产教融合平台建设网站/定制企业网站建设制作
struct person
{char name[16]; //名字float height; //身高float height; //体重char cell[32]; //手机号
};
class person
{private:char name[16]; //名字float height; //身高float weight; //体重char cell[32]; //手机号public:int running(char *WayName,int time); //跑步行为int eat(char *ObjectName,int l); //存放行为
};
class 和 struct 在 C++中的区别
类的访问修饰符
构造函数和析构函数
#include <iostream>
#include<string.h>
using namespace std;
class person
{private:char name[16]; //名字float height; //身高float weight; //体重char cell[32]; //手机号public:person();person(const char *ne,float h,float w,const char *ce);~person(){cout << name <<":执行了析构" << endl;}void run(){cout << "名字:"<< name << endl;cout << "身高:"<< height << endl;cout << "体重:"<< weight << endl;cout << "手机号:"<< cell << endl;}int running(char *WayName,int time); //跑步行为int eat(char *ObjectName,int l); //存放行为
};
person::person()
{strcpy(name,"zhangsan");height = 168.4;weight = 64.2;strcpy(cell,"17873432557");
}
person::person(const char *ne,float h,float w,const char *ce)
{strcpy(name,ne);height = h;weight = w;strcpy(cell,ce);
}
int main()
{person a; //创建一个对象,执行的是没有参数的构造函数person b{"jiuyue",178.7,76.6,"110"}; //创建一个对象执行有参数的构造函数a.run();b.run();return 0;
}
person a ();
person a ;// 或person a {};
构造函数初始化列表
class CExample
{public:int a;float b;
//构造函数初始化列表CExample(): a(0),b(8.8){}
//构造函数内部赋值CExample(){a=0;b=8.8;}
};
我们来看第一种情况为什么要使用构造函数初始化列表:(成员对象没有默认构造函数)
#include <iostream>
#include<string.h>
using namespace std;
class A
{private:int a;char b;public:A(int x,char y){a = x;b = y;cout << "a 的构造" << endl;}
};
class B
{private:A a;int c;public:B(int z,char n,int x):a(z,n){ c = x;}
};
int main(int argc, char const *argv[])
{B b(1,'2',3);return 0;
}
#include <iostream>
#include<string.h>
using namespace std;
class A
{private:int a;const int b;char& c;public:A(int x,char y):b(x),c(y){a = 3;//b = 4; //const 常量只能在声明时初始化,之后不能改变//c = ? //引用只能在声明的时候进行初始化}
};
int main(int argc, char const *argv[])
{char i = '1';A(4,i);return 0;
}
#include <iostream>
#include<string.h>
using namespace std;
class A
{private:int a;const int b;char& c;public:A(int x,char y):b(x),c(y){a = 3;//b = 4; //const 常量只能在声明时初始化,之后不能改变//c = ? //引用只能在声明的时候进行初始化}
};
class B:public A
{private:int x;public:B(int a1,char b1):A(a1,b1){x = 2;}
};
int main(int argc, char const *argv[])
{char i = '1';B(4,i);return 0;
}
结论
int a = 3;
const char c = '1';
A b(2,5);
int a;
const char c;
A b;
a = 3;
c = '1' //error
b(3,5); //error
临时对象
例如:
#include <iostream>
#include<string.h>
using namespace std;
class A
{private:int a;int b;public:A(int x,int y):a(x),b(y){cout << "构造" <<endl;}~A(){cout << "析构" <<endl;}
A fun()
{return A(a,b);
}
};
int main(int argc, char const *argv[])
{A i = A(1,2);A j = i.fun();return 0;
}
类的只读成员函数
class A
{private:int a;int b;public:A(int x,int y):a(x),b(y){}int fun() const{//...}
};
拷贝构造函数
类名 (const 类名 &) ;
class A
{private:int a;int b;public:A(int x,int y):a(x),b(y){} //构造函数初始化列表A(const A &obj) //拷贝构造函数{// 构造函数的主体}
};
int main(int argc, char const *argv[])
{A i(1,2);A j = i; //调用了拷贝构造A k(i); //调用了拷贝构造return 0;
}
省略复制
#include <iostream>
#include<string.h>
using namespace std;
class A
{private:char buf[16];public:A(const char *str){strcpy(buf,str);cout << "构造函数" << endl;}A(const A& a){strcpy(this->buf,a.buf);cout << "拷贝构造" << endl;}
};
int main()
{A ob = "nihao";return 0;
}
#include <iostream>
#include<string.h>
using namespace std;
class A
{private:char buf[16];public:A(const char *str){strcpy(buf,str);cout << "构造函数" << endl;}A(const A& a){strcpy(this->buf,a.buf);cout << "拷贝构造" << endl;}
};
A fnn()
{return A("nihao");
}
//或
A fuu()
{A a("nihao");return a;
}
int main()
{A ob = fuu();return 0;
}
this 指针
class A
{private:int a;int b;public:A(int x,int y):a(x),b(y){} //构造函数初始化列表void fun(){this->a = 4; //this 就是该对象的地址(*this).b = 5; //*this 就相当于对象本身}
};
class A
{private:int a;int b;public:A(int x,int y):a(x),b(y){} //构造函数初始化列表void fun(int a,int b){//a = a; //erreor ,系统会以为是形参 a 赋值给形参 a,并不会改变成员变量的值//b = b; //同上理this->a = a;this->b = b;}
};
void sun ( A * this );void fun ( int a , int b , A * this );
A a ( 1 , 2 );a . sun (& a );a . fun ( 1 , 2 ,& a );
类的静态成员函数
class person
{
public:static void func(); //只需要在类内进行声明
};
int main(int argc, char const *argv[])
{A::fun();return 0;
}
class A
{private:int a;int b;static int c;public:static void fun();
};
int A::c = 0; //静态成员变量初始化
#include <iostream>
#include<string.h>
using namespace std;
class A
{private:int a;int b;public:static int c;static void fun(){cout << c << endl;}
};
int A::c = 0;
int main(int argc, char const *argv[])
{A a;a.c = 0;A::c = 5;a.fun();return 0;
}
友元函数和友元类(friend)
友元函数
#include <iostream>
#include<string.h>
using namespace std;
class A; //对 A 进行超前声明,不然 sun 参数找不到
int sun(int a,int b,A& c);
class A
{private:int x;int y;public:A(int a,int b):x(a),y(b){}~A(){}friend int sun(int a,int b,A& c);
};
int sun(int a,int b, A& c)
{c.x = a;c.y = b;return c.x+c.y;
}
int main(int argc, char const *argv[])
{A a(2,4);sun(1,2,a);return 0;
}
#include <iostream>
#include<string.h>
using namespace std;
class A; //对 A 进行超前声明,不然 sun 参数找不到
class B
{private:int i; char j;public:B(int a,char c){i = a;j = c;}~B(){}int fun(A *rm);
};
class A
{private:int x;int y;public:A(int a,int b):x(a),y(b){}~A(){}friend int B::fun(A* rm); //友元
};
int B::fun(A *rm)
{i = rm->x + rm->y;j = 'a';return i;
}
int main(int argc, char const *argv[])
{A a(2,4);B b(2,'A');b.fun(&a);return 0;
}
友元类
#include <iostream>
#include<string.h>
using namespace std;
class A; //对 A 进行超前声明,不然 sun 参数找不到
class B
{private:int i; char j;public:B(int a,char c){i = a;j = c;}~B(){}int fun(A &a);
};
class A
{private:int x;int y;friend B;public:A(int a,int b):x(a),y(b){}~A(){}
};
int B::fun(A &a)
{i = a.x + a.y;return i;
}
int main(int argc, char const *argv[])
{A a(2,4);B b(2,'A');b.fun(a);return 0;
}
友元注意事项
相关文章:

类(class)
类是 C中一个非常重要的元素,可以说是 C的灵魂所在了,我们都知道 C说一种面向对象的编程语言,那么面向对象是一种什么概念呢?在 C程序设计中,所有一切东西都可以称之为对象,任何对象都应该具有属性和行为。…...
