《第一行代码》 第八章:应用手机多媒体
一,使用通知
第一步,创建项目,书写布局
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"android:orientation="vertical"android:layout_width="match_parent"android:layout_height="match_parent"><Buttonandroid:id="@+id/send_notice"android:layout_width="wrap_content"android:layout_height="wrap_content"android:text="发送通知"/>
</LinearLayout>
第二步:修改活动代码,写发起通知
public class MainActivity extends AppCompatActivity implements View.OnClickListener{@Overrideprotected void onCreate(Bundle savedInstanceState) {super.onCreate(savedInstanceState);setContentView(R.layout.activity_main);Button sendNotice=(Button) findViewById(R.id.send_notice);sendNotice.setOnClickListener(this);}@Overridepublic void onClick(View view) {switch(view.getId()){case R.id.send_notice://接收一个字符串用于明确是哪个服务,创建一个通知管理器manager,对通知进行管理NotificationManager manager =(NotificationManager) getSystemService(NOTIFICATION_SERVICE);//高版本需要渠道,注意这里Build.VERSION_CODES.O是opq的o,不是数字0if(Build.VERSION.SDK_INT >= Build.VERSION_CODES.O){NotificationChannel notificationChannel=new NotificationChannel("1","name",NotificationManager.IMPORTANCE_HIGH);//如果这里用IMPORTANCE_HIGH就需要在系统的设置里开启渠道,通知才能正常弹出if(manager!=null){manager.createNotificationChannel(notificationChannel);}}//使用Builder来创建构造器,并完成基本的参数配置Notification notification=new NotificationCompat.Builder(MainActivity.this,"1").setContentTitle("这是标题").setContentText("这是内容").setWhen(System.currentTimeMillis()).setSmallIcon(R.mipmap.ic_launcher).setLargeIcon(BitmapFactory.decodeResource(getResources(),R.mipmap.ic_launcher)).build();if(manager!=null){manager.notify(1,notification);}break;default:break;}}
}
第三步,书写点击通知后的回调事件
新建NotificationActivity活动,修改对应的布局:
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"android:layout_width="match_parent"android:layout_height="match_parent"><TextViewandroid:layout_width="wrap_content"android:layout_height="wrap_content"android:layout_centerInParent="true"android:textSize="24sp"android:text="文本内容"/>
</RelativeLayout>
修改活动代码:
public class MainActivity extends AppCompatActivity implements View.OnClickListener{@Overrideprotected void onCreate(Bundle savedInstanceState) {super.onCreate(savedInstanceState);setContentView(R.layout.activity_main);Button sendNotice=(Button) findViewById(R.id.send_notice);sendNotice.setOnClickListener(this);}@Overridepublic void onClick(View view) {switch(view.getId()){case R.id.send_notice://回调事件,打开新的活动Intent intent =new Intent(this,NotificationActivity.class);PendingIntent pi=PendingIntent.getActivity(this,0,intent,0);//接收一个字符串用于明确是哪个服务,创建一个通知管理器manager,对通知进行管理NotificationManager manager =(NotificationManager) getSystemService(NOTIFICATION_SERVICE);//高版本需要渠道,注意这里Build.VERSION_CODES.O是opq的o,不是数字0if(Build.VERSION.SDK_INT >= Build.VERSION_CODES.O){NotificationChannel notificationChannel=new NotificationChannel("1","name",NotificationManager.IMPORTANCE_HIGH);//如果这里用IMPORTANCE_HIGH就需要在系统的设置里开启渠道,通知才能正常弹出if(manager!=null){manager.createNotificationChannel(notificationChannel);}}//使用Builder来创建构造器,并完成基本的参数配置Notification notification=new NotificationCompat.Builder(MainActivity.this,"1").setContentTitle("这是标题").setContentText("这是内容").setWhen(System.currentTimeMillis()).setSmallIcon(R.mipmap.ic_launcher).setLargeIcon(BitmapFactory.decodeResource(getResources(),R.mipmap.ic_launcher)).setContentIntent(pi).build();if(manager!=null){manager.notify(1,notification);}break;default:break;}}
}
主要是这几行代码:
//回调事件,打开新的活动
Intent intent =new Intent(this,NotificationActivity.class);
PendingIntent pi=PendingIntent.getActivity(this,0,intent,0);
//....
Notification notification=new NotificationCompat.Builder(MainActivity.this,"1").setContentIntent(pi)//这里注册回调函数.build();
第四步:关闭通知
在我们点击通知之后,需要把该通知移除掉,这个移除有两种方法。
【1】注册时声明
Notification notification=new NotificationCompat.Builder(MainActivity.this,"1").setAutoCancel(true).build();
【2】执行好回调后执行
我们点击通知是打开一个新的活动页,于是可以在新的活动页中,拿到该通知管理器,然后调用通知管理器的关闭通知,找到对应要关闭的通知就行关闭即可:
public class NotificationActivity extends AppCompatActivity {@Overrideprotected void onCreate(Bundle savedInstanceState) {super.onCreate(savedInstanceState);setContentView(R.layout.notification_layout);NotificationManager manager=(NotificationManager) getSystemService(NOTIFICATION_SERVICE);manager.cancel(1);}
}
二,调用摄像头和相册
第一步:新建项目,修改布局
新建CameraAlbumTest项目,修改布局:
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"android:orientation="vertical"android:layout_width="match_parent"android:layout_height="match_parent"><Buttonandroid:id="@+id/take_photo"android:layout_width="match_parent"android:layout_height="wrap_content"android:text="照相"/><ImageViewandroid:id="@+id/picture"android:layout_width="wrap_content"android:layout_height="wrap_content"android:layout_gravity="center_horizontal"/></LinearLayout>
第二步:修改对应的activity
public class MainActivity extends AppCompatActivity {public static final int TAKE_PHOTO=1;private ImageView picture;private Uri imageUri;@Overrideprotected void onCreate(Bundle savedInstanceState) {super.onCreate(savedInstanceState);setContentView(R.layout.activity_main);Button takePhoto =(Button) findViewById(R.id.take_photo);picture=(ImageView) findViewById(R.id.picture);takePhoto.setOnClickListener(new View.OnClickListener(){@Overridepublic void onClick(View view) {//创建file对象用于存储拍照后的图片File outputImage=new File(getExternalCacheDir(),"output_image.jpg");try {if(outputImage.exists()){outputImage.delete();}} catch (IOException e){e.printStackTrace();}if(Build.VERSION.SDK_INT>=24){imageUri= FileProvider.getUriForFile(MainActivity.this,"com.example.cameraalbumtest.fileprovider",outputImage);}else{imageUri=Uri.fromFile(outputImage);}//启动相机程序Intent intent =new Intent("android.media.action.IMAGE_CAPTURE");intent.putExtra(MediaStore.EXTRA_OUTPUT,imageUri);startActivityForResult(intent,TAKE_PHOTO);}});}@Overrideprotected void onActivityResult(int requestCode, int resultCode,Intent data) {super.onActivityResult(requestCode, resultCode, data);switch (requestCode) {case TAKE_PHOTO:if (resultCode == RESULT_OK) {try {//将拍摄的照片显示出来Bitmap bitmap = BitmapFactory.decodeStream(getContentResolver().openInputStream(imageUri));picture.setImageBitmap(bitmap);} catch (FileNotFoundException e) {e.printStackTrace();}}break;default:break;}}
