利用easy excel 实现文件导出
一.创建实体类
package com.siact.product.jwp.module.report.dto;import com.alibaba.excel.annotation.ExcelProperty;
import com.alibaba.excel.annotation.write.style.ColumnWidth;
import com.alibaba.excel.annotation.write.style.ContentRowHeight;
import com.alibaba.excel.annotation.write.style.HeadRowHeight;
import lombok.AllArgsConstructor;
import lombok.Data;
import lombok.NoArgsConstructor;/*** @Description:*/
@Data
@AllArgsConstructor
@NoArgsConstructor
@ContentRowHeight(30)
@HeadRowHeight(25)
@ColumnWidth(25)
public class PatrolProcessDTO {@ExcelProperty(index = 0,value = "序号")private int orderNum;@ExcelProperty(index = 1,value = "工单名称")private String workOrderName;@ExcelProperty(index = 2,value = "巡检路线")private String patrolRoute;@ExcelProperty(index = 3,value = "维修技师")private String maintenanceUserName;@ExcelProperty(index = 4,value = "打点情况")private String content;@ExcelProperty(index = 5,value = "处理结果(工单状态)")private String workOrderSolveStatusName;@ExcelProperty(index = 6,value = "维修技师处理时长")private String maintenanceTime;@ExcelProperty(index = 7,value = "维修班长确认时长")private String confirmTime;@ExcelProperty(index = 8,value = "总用时")private String workOrderSpendTime;}
二、引入合并策略
package com.siact.product.jwp.module.report.service.impl.StyleUtils;import com.alibaba.excel.metadata.Head;
import com.alibaba.excel.metadata.data.CellData;
import com.alibaba.excel.metadata.data.WriteCellData;
import com.alibaba.excel.write.handler.CellWriteHandler;
import com.alibaba.excel.write.metadata.holder.WriteSheetHolder;
import com.alibaba.excel.write.metadata.holder.WriteTableHolder;
import org.apache.poi.ss.usermodel.Cell;
import org.apache.poi.ss.usermodel.CellType;
import org.apache.poi.ss.usermodel.Row;
import org.apache.poi.ss.usermodel.Sheet;
import org.apache.poi.ss.util.CellRangeAddress;import java.util.List;/*** @Description:*/public class ExcelFillCellMergeStrategy implements CellWriteHandler {
// /*
// * 要合并的列 (下表也是从0开始)
// */
// private Set<Integer> mergeColumnIndex;
// /*
// * 用第几行开始合并 ,默认为1,因为第0行是标题,EasyExcel 的默认也是
// */
// private int mergeBeginRowIndex = 1;
//
// public ExcelFillCellMergeStrategy(Set<Integer> mergeColumnIndex) {
// this.mergeColumnIndex = mergeColumnIndex;
// }
//
//
// /*
// * 在创建单元格之前调用
// */
// @Override
// public void beforeCellCreate(WriteSheetHolder writeSheetHolder, WriteTableHolder writeTableHolder, Row row, Head head, Integer columnIndex, Integer relativeRowIndex, Boolean isHead) {
// }
//
// /*
// * 在创建单元格之后调用
// */
// @Override
// public void afterCellCreate(WriteSheetHolder writeSheetHolder, WriteTableHolder writeTableHolder, Cell cell, Head head, Integer relativeRowIndex, Boolean isHead) {
//
// }
//
//
//
// /*
// * 在对单元格的所有操作完成后调用
// */
// @Override
// public void afterCellDispose(WriteSheetHolder writeSheetHolder, WriteTableHolder writeTableHolder, List<WriteCellData<?>> list, Cell cell, Head head, Integer integer, Boolean aBoolean) {
// //当前行
// int curRowIndex = cell.getRowIndex();
// //当前列
// int curColIndex = cell.getColumnIndex();
//
// if (curRowIndex > mergeBeginRowIndex) {
// if (mergeColumnIndex.contains(curColIndex)) {
// mergeWithPrevRow(writeSheetHolder, cell, curRowIndex, curColIndex);
// }
// }
// }
//
// /**
// * 当前单元格向上合并
// *
// * @param writeSheetHolder
// * @param cell 当前单元格
// * @param curRowIndex 当前行
// * @param curColIndex 当前列
// */
// private void mergeWithPrevRow(WriteSheetHolder writeSheetHolder, Cell cell, int curRowIndex, int curColIndex) {
// //获取当前行的当前列的数据和上一行的当前列列数据,通过上一行数据是否相同进行合并
// Object curData = cell.getCellTypeEnum() == CellType.STRING ? cell.getStringCellValue() : cell.getNumericCellValue();
// Cell preCell = cell.getSheet().getRow(curRowIndex - 1).getCell(curColIndex);
// Object preData = preCell.getCellTypeEnum() == CellType.STRING ? preCell.getStringCellValue() : preCell.getNumericCellValue();
// // 比较当前行的第一列的单元格与上一行是否相同,相同合并当前单元格与上一行
// if (curData.equals(preData)) {
// Sheet sheet = writeSheetHolder.getSheet();
// // 获取合并信息
// List<CellRangeAddress> mergeRegions = sheet.getMergedRegions();
// int size = mergeRegions.size();
// CellRangeAddress cellRangeAddr;
// if (size > 0) {
// cellRangeAddr = mergeRegions.get(size - 1);
// // 若上一个单元格已经被合并,则先移出原有的合并单元,再重新添加合并单元
// if (cellRangeAddr.isInRange(curRowIndex - 1, curColIndex)) {
// // 移除当前合并信息
// sheet.removeMergedRegion(size - 1);
// // 重新设置当前结束行
// cellRangeAddr.setLastRow(curRowIndex);
// } else {
// // 若上一个单元格未被合并,则新增合并单元
// cellRangeAddr = new CellRangeAddress(curRowIndex - 1, curRowIndex, curColIndex, curColIndex);
// }
// } else {
// // 若上一个单元格未被合并,则新增合并单元
// cellRangeAddr = new CellRangeAddress(curRowIndex - 1, curRowIndex, curColIndex, curColIndex);
// }
// // 添加新的合并信息
// sheet.addMergedRegion(cellRangeAddr);
// }
// }
// 需要从第几行开始合并,0表示第1行
private final int mergeRowIndex;// 合并的哪些列,比如为4时,当前行id和上一行id相同则合并前五列private final int mergeColumnRegion;private final List<Integer> ignoreColumn;public ExcelFillCellMergeStrategy(int mergeRowIndex, int mergeColumnRegion, List<Integer> ignoreColumn) {this.mergeRowIndex = mergeRowIndex;this.mergeColumnRegion = mergeColumnRegion;this.ignoreColumn = ignoreColumn;}@Overridepublic void afterCellCreate(WriteSheetHolder writeSheetHolder, WriteTableHolder writeTableHolder, Cell cell, Head head, Integer relativeRowIndex, Boolean isHead) {}// @Override
// public void afterCellDataConverted(WriteSheetHolder writeSheetHolder, WriteTableHolder writeTableHolder, CellData cellData, Cell cell, Head head, Integer relativeRowIndex, Boolean isHead) {
// // 隐藏id列writeSheetHolder.getSheet().setColumnHidden(0, true);
// }@Overridepublic void afterCellDispose(WriteSheetHolder writeSheetHolder, WriteTableHolder writeTableHolder, List<WriteCellData<?>> cellDataList, Cell cell, Head head, Integer relativeRowIndex, Boolean isHead) {//当前行int curRowIndex = cell.getRowIndex();//当前列int curColIndex = cell.getColumnIndex();if (!ignoreColumn.contains(curColIndex) && curRowIndex > mergeRowIndex) {for (int i = 0; i < mergeColumnRegion; i++) {if (curColIndex <= mergeColumnRegion) {mergeWithPreviousRow(writeSheetHolder, cell, curRowIndex, curColIndex);break;}}}}/*** 当前单元格向上合并:当前行的id和上一行的id相同则合并前面(mergeColumnRegion+1)列** @param cell 当前单元格* @param curRowIndex 当前行* @param curColIndex 当前列*/private void mergeWithPreviousRow(WriteSheetHolder writeSheetHolder, Cell cell, int curRowIndex, int curColIndex) {// 当前行的第一个CellCell curFirstCell = cell.getSheet().getRow(curRowIndex).getCell(0);Object curFirstData = curFirstCell.getCellType().getCode() == CellType.STRING.getCode() ? curFirstCell.getStringCellValue() : curFirstCell.getNumericCellValue();// 上一行的第一个CellCell preFirstCell = cell.getSheet().getRow(curRowIndex - 1).getCell(0);Object preFirstData = preFirstCell.getCellType().getCode() == CellType.STRING.getCode() ? preFirstCell.getStringCellValue() : preFirstCell.getNumericCellValue();// 当前cellObject data = cell.getCellType().getCode() == CellType.STRING.getCode() ? cell.getStringCellValue() : cell.getNumericCellValue();// 上面的CellCell upCell = cell.getSheet().getRow(curRowIndex - 1).getCell(curColIndex);Object upData = upCell.getCellType().getCode() == CellType.STRING.getCode() ? upCell.getStringCellValue() : upCell.getNumericCellValue();// 当前行的id和上一行的id相同则合并前面(mergeColumnRegion+1)列 且上一行值相同if (curFirstData.equals(preFirstData) && data.equals(upData)) {Sheet sheet = writeSheetHolder.getSheet();List<CellRangeAddress> mergeRegions = sheet.getMergedRegions();boolean isMerged = false;for (int i = 0; i < mergeRegions.size() && !isMerged; i++) {CellRangeAddress cellRangeAddr = mergeRegions.get(i);// 若上一个单元格已经被合并,则先移出原有的合并单元,再重新添加合并单元if (cellRangeAddr.isInRange(curRowIndex - 1, curColIndex)) {sheet.removeMergedRegion(i);cellRangeAddr.setLastRow(curRowIndex);sheet.addMergedRegion(cellRangeAddr);isMerged = true;}}// 若上一个单元格未被合并,则新增合并单元if (!isMerged) {CellRangeAddress cellRangeAddress = new CellRangeAddress(curRowIndex - 1, curRowIndex, curColIndex, curColIndex);sheet.addMergedRegion(cellRangeAddress);}}}@Overridepublic void beforeCellCreate(WriteSheetHolder writeSheetHolder, WriteTableHolder writeTableHolder, Row row, Head head, Integer integer, Integer integer1, Boolean aBoolean) {}}
三、引入其他样式
package com.siact.product.jwp.module.report.service.impl.StyleUtils;import cn.hutool.core.util.StrUtil;
import com.alibaba.excel.write.handler.AbstractRowWriteHandler;
import com.alibaba.excel.write.metadata.holder.WriteSheetHolder;
import com.alibaba.excel.write.metadata.holder.WriteTableHolder;
import com.siact.product.jwp.module.report.service.impl.StyleUtils.CellStyleModel;
import org.apache.poi.ss.usermodel.*;
import org.apache.poi.xssf.usermodel.XSSFCellStyle;
import org.apache.poi.xssf.usermodel.XSSFColor;
import org.apache.poi.xssf.usermodel.XSSFFont;
import org.camunda.bpm.engine.impl.util.CollectionUtil;
import org.springframework.stereotype.Component;import java.util.ArrayList;
import java.util.List;
import java.util.stream.Collectors;/*** @Author: 刘 旺* @CreateTime: 2023-08-04 17:50* @Description: 自定义单元格样式处理器(支持字体样式、背景颜色、边框样式、对齐方式、自动换行)*/@Component
