10 mybatis 日志
文章目录
- product.sql
- pom.xml
- logback.xml
- mybatis-config.xml
- ProductsMapper.xml
- Products
- ProductsMapper.java
product.sql
create table products
(product_id int auto_increment comment '产品ID'primary key,product_name varchar(100) null comment '产品名称',brand varchar(50) null comment '品牌',price decimal(10, 2) null comment '价格',color varchar(20) null comment '颜色',storage_capacity varchar(10) null comment '存储容量',description text null comment '描述'
)comment '手机产品表';
pom.xml
<?xml version="1.0" encoding="UTF-8"?>
<project xmlns="http://maven.apache.org/POM/4.0.0"xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd"><modelVersion>4.0.0</modelVersion><groupId>com.aistat</groupId><artifactId>mybatis_tech</artifactId><version>1.0-SNAPSHOT</version><!--打包类型--><packaging>jar</packaging><properties><maven.compiler.source>8</maven.compiler.source><maven.compiler.target>8</maven.compiler.target><project.build.sourceEncoding>UTF-8</project.build.sourceEncoding></properties><!--所有依赖--><dependencies><!-- MySQL驱动--><dependency><groupId>com.mysql</groupId><artifactId>mysql-connector-j</artifactId><version>8.0.31</version></dependency><!-- mybatis依赖--><dependency><groupId>org.mybatis</groupId><artifactId>mybatis</artifactId><version>3.5.15</version></dependency><!--测试环境--><dependency><groupId>junit</groupId><artifactId>junit</artifactId><version>4.13.2</version><scope>test</scope></dependency>
<!--Lombok--><dependency><groupId>org.projectlombok</groupId><artifactId>lombok</artifactId><version>1.14.8</version><scope>provided</scope></dependency><!-- logback日志 slf规范-->
<!-- 配置文件也是在resouce目录下,且只能以logback.xml/logback-test.xml命名--><dependency><groupId>ch.qos.logback</groupId><artifactId>logback-classic</artifactId><version>1.2.11</version></dependency></dependencies></project>
logback.xml
<?xml version="1.0" encoding="UTF-8"?>
<configuration ><!--0. 日志格式和颜色渲染 --><!-- 彩色日志依赖的渲染类 --><conversionRule conversionWord="clr" converterClass="org.springframework.boot.logging.logback.ColorConverter" /><conversionRule conversionWord="wex" converterClass="org.springframework.boot.logging.logback.WhitespaceThrowableProxyConverter" /><conversionRule conversionWord="wEx" converterClass="org.springframework.boot.logging.logback.ExtendedWhitespaceThrowableProxyConverter" /><!-- 彩色日志格式 --><property name="CONSOLE_LOG_PATTERN" value="${CONSOLE_LOG_PATTERN:-%clr(%d{yyyy-MM-dd HH:mm:ss.SSS}){faint} %clr(${LOG_LEVEL_PATTERN:-%5p}) %clr(${PID:- }){magenta} %clr(---){faint} %clr([%15.15t]){faint} %clr(%-40.40logger{39}){cyan} %clr(:){faint} %m%n${LOG_EXCEPTION_CONVERSION_WORD:-%wEx}}"/><!--CONSOLE :表示当前的日志信息是可以输出到控制台的。--><appender name="Console" class="ch.qos.logback.core.ConsoleAppender"><encoder><pattern>[%level] %blue(%d{HH:mm:ss.SSS}) %cyan([%thread]) %boldGreen(%logger{15}) - %msg %n</pattern></encoder></appender><!--触动到定义部分,就输出对应级别日志--><logger name="org.apache.ibatis" level="TRACE"/><logger name ="java.sql.Connection" level="DEBUG"/><logger name="java.sql.PreparedStatement" level="DEBUG"/><logger name="java.sql.Statement" level="DEBUG"/><logger name="com.aistart.tech.mapper" level="DEBUG"/><!--level:用来设置打印级别,大小写无关:TRACE, DEBUG, INFO, WARN, ERROR, ALL 和 OFF, 默认debugINFO类似于sout<root>可以包含零个或多个<appender-ref>元素,标识这个输出位置将会被本日志级别控制。--><root level="DEBUG"><appender-ref ref="Console"/></root>
</configuration>
mybatis-config.xml
<?xml version="1.0" encoding="UTF-8" ?>
<!DOCTYPE configurationPUBLIC "-//mybatis.org//DTD Config 3.0//EN""http://mybatis.org/dtd/mybatis-3-config.dtd">
<configuration><settings><setting name="mapUnderscoreToCamelCase" value="true"/></settings><typeAliases><package name="com.aistart.tech.pojo"/></typeAliases><!-- <typeAliases>-->
<!-- <typeAlias alias="Products" type="com.aistart.tech.pojo.Products"/>--><!-- </typeAliases>--><environments default="development"><environment id="development"><transactionManager type="JDBC"/><dataSource type="POOLED"><property name="driver" value="com.mysql.cj.jdbc.Driver"/><property name="url" value="jdbc:mysql://localhost:3306/mybatisdb"/><property name="username" value="root"/><property name="password" value="root"/><property name="poolMaximumActiveConnections" value="1"/></dataSource></environment><environment id="testdevelopment"><transactionManager type="JDBC"/><dataSource type="POOLED"><property name="driver" value="com.mysql.cj.jdbc.Driver"/><property name="url" value="jdbc:mysql://localhost:3306/test"/><property name="username" value="root"/><property name="password" value="root"/></dataSource></environment></environments><mappers><mapper class="com.aistart.tech.mapper.ProductsMapper"/><!-- <mapper resource="com/aistart/tech/mapper/ProductsMapper.xml"></mapper>--></mappers>
