postman断言脚本(2)
https://learning.postman.com/docs/writing-scripts/script-references/test-examples/#parsing-response-body-data
状态码
pm.test("Status code is 200",function(){pm.response.to.have.status(200);});
pm.test("Status code is 200",()=>{pm.expect(pm.response.code).to.eql(200);});
多重断言
pm.test("The response has all properties",()=>{//parse the response JSON and test three propertiesconst responseJson = pm.response.json();pm.expect(responseJson.type).to.eql('vip');pm.expect(responseJson.name).to.be.a('string');pm.expect(responseJson.id).to.have.lengthOf(1);});
解析response body
To parse JSON data, use the following syntax:
const responseJson = pm.response.json();
To parse XML, use the following:
const responseJson =xml2Json(pm.response.text());
If you're dealing with complex XML responses you may find console logging useful.
To parse CSV, use the CSV parse utility:
const parse =require('csv-parse/lib/sync');const responseJson =parse(pm.response.text());
To parse HTML, use cheerio:
const $ = cheerio.load(pm.response.text());//output the html for testing
console.log($.html());
If you can't parse the response body to JavaScript because it's not formatted as JSON, XML, HTML, CSV, or any other parsable data format, you can still make assertions on the data.
Test if the response body contains a string:
pm.test("Body contains string",()=>{pm.expect(pm.response.text()).to.include("customer_id");});
This doesn't tell you where the string was encountered because it carries out the test on the whole response body. Test if a response matches a string (which will typically only be effective with short responses):
pm.test("Body is string",function(){pm.response.to.have.body("whole-body-text");});
断言 HTTP response
Your tests can check various aspects of a request response, including the body, status codes, headers, cookies, response times, and more.
响应体
Check for particular values in the response body:
pm.test("Person is Jane",()=>{const responseJson = pm.response.json();pm.expect(responseJson.name).to.eql("Jane");pm.expect(responseJson.age).to.eql(23);});
状态码
Test for the response status code:
pm.test("Status code is 201",()=>{pm.response.to.have.status(201);});
If you want to test for the status code being one of a set, include them all in an array and use oneOf:
pm.test("Successful POST request",()=>{pm.expect(pm.response.code).to.be.oneOf([201,202]);});
Check the status code text:
pm.test("Status code name has string",()=>{pm.response.to.have.status("Created");});
响应头
Check that a response header is present:
pm.test("Content-Type header is present",()=>{pm.response.to.have.header("Content-Type");});
Test for a response header having a particular value:
pm.test("Content-Type header is application/json",()=>{pm.expect(pm.response.headers.get('Content-Type')).to.eql('application/json');});
cookie
Test if a cookie is present in the response:
pm.test("Cookie JSESSIONID is present",()=>{pm.expect(pm.cookies.has('JSESSIONID')).to.be.true;});
Test for a particular cookie value:
pm.test("Cookie isLoggedIn has value 1",()=>{pm.expect(pm.cookies.get('isLoggedIn')).to.eql('1');});
响应时间
Test for the response time to be within a specified range:
pm.test("Response time is less than 200ms",()=>{pm.expect(pm.response.responseTime).to.be.below(200);});
一般性断言
断言value type
/* response has this structure:
{"name": "Jane","age": 29,"hobbies": ["skating","painting"],"email": null
}
*/const jsonData = pm.response.json();
pm.test("Test data type of the response",()=>{pm.expect(jsonData).to.be.an("object");pm.expect(jsonData.name).to.be.a("string");pm.expect(jsonData.age).to.be.a("number");pm.expect(jsonData.hobbies).to.be.an("array");pm.expect(jsonData.website).to.be.undefined;pm.expect(jsonData.email).to.be.null;});
断言数组属性
Check if an array is empty, and if it contains particular items:
/*
response has this structure:
{"errors": [],"areas": [ "goods", "services" ],"settings": [{"type": "notification","detail": [ "email", "sms" ]},{"type": "visual","detail": [ "light", "large" ]}]
}
*/const jsonData = pm.response.json();
pm.test("Test array properties",()=>{//errors array is emptypm.expect(jsonData.errors).to.be.empty;//areas includes "goods"pm.expect(jsonData.areas).to.include("goods");//get the notification settings
objectconst notificationSettings = jsonData.settings.find(m=> m.type ==="notification");pm.expect(notificationSettings).to.be.an("object","Could not find the setting");//detail array must include "sms"pm.expect(notificationSettings.detail).to.include("sms");//detail array must include all listedpm.expect(notificationSettings.detail).to.have.members(["email","sms"]);});
断言object属性
Assert that an object contains keys or properties:
pm.expect({a:1,b:2}).to.have.all.keys('a','b');
pm.expect({a:1,b:2}).to.have.any.keys('a','b');
pm.expect({a:1,b:2}).to.not.have.any.keys('c','d');
pm.expect({a:1}).to.have.property('a');
pm.expect({a:1,b:2}).to.be.an('object').that.has.all.keys('a','b');
Target can be an object, set, array or map. If .keys is run without .all or .any, the expression defaults to .all. As .keys behavior varies based on the target type, it's recommended to check the type before using .keys with .a.
断言值在集合里
check a response value against a list of valid options:
pm.test("Value is in valid list",()=>{pm.expect(pm.response.json().type).to.be.oneOf(["Subscriber","Customer","User"]);});
断言包含object
Check that an object is part of a parent object:
/*
response has the following structure:
{"id": "d8893057-3e91-4cdd-a36f-a0af460b6373","created": true,"errors": []
}
*/pm.test("Object is contained",()=>{const expectedObject ={"created":true,"errors":[]};pm.expect(pm.response.json()).to.deep.include(expectedObject);});
Using .deep causes all .equal, .include, .members, .keys, and .property assertions that follow in the chain to use deep equality (loose equality) instead of strict (===) equality. While the .eql also compares loosely, .deep.equal causes deep equality comparisons to also be used for any other assertions that follow in the chain, while .eql doesn't.