public class CustomCellStyleHandler extends AbstractRowWriteHandler {/*** sheet页名称列表*/private List<String> sheetNameList;/*** 样式信息*/private List<CellStyleModel> cellStyleList = new ArrayList<>();/*** 自定义样式适配器构造方法** @param cellStyleList 样式信息*/public CustomCellStyleHandler(List<CellStyleModel> cellStyleList) {if (CollectionUtil.isEmpty(cellStyleList)) {return;}cellStyleList = cellStyleList.stream().filter(x -> x != null//判断sheet名称KEY是否存在&& StrUtil.isNotBlank(x.getSheetName())//字体样式//判断字体颜色KEY是否存在&& (x.getFontColor() == null || x.getFontColor() instanceof IndexedColors|| x.getFontColor() instanceof XSSFColor)//判断背景颜色KEY是否存在&& (x.getBackgroundColor() == null || x.getBackgroundColor() instanceof IndexedColors|| x.getBackgroundColor() instanceof XSSFColor)//边框样式// 判断上边框线条颜色KEY是否存在&& (x.getTopBorderColor() == null || x.getTopBorderColor() instanceof IndexedColors|| x.getTopBorderColor() instanceof XSSFColor)// 判断右边框线条颜色KEY是否存在&& (x.getRightBorderColor() == null || x.getRightBorderColor() instanceof IndexedColors|| x.getRightBorderColor() instanceof XSSFColor)// 判断下边框线条颜色KEY是否存在&& (x.getBottomBorderColor() == null || x.getBottomBorderColor() instanceof IndexedColors|| x.getBottomBorderColor() instanceof XSSFColor)// 判断左边框线条颜色KEY是否存在&& (x.getLeftBorderColor() == null || x.getLeftBorderColor() instanceof IndexedColors|| x.getLeftBorderColor() instanceof XSSFColor)).collect(Collectors.toList());this.cellStyleList = cellStyleList;sheetNameList = this.cellStyleList.stream().map(x -> x.getSheetName()).distinct().collect(Collectors.toList());}@Overridepublic void afterRowDispose(WriteSheetHolder writeSheetHolder, WriteTableHolder writeTableHolder, Row row, Integer relativeRowIndex, Boolean isHead) {Sheet sheet = writeSheetHolder.getSheet();//不需要添加样式,或者当前sheet页不需要添加样式if (cellStyleList == null || cellStyleList.size() <= 0 || sheetNameList.contains(sheet.getSheetName()) == false) {return;}//获取当前行的样式信息List<CellStyleModel> rowCellStyleList = cellStyleList.stream().filter(x ->StrUtil.equals(x.getSheetName(), sheet.getSheetName()) && x.getRowIndex() == relativeRowIndex).collect(Collectors.toList());//该行不需要设置样式if (rowCellStyleList == null || rowCellStyleList.size() <= 0) {return;}for (CellStyleModel cellStyleModel : rowCellStyleList) {//设置单元格样式setCellStyle(cellStyleModel, row);}//删除已添加的样式信息cellStyleList.removeAll(rowCellStyleList);//重新获取要添加的sheet页姓名sheetNameList = cellStyleList.stream().map(x -> x.getSheetName()).distinct().collect(Collectors.toList());}/*** 给单元格设置样式** @param cellStyleModel 样式信息* @param row 行对象*/private void setCellStyle(CellStyleModel cellStyleModel, Row row) {//背景颜色Object backgroundColor = cellStyleModel.getBackgroundColor();//自动换行Boolean wrapText = cellStyleModel.getWrapText();//列索引int colIndex = cellStyleModel.getColIndex();//边框样式Cell cell = row.getCell(colIndex);if (cell == null) {cell = row.createCell(colIndex);}XSSFCellStyle style = (XSSFCellStyle) cell.getRow().getSheet().getWorkbook().createCellStyle();// 克隆出一个 stylestyle.cloneStyleFrom(cell.getCellStyle());//设置背景颜色if (backgroundColor != null) {//使用IndexedColors定义的颜色if (backgroundColor instanceof IndexedColors) {style.setFillForegroundColor(((IndexedColors) backgroundColor).getIndex());}//使用自定义的RGB颜色else if (backgroundColor instanceof XSSFColor) {style.setFillForegroundColor((XSSFColor) backgroundColor);}style.setFillPattern(FillPatternType.SOLID_FOREGROUND);}//设置自动换行if (wrapText != null) {style.setWrapText(wrapText);}//设置字体样式setFontStyle(row, style, cellStyleModel);//设置边框样式setBorderStyle(style, cellStyleModel);//设置对齐方式setAlignmentStyle(style, cellStyleModel);cell.setCellStyle(style);}/*** 设置字体样式** @param row 行对象* @param style 单元格样式* @param cellStyleModel 样式信息*/private void setFontStyle(Row row, XSSFCellStyle style, CellStyleModel cellStyleModel) {//字体名称String fontName = cellStyleModel.getFontName();//字体大小Double fontHeight = cellStyleModel.getFontHeight();//字体颜色Object fontColor = cellStyleModel.getFontColor();//字体加粗Boolean fontBold = cellStyleModel.getFontBold();//字体斜体Boolean fontItalic = cellStyleModel.getFontItalic();//字体下划线Byte fontUnderLine = cellStyleModel.getFontUnderLine();//字体上标下标Short fontTypeOffset = cellStyleModel.getFontTypeOffset();//字体删除线Boolean fontStrikeout = cellStyleModel.getFontStrikeout();//不需要设置字体样式if (fontName == null && fontHeight == null && fontColor == null && fontBold == null && fontItalic == null&& fontUnderLine == null && fontTypeOffset == null && fontStrikeout == null) {return;}XSSFFont font = null;//样式存在字体对象时,使用原有的字体对象if (style.getFontIndex() != 0) {font = style.getFont();}//样式不存在字体对象时,创建字体对象else {font = (XSSFFont) row.getSheet().getWorkbook().createFont();//默认字体为宋体font.setFontName("宋体");}//设置字体名称if (fontName != null) {font.setFontName(fontName);}//设置字体大小if (fontHeight != null) {font.setFontHeight(fontHeight);}//设置字体颜色if (fontColor != null) {//使用IndexedColors定义的颜色if (fontColor instanceof IndexedColors) {font.setColor(((IndexedColors) fontColor).getIndex());}//使用自定义的RGB颜色else if (fontColor instanceof XSSFColor) {font.setColor((XSSFColor) fontColor);}}//设置字体加粗if (fontBold != null) {font.setBold(fontBold);}//设置字体斜体if (fontItalic != null) {font.setItalic(fontItalic);}//设置字体下划线if (fontUnderLine != null) {font.setUnderline(fontUnderLine);}//设置字体上标下标if (fontTypeOffset != null) {font.setTypeOffset(fontTypeOffset);}//设置字体删除线if (fontStrikeout != null) {font.setStrikeout(fontStrikeout);}style.setFont(font);}/*** 设置边框样式** @param style 单元格样式* @param cellStyleModel 样式信息*/private void setBorderStyle(XSSFCellStyle style, CellStyleModel cellStyleModel) {//上边框线条类型BorderStyle borderTop = cellStyleModel.getBorderTop();//右边框线条类型BorderStyle borderRight = cellStyleModel.getBorderRight();//下边框线条类型BorderStyle borderBottom = cellStyleModel.getBorderBottom();//左边框线条类型BorderStyle borderLeft = cellStyleModel.getBorderLeft();//上边框颜色类型Object topBorderColor = cellStyleModel.getTopBorderColor();//右边框颜色类型Object rightBorderColor = cellStyleModel.getRightBorderColor();//下边框颜色类型Object bottomBorderColor = cellStyleModel.getBottomBorderColor();//左边框颜色类型Object leftBorderColor = cellStyleModel.getLeftBorderColor();//不需要设置边框样式if (borderTop == null && borderRight == null && borderBottom == null && borderLeft == null && topBorderColor == null&& rightBorderColor == null && bottomBorderColor == null && leftBorderColor == null) {return;}//设置上边框线条类型if (borderTop != null) {style.setBorderTop(borderTop);}//设置右边框线条类型if (borderRight != null) {style.setBorderRight(borderRight);}//设置下边框线条类型if (borderBottom != null) {style.setBorderBottom(borderBottom);}//设置左边框线条类型if (borderLeft != null) {style.setBorderLeft(borderLeft);}//设置上边框线条颜色if (topBorderColor != null) {//使用IndexedColors定义的颜色if (topBorderColor instanceof IndexedColors) {style.setTopBorderColor(((IndexedColors) topBorderColor).getIndex());}//使用自定义的RGB颜色else if (topBorderColor instanceof XSSFColor) {style.setTopBorderColor((XSSFColor) topBorderColor);}}//设置右边框线条颜色if (rightBorderColor != null) {//使用IndexedColors定义的颜色if (rightBorderColor instanceof IndexedColors) {style.setRightBorderColor(((IndexedColors) rightBorderColor).getIndex());}//使用自定义的RGB颜色else if (rightBorderColor instanceof XSSFColor) {style.setRightBorderColor((XSSFColor) rightBorderColor);}}//设置下边框线条颜色if (bottomBorderColor != null) {//使用IndexedColors定义的颜色if (bottomBorderColor instanceof IndexedColors) {style.setBottomBorderColor(((IndexedColors) bottomBorderColor).getIndex());}//使用自定义的RGB颜色else if (bottomBorderColor instanceof XSSFColor) {style.setBottomBorderColor((XSSFColor) bottomBorderColor);}}//设置左边框线条颜色if (leftBorderColor != null) {//使用IndexedColors定义的颜色if (leftBorderColor instanceof IndexedColors) {style.setLeftBorderColor(((IndexedColors) leftBorderColor).getIndex());}//使用自定义的RGB颜色else if (topBorderColor instanceof XSSFColor) {style.setLeftBorderColor((XSSFColor) leftBorderColor);}}}/*** 设置对齐方式** @param style 单元格样式* @param cellStyleModel 样式信息*/private void setAlignmentStyle(XSSFCellStyle style, CellStyleModel cellStyleModel) {//水平对齐方式HorizontalAlignment horizontalAlignment = cellStyleModel.getHorizontalAlignment();//垂直对齐方式VerticalAlignment verticalAlignment = cellStyleModel.getVerticalAlignment();//不需要设置对齐方式if (horizontalAlignment == null && verticalAlignment == null) {return;}//设置水平对齐方式if (horizontalAlignment != null) {style.setAlignment(horizontalAlignment);}//设置垂直对齐方式if (verticalAlignment != null) {style.setVerticalAlignment(verticalAlignment);}}}
四、如有换行及其他背景字体样式,需引入换行样式(换行识别标识‘\n’)
package com.siact.product.jwp.module.report.service.impl.StyleUtils;import cn.hutool.core.util.StrUtil;
import lombok.Data;
import org.apache.poi.ss.usermodel.*;
import org.apache.poi.xssf.usermodel.DefaultIndexedColorMap;
import org.apache.poi.xssf.usermodel.XSSFColor;/*** @Description:*/
@Data
public class CellStyleModel {/*** sheet名称*/private String sheetName;/*** 列索引*/private int colIndex;/*** 行索引*/private int rowIndex;/*** 字体名称*/private String fontName;/*** 字体大小*/private Double fontHeight;/*** 字体颜色*/private Object fontColor;/*** 字体加粗*/private Boolean fontBold;/*** 字体斜体*/private Boolean fontItalic;/*** 字体下划线*/private Byte fontUnderLine;/*** 字体上标下标*/private Short fontTypeOffset;/*** 字体删除线*/private Boolean fontStrikeout;/*** 背景颜色*/private Object backgroundColor;/*** 上边框线条类型*/private BorderStyle borderTop;/*** 右边框线条类型*/private BorderStyle borderRight;/*** 下边框线条类型*/private BorderStyle borderBottom;/*** 左边框线条类型*/private BorderStyle borderLeft;/*** 上边框线条颜色*/private Object topBorderColor;/*** 上边框线条颜色*/private Object rightBorderColor;/*** 下边框线条颜色*/private Object bottomBorderColor;/***/private Object leftBorderColor;/*** 水平对齐方式*/private HorizontalAlignment horizontalAlignment;/*** 垂直对齐方式*/private VerticalAlignment verticalAlignment;/*** 自动换行方式*/private Boolean wrapText;/*** 生成字体名称样式信息** @param sheetName sheet页名称* @param rowIndex 行号* @param columnIndex 列号* @param fontName 字体名称(默认宋体)* @return*/public static CellStyleModel createFontNameCellStyleModel(String sheetName, int rowIndex, int columnIndex, String fontName) {return createFontCellStyleModel(sheetName, rowIndex, columnIndex, fontName, null, null, null, null, null, null, null);}/*** 生成字体名称大小信息** @param sheetName sheet页名称* @param rowIndex 行号* @param columnIndex 列号* @param fontHeight 字体大小* @return*/public static CellStyleModel createFontHeightCellStyleModel(String sheetName, int rowIndex, int columnIndex, Double fontHeight) {return createFontCellStyleModel(sheetName, rowIndex, columnIndex, null, fontHeight, null, null, null, null, null, null);}/*** 得到RBG自定义颜色** @param redNum 红色数值* @param greenNum 绿色数值* @param blueNum 蓝色数值* @return*/public static XSSFColor getRGBColor(int redNum, int greenNum, int blueNum) {XSSFColor color = new XSSFColor(new byte[]{(byte) redNum, (byte) greenNum, (byte) blueNum}, new DefaultIndexedColorMap());return color;}/*** 生成字体颜色样式信息(支持自定义RGB颜色)** @param sheetName sheet页名称* @param rowIndex 行号* @param columnIndex 列号* @param redNum 红色数值* @param greenNum 绿色数值* @param blueNum 蓝色数值* @return*/public static CellStyleModel createFontColorCellStyleModel(String sheetName, int rowIndex, int columnIndex, int redNum, int greenNum, int blueNum) {XSSFColor fontColor = getRGBColor(redNum, greenNum, blueNum);return createFontColorCellStyleModel(sheetName, rowIndex, columnIndex, fontColor);}/*** 生成字体颜色样式信息** @param sheetName sheet页名称* @param rowIndex 行号* @param columnIndex 列号* @param fontColor 字体颜色* @return*/public static CellStyleModel createFontColorCellStyleModel(String sheetName, int rowIndex, int columnIndex, Object fontColor) {return createFontCellStyleModel(sheetName, rowIndex, columnIndex, null, null, fontColor, null, null, null, null, null);}/*** 生成字体加粗样式信息** @param sheetName sheet页名称* @param rowIndex 行号* @param columnIndex 列号* @param fontBold 字体加粗* @return*/public static CellStyleModel createFontBoldCellStyleModel(String sheetName, int rowIndex, int columnIndex, Boolean fontBold) {return createFontCellStyleModel(sheetName, rowIndex, columnIndex, null, null, null, fontBold, null, null, null, null);}/*** 生成字体斜体样式信息** @param sheetName sheet页名称* @param rowIndex 行号* @param columnIndex 列号* @param fontItalic 字体斜体* @return*/public static CellStyleModel