</configuration>
ProductsMapper.xml
<?xml version="1.0" encoding="UTF-8" ?>
<!DOCTYPE mapperPUBLIC "-//mybatis.org//DTD Mapper 3.0//EN""http://mybatis.org/dtd/mybatis-3-mapper.dtd">
<mapper namespace="com.aistart.tech.mapper.ProductsMapper"><sql id="all">product_id, product_name, brand, price, color, storage_capacity, description</sql><resultMap id="selectOneMap" type="com.aistart.tech.dto.PhoneDto"><result column="product_name" property="name"/><result column="price" property="jg"/></resultMap><sql id="select">select <include refid="all"/> from productswhere</sql><!--XML tag has empty body一个标签中resultmap和resultType只能存在一个用到了resultmap就写resultmap--><select id="selectOneMap" resultMap="selectOneMap"><include refid="select"/>product_id = 1;</select><select id="selectOneByNameAndPrice" resultType="Products"><include refid="select"/>product_name = #{productName}andprice > #{price}</select><!--调用 sqlSession.selectOne("com.aistart.tech.mapper.ProductsMapper.selectOne",products)--><!--id作为这个sql的唯一id,调用也是通过它--><insert id="insertOne" parameterType="Products" ><selectKey keyProperty="productId" keyColumn="product_id" resultType="int" order="AFTER">select last_insert_id();</selectKey>insert into products (product_name,color,price)values (#{productName},#{color},#{price})</insert><delete id="deleteOneById">delete from products where product_id = #{askdksad}</delete><update id="updateProductName" parameterType="Products">update products set product_name = #{productName} where product_id = #{productId}</update><select id="selectOneById" parameterType="int" resultType="com.aistart.tech.pojo.Products">/*参数名一个时虽然可以随便写,但是不推荐*/select<include refid="all"/>from products where product_id = #{productId}/*默认调用了get函数,利用反射,根据get+param()的形式找函数并且执行*/</select><!--JDBC>>>>sql>>>>resultSet>>>获取列并遍历>>>>>>--><select id="selectAll" resultType="com.aistart.tech.pojo.Products">select<include refid="all"></include>from products</select><select id="selectOneBynName" resultType="Products"><include refid="select"></include>product_name = #{name}</select>
</mapper>
Products
package com.aistart.tech.pojo;import lombok.*;@Data
@AllArgsConstructor
@NoArgsConstructor
public class Products {private Integer productId;private String productName;private String brand;private Double price;private String color;private String storageCapacity;private String description;}
ProductsMapper.java
package com.aistart.tech.mapper;import com.aistart.tech.dto.PhoneDto;
import com.aistart.tech.pojo.Products;
import org.apache.ibatis.annotations.*;public interface ProductsMapper {/** 三种传参数方式** 1.直接对象传参* 2.map传参* 3.参数传参* a.单一传值,不用在乎变量名* b.多值时,必须指明参数*** */public int insertOne(Products products);public Products selectOneById(int num);/*** todo:* 重要字段: id(函数名) 传入类型(参数) 返回类型(返回类型)* sql语句 select标签 @Select sql语句("")**** 跟之前用mapper语法一致,传参数有以下几个条件* 1.单一值时,随便,自动匹配* 2.多参数时* a.多个参数@Param("映射的#{}中的`字段")* b.对象/map,注意映射字段名* @param name(mapper中的paramType)* @return (resultType)*/@Select("select * from products where product_name = #{name}")public Products selectOneByName(String name);//在参数前指定映射
// @Select("select product_name from products where product_name = #{productName} and price > #{price}")public Products selectOneByNameAndPrice(@Param("productName") String productName,@Param("price") double price);@Results({@Result(property = "name", column = "product_name"),@Result(property = "jg", column = "price")})@Select("select product_name,price from products where product_name = #{productName}")public PhoneDto selectDtoByName(@Param("productName") String productName);/** <select id = "selectDtoByName" paramType ="String" resultType = "PhoneDto" >* select product_name from products where product_name = #{productName}** </select>* */
}
相关文章:
10 mybatis 日志
文章目录 product.sqlpom.xmllogback.xmlmybatis-config.xmlProductsMapper.xmlProductsProductsMapper.java product.sql create table products (product_id int auto_increment comment 产品IDprimary key,product_name varchar(100) null comment 产品名称,bra…...
