debug("Creating nested transaction with name [" + definition.getName() + "]");}// 嵌套事务的处理if (useSavepointForNestedTransaction()) {// Create savepoint within existing Spring-managed transaction,// through the SavepointManager API implemented by TransactionStatus.// Usually uses JDBC 3.0 savepoints. Never activates Spring synchronization.// 如果没有可以使用保存点的方式控制事务回滚,那么在嵌入式事务的建立初始简历保存点DefaultTransactionStatus status =prepareTransactionStatus(definition, transaction, false, false, debugEnabled, null);// 为事务设置一个回退点status.createAndHoldSavepoint();return status;}else {// Nested transaction through nested begin and commit/rollback calls.// Usually only for JTA: Spring synchronization might get activated here// in case of a pre-existing JTA transaction.// 有些情况是不能使用保存点操作return startTransaction(definition, transaction, debugEnabled, null);}}// Assumably PROPAGATION_SUPPORTS or PROPAGATION_REQUIRED.if (debugEnabled) {logger.debug("Participating in existing transaction");}if (isValidateExistingTransaction()) {if (definition.getIsolationLevel() != TransactionDefinition.ISOLATION_DEFAULT) {Integer currentIsolationLevel = TransactionSynchronizationManager.getCurrentTransactionIsolationLevel();if (currentIsolationLevel == null || currentIsolationLevel != definition.getIsolationLevel()) {Constants isoConstants = DefaultTransactionDefinition.constants;throw new IllegalTransactionStateException("Participating transaction with definition [" +definition + "] specifies isolation level which is incompatible with existing transaction: " +(currentIsolationLevel != null ?isoConstants.toCode(currentIsolationLevel, DefaultTransactionDefinition.PREFIX_ISOLATION) :"(unknown)"));}}if (!definition.isReadOnly()) {if (TransactionSynchronizationManager.isCurrentTransactionReadOnly()) {throw new IllegalTransactionStateException("Participating transaction with definition [" +definition + "] is not marked as read-only but existing transaction is");}}}boolean newSynchronization = (getTransactionSynchronization() != SYNCHRONIZATION_NEVER);return prepareTransactionStatus(definition, transaction, false, newSynchronization, debugEnabled, null);}
最后来看看 startTransaction() 方法
/*** Start a new transaction.*/private TransactionStatus startTransaction(TransactionDefinition definition, Object transaction,boolean debugEnabled, @Nullable SuspendedResourcesHolder suspendedResources) {// 是否需要新同步boolean newSynchronization = (getTransactionSynchronization() != SYNCHRONIZATION_NEVER);// 创建新的事务DefaultTransactionStatus status = newTransactionStatus(definition, transaction, true, newSynchronization, debugEnabled, suspendedResources);// 开启事务和连接doBegin(transaction, definition);// 新同步事务的设置,针对于当前线程的设置prepareSynchronization(status, definition);return status;}
doBegin方法开启和连接事务
@Overrideprotected void doBegin(Object transaction, TransactionDefinition definition) {// 强制转化事务对象DataSourceTransactionObject txObject = (DataSourceTransactionObject) transaction;Connection con = null;try {// 判断事务对象没有数据库连接持有器if (!txObject.hasConnectionHolder() ||txObject.getConnectionHolder().isSynchronizedWithTransaction()) {// 通过数据源获取一个数据库连接对象Connection newCon = obtainDataSource().getConnection();if (logger.isDebugEnabled()) {logger.debug("Acquired Connection [" + newCon + "] for JDBC transaction");}// 把我们的数据库连接包装成一个ConnectionHolder对象 然后设置到我们的txObject对象中去txObject.setConnectionHolder(new ConnectionHolder(newCon), true);}// 标记当前的连接是一个同步事务txObject.getConnectionHolder().setSynchronizedWithTransaction(true);con = txObject.getConnectionHolder().getConnection();// 为当前的事务设置隔离级别Integer previousIsolationLevel = DataSourceUtils.prepareConnectionForTransaction(con, definition);// 设置先前隔离级别txObject.setPreviousIsolationLevel(previousIsolationLevel);// 设置是否只读txObject.setReadOnly(definition.isReadOnly());// Switch to manual commit if necessary. This is very expensive in some JDBC drivers,// so we don't want to do it unnecessarily (for example if we've explicitly// configured the connection pool to set it already).// 关闭自动提交if (con.getAutoCommit()) {//设置需要恢复自动提交txObject.setMustRestoreAutoCommit(true);if (logger.isDebugEnabled()) {logger.debug("Switching JDBC Connection [" + con + "] to manual commit");}// 关闭自动提交con.setAutoCommit(false);}// 判断事务是否需要设置为只读事务prepareTransactionalConnection(con, definition);// 标记激活事务txObject.getConnectionHolder().setTransactionActive(true);// 设置事务超时时间int timeout = determineTimeout(definition);if (timeout != TransactionDefinition.TIMEOUT_DEFAULT) {txObject.getConnectionHolder().setTimeoutInSeconds(timeout);}// Bind the connection holder to the thread.// 绑定我们的数据源和连接到我们的同步管理器上,把数据源作为key,数据库连接作为value 设置到线程变量中if (txObject.isNewConnectionHolder()) {// 将当前获取到的连接绑定到当前线程TransactionSynchronizationManager.bindResource(obtainDataSource(), txObject.getConnectionHolder());}}catch (Throwable ex) {if (txObject.isNewConnectionHolder()) {// 释放数据库连接DataSourceUtils.releaseConnection(con, obtainDataSource());txObject.setConnectionHolder(null, false);}throw new CannotCreateTransactionException("Could not open JDBC Connection for transaction", ex);}}
注意:在上述doBegin方法源码中的第42行关闭了自动提交,同时第60行把连接绑定到本地线程中bindResource方法
业务代码使用事务
业务代码使用事务的方式有如下2种,一种是编程式事务,另一种是AOP事务。
编程式事务
编程式事务要求开发人员手动使用事务管理器,创建事务,开始事务和结束事务。Spring只需要提前配置并实例化好事务管理器Bean,然后注入到Service中即可。参考如下案例代码:insertUser()方法为主要业务方法,其中调用了2个方法分别负责插入用户和插入业务日志。
@Autowiredprivate UserDao userDao;@Autowiredprivate PlatformTransactionManager txManager;@Autowiredprivate LogService logService;@Transactionalpublic void insertUser(User u) {// 1、创建事务定义DefaultTransactionDefinition definition = new DefaultTransactionDefinition();// 2、根据定义开启事务TransactionStatus status = txManager.getTransaction(definition);try {this.userDao.insert(u);Log log = new Log(System.currentTimeMillis() + "", System.currentTimeMillis() + "-" + u.getUserName());// this.doAddUser(u);this.logService.insertLog(log);// 3、提交事务txManager.commit(status);} catch (Exception e) {// 4、异常了,回滚事务txManager.rollback(status);throw e;}}
AOP事务
接下来是AOP事务,给事务方法加上@Transactional注解之后。来看看在Spring中这块是如何处理的。
通过Debug的方式可以看到,在调用@Transactional标记的方法之前,Spring自动创建了Cglib代理对象,调用的是代理对象的insertUser方法,处理的关键流程 org.springframework.transaction.interceptor.TransactionInterceptor
