超级seo外链/镇江百度关键词优化
openssl crl2pkcs7 -nocrl -certfile /etc/ssl/certs/ca-certificates.crt | openssl pkcs7 -print_certs -noout -text
ca-certificates.crt为操作系统根证书列表。
获取证书以后使用PK11_ImportDERCert将证书导入到nssdb中
base::FilePath cert_path = base::FilePath("/etc/ssl/certs/ca-certificates.crt");std::string cert_data;if (base::ReadFileToString(cert_path, &cert_data)){base::span<const uint8_t> datas = base::as_bytes(base::make_span(cert_data));base::StringPiece data_string(reinterpret_cast<const char*>(datas.data()),datas.size());std::vector<std::string> pem_headers;// To maintain compatibility with NSS/Firefox, CERTIFICATE is a universally// valid PEM block header for any format.pem_headers.push_back(kCertificateHeader);pem_headers.push_back(kPKCS7Header);PEMTokenizer pem_tokenizer(data_string, pem_headers);int i = 0;while (pem_tokenizer.GetNext()) {std::string decoded(pem_tokenizer.data());LOG(INFO)<<decoded;SECItem certData;certData.data = reinterpret_cast<unsigned char*>(const_cast<char*>(decoded.c_str()));certData.len = decoded.size();certData.type = siDERCertBuffer;std::string name = "cert"+std::to_string(i);std::string fileName = "/home/arv000/Desktop/cc/"+name;std::ofstream outFile(fileName);if (outFile.is_open()) {// 写入字符串到文件outFile << decoded;// 关闭文件流outFile.close();}SECStatus status = PK11_ImportDERCert(slot, &certData, CK_INVALID_HANDLE ,const_cast<char*>(name.c_str()) /* is_perm */, PR_TRUE /* copyDER */);i++;}}
// Copyright (c) 2010 The Chromium Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.#include "crypto/cert/pem.h"#include "base/base64.h"
#include "base/strings/string_piece.h"
#include "base/strings/string_util.h"
#include "base/strings/stringprintf.h"namespace {const char kPEMSearchBlock[] = "-----BEGIN ";
const char kPEMBeginBlock[] = "-----BEGIN %s-----";
const char kPEMEndBlock[] = "-----END %s-----";} // namespacenamespace crypto {using base::StringPiece;struct PEMTokenizer::PEMType {std::string type;std::string header;std::string footer;
};PEMTokenizer::PEMTokenizer(const StringPiece& str,const std::vector<std::string>& allowed_block_types) {Init(str, allowed_block_types);
}PEMTokenizer::~PEMTokenizer() = default;bool PEMTokenizer::GetNext() {while (pos_ != StringPiece::npos) {// Scan for the beginning of the next PEM encoded block.pos_ = str_.find(kPEMSearchBlock, pos_);if (pos_ == StringPiece::npos)return false; // No more PEM blocksstd::vector<PEMType>::const_iterator it;// Check to see if it is of an acceptable block type.for (it = block_types_.begin(); it != block_types_.end(); ++it) {if (!base::StartsWith(str_.substr(pos_), it->header))continue;// Look for a footer matching the header. If none is found, then all// data following this point is invalid and should not be parsed.StringPiece::size_type footer_pos = str_.find(it->footer, pos_);if (footer_pos == StringPiece::npos) {pos_ = StringPiece::npos;return false;}// Chop off the header and footer and parse the data in between.StringPiece::size_type data_begin = pos_ + it->header.size();pos_ = footer_pos + it->footer.size();block_type_ = it->type;StringPiece encoded = str_.substr(data_begin, footer_pos - data_begin);if (!base::Base64Decode(base::CollapseWhitespaceASCII(encoded, true),&data_)) {// The most likely cause for a decode failure is a datatype that// includes PEM headers, which are not supported.break;}return true;}// If the block did not match any acceptable type, move past it and// continue the search. Otherwise, |pos_| has been updated to the most// appropriate search position to continue searching from and should not// be adjusted.if (it == block_types_.end())pos_ += sizeof(kPEMSearchBlock);}return false;
}void PEMTokenizer::Init(const StringPiece& str,const std::vector<std::string>& allowed_block_types) {str_ = str;pos_ = 0;// Construct PEM header/footer strings for all the accepted types, to// reduce parsing later.for (auto it = allowed_block_types.begin(); it != allowed_block_types.end();++it) {PEMType allowed_type;allowed_type.type = *it;allowed_type.header = base::StringPrintf(kPEMBeginBlock, it->c_str());allowed_type.footer = base::StringPrintf(kPEMEndBlock, it->c_str());block_types_.push_back(allowed_type);}
}std::string PEMEncode(base::StringPiece data, const std::string& type) {std::string b64_encoded;base::Base64Encode(data, &b64_encoded);// Divide the Base-64 encoded data into 64-character chunks, as per// 4.3.2.4 of RFC 1421.static const size_t kChunkSize = 64;size_t chunks = (b64_encoded.size() + (kChunkSize - 1)) / kChunkSize;std::string pem_encoded;pem_encoded.reserve(// header & footer17 + 15 + type.size() * 2 +// encoded datab64_encoded.size() +// newline characters for line wrapping in encoded datachunks);pem_encoded = "-----BEGIN ";pem_encoded.append(type);pem_encoded.append("-----\n");for (size_t i = 0, chunk_offset = 0; i < chunks;++i, chunk_offset += kChunkSize) {pem_encoded.append(b64_encoded, chunk_offset, kChunkSize);pem_encoded.append("\n");}pem_encoded.append("-----END ");pem_encoded.append(type);pem_encoded.append("-----\n");return pem_encoded;
}} // namespace net
// Copyright (c) 2011 The Chromium Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.#ifndef NET_CERT_PEM_H_
#define NET_CERT_PEM_H_#include <stddef.h>#include <string>
#include <vector>#include "base/macros.h"
#include "base/strings/string_piece.h"namespace crypto {// PEMTokenizer is a utility class for the parsing of data encapsulated
// using RFC 1421, Privacy Enhancement for Internet Electronic Mail. It
// does not implement the full specification, most notably it does not
// support the Encapsulated Header Portion described in Section 4.4.
class PEMTokenizer {public:// Create a new PEMTokenizer that iterates through |str| searching for// instances of PEM encoded blocks that are of the |allowed_block_types|.// |str| must remain valid for the duration of the PEMTokenizer.PEMTokenizer(const base::StringPiece& str,const std::vector<std::string>& allowed_block_types);~PEMTokenizer();// Attempts to decode the next PEM block in the string. Returns false if no// PEM blocks can be decoded. The decoded PEM block will be available via// data().bool GetNext();// Returns the PEM block type (eg: CERTIFICATE) of the last successfully// decoded PEM block.// GetNext() must have returned true before calling this method.const std::string& block_type() const { return block_type_; }// Returns the raw, Base64-decoded data of the last successfully decoded// PEM block.// GetNext() must have returned true before calling this method.const std::string& data() const { return data_; }private:void Init(const base::StringPiece& str,const std::vector<std::string>& allowed_block_types);// A simple cache of the allowed PEM header and footer for a given PEM// block type, so that it is only computed once.struct PEMType;// The string to search, which must remain valid for as long as this class// is around.base::StringPiece str_;// The current position within |str_| that searching should begin from,// or StringPiece::npos if iteration is completebase::StringPiece::size_type pos_;// The type of data that was encoded, as indicated in the PEM// Pre-Encapsulation Boundary (eg: CERTIFICATE, PKCS7, or// PRIVACY-ENHANCED MESSAGE).std::string block_type_;// The types of PEM blocks that are allowed. PEM blocks that are not of// one of these types will be skipped.std::vector<PEMType> block_types_;// The raw (Base64-decoded) data of the last successfully decoded block.std::string data_;DISALLOW_COPY_AND_ASSIGN(PEMTokenizer);
};// Encodes |data| in the encapsulated message format described in RFC 1421,
// with |type| as the PEM block type (eg: CERTIFICATE).std::string PEMEncode(base::StringPiece data,const std::string& type);} // namespace net#endif // NET_CERT_PEM_H_
相关文章:

ca-certificates.crt解析加载到nssdb中
openssl crl2pkcs7 -nocrl -certfile /etc/ssl/certs/ca-certificates.crt | openssl pkcs7 -print_certs -noout -text ca-certificates.crt为操作系统根证书列表。 获取证书以后使用PK11_ImportDERCert将证书导入到nssdb中 base::FilePath cert_path base::FilePath("…...
