C# Onnx 使用onnxruntime部署实时视频帧插值
目录
介绍
效果
模型信息
项目
代码
下载
C# Onnx 使用onnxruntime部署实时视频帧插值
介绍
github地址:https://github.com/google-research/frame-interpolation
FILM: Frame Interpolation for Large Motion, In ECCV 2022.
The official Tensorflow 2 implementation of our high quality frame interpolation neural network. We present a unified single-network approach that doesn't use additional pre-trained networks, like optical flow or depth, and yet achieve state-of-the-art results. We use a multi-scale feature extractor that shares the same convolution weights across the scales. Our model is trainable from frame triplets alone.
FILM transforms near-duplicate photos into a slow motion footage that look like it is shot with a video camera.
效果
模型信息
Model Properties
-------------------------
---------------------------------------------------------------
Inputs
-------------------------
name:I0
tensor:Float[1, 3, -1, -1]
name:I1
tensor:Float[1, 3, -1, -1]
---------------------------------------------------------------
Outputs
-------------------------
name:merged
tensor:Float[1, -1, -1, -1]
---------------------------------------------------------------
项目
代码
using Microsoft.ML.OnnxRuntime;
using Microsoft.ML.OnnxRuntime.Tensors;
using OpenCvSharp;
using OpenCvSharp.Dnn;
using System;
using System.Collections.Generic;
using System.Drawing;
using System.Drawing.Imaging;
using System.Linq;
using System.Numerics;
using System.Windows.Forms;
namespace Onnx_Yolov8_Demo
{
public partial class Form1 : Form
{
public Form1()
{
InitializeComponent();
}
string fileFilter = "*.*|*.bmp;*.jpg;*.jpeg;*.tiff;*.tiff;*.png";
string image_path = "";
string startupPath;
DateTime dt1 = DateTime.Now;
DateTime dt2 = DateTime.Now;
string model_path;
Mat image;
Mat result_image;
SessionOptions options;
InferenceSession onnx_session;
Tensor<float> input_tensor;
Tensor<float> input_tensor2;
List<NamedOnnxValue> input_container;
IDisposableReadOnlyCollection<DisposableNamedOnnxValue> result_infer;
DisposableNamedOnnxValue[] results_onnxvalue;
Tensor<float> result_tensors;
float[] result_array;
float[] input1_image;
float[] input2_image;
int inpWidth;
int inpHeight;
private void button1_Click(object sender, EventArgs e)
{
OpenFileDialog ofd = new OpenFileDialog();
ofd.Filter = fileFilter;
if (ofd.ShowDialog() != DialogResult.OK) return;
pictureBox1.Image = null;
image_path = ofd.FileName;
pictureBox1.Image = new Bitmap(image_path);
textBox1.Text = "";
image = new Mat(image_path);
pictureBox2.Image = null;
}
void Preprocess(Mat img, ref float[] input_img)
{
Mat rgbimg = new Mat();
Cv2.CvtColor(img, rgbimg, ColorConversionCodes.BGR2RGB);
int h = rgbimg.Rows;
int w = rgbimg.Cols;
int align = 32;
if (h % align != 0 || w % align != 0)
{
int ph = ((h - 1) / align + 1) * align;
int pw = ((w - 1) / align + 1) * align;
Cv2.CopyMakeBorder(rgbimg, rgbimg, 0, ph - h, 0, pw - w, BorderTypes.Constant, 0);
}
inpHeight = rgbimg.Rows;
inpWidth = rgbimg.Cols;
rgbimg.ConvertTo(rgbimg, MatType.CV_32FC3, 1 / 255.0);
int image_area = rgbimg.Rows * rgbimg.Cols;
//input_img = new float[3 * image_area];
input_img = Common.ExtractMat(rgbimg);
}
Mat Interpolate(Mat srcimg1, Mat srcimg2)
{
int srch = srcimg1.Rows;
int srcw = srcimg1.Cols;
Preprocess(srcimg1, ref input1_image);
Preprocess(srcimg2, ref input2_image);
// 输入Tensor
input_tensor = new DenseTensor<float>(input1_image, new[] { 1, 3, inpHeight, inpWidth });
input_tensor2 = new DenseTensor<float>(input2_image, new[] { 1, 3, inpHeight, inpWidth });
//将tensor 放入一个输入参数的容器,并指定名称
input_container.Add(NamedOnnxValue.CreateFromTensor("I0", input_tensor));
input_container.Add(NamedOnnxValue.CreateFromTensor("I1", input_tensor2));
//运行 Inference 并获取结果
result_infer = onnx_session.Run(input_container);
// 将输出结果转为DisposableNamedOnnxValue数组
results_onnxvalue = result_infer.ToArray();
// 读取第一个节点输出并转为Tensor数据
result_tensors = results_onnxvalue[0].AsTensor<float>();
int out_h = results_onnxvalue[0].AsTensor<float>().Dimensions[2];
int out_w = results_onnxvalue[0].AsTensor<float>().Dimensions[3];
result_array = result_tensors.ToArray();
for (int i = 0; i < result_array.Length; i++)
{
result_array[i] = result_array[i] * 255;
if (result_array[i] < 0)
{
result_array[i] = 0;
}
else if (result_array[i] > 255)
{
result_array[i] = 255;
}
result_array[i] = result_array[i] + 0.5f;
}
float[] temp_r = new float[out_h * out_w];
float[] temp_g = new float[out_h * out_w];
float[] temp_b = new float[out_h * out_w];
Array.Copy(result_array, temp_r, out_h * out_w);
Array.Copy(result_array, out_h * out_w, temp_g, 0, out_h * out_w);
Array.Copy(result_array, out_h * out_w * 2, temp_b, 0, out_h * out_w);
Mat rmat = new Mat(out_h, out_w, MatType.CV_32F, temp_r);
Mat gmat = new Mat(out_h, out_w, MatType.CV_32F, temp_g);
Mat bmat = new Mat(out_h, out_w, MatType.CV_32F, temp_b);
result_image = new Mat();
Cv2.Merge(new Mat[] { bmat, gmat, rmat }, result_image);
result_image.ConvertTo(result_image, MatType.CV_8UC3);
Mat mid_img = new Mat(result_image, new Rect(0, 0, srcw, srch));
return mid_img;
}
private void button2_Click(object sender, EventArgs e)
{
button2.Enabled = false;
pictureBox2.Image = null;
