perl脚本中使用eval函数执行可能有异常的操作
perl脚本中有时候执行的操作可能会引发异常,为了直观的说明,这里举一个json反序列化的例子,脚本如下:
#! /usr/bin/perl
use v5.14;
use JSON;
use Data::Dumper;# 读取json字符串数据
my $json_str = join('', <DATA>);
# 反序列化操作
my $json = from_json($json_str);say to_json($json, { pretty => 1 });__DATA__
bad {"id" : 1024,"desc" : "hello world","other" : {"test_null" : null,"test_false" : false,"test_true" : true}
}
脚本中有意把正确json字符串之前加了几个字符,显然这个json字符串是不符合规范格式的,在git bash中执行这个脚本,结果如下:
下面用perl的eval函数改造这个脚本:
#! /usr/bin/perl
use v5.14;
use JSON;
use Data::Dumper;# 读取json字符串数据
my $json_str = join('', <DATA>);
# 反序列化操作
my $json = eval {return from_json($json_str);
};unless (defined $json) {say "from_json failed !!!!";
} else {say to_json($json, { pretty => 1 });
}__DATA__
bad {"id" : 1024,"desc" : "hello world","other" : {"test_null" : null,"test_false" : false,"test_true" : true}
}
脚本中用把from_json的操作放在eval函数中,输出结果如下:
显然,这个结果是可控的,可预期的。比如就可以使用这种方法判断json字符串是否合法,能够正常反序列化的就是合法的,否则就是非法的。eval函数的具体使用可以使用perldoc -f eval查看。
eval EXPReval BLOCKeval "eval" in all its forms is used to execute a little Perlprogram, trapping any errors encountered so they don't crash thecalling program.Plain "eval" with no argument is just "eval EXPR", where theexpression is understood to be contained in $_. Thus there areonly two real "eval" forms; the one with an EXPR is often called"string eval". In a string eval, the value of the expression(which is itself determined within scalar context) is firstparsed, and if there were no errors, executed as a block withinthe lexical context of the current Perl program. This form istypically used to delay parsing and subsequent execution of thetext of EXPR until run time. Note that the value is parsed everytime the "eval" executes.The other form is called "block eval". It is less general thanstring eval, but the code within the BLOCK is parsed only once(at the same time the code surrounding the "eval" itself wasparsed) and executed within the context of the current Perlprogram. This form is typically used to trap exceptions moreefficiently than the first, while also providing the benefit ofchecking the code within BLOCK at compile time. BLOCK is parsedand compiled just once. Since errors are trapped, it often isused to check if a given feature is available.In both forms, the value returned is the value of the lastexpression evaluated inside the mini-program; a return statementmay also be used, just as with subroutines. The expressionproviding the return value is evaluated in void, scalar, or listcontext, depending on the context of the "eval" itself. See"wantarray" for more on how the evaluation context can bedetermined.If there is a syntax error or runtime error, or a "die"statement is executed, "eval" returns "undef" in scalar context,or an empty list in list context, and $@ is set to the errormessage. (Prior to 5.16, a bug caused "undef" to be returned inlist context for syntax errors, but not for runtime errors.) Ifthere was no error, $@ is set to the empty string. A controlflow operator like "last" or "goto" can bypass the setting of$@. Beware that using "eval" neither silences Perl from printingwarnings to STDERR, nor does it stuff the text of warningmessages into $@. To do either of those, you have to use the$SIG{__WARN__} facility, or turn off warnings inside the BLOCKor EXPR using "no warnings 'all'". See "warn", perlvar, andwarnings.Note that, because "eval" traps otherwise-fatal errors, it isuseful for determining whether a particular feature (such as"socket" or "symlink") is implemented. It is also Perl'sexception-trapping mechanism, where the "die" operator is usedto raise exceptions.Before Perl 5.14, the assignment to $@ occurred beforerestoration of localized variables, which means that for yourcode to run on older versions, a temporary is required if youwant to mask some, but not all errors:# alter $@ on nefarious repugnancy only{my $e;{local $@; # protect existing $@eval { test_repugnancy() };# $@ =~ /nefarious/ and die $@; # Perl 5.14 and higher only$@ =~ /nefarious/ and $e = $@;}die $e if defined $e}There are some different considerations for each form:String evalSince the return value of EXPR is executed as a block withinthe lexical context of the current Perl program, any outerlexical variables are visible to it, and any packagevariable settings or subroutine and format definitionsremain afterwards.Under the "unicode_eval" featureIf this feature is enabled (which is the default under a"use 5.16" or higher declaration), EXPR is considered tobe in the same encoding as the surrounding program. Thusif "use utf8" is in effect, the string will be treatedas being UTF-8 encoded. Otherwise, the string isconsidered to be a sequence of independent bytes. Bytesthat correspond to ASCII-range code points will havetheir normal meanings for operators in the string. Thetreatment of the other bytes depends on if the"'unicode_strings"" feature is in effect.In a plain "eval" without an EXPR argument, being in"use utf8" or not is irrelevant; the UTF-8ness of $_itself determines the behavior.Any "use utf8" or "no utf8" declarations within thestring have no effect, and source filters are forbidden.("unicode_strings", however, can appear within thestring.) See also the "evalbytes" operator, which worksproperly with source filters.Variables defined outside the "eval" and used inside itretain their original UTF-8ness. Everything inside thestring follows the normal rules for a Perl program withthe given state of "use utf8".Outside the "unicode_eval" featureIn this case, the behavior is problematic and is not soeasily described. Here are two bugs that cannot easilybe fixed without breaking existing programs:* It can lose track of whether something should beencoded as UTF-8 or not.* Source filters activated within "eval" leak out intowhichever file scope is currently being compiled. Togive an example with the CPAN moduleSemi::Semicolons:BEGIN { eval "use Semi::Semicolons; # not filtered" }# filtered here!"evalbytes" fixes that to work the way one wouldexpect:use feature "evalbytes";BEGIN { evalbytes "use Semi::Semicolons; # filtered" }# not filteredProblems can arise if the string expands a scalar containinga floating point number. That scalar can expand to letters,such as "NaN" or "Infinity"; or, within the scope of a "uselocale", the decimal point character may be something otherthan a dot (such as a comma). None of these are likely toparse as you are likely expecting.You should be especially careful to remember what's beinglooked at when:eval $x; # CASE 1eval "$x"; # CASE 2eval '$x'; # CASE 3eval { $x }; # CASE 4eval "\$$x++"; # CASE 5$$x++; # CASE 6Cases 1 and 2 above behave identically: they run the codecontained in the variable $x. (Although case 2 hasmisleading double quotes making the reader wonder what elsemight be happening (nothing is).) Cases 3 and 4 likewisebehave in the same way: they run the code '$x', which doesnothing but return the value of $x. (Case 4 is preferred forpurely visual reasons, but it also has the advantage ofcompiling at compile-time instead of at run-time.) Case 5 isa place where normally you *would* like to use doublequotes, except that in this particular situation, you canjust use symbolic references instead, as in case 6.An "eval ''" executed within a subroutine defined in the"DB" package doesn't see the usual surrounding lexicalscope, but rather the scope of the first non-DB piece ofcode that called it. You don't normally need to worry aboutthis unless you are writing a Perl debugger.The final semicolon, if any, may be omitted from the valueof EXPR.Block evalIf the code to be executed doesn't vary, you may use theeval-BLOCK form to trap run-time errors without incurringthe penalty of recompiling each time. The error, if any, isstill returned in $@. Examples:# make divide-by-zero nonfataleval { $answer = $a / $b; }; warn $@ if $@;# same thing, but less efficienteval '$answer = $a / $b'; warn $@ if $@;# a compile-time erroreval { $answer = }; # WRONG# a run-time erroreval '$answer ='; # sets $@If you want to trap errors when loading an XS module, someproblems with the binary interface (such as Perl versionskew) may be fatal even with "eval" unless$ENV{PERL_DL_NONLAZY} is set. See perlrun.Using the "eval {}" form as an exception trap in librariesdoes have some issues. Due to the current arguably brokenstate of "__DIE__" hooks, you may wish not to trigger any"__DIE__" hooks that user code may have installed. You canuse the "local $SIG{__DIE__}" construct for this purpose, asthis example shows:# a private exception trap for divide-by-zeroeval { local $SIG{'__DIE__'}; $answer = $a / $b; };warn $@ if $@;This is especially significant, given that "__DIE__" hookscan call "die" again, which has the effect of changing theirerror messages:# __DIE__ hooks may modify error messages{local $SIG{'__DIE__'} =sub { (my $x = $_[0]) =~ s/foo/bar/g; die $x };eval { die "foo lives here" };print $@ if $@; # prints "bar lives here"}Because this promotes action at a distance, thiscounterintuitive behavior may be fixed in a future release."eval BLOCK" does *not* count as a loop, so the loop controlstatements "next", "last", or "redo" cannot be used to leaveor restart the block.The final semicolon, if any, may be omitted from within theBLOCK.
