RustDay04------Exercise[11-20]
11.函数原型有参数时需要填写对应参数进行调用
这里原先call_me函数没有填写参数导致报错 添加一个usize即可
// functions3.rs
// Execute `rustlings hint functions3` or use the `hint` watch subcommand for a hint.fn main() {call_me(10);
}fn call_me(num: u32) {for i in 0..num {println!("Ring! Call number {}", i + 1);}
}
12.函数需要返回值
fn sale_price(price: i32) -> i32前面括号内是传入参数类型,后面是返回值类型
// functions4.rs
// Execute `rustlings hint functions4` or use the `hint` watch subcommand for a hint.// This store is having a sale where if the price is an even number, you get
// 10 Rustbucks off, but if it's an odd number, it's 3 Rustbucks off.
// (Don't worry about the function bodies themselves, we're only interested
// in the signatures for now. If anything, this is a good way to peek ahead
// to future exercises!)fn main() {let original_price = 51;println!("Your sale price is {}", sale_price(original_price));
}fn sale_price(price: i32) -> i32{if is_even(price) {price - 10} else {price - 3}
}fn is_even(num: i32) -> bool {num % 2 == 0
}
13.函数隐式返回,不能使用逗号作为默认返回
这里square函数隐式返回num*num,如果加上分号会返回()
// functions5.rs
// Execute `rustlings hint functions5` or use the `hint` watch subcommand for a hint.// I AM NOT DONEfn main() {let answer = square(3);println!("The square of 3 is {}", answer);
}fn square(num: i32) -> i32 {num * num
}
14.使用if编写函数功能
这里使用if判断a>b的情况 然后分情况讨论
// if1.rs
// Execute `rustlings hint if1` or use the `hint` watch subcommand for a hint.pub fn bigger(a: i32, b: i32) -> i32 {// Complete this function to return the bigger number!// Do not use:// - another function call// - additional variablesif a>b {a}else {b}
}// Don't mind this for now :)
#[cfg(test)]
mod tests {use super::*;#[test]fn ten_is_bigger_than_eight() {assert_eq!(10, bigger(10, 8));}#[test]fn fortytwo_is_bigger_than_thirtytwo() {assert_eq!(42, bigger(32, 42));}
}
15.嵌套if返回条件
// if2.rs// Step 1: Make me compile!
// Step 2: Get the bar_for_fuzz and default_to_baz tests passing!
// Execute `rustlings hint if2` or use the `hint` watch subcommand for a hint.pub fn foo_if_fizz(fizzish: &str) -> &str {if fizzish == "fizz" {"foo"} else {if fizzish =="fuzz"{"bar"}else {"baz"}}
}// No test changes needed!
#[cfg(test)]
mod tests {use super::*;#[test]fn foo_for_fizz() {assert_eq!(foo_if_fizz("fizz"), "foo")}#[test]fn bar_for_fuzz() {assert_eq!(foo_if_fizz("fuzz"), "bar")}#[test]fn default_to_baz() {assert_eq!(foo_if_fizz("literally anything"), "baz")}
}
其中assert_eq!(a,b)是在比较a,b两个数值是否相等,用于做单元测试
16.使用if进行简单应用场景功能实现
自己编写calculate_price_of_apples(price:i32)->i32即可
// quiz1.rs
// This is a quiz for the following sections:
// - Variables
// - Functions
// - If// Mary is buying apples. The price of an apple is calculated as follows:
// - An apple costs 2 rustbucks.
// - If Mary buys more than 40 apples, each apple only costs 1 rustbuck!
// Write a function that calculates the price of an order of apples given
// the quantity bought. No hints this time!// Put your function here!
fn calculate_price_of_apples(price:i32)->i32 {if (price<=40){return price*2;}return price;}// Don't modify this function!
#[test]
fn verify_test() {let price1 = calculate_price_of_apples(35);let price2 = calculate_price_of_apples(40);let price3 = calculate_price_of_apples(41);let price4 = calculate_price_of_apples(65);assert_eq!(70, price1);assert_eq!(80, price2);assert_eq!(41, price3);assert_eq!(65, price4);
}
17.利用boolean类型变量做判断
// primitive_types1.rs
// Fill in the rest of the line that has code missing!
// No hints, there's no tricks, just get used to typing these :)fn main() {// Booleans (`bool`)let is_morning = true;if is_morning {println!("Good morning!");}let is_evening = false;// let // Finish the rest of this line like the example! Or make it be false!if is_evening {println!("Good evening!");}
}
18.判断字符类型
我们在这里只需要填一个字符即可,即使是emjoy
// primitive_types2.rs
// Fill in the rest of the line that has code missing!
// No hints, there's no tricks, just get used to typing these :)fn main() {// Characters (`char`)// Note the _single_ quotes, these are different from the double quotes// you've been seeing around.let my_first_initial = 'C';if my_first_initial.is_alphabetic() {println!("Alphabetical!");} else if my_first_initial.is_numeric() {println!("Numerical!");} else {println!("Neither alphabetic nor numeric!");}let your_character='u';// Finish this line like the example! What's your favorite character?// Try a letter, try a number, try a special character, try a character// from a different language than your own, try an emoji!if your_character.is_alphabetic() {println!("Alphabetical!");} else if your_character.is_numeric() {println!("Numerical!");} else {println!("Neither alphabetic nor numeric!");}
}
19.获取字符串长度
// primitive_types3.rs
// Create an array with at least 100 elements in it where the ??? is.
// Execute `rustlings hint primitive_types3` or use the `hint` watch subcommand for a hint.fn main() {let a = "99999999999999999999999999999999";if a.len() >= 100 {println!("Wow, that's a big array!");} else {println!("Meh, I eat arrays like that for breakfast.");}
}
20.字符串切片
使用&引用变量 [leftIndex..rightIndex)区间内切片
// primitive_types4.rs
// Get a slice out of Array a where the ??? is so that the test passes.
// Execute `rustlings hint primitive_types4` or use the `hint` watch subcommand for a hint.#[test]
fn slice_out_of_array() {let a = [1, 2, 3, 4, 5];let nice_slice = &a[1..4];assert_eq!([2, 3, 4], nice_slice)
}
相关文章:

RustDay04------Exercise[11-20]
11.函数原型有参数时需要填写对应参数进行调用 这里原先call_me函数没有填写参数导致报错 添加一个usize即可 // functions3.rs // Execute rustlings hint functions3 or use the hint watch subcommand for a hint.fn main() {call_me(10); }fn call_me(num: u32) {for i i…...