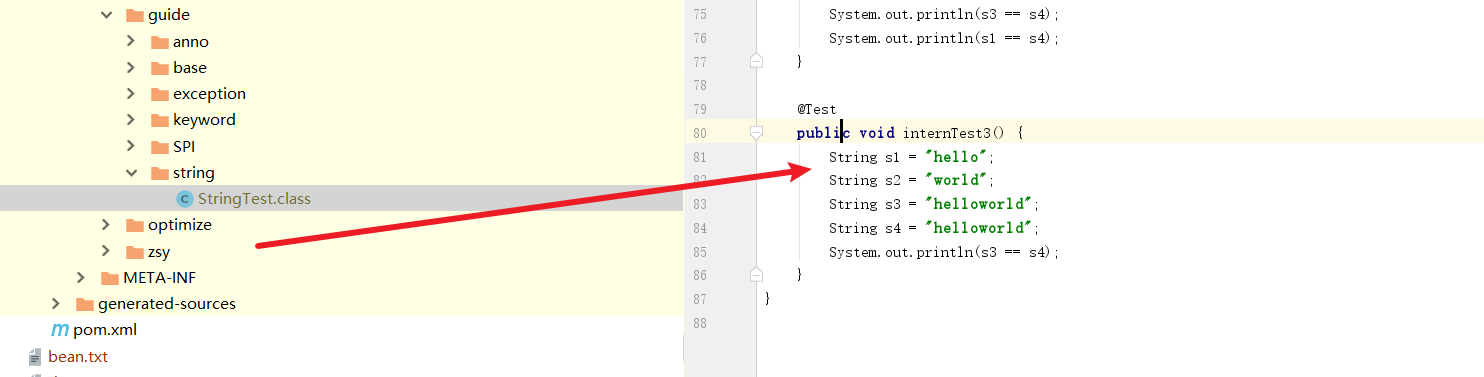
聊聊Java中的常用类String
String、StringBuffer、StringBuilder 的区别 从可变性分析 String不可变。StringBuffer、StringBuilder都继承自AbstractStringBuilder ,两者的底层的数组value并没有使用private和final修饰,所以是可变的。 AbstractStringBuilder 源码如下所示 ab…...

R语言piecewiseSEM结构方程模型在生态环境领域实践技术
结构方程模型(Sructural Equation Modeling,SEM)可分析系统内变量间的相互关系,并通过图形化方式清晰展示系统中多变量因果关系网,具有强大的数据分析功能和广泛的适用性,是近年来生态、进化、环境、地学、…...
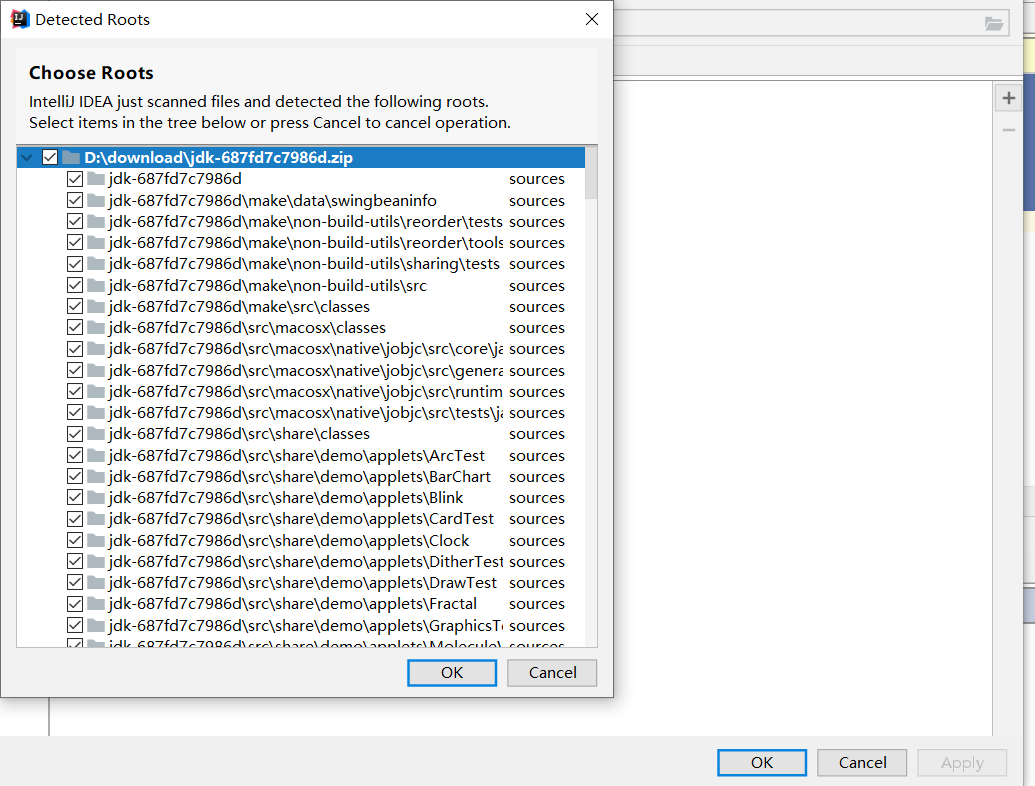
IDEA设置查看JDK源码
问题 我们在查看JDK源码时,可能会遇到这种情况,步入底层查看JDK源码时,出现一堆var变量,可读性非常之差,例如笔者最近想看到nio包下的SocketChannelImpl的write方法,结果看到这样一番景象: pu…...

SSM—Mybatis
目录 和其它持久化层技术对比 搭建MyBatis 开发环境 创建maven工程 创建MyBatis的核心配置文件 创建mapper接口 创建MyBatis的映射文件 通过junit测试功能 加入log4j日志功能 核心配置文件详解 MyBatis的增删改查 新增 删除 修改 查询一个实体类对象 查询list集…...

MYSQL在不删除数据的情况下,重置主键自增id
MYSQL在不删除数据的情况下,重置主键自增id 方法一: SET num : 0; UPDATE table_name SET id num : (num1); ALTER TABLE table_name AUTO_INCREMENT 1; 方法二: 背景(mysql 数据在进行多次删除新增之后id变得很大,但是并没…...

SpringMVC-servlet交互
servlet交互 1.1 引入servlet依赖 <dependency><groupId>javax.servlet</groupId><artifactId>javax.servlet-api</artifactId><version>4.0.1</version><scope>provided</scope></dependency>1.2 创建testservl…...

DICOM 文件中,VR,VL,SQ,图像二进制的几个注意点
DICOM 文件的结构,在网上有很多的学习资料,这里只介绍些容易混淆的概念,作为回看笔记。 1. 传输语法 每个传输语法,起都是表达的三个概念:大小端、显隐式、压缩算法 DICOM Implicit VR Little Endian: 1.2.840.1000…...
