Python tkinter控件全集之组合选择框 ttk.ComboBox
Tkinter标准库
Tkinter是Python的标准GUI库,也是最常用的Python GUI库之一,提供了丰富的组件和功能,包括窗口、按钮、标签、文本框、列表框、滚动条、画布、菜单等,方便开发者进行图形界面的开发。Tkinter库基于Tk for Unix/Windows/macOS,由Tcl语言编写。使用Tkinter,可以快速创建桌面应用程序,并支持多平台Windows、macOS、Linux等。
tkinter控件全集
在python中导入tkinter库后,有18种控件(也称组件):
导入方式:import tkinter as tk
Button、Canvas、Checkbutton、Entry、Frame、Label、LabelFrame、Listbox、Menu、Menubutton、Message、OptionMenu、PanedWindow、Radiobutton、Scale、Scrollbar、Spinbox、Text
最学见的按钮、文本框、标签、列表框等都在里边,唯独没看到组合框ComboBox。查过资料后才知道,tkinter库中还有一子模块tkinter.ttk,它包含有包括Combobox在内的20种控件:
导入方式:from tkinter import ttk
Button、Checkbutton、Combobox、Entry、Frame、Label、LabelFrame、LabeledScale、Labelframe、Menubutton、Notebook、OptionMenu、PanedWindow、Progressbar、Radiobutton、Scale、Scrollbar、Separator、Sizegrip、Spinbox、Treeview
请注意,某些控件在两个模块中都存在(如Button, Checkbutton, Entry等),但它们在外观和行为上可能会有所不同,ttk模块中的控件可以提供更加现代和可定制的外观。以Button为例,都以简单的方式表达,运行后的外观和行为都有些不同。
import tkinter as tk
from tkinter import ttk
button1 = tk.Button(root, text="Click Me", command=button_click)
button2 = ttk.Button(root, text="Click Me", command=button_click)
如图所示:
完整代码如下:
import tkinter as tk
from tkinter import ttk def button_click(): print("Button clicked!") if __name__=='__main__':root = tk.Tk()root.title("Button vs ttk.Button")root.geometry("400x300")button1 = tk.Button(root, text="Click Me", command=button_click)button1.pack(pady=60)button2 = ttk.Button(root, text="Click Me", command=button_click)button2.pack(pady=20)root.mainloop()
以下将介绍今天的主角:ttk.Combobox 组合选择框。
ComboBox 组合选择框
ComboBox 将几个文本字段组合成为一个可弹出的选择列表。它的原版帮助有3万多字呢,以下挑一些主要的操作方法简单介绍一下:
创建并设置选择项
values = ["Option 1", "Option 2", "Option 3", "Option 4"]
combobox = ttk.Combobox(root, values=values)
combobox.pack()
绑定事件ComboboxSelected
combobox.bind("<<ComboboxSelected>>", on_select)
获取当前选择项combobox.get()
combobox.get()
以上插图的代码如下:
import tkinter as tk
from tkinter import ttkdef on_select(event):label.config(text = "当前选择为:" + combobox.get())if __name__=='__main__':# 创建主窗口root = tk.Tk()root.title("Combobox Example")root.geometry("400x300")label = tk.Label(root, text="请点击下拉框选择:")label.pack()# 创建多选下拉框values = ["Option 1", "Option 2", "Option 3", "Option 4"]combobox = ttk.Combobox(root, values=values)combobox.pack()combobox.bind("<<ComboboxSelected>>", on_select)# 运行主循环root.mainloop()
设置当前显示项combobox.set()
combobox.set()
自定义类 SelectCombobox
把以上内容综合起来,自定义一个组合框类:
import tkinter as tk
from tkinter import ttkclass SelectCombobox(ttk.Combobox):def __init__(self, master=None, values=None):super().__init__(master, values=values)self.bind("<<ComboboxSelected>>", self.on_select)def on_select(self, event):label.config(text = "当前选择为:" + self.get())if __name__=='__main__':# 创建主窗口root = tk.Tk()root.title("Combobox Example")root.geometry("400x300")label = tk.Label(root, text="请点击下拉框选择:")label.pack()# 创建多选下拉框values = ["Option 1", "Option 2", "Option 3", "Option 4"]combobox = SelectCombobox(root, values=values)combobox.pack()# 运行主循环root.mainloop()
扩展Combobox组合框
拓展成多项选择
Combobox本身不支持多项选择,变通方法:引入一个列表,用于存放已选项,奇数次选择一个项则存入列表、反之偶数次选择则移除。
代码如下:
import tkinter as tk
from tkinter import ttkclass MultiSelectCombobox(ttk.Combobox):def __init__(self, master=None, values=None, **kwargs):super().__init__(master, values=values, **kwargs)self.bind("<<ComboboxSelected>>", self.on_select)self.selected_values = []def on_select(self, event):selected_value = self.get() # 使用 get 方法获取选中项的值if selected_value in self.selected_values:self.selected_values.remove(selected_value) # 如果已选中,则移除该选项else:self.selected_values.append(selected_value) # 如果未选中,则添加到已选项列表self.selected_values.sort()if self.selected_values:text = '、'.join(self.selected_values)else:text = '空'label2.config(text = "当前选择为:" + text)if __name__=='__main__':# 创建主窗口root = tk.Tk()root.title("Multi-Select Combobox Example")root.geometry("400x300")label = tk.Label(root, text="请选择:")label.pack()# 创建多选下拉框values = ["Option 1", "Option 2", "Option 3", "Option 4"]combobox = MultiSelectCombobox(root, values=values)combobox.pack()text = "当前选择为:空"label2 = tk.Label(root, text=text)label2.pack()# 运行主循环root.mainloop()
选项前添加符号
Combobox本身不支持修改选择项,变通方法:在每次变更选项时都重建一个新的组合框,同时把所有的选项前都加上一个全角空格(\u3000),对于已选中的选项,则把前面的全角空格替换成对勾符号(\u2713)。
这样子可能更像一个多项选择框,如下图:
代码如下:
import tkinter as tk
from tkinter import ttkdef on_select(event):selected_value = combobox.get()selected_value = selected_value.replace("\u2713","\u3000")if selected_value in selected_values:selected_values.remove(selected_value)else:selected_values.append(selected_value)selected_values.sort()if selected_values:text = '、'.join(selected_values).replace('tion ','')else:text = '空'label.config(text = "当前选择为:" + text)update_combobox_options()def update_combobox_options():global comboboxcombobox.grid_forget()new_values = []for v in values:if v in selected_values:new_values.append(v.replace("\u3000","\u2713"))else:new_values.append(v)combobox = ttk.Combobox(root, values=new_values, state='readonly')combobox.place(x=100, y=30)combobox.set('请点击选择:')combobox.bind("<<ComboboxSelected>>", on_select)if __name__=='__main__':# 创建主窗口root = tk.Tk()root.title("Multi-Select Combobox Example")root.geometry("400x300")# 创建多选下拉框values = ["Option 1", "Option 2", "Option 3", "Option 4"]values = ["\u3000"+v for v in values]selected_values = []combobox = ttk.Combobox(root, values=values, state='readonly')combobox.place(x=100, y=30)combobox.set('请点击选择:')combobox.bind("<<ComboboxSelected>>", on_select)text = "当前选择为:空"label = tk.Label(root, text=text)label.pack(side=tk.BOTTOM)# 运行主循环root.mainloop()
题外话
以上这些是我自己瞎写的变通代码,可能有更好的办法来实现这些功能,也有可能ttk模块本身就有这些功能,只是我没发现而已。另外:如果选择项不至是四个而是有很多,最好能在最前面设置两个选项,全部选择和取消全选,它们不参与进入已选项列表,点击它们只为在其它选项上标注对勾同时填满或清空已选项列表。感兴趣的朋友,可以自己动手实现这两个功能。
附件一
Combobox 英文帮助
Help on class Combobox in module tkinter.ttk:
class Combobox(Entry)
| Combobox(master=None, **kw)
|
| Ttk Combobox widget combines a text field with a pop-down list of values.
|
| Method resolution order:
| Combobox
| Entry
| Widget
| tkinter.Entry
| tkinter.Widget
| tkinter.BaseWidget
| tkinter.Misc
| tkinter.Pack
| tkinter.Place
| tkinter.Grid
| tkinter.XView
| builtins.object
|
| Methods defined here:
|
| __init__(self, master=None, **kw)
| Construct a Ttk Combobox widget with the parent master.
|
| STANDARD OPTIONS
|
| class, cursor, style, takefocus
|
| WIDGET-SPECIFIC OPTIONS
|
| exportselection, justify, height, postcommand, state,
| textvariable, values, width
|
| current(self, newindex=None)
| If newindex is supplied, sets the combobox value to the
| element at position newindex in the list of values. Otherwise,
| returns the index of the current value in the list of values
| or -1 if the current value does not appear in the list.
|
| set(self, value)
| Sets the value of the combobox to value.
|
| ----------------------------------------------------------------------
| Methods inherited from Entry:
|
| bbox(self, index)
| Return a tuple of (x, y, width, height) which describes the
| bounding box of the character given by index.
|
| identify(self, x, y)
| Returns the name of the element at position x, y, or the
| empty string if the coordinates are outside the window.
|
| validate(self)
| Force revalidation, independent of the conditions specified
| by the validate option. Returns False if validation fails, True
| if it succeeds. Sets or clears the invalid state accordingly.
|
| ----------------------------------------------------------------------
| Methods inherited from Widget:
|
| instate(self, statespec, callback=None, *args, **kw)
| Test the widget's state.
|
| If callback is not specified, returns True if the widget state
| matches statespec and False otherwise. If callback is specified,
| then it will be invoked with *args, **kw if the widget state
| matches statespec. statespec is expected to be a sequence.
|
| state(self, statespec=None)
| Modify or inquire widget state.
|
| Widget state is returned if statespec is None, otherwise it is
| set according to the statespec flags and then a new state spec
| is returned indicating which flags were changed. statespec is
| expected to be a sequence.
|
| ----------------------------------------------------------------------
| Methods inherited from tkinter.Entry:
|
| delete(self, first, last=None)
| Delete text from FIRST to LAST (not included).
|
| get(self)
| Return the text.
|
| icursor(self, index)
| Insert cursor at INDEX.
|
| index(self, index)
| Return position of cursor.
|
| insert(self, index, string)
| Insert STRING at INDEX.
|
| scan_dragto(self, x)
| Adjust the view of the canvas to 10 times the
| difference between X and Y and the coordinates given in
| scan_mark.
|
| scan_mark(self, x)
| Remember the current X, Y coordinates.
|
| select_adjust = selection_adjust(self, index)
|
| select_clear = selection_clear(self)
|
| select_from = selection_from(self, index)
|
| select_present = selection_present(self)
|
| select_range = selection_range(self, start, end)
|
| select_to = selection_to(self, index)
|
| selection_adjust(self, index)
| Adjust the end of the selection near the cursor to INDEX.
|
| selection_clear(self)
| Clear the selection if it is in this widget.
|
| selection_from(self, index)
| Set the fixed end of a selection to INDEX.
|
| selection_present(self)
| Return True if there are characters selected in the entry, False
| otherwise.
|
| selection_range(self, start, end)
| Set the selection from START to END (not included).
|
| selection_to(self, index)
| Set the variable end of a selection to INDEX.
|
| ----------------------------------------------------------------------
| Methods inherited from tkinter.BaseWidget:
|
| destroy(self)
| Destroy this and all descendants widgets.
|
| ----------------------------------------------------------------------
| Methods inherited from tkinter.Misc:
|
| __getitem__ = cget(self, key)
|
| __repr__(self)
| Return repr(self).
|
| __setitem__(self, key, value)
|
| __str__(self)
| Return the window path name of this widget.
|
| after(self, ms, func=None, *args)
| Call function once after given time.
|
| MS specifies the time in milliseconds. FUNC gives the
| function which shall be called. Additional parameters
| are given as parameters to the function call. Return
| identifier to cancel scheduling with after_cancel.
|
| after_cancel(self, id)
| Cancel scheduling of function identified with ID.
|
| Identifier returned by after or after_idle must be
| given as first parameter.
|
| after_idle(self, func, *args)
| Call FUNC once if the Tcl main loop has no event to
| process.
|
| Return an identifier to cancel the scheduling with
| after_cancel.
|
| anchor = grid_anchor(self, anchor=None)
|
| bell(self, displayof=0)
| Ring a display's bell.
|
| bind(self, sequence=None, func=None, add=None)
| Bind to this widget at event SEQUENCE a call to function FUNC.
|
| SEQUENCE is a string of concatenated event
| patterns. An event pattern is of the form
| <MODIFIER-MODIFIER-TYPE-DETAIL> where MODIFIER is one
| of Control, Mod2, M2, Shift, Mod3, M3, Lock, Mod4, M4,
| Button1, B1, Mod5, M5 Button2, B2, Meta, M, Button3,
| B3, Alt, Button4, B4, Double, Button5, B5 Triple,
| Mod1, M1. TYPE is one of Activate, Enter, Map,
| ButtonPress, Button, Expose, Motion, ButtonRelease
| FocusIn, MouseWheel, Circulate, FocusOut, Property,
| Colormap, Gravity Reparent, Configure, KeyPress, Key,
| Unmap, Deactivate, KeyRelease Visibility, Destroy,
| Leave and DETAIL is the button number for ButtonPress,
| ButtonRelease and DETAIL is the Keysym for KeyPress and
| KeyRelease. Examples are
| <Control-Button-1> for pressing Control and mouse button 1 or
| <Alt-A> for pressing A and the Alt key (KeyPress can be omitted).
| An event pattern can also be a virtual event of the form
| <<AString>> where AString can be arbitrary. This
| event can be generated by event_generate.
| If events are concatenated they must appear shortly
| after each other.
|
| FUNC will be called if the event sequence occurs with an
| instance of Event as argument. If the return value of FUNC is
| "break" no further bound function is invoked.
|
| An additional boolean parameter ADD specifies whether FUNC will
| be called additionally to the other bound function or whether
| it will replace the previous function.
|
| Bind will return an identifier to allow deletion of the bound function with
| unbind without memory leak.
|
| If FUNC or SEQUENCE is omitted the bound function or list
| of bound events are returned.
|
| bind_all(self, sequence=None, func=None, add=None)
| Bind to all widgets at an event SEQUENCE a call to function FUNC.
| An additional boolean parameter ADD specifies whether FUNC will
| be called additionally to the other bound function or whether
| it will replace the previous function. See bind for the return value.
|
| bind_class(self, className, sequence=None, func=None, add=None)
| Bind to widgets with bindtag CLASSNAME at event
| SEQUENCE a call of function FUNC. An additional
| boolean parameter ADD specifies whether FUNC will be
| called additionally to the other bound function or
| whether it will replace the previous function. See bind for
| the return value.
|
| bindtags(self, tagList=None)
| Set or get the list of bindtags for this widget.
|
| With no argument return the list of all bindtags associated with
| this widget. With a list of strings as argument the bindtags are
| set to this list. The bindtags determine in which order events are
| processed (see bind).
|
| cget(self, key)
| Return the resource value for a KEY given as string.
|
| clipboard_append(self, string, **kw)
| Append STRING to the Tk clipboard.
|
| A widget specified at the optional displayof keyword
| argument specifies the target display. The clipboard
| can be retrieved with selection_get.
|
| clipboard_clear(self, **kw)
| Clear the data in the Tk clipboard.
|
| A widget specified for the optional displayof keyword
| argument specifies the target display.
|
| clipboard_get(self, **kw)
| Retrieve data from the clipboard on window's display.
|
| The window keyword defaults to the root window of the Tkinter
| application.
|
| The type keyword specifies the form in which the data is
| to be returned and should be an atom name such as STRING
| or FILE_NAME. Type defaults to STRING, except on X11, where the default
| is to try UTF8_STRING and fall back to STRING.
|
| This command is equivalent to:
|
| selection_get(CLIPBOARD)
|
| columnconfigure = grid_columnconfigure(self, index, cnf={}, **kw)
|
| config = configure(self, cnf=None, **kw)
|
| configure(self, cnf=None, **kw)
| Configure resources of a widget.
|
| The values for resources are specified as keyword
| arguments. To get an overview about
| the allowed keyword arguments call the method keys.
|
| deletecommand(self, name)
| Internal function.
|
| Delete the Tcl command provided in NAME.
|
| event_add(self, virtual, *sequences)
| Bind a virtual event VIRTUAL (of the form <<Name>>)
| to an event SEQUENCE such that the virtual event is triggered
| whenever SEQUENCE occurs.
|
| event_delete(self, virtual, *sequences)
| Unbind a virtual event VIRTUAL from SEQUENCE.
|
| event_generate(self, sequence, **kw)
| Generate an event SEQUENCE. Additional
| keyword arguments specify parameter of the event
| (e.g. x, y, rootx, rooty).
|
| event_info(self, virtual=None)
| Return a list of all virtual events or the information
| about the SEQUENCE bound to the virtual event VIRTUAL.
|
| focus = focus_set(self)
|
| focus_displayof(self)
| Return the widget which has currently the focus on the
| display where this widget is located.
|
| Return None if the application does not have the focus.
|
| focus_force(self)
| Direct input focus to this widget even if the
| application does not have the focus. Use with
| caution!
|
| focus_get(self)
| Return the widget which has currently the focus in the
| application.
|
| Use focus_displayof to allow working with several
| displays. Return None if application does not have
| the focus.
|
| focus_lastfor(self)
| Return the widget which would have the focus if top level
| for this widget gets the focus from the window manager.
|
| focus_set(self)
| Direct input focus to this widget.
|
| If the application currently does not have the focus
| this widget will get the focus if the application gets
| the focus through the window manager.
|
| getboolean(self, s)
| Return a boolean value for Tcl boolean values true and false given as parameter.
|
| getdouble(self, s)
|
| getint(self, s)
|
| getvar(self, name='PY_VAR')
| Return value of Tcl variable NAME.
|
| grab_current(self)
| Return widget which has currently the grab in this application
| or None.
|
| grab_release(self)
| Release grab for this widget if currently set.
|
| grab_set(self)
| Set grab for this widget.
|
| A grab directs all events to this and descendant
| widgets in the application.
|
| grab_set_global(self)
| Set global grab for this widget.
|
| A global grab directs all events to this and
| descendant widgets on the display. Use with caution -
| other applications do not get events anymore.
|
| grab_status(self)
| Return None, "local" or "global" if this widget has
| no, a local or a global grab.
|
| grid_anchor(self, anchor=None)
| The anchor value controls how to place the grid within the
| master when no row/column has any weight.
|
| The default anchor is nw.
|
| grid_bbox(self, column=None, row=None, col2=None, row2=None)
| Return a tuple of integer coordinates for the bounding
| box of this widget controlled by the geometry manager grid.
|
| If COLUMN, ROW is given the bounding box applies from