textBox1.Text = "正在运行,请稍后……";
Application.DoEvents();
dt1 = DateTime.Now;
List<String> inputs_imgpath = new List<String>() { "test_img/frame07.png", "test_img/frame08.png", "test_img/frame09.png", "test_img/frame10.png", "test_img/frame11.png", "test_img/frame12.png", "test_img/frame13.png", "test_img/frame14.png" };
int imgnum = inputs_imgpath.Count();
for (int i = 0; i < imgnum - 1; i++)
{
Mat srcimg1 = Cv2.ImRead(inputs_imgpath[i]);
Mat srcimg2 = Cv2.ImRead(inputs_imgpath[i + 1]);
Mat mid_img = Interpolate(srcimg1, srcimg2);
string save_imgpath = "imgs_results/mid" + i + ".jpg";
Cv2.ImWrite(save_imgpath, mid_img);
}
dt2 = DateTime.Now;
textBox1.Text = "推理耗时:" + (dt2 - dt1).TotalMilliseconds + "ms";
button2.Enabled = true;
}
private void Form1_Load(object sender, EventArgs e)
{
model_path = "model/RIFE_HDv3.onnx";
// 创建输出会话,用于输出模型读取信息
options = new SessionOptions();
options.LogSeverityLevel = OrtLoggingLevel.ORT_LOGGING_LEVEL_INFO;
options.AppendExecutionProvider_CPU(0);// 设置为CPU上运行
// 创建推理模型类,读取本地模型文件
onnx_session = new InferenceSession(model_path, options);//model_path 为onnx模型文件的路径
// 创建输入容器
input_container = new List<NamedOnnxValue>();
pictureBox1.Image = new Bitmap("test_img/frame11.png");
pictureBox3.Image = new Bitmap("test_img/frame12.png");
}
private void pictureBox1_DoubleClick(object sender, EventArgs e)
{
Common.ShowNormalImg(pictureBox1.Image);
}
private void pictureBox2_DoubleClick(object sender, EventArgs e)
{
Common.ShowNormalImg(pictureBox2.Image);
}
SaveFileDialog sdf = new SaveFileDialog();
private void button3_Click(object sender, EventArgs e)
{
if (pictureBox2.Image == null)
{
return;
}
Bitmap output = new Bitmap(pictureBox2.Image);
sdf.Title = "保存";
sdf.Filter = "Images (*.jpg)|*.jpg|Images (*.png)|*.png|Images (*.bmp)|*.bmp|Images (*.emf)|*.emf|Images (*.exif)|*.exif|Images (*.gif)|*.gif|Images (*.ico)|*.ico|Images (*.tiff)|*.tiff|Images (*.wmf)|*.wmf";
if (sdf.ShowDialog() == DialogResult.OK)
{
switch (sdf.FilterIndex)
{
case 1:
{
output.Save(sdf.FileName, ImageFormat.Jpeg);
break;
}
case 2:
{
output.Save(sdf.FileName, ImageFormat.Png);
break;
}
case 3:
{
output.Save(sdf.FileName, ImageFormat.Bmp);
break;
}
case 4:
{
output.Save(sdf.FileName, ImageFormat.Emf);
break;
}
case 5:
{
output.Save(sdf.FileName, ImageFormat.Exif);
break;
}
case 6:
{
output.Save(sdf.FileName, ImageFormat.Gif);
break;
}
case 7:
{
output.Save(sdf.FileName, ImageFormat.Icon);
break;
}
case 8:
{
output.Save(sdf.FileName, ImageFormat.Tiff);
break;
}
case 9:
{
output.Save(sdf.FileName, ImageFormat.Wmf);
break;
}
}
MessageBox.Show("保存成功,位置:" + sdf.FileName);
}
}
private void button4_Click(object sender, EventArgs e)
{
button2.Enabled = false;
pictureBox2.Image = null;
textBox1.Text = "正在运行,请稍后……";
Application.DoEvents();
dt1 = DateTime.Now;
Mat srcimg1 = Cv2.ImRead("test_img/frame11.png");
Mat srcimg2 = Cv2.ImRead("test_img/frame12.png");
Mat mid_img = Interpolate(srcimg1, srcimg2);
dt2 = DateTime.Now;
pictureBox2.Image = new Bitmap(mid_img.ToMemoryStream());
textBox1.Text = "推理耗时:" + (dt2 - dt1).TotalMilliseconds + "ms";
button2.Enabled = true;
}
}
}
using Microsoft.ML.OnnxRuntime;
using Microsoft.ML.OnnxRuntime.Tensors;
using OpenCvSharp;
using OpenCvSharp.Dnn;
using System;
using System.Collections.Generic;
using System.Drawing;
using System.Drawing.Imaging;
using System.Linq;
using System.Numerics;
using System.Windows.Forms;namespace Onnx_Yolov8_Demo
{public partial class Form1 : Form{public Form1(){InitializeComponent();}string fileFilter = "*.*|*.bmp;*.jpg;*.jpeg;*.tiff;*.tiff;*.png";string image_path = "";string startupPath;DateTime dt1 = DateTime.Now;DateTime dt2 = DateTime.Now;string model_path;Mat image;Mat result_image;SessionOptions options;InferenceSession onnx_session;Tensor<float> input_tensor;Tensor<float> input_tensor2;List<NamedOnnxValue> input_container;IDisposableReadOnlyCollection<DisposableNamedOnnxValue> result_infer;DisposableNamedOnnxValue[] results_onnxvalue;Tensor<float> result_tensors;float[] result_array;float[] input1_image;float[] input2_image;int inpWidth;int inpHeight;private void button1_Click(object sender, EventArgs e){OpenFileDialog ofd = new OpenFileDialog();ofd.Filter = fileFilter;if (ofd.ShowDialog() != DialogResult.OK) return;pictureBox1.Image = null;image_path = ofd.FileName;pictureBox1.Image = new Bitmap(image_path);textBox1.Text = "";image = new Mat(image_path);pictureBox2.Image = null;}void Preprocess(Mat img, ref float[] input_img){Mat rgbimg = new Mat();Cv2.CvtColor(img, rgbimg, ColorConversionCodes.BGR2RGB);int h = rgbimg.Rows;int w = rgbimg.Cols;int align = 32;if (h % align != 0 || w % align != 0){int ph = ((h - 1) / align + 1) * align;int pw = ((w - 1) / align + 1) * align;Cv2.CopyMakeBorder(rgbimg, rgbimg, 0, ph - h, 0, pw - w, BorderTypes.Constant, 0);}inpHeight = rgbimg.Rows;inpWidth = rgbimg.Cols;rgbimg.ConvertTo(rgbimg, MatType.CV_32FC3, 1 / 255.0);int image_area = rgbimg.Rows * rgbimg.Cols;//input_img = new float[3 * image_area];input_img = Common.ExtractMat(rgbimg);}Mat Interpolate(Mat srcimg1, Mat srcimg2){int srch = srcimg1.Rows;int srcw = srcimg1.Cols;Preprocess(srcimg1, ref