}
第三步:在menifest文件中对内容提供器进行注册
<providerandroid:authorities="com.example.cameraalbumtest.fileprovider"android:name="androidx.core.content.FileProvider"android:exported="false"android:grantUriPermissions="true"><meta-data android:name="android.support.FILE_PROVIDER_PATHS" android:resource="@xml/file_paths"/></provider>
其中meta-data来指定uri的共享路径,下面我们来创建它:
右击 res目录一New-Directory,创建一个xml目录,接着右击xml目录-New-File,创建一个file paths.xml文件。然后修改file paths.xml文件中的内容,如下所示:
<?xml version="1.0" encoding="utf-8"?>
<paths xmlns:android="http://schemas.android.com/apk/res/android"><external-pathname="my_images"path=""/>
</paths>
其中,external-path 就是用来指定 Uri 共享的name 属性的值可以随便填,path 属性的值表示共享的具体路径。这里设置空值就表示将整个 SD卡进行共享,当然你可以仅共享我们存放output imagejpg这张图片的路径
另外还有一点要注意,在Android 4.4系统之前,访问SD卡的应用关联目录也是要声明权限的,从 4.4 系统开始不再需要权限声明。那么我们为了能够兼容老版本系统的手机,还需要在AndroidManifest.xml中声明一下访问SD卡的权限:
<uses-permission android:name="android.permission.WRITE_EXTERNAL_STORAGE"/>
实现的效果:
第四步:从相册中选择照片
修改布局,增加一个点击打开相册的按钮:
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"android:orientation="vertical"android:layout_width="match_parent"android:layout_height="match_parent"><Buttonandroid:id="@+id/take_photo"android:layout_width="match_parent"android:layout_height="wrap_content"android:text="照相"/><Buttonandroid:id="@+id/choose_from_album"android:layout_width="match_parent"android:layout_height="wrap_content"android:text="choose photos"></Button><ImageViewandroid:id="@+id/picture"android:layout_width="wrap_content"android:layout_height="wrap_content"android:layout_gravity="center_horizontal"/></LinearLayout>
1.建立点击事件及布局,进行运行时权限处理,WRITE_EXTERNAL_STORAGE为程序对SD卡的读写能力。大于6.0动态申请权限后调用openAlbum()方法,小于6.0直接调用openAlbum()方法
Button chooseFromAlbum =(Button) findViewById(R.id.choose_from_album);
chooseFromAlbum.setOnClickListener(new View.OnClickListener(){@Overridepublic void onClick(View view) {if (ContextCompat.checkSelfPermission(MainActivity.this, Manifest.permission.WRITE_EXTERNAL_STORAGE) != PackageManager.PERMISSION_GRANTED) {ActivityCompat.requestPermissions(MainActivity.this, new String[]{Manifest.permission.WRITE_EXTERNAL_STORAGE}, 1);} else {openAlbum();}}
});
2.openAlbum中构建Intent对象,指定action为android.intent.action.GET_CONTENT,为Intent设定必要参数,调用startActivityForResult来打开相册程序选择照片,其中第二个参数为CHOOSE_PHOTO的2
private void openAlbum() {Intent intent01 = new Intent("android.intent.action.GET_CONTENT");intent01.setType("image/*");startActivityForResult(intent01, CHOOSE_PHOTO);
}
3.这样在onActivityResult中进入CHOOSE_PHOTO进行处理,为了兼容新旧版本,4.4以上调handleImageOnKitKat方法; 4.4以下调handleImageBeforeKitKat方法。因为4.4以后选择相册中的照片不在返回真实的Uri,因此需要解析。
case CHOOSE_PHOTO:if (resultCode == RESULT_OK) {if (Build.VERSION.SDK_INT >= 19) {handleImageOnKitKat(data);} else {handleImageBeforeKitKat(data);}}
4.1.handleImageOnKitKat解析了封装的Uri,(1)如果是document类型的Uri,则通过document id处理。若URi的authority是media格式,还需要进一步的解析,通过字符串分割获得真实ID,用ID构建新Uri和判断语句,将其传至getImagePath方法中。可以获取真实路径了(2)如果是content类型的uri,则使用普通方法去处理(3)如果是File类型的uri,直接获取图片路径即可,最后调用displayImage根据图片路径显示图片。
4.2.handleImageBeforeKitKat方法中Uri未经封装,无需解析,直接getIamgePath获取真实路径,再调用displayImage方法显示于界面。
@TargetApi(19)private void handleImageOnKitKat (Intent data){String imagePath = null;Uri uri = data.getData();if (DocumentsContract.isDocumentUri(this, uri)) {//如果是document类型的Uri,则通过document id处理String docId = DocumentsContract.getDocumentId(uri);if ("com.android.providers.media.documents".equals(uri.getAuthority())) {String id = docId.split(":")[1];//解析出数字格式的idString selection = MediaStore.Images.Media._ID + "=" + id;imagePath = getImagePath(MediaStore.Images.Media.EXTERNAL_CONTENT_URI, selection);} else ("com.android.providers.downloads.documents".equals(uri.getAuthority())) {Uri contentUri = ContentUris.withAppendedId(Uri.parse("content://downloads/public downloads"), Long.valueOf(docId));imagePath = getImagePath(contentUri, null);}}else if ("content".equalsIgnoreCase(uri.getScheme())) {imagePath = getImagePath(uri,null);}else if("file".equalsIgnoreCase(uri.getScheme())) {imagePath=uri.getPath();}displayImage(imagePath);//根据图片路径显示图片}private void handleImageBeforeKitKat(Intent data) {Uri uri = data.getData();String imagePath = getImagePath(uri,null);displayImage(imagePath);}
对应的displayImage方法:
@SuppressLint("Range")private String getImagePath(Uri uri, String selection) {String path = null;//通过Uri和Selection来获取真是的路径图片Cursor cursor = getContentResolver().query(uri,null,selection,null,null);if (cursor != null) {if (cursor.moveToFirst()) {path = cursor.getString(cursor.getColumnIndex(MediaStore.Images.Media.DATA));}cursor.close();}return path;}private void displayImage(String imagePath) {if (imagePath != null) {if (Build.VERSION.SDK_INT >= 29) {picture.setImageURI(getImageContentUri(MainActivity.this, imagePath));} else {Bitmap bitmap = BitmapFactory.decodeFile(imagePath);picture.setImageBitmap(bitmap);}} else {Toast.makeText(this, "failed to get image", Toast.LENGTH_SHORT).show();}}/*** 将图片转换成Uri* @param context 传入上下文参数* @param path 图片的路径* @return 返回的就是一个Uri对象*/public static Uri getImageContentUri(Context context, String path) {Cursor cursor = context.getContentResolver().query(MediaStore.Images.Media.EXTERNAL_CONTENT_URI,new String[] { MediaStore.Images.Media._ID }, MediaStore.Images.Media.DATA + "=? ",new String[] { path }, null);if (cursor != null && cursor.moveToFirst()) {int id = cursor.getInt(cursor.getColumnIndex(MediaStore.MediaColumns._ID));Uri baseUri = Uri.parse("content://media/external/images/media");return Uri.withAppendedPath(baseUri, "" + id);} else {// 如果图片不在手机的共享图片数据库,就先把它插入。if (new File(path).exists()) {ContentValues values = new ContentValues();values.put(MediaStore.Images.Media.DATA, path);return context.getContentResolver().insert(MediaStore.Images.Media.EXTERNAL_CONTENT_URI, values);} else {return null;}}}
