leetcode刷题记录:hot100强化训练2:二叉树+图论
二叉树
36. 二叉树的中序遍历
递归就不写了,写一下迭代法
class Solution(object):def inorderTraversal(self, root):""":type root: TreeNode:rtype: List[int]"""if not root:return res = []cur = rootstack = []while cur or stack:if cur:stack.append(cur)cur = cur.left else:cur = stack.pop()res.append(cur.val)cur = cur.rightreturn res
顺便再写一下前序和后序的迭代法
前序:
class Solution(object):def preorderTraversal(self, root):""":type root: TreeNode:rtype: List[int]"""if not root:returnst = [root]res = []# cur = while st:cur = st.pop()res.append(cur.val) if cur.right:st.append(cur.right)if cur.left:st.append(cur.left)return res
后序:
class Solution(object):def postorderTraversal(self, root):""":type root: TreeNode:rtype: List[int]"""if not root:returncur = rootst = []prev = Noneres = []while st or cur:if cur:st.append(cur)cur = cur.leftelse:cur = st.pop()#现在需要确定的是是否有右子树,或者右子树是否访问过#如果没有右子树,或者右子树访问完了,也就是上一个访问的节点是右子节点时#说明可以访问当前节点if not cur.right or cur.right == prev:res.append(cur.val)prev = curcur = Noneelse:st.append(cur)cur = cur.right return res
37. 二叉树的最大深度
分解很简单,直接交给递归函数。但是遍历的思路都要会
- 分解(动态规划思路)
class Solution(object):def maxDepth(self, root):""":type root: TreeNode:rtype: int"""if not root:return 0return max(self.maxDepth(root.left), self.maxDepth(root.right)) + 1
- 遍历(回溯思路)
走完之后depth-1
class Solution(object):def maxDepth(self, root):""":type root: TreeNode:rtype: int"""def helper(root):if not root:self.res = max(self.res, self.depth)returnself.depth += 1helper(root.left) helper(root.right) self.depth -= 1self.depth = 0self.res = 0helper(root)return self.res
- 延伸:可否不用递归来做这道题?
这个解法是chatgpt写的,将depth和node一起压入栈,避免对depth +1, -1的操作
class Solution(object):def maxDepth(self, root):""":type root: TreeNode:rtype: int"""if not root:return 0stack = [(root, 1)]max_depth = 0while stack:node, depth = stack.pop()if node:max_depth = max(max_depth, depth)if node.right:stack.append((node.right, depth + 1))if node.left:stack.append((node.left, depth + 1)) return max_depth
- 反转二叉树
递归函数的定义:给定二叉树根节点,反转它的左右子树。
后续位置递归
class Solution(object):def invertTree(self, root):""":type root: TreeNode:rtype: TreeNode"""if not root:returnself.invertTree(root.left) self.invertTree(root.right) root.left, root.right = root.right, root.leftreturn root
- 对称二叉树
核心思路:
如果一个树的左子树与右子树镜像对称,那么这个树是对称的。该问题可以转化为:两个树在什么情况下互为镜像?
如果同时满足下面的条件,两个树互为镜像: - 它们的两个根结点具有相同的值
- 每个树的右子树都与另一个树的左子树镜像对称
创建一个函数,传入2个节点,判断是否对称
class Solution(object):def isSymmetric(self, root):""":type root: TreeNode:rtype: bool"""def check(p, q):if p and q:a = p.val == q.valb = check(p.left, q.right)c = check(p.right, q.left)return a and b and celif (not p) and (not q):return Trueelse:return Falsereturn check(root, root)
40 二叉树的直径
class Solution(object):def diameterOfBinaryTree(self, root):""":type root: TreeNode:rtype: int"""def helper(root):if not root:return 0l = helper(root.left)r = helper(root.right)d = max(l, r) + 1self.res = max(self.res, l + r)return dself.res = 0helper(root)return self.res
41. 二叉树的层序遍历
class Solution(object):def levelOrder(self, root):""":type root: TreeNode:rtype: List[List[int]]"""if not root:returnq = [root]res = []while q:sz = len(q)temp = []for i in range(sz):cur = q.pop(0)temp.append(cur.val)if cur.left:q.append(cur.left)if cur.right:q.append(cur.right)res.append(temp[:])return res
42. 构造二叉搜索树
有序数组,找到mid,nums[mid]是根节点,左边和右边分别是左子树和右子树
class Solution(object):def sortedArrayToBST(self, nums):""":type nums: List[int]:rtype: TreeNode"""def construct(nums, lo, hi):if lo > hi:returnmid = lo + (hi-lo)//2root = TreeNode(nums[mid])root.left = construct(nums, lo, mid-1)root.right = construct(nums, mid+1, hi)return rootif not nums:returnn = len(nums)return construct(nums, 0, n-1)
43. 判断二叉搜索树
迭代法写中序遍历
class Solution(object):def isValidBST(self, root):""":type root: TreeNode:rtype: bool"""if not root:return Trueprev = Nonest = []cur = rootwhile st or cur:if cur:st.append(cur)cur = cur.leftelse:cur = st.pop() if prev and prev.val >= cur.val: return Falseprev = curcur = cur.rightreturn True
44. bst第k小元素
迭代法遍历,太爽了
class Solution(object):def kthSmallest(self, root, k):""":type root: TreeNode:type k: int:rtype: int"""st = []cur = rooti = 0while st or cur:if cur:st.append(cur)cur = cur.leftelse:cur = st.pop()i += 1if i == k:return cur.valcur = cur.right
45. 二叉树的右视图
层序遍历,从右往左
class Solution(object):def rightSideView(self, root):""":type root: TreeNode:rtype: List[int]"""if not root:returnq = deque()q.append(root)res = []while q:sz = len(q)res.append(q[0].val)for i in range(sz):cur = q.popleft()if cur.right:q.append(cur.right)if cur.left:q.append(cur.left)return res
46. 二叉树展开为链表
class Solution(object):def flatten(self, root):""":type root: TreeNode:rtype: None Do not return anything, modify root in-place instead."""if not root:returnl = self.flatten(root.left)r = self.flatten(root.right) root.left = Noneroot.right = lnode = rootwhile node.right:node = node.rightnode.right = rreturn root
47. 从前序和中序构造二叉树
class Solution(object):def buildTree(self, preorder, inorder):""":type preorder: List[int]:type inorder: List[int]:rtype: TreeNode"""def helper(preorder, prelo, prehi, inorder, inlo, inhi):if prelo > prehi or inlo > inhi:returnroot_val = preorder[prelo] root_idx = inorder.index(root_val)left_size = root_idx - inloroot = TreeNode(root_val)root.left = helper(preorder, prelo+1, prelo+left_size, inorder, inlo, root_idx-1)root.right = helper(preorder, prelo+left_size+1, prehi, inorder, root_idx+1, inhi)return rootn = len(preorder)return helper(preorder, 0, n-1, inorder, 0, n-1)