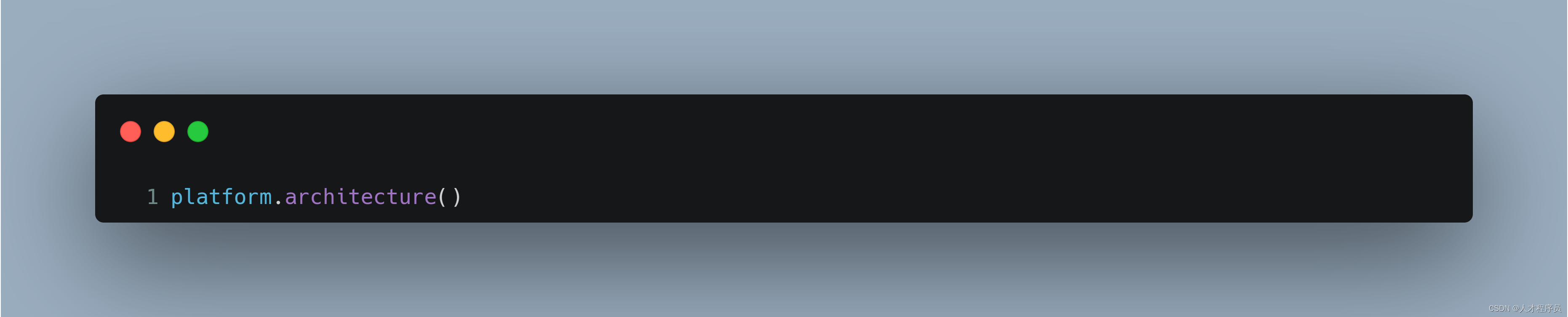
【Python第三方包】快速获取硬件信息和使用情况(psutil、platform)
文章目录 前言一、psutil包1.1 安装psutil包1.2 psutil 使用方式获取CPU使用率获取内存使用情况将内存的获取的使用情况变成GB和MB获取磁盘使用情况磁盘内存进行转换获取网络信息网络info 二、platform2.1 platform的介绍2.2 platform 使用方式获取操作系统的名称获取架构的名称…...
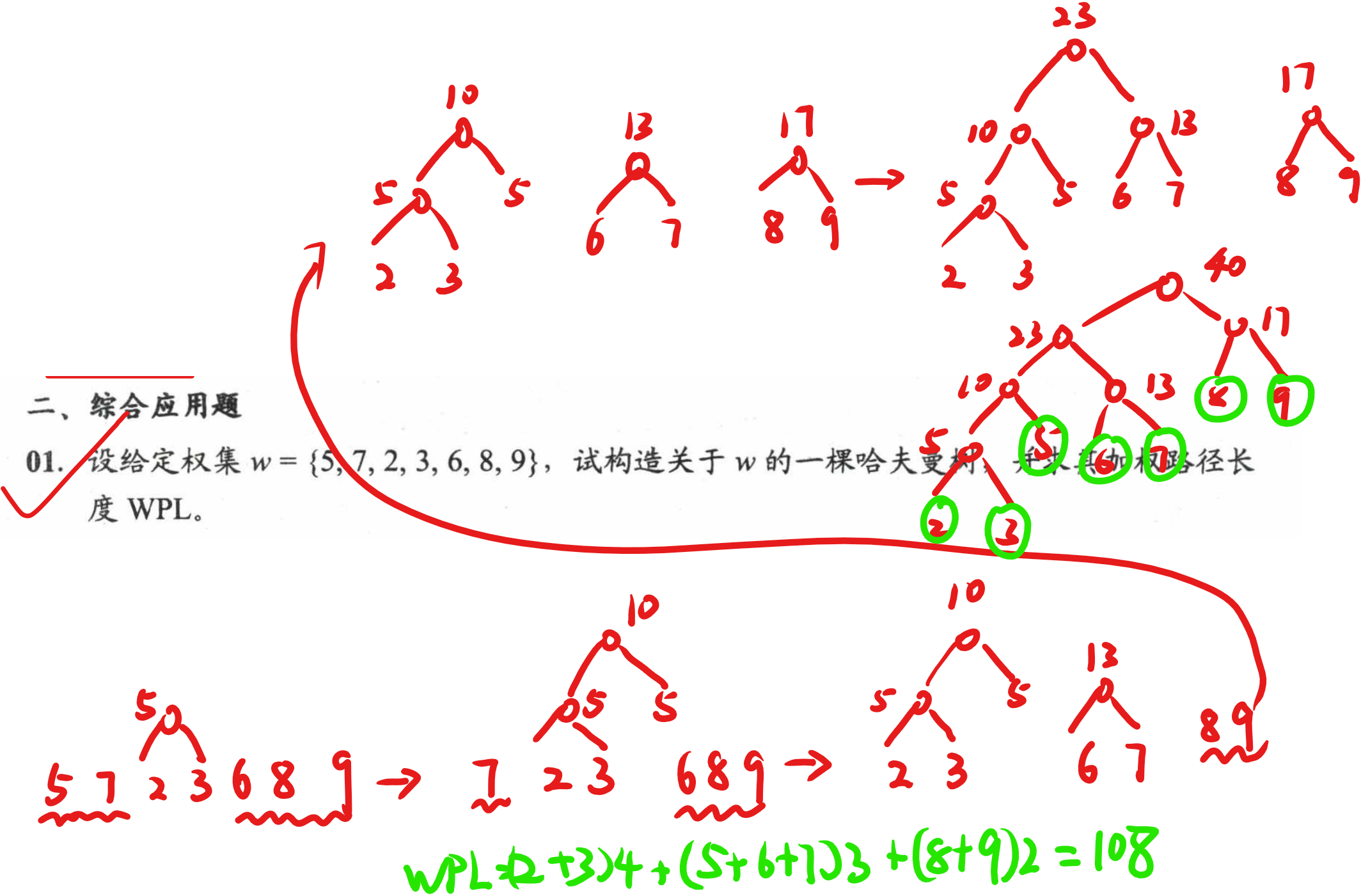
数据结构与算法课后题-第五章(哈夫曼树和哈夫曼编码)
文章目录 选择题1选择题2选择题3选择题4选择题5选择题6选择题7应用题7 选择题1 选择题2 选择题3 需要深究 选择题4 选择题5 选择题6 选择题7 应用题7...
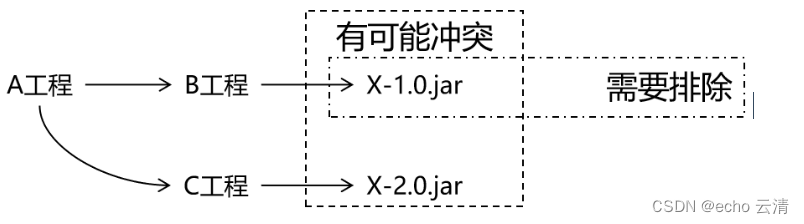
07测试Maven中依赖的范围,依赖的传递原则,依赖排除的配置
依赖的特性 scope标签在dependencies/dependency标签内,可选值有compile(默认值),test,provided,system,runtime,import compile:在项目实际运行时真正要用到的jar包都是以compile的范围进行依赖 ,比如第三方框架SSM所需的jar包test:测试过程中使用的j…...
