【人工智能 Open AI 】我们程序员真的要下岗了- 全能写Go / C / Java / C++ / Python / JS 人工智能机器人
文章目录
- @[toc]
- 人工智能 AI Code 写代码测试
- 用golang实现冒泡排序
- 用golang实现计算环比函数
- goroutine and channel
- 用golang实现二叉树遍历代码
- 用golang实现线程安全的HashMap操作代码
- using C programming language write a tiny Operation System
- use C language write a tiny compiler
- 用golang实现B+树代码
- 使用 golang 写一个开启10个协程请求rpc接口的代码
- 用 C 语言实现一个HashMap 代码
- use C language write a Collection Operation API
文章目录
- @[toc]
- 人工智能 AI Code 写代码测试
- 用golang实现冒泡排序
- 用golang实现计算环比函数
- goroutine and channel
- 用golang实现二叉树遍历代码
- 用golang实现线程安全的HashMap操作代码
- using C programming language write a tiny Operation System
- use C language write a tiny compiler
- 用golang实现B+树代码
- 使用 golang 写一个开启10个协程请求rpc接口的代码
- 用 C 语言实现一个HashMap 代码
- use C language write a Collection Operation API
人工智能 AI Code 写代码测试
用golang实现冒泡排序
package mainimport "fmt"func main() {arr := []int{3,2,1,3,2,4,8,7,9,2,3}bubbleSort(arr) //冒泡排序fmt.Println("排序后:",arr)
}func bubbleSort(arr []int){for i:= 0; i < len(arr)-1; i++{for j:=0;j < len(arr)-1-i ;j++{if arr[j] > arr[j+1]{arr[j],arr[j+1] = arr[j+1],arr[j]}}}
}
用golang实现计算环比函数
package mainimport "fmt"func main() {fmt.Println(calcLinkRatio(5,3))
}//计算环比值(升、降)
func calcLinkRatio(a, b float64) float64 {//环比linkRatio := (b - a) / a * 100return linkRatio
}
goroutine and channel
package mainimport "fmt"// create a channel
var ch = make(chan int)// send data to channel
func sendData(nums ...int) {for _, n := range nums {ch <- n}
}// receive data from channel
func receiveData() {for {num := <-chfmt.Println("receive data from channel:", num)}
}// main function
func main() {// create go routinego sendData(1, 2, 3, 4, 5, 6)go receiveData()// wait for all routines to finishvar input stringfmt.Scanln(&input)fmt.Println("Done")
}
用golang实现二叉树遍历代码
type TreeNode struct
{Val intLeft *TreeNodeRight *TreeNode
}//先序遍历
func PreOrder(node *TreeNode) {if node == nil {return}//访问根节点fmt.Printf("%d ", node.Val)PreOrder(node.Left)PreOrder(node.Right)
}//中序遍历
func InOrder(node *TreeNode) {if node == nil {return}InOrder(node.Left)fmt.Printf("%d ", node.Val)InOrder(node.Right)
}//后序遍历
func PostOrder(node *TreeNode) {if node == nil {return}PostOrder(node.Left)PostOrder(node.Right)fmt.Printf("%d ", node.Val)
}
用golang实现线程安全的HashMap操作代码
go
package mainimport ("fmt""sync"
)type SafeHashMap struct {lock *sync.Mutexm map[int]int
}// 创建一个新的 HashMap,并初始化 Mutex 锁
func NewSafeHashMap() *SafeHashMap {return &SafeHashMap{ lock: new(sync.Mutex), m : make(map[int]int)}
}// 根据键值对 kv 向 HashMap 中插入一项数据
// 返回 bool 值,若插入成功则返回 true,否则返回 false
func (shm *SafeHashMap) Put(k int, v int) bool {// 上锁shm.lock.Lock()defer shm.lock.Unlock()// 若 key 已经存在,则返回 false_, ok := shm.m[k]if ok {return false}// 插入 key-value 键值对shm.m[k] = vreturn true
}// 根据 key 从 HashMap 中取出一项 key-value 键值对,
// 若 key 存在则返回对应的 value 以及 true 值,
// 否则返回零值以及 false 值
func (shm *SafeHashMap) Get(k int) (int, bool) {// 上锁shm.lock.Lock()defer shm.lock.Unlock()// 根据 key 取出 valuev, ok := shm.m[k]return v, ok
}// 从 HashMap 中移除一项 key-value 键值对,
// 若 key 存在则移除该项并返回 true 值,
// 否则返回 false 值
func (shm *SafeHashMap) Remove(k int) bool {// 上锁shm.lock.Lock()defer shm.lock.Unlock()_, ok := shm.m[k]if ok {delete(shm.m, k)}return ok
}// 返回 HashMap 中 key-value 键值对的项数
func (shm *SafeHashMap) Size() int {return len(shm.m)
}func main() {// 创建一个空的安全的 HashMapshm := NewSafeHashMap()// 插入 key-value 键值对shm.Put(1, 10)v, ok := shm.Get(1)fmt.Println(v, ok)// 移除 keyok = shm.Remove(1)fmt.Println(ok)// 获取 key 的数量fmt.Println(shm.Size())
}
using C programming language write a tiny Operation System
哈哈,让 AI 写一个操作系统,估计有点难为它了。目前来看,还达不到MOSS的水平,自动生成操作系统。
#include <stdio.h>
#include <stdlib.h>
#include <string.h>/******************** Function declarations ********************/
void clear(void); // clears the console
void print_welcome_message (void); // prints an introductory message
void command_input(void); // get an input from the user
int run_command (char *inp); // runs the command which is given by the user/******************** Main code ********************/
int main(void)
{clear();print_welcome_message();command_input();return 0;
}/******************** Function definitions ********************/
void clear()
{#ifdef WINDOWSsystem("cls");#elsesystem("clear");#endif
}void print_welcome_message()
{printf("Welcome to our Tiny OS!\n");printf("Start typing the commands:\n\n");
}void command_input()
{char input_str[20];while(1){printf("> ");scanf("%s", input_str);if(run_command(input_str) == -1){break;}}
}int run_command(char *inp)
{if(strcmp(inp, "exit") == 0){printf("\nbye!\n\n");return -1;}else{printf("Invalid Command.\nType \"exit\" to Exit.\n\n");return 0;}}
用 C 语言实现操作系统的自举启动的代码。
#include "boot.h"// 加载操作系统
void boot_load_os()
{ // 这里是一大堆代码// ...// 执行操作系统execute_os();
}
use C language write a tiny compiler
写个编译器看看。
#include <stdio.h>
#include <string.h>const int MAX_SIZE = 1000;// creating a structure to store the tokens.
