LLM之RAG实战(九)| 高级RAG 03:多文档RAG体系结构
在RAG(检索和生成)这样的框架内管理和处理多个文档有很大的挑战。关键不仅在于提取相关内容,还在于选择包含用户查询所寻求的信息的适当文档。基于用户查询对齐的多粒度特性,需要动态选择文档,本文将介绍结构化层次检索来解决多文档RAG问题。
一、Llamaindex结构化检索介绍
Llamaindex支持多层次信息检索。它不只是筛选文档,而是利用元数据过滤来简化选择过程。通过使用自动检索机制,这些过滤器可以根据用户查询检索出最相关的文档。这个过程包括推断语义查询,在矢量数据库中确定最佳过滤器集,有效地将文本到SQL和语义搜索的能力结合起来。
二、结构化层次检索的优点
下面介绍Llamaindex提供的结构化分层检索的一些好处:
-
增强相关性:通过利用元数据驱动的过滤器,可以准确地识别和检索符合用户查询细微要求的文档。这确保了内容选择中更高的相关性和准确性;
-
动态文档选择:与传统的静态文档检索是不同,Llamaindex支持动态文档选择。Llamaindex通过根据相关文档的属性和结构化元数据灵活选择相关文档,智能地适应不同的用户查询;
-
高效信息检索:结构化层次检索显著提高了信息检索的效率。通过将文档预处理到元数据字典中并将其存储在矢量数据库中,该系统简化了检索过程,最大限度地减少了计算开销并优化了搜索效率;
-
语义查询优化:文本到SQL和语义搜索的融合使系统能够更好地理解用户意图。Llamaindex的自动检索机制将用户查询细化为语义结构,从而能够从文档存储库中精确而细致地检索信息。
三、结构化层次检索代码实现
下面使用Python代码来展示Llamaindex的基本概念,并实现一个结构化的分层检索系统。使用Llamaindex类初始化来管理矢量数据库中的文档元数据。
- 文档添加:add_document方法通过创建包含摘要和关键字等关键信息的元数据字典,将文档添加到Llamaindex;
- 检索逻辑:retrieve_documents方法通过将用户查询与矢量数据库中的元数据过滤器进行匹配来处理用户查询。为了演示目的,使用了一个基本的模拟匹配逻辑;
- 匹配机制:match_metadata方法模拟用户查询和文档元数据之间的匹配过程。这是一个简化的演示逻辑,通常会使用更高级的NLP或语义分析技术。
本示例旨在说明Llamaindex的核心概念,展示如何通过Python中的简化实现来存储文档元数据并基于用户查询检索相关文档。
步骤1:安装库
!pip install llama-index wandb llama_hub weaviate-client --quiet
步骤2:导入库
import os
import openai
import logging
import sys
from IPython.display import Markdown, display
from llama_index.llms import OpenAI
from llama_index.callbacks import CallbackManager, WandbCallbackHandler
from llama_index import load_index_from_storage
import pandas as pd
from llama_index.query_engine import PandasQueryEngine
from pprint import pprint
from llama_index import (
VectorStoreIndex,
SimpleKeywordTableIndex,
SimpleDirectoryReader,
StorageContext,
ServiceContext,
)
import nest_asyncio
nest_asyncio.apply()
#Setup OPEN API Key
os.environ["OPENAI_API_KEY"] = ""
# openai_key = "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" #<--- Your API KEY
# openai.api_key = openai_key
logging.basicConfig(stream=sys.stdout, level=logging.INFO)
logging.getLogger().addHandler(logging.StreamHandler(stream=sys.stdout))
# initialise WandbCallbackHandler and pass any wandb.init args
wandb_args = {"project":"llama-index-report"}
wandb_callback = WandbCallbackHandler(run_args=wandb_args)
# pass wandb_callback to the service context
callback_manager = CallbackManager([wandb_callback])
service_context = ServiceContext.from_defaults(llm=OpenAI(model="gpt-3.5-turbo-0613", temperature=0), chunk_size=1024, callback_manager=callback_manager)
步骤3:下载Github issues
os.environ["GITHUB_TOKEN"] = ""
from llama_hub.github_repo_issues import (
GitHubRepositoryIssuesReader,
GitHubIssuesClient,
)
github_client = GitHubIssuesClient()
loader = GitHubRepositoryIssuesReader(
github_client,
owner="run-llama",
repo="llama_index",
verbose=True,
)
orig_docs = loader.load_data()
limit = 100
docs = []
for idx, doc in enumerate(orig_docs):
doc.metadata["index_id"] = doc.id_
if idx >= limit:
break
docs.append(doc)
# Output
Found 100 issues in the repo page 1
Resulted in 100 documents
Found 100 issues in the repo page 2
Resulted in 200 documents
Found 100 issues in the repo page 3
Resulted in 300 documents
Found 8 issues in the repo page 4
Resulted in 308 documents
No more issues found, stopping
from copy import deepcopy
import asyncio
from tqdm.asyncio import tqdm_asyncio
from llama_index import SummaryIndex, Document, ServiceContext
from llama_index.llms import OpenAI
from llama_index.async_utils import run_jobs
async def aprocess_doc(doc, include_summary: bool = True):
"""Process doc."""
print(f"Processing {doc.id_}")
metadata = doc.metadata
date_tokens = metadata["created_at"].split("T")[0].split("-")
year = int(date_tokens[0])
month = int(date_tokens[1])
day = int(date_tokens[2])
assignee = (
"" if "assignee" not in doc.metadata else doc.metadata["assignee"]
)
size = ""
if len(doc.metadata["labels"]) > 0:
size_arr = [l for l in doc.metadata["labels"] if "size:" in l]
size = size_arr[0].split(":")[1] if len(size_arr) > 0 else ""
new_metadata = {
"state": metadata["state"],
"year": year,
"month": month,
"day": day,
"assignee": assignee,
"size": size,
"index_id": doc.id_,
}
# now extract out summary
summary_index = SummaryIndex.from_documents([doc])
query_str = "Give a one-sentence concise summary of this issue."
query_engine = summary_index.as_query_engine(
service_context=ServiceContext.from_defaults(
llm=OpenAI(model="gpt-3.5-turbo")
)
)
summary_txt = str(query_engine.query(query_str))
new_doc = Document(text=summary_txt, metadata=new_metadata)
return new_doc
async def aprocess_docs(docs):
"""Process metadata on docs."""
new_docs = []
tasks = []
for doc in docs:
task = aprocess_doc(doc)
tasks.append(task)
new_docs = await run_jobs(tasks, show_progress=True, workers=5)
# new_docs = await tqdm_asyncio.gather(*tasks)
return new_docs
new_docs = await aprocess_docs(docs)
# Output
Processing 9398
Processing 9427
Processing 9613
Processing 9417
Processing 9612
Processing 8832
Processing 9609
Processing 9353
Processing 9431
Processing 9426
Processing 9425
Processing 9435
Processing 9419
Processing 9571
Processing 9373
Processing 9383
Processing 9408
Processing 9405
Processing 9372
Processing 9546
Processing 9565
Processing 9664
Processing 9560
Processing 9470
Processing 9343
Processing 9518
Processing 9358
Processing 8536
Processing 9385
Processing 9380
Processing 9510
Processing 9352
Processing 9368
Processing 7457
Processing 8893
Processing 9583
Processing 9312
Processing 7720
Processing 9219
Processing 9481
Processing 9469
Processing 9655
Processing 9477
Processing 9670
Processing 9475
Processing 9667
Processing 9665
Processing 9348
Processing 9471
Processing 9342
Processing 9488
Processing 9338
Processing 9523
Processing 9416
Processing 7726
Processing 9522
Processing 9652
Processing 9520
Processing 9651
Processing 7244
Processing 9650
Processing 9519
Processing 9649
Processing 9492
Processing 9603
Processing 9509
Processing 9269
Processing 9491
Processing 8802
Processing 9525
Processing 9611
Processing 9543
Processing 8551
Processing 9627
Processing 9450
Processing 9658
Processing 9421
Processing 9394
Processing 9653
Processing 9439
Processing 9604
Processing 9413
Processing 9507
Processing 9625
Processing 9490
Processing 9626
Processing 9483
Processing 9638
Processing 7744
Processing 9472
Processing 8475
Processing 9244
Processing 9618
100%|██████████| 100/100 [02:07<00:00, 1.27s/it]
步骤4:将数据加载到Weaviate Vector Store
from llama_index.vector_stores import WeaviateVectorStore
from llama_index.storage import StorageContext
from llama_index import VectorStoreIndex
import weaviate
# cloud
auth_config = weaviate.AuthApiKey(api_key="")
client = weaviate.Client(
"https://<weaviate-cluster>.weaviate.network",
auth_client_secret=auth_config,
)
class_name = "LlamaIndex_auto"
vector_store = WeaviateVectorStore(
weaviate_client=client, index_name=class_name
)
storage_context = StorageContext.from_defaults(vector_store=vector_store)
# Since "new_docs" are concise summaries, we can directly feed them as nodes into VectorStoreIndex
index = VectorStoreIndex(new_docs, storage_context=storage_context)
docs[0].metadata
# Output
{'state': 'open',
'created_at': '2023-12-21T20:18:03Z',
'url': 'https://api.github.com/repos/run-llama/llama_index/issues/9655',
'source': 'https://github.com/run-llama/llama_index/pull/9655',
'labels': ['size:L'],
'index_id': '9655'}
步骤5:对原始文档建立Weaviate Index
vector_store = WeaviateVectorStore(
weaviate_client=client, index_name=doc_class_name
)
storage_context = StorageContext.from_defaults(vector_store=vector_store)
doc_index = VectorStoreIndex.from_documents(
docs, storage_context=storage_context
)
步骤6:建立自动检索机制
自动检索器的设置过程通过分为以下几个关键步骤:
-
定义Schema:定义向量数据库模式,包括元数据字段;
-
VectorIndexAutoRetriever初始化:实例化此类将创建一个利用压缩元数据索引的检索器。需要定义的Schema作为其输入;
-
创建Wrapper Retriever:该步骤主要将每个节点后处理为IndexNode。此转换包含一个链接回源文档的索引ID,此链接支持在后面的部分中进行递归检索,依靠IndexNode对象与下游检索器、查询引擎或其他节点连接。
6(a)定义Schema
from llama_index.vector_stores.types import MetadataInfo, VectorStoreInfo
vector_store_info = VectorStoreInfo(
content_info="Github Issues",
metadata_info=[
MetadataInfo(
name="state",
description="Whether the issue is `open` or `closed`",
type="string",
),
MetadataInfo(
name="year",
description="The year issue was created",
type="integer",
),
MetadataInfo(
name="month",
description="The month issue was created",
type="integer",
),
MetadataInfo(
name="day",
description="The day issue was created",
type="integer",
),
MetadataInfo(
name="assignee",
description="The assignee of the ticket",
type="string",
),
MetadataInfo(
name="size",
description="How big the issue is (XS, S, M, L, XL, XXL)",
type="string",
),
],
)
6(b)实例化 VectorIndexAutoRetriever
from llama_index.retrievers import VectorIndexAutoRetriever
retriever = VectorIndexAutoRetriever(
index,
vector_store_info=vector_store_info,
similarity_top_k=2,
empty_query_top_k=10, # if only metadata filters are specified, this is the limit
verbose=True,
)
nodes = retriever.retrieve("Tell me about some issues on 12/11")
print(f"Number retrieved: {len(nodes)}")
print(nodes[0].metadata)
# Output
Using query str:
Using filters: [('month', '==', 12), ('day', '==', 11)]
Number retrieved: 6
{'state': 'open', 'year': 2023, 'month': 12, 'day': 11, 'assignee': '', 'size': 'XL', 'index_id': '9431'}
6(c)定义Wrapper Retriever
from llama_index.retrievers import BaseRetriever
from llama_index.indices.query.schema import QueryBundle
from llama_index.schema import IndexNode, NodeWithScore
class IndexAutoRetriever(BaseRetriever):
"""Index auto-retriever."""
def __init__(self, retriever: VectorIndexAutoRetriever):
"""Init params."""
self.retriever = retriever
def _retrieve(self, query_bundle: QueryBundle):
"""Convert nodes to index node."""
retrieved_nodes = self.retriever.retrieve(query_bundle)
new_retrieved_nodes = []
for retrieved_node in retrieved_nodes:
index_id = retrieved_node.metadata["index_id"]
index_node = IndexNode.from_text_node(
retrieved_node.node, index_id=index_id
)
new_retrieved_nodes.append(
NodeWithScore(node=index_node, score=retrieved_node.score)
)
return new_retrieved_nodes
index_retriever = IndexAutoRetriever(retriever=retriever)
步骤7:建立递归检索机制
这种类型的检索器将检索器的每个节点连接到另一个检索器、查询引擎或节点。该设置包括将每个汇总的元数据节点链接到与相应文档对应的RAG管道对齐的检索器。
配置过程如下:
-
为每个文档定义一个检索器,并把他们添加到字典中;
-
定义递归检索器:在参数中定义包括root检索器(汇总元数据检索器)和其他文档检索器。
from llama_index.vector_stores.types import (
MetadataFilter,
MetadataFilters,
FilterOperator,
)
retriever_dict = {}
query_engine_dict = {}
for doc in docs:
index_id = doc.metadata["index_id"]
# filter for the specific doc id
filters = MetadataFilters(
filters=[
MetadataFilter(
key="index_id", operator=FilterOperator.EQ, value=index_id
),
]
)
retriever = doc_index.as_retriever(filters=filters)
query_engine = doc_index.as_query_engine(filters=filters)
retriever_dict[index_id] = retriever
query_engine_dict[index_id] = query_engine
from llama_index.retrievers import RecursiveRetriever
# note: can pass `agents` dict as `query_engine_dict` since every agent can be used as a query engine
recursive_retriever = RecursiveRetriever(
"vector",
retriever_dict={"vector": index_retriever, **retriever_dict},
# query_engine_dict=query_engine_dict,
verbose=True,
)
nodes = recursive_retriever.retrieve("Tell me about some issues on 12/11")
print(f"Number of source nodes: {len(nodes)}")
nodes[0].node.metadata
# Output
Retrieving with query id None: Tell me about some issues on 12/11
Using query str:
Using filters: [('month', '==', 12), ('day', '==', 11)]
Retrieved node with id, entering: 9431
Retrieving with query id 9431: Tell me about some issues on 12/11
Retrieving text node: Dev awiss
# Description
Try to use clickhouse as vectorDB.