input1_image);Preprocess(srcimg2, ref input2_image);// 输入Tensorinput_tensor = new DenseTensor<float>(input1_image, new[] { 1, 3, inpHeight, inpWidth });input_tensor2 = new DenseTensor<float>(input2_image, new[] { 1, 3, inpHeight, inpWidth });//将tensor 放入一个输入参数的容器,并指定名称input_container.Add(NamedOnnxValue.CreateFromTensor("I0", input_tensor));input_container.Add(NamedOnnxValue.CreateFromTensor("I1", input_tensor2));//运行 Inference 并获取结果result_infer = onnx_session.Run(input_container);// 将输出结果转为DisposableNamedOnnxValue数组results_onnxvalue = result_infer.ToArray();// 读取第一个节点输出并转为Tensor数据result_tensors = results_onnxvalue[0].AsTensor<float>();int out_h = results_onnxvalue[0].AsTensor<float>().Dimensions[2];int out_w = results_onnxvalue[0].AsTensor<float>().Dimensions[3];result_array = result_tensors.ToArray();for (int i = 0; i < result_array.Length; i++){result_array[i] = result_array[i] * 255;if (result_array[i] < 0){result_array[i] = 0;}else if (result_array[i] > 255){result_array[i] = 255;}result_array[i] = result_array[i] + 0.5f;}float[] temp_r = new float[out_h * out_w];float[] temp_g = new float[out_h * out_w];float[] temp_b = new float[out_h * out_w];Array.Copy(result_array, temp_r, out_h * out_w);Array.Copy(result_array, out_h * out_w, temp_g, 0, out_h * out_w);Array.Copy(result_array, out_h * out_w * 2, temp_b, 0, out_h * out_w);Mat rmat = new Mat(out_h, out_w, MatType.CV_32F, temp_r);Mat gmat = new Mat(out_h, out_w, MatType.CV_32F, temp_g);Mat bmat = new Mat(out_h, out_w, MatType.CV_32F, temp_b);result_image = new Mat();Cv2.Merge(new Mat[] { bmat, gmat, rmat }, result_image);result_image.ConvertTo(result_image, MatType.CV_8UC3);Mat mid_img = new Mat(result_image, new Rect(0, 0, srcw, srch));return mid_img;}private void button2_Click(object sender, EventArgs e){button2.Enabled = false;pictureBox2.Image = null;textBox1.Text = "正在运行,请稍后……";Application.DoEvents();dt1 = DateTime.Now;List<String> inputs_imgpath = new List<String>() { "test_img/frame07.png", "test_img/frame08.png", "test_img/frame09.png", "test_img/frame10.png", "test_img/frame11.png", "test_img/frame12.png", "test_img/frame13.png", "test_img/frame14.png" };int imgnum = inputs_imgpath.Count();for (int i = 0; i < imgnum - 1; i++){Mat srcimg1 = Cv2.ImRead(inputs_imgpath[i]);Mat srcimg2 = Cv2.ImRead(inputs_imgpath[i + 1]);Mat mid_img = Interpolate(srcimg1, srcimg2);string save_imgpath = "imgs_results/mid" + i + ".jpg";Cv2.ImWrite(save_imgpath, mid_img);}dt2 = DateTime.Now;textBox1.Text = "推理耗时:" + (dt2 - dt1).TotalMilliseconds + "ms";button2.Enabled = true;}private void Form1_Load(object sender, EventArgs e){model_path = "model/RIFE_HDv3.onnx";// 创建输出会话,用于输出模型读取信息options = new SessionOptions();options.LogSeverityLevel = OrtLoggingLevel.ORT_LOGGING_LEVEL_INFO;options.AppendExecutionProvider_CPU(0);// 设置为CPU上运行// 创建推理模型类,读取本地模型文件onnx_session = new InferenceSession(model_path, options);//model_path 为onnx模型文件的路径// 创建输入容器input_container = new List<NamedOnnxValue>();pictureBox1.Image = new Bitmap("test_img/frame11.png");pictureBox3.Image = new Bitmap("test_img/frame12.png");}private void pictureBox1_DoubleClick(object sender, EventArgs e){Common.ShowNormalImg(pictureBox1.Image);}private void pictureBox2_DoubleClick(object sender, EventArgs e){Common.ShowNormalImg(pictureBox2.Image);}SaveFileDialog sdf = new SaveFileDialog();private void button3_Click(object sender, EventArgs e){if (pictureBox2.Image == null){return;}Bitmap output = new Bitmap(pictureBox2.Image);sdf.Title = "保存";sdf.Filter = "Images (*.jpg)|*.jpg|Images (*.png)|*.png|Images (*.bmp)|*.bmp|Images (*.emf)|*.emf|Images (*.exif)|*.exif|Images (*.gif)|*.gif|Images (*.ico)|*.ico|Images (*.tiff)|*.tiff|Images (*.wmf)|*.wmf";if (sdf.ShowDialog() == DialogResult.OK){switch (sdf.FilterIndex){case 1:{output.Save(sdf.FileName, ImageFormat.Jpeg);break;}case 2:{output.Save(sdf.FileName, ImageFormat.Png);break;}case 3:{output.Save(sdf.FileName, ImageFormat.Bmp);break;}case 4:{output.Save(sdf.FileName, ImageFormat.Emf);break;}case 5:{output.Save(sdf.FileName, ImageFormat.Exif);break;}case 6:{output.Save(sdf.FileName, ImageFormat.Gif);break;}case 7:{output.Save(sdf.FileName, ImageFormat.Icon);break;}case 8:{output.Save(sdf.FileName, ImageFormat.Tiff);break;}case 9:{output.Save(sdf.FileName, ImageFormat.Wmf);break;}}MessageBox.Show("保存成功,位置:" + sdf.FileName);}}private void button4_Click(object sender, EventArgs e){button2.Enabled = false;pictureBox2.Image = null;textBox1.Text = "正在运行,请稍后……";Application.DoEvents();dt1 = DateTime.Now;Mat srcimg1 = Cv2.ImRead("test_img/frame11.png");Mat srcimg2 = Cv2.ImRead("test_img/frame12.png");Mat mid_img = Interpolate(srcimg1, srcimg2);dt2 = DateTime.Now;pictureBox2.Image = new Bitmap(mid_img.ToMemoryStream());textBox1.Text = "推理耗时:" + (dt2 - dt1).TotalMilliseconds + "ms";button2.Enabled = true;}}
}
下载
源码下载
相关文章:
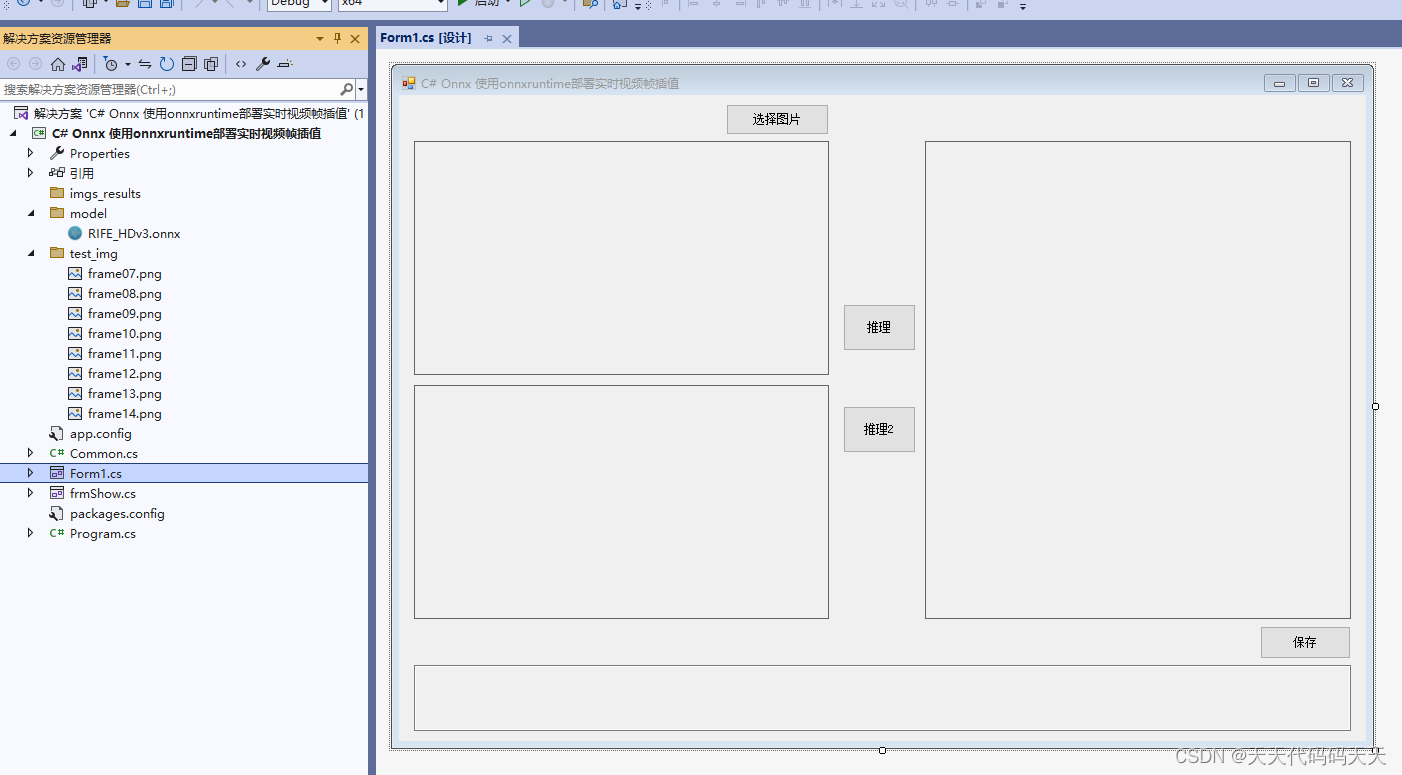
C# Onnx 使用onnxruntime部署实时视频帧插值
目录 介绍 效果 模型信息 项目 代码 下载 C# Onnx 使用onnxruntime部署实时视频帧插值 介绍 github地址:https://github.com/google-research/frame-interpolation FILM: Frame Interpolation for Large Motion, In ECCV 2022. The official Tensorflow 2…...