struct tokens {char* type;char* value;
};//function to lex the program
struct tokens lex(char* input)
{struct tokens token;int i = 0;int startIndex = 0;while(input[i] != '\0'){char currentChar = input[i];if (currentChar == ' '){char* str = malloc(i - startIndex);memcpy(str, &input[startIndex], i - startIndex);token.type = str;token.value = NULL;break;}else if (currentChar == '+' || currentChar == '-'){token.type = "op";token.value = malloc(1);token.value[1] = '\0';token.value = currentChar;break;}i++;}return token;
}int main() {char input[MAX_SIZE];printf("Enter a simple expression:\t");scanf("%s", input);struct tokens token = lex(input);printf("Type: %s, Value: %s\n", token.type, token.value);return 0;
}
用golang实现B+树代码
package bptree// B+树定义
type BPTree struct {Order int // B+树的阶数Root *Node // 根节点Height int // 树的高度Size int // 树的大小Sequence func(interface{}, int) // B+树键比较函数
}// B+树中的节点
type Node struct {IsLeaf bool // 标识该节点是否为叶子节点Entries []*Entry // 节点上的数据条目Next *Node // 指向右兄弟节点Children []*Node // 不是叶子节点时有效,存放子节点指针Parent *Node // 指向父节点Items map[interface{}]*Entry // 键值对
}// 数据条目
type Entry struct {Key interface{} // B+树索引键Value interface{} // 数据值
}// 构造函数,构建一棵B+树
func NewTree(order int, sequence func(interface{}, int)) *BPTree {tree := &BPTree{Order: order,Root: nil,Height: 0,Size: 0,Sequence: sequence,}return tree
}// 搜索数据,返回搜索键的数据记录
func (tree *BPTree) Search(key interface{}) interface{} {node := tree._search(tree.Root, key)if node == nil {return nil}entry := node.Items[key]if entry == nil {return nil}return entry.Value
}// 内部搜索函数,返回搜索键所在节点
func (tree *BPTree) _search(node *Node, key interface{}) *Node {if node == nil {return nil}// 在节点上搜索键i, _ := tree._binarySearch(node, key)// 找到键if i >= 0 && node.Entries[i].Key == key {return node}// 不是叶子节点,从子节点继续搜索if !node.IsLeaf {return tree._search(node.Children[i], key)}// 未找到键return nil
}// 二分搜索函数,在节点上搜索键
func (tree *BPTree) _binarySearch(node *Node, key interface{}) (int, bool) {low := 0high := len(node.Entries) - 1for low <= high {mid := (low + high) / 2result := tree.cmpFunc(key, node.Entries[mid])if result == 0 {return mid, true // 搜索成功} else if result < 0 {high = mid - 1} else {low = mid + 1}}return low, false // 搜索失败
}
使用 golang 写一个开启10个协程请求rpc接口的代码
// 请求rpc
func RpcRequest(aid int, ch chan int) {resp := sendRpc(aid) // 发送rpcch <- resp
}func main() {ch := make(chan int, 10)for i := 0; i < 10; i++ {go RpcRequest(i, ch)}for i := 0; i < 10; i++ {resp := <- chfmt.Println("resp:", resp)}
}
用 C 语言实现一个HashMap 代码
/* 定义key-value字段 */
typedef struct
{void *key; //key void *value; //valuestruct ht_node *next; //单链表
} ht_node;/* 定义hashmap */
typedef struct
{int size; //字典的大小 ht_node **head; //字典的头int count; //元素的个数
}ht_hash_map;/*hash函数,计算键值*/
int hash_int_func(int key)
{key += ~(key << 15);key ^= (key >> 10);key += (key << 3);key ^= (key >> 6);key += ~(key << 11);key ^= (key >> 16);return (key & 0x7FFFFFFF);
}/* 创建hashmap */
ht_hash_map *ht_create(int size)
{ht_hash_map *ht = (ht_hash_map*)malloc(sizeof(ht_hash_map));ht->count = 0;ht->size = size;ht->head = (ht_node**)malloc(sizeof(ht_node*)*size);int i;for(i = 0; i < ht->size; i++){ht->head[i] = NULL;}return ht;
}/* 设置hashmap */
int ht_set(ht_hash_map *ht,void *key, void *value)
{int index = hash_int_func(*(int*)key) % ht->size;ht_node *tmp = ht->head[index];ht_node *last = NULL;while(tmp){if(*(int*)tmp->key == *(int*)key){tmp->value = value;return 0;}last = tmp;tmp = tmp->next;}// 没有重复映射ht_node *node = (ht_node*)malloc(sizeof(ht_node));node->key = key;node->value = value;node->next = NULL;// head为空if(ht->head[index] == NULL){ht->head[index] = node;}else{last->next = node;}ht->count++;return 1;
}/* 获取hashmap */
void *ht_get(ht_hash_map *ht, void *key)
{int index = hash_int_func(*(int*)key) % ht->size;ht_node *tmp = ht->head[index];while(tmp){if(*(int*)tmp->key == *(int*)key)return tmp->value;tmp = tmp->next;}return NULL;
}/* 删除hashmap */
int ht_remove(ht_hash_map *ht, void *key)
{int index = hash_int_func(*(int*)key) % ht->size;ht_node *tmp = ht->head[index];ht_node *last = NULL;while(tmp){if(*(int*)tmp->key == *(int*)key){if(last == NULL){//头节点ht->head[index] = tmp->next;}else{last->next = tmp->next;}free(tmp);return 0;}last = tmp;tmp = tmp->next;}return 1;
}/* 销毁hashmap */
void ht_destory(ht_hash_map *ht) {int i;for (i = 0; i < ht.size; i++) {item *cur_item, *cur_item_tmp;cur_item_tmp = ht->items[i];while (cur_item_tmp) {cur_item = cur_item_tmp;cur_item_tmp = cur_item_tmp->next;free(cur_item);}}free(ht);
}/* 查找hashmap中的key-value */
ht_item *ht_search(ht_hash_map *ht, const char *key) {int index;index = hash(key, ht->size);item *cur_item;cur_item = ht->items[index];while (cur_item) {if (strcmp(cur_item->key, key) == 0) { return cur_item; }cur_item = cur_item->next;}return NULL;
}
use C language write a Collection Operation API
#include <stdlib.h>typedef struct item {void *data;
} Item;typedef struct Collection{void* data;int size;
} Collection;// Create a new Collection
Collection* Collection_create() {Collection *collection = (Collection*)malloc(sizeof(Collection));if (collection == NULL) {return NULL;}collection->data = NULL;collection->size = 0;return collection;
}// Add an item to the Collection
int Collection_add(Collection *collection, void* item) {if (collection == NULL) return 0;// Increase the size of the collectionint newSize = collection->size + 1;Item *items = (Item*)realloc(collection->data, newSize*sizeof(Item));if (items == NULL) {return 0;}collection->data = items;// Add the itemItem *item_ptr = &items[collection->size];item_ptr->data = item;collection->size = newSize;return 1;
}// Remove an item from the Collection
int Collection_remove(Collection *collection, void* item) {if (collection == NULL || collection->size == 0) return 0;// Find the item to removeItem *items = (Item*)collection->data;int removeIndex = -1;int i;for (i = 0; i < collection->size; i++) {Item *item_ptr = &items[i];if (item_ptr->data == item) {removeIndex = i;break;}}if (removeIndex == -1) {return 0;}// Remove the itemint newSize = collection->size - 1;Item *newItems = (Item*)malloc(newSize*sizeof(Item));if (newItems == NULL) return 0;// Copy the elements before the removeIndexfor (i = 0; i < removeIndex; i++) {newItems[i] = items[i];}// Copy the elements after the removeIndexfor (i = removeIndex; i < newSize; i++) {newItems[i] = items[i+1];}free(collection->data);collection->data = newItems;collection->size = newSize;return 1;
}// Clear all items from the Collection
int Collection_clear(Collection *collection) {if (collection == NULL) return 0;free(collection->data);collection->data = NULL;collection->size = 0;return 1;
}// Delete the Collection
void Collection_delete(Collection *collection) {if (collection == NULL) return;free(collection->data);free(collection);
}
估计 csdn 封掉链接,不让贴,请私信我!