就是事务处理的Advisor。下面是的他的invoke方法。
@Override@Nullablepublic Object invoke(MethodInvocation invocation) throws Throwable {// Work out the target class: may be {@code null}.// The TransactionAttributeSource should be passed the target class// as well as the method, which may be from an interface.Class<?> targetClass = (invocation.getThis() != null ? AopUtils.getTargetClass(invocation.getThis()) : null);// Adapt to TransactionAspectSupport's invokeWithinTransaction...return invokeWithinTransaction(invocation.getMethod(), targetClass, invocation::proceed);}
进入到TransactionAspectSupport.invokeWithinTransaction()方法中,其中第46行,会调用具体的业务方法完成事务操作。
@Nullableprotected Object invokeWithinTransaction(Method method, @Nullable Class<?> targetClass,final InvocationCallback invocation) throws Throwable {// If the transaction attribute is null, the method is non-transactional.// 获取我们的事务属性源对象TransactionAttributeSource tas = getTransactionAttributeSource();// 通过事务属性源对象获取到当前方法的事务属性信息final TransactionAttribute txAttr = (tas != null ? tas.getTransactionAttribute(method, targetClass) : null);// 获取我们配置的事务管理器对象final TransactionManager tm = determineTransactionManager(txAttr);if (this.reactiveAdapterRegistry != null && tm instanceof ReactiveTransactionManager) {ReactiveTransactionSupport txSupport = this.transactionSupportCache.computeIfAbsent(method, key -> {if (KotlinDetector.isKotlinType(method.getDeclaringClass()) && KotlinDelegate.isSuspend(method)) {throw new TransactionUsageException("Unsupported annotated transaction on suspending function detected: " + method +". Use TransactionalOperator.transactional extensions instead.");}ReactiveAdapter adapter = this.reactiveAdapterRegistry.getAdapter(method.getReturnType());if (adapter == null) {throw new IllegalStateException("Cannot apply reactive transaction to non-reactive return type: " +method.getReturnType());}return new ReactiveTransactionSupport(adapter);});return txSupport.invokeWithinTransaction(method, targetClass, invocation, txAttr, (ReactiveTransactionManager) tm);}PlatformTransactionManager ptm = asPlatformTransactionManager(tm);// 获取连接点的唯一标识 类名+方法名final String joinpointIdentification = methodIdentification(method, targetClass, txAttr);// 声明式事务处理if (txAttr == null || !(ptm instanceof CallbackPreferringPlatformTransactionManager)) {// Standard transaction demarcation with getTransaction and commit/rollback calls.// 创建TransactionInfoTransactionInfo txInfo = createTransactionIfNecessary(ptm, txAttr, joinpointIdentification);Object retVal;try {// This is an around advice: Invoke the next interceptor in the chain.// This will normally result in a target object being invoked.// 执行被增强方法,调用具体的业务处理逻辑retVal = invocation.proceedWithInvocation();}catch (Throwable ex) {// target invocation exception// 异常回滚completeTransactionAfterThrowing(txInfo, ex);throw ex;}finally {//清除事务信息,恢复线程私有的老的事务信息cleanupTransactionInfo(txInfo);}if (retVal != null && vavrPresent && VavrDelegate.isVavrTry(retVal)) {// Set rollback-only in case of Vavr failure matching our rollback rules...TransactionStatus status = txInfo.getTransactionStatus();if (status != null && txAttr != null) {retVal = VavrDelegate.evaluateTryFailure(retVal, txAttr, status);}}//成功后提交,会进行资源储量,连接释放,恢复挂起事务等操作commitTransactionAfterReturning(txInfo);return retVal;}else {// 编程式事务处理Object result;final ThrowableHolder throwableHolder = new ThrowableHolder();// It's a CallbackPreferringPlatformTransactionManager: pass a TransactionCallback in.try {result = ((CallbackPreferringPlatformTransactionManager) ptm).execute(txAttr, status -> {TransactionInfo txInfo = prepareTransactionInfo(ptm, txAttr, joinpointIdentification, status);try {Object retVal = invocation.proceedWithInvocation();if (retVal != null && vavrPresent && VavrDelegate.isVavrTry(retVal)) {// Set rollback-only in case of Vavr failure matching our rollback rules...retVal = VavrDelegate.evaluateTryFailure(retVal, txAttr, status);}return retVal;}catch (Throwable ex) {if (txAttr.rollbackOn(ex)) {// A RuntimeException: will lead to a rollback.if (ex instanceof RuntimeException) {throw (RuntimeException) ex;}else {throw new ThrowableHolderException(ex);}}else {// A normal return value: will lead to a commit.throwableHolder.throwable = ex;return null;}}finally {cleanupTransactionInfo(txInfo);}});}catch (ThrowableHolderException ex) {throw ex.getCause();}catch (TransactionSystemException ex2) {if (throwableHolder.throwable != null) {logger.error("Application exception overridden by commit exception", throwableHolder.throwable);ex2.initApplicationException(throwableHolder.throwable);}throw ex2;}catch (Throwable ex2) {if (throwableHolder.throwable != null) {logger.error("Application exception overridden by commit exception", throwableHolder.throwable);}throw ex2;}// Check result state: It might indicate a Throwable to rethrow.if (throwableHolder.throwable != null) {throw throwableHolder.throwable;}return result;}}
然后进入到createTransactionIfNecessary方法中
protected TransactionInfo createTransactionIfNecessary(@Nullable PlatformTransactionManager tm,@Nullable TransactionAttribute txAttr, final String joinpointIdentification) {// If no name specified, apply method identification as transaction name.// 如果没有名称指定则使用方法唯一标识,并使用DelegatingTransactionAttribute封装txAttrif (txAttr != null && txAttr.getName() == null) {txAttr = new DelegatingTransactionAttribute(txAttr) {@Overridepublic String getName() {return joinpointIdentification;}};}TransactionStatus status = null;if (txAttr != null) {if (tm != null) {// 获取TransactionStatus事务状态信息status = tm.getTransaction(txAttr);}else {if (logger.isDebugEnabled()) {logger.debug("Skipping transactional joinpoint [" + joinpointIdentification +"] because no transaction manager has been configured");}}}// 根据指定的属性与status准备一个TransactionInfo,return prepareTransactionInfo(tm, txAttr, joinpointIdentification, status);}
其中第19行,进入 getTransaction()这个方法,开始事务。
核心的是doBegin方法。完成 自动提交的关闭和 本地线程 对象的存储
TransactionInterceptor
既然事务是通过TransactionInterceptor来创建的。那TransactionInterceptor是如何注入到容器中的?