createFontItalicCellStyleModel(String sheetName, int rowIndex, int columnIndex, Boolean fontItalic) {return createFontCellStyleModel(sheetName, rowIndex, columnIndex, null, null, null, null, fontItalic, null, null, null);}/*** 生成字体下划线样式信息** @param sheetName sheet页名称* @param rowIndex 行号* @param columnIndex 列号* @param fontUnderLine 字体下划线* @return*/public static CellStyleModel createFontUnderLineCellStyleModel(String sheetName, int rowIndex, int columnIndex, Byte fontUnderLine) {return createFontCellStyleModel(sheetName, rowIndex, columnIndex, null, null, null, null, null, fontUnderLine, null, null);}/*** 生成字体上标下标样式信息** @param sheetName sheet页名称* @param rowIndex 行号* @param columnIndex 列号* @param fontTypeOffset 字体上标下标* @return*/public static CellStyleModel createFontTypeOffsetCellStyleModel(String sheetName, int rowIndex, int columnIndex, Short fontTypeOffset) {return createFontCellStyleModel(sheetName, rowIndex, columnIndex, null, null, null, null, null, null, fontTypeOffset, null);}/*** 生成字体删除线样式信息** @param sheetName sheet页名称* @param rowIndex 行号* @param columnIndex 列号* @param fontStrikeout 字体删除线* @return*/public static CellStyleModel createFontStrikeoutCellStyleModel(String sheetName, int rowIndex, int columnIndex, Boolean fontStrikeout) {return createFontCellStyleModel(sheetName, rowIndex, columnIndex, null, null, null, null, null, null, null, fontStrikeout);}/*** 生成字体样式信息** @param sheetName sheet页名称* @param rowIndex 行号* @param columnIndex 列号* @param fontName 字体名称(默认宋体)* @param fontHeight 字体大小* @param fontColor 字体颜色* @param fontItalic 字体斜体* @param fontBold 字体加粗* @param fontUnderLine 字体下划线* @param fontTypeOffset 字体上标下标* @param fontStrikeout 字体删除线* @return*/public static CellStyleModel createFontCellStyleModel(String sheetName, int rowIndex, int columnIndex, String fontName, Double fontHeight, Object fontColor, Boolean fontBold, Boolean fontItalic, Byte fontUnderLine, Short fontTypeOffset, Boolean fontStrikeout) {return createCellStyleModel(sheetName, rowIndex, columnIndex, fontName, fontHeight, fontColor, fontBold, fontItalic, fontUnderLine, fontTypeOffset, fontStrikeout, null);}/*** 生成背景颜色样式信息** @param sheetName sheet页名称* @param rowIndex 行号* @param columnIndex 列号* @param backgroundColor 背景颜色* @return*/public static CellStyleModel createBackgroundColorCellStyleModel(String sheetName, int rowIndex, int columnIndex, Object backgroundColor) {return createCellStyleModel(sheetName, rowIndex, columnIndex, null, null, null, null, null, null, null, null, backgroundColor);}/*** 生成背景颜色样式信息(支持自定义RGB颜色)** @param sheetName sheet页名称* @param rowIndex 行号* @param columnIndex 列号* @param redNum 红色数值* @param greenNum 绿色数值* @param blueNum 蓝色数值* @return*/public static CellStyleModel createBackgroundColorCellStyleModel(String sheetName, int rowIndex, int columnIndex, int redNum, int greenNum, int blueNum) {XSSFColor backgroundColor = getRGBColor(redNum, greenNum, blueNum);return createBackgroundColorCellStyleModel(sheetName, rowIndex, columnIndex, backgroundColor);}/*** 生成样式信息** @param sheetName sheet页名称* @param rowIndex 行号* @param columnIndex 列号* @param fontName 字体名称(宋体)* @param fontHeight 字体大小* @param fontColor 字体颜色* @param fontBold 字体加粗* @param fontItalic 字体斜体* @param fontUnderLine 字体下划线* @param fontTypeOffset 字体上标下标* @param fontStrikeout 字体删除线* @param backgroundColor 背景颜色* @return*/public static CellStyleModel createCellStyleModel(String sheetName, int rowIndex, int columnIndex, String fontName, Double fontHeight, Object fontColor, Boolean fontBold, Boolean fontItalic, Byte fontUnderLine, Short fontTypeOffset, Boolean fontStrikeout, Object backgroundColor) {return createCellStyleModel(sheetName, rowIndex, columnIndex, fontName, fontHeight, fontColor, fontBold, fontItalic, fontUnderLine, fontTypeOffset, fontStrikeout, backgroundColor, null, null, null, null, null, null, null, null);}/*** 生成上边框线条颜色样式信息** @param sheetName sheet页名称* @param rowIndex 行号* @param columnIndex 列号* @param topBorderColor 上边框线条颜色* @return*/public static CellStyleModel createTopBorderColorCellStyleModel(String sheetName, int rowIndex, int columnIndex, Object topBorderColor) {return createBorderColorCellStyleModel(sheetName, rowIndex, columnIndex, topBorderColor, null, null, null);}/*** 生成右边框线条颜色样式信息** @param sheetName sheet页名称* @param rowIndex 行号* @param columnIndex 列号* @param rightBorderColor 右边框线条颜色* @return*/public static CellStyleModel createRightBorderColorCellStyleModel(String sheetName, int rowIndex, int columnIndex, Object rightBorderColor) {return createBorderColorCellStyleModel(sheetName, rowIndex, columnIndex, null, rightBorderColor, null, null);}/*** 生成下边框线条颜色样式信息** @param sheetName sheet页名称* @param rowIndex 行号* @param columnIndex 列号* @param bottomBorderColor 下边框线条颜色* @return*/public static CellStyleModel createBottomBorderColorCellStyleModel(String sheetName, int rowIndex, int columnIndex, Object bottomBorderColor) {return createBorderColorCellStyleModel(sheetName, rowIndex, columnIndex, null, null, bottomBorderColor, null);}/*** 生成左边框线条颜色样式信息** @param sheetName sheet页名称* @param rowIndex 行号* @param columnIndex 列号* @param leftBorderColor 左边框线条颜色* @return*/public static CellStyleModel createLeftBorderColorCellStyleModel(String sheetName, int rowIndex, int columnIndex, Object leftBorderColor) {return createBorderColorCellStyleModel(sheetName, rowIndex, columnIndex, null, null, null, leftBorderColor);}/*** 生成上边框线条类型样式信息** @param sheetName sheet页名称* @param rowIndex 行号* @param columnIndex 列号* @param borderTop 上边框线条类型* @return*/public static CellStyleModel createTopBorderLineTypeCellStyleModel(String sheetName, int rowIndex, int columnIndex, BorderStyle borderTop) {return createBorderLineTypeCellStyleModel(sheetName, rowIndex, columnIndex, borderTop, null, null, null);}/*** 生成右边框线条类型样式信息** @param sheetName sheet页名称* @param rowIndex 行号* @param columnIndex 列号* @param borderRight 右边框线条类型* @return*/public static CellStyleModel createRightBorderLineTypeCellStyleModel(String sheetName, int rowIndex, int columnIndex, BorderStyle borderRight) {return createBorderLineTypeCellStyleModel(sheetName, rowIndex, columnIndex, null, borderRight, null, null);}/*** 生成下边框线条类型样式信息** @param sheetName sheet页名称* @param rowIndex 行号* @param columnIndex 列号* @param borderBottom 下边框线条类型* @return*/public static CellStyleModel createBottomBorderLineTypeCellStyleModel(String sheetName, int rowIndex, int columnIndex, BorderStyle borderBottom) {return createBorderLineTypeCellStyleModel(sheetName, rowIndex, columnIndex, null, null, borderBottom, null);}/*** 生成左边框线条类型样式信息** @param sheetName sheet页名称* @param rowIndex 行号* @param columnIndex 列号* @param borderLeft 左边框线条类型* @return*/public static CellStyleModel createLeftBorderLineTypeCellStyleModel(String sheetName, int rowIndex, int columnIndex, BorderStyle borderLeft) {return createBorderLineTypeCellStyleModel(sheetName, rowIndex, columnIndex, null, null, null, borderLeft);}/*** 生成边框线条颜色样式信息** @param sheetName sheet页名称* @param rowIndex 行号* @param columnIndex 列号* @param borderColor 边框线条颜色* @return*/public static CellStyleModel createBorderColorCellStyleModel(String sheetName, int rowIndex, int columnIndex, Object borderColor) {return createBorderCellStyleModel(sheetName, rowIndex, columnIndex, null, borderColor);}/*** 生成边框线条颜色样式信息** @param sheetName sheet页名称* @param rowIndex 行号* @param columnIndex 列号* @param topBorderColor 上边框线条颜色* @param rightBorderColor 右边框线条颜色* @param bottomBorderColor 下边框线条颜色* @param leftBorderColor 左边框线条颜色* @return*/public static CellStyleModel createBorderColorCellStyleModel(String sheetName, int rowIndex, int columnIndex, Object topBorderColor, Object rightBorderColor, Object bottomBorderColor, Object leftBorderColor) {return createBorderCellStyleModel(sheetName, rowIndex, columnIndex, null, null, null, null, topBorderColor, rightBorderColor, bottomBorderColor, leftBorderColor);}/*** 生成边框线条类型样式信息** @param sheetName sheet页名称* @param rowIndex 行号* @param columnIndex 列号* @param borderLineType 边框线条类型* @return*/public static CellStyleModel createBorderLineTypeCellStyleModel(String sheetName, int rowIndex, int columnIndex, BorderStyle borderLineType) {return createBorderCellStyleModel(sheetName, rowIndex, columnIndex, borderLineType, null);}/*** 生成边框线条类型样式信息** @param sheetName sheet页名称* @param rowIndex 行号* @param columnIndex 列号* @param borderTop 上边框线条类型* @param borderRight 右边框线条类型* @param borderBottom 下边框线条类型* @param borderLeft 左边框线条类型* @return*/public static CellStyleModel createBorderLineTypeCellStyleModel(String sheetName, int rowIndex, int columnIndex, BorderStyle borderTop, BorderStyle borderRight, BorderStyle borderBottom, BorderStyle borderLeft) {return createBorderCellStyleModel(sheetName, rowIndex, columnIndex, borderTop, borderRight, borderBottom, borderLeft, null, null, null, null);}/*** 生成边框样式信息** @param sheetName sheet页名称* @param rowIndex 行号* @param columnIndex 列号* @param borderLineType 边框线条类型* @param borderColor 边框线条颜色* @return*/public static CellStyleModel createBorderCellStyleModel(String sheetName, int rowIndex, int columnIndex, BorderStyle borderLineType, Object borderColor) {return createBorderCellStyleModel(sheetName, rowIndex, columnIndex, borderLineType, borderLineType, borderLineType, borderLineType, borderColor, borderColor, borderColor, borderColor);}/*** 生成边框样式信息** @param sheetName sheet页名称* @param rowIndex 行号* @param columnIndex 列号* @param borderTop 上边框线条类型* @param borderRight 右边框线条类型* @param borderBottom 下边框线条类型* @param borderLeft 左边框线条类型* @param topBorderColor 上边框线条颜色* @param rightBorderColor 右边框线条颜色* @param bottomBorderColor 下边框线条颜色* @param leftBorderColor 左边框线条颜色* @return*/public static CellStyleModel createBorderCellStyleModel(String sheetName, int rowIndex, int columnIndex, BorderStyle borderTop, BorderStyle borderRight, BorderStyle borderBottom, BorderStyle borderLeft, Object topBorderColor, Object rightBorderColor, Object bottomBorderColor, Object leftBorderColor) {return createCellStyleModel(sheetName, rowIndex, columnIndex, null, null, null, null, null, null, null, null, null, borderTop, borderRight, borderBottom, borderLeft, topBorderColor, rightBorderColor, bottomBorderColor, leftBorderColor);}/*** 生成样式信息** @param sheetName sheet页名称* @param rowIndex 行号* @param columnIndex 列号* @param fontName 字体名称(宋体)* @param fontHeight 字体大小* @param fontColor 字体颜色* @param fontBold 字体加粗* @param fontItalic 字体斜体* @param fontUnderLine 字体下划线* @param fontTypeOffset 字体上标下标* @param fontStrikeout 字体删除线* @param backgroundColor 背景颜色* @param borderTop 上边框线条类型* @param borderRight 右边框线条类型* @param borderBottom 下边框线条类型* @param borderLeft 左边框线条类型* @param topBorderColor 上边框线条颜色* @param