相关文章:

perl脚本中使用eval函数执行可能有异常的操作
perl脚本中有时候执行的操作可能会引发异常,为了直观的说明,这里举一个json反序列化的例子,脚本如下: #! /usr/bin/perl use v5.14; use JSON; use Data::Dumper;# 读取json字符串数据 my $json_str join(, <DATA>); # 反…...
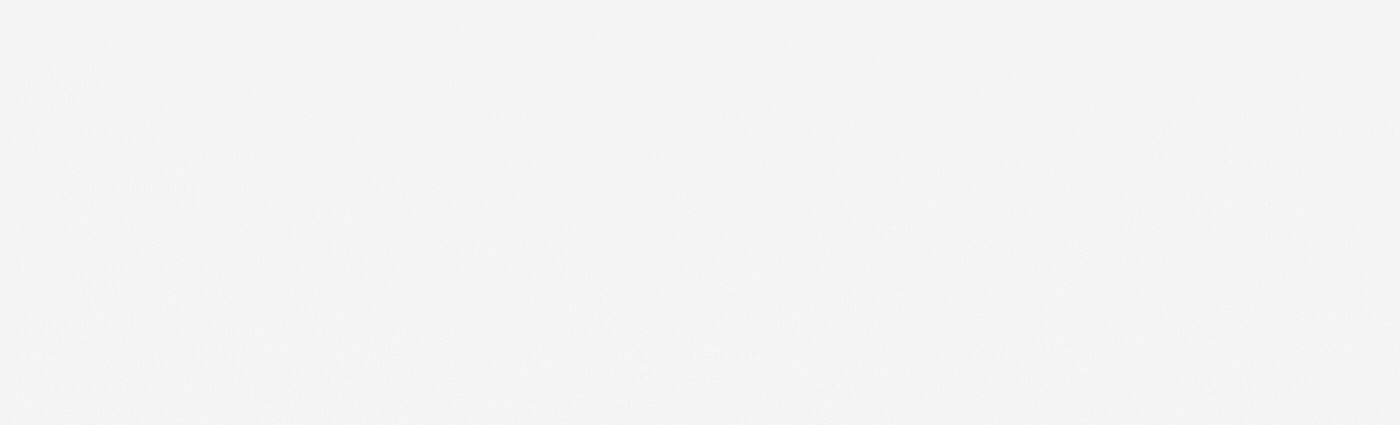
『Redis』在Docker中快速部署Redis并进行数据持久化挂载
📣读完这篇文章里你能收获到 在Docke中快速部署Redis如何将Redis的数据进行持久化 文章目录 一、拉取镜像二、创建挂载目录1 宿主机与容器挂载映射2 挂载命令执行 三、创建容器—运行Redis四、查看运行情况 一、拉取镜像 版本号根据需要自己选择,这里以…...
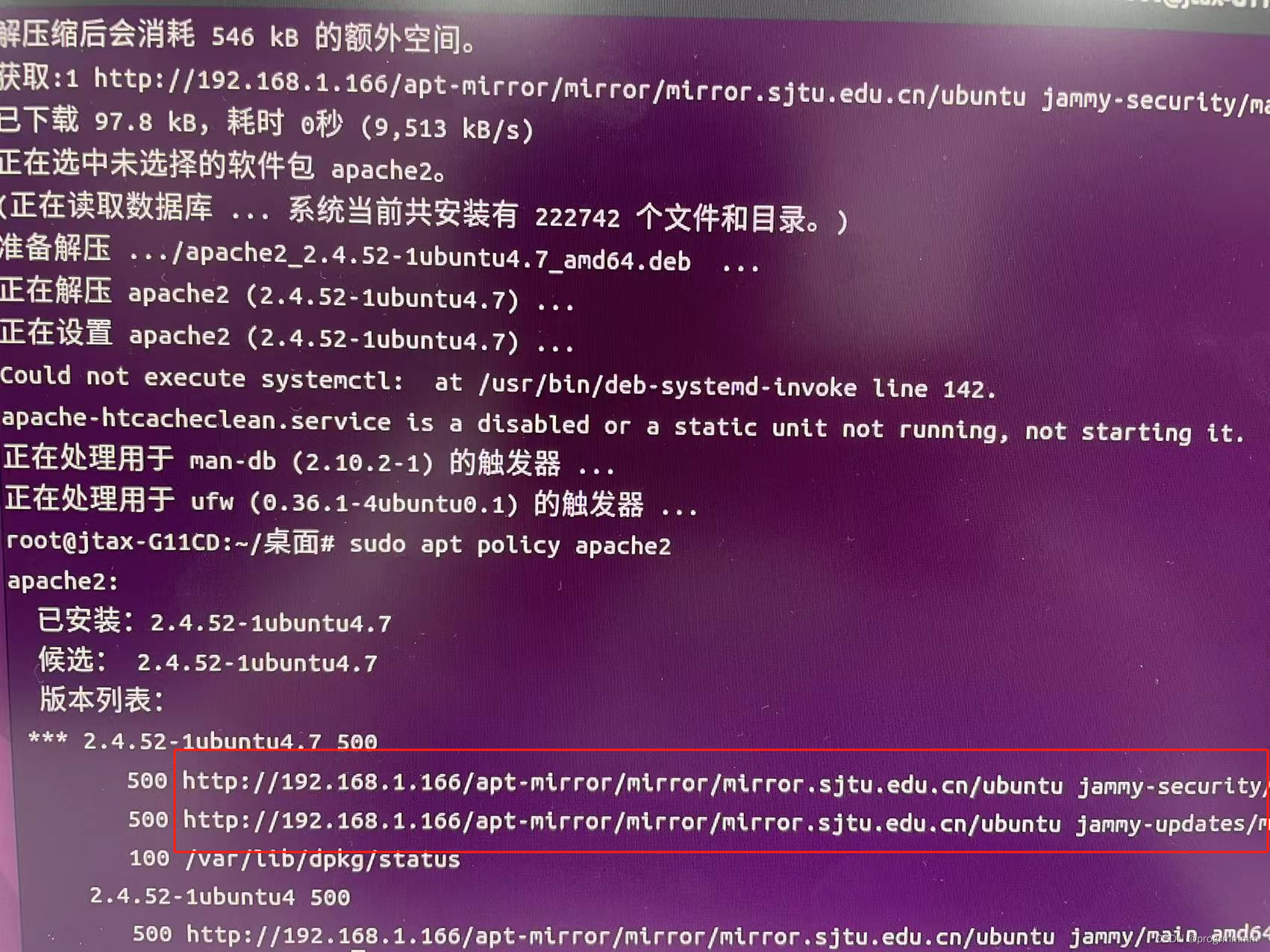
ubuntu创建apt-mirror本地仓库
首先创建apt-mirror的服务端,也就是存储所有apt-get下载的文件和依赖。大约需要300G,预留400G左右空间就可以开始了。 安装ubuntu省略,用的是ubuntu202204 ubuntu挂载硬盘(不需要的可以跳过): #下载挂载工具 sudo apt…...