48. 路径总和III
后序遍历,helper返回以root为开始的路径和的哈希表
class Solution(object):def pathSum(self, root, targetSum):""":type root: TreeNode:type targetSum: int:rtype: int"""def helper(root):if not root:return {}l = helper(root.left)r = helper(root.right)res = {}for k, v in l.items():x = root.val + kres[x] = v for k, v in r.items():x = root.val + kres[x] = res.get(x, 0) + vres[root.val] = res.get(root.val, 0) + 1self.cnt += res.get(targetSum, 0)return resself.cnt = 0x = helper(root) return self.cnt
49. 二叉树的最近公共祖先
思路:如果一个节点能够在它的左右子树中分别找到 p 和 q,则该节点为 LCA 节点。
class Solution(object):def lowestCommonAncestor(self, root, p, q):""":type root: TreeNode:type p: TreeNode:type q: TreeNode:rtype: TreeNode"""def helper(root, p, q):if not root:return# 说明p或者q本身就是LCAif root == p or root == q:return rootleft = helper(root.left, p, q)right = helper(root.right, p, q) if left and right:# 说明p和q一个在左子树,另一个在右子树,root就是LCAreturn rootreturn left if left else right return helper(root, p, q)
50. 二叉树的最大路径和
自己ac的hard题目。
class Solution(object):def maxPathSum(self, root):""":type root: TreeNode:rtype: int"""def helper(root):if not root:return 0leftMax = helper(root.left)rightMax = helper(root.right)x = max(root.val, root.val+leftMax, root.val+rightMax) self.res = max(self.res, x, root.val+leftMax+rightMax)return xself.res = -1001helper(root)return self.res
图论
51. 岛屿数量
dfs
class Solution(object):def numIslands(self, grid):""":type grid: List[List[str]]:rtype: int"""def dfs(grid, i, j):m, n = len(grid), len(grid[0])if i < 0 or i >= m or j < 0 or j >= n:return if grid[i][j] == "0":return grid[i][j] = "0"dfs(grid, i+1, j)dfs(grid, i-1, j)dfs(grid, i, j+1)dfs(grid, i, j-1)m, n = len(grid), len(grid[0])res = 0for i in range(m):for j in range(n):if grid[i][j] == "1":dfs(grid, i, j)res += 1return res
52 腐烂橘子
来自 作者:nettee的题解
返回直到单元格中没有新鲜橘子为止所必须经过的最小分钟数。翻译一下,实际上就是求腐烂橘子到所有新鲜橘子的最短路径。要用bfs
一开始,我们找出所有腐烂的橘子,将它们放入队列,作为第 0 层的结点。
然后进行 BFS 遍历,每个结点的相邻结点可能是上、下、左、右四个方向的结点,注意判断结点位于网格边界的特殊情况。
由于可能存在无法被污染的橘子,我们需要记录新鲜橘子的数量。在 BFS 中,每遍历到一个橘子(污染了一个橘子),就将新鲜橘子的数量减一。如果 BFS 结束后这个数量仍未减为零,说明存在无法被污染的橘子
class Solution(object):def orangesRotting(self, grid):""":type grid: List[List[int]]:rtype: int""" m, n = len(grid), len(grid[0])q = deque()fresh_cnt = 0for i in range(m):for j in range(n):if grid[i][j] == 1:fresh_cnt += 1elif grid[i][j] == 2:q.append((i, j))res = 0while fresh_cnt > 0 and q:res += 1sz = len(q)for i in range(sz):cur = q.popleft()i, j = cur[0], cur[1]if i > 0 and grid[i-1][j] == 1:q.append((i-1, j))grid[i-1][j] = 2fresh_cnt -= 1 if i < m-1 and grid[i+1][j] == 1:q.append((i+1, j))grid[i+1][j] = 2fresh_cnt -= 1 if j > 0 and grid[i][j-1] == 1:q.append((i, j-1))grid[i][j-1] = 2fresh_cnt -= 1 if j < n-1 and grid[i][j+1] == 1:q.append((i, j+1))grid[i][j+1] = 2fresh_cnt -= 1 if fresh_cnt == 0:return reselse:return -1
53. 课程表
自己写的暴力解法:
class Solution(object):def canFinish(self, numCourses, prerequisites):""":type numCourses: int:type prerequisites: List[List[int]]:rtype: bool"""d = {}for i in prerequisites:a, b = i[0], i[1]if a not in d:d[a] = [b]else:d[a].append(b)learned = set()while len(learned) < numCourses:L = len(learned)# print(learned, x)for n in range(numCourses):if n not in d:learned.add(n)else:flag = Truefor x in d[n]:if x not in learned: flag = Falsebreakif flag:learned.add(n)# print(learned, x)if len(learned) == L:return Falsereturn True
看题解:有向图+bfs。用一个in_degree数组存储每个节点(每节课)的入度,再用一个map d存储每个节点的出度。
每次入度为0的课程入队,bfs的思路。
class Solution(object):def canFinish(self, numCourses, prerequisites):""":type numCourses: int:type prerequisites: List[List[int]]:rtype: bool"""d = {}in_degree = [0] * numCoursesfor i in prerequisites: a, b = i[0], i[1]in_degree[a] += 1if b not in d:d[b] = [a]else:d[b].append(a)q = deque()for n in range(numCourses):if in_degree[n] == 0:q.append(n)cnt = 0while q:cur = q.popleft()cnt += 1if cur in d:for k in d[cur]:in_degree[k] -= 1if in_degree[k] == 0:q.append(k)return cnt == numCourses
54. Trie前缀树
面试大概率不会考,跳过
相关文章:

leetcode刷题记录:hot100强化训练2:二叉树+图论
二叉树 36. 二叉树的中序遍历 递归就不写了,写一下迭代法 class Solution(object):def inorderTraversal(self, root):""":type root: TreeNode:rtype: List[int]"""if not root:return res []cur rootstack []while cur or st…...
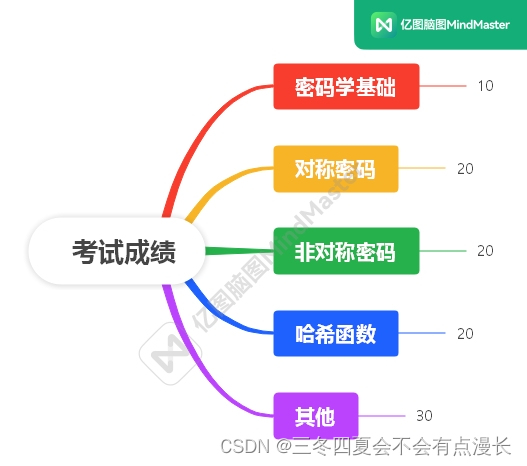
湘潭大学信息与网络安全复习笔记2(总览)
前面的实验和作业反正已经结束了,现在就是集中火力把剩下的内容复习一遍,这一篇博客的内容主要是参考教学大纲和教学日历 文章目录 教学日历教学大纲 教学日历 总共 12 次课,第一次课是概述,第二次和第三次课是密码学基础&#x…...

C语言:头歌使用函数找出数组中的最大值
任务描述 本关任务:本题要求实现一个找出整型数组中最大值的函数。 函数接口定义: int FindArrayMax( int a[], int n ); 其中a是用户传入的数组,n是数组a中元素的个数。函数返回数组a中的最大值。 主程序样例: #include <stdio.h>#…...

【技巧】Leetcode 191. 位1的个数【简单】
位1的个数 编写一个函数,输入是一个无符号整数(以二进制串的形式),返回其二进制表达式中 设置位 的个数(也被称为汉明重量)。 示例 1: 输入:n 11 输出:3 解释&#x…...

【Pandas驯化-02】pd.read_csv读取中文出现error解决方法
【Pandas】驯化-02pd.read_csv读取中文出现error解决方法 本次修炼方法请往下查看 🌈 欢迎莅临我的个人主页 👈这里是我工作、学习、实践 IT领域、真诚分享 踩坑集合,智慧小天地! 🎇 相关内容文档获取 微信公众号 &…...

linux下C语言如何操作文件(三)
我们继续介绍file_util.c中的函数: bool create_dir(const char* path):创建目录,根据给定的path创建目录,成功返回true,否则返回false。如果有父目录不存在,该函数不会创建。 /*** 创建目录* @param path 目录路径* @return true 创建成功,false 创建失败*/ bool cre…...