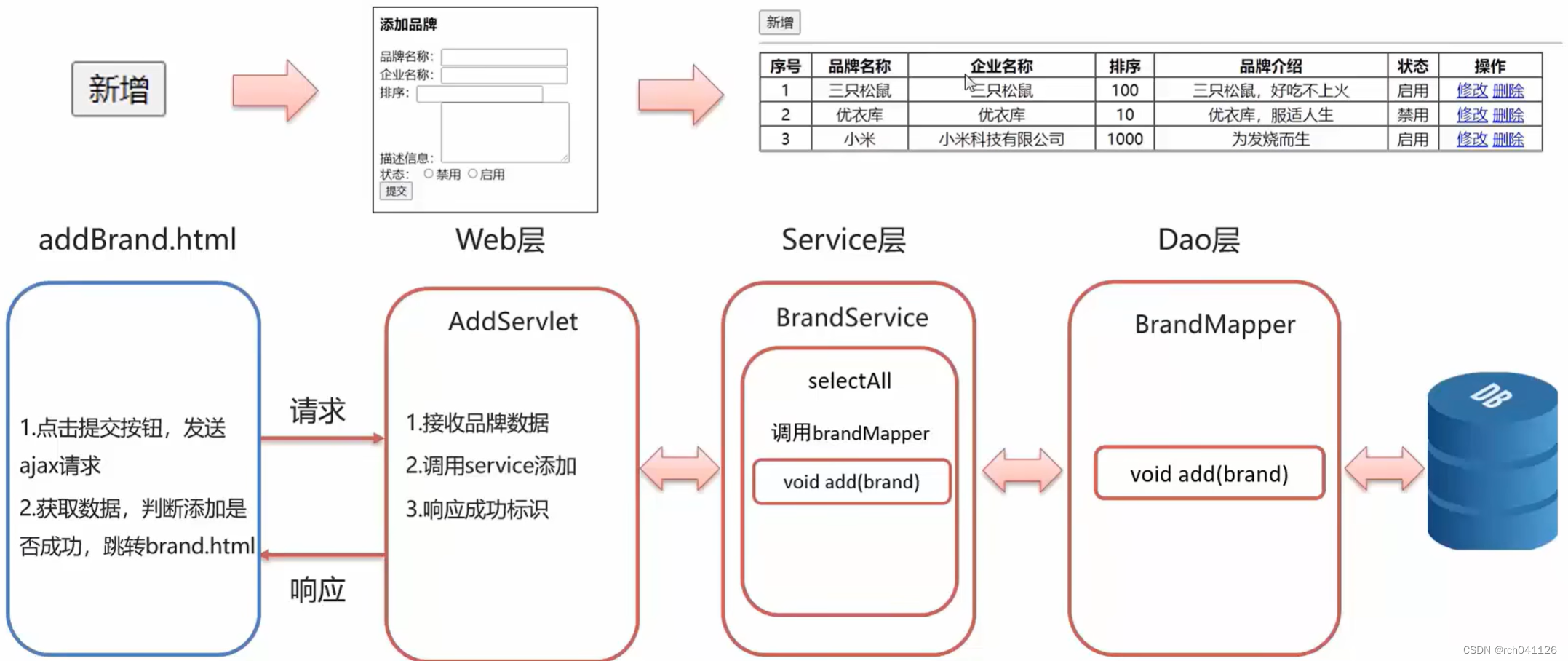
AJAX介绍使用案例
文章目录 一、AJAX概念二、AJAX快速入门1、编写AjaxServlet,并使用response输出字符(后台代码)2、创建XMLHttpRequest对象:用于和服务器交换数据 & 3、向服务器发送请求 & 4、获取服务器响应数据 三、案例-验证用户是否存…...
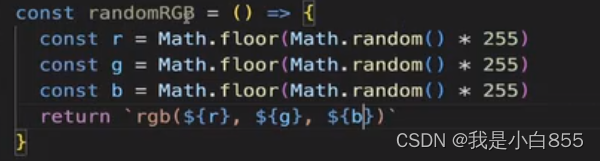
【echart】数据可视化
什么是数据可视化? 数据可视化主要目的:借助于图形化手段,清晰有效地传达与沟通信息。 数据可视化可以把数据从冰冷的数字转换成图形,揭示蕴含在数据中的规律和道理。 如何绘制? echarts 图表的绘制,大体分为三步:…...
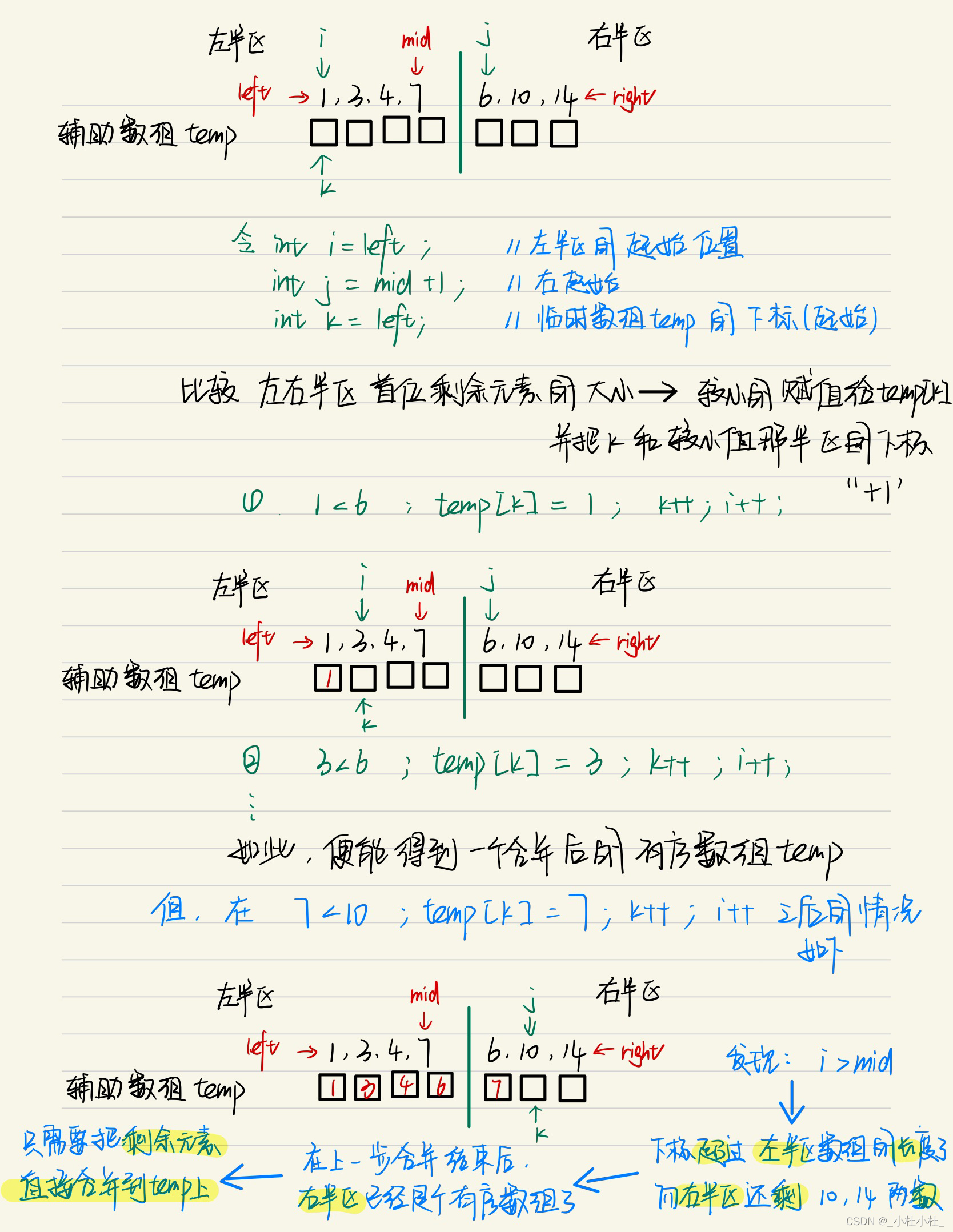
排序(冒泡/快速/归并)
冒泡排序 时间复杂度为 O(n^2) 原理 比较相邻的元素. 如果第一个比第二个大,就交换他们两个.依次比较每一对相邻的元素—>结果 : 最后的元素是这组数中最大的重复上述步骤 , 除了最后一个[]因为最后一个已经是排好序的了(这组数中最大的那个)]持续对越来越少的元素进行如上…...

jq中的跨域
跨域 1.从一个地址到另外一个第一请求资源或者数据时,就有可能发生跨域 <!DOCTYPE html> <html lang"en"> <head><meta charset"UTF-8"><title>跨域</title><script src"jquery/jquery-1.11.3.j…...