编程笔记 Golang基础 016 数据类型:数字类型
编程笔记 Golang基础 016 数据类型:数字类型 1. 整数类型(Integer Types)a) 固定长度整数:b) 变长整数: 2. 浮点数类型(Floating-Point Types)3. 复数类型(Complex Number Types&…...
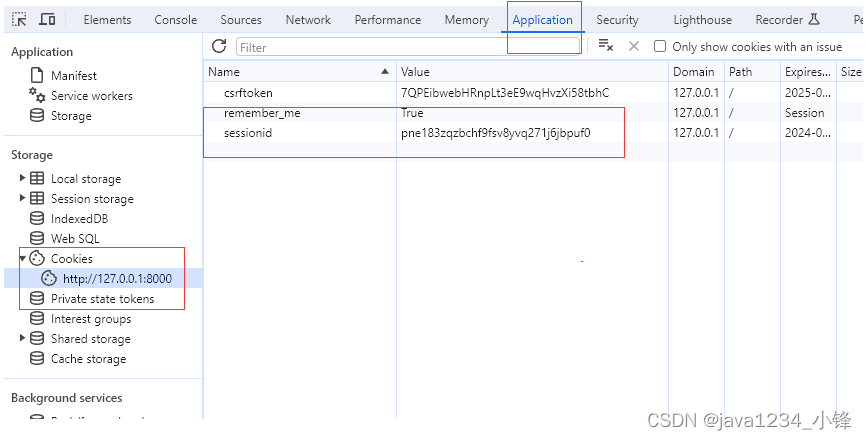
一周学会Django5 Python Web开发-会话管理(CookiesSession)
锋哥原创的Python Web开发 Django5视频教程: 2024版 Django5 Python web开发 视频教程(无废话版) 玩命更新中~_哔哩哔哩_bilibili2024版 Django5 Python web开发 视频教程(无废话版) 玩命更新中~共计26条视频,包括:2024版 Django5 Python we…...
QT之QString.arg输出固定位数
问题描述 我需要用QString输出一个固定位数的数字字符串。起初我的代码是这样: int img_num 1 auto new_name QString("%1.png").arg((int)img_num, 3, 10, 0); //最后一个参数用u0也是一样的 qDebug() << "new_name:" << new…...
Linux下各种压缩包的压缩与解压
tar 归档,不压缩,常见后缀 .tar # 将文件夹归档成为一个包 tar cf rootfs.tar rootfs # 将归档包还原为文件夹 tar xf rootfs.tar # 将归档包还原到路径 a/b/c tar xf rootfs.tar -C a/b/cgzip压缩, 常见后缀 .tar.gz .tgz # 压缩 tar czf …...
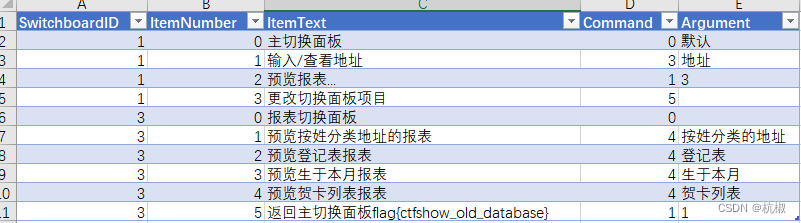
【ctfshow—web】——信息搜集篇1(web1~20详解)
ctfshow—web题解 web1web2web3web4web5web6web7web8web9web10web11web12web13web14web15web16web17web18web19web20 web1 题目提示 开发注释未及时删除 那就找开发注释咯,可以用F12来查看,也可以CtrlU直接查看源代码呢 就拿到flag了 web2 题目提示 j…...
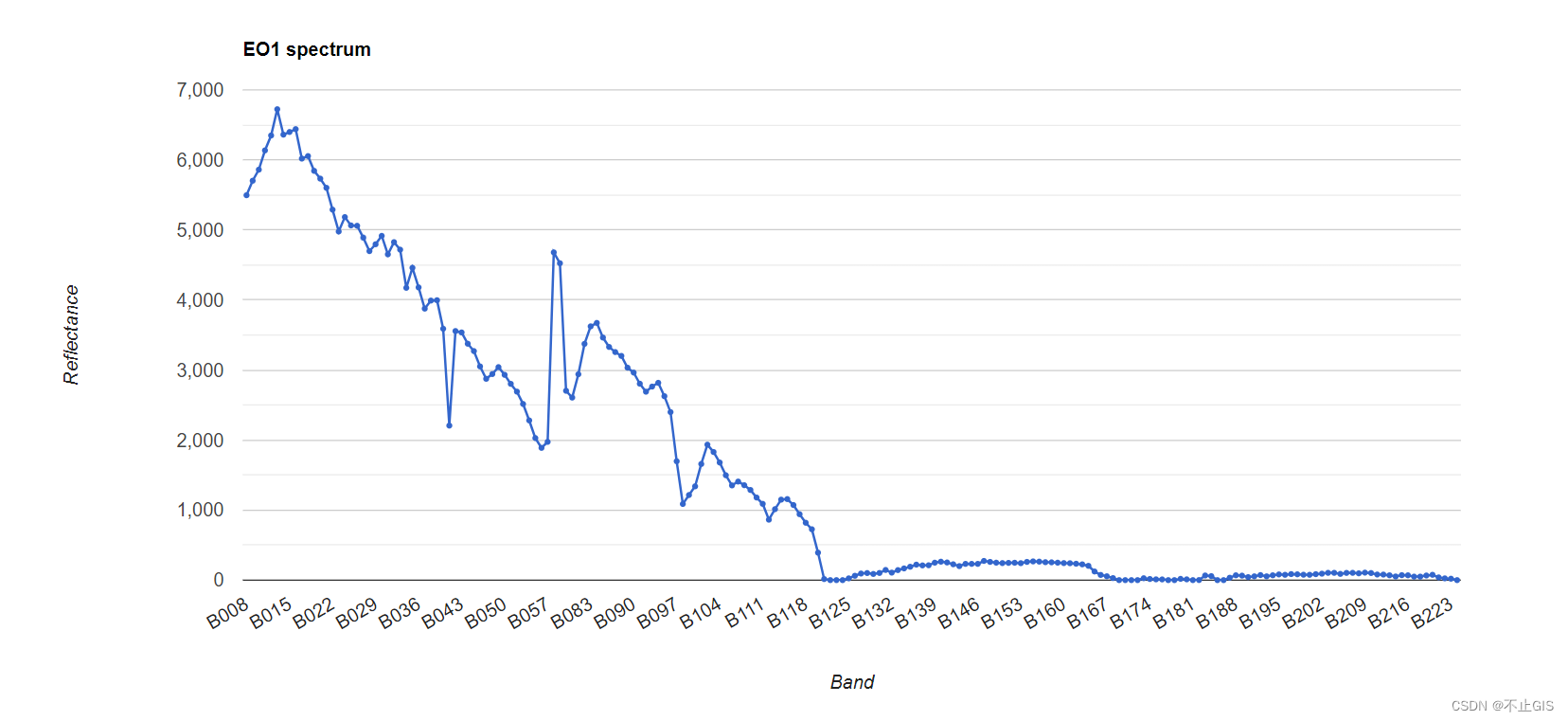
GEE入门篇|遥感专业术语(实践操作4):光谱分辨率(Spectral Resolution)
目录 光谱分辨率(Spectral Resolution) 1.MODIS 2.EO-1 光谱分辨率(Spectral Resolution) 光谱分辨率是指传感器进行测量的光谱带的数量和宽度。 您可以将光谱带的宽度视为每个波段的波长间隔,在多个波段测量辐射亮…...
c++中模板的注意事项
1. 模板定义时,<>中的虚拟类型参数不能为空。(因为我们使用模板就是希望使用模拟类型代替其它的类型,如果我们不定义就没有意义了) 2. 无论是定义函数模板还是类模板,其实template定义与后面使用虚拟类型的类或者函数,是…...