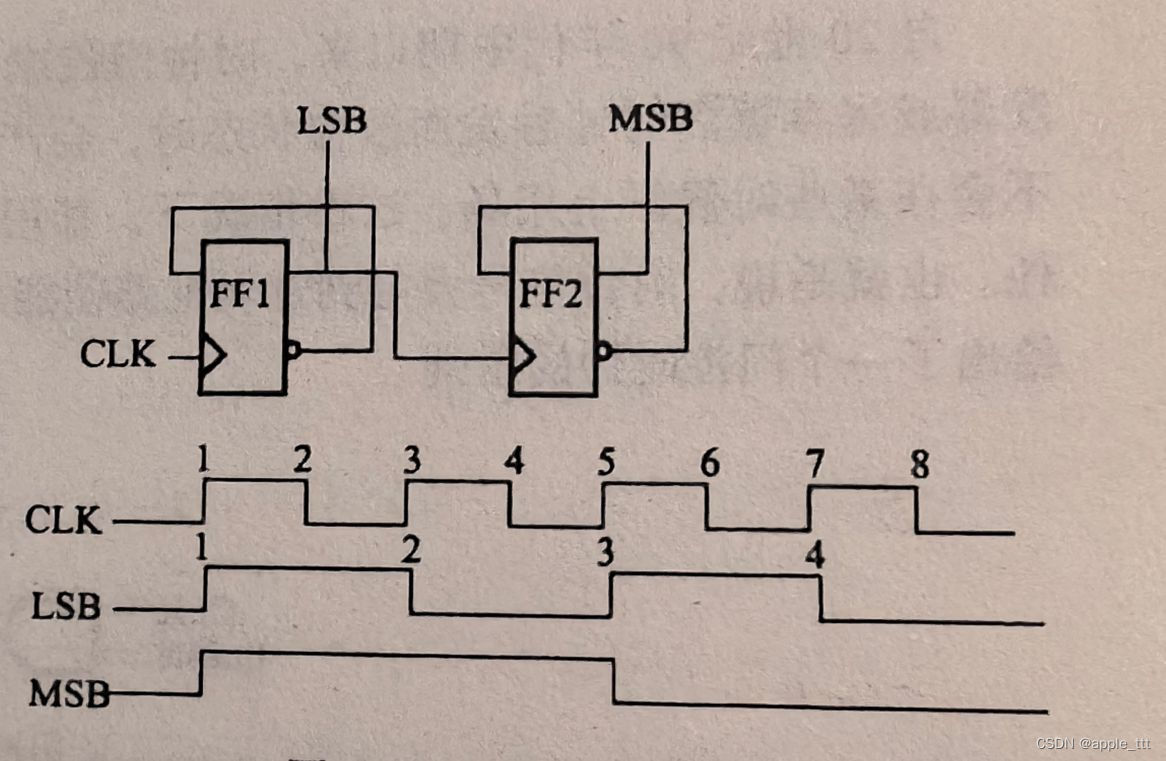
FPGA时序分析与约束(10)——生成时钟
一、概述 最复杂的设计往往需要多个时钟来完成相应的功能。当设计中存在多个时钟的时候,它们需要相互协作或各司其职。异步时钟是不能共享确定相位关系的时钟信号,当多个时钟域交互时,设计中只有异步时钟很难满足建立和保持要求。我们将在后面…...
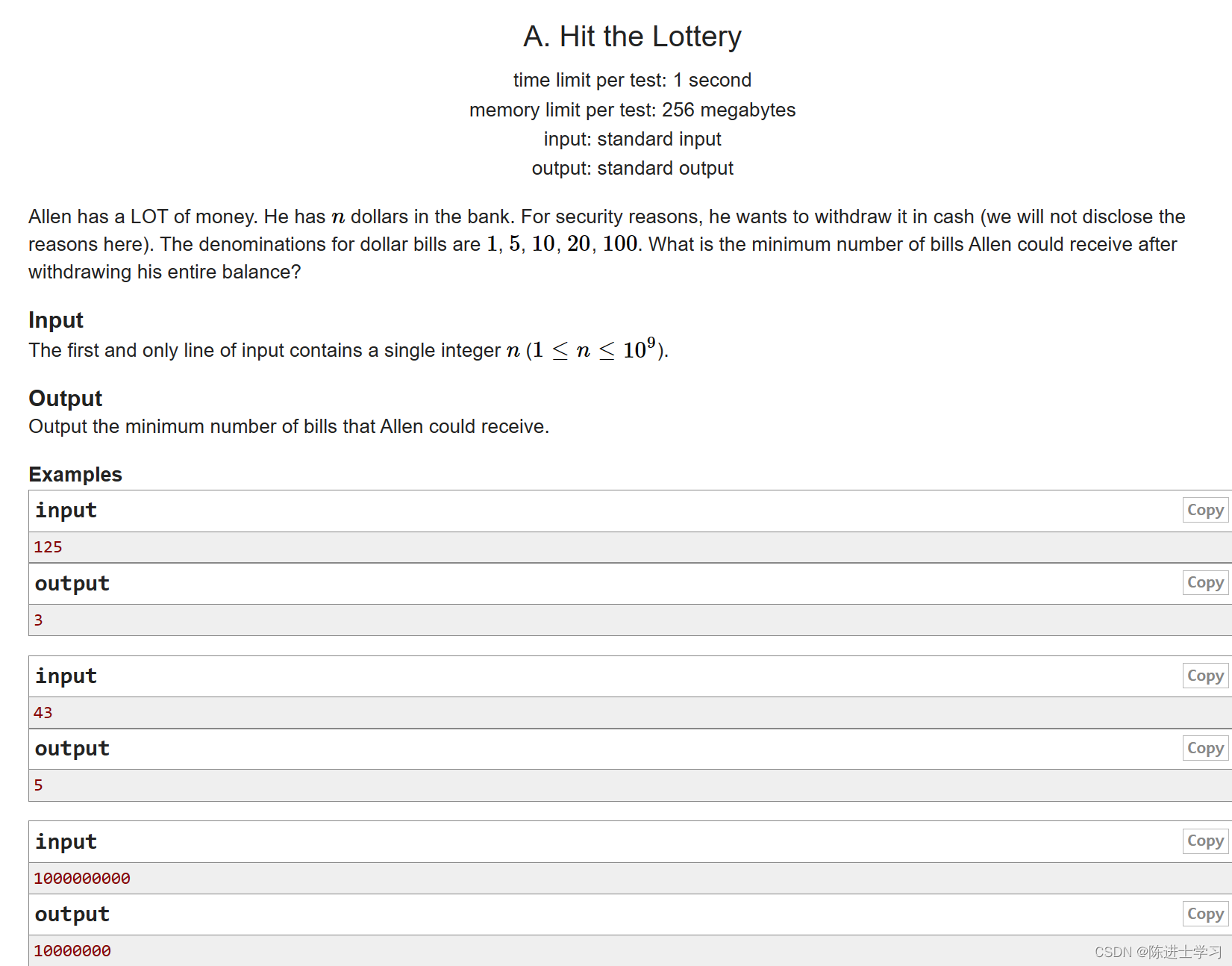
A. Hit the Lottery
#include<bits/stdc.h> using namespace std; const int N1e55; int n,a[N],res; int main(){scanf("%d",&n);int an/100;n%100;int bn/20;n%20;int cn/10;n%10;int dn/5;n%5;int en;cout<<abcde;return 0; }...