Try to chunk docs with independent parser service.
Special designed schema and tricks for better query and retriever.
Fixes # (issue)
## Type of Change
Please delete options that are not relevant.
- [ ] Bug fix (non-breaking change which fixes an issue)
- [ ] New feature (non-breaking change which adds functionality)
- [ ] Breaking change (fix or feature that would cause existing functionality to not work as expected)
- [ ] This change requires a documentation update
# How Has This Been Tested?
Please describe the tests that you ran to verify your changes. Provide instructions so we can reproduce. Please also list any relevant details for your test configuration
- [ ] Added new unit/integration tests
- [ ] Added new notebook (that tests end-to-end)
- [ ] I stared at the code and made sure it makes sense
# Suggested Checklist:
- [ ] I have performed a self-review of my own code
- [ ] I have commented my code, particularly in hard-to-understand areas
- [ ] I have made corresponding changes to the documentation
- [ ] I have added Google Colab support for the newly added notebooks.
- [ ] My changes generate no new warnings
- [ ] I have added tests that prove my fix is effective or that my feature works
- [ ] New and existing unit tests pass locally with my changes
- [ ] I ran `make format; make lint` to appease the lint gods
Retrieved node with id, entering: 9435
Retrieving with query id 9435: Tell me about some issues on 12/11
Retrieving text node: [Bug]: [nltk_data] Error loading punkt: <urlopen error [WinError 10060] A
### Bug Description
I am using a vector Index which connects to a chromaDB client as my database. I have initialized the index as a chat engine. When the query the chat engine, two things happen:
1. The response time is nearly 2-3mins.
2. It throws the below warning
```
[nltk_data] Error loading punkt: <urlopen error [WinError 10060] A
[nltk_data] connection attempt failed because the connected party
[nltk_data] did not properly respond after a period of time, or
[nltk_data] established connection failed because connected host
[nltk_data] has failed to respond>
```
### Version
0.9.8.post1
### Steps to Reproduce
Clone, setup and run the below repository: (Follow readme for instructions)
https://github.com/umang299/document-gpt
### Relevant Logs/Tracbacks
_No response_
Retrieved node with id, entering: 9426
Retrieving with query id 9426: Tell me about some issues on 12/11
Retrieving text node: Slack Loader with large lack channels
### Question Validation
- [X] I have searched both the documentation and discord for an answer.
### Question
Hi team,
I am using the [Slack Loader ](https://llamahub.ai/l/slack)from Llama Hub. For smaller Slack channels it works fine. However, for larger channels with lots of messages created over months, I keep seeing this message:
`Rate limit error reached, sleeping for: 10 seconds`
Is there a recommended / idiomatic way to load larger Slack channels to avoid this issue?
Retrieved node with id, entering: 9425
Retrieving with query id 9425: Tell me about some issues on 12/11
Retrieving text node: [Feature Request]: Make llama-index compartible with models finetuned and hosted on modal.com
### Feature Description
Modal.com is a cloud computing service that allows you to finetune and host models on their workers. They provide inference points for any models finetuned on their platform.
### Reason
I have not tried implementing the feature. I just read about the capabilities on modal.com and thought it would be a good integration feature for llama-index to allow for more configuration.
### Value of Feature
An integration feature to allow users who host their models on modal to use llama-index for their RAG and prompt engineering pipelines.
Retrieved node with id, entering: 9439
Retrieving with query id 9439: Tell me about some issues on 12/11
Retrieving text node: [Bug]: Metadata filter not working with Elastic search indexing
### Bug Description
While retrieving from ES with multiple metadatafilter condition(OR/AND) its not taking it into account. It always performs an AND operation even if its explicitly mentioned OR.
Example below code should filter and retrieve only 'mafia' or "Stephen King" bit its not doing as expected.
filters = MetadataFilters(
filters=[
MetadataFilter(key="theme", value="Mafia"),
MetadataFilter(key="author", value="Stephen King"),
],
condition=FilterCondition.OR,
)
retriever = index.as_retriever(filters=filters)
### Version
0.9.13
### Steps to Reproduce
nodes = [
TextNode(
text="The Shawshank Redemption",
metadata={
"author": "Stephen King",
"theme": "Friendship",
},
),
TextNode(
text="The Godfather",
metadata={
"director": "Francis Ford Coppola",
"theme": "Mafia",
},
),
TextNode(
text="Inception",
metadata={
"director": "Christopher Nolan",
},
),
]
filters = MetadataFilters(
filters=[
MetadataFilter(key="theme", value="Mafia"),
MetadataFilter(key="author", value="Stephen King"),
],
condition=FilterCondition.OR,
)
retriever = index.as_retriever(filters=filters)
### Relevant Logs/Tracbacks
_No response_
Retrieved node with id, entering: 9427
Retrieving with query id 9427: Tell me about some issues on 12/11
Retrieving text node: [Feature Request]: Postgres BM25 support
### Feature Description
Feature: add a variation of PGVectorStore which uses ParadeDB's BM25 extension.
BM25 is now possible in Postgres with a Rust extension [pg_bm25): https://github.com/paradedb/paradedb/tree/dev/pg_bm25
Unsure if it might be better to use [pg_search](https://github.com/paradedb/paradedb/tree/dev/pg_search) and get HNSW at the same time..
I'm interested in contributing on this myself, but am just starting to look into it. Interested to hear others' thoughts.
### Reason
Although the code comments for the PGVectorStore class currently suggest BM25 search is present in Postgres - it is not.
### Value of Feature
BM25 retrieval hit rate and MRR is measurable better than Postgres full text search with tsvector and tsquery. Indexing is also supposed to be faster with pg_bm25.
Number of source nodes: 6
{'state': 'open',
'created_at': '2023-12-11T10:17:52Z',
'url': 'https://api.github.com/repos/run-llama/llama_index/issues/9431',
'source': 'https://github.com/run-llama/llama_index/pull/9431',
'labels': ['size:XL'],
'index_id': '9431'}
步骤8:插入RetrieverQueryEngine
from llama_index.query_engine import RetrieverQueryEngine
from llama_index import ServiceContext
llm = OpenAI(model="gpt-3.5-turbo")
service_context = ServiceContext.from_defaults(llm=llm)
query_engine = RetrieverQueryEngine.from_args(recursive_retriever, llm=llm)
response = query_engine.query(
"Tell me about some open issues related to agents"
)
print(str(response))
# Output
There were several issues created on 12/11. One of them is a bug where the metadata filter is not working correctly with Elastic search indexing. Another bug involves an error loading the 'punkt' module in the NLTK library. There are also a couple of feature requests, one for adding Postgres BM25 support and another for making llama-index compatible with models finetuned and hosted on modal.com. Additionally, there is a question about using the Slack Loader with large Slack channels.
四、结论
总之,将Llamaindex集成到多文档RAG架构的结构中预示着信息检索的新时代。它能够基于结构化元数据动态选择文档,再加上语义查询优化的技巧,重塑了我们如何利用庞大文档存储库中的知识,提高了检索过程的效率、相关性和准确性。
参考文献:
[1] https://ai.gopubby.com/structured-hierarchical-retrieval-revolutionizing-multi-document-rag-architectures-f101463db689
[2] https://weaviate.io/developers/wcs/quickstart
[3] https://docs.llamaindex.ai/en/stable/examples/query_engine/multi_doc_auto_retrieval/multi_doc_auto_retrieval.html
相关文章:
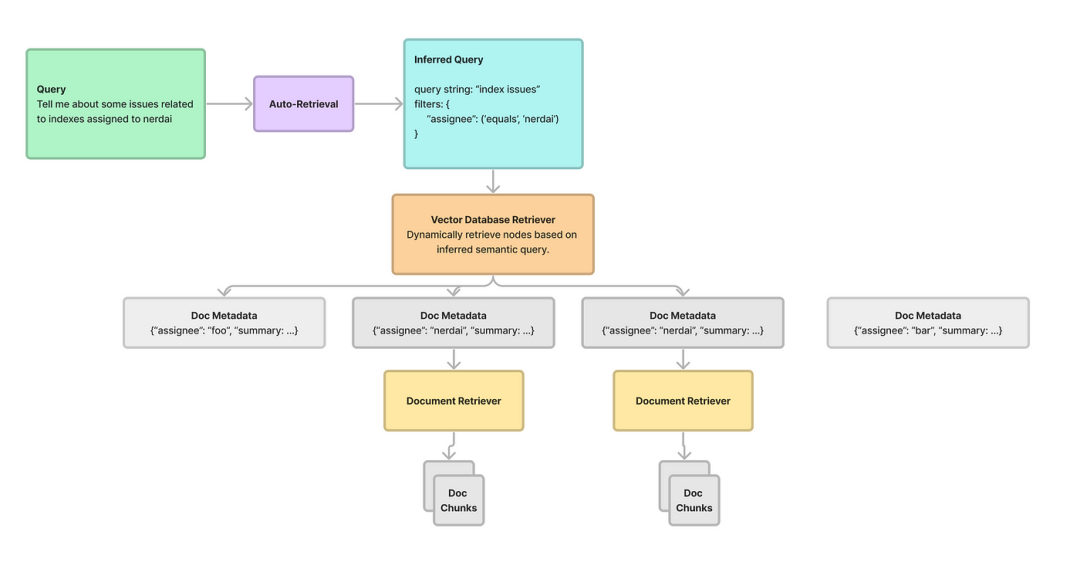
LLM之RAG实战(九)| 高级RAG 03:多文档RAG体系结构
在RAG(检索和生成)这样的框架内管理和处理多个文档有很大的挑战。关键不仅在于提取相关内容,还在于选择包含用户查询所寻求的信息的适当文档。基于用户查询对齐的多粒度特性,需要动态选择文档,本文将介绍结构化层次检索…...
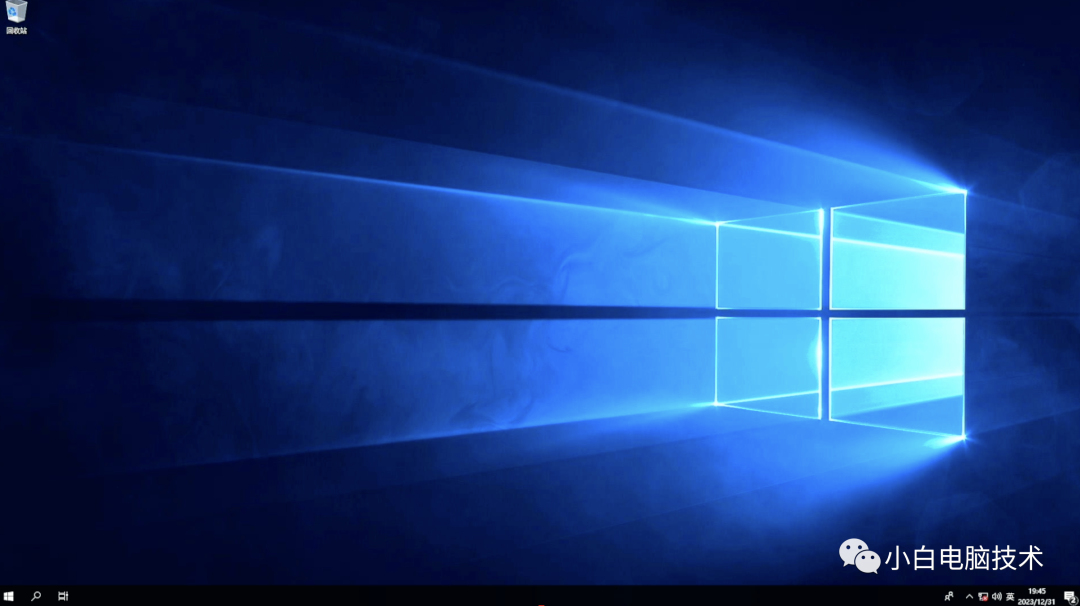
Windows电脑引导损坏?按照这个教程能修复
前言 Windows系统的引导一般情况下是不会坏的,小伙伴们可以不用担心。发布这个帖子是因为要给接下来的文章做点铺垫。 关注小白很久的小伙伴应该都知道,小白的文章都讲得比较细。而且文章与文章之间的关联度其实还是蛮高的。在文章中,你会遇…...

记Android字符串资源支持的参数类型
参数以%开头,后拼接对应的参数类型名称,如下所示: <string name"tips">Hello, %s! You have some new messages.</string> 类型名称如下所示: s字符串格式用于插入字符串值。例如,"Hel…...