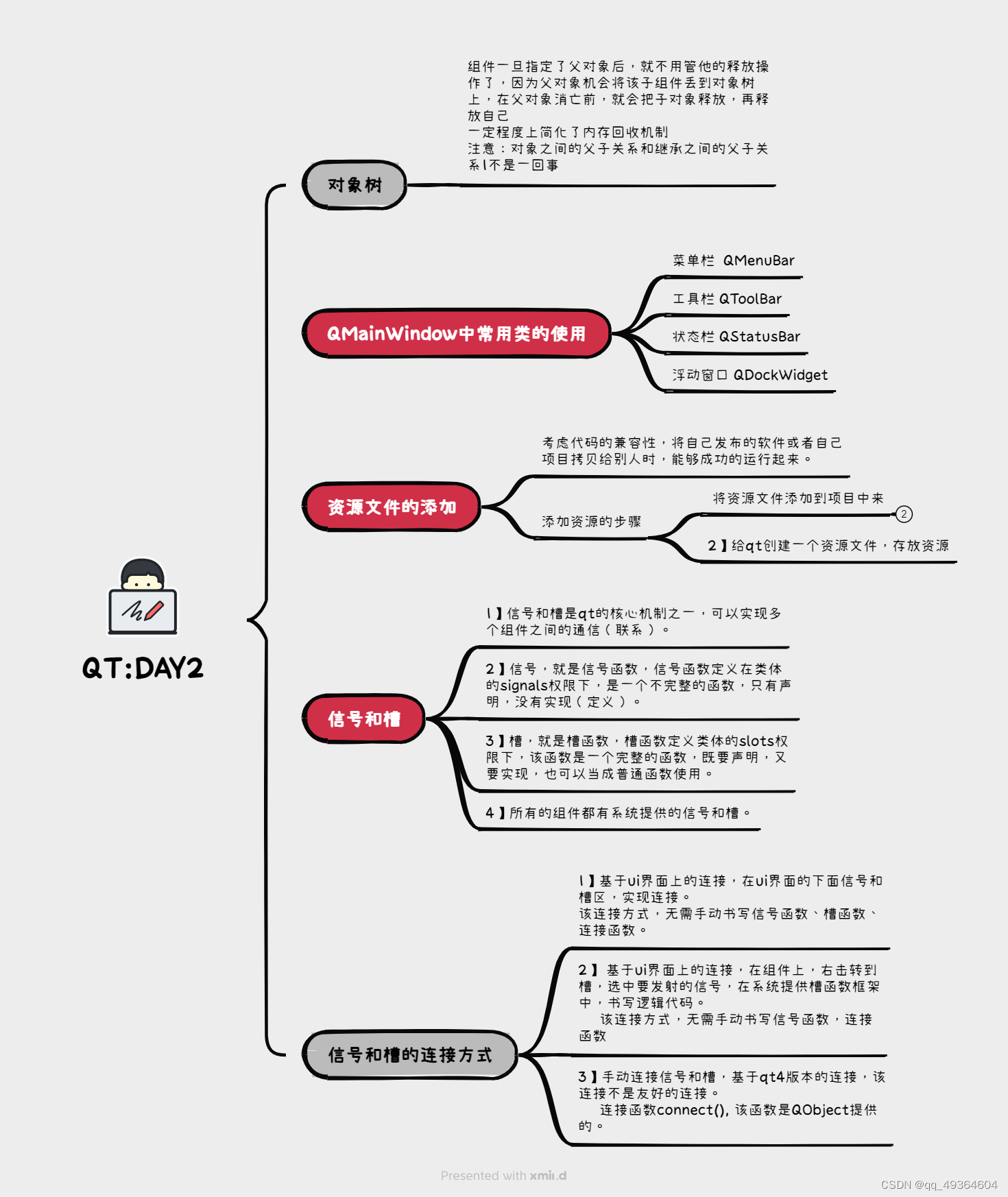
6.14作业
使用手动连接,将登录框中的取消按钮使用第二中连接方式,右击转到槽,在该槽函数中,调用关闭函数 将登录按钮使用qt4版本的连接到自定义的槽函数中,在槽函数中判断ui界面上输入的账号是否为"admin"࿰…...
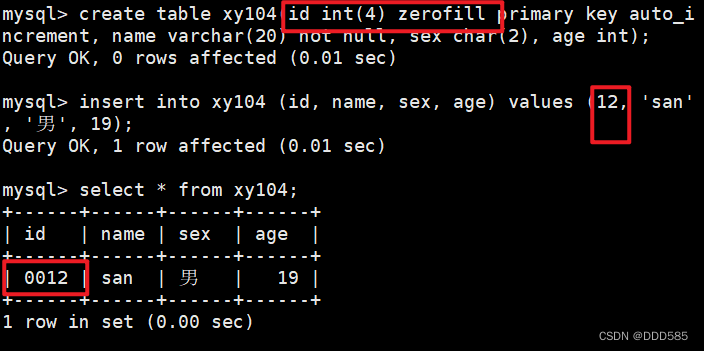
MySQL数据库管理(一)
目录 1.MySQL数据库管理 1.1 常用的数据类型编辑 1.2 char和varchar区别 2. 增删改查命令操作 2.1 查看数据库结构 2.2 SQL语言 2.3 创建及删除数据库和表 2.4 管理表中的数据记录 2.5 修改表名和表结构 3.MySQL的6大约束属性 1.MySQL数据库管理 1.1 常用的数据类…...

Kafka使用教程和案例详解
Kafka 使用教程和案例详解 Kafka 使用教程和案例详解1. Kafka 基本概念1.1 Kafka 是什么?1.2 核心组件2. Kafka 安装与配置2.1 安装 Kafka使用包管理器(如 yum)安装使用 Docker 安装2.2 配置 Kafka2.3 启动 Kafka3. Kafka 使用教程3.1 创建主题3.2 生产消息3.3 消费消息3.4 …...

TGI模型- 同期群-评论文本
用户偏好分析 TGI 1.1 用户偏好分析介绍 要分析的目标,在目标群体中的均值 和 全部群体里的均值进行比较, 差的越多说明 目标群体偏好越明显 TGI(Target Group Index,目标群体指数)用于反映目标群体在特定研究范围内…...
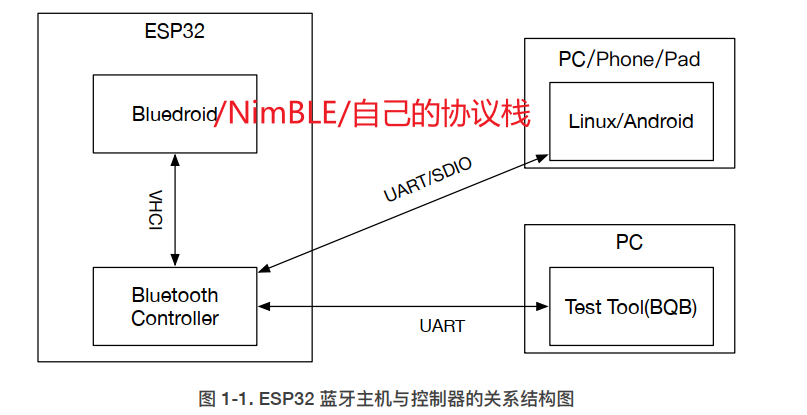
ESP32 BLE学习(0) — 基础架构
前言 (1)学习本文之前,需要先了解一下蓝牙的基本概念:BLE学习笔记(0.0) —— 基础概念(0) (2) 学习一款芯片的蓝牙肯定需要先简单了解一下该芯片的体系结构&a…...

【JAVA】Java中Spring Boot如何设置全局的BusinessException
文章目录 前言一、函数解释二、代码实现三、总结 前言 在Java应用开发中,我们常常需要读取配置文件。Spring Boot提供了一种方便的方式来读取配置。在本文中,我们将探讨如何在Spring Boot中使用Value和ConfigurationProperties注解来读取配置。 一、函数…...

pdf.js实现web h5预览pdf文件(兼容低版本浏览器)
注意 使用的是pdf.js 版本为 v2.16.105。因为新版本 兼容性不太好,部分手机预览不了,所以采用v2版本。 相关依赖 "canvas": "^2.11.2", "pdfjs-dist": "^2.16.105", "core-js-pure": "^3.37.…...

SSID简介
一、 SSID 概念定义 SSID(Service Set Identifier)即服务集标识符。它是无线网络中的一个重要标识,用于区分不同的无线网络。 相当于无线网络的名称,用于区分不同的无线网络。用户在众多可用网络中识别和选择特定网络的依据。通…...