计算机网络 internet应用 (水
ARPA net ---Internet 前身 发展史: ARPA net 第一个主干网..美国军方NSFnet 美国国家科学基金会NSFANSnet 美国全国 (internet 叫法开始出现) 第二代互联网(现在() IP地址 IP地址 最高管理机构 - InterNIC IPV4 32位 IPV6 128位 域名 起名 解析 domain name sys…...
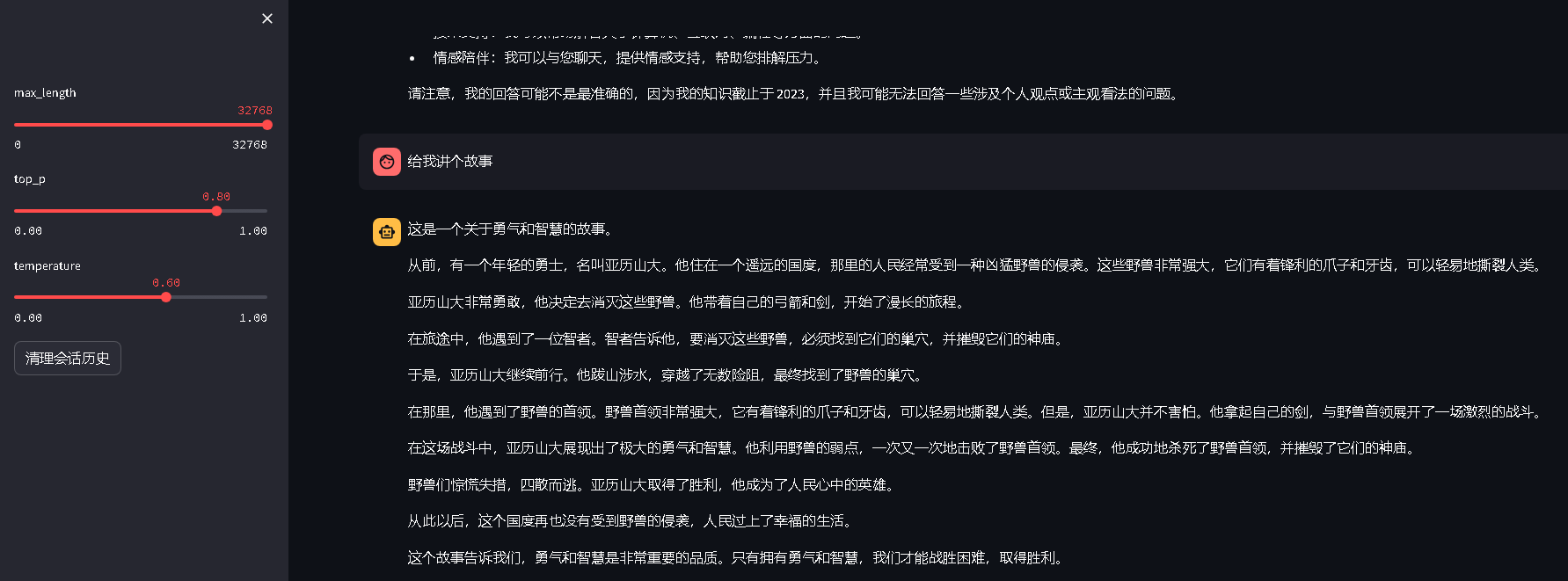
【ChatGLM3】第三代大语言模型多GPU部署指南
关于ChatGLM3 ChatGLM3是智谱AI与清华大学KEG实验室联合发布的新一代对话预训练模型。在第二代ChatGLM的基础之上, 更强大的基础模型: ChatGLM3-6B 的基础模型 ChatGLM3-6B-Base 采用了更多样的训练数据、更充分的训练步数和更合理的训练策略。在语义、…...
云原生Kubernetes系列 | Docker/Kubernetes的卷管理
云原生Kubernetes系列 | Docker/Kubernetes的卷管理 1. Docker卷管理2. Kubernetes卷管理2.1. 本地存储2.1.1. emptyDir2.1.2. hostPath2.2. 网络存储2.2.1. 使用NFS2.2.2. 使用ISCSI2.3. 持久化存储2.3.1. PV和PVC2.3.2. 访问模式2.3.3. 回收策略1. Docker卷管理...
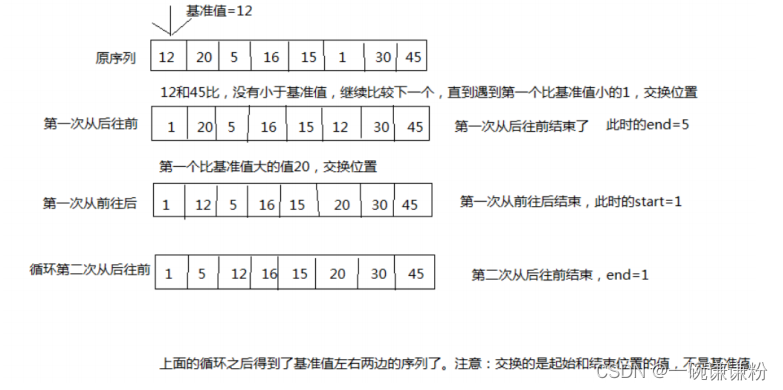
Java实现快速排序算法
快速排序算法 (1)概念:快速排序是指通过一趟排序将要排序的数据分割成独立的两部分,其中一部分的所有数据都比另外一部分的所有数据都要小,然后再按此方法对这两部分数据分别进行快速排序。整个排序过程可以递归进行&…...