相关文章:
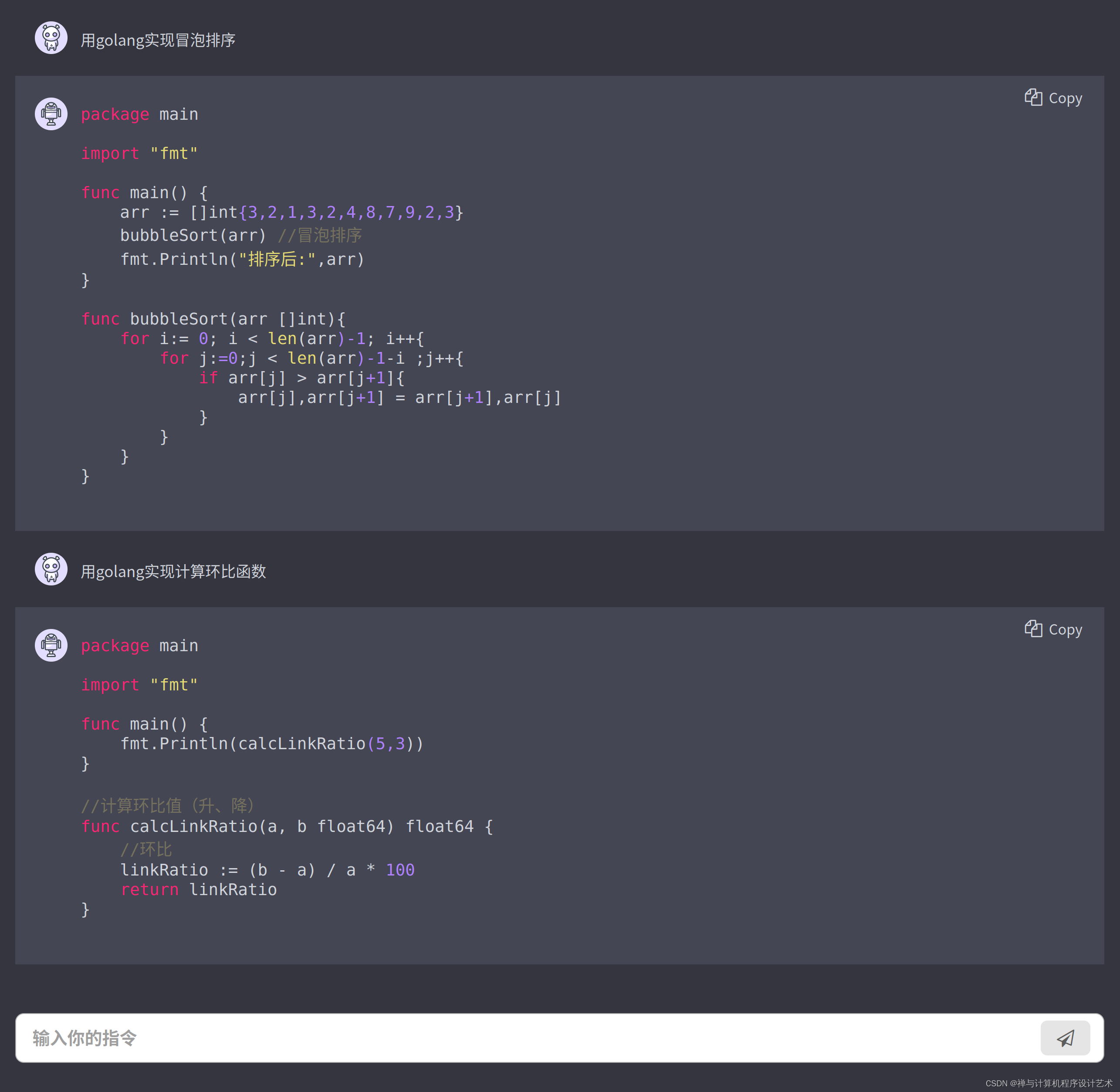
【人工智能 Open AI 】我们程序员真的要下岗了- 全能写Go / C / Java / C++ / Python / JS 人工智能机器人
文章目录[toc]人工智能 AI Code 写代码测试用golang实现冒泡排序用golang实现计算环比函数goroutine and channel用golang实现二叉树遍历代码用golang实现线程安全的HashMap操作代码using C programming language write a tiny Operation Systemuse C language write a tiny co…...
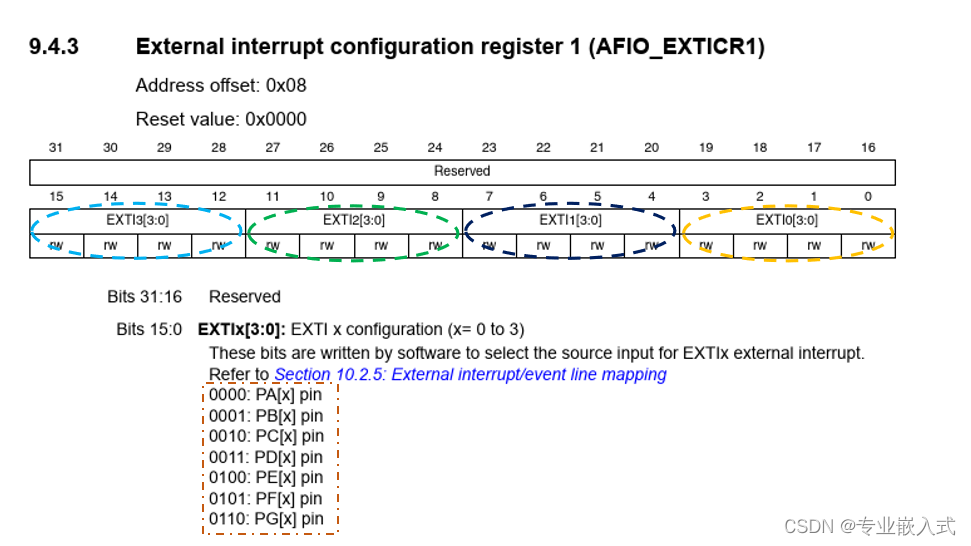
STM32 EXTI外部中断
本文代码使用 HAL 库。 文章目录前言一、什么是外部中断?二、外部中断中断线三、STM32F103的引脚复用四、相关函数:总结前言 一、什么是外部中断? 外部中断 是单片机实时地处理外部事件的一种内部机制。当某种外部事件发生时,单片…...