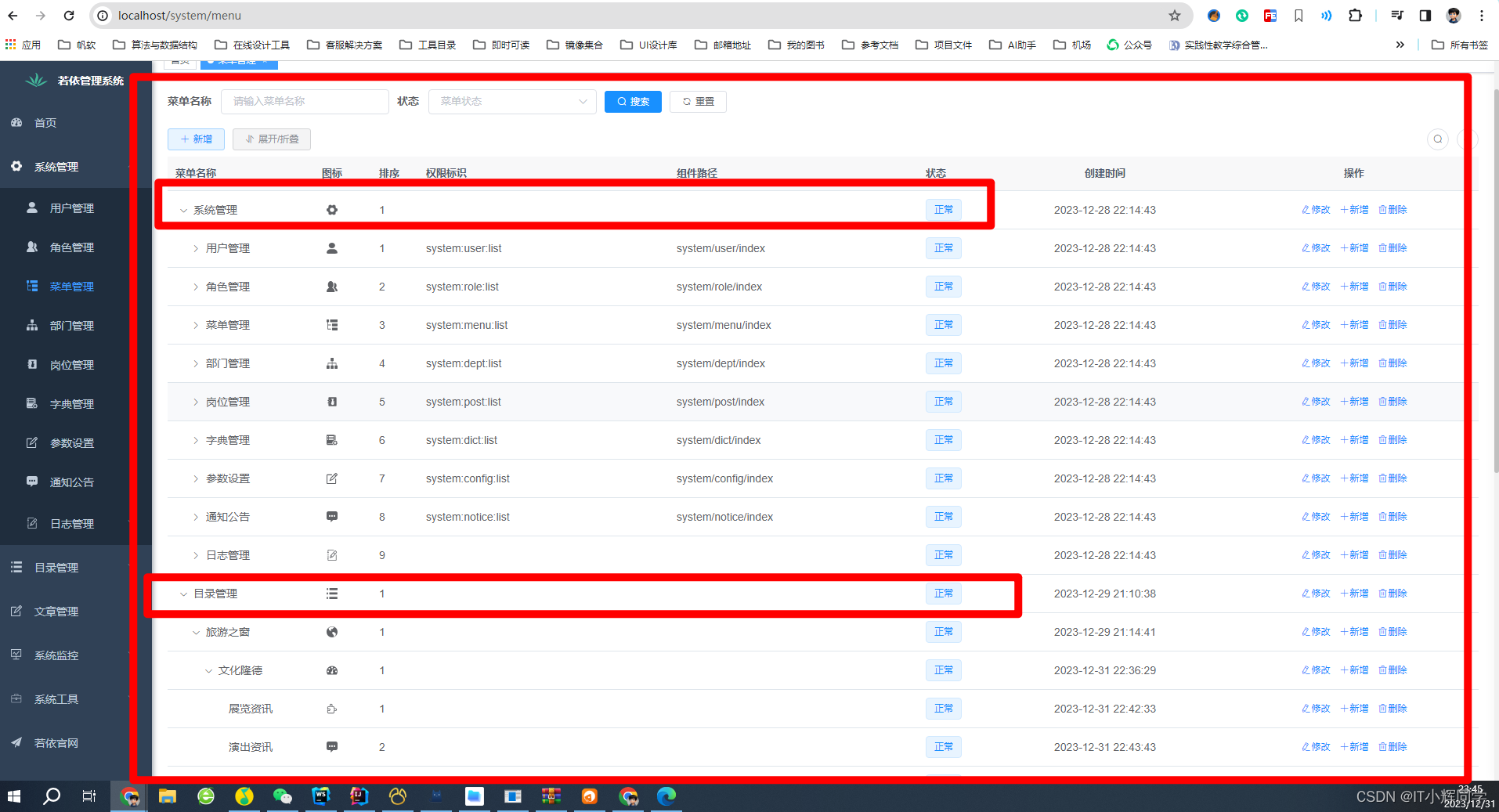
Java实现树结构(为前端实现级联菜单或者是下拉菜单接口)
Java实现树结构(为前端实现级联菜单或者是下拉菜单接口) 我们常常会遇到这样一个问题,就是前端要实现的样式是一个级联菜单或者是下拉树,如图 这样的数据接口是怎么实现的呢,是什么样子的呢? 我们可以看看 …...

MySQL中常用的数据类型
整型 int 有符号范围: -2147483648 ~ 2147483647 int unsigned 无符号范围: 0 ~ 4294967295 int(5) zerofill 仅用于显示,当不满足5位时,按照左边补0,例如: 00002满足时,正常显示 tinyint[(m)] [unsigned] [zerofill] 有符号&a…...
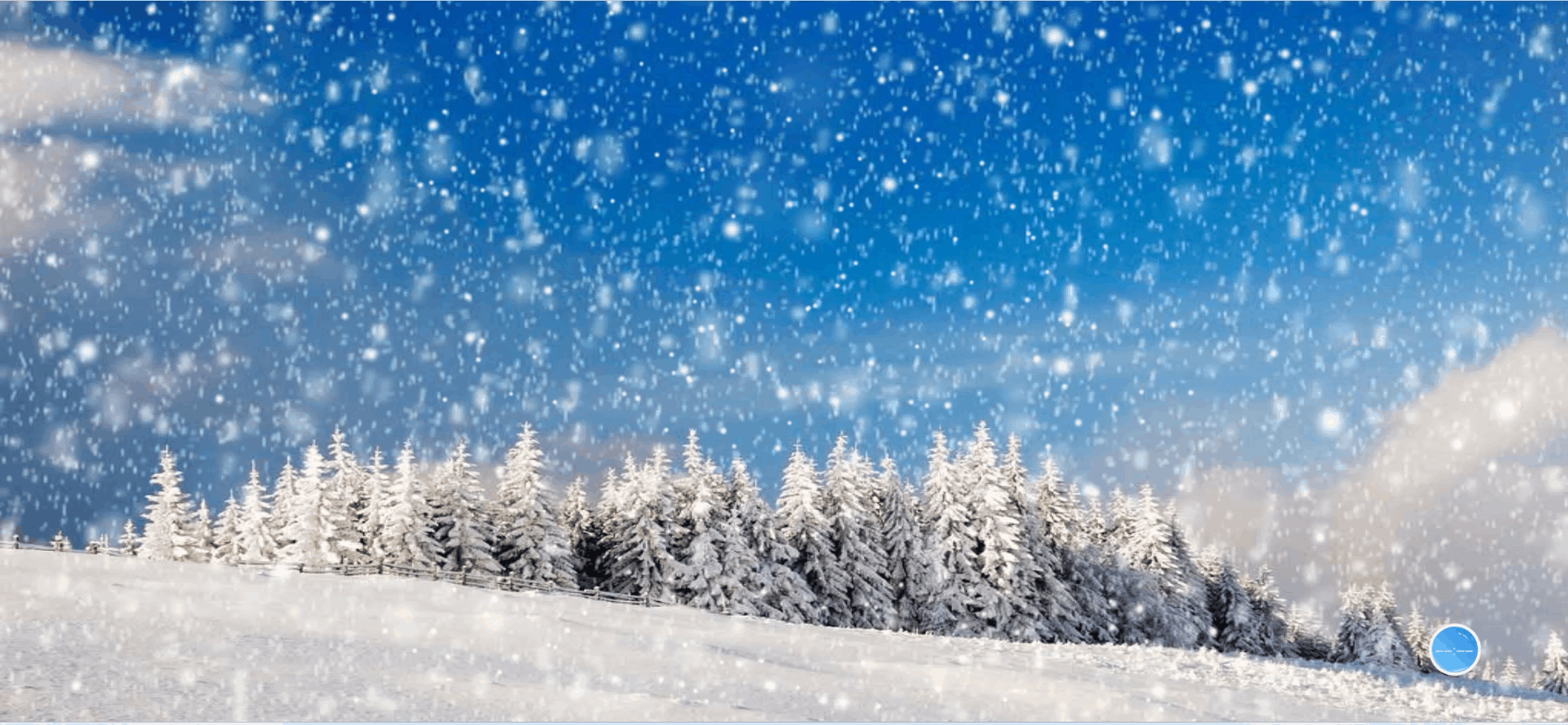
HTML+CSS+JS制作三款雪花酷炫特效
🎀效果展示 🎀代码展示 <!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd"> <html...
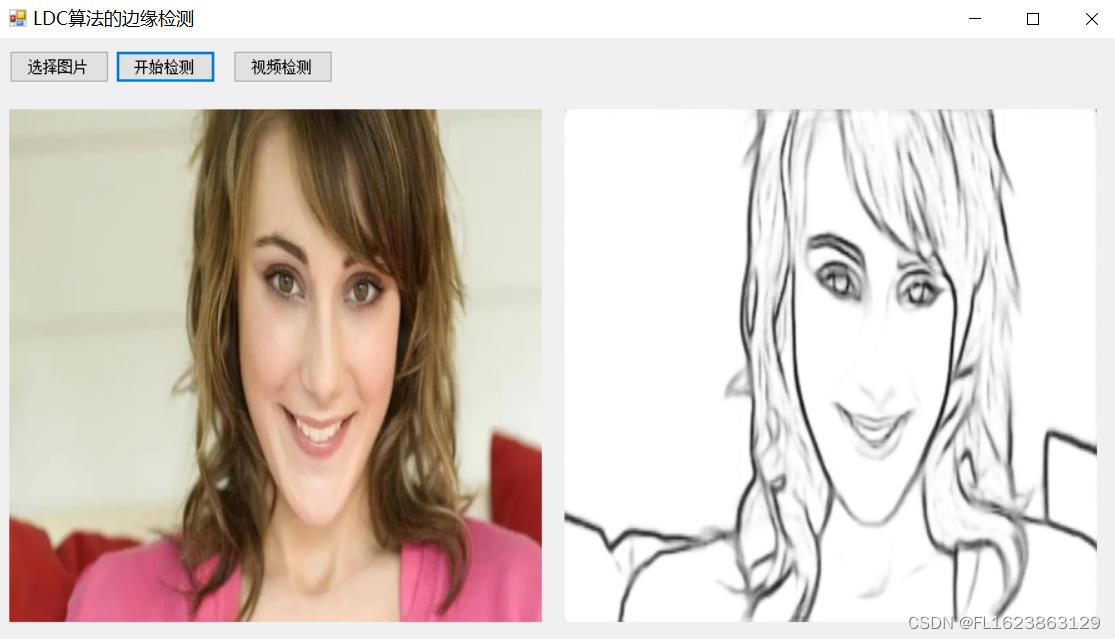
[C#]使用ONNXRuntime部署一种用于边缘检测的轻量级密集卷积神经网络LDC
源码地址: github.com/xavysp/LDC LDC: Lightweight Dense CNN for Edge Detection算法介绍: 由于深度学习方法的快速发展,近年来,用于执行图像边缘检测的卷积神经网络(CNN)模型爆炸性地传播。但边缘检测…...
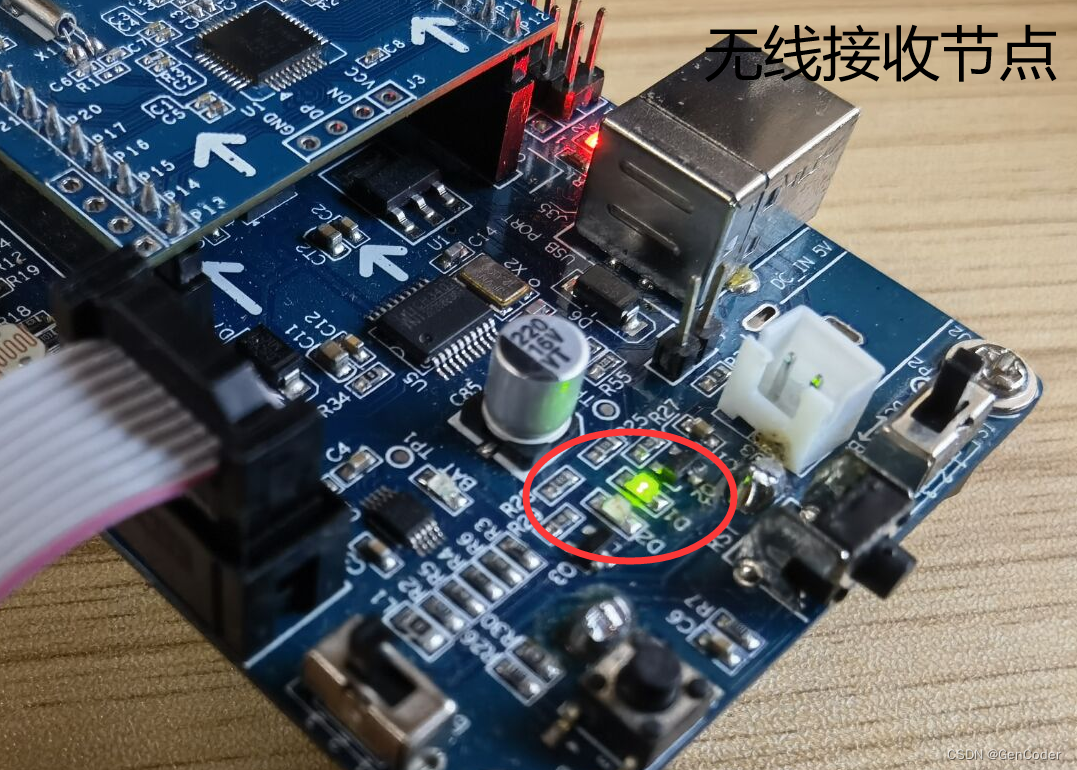
ZigBee案例笔记 - 无线点灯
文章目录 无线点灯实验概述工程关键字工程文件夹介绍Basic RF软件设计框图简单说明工程操作Basic RF启动流程Basic RF发送流程Basic RF接收流程 无线点灯案例无线点灯现象 无线点灯实验概述 ZigBee无线点灯实验(即Basic RF工程),由TI公司提供…...