CUDA学习笔记08: 原子规约/向量求和
参考资料 CUDA编程模型系列一(核心函数)_哔哩哔哩_bilibili 代码 #include <iostream> #include <cuda_runtime.h> #include <device_launch_parameters.h> #include <stdio.h> #include <math.h>#define N 10000000 #define BLOCK 256 #def…...
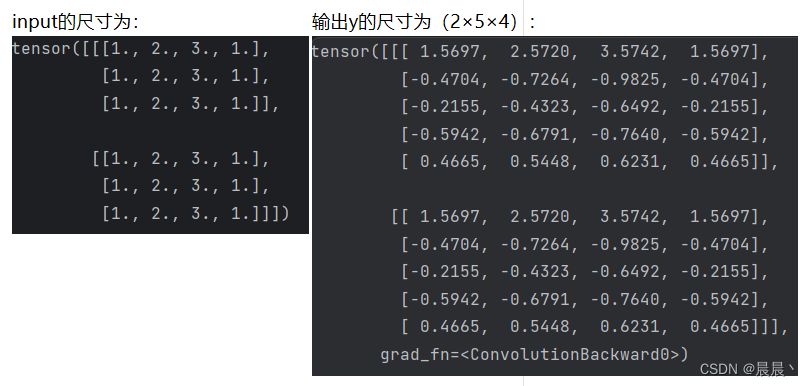
PointNet++论文复现(一)【PontNet网络模型代码详解 - 分类部分】
PontNet网络模型代码详解 - 分类部分 专栏持续更新中!关注博主查看后续部分! 分类模型的训练: ## e.g., pointnet2_ssg without normal features python train_classification.py --model pointnet2_cls_ssg --log_dir pointnet2_cls_ssg python test_classification.py…...
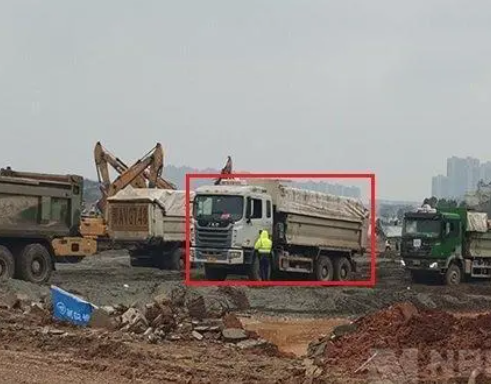
AI渣土车监测报警摄像机
随着城市建设的不断发展和交通运输的快速增长,渣土车作为建筑行业中不可或缺的运输工具,承担着大量的渣土运输任务。然而,由于渣土车在运输过程中存在超速、违规变道、碾压行人等交通安全问题,给道路交通和行人安全带来了严重的隐…...