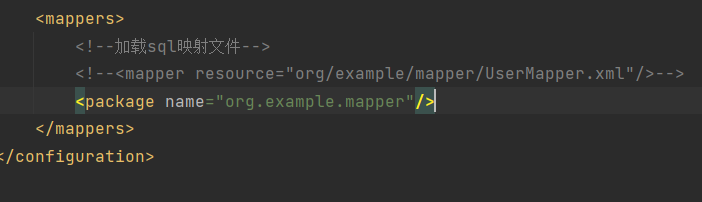
Mapper代理开发——书接MaBatis的简单使用
在这个mybatis的普通使用中依旧存在硬编码问题,虽然静态语句比原生jdbc都写更少了但是还是要写,Mapper就是用来解决原生方式中的硬编码还有简化后期执行SQL UserMapper是一个接口,里面有很多方法,都是一一和配置文件里面的sql语句的id名称所对…...

实体对象说明
1.工具类层Utilutil 工具顾明思义,util层就是存放工具类的地方,对于一些独立性很高的小功能,或重复性很高的代码片段,可以提取出来放到Util层中。2.数据层POJO对象(概念比较大) 包含了以下POJO plain ord…...
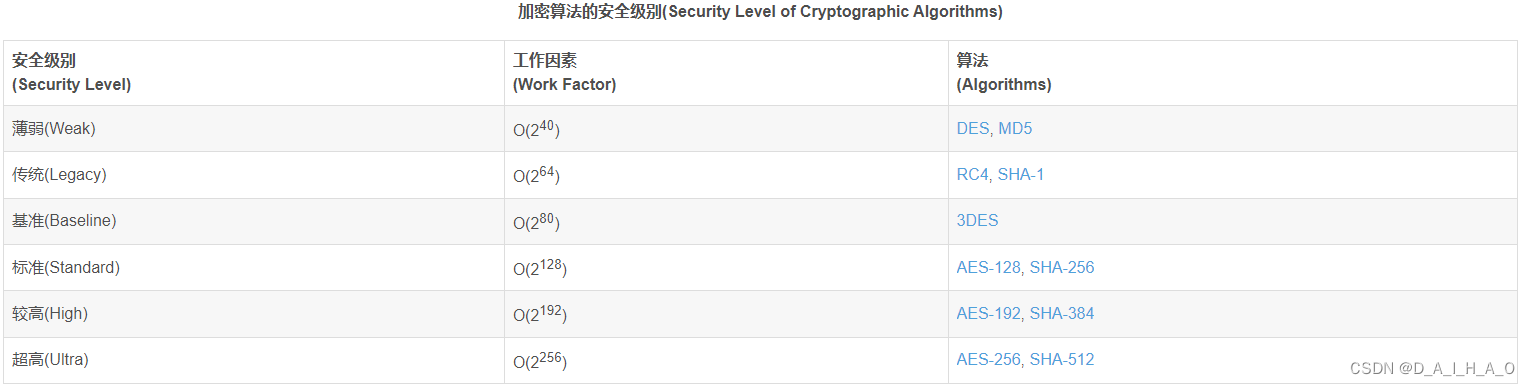
JAVA中加密与解密
BASE64加密/解密 Base64 编码会将字符串编码得到一个含有 A-Za-z0-9/ 的字符串。标准的 Base64 并不适合直接放在URL里传输,因为URL编码器会把标准 Base64 中的“/”和“”字符变为形如 “%XX” 的形式,而这些 “%” 号在存入数据库时还需要再进行转换&…...
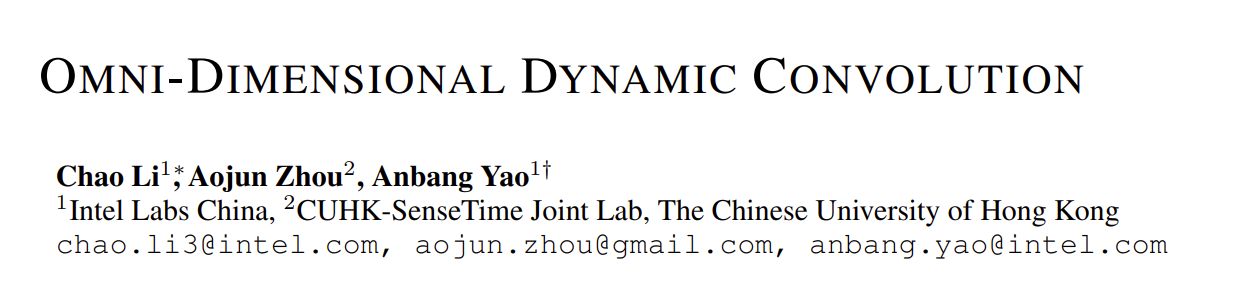
改进YOLO系列 | ICLR2022 | OMNI-DIMENSIONAL DYNAMIC CONVOLUTION: 全维动态卷积
单个静态卷积核是现代卷积神经网络(CNNs)的常见训练范式。然而,最近的动态卷积研究表明,学习加权为其输入依赖注意力的n个卷积核的线性组合可以显著提高轻量级CNNs的准确性,同时保持高效的推理。然而,我们观察到现有的作品通过卷积核空间的一个维度(关于卷积核数量)赋予…...

信息收集之Github搜索语法
信息收集之Github搜索语法1.Github的搜索语法2.使用 Github 进行邮件配置信息收集3.使用Github进行数据库信息收集4.使用Github进行 SVN 信息收集5.使用Github进行综合信息收集在测试的信息收集阶段,可以去Github和码云上搜索与目标有关的信息,或者就有意…...
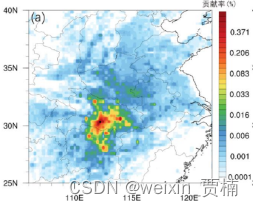
【案例教程】拉格朗日粒子扩散模式FLEXPART
拉格朗日粒子扩散模式FLEXPART通过计算点、线、面或体积源释放的大量粒子的轨迹,来描述示踪物在大气中长距离、中尺度的传输、扩散、干湿沉降和辐射衰减等过程。该模式既可以通过时间的前向运算来模拟示踪物由源区向周围的扩散,也可以通过后向运算来确定…...
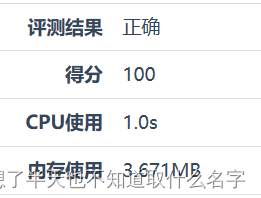
试题 算法训练 自行车停放
问题描述 有n辆自行车依次来到停车棚,除了第一辆自行车外,每辆自行车都会恰好停放在已经在停车棚里的某辆自行车的左边或右边。(e.g.停车棚里已经有3辆自行车,从左到右编号为:3,5,1。现在编号为2的第4辆自行车要停在5号自行车的左…...