【代码随想录python笔记整理】第十三课 · 链表的基础操作 1
前言:本笔记仅仅只是对内容的整理和自行消化,并不是完整内容,如有侵权,联系立删。 一、链表 在之前的学习中,我们接触到了字符串和数组(列表)这两种结构,它们具有着以下的共同点:1、元素按照一定的顺序来排列。2、可以通过索引来访问数组中的元素和字符串中的字符。由此,…...
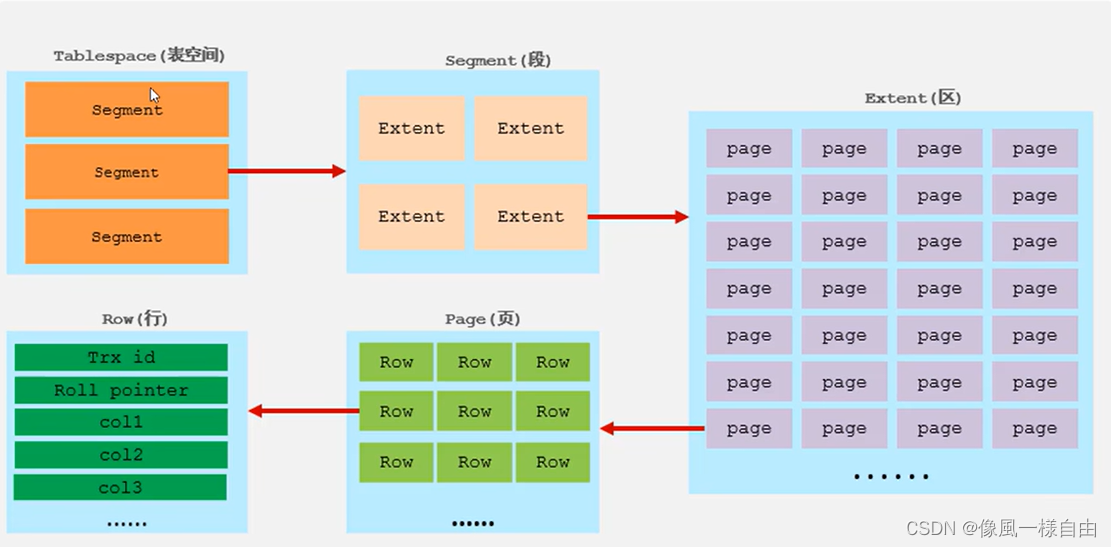
JAVA工程师面试专题-《Mysql》篇
目录 一、基础 1、mysql可以使用多少列创建索引? 2、mysql常用的存储引擎有哪些 3、MySQL 存储引擎,两者区别 4、mysql默认的隔离级别 5、数据库三范式 6、drop、delete 与 truncate 区别? 7、IN与EXISTS的区别 二、索引 1、索引及索…...
@ 代码随想录算法训练营第4周(C语言)|Day22(二叉树)
代码随想录算法训练营第4周(C语言)|Day22(二叉树) Day22、二叉树(包含题目 ● 235. 二叉搜索树的最近公共祖先 ● 701.二叉搜索树中的插入操作 ● 450.删除二叉搜索树中的节点 ) 235. 二叉搜索树的最近公…...
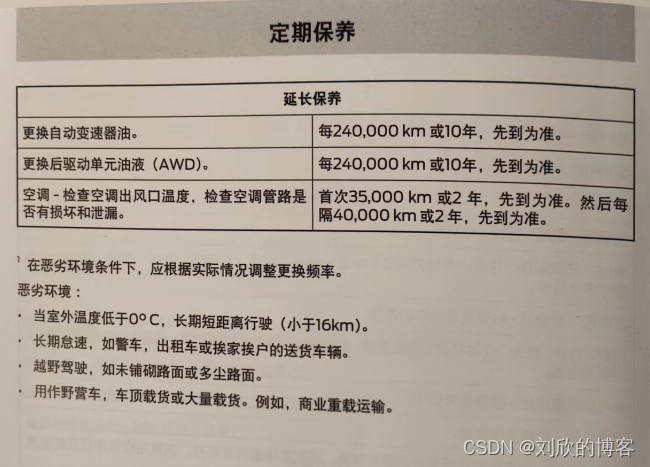
福特锐界2021plus 汽车保养手册
福特锐界2021plus汽车保养手册两页,零部件保养要求,电子版放这里方便查询:...
c++进阶路线
学完C后的进阶路线-初学者勿入【程序员Rock】_哔哩哔哩_bilibili 1.系统训练代码阅读能力 代码阅读工具: 1).Source Insight(阅读大型源码) 2).understand(整体代码模块关系构建) 3).SOURCETRAIL 代码阅读能力--千行级 嵌入…...
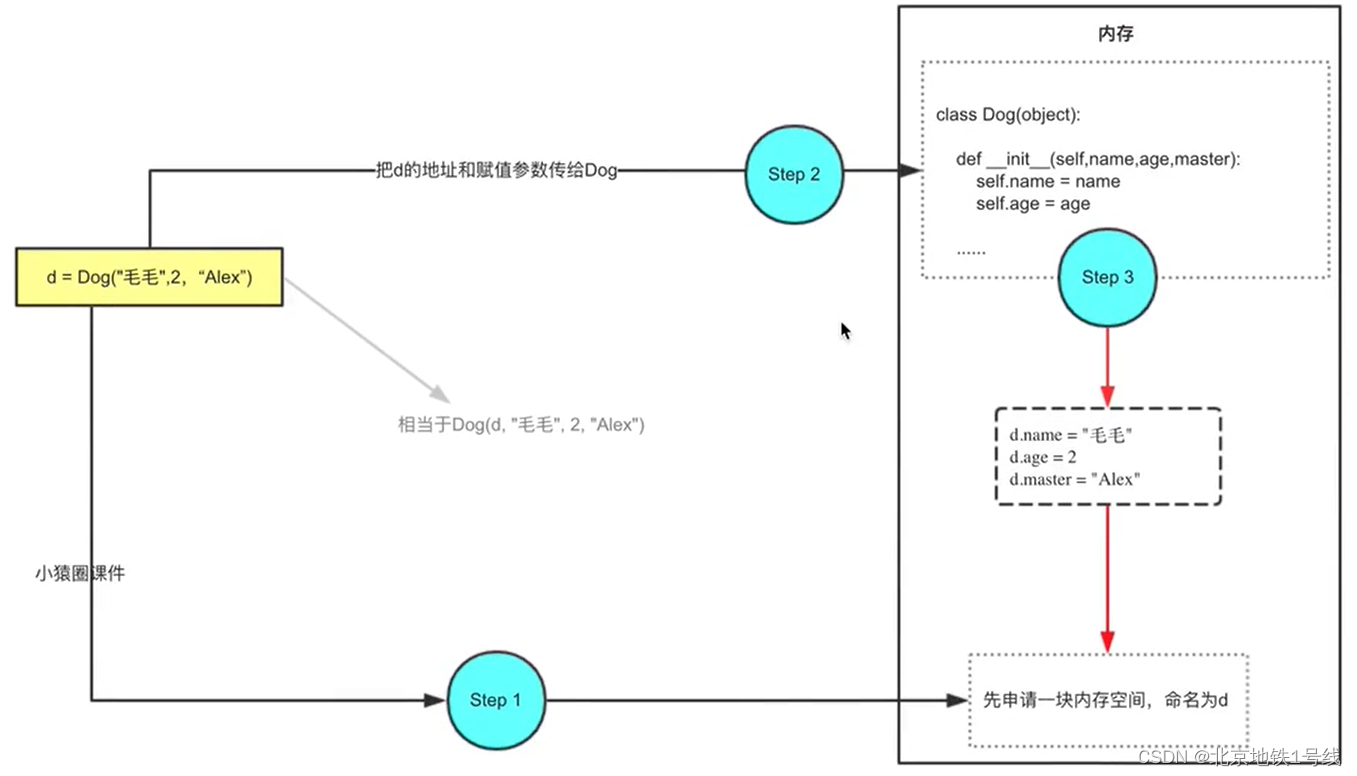
python中的类与对象(2)
目录 一. 类的基本语法 二. 类属性的应用场景 三. 类与类之间的依赖关系 (1)依赖关系 (2)关联关系 (3)组合关系 四. 类的继承 一. 类的基本语法 先看一段最简单的代码: class Dog():d_…...