验证响应结构
Carry out JSON schema validation with Tiny Validator V4 (tv4):
const schema ={"items":{"type":"boolean"}};
const data1 =[true,false];
const data2 =[true,123];pm.test('Schema is valid',function(){pm.expect(tv4.validate(data1, schema)).to.be.true;pm.expect(tv4.validate(data2, schema)).to.be.true;});
Validate JSON schema with the Ajv JSON schema validator:
const schema ={"properties":{"alpha":{"type":"boolean"}}};
pm.test('Schema is valid',function(){pm.response.to.have.jsonSchema(schema);});
发送异步请求
Send a request from your test code and log the response.
pm.sendRequest("https://postman-echo.com/get",function(err, response){console.log(response.json());});
相关文章:

postman断言脚本(2)
https://learning.postman.com/docs/writing-scripts/script-references/test-examples/#parsing-response-body-data状态码pm.test("Status code is 200",function(){pm.response.to.have.status(200);});pm.test("Status code is 200",()>{pm.expect(…...
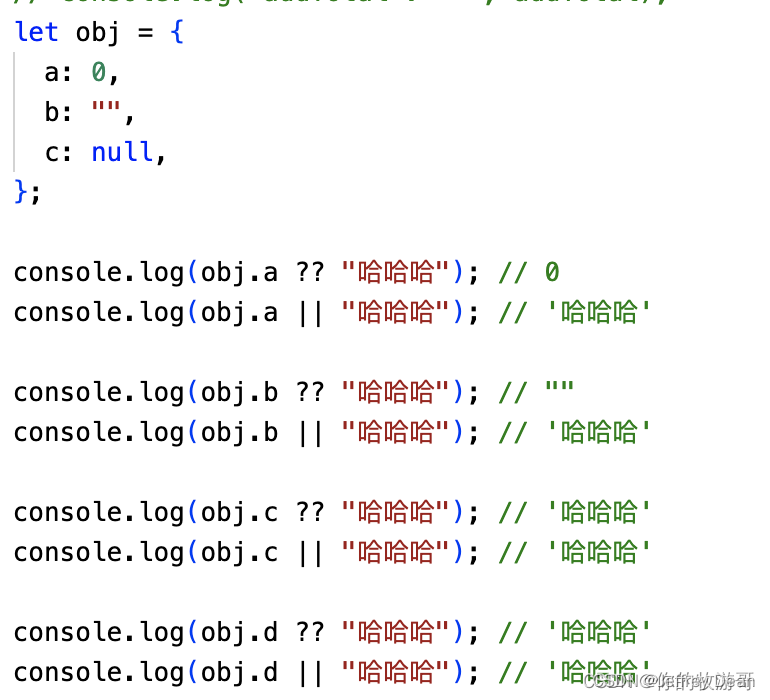
js中?.、??的具体用法
1、?. (可选链运算符) 在javascript中如果一个值为null、undefined,直接访问下面的属性, 会报 Uncaught TypeError: Cannot read properties of undefined 异常错误。 而在真实的项目中是会出现这种情况,有这个值就…...
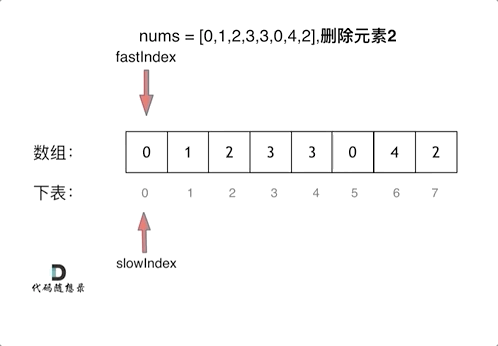
刷题笔记1 | 704. 二分查找,27. 移除元素
704. 二分查找 给定一个 n 个元素有序的(升序)整型数组 nums 和一个目标值 target ,写一个函数搜索 nums 中的 target,如果目标值存在返回下标,否则返回 -1。 输入: nums [-1,0,3,5,9,12], target 9 输出: 4 …...
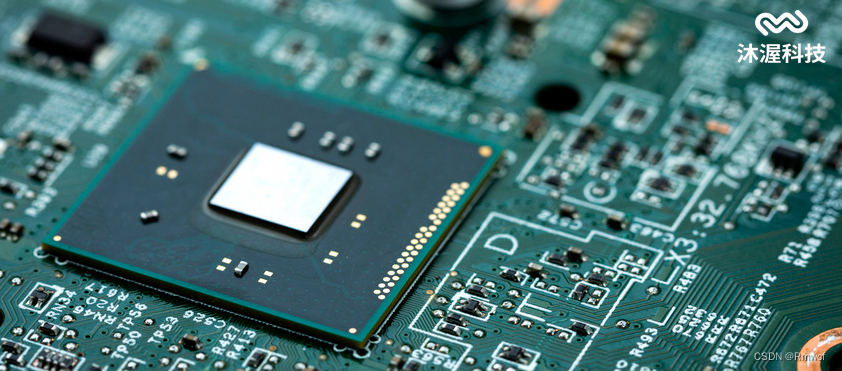
柔性电路板的优点、分类和发展方向
柔性电路板是pcb电路板的一种,又称为软板、柔性印刷电路板,主要是由柔性基材制作而成的一种具有高可靠性、高可挠性的印刷电路板,具有厚度薄、可弯曲、配线密度高、重量轻、灵活度高等特点,主要用在手机、电脑、数码相机、家用电器…...