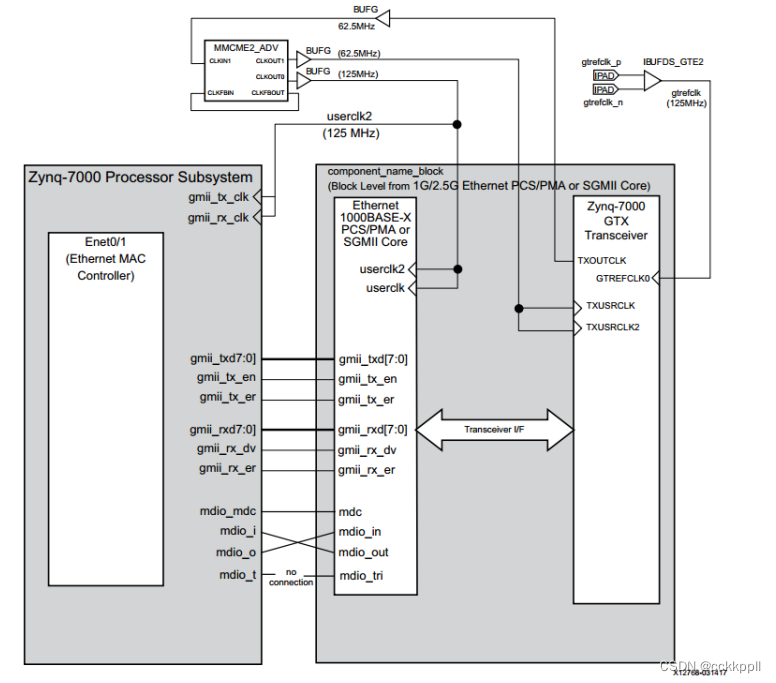
PS通过GTX实现SFP网络通信1
将 PS ENET1 的 GMII 接口和 MDIO 接口 通过 EMIO 方 式引出。在 PL 端将引出的 GMII 接口和 MDIO 接口与 IP 核 1G/2.5G Ethernet PCS/PMA or SGMII 连接, 1G/2.5G Ethernet PCS/PMA or SGMII 通过高速串行收发器 GTX 与 MIZ7035/7100 开发…...
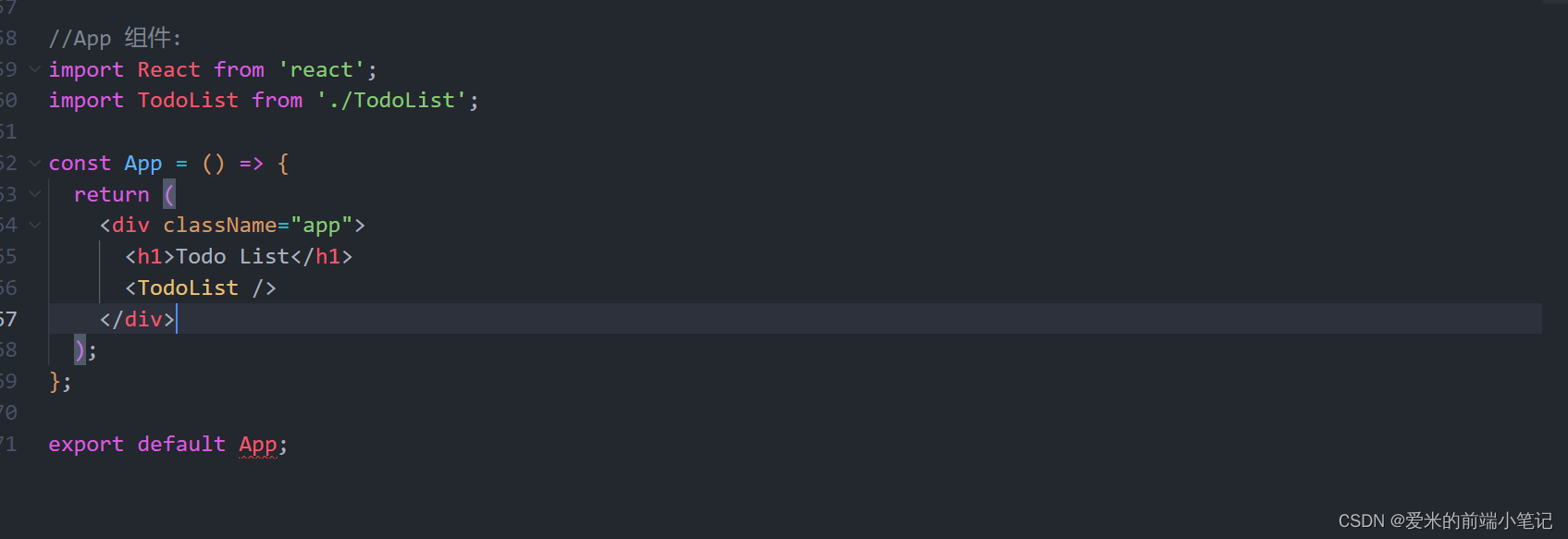
前端面试项目细节重难点(已工作|做分享)(九)
面试官:请你讲讲你在工作中如何开发一个新需求,你的整个开发过程是什么样的? 答:仔细想想,我开发新需求的过程如下: (1)第一步:理解需求文档: 首先&#x…...
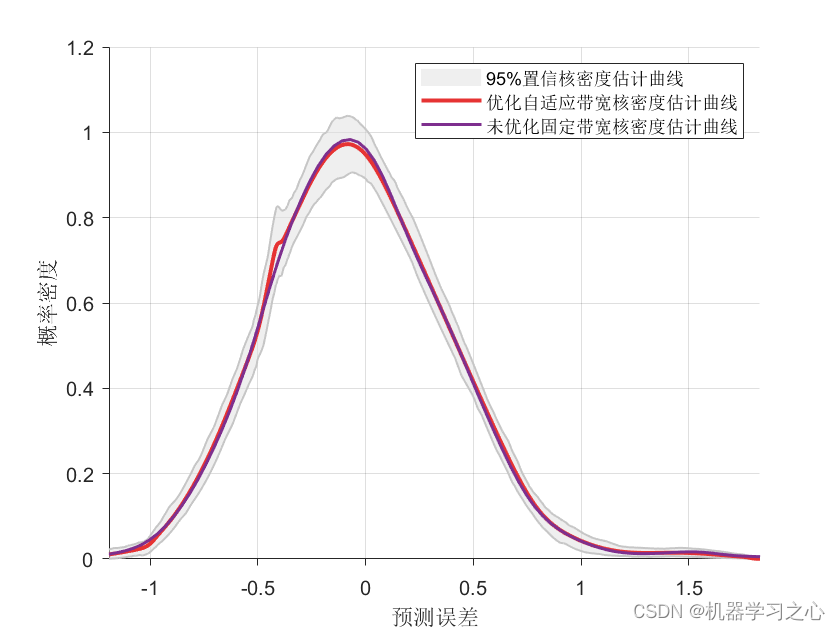
区间预测 | Matlab实现BP-ABKDE的BP神经网络自适应带宽核密度估计多变量回归区间预测
区间预测 | Matlab实现BP-ABKDE的BP神经网络自适应带宽核密度估计多变量回归区间预测 目录 区间预测 | Matlab实现BP-ABKDE的BP神经网络自适应带宽核密度估计多变量回归区间预测效果一览基本介绍程序设计参考资料 效果一览 基本介绍 1.Matlab实现BP-ABKDE的BP神经网络自适应带…...
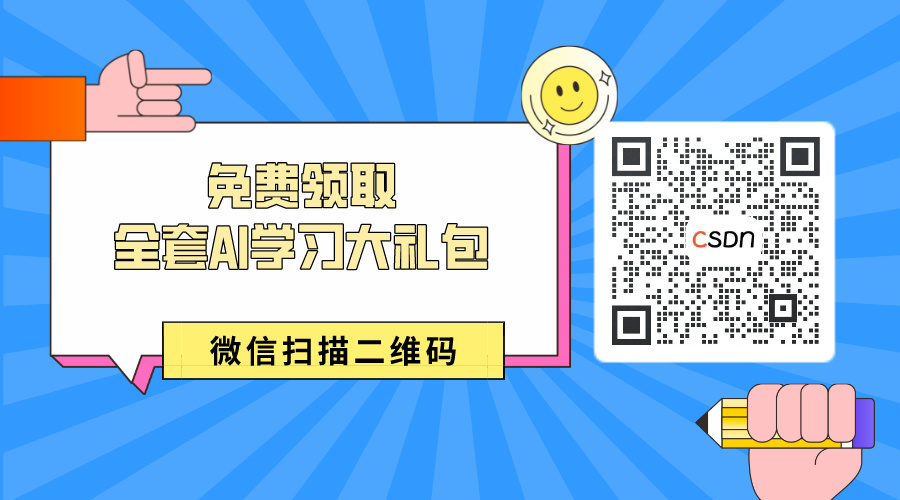
抢占人工智能行业红利,前阿里巴巴产品专家带你15天入门AI产品经理
前言 当互联网行业巨头纷纷布局人工智能,国家将人工智能上升为国家战略,藤校核心课程涉足人工智能…人工智能领域蕴含着巨大潜力,早已成为业内共识。 面对极大的行业空缺,不少人都希望能抢占行业红利期,进入AI领域。…...