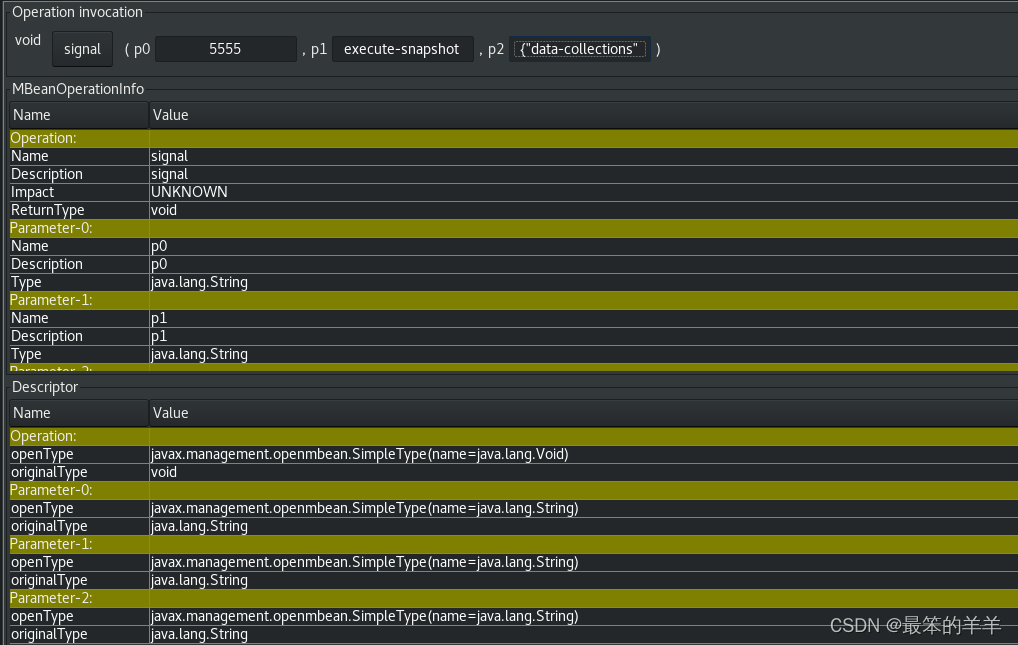
Debezium日常分享系列之:向 Debezium 连接器发送信号
Debezium日常分享系列之:向 Debezium 连接器发送信号 一、概述二、激活源信号通道三、信令数据集合的结构四、创建信令数据集合五、激活kafka信号通道六、数据格式七、激活JMX信号通道八、自定义信令通道九、Debezium 核心模块依赖项十、部署自定义信令通道十一、信…...

《C#程序设计教程》总复习
一、单项选择题 1.short 类型的变量在内存中占据的位数是 ( )。 A. 8 B. 16 C. 32 D. 64 2.对千 int[ 4,5]型的数组 a, 数组元素 a[2,3] 存在数组第 ( )个位置上。 A. 11 B. 12 C. 14 D. 15 3.设 int 类型变量 x,y,z 的值分别是2、3、6 , 那么…...

为什么ChatGPT选择了SSE,而不是WebSocket?
我在探索ChatGPT的使用过程中,发现了一个有趣的现象:ChatGPT在实现流式返回的时候,选择了SSE(Server-Sent Events),而非WebSocket。 那么问题来了:为什么ChatGPT选择了SSE,而不是We…...
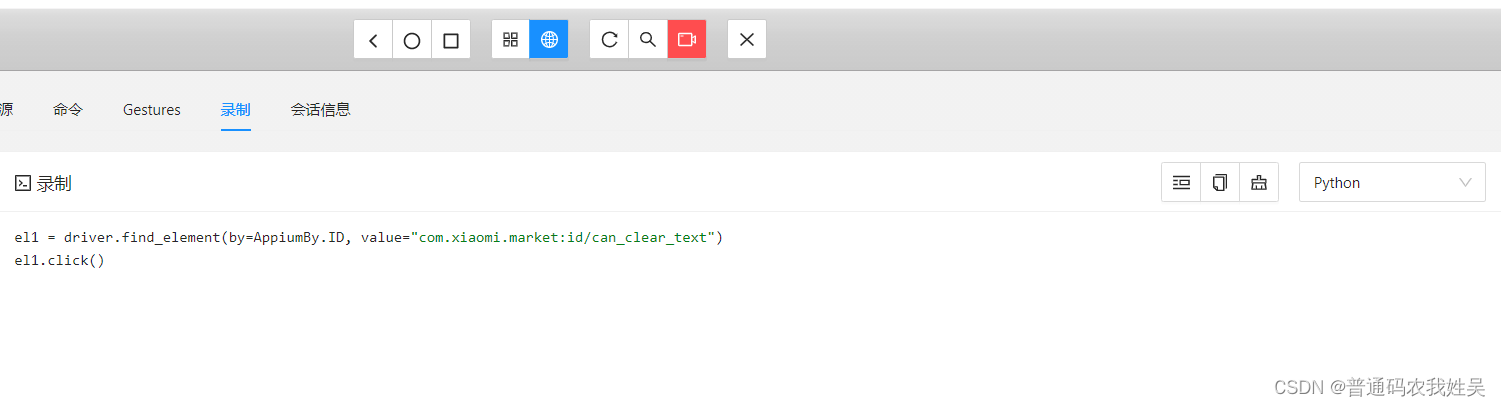
appium入门基础
介绍 appium支持在不同平台的UI自动化,如web,移动端,桌面端等。还支持使用java,python,js等语言编写自动化代码。主要用于自动化测试脚本,省去重复的手动操作。 Appium官网 安装 首先必须环境有Node.js用于安装Appium。 总体来…...
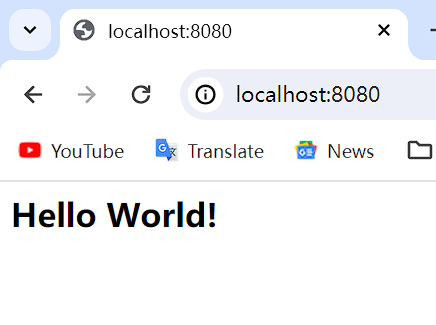
jsp介绍
JSP 一种编写动态网页的语言,可以嵌入java代码和html代码,其底层本质上为servlet,html部分为输出流,编译为java文件 例如 源jsp文件 <% page contentType"text/html; charsetutf-8" language"java" pageEncoding&…...

Debian安装k8s记录
Debian安装k8s记录 在master和node上安装kube安装master安装node遇到的问题汇总1、kubelet.service报错 failed to pull image "registry.k8s.io/pause:3.6"2、node重启后报错,failed: open /run/flannel/subnet.env: no such file or directory 在master…...
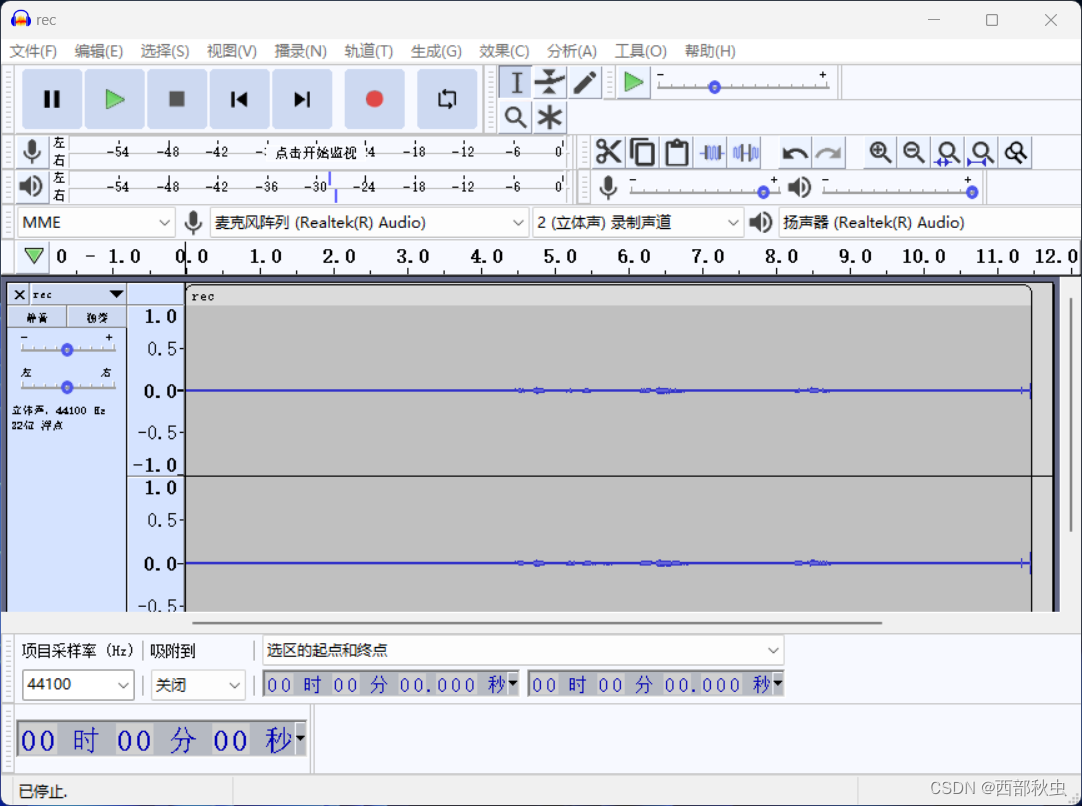
第6课 用window API捕获麦克风数据并加入队列备用
今天是2024年1月1日,新年的第一缕阳光已经普照大地,祝愿看到这篇文章的所有程序员或程序爱好者都能在新的一年里持之以恒,事业有成。 今天也是我加入CSDN的第4100天,但回过头看一看,这么长的时间也没有在CSDN写下几篇…...
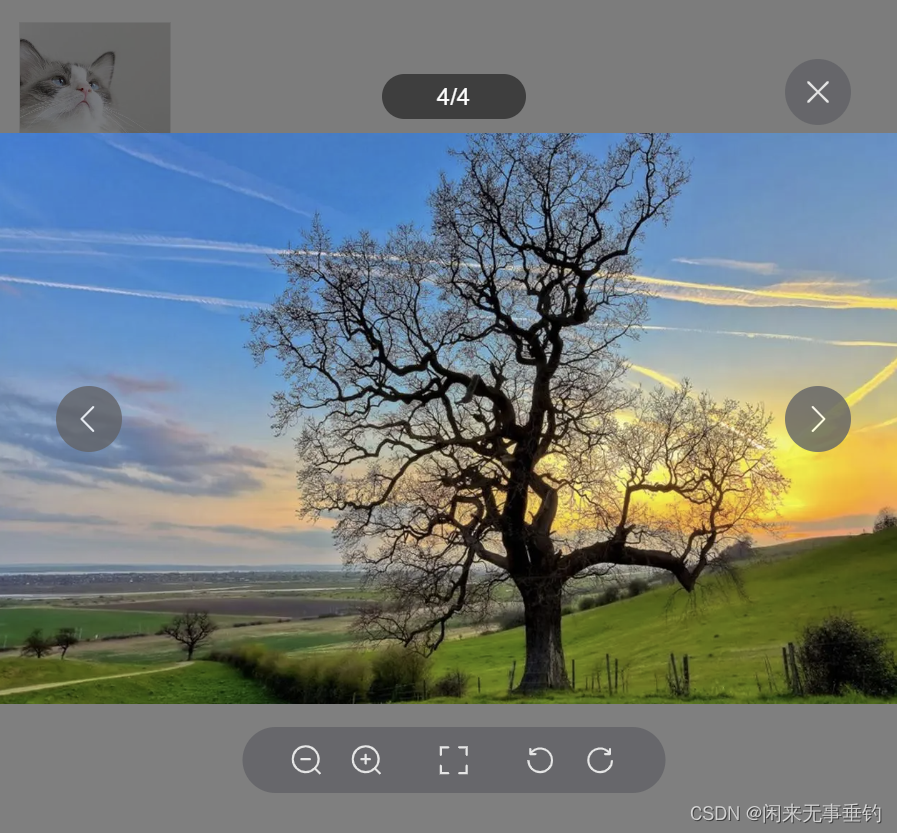
图片预览 element-plus 带页码
vue3、element-plus项目中,点击预览图片,并显示页码效果如图 安装 | Element Plus <div class"image__preview"><el-imagestyle"width: 100px; height: 100px":src"imgListArr[0]":zoom-rate"1.2":max…...
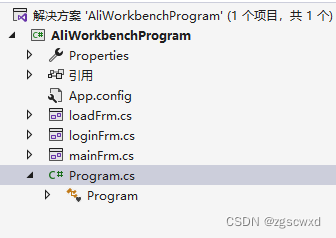
【小白专用】winform启动界面+登录窗口 更新2024.1.1
需求场景:先展示启动界面,然后打开登录界面,如果登录成功就跳转到主界面 首先在程序的入口路径加载启动界面,使用ShowDialog显示界面, 然后在启动界面中添加定时器,来实现显示一段时间的效果,等…...