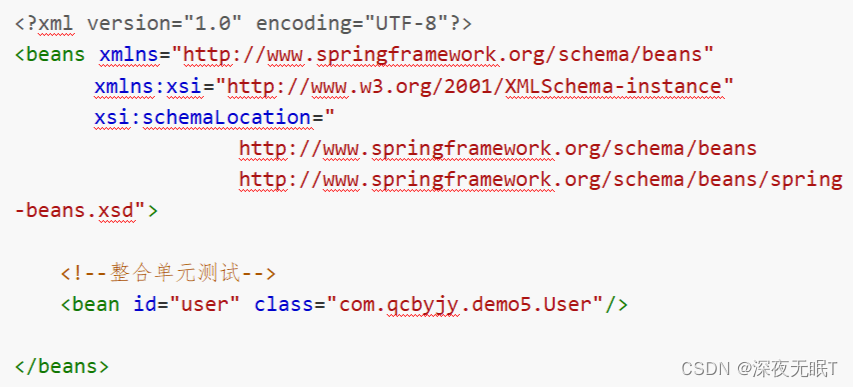
Spring框架介绍及详细使用
前言 本篇文章将会对spring框架做出一个比较详细的讲解,并且每个知识点基本都会有例子演示,详细记录下了我在学习Spring时所了解到全部知识点。 在了解是什么spring之前,我们要先知道spring框架在开发时,服务器端采用三层架构的方…...
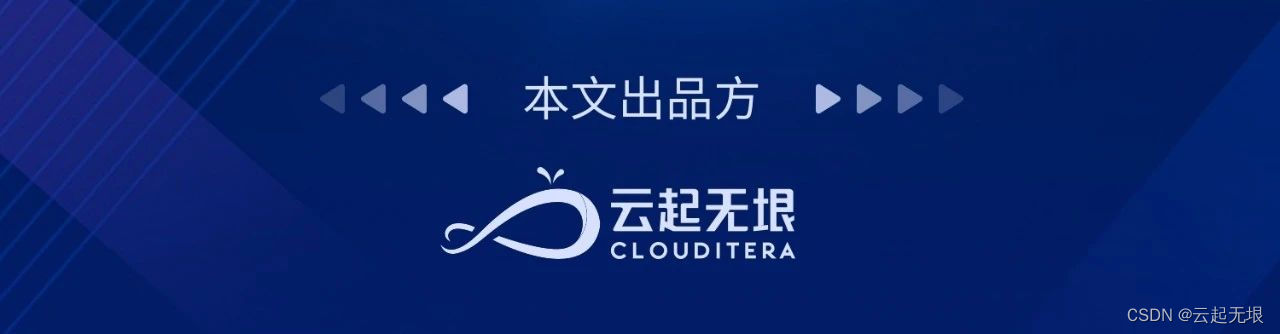
【论文速读】| 对大语言模型解决攻击性安全挑战的实证评估
本次分享论文为:An Empirical Evaluation of LLMs for Solving Offensive Security Challenges 基本信息 原文作者:Minghao Shao, Boyuan Chen, Sofija Jancheska, Brendan Dolan-Gavitt, Siddharth Garg, Ramesh Karri, Muhammad Shafique 作者单位&a…...

小迪安全48WEB 攻防-通用漏洞Py 反序列化链构造自动审计 bandit魔术方法
#知识点: 1、Python-反序列化函数使用 2、Python-反序列化魔术方法 3、Python-反序列化 POP 链构造(payload构造) 4、Python-自动化审计 bandit 使用 #前置知识: 函数使用: pickle.dump(obj, file) : 将对…...
微服务:解放软件开发的神器,引领企业级应用的未来(二)
本系列文章简介: 本系列文章将深入剖析微服务架构的原理、设计和实践,向大家介绍微服务的核心概念和关键技术,以及在实际项目中的应用和实践经验。我们将通过具体的案例和实例,帮助大家理解微服务架构的优势和挑战,掌握…...
easyexcel与vue配合下载excel
后端 设置响应 // 设置响应头 response.setContentType("application/octet-stream;charsetUTF-8"); String returnName null; try {returnName URLEncoder.encode(fileName, "UTF-8"); } catch (UnsupportedEncodingException e) {throw new RuntimeExc…...
Vue.js 模板语法
Vue.js 使用了基于 HTML 的模板语法,允许开发者声明式地将 DOM 绑定至底层 Vue 实例的数据。 Vue.js 的核心是一个允许你采用简洁的模板语法来声明式的将数据渲染进 DOM 的系统。 结合响应系统,在应用状态改变时, Vue 能够智能地计算出重新…...
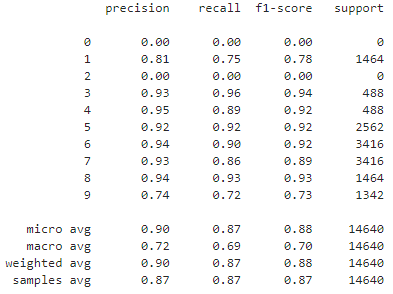
信号处理--基于DEAP数据集的情绪分类的典型深度学习模型构建
关于 本实验采用DEAP情绪数据集进行数据分类任务。使用了三种典型的深度学习网络:2D 卷积神经网络;1D卷积神经网络GRU; LSTM网络。 工具 数据集 DEAP数据 图片来源: DEAP: A Dataset for Emotion Analysis using Physiological…...
Spring设计模式-实战篇之模板方法模式
什么是模板方法模式? 模板方法模式用于定义一个算法的框架,并允许子类在不改变该算法结构的情况下重新定义算法中的某些步骤。这种模式提供了一种将算法的通用部分封装在一个模板方法中,而将具体步骤的实现延迟到子类中的方式。 模板方法模式…...