| the cell with row and column 0 to the specified
| cell. If COL2 and ROW2 are given the bounding box
| starts at that cell.
|
| The returned integers specify the offset of the upper left
| corner in the master widget and the width and height.
|
| grid_columnconfigure(self, index, cnf={}, **kw)
| Configure column INDEX of a grid.
|
| Valid resources are minsize (minimum size of the column),
| weight (how much does additional space propagate to this column)
| and pad (how much space to let additionally).
|
| grid_location(self, x, y)
| Return a tuple of column and row which identify the cell
| at which the pixel at position X and Y inside the master
| widget is located.
|
| grid_propagate(self, flag=['_noarg_'])
| Set or get the status for propagation of geometry information.
|
| A boolean argument specifies whether the geometry information
| of the slaves will determine the size of this widget. If no argument
| is given, the current setting will be returned.
|
| grid_rowconfigure(self, index, cnf={}, **kw)
| Configure row INDEX of a grid.
|
| Valid resources are minsize (minimum size of the row),
| weight (how much does additional space propagate to this row)
| and pad (how much space to let additionally).
|
| grid_size(self)
| Return a tuple of the number of column and rows in the grid.
|
| grid_slaves(self, row=None, column=None)
| Return a list of all slaves of this widget
| in its packing order.
|
| image_names(self)
| Return a list of all existing image names.
|
| image_types(self)
| Return a list of all available image types (e.g. photo bitmap).
|
| info_patchlevel(self)
| Returns the exact version of the Tcl library.
|
| keys(self)
| Return a list of all resource names of this widget.
|
| lift = tkraise(self, aboveThis=None)
|
| lower(self, belowThis=None)
| Lower this widget in the stacking order.
|
| mainloop(self, n=0)
| Call the mainloop of Tk.
|
| nametowidget(self, name)
| Return the Tkinter instance of a widget identified by
| its Tcl name NAME.
|
| option_add(self, pattern, value, priority=None)
| Set a VALUE (second parameter) for an option
| PATTERN (first parameter).
|
| An optional third parameter gives the numeric priority
| (defaults to 80).
|
| option_clear(self)
| Clear the option database.
|
| It will be reloaded if option_add is called.
|
| option_get(self, name, className)
| Return the value for an option NAME for this widget
| with CLASSNAME.
|
| Values with higher priority override lower values.
|
| option_readfile(self, fileName, priority=None)
| Read file FILENAME into the option database.
|
| An optional second parameter gives the numeric
| priority.
|
| pack_propagate(self, flag=['_noarg_'])
| Set or get the status for propagation of geometry information.
|
| A boolean argument specifies whether the geometry information
| of the slaves will determine the size of this widget. If no argument
| is given the current setting will be returned.
|
| pack_slaves(self)
| Return a list of all slaves of this widget
| in its packing order.
|
| place_slaves(self)
| Return a list of all slaves of this widget
| in its packing order.
|
| propagate = pack_propagate(self, flag=['_noarg_'])
|
| quit(self)
| Quit the Tcl interpreter. All widgets will be destroyed.
|
| register = _register(self, func, subst=None, needcleanup=1)
|
| rowconfigure = grid_rowconfigure(self, index, cnf={}, **kw)
|
| selection_get(self, **kw)
| Return the contents of the current X selection.
|
| A keyword parameter selection specifies the name of
| the selection and defaults to PRIMARY. A keyword
| parameter displayof specifies a widget on the display
| to use. A keyword parameter type specifies the form of data to be
| fetched, defaulting to STRING except on X11, where UTF8_STRING is tried
| before STRING.
|
| selection_handle(self, command, **kw)
| Specify a function COMMAND to call if the X
| selection owned by this widget is queried by another
| application.
|
| This function must return the contents of the
| selection. The function will be called with the
| arguments OFFSET and LENGTH which allows the chunking
| of very long selections. The following keyword
| parameters can be provided:
| selection - name of the selection (default PRIMARY),
| type - type of the selection (e.g. STRING, FILE_NAME).
|
| selection_own(self, **kw)
| Become owner of X selection.
|
| A keyword parameter selection specifies the name of
| the selection (default PRIMARY).
|
| selection_own_get(self, **kw)
| Return owner of X selection.
|
| The following keyword parameter can
| be provided:
| selection - name of the selection (default PRIMARY),
| type - type of the selection (e.g. STRING, FILE_NAME).
|
| send(self, interp, cmd, *args)
| Send Tcl command CMD to different interpreter INTERP to be executed.
|
| setvar(self, name='PY_VAR', value='1')
| Set Tcl variable NAME to VALUE.
|
| size = grid_size(self)
|
| slaves = pack_slaves(self)
|
| tk_bisque(self)
| Change the color scheme to light brown as used in Tk 3.6 and before.
|
| tk_focusFollowsMouse(self)
| The widget under mouse will get automatically focus. Can not
| be disabled easily.
|
| tk_focusNext(self)
| Return the next widget in the focus order which follows
| widget which has currently the focus.
|
| The focus order first goes to the next child, then to
| the children of the child recursively and then to the
| next sibling which is higher in the stacking order. A
| widget is omitted if it has the takefocus resource set
| to 0.
|
| tk_focusPrev(self)
| Return previous widget in the focus order. See tk_focusNext for details.
|
| tk_setPalette(self, *args, **kw)
| Set a new color scheme for all widget elements.
|
| A single color as argument will cause that all colors of Tk
| widget elements are derived from this.
| Alternatively several keyword parameters and its associated
| colors can be given. The following keywords are valid:
| activeBackground, foreground, selectColor,
| activeForeground, highlightBackground, selectBackground,
| background, highlightColor, selectForeground,
| disabledForeground, insertBackground, troughColor.
|
| tk_strictMotif(self, boolean=None)
| Set Tcl internal variable, whether the look and feel
| should adhere to Motif.
|
| A parameter of 1 means adhere to Motif (e.g. no color
| change if mouse passes over slider).
| Returns the set value.
|
| tkraise(self, aboveThis=None)
| Raise this widget in the stacking order.
|
| unbind(self, sequence, funcid=None)
| Unbind for this widget for event SEQUENCE the
| function identified with FUNCID.
|
| unbind_all(self, sequence)
| Unbind for all widgets for event SEQUENCE all functions.
|
| unbind_class(self, className, sequence)
| Unbind for all widgets with bindtag CLASSNAME for event SEQUENCE
| all functions.
|
| update(self)
| Enter event loop until all pending events have been processed by Tcl.
|
| update_idletasks(self)
| Enter event loop until all idle callbacks have been called. This
| will update the display of windows but not process events caused by
| the user.
|
| wait_variable(self, name='PY_VAR')
| Wait until the variable is modified.
|
| A parameter of type IntVar, StringVar, DoubleVar or
| BooleanVar must be given.
|
| wait_visibility(self, window=None)
| Wait until the visibility of a WIDGET changes
| (e.g. it appears).
|
| If no parameter is given self is used.
|
| wait_window(self, window=None)
| Wait until a WIDGET is destroyed.
|
| If no parameter is given self is used.
|
| waitvar = wait_variable(self, name='PY_VAR')
|
| winfo_atom(self, name, displayof=0)
| Return integer which represents atom NAME.
|
| winfo_atomname(self, id, displayof=0)
| Return name of atom with identifier ID.
|
| winfo_cells(self)
| Return number of cells in the colormap for this widget.
|
| winfo_children(self)
| Return a list of all widgets which are children of this widget.
|
| winfo_class(self)
| Return window class name of this widget.
|
| winfo_colormapfull(self)
| Return True if at the last color request the colormap was full.
|
| winfo_containing(self, rootX, rootY, displayof=0)
| Return the widget which is at the root coordinates ROOTX, ROOTY.
|
| winfo_depth(self)
| Return the number of bits per pixel.
|
| winfo_exists(self)
| Return true if this widget exists.
|
| winfo_fpixels(self, number)
| Return the number of pixels for the given distance NUMBER
| (e.g. "3c") as float.
|
| winfo_geometry(self)
| Return geometry string for this widget in the form "widthxheight+X+Y".
|
| winfo_height(self)
| Return height of this widget.
|
| winfo_id(self)
| Return identifier ID for this widget.
|
| winfo_interps(self, displayof=0)
| Return the name of all Tcl interpreters for this display.
|
| winfo_ismapped(self)
| Return true if this widget is mapped.
|
| winfo_manager(self)
| Return the window manager name for this widget.
|
| winfo_name(self)
| Return the name of this widget.
|
| winfo_parent(self)
| Return the name of the parent of this widget.
|
| winfo_pathname(self, id, displayof=0)
| Return the pathname of the widget given by ID.
|
| winfo_pixels(self, number)
| Rounded integer value of winfo_fpixels.
|
| winfo_pointerx(self)
| Return the x coordinate of the pointer on the root window.
|
| winfo_pointerxy(self)
| Return a tuple of x and y coordinates of the pointer on the root window.
|
| winfo_pointery(self)
| Return the y coordinate of the pointer on the root window.
|
| winfo_reqheight(self)
| Return requested height of this widget.
|
| winfo_reqwidth(self)
| Return requested width of this widget.
|
| winfo_rgb(self, color)
| Return a tuple of integer RGB values in range(65536) for color in this widget.
|
| winfo_rootx(self)
| Return x coordinate of upper left corner of this widget on the
| root window.
|
| winfo_rooty(self)
| Return y coordinate of upper left corner of this widget on the
| root window.
|
| winfo_screen(self)
| Return the screen name of this widget.
|
| winfo_screencells(self)
| Return the number of the cells in the colormap of the screen
| of this widget.
|
| winfo_screendepth(self)
| Return the number of bits per pixel of the root window of the
| screen of this widget.
|
| winfo_screenheight(self)
| Return the number of pixels of the height of the screen of this widget
| in pixel.
|
| winfo_screenmmheight(self)
| Return the number of pixels of the height of the screen of
| this widget in mm.
|
| winfo_screenmmwidth(self)
| Return the number of pixels of the width of the screen of
| this widget in mm.
|
| winfo_screenvisual(self)
| Return one of the strings directcolor, grayscale, pseudocolor,
| staticcolor, staticgray, or truecolor for the default
| colormodel of this screen.
|
| winfo_screenwidth(self)
| Return the number of pixels of the width of the screen of
| this widget in pixel.
|
| winfo_server(self)
| Return information of the X-Server of the screen of this widget in
| the form "XmajorRminor vendor vendorVersion".
|
| winfo_toplevel(self)
| Return the toplevel widget of this widget.
|
| winfo_viewable(self)
| Return true if the widget and all its higher ancestors are mapped.
|
| winfo_visual(self)
| Return one of the strings directcolor, grayscale, pseudocolor,
| staticcolor, staticgray, or truecolor for the
| colormodel of this widget.
|
| winfo_visualid(self)
| Return the X identifier for the visual for this widget.
|
| winfo_visualsavailable(self, includeids=False)
| Return a list of all visuals available for the screen
| of this widget.
|
| Each item in the list consists of a visual name (see winfo_visual), a
| depth and if includeids is true is given also the X identifier.
|
| winfo_vrootheight(self)
| Return the height of the virtual root window associated with this
| widget in pixels. If there is no virtual root window return the
| height of the screen.
|
| winfo_vrootwidth(self)
| Return the width of the virtual root window associated with this
| widget in pixel. If there is no virtual root window return the
| width of the screen.
|
| winfo_vrootx(self)
| Return the x offset of the virtual root relative to the root
| window of the screen of this widget.
|
| winfo_vrooty(self)
| Return the y offset of the virtual root relative to the root
| window of the screen of this widget.
|
| winfo_width(self)
| Return the width of this widget.
|
| winfo_x(self)
| Return the x coordinate of the upper left corner of this widget
| in the parent.
|
| winfo_y(self)
| Return the y coordinate of the upper left corner of this widget
| in the parent.
|
| ----------------------------------------------------------------------
| Data descriptors inherited from tkinter.Misc:
|
| __dict__
| dictionary for instance variables (if defined)
|
| __weakref__
| list of weak references to the object (if defined)
|
| ----------------------------------------------------------------------
| Methods inherited from tkinter.Pack:
|
| forget = pack_forget(self)
|
| info = pack_info(self)
|
| pack = pack_configure(self, cnf={}, **kw)
|
| pack_configure(self, cnf={}, **kw)
| Pack a widget in the parent widget. Use as options:
| after=widget - pack it after you have packed widget
| anchor=NSEW (or subset) - position widget according to
| given direction
| before=widget - pack it before you will pack widget
| expand=bool - expand widget if parent size grows
| fill=NONE or X or Y or BOTH - fill widget if widget grows
| in=master - use master to contain this widget
| in_=master - see 'in' option description
| ipadx=amount - add internal padding in x direction
| ipady=amount - add internal padding in y direction
| padx=amount - add padding in x direction
| pady=amount - add padding in y direction
| side=TOP or BOTTOM or LEFT or RIGHT - where to add this widget.
|
| pack_forget(self)
| Unmap this widget and do not use it for the packing order.
|
| pack_info(self)
| Return information about the packing options
| for this widget.
|
| ----------------------------------------------------------------------
| Methods inherited from tkinter.Place:
|
| place = place_configure(self, cnf={}, **kw)
|
| place_configure(self, cnf={}, **kw)
| Place a widget in the parent widget. Use as options:
| in=master - master relative to which the widget is placed
| in_=master - see 'in' option description
| x=amount - locate anchor of this widget at position x of master
| y=amount - locate anchor of this widget at position y of master
| relx=amount - locate anchor of this widget between 0.0 and 1.0
| relative to width of master (1.0 is right edge)
| rely=amount - locate anchor of this widget between 0.0 and 1.0
| relative to height of master (1.0 is bottom edge)
| anchor=NSEW (or subset) - position anchor according to given direction
| width=amount - width of this widget in pixel
| height=amount - height of this widget in pixel
| relwidth=amount - width of this widget between 0.0 and 1.0
| relative to width of master (1.0 is the same width
| as the master)
| relheight=amount - height of this widget between 0.0 and 1.0
| relative to height of master (1.0 is the same
| height as the master)
| bordermode="inside" or "outside" - whether to take border width of
| master widget into account
|
| place_forget(self)
| Unmap this widget.
|
| place_info(self)
| Return information about the placing options
| for this widget.
|
| ----------------------------------------------------------------------
| Methods inherited from tkinter.Grid:
|
| grid = grid_configure(self, cnf={}, **kw)
|
| grid_configure(self, cnf={}, **kw)
| Position a widget in the parent widget in a grid. Use as options:
| column=number - use cell identified with given column (starting with 0)
| columnspan=number - this widget will span several columns
| in=master - use master to contain this widget
| in_=master - see 'in' option description
| ipadx=amount - add internal padding in x direction
| ipady=amount - add internal padding in y direction
| padx=amount - add padding in x direction
| pady=amount - add padding in y direction
| row=number - use cell identified with given row (starting with 0)
| rowspan=number - this widget will span several rows
| sticky=NSEW - if cell is larger on which sides will this
| widget stick to the cell boundary
|
| grid_forget(self)
| Unmap this widget.
|
| grid_info(self)
| Return information about the options
| for positioning this widget in a grid.
|
| grid_remove(self)
| Unmap this widget but remember the grid options.
|
| location = grid_location(self, x, y)
|
| ----------------------------------------------------------------------
| Methods inherited from tkinter.XView:
|
| xview(self, *args)
| Query and change the horizontal position of the view.
|
| xview_moveto(self, fraction)
| Adjusts the view in the window so that FRACTION of the
| total width of the canvas is off-screen to the left.
|
| xview_scroll(self, number, what)
| Shift the x-view according to NUMBER which is measured in "units"
| or "pages" (WHAT).
附件二
tkinter.rar下载
tkinter全部控件的英文帮助全集下载地址:
https://download.csdn.net/download/boysoft2002/88640897
相关文章:
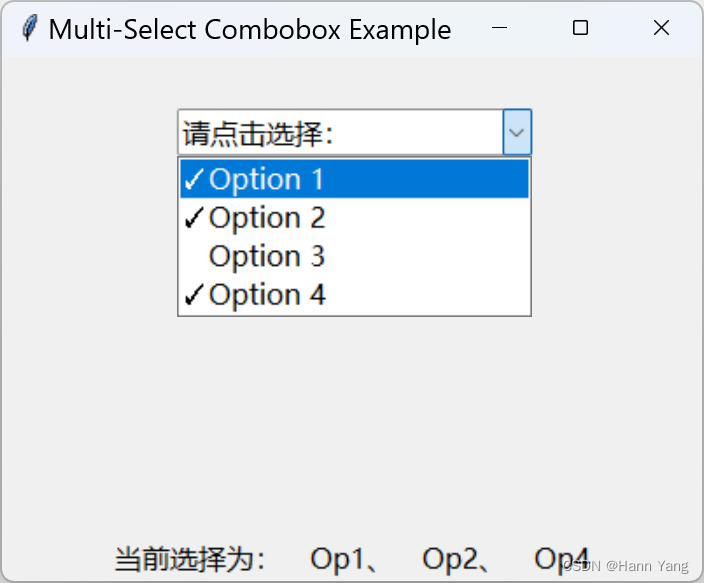
Python tkinter控件全集之组合选择框 ttk.ComboBox
Tkinter标准库 Tkinter是Python的标准GUI库,也是最常用的Python GUI库之一,提供了丰富的组件和功能,包括窗口、按钮、标签、文本框、列表框、滚动条、画布、菜单等,方便开发者进行图形界面的开发。Tkinter库基于Tk for Unix/Wind…...
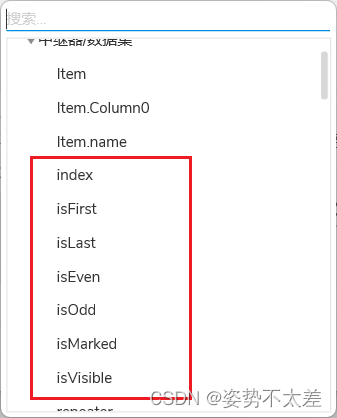
Axure之中继器的使用(交互动作reperter属性Item属性)
目录 一.中继器的基本使用 二.中继器的动作(增删改查) 2.1 新增 2.2 删除 2.3 更新行 2.4 效果展示 2.5 模糊查询 三.reperter属性 在Axure中,中继器(Repeater)是一种功能强大的组件,用于创建重复…...
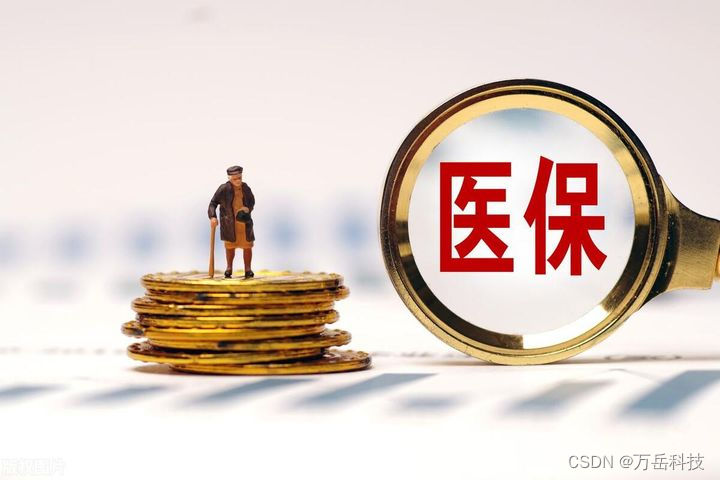
数字化医疗新篇章:构建智能医保支付购药系统
在迎接数字化医疗时代的挑战和机遇中,智能医保支付购药系统的建设显得尤为重要。本文将深入介绍如何通过先进的技术实现,构建一套智能、高效的医保支付购药系统,为全面建设健康中国贡献力量。 1. 引言 随着医疗科技的飞速发展,…...