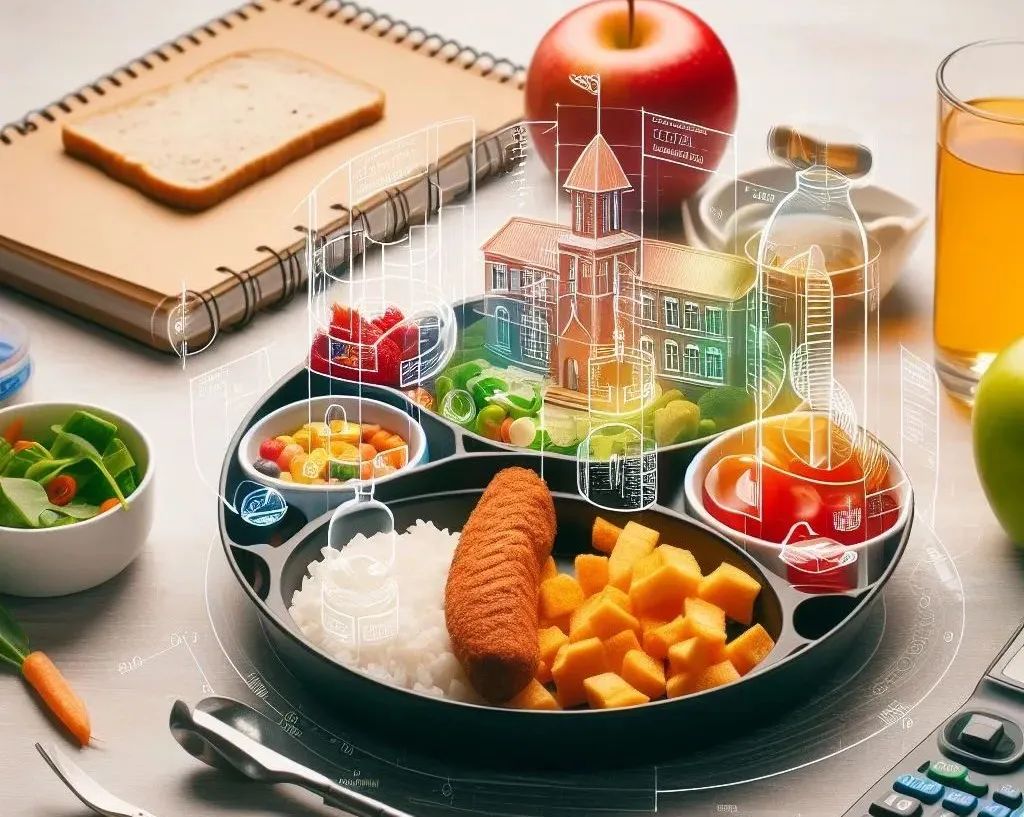
科技为饮食带来创新,看AI如何打造智能营养时代
在当今社会,快节奏的生活方式、便捷的食品选择以及现代科技的快速发展正深刻地重塑着我们对健康的认知和实践,它已经不再仅仅是一个话题,而是一个备受关注的社会焦点。在这个纷繁复杂的交汇点上,AI技术的介入为我们开辟了前所未有…...
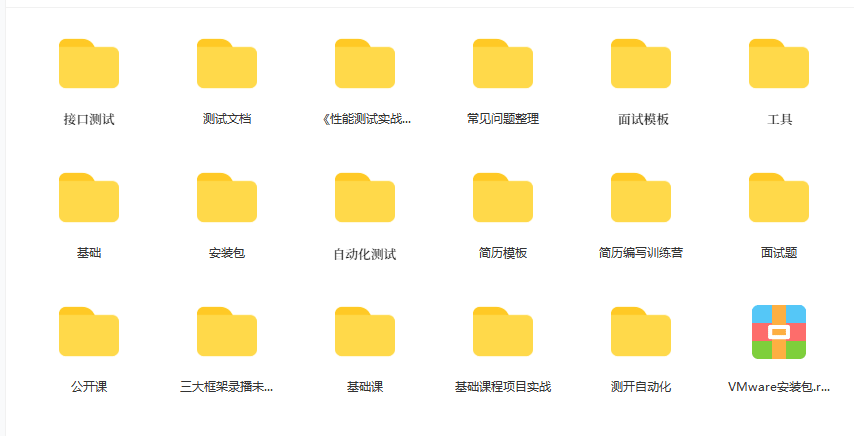
软件测试知识库+1,5款顶级自动化测试工具推荐和使用分析
“工欲善其事必先利其器”,在自动化测试领域,自动化测试工具的核心地位不容置疑的。目前市面上有很多可以支持接口测试的工具,在网上随便一搜就可以出来很多,利用自动化测试工具进行接口测试,可以很好的提高测试效率&a…...

代码随想录算法训练营第23期day22|669. 修剪二叉搜索树、108.将有序数组转换为二叉搜索树、538.把二叉搜索树转换为累加树
目录 一、(leetcode 669)修剪二叉搜索树 二、(leetcode 108)将有序数组转换为二叉搜索树 三、(leetcode 538)把二叉搜索树转换为累加树 一、(leetcode 669)修剪二叉搜索树 力扣题…...
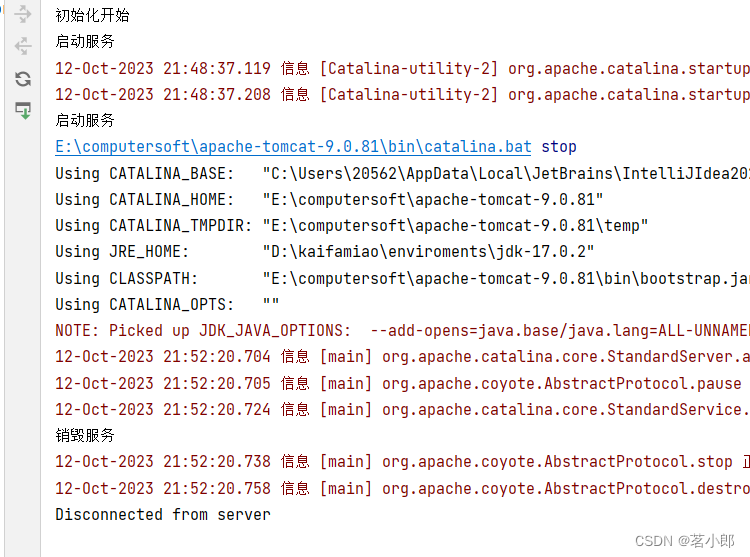
IDEA中创建Web工程流程
第一步:File--》New--》Project 第二步:填写信息,点击Create 第三步:点击File,点击Project Structure 出现该界面 选择相应的版本,这里我用jdk17,点击apply ,点击ok 第三步:右键文件…...