总体的所有代码:
public class MainActivity extends AppCompatActivity {public static final int TAKE_PHOTO=1;private ImageView picture;private Uri imageUri;public static final int CHOOSE_PHOTO = 2;@Overrideprotected void onCreate(Bundle savedInstanceState) {super.onCreate(savedInstanceState);setContentView(R.layout.activity_main);Button takePhoto =(Button) findViewById(R.id.take_photo);picture=(ImageView) findViewById(R.id.picture);Button chooseFromAlbum =(Button) findViewById(R.id.choose_from_album);chooseFromAlbum.setOnClickListener(new View.OnClickListener(){@Overridepublic void onClick(View view) {if (ContextCompat.checkSelfPermission(MainActivity.this, Manifest.permission.WRITE_EXTERNAL_STORAGE) != PackageManager.PERMISSION_GRANTED) {ActivityCompat.requestPermissions(MainActivity.this, new String[]{Manifest.permission.WRITE_EXTERNAL_STORAGE}, 1);} else {openAlbum();}}});takePhoto.setOnClickListener(new View.OnClickListener(){@Overridepublic void onClick(View view) {//创建file对象用于存储拍照后的图片File outputImage=new File(getExternalCacheDir(),"output_image.jpg");try {if(outputImage.exists()){outputImage.delete();}outputImage.createNewFile();}catch(IOException e){e.printStackTrace();}if(Build.VERSION.SDK_INT>=24){imageUri= FileProvider.getUriForFile(MainActivity.this,"com.example.cameraalbumtest.fileprovider",outputImage);}else{imageUri= Uri.fromFile(outputImage);}//启动相机程序Intent intent =new Intent("android.media.action.IMAGE_CAPTURE");intent.putExtra(MediaStore.EXTRA_OUTPUT,imageUri);startActivityForResult(intent,TAKE_PHOTO);}});}private void openAlbum() {Intent intent01 = new Intent("android.intent.action.GET_CONTENT");intent01.setType("image/*");startActivityForResult(intent01, CHOOSE_PHOTO);}@Overrideprotected void onActivityResult(int requestCode, int resultCode,Intent data) {super.onActivityResult(requestCode, resultCode, data);switch (requestCode) {case TAKE_PHOTO:if (resultCode == RESULT_OK) {try {//将拍摄的照片显示出来Bitmap bitmap = BitmapFactory.decodeStream(getContentResolver().openInputStream(imageUri));picture.setImageBitmap(bitmap);} catch (FileNotFoundException e) {e.printStackTrace();}}break;case CHOOSE_PHOTO:if (resultCode == RESULT_OK) {if (Build.VERSION.SDK_INT >= 19) {handleImageOnKitKat(data);} else {Toast.makeText(this, "2", Toast.LENGTH_SHORT).show();handleImageBeforeKitKat(data);}}break;default:break;}}@TargetApi(19)private void handleImageOnKitKat (Intent data){String imagePath = null;Uri uri = data.getData();if (DocumentsContract.isDocumentUri(this, uri)) {//如果是document类型的Uri,则通过document id处理String docId = DocumentsContract.getDocumentId(uri);if ("com.android.providers.media.documents".equals(uri.getAuthority())) {String id = docId.split(":")[1];//解析出数字格式的idString selection = MediaStore.Images.Media._ID + "=" + id;imagePath = getImagePath(MediaStore.Images.Media.EXTERNAL_CONTENT_URI, selection);} else if("com.android.providers.downloads.documents".equals(uri.getAuthority())){Uri contentUri = ContentUris.withAppendedId(Uri.parse("content://downloads/public downloads"), Long.valueOf(docId));imagePath = getImagePath(contentUri, null);}}else if ("content".equalsIgnoreCase(uri.getScheme())) {imagePath = getImagePath(uri,null);}else if("file".equalsIgnoreCase(uri.getScheme())) {imagePath=uri.getPath();}displayImage(imagePath);//根据图片路径显示图片}private void handleImageBeforeKitKat(Intent data) {Uri uri = data.getData();String imagePath = getImagePath(uri,null);displayImage(imagePath);}@SuppressLint("Range")private String getImagePath(Uri uri, String selection) {String path = null;//通过Uri和Selection来获取真是的路径图片Cursor cursor = getContentResolver().query(uri,null,selection,null,null);if (cursor != null) {if (cursor.moveToFirst()) {path = cursor.getString(cursor.getColumnIndex(MediaStore.Images.Media.DATA));}cursor.close();}return path;}private void displayImage(String imagePath) {if (imagePath != null) {if (Build.VERSION.SDK_INT >= 29) {picture.setImageURI(getImageContentUri(MainActivity.this, imagePath));} else {Bitmap bitmap = BitmapFactory.decodeFile(imagePath);picture.setImageBitmap(bitmap);}} else {Toast.makeText(this, "failed to get image", Toast.LENGTH_SHORT).show();}}/*** 将图片转换成Uri* @param context 传入上下文参数* @param path 图片的路径* @return 返回的就是一个Uri对象*/public static Uri getImageContentUri(Context context, String path) {Cursor cursor = context.getContentResolver().query(MediaStore.Images.Media.EXTERNAL_CONTENT_URI,new String[] { MediaStore.Images.Media._ID }, MediaStore.Images.Media.DATA + "=? ",new String[] { path }, null);if (cursor != null && cursor.moveToFirst()) {int id = cursor.getInt(cursor.getColumnIndex(MediaStore.MediaColumns._ID));Uri baseUri = Uri.parse("content://media/external/images/media");return Uri.withAppendedPath(baseUri, "" + id);} else {// 如果图片不在手机的共享图片数据库,就先把它插入。if (new File(path).exists()) {ContentValues values = new ContentValues();values.put(MediaStore.Images.Media.DATA, path);return context.getContentResolver().insert(MediaStore.Images.Media.EXTERNAL_CONTENT_URI, values);} else {return null;}}}
}
相关文章:
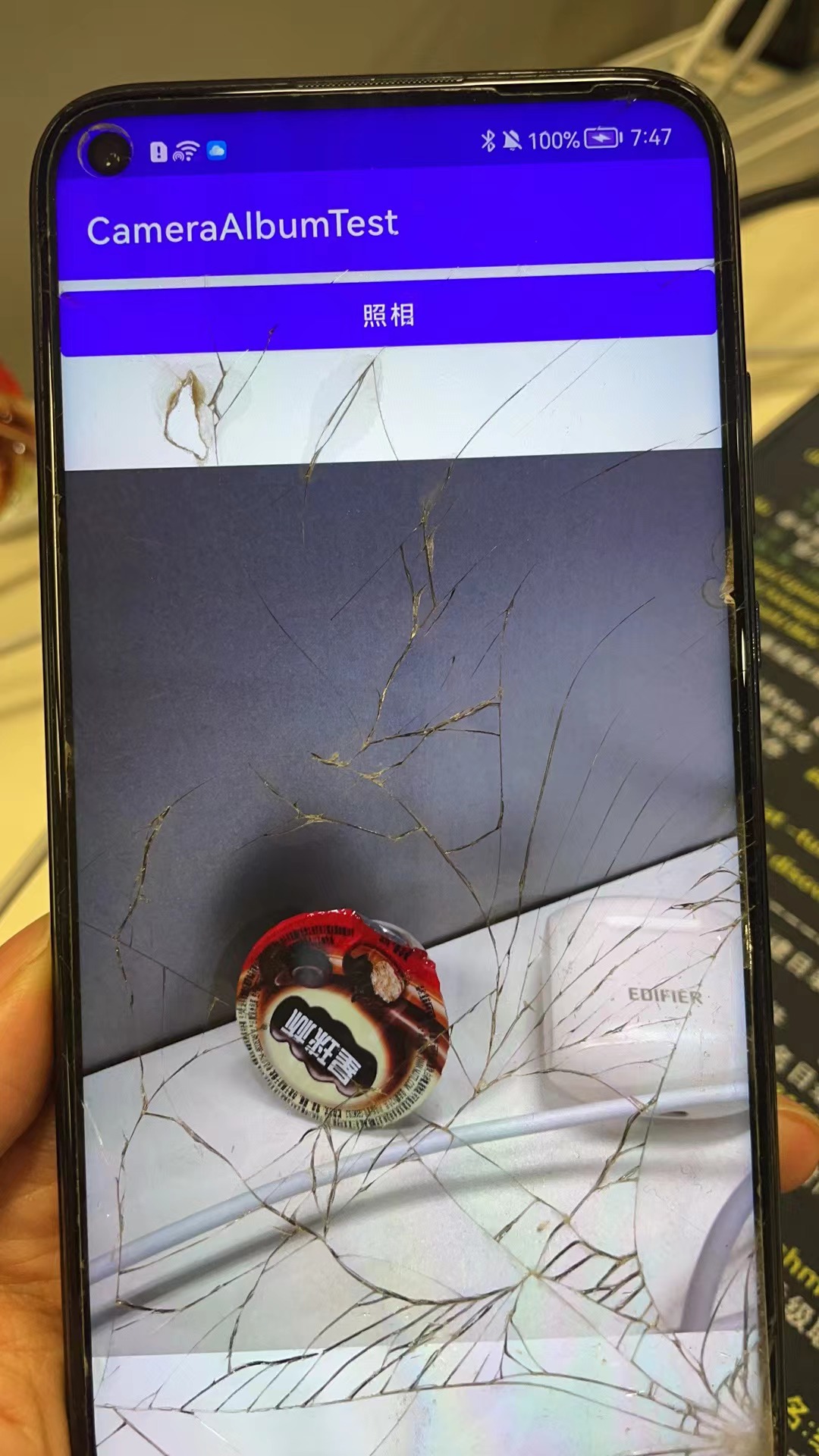
《第一行代码》 第八章:应用手机多媒体
一,使用通知 第一步,创建项目,书写布局 <LinearLayout xmlns:android"http://schemas.android.com/apk/res/android"android:orientation"vertical"android:layout_width"match_parent"android:layout_he…...