rightBorderColor 右边框线条颜色* @param bottomBorderColor 下边框线条颜色* @param leftBorderColor 左边框线条颜色* @return*/public static CellStyleModel createCellStyleModel(String sheetName, int rowIndex, int columnIndex, String fontName, Double fontHeight, Object fontColor, Boolean fontBold, Boolean fontItalic, Byte fontUnderLine, Short fontTypeOffset, Boolean fontStrikeout, Object backgroundColor, BorderStyle borderTop, BorderStyle borderRight, BorderStyle borderBottom, BorderStyle borderLeft, Object topBorderColor, Object rightBorderColor, Object bottomBorderColor, Object leftBorderColor) {return createCellStyleModel(sheetName, rowIndex, columnIndex, fontName, fontHeight, fontColor, fontBold, fontItalic, fontUnderLine, fontTypeOffset, fontStrikeout, backgroundColor, borderTop, borderRight, borderBottom, borderLeft, topBorderColor, rightBorderColor, bottomBorderColor, leftBorderColor, null, null);}/*** 生成水平对齐方式信息** @param sheetName sheet页名称* @param rowIndex 行号* @param columnIndex 列号* @param horizontalAlignment 水平对齐方式* @return*/public static CellStyleModel createHorizontalAlignmentCellStyleModel(String sheetName, int rowIndex, int columnIndex, HorizontalAlignment horizontalAlignment) {return createAlignmentCellStyleModel(sheetName, rowIndex, columnIndex, horizontalAlignment, null);}/*** 生成垂直对齐方式信息** @param sheetName sheet页名称* @param rowIndex 行号* @param columnIndex 列号* @param verticalAlignment 垂直对齐方式* @return*/public static CellStyleModel createVerticalAlignmentCellStyleModel(String sheetName, int rowIndex, int columnIndex, VerticalAlignment verticalAlignment) {return createAlignmentCellStyleModel(sheetName, rowIndex, columnIndex, null, verticalAlignment);}/*** 生成对齐方式信息** @param sheetName sheet页名称* @param rowIndex 行号* @param columnIndex 列号* @param horizontalAlignment 水平对齐方式* @param verticalAlignment 垂直对齐方式* @return*/public static CellStyleModel createAlignmentCellStyleModel(String sheetName, int rowIndex, int columnIndex, HorizontalAlignment horizontalAlignment, VerticalAlignment verticalAlignment) {return createCellStyleModel(sheetName, rowIndex, columnIndex, null, null, null, null, null, null, null, null, null, null, null, null, null, null, null, null, null, horizontalAlignment, verticalAlignment);}/*** 生成样式信息** @param sheetName sheet页名称* @param rowIndex 行号* @param columnIndex 列号* @param fontName 字体名称(宋体)* @param fontHeight 字体大小* @param fontColor 字体颜色* @param fontBold 字体加粗* @param fontItalic 字体斜体* @param fontUnderLine 字体下划线* @param fontTypeOffset 字体上标下标* @param fontStrikeout 字体删除线* @param backgroundColor 背景颜色* @param borderTop 上边框线条类型* @param borderRight 右边框线条类型* @param borderBottom 下边框线条类型* @param borderLeft 左边框线条类型* @param topBorderColor 上边框线条颜色* @param rightBorderColor 右边框线条颜色* @param bottomBorderColor 下边框线条颜色* @param leftBorderColor 左边框线条颜色* @param horizontalAlignment 水平对齐方式* @param verticalAlignment 垂直对齐方式* @return*/public static CellStyleModel createCellStyleModel(String sheetName, int rowIndex, int columnIndex, String fontName, Double fontHeight, Object fontColor, Boolean fontBold, Boolean fontItalic, Byte fontUnderLine, Short fontTypeOffset, Boolean fontStrikeout, Object backgroundColor, BorderStyle borderTop, BorderStyle borderRight, BorderStyle borderBottom, BorderStyle borderLeft, Object topBorderColor, Object rightBorderColor, Object bottomBorderColor, Object leftBorderColor, HorizontalAlignment horizontalAlignment, VerticalAlignment verticalAlignment) {return createCellStyleModel(sheetName, rowIndex, columnIndex, fontName, fontHeight, fontColor, fontBold, fontItalic, fontUnderLine, fontTypeOffset, fontStrikeout, backgroundColor, borderTop, borderRight, borderBottom, borderLeft, topBorderColor, rightBorderColor, bottomBorderColor, leftBorderColor, horizontalAlignment, verticalAlignment, null);}/*** 生成自动换行样式信息** @param sheetName sheet页名称* @param rowIndex 行号* @param columnIndex 列号* @param wrapText 自动换行* @return*/public static CellStyleModel createWrapTextCellStyleModel(String sheetName, int rowIndex, int columnIndex, Boolean wrapText) {return createCellStyleModel(sheetName, rowIndex, columnIndex, null, null, null, null, null, null, null, null, null, null, null, null, null, null, null, null, null, null, null, wrapText);}/*** 生成样式信息** @param sheetName sheet页名称* @param rowIndex 行号* @param columnIndex 列号* @param fontName 字体名称(宋体)* @param fontHeight 字体大小* @param fontColor 字体颜色* @param fontBold 字体加粗* @param fontItalic 字体斜体* @param fontUnderLine 字体下划线* @param fontTypeOffset 字体上标下标* @param fontStrikeout 字体删除线* @param backgroundColor 背景颜色* @param borderTop 上边框线条类型* @param borderRight 右边框线条类型* @param borderBottom 下边框线条类型* @param borderLeft 左边框线条类型* @param topBorderColor 上边框线条颜色* @param rightBorderColor 右边框线条颜色* @param bottomBorderColor 下边框线条颜色* @param leftBorderColor 左边框线条颜色* @param horizontalAlignment 水平对齐方式* @param verticalAlignment 垂直对齐方式* @param wrapText 自动换行* @return*/public static CellStyleModel createCellStyleModel(String sheetName, int rowIndex, int columnIndex, String fontName, Double fontHeight, Object fontColor, Boolean fontBold, Boolean fontItalic, Byte fontUnderLine, Short fontTypeOffset, Boolean fontStrikeout, Object backgroundColor, BorderStyle borderTop, BorderStyle borderRight, BorderStyle borderBottom, BorderStyle borderLeft, Object topBorderColor, Object rightBorderColor, Object bottomBorderColor, Object leftBorderColor, HorizontalAlignment horizontalAlignment, VerticalAlignment verticalAlignment, Boolean wrapText) {CellStyleModel cellStyleModel = new CellStyleModel();//sheet页名称cellStyleModel.setSheetName(sheetName);//行号cellStyleModel.setRowIndex(rowIndex);//列号cellStyleModel.setColIndex(columnIndex);//设置字体样式//字体名称(比如宋体)fontName = fontName != null && StrUtil.equals(fontName, "") ? "宋体" : fontName;cellStyleModel.setFontName(fontName);//字体大小fontHeight = fontHeight != null && fontHeight <= 0 ? null : fontHeight;cellStyleModel.setFontHeight(fontHeight);//字体颜色fontColor = fontColor != null && (fontColor instanceof IndexedColors == false && fontColor instanceof XSSFColor == false)? null : fontColor;cellStyleModel.setFontColor(fontColor);//字体加粗cellStyleModel.setFontBold(fontBold);//字体斜体cellStyleModel.setFontItalic(fontItalic);//字体下划线fontUnderLine = fontUnderLine != null && (fontUnderLine != Font.U_NONE && fontUnderLine != Font.U_SINGLE && fontUnderLine != Font.U_DOUBLE&& fontUnderLine != Font.U_DOUBLE_ACCOUNTING && fontUnderLine != Font.U_SINGLE_ACCOUNTING) ? null : fontUnderLine;cellStyleModel.setFontUnderLine(fontUnderLine);//字体上标下标fontTypeOffset = fontTypeOffset != null && (fontTypeOffset != Font.SS_NONE && fontTypeOffset != Font.SS_SUB && fontTypeOffset != Font.SS_SUPER)? null : fontTypeOffset;cellStyleModel.setFontTypeOffset(fontTypeOffset);//字体删除线cellStyleModel.setFontStrikeout(fontStrikeout);//背景颜色backgroundColor = backgroundColor != null && (backgroundColor instanceof IndexedColors == false && backgroundColor instanceof XSSFColor == false)? null : backgroundColor;cellStyleModel.setBackgroundColor(backgroundColor);//边框样式//上边框线条类型cellStyleModel.setBorderTop(borderTop);//右边框线条类型cellStyleModel.setBorderRight(borderRight);//下边框线条类型cellStyleModel.setBorderBottom(borderBottom);//左边框线条类型cellStyleModel.setBorderLeft(borderLeft);//上边框颜色类型cellStyleModel.setTopBorderColor(topBorderColor);//右边框颜色类型cellStyleModel.setRightBorderColor(rightBorderColor);//下边框颜色类型cellStyleModel.setBottomBorderColor(bottomBorderColor);//左边框颜色类型cellStyleModel.setLeftBorderColor(leftBorderColor);//对齐方式//水平对齐方式cellStyleModel.setHorizontalAlignment(horizontalAlignment);//垂直对齐方式cellStyleModel.setVerticalAlignment(verticalAlignment);//自动换行cellStyleModel.setWrapText(wrapText);return cellStyleModel;}}
五、导出实现
public void exportPatrolWorkOrderInfo(String startTime, String endTime, HttpServletResponse response) {List<PatrolProcessDTO> allContentList = new ArrayList<>();//设置合并策略List<CellStyleModel> cellStyleList = new ArrayList<>();//根据时间获取工单List<PatrolProcessExportDTO> patrolProcessExportDTOList = analysisPatrolProcessExportInfo.analyzeExportProcessInfo(new ExportWorkOrderInfoDTO(startTime, endTime));if (!CollectionUtils.isEmpty(patrolProcessExportDTOList)) {patrolProcessExportDTOList = patrolProcessExportDTOList.stream().sorted(Comparator.comparing(PatrolProcessExportDTO::getProcessInstanceStartTime)).collect(Collectors.toList());int i = 1;for (PatrolProcessExportDTO patrolProcessExportDTO : patrolProcessExportDTOList) {Map<String, List<List<String>>> nfcMap = patrolProcessExportDTO.getNfcMap();if (!CollectionUtils.isEmpty(nfcMap)) {int finalI = i;nfcMap.forEach((k, v) -> {PatrolProcessDTO patrolProcessDTO = new PatrolProcessDTO();BeanUtils.copyProperties(patrolProcessExportDTO, patrolProcessDTO);patrolProcessDTO.setOrderNum(finalI);//设置打卡点情况if (v.size() == 0) {patrolProcessDTO.setContent(k + "未打卡");//设置单元格背景颜色cellStyleList.add(CellStyleModel.createBackgroundColorCellStyleModel("巡检工单", allContentList.size(), 4, IndexedColors.YELLOW));} else {patrolProcessDTO.setContent(getNfcInfo(v, k));}allContentList.add(patrolProcessDTO);});} else {PatrolProcessDTO patrolProcessDTO = new PatrolProcessDTO();BeanUtils.copyProperties(patrolProcessExportDTO, patrolProcessDTO);patrolProcessDTO.setOrderNum(i);allContentList.add(patrolProcessDTO);}i++;}}for (int i = 0; i <= allContentList.size(); i++) {for (int j = 0; j < 9; j++) {
// cellStyleList.add(CellStyleModel.createBorderCellStyleModel("巡检工单", i, j, BorderStyle.THIN, IndexedColors.BLACK));//设置换行策略cellStyleList.add(CellStyleModel.createWrapTextCellStyleModel("巡检工单", i, j, true));}}//向会话写入try {response.setContentType("application/vnd.ms-excel");response.setCharacterEncoding("utf-8");String fileName = URLEncoder.encode("巡检工单", "UTF-8"); //.replaceAll("\\+", "%20")response.setHeader("Content-disposition", "attachment;filename=" + fileName + ".xlsx");EasyExcel.write(response.getOutputStream(), PatrolProcessDTO.class).excelType(ExcelTypeEnum.XLSX).autoCloseStream(Boolean.TRUE)// 添加自定义处理程序,相当于Spring的AOP切面.registerWriteHandler(new ExcelFillCellMergeStrategy(0, 8, new ArrayList<>())).registerWriteHandler(new CustomCellStyleHandler(cellStyleList)).sheet("巡检工单").doWrite(allContentList);} catch (Exception e) {log.error("导出出错-{}", ExceptionUtils.getStackTrace(e));renderString(response, JSON.toJSONString(new ResultInfo().error(SystemError.SYS_10055)));}}
备注:由于合并策略是自定义得,在效率上没法保证,当牵扯到大数据量合并导出时,用时较长。
相关文章:

利用easy excel 实现文件导出
一.创建实体类 package com.siact.product.jwp.module.report.dto;import com.alibaba.excel.annotation.ExcelProperty; import com.alibaba.excel.annotation.write.style.ColumnWidth; import com.alibaba.excel.annotation.write.style.ContentRowHeight; import com.alib…...