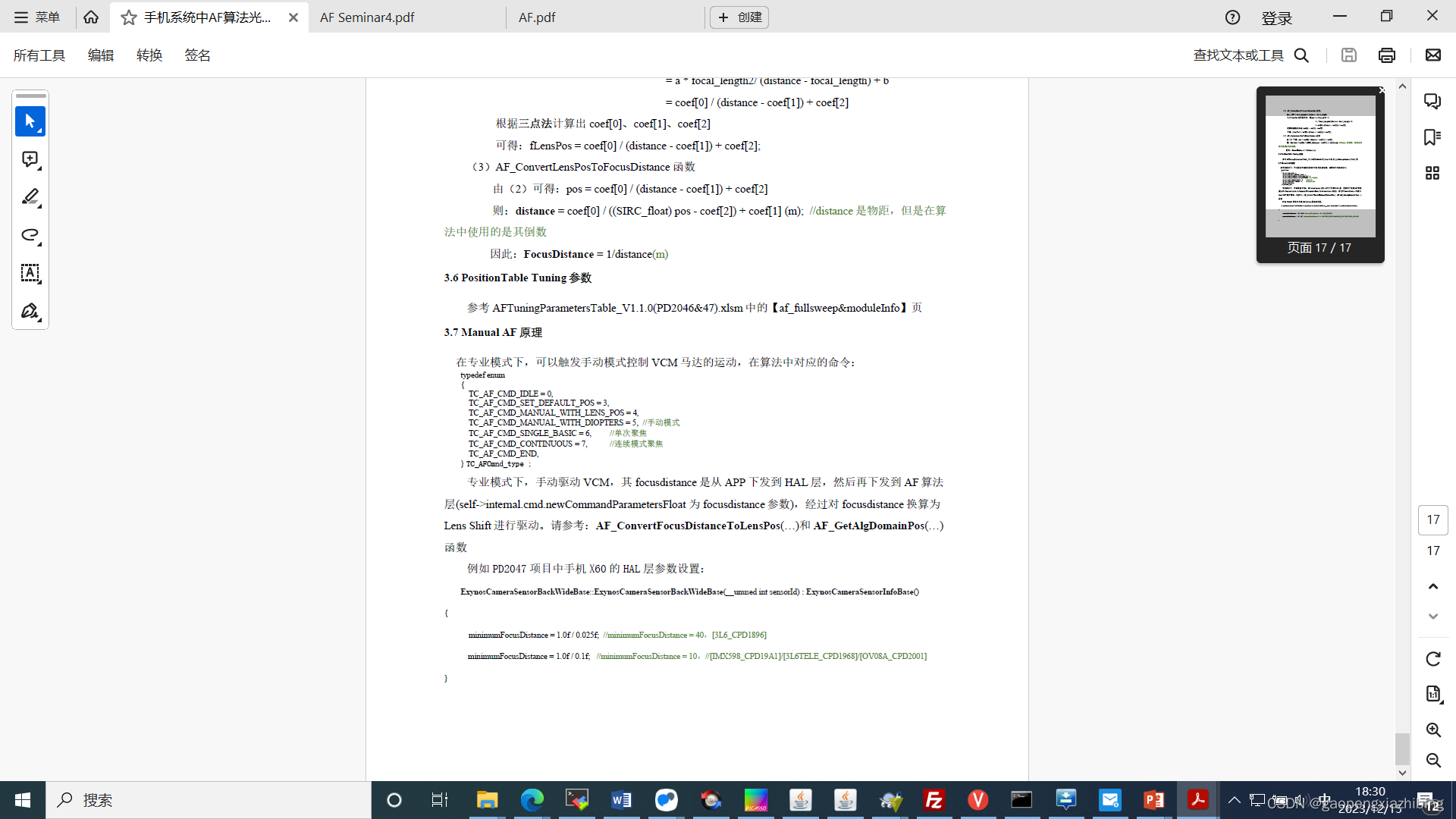
git 的使用
git reset详解-CSDN博客 git reset 命令详解 git revert命令详解。-CSDN博客 关于Git分支中HEAD和Master的理解 - 知乎 (zhihu.com) 一文带你精通 Git(Git 安装与使用、Git 命令精讲、项目的推送与克隆)-CSDN博客 Git 常用操作(5ÿ…...
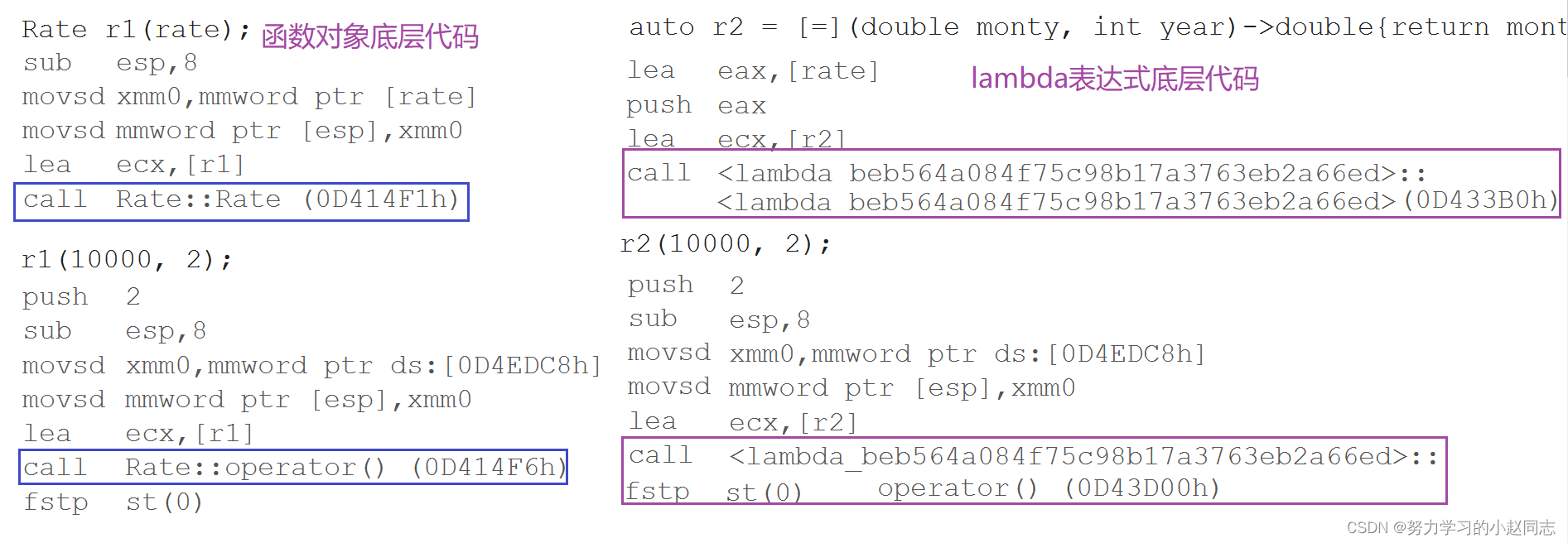
详解—【C++】lambda表达式
目录 前言 一、lambda表达式 二、lambda表达式语法 2.1. lambda表达式各部分说明 2.2. 捕获列表说明 三、函数对象与lambda表达式 前言 在C98中,如果想要对一个数据集合中的元素进行排序,可以使用std::sort方法。 #include <algorithm> #i…...