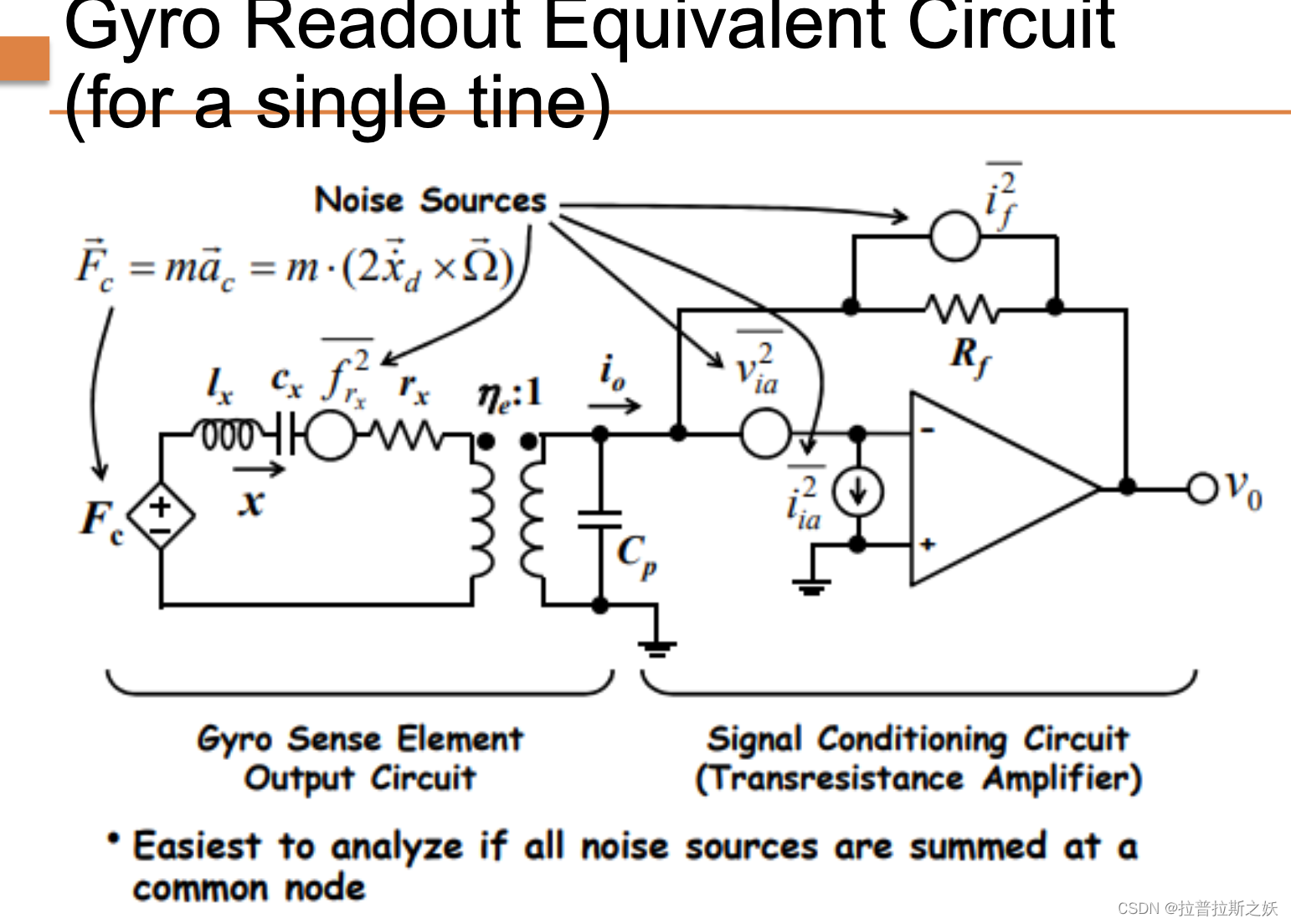
MEMS:Lecture 16 Gyros
陀螺仪原理 A classic spinning gyroscope measures the rotation rate by utilizing the conservation of angular momentum. 经典旋转陀螺仪通过利用角动量守恒来测量旋转速率。 Coriolis Effect and Coriolis Force 科里奥利效应是一种出现在旋转参考系中的现象。它描述了…...
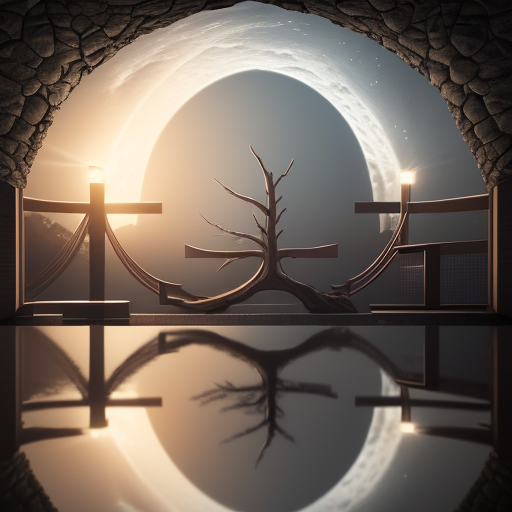
Java中List流式转换为Map的终极指南
哈喽,大家好,我是木头左! 在Java编程中,经常需要将一个List对象转换为另一个Map对象。这可能是因为需要根据List中的元素的某些属性来创建一个新的键值对集合。在本文中,我将向您展示如何使用Java 中的流式API轻松地实…...
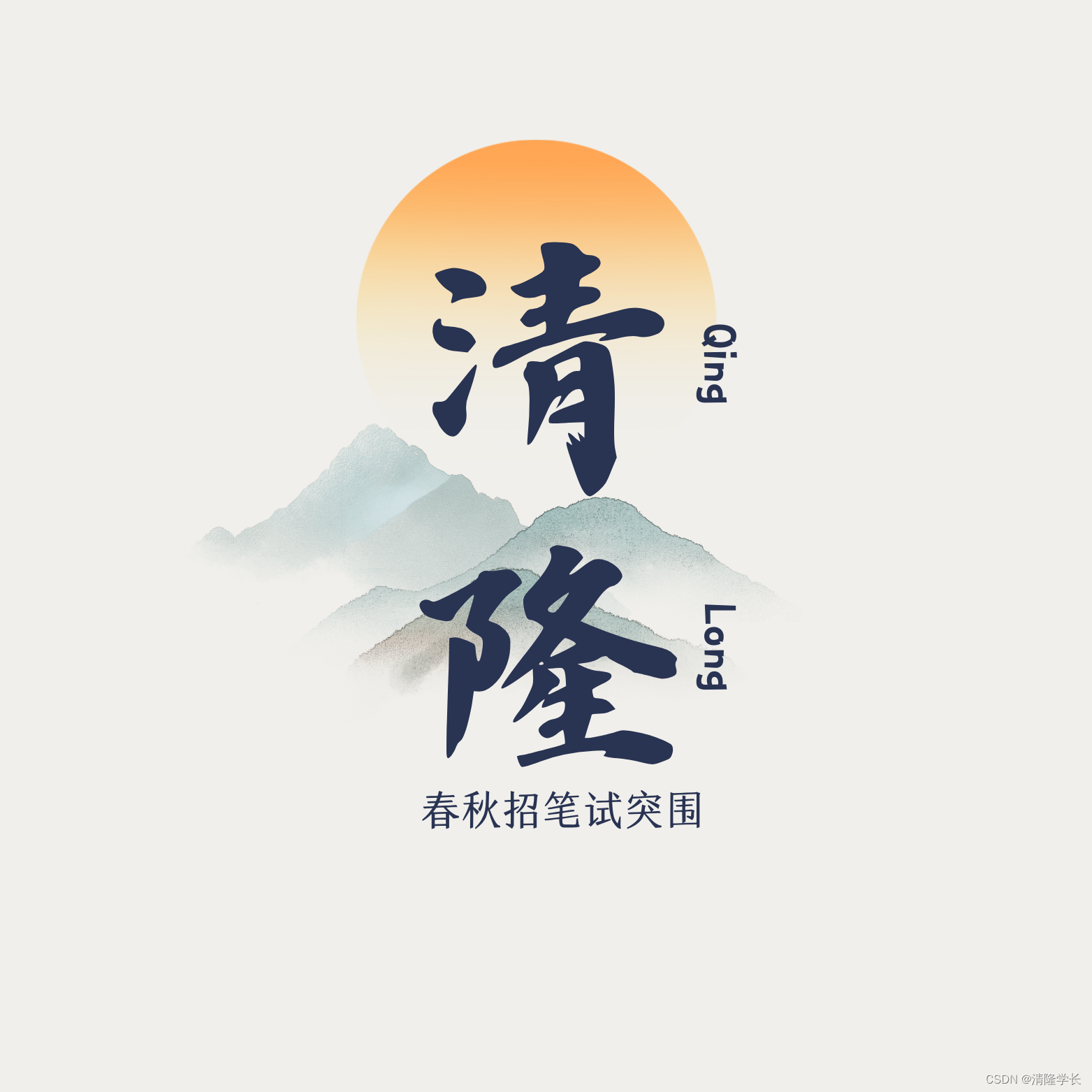
【秋招突围】2024届秋招笔试-小红书笔试题-第一套-三语言题解(Java/Cpp/Python)
🍭 大家好这里是清隆学长 ,一枚热爱算法的程序员 ✨ 本系计划跟新各公司春秋招的笔试题 💻 ACM银牌🥈| 多次AK大厂笔试 | 编程一对一辅导 👏 感谢大家的订阅➕ 和 喜欢💗 📧 清隆这边…...
