学习Rust的第三天:猜谜游戏
基于Steve Klabnik的《The Rust Programming Language》一书。今天我们在rust中建立一个猜谜游戏。
Introduction 介绍
We will build a game that will pick a random number between 1 to 100 and the user has to guess the number on a correct guess the user wins.
我们将构建一个游戏,它将选择1到100之间的随机数字,用户必须猜测正确的猜测用户获胜的数字。
Algorithm 算法
This is what the pseudo code would look like
这就是伪代码的样子
secret_number = (Generate a random number)
loop {Write “Please input your guess”Read guessWrite "Your guess : ${guess}"if(guess > secret_number){Write "Too high!"}else if(guess < secret_number){Write "Too low!"}else if(guess==number){Write "You win"break}
}
Step 1 : Set up a new project
步骤1:创建一个新项目
Use the cargo new
command to set up a project
使用 cargo new
命令设置项目
$ cargo new guessing_game
$ cd guessing_game
Step 2 : Declaring variables and reading user input
步骤2:声明变量并阅读用户输入
Filename : main.rs Film:main.rs
use std::io;fn main() {println!("Guess the number");println!("Please input your guess");let mut guess = String::new();io::stdin().read_line(&mut guess).expect("Failed to read line");println!("Your guess: {}", guess);
}
Let’s break the code down line by line :
让我们逐行分解代码:
use std::io;
This line brings thestd::io
(standard input/output) library into scope. Thestd::io
library provides a number of useful features for handling input/output.use std::io;
此行将std::io
(标准输入/输出)库带入范围。std::io
库提供了许多用于处理输入/输出的有用特性。fn main(){...}
This is the main function where the program execution begins.fn main(){...}
这是程序开始执行的main函数。println!("Guess the number");
println!
is a macro that prints the text to the console.println!("Guess the number");
println!
是一个宏,它将文本打印到控制台。println!("Please input your guess");
This line prompts the user to enter their guess.println!("Please input your guess");
这一行提示用户输入他们的猜测。let mut guess = String::new();
This line declares a mutable variableguess
and initializes it to an empty string.*mut
means the variable is mutable*, i.e., its value can be changed.String::new()
creates a new, empty string.let mut guess = String::new();
这一行声明了一个可变变量guess
,并将其转换为空字符串。*mut
表示变量是可变的 *,即,其值可以改变。String::new()
创建一个新的空字符串。io::stdin().read_line(&mut guess).expect("Failed to read line");
This line reads the user input from the standard input (keyboard). The entered input is put into theguess
string. If this process fails, the program will stop execution and display the message "Failed to read line".io::stdin().read_line(&mut guess).expect("Failed to read line");
这一行从标准输入(键盘)读取用户输入。输入的输入被放入guess
字符串中。如果此过程失败,程序将停止执行,并显示消息“读取行失败”。println!("Your guess : {guess}");
This line prints out the string "Your guess: ", followed by whatever the user inputted.println!("Your guess : {guess}");
这一行打印出字符串“Your guess:“,后跟用户输入的任何内容。
Step 3 : Generating a random number
步骤3:生成随机数
The number should be different each time for replayability. Rust’s standard library doesn’t include random number functionality, but the Rust team provides a rand
crate for this purpose.
为了可重玩性,每次的数字应该不同。Rust的标准库不包含随机数功能,但Rust团队为此提供了一个 rand
crate。
A Rust crate is like a neatly packaged box of code that you can easily use and share with others in the Rust programming language.
Rust crate就像一个整齐打包的代码盒,您可以轻松使用Rust编程语言并与其他人共享。
To use the rand
crate :
使用 rand
crate:
Filename: Cargo.toml Filtrate:Cargo.toml
[package]
name = "guessing_game"
version = "0.1.0"
edition = "2021"[dependencies]
rand = "0.8.5" #append this line
Understanding the Cargo.toml
file :
了解 Cargo.toml
文件:
- [package] [包]
name = "guessing_game"
: The name of the Rust package (or crate) is set to "guessing_game".name = "guessing_game"
:Rust包(或crate)的名称设置为“guessing_game”。version = "0.1.0"
: The version of the crate is specified as "0.1.0".version = "0.1.0"
:机箱的版本指定为“0.1.0”。edition = "2021"
: Specifies the Rust edition to use (in this case, the 2021 edition).edition = "2021"
:指定要使用的Rust版本(在本例中为2021版)。
2. [dependencies] 2. [依赖关系]
rand = "0.8.5"
: Adds a dependency on the "rand" crate with version "0.8.5". This means the "guessing_game" crate relies on the functionality provided by the "rand" crate, and version 0.8.5 specifically.rand = "0.8.5"
:在版本为“0.8.5”的“兰德”crate上添加依赖项。这意味着“guessing_game”crate依赖于“兰德”crate提供的功能,特别是0.8.5版本。
In simpler terms, this configuration file (Cargo.toml
) is like a recipe for your Rust project, specifying its name, version, Rust edition, and any external dependencies it needs (in this case, the "rand" crate).
简单地说,这个配置文件( Cargo.toml
)就像是Rust项目的配方,指定了它的名称、版本、Rust版本以及它需要的任何外部依赖(在本例中是“兰德”crate)。
After this without changing any code run cargo build
, Why do we do that?
在此之后,不改变任何代码运行 cargo build
,为什么我们这样做?
- Fetch Dependencies:
cargo build
fetches and updates the project's dependencies specified inCargo.toml
.
获取依赖项:cargo build
获取并更新Cargo.toml
中指定的项目依赖项。 - Dependency Resolution: It resolves and ensures the correct versions of dependencies are installed.
依赖项解析:它解析并确保安装了正确版本的依赖项。 - Build Process: Compiles Rust code and dependencies into executable artifacts or libraries.
构建过程:将Rust代码和依赖项编译为可执行工件或库。 - Check for Errors: Identifies and reports compilation errors, ensuring code consistency.
检查错误:检查并报告编译错误,确保代码一致性。 - Update lock file: Updates the
Cargo.lock
file to record the exact dependency versions for reproducibility.
更新锁定文件:更新Cargo.lock
文件以记录精确的依赖性版本,以实现再现性。
Now let’s talk code 现在我们来谈谈代码
use std::io;
use rand::Rng;fn main() {println!("Guess the number!");let secret_number = rand::thread_rng().gen_range(1..=100);println!("Secret number: {}", secret_number);println!("Please input your guess");let mut guess = String::new();io::stdin().read_line(&mut guess).expect("Failed to read line");println!("Your guess: {}", guess);
}
Running the program : 运行程序:
$ cargo run
Guess the number!
Secret number : 69
Please input your guess
32
Your guess : 32
Let’s see what we did here :
让我们看看我们在这里做了什么:
use rand::Rng;
: This line is an import statement that brings theRng
trait into scope, allowing you to use its methods.use rand::Rng;
:这一行是一个import语句,它将Rng
trait带入作用域,允许你使用它的方法。rand::thread_rng()
: This part initializes a random number generator specific to the current thread. Therand::thread_rng()
function returns a type that implements theRng
trait.rand::thread_rng()
:这部分提供了一个特定于当前线程的随机数生成器。rand::thread_rng()
函数返回一个实现了Rng
trait的类型。.gen_range(1..=100)
: Using the random number generator (Rng
trait), this code calls thegen_range
method to generate a random number within a specified range. The range is defined as1..=100
, meaning the generated number should be between 1 and 100 (inclusive)..gen_range(1..=100)
:使用随机数生成器(Rng
trait),这段代码调用gen_range
方法来生成指定范围内的随机数。范围被定义为1..=100
,这意味着生成的数字应该在1和100之间(包括1和100)。
Step 4 : Comparing the guess to the user input
步骤4:将猜测与用户输入进行比较
Now that we have the input, the program compares the user’s guess to the secret number to determine if they guessed correctly. If the guess matches the secret number, the user is successful; otherwise, the program evaluates whether the guess is too high or too low.