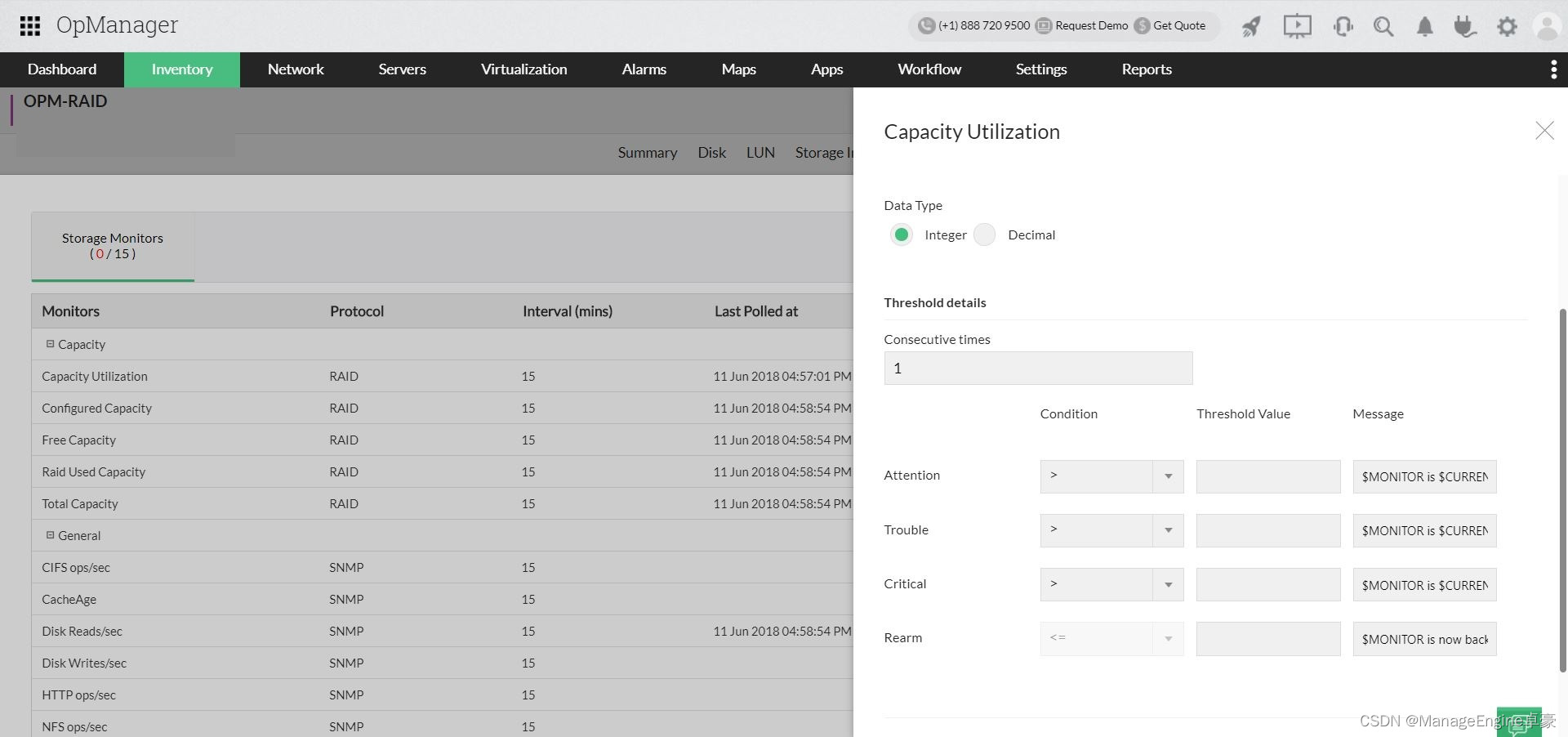
自动化网络故障修复管理
什么是故障管理 故障管理是网络管理的组成部分,涉及检测、隔离和解决问题。如果实施得当,网络故障管理可以使连接、应用程序和服务保持在最佳水平,提供容错能力并最大限度地减少停机时间。专门为此目的设计的平台或工具称为故障管理系统。 …...
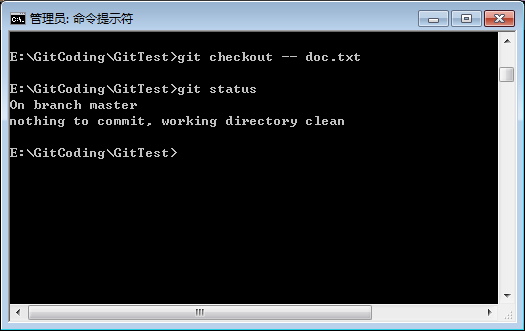
Git:常用命令(二)
查看提交历史 1 git log 撤消操作 任何时候,你都有可能需要撤消刚才所做的某些操作。接下来,我们会介绍一些基本的撤消操作相关的命令。请注意,有些操作并不总是可以撤消的,所以请务必谨慎小心,一旦失误,…...

Oracle 12c rac 搭建 dg
环境 rac 环境 (主)byoradbrac 系统版本:Red Hat Enterprise Linux Server release 6.5 软件版本:Oracle Database 12c Enterprise Edition Release 12.1.0.2.0 - 64bit byoradb1:172.17.38.44 byoradb2:…...
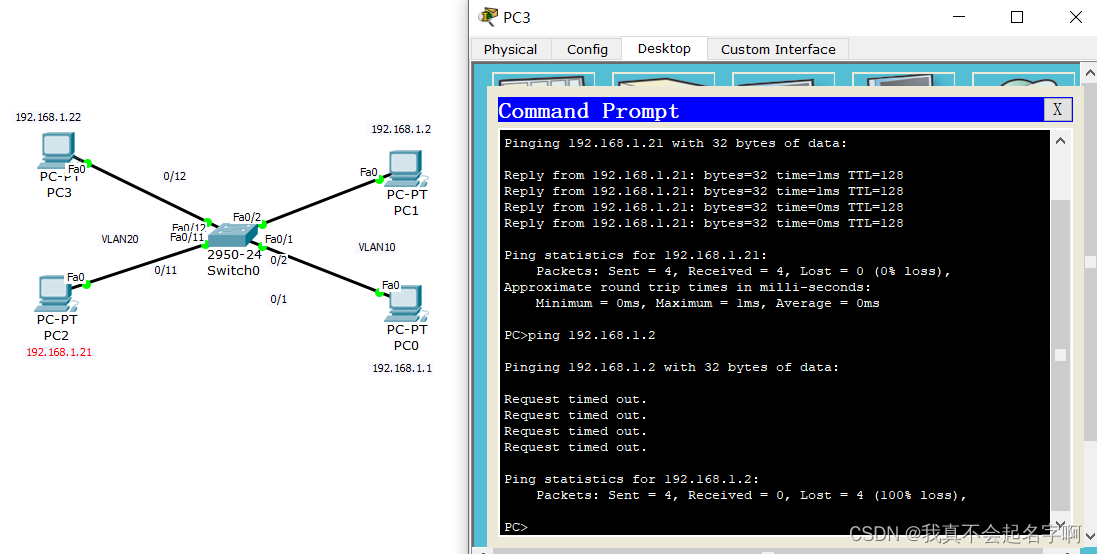
Cisco模拟器-交换机端口的隔离
设计要求将某台交换机的端口划分在不同的VLAN。以实现连接在相同VLAN端口上的计算机可以通信,而连接在不同VLAN端口上的计算机无法通信的目的。 通过设计,一方面可以加强计算机网络的安全,另一方面通过隔绝不同VLAN间的广播包也可以提高网络…...

zdppy_api框架快速入门
概述 zdppy_api是一款为了快速开发而生的,基于异步的,使用简单的Python后端API接口开发框架。 本框架的目标是让Python后端开发变得越来越简单,直到发现原来还可以更简单! 一切都是为了提高开发效率!!&…...

https证书配置过程
相关网址: FreeSSL首页 - FreeSSL.cn一个提供免费HTTPS证书申请的网站 ACME v2证书自动化快速入门 acme.sh简单教程-CSDN博客...

如何用C语言程序生成任意手性(即具有任意m和n值),任意长度的碳纳米管,并输出三维空间坐标呢?
如何用C语言程序生成任意手性(即具有任意m和n值),任意长度的碳纳米管,并输出三维空间坐标呢? 生成任意手性、任意长度的碳纳米管可以使用 Chirality Vector 和 Unit Vector 的概念来表示。Chirality Vector (n, m) 描述…...
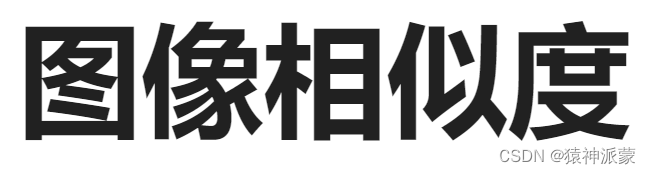
C++每日一练(8):图像相似度
题目描述 给出两幅相同大小的黑白图像(用0-1矩阵)表示,求它们的相似度。 说明:若两幅图像在相同位置上的像素点颜色相同,则称它们在该位置具有相同的像素点。两幅图像的相似度定义为相同像素点数占总像素点数的百分比。…...
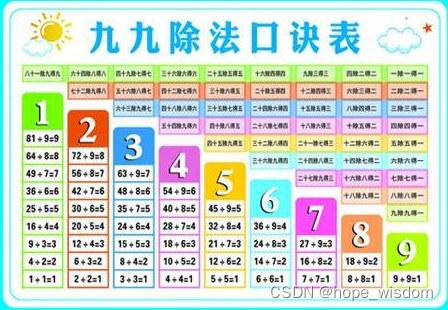
C++面试宝典第12题:数组元素相除
题目 从控制台输入若干个整数作为数组,将数组中每一个元素除以第一个元素的结果,作为新的数组元素值。比如:可以先输入3,作为数组元素的个数;然后输入3个整数,作为数组元素的值。 解析 这道题本身并不复杂,但里面隐藏了不少“坑点”和“雷区”,主要考察应聘者全面思考问…...

oCPC实践录 | 目标ROI的出价与转化回传调控算法
这篇文章我们聊聊广告主在oCPC下,怎么调控自己的出价或者回传转化优化自己的ROI。 ROI是广告主最关心的指标了,根据oCPC出价的基本原理ocpc_bid pcvr * given_cpa * k, 广告主在整个出价中有两个可以控制的变量来影响出价,一个是直接的give…...

百倍量化之Dbcd-v2中性策略
Dbcd-v2中性策略 1. 指标含义 该指标主要是计算偏置的因子,并根据偏置的平均来分析这个股票的稳定性。相比于v1,策略是更换了dbcd的计算方式 第一步主要操作就是计算当前值和前段时间的平均值的偏置 ma = bt.indicators.SimpleMovingAverage(self.data, period=self.p.peri…...

系统学习Python——装饰器:函数装饰器-[装饰器状态保持方案:函数属性]
分类目录:《系统学习Python》总目录 如果我们没有在使用Python3.X并因此无法利用一条nonlocal语句,或者我们希望代码具有可移植性,能在Python3.X和Python2.X上同时工作一一我们仍然能够针对某些可改变的状态使用函数属性来避免使用全局变量和…...
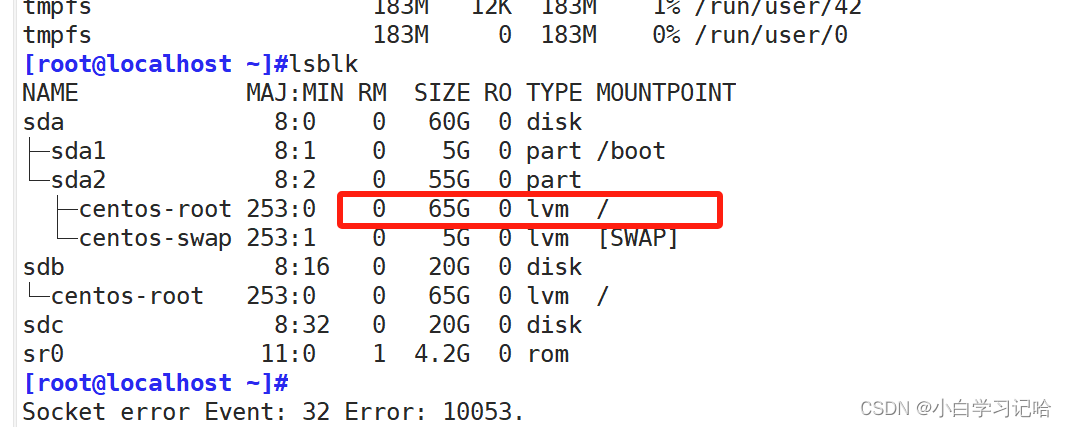
逻辑卷学习后续----------缩容
一、缩容:缩减大小 ext4可以 , xfs无法缩减,缩减会影响业务 1.解挂载 2.检查文件系统完整性 3.缩减文件系统 4.缩减逻辑卷上下一致 5.再挂载回去 添加磁盘 文件系统只能装ext4 缩减文件系统 resize2fs 挂载失败需要重新安装文件系统…...
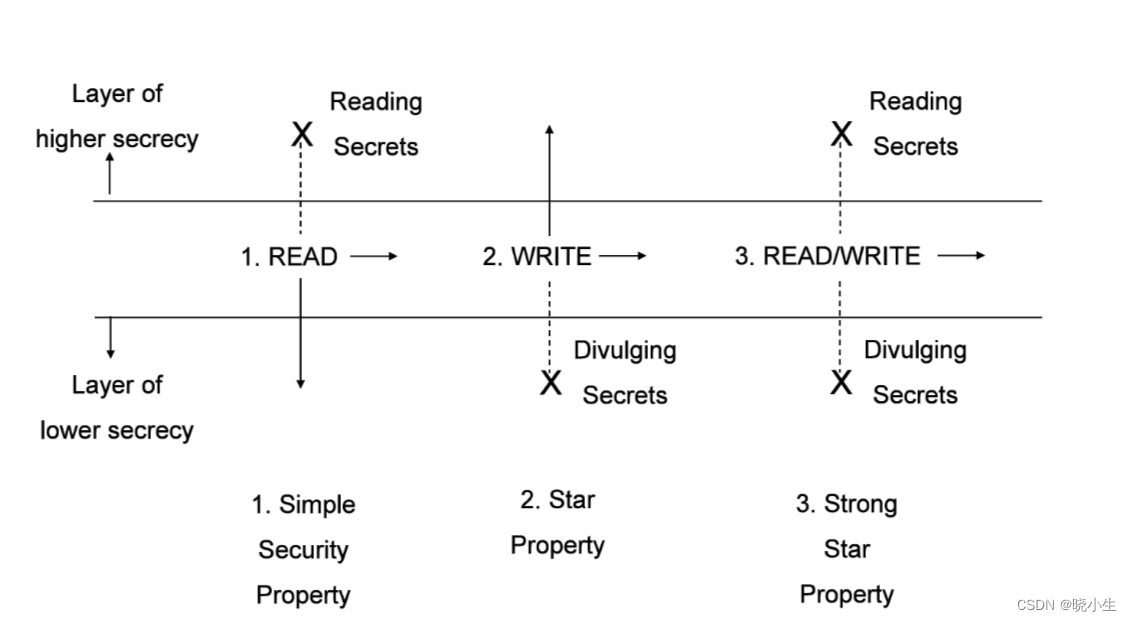
15-网络安全框架及模型-BLP机密性模型
目录 BLP机密性模型 1 背景概述 2 模型原理 3 主要特性 4 优势和局限性 5 困难和挑战 6 应用场景 7 应用案例 BLP机密性模型 1 背景概述 BLP模型,全称为Bell-LaPadula模型,是在1973年由D.Bell和J.LaPadula在《Mathematical foundations and mod…...