11_12-Golang中的运算符
**Golang **中的运算符 主讲教师:(大地) 合作网站:www.itying.com** **(IT 营) 我的专栏:https://www.itying.com/category-79-b0.html 1、Golang 内置的运算符 算术运算符关系运算符逻辑运…...
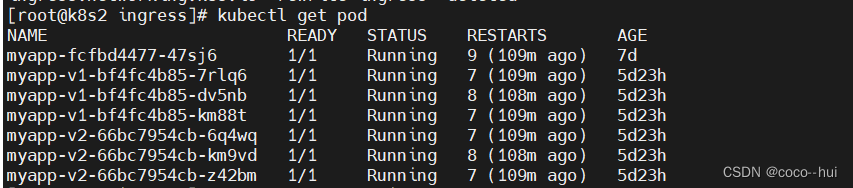
k8s-ingress特性 9
TLS加密 创建证书 测试访问 auth认证 创建认证文件 rewrite重定向 进入域名时,会自动重定向到hostname.html 示例: 测试 版本的升级迭代,之前利用控制器进行滚动更新,在升级过程中无法做到快速回滚 更加平滑的升级࿱…...

【redis】redis系统实现发布订阅的标准模板
目录 简介参数配置代码模板 简介 Redis发布订阅功能是Redis的一种消息传递模式,允许多个客户端之间通过消息通道进行实时的消息传递。在发布订阅模式下,消息的发送者被称为发布者(publisher),而接收消息的客户端被称为…...
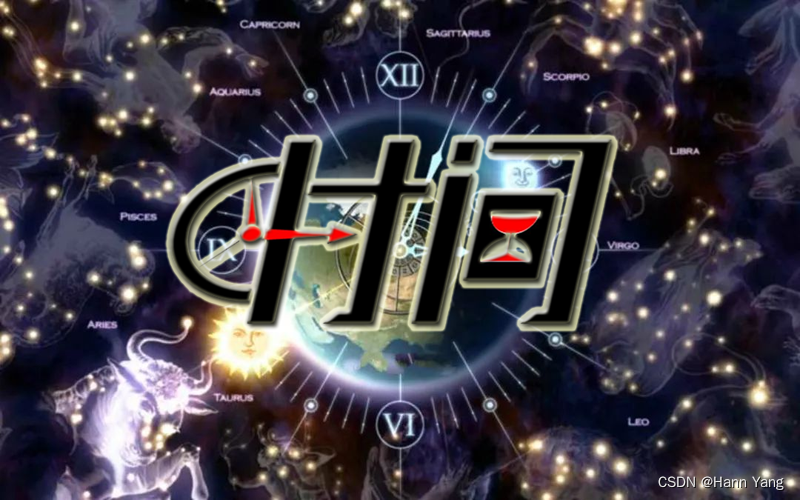
Python 时间日期处理库函数
标准库 datetime >>> import datetime >>> date datetime.date(2023, 12, 20) >>> print(date) 2023-12-20 >>> date datetime.datetime(2023, 12, 20) >>> print(date) 2023-12-20 00:00:00 >>> print(date.strfti…...