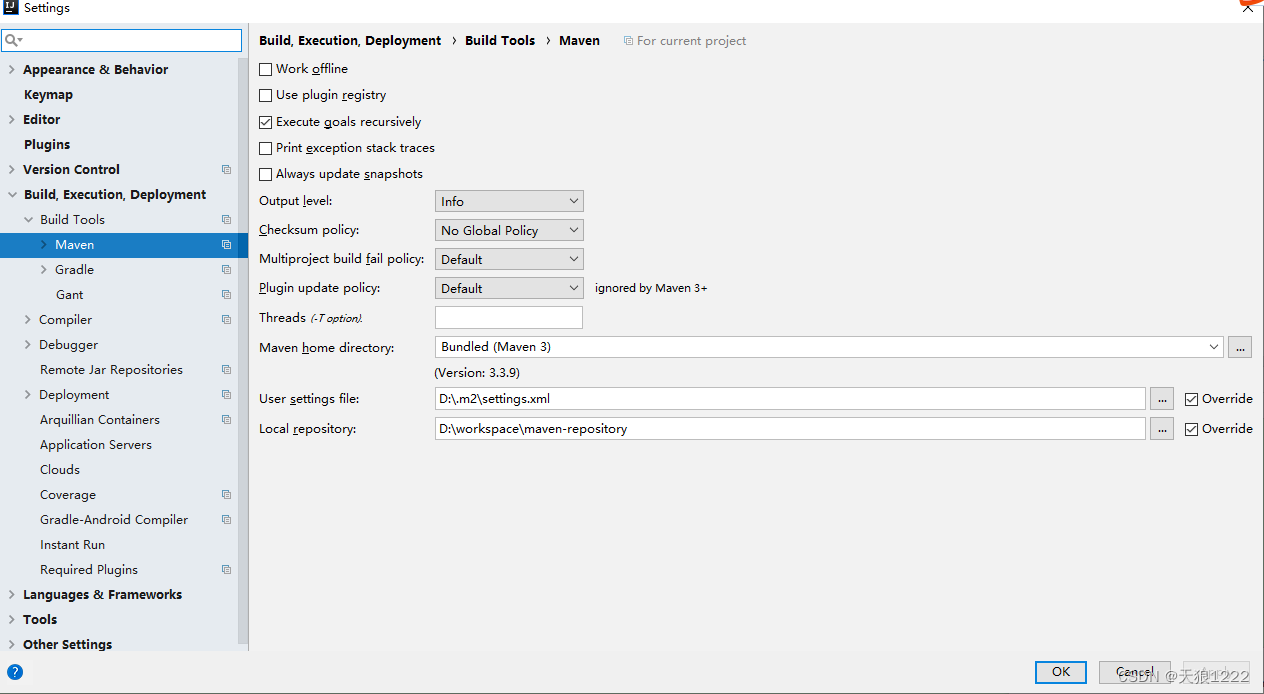
mvn: Downloading from pluginRepository
场景 maven 项目打包 mvn package 报git地址错误(有换新的git地址) 检查了下 settting.xml的配置没问题。是新的git地址。 处理: 用命令: mvn -X [DEBUG] Message styles: debug info warning error success failure stron…...

docker相关知识
docker-compose https://www.runoob.com/docker/docker-compose.html Compose 使用的三个步骤: 使用 Dockerfile 定义应用程序的环境。 使用 docker-compose.yml 定义构成应用程序的服务,这样它们可以在隔离环境中一起运行。 最后,执行 …...

Springboot 集成 RocketMQ(进阶-消息)
0. 入门篇 Springboot 集成 RocketMq(入门)-CSDN博客 1. 异步消息 1.1 生产者 GetMapping("/send/async/{messageBody}")public String sendAsyncMsg(PathVariable("messageBody") String messageBody) {// 构建消息对象Message m…...
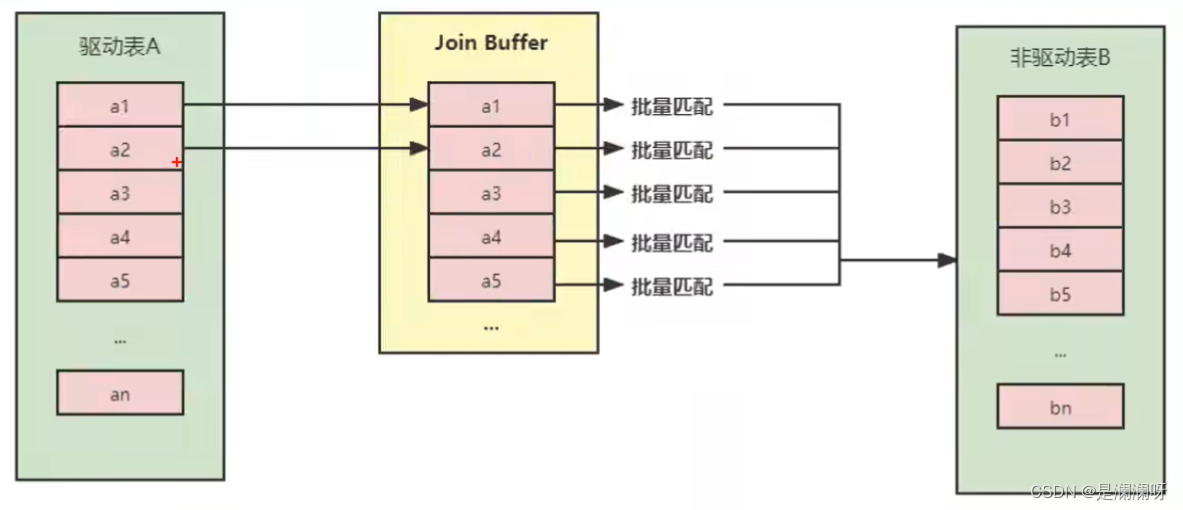
10 索引优化与查询优化
文章目录 索引失效案例关联查询优化对于左外连接对于内连接JOIN语句原理简单嵌套循环连接SNLJ索引嵌套循环连接INLJ块嵌套循环连接BNLJHash Join 子查询优化排序优化filesort算法:双路排序和单路排序 分组优化分页优化优先考虑覆盖索引索引下推ICP使用条件 其他查询…...