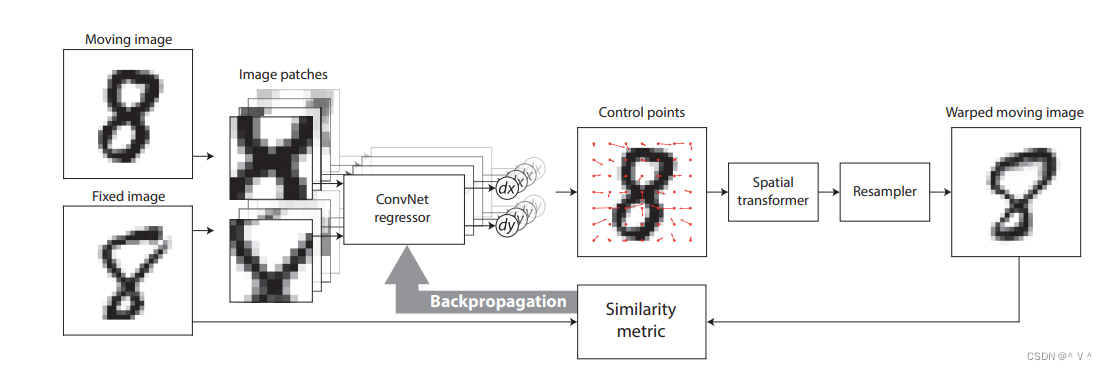
【论文阅读】基于卷积神经的端到端无监督变形图像配准
📘End-to-End Unsupervised Deformable ImageRegistration with a Convolutional NeuralNetwork 📕《基于卷积神经的端到端无监督变形图像配准》 文章目录 摘要 Abstract. 1.导言 Introduction 附录 References未完待续 to be continued ... 摘要 Abstr…...
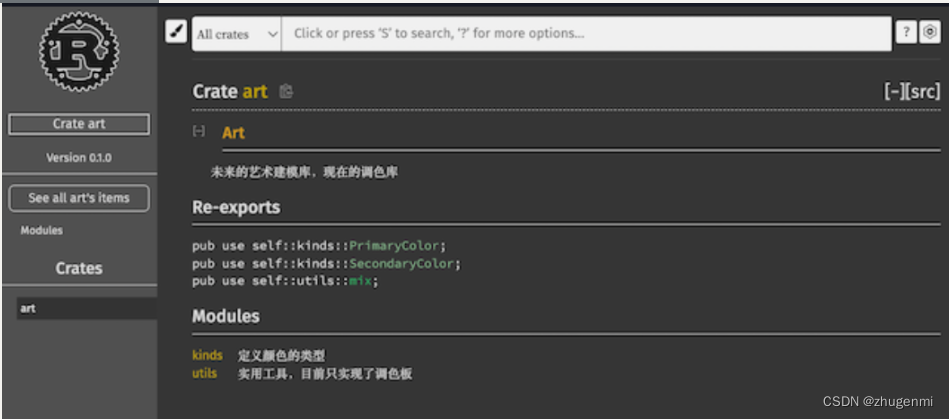
【Rust】包和模块,文档注释,Rust格式化输出
文章目录 包和模块包 CrateRust 的标准目录结构 模块 Module用路径引用模块使用super引用模块使用self引用模块结构体和枚举的可见性 使用 use 引入模块及受限可见性基本引入方式绝对路径引入模块相对路径引入模块中的函数 避免同名引用 注释和文档文档注释包和模块级别的注释注…...

leetcode221.最大正方形
最大正方形 可以使用动态规划降低时间复杂度。用 dp(i,j) 表示以 (i,j)为右下角,且只包含 111 的正方形的边长最大值。能计算出所有 dp(i,j)的值,那么其中的最大值即为矩阵中只包含 111 的正方形的边长最大值,其平方即为最大正方形的面积。 …...
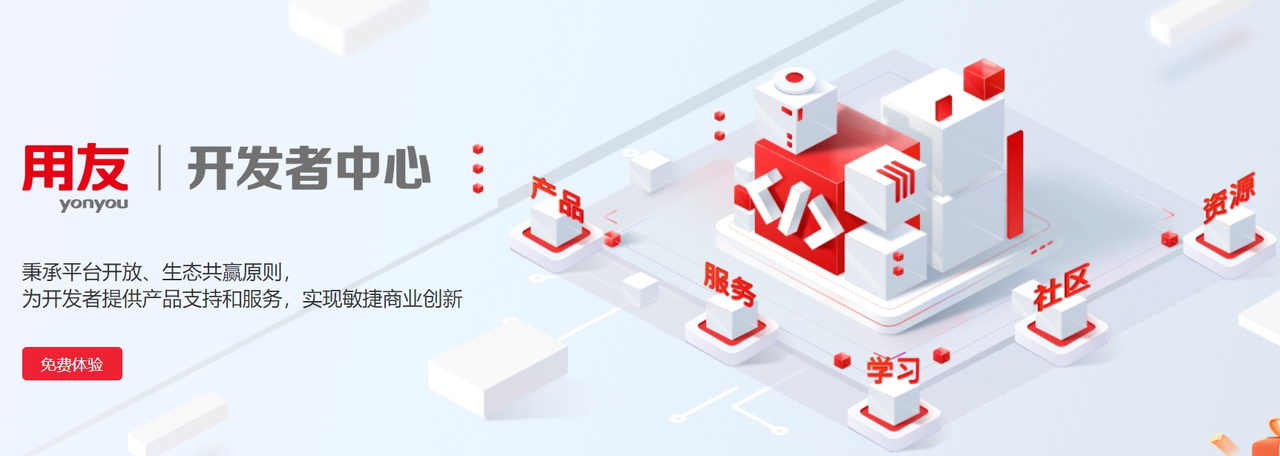
低代码技术这么香,如何把它的开发特点发挥到极致?
前言 什么是低代码技术? 低代码是一种可视化软件开发方法,通过最少的编码更快地交付应用程序。图形用户界面和拖放功能使开发过程的各个方面自动化,消除了对传统计算机编程方法的依赖。 文章目录 前言低代码平台怎么选?用友Yonbu…...