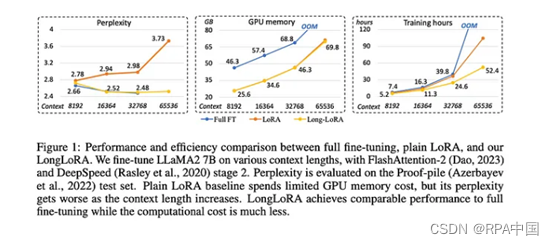
LongLoRA:超长上下文,大语言模型高效微调方法
麻省理工学院和香港中文大学联合发布了LongLoRA,这是一种全新的微调方法,可以增强大语言模型的上下文能力,而无需消耗大量算力资源。 通常,想增加大语言模型的上下文处理能力,需要更多的算力支持。例如,将…...
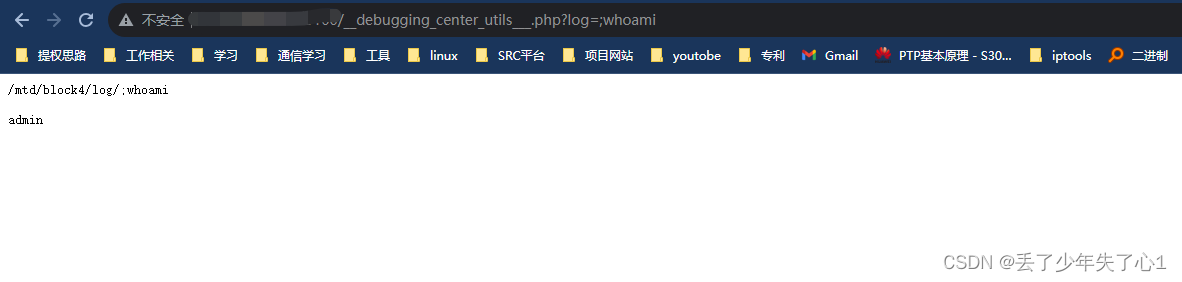
【漏洞复现】某 NVR 视频存储管理设备远程命令执行
漏洞描述 NUUO NVR是中国台湾NUUO公司旗下的一款网络视频记录器,该设备存在远程命令执行漏洞,攻击者可利用该漏洞执行任意命令,进而获取服务器的权限。 免责声明 技术文章仅供参考,任何个人和组织使用网络应当遵守宪法法律&am…...

若依前端-应用路径发布和使用
官网的路径:前端手册 | RuoYi 应用路径 有些特殊情况需要部署到子路径下,例如:https://www.ruoyi.vip/admin,可以按照下面流程修改。 1、修改vue.config.js中的publicPath属性 publicPath: process.env.NODE_ENV "produ…...
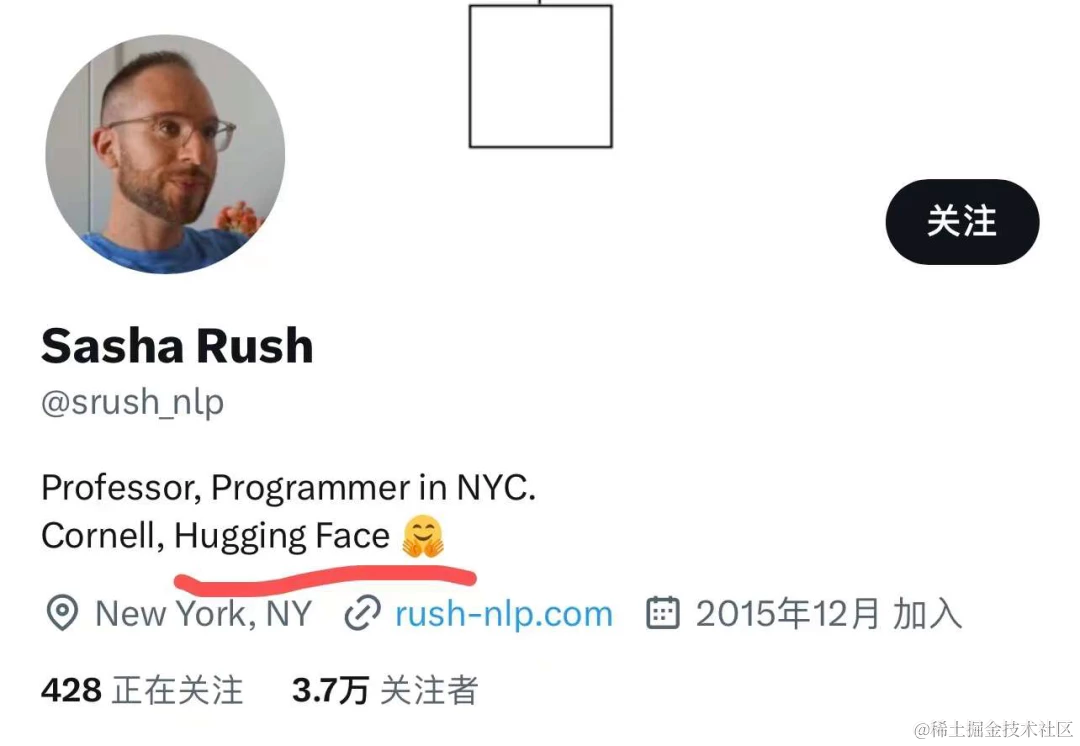
Mojo 正式发布,Rust 能否与之匹敌?
9 月 7 日,Modular 公司宣布正式发布 Mojo:Mojo 现在已经开放本地下载——初步登陆 Linux 系统,并将很快提供 Mac 与 Windows 版本。据介绍,Mojo 最初的目标是比 Python 快 35000 倍,近日该团队表示,Mojo 将…...

opencv实现抠图,图像拼接,图像融合
在OpenCV中,你可以使用图像拼接、抠图和将图像的一部分放在另一张图片的指定位置。以下是示例代码,演示如何执行这些操作: 图像拼接 要将两张图像拼接在一起,你可以使用 cv::hconcat(水平拼接)和 cv::vco…...
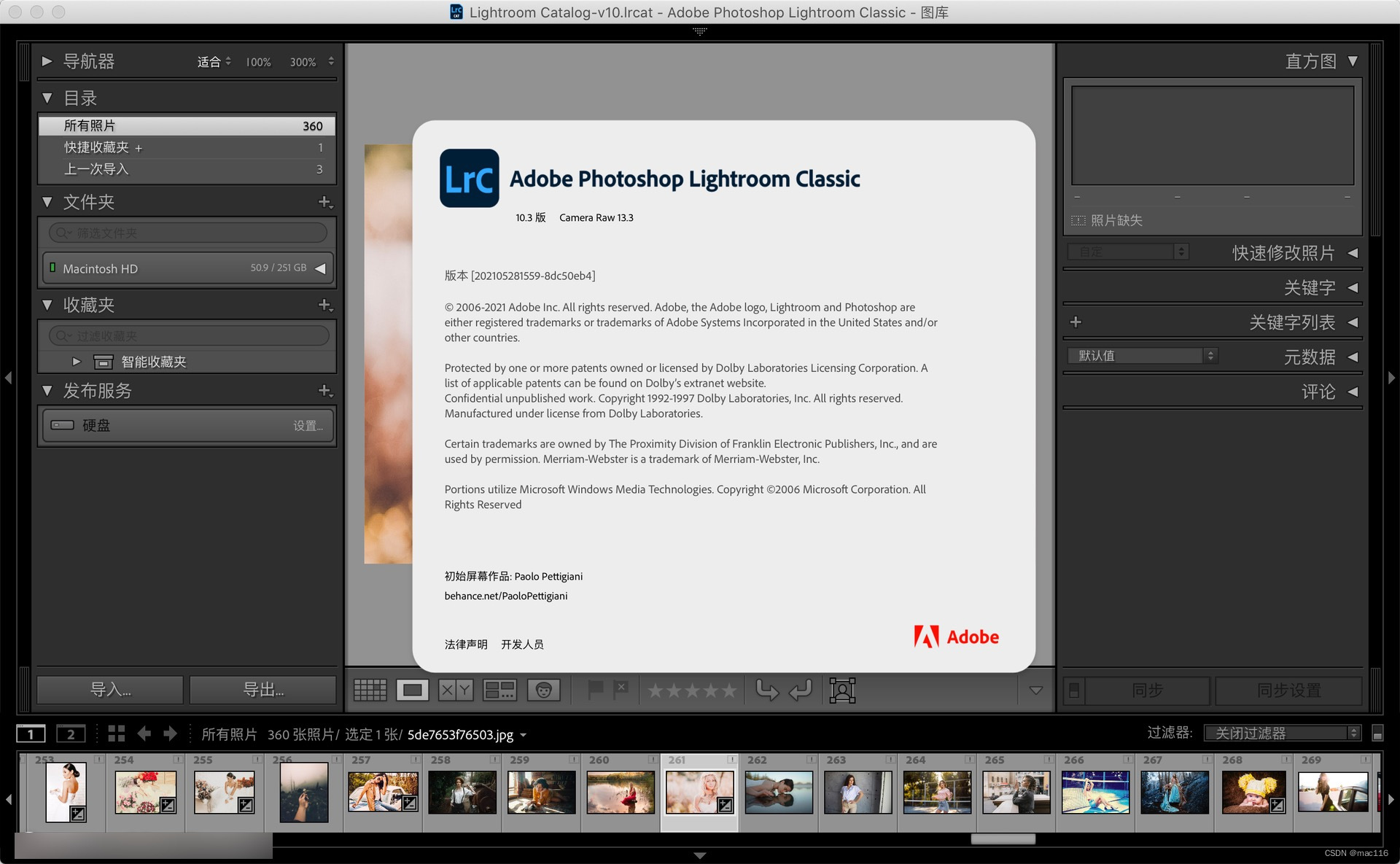
照片处理软件Lightroom Classic mac中文版功能介绍(Lrc2021)
Lightroom Classic 2022 mac是一款桌面编辑工具,lrc2021 mac包括提亮颜色、使灰暗的摄影更加生动、删除瑕疵、将弯曲的画面拉直等。您可以在电脑桌面上轻松整理所有照片。使用Lightroom Classic, 轻松整理编辑照片,为您的作品锦上添花。 Ligh…...
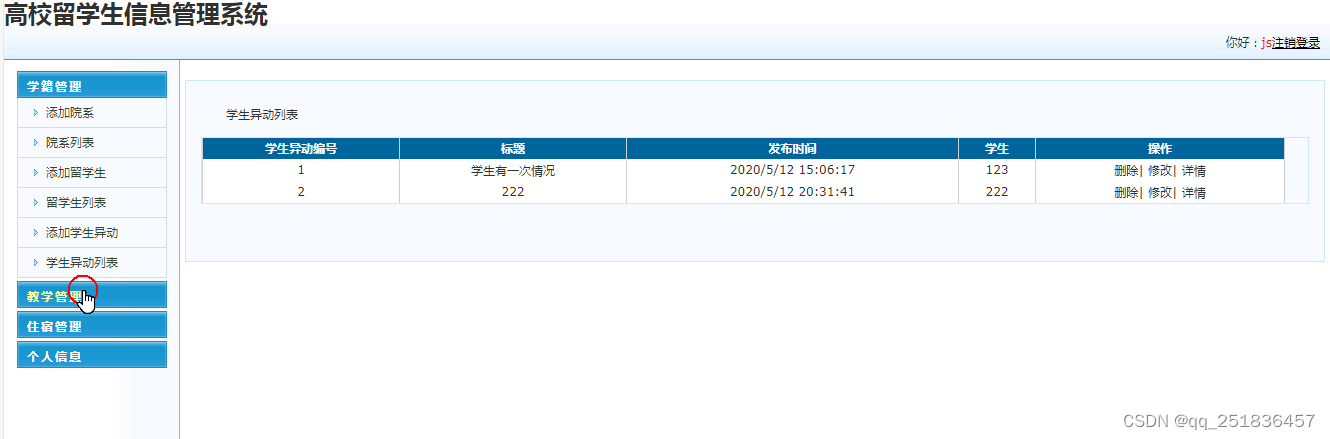
asp.net高校留学生信息管理系统VS开发sqlserver数据库web结构c#编程Microsoft Visual Studio
一、源码特点 asp.net 高校留学生信息管理系统 是一套完善的web设计管理系统,系统具有完整的源代码和数据库,系统主要采用B/S模式开发。开发环境为vs2010,数据库为sqlserver2008,使 用c#语言开发 asp.net留学生信息管理系…...