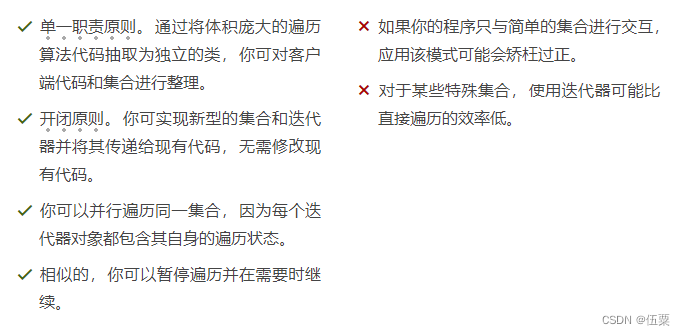
C++设计模式(20)——迭代器模式
亦称: Iterator 意图 迭代器模式是一种行为设计模式, 让你能在不暴露集合底层表现形式 (列表、 栈和树等) 的情况下遍历集合中所有的元素。 问题 集合是编程中最常使用的数据类型之一。 尽管如此, 集合只是一组对…...
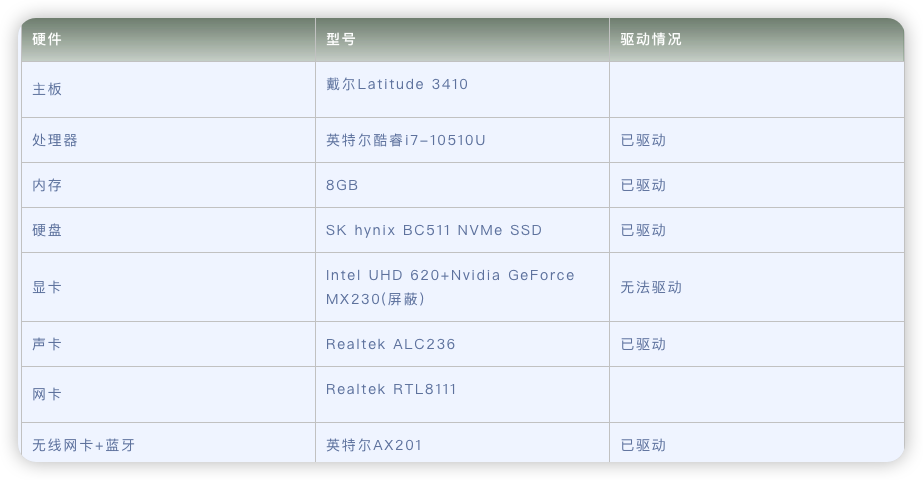
戴尔Latitude 3410电脑 Hackintosh 黑苹果efi引导文件
原文来源于黑果魏叔官网,转载需注明出处。硬件型号驱动情况主板戴尔Latitude 3410处理器英特尔酷睿i7-10510U已驱动内存8GB已驱动硬盘SK hynix BC511 NVMe SSD已驱动显卡Intel UHD 620Nvidia GeForce MX230(屏蔽)无法驱动声卡Realtek ALC236已驱动网卡Realtek RTL81…...
一起Talk Android吧(第五百零四回:如何调整组件在约束布局中的位置)
文章目录 背景介绍调整方法一调整方法二经验分享各位看官们大家好,上一回中咱们说的例子是"解决retrofit被混淆后代码出错的问题",这一回中咱们说的例子是" 如何调整组件在约束布局中的位置"。闲话休提,言归正转, 让我们一起Talk Android吧! 背景介绍…...
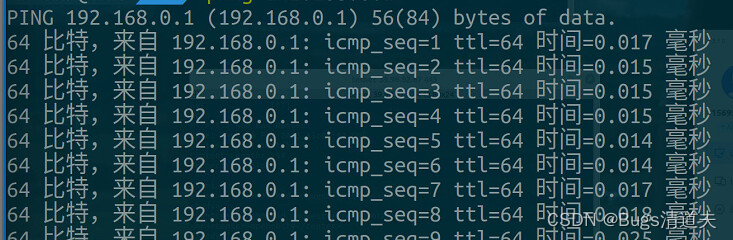
ssh连不上实验室的物理机了
实验室的电脑,不能在校外用 ssh 连接了 192.168.1.33 是本地地址,掩码16位,图1。 192.168.1.14 是实验室的另一台可以ssh连接的物理机,掩码16。 192.168.0.1 是无线路由器地址。 192.168.0.2 是192.168.1.14上的虚拟机地址&#…...
selinux讲解
Selinux讲解 1、selinux的概述 Selinux的历史 Linux安全性与windows在不开启防御措施的时候是一样的;同样是C2级别的安全防护安全级别评定: D–>C1–>C2–>B1–>B2–>B3–>A1 D级,最低安全性C1级,主存取控制…...
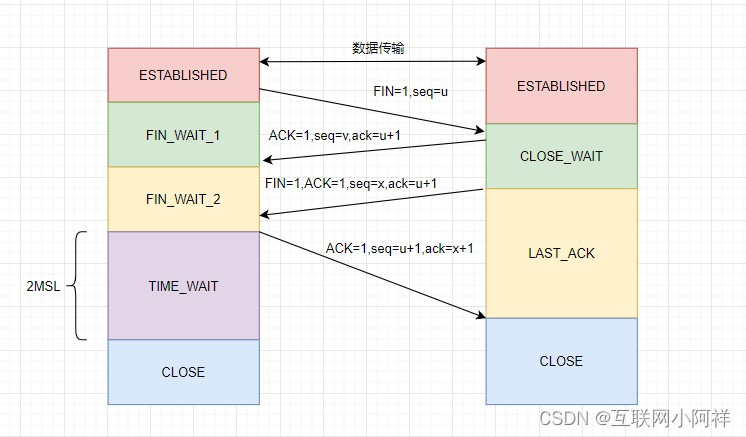
【计算机网络】TCP底层设计交互原理
文章目录1.TCP底层三次握手详细流程2.TCP洪水攻击介绍和ss命令浅析3.Linux服务器TCP洪水攻击入侵案例4.TCP洪水攻击结果分析和解决方案5.TCP底层四次挥手详细流程1.TCP底层三次握手详细流程 TCP的可靠性传输机制:TCP三次我手的流程 一次握手:客户端发送一…...
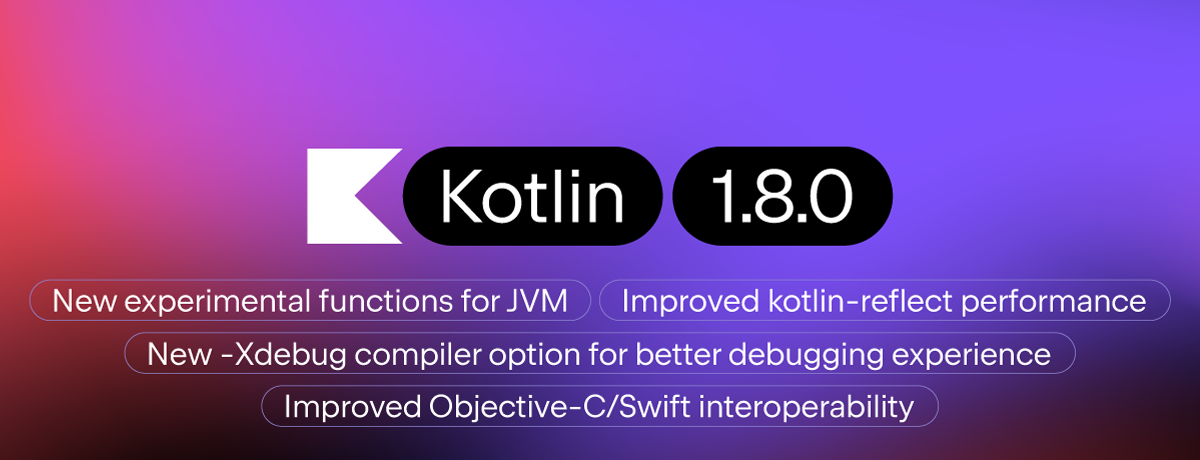
Kotlin1.8新特性
Kotlin1.8.0新特性 新特性概述 JVM 的新实验性功能:递归复制或删除目录内容提升了 kotlin-reflect 性能新的 -Xdebug 编译器选项,提供更出色的调试体验kotlin-stdlib-jdk7 与 kotlin-stdlib-jdk8 合并为 kotlin-stdlib提升了 Objective-C/Swift 互操作…...