linux PVE安装
先下载安装包: ISO - Proxmox Virtual Environment 普通电脑主机的话,做个U盘启动盘,进行刷机即可。 如果还没制作U盘启动盘,建议用这个,方便多个镜像切换 Download . Ventoy 按照刷机提示页面一步步配置即可&#…...
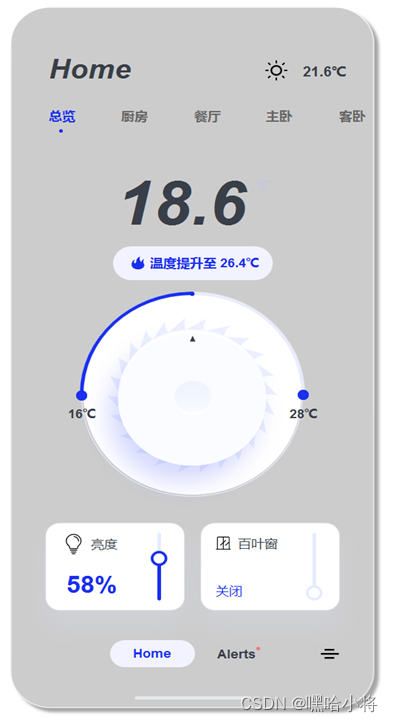
ZZ038 物联网应用与服务赛题第J套
2023年全国职业院校技能大赛 中职组 物联网应用与服务 任 务 书 (J卷) 赛位号:______________ 竞赛须知 一、注意事项 1.检查硬件设备、电脑设备是否正常。检查竞赛所需的各项设备、软件和竞赛材料等; 2.竞赛任务中所使用…...
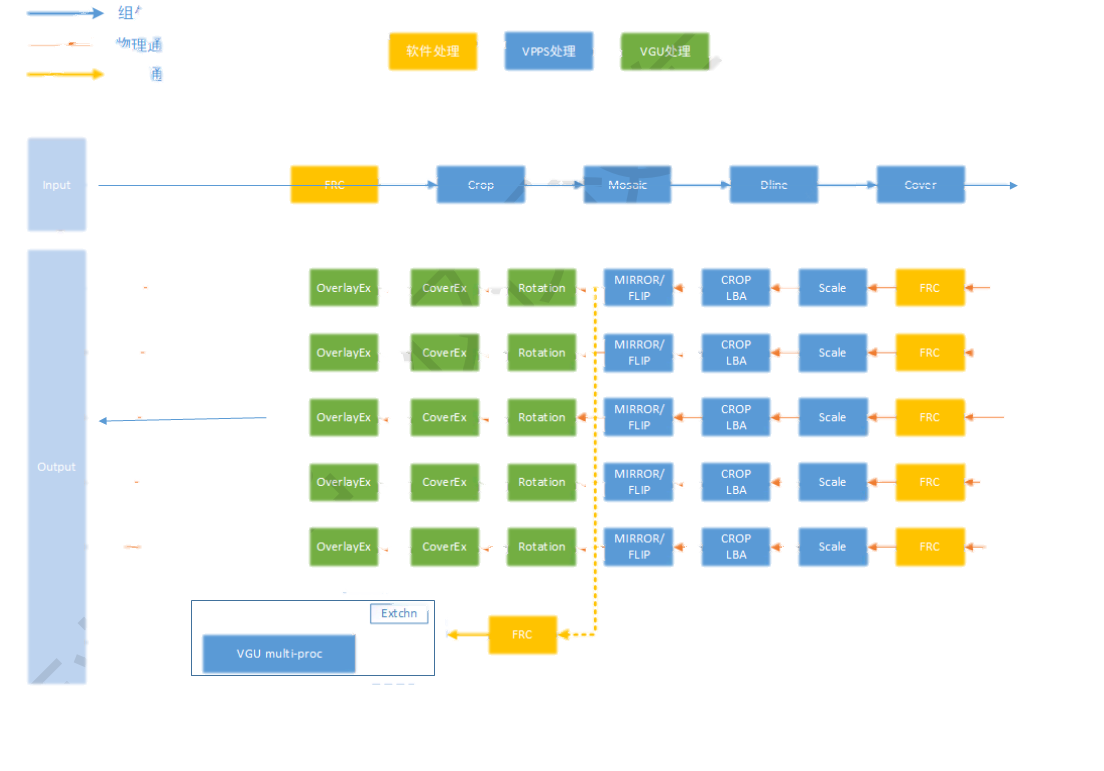
【寒武纪(3)】媒体处理系统的系统控制、视频输入和后处理子系统
系统控制 文章目录 系统控制1、配置视频缓存池Video Pool2、配置硬件IP为在线工作(不通过DDR数据交互)/ 离线工作(写入DDR)模式3、硬IP可以使用 非Video Block (VB)内存4、配置是否启动内存传递的压缩 视频…...
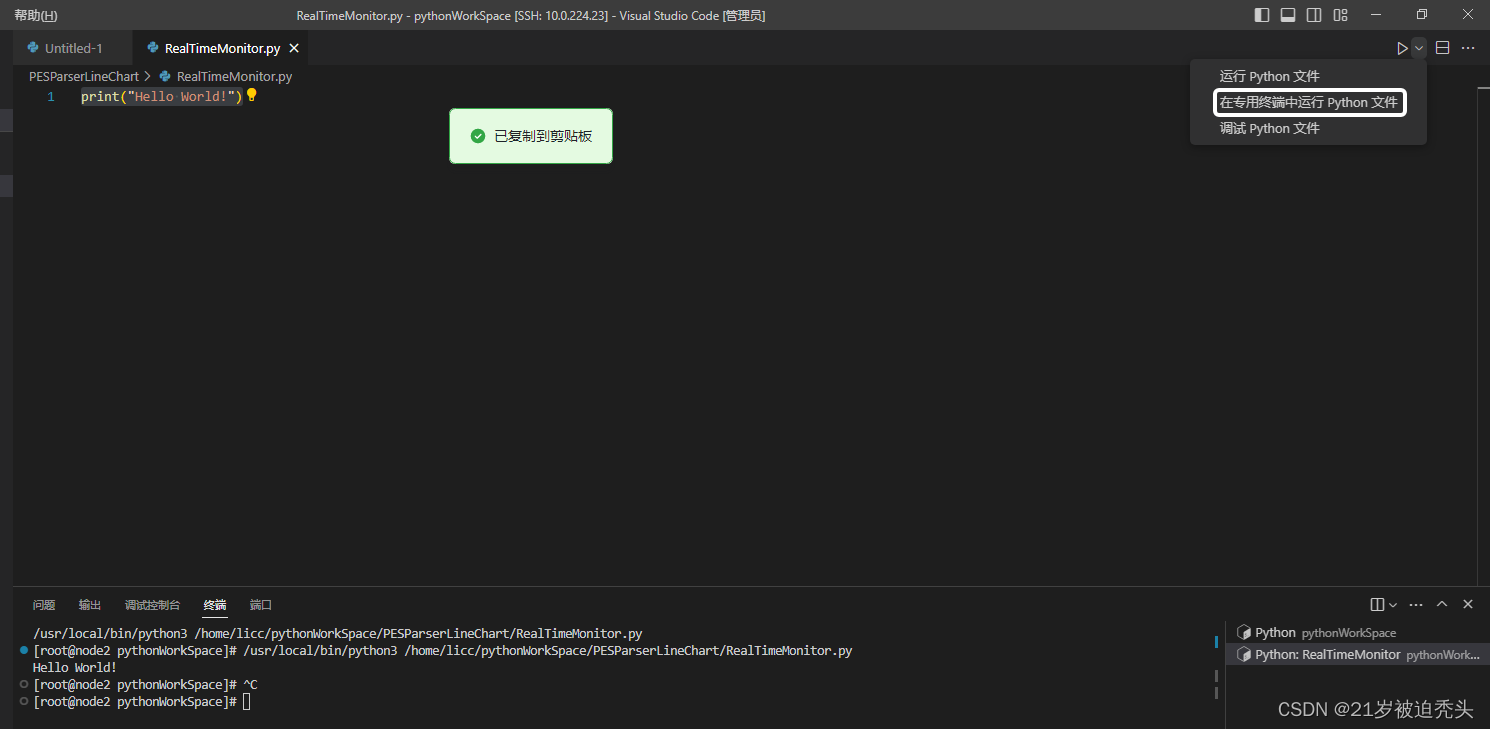
Linux下使用vscode编写Python项目
我此处是使用VScode远程连接的服务器,具体方法可看如下: 1、vscode中安装Python插件 按上面步骤安装好Python插件后,重启vscode; 2、选择Python解释器 创建Python项目结构: 按下F1,打开vscode命令栏&am…...