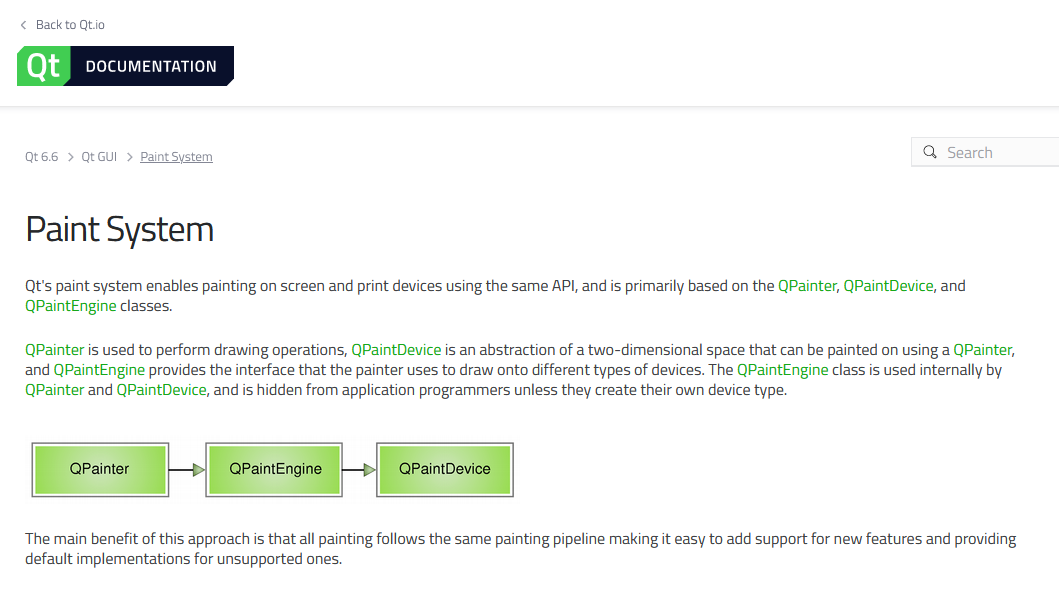
Qt Desktop Widgets 控件绘图原理逐步分析拆解
Qt 是目前C语言首选的框架库。之所以称为框架库而不单单是GUI库,是因为Qt提供了远远超过GUI的功能封装,即使不使用GUI的后台服务,也可以用Qt大大提高跨平台的能力。 仅就界面来说,Qt 保持各个平台绘图等效果的统一,并…...
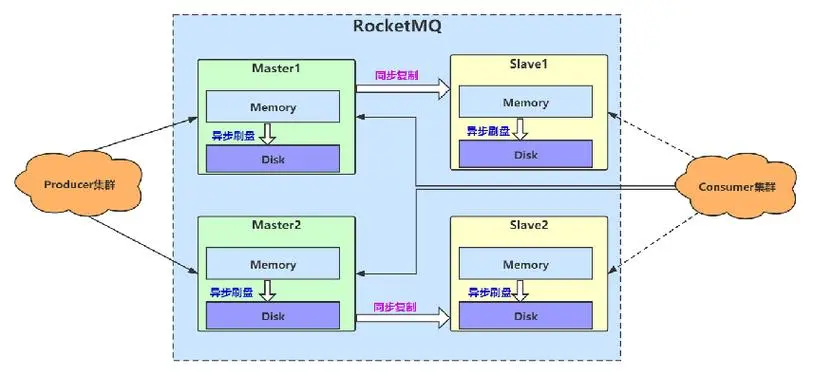
什么是rocketmq❓
在大规模分布式系统中,各个服务之间的通信是至关重要的,而RocketMQ作为一款分布式消息中间件,为解决这一问题提供了强大的解决方案。本文将深入探讨RocketMQ的基本概念、用途,以及在实际分布式系统中的作用,并对Produc…...
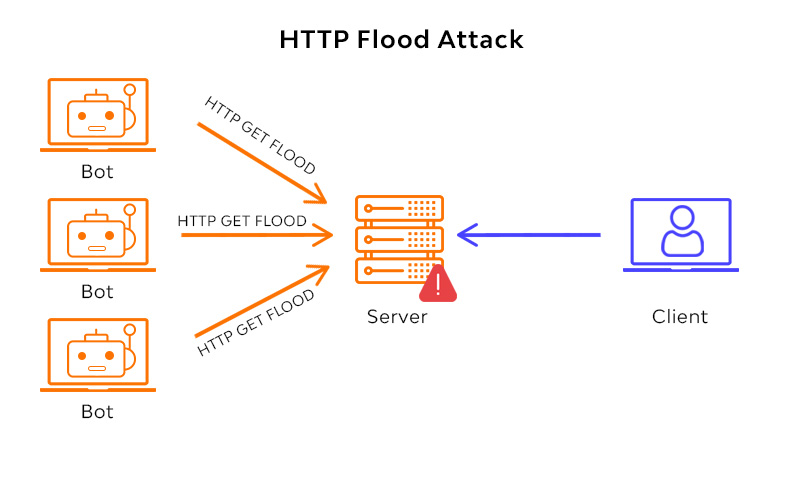
【网络安全】HTTP Slowloris攻击原理解析
文章目录 Slowloris攻击的概念Slowloris攻击原理Slowloris攻击的步骤其他的DDoS攻击类型UDP FloodICMP (Ping) FloodSYN FloodPing of DeathNTP AmplificationHTTP FloodZero-day DDoS 攻击 推荐阅读 Slowloris攻击的概念 Slowloris是在2009年由著名Web安全专家RSnake提出的一…...
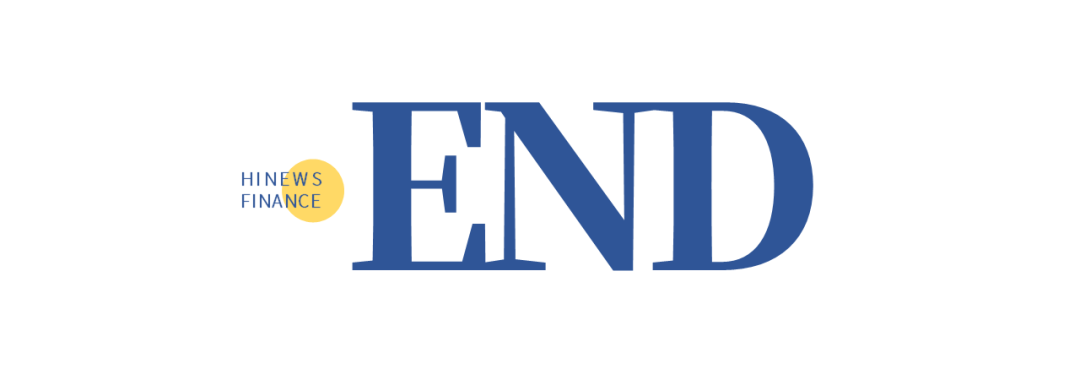
从最近爆火的ChatGPT,我看到了电商的下一个形态
爆火的ChatGPT似乎让每个行业有了改造的可能性,电商行业也不例外。 在讨论了很多流量红利消失的话题后,我们看到互联网电商行业不再性感,从淘宝天猫,京东,到拼多多,再到抖音,快手,电…...