Android横竖屏切换configChanges=“screenSize|orientation“避免activity销毁重建,Kotlin
Android横竖屏切换configChanges"screenSize|orientation"避免activity销毁重建,Kotlin 如果不在Androidmanifest.xml设置activity的: android:configChanges"screenSize|orientation" 那么,每次横竖屏切换activity都会…...
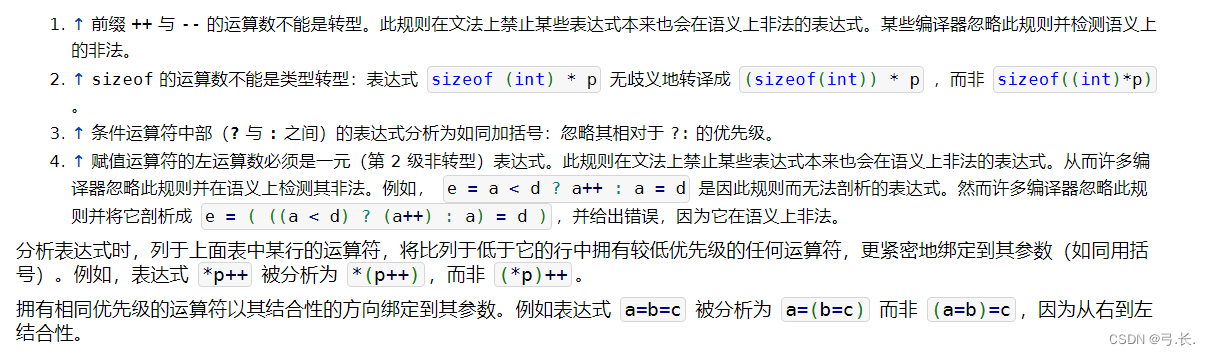
【C语言基础】:操作符详解(二)
文章目录 操作符详解一、上期扩展二、单目操作符三、逗号表达式四、下标访问[]、 函数调用()五、结构成员访问操作符六、操作符的属性:优先级、结合性1. 优先级2. 结合性 操作符详解 上期回顾:【C语言基础】:操作符详解(一) 一、上期扩展 …...
模型训练基本结构
project_name/ │ ├── data/ │ ├── raw/ # 存放原始数据 │ ├── processed/ # 存放预处理后的数据 │ └── splits/ # 存放数据集划分(训练集、验证集、测试集等) │ ├── noteboo…...
Redis 数据结构详解:底层实现与高效使用场景
String(字符串) 底层实现细节: 动态字符串(SDS): SDS相比于C语言的原生字符串,提供了自动内存管理和预分配机制。当字符串长度增加时,SDS会预先分配额外的空间,以减少内存重新分配…...
Vue2:router-link的replace属性
一、情景说明 我们在用浏览器访问网站的时候 知道浏览器会记录访问的历史路径,从而,可以退回到之前的页面 那么,Vue项目中的路由组件,通过router-link跳转,也是可以退回的 这里,我们用replace来屏蔽退回的…...
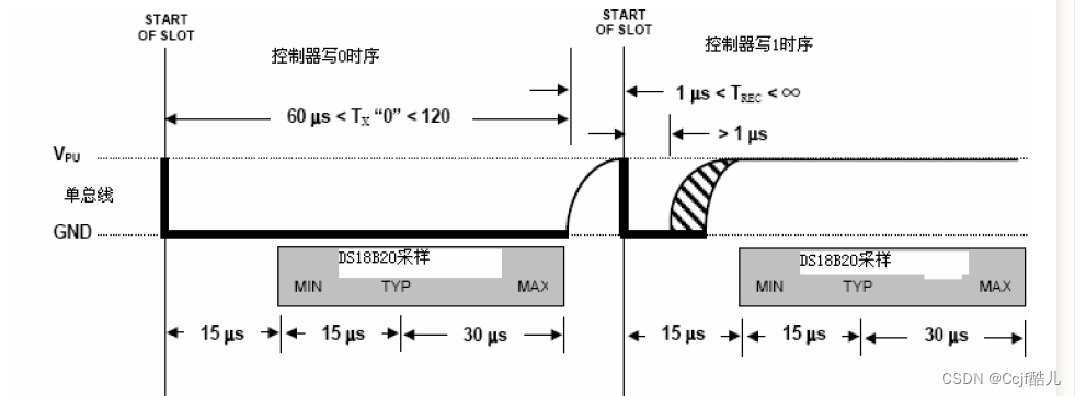
普中51单片机(DS18B20温度传感器)
DS18B20温度传感器原理 内部结构 64位(激)光刻只读存储器 光刻ROM中的64位序列号是出厂前被光刻好的,它可以看作是该DS18B20的地址序列号。64位光刻ROM的排列是:开始8位(28H)是产品类型标号,接着的48位是该DS18B20自身…...
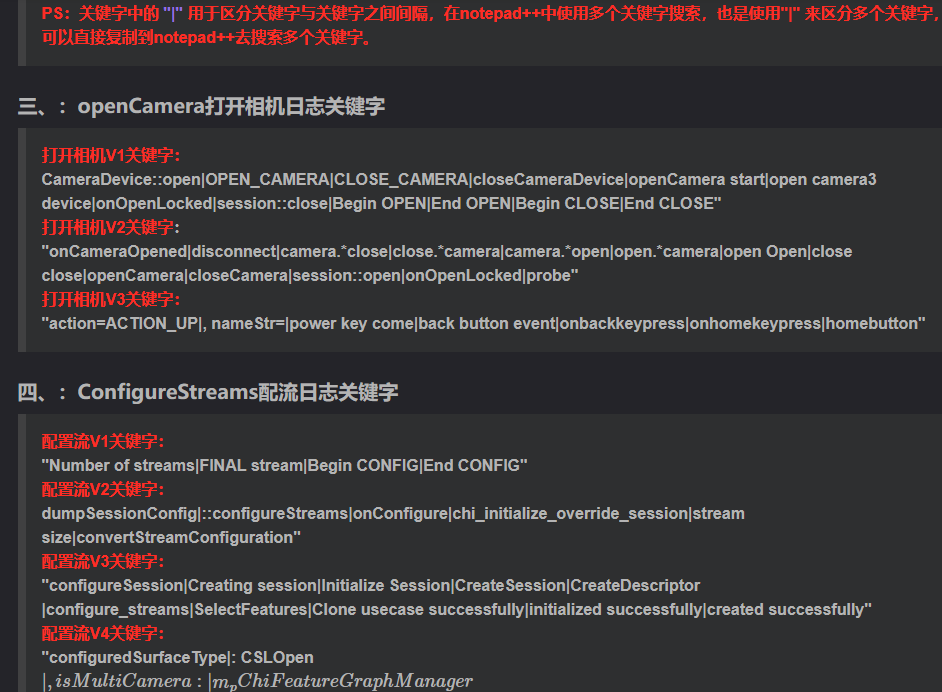
相机Camera日志实例分析之二:相机Camx【专业模式开启直方图拍照】单帧流程日志详解
【关注我,后续持续新增专题博文,谢谢!!!】 上一篇我们讲了: 这一篇我们开始讲: 目录 一、场景操作步骤 二、日志基础关键字分级如下 三、场景日志如下: 一、场景操作步骤 操作步…...