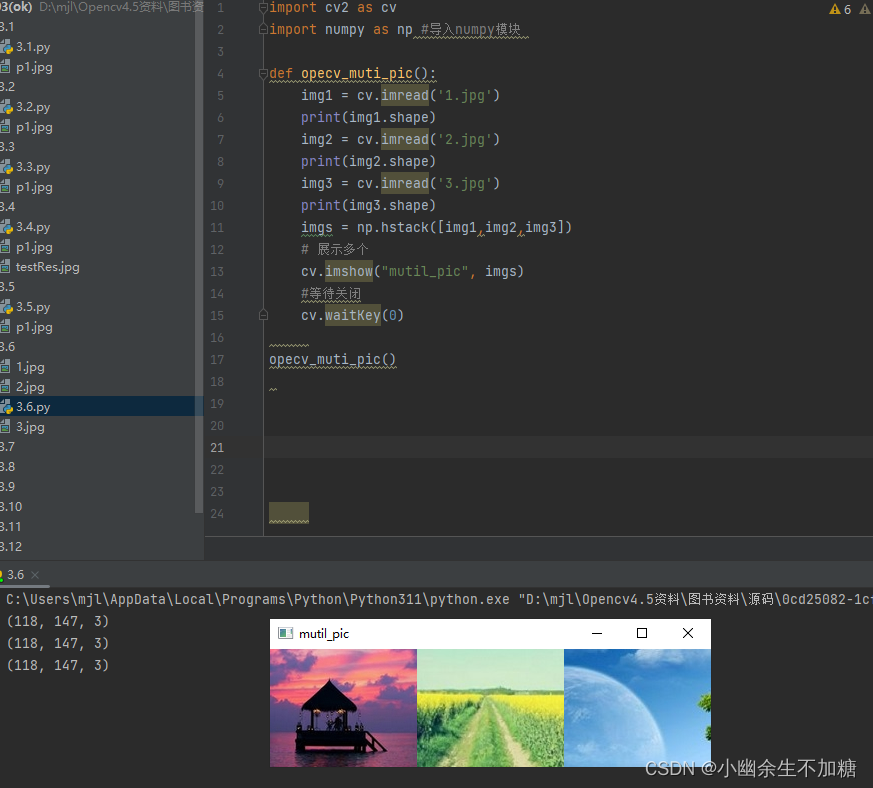
OpenCV入门(二)快速学会OpenCV1图像基本操作
OpenCV入门(一)快速学会OpenCV1图像基本操作 不讲大道理,直接上干货。操作起来。 众所周知,OpenCV 是一个跨平台的计算机视觉库, 支持多语言, 功能强大。今天就从读取图片,显示图片,输出图片信息和简单的…...
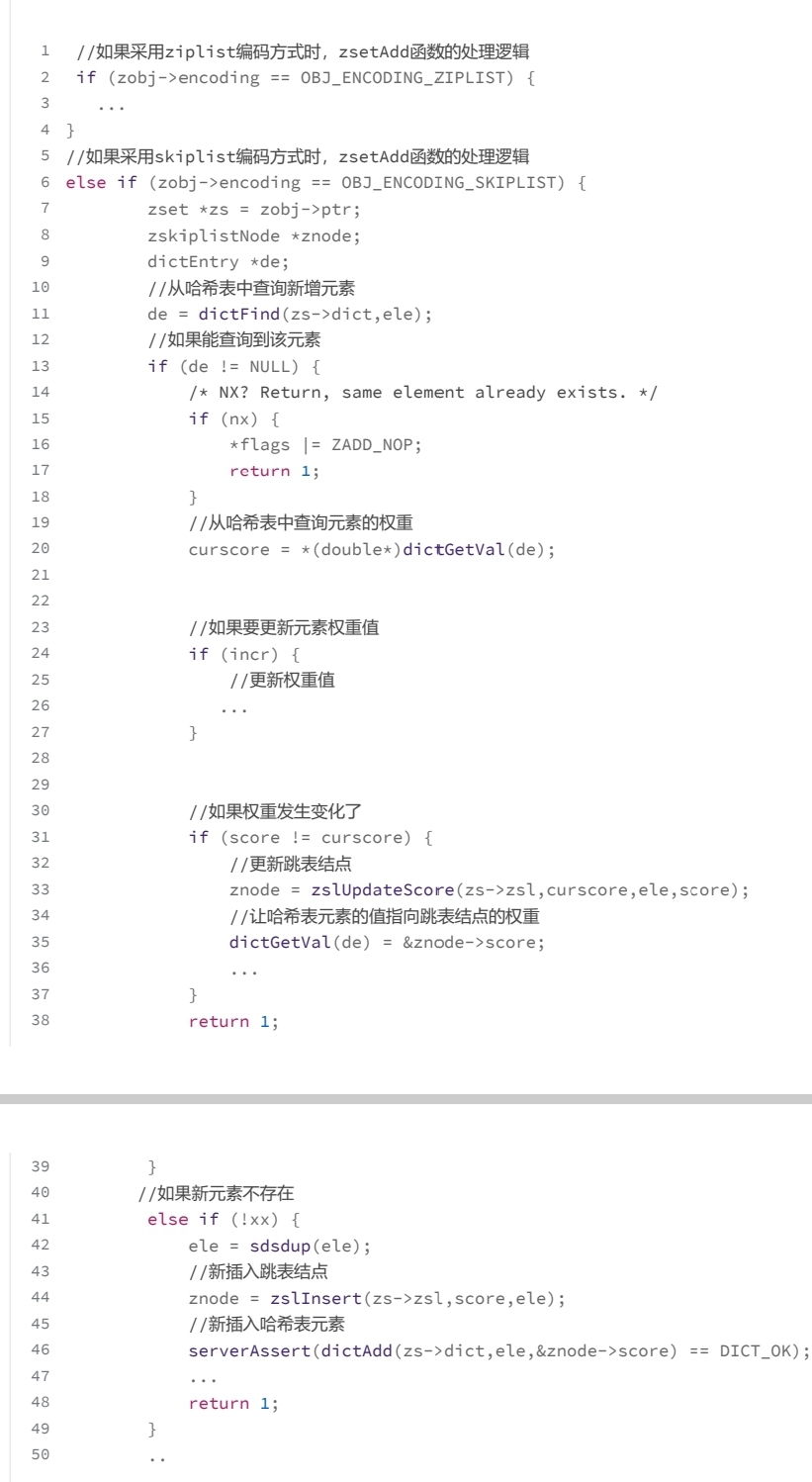
Redis源码---有序集合为何能同时支持点查询和范围查询
目录 前言 Sorted Set 基本结构 跳表的设计与实现 跳表数据结构 跳表结点查询 跳表结点层数设置 哈希表和跳表的组合使用 前言 有序集合(Sorted Set)是 Redis 中一种重要的数据类型,它本身是集合类型,同时也可以支持集合中…...