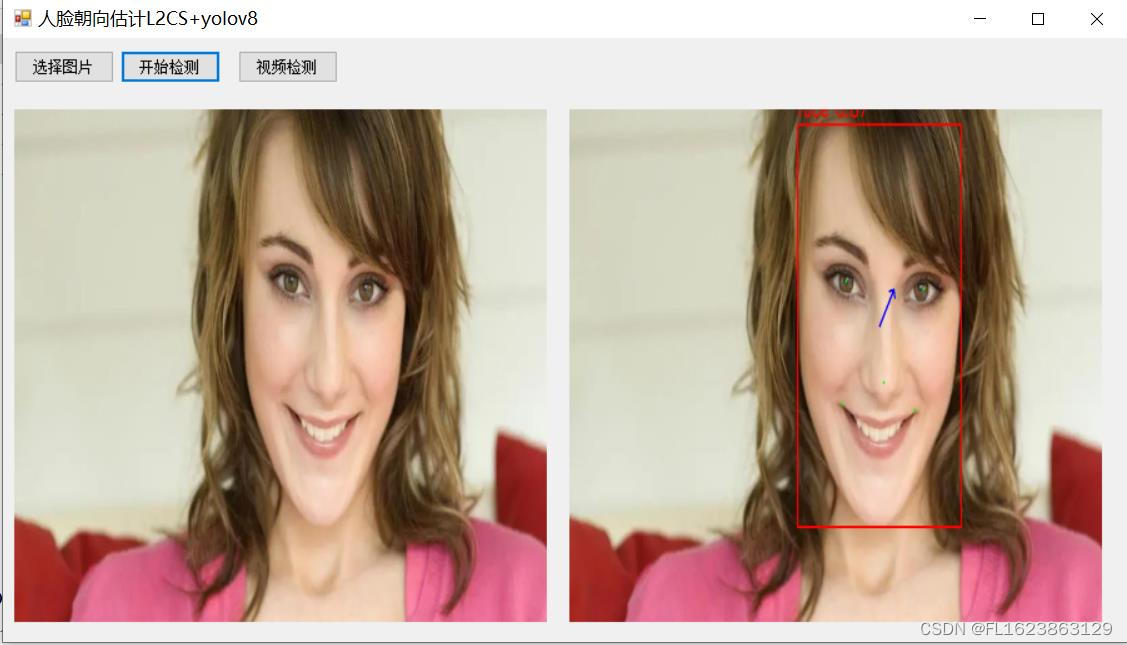
[C#]OpenCvSharp结合yolov8-face实现L2CS-Net眼睛注视方向估计或者人脸朝向估计
源码地址: github地址:https://github.com/Ahmednull/L2CS-Net L2CS-Net介绍: 眼睛注视(eye gaze) 是在各种应用中使用的基本线索之一。 它表示用户在人机交互和开放对话系统中的参与程度。此外,它还被用…...

[2024区块链开发入门指引] - 比特币与区块链诞生
一份为小白用户准备的免费区块链基础教程 工欲善其事,必先利其器 Web3开发中,各种工具、教程、社区、语言框架.。。。 种类繁多,是否有一个包罗万象的工具专注与Web3开发和相关资讯能毕其功于一役? 参见另一篇博文👉 2024最全面…...
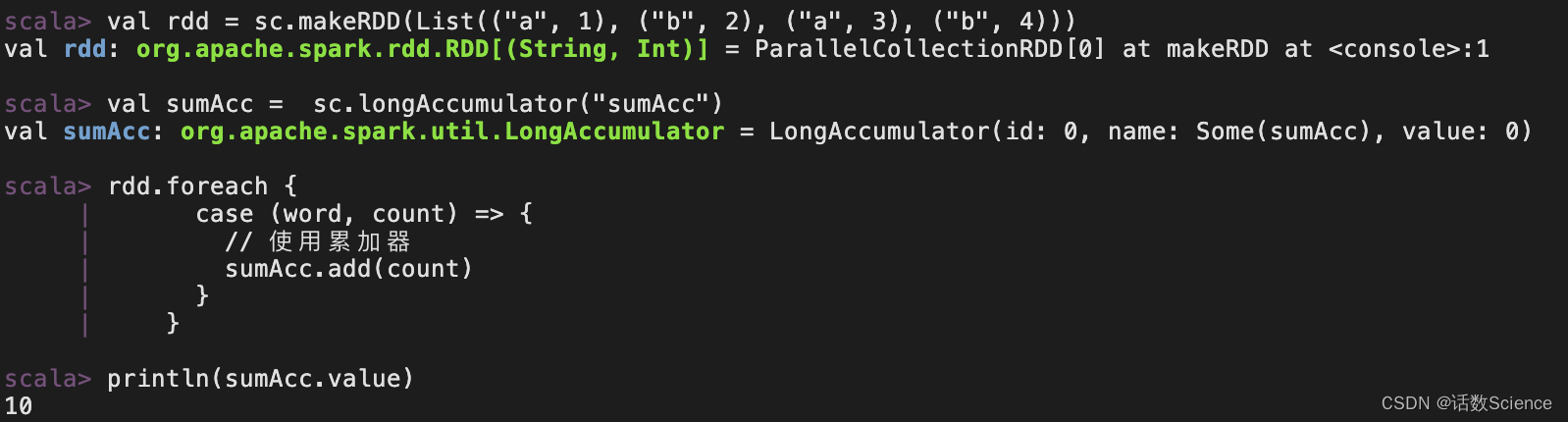
【大数据面试知识点】Spark中的累加器
Spark累加器 累加器用来把Executor端变量信息聚合到Driver端,在driver程序中定义的变量,在Executor端的每个task都会得到这个变量的一份新的副本,每个task更新这些副本的值后,传回driver端进行merge。 累加器一般是放在行动算子…...
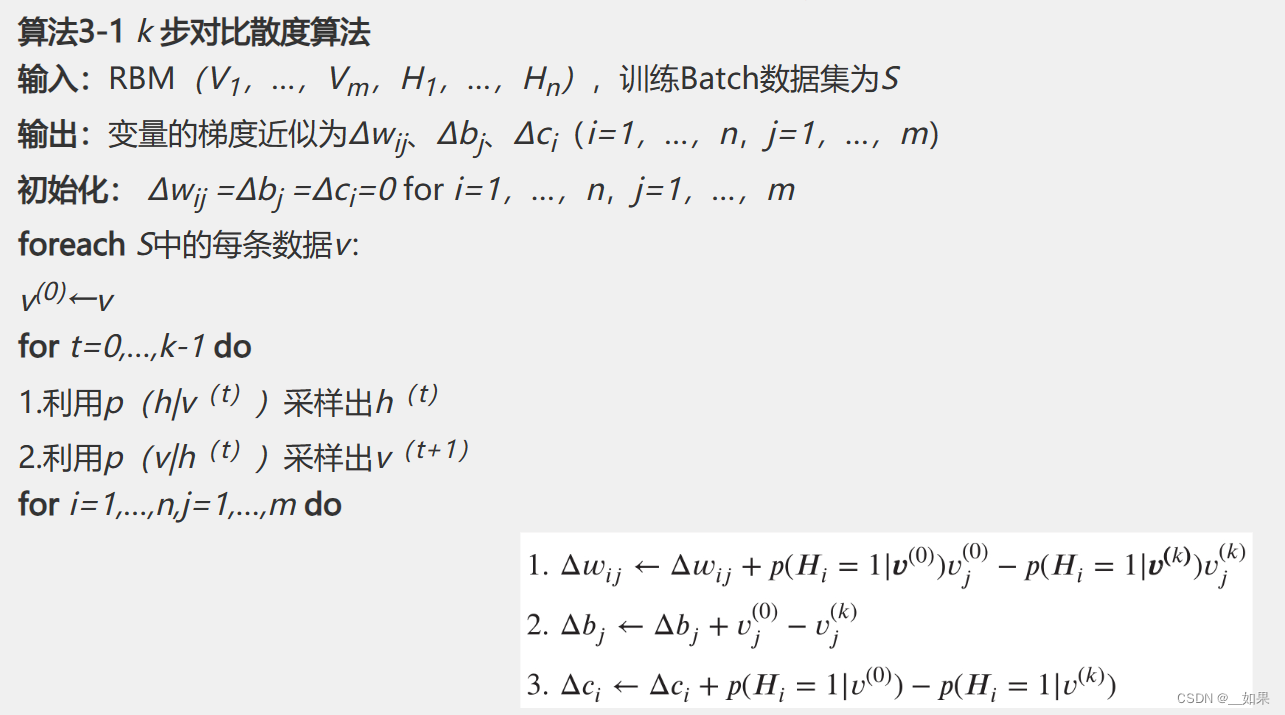
深度学习核心技术与实践之深度学习基础篇
非书中全部内容,只是写了些自认为有收获的部分 神经网络 生物神经元的特点 (1)人体各种神经元本身的构成很相似 (2)早期的大脑损伤,其功能可能是以其他部位的神经元来代替实现的 (3&#x…...
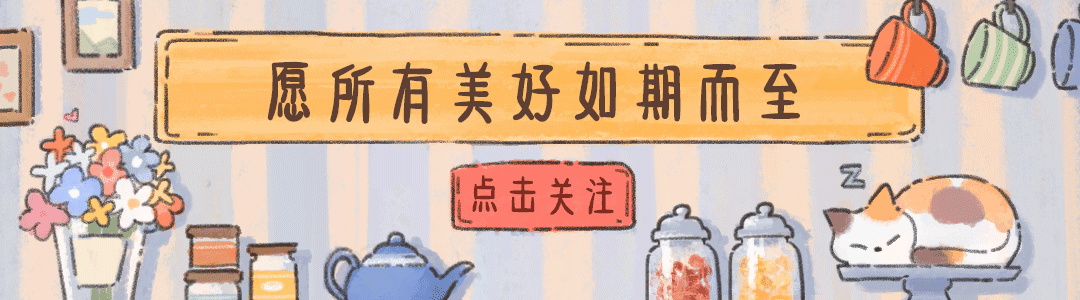
Kafka安装及简单使用介绍
🍓 简介:java系列技术分享(👉持续更新中…🔥) 🍓 初衷:一起学习、一起进步、坚持不懈 🍓 如果文章内容有误与您的想法不一致,欢迎大家在评论区指正🙏 🍓 希望这篇文章对你有所帮助,欢…...
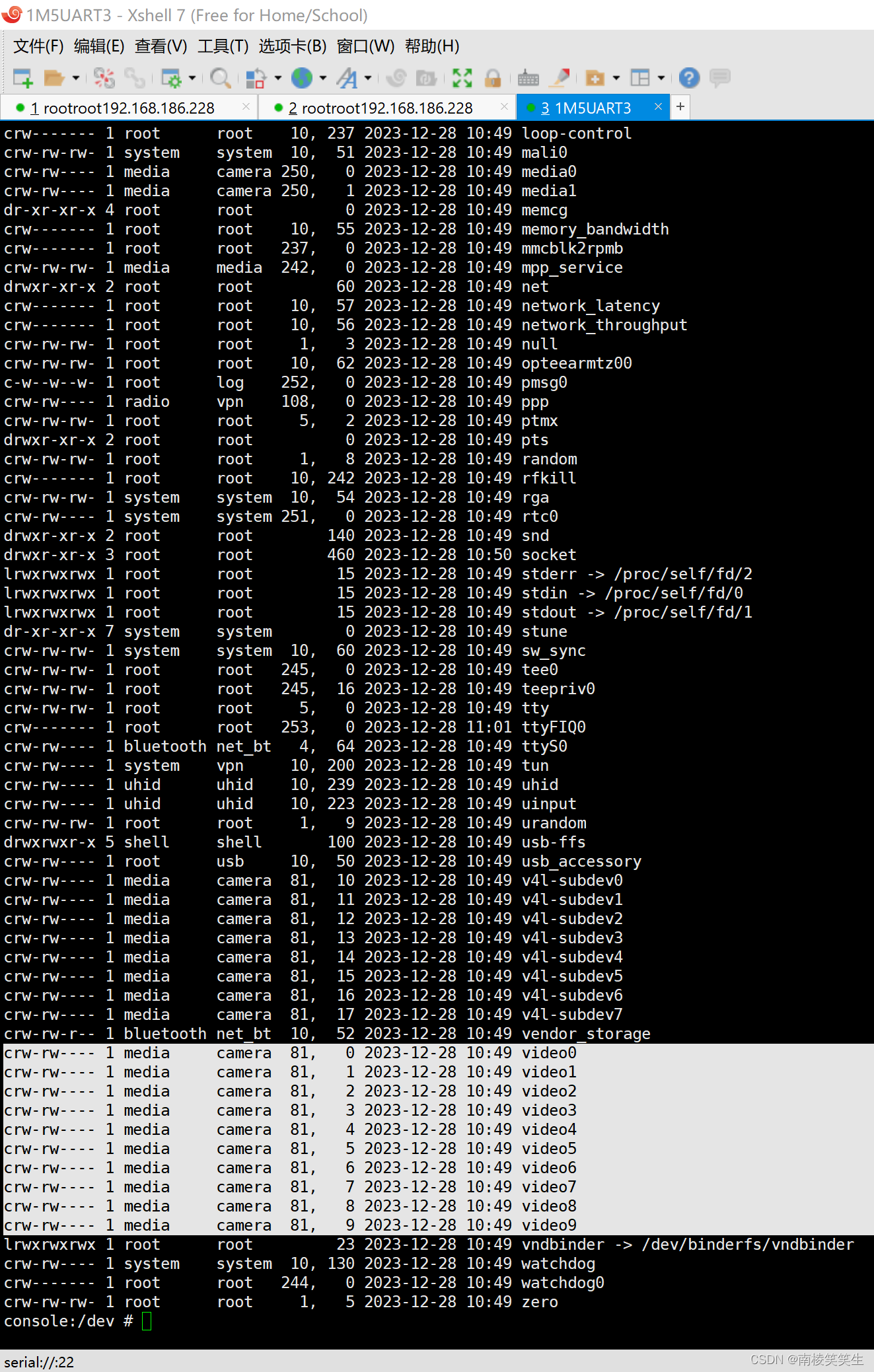
20231229在Firefly的AIO-3399J开发板的Android11使用挖掘机的DTS配置单前后摄像头ov13850
20231229在Firefly的AIO-3399J开发板的Android11使用挖掘机的DTS配置单前后摄像头ov13850 2023/12/29 11:10 开发板:Firefly的AIO-3399J【RK3399】 SDK:rk3399-android-11-r20211216.tar.xz【Android11】 Android11.0.tar.bz2.aa【ToyBrick】 Android11.…...