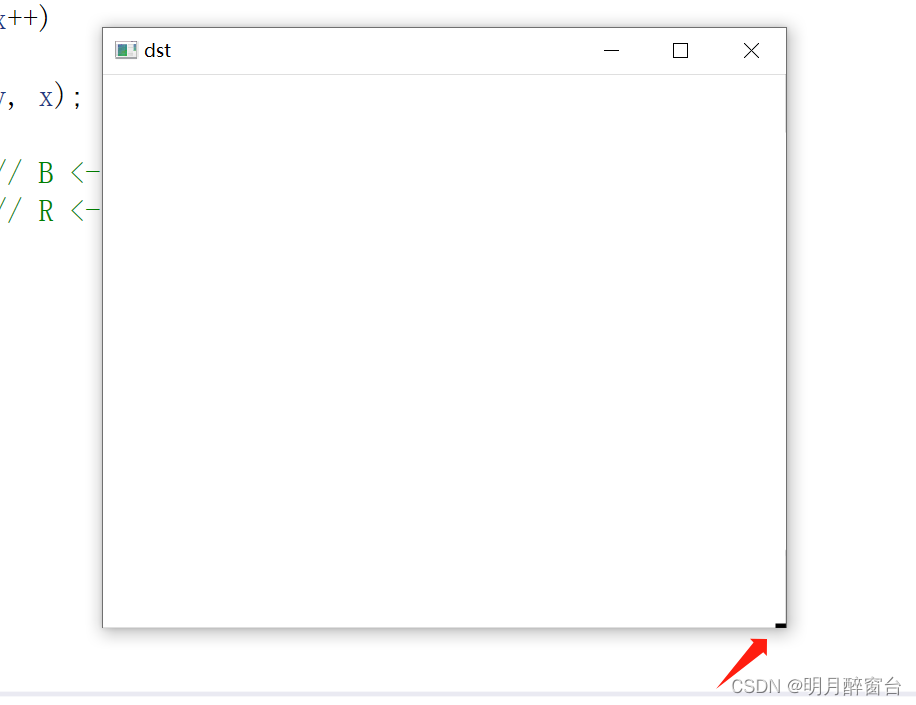
C# - Opencv应用(1) 之VS下环境配置详解
C# - Opencv应用(1) 之VS下环境配置详解 有时候,单纯c#做前端时会联合C实现的dll来落地某些功能由于有时候会用C - Opencv实现算法后封装成dll,但是有时候会感觉麻烦,不如直接通过C#直接调用Opencv在此慢慢总结下C# -…...
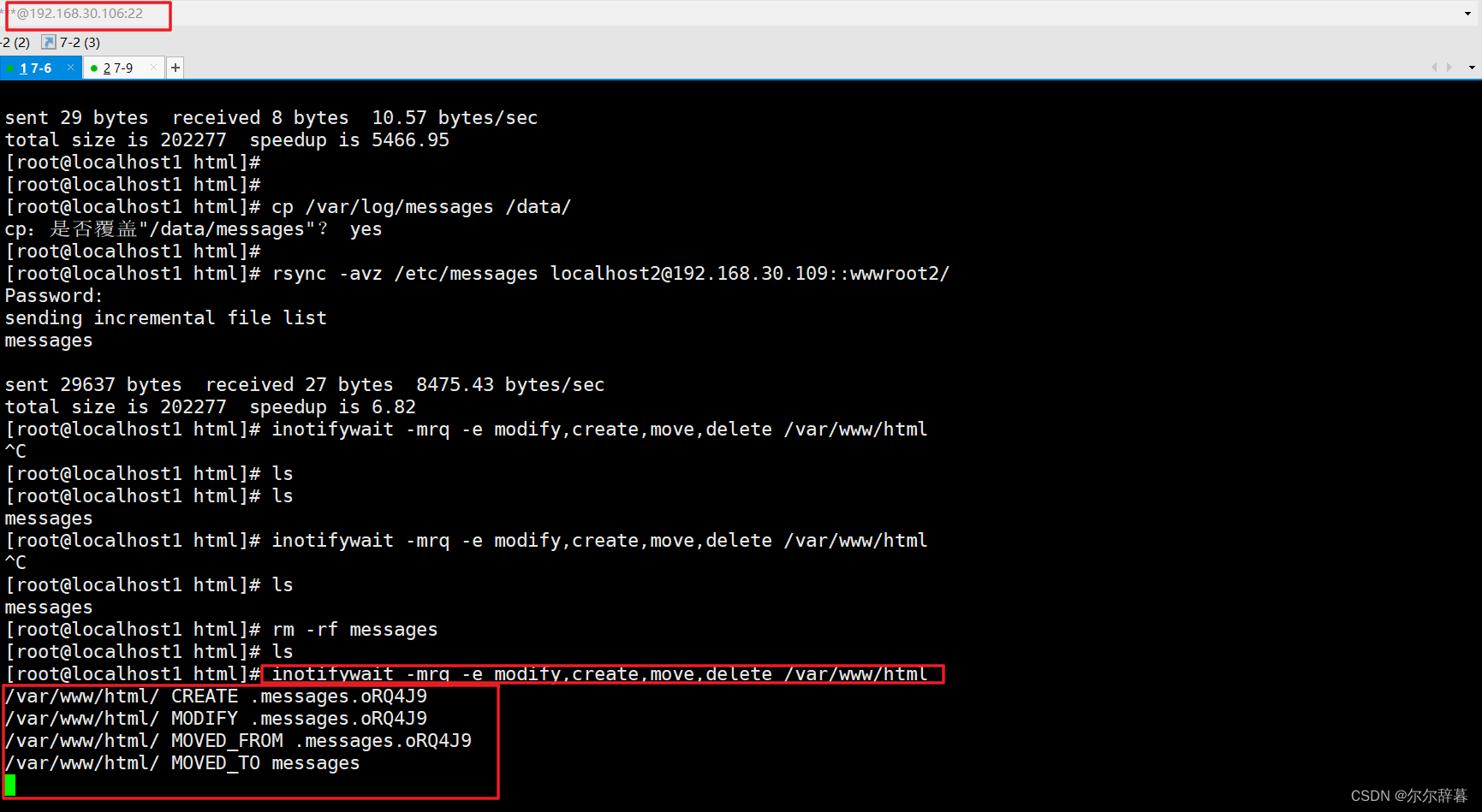
rsync 远程同步实现快速、安全、高效的异地备份
目录 1 rsync 远程同步 1.1 rsync是什么? 1.2 rsync同步方式 1.3 rsync的特性 1.4 rsync的应用场景 1.5 rsync与cp、scp对比 1.6 rsync同步源 2 配置rsync源服务器 2.1 建立/etc/rsyncd.conf 配置文件 3 发起端 4 发起端配置 rsyncinotify 4.1 修改rsync…...
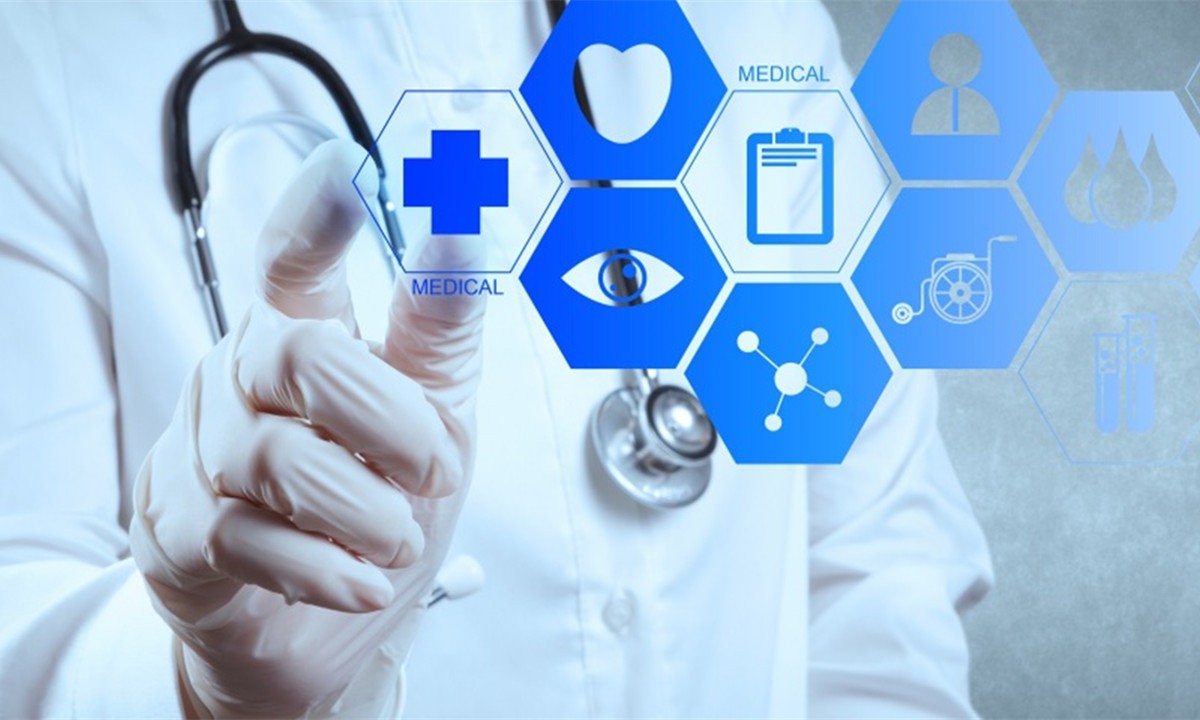
医学访问学者面试技巧
医学访问学者面试是一个非常重要的环节,它决定了你是否能够获得这个宝贵的机会去国外的大学或研究机构学习和研究。在这篇文章中,知识人网小编将分享一些关于医学访问学者面试的技巧,帮助你在面试中表现出色。 1. 准备充分 在参加医学访问学…...
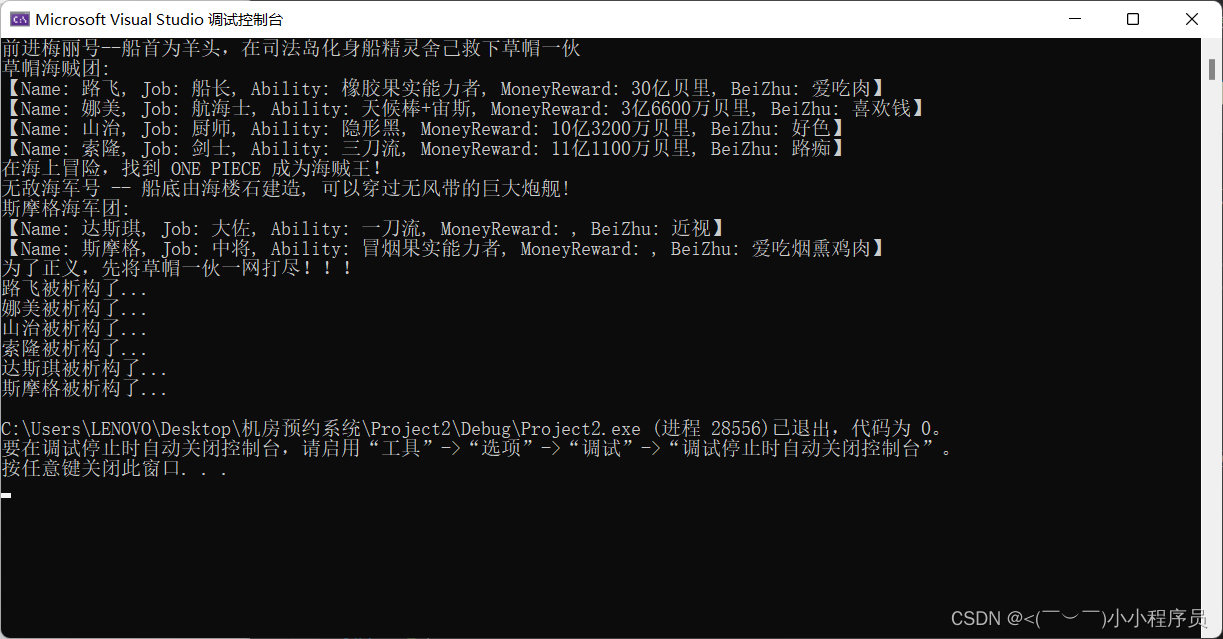
【19】c++设计模式——>桥接模式
桥接模式的定义 C的桥接模式(Bridge Pattern)是一种结构型设计模式,它将抽象部分与实现部分分离,使得它们可以独立地变化。桥接模式的核心思想是利用组合关系代替继承关系,将系统划分成多个独立的、功能不同的类层次结…...