PTA天梯赛习题 L2-006 树的遍历
先序遍历:根-左-右 > 序列的第一个数就是根 中序遍历:左-根-右 > 知道中间某一个数为根,则这个数的左边就是左子树,右边则是右子树 后序遍历:左-右-根 > 序列的最后一个数就是根 题目 给定一棵…...
js相关的dom方法
查找元素 //获取元素id为box的元素 document.getElementById(box) //获取元素类名为box的元素 document.getElementsByClassName(box) //获取标签名为div的元素 document.getElementsByTagName(div)改变元素 //设置id为box的元素内容 document.getElementById("box"…...
Django——Ajax请求
Django——Ajax请求 一、响应 Json 数据 path(str/ , views.str_view), path(json/ , views.json_view), path(jsonresponse/ , views.jsonresponse_view), path(ls/ , views.ls),from django.shortcuts import render , HttpResponse from django.http import JsonResponse …...
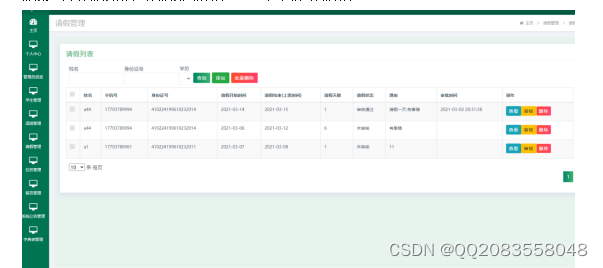
基于java多角色学生管理系统论文
摘 要 现代经济快节奏发展以及不断完善升级的信息化技术,让传统数据信息的管理升级为软件存储,归纳,集中处理数据信息的管理方式。本学生管理系统就是在这样的大环境下诞生,其可以帮助管理者在短时间内处理完毕庞大的数据信息&am…...
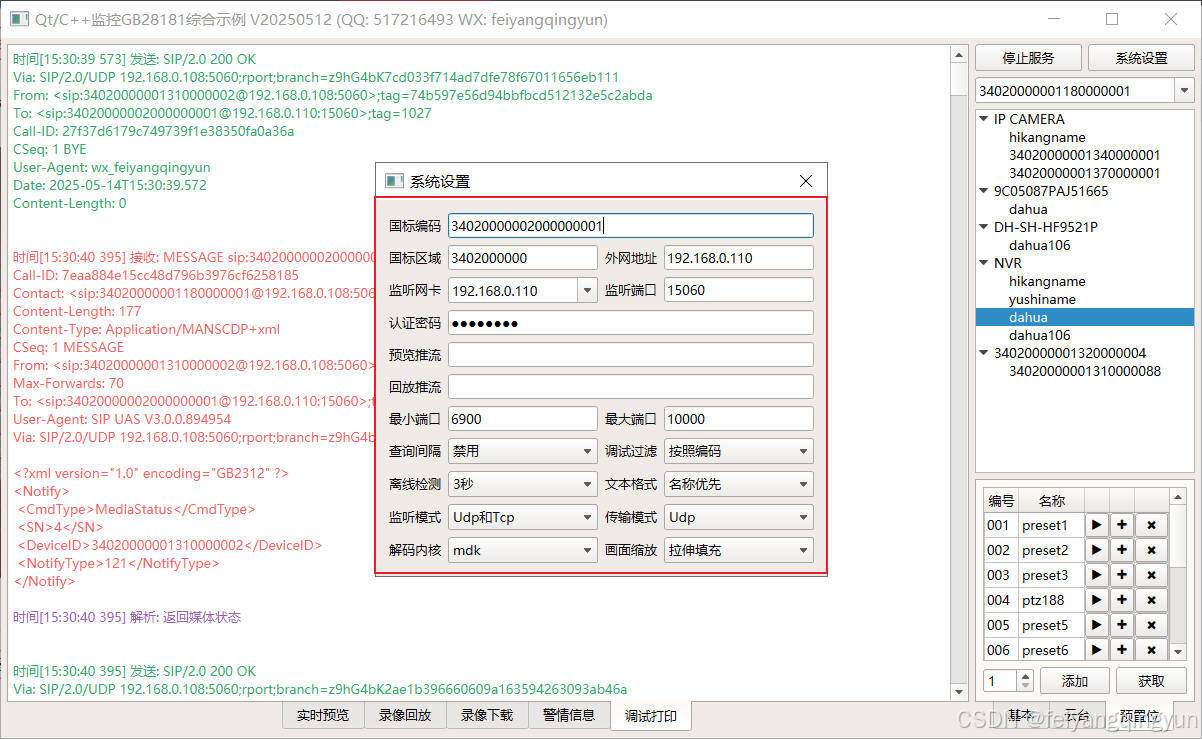
Qt/C++开发监控GB28181系统/取流协议/同时支持udp/tcp被动/tcp主动
一、前言说明 在2011版本的gb28181协议中,拉取视频流只要求udp方式,从2016开始要求新增支持tcp被动和tcp主动两种方式,udp理论上会丢包的,所以实际使用过程可能会出现画面花屏的情况,而tcp肯定不丢包,起码…...
前端倒计时误差!