九台虚拟机网站流量分析项目启动步骤
文章目录 零、操作概述一、服务器分配二、9台虚拟机相互免密登录三、Nginx(反向代理服务器)四、Tomcat(Web服务器)五、测试Nginx反向代理是否成功六、Flume集群配置七、修改LogDemo项目八、项目1703FluxStorm九、Hadoop集群十、整个集群的启动十一、部署项目十二、测试项目…...
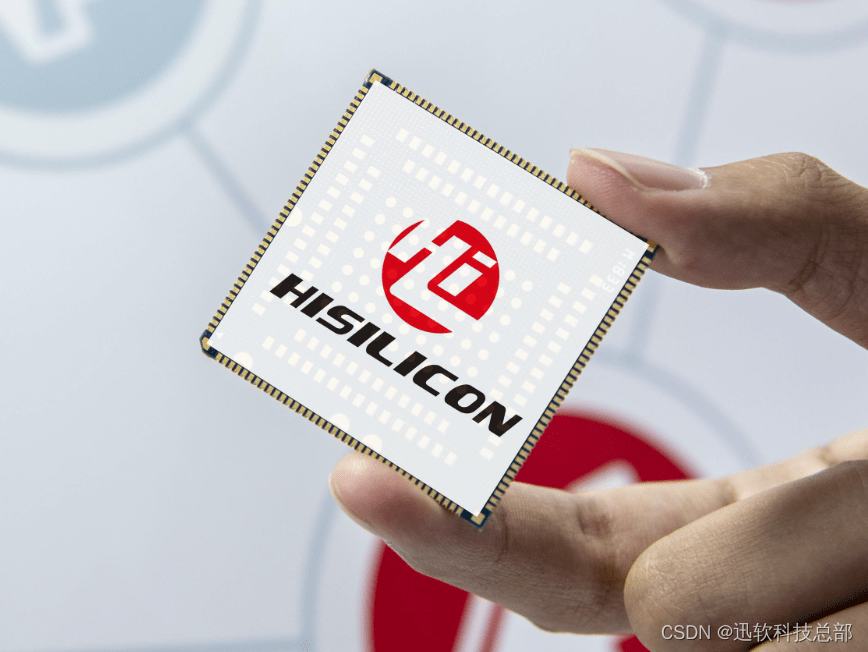
迅软科技助力高科技防泄密:从华为事件中汲取经验教训
近期,涉及华为芯片技术被窃一事引起广泛关注。据报道,华为海思的两个高管张某、刘某离职后成立尊湃通讯,然后以支付高薪、股权支付等方式,诱导多名海思研发人员跳槽其公司,并指使这些人员在离职前通过摘抄、截屏等方式…...
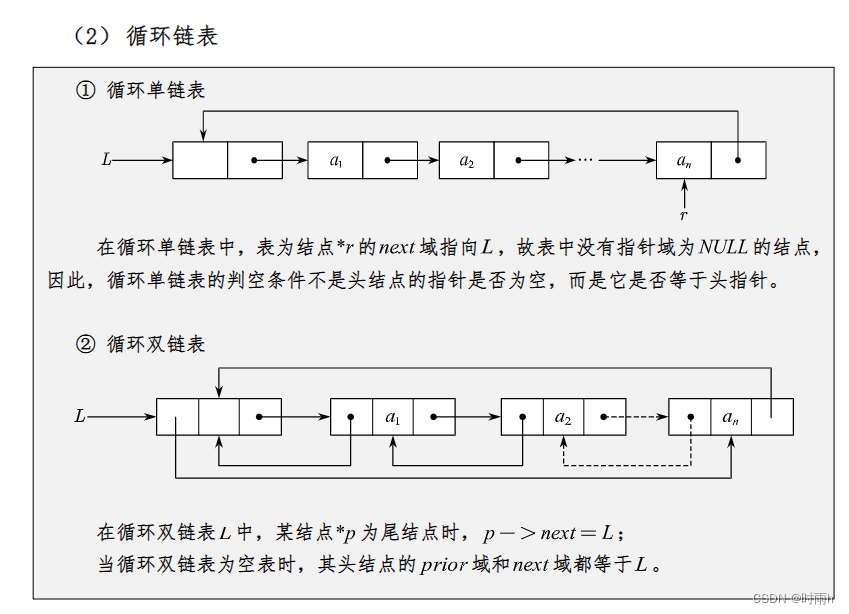
数据结构期末复习(2)链表
链表 链表(Linked List)是一种常见的数据结构,用于存储一系列具有相同类型的元素。链表由节点(Node)组成,每个节点包含两部分:数据域(存储元素值)和指针域(指…...
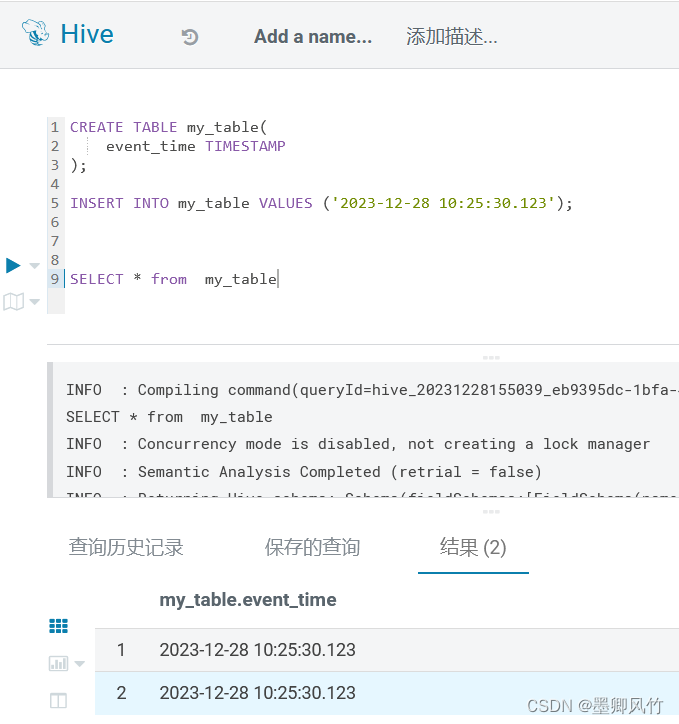
Hive中支持毫秒级别的时间精度
实际上,Hive 在较新的版本中已经支持毫秒级别的时间精度。你可以通过设置 hive.exec.default.serialization.format 和 mapred.output.value.format 属性为 1,启用 Hive 的时间精度为毫秒级。可以使用以下命令进行设置: set hive.exec.defau…...
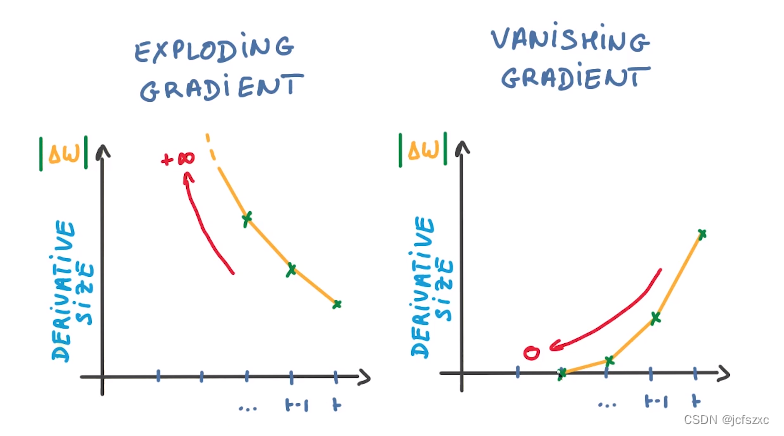
【深度学习:Recurrent Neural Networks】循环神经网络(RNN)的简要概述
【深度学习】循环神经网络(RNN):连接过去与未来的桥梁 循环神经网络简介什么是循环神经网络 (RNN)?传统 RNN 的架构循环神经网络如何工作?常用激活函数RNN的优点和缺点RNN 的优点:RNN 的缺点: 循…...
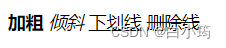
HTML 基础
文章目录 01-标签语法标签结构 03-HTML骨架04-标签的关系05-注释06-标题标签07-段落标签08-换行和水平线09-文本格式化标签10-图像标签图像属性 11-路径相对路径绝对路径 12-超链接标签13-音频14-视频 01-标签语法 HTML 超文本标记语言——HyperText Markup Language。 超文本…...
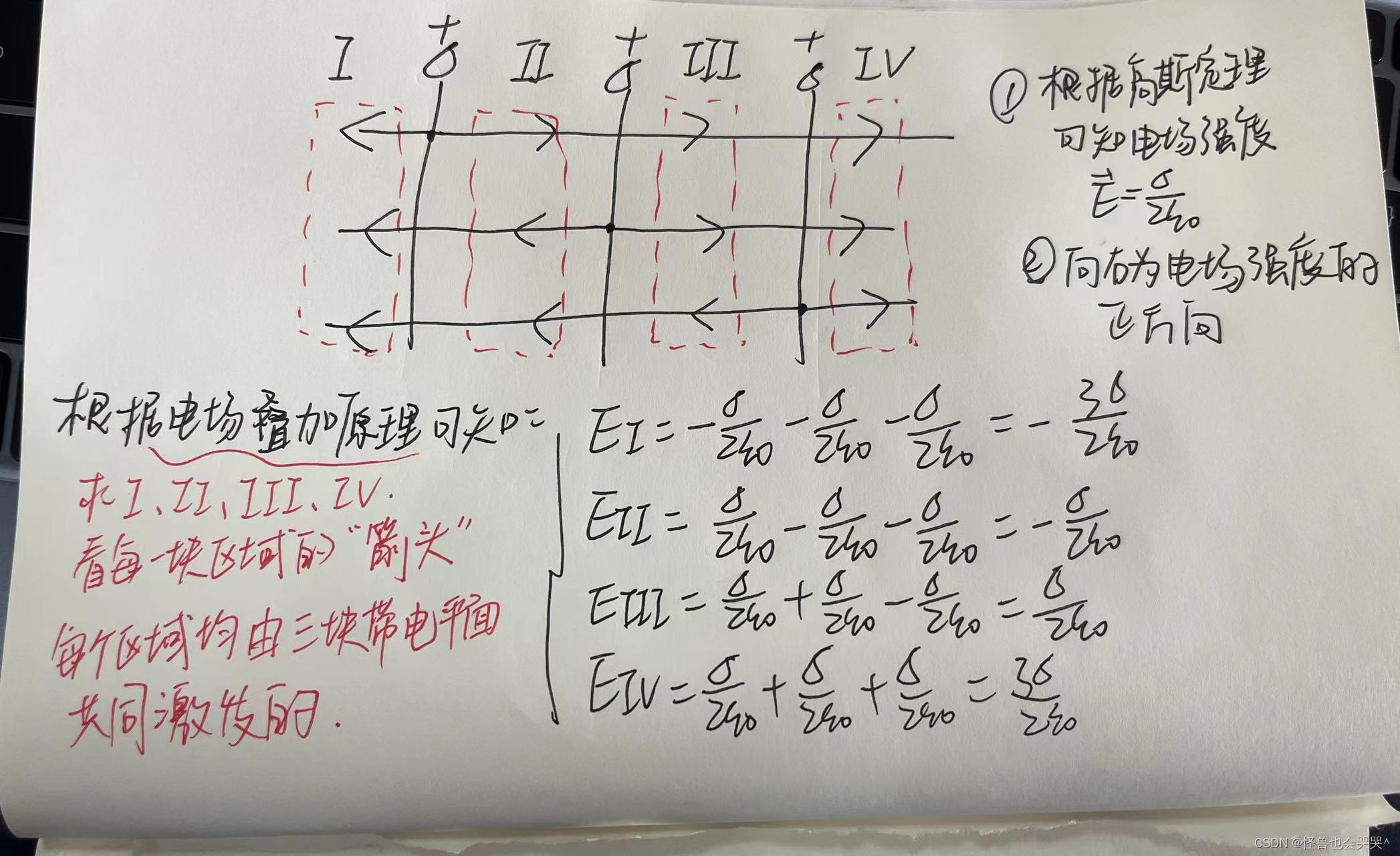
大学物理II-作业1【题解】
1.【单选题】——考查高斯定理 下面关于高斯定理描述正确的是(D )。 A.高斯面上的电场强度是由高斯面内的电荷激发的 B.高斯面上的各点电场强度为零时,高斯面内一定没有电荷 C.通过高斯面的电通量为零时,高斯面上各点电场强度…...