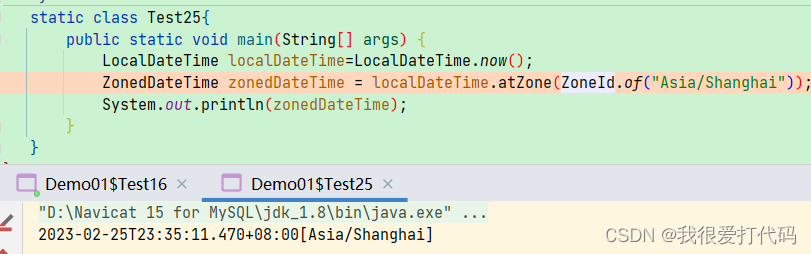
【Java8】
1、接口中默认方法修饰为普通方法 在jdk8之前,interface之中可以定义变量和方法,变量必须是public、static、final的,方法必须是public、abstract的,由于这些修饰符都是默认的。 接口定义方法: public抽象方法需要子类实现 接口定…...
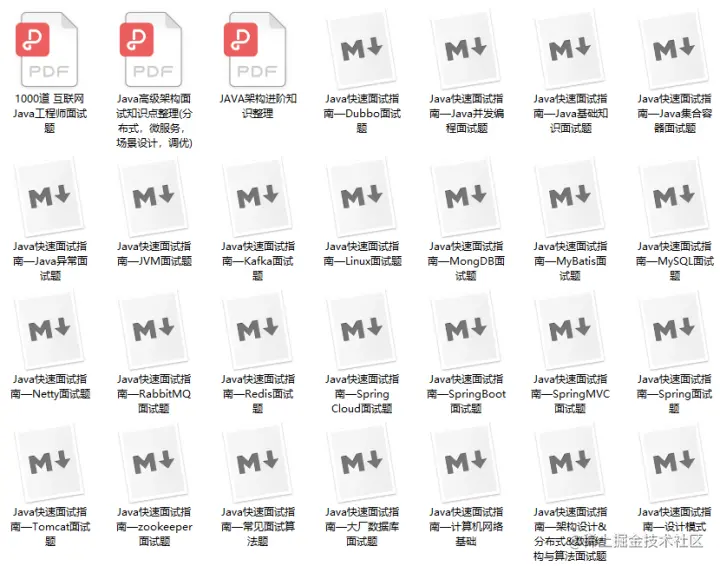
阿里 Java 程序员面试经验分享,附带个人学习笔记、路线大纲
背景经历 当时我工作近5年,明显感觉到了瓶颈期。说句不好听的成了老油条,可以每天舒服的混日子(这也有好处,有时间准备面试)。这对于个人成长不利,长此以往可能面临大龄失业。所以我觉得需要痛下决心改变一…...
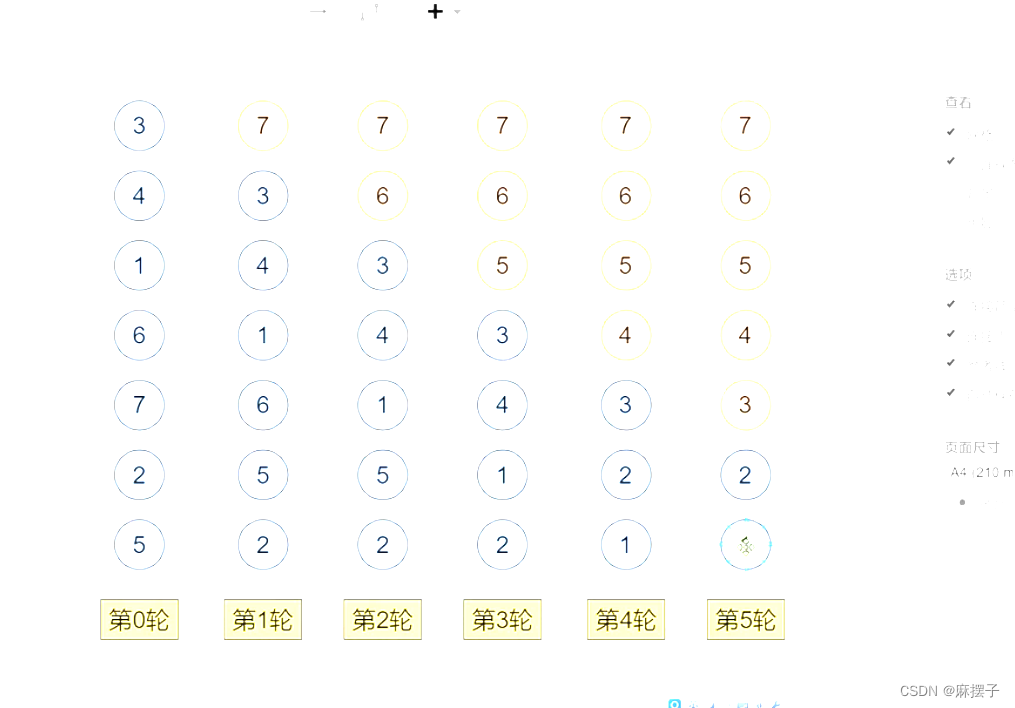
十大算法基础——上(共有20道例题,大多数为简单题)
一、枚举(Enumerate)算法 定义:就是一个个举例出来,然后看看符不符合条件。 举例:一个数组中的数互不相同,求其中和为0的数对的个数。 for (int i 0; i < n; i)for (int j 0; j < i; j)if (a[i] …...
【PAT甲级题解记录】1018 Public Bike Management (30 分)
【PAT甲级题解记录】1018 Public Bike Management (30 分) 前言 Problem:1018 Public Bike Management (30 分) Tags:dijkstra最短路径 DFS Difficulty:剧情模式 想流点汗 想流点血 死而无憾 Address:1018 Public Bike Managemen…...
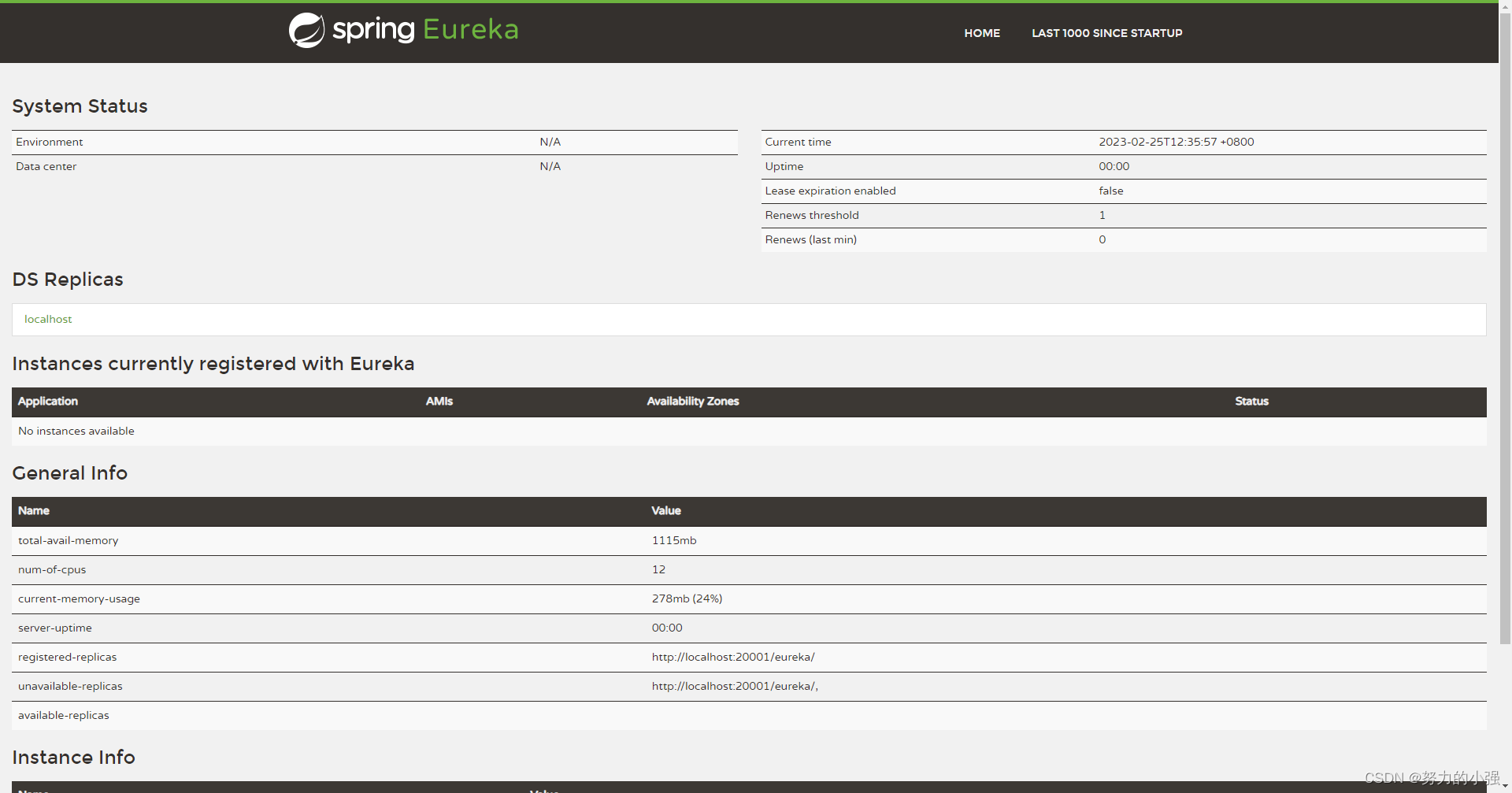
SpringCloud————Eureka概述及单机注册中心搭建
Spring Cloud Eureka是Netflix开发的注册发现组件,本身是一个基于REST的服务。提供注册与发现,同时还提供了负载均衡、故障转移等能力。 Eureka组件的三个角色 服务中心服务提供者服务消费者 Eureka Server:服务器端。提供服务的注册和发现…...