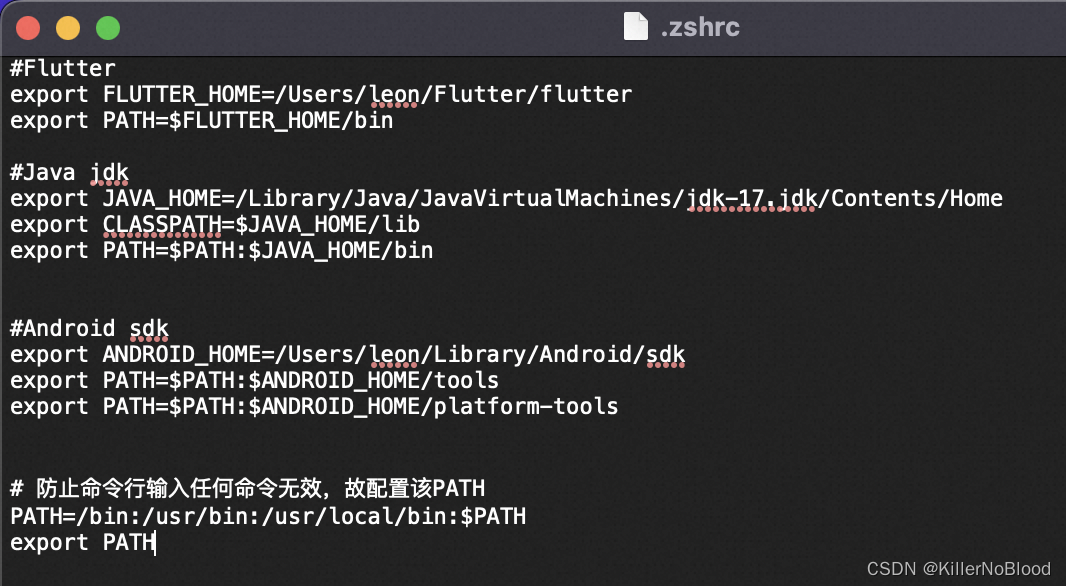
MAC配置环境变量
1、配置 JAVA JDK 1.1、查看 JDK 安装目录 (1)可以在Android Studio中查看,复制该路径 (2)也可以在官网下载 Java JDK下载地址 mac中的安装地址是"资源库->Java->JavaVirtualMachines"中 1.2、…...
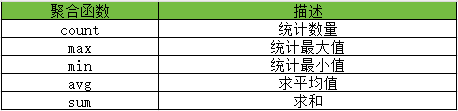
系列五、DQL
一、DQL 1.1、概述 DQL的英文全称为:Data Query Language,中文意思为:数据查询语言,用大白话讲就是查询数据。对于大多数系统来说,查询操作的频次是要远高于增删改的,当我们去访问企业官网、电商网站&…...
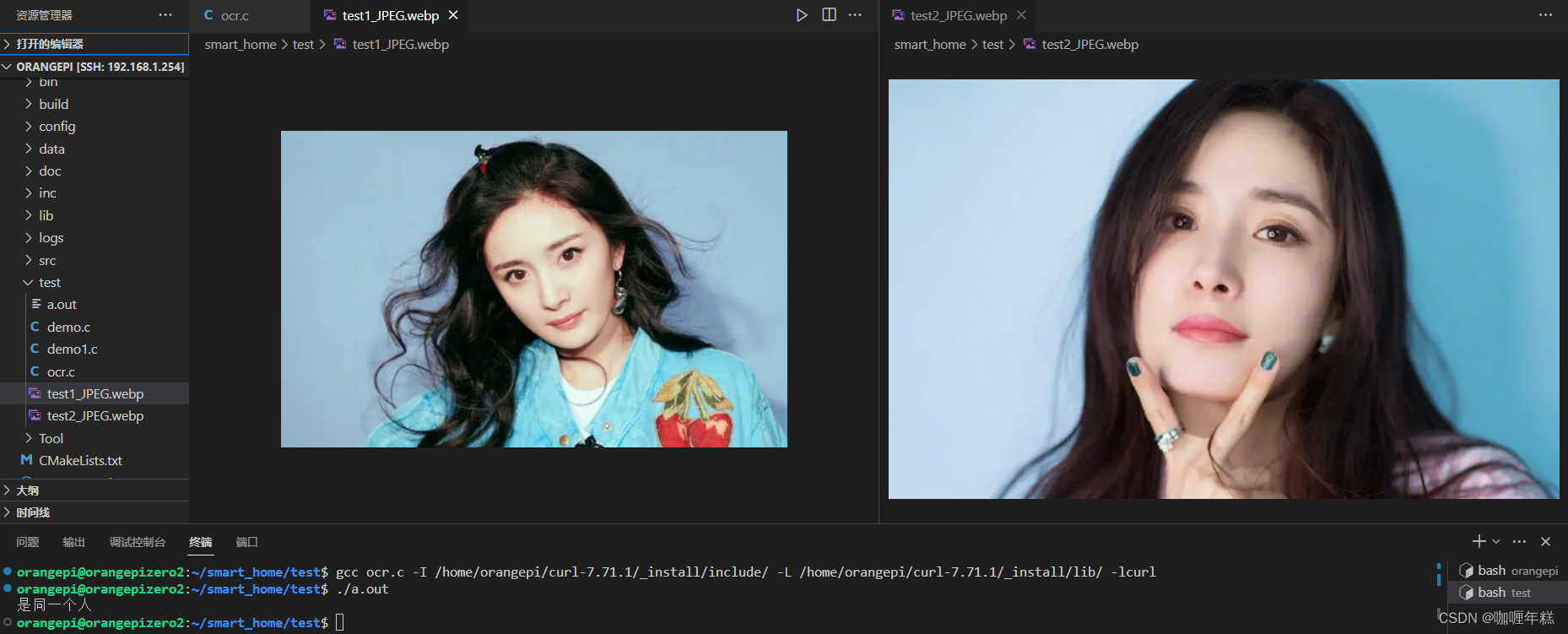
【智能家居】七、人脸识别 翔云平台编程使用(编译openSSL支持libcurl的https访问、安装SSL依赖库openSSL)
一、翔云 人工智能开放平台 API文档开发示例下载 二、编译openSSL支持libcurl的https访问 安装SSL依赖库openSSL(使用工具wget)libcurl库重新配置,编译,安装运行(运行需添加动态库为环境变量) 三、编程实现人脸识别 四、Base6…...