首先来看看事务的开启注解@EnableTransactionManagement
可以看到这个注解导入了一个TransactionManagementConfigurationSelector类。
这个TransactionManagementConfigurationSelector的selectImports方法内部,引入了一个重要类,ProxyTransactionManagementConfiguration
@Overrideprotected String[] selectImports(AdviceMode adviceMode) {switch (adviceMode) {case PROXY:return new String[] {AutoProxyRegistrar.class.getName(),ProxyTransactionManagementConfiguration.class.getName()};case ASPECTJ:return new String[] {determineTransactionAspectClass()};default:return null;}}
这个类是一个配置类,注册了事务的一些相关类,上文提到的TransactionInterceptor是其中之一。
/*** 代理事务配置,注册事务需要用的一些类,而且Role=ROLE_INFRASTRUCTURE都是属于内部级别的*/
@Configuration(proxyBeanMethods = false)
@Role(BeanDefinition.ROLE_INFRASTRUCTURE)
public class ProxyTransactionManagementConfiguration extends AbstractTransactionManagementConfiguration {/*** 配置事务属性通知器,存放事务注解的方法相关的属性* @return*/@Bean(name = TransactionManagementConfigUtils.TRANSACTION_ADVISOR_BEAN_NAME)@Role(BeanDefinition.ROLE_INFRASTRUCTURE)public BeanFactoryTransactionAttributeSourceAdvisor transactionAdvisor(TransactionAttributeSource transactionAttributeSource, TransactionInterceptor transactionInterceptor) {BeanFactoryTransactionAttributeSourceAdvisor advisor = new BeanFactoryTransactionAttributeSourceAdvisor();advisor.setTransactionAttributeSource(transactionAttributeSource);advisor.setAdvice(transactionInterceptor);if (this.enableTx != null) {advisor.setOrder(this.enableTx.<Integer>getNumber("order"));}return advisor;}@Bean@Role(BeanDefinition.ROLE_INFRASTRUCTURE)public TransactionAttributeSource transactionAttributeSource() {return new AnnotationTransactionAttributeSource();}/*** 配置事务拦截器,实现了方法拦截器*/@Bean@Role(BeanDefinition.ROLE_INFRASTRUCTURE)public TransactionInterceptor transactionInterceptor(TransactionAttributeSource transactionAttributeSource) {TransactionInterceptor interceptor = new TransactionInterceptor();interceptor.setTransactionAttributeSource(transactionAttributeSource);if (this.txManager != null) {interceptor.setTransactionManager(this.txManager);}return interceptor;}
}
到这分析结束。
相关文章:
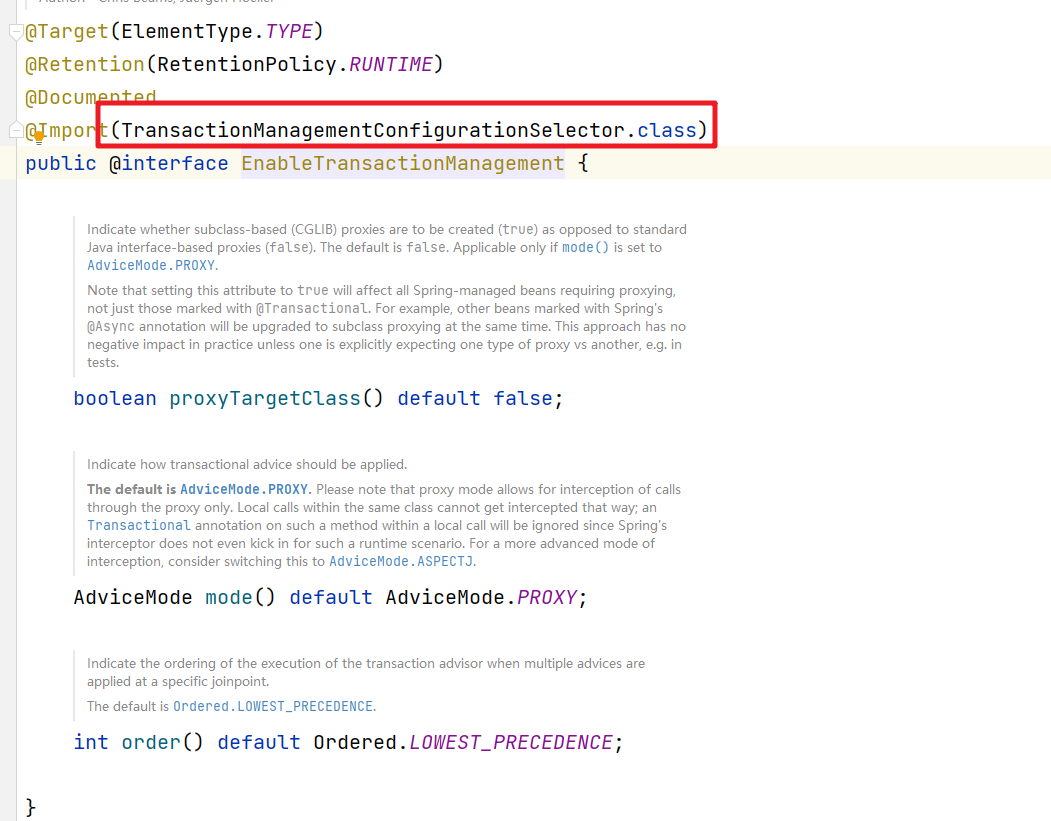
Spring源码分析 事务 实现原理
文章目录 什么是事务Spring事务管理Spring事务实现原理事务管理器事务定义事务的开启事务核心方法业务代码使用事务TransactionInterceptor 什么是事务 一般所指的事务是数据库事务,是指一批不可分割的数据库操作序列,也是数据库并发控制的基本单位。其…...
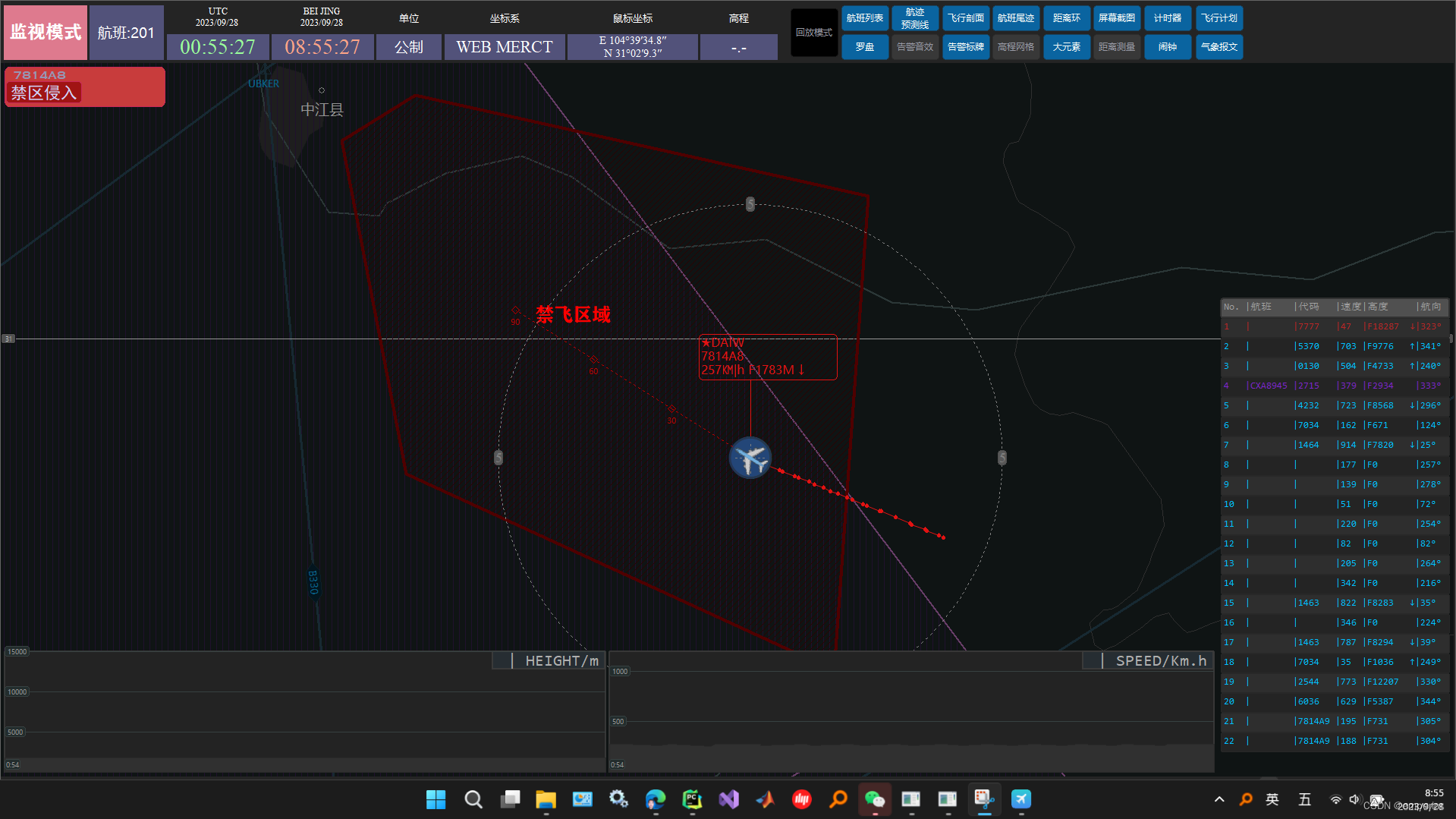
ADS-B及雷达显示终端8.3
新版本功能升级主要有如下: 1、地图更新 在上一版本8.2中使用的高程地图为由SRTM经过地形晕渲后,生成地形图片,然后对图片进行贴图,一一按规定位置、大小将地形图贴至底图上,而后在底图上进行二维矢量地图的绘制,包括…...