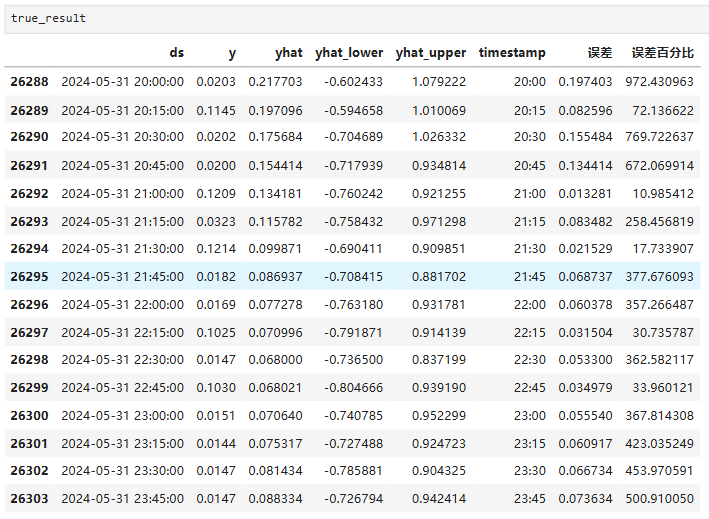
Python实现prophet 理论及参数优化
文章目录 Prophet理论及模型参数介绍Python代码完整实现prophet 添加外部数据进行模型优化 之前初步学习prophet的时候,写过一篇简单实现,后期随着对该模型的深入研究,本次记录涉及到prophet 的公式以及参数调优,从公式可以更直观…...
鱼香ros docker配置镜像报错:https://registry-1.docker.io/v2/
使用鱼香ros一件安装docker时的https://registry-1.docker.io/v2/问题 一键安装指令 wget http://fishros.com/install -O fishros && . fishros出现问题:docker pull 失败 网络不同,需要使用镜像源 按照如下步骤操作 sudo vi /etc/docker/dae…...
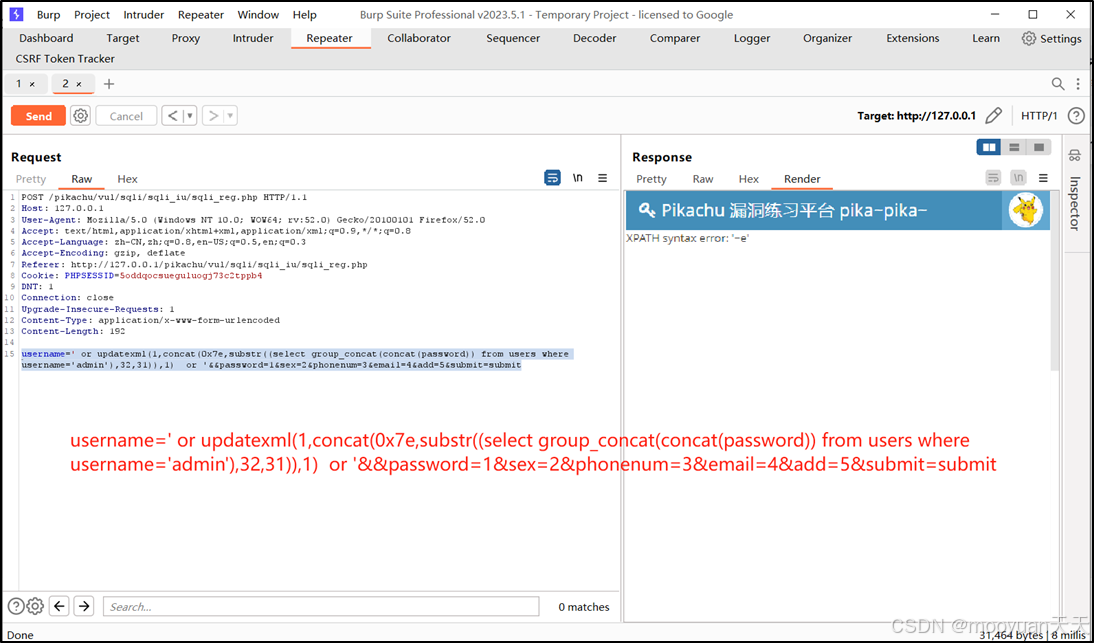
pikachu靶场通关笔记22-1 SQL注入05-1-insert注入(报错法)
目录 一、SQL注入 二、insert注入 三、报错型注入 四、updatexml函数 五、源码审计 六、insert渗透实战 1、渗透准备 2、获取数据库名database 3、获取表名table 4、获取列名column 5、获取字段 本系列为通过《pikachu靶场通关笔记》的SQL注入关卡(共10关࿰…...
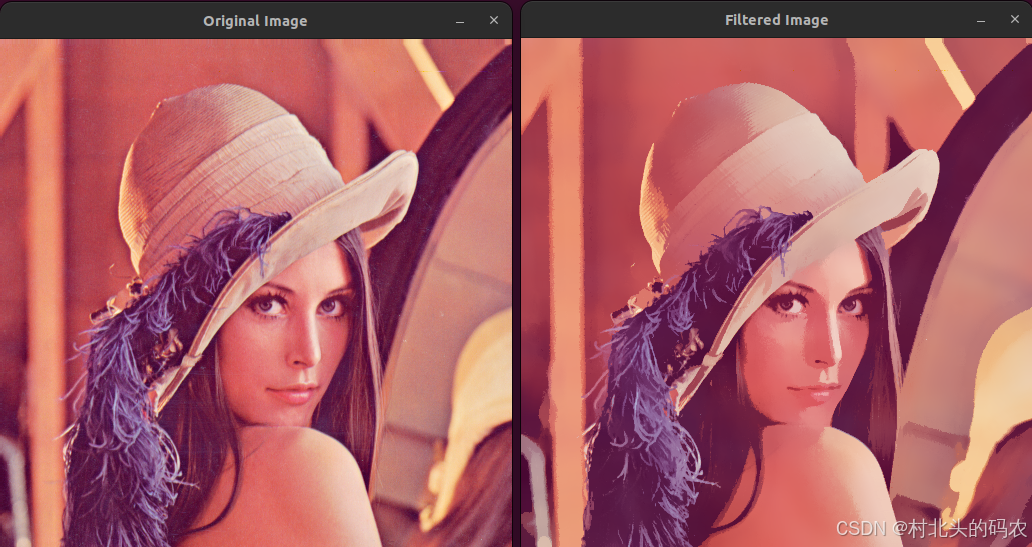
OPenCV CUDA模块图像处理-----对图像执行 均值漂移滤波(Mean Shift Filtering)函数meanShiftFiltering()
操作系统:ubuntu22.04 OpenCV版本:OpenCV4.9 IDE:Visual Studio Code 编程语言:C11 算法描述 在 GPU 上对图像执行 均值漂移滤波(Mean Shift Filtering),用于图像分割或平滑处理。 该函数将输入图像中的…...
React---day11
14.4 react-redux第三方库 提供connect、thunk之类的函数 以获取一个banner数据为例子 store: 我们在使用异步的时候理应是要使用中间件的,但是configureStore 已经自动集成了 redux-thunk,注意action里面要返回函数 import { configureS…...