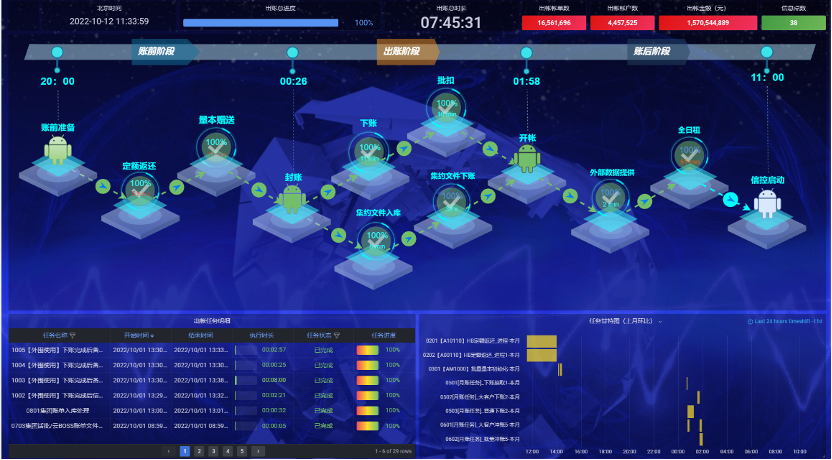
从计费出账加速的设计谈周期性业务的优化思考
1号恐惧症 你有没有这样的做IT的朋友?年纪轻轻,就头发花白或者秃顶,然后每个月周期性的精神不振,一到月底,就有明显的焦虑。如果有,他可能就是运营商行业做计费运营的,请对他好点,特…...

垃圾回收的概念与算法(第四章)
《实战Java虚拟机:JVM故障诊断与性能优化 (第2版)》 第4章 垃圾回收的概念与算法 目标: 了解什么是垃圾回收学习几种常用的垃圾回收算法掌握可触及性的概念理解 Stop-The-World(STW) 4.1. 认识垃圾回收 - 内存管理清洁工 垃圾…...
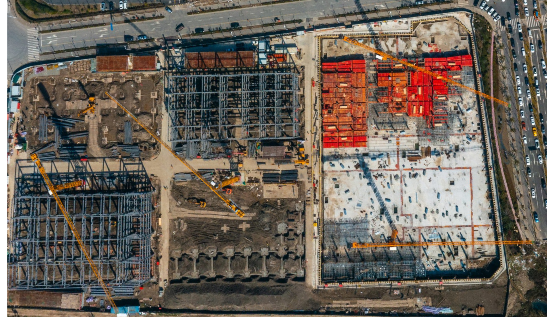
让您的客户了解您的制造过程“VR云看厂实时数字化展示”
一、工厂云考察,成为市场热点虚拟现实(VR)全景技术问世已久,但由于应用范围较为狭窄,一直未得到广泛应用。国外客户无法亲自到访,从而导致考察难、产品取样难等问题,特别是对于大型制造企业来说…...
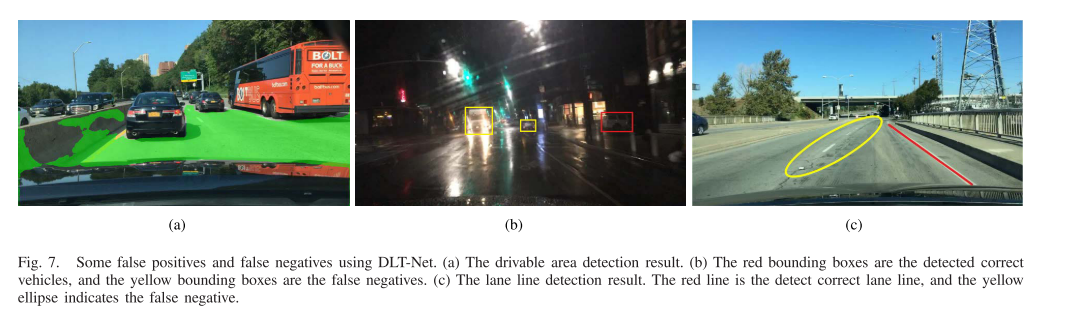
CV——day80 读论文:DLT-Net:可行驶区域、车道线和交通对象的联合检测
DLT-Net:可行驶区域、车道线和交通对象的联合检测I. INTRODUCTIONII. ANALYSIS OF PERCEPTIONIV. DLT-NETA. EncoderB. Decoder1) Drivable Area Branch(可行驶区域分支)2) Context Tensor(上下文张量)3) Lane Line Branch(车道线分支)4) Traffic Object Branch(目标检测对象分…...
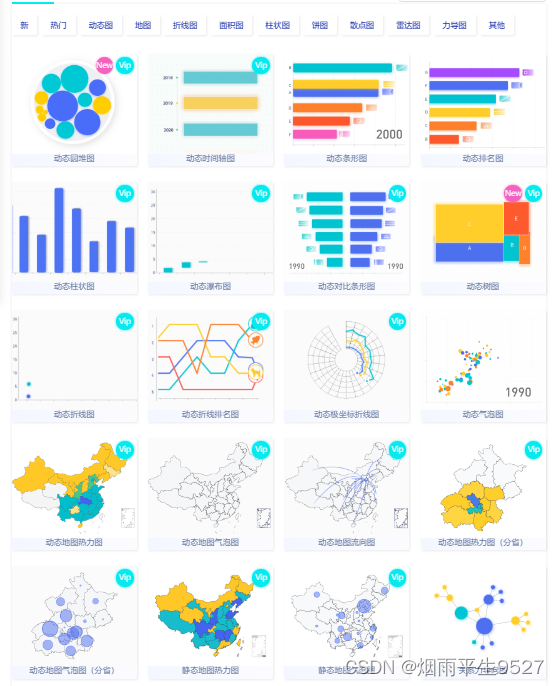
工具篇4.5数据可视化工具大全
1.1 Flourish 数据可视化不仅是一项技术,也是一门艺术。当然,数据可视化的工具也非常多,仅 Python 就有 matplotlib、plotly、seaborn、bokeh 等多种可视化库,我们可以根据自己的需要进行选择。但不是所有的人都擅长写代码完成数…...

京东前端二面常考手写面试题(必备)
实现发布-订阅模式 class EventCenter{// 1. 定义事件容器,用来装事件数组let handlers {}// 2. 添加事件方法,参数:事件名 事件方法addEventListener(type, handler) {// 创建新数组容器if (!this.handlers[type]) {this.handlers[type] …...