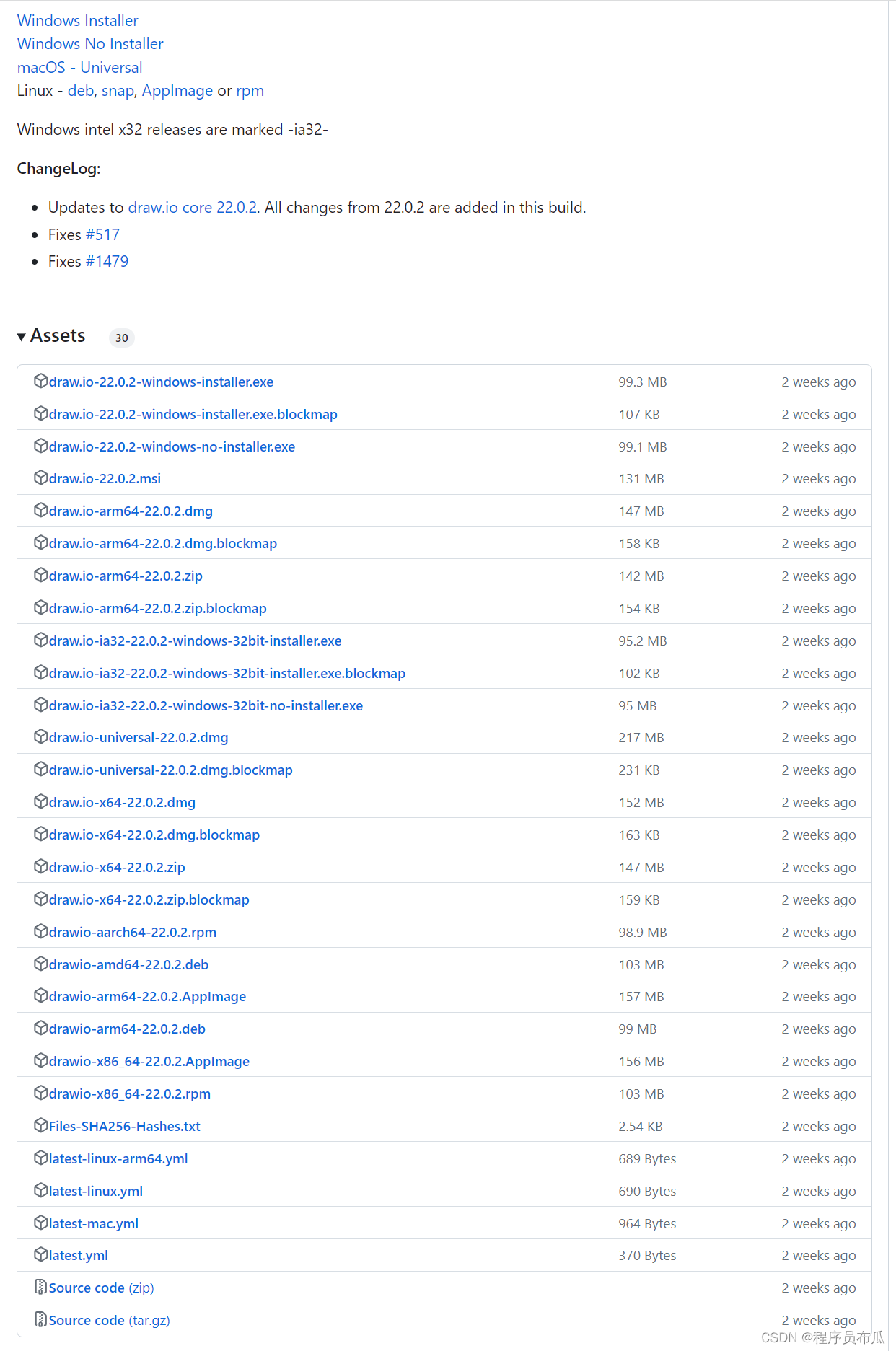
drawio简介以及下载安装
drawio简介以及下载安装 drawio是一款非常强大的开源在线的流程图编辑器,支持绘制各种形式的图表,提供了 Web端与客户端支持,同时也支持多种资源类型的导出。 访问网址:draw.io或者直接使用app.diagrams.net直接打开可以使用在线版…...
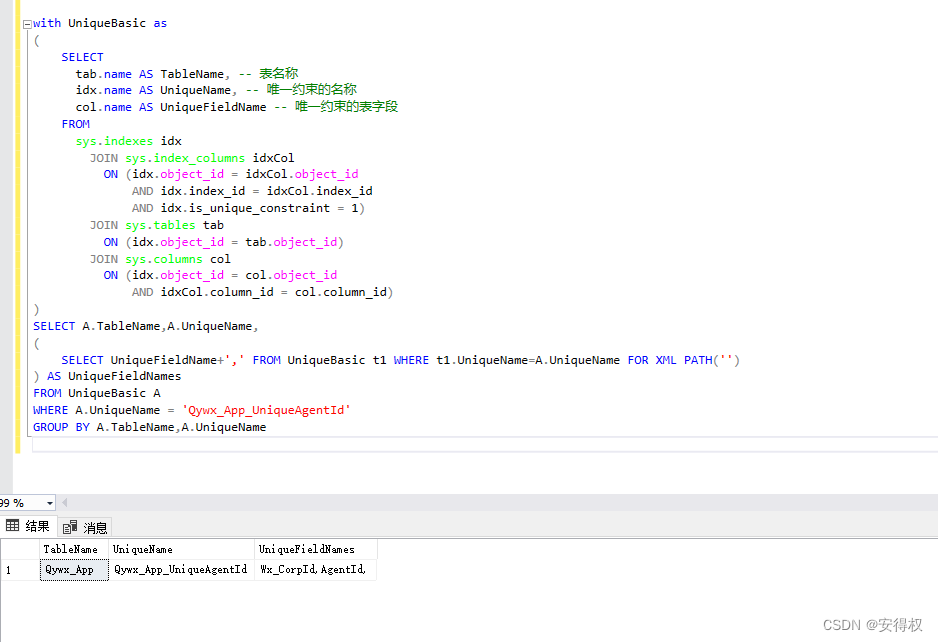
Sql Server 数据库中的所有已定义的唯一约束 (列名称 合并过了)
查询Sql Server Database中的唯一约束 with UniqueBasic as (SELECTtab.name AS TableName, -- 表名称idx.name AS UniqueName, -- 唯一约束的名称col.name AS UniqueFieldName -- 唯一约束的表字段FROMsys.indexes idxJOIN sys.index_columns idxColON (idx.object_id idxCo…...
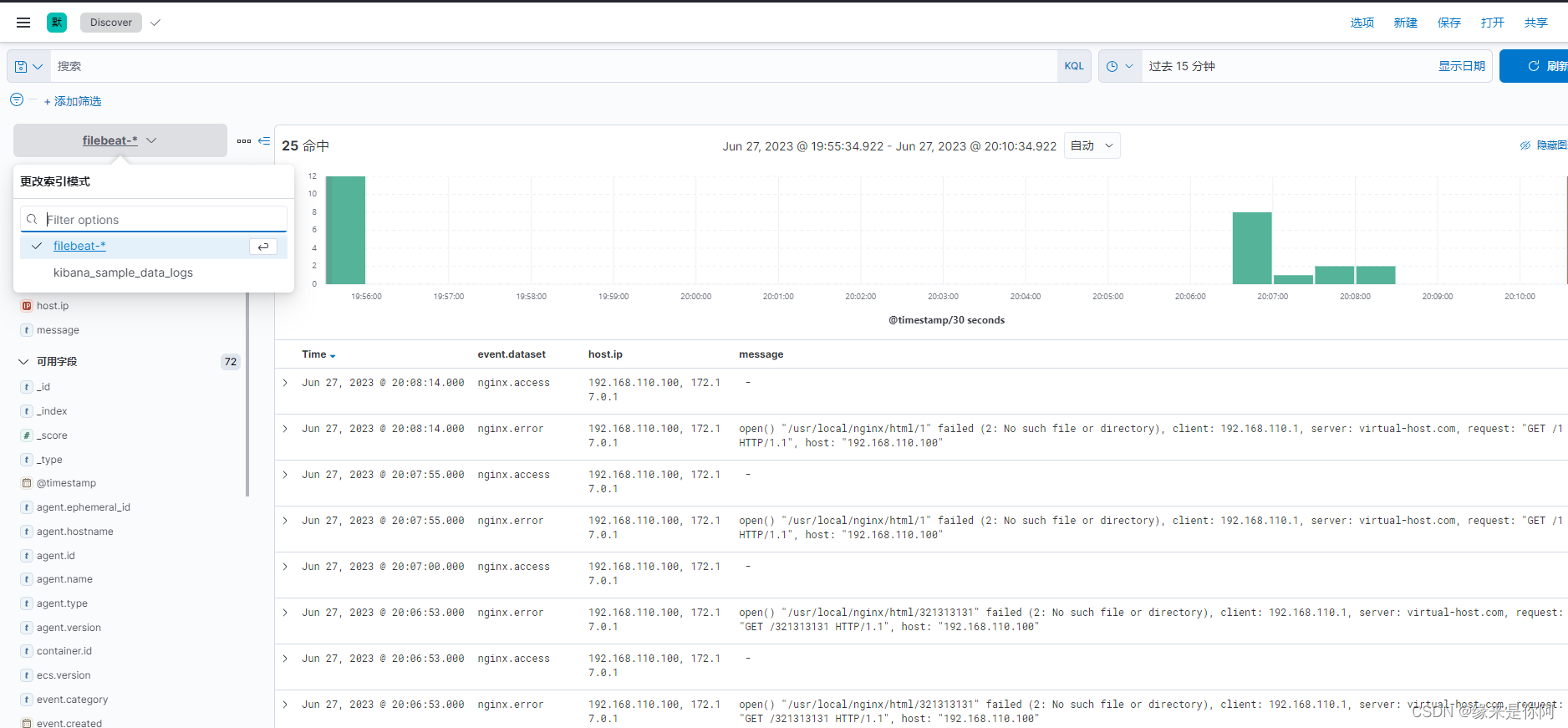
elasticsearch (六)filebeat 安装学习
filebeat 安装:文件节拍快速入门:安装和配置 |文件节拍参考 [7.17] |弹性的 (elastic.co) 解压缩后,以配置nginx日志为例。 Nginx module | Filebeat Reference [7.17] | Elastic filebeat 配置中, - module: nginx access: …...

