做蔬菜线上的网站/合肥新闻 今天 最新消息
1. 问题背景
有一天给同事CR,看到一段这样的代码
try {for (param : params) {//并发处理,func无返回值ThreadPool.submit(func(param));}
} catch (Exception e) {log.info("func抛异常啦,参数是:{}", param)
}
我:你这段代码是利用并发降低RT对吧,如果func内部抛异常,你确定可以catch到吗
同事:可以啊, 为什么不可以(...
我:不如你run一把,在func mock一个异常出来试试
同事:我靠还真是
我:你可以用execute,改动比较小
同事:那么是为什么呢
2. 同事的例子
import java.util.concurrent.*;public class ThreadPoolTest {public static void main(String[] args) throws Exception {ExecutorService executorService = new ThreadPoolExecutor(10, 20, 10, TimeUnit.SECONDS, new LinkedBlockingQueue<>());testExecute(executorService);Thread.sleep(2000);testSubmit1(executorService);Thread.sleep(2000);testSubmit2(executorService);}private static void testExecute(ExecutorService executorService) {executorService.execute(() -> {System.out.println("执行线程池execute方法");throw new RuntimeException("execute方法抛出异常");});}private static void testSubmit1(ExecutorService executorService) {executorService.submit(() -> {System.out.println("执行线程池submit方法1");throw new RuntimeException("submit方法1抛出异常");});}private static void testSubmit2(ExecutorService executorService) throws Exception {Future<Object> feature = executorService.submit(() -> {System.out.println("执行线程池submit方法2");throw new RuntimeException("submit方法2抛出异常");});feature.get();}
}
执行结果:
执行线程池execute方法
Exception in thread "pool-1-thread-1" java.lang.RuntimeException: execute方法抛出异常at ThreadPoolTest.lambda$testExecute$0(ThreadPoolTest.java:23)at java.util.concurrent.ThreadPoolExecutor.runWorker(ThreadPoolExecutor.java:1149)at java.util.concurrent.ThreadPoolExecutor$Worker.run(ThreadPoolExecutor.java:624)at java.lang.Thread.run(Thread.java:748)
执行线程池submit方法1
执行线程池submit方法2
Exception in thread "main" java.util.concurrent.ExecutionException: java.lang.RuntimeException: submit方法2抛出异常at java.util.concurrent.FutureTask.report(FutureTask.java:122)at java.util.concurrent.FutureTask.get(FutureTask.java:192)at ThreadPoolTest.testSubmit2(ThreadPoolTest.java:39)at ThreadPoolTest.main(ThreadPoolTest.java:17)
Caused by: java.lang.RuntimeException: submit方法2抛出异常at ThreadPoolTest.lambda$testSubmit2$2(ThreadPoolTest.java:37)at java.util.concurrent.FutureTask.run$$$capture(FutureTask.java:266)at java.util.concurrent.FutureTask.run(FutureTask.java)at java.util.concurrent.ThreadPoolExecutor.runWorker(ThreadPoolExecutor.java:1149)at java.util.concurrent.ThreadPoolExecutor$Worker.run(ThreadPoolExecutor.java:624)at java.lang.Thread.run(Thread.java:748)
3. 原理分析
3.1 线程池包的继承结构
3.2 submit和execute方法的差异
3.2.1 execute
方法定义在最顶层的Executor接口,并且Executor接口有且仅有这一个方法
public interface Executor {/*** Executes the given command at some time in the future. The command* may execute in a new thread, in a pooled thread, or in the calling* thread, at the discretion of the {@code Executor} implementation.** @param command the runnable task* @throws RejectedExecutionException if this task cannot be* accepted for execution* @throws NullPointerException if command is null*/void execute(Runnable command);