现在我们有了输入,程序将用户的猜测与秘密数字进行比较,以确定他们是否猜对了。如果猜测与秘密数字匹配,则用户成功;否则,程序评估猜测是否过高或过低。
Let’s take a look at the code and then break it down :
让我们看看代码,然后分解它:
use std::io;
use std::cmp::Ordering;
use rand::Rng;fn main() {println!("Guess the number!");let secret_number = rand::thread_rng().gen_range(1..=100);println!("Secret number: {}", secret_number);println!("Please input your guess");let mut guess = String::new();io::stdin().read_line(&mut guess).expect("Failed to read line");let guess: u32 = guess.trim().parse().expect("Please type a number!");println!("Your guess: {}", guess);match guess.cmp(&secret_number) {Ordering::Less => println!("Too small!"),Ordering::Greater => println!("Too big!"),Ordering::Equal => println!("You win!"),}
}
Running the program : 运行程序:
$ cargo run
Guess the number!
Secret number : 48
Please input your guess
98
Your guess : 98
Too big!
Explanation : 说明:
let guess: u32 = guess.trim().parse().expect("Please type a number!");
: Shadowing the variableguess
, it parses the string into an unsigned 32-bit integer. If parsing fails, it prints an error message.let guess: u32 = guess.trim().parse().expect("Please type a number!");
:隐藏变量guess
,将字符串解析为无符号32位整数。如果解析失败,它将打印一条错误消息。match guess.cmp(&secret_number) { ... }
: Compares the user's guess to the secret number using amatch
statement, handling three cases: less than, greater than, or equal to the secret number.
第0号:使用match
语句将用户的猜测与秘密数字进行比较,处理三种情况:小于、大于或等于秘密数字。
Shadowing: 阴影:
- Shadowing is when a new variable is declared with the same name as an existing variable, effectively “shadowing” the previous one.
隐藏是当一个新变量与现有变量同名时,有效地“隐藏”前一个变量。 - In this code,
let guess: u32 = ...
is an example of shadowing. The secondguess
shadows the first one, changing its type fromString
tou32
. Shadowing is often used to reassign a variable with a new value and type while keeping the same name.
在这段代码中,let guess: u32 = ...
是阴影的一个例子。第二个guess
隐藏第一个,将其类型从String
更改为u32
。隐藏通常用于为变量重新分配新的值和类型,同时保持名称不变。
Step 5 : Looping the guesses till the user wins
第5步:循环猜测,直到用户获胜
In Step 5, the program implements a loop structure to repeatedly prompt the user for guesses until they correctly guess the secret number as we saw in the algorithm
在步骤5中,程序实现了一个循环结构,反复提示用户进行猜测,直到他们正确地猜出密码,就像我们在算法中看到的那样
As always code then explanation :
一如既往的代码然后解释:
use std::io;
use std::cmp::Ordering;
use rand::Rng;fn main() {println!("Guess the number!");let secret_number = rand::thread_rng().gen_range(1..=100);println!("Secret number: {}", secret_number);loop {println!("Please input your guess");let mut guess = String::new();io::stdin().read_line(&mut guess).expect("Failed to read line");let guess: u32 = guess.trim().parse().expect("Please type a number!");println!("Your guess: {}", guess);match guess.cmp(&secret_number) {Ordering::Less => println!("Too small!"),Ordering::Greater => println!("Too big!"),Ordering::Equal => {println!("You win!");break;},}}
}
Running the program: 运行程序:
$ cargo run
Guess the number!
Secret number : 23
Please input your guess
4
Your guess : 4
Too small!
Please input your guess
76
Your guess : 76
Too big!
Please input your guess
23
Your guess : 4
You win!
Explanation: 说明:
loop{...}
statement is used to create an infinite looploop{...}
语句用于创建无限循环- We are using the
break
statement to exit out of the program when the variablesguess
andsecret_number
are the same.
当变量guess
和secret_number
相同时,我们使用break
语句退出程序。
Step 6 : Handling invalid input
步骤6:处理无效输入
In Step 6, we want to handle invalid inputs and errors because of them. For example : entering a string in the prompt
在第6步中,我们要处理无效输入和由此产生的错误。例如:在提示符中输入字符串
use std::io;
use std::cmp::Ordering;
use rand::Rng;fn main() {println!("Guess the number!");let secret_number = rand::thread_rng().gen_range(1..=100);println!("Secret number: {}", secret_number);loop {println!("Please input your guess");let mut guess = String::new();io::stdin().read_line(&mut guess).expect("Failed to read line");let guess: u32 = match guess.trim().parse() {Ok(num) => num,Err(_) => continue,};println!("Your guess: {}", guess);match guess.cmp(&secret_number) {Ordering::Less => println!("Too small!"),Ordering::Greater => println!("Too big!"),Ordering::Equal => {println!("You win!");break;},}}
}
Running the program: 运行程序:
$ cargo run
Guess the number!
Secret number : 98
Please input your guess
meow
Please input your guess
43
Your guess : 43
Too small!
Parse User Input: 解析用户输入:
- Attempts to parse the string in
guess
into an unsigned 32-bit integer.
尝试将guess
中的字符串解析为无符号32位整数。 - Uses the
trim
method to remove leading and trailing whitespaces from the user's input.
使用trim
方法从用户输入中删除前导和尾随空格。
Match Statement: 匹配声明:
- The
match
statement checks the result of the parsing operation.match
语句检查解析操作的结果。 Ok(num) => num
: If parsing is successful, assigns the parsed number to the variableguess
.Ok(num) => num
:如果解析成功,将解析后的数字赋给变量guess
。
Error Handling: 错误处理:
Err(_) => continue
: If an error occurs during parsing, the placeholder '_' matches any error, and the code inside the loop continues, prompting the user for input again.
第0号:如果在解析过程中出现错误,占位符'_'将匹配任何错误,循环中的代码将继续执行,提示用户再次输入。
Summary 总结
In this article, we embarked on our third day of learning Rust by building a guessing game. Here’s a summary of the key steps and concepts covered:Introduction
在本文中,我们通过构建一个猜谜游戏开始了学习Rust的第三天。以下是所涵盖的关键步骤和概念的摘要:简介
- The goal is to create a game where the user guesses a randomly generated number between 1 and 100.
我们的目标是创建一个游戏,让用户猜测1到100之间随机生成的数字。
Algorithm 算法
- A generic algorithm was outlined, providing a high-level overview of the game’s logic.
概述了一个通用算法,提供了游戏逻辑的高级概述。
Step 1: Set up a new project
步骤1:创建一个新项目
- Used
cargo new
to create a new Rust project named "guessing_game."
使用cargo new
创建一个名为“guessing_game”的新Rust项目。“
Step 2: Declaring variables and reading user input
步骤2:声明变量并阅读用户输入
- Introduced basic input/output functionality using
std::io
.
使用std::io
引入基本输入/输出功能。 - Demonstrated reading user input, initializing variables, and printing messages.
演示了阅读用户输入、初始化变量和打印消息。
Step 3: Generating a random number
步骤3:生成随机数
- Added the
rand
crate as a dependency to generate random numbers.
添加了rand
crate作为依赖项来生成随机数。 - Used
rand::thread_rng().gen_range(1..=100)
to generate a random number between 1 and 100.
使用rand::thread_rng().gen_range(1..=100)
生成1到100之间的随机数。
Step 4: Comparing the guess to the user input
步骤4:将猜测与用户输入进行比较
- Introduced variable shadowing and compared user input to the secret number.
引入了变量跟踪,并将用户输入与秘密数字进行比较。 - Used a
match
statement to handle different comparison outcomes.