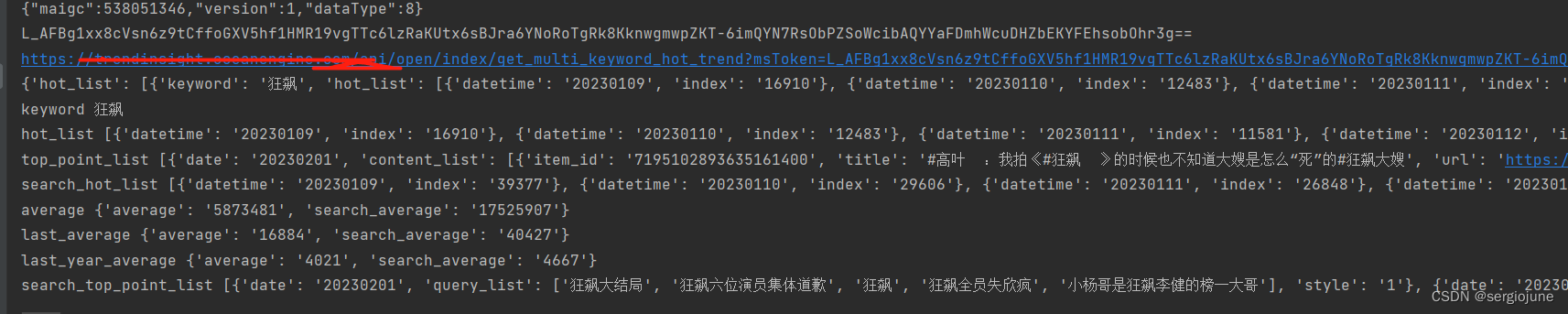
如何用AST还原某音的JSVMP
1. 什么是JSVMP vmp简单来说就是将一些高级语言的代码通过自己实现的编译器进行编译得到字节码,这样就可以更有效的保护原有代码,而jsvmp自然就是对JS代码的编译保护,具体的可以看看H5应用加固防破解-JS虚拟机保护方案。 如何区分是不是jsv…...
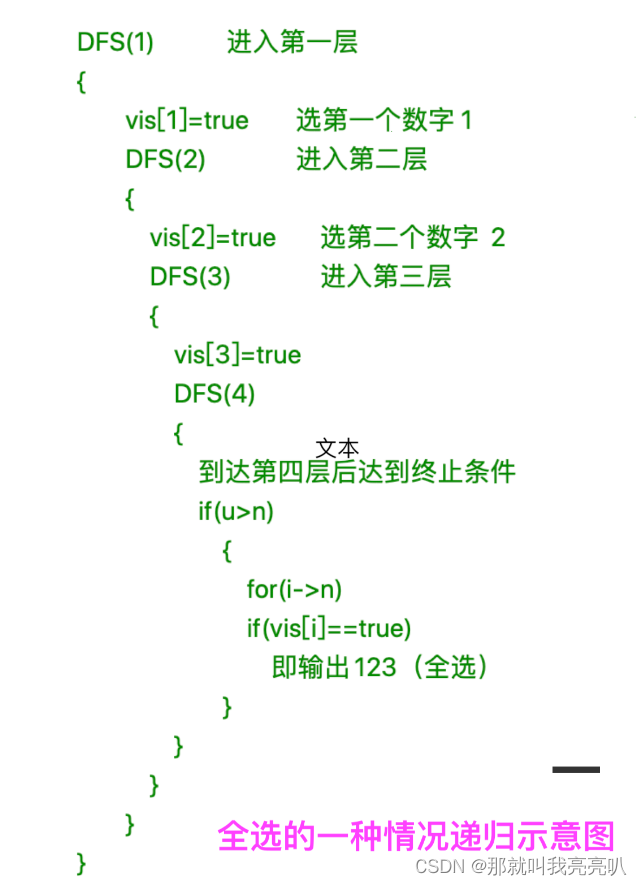
【蓝桥杯试题】 递归实现指数型枚举例题
💃🏼 本人简介:男 👶🏼 年龄:18 🤞 作者:那就叫我亮亮叭 📕 专栏:蓝桥杯试题 文章目录1. 题目描述2. 思路解释2.1 时间复杂度2.2 递归3. 代码展示最后&#x…...
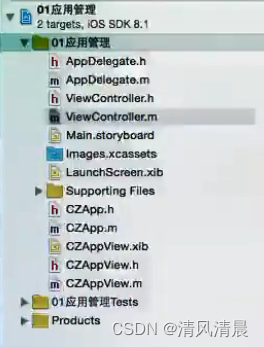
【用Group整理目录结构 Objective-C语言】
一、接下来,我们看另外一个知识点,怎么用Group把这一堆乱七八糟的文件给它整理一下,也算是封装一下吧, 1.这一堆杂乱无章的文件: 那么,哪些类是属于模型呢,哪些类是属于视图呢,哪些类是属于控制器呢, 我们接下来通过Group的方式,来给它们分一下类, 这样看起来就好…...
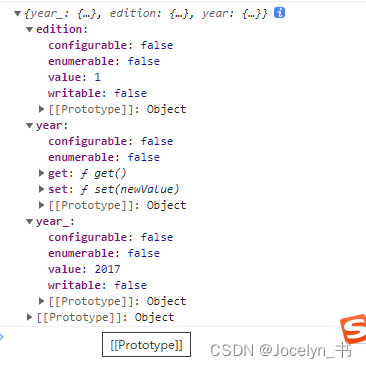
JavaScript高级程序设计读书分享之8章——8.1理解对象
JavaScript高级程序设计(第4版)读书分享笔记记录 适用于刚入门前端的同志 创建自定义对象的通常方式是创建 Object 的一个新实例,然后再给它添加属性和方法。 let person new Object() person.name Tom person.age 18 person.sayName function(){//示 this.name…...
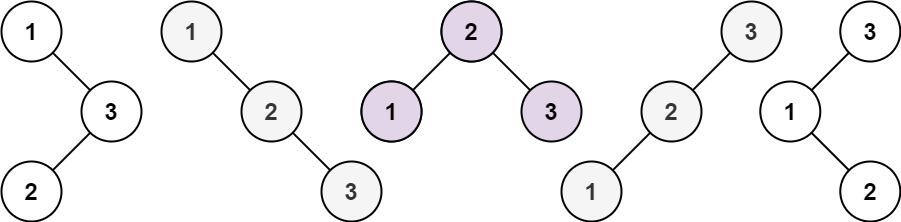
代码随想录算法训练营第四十天 | 343. 整数拆分,96.不同的二叉搜索树
一、参考资料整数拆分https://programmercarl.com/0343.%E6%95%B4%E6%95%B0%E6%8B%86%E5%88%86.html 视频讲解:https://www.bilibili.com/video/BV1Mg411q7YJ不同的二叉搜索树https://programmercarl.com/0096.%E4%B8%8D%E5%90%8C%E7%9A%84%E4%BA%8C%E5%8F%89%E6%90…...