}
方法实现在ThreadPoolExecutor:
public void execute(Runnable command) {if (command == null)throw new NullPointerException();int c = ctl.get();if (workerCountOf(c) < corePoolSize) {if (addWorker(command, true))return;c = ctl.get();}if (isRunning(c) && workQueue.offer(command)) {int recheck = ctl.get();if (! isRunning(recheck) && remove(command))reject(command);else if (workerCountOf(recheck) == 0)addWorker(null, false);}else if (!addWorker(command, false))reject(command);}
实际执行的过程,在worker(是runnable的实现类)的run方法,run方法实际执行的是runWorker方法
final void runWorker(Worker w) {Thread wt = Thread.currentThread();Runnable task = w.firstTask;w.firstTask = null;w.unlock(); // allow interruptsboolean completedAbruptly = true;try {while (task != null || (task = getTask()) != null) {w.lock();// If pool is stopping, ensure thread is interrupted;// if not, ensure thread is not interrupted. This// requires a recheck in second case to deal with// shutdownNow race while clearing interruptif ((runStateAtLeast(ctl.get(), STOP) ||(Thread.interrupted() &&runStateAtLeast(ctl.get(), STOP))) &&!wt.isInterrupted())wt.interrupt();try {beforeExecute(wt, task);Throwable thrown = null;try {task.run();} catch (RuntimeException x) {thrown = x; throw x;} catch (Error x) {thrown = x; throw x;} catch (Throwable x) {thrown = x; throw new Error(x);} finally {afterExecute(task, thrown);}} finally {task = null;w.completedTasks++;w.unlock();}}completedAbruptly = false;} finally {processWorkerExit(w, completedAbruptly);}}
可以看到执行过程中,如果task.run();发生异常,没有catch处理,异常会层层向外抛出;最终进入finally块,执行processWorkerExit;
3.2.2 submit
submit方法定义在ExecutorService
public interface ExecutorService extends Executor {/*** Submits a value-returning task for execution and returns a* Future representing the pending results of the task. The* Future's {@code get} method will return the task's result upon* successful completion.** <p>* If you would like to immediately block waiting* for a task, you can use constructions of the form* {@code result = exec.submit(aCallable).get();}** <p>Note: The {@link Executors} class includes a set of methods* that can convert some other common closure-like objects,* for example, {@link java.security.PrivilegedAction} to* {@link Callable} form so they can be submitted.** @param task the task to submit* @param <T> the type of the task's result* @return a Future representing pending completion of the task* @throws RejectedExecutionException if the task cannot be* scheduled for execution* @throws NullPointerException if the task is null*/<T> Future<T> submit(Callable<T> task);/*** Submits a Runnable task for execution and returns a Future* representing that task. The Future's {@code get} method will* return the given result upon successful completion.