算法通关村第一关|青铜|链表笔记
1.理解 Java 如何构造出链表 在 Java 中,我们创建一个链表类,类中应当有两个属性,一个是结点的值 val ,一个是该结点指向的下一个结点 next 。 next 通俗讲是一个链表中的指针,但是在链表类中是一个链表类型的引用变量…...

【记录】使用Python读取Tiff图像的几种方法
文章目录 PIL.Imagecv2gdal 本文总结了使用 PIL Image, cv2, gdal.Open三种python package 读取多通道Tiff格式遥感影像的方法。 PIL.Image PIL对Tiff只支持两种格式的图像: 多通道8bit图像单通道int16, int32, float32图像 多通道多bit的tiff图像PIL不支持读取…...
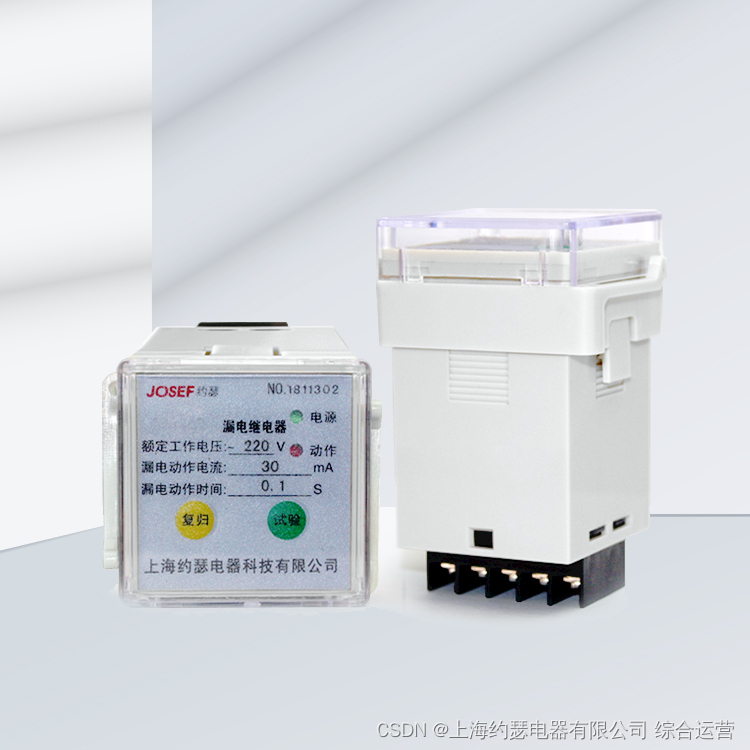
JOSEF约瑟 多档切换式漏电(剩余)继电器JHOK-ZBL1 30/100/300/500mA
系列型号: JHOK-ZBL多档切换式漏电(剩余)继电器(导轨) JHOK-ZBL1多档切换式漏电(剩余)继电器 JHOK-ZBL2多档切换式漏电(剩余)继电器 JHOK-ZBM多档切换式漏电…...
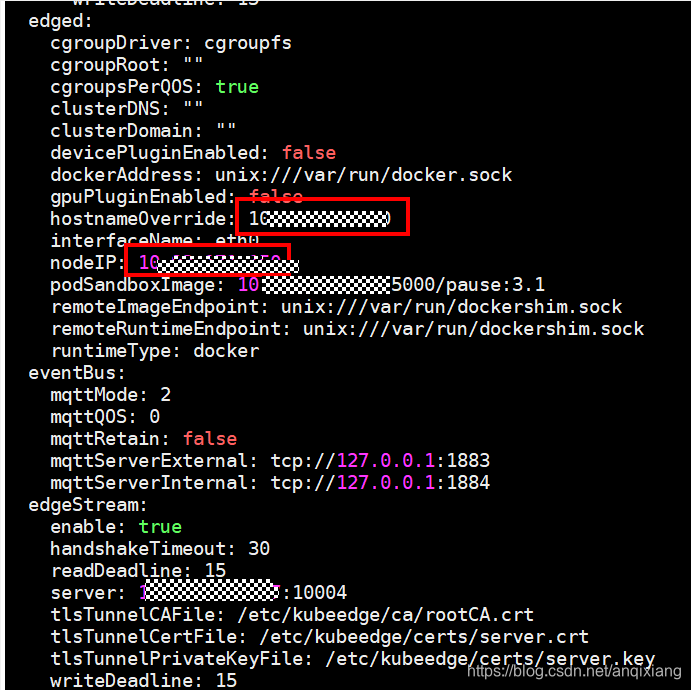
Linux部署kubeedge 1.4
文章目录 一、机器信息二、环境准备(所有节点操作)2.1. 修改主机名2.2. 开启路由转发2.3.安装Docker(所有节点)2.4.部署K8S集群(单机版,云端节点) 2.5.安装Mosquitto(只在边缘节点安装)三、安装kubeedge 1.…...

第一章习题
文章目录 x ( t ) j e j w 0 t x(t)je^{jw_0t} x(t)jejw0t x [ n ] j e j w 0 n x[n]je^{jw_0n} x[n]jejw0n 求基本周期: T 2 Π w 0 T\frac{2Π}{w_0} Tw02Π 对x[n],T为有理数才算 1、求信号x(t)2cos(10t1)-sin(4t-1)的基波周期 2 Π 10 Π 5 \frac{2…...

nvm、node、npm解决问题过程记录
在Windows10如何降级Node.js版本:可以尝试将Node.js版本降级到一个较旧的版本,以查看问题是否得以解决。可以使用Node Version Manager (nvm) 来轻松切换Node.js版本,具体完整步骤: 首先,需要安装Node Version Manager…...