原生django raw() 分页
def change_obj_to_dict(self,temp):dict {}dict["wxh_name"] temp.wxh_namedict["types"] temp.typesdict["subject"] temp.subjectdict["ids"] temp.ids# 虽然产品表里没有替代型号,但是通过sql语句的raw()查询可以…...
Android 9.0 Settings 搜索功能屏蔽某个app
1.概述 在9.0的系统rom产品定制化开发过程中,在系统Settings的开发功能中,最近产品需求要求去掉搜索中屏蔽某个app的搜索,就是根据包名,不让搜索出某个app., 在系统setting中,搜索功能中,根据包名过滤掉某个app的搜索功能,所以需要熟悉系统Settings中的搜索的相关功能,…...
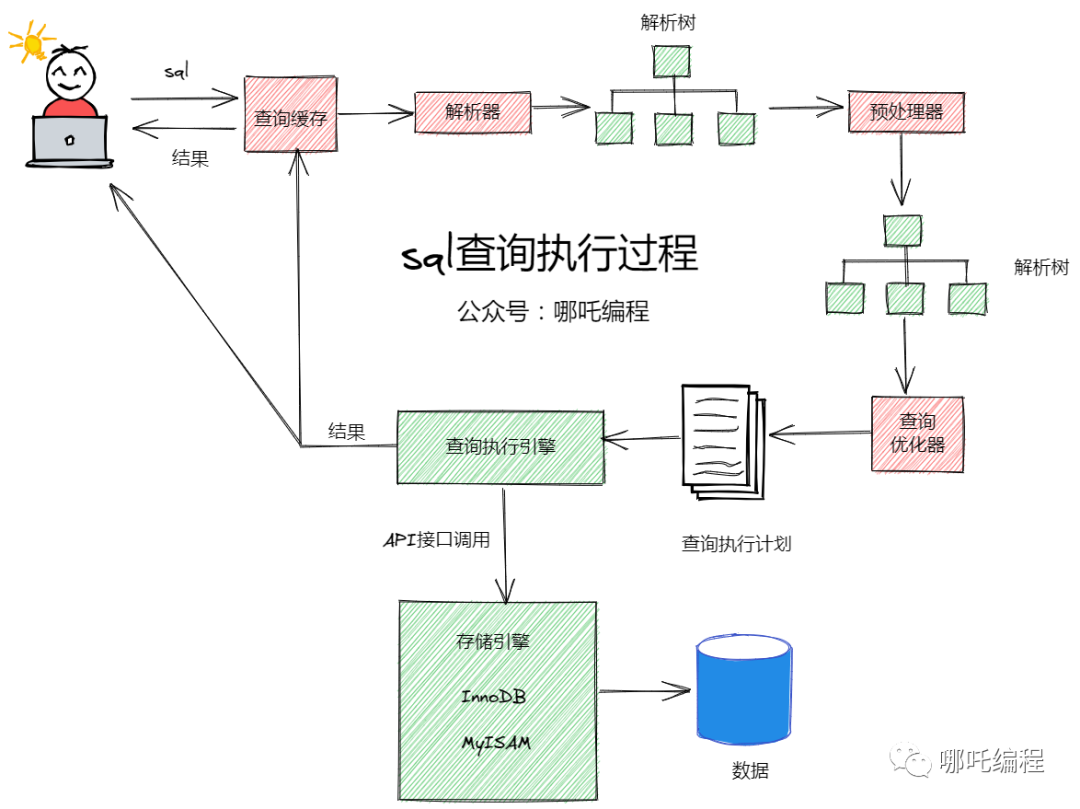
SQL性能优化的47个小技巧,果断收藏!
1、先了解MySQL的执行过程 了解了MySQL的执行过程,我们才知道如何进行sql优化。 客户端发送一条查询语句到服务器; 服务器先查询缓存,如果命中缓存,则立即返回存储在缓存中的数据; 未命中缓存后,MySQL通…...

SE | 哇哦!让人不断感叹真香的数据格式!~
1写在前面 最近在用的包经常涉及到SummarizedExperiment格式的文件,不知道大家有没有遇到过。🤒 一开始觉得这种格式真麻烦,后面搞懂了之后发现真是香啊,爱不释手!~😜 2什么是SummarizedExperiment 这种cla…...
运行Qt后出现无法显示字库问题的解决方案
问题描述:运行后字体出现问题QFontDatabase: Cannot find font directory解决前提: 其实就是移植后字体库中是空的,字没办法进行显示本质就是我们只需要通过某种手段将QT界面中的字母所调用的库进行填充即可此处需要注意的是,必须…...
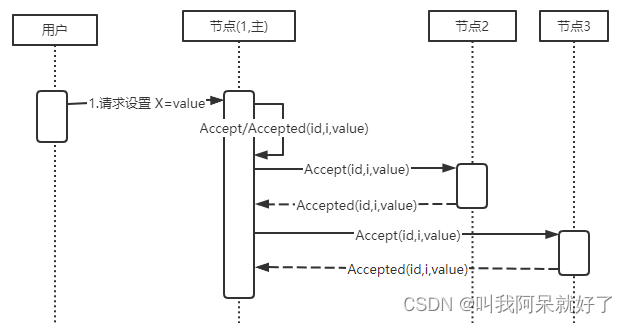
数据库浅谈之共识算法
数据库浅谈之共识算法 HELLO,各位博友好,我是阿呆 🙈🙈🙈 这里是数据库浅谈系列,收录在专栏 DATABASE 中 😜😜😜 本系列阿呆将记录一些数据库领域相关的知识 …...
代码随想录算法训练营 || 贪心算法 455 376 53
Day27贪心算法基础贪心的本质是选择每一阶段的局部最优,从而达到全局最优。刷题或者面试的时候,手动模拟一下感觉可以局部最优推出整体最优,而且想不到反例,那么就试一试贪心。做题的时候,只要想清楚 局部最优 是什么&…...