第二章:最新版零基础学习 PYTHON 教程(第二节 - Python 输入/输出–从 Python 控制台获取输入)
目录 Python 中的控制台是什么? 接受来自控制台的输入: 1. 将输入类型转换为整数:...
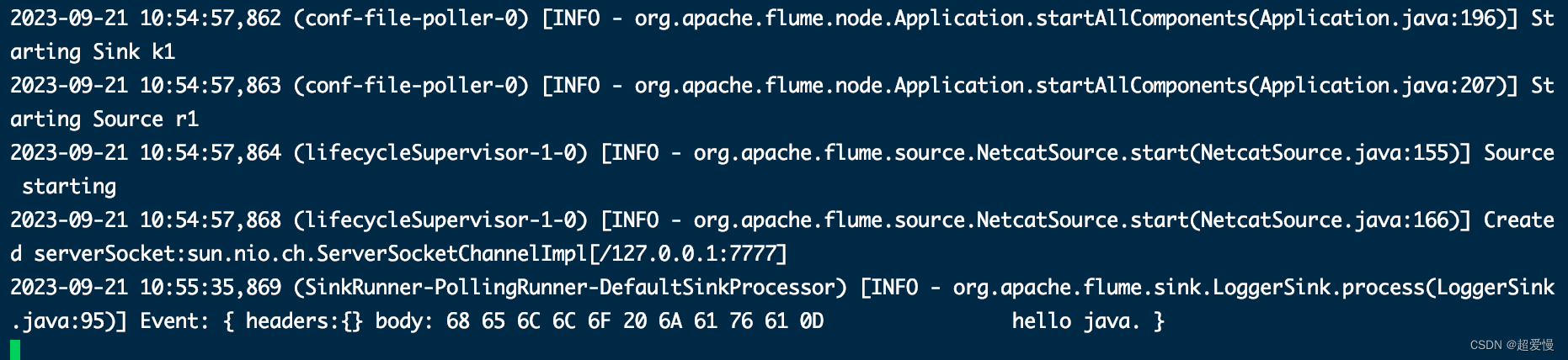
linux安装配置 flume
目录 一 解压安装包 二 配置部署 (1)修改配置 (2)下载工具 (3)创建配置文件 (4)启动监听测试 (5)flume监控文件 一 解压安装包 这里提供了网盘资源 链…...
SSM - Springboot - MyBatis-Plus 全栈体系(十五)
第三章 MyBatis 二、MyBatis 基本使用 4. CRUD 强化练习 4.1 准备数据库数据 首先,我们需要准备一张名为 user 的表。该表包含字段 id(主键)、username、password。创建SQL如下: CREATE TABLE user (id INT(11) NOT NULL AUT…...
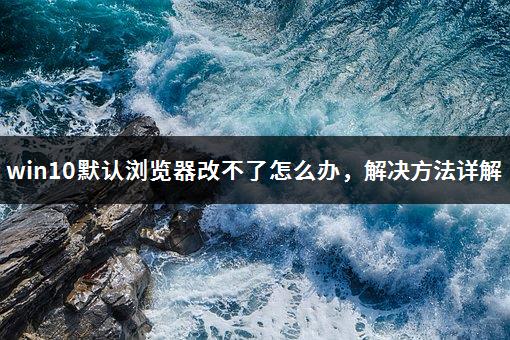
win10默认浏览器改不了怎么办,解决方法详解
win10默认浏览器改不了怎么办,解决方法详解_蓝天网络 在使用Windows 10操作系统时,你可能会遇到无法更改默认浏览器的情况。这可能是因为其他程序或设置正在干扰更改。如果你也遇到了这个问题,不要担心,本文将为你提供详细的解决…...