使用match
语句处理不同的比较结果。
Step 5: Looping the guesses till the user wins
第5步:循环猜测,直到用户获胜
- Implemented a loop structure to allow the user to make repeated guesses until they correctly guess the secret number.
实现了一个循环结构,允许用户重复猜测,直到他们正确地猜测秘密数字。
Step 6: Handling invalid input
步骤6:处理无效输入
- Addressed potential errors by adding error handling to the user input parsing process.
通过向用户输入分析过程添加错误处理来解决潜在错误。 - Used the
continue
statement to skip the current iteration and prompt the user again in case of an error.
使用continue
语句跳过当前迭代,并在出现错误时再次提示用户。
Final code : 最终代码:
use std::io;
use std::cmp::Ordering;
use rand::Rng;fn main() {println!("Guess the number!");let secret_number = rand::thread_rng().gen_range(1..=100);loop {println!("Please input your guess");let mut guess = String::new();io::stdin().read_line(&mut guess).expect("Failed to read line");let guess: u32 = match guess.trim().parse() {Ok(num) => num,Err(_) => continue,};println!("Your guess: {}", guess);match guess.cmp(&secret_number) {Ordering::Less => println!("Too small!"),Ordering::Greater => println!("Too big!"),Ordering::Equal => {println!("You win!");break;},}}
}
相关文章:
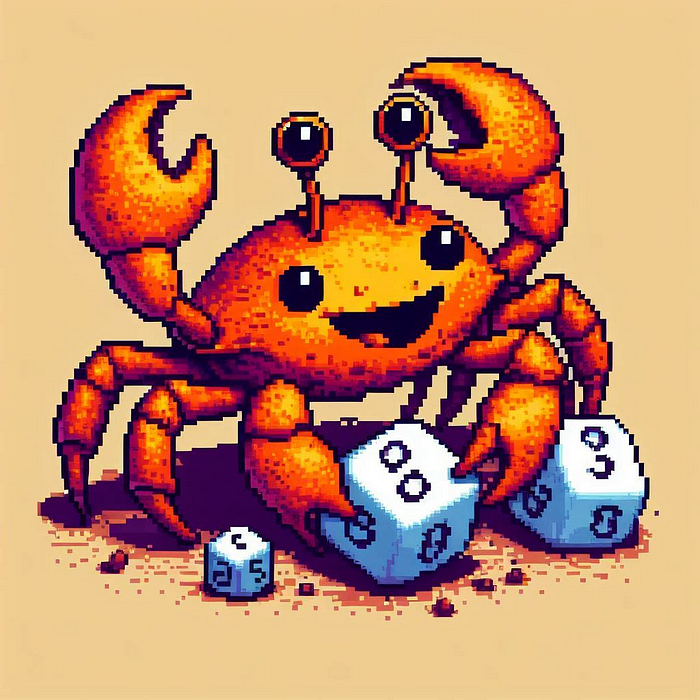
学习Rust的第三天:猜谜游戏
基于Steve Klabnik的《The Rust Programming Language》一书。今天我们在rust中建立一个猜谜游戏。 Introduction 介绍 We will build a game that will pick a random number between 1 to 100 and the user has to guess the number on a correct guess the user wins. 我们将…...
React中子传父的方式及原理
方式挺多的,先说最常用的通过props进行父子组件的数据传递和修改以及原理 在React中,props不仅用于传递数据,它们也可以传递可以执行的函数,这使得子组件能够间接更新父组件的状态。这种方法强化了React的单向数据流策略…...
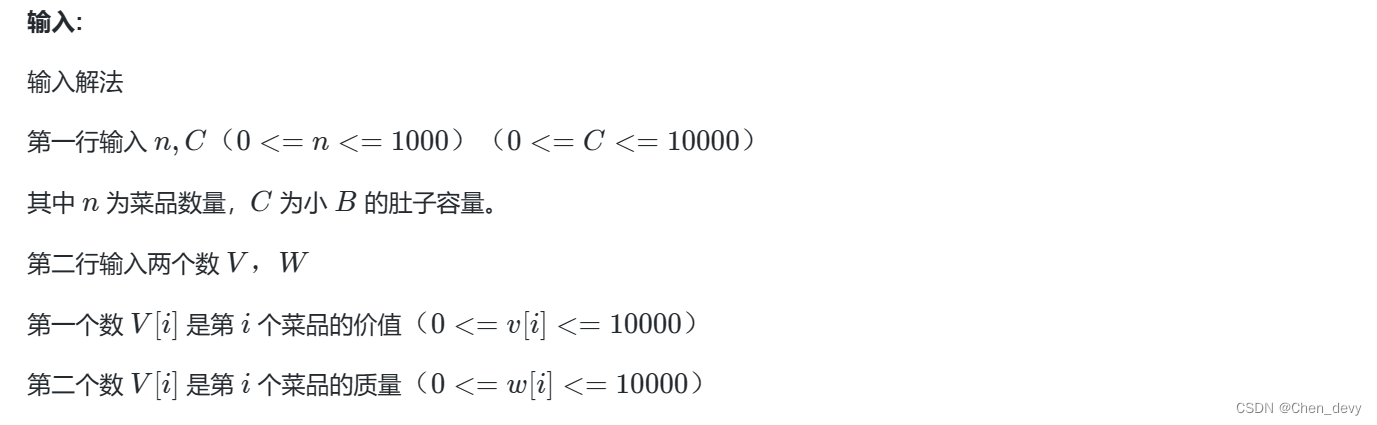
【数据结构与算法】贪心算法及例题
目录 贪心算法例题一:找零问题例题二:走廊搬运物品最优方案问题输入样例例题三:贪心自助餐 贪心算法 贪心算法是一种在每一步选择中都采取当前状态下最优的选择,以期望最终达到全局最优解的算法。它的核心思想是每次都选择当前最…...
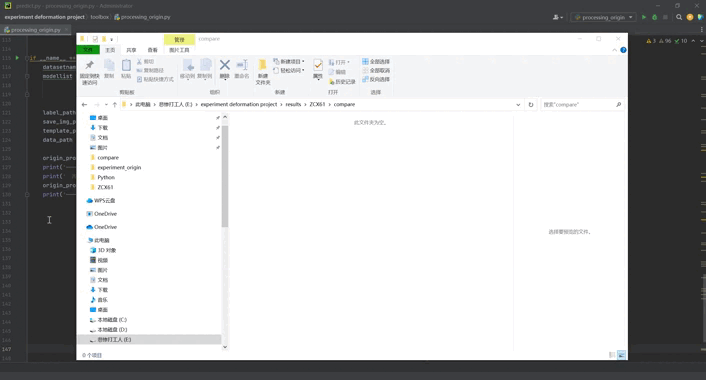
【Origin+Python】使用External Python批量出图代码参考
目录 基本介绍环境配置官方代码示例基础代码详解我的代码效果视频进阶代码及去水印 基本介绍 origin2021后可以使用python实现批量绘图,一共有两种方式:一种是嵌入式Python,一种是外部Python访问Origin。详细介绍可以自己去查看,打…...
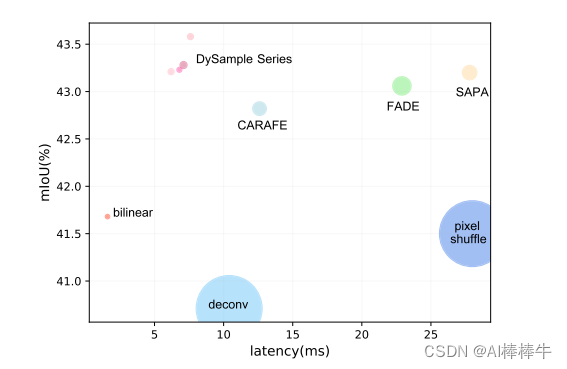
YOLOv8最新改进系列:融合DySample超轻量动态上采样算子,低延迟、高性能,目前最新上采样方法!!!遥遥领先!