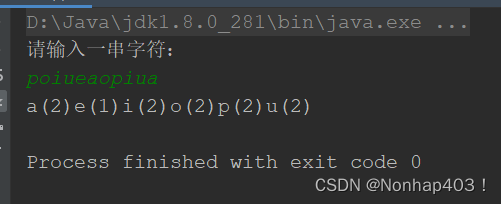
泛型与Map接口
Java学习之道 泛型 泛型这种参数类型可以用在类、方法和接口中,分别被称为泛型类,泛型方法,泛型接口 参数化类型:将类型由原来的具体的类型参数化,在使用/调用时传入具体的类型JDK5引入特性提供了安全检测机制…...

Unity Bug记录本
//个人记录,持续更新 1、将此代码挂载到空脚本上: bool flag (object)GetComponent<Camera>() null; bool flag1 (object)GetComponent<Text>() null; Debug.Log(flag"::"flag1); //输出结果:False::True bool…...

B. The Number of Products)厉害
You are given a sequence a1,a2,…,ana1,a2,…,an consisting of nn non-zero integers (i.e. ai≠0ai≠0). You have to calculate two following values: the number of pairs of indices (l,r)(l,r) (l≤r)(l≤r) such that al⋅al1…ar−1⋅aral⋅al1…ar−1⋅ar is neg…...

一起Talk Android吧(第五百一十二回:自定义Dialog)
文章目录整体思路实现方法第一步第二步第三步第四步各位看官们大家好,上一回中咱们说的例子是"自定义Dialog主题",这一回中咱们说的例子是" 自定义Dialog"。闲话休提,言归正转, 让我们一起Talk Android吧!整体…...
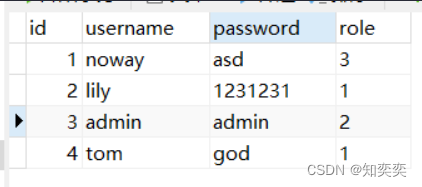
GinVueAdmin源码分析3-整合MySQL
目录文件结构数据库准备配置文件处理config.godb_list.gogorm_mysql.gosystem.go初始化数据库gorm.gogorm_mysql.go开始初始化测试数据库定义实体类 Userserviceapi开始测试!文件结构 本文章将使用到上一节创建的 CommonService 接口,用于测试连接数据库…...

大数据框架之Hadoop:MapReduce(三)MapReduce框架原理——MapReduce开发总结
在编写MapReduce程序时,需要考虑如下几个方面: 1、输入数据接口:InputFormat 默认使用的实现类是:TextInputFormatTextInputFormat的功能逻辑是:一次读一行文本,然后将该行的起始偏移量作为key࿰…...

requests---(4)发送post请求完成登录
前段时间写过一个通过cookies完成登录,今天我们写一篇通过post发送请求完成登录豆瓣网 模拟登录 1、首先找到豆瓣网的登录接口 打开豆瓣网站的登录接口,请求错误的账号密码,通过F12或者抓包工具找到登录接口 通过F12抓包获取到请求登录接口…...
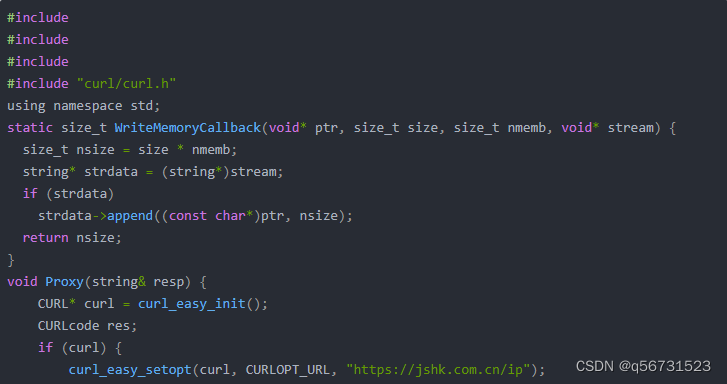
Python抓取数据具体流程
之前看了一段有关爬虫的网课深有启发,于是自己也尝试着如如何过去爬虫百科“python”词条等相关页面的整个过程记录下来,方便后期其他人一起来学习。 抓取策略 确定目标:重要的是先确定需要抓取的网站具体的那些部分,下面实例是…...
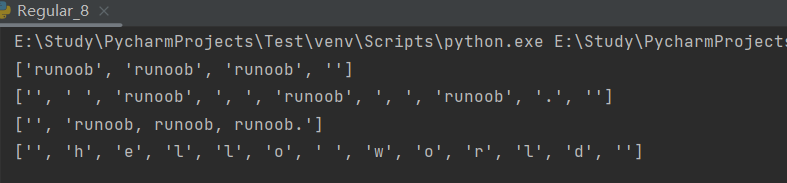
【Python学习笔记】第二十四节 Python 正则表达式
一、正则表达式简介正则表达式(regular expression)是一个特殊的字符序列,它能帮助你方便的检查一个字符串是否与某种模式匹配。正则表达式是对字符串(包括普通字符(例如,a 到 z 之间的字母)和特…...