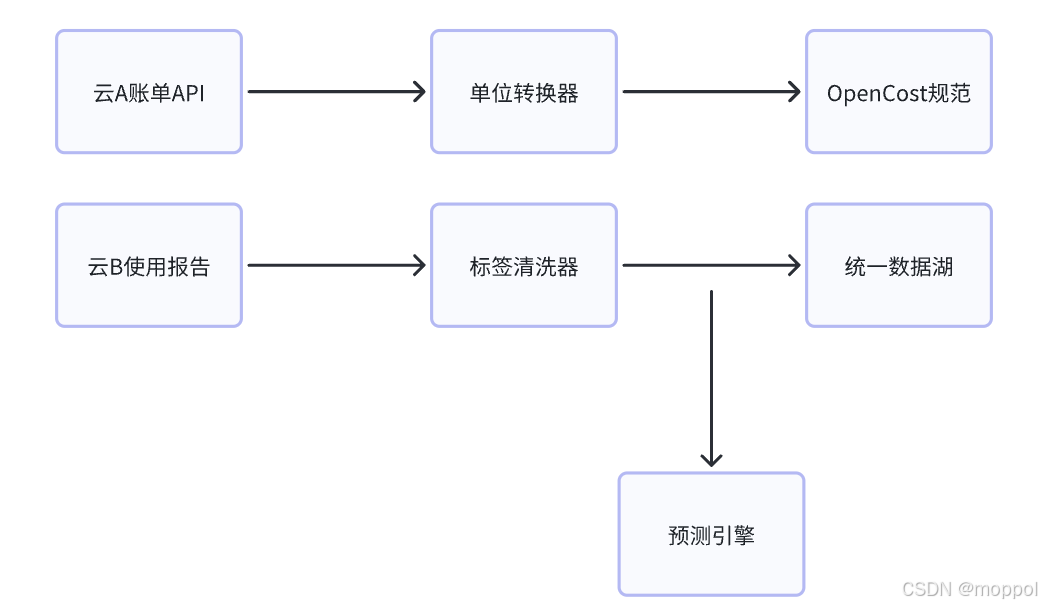
多云管理“拦路虎”:深入解析网络互联、身份同步与成本可视化的技术复杂度
一、引言:多云环境的技术复杂性本质 企业采用多云策略已从技术选型升维至生存刚需。当业务系统分散部署在多个云平台时,基础设施的技术债呈现指数级积累。网络连接、身份认证、成本管理这三大核心挑战相互嵌套:跨云网络构建数据…...
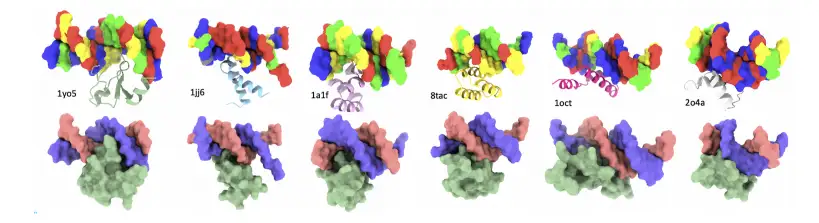
8k长序列建模,蛋白质语言模型Prot42仅利用目标蛋白序列即可生成高亲和力结合剂
蛋白质结合剂(如抗体、抑制肽)在疾病诊断、成像分析及靶向药物递送等关键场景中发挥着不可替代的作用。传统上,高特异性蛋白质结合剂的开发高度依赖噬菌体展示、定向进化等实验技术,但这类方法普遍面临资源消耗巨大、研发周期冗长…...
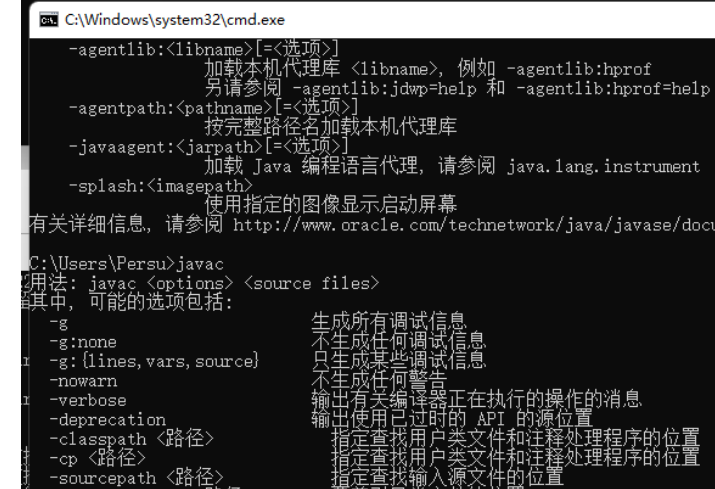
python/java环境配置
环境变量放一起 python: 1.首先下载Python Python下载地址:Download Python | Python.org downloads ---windows -- 64 2.安装Python 下面两个,然后自定义,全选 可以把前4个选上 3.环境配置 1)搜高级系统设置 2…...
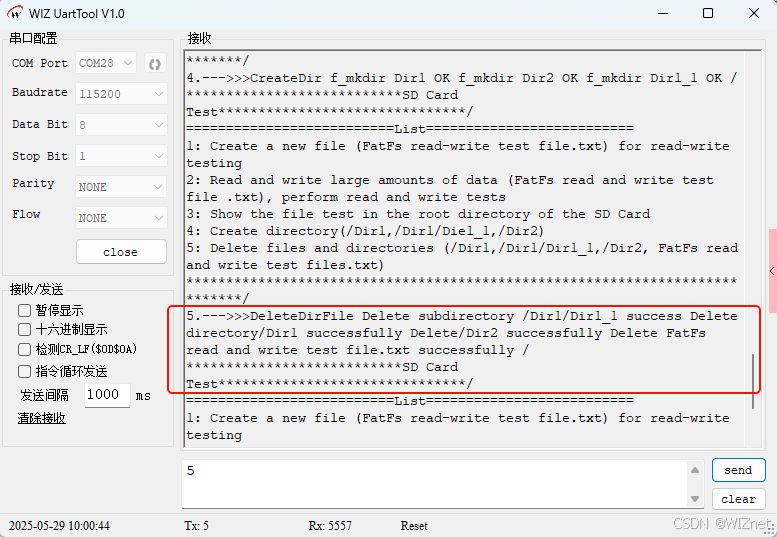
【第二十一章 SDIO接口(SDIO)】
第二十一章 SDIO接口 目录 第二十一章 SDIO接口(SDIO) 1 SDIO 主要功能 2 SDIO 总线拓扑 3 SDIO 功能描述 3.1 SDIO 适配器 3.2 SDIOAHB 接口 4 卡功能描述 4.1 卡识别模式 4.2 卡复位 4.3 操作电压范围确认 4.4 卡识别过程 4.5 写数据块 4.6 读数据块 4.7 数据流…...
【磁盘】每天掌握一个Linux命令 - iostat
目录 【磁盘】每天掌握一个Linux命令 - iostat工具概述安装方式核心功能基础用法进阶操作实战案例面试题场景生产场景 注意事项 【磁盘】每天掌握一个Linux命令 - iostat 工具概述 iostat(I/O Statistics)是Linux系统下用于监视系统输入输出设备和CPU使…...