YOLOv8最新改进系列:融合DySample超轻量动态上采样算子,低延迟、高性能,目前最新上采样方法!!!遥遥领先! DySample超轻量动态上采样算子全文戳这!here! 详细的改进教程以及源码&am…...
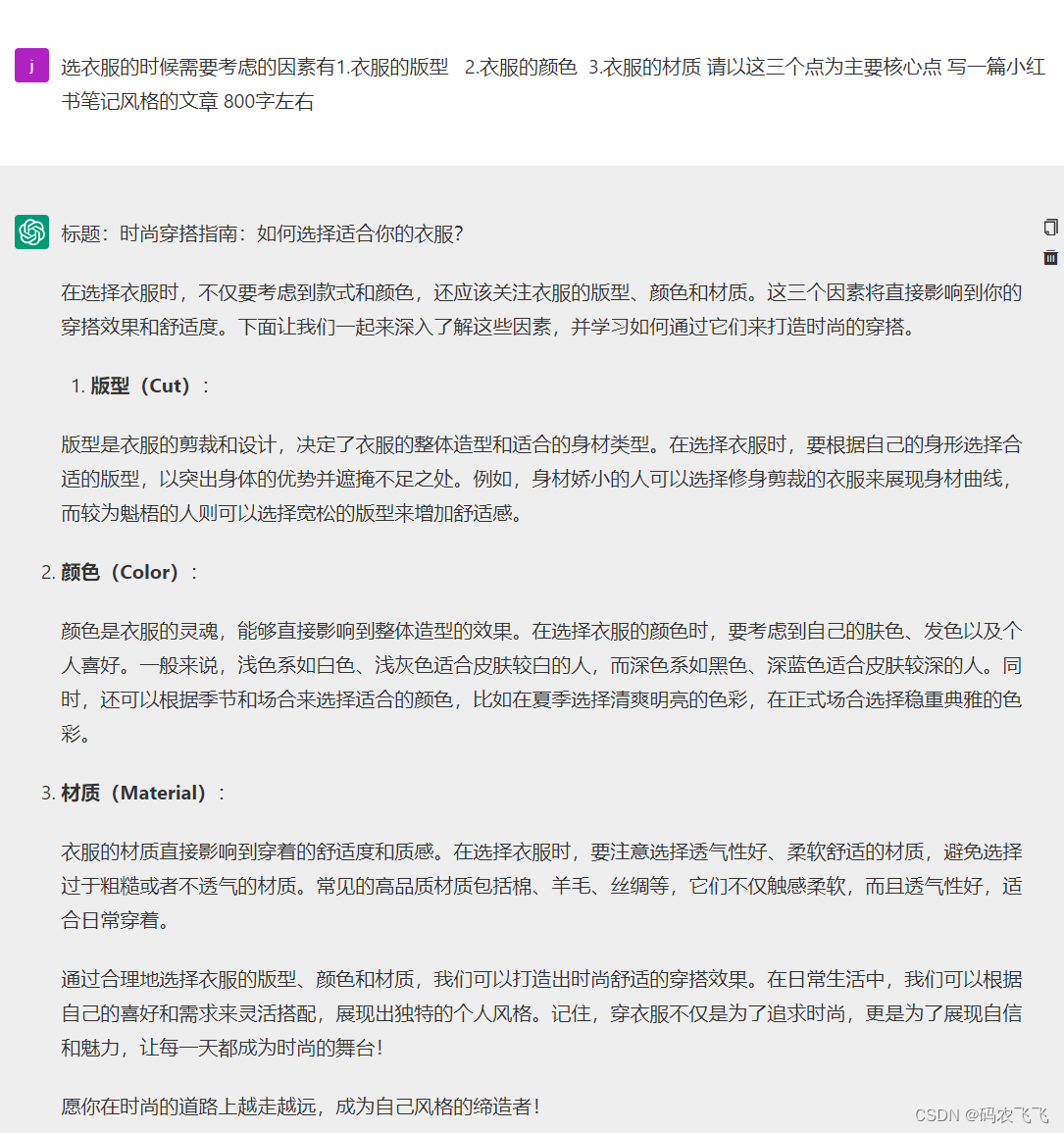
ChatGPT基础(二) ChatGPT的使用和调优
文章目录 ChatGPT的特性采用关键词进行提问给ChatGPT指定身份提升问答质量的策略1.表述方式上的优化2.用"继续"输出长内容3.营造场景4.由浅入深,提升问题质量5.预设回答框架和风格 ChatGPT的特性 1.能够联系上下文进行回答 ChatGPT回答问题是有上下文的&…...
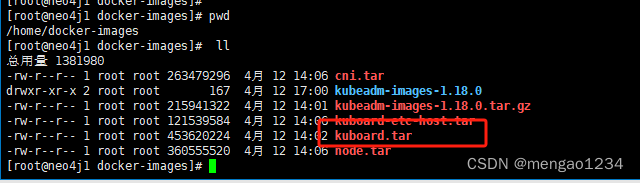
麒麟 V10 离线 安装 k8s 和kuboard
目录 安装文件准备 主机准备 主机配置 修改主机名(三个节点分别执行) 配置hosts(所有节点) 关闭防火墙、selinux、swap、dnsmasq(所有节点) 安装依赖包(所有节点) 系统参数设置(所有节点) 时间同步…...
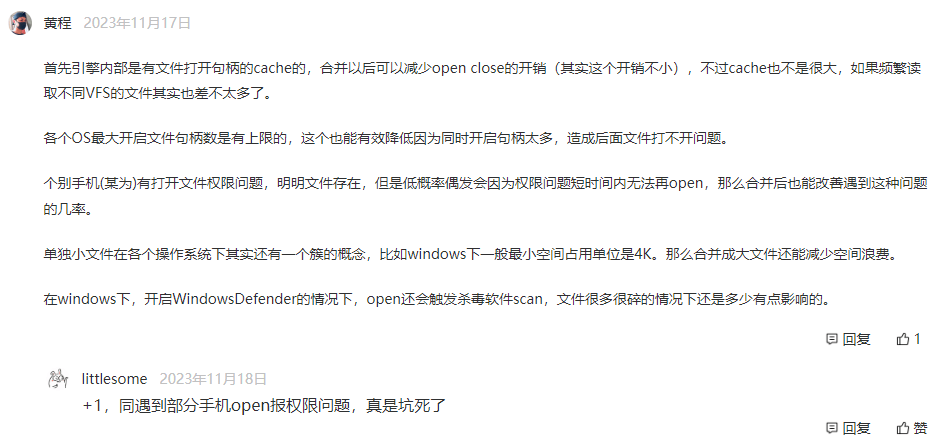
PlayerSettings.WebGL.emscriptenArgs设置无效的问题
1)PlayerSettings.WebGL.emscriptenArgs设置无效的问题 2)多个小资源包合并为大资源包的疑问 3)AssetBundle在移动设备上丢失 4)Unity云渲染插件RenderStreaming,如何实现多用户分别有独立的操作 这是第381篇UWA技术知…...