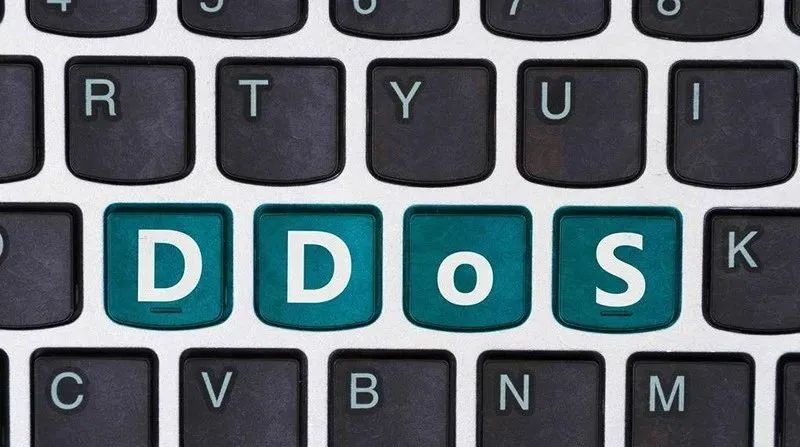
网络安全:六种常见的网络攻击手段
1、什么是VPN服务? 虚拟专用网络(或VPN)是您的设备与另一台计算机之间通过互联网的安全连接。VPN服务可用于在离开办公室时安全地访问工作计算机系统。但它们也常用于规避政府审查制度,或者在电影流媒体网站上阻止位置封锁&#…...
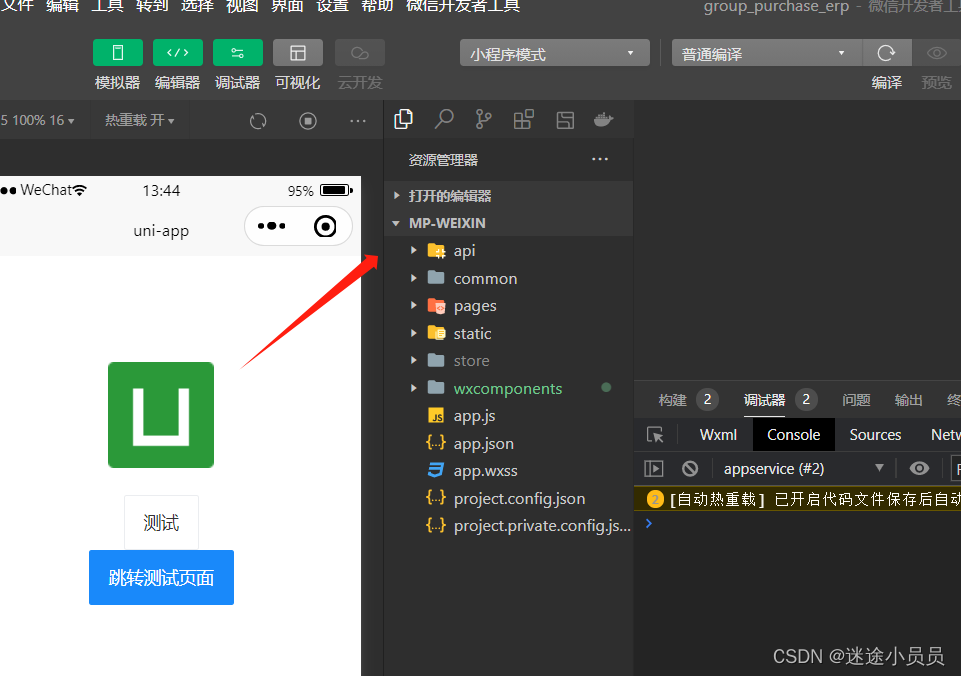
使用HbuilderX运行uniapp中小程序项目
下载HbuilderX,下载链接: HBuilderX-高效极客技巧 导入相关项目。下载微信开发者工具。使用微信开发者工具打开:注意:如果是第一次使用,需要先配置小程序ide的相关路径,才能运行成功。如下图,需…...
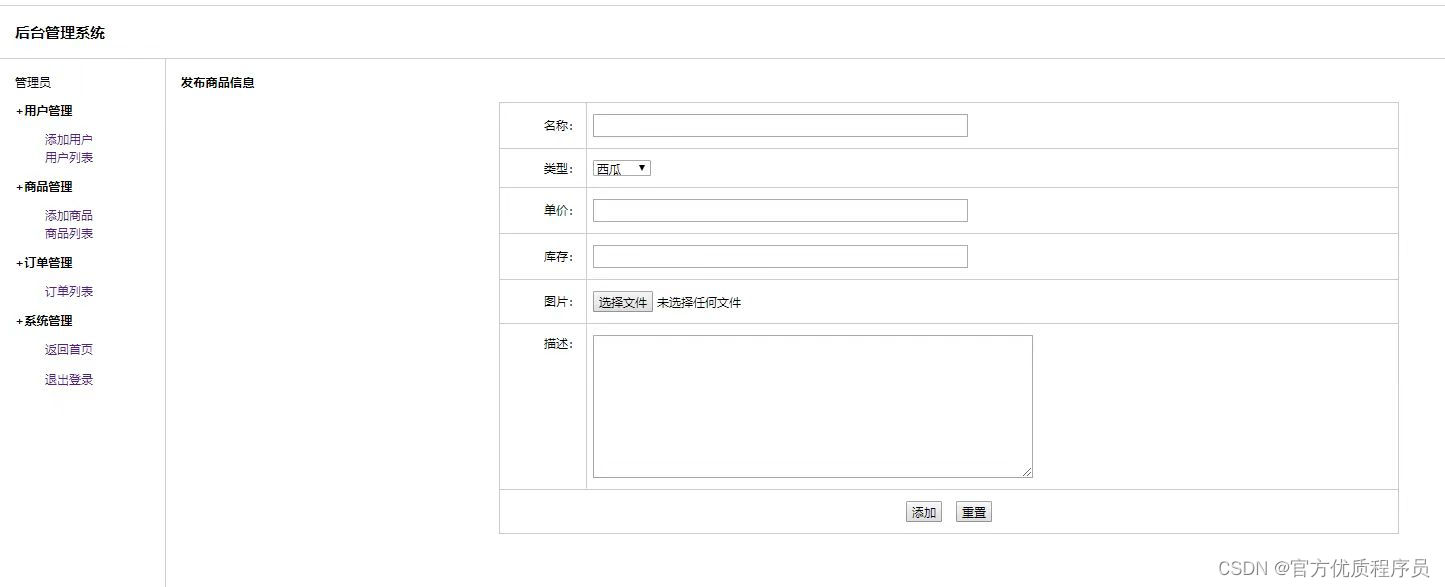
基于PHP的水果商城网站,mysql数据库,前台+后台,原生PHP,PHP study(小皮),完美运行,有一万字报告。
目录 演示视频 基本介绍 论文目录 购买流程 系统截图 演示视频 基本介绍 基于PHP的水果商城网站,mysql数据库,前台后台,原生PHP,PHP study(小皮),完美运行,有一万字报告。 前…...
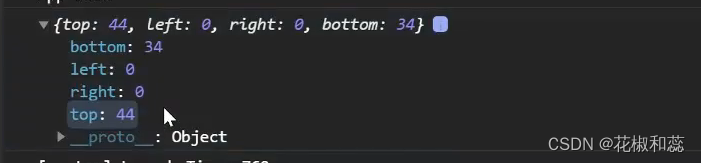
【uniapp】自定义导航栏时,设置安全距离,适配不同机型
1、在pages.json中,给对应的页面设置自定义导航栏样式 {"path": "pages/index/index","style": {"navigationStyle": "custom","navigationBarTextStyle": "white","navigationBarTitl…...

JAVA经典百题之数组逆序输出
题目:将一个数组逆序输出。 程序分析 要将一个数组逆序输出,即将数组中的元素顺序颠倒过来,可以使用多种方法。基本思路是创建一个新数组或修改原数组,将元素的顺序颠倒。 方法1: 创建新数组实现 思路 创建一个新的数组,长度…...

vue run dev 配置nginx
由于项目有多个vue项目,在开发过程中需要联调,而不是每次发布后再联调. 比如有2个项目: main-project(主项目),test1-project. 由于是多项目,每个项目相当于有一个独立的域,这里test1-project设置域名称为test1 vue修改 在vite.config.ts中配置一个base:"/test1/"…...

Python实现RNN算法对MFCC特征的简单语音识别
Python实现RNN算法对MFCC特征的简单语音识别 1、实现步骤 借助深度学习库 TensorFlow/Keras 来构建模型 1.对标签进行编码,将文本标签转换为整数标签。 2.对 MFCC 特征数据进行填充或截断,使其长度一致,以便于输入到 RNN 模型中 3.如果是二维数据需要转成三维: Simpl…...
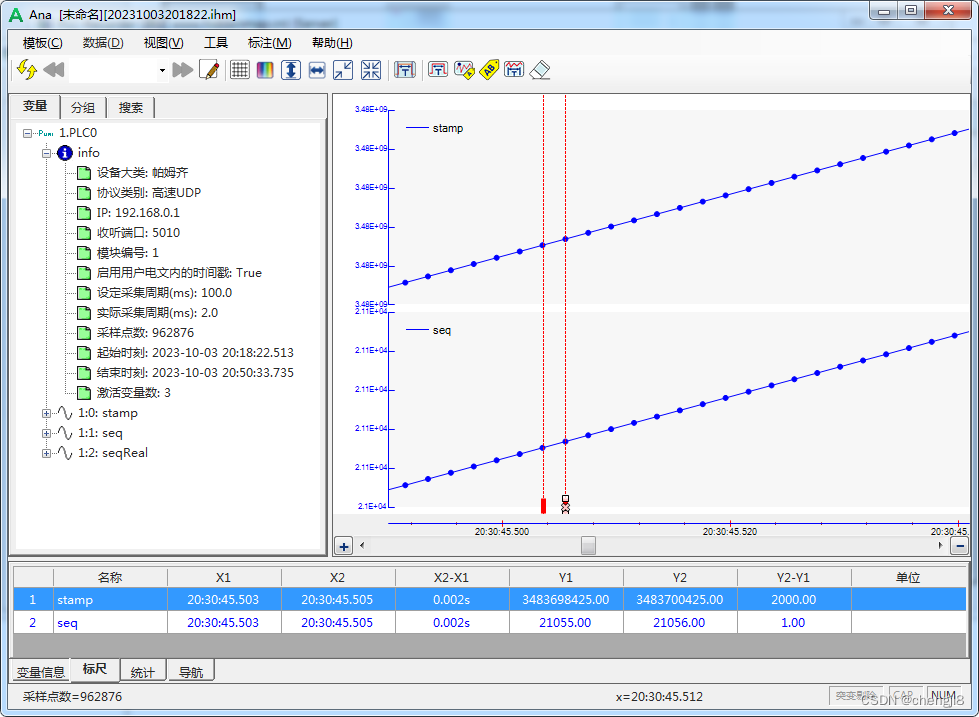
借助PLC-Recorder,汇川中型PLC(AM、AC系列,CODESYS平台)2ms高速采集的方法
高速数据采集要保证速度,也要保证时刻的准确性。在windows系统里,时间稳定性是个很难的问题。如果PLC发送的数据里带有时间信息,则可以由PLC来保证采样周期的稳定性。 从V2.12版本开始,PLC-Recorder软件可以处理发送电文里的时间…...
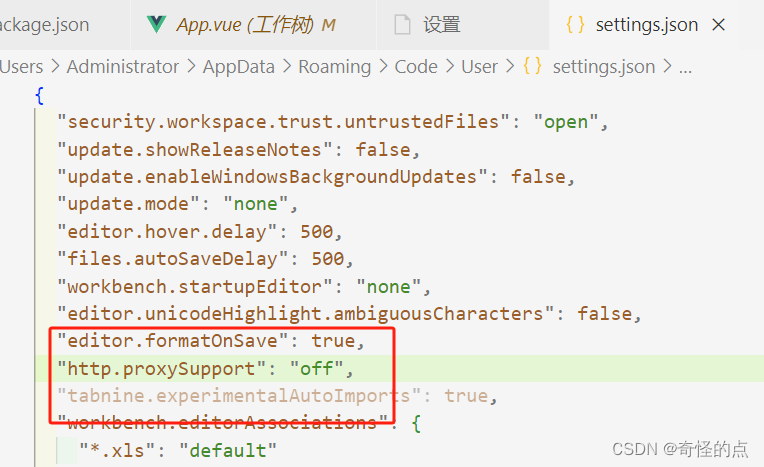
vscode package.json文件开头的{总是提升警告
警告如下 Problems loading reference https://json.schemastore.org/stylelintrc.json: Unable to load schema from https://json.schemastore.org/stylelintrc.json: read ECONNRESET. 解决如下 在设置(settings.json)里 新增一条属性 "ht…...
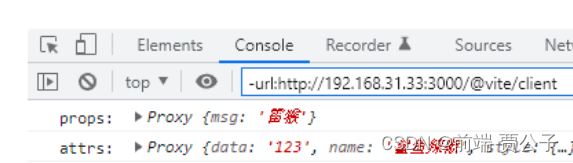
$attrs 和 $listeners (vue2vue3)
目录 透传 Attributes Attributes 继承 对 class 和 style 的合并 v-on 监听器继承 深层组件继承 禁用 Attributes 继承 多根节点的 Attributes 继承 vue2 $attrs 和 $listeners $attrs 概念说明 $attrs 案例 $listeners 概念说明 $listeners案例 vue3 $attr…...