** @param task the task to submit* @param result the result to return* @param <T> the type of the result* @return a Future representing pending completion of the task* @throws RejectedExecutionException if the task cannot be* scheduled for execution* @throws NullPointerException if the task is null*/<T> Future<T> submit(Runnable task, T result);/*** Submits a Runnable task for execution and returns a Future* representing that task. The Future's {@code get} method will* return {@code null} upon <em>successful</em> completion.** @param task the task to submit* @return a Future representing pending completion of the task* @throws RejectedExecutionException if the task cannot be* scheduled for execution* @throws NullPointerException if the task is null*/Future<?> submit(Runnable task);
}
实现在AbstractExecutorService
public Future<?> submit(Runnable task) {if (task == null) throw new NullPointerException();RunnableFuture<Void> ftask = newTaskFor(task, null);execute(ftask);return ftask;}
可以看到这里创建了RunnableFuture(而不是基础的worker),顾名思义,RunnableFuture同时实现了Runnable和Future接口,也就意味着可以对该任务执行get操作,看看RunnableFuture的run方法:
public void run() {if (state != NEW ||!UNSAFE.compareAndSwapObject(this, runnerOffset,null, Thread.currentThread()))return;try {Callable<V> c = callable;if (c != null && state == NEW) {V result;boolean ran;try {result = c.call();ran = true;} catch (Throwable ex) {result = null;ran = false;setException(ex);}if (ran)set(result);}} finally {// runner must be non-null until state is settled to// prevent concurrent calls to run()runner = null;// state must be re-read after nulling runner to prevent// leaked interruptsint s = state;if (s >= INTERRUPTING)handlePossibleCancellationInterrupt(s);}}
catch块对方法异常做了处理,与执行结果一同在Future中暂存起来;submit()执行完毕后返回Future对象,执行future.get()会触发异常的抛出;
当然了,如果你只是执行了submit,没有获取future,异常就会“神奇地”消失。
参考:
Java线程池实现原理及其在美团业务中的实践 - 美团技术团队
https://zhuanlan.zhihu.com/p/651997713
相关文章:
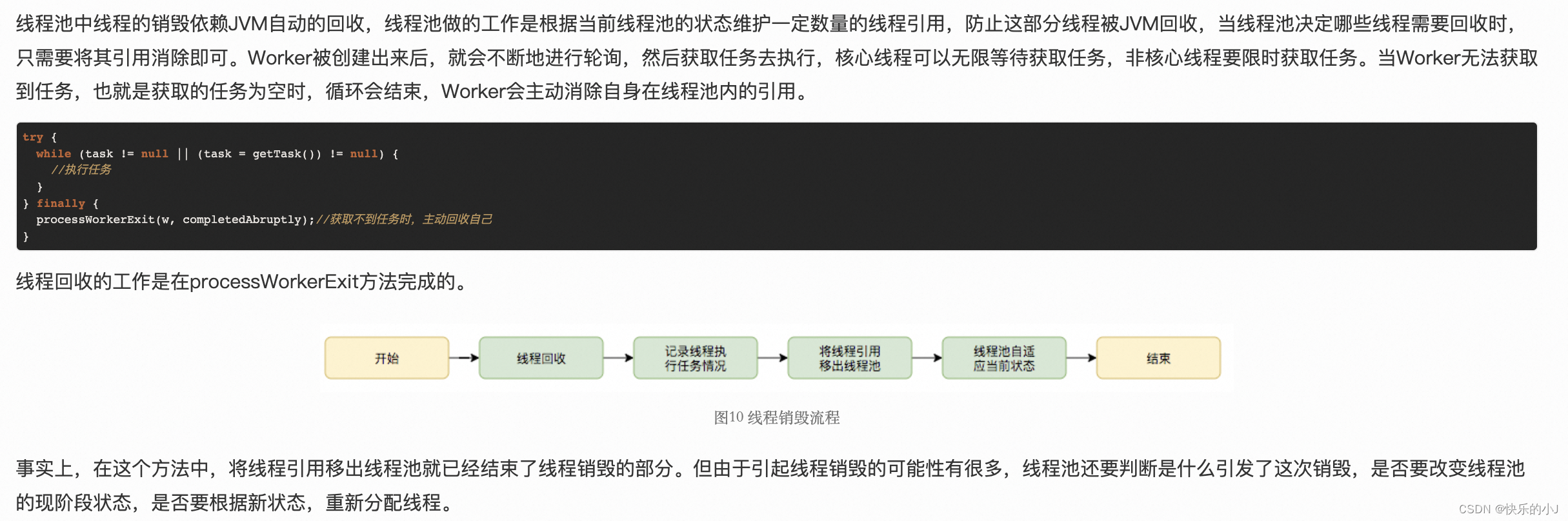
线程池吞掉异常的case:源码阅读与解决方法
1. 问题背景 有一天给同事CR,看到一段这样的代码 try {for (param : params) {//并发处理,func无返回值ThreadPool.submit(func(param));} } catch (Exception e) {log.info("func抛异常啦,参数是:{}", param) } 我:你这段代码是…...

基于mysqlbinlog恢复数据
1、把binlog转换为SQL mysqlbinlog --base64-outputdecode-rows -vv /usr/local/mysql/log-bin/mysql-bin.000003 >result.sql find / -name result.sql 2、查看events show binlog events in mysql-bin.000003; 3、回滚到3667那一行的数据 mysqlbinlog -v /usr/local/mys…...

Android_Android Studio 常用快捷键 for mac
功能快捷键运行ctrl R优化importctrl opt O格式化opt cmd L自动修正opt enter自动补齐cmd J自动生成代码cmd N搜索使用到的地方fn opt F7 ( cmd)搜索使用到的地方2shift cmd F搜索类cmd O当前文件搜索cmd F全局搜索按两下 shift搜索文件shift cmd O搜索符号opt…...