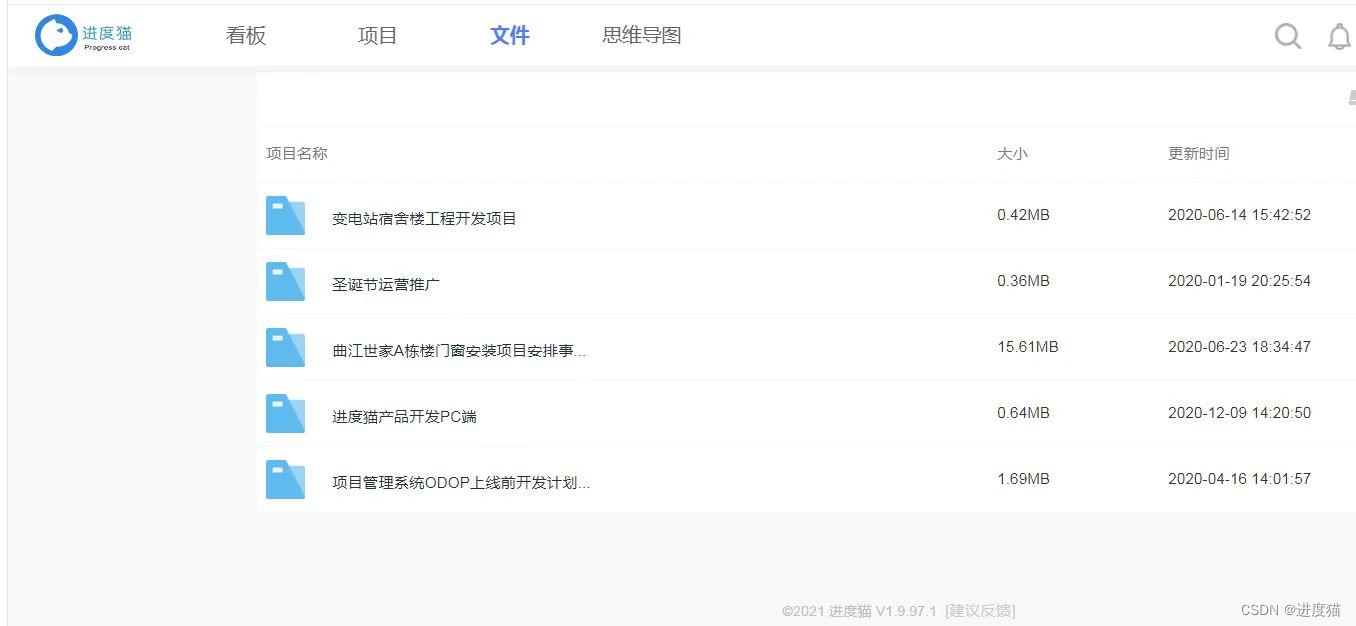
项目管理工具——使用甘特图制定项目计划的详细步骤
甘特图是一种直观的项目管理工具,它有助于我们清晰地展示任务安排、时间管理和项目的进度。以下是使用甘特图制定项目计划的详细步骤: 1、创建项目:首先,在进度猫中创建新的项目,并设置项目的时间、工作日等参数。根据…...

python读取文件数据写入到数据库中,并反向从数据库读取保存到本地
学python,操作数据库是必不可少的,不光要会写python代码,还要会写SQL语句,本篇文章主要讲如何把本地txt文件中的数据读取出来并写入到对应的数据库中,同时将数据库单个表中的数据读出来保存在本地txt文件中。 话不多说…...
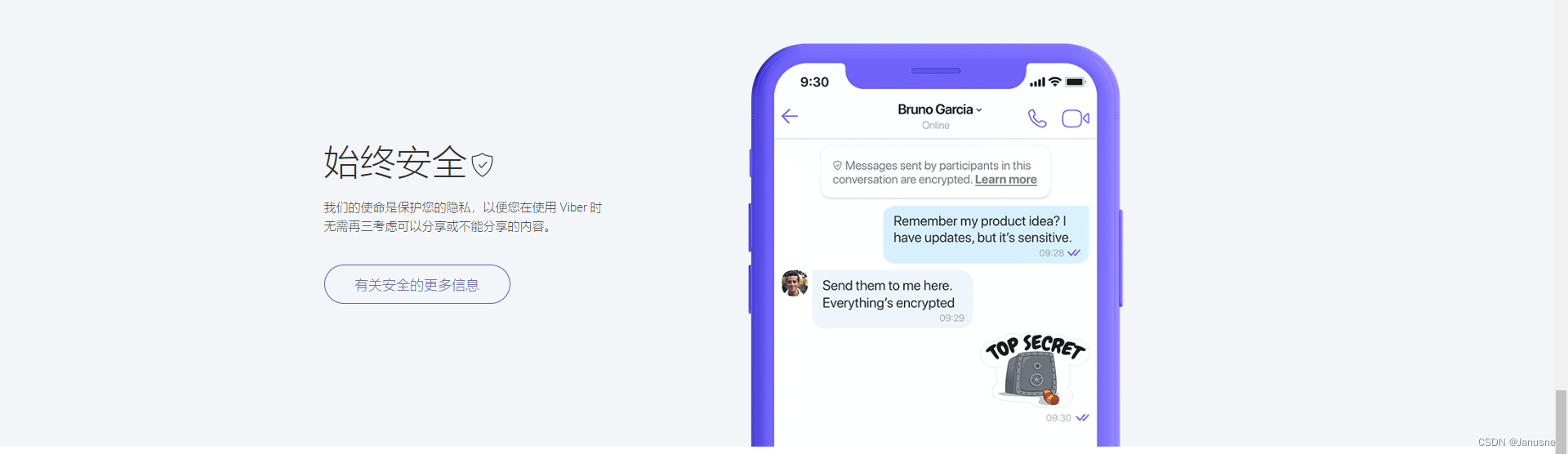
社交媒体数据恢复:Viber
Viber是一款流行的即时通讯应用,用于发送消息、语音通话和视频通话。然而,有时候我们会不小心删除一些重要的Viber聊天记录,这时候就需要进行数据恢复。本文将介绍如何在安卓设备上进行Viber数据恢复。 一、使用安卓数据恢复软件 安卓数据恢…...
蓝桥杯赛事介绍
蓝桥杯是由工业和信息化部人才交流中心主办的全国性IT学科赛事,全称为“蓝桥杯全国软件和信息技术专业人才大赛”。该赛事旨在推动软件和信息领域专业技术人才培养,提升大学生的创新能力和就业竞争力,为行业输送具有创新能力和实践能力的高端…...
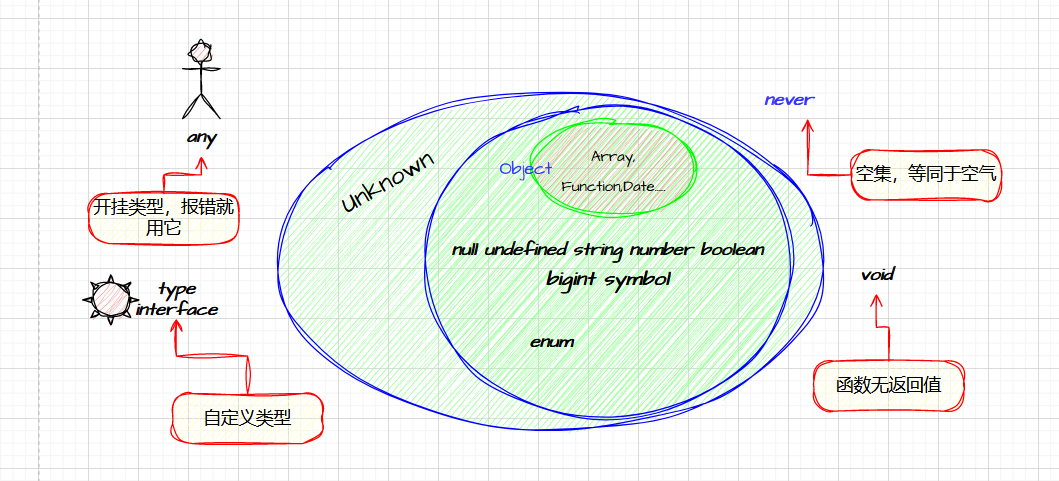
TypeScript系列之-深度理解基本类型画图讲解
JS的类型(8): null undefined string number boolean bigint symbol object(含 Array, Function,Date.....) TS的类型(87): 以上所有,加上 void, never, enum, unknown, any 再加上自定义类型 type interface 上一节我们说…...