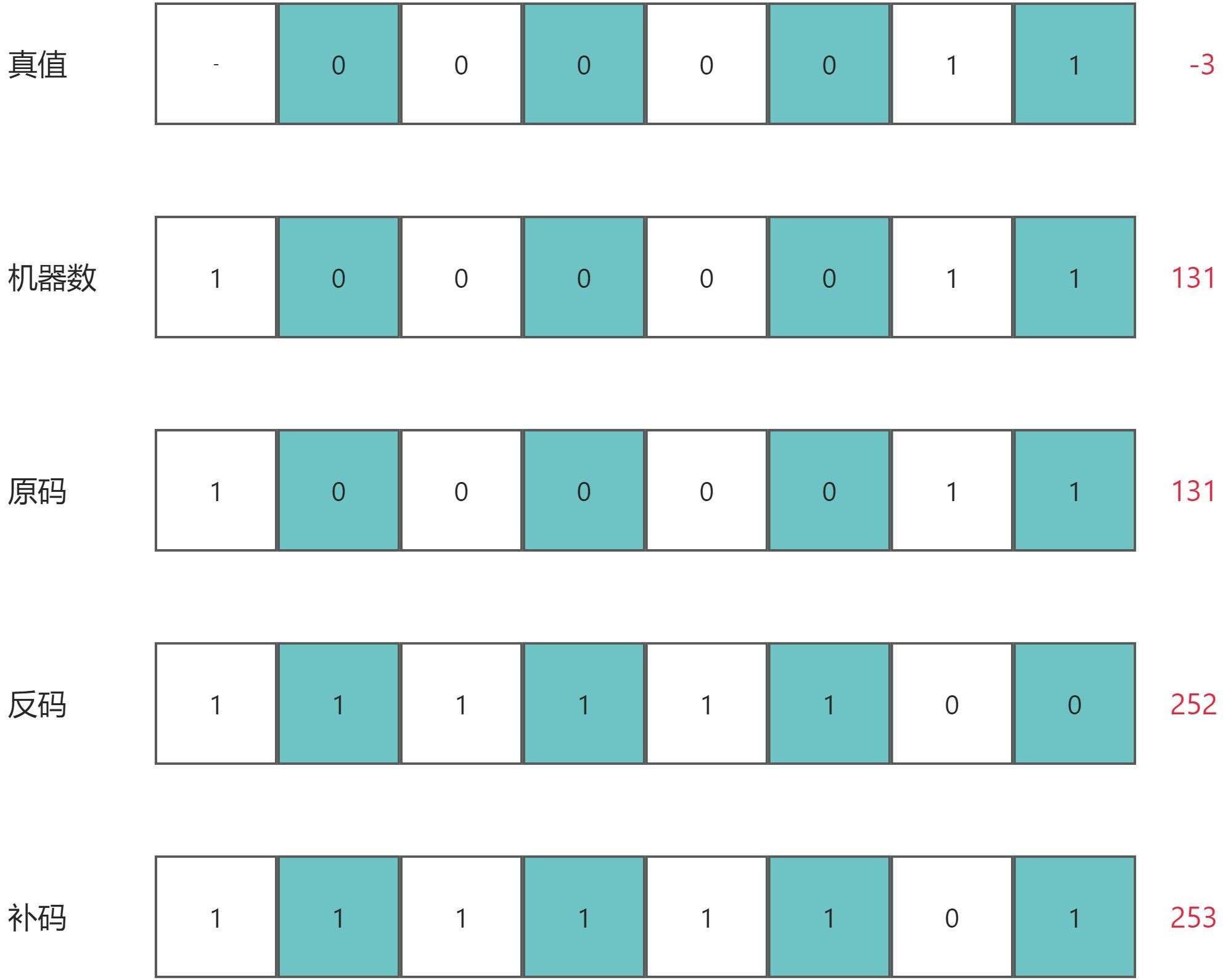
数字逻辑基础:原码、反码、补码
时间紧、不理解可以只看这里的结论 正数的原码、反码、补码相同。等于真值对应的机器码。 负数的原码等于机器码,反码为原码的符号位不变,其余各位按位取反。补码为反码1。 三种码的出现是为了解决计算问题并简化电路结构。 在原码和反码中,存…...

有限差分法-差商公式及其Matlab实现
2.1 有限差分法 有限差分法 (finite difference method)是一种数值求解偏微分方程的方法,它将偏微分方程中的连续变量离散化为有限个点上的函数值,然后利用差分逼近导数,从而得到一个差分方程组。通过求解差分方程组,可以得到原偏微分方程的数值解。 有限差分法是一种历史…...

高校就业信息管理系统
1引言 1.1编写目的 1.2背景 1.3定义 1.4参考资料 2程序系统的结构 3登录模块设计说明一 3.1程序描述 3.2功能 3.3性能 3.4输人项 3.5输出项 3.6算法 3.7流程逻辑 3.8接口 3.10注释设计 3.11限制条件 3.12测试计划 3.13尚未解决的问题 4注册模块设计说明 4.…...
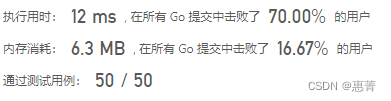
【Java|golang】2373. 矩阵中的局部最大值
给你一个大小为 n x n 的整数矩阵 grid 。 生成一个大小为 (n - 2) x (n - 2) 的整数矩阵 maxLocal ,并满足: maxLocal[i][j] 等于 grid 中以 i 1 行和 j 1 列为中心的 3 x 3 矩阵中的 最大值 。 换句话说,我们希望找出 grid 中每个 3 x …...
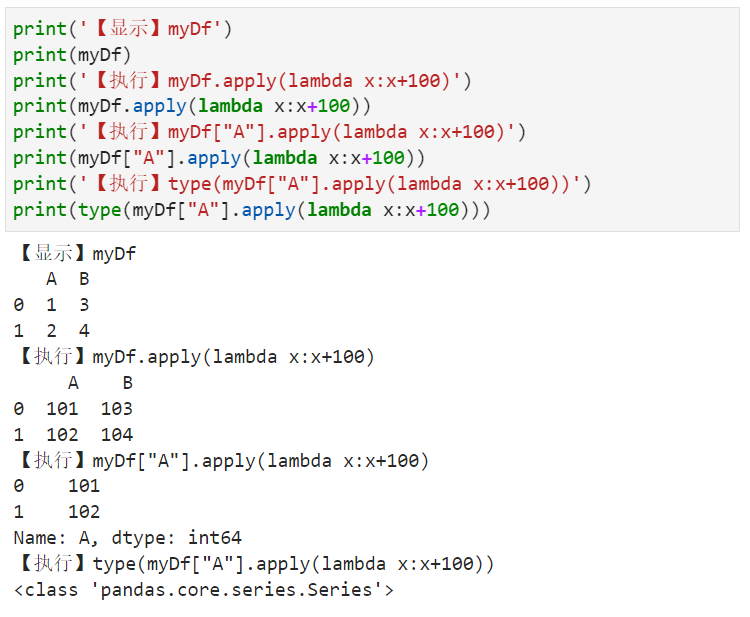
根据指定函数对DataFrame中各元素进行计算
【小白从小学Python、C、Java】【计算机等级考试500强双证书】【Python-数据分析】根据指定函数对DataFrame中各元素进行计算以下错误的一项是?import numpy as npimport pandas as pdmyDict{A:[1,2],B:[3,4]}myDfpd.DataFrame(myDict)print(【显示】myDf)print(myDf)print(【…...

【蓝桥杯集训·每日一题】AcWing 3502. 不同路径数
文章目录一、题目1、原题链接2、题目描述二、解题报告1、思路分析2、时间复杂度3、代码详解三、知识风暴一、题目 1、原题链接 3502. 不同路径数 2、题目描述 给定一个 nm 的二维矩阵,其中的每个元素都是一个 [1,9] 之间的正整数。 从矩阵中的任意位置出发…...