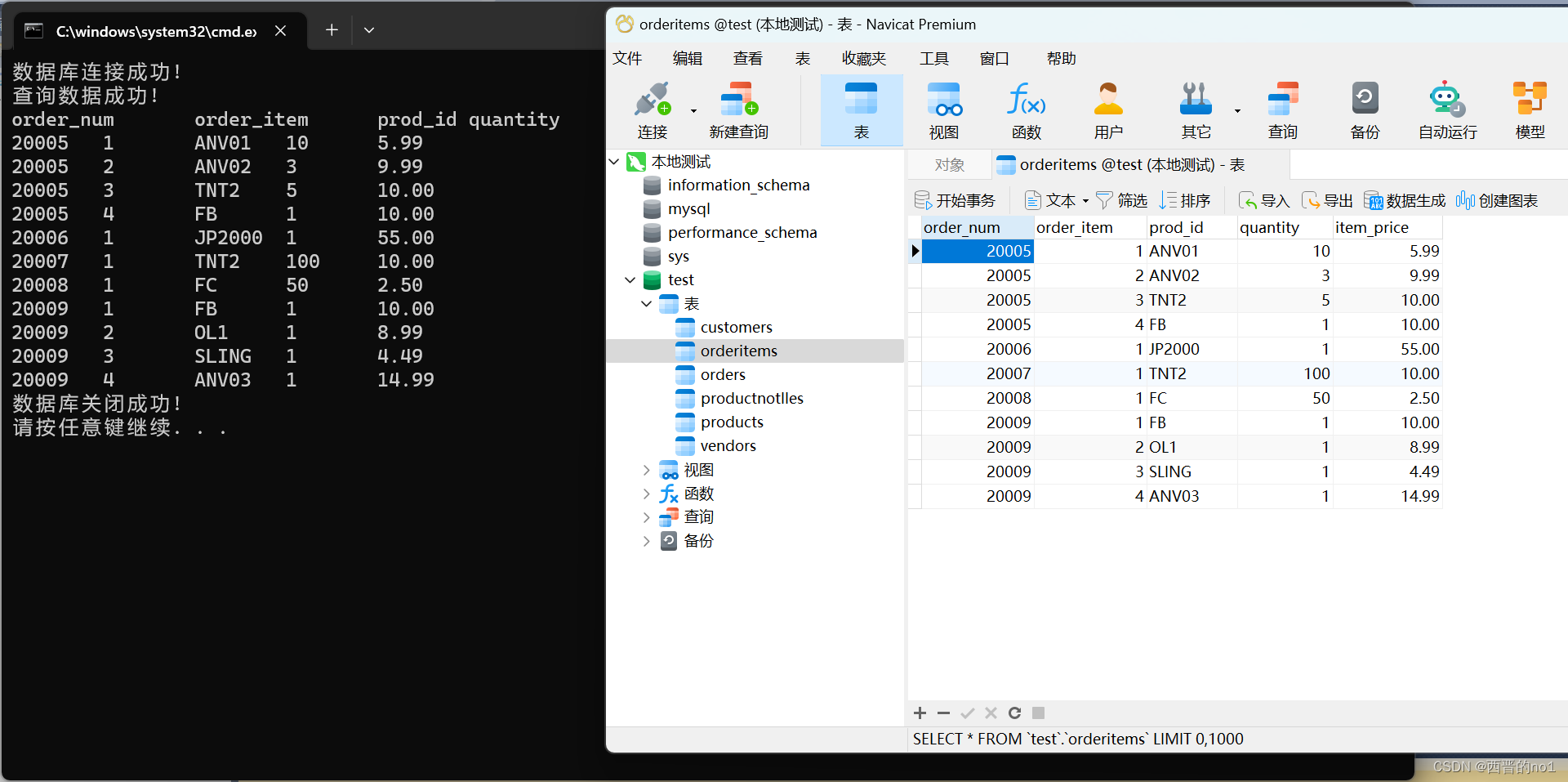
C语言连接MySQL并执行SQL语句(hello world)
1.新建一个控制台项目 参考【VS2022 和 VS2010 C语言控制台输出 Hello World】VS2022 和 VS2010 C语言控制台输出 Hello World_vs2022源文件在哪_西晋的no1的博客-CSDN博客 2.安装MySQL 参考【MySQL 8.0.34安装教程】MySQL 8.0.34安装教程_西晋的no1的博客-CSDN博客 3.复制MySQ…...
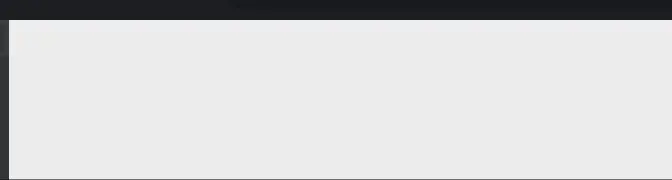
react实现动态递增展示数字特效
在可视化展示界面时有一种场景,就是页面在初始化的时候,有些数字展示想要从某个值开始动态递增到实际值,形成一种动画效果。例如: 写一个数字递增的组件,有两种方式:1.固定步长,代码如下&#x…...
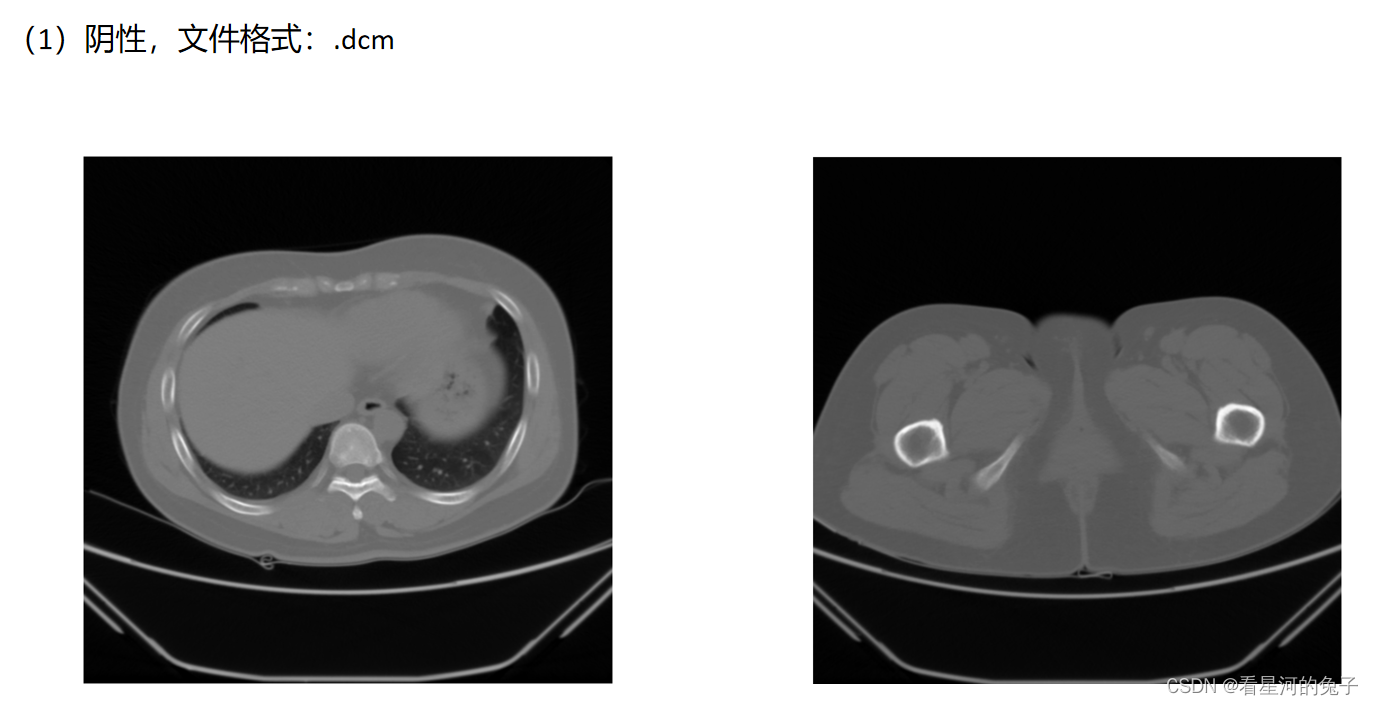
读取.nrrd和.dcm文件格式医学图片可视化与预处理
nrrd数据格式 MITK默认会将医学图像保存为格式为NRRD的图像,在这个数据格式中包含: 1、一个单个的数据头文件:为科学可视化和医学图像处理准确地表示N维度的栅格信息。 2、既能分开又能合并的图像文件。 nrrd_options输出 {u’dimension’:…...
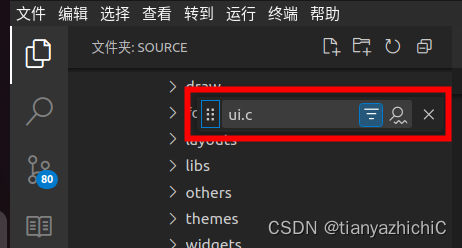
VS CODE中的筛选器如何打开?
最近更新了vscode1.82版本,发现在git管理界面有一个“筛选器”功能,十分好用,后来关掉了,找了好久都没有找到办法打开这个筛选器功能,今天无意中不知道按到了哪个快捷键,打开了,就是下图这个&am…...