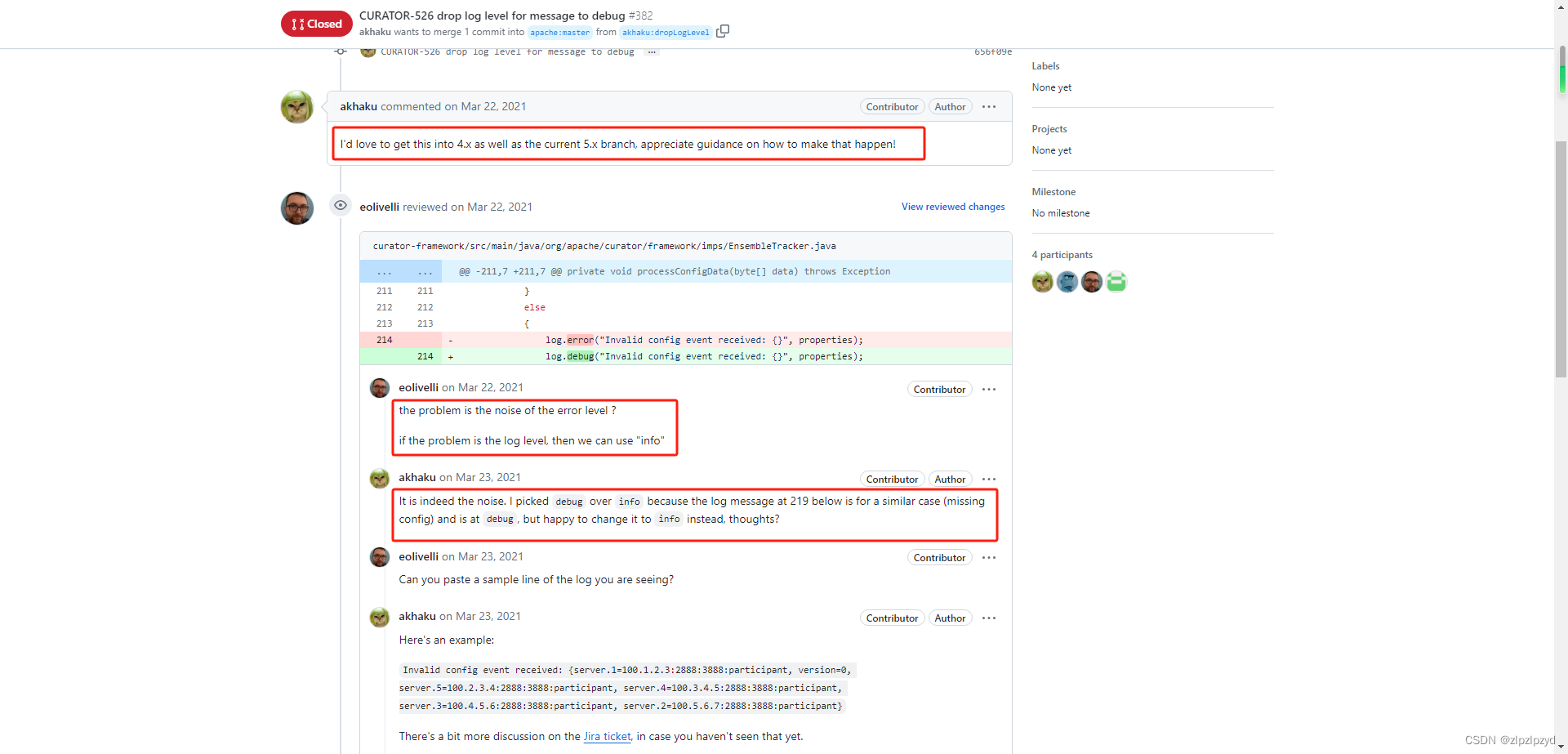
使用 curator 连接 zookeeper 集群 Invalid config event received
dubbo整合zookeeper 如图,错误日志 2023-11-04 21:16:18.699 ERROR 7459 [main-EventThread] org.apache.curator.framework.imps.EnsembleTracker Caller0 at org.apache.curator.framework.imps.EnsembleTracker.processConfigData(EnsembleTracker.java…...