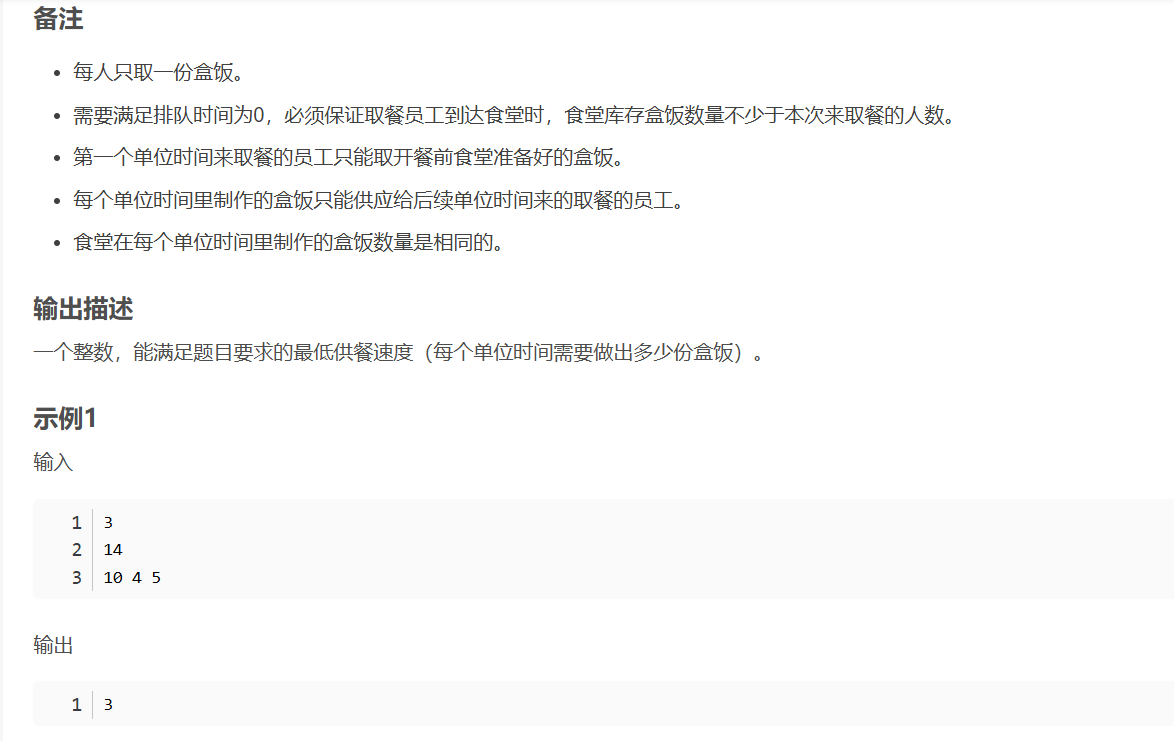
华为OD机试-食堂供餐-二分法
import java.util.Arrays; import java.util.Scanner;public class DemoTest3 {public static void main(String[] args) {Scanner in new Scanner(System.in);// 注意 hasNext 和 hasNextLine 的区别while (in.hasNextLine()) { // 注意 while 处理多个 caseint a in.nextIn…...
TRS收益互换:跨境资本流动的金融创新工具与系统化解决方案
一、TRS收益互换的本质与业务逻辑 (一)概念解析 TRS(Total Return Swap)收益互换是一种金融衍生工具,指交易双方约定在未来一定期限内,基于特定资产或指数的表现进行现金流交换的协议。其核心特征包括&am…...
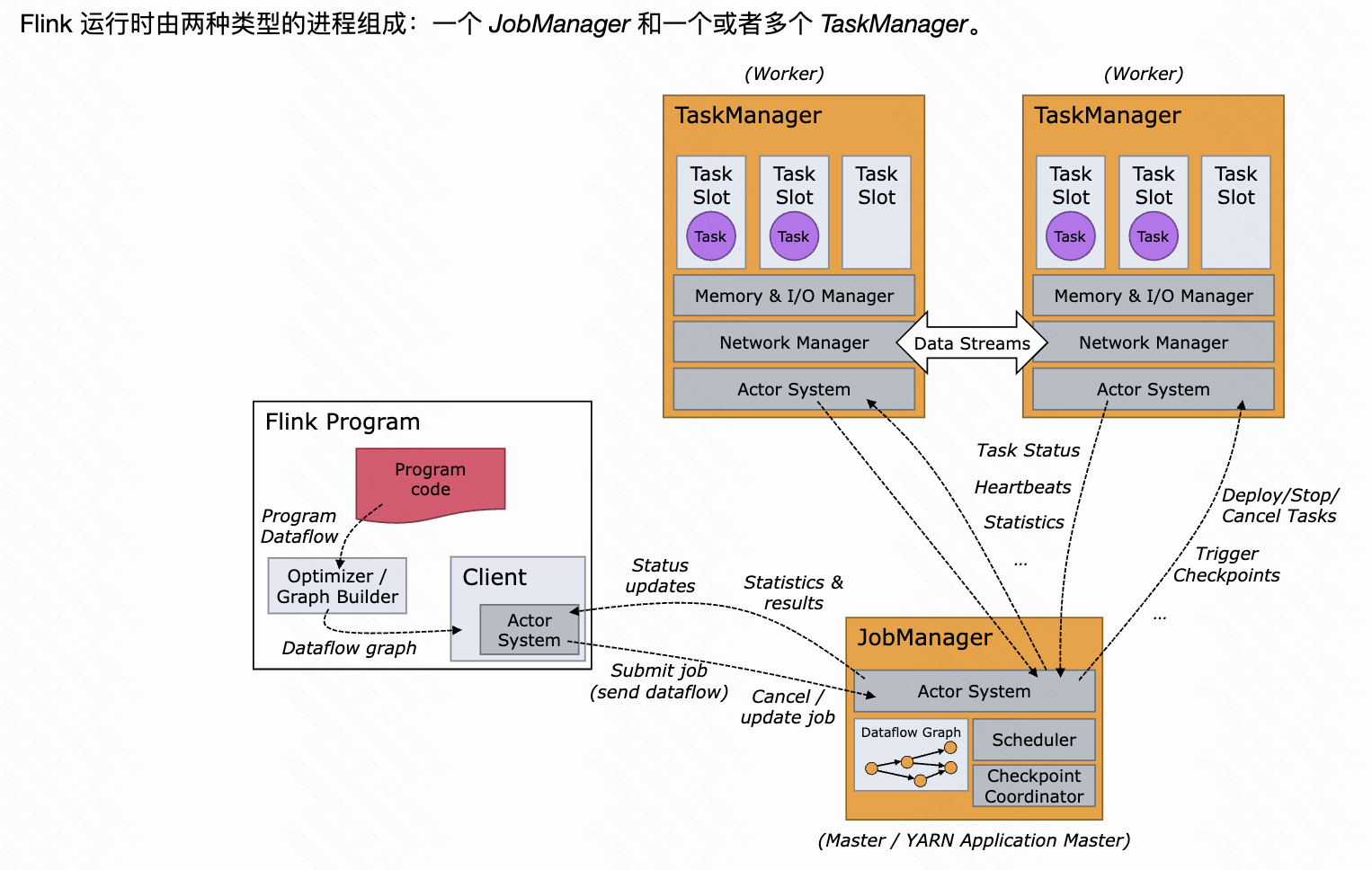
《基于Apache Flink的流处理》笔记
思维导图 1-3 章 4-7章 8-11 章 参考资料 源码: https://github.com/streaming-with-flink 博客 https://flink.apache.org/bloghttps://www.ververica.com/blog 聚会及会议 https://flink-forward.orghttps://www.meetup.com/topics/apache-flink https://n…...
CRMEB 框架中 PHP 上传扩展开发:涵盖本地上传及阿里云 OSS、腾讯云 COS、七牛云
目前已有本地上传、阿里云OSS上传、腾讯云COS上传、七牛云上传扩展 扩展入口文件 文件目录 crmeb\services\upload\Upload.php namespace crmeb\services\upload;use crmeb\basic\BaseManager; use think\facade\Config;/*** Class Upload* package crmeb\services\upload* …...
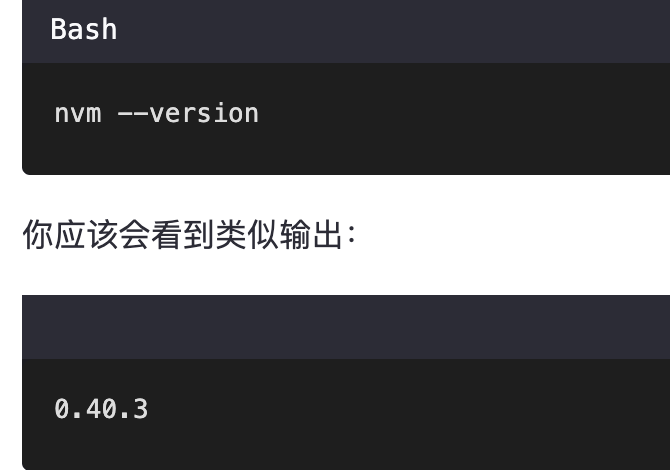
mac 安装homebrew (nvm 及git)
mac 安装nvm 及git 万恶之源 mac 安装这些东西离不开Xcode。及homebrew 一、先说安装git步骤 通用: 方法一:使用 Homebrew 安装 Git(推荐) 步骤如下:打开终端(Terminal.app) 1.安装 Homebrew…...