vue 多环境文件配置(开发,测试,生产)
1.经常我们在开发时候会有不同环境,要代理的路由等等都会出现不同 配置一下三个文件打包的时候,执行三个不同的指令就会打包不同的环境 npm run build:dev npm run build:test npm run build:prodpackage.json 中配置scripts 指令 以,env.development…...
在服务器上搭建pulseaudio的运行环境,指定其运行目录、状态目录和模块目录
如果想在搭建 PulseAudio 的服务器上指定其运行目录、状态目录和模块目录,可以通过修改 PulseAudio 的配置文件来实现。一般情况下所涉及的配置文件和相关选项如下所示: 1、配置文件路径:通常情况下,PulseAudio 的配置文件位于 /…...
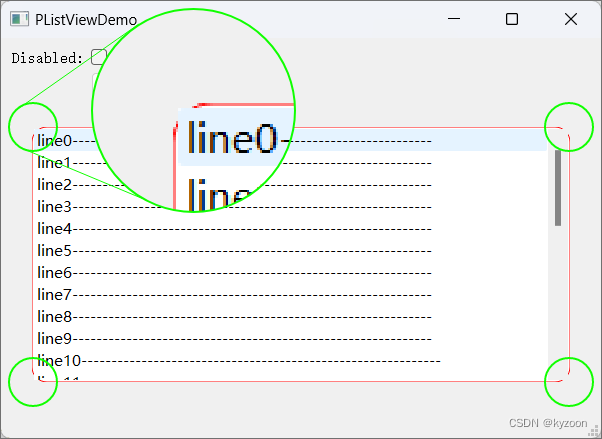
[Qt]QListView 重绘实例之一:背景重绘
0 环境 Windows 11Qt 5.15.2 MinGW x64 1 系列文章 简介:本系列文章,是以纯代码方式实现 Qt 控件的重构,尽量不使用 Qss 方式。 《[Qt]QListView 重绘实例之一:背景重绘》 《[Qt]QListView 重绘实例之二:列表项覆…...
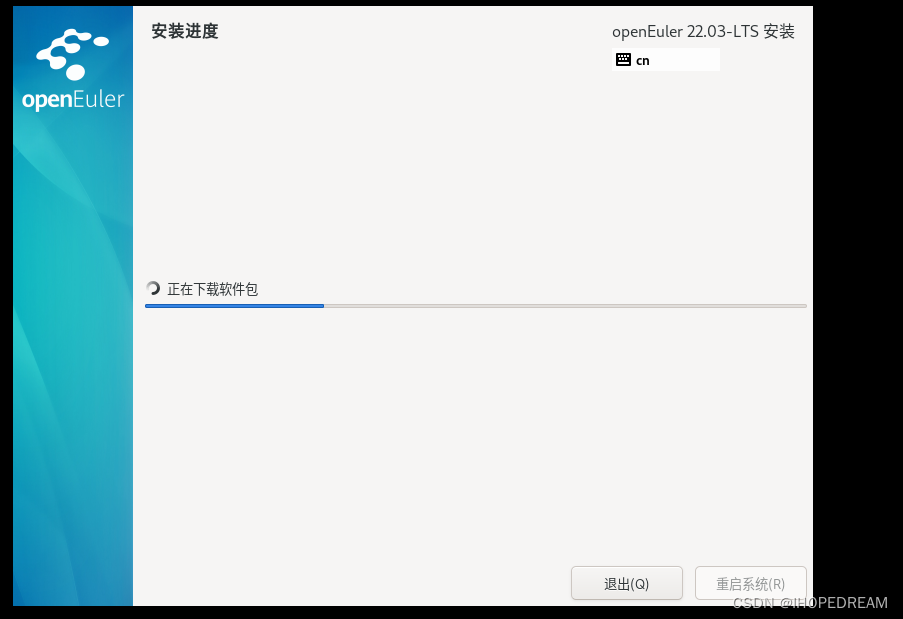
国庆周《Linux学习第二课》
Linux开篇指南针环境安装(第一课)-CSDN博客 Linux详细的环境安装介绍在上面 第一 环境准备过程 安装过程...
6年前的麒麟980依旧可以再战
麒麟980,使用6年后的今天,我对它进行跑分测试。 在bench旗下的VRMark跑分中,麒麟980荣获5023分,同款跑分APP,要知道同一时期的高通骁龙855只有4937分, 打游戏,以和平精英为例,帧率3…...
JS计算任意多边形的面积
计算任意多边形的面积需要使用一些几何数学公式。具体的计算方法取决于多边形的形状和提供的顶点坐标。下面是一个通用的 JavaScript 函数,用于计算任意多边形的面积,假设你提供多边形的顶点坐标数组: function calculatePolygonArea(vertic…...
ios xcode15 navigationController?.navigationBar.isHidden = false无效
xcode 15 用 navigationController?.setNavigationBarHidden(true, animated: false)隐藏navigationBar后,再调用 navigationController?.navigationBar.isHidden false无效 解决 用 navigationController?.navigationBar.isHidden true隐藏navigationBar...
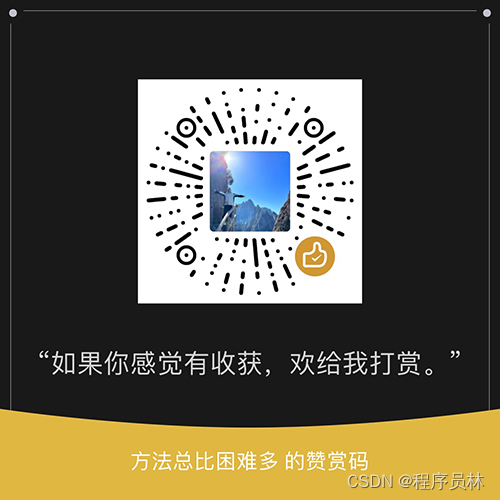
Python二级 每周练习题20
练习一: 日期计算器 设计一款日期计算程序,能否实现下面的功能: (1)要求用户分别输入年、月、日(分三次输入); (2)程序自动会根据输入的年月日计算出这一天是这一年的第几天; (3)输出格式为:这…...
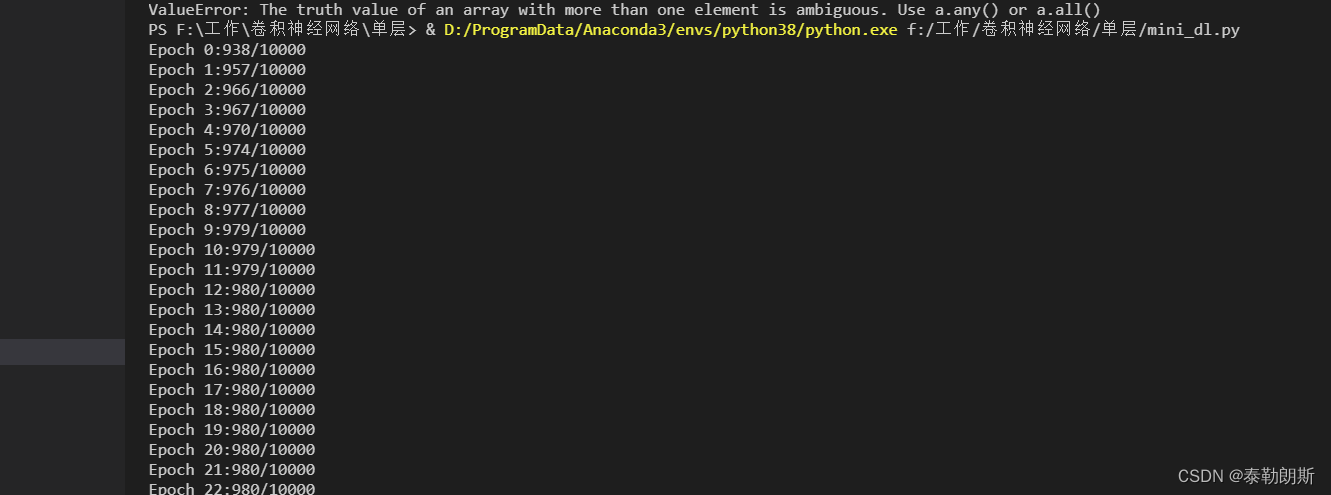
深度学习-一个简单的深度学习推导
文章目录 前言1.sigmod函数2.sigmoid求导3.损失函数loss4.神经网络1.神经网络结构2.公式表示-正向传播3.梯度计算1.Loss 函数2.梯度1.反向传播第2-3层2.反向传播第1-2层 3.python代码4.MNIST 数据集 前言 本章主要推导一个简单的两层神经网络。 其中公式入口【入口】 1.sigmod…...