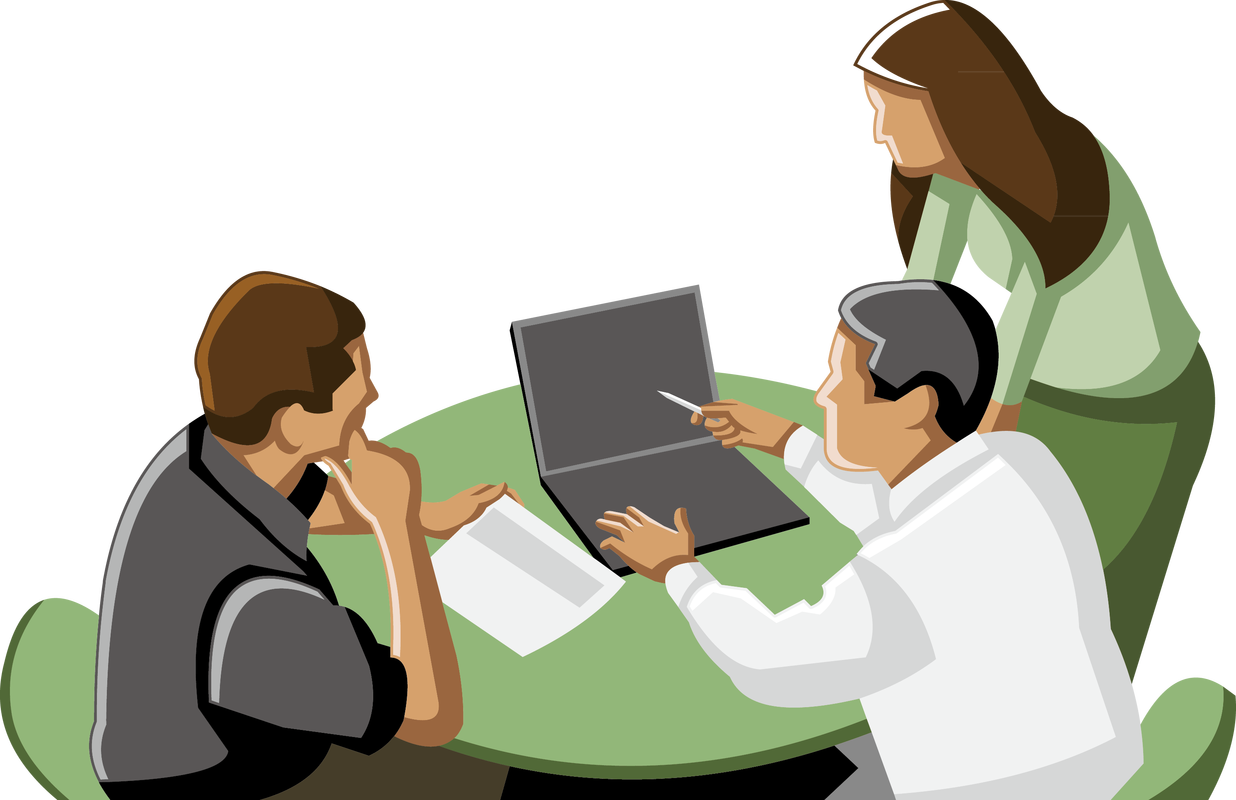
大促期间也要做好低价治理
低价链接无处不在,对于品牌来说,日常治理低价是常规操作,那面对价格变化更快、促销信息更丰富的大促,对低价的治理要求会更高。否则容易被未授权在大促双十一、六一八期间分食流量。 力维网络有专业的团队为品牌提供低价治理服务&…...
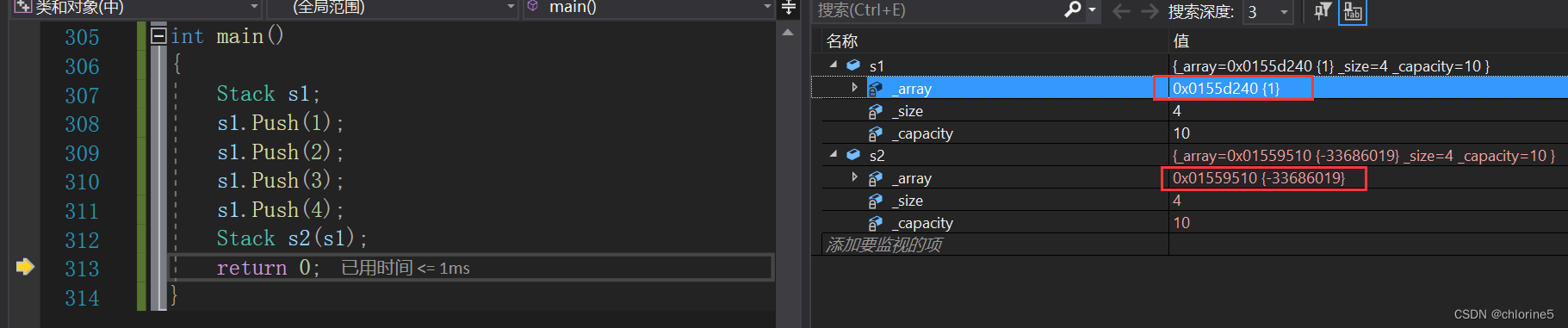
【c++】——类和对象(中)——默认成员函数(上)
【学习目标】 1. 类的6个默认成员函数 2. 构造函数 3. 析构函数 4. 拷贝构造函数 目录 一.类的6个默认成员函数 二. 构造函数 2.1 概念 2.2.特性 三.析构函数 3.1.概念 3.2 特性 四.拷贝构造函数 4.1.概念 4.2.特性 一.类的6个默认成员函数 如果一个类中什么成员…...

钉钉企业微应用开发C#-HTTP回调接口
官方的STREAM回调推送的方式,试了几次都认证不过,就放弃了还是用HTTP的模式吧。 /// <summary>/// 应用回调/// </summary>/// <param name"model"></param>/// <returns></returns>public static Dictio…...
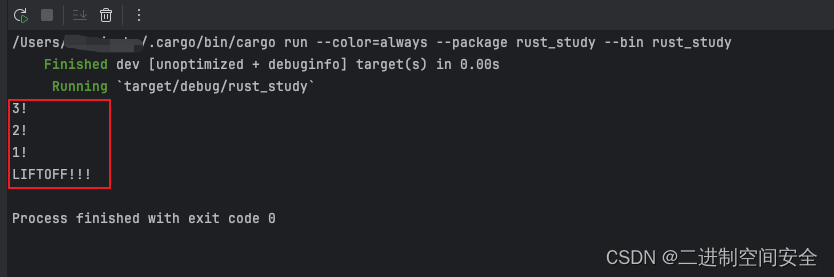
Rust编程基础之条件表达式和循环
1.if表达式 if 表达式允许根据条件执行不同的代码分支, 以下代码是一个典型的使用if表达式的例子: fn main() {let number 3; if number < 5 {println!("condition was true");} else {println!("condition was false");} } 所有的 if 表达式都以…...

MATLAB算法实战应用案例精讲-【人工智能】ROS机器人(补充篇)
目录 前言 ROS 机器人导航调参 1 速度和加速度 2 全局路径规划 3 局部路径规划...
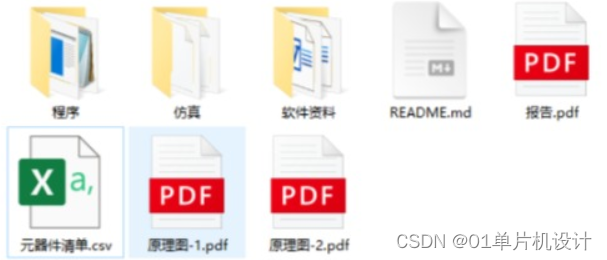
基于8086汽车智能小车控制系统
**单片机设计介绍,基于8086汽车智能小车控制系统 文章目录 一 概要二、功能设计设计思路 三、 软件设计原理图 五、 程序六、 文章目录 一 概要 基于 8086 的汽车智能小车控制系统是一种将微处理器技术应用于汽车控制的系统。下面是其主要的设计介绍: 硬…...