第二十二章 : Spring Boot 集成定时任务(一)
第二十二章 : Spring Boot 集成定时任务(一) 前言 本章知识点: 介绍使用Spring Boot内置的Scheduled注解来实现定时任务-单线程和多线程;以及介绍Quartz定时任务调度框架:简单定时调度器(Simp…...
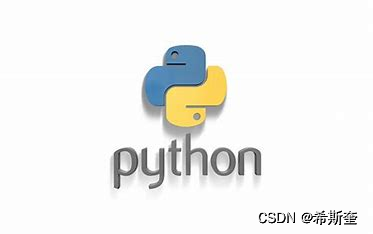
关于“Python”的核心知识点整理大全32
目录 12.6.4 调整飞船的速度 settings.py ship.py alien_invasion.py 12.6.5 限制飞船的活动范围 ship.py 12.6.6 重构 check_events() game_functions.py 12.7 简单回顾 12.7.1 alien_invasion.py 12.7.2 settings.py 12.7.3 game_functions.py 12.7.4 ship.py …...
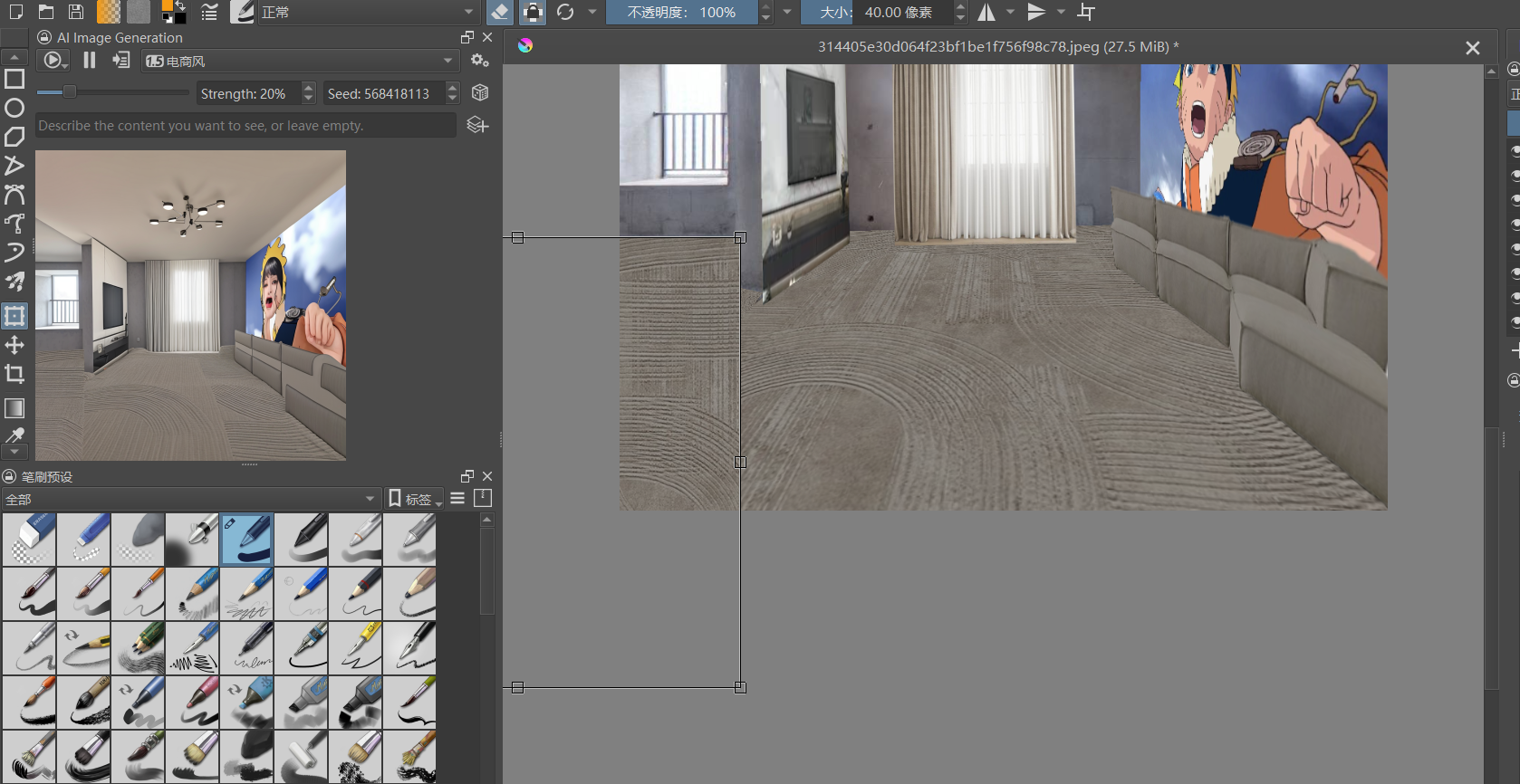
【krita】实时绘画 入门到精通 海报+电商+装修+人物
安装插件 首先打开comfyUI,再打开krita,出现问题提示, 打开 cd custom_nodes 输入命令 安装控件 git clone https://github.com/Acly/comfyui-tooling-nodes.git krita基础设置 设置模型 设置lora (可设置lora强度 增加更多…...
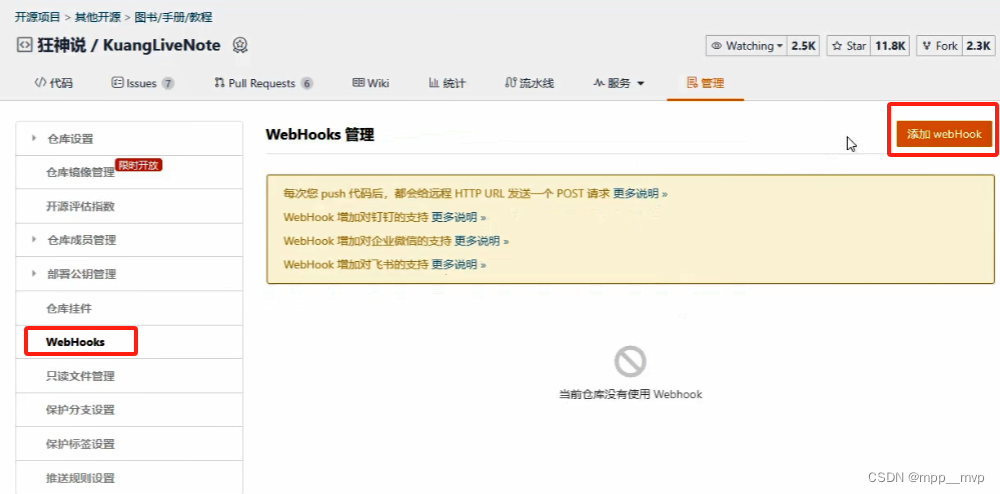
云原生系列2-CICD持续集成部署-GitLab和Jenkins
1、CICD持续集成部署 传统软件开发流程: 1、项目经理分配模块开发任务给开发人员(项目经理-开发) 2、每个模块单独开发完毕(开发),单元测试(测试) 3、开发完毕后,集成部…...
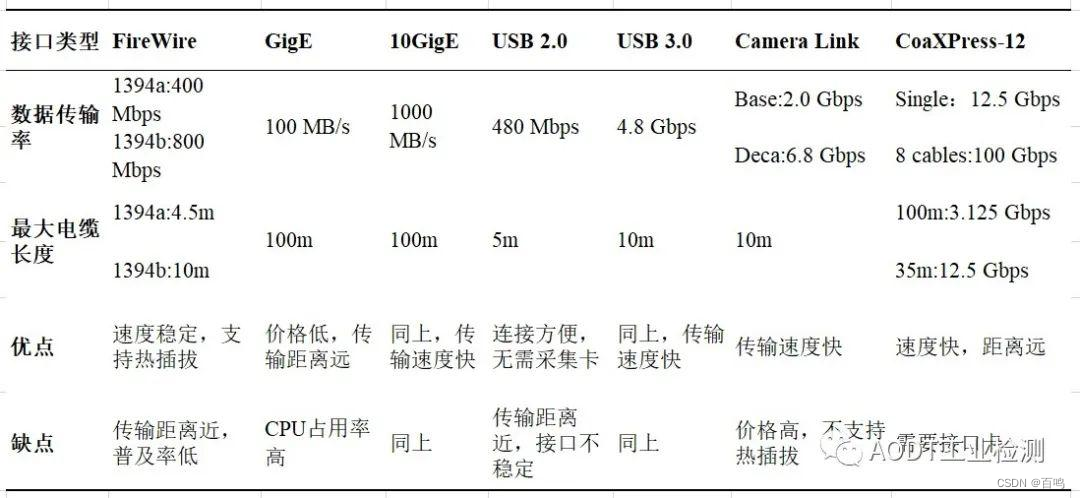
50ms时延工业相机
华睿工业相机A3504CG000 参数配置: 相机端到端理论时延:80ms 厂家同步信息,此款设备帧率上线23fps,单帧时延:43.48ms,按照一图缓存加上传输显示的话,厂家预估时延在:80ms 厂家还有…...
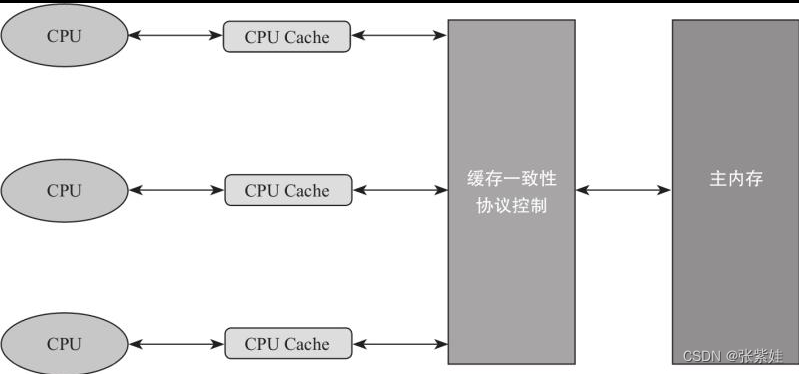
CPU缓存一致性问题
什么是可见性问题? Further Reading :什么是可见性问题? 缓存一致性 内存一致性 内存可见性 顺序一致性区别 CPU缓存一致性问题 由于CPU缓存的出现,很好地解决了处理器与内存速度之间的矛盾,极大地提高了CPU的吞吐能…...