ES写入数据报错:retrying failed action with response code: 429
报错: 使用logstash导入分片数量为9的index发生错误,[logstash.outputs.elasticsearch] retrying failed action with response code: 429 ({"type">"es_rejected_execution_exception", "reason">"rejected execution …...
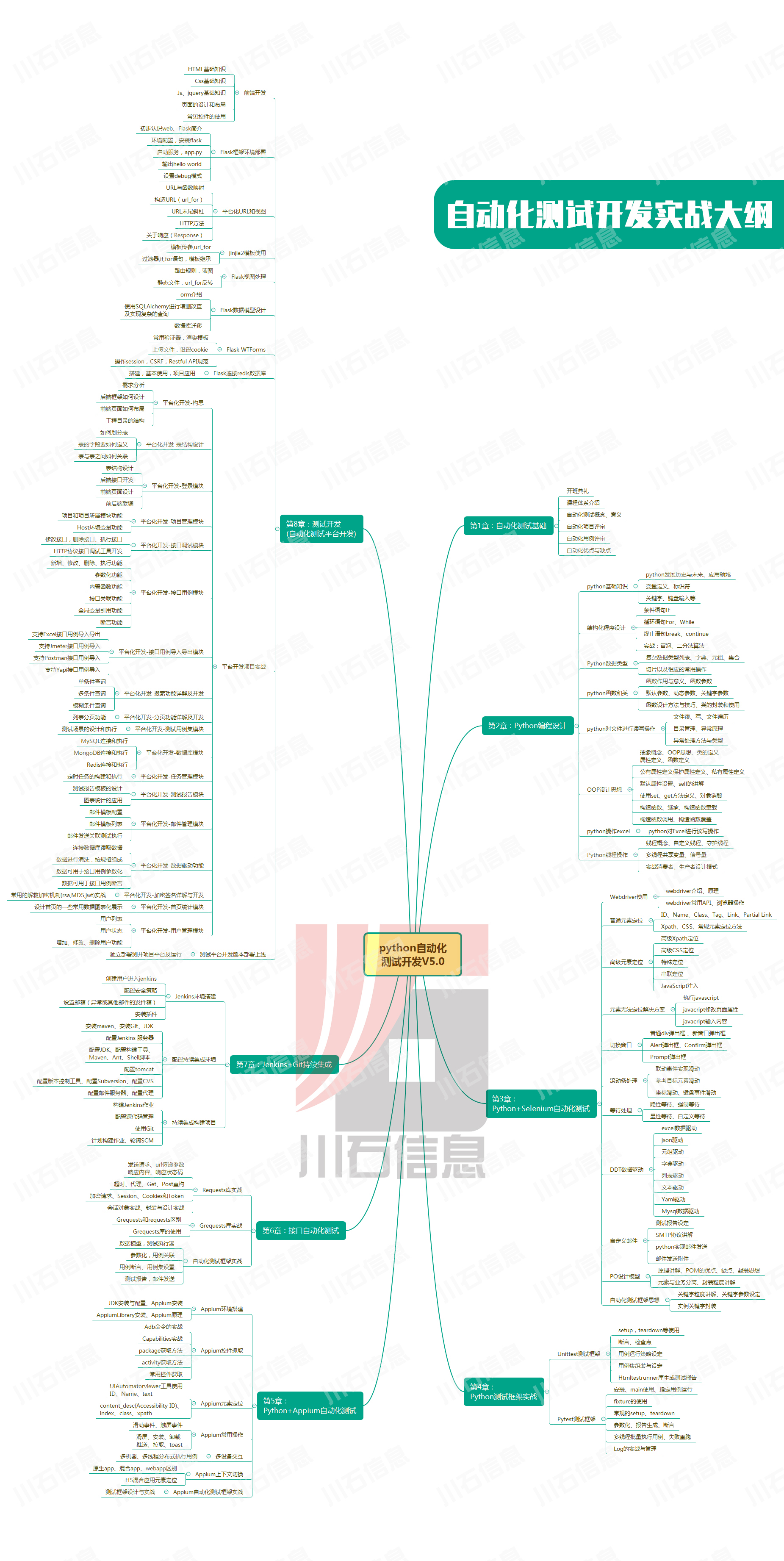
接口测试中缓存处理策略
在接口测试中,缓存处理策略是一个关键环节,直接影响测试结果的准确性和可靠性。合理的缓存处理策略能够确保测试环境的一致性,避免因缓存数据导致的测试偏差。以下是接口测试中常见的缓存处理策略及其详细说明: 一、缓存处理的核…...
反向工程与模型迁移:打造未来商品详情API的可持续创新体系
在电商行业蓬勃发展的当下,商品详情API作为连接电商平台与开发者、商家及用户的关键纽带,其重要性日益凸显。传统商品详情API主要聚焦于商品基本信息(如名称、价格、库存等)的获取与展示,已难以满足市场对个性化、智能…...
【Linux】C语言执行shell指令
在C语言中执行Shell指令 在C语言中,有几种方法可以执行Shell指令: 1. 使用system()函数 这是最简单的方法,包含在stdlib.h头文件中: #include <stdlib.h>int main() {system("ls -l"); // 执行ls -l命令retu…...
ssc377d修改flash分区大小
1、flash的分区默认分配16M、 / # df -h Filesystem Size Used Available Use% Mounted on /dev/root 1.9M 1.9M 0 100% / /dev/mtdblock4 3.0M...