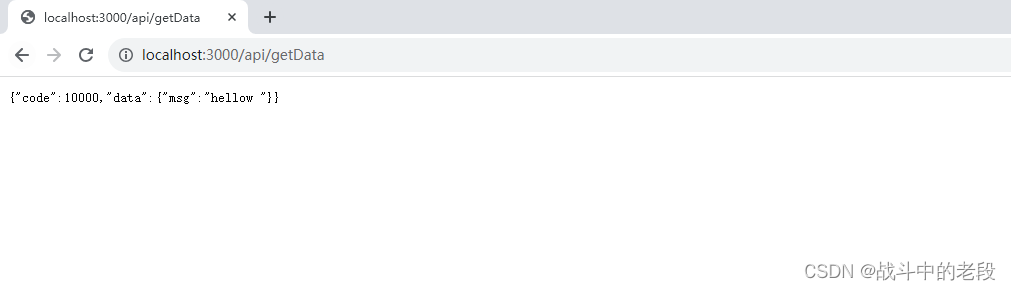
基于node 安装express后端脚手架
1.首先创建文件件 2.在文件夹内打开终端 npm init 3.安装express: npm install -g express-generator注意的地方:这个时候安装特别慢,最后导致不成功 解决方法:npm config set registry http://registry.npm.taobao.org/ 4.依次执行 npm install -g ex…...
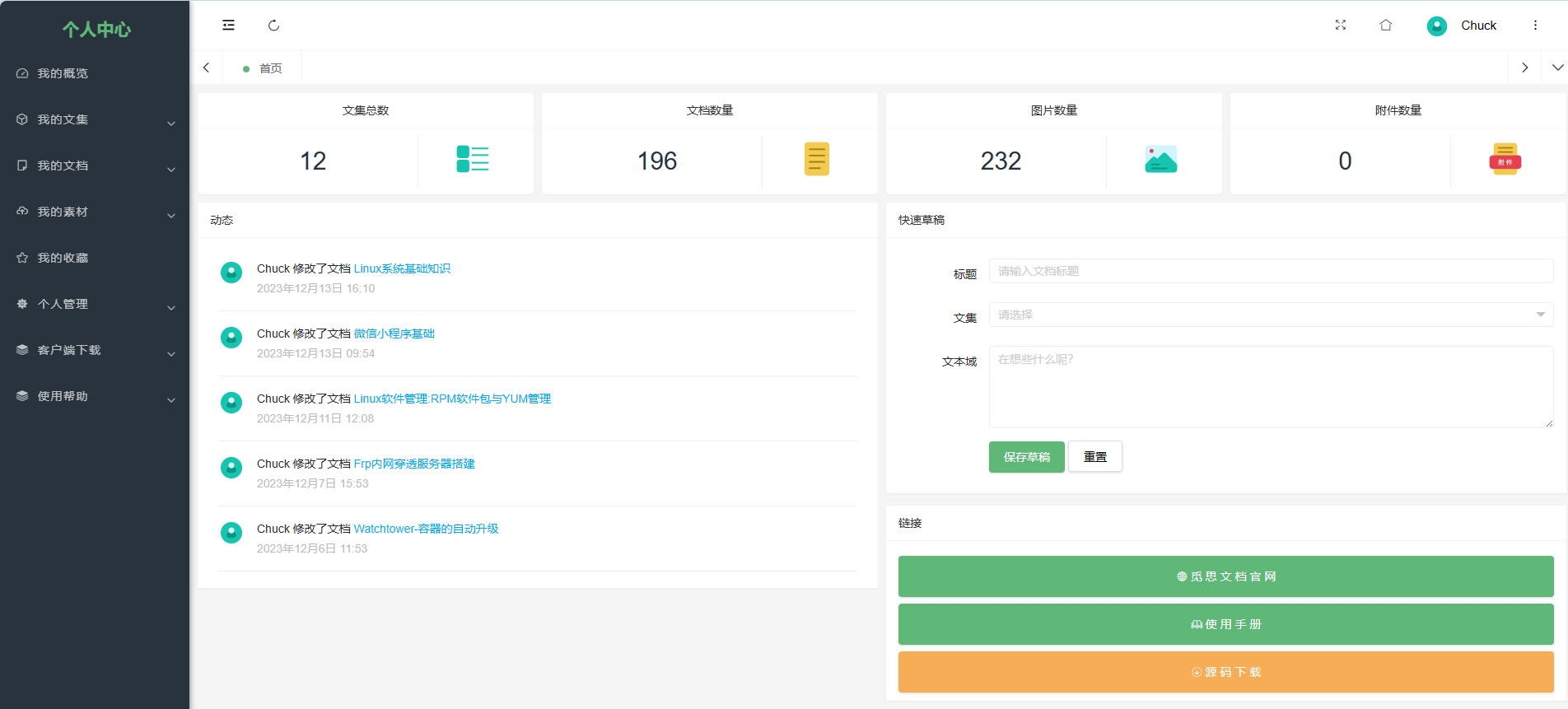
Mrdoc知识文档
MrDoc知识文档平台是一款基于Python开发的在线文档系统,适合作为个人和中小型团队的私有云文档、云笔记和知识管理工具,致力于成为优秀的私有化在线文档部署方案。我现在主要把markdown笔记放在上面,因为平时老是需要查询一些知识点ÿ…...
C语言中getchar函数
在 C 语言中,getchar() 是一个标准库函数,用于从标准输入(通常是键盘)读取单个字符。它的函数原型如下: int getchar(void);getchar() 函数的工作原理如下: 当调用 getchar() 函数时,它会等待…...
全栈开发组合
SpringBoot是什么? SpringBoot是一个基于Spring框架的开源框架,由Pivotal团队开发。它的设计目的是用来简化Spring应用的初始搭建以及开发过程。SpringBoot提供了丰富的Spring模块化支持,可以帮助开发者更轻松快捷地构建出企业级应用 Sprin…...
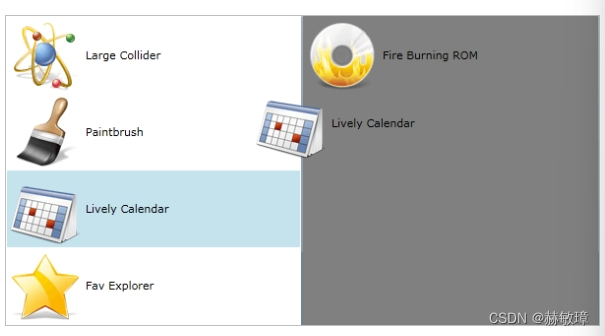
wpf TelerikUI使用DragDropManager
首先,我先创建事务对象ApplicationInfo,当暴露出一对属性当例子集合对于构成ListBoxes。这个类在例子中显示如下代码: public class ApplicationInfo { public Double Price { get; set; } public String IconPath { get; set; } public …...
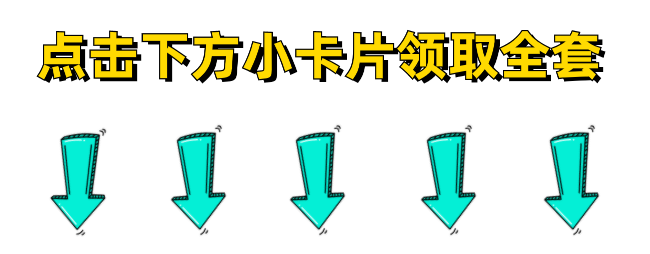
Python+Appium自动化测试之元素等待方法与重新封装元素定位方法
在appium自动化测试脚本运行的过程中,因为网络不稳定、测试机或模拟器卡顿等原因,有时候会出现页面元素加载超时元素定位失败的情况,但实际这又不是bug,只是元素加载较慢,这个时候我们就会使用元素等待的方法来避免这种…...