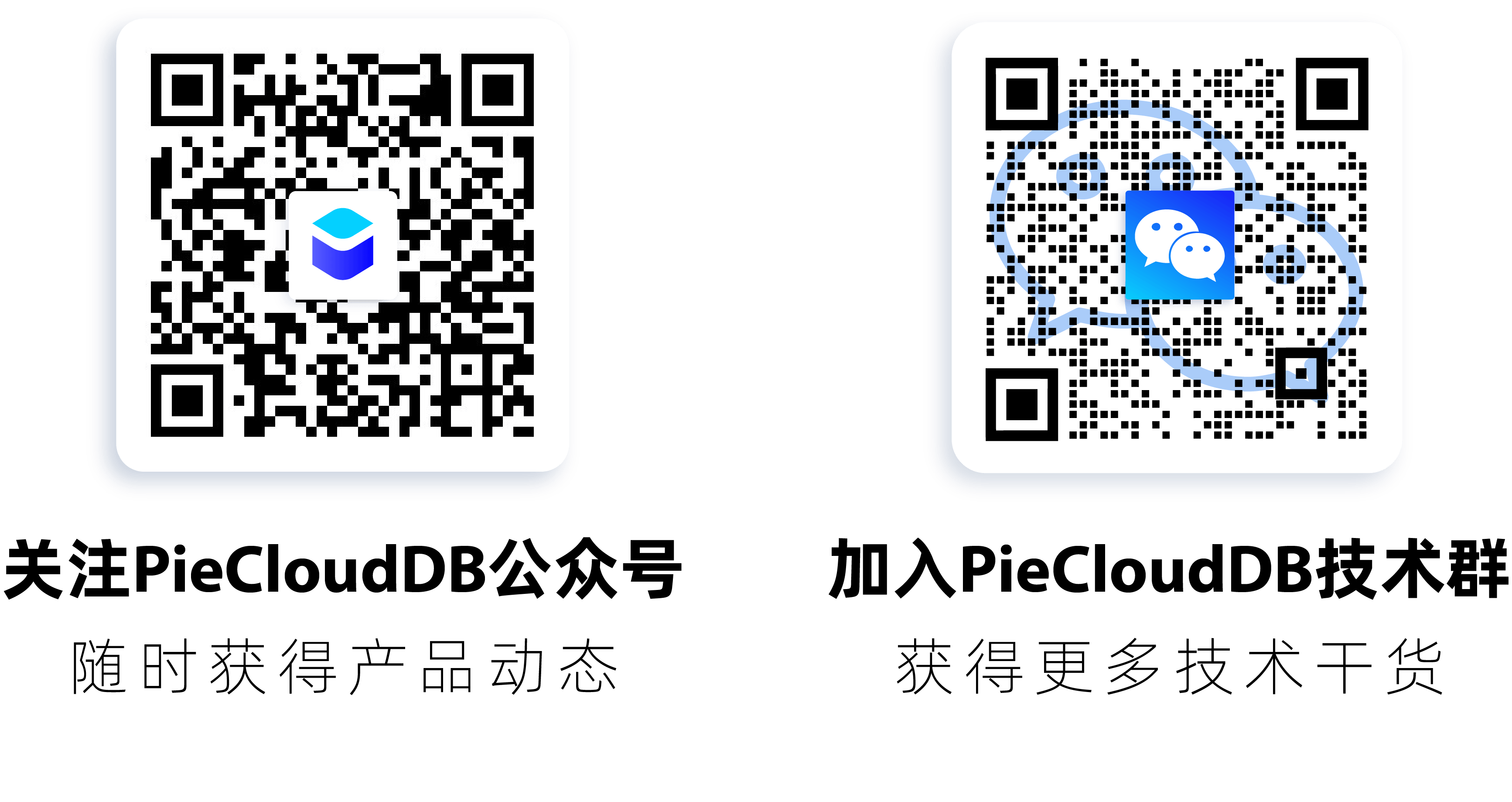
云原生向量计算引擎 PieCloudVector:为大模型提供独特记忆
拓数派大模型数据计算系统(PieDataComputingSystem,缩写:πDataCS)在10月24日程序员节「大模型数据计算系统」2023拓数派年度技术论坛正式发布。πDataCS 以云原生技术重构数据存储和计算,「一份存储,多引擎…...
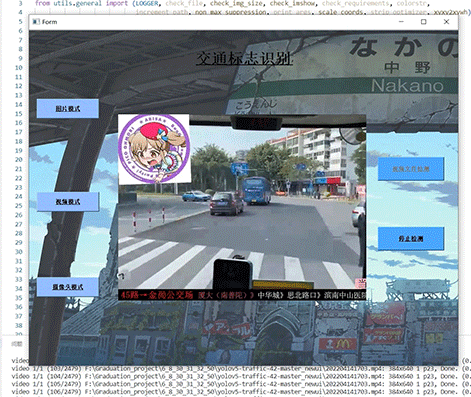
大创项目推荐 深度学习 opencv python 实现中国交通标志识别
文章目录 0 前言1 yolov5实现中国交通标志检测2.算法原理2.1 算法简介2.2网络架构2.3 关键代码 3 数据集处理3.1 VOC格式介绍3.2 将中国交通标志检测数据集CCTSDB数据转换成VOC数据格式3.3 手动标注数据集 4 模型训练5 实现效果5.1 视频效果 6 最后 0 前言 🔥 优质…...
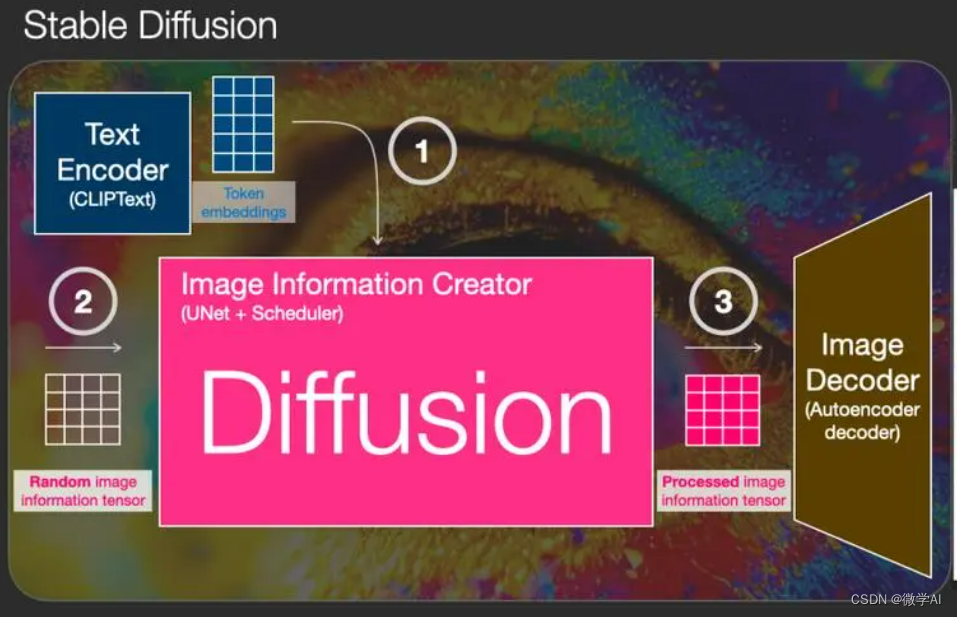
深度学习实战67-基于Stable-diffusion的图像生成应用模型的搭建,在Kaggle平台的搭建部署,解决本地没有算力资源问题
大家好,我是微学AI,今天给大家介绍一下深度学习实战67-基于Stable-diffusion的图像生成应用模型的搭建,在Kaggle平台的搭建部署,解决本地没有算力资源问题。稳定扩散模型(Stable Diffusion Model)是一种用于图像增强和去噪的计算机视觉算法。它通过对输入图像进行扩散过程…...