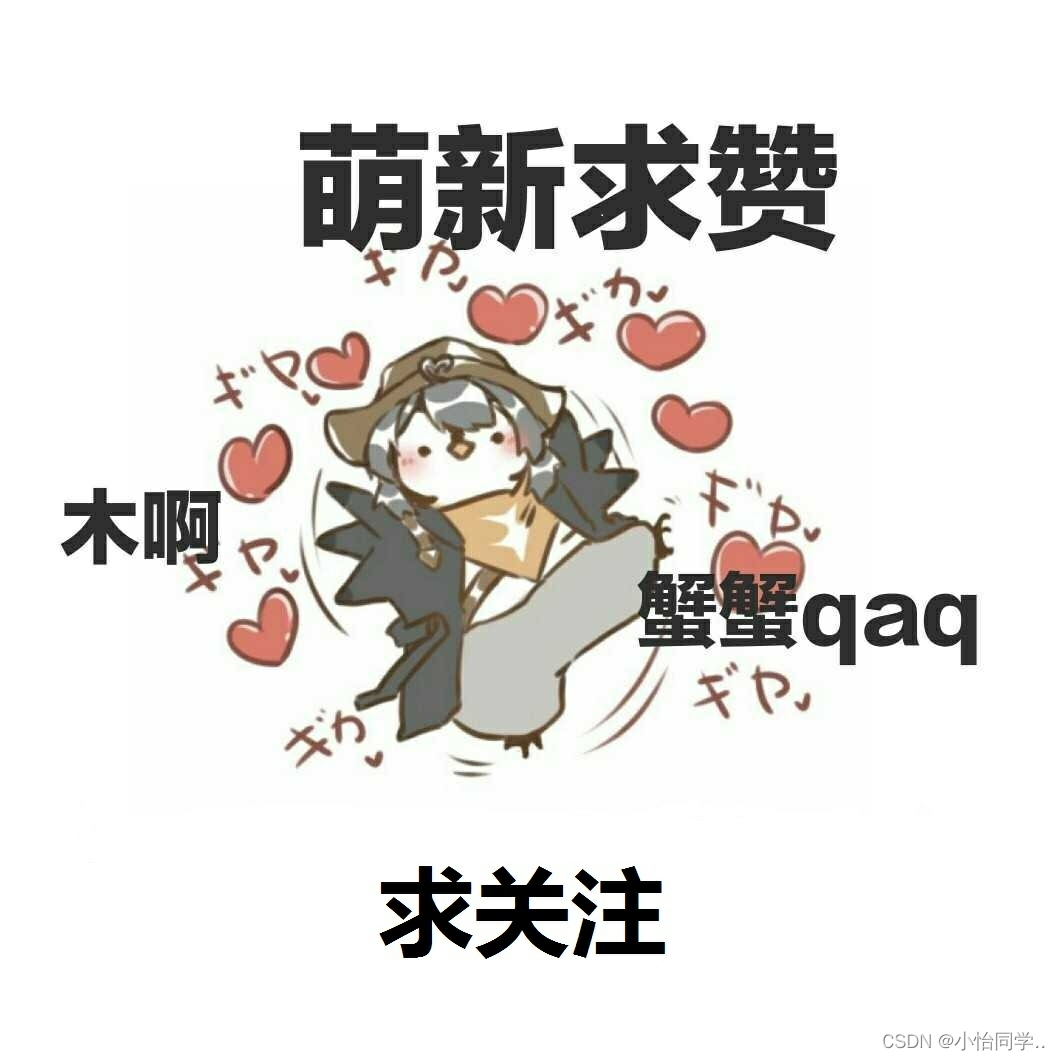
数据结构与算法系列之顺序表的实现
这里写目录标题顺序表的优缺点:注意事项test.c(动态顺序表)SeqList.hSeqList.c各接口函数功能详解void SLInit(SL* ps);//定义void SLDestory(SL* ps);void SLPrint(SL* ps);void SLPushBack(SL* ps ,SLDataType * x );void SLPopBack(SL* ps…...
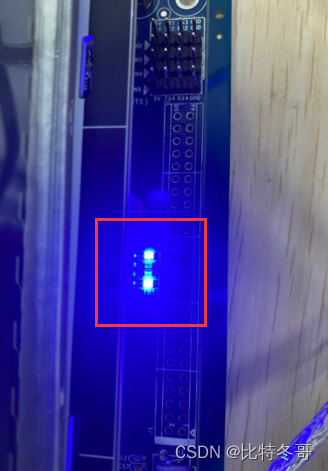
基于Linux_ARM板的驱动烧写及连接、挂载详细过程(附带驱动程序)
文章目录前言一、搭建nfs服务二、ARM板的硬件连接三、putty连接四、挂载共享文件夹五、烧写驱动程序六、驱动程序示例前言 本文操作环境:Ubuntu14.04、GEC6818 这里为似懂非懂的朋友简单叙述该文章的具体操作由来,我们的主要目的是将写好的驱动程序烧进…...

python-爬虫-字体加密
直接点 某8网 https://*****.b*b.h*****y*8*.com/ 具体网址格式就是这样的但是为了安全起见,我就这样打码了. 抛出问题 我们看到这个号码是在页面上正常显示的 F12 又是这样就比较麻烦,不能直接获取.用requests库也是获取不到正常想要的 源码的,因为字体加密了. 查看页面源代码…...