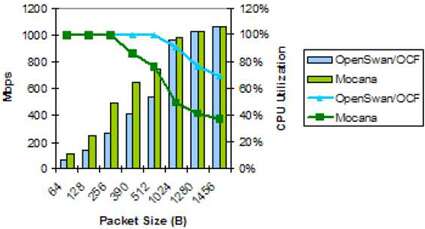
嵌入式系统中的加密性能:第2部分
本系列的第1部分讨论了影响系统级密码性能的硬件和软件变量。现在,在第2部分中,我们将重点介绍两种用于测量高级后备加速器性能的方法:1)驱动器级加速器测试以识别加速器或SoC内存带宽约束,以及2)应用程序/…...
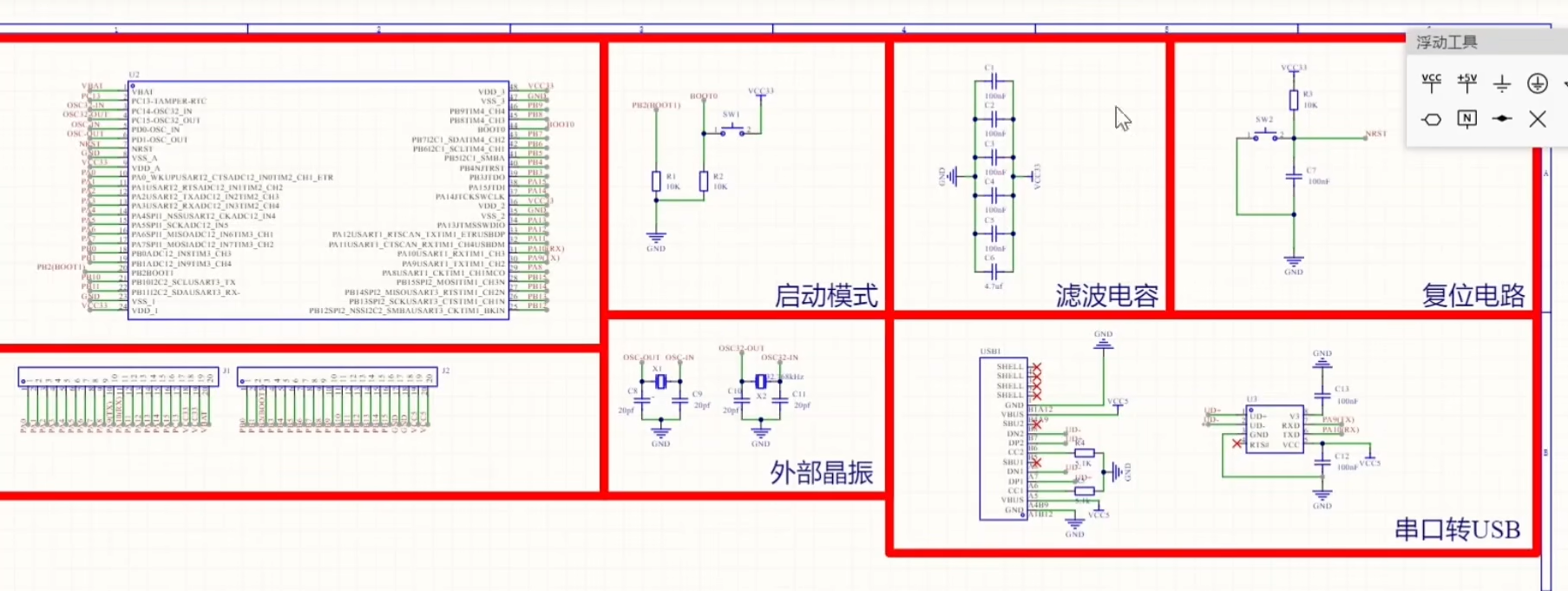
STM32F103 最小系统 PCB 设计与原理
这篇文章是来自我学习: 带着你从手册开始画板 STM最小系统板教程系列(一)_哔哩哔哩_bilibili 这套教程的笔记,同时本文中也参考了其他教程以及我遇到的困惑与自答,最终汇总。 一、单片机最小系统 单片机最小系统是由…...

JVM篇---第十一篇
系列文章目录 文章目录 系列文章目录一、如何选择垃圾收集器?二、什么是类加载器?三、什么是 tomcat 类加载机制?一、如何选择垃圾收集器? 如果你的堆大小不是很大(比如 100MB ),选择串行收集器一般是效率最高的。 参数: -XX:+UseSerialGC 。如果你的应用运行在单核的机…...
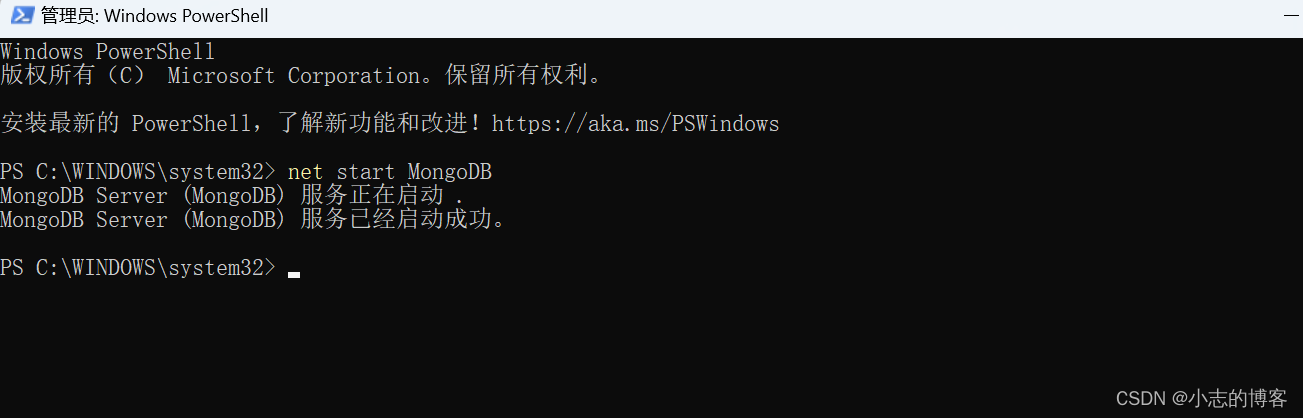
MongoDB——window11安装mongodb5.0.21版本服务端(图解版)
目录 一、mongodb官网下载地址二、安装步骤三、配置环境变量四、运行mongodb 一、mongodb官网下载地址 mongodb官网下载地址:https://www.mongodb.com/try/download/community 二、安装步骤 双击运行下载好的mongodb-windows-x86_64-5.0.21-signed.msi安装包&am…...

第1次 更多的bash shell命令
1.检测程序 程序都是进程在运行,进程里面有很多线程,面试经常会问进程和线程的区别,线程可以访问另一个线程的什么什么的,这些我都听腻了,区别就是进程会分配程序需要的空间,创建线程需要的资源,…...
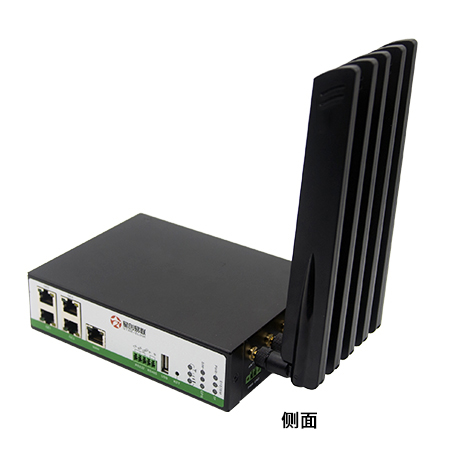
工业路由器项目应用(4g+5g两种工业路由器项目介绍)
引言: 随着工业智能化的不断发展,工业路由器在各个领域的应用越来越广泛。本文将介绍两个工业路由器项目的应用案例,一个是使用SR500 4g工业路由器,另一个是使用SR800 5g工业路由器。 详情:https://www.key-iot.com/i…...
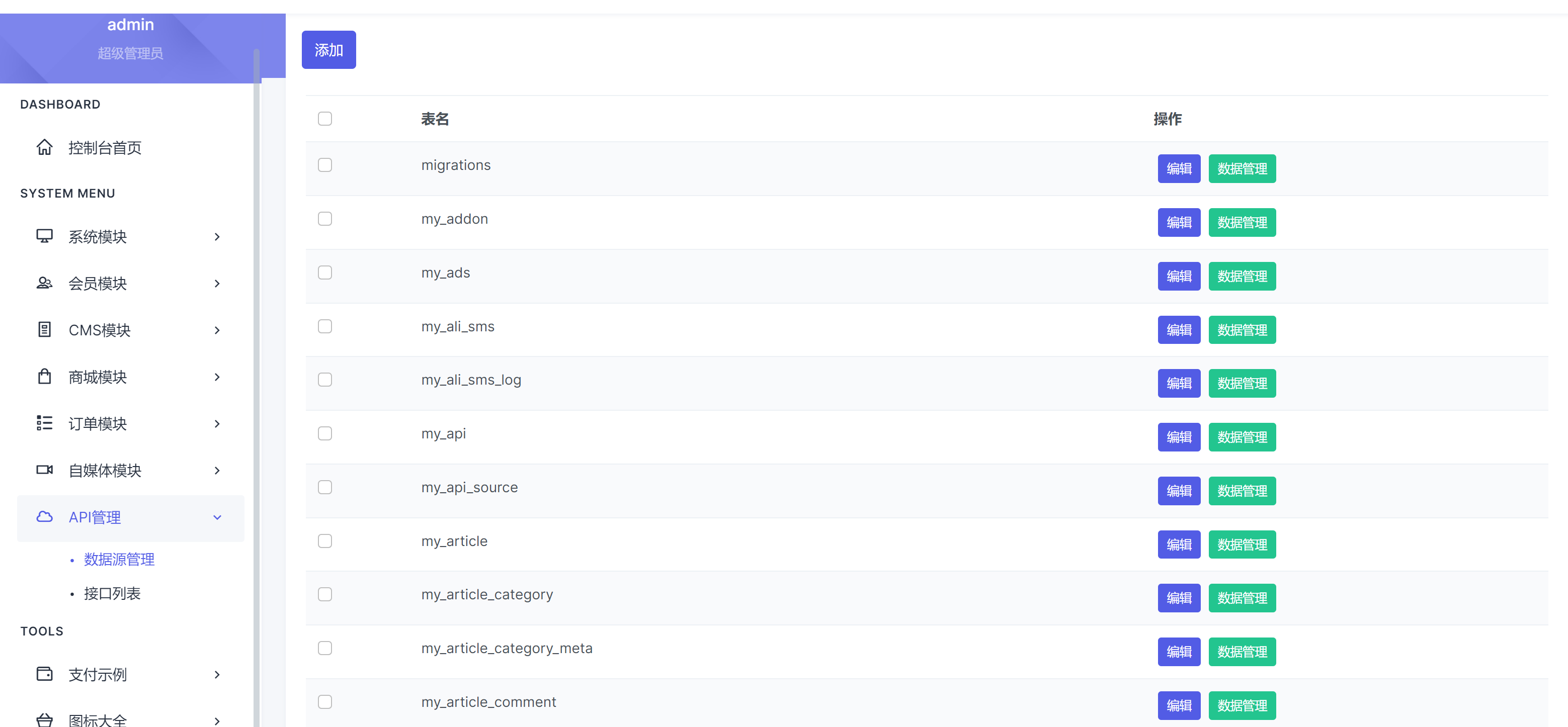
国产开源无头CMS,MyCms v4.7 快捷生成接口开发后台
MyCms 是一款基于 Laravel 开发的开源免费的开源多语言商城 CMS 企业建站系统。 MyCms 基于 Apache2.0 开源协议发布,免费且可商业使用,欢迎持续关注我们。技术交流 QQ 群:887522124 加群请备注来源:如gitee、github、官网等 v4…...
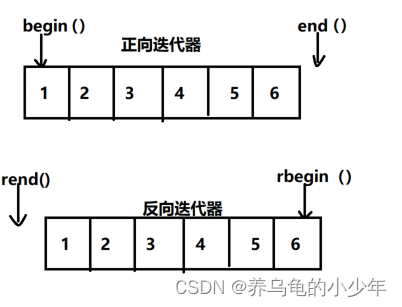
C++(反向迭代器)
前言: 上一章我们介绍了适配器,也提了一下迭代器适配器,今天我们就从反向迭代器把迭代器适配器给解释一下。 既然 都叫迭代器容器了 就说名只要接口合适他可以封装实现各种容器需求包括vector list 。 目录 1.反向迭代器设计 1.1反向迭代…...