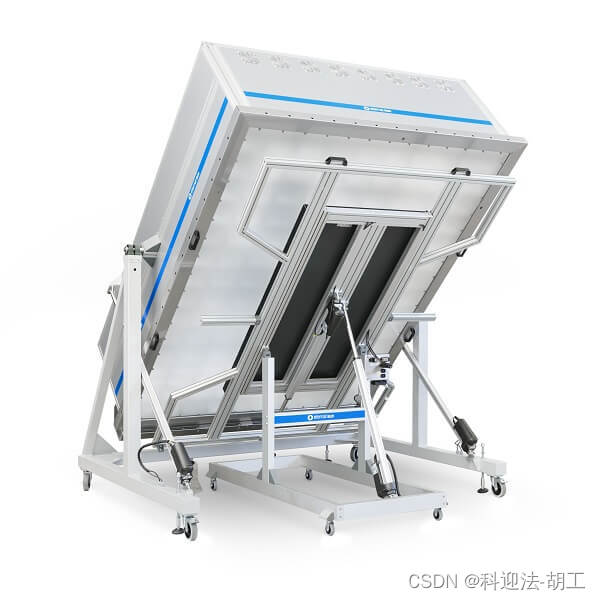
全光谱大面积氙光灯太阳光模拟器老化测试
氙灯光源太阳光模拟器广泛应用于光解水产氢、光化学催化、二氧化碳制甲醇、光化学合成、光降解污染物、 水污染处理、生物光照,光学检测、太阳能电池研究、荧光材料测试(透射、反射、吸收) 太阳能电池特性测试,光热转化,光电材料特性测试,生物…...

linux添加一条到中间路由器的路由
有时候需要配置一些明细路由,不能直接通过网关进行路由转发 配置示例 ip route add 10.0.12.0/24 via 10.0.41.1 dev bond0 这个命令是用于在Linux操作系统上配置IP路由的命令。具体来说,这个命令的含义是: ip route add: 这部分表示要添加…...

不同MySQL服务的表以及库的数据迁移(/备份)
目标: 将本地主机上usernameroot,passwordroot,port3307的MySQL服务中migration_one数据库的table_11数据表导出到本地的D:\start_java\XinQiUtilsOrDemo\testMigrationMySQL\table_11.bak注意:目前D:\start_java\XinQiUtilsOrDemo\testMigrationMySQL该…...
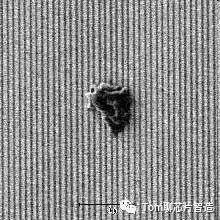
聊聊芯片超净间的颗粒(particle)
在芯片制造领域,颗粒的存在可能对生产过程产生巨大影响。其中,每个微小的颗粒,无论是来自人员、设备,还是自然环境,都有可能在制程中引发故障,从而对产品性能产生负面影响。这就是为什么在芯片厂中…...
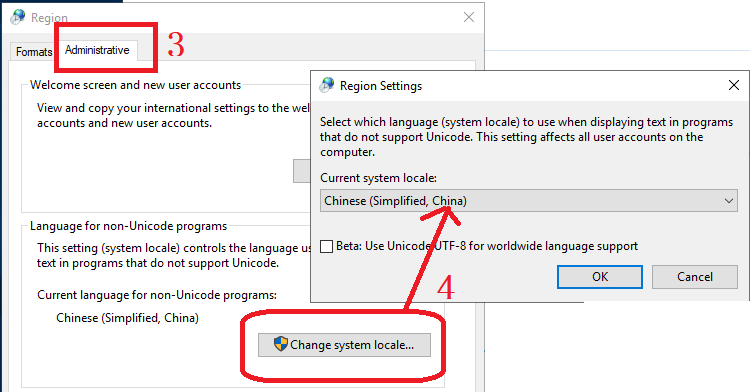
服务器(windows Server 2019为例)中的日志中文乱码的解决办法
1. 首先,打开控制面板,找到区域(Region),把Format设置为国语简体中文,点击高级(Administrative)后设置Current system locale为国语简体中文,按照图中步骤:...
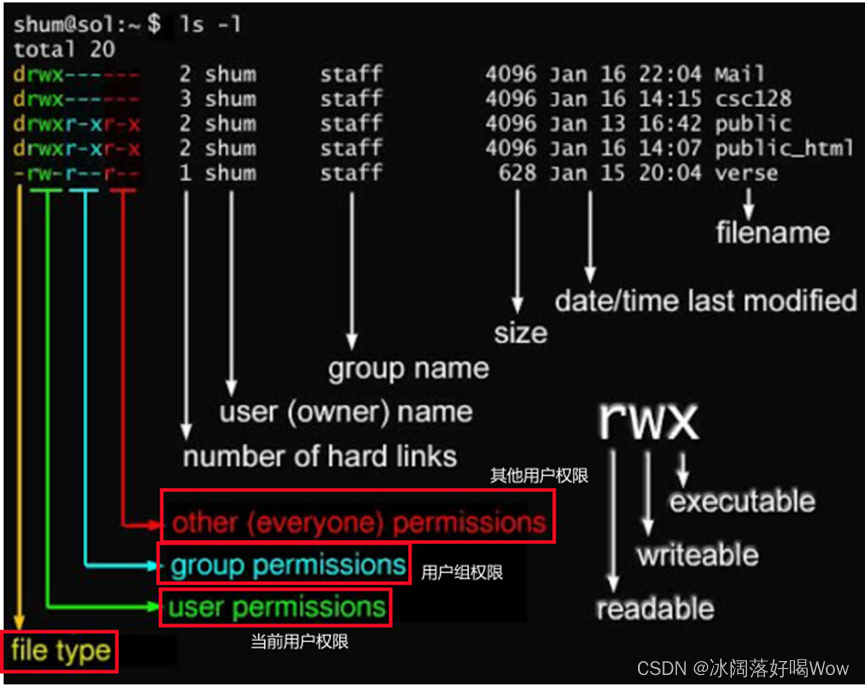
Linux 学习(CentOS 7)
CentOS 7 学习 Linux系统内核作者: Linux内核版本 内核(kernel)是系统的心脏,是运行程序和管理像磁盘和打印机等硬件设备的核心程序,它提供了一个在裸设备与应用程序间的抽象层。 Linux内核版本又分为稳定版和开发版,两种版本是相互关联&am…...

架构决策记录 ADR
在项目和产品开发过程中,软件工程团队需要做出架构决策以实现其目标。这些决策可以是技术性的,也可以与流程相关。 技术决策:例如决定使用JBOSS Data Grid作为缓存解决方案还是选择Amazon Elasticache,或者决定使用AWS Network L…...