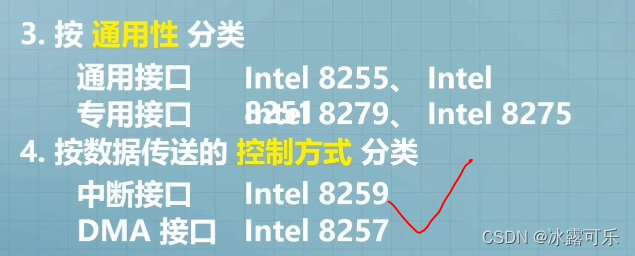
计算机组成原理4小时速成5:输入输出系统,io设备与cpu的链接方式,控制方式,io设备,io接口,并行串行总线
计算机组成原理4小时速成5:输入输出系统,io设备与cpu的链接方式,控制方式,io设备,io接口,并行串行总线 2022找工作是学历、能力和运气的超强结合体,遇到寒冬,大厂不招人,…...
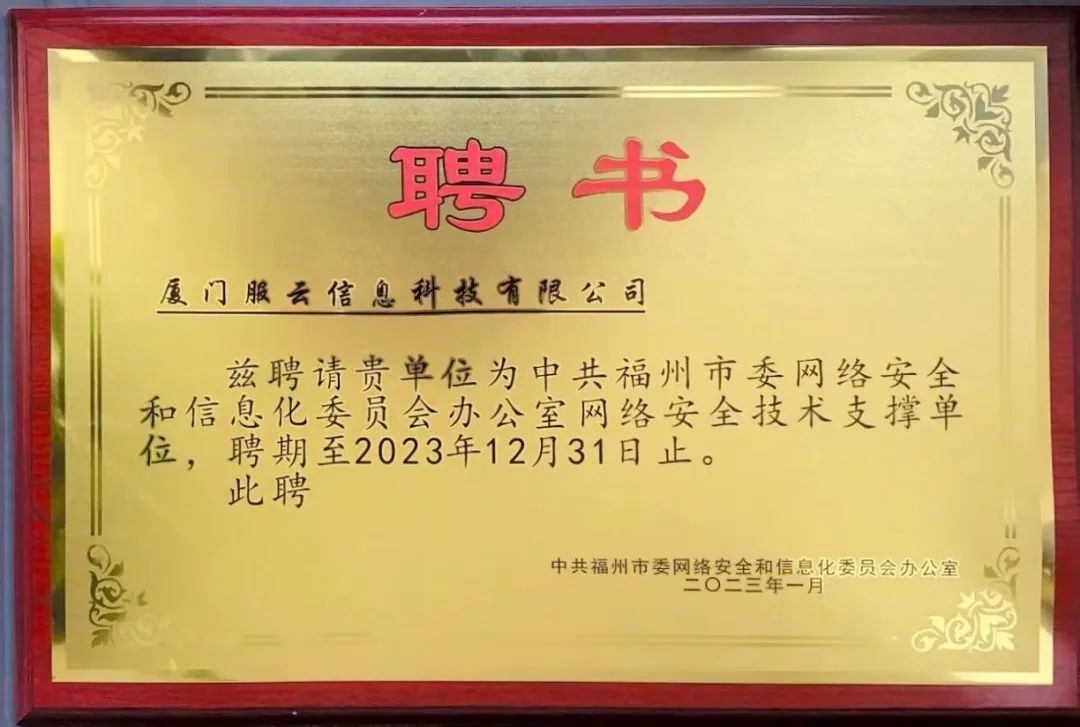
安全狗受聘成为福州网信办网络安全技术支撑单位
近日,福州市委网信办召开了2022年度网络安全技术支撑单位总结表彰大会。 作为国内云原生安全领导厂商,安全狗也出席了此次活动。 据悉,会议主要对2022年度优秀支撑单位进行表彰,并为2023年度支撑单位举行授牌仪式。 本次遴选工…...

RV1126 在Ubuntu18.04开发环境搭建
1:安装软件终端下输入安装命名:sudo apt install openssh-serversudo apt install android-tools-adbsudo apt install vim net-tools gitsudo apt install cmakesudo apt install treesudo apt install minicomsudo apt install gawksudo apt install bisonsudo ap…...

如何在 C++ 中调用 python 解析器来执行 python 代码(一)?
实现 Python UDF 中的一步就是学习如何在 C 语言中调用 python 解析器。本文根据 Python 官方文档做了一次实验,记录如下: 1. 安装依赖包 $sudo yum install python3-devel.x86_642. 使用 python-config 来生成编译选项 $python3.6-config --cflags -…...
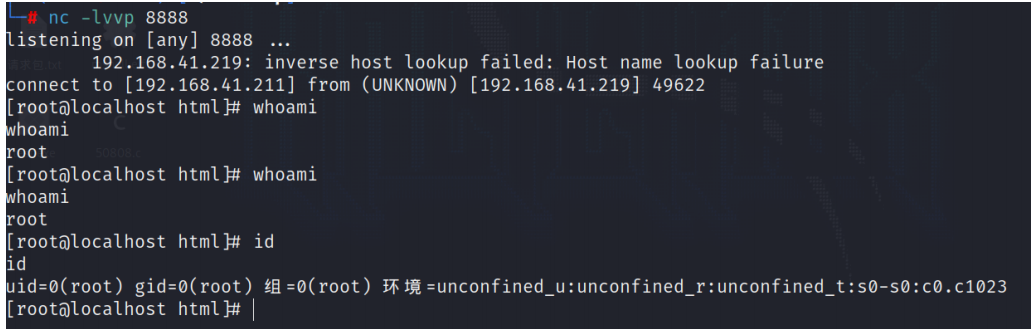
操作系统权限提升(二十三)之Linux提权-通配符(ws)提权
系列文章 操作系统权限提升(十八)之Linux提权-内核提权 操作系统权限提升(十九)之Linux提权-SUID提权 操作系统权限提升(二十)之Linux提权-计划任务提权 操作系统权限提升(二十一)之Linux提权-环境变量劫持提权 操作系统权限提升(二十二)之Linux提权-SUDO滥用提权 利用通配符…...

Zookeeper下载和安装
Zookeeper 1.下载 官方下载地址:https://zookeeper.apache.org/ 版本:apache-zookeeper-3.7.1-bin.tar.gz 2. 安装 2.1 本地安装 2.1.1 安装JDK 见:Hadoop集群搭建 2.1.2 上传安装包 使用远程工具拷贝安装包到Linux指定路径 /opt/s…...