35道HTML高频题整理(附答案背诵版)
1、简述 HTML5 新特性 ? HTML5 是 HTML 的最新版本,它引入了很多新的特性和元素,以提供更丰富的网页内容和更好的用户体验。以下是一些主要的新特性: 语义元素:HTML5 引入了新的语义元素,像 <article&g…...
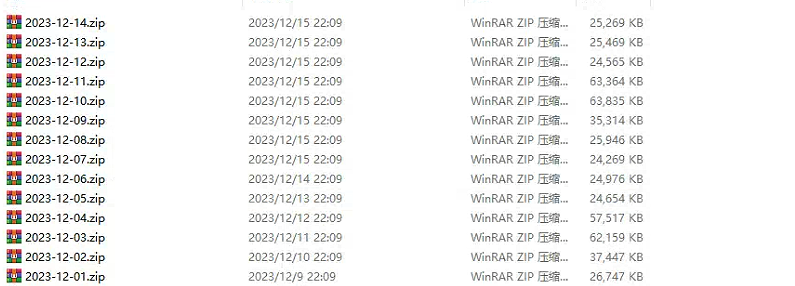
【powershell】Windows环境powershell 运维之历史文件压缩清理
🦄 个人主页——🎐开着拖拉机回家_Linux,大数据运维-CSDN博客 🎐✨🍁 🪁🍁🪁🍁🪁🍁🪁🍁 🪁🍁🪁&am…...
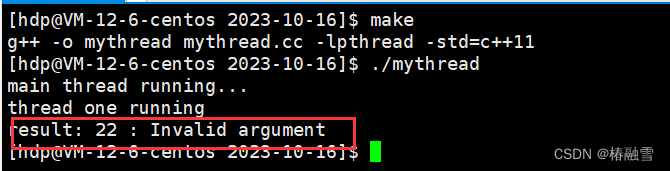
【Linux】Linux线程概念和线程控制
文章目录 一、Linux线程概念1.什么是线程2.线程的优缺点3.线程异常4.线程用途5.Linux进程VS线程 二、线程控制1.线程创建2.线程终止3.线程等待4.线程分离 一、Linux线程概念 1.什么是线程 线程是进程内的一个执行流。 我们知道,一个进程会有对应的PCB,…...
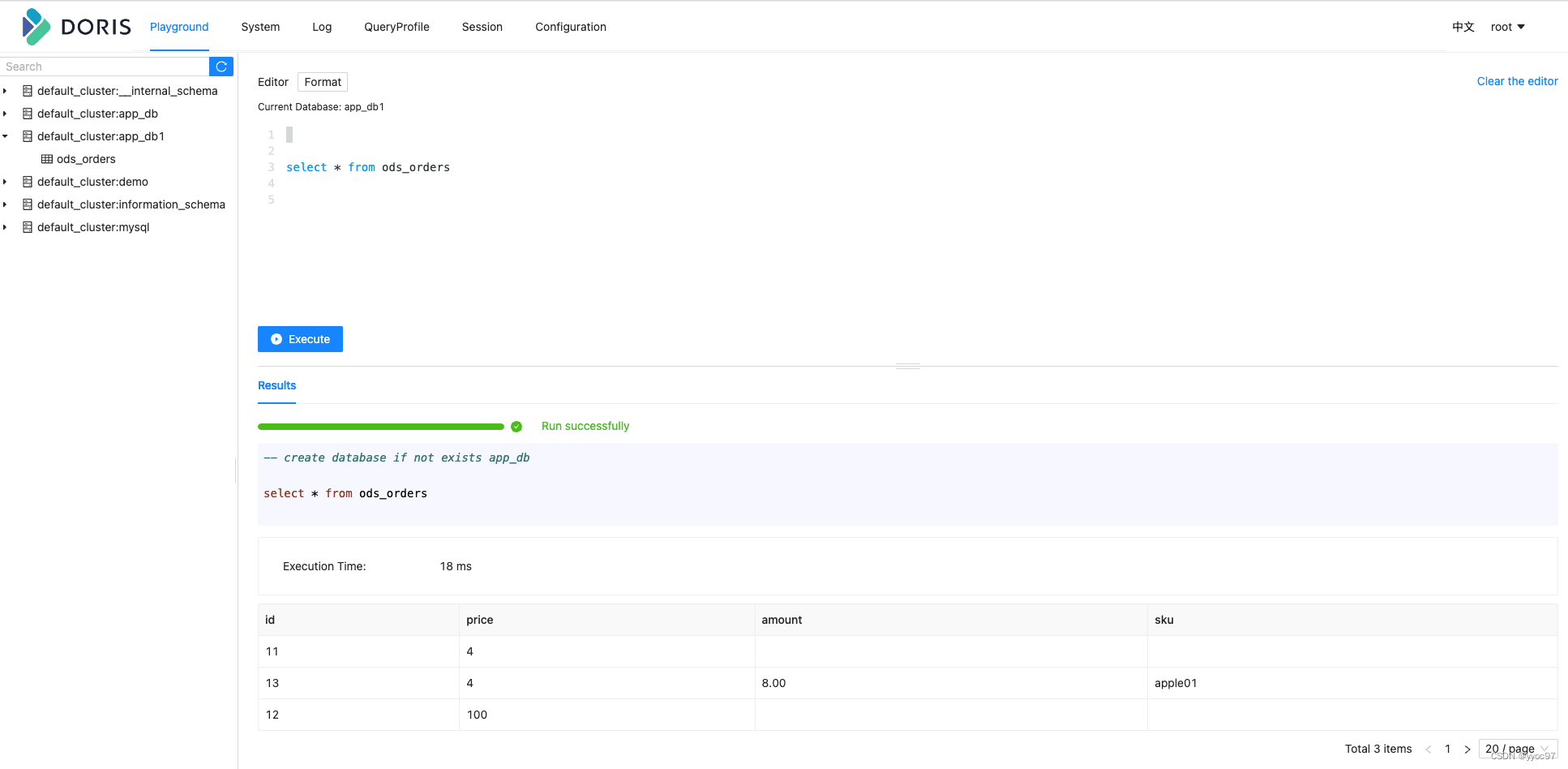
Flink cdc3.0同步实例(动态变更表结构、分库分表同步)
文章目录 前言准备flink环境docker构建mysql、doris环境数据准备 通过 FlinkCDC cli 提交任务整库同步同步变更路由变更路由表结构不一致无法同步 结尾 前言 最近Flink CDC 3.0发布, 不仅提供基础的数据同步能力。schema 变更自动同步、整库同步、分库分表等增强功…...
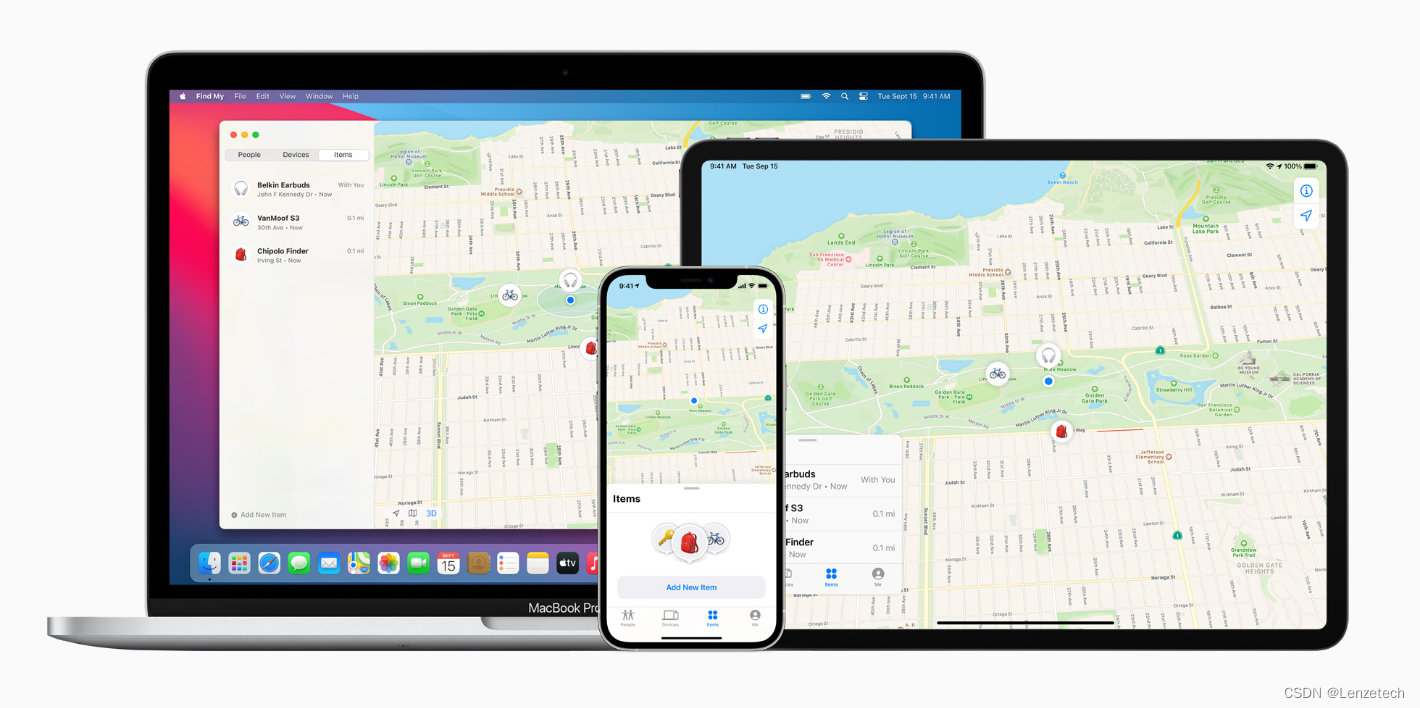
国产Apple Find My认证芯片哪里找,伦茨科技ST17H6x芯片可以帮到您
深圳市伦茨科技有限公司(以下简称“伦茨科技”)发布ST17H6x Soc平台。成为继Nordic之后全球第二家取得Apple Find My「查找」认证的芯片厂家,该平台提供可通过Apple Find My认证的Apple查找(Find My)功能集成解决方案。…...