详解Maven如何打包SpringBoot工程
目录 一、spring-boot-maven-plugin详解 1、添加spring-boot-maven-plugin插件到pom.xml 2、配置主类(Main Class) 3、配置打包的JAR文件名 4、包含或排除特定的资源文件 5、指定额外的依赖项 6、配置运行参数 7、自定义插件执行阶段 二、Maven打…...
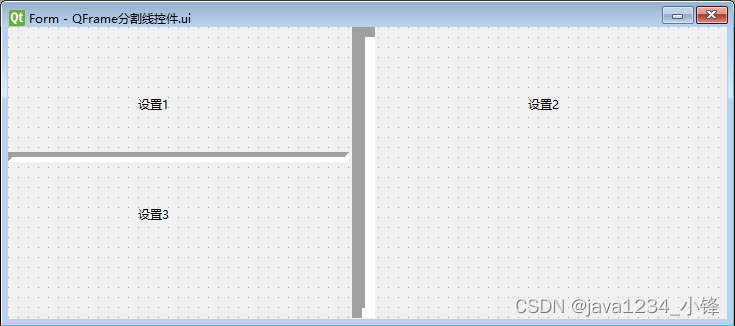
PyQt6 QFrame分割线控件
锋哥原创的PyQt6视频教程: 2024版 PyQt6 Python桌面开发 视频教程(无废话版) 玩命更新中~_哔哩哔哩_bilibili2024版 PyQt6 Python桌面开发 视频教程(无废话版) 玩命更新中~共计46条视频,包括:2024版 PyQt6 Python桌面开发 视频教程(无废话版…...
PostgreSql 序列
一、概述 在 PostgreSQL 中,序列用于生成唯一标识符,通常用于为表的主键列生成连续的唯一值。若目的仅是为表字段设置自增 id,可考虑序列类型来实现,可参考《PostgreSql 设置自增字段》 二、创建序列 2.1 语法 CREATE [ TEMPOR…...

【深度学习目标检测】六、基于深度学习的路标识别(python,目标检测,yolov8)
YOLOv8是一种物体检测算法,是YOLO系列算法的最新版本。 YOLO(You Only Look Once)是一种实时物体检测算法,其优势在于快速且准确的检测结果。YOLOv8在之前的版本基础上进行了一系列改进和优化,提高了检测速度和准确性。…...
后进先出(LIFO)详解
LIFO 是 Last In, First Out 的缩写,中文译为后进先出。这是一种数据结构的工作原则,类似于一摞盘子或一叠书本: 最后放进去的元素最先出来 -想象往筒状容器里放盘子: (1)你放进的最后一个盘子(…...
CVPR 2025 MIMO: 支持视觉指代和像素grounding 的医学视觉语言模型
CVPR 2025 | MIMO:支持视觉指代和像素对齐的医学视觉语言模型 论文信息 标题:MIMO: A medical vision language model with visual referring multimodal input and pixel grounding multimodal output作者:Yanyuan Chen, Dexuan Xu, Yu Hu…...
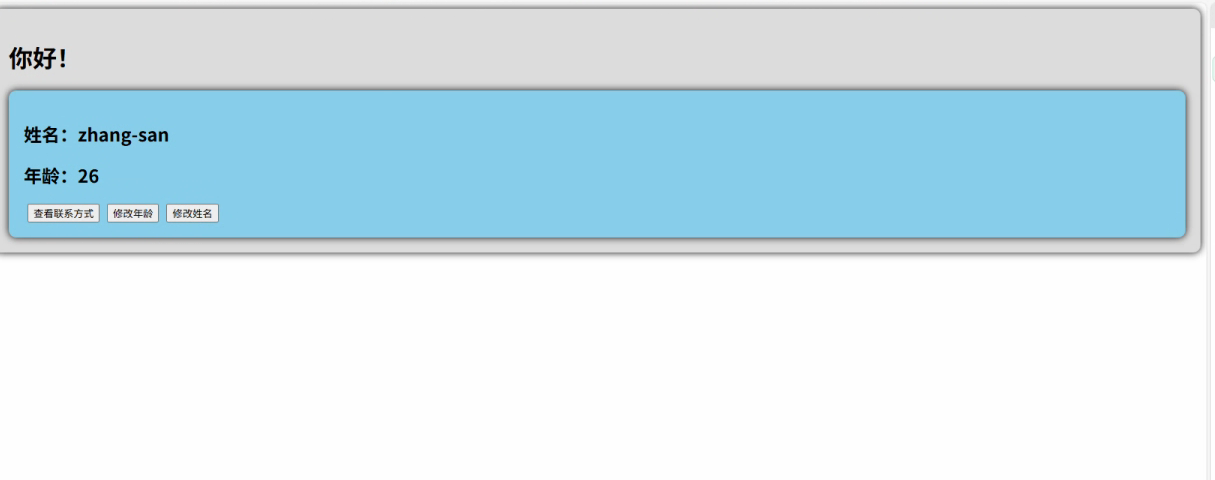
2.Vue编写一个app
1.src中重要的组成 1.1main.ts // 引入createApp用于创建应用 import { createApp } from "vue"; // 引用App根组件 import App from ./App.vue;createApp(App).mount(#app)1.2 App.vue 其中要写三种标签 <template> <!--html--> </template>…...
C# SqlSugar:依赖注入与仓储模式实践
C# SqlSugar:依赖注入与仓储模式实践 在 C# 的应用开发中,数据库操作是必不可少的环节。为了让数据访问层更加简洁、高效且易于维护,许多开发者会选择成熟的 ORM(对象关系映射)框架,SqlSugar 就是其中备受…...
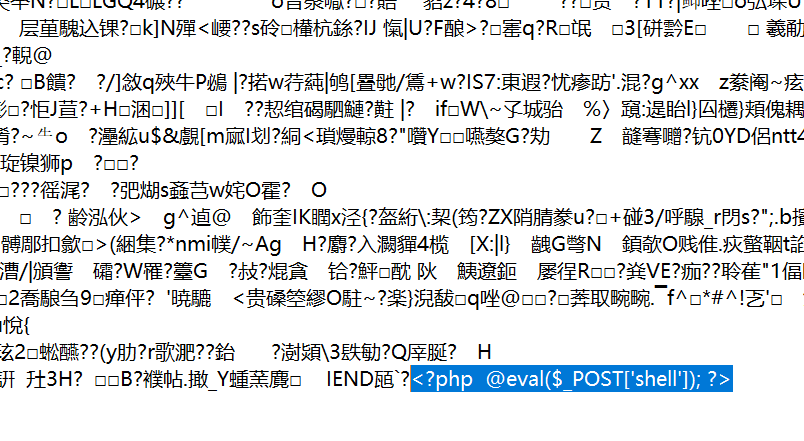
零基础在实践中学习网络安全-皮卡丘靶场(第九期-Unsafe Fileupload模块)(yakit方式)
本期内容并不是很难,相信大家会学的很愉快,当然对于有后端基础的朋友来说,本期内容更加容易了解,当然没有基础的也别担心,本期内容会详细解释有关内容 本期用到的软件:yakit(因为经过之前好多期…...