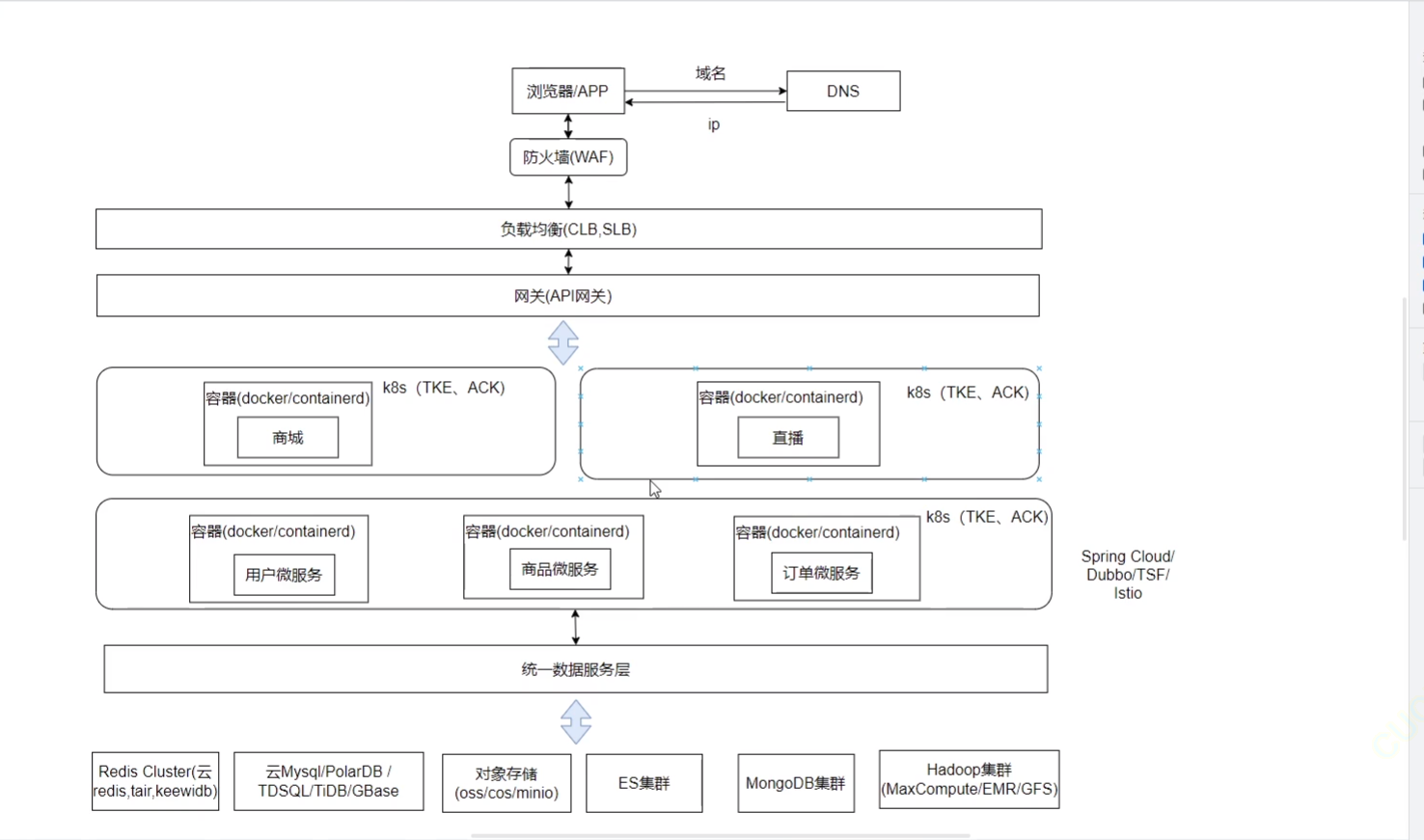
《Docker》架构
文章目录 架构模式单机架构应用数据分离架构应用服务器集群架构读写分离/主从分离架构冷热分离架构垂直分库架构微服务架构容器编排架构什么是容器,docker,镜像,k8s 架构模式 单机架构 单机架构其实就是应用服务器和单机服务器都部署在同一…...
二维FDTD算法仿真
二维FDTD算法仿真,并带完全匹配层,输入波形为高斯波、平面波 FDTD_二维/FDTD.zip , 6075 FDTD_二维/FDTD_31.m , 1029 FDTD_二维/FDTD_32.m , 2806 FDTD_二维/FDTD_33.m , 3782 FDTD_二维/FDTD_34.m , 4182 FDTD_二维/FDTD_35.m , 4793...
Python学习(8) ----- Python的类与对象
Python 中的类(Class)与对象(Object)是面向对象编程(OOP)的核心。我们可以通过“类是模板,对象是实例”来理解它们的关系。 🧱 一句话理解: 类就像“图纸”,对…...
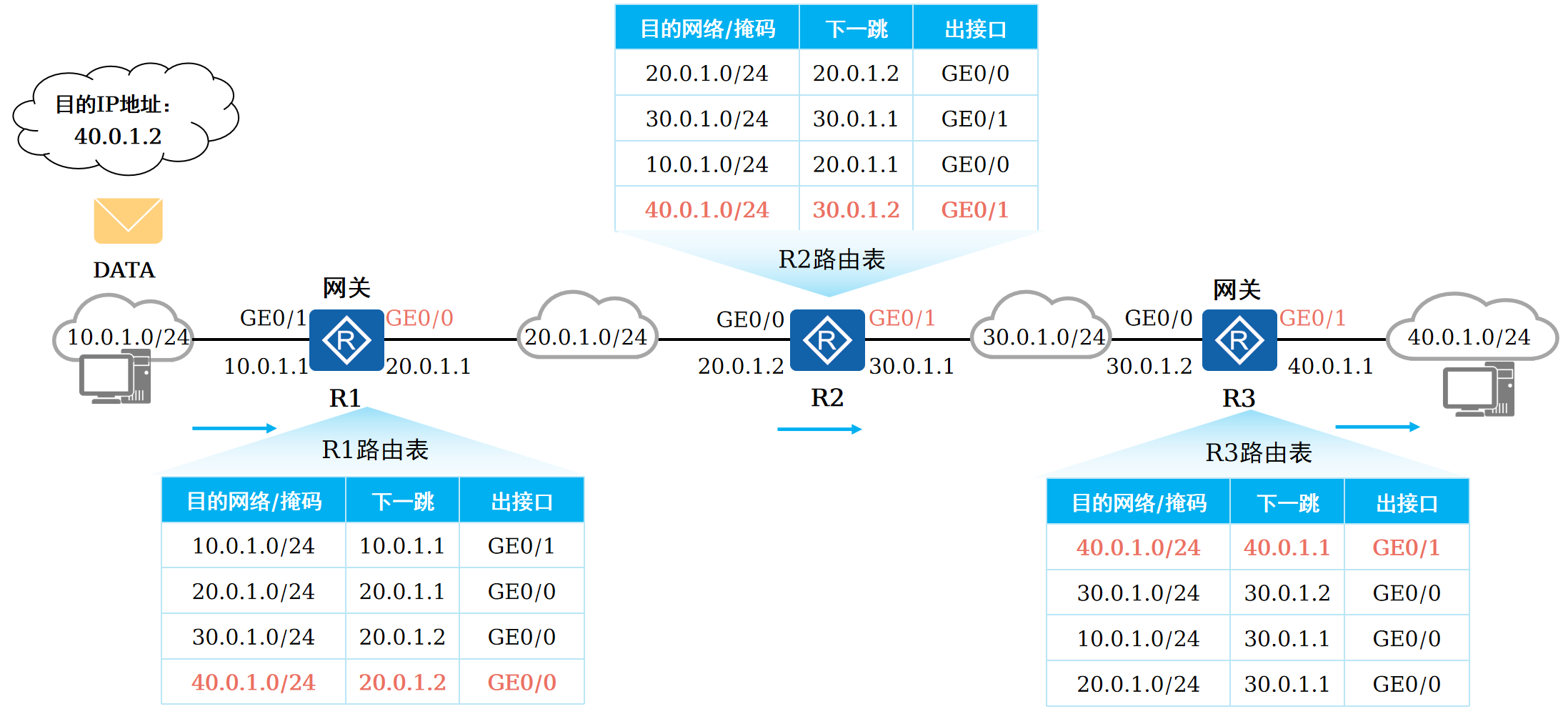
路由基础-路由表
本篇将会向读者介绍路由的基本概念。 前言 在一个典型的数据通信网络中,往往存在多个不同的IP网段,数据在不同的IP网段之间交互是需要借助三层设备的,这些设备具备路由能力,能够实现数据的跨网段转发。 路由是数据通信网络中最基…...