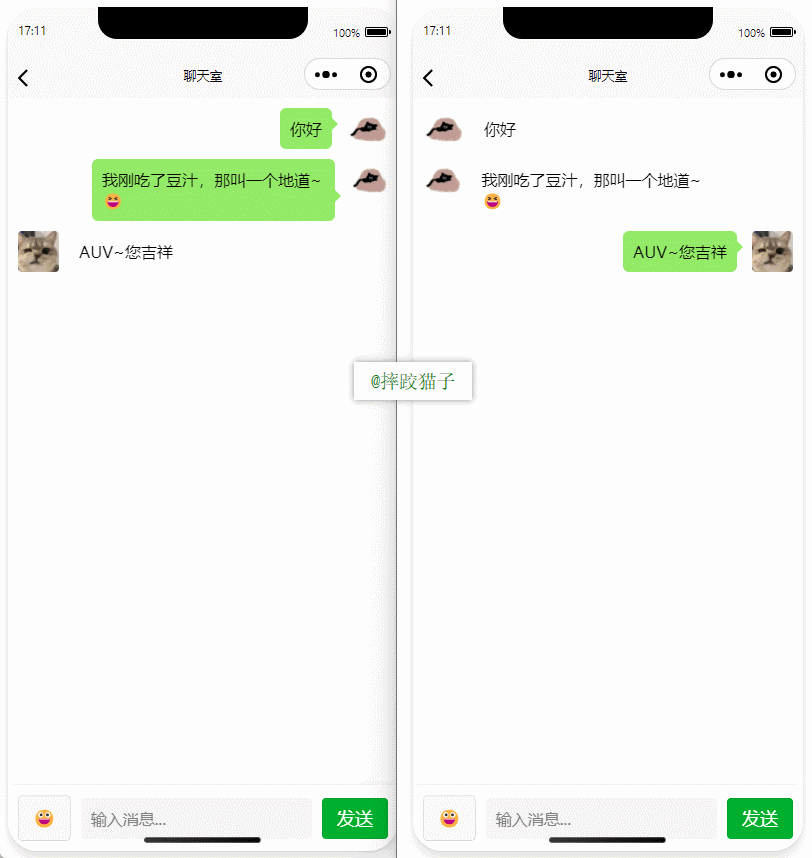
基于uniapp+WebSocket实现聊天对话、消息监听、消息推送、聊天室等功能,多端兼容
基于 UniApp + WebSocket实现多端兼容的实时通讯系统,涵盖WebSocket连接建立、消息收发机制、多端兼容性配置、消息实时监听等功能,适配微信小程序、H5、Android、iOS等终端 目录 技术选型分析WebSocket协议优势UniApp跨平台特性WebSocket 基础实现连接管理消息收发连接…...
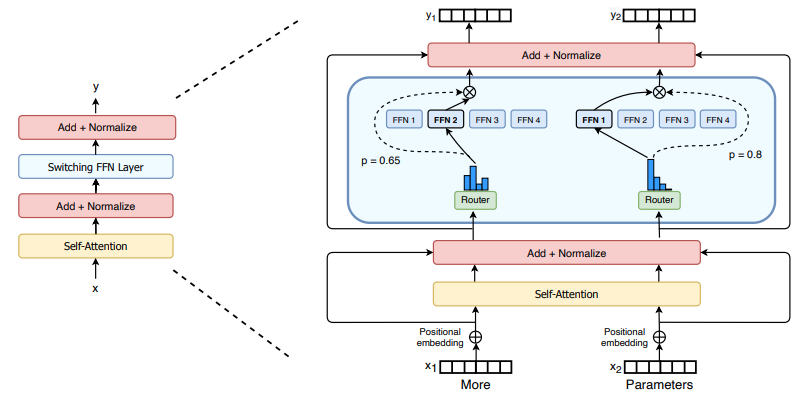
(二)TensorRT-LLM | 模型导出(v0.20.0rc3)
0. 概述 上一节 对安装和使用有个基本介绍。根据这个 issue 的描述,后续 TensorRT-LLM 团队可能更专注于更新和维护 pytorch backend。但 tensorrt backend 作为先前一直开发的工作,其中包含了大量可以学习的地方。本文主要看看它导出模型的部分&#x…...
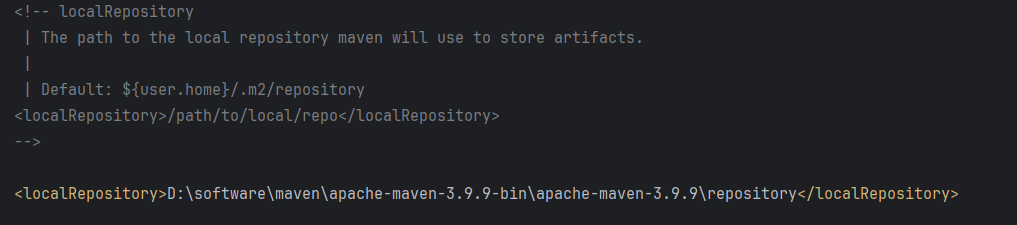
Maven 概述、安装、配置、仓库、私服详解
目录 1、Maven 概述 1.1 Maven 的定义 1.2 Maven 解决的问题 1.3 Maven 的核心特性与优势 2、Maven 安装 2.1 下载 Maven 2.2 安装配置 Maven 2.3 测试安装 2.4 修改 Maven 本地仓库的默认路径 3、Maven 配置 3.1 配置本地仓库 3.2 配置 JDK 3.3 IDEA 配置本地 Ma…...
Linux C语言网络编程详细入门教程:如何一步步实现TCP服务端与客户端通信
文章目录 Linux C语言网络编程详细入门教程:如何一步步实现TCP服务端与客户端通信前言一、网络通信基础概念二、服务端与客户端的完整流程图解三、每一步的详细讲解和代码示例1. 创建Socket(服务端和客户端都要)2. 绑定本地地址和端口&#x…...
Spring是如何解决Bean的循环依赖:三级缓存机制
1、什么是 Bean 的循环依赖 在 Spring框架中,Bean 的循环依赖是指多个 Bean 之间互相持有对方引用,形成闭环依赖关系的现象。 多个 Bean 的依赖关系构成环形链路,例如: 双向依赖:Bean A 依赖 Bean B,同时 Bean B 也依赖 Bean A(A↔B)。链条循环: Bean A → Bean…...
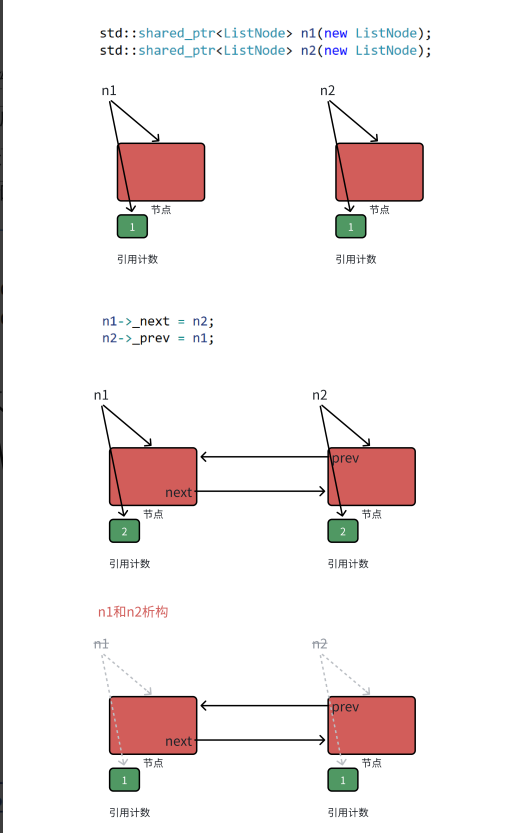
【C++进阶篇】智能指针
C内存管理终极指南:智能指针从入门到源码剖析 一. 智能指针1.1 auto_ptr1.2 unique_ptr1.3 shared_ptr1.4 make_shared 二. 原理三. shared_ptr循环引用问题三. 线程安全问题四. 内存泄漏4.1 什么是内存泄漏4.2 危害4.3 避免内存泄漏 五. 最后 一. 智能指针 智能指…...