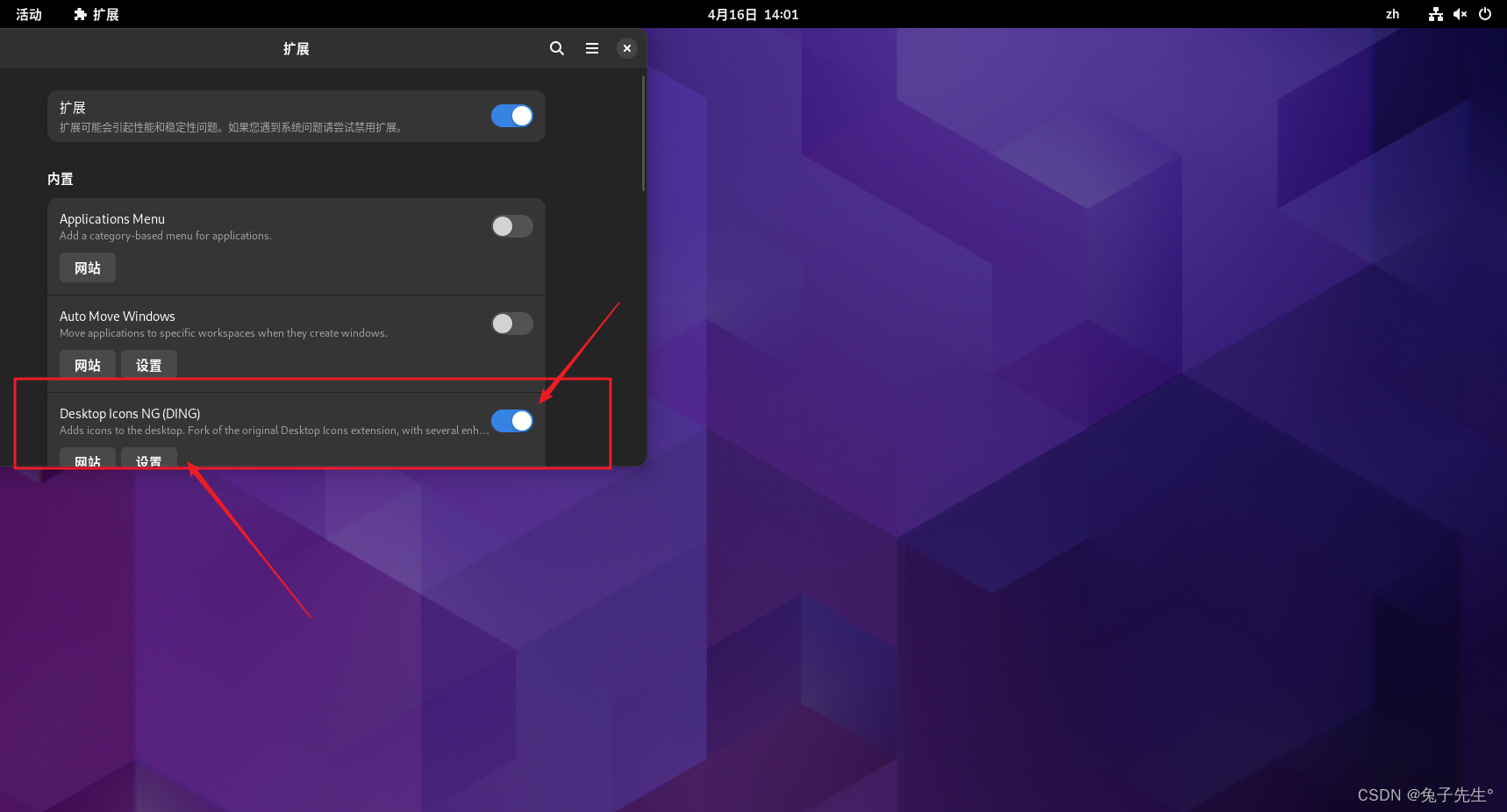
Debian
使用root用户操作 直接使用su命令进行切换。 配置用户使用sudo命令 在安装好系统之后,使用用户名登录之后。需要执行需要root权限的命令,会发现无法执行成功。原因是没有配置用户使用sudo的权限。 编辑bash /etc/sudoers文件 可以先切换root用户安装…...
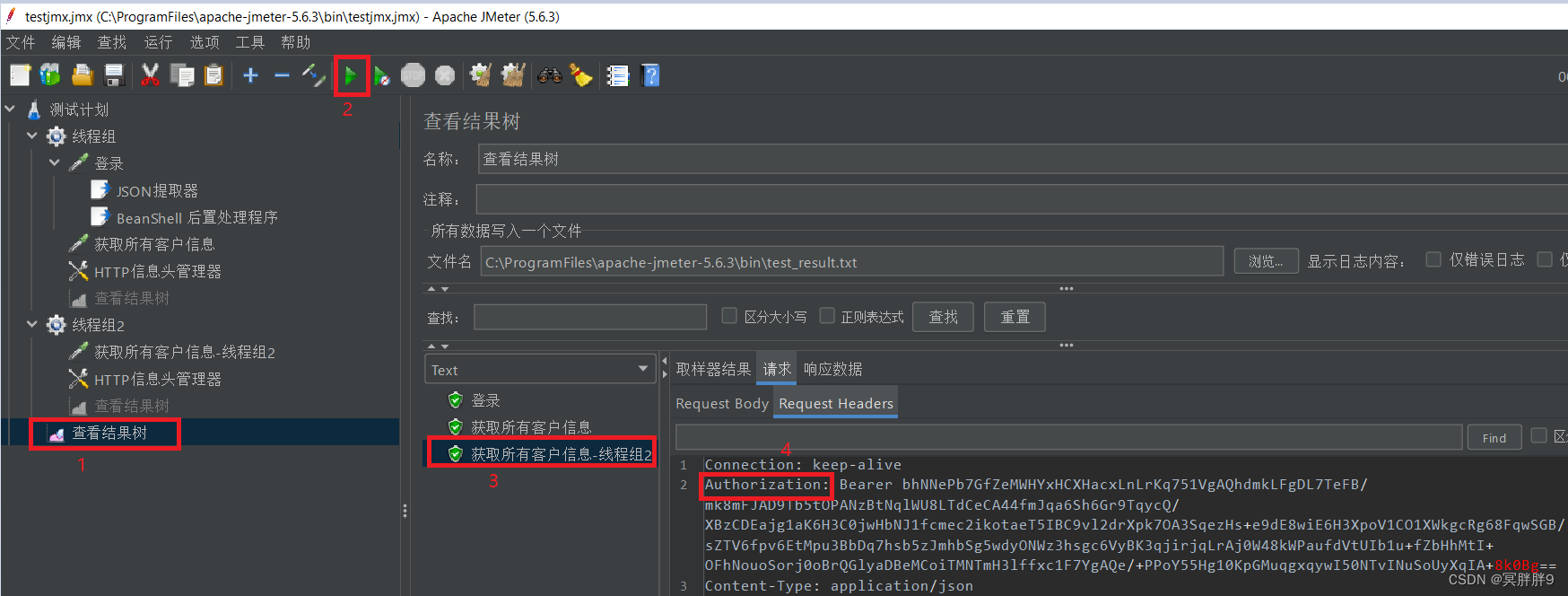
怎么使用JMeter进行性能测试?
一、简介 JMeter是Apache软件基金会下的一款开源的性能测试工具,完全由Java开发。它专注于对我们应用程序进行负载测试和性能测量,最初设计用于web应用程序,现在已经扩展到其他测试功能,比如:FTP、Database和LDAP等。…...
MySQL:锁的分类
文章目录 行级锁Record LockGap LockNext-Key Lock插入意向锁 表级锁表锁元数据锁(MDL)意向锁AUTO-INC 锁 全局锁 行级锁 Record Lock 记录锁有S锁(共享锁/读锁)和X锁(排他锁/写锁)之分,加完S…...