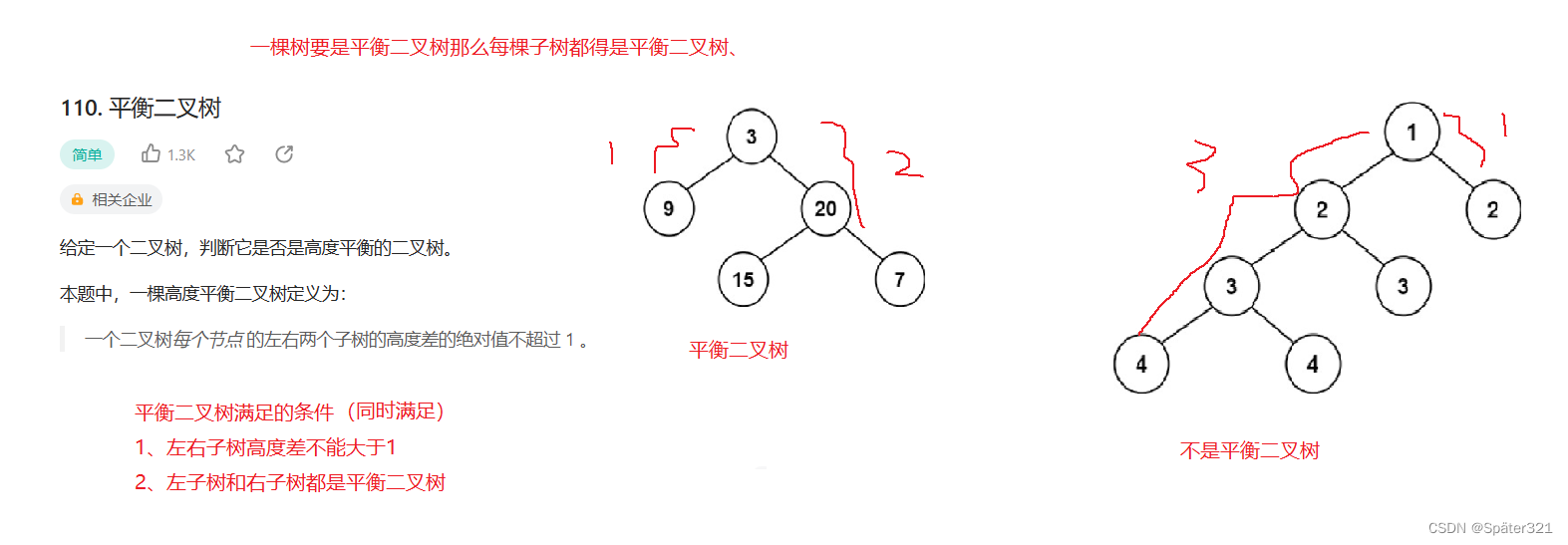
Java - 数据结构,二叉树
一、什么是树 概念 树是一种非线性的数据结构,它是由n(n>0)个有限结点组成一个具有层次关系的集合。把它叫做树是因为它看起来像一棵倒挂的树,也就是说它是根朝上,而叶朝下的。它具有以下的特点: 1、有…...
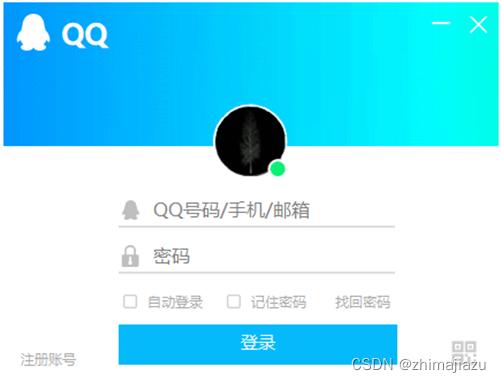
模拟QQ登录-课后程序(JAVA基础案例教程-黑马程序员编著-第十一章-课后作业)
【案例11-3】 模拟QQ登录 【案例介绍】 1.案例描述 QQ是现实生活中常用的聊天工具,QQ登录界面看似小巧、简单,但其中涉及的内容却很多,对于初学者练习Java Swing工具的使用非常合适。本案例要求使用所学的Java Swing知识,模拟实…...
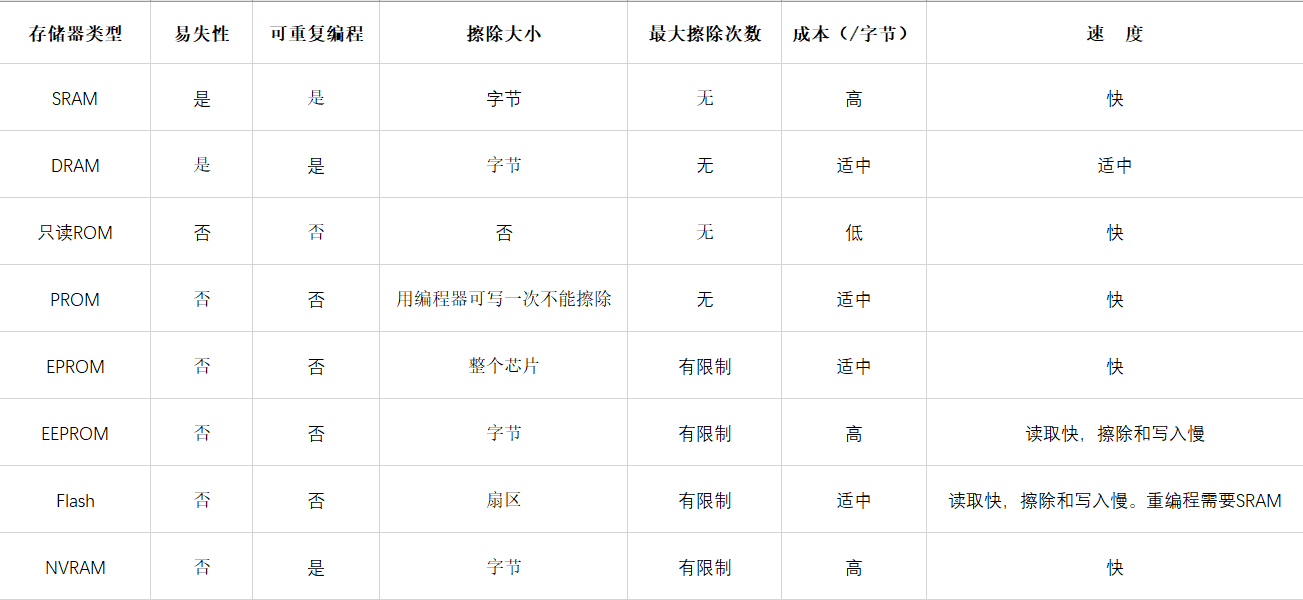
【壹】嵌入式系统硬件基础
随手拍拍💁♂️📷 日期: 2023.2.28 地点: 杭州 介绍: 日子像旋转毒马🐎,在脑海里转不停🤯 🌲🌲🌲🌲🌲 往期回顾 🌲🌲🌲…...