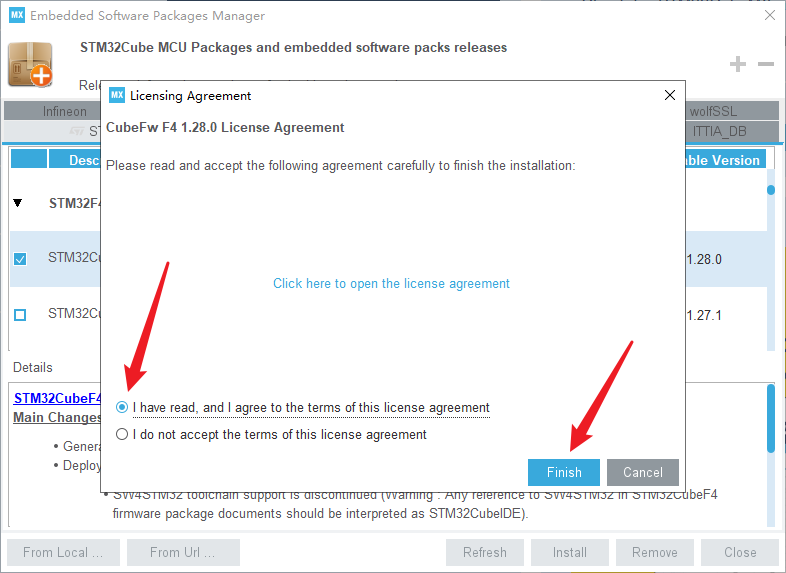
HAL库开发--STM32的HAL环境搭建
知不足而奋进 望远山而前行 目录 文章目录 前言 下载 安装 解压 安装 添加开发包 修改仓库路径 下载软件开发包(慢,不推荐) 解压已有软件开发包(快,推荐) 总结 前言 在嵌入式系统开发中&#x…...

【DPDK学习路径】七、创建RX/TX队列
上一节我们讲述了如何申请内存池缓冲区以便接下来创建 RX 队列,这一节我们将给出具体如何创建 RX/TX 队列。 在 DPDK 中提供了 rte_eth_rx_queue_setup 及 rte_eth_tx_queue_setup 这两个接口用于接收/发送队列的创建。 下面给出一个为各个网卡创建RX/TX 队列的实例…...
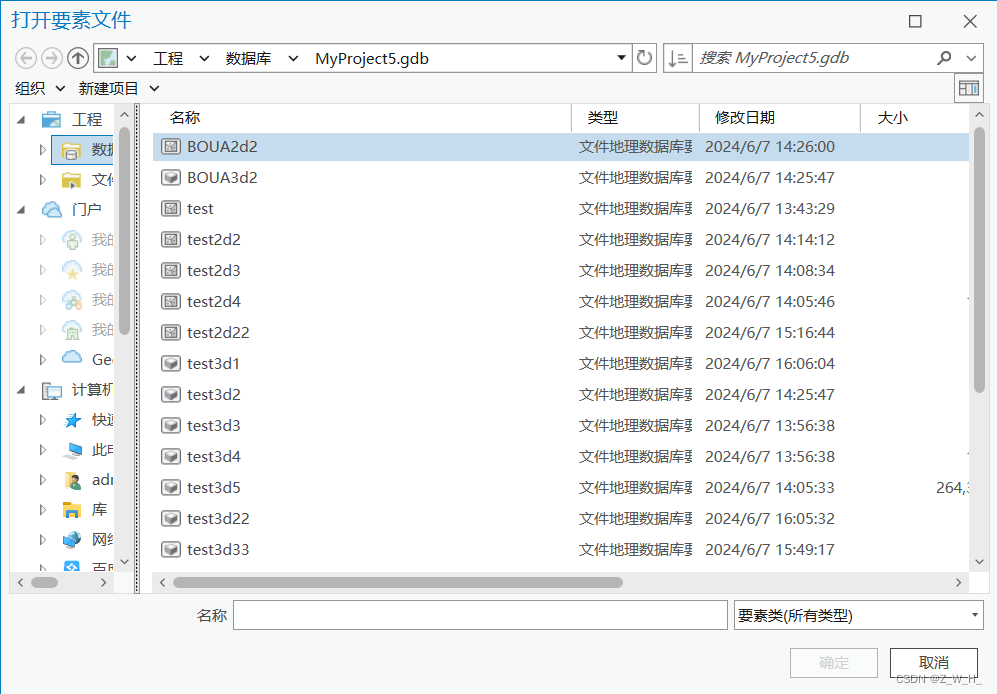
【ArcGISProSDK】OpenItemDialog打开文件对话框
打开单个文件 效果 代码 public async void OpenFunction() {// 获取默认数据库var gdbPath Project.Current.DefaultGeodatabasePath;OpenItemDialog openItemDialog new OpenItemDialog() { Title "打开要素文件",InitialLocation gdbPath,Filter ItemFilte…...