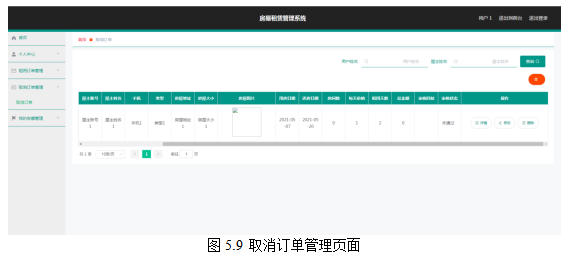
基于springboot实现房屋租赁管理系统设计项目【项目源码+论文说明】
基于springboot实现房屋租赁管理系统设计演示 摘要 互联网发展至今,无论是其理论还是技术都已经成熟,而且它广泛参与在社会中的方方面面。它让信息都可以通过网络传播,搭配信息管理工具可以很好地为人们提供服务。针对房屋租赁信息管理混乱&…...
揭秘Redis底层:一窥数据结构的奥秘与魅力
一、引言 Redis,以其高性能、高可靠、丰富的数据结构等特点,成为现代应用程序中不可或缺的缓存与存储组件。然而,Redis之所以能够实现如此卓越的性能,离不开其底层精巧的数据结构设计。本文将深入浅出地解析Redis底层五大核心数据…...
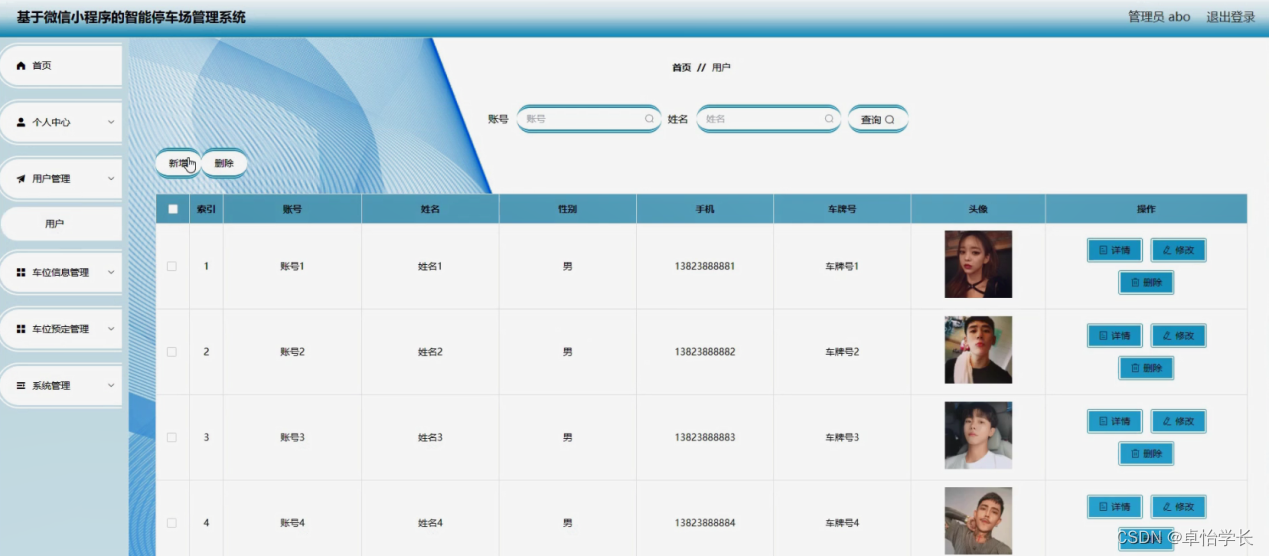
【网站项目】智能停车场管理系统小程序
🙊作者简介:拥有多年开发工作经验,分享技术代码帮助学生学习,独立完成自己的项目或者毕业设计。 代码可以私聊博主获取。🌹赠送计算机毕业设计600个选题excel文件,帮助大学选题。赠送开题报告模板ÿ…...
芒果YOLOv5改进94:检测头篇DynamicHead为目标检测统一检测头:即插即用|DynamicHead检测头,尺度感知、空间感知、任务感知
该专栏完整目录链接: 芒果YOLOv5深度改进教程 该创新点:在原始的Dynamic Head的基础上,对核心部位进行了二次的改进,在 原论文 《尺度感知、空间感知、任务感知》 的基础上,在 通道感知 的层级上进行了增强,关注每个像素点的比重。 在自己的数据集上改进,有效涨点就可以…...
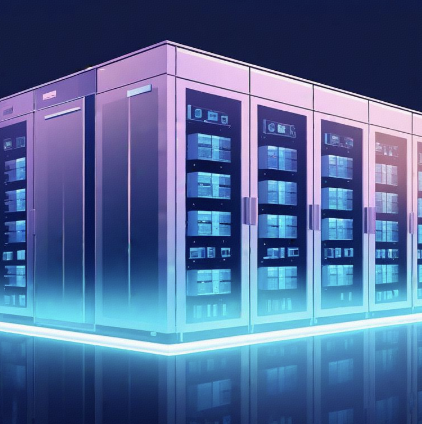
华为云AI开发平台ModelArts
华为云ModelArts:重塑AI开发流程的“智能引擎”与“创新加速器”! 在人工智能浪潮席卷全球的2025年,企业拥抱AI的意愿空前高涨,但技术门槛高、流程复杂、资源投入巨大的现实,却让许多创新构想止步于实验室。数据科学家…...
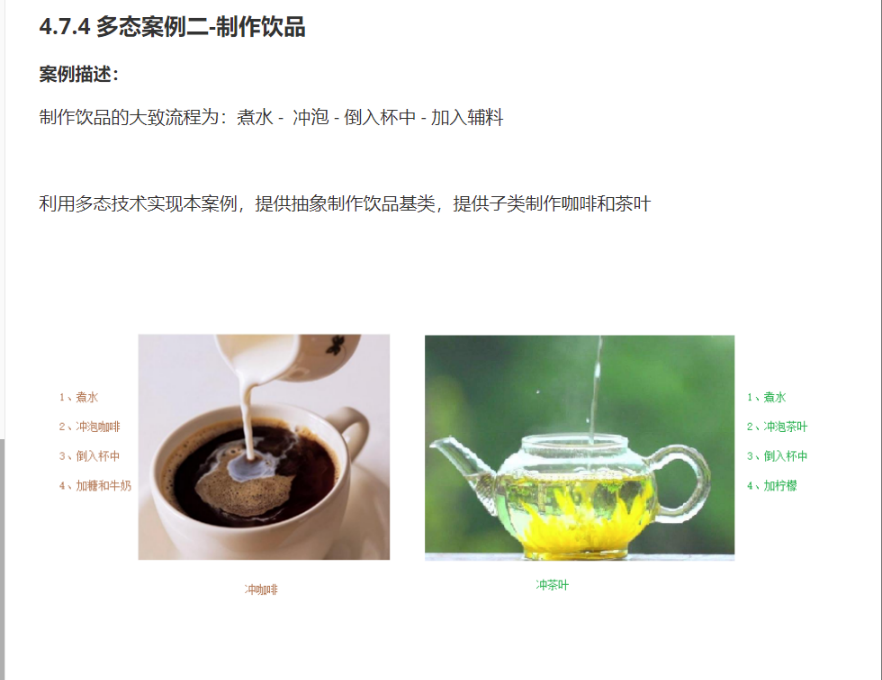
C++_核心编程_多态案例二-制作饮品
#include <iostream> #include <string> using namespace std;/*制作饮品的大致流程为:煮水 - 冲泡 - 倒入杯中 - 加入辅料 利用多态技术实现本案例,提供抽象制作饮品基类,提供子类制作咖啡和茶叶*//*基类*/ class AbstractDr…...