Linux- DWARF调试文件格式
基本概念 DWARF是一个用于在可执行程序和其源代码之间进行关联的调试文件格式。当开发者使用调试编译选项(例如,使用gcc时的-g标志)编译程序时,编译器会生成这种格式的调试信息。这些信息在后续的调试过程中非常有用,…...
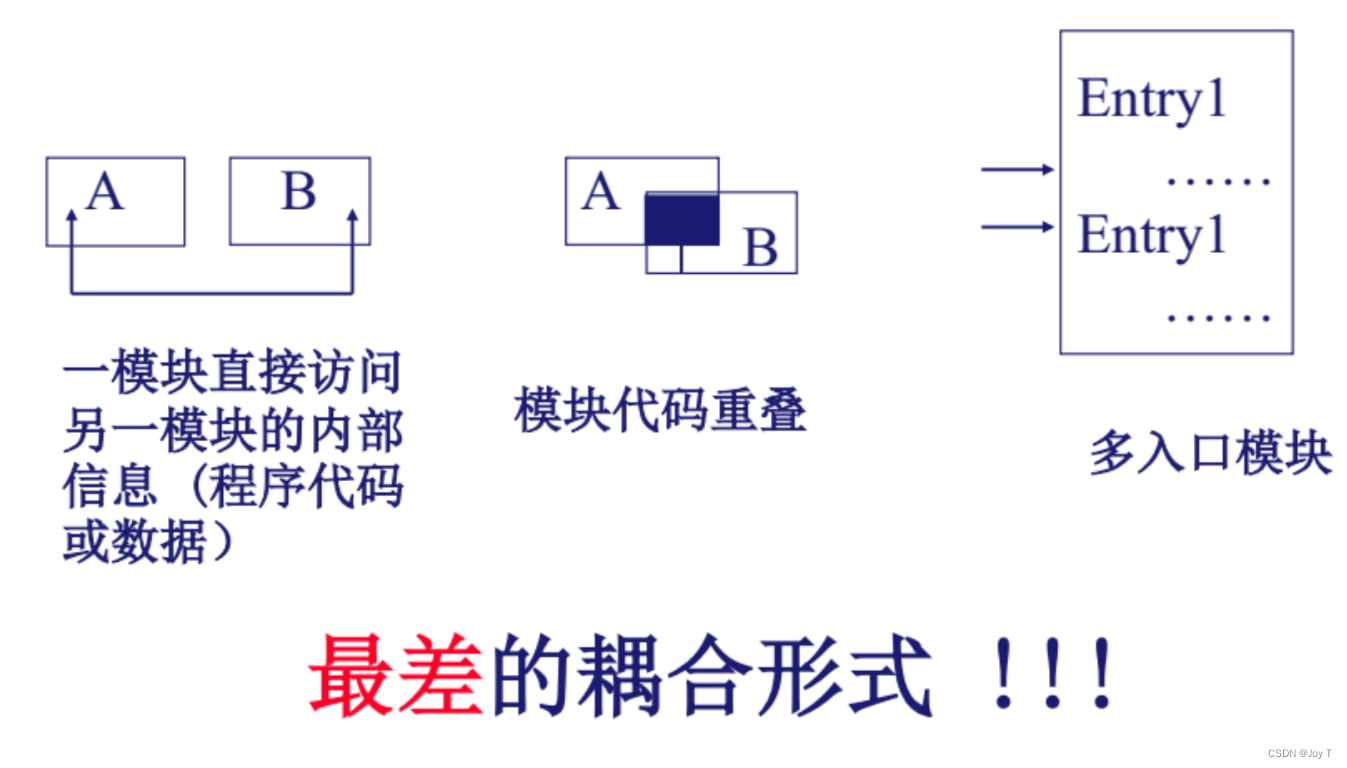
软件工程第六周
软件体系结构概述 体系结构:一种思想,而框架就是思想的实现,设计模式就是根据某一特殊问题实现的框架。 体系结构:体系结构是软件系统的高级结构。它定义了系统的主要组成部分,以及这些部分之间的关系和交互方式。 框…...

node+pm2安装部署
1、安装node 下载node安装包: wget https://nodejs.org/dist/v16.14.0/node-v16.14.0-linux-x64.tar.xz 解压: tar -xvJf node-v14.17.0-linux-x64.tar.xz 配置环境变量,在/etc/profile文件最后添加以下脚本: export PATH$P…...

大数据学习(11)-hive on mapreduce详解
&&大数据学习&& 🔥系列专栏: 👑哲学语录: 承认自己的无知,乃是开启智慧的大门 💖如果觉得博主的文章还不错的话,请点赞👍收藏⭐️留言📝支持一下博>主哦&#x…...
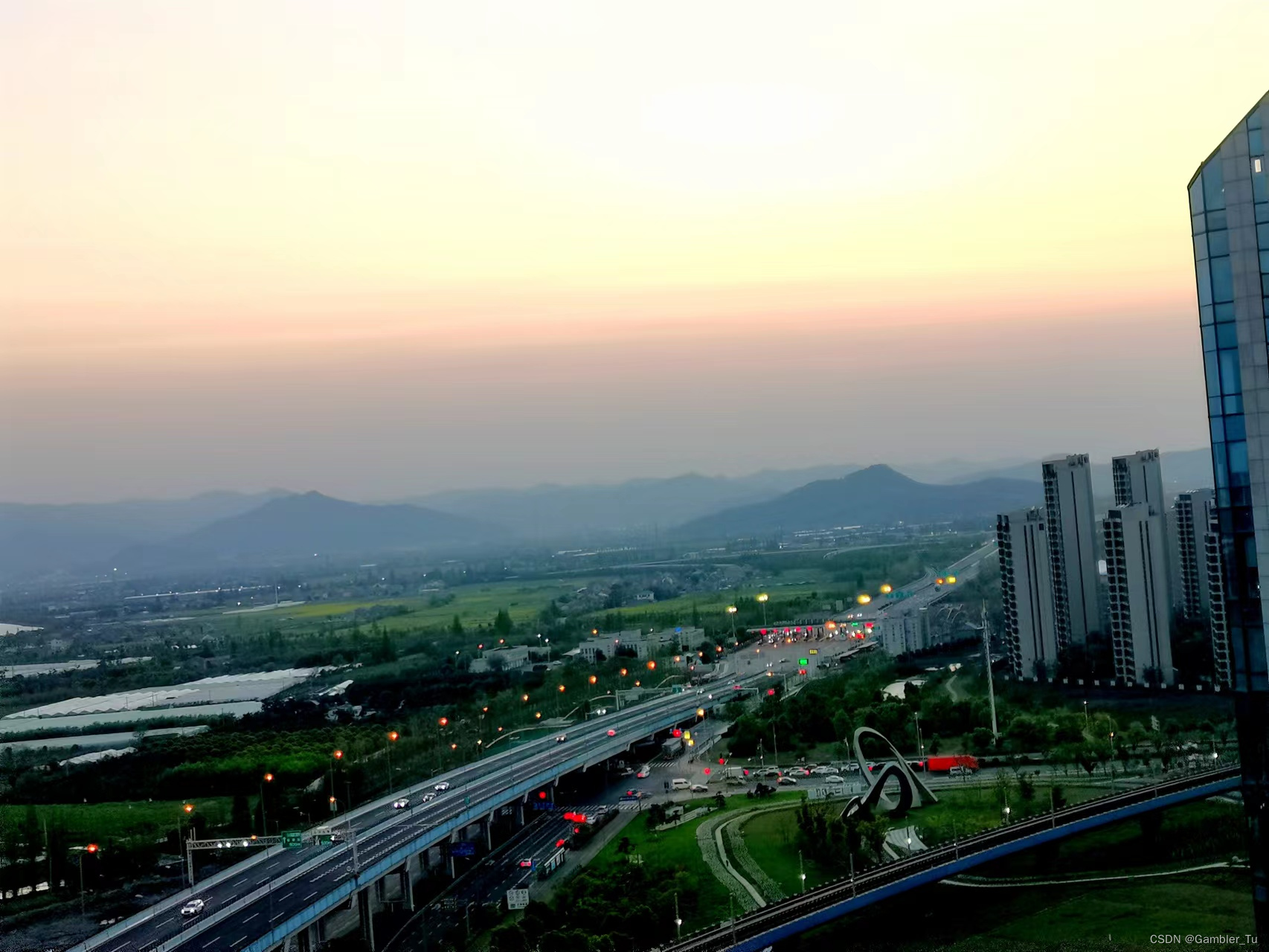
MyBatis基础之自动映射、映射类型、文件注解双配置
文章目录 自动映射原理jdbcType同时启用配置文件和注解两种配置方式 自动映射原理 在 MyBatis 的配置文件(settings 元素部分)中,有一个 autoMappingBehavior 配置,其默认值为 PARTIAL ,表示 MyBatis 会自动映射&…...