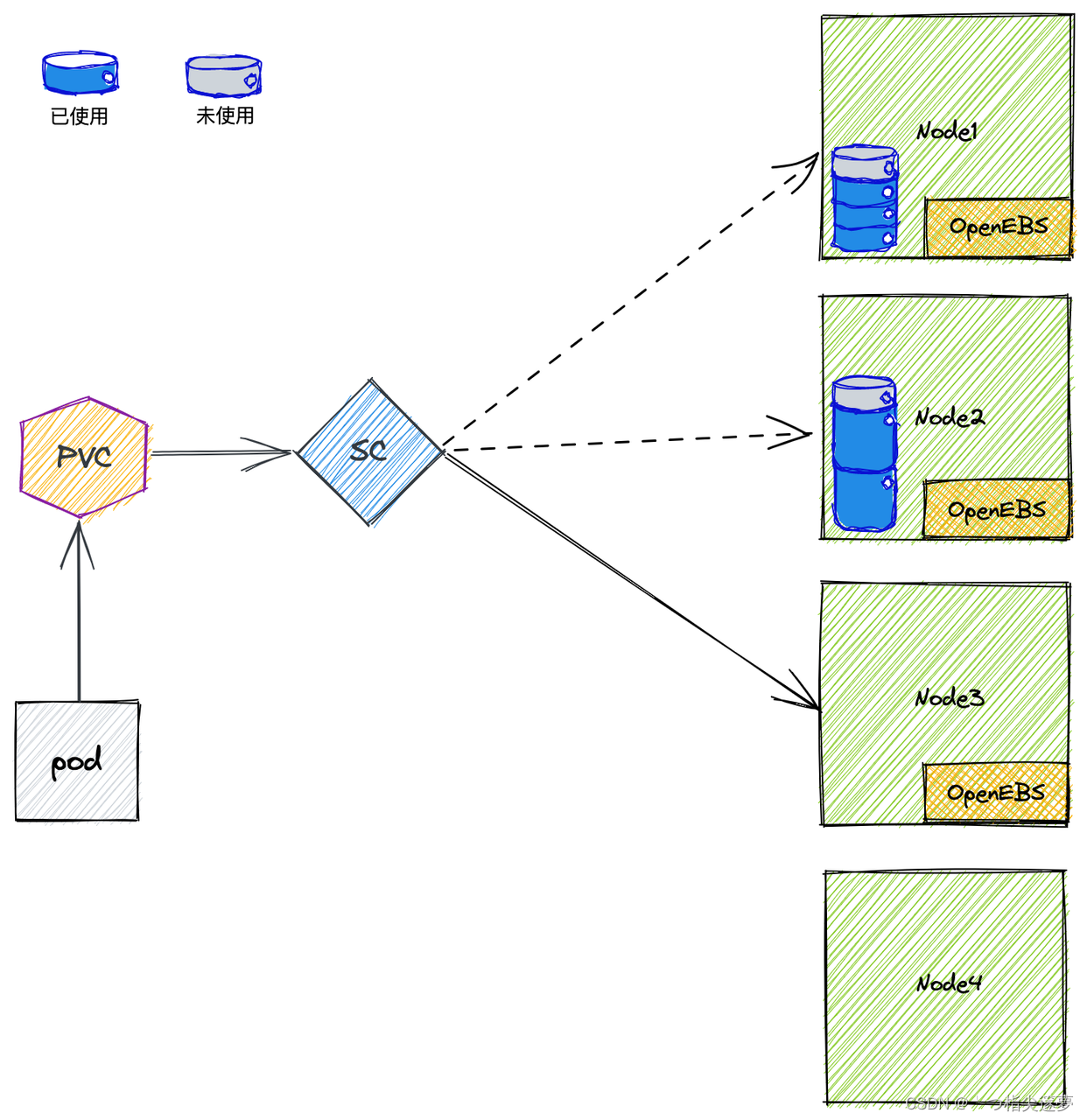
云原生之深入解析Kubernetes本地持久化存储方案OpenEBS LocalPV的最佳实践
一、K8s 本地存储 K8s 支持多达 20 种类型的持久化存储,如常见的 CephFS 、Glusterfs 等,不过这些大都是分布式存储,随着社区的发展,越来越多的用户期望将 K8s 集群中工作节点上挂载的数据盘利用起来,于是就有了 loca…...
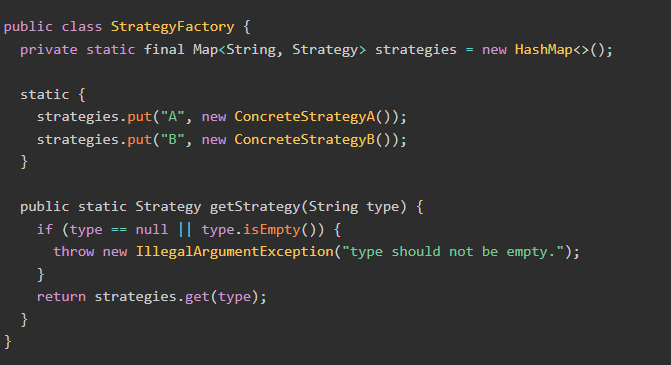
设计模式-策略(Strategy)模式
又被称为政策(方针)模式策略模式(Strategy Design Pattern):封装可以互换的行为,并使用委托来决定要使用哪一个策略模式是一种行为设计模式,它能让你定义一系列算法,并将每种算法分别放入独立的类中&#x…...
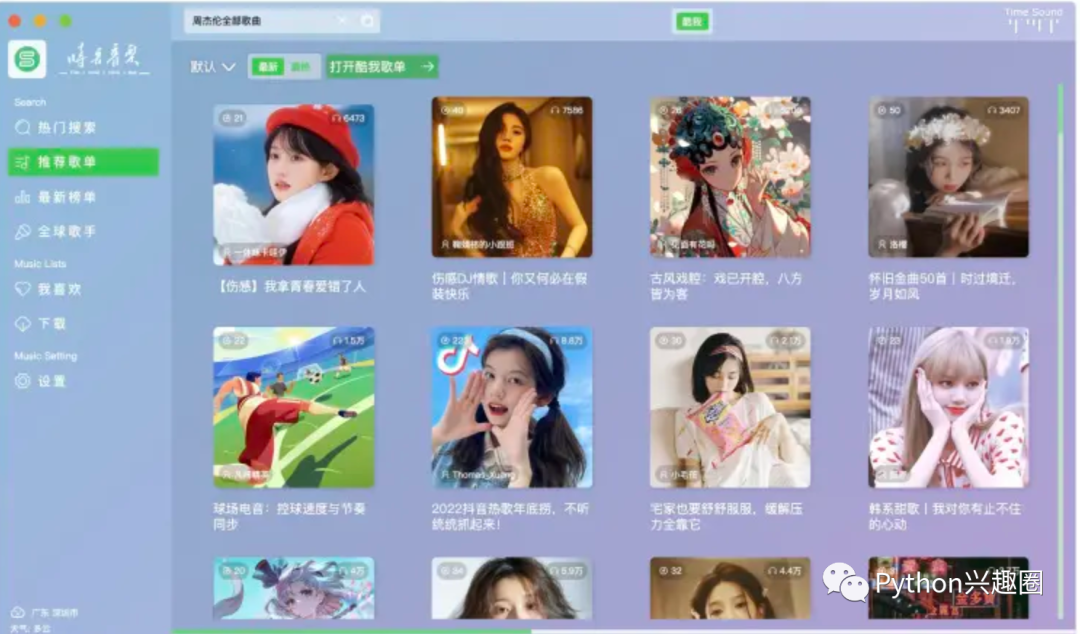
Star 4.1k!Gitee GVP开源项目!新一代桌面应用开发框架 ElectronEgg!
前言 随着现代技术的快速升级迭代及发展,桌面应用开发已经变得越来越普及。然而对于非专业桌面应用开发工程师在面对这项任务时,可能会感到无从下手,甚至觉得这是一项困难的挑战。 本篇文章将分享一种新型桌面应用开发框架 ElectronEgg&…...
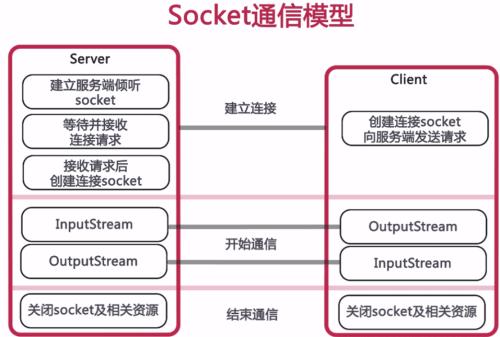
node.js学习(简单聊天室)
在掘金查看该文章 1. TCP服务搭建 1.1 socket 先来粗略了解下socket 套接字(socket)是一个抽象层,应用程序可以通过它发送或接收数据,可对其进行像对文件一样的打开、读写和关闭等操作。套接字允许应用程序将I/O插入到网络中&am…...

cfa一级考生复习经验分享系列(四)
备考CFA一级满打满算用了一个多月,每天八个小时以上。可能如果仅以通过为目标的话完全不用这样,看过太多类似于只看了一周就通过了考试又或是放弃了好几门飘过了考试的情况,我觉得这是不正确的考试状态,完全不必惊叹,踏…...