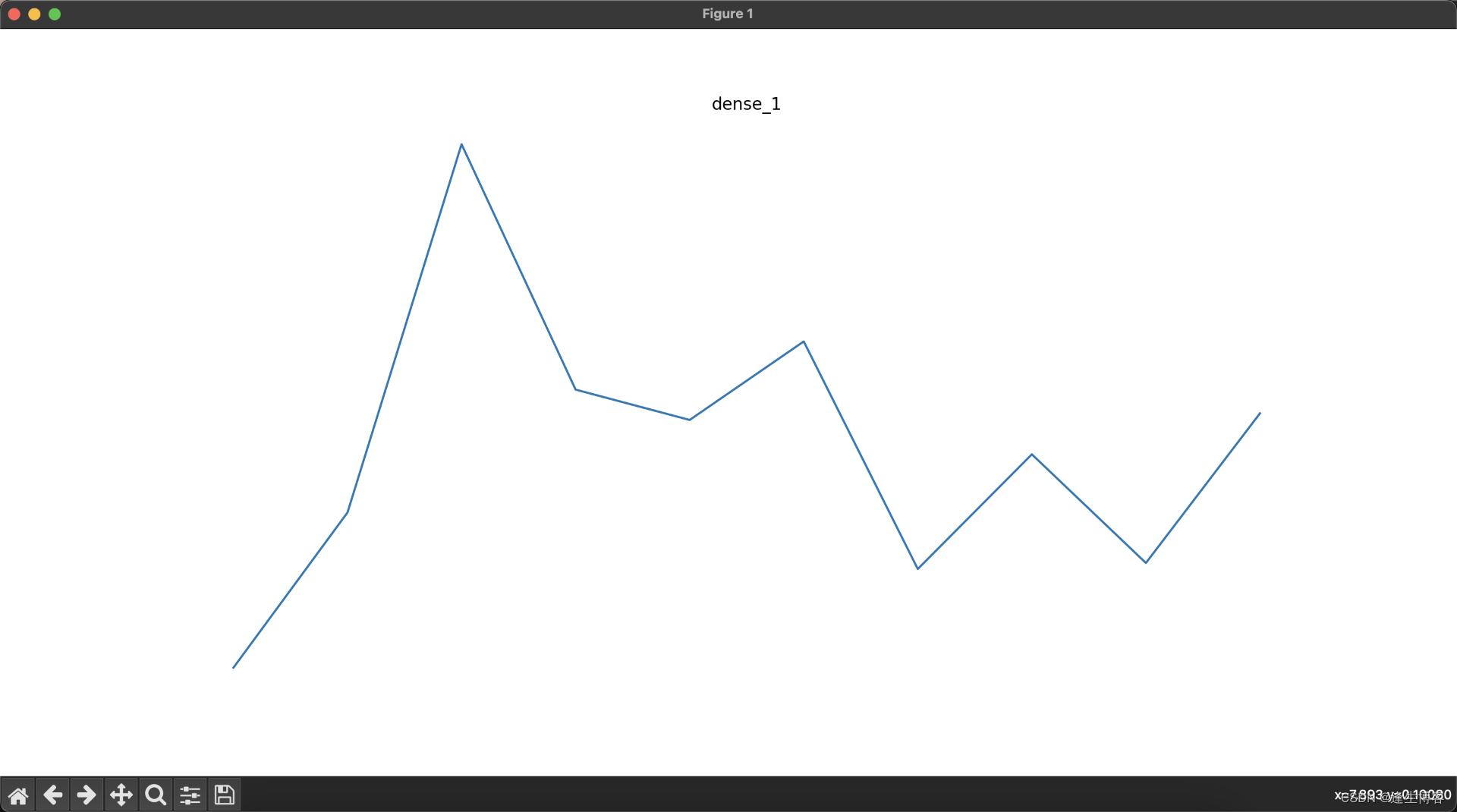
TensorFlow2.x基础与mnist手写数字识别示例
文章目录 Github官网文档Playground安装声明张量常量变量 张量计算张量数据类型转换张量数据维度转换ReLU 函数Softmax 函数卷积神经网络训练模型测试模型数据集保存目录显示每层网络的结果 TensorFlow 是一个开源的深度学习框架,由 Google Brain 团队开发和维护。它…...

大数据开发语言Scala入门
Scala是一种多范式编程语言,它集成了面向对象编程和函数式编程的特性。Scala运行在Java虚拟机上,并且可以与Java代码无缝交互,这使得它成为大数据处理和分析领域中非常受欢迎的语言,尤其是在使用Apache Spark这样的框架时。 Scal…...
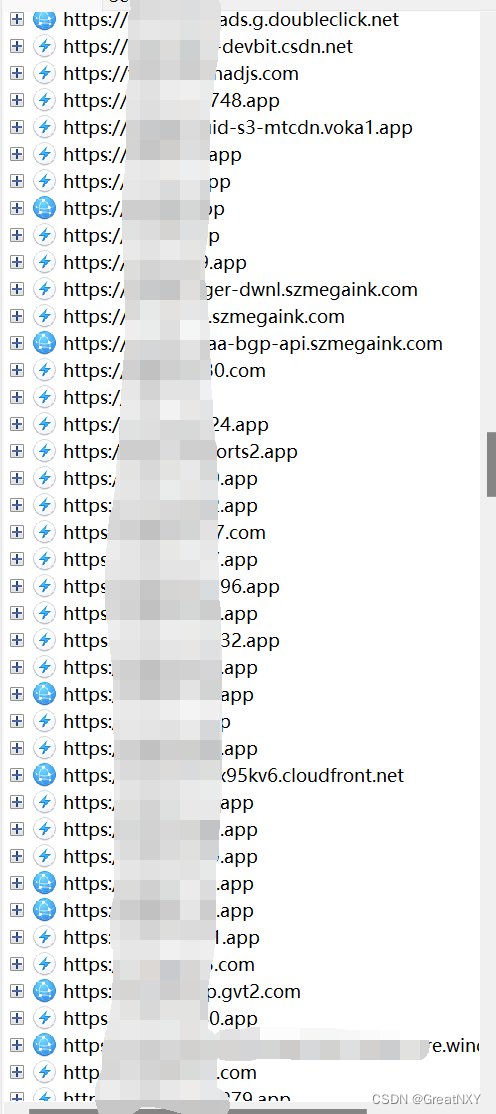
【CDN】逆天 CDN !BootCDN 向 JS 文件中植入恶意代码
今天在调试代码,突然控制台出现了非常多报错。 这非常可疑,报错指向的域名也证实了这一点。 因为我的 HTML 中只有一个外部开源库(qrcode.min.js),因此只有可能是它出现了问题。 我翻看了请求记录,发现这…...
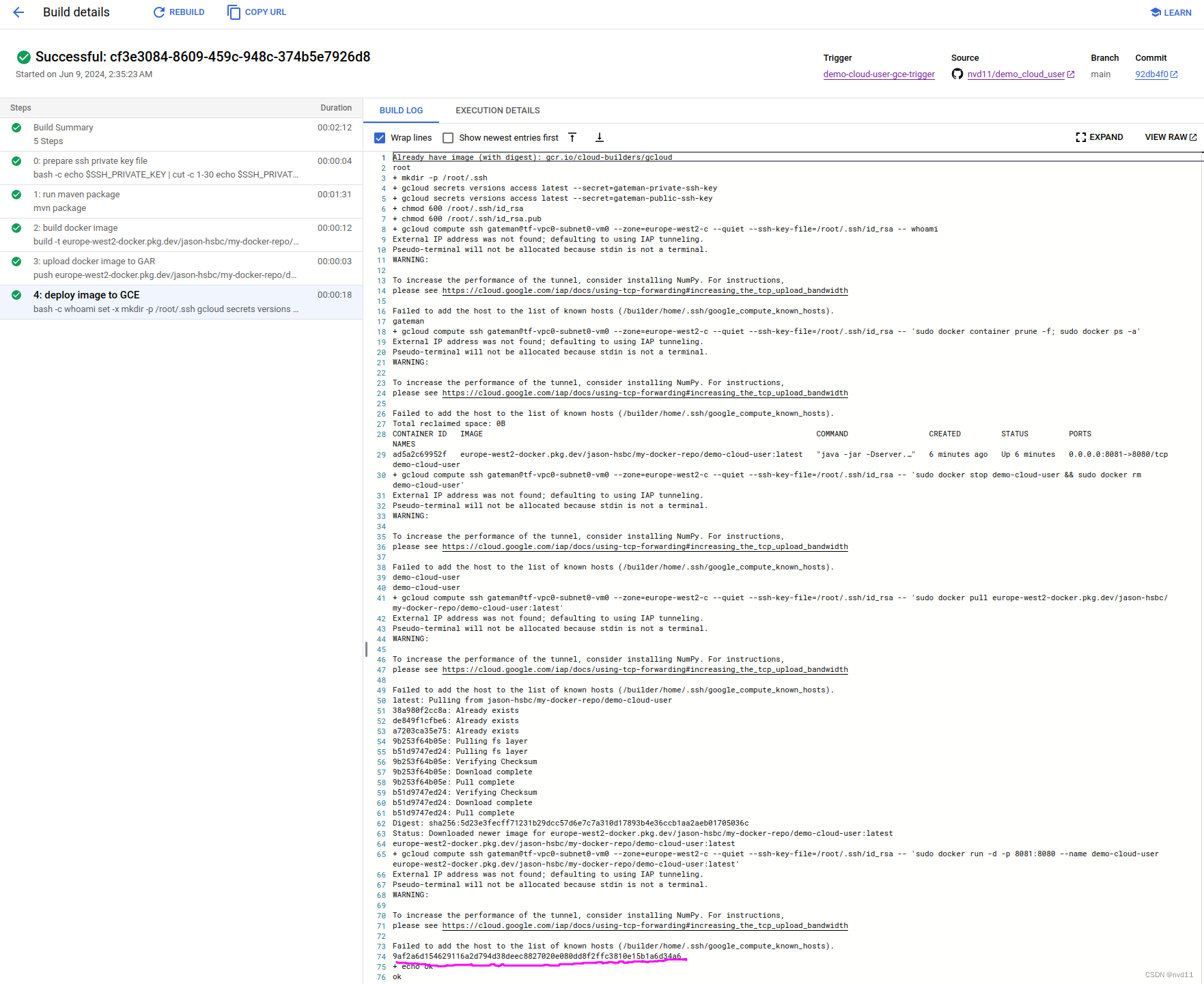
摆脱Jenkins - 使用google cloudbuild 部署 java service 到 compute engine VM
在之前 介绍 cloud build 的文章中 初探 Google 云原生的CICD - CloudBuild 已经介绍过, 用cloud build 去部署1个 spring boot service 到 cloud run 是很简单的, 因为部署cloud run 无非就是用gcloud 去部署1个 GAR 上的docker image 到cloud run 容…...