肺癌相关知识
写在前面 大概想了解下肺癌相关的知识,开此贴做记录,看看后续有没有相关的生信文章思路。 综述 文章名期刊影响因子Lung cancer immunotherapy: progress, pitfalls, and promisesMol Cancer37.3 常见治疗手段有surgery, radiation therapy, chemoth…...
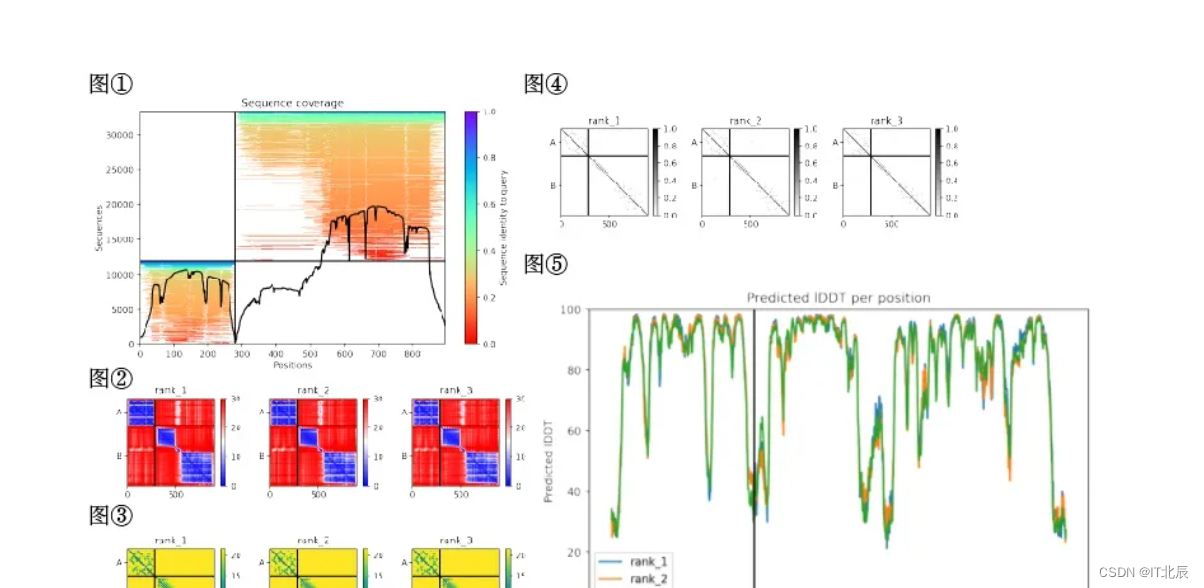
ChimeraX使用教程-安装及基本操作
ChimeraX使用教程-安装及基本操作 1、访问https://www.cgl.ucsf.edu/chimerax/download.html进行下载,然后安装 安装完成后,显示界面 2、基本操作 1、点击file,导入 .PDB 文件。 (注:在 alphafold在线预测蛋白》点…...
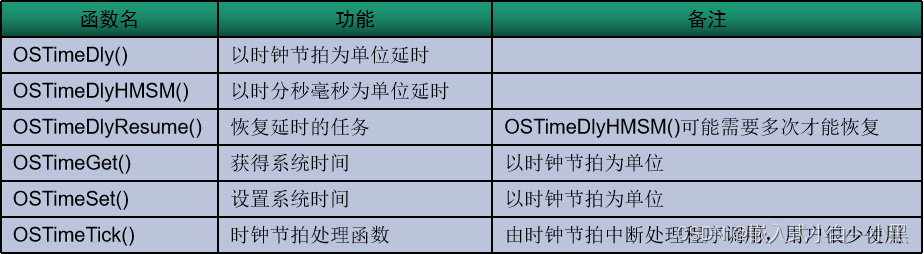
【小黑嵌入式系统第十一课】μC/OS-III程序设计基础(一)——任务设计、任务管理(创建基本状态内部任务)、任务调度、系统函数
上一课: 【小黑嵌入式系统第十课】μC/OS-III概况——实时操作系统的特点、基本概念(内核&任务&中断)、与硬件的关系&实现 文章目录 一、任务设计1.1 任务概述1.2 任务的类型1.2.1 单次执行类任务(运行至完成型&#…...
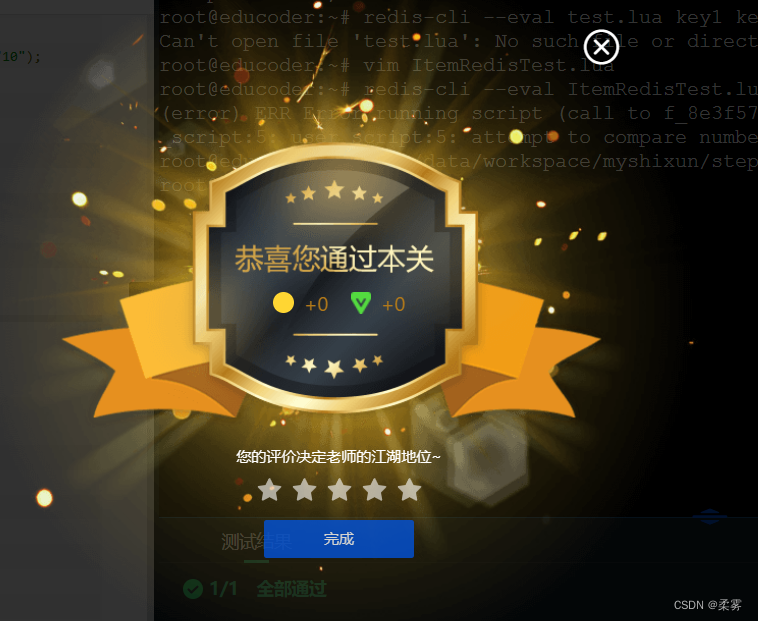
Redis一些常用的技术
文章目录 第1关:Redis 事务与锁机制第2关:流水线第3关:发布订阅第4关:超时命令第5关:使用Lua语言 第1关:Redis 事务与锁机制 编程要求 根据提示,在右侧编辑器Begin-End补充代码,根据…...