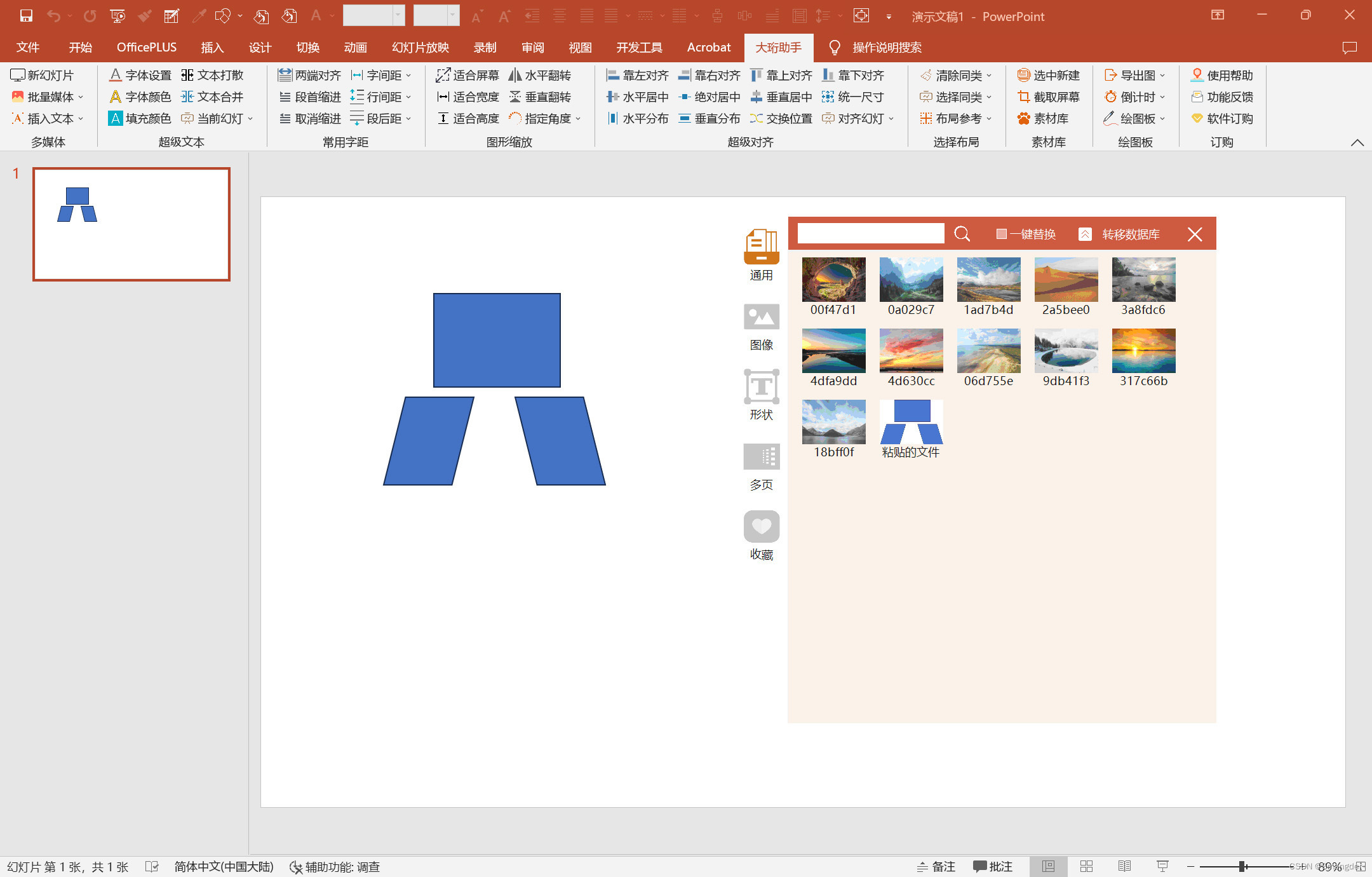
PPT插件-好用的插件-放映笔、绘图板-大珩助手
放映笔 幻灯片放映时,工具在幻灯片的左下方,本工具在幻灯片的右侧,可以移动,可以方便在右侧讲课时候使用 绘图板 可在绘图板上写签名、绘制图画、写字等等,点画笔切换橡皮擦,点插入绘图,将背景…...
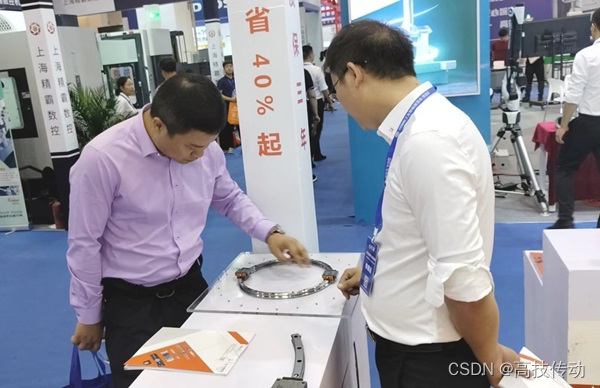
弧形导轨的安装注意事项
随着弧形导轨的应用日渐普遍,在日常使用中总会遇到很多各种各样的问题,原因很多是安装不正确或者使用不恰当。不合理的使用不但不能充分发挥其价值还会导致使用寿命大打折扣,使企业造成不必要的损失,因此大伙有必要了解一些安装的…...
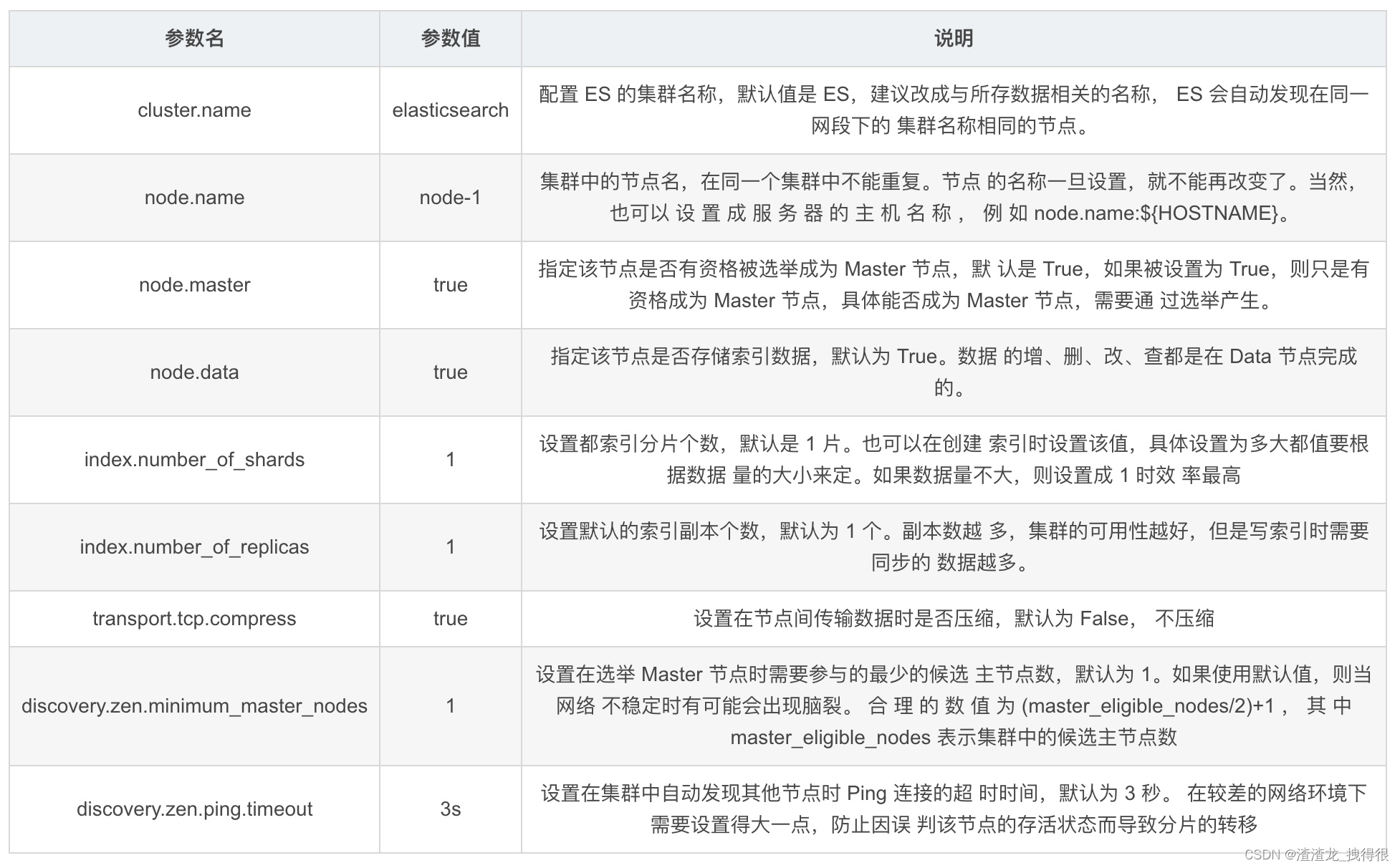
Elasticsearch优化-04
Elasticsearch优化 1、优化-硬件选择 Elasticsearch 的基础是 Lucene,所有的索引和文档数据是存储在本地的磁盘中,具体的路径可在 ES 的配置文件…/config/elasticsearch.yml中配置,如下: # #Path to directory where to store …...