8、docker 安装 nginx
1、下载镜像 docker pull nginx 2、本机创建目录 1)创建nginx挂载目录 mkdir /usr/local/nginx 2)进入nginx目录 cd /usr/local/nginx 3)创建 www和logs目录 mkdir -p www logs 3、创建nginx容器 此容器用于复制配置文件,复…...
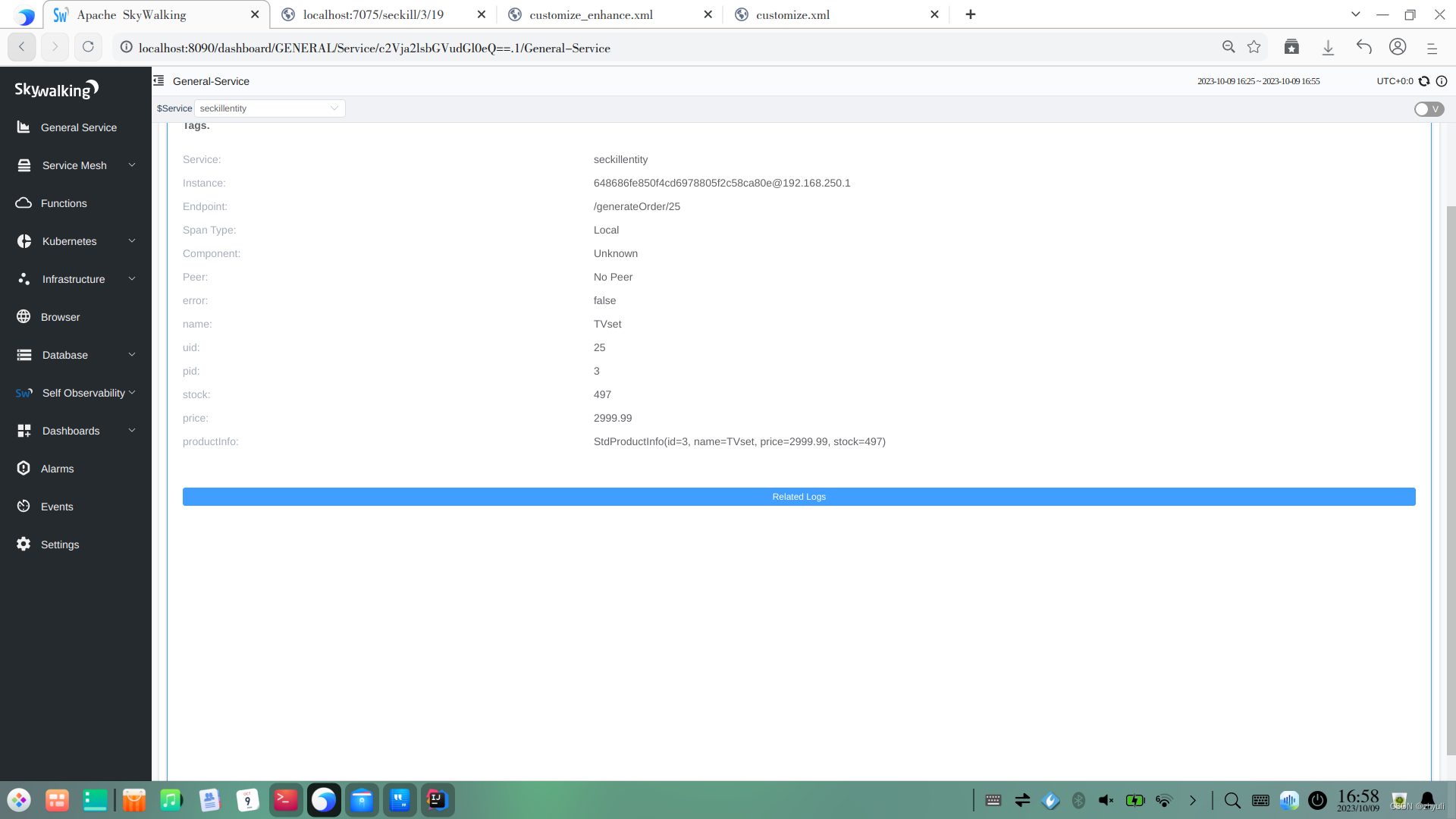
关于Skywalking Agent customize-enhance-trace对应用复杂参数类型取值
对于Skywalking Agent customize-enhance-trace 大家应该不陌生了,主要支持以非入侵的方式按用户自定义的Span跟踪对应的应用方法,并获取数据。 参考https://skywalking.apache.org/docs/skywalking-java/v9.0.0/en/setup/service-agent/java-agent/cust…...

手机路径、Windows路径知识及delphiXE跨设备APP自动下载和升级
手机路径、Windows路径知识 及delphiXE跨设备APP自动下载和升级 一、APP安装程序文件版本和权限信息 1、运行时动态调用Android apk的AndroidManifest.xml获取versionName 2、运行时动态调用IOS ipa的info.plist获取CFBundleVersion (和entitlements)…...

GitLab 502问题解决方案
由于最近 gitlab 切换到另一台服务器上部署的 gitlab 后,经常出现 502。平时重启 gitlab 后都能解决,今天突然重启多次后都还是 502(重启日志是正常的),遂通过 gitlab-ctl tail 查看日志进行排查。 gitlab-ctl tail通…...