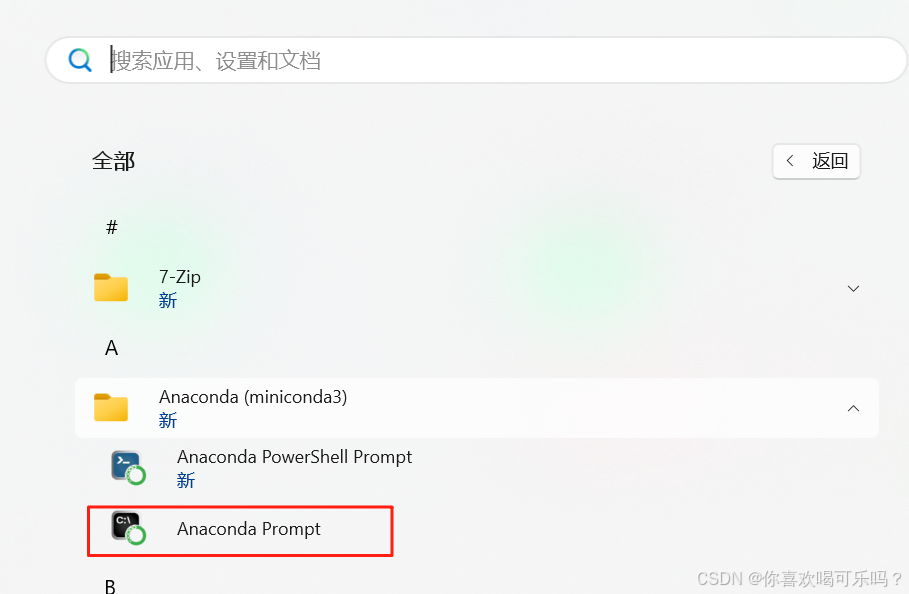
Windows安装Miniconda
一、下载 https://www.anaconda.com/download/success 二、安装 三、配置镜像源 Anaconda/Miniconda pip 配置清华镜像源_anaconda配置清华源-CSDN博客 四、常用操作命令 Anaconda/Miniconda 基本操作命令_miniconda创建环境命令-CSDN博客...
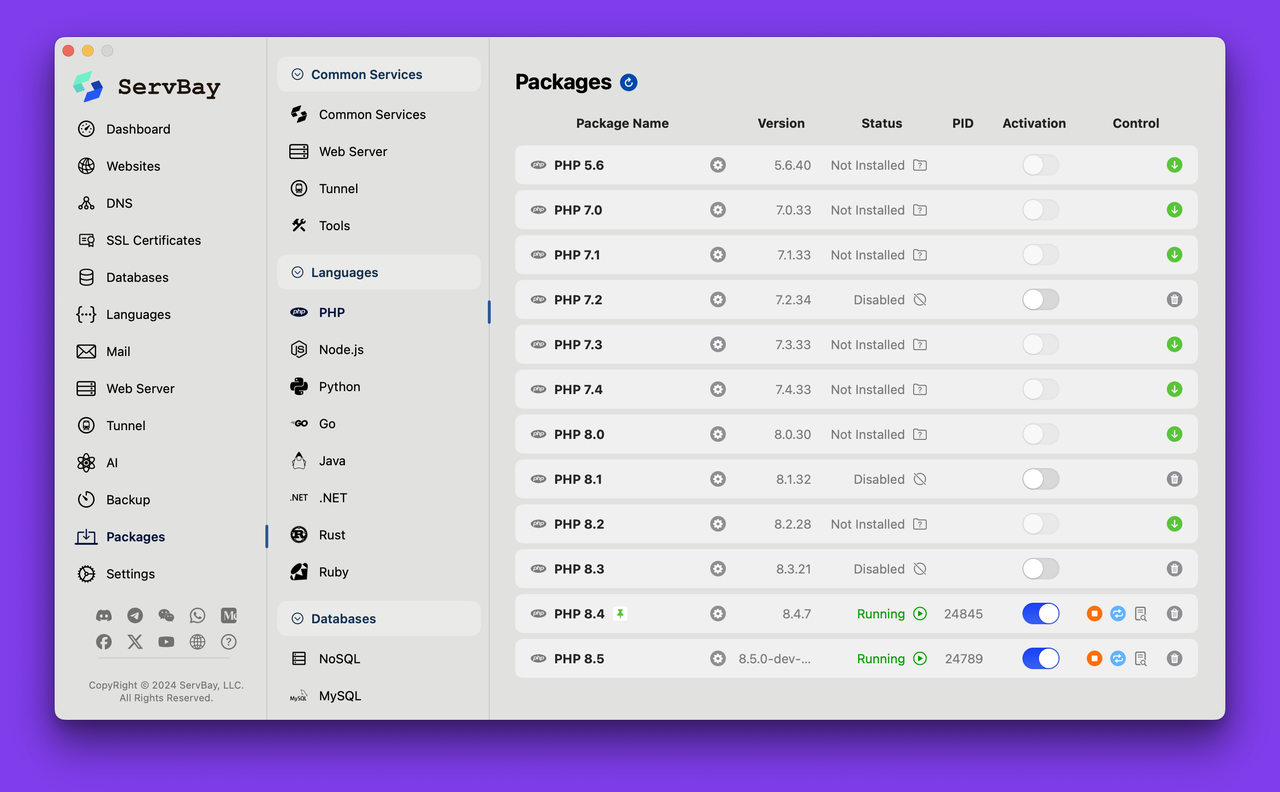
PHP 8.5 即将发布:管道操作符、强力调试
前不久,PHP宣布了即将在 2025 年 11 月 20 日 正式发布的 PHP 8.5!作为 PHP 语言的又一次重要迭代,PHP 8.5 承诺带来一系列旨在提升代码可读性、健壮性以及开发者效率的改进。而更令人兴奋的是,借助强大的本地开发环境 ServBay&am…...
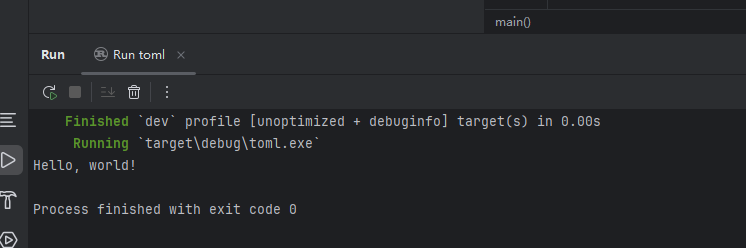
Rust 开发环境搭建
环境搭建 1、开发工具RustRover 或者vs code 2、Cygwin64 安装 https://cygwin.com/install.html 在工具终端执行: rustup toolchain install stable-x86_64-pc-windows-gnu rustup default stable-x86_64-pc-windows-gnu 2、Hello World fn main() { println…...