基于QPainter 绘图图片绕绘制设备中心旋转
项目地址:https://gitcode.com/m0_45463480/QPainter/tree/main 获取途径:进入CSDN->GitCode直接下载或者通过git拉取仓库内容。 QPainter是Qt框架中的一个类,用于在QWidget或QPixmap等设备上进行绘图操作。它提供了丰富的绘图功能,可以用于绘制线条、图形、文本等。Q…...
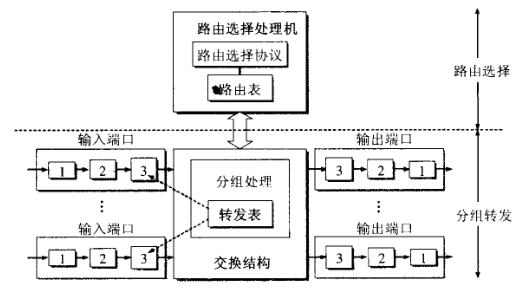
计算机网络(4):网络层
网络层提供的两种服务 虚电路服务(Virtual Circuit Service)和数据报服务(Datagram Service)是在网络层(第三层)提供的两种不同的通信服务。它们主要区别在于建立连接的方式和数据传输的方式。 虚电路服务…...
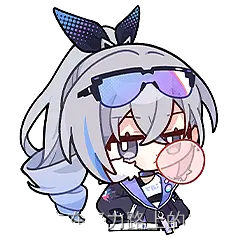
动态内存分配(malloc和free、calloc和realloc)
目录 一、为什么要有动态内存分配 二、C/C中程序内存区域划分 三、malloc和free 2.1、malloc 2.2、free 四、calloc和realloc 3.1、calloc 3.2、realloc 3.3realloc在调整内存空间的是存在两种情况: 3.4realloc有malloc的功能 五、常见的动…...
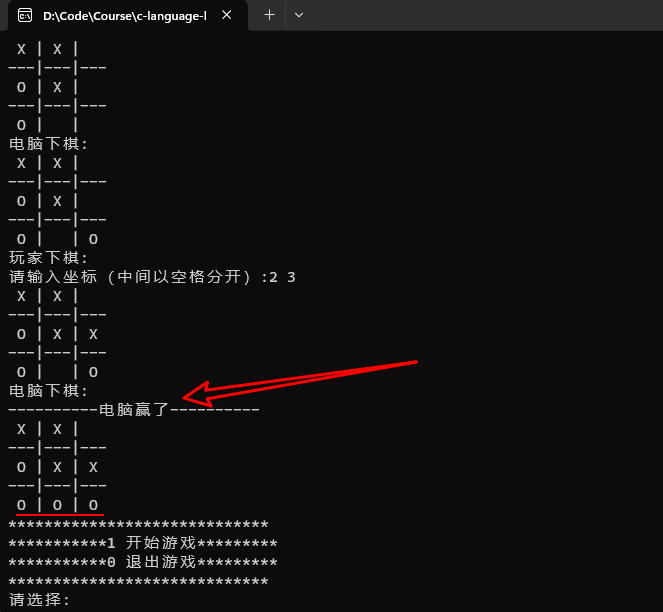
C语言---井字棋(三子棋)
Tic-Tac-Toe 1 游戏介绍和随机数1.1 游戏介绍1.2 随机数的生成1.3 棋盘大小和符号 2 设计游戏2.1 初始化棋盘2.2 打印棋盘2.3 玩家下棋2.4 电脑下棋2.5 判断输赢2.6 game()函数2.7 main()函数 3 完整三子棋代码3.1 Tic_Tac_Toe.h3.2 Tic_Tac_Toe.c3.3 Test.c 4 游戏代码的缺陷 …...
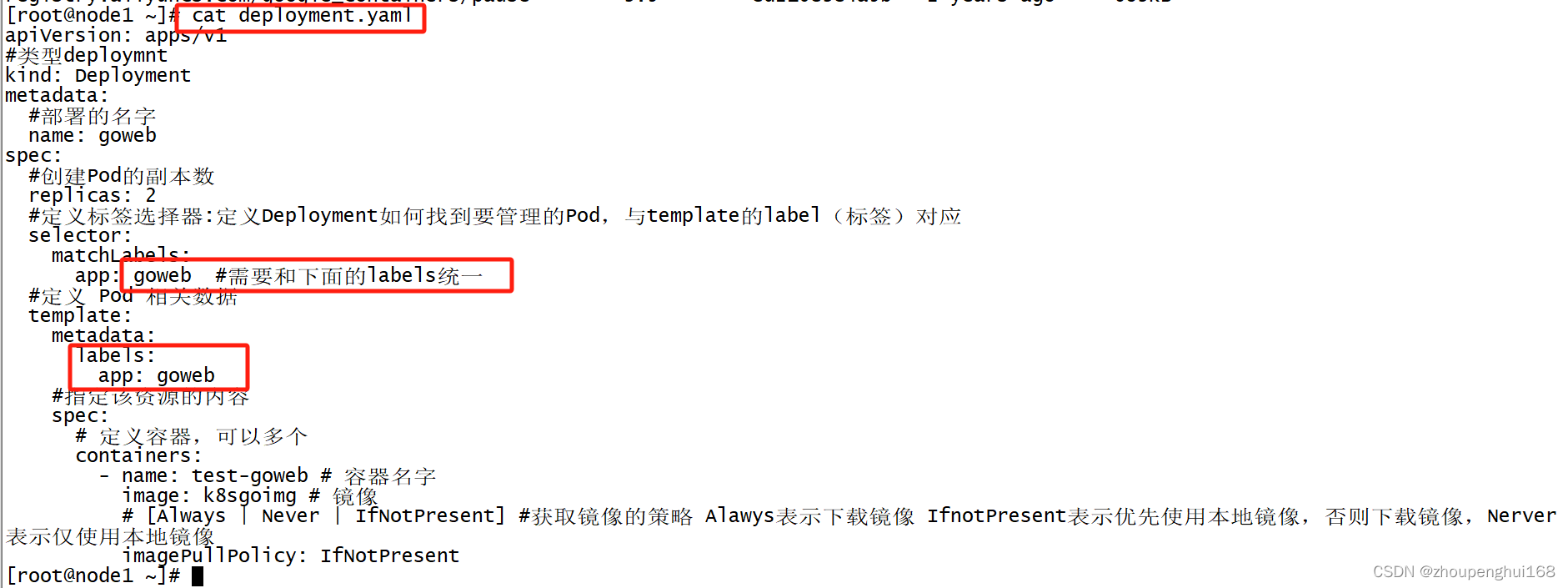
[Kubernetes]3. k8s集群Service详解
在上一节讲解了k8s 的pod,deployment,以及借助pod,deployment来部署项目,但会存在问题: 每次只能访问一个 pod,没有负载均衡自动转发到不同 pod访问还需要端口转发Pod重创后IP变了,名字也变了针对上面的问题,可以借助Service来解决,下面就来看看Service怎么使用 一.Service详…...