提示:记录工作中遇到的需求及解决办法 文章目录 前言一、误差从何而来?二、五大解决方案1. 动态校准法(基础版)2. Web Worker 计时3. 服务器时间同步4. Performance API 高精度计时5. 页面可见性API优化三、生产环境最佳实践四、终极解决方案架构前言 前几天听说公司某个项…...
IGP(Interior Gateway Protocol,内部网关协议)
IGP(Interior Gateway Protocol,内部网关协议) 是一种用于在一个自治系统(AS)内部传递路由信息的路由协议,主要用于在一个组织或机构的内部网络中决定数据包的最佳路径。与用于自治系统之间通信的 EGP&…...
Objective-C常用命名规范总结
【OC】常用命名规范总结 文章目录 【OC】常用命名规范总结1.类名(Class Name)2.协议名(Protocol Name)3.方法名(Method Name)4.属性名(Property Name)5.局部变量/实例变量(Local / Instance Variables&…...
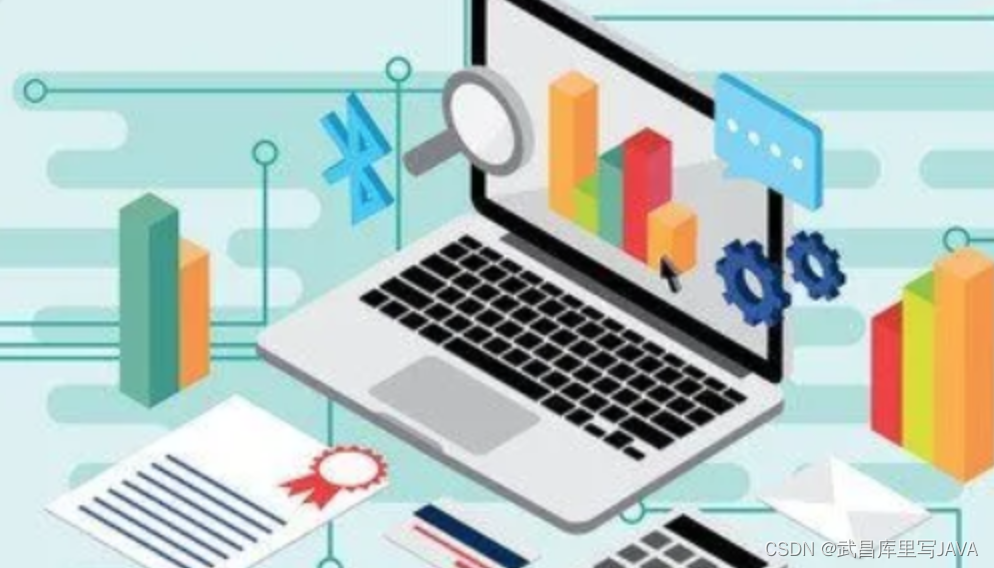
2021-03-15 iview一些问题
1.iview 在使用tree组件时,发现没有set类的方法,只有get,那么要改变tree值,只能遍历treeData,递归修改treeData的checked,发现无法更改,原因在于check模式下,子元素的勾选状态跟父节…...
Python 包管理器 uv 介绍
Python 包管理器 uv 全面介绍 uv 是由 Astral(热门工具 Ruff 的开发者)推出的下一代高性能 Python 包管理器和构建工具,用 Rust 编写。它旨在解决传统工具(如 pip、virtualenv、pip-tools)的性能瓶颈,同时…...
动态 Web 开发技术入门篇
一、HTTP 协议核心 1.1 HTTP 基础 协议全称 :HyperText Transfer Protocol(超文本传输协议) 默认端口 :HTTP 使用 80 端口,HTTPS 使用 443 端口。 请求方法 : GET :用于获取资源,…...
JS手写代码篇----使用Promise封装AJAX请求
15、使用Promise封装AJAX请求 promise就有reject和resolve了,就不必写成功和失败的回调函数了 const BASEURL ./手写ajax/test.jsonfunction promiseAjax() {return new Promise((resolve, reject) > {const xhr new XMLHttpRequest();xhr.open("get&quo…...