Biomod2 (上):物种分布模型预备知识总结
Biomod11.栅格数据处理1.1 读取一个栅格图片1.2 计算数据间的相关系数1.3 生成多波段的栅格图像1.4 修改变量名称1.4.1 计算多个变量之间的相关性2. 矢量数据处理2.1 提取矢量数据2.2 数据掩膜2.2 栅格计算2.3 拓展插件的使用3. 图表绘制3.1 遥感影像绘制3.2 柱状图分析图绘制3…...
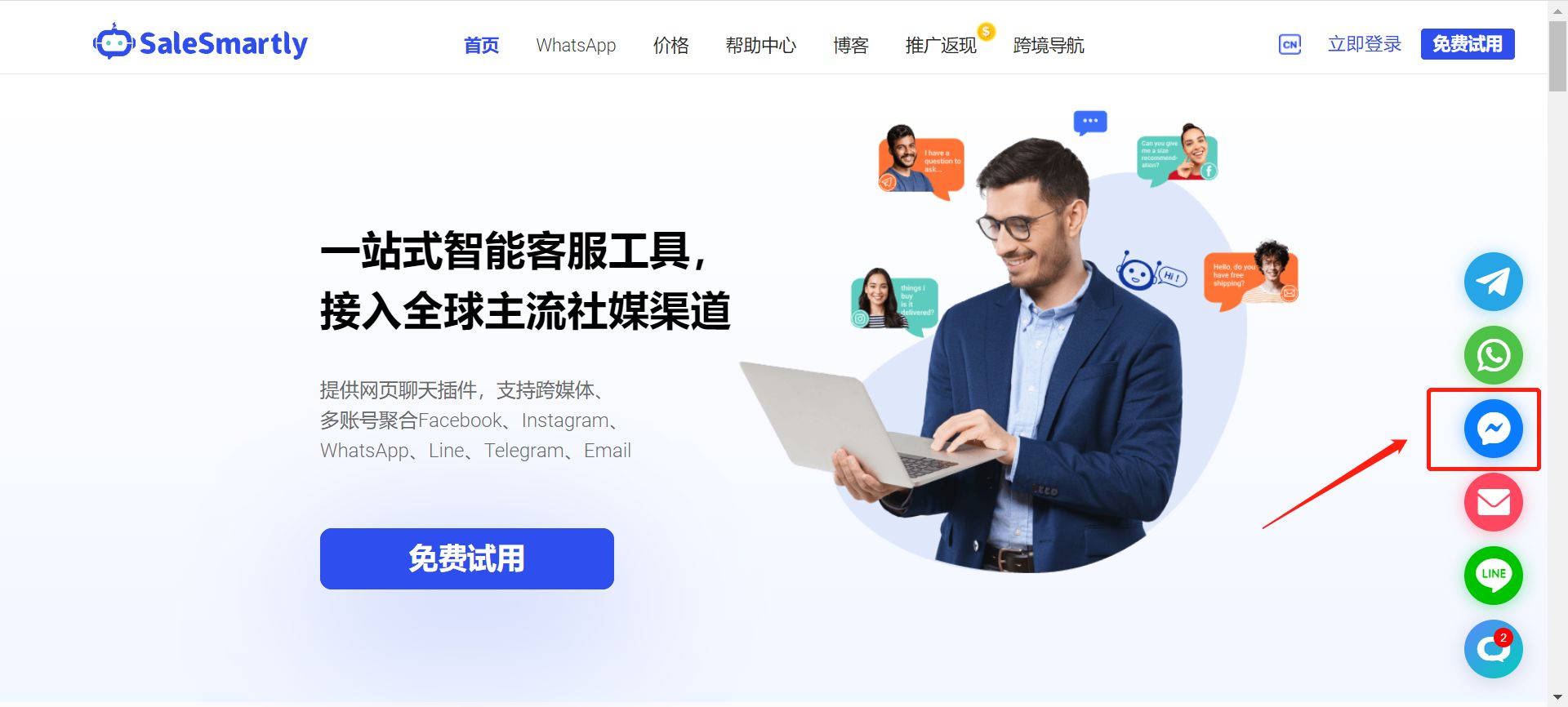
操作指南:如何高效使用Facebook Messenger销售(二)
上一篇文章我们介绍了使用Facebook Messenger作为销售渠道的定义、好处及注意事项,本节我们将详细介绍怎么将Facebook Messenger销售与SaleSmartly(ss客服)结合,实现一站式管理多主页配图来源:SaleSmartly(…...

计算机三级|网络技术|中小型网络系统总体规划与设计方案|IP地址规划技术|2|3
p3 p4一、中小型网络系统总体规划与设计方案网络关键的设备选型路由器技术指标性能指标综述吞吐量背板能力丢包率时延抖动突发处理能力路由表容量服务质量网管能力可靠性和可用性1 吞吐量指路由器的包转发能力,涉及两个内容:端口吞吐量和整机吞吐量&…...
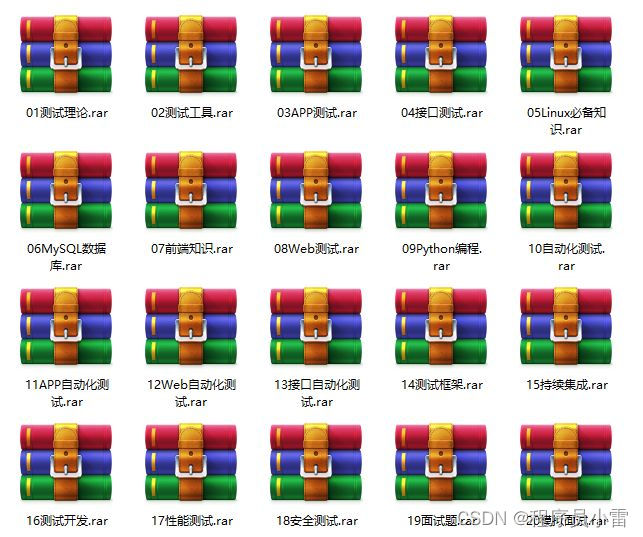
为什么一定要做集成测试?
集成测试,我们都不陌生,几乎我们产品每天都在进行。但是我们真的有好好思考:为什么一定要做集成测试吗?只是为了简单的将“积木”搭起来就行,还是有什么其他的深意? 深意可能不一定会有,但是意…...