C++ 指定范围内递增初始化一个vector<int> | Python: list(range(31, 90))
通过lambda表达式 std::iota()实现: template <typename Tp> inline void print_vec(const std::vector<Tp>& vec) {fmt::print("[{}]\n", fmt::join(vec, ", ")); }// 相当于Python的lst list(range(31, 90))const std::ve…...

【Java之数据结构与算法】
选择排序 package Code01;public class Code01_SelectionSort {public static void selectionSort(int[] arr) {if(arrnull||arr.length<2) {return;}for(int i0;i<arr.length;i) {int minIndex i;for(int ji1;j<arr.length;j) {minIndex arr[minIndex] > arr[j…...

爬虫scrapy中间件的使用
爬虫scrapy中间件的使用 学习目标: 应用 scrapy中使用间件使用随机UA的方法应用 scrapy中使用代理ip的的方法应用 scrapy与selenium配合使用 1. scrapy中间件的分类和作用 1.1 scrapy中间件的分类 根据scrapy运行流程中所在位置不同分为: 下载中间件…...

如何提高wordpress访问速度/it教育培训机构排名
转载原文在win环境下使用Git与GitHub建立关联or第二篇文章or图解说...

怎做不下网站刷枪/网络销售怎么才能找到客户
具体的报错信息:ArgumentException: Getting control 0s position in a group with only 0 controls when doing Repaint A OnGUI在每次绘制时会调用2次,第1次计算所有控件的位置,所有EdtorGUILayout控件方法返回的Rect均为0;第2次根据位置来…...

重庆网站seo公司/招工 最新招聘信息
1. 账户与账户安全 账户和组是操作系统的基本概念,linux的组有基本组和附加组之分,一个用户只可以加入到一个基本组中国,但是可以加入到多个附加组中.创建用户时,系统默认会自动创建同名的组,并设置用户加入该基本组中. 1.1 创建账户和组 1. useraddm-c 设置账户描述信息,一…...

做网站案例/百度网址输入
获取表字段:select *from user_tab_columnswhere Table_Name用户表order by column_name获取表注释:select *from user_tab_commentswhere Table_Name用户表order by Table_Name获取字段注释:select *from user_col_commentswhere Table_Name…...

口碑营销的策略技巧/seo是搜索引擎营销吗
yum -y install screen screen -S lnmp 记录session会话 ssh客户端中断再连接后 screen -r lnmp 恢复会话转载于:https://blog.51cto.com/kongdq/967746...

做零售出口的网站/武汉seo优化
1,第一个问题---报错---现象,目标mysql库两个表,一个有数据,一个没有数据,切复制进程RBA为0查看源端ggserr.log 发现端倪2018-06-27 15:47:42 INFO OGG-00987 Oracle GoldenGate Command Interpreter for Oracle: GGSCI command (…...