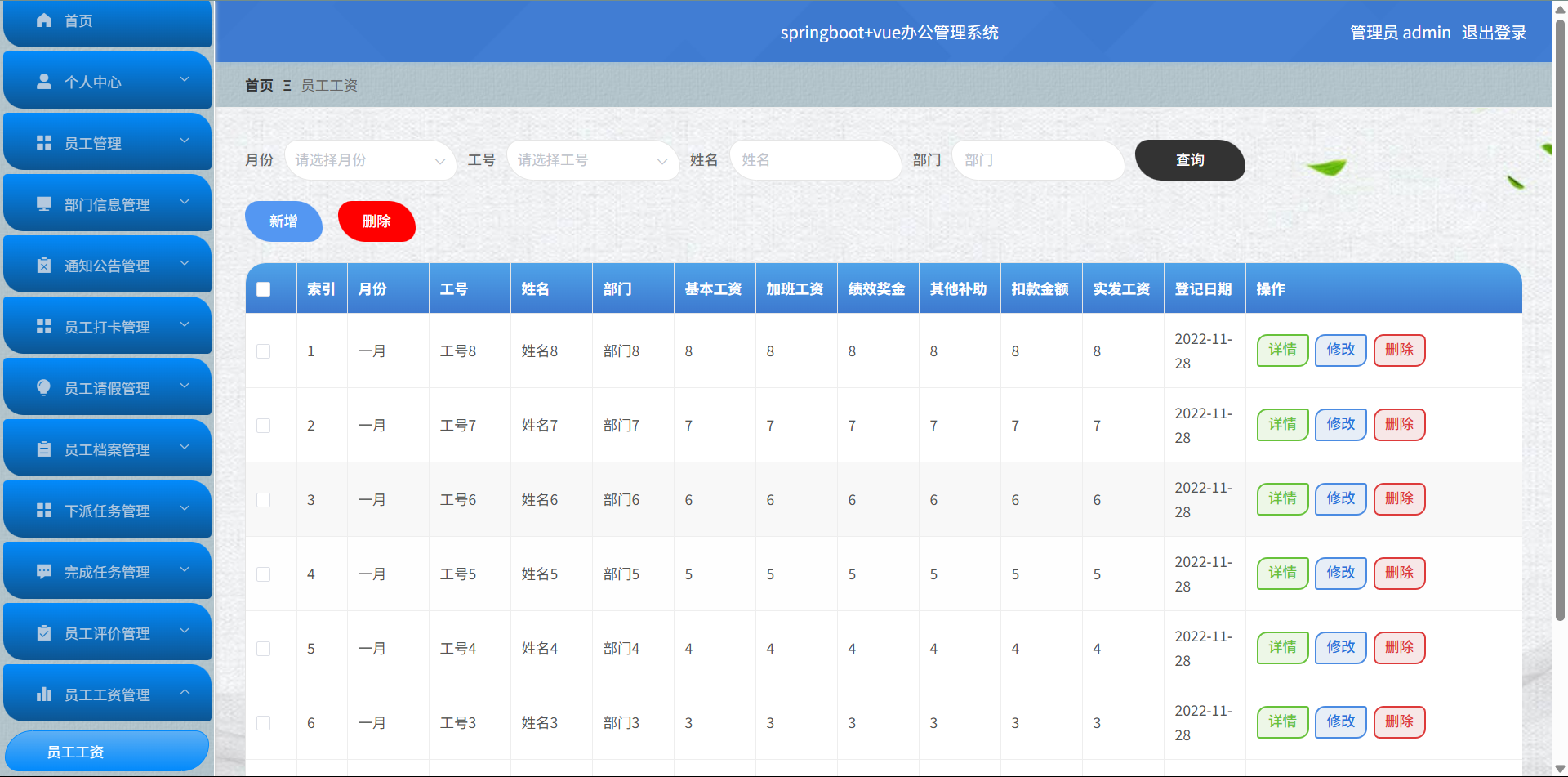
基于Springboot+Vue的办公管理系统
角色: 管理员、员工 技术: 后端: SpringBoot, Vue2, MySQL, Mybatis-Plus 前端: Vue2, Element-UI, Axios, Echarts, Vue-Router 核心功能: 该办公管理系统是一个综合性的企业内部管理平台,旨在提升企业运营效率和员工管理水…...
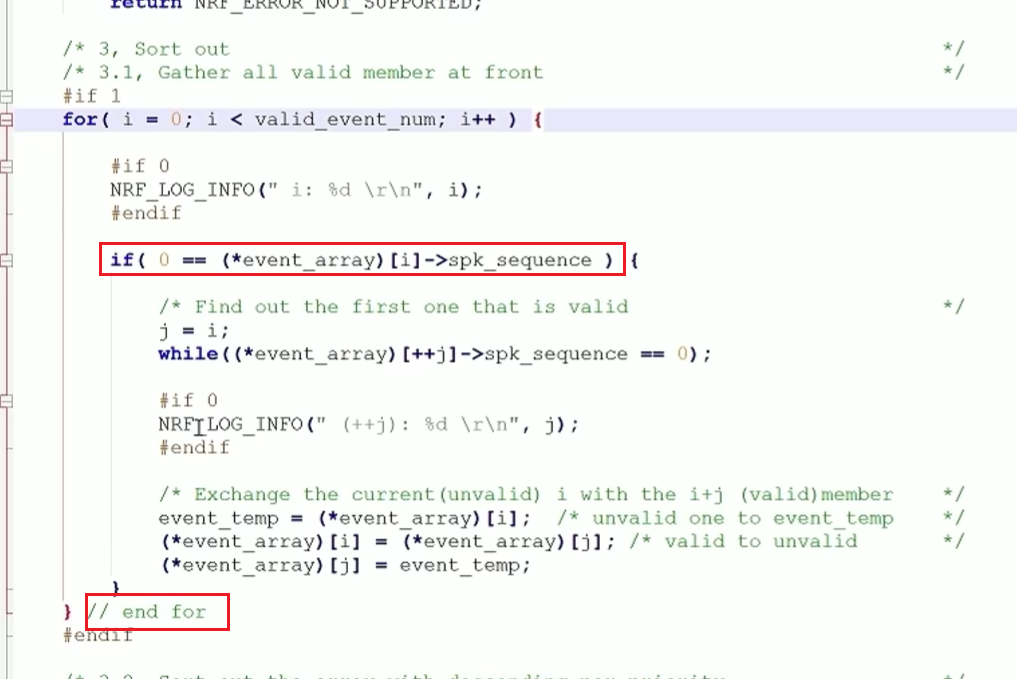
代码规范和架构【立芯理论一】(2025.06.08)
1、代码规范的目标 代码简洁精炼、美观,可持续性好高效率高复用,可移植性好高内聚,低耦合没有冗余规范性,代码有规可循,可以看出自己当时的思考过程特殊排版,特殊语法,特殊指令,必须…...