CTF show Web 红包题第六弹
提示 1.不是SQL注入 2.需要找关键源码 思路 进入页面发现是一个登录框,很难让人不联想到SQL注入,但提示都说了不是SQL注入,所以就不往这方面想了 先查看一下网页源码,发现一段JavaScript代码,有一个关键类ctfs…...
模型参数、模型存储精度、参数与显存
模型参数量衡量单位 M:百万(Million) B:十亿(Billion) 1 B 1000 M 1B 1000M 1B1000M 参数存储精度 模型参数是固定的,但是一个参数所表示多少字节不一定,需要看这个参数以什么…...
Neo4j 集群管理:原理、技术与最佳实践深度解析
Neo4j 的集群技术是其企业级高可用性、可扩展性和容错能力的核心。通过深入分析官方文档,本文将系统阐述其集群管理的核心原理、关键技术、实用技巧和行业最佳实践。 Neo4j 的 Causal Clustering 架构提供了一个强大而灵活的基石,用于构建高可用、可扩展且一致的图数据库服务…...
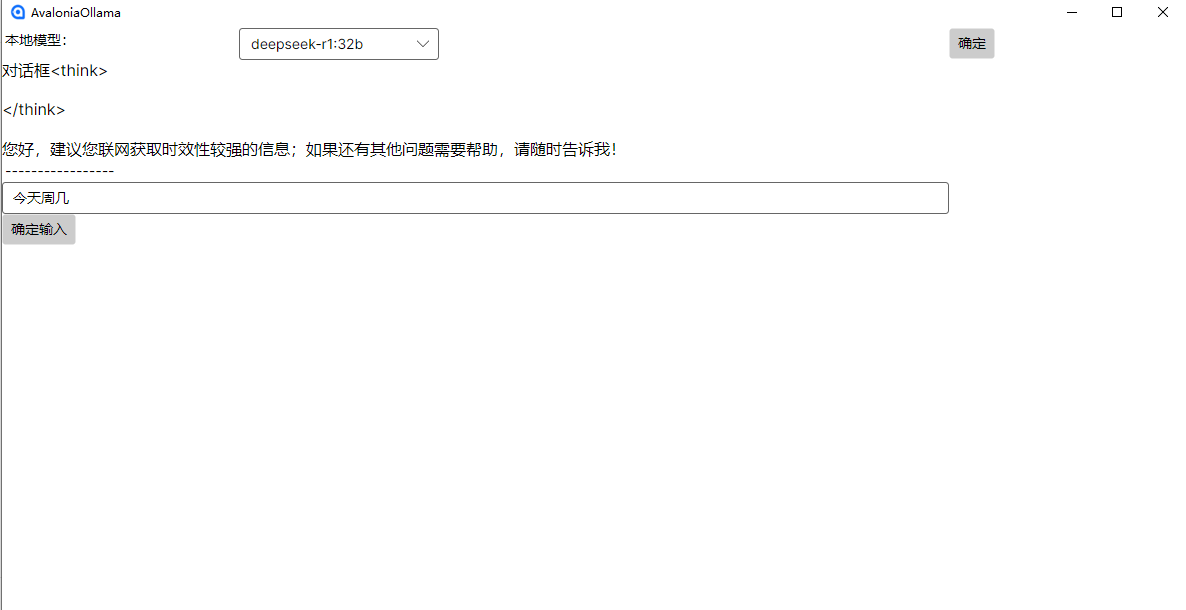
c#开发AI模型对话
AI模型 前面已经介绍了一般AI模型本地部署,直接调用现成的模型数据。这里主要讲述讲接口集成到我们自己的程序中使用方式。 微软提供了ML.NET来开发和使用AI模型,但是目前国内可能使用不多,至少实践例子很少看见。开发训练模型就不介绍了&am…...
Swagger和OpenApi的前世今生
Swagger与OpenAPI的关系演进是API标准化进程中的重要篇章,二者共同塑造了现代RESTful API的开发范式。 本期就扒一扒其技术演进的关键节点与核心逻辑: 🔄 一、起源与初创期:Swagger的诞生(2010-2014) 核心…...
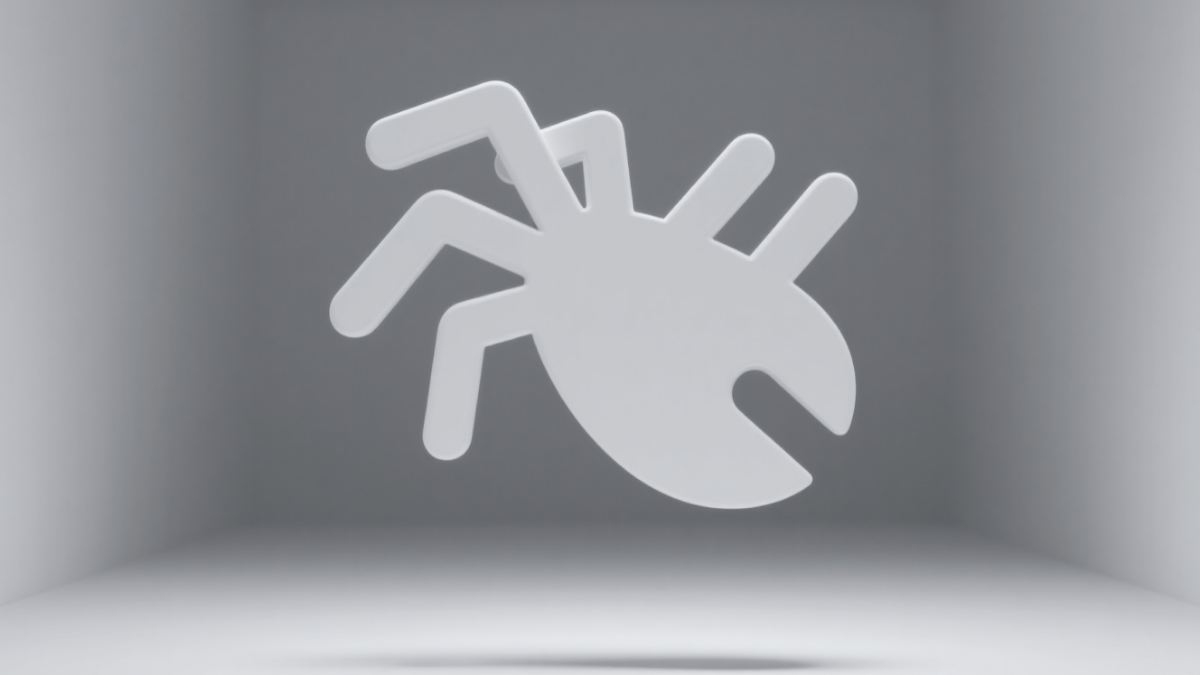
智能分布式爬虫的数据处理流水线优化:基于深度强化学习的数据质量控制
在数字化浪潮席卷全球的今天,数据已成为企业和研究机构的核心资产。智能分布式爬虫作为高效的数据采集工具,在大规模数据获取中发挥着关键作用。然而,传统的数据处理流水线在面对复杂多变的网络环境和海量异构数据时,常出现数据质…...
Python 包管理器 uv 介绍
Python 包管理器 uv 全面介绍 uv 是由 Astral(热门工具 Ruff 的开发者)推出的下一代高性能 Python 包管理器和构建工具,用 Rust 编写。它旨在解决传统工具(如 pip、virtualenv、pip-tools)的性能瓶颈,同时…...
Python+ZeroMQ实战:智能车辆状态监控与模拟模式自动切换
目录 关键点 技术实现1 技术实现2 摘要: 本文将介绍如何利用Python和ZeroMQ消息队列构建一个智能车辆状态监控系统。系统能够根据时间策略自动切换驾驶模式(自动驾驶、人工驾驶、远程驾驶、主动安全),并通过实时消息推送更新车…...