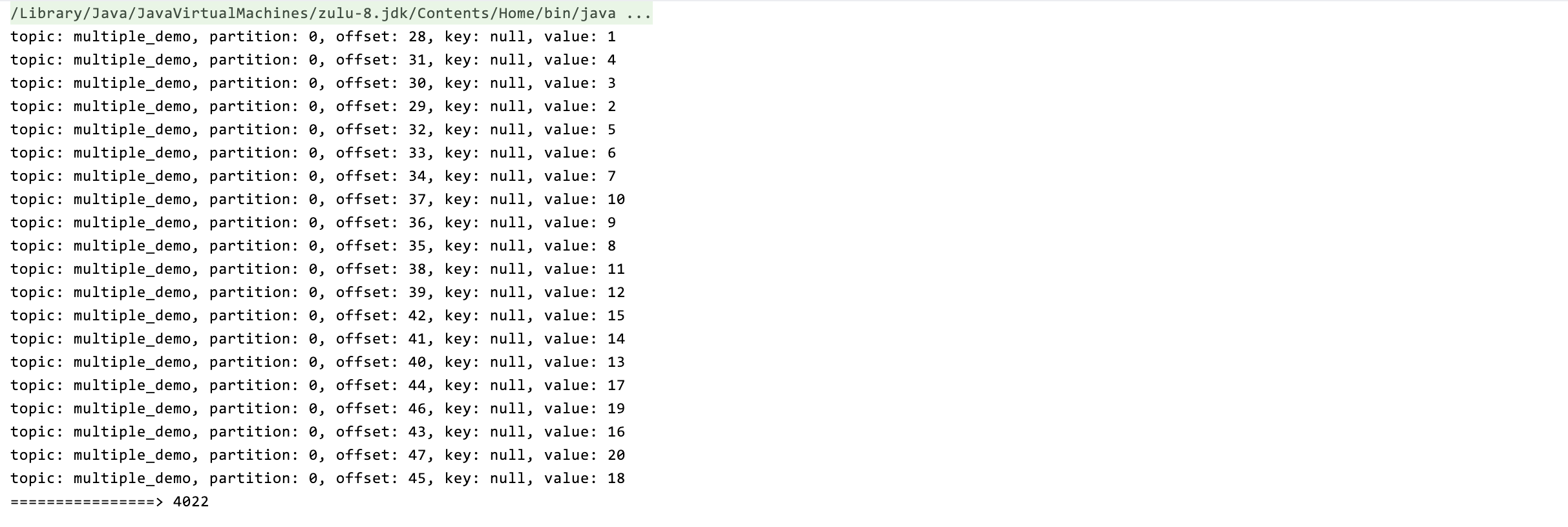
当参数调优无法解决kafka消息积压时可以这么做
今天的议题是:如何快速处理kafka的消息积压 通常的做法有以下几种: 增加消费者数增加 topic 的分区数,从而进一步增加消费者数调整消费者参数,如max.poll.records增加硬件资源 常规手段不是本文的讨论重点或者当上面的手段已经使…...
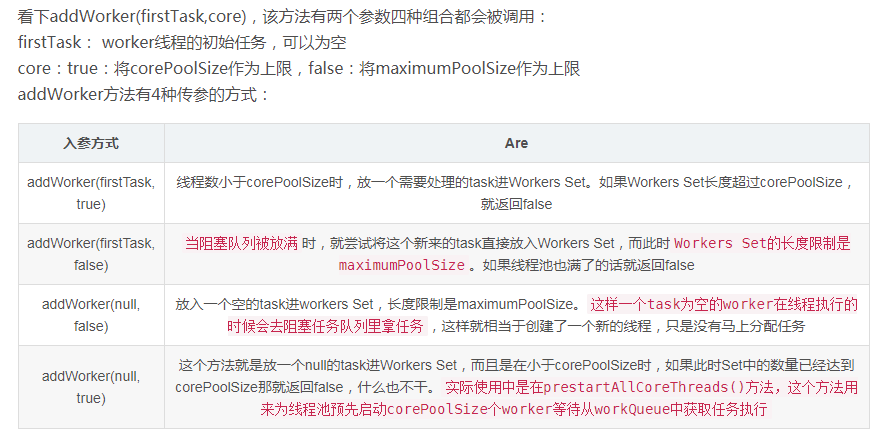
Java线程池源码分析
Java 线程池的使用,是面试必问的。下面我们来从使用到源码整理一下。 1、构造线程池 通过Executors来构造线程池 1、构造一个固定线程数目的线程池,配置的corePoolSize与maximumPoolSize大小相同, 同时使用了一个无界LinkedBlockingQueue存…...
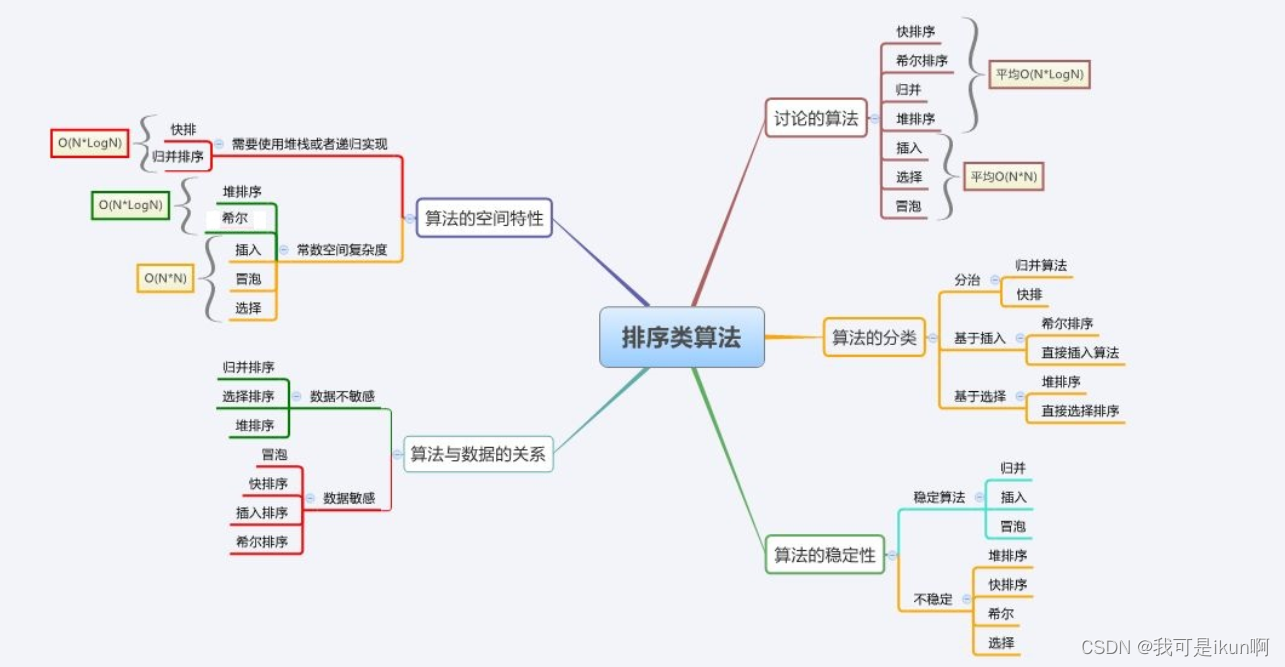
手撕八大排序(下)
目录 交换排序 冒泡排序: 快速排序 Hoare法 挖坑法 前后指针法【了解即可】 优化 再次优化(插入排序) 迭代法 其他排序 归并排序 计数排序 排序总结 结束了上半章四个较为简单的排序,接下来的难度将会大幅度上升&…...