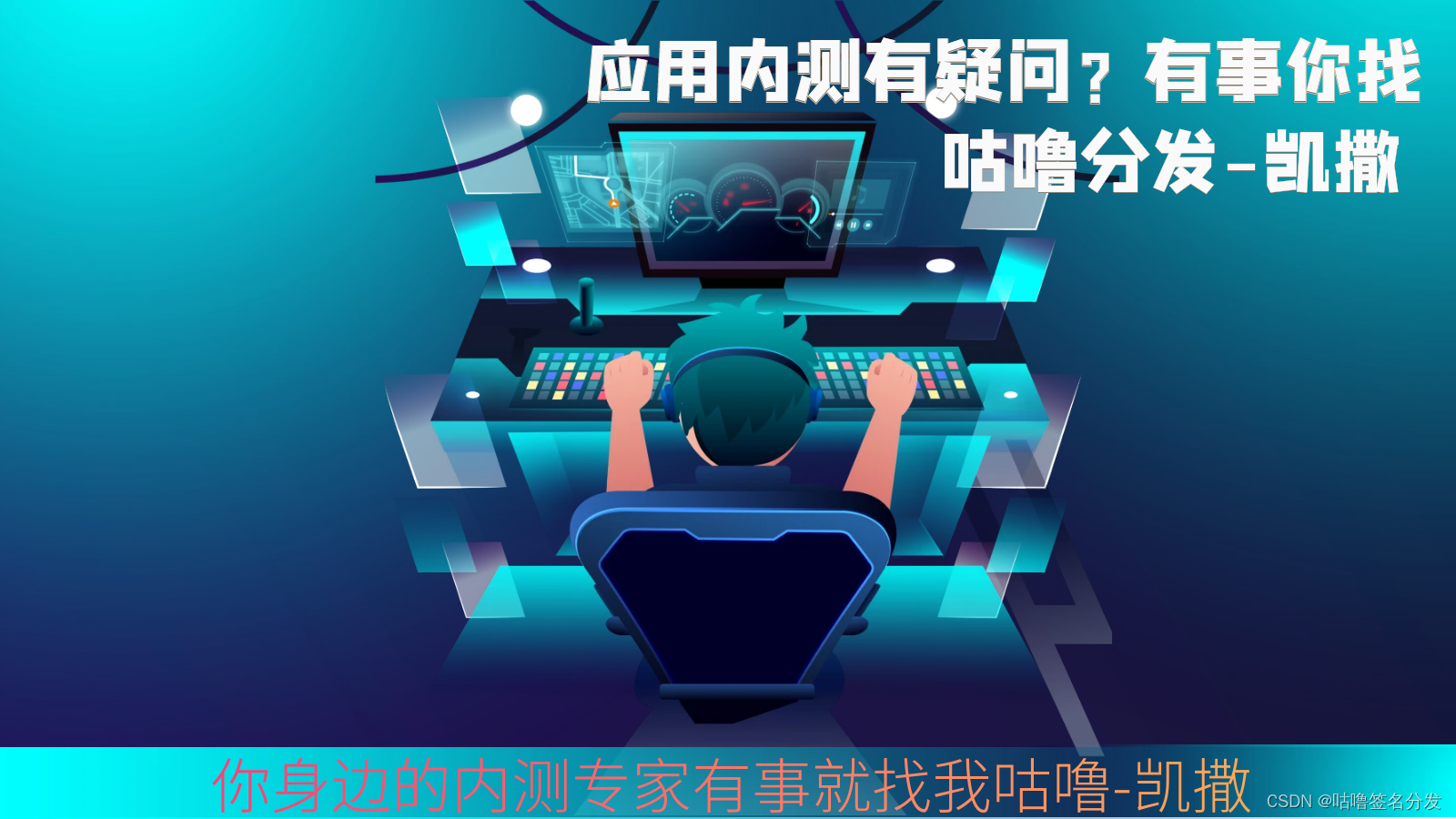
Unity引擎有哪些优点
Unity引擎是一款跨平台的游戏引擎,拥有很多的优点,如跨平台支持、强大的工具和编辑器、灵活的脚本支持、丰富的资源库和强大的社区生态系统等,让他成为众多开发者选择的游戏开发引擎。下面我简单的介绍一下Unity引擎的优点。 跨平台支持 跨…...
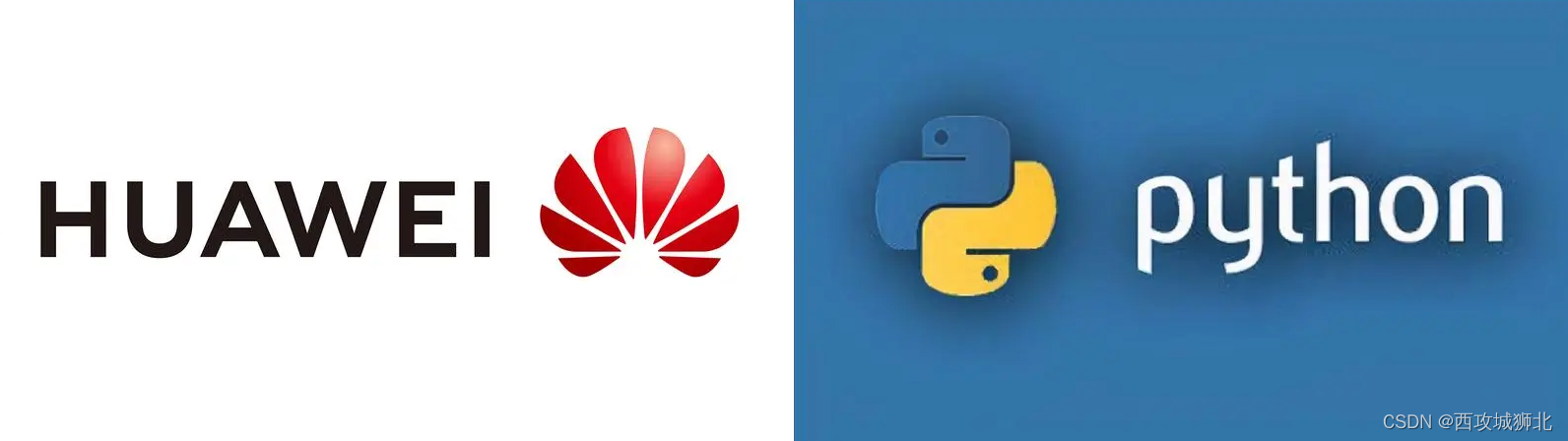
【华为机试】2023年真题B卷(python)-猴子爬山
一、题目 题目描述: 一天一只顽猴想去从山脚爬到山顶,途中经过一个有个N个台阶的阶梯,但是这猴子有一个习惯: 每一次只能跳1步或跳3步,试问猴子通过这个阶梯有多少种不同的跳跃方式? 二、输入输出 输入描述…...
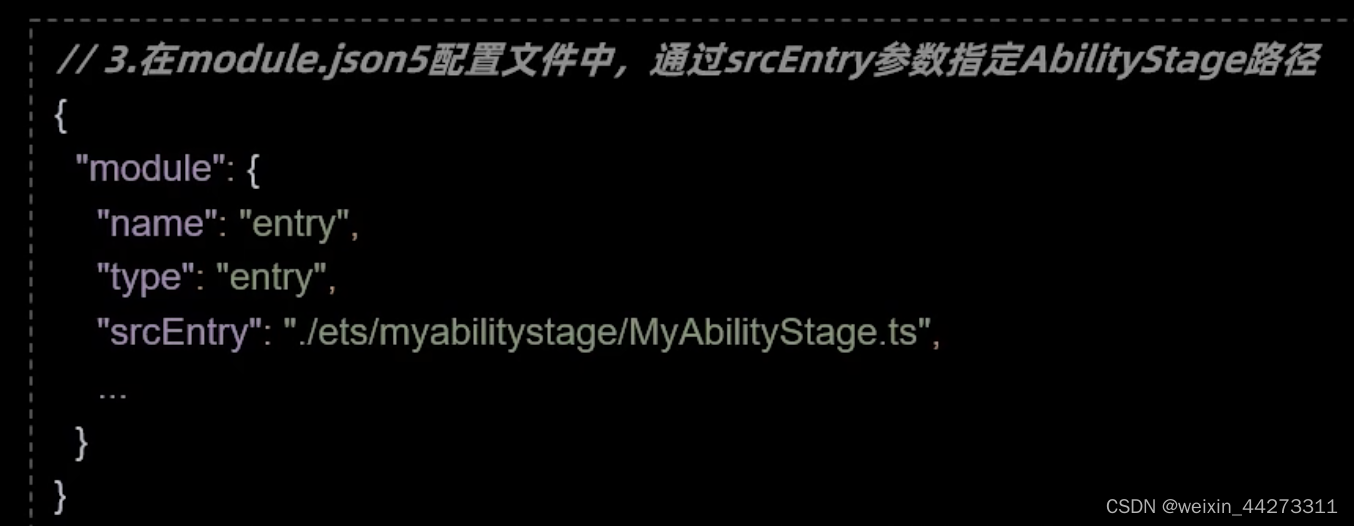
【Harmony OS - Stage应用模型】
基本概念 大类分为: Ability Module: 功能模块 、Library Module: 共享功能模块 编译时概念: Ability Module在编译时打包生成HAP(Harmony Ability Package),一个应用可能会有多个HAP…...
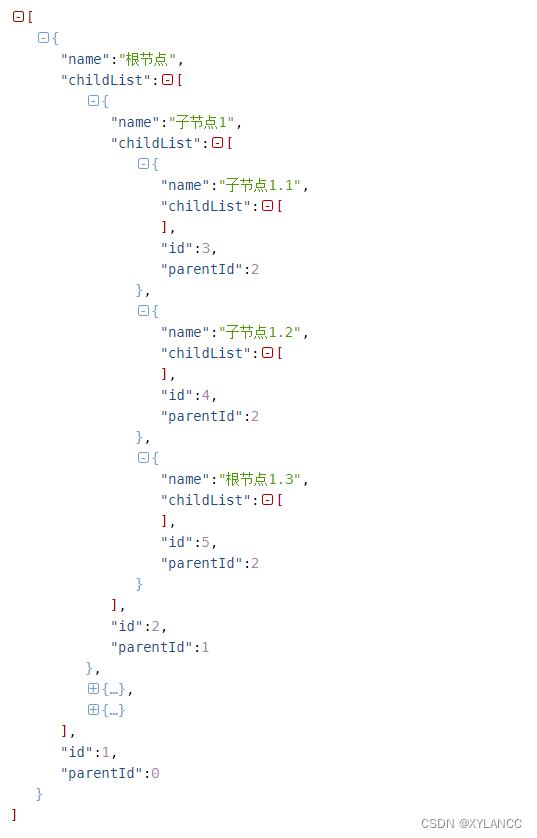
Java 8 中的 Stream 轻松遍历树形结构!
可能平常会遇到一些需求,比如构建菜单,构建树形结构,数据库一般就使用父id来表示,为了降低数据库的查询压力,我们可以使用Java8中的Stream流一次性把数据查出来,然后通过流式处理,我们一起来看看…...
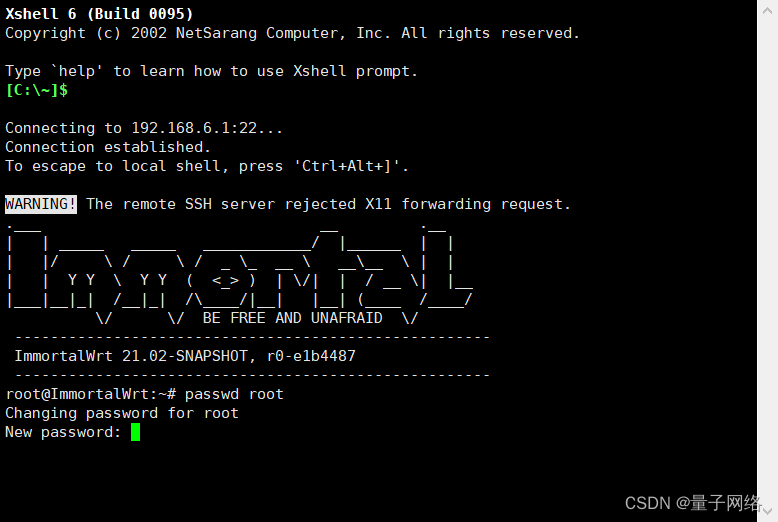
Openwrt修改Dropbear ssh root密码
使用ssh工具连接路由器 输入:passwd root 输入新密码 重复新密码 设置完成 rootImmortalWrt:~# passwd root Changing password for root New password:...
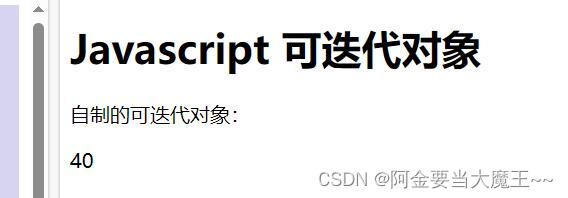
js 对象
js 对象定义 <!DOCTYPE html> <html> <body><h1>JavaScript 对象创建</h1><p id"demo1"></p> <p>new</p> <p id"demo"></p><script> // 创建对象: var persona {fi…...