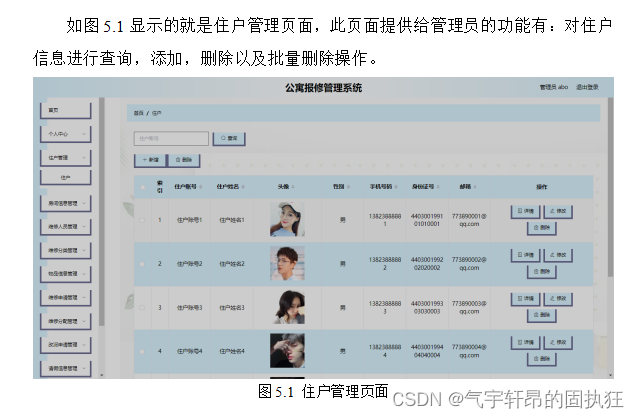
Springboot+vue的公寓报修管理系统(有报告)。Javaee项目,springboot vue前后端分离项目
演示视频: Springbootvue的公寓报修管理系统(有报告)。Javaee项目,springboot vue前后端分离项目 项目介绍: 本文设计了一个基于Springbootvue的前后端分离的公寓报修管理系统,采用M(model&…...

uniapp腾讯地图路线规划
在uniapp中使用腾讯地图进行路线规划需要通过腾讯地图API进行操作。以下是基本的步骤: 在腾讯地图开放平台上注册账号,并创建应用获取API key。 在uniapp的项目中引入腾讯地图API的JS文件,例如在index.html中添加以下代码: <…...

Python 全栈体系【四阶】(五)
第四章 机器学习 三、数据预处理 1. 数据预处理的目的 去除无效数据、不规范数据、错误数据 补齐缺失值 对数据范围、量纲、格式、类型进行统一化处理,更容易进行后续计算 2. 预处理方法 2.1 标准化(均值移除) 让样本矩阵中的每一列的…...

原点处可微问题
文章目录 原点可微问题例例 原点可微问题 lim x → 0 , y → 0 f ( x , y ) − f ( 0 , 0 ) x 2 y 2 \lim\limits_{x\to{0},y\to{0}} \frac{f(x,y)-f(0,0)}{\sqrt{x^2y^2}} x→0,y→0limx2y2 f(x,y)−f(0,0) 0 0 0(1)是函数 f ( x , y ) f(x,y) f(x,y)在 ( 0 , 0 ) (…...
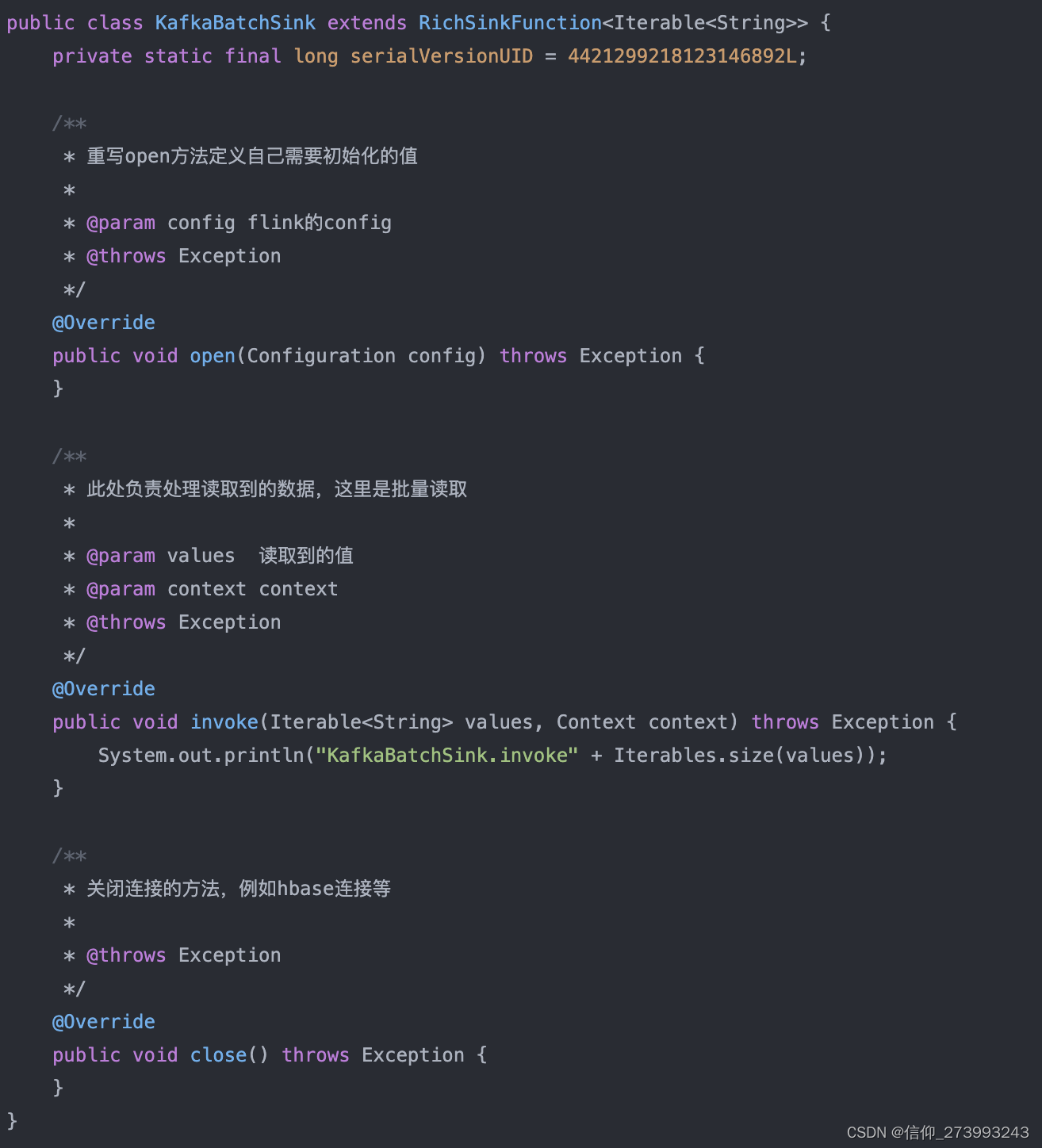
Flink+Kafka消费
引入jar <dependency><groupId>org.apache.flink</groupId><artifactId>flink-java</artifactId><version>1.8.0</version> </dependency> <dependency><groupId>org.apache.flink</groupId><artifactI…...