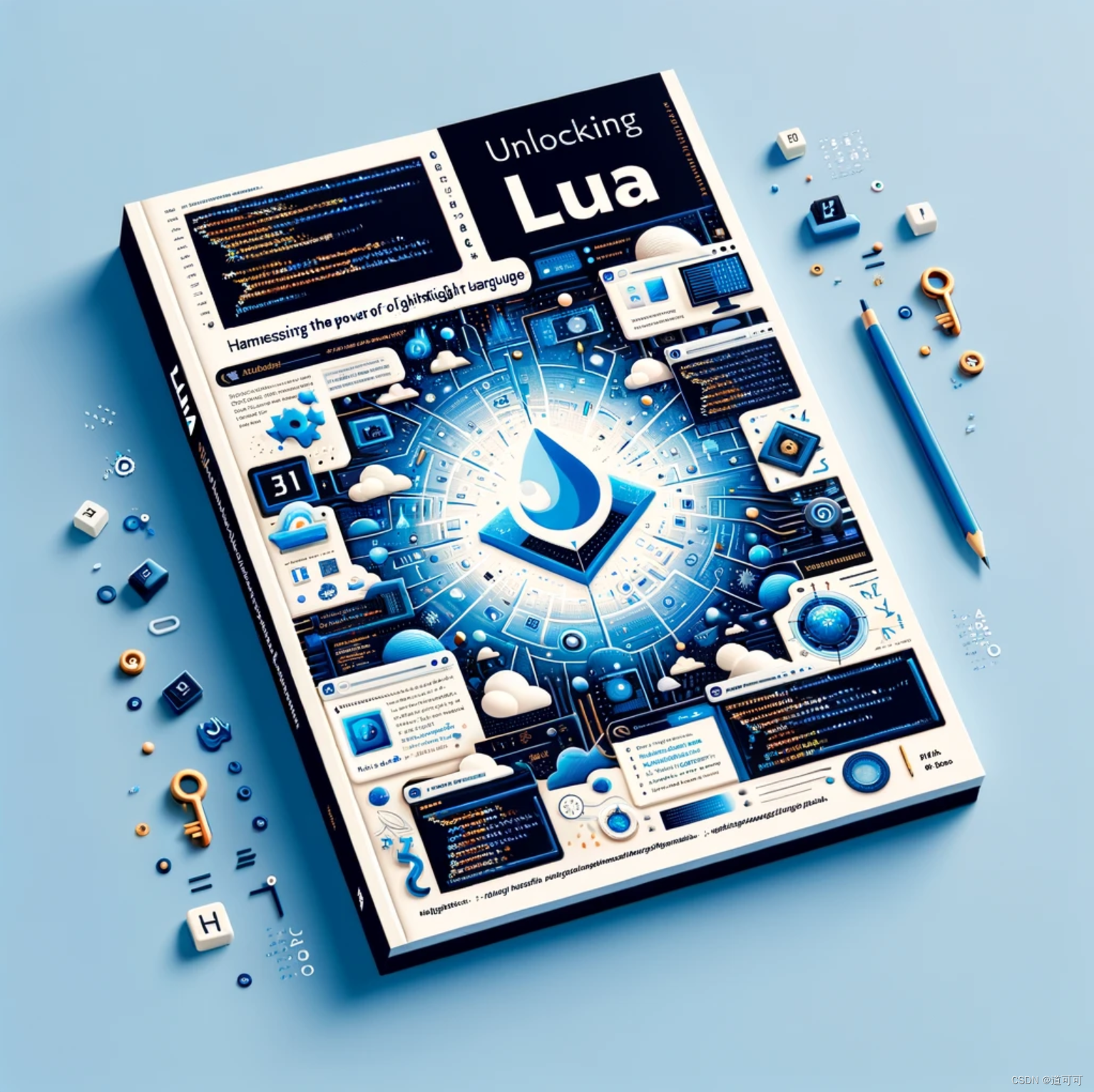
【CS.PL】Lua 编程之道: 控制结构 - 进度24%
3 初级阶段 —— 控制结构 文章目录 3 初级阶段 —— 控制结构3.1 条件语句:if、else、elseif3.2 循环语句:for、while、repeat-until3.2.1 输出所有的命令行参数3.2.2 while.lua3.2.3 repeat.lua及其作用域 🔥3.2.4 for.lua (For Statement)…...
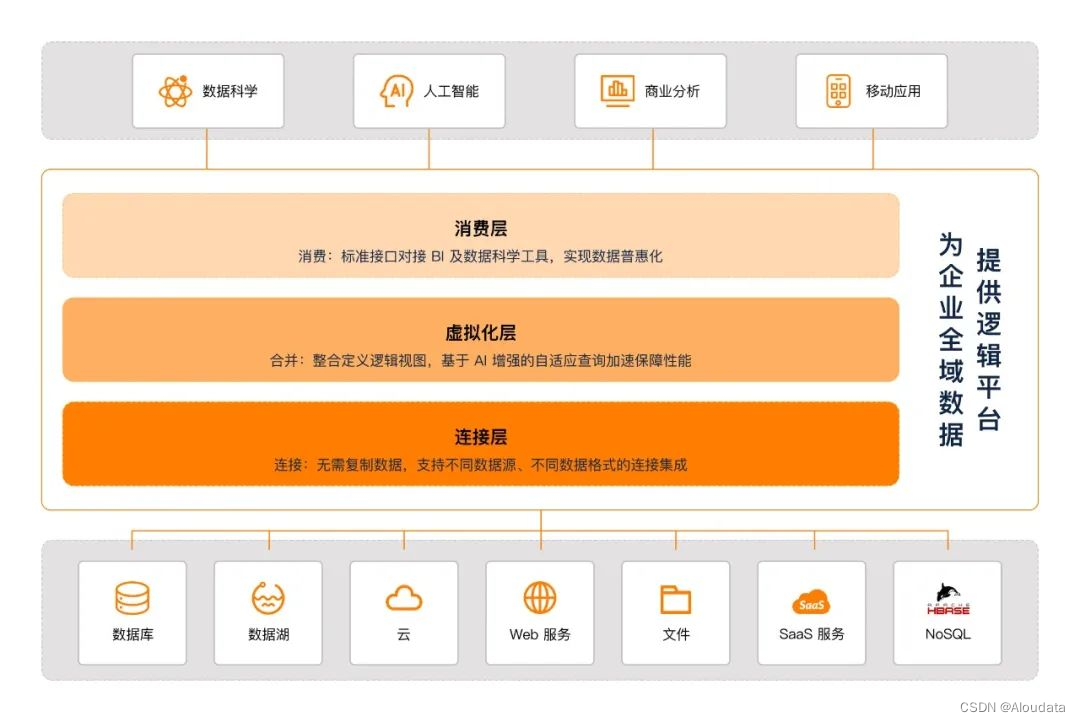
从“数据孤岛”、Data Fabric(数据编织)谈逻辑数据平台
提到逻辑数据平台,其核心在于“逻辑”,与之相对的便是“物理”。在过去,为了更好地利用和管理数据,我们通常会选择搭建数据仓库和数据湖,将所有数据物理集中起来。但随着数据量、用数需求和用数人员的持续激增…...