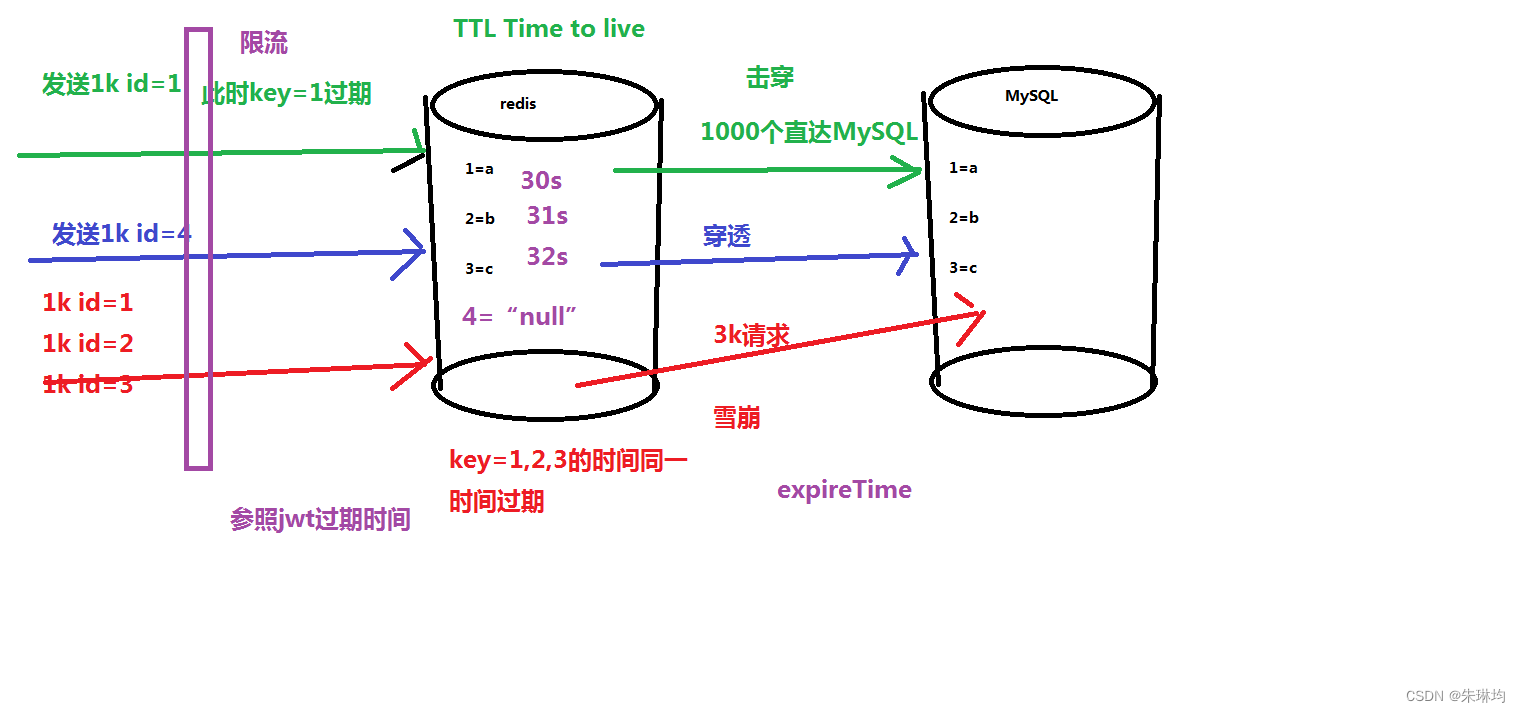
SSM之spring注解式缓存redis->redis整合,redis的注解式开发及应用场景,redis的击穿穿透雪崩
redis整合redis的注解式开发及应用场景redis的击穿穿透雪崩 1.redis整合 mysql整合 pom配置; String-fmybatis.xml --> mybatis.cfg.xml: 包扫描; 注册了一个jdbc.properties(url/password/username/...); 配置数据源(数据库连…...

数据库性能优化(查询优化、索引优化、负载均衡、硬件升级等方面)
数据库性能优化是提升数据库系统整体性能和响应速度的一系列技术和策略。它可以通过多种方式来实现,包括优化查询语句、索引设计、硬件升级、负载均衡等手段。 合适的数据模型设计 正确的数据模型设计是性能优化的基石。合理的表结构和关系设计可以减少冗余数据&…...
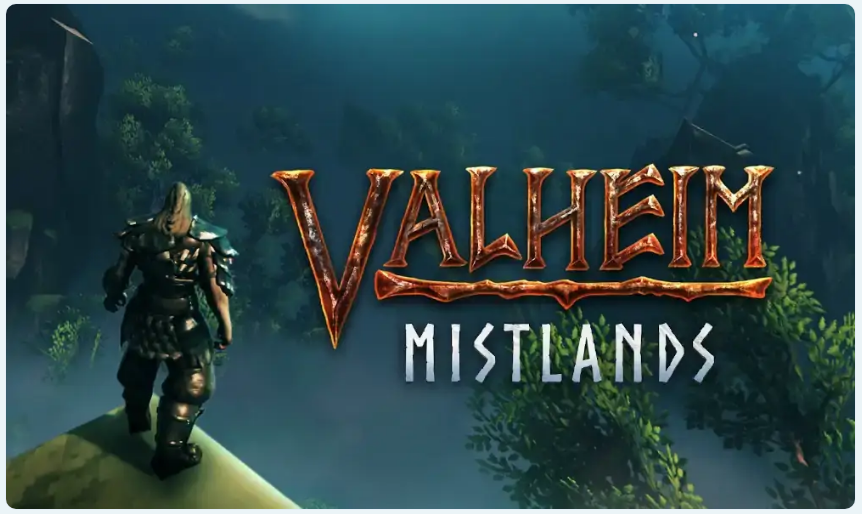
谁说 Linux 不能玩游戏?
在上个世纪最早推出视频游戏的例子是托马斯戈德史密斯(Thomas T. Goldsmith Jr.)于1947年开发的“「Cathode Ray Tube Amusement Device」”,它已经显着发展,并且已成为人类生活中必不可少的一部分。 通过美国游戏行业的统计数据&…...
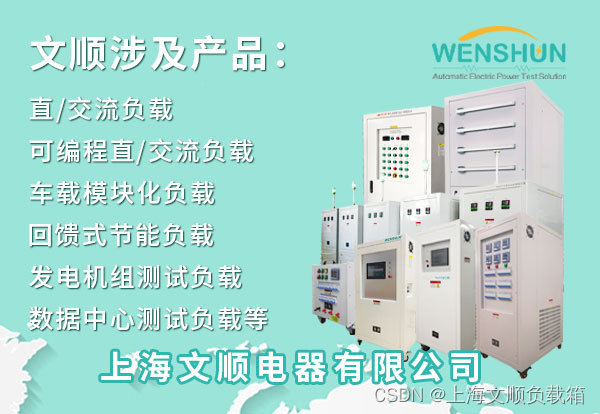
发电机负载测试方案
发电机负载测试是为了评估发电机在不同负载条件下的性能和稳定性。下面是一个可能的发电机负载测试方案: 测试前准备: - 确定测试的负载范围和条件,包括负载大小、负载类型(如电阻性、感性或容性负载)、负载持续时间等…...

Flask三种文件下载方法
Flask 是一个流行的 Python Web 框架,它提供了多种方法来实现文件下载。在本文中,我们将介绍三种不同的方法,以便你能够选择最适合你应用程序的方法。 方法一:使用 send_file 函数 send_file 函数是 Flask 中最常用的文件下载方法…...