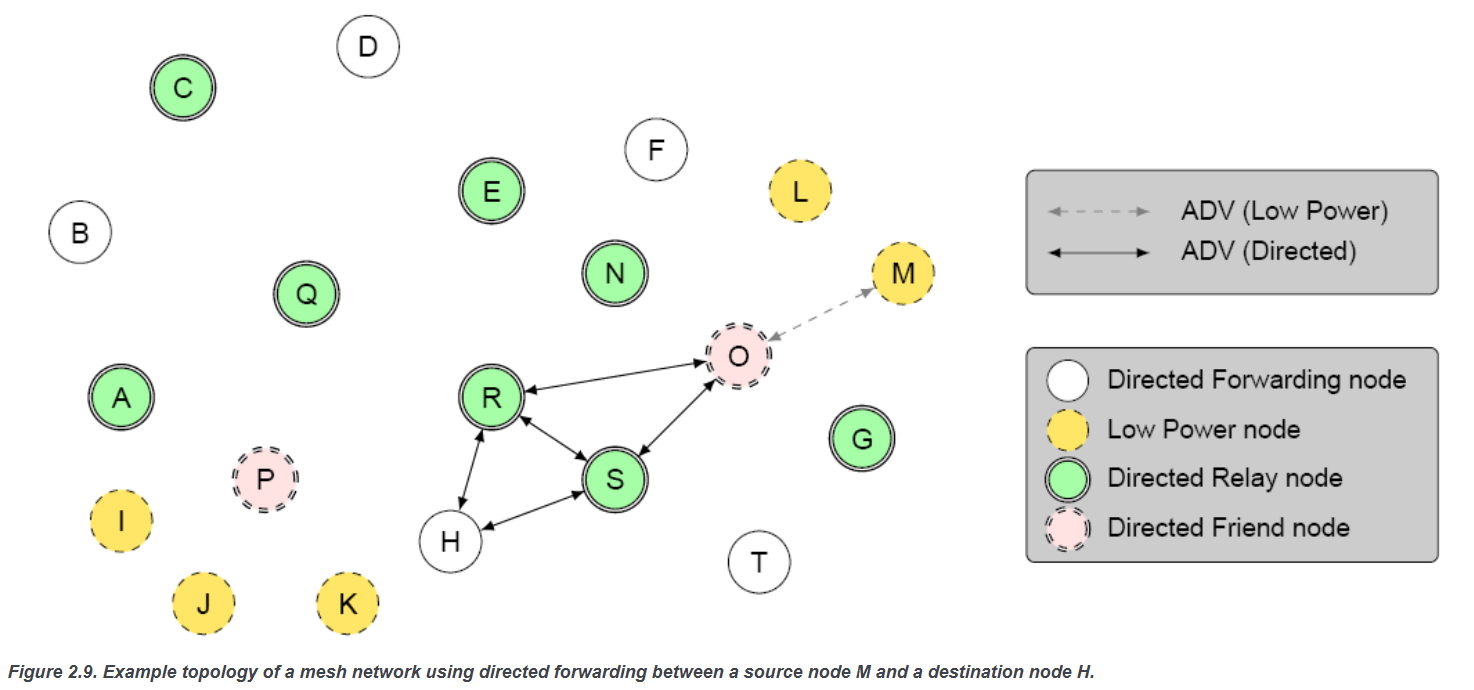
【p2p、分布式,区块链笔记 MESH】Bluetooth蓝牙通信 BLE Mesh协议的拓扑结构 定向转发机制
目录 节点的功能承载层(GATT/Adv)局限性: 拓扑关系定向转发机制定向转发意义 CG 节点的功能 节点的功能由节点支持的特性和功能决定。所有节点都能够发送和接收网格消息。节点还可以选择支持一个或多个附加功能,如 Configuration …...
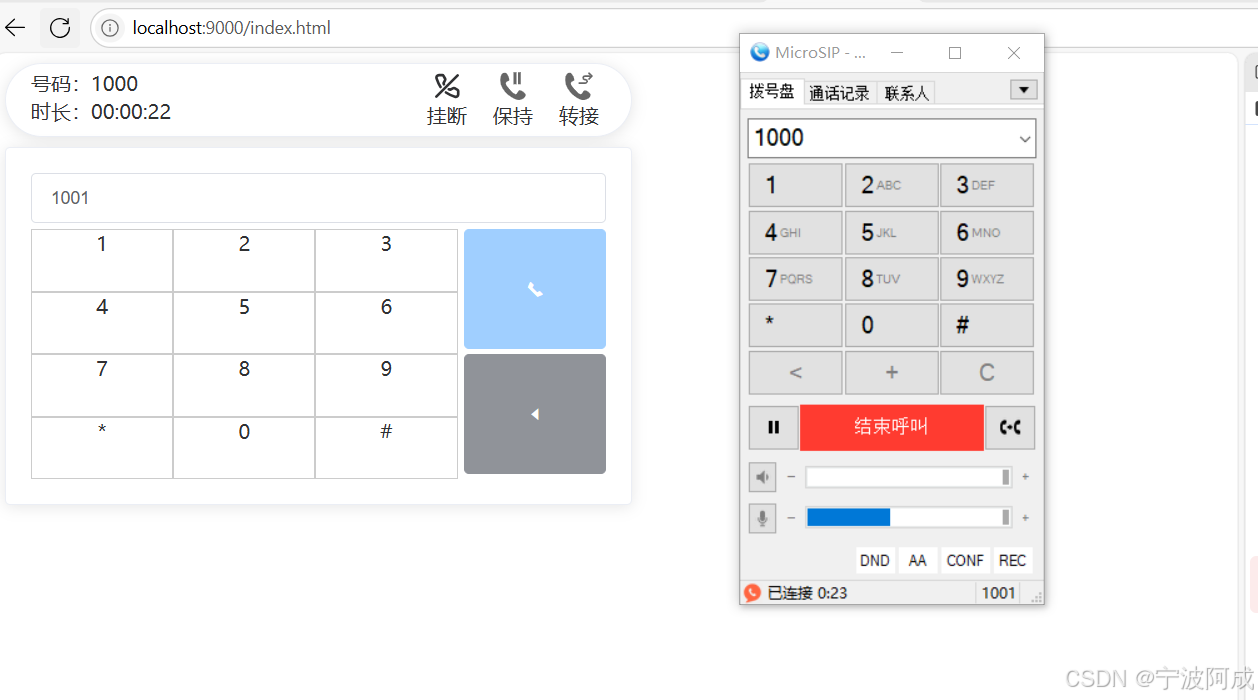
通过MicroSip配置自己的freeswitch服务器进行调试记录
之前用docker安装的freeswitch的,启动是正常的, 但用下面的Microsip连接不上 主要原因有可能一下几个 1、通过下面命令可以看 [rootlocalhost default]# docker exec -it freeswitch fs_cli -x "sofia status profile internal"Name …...