[EFI]NUC11电脑 Hackintosh 黑苹果efi引导文件
硬件型号驱动情况主板 英特尔 NUC11DBBi9(LPC Controller WM590芯片组) 处理器 11th Gen Intel Core i9-11900KB 3.30GHz 八核 已驱动内存32 GB ( 三星 DDR4 3200MHz 16GB x 2 )已驱动硬盘三星 MZVL21T0HCLR-00B00 (1024 GB / 固态硬盘)已驱动显卡AMD R…...

在Ubuntu上配置和设置防火墙UFW
在本文我们学习如何在Ubuntu上配置和设置UFW(防火墙),UFW代表“不复杂的防火墙”,它充当IPTABLES的接口,从而简化了防火墙的配置过程,对于防火墙来说,这是非常困难的。初学者学习和配置防火墙规…...
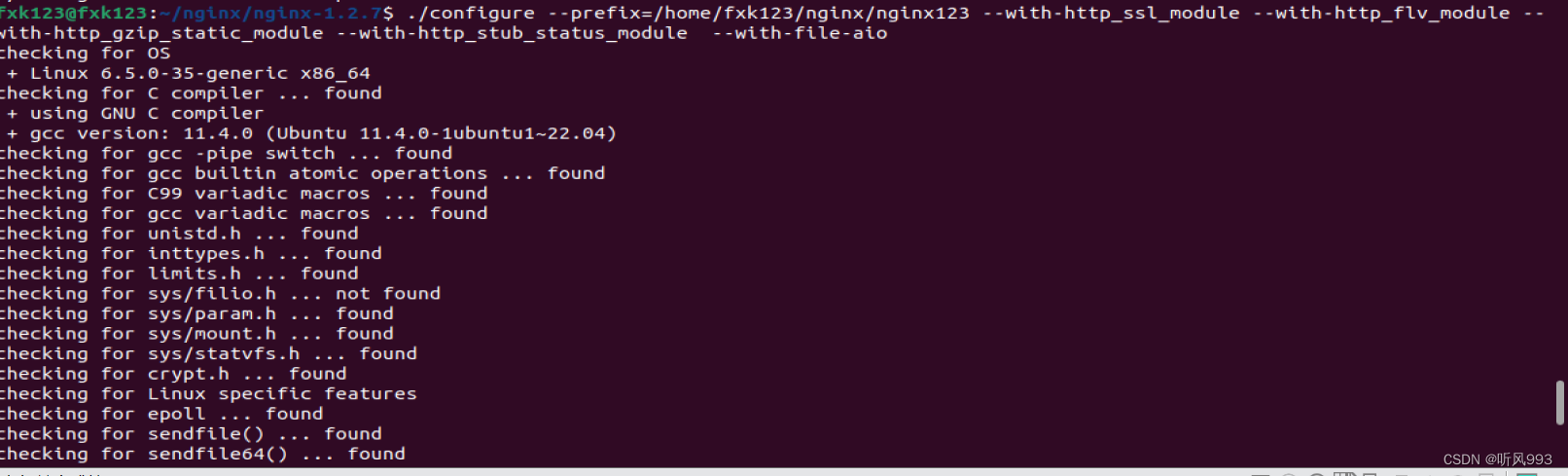
nginx安装环境部署(完整步骤)
在部署nginx前,我们需要进行环境的部署 1.编译工具gcc,g,autoconf,automake ,make sudo apt-get install gcc g autoconf automake make 2.依赖库zlib,openssl,pcre 2.1 openssl下载地址 https://www.open…...

如何做电子骑缝章?
制作电子骑缝章的过程可以依据不同情况和所使用的工具而有所不同,但基本思路是确保印章能够跨过页面接缝,以验证文档的完整性。以下是几种常见的方法: 使用专业电子合同平台 选择平台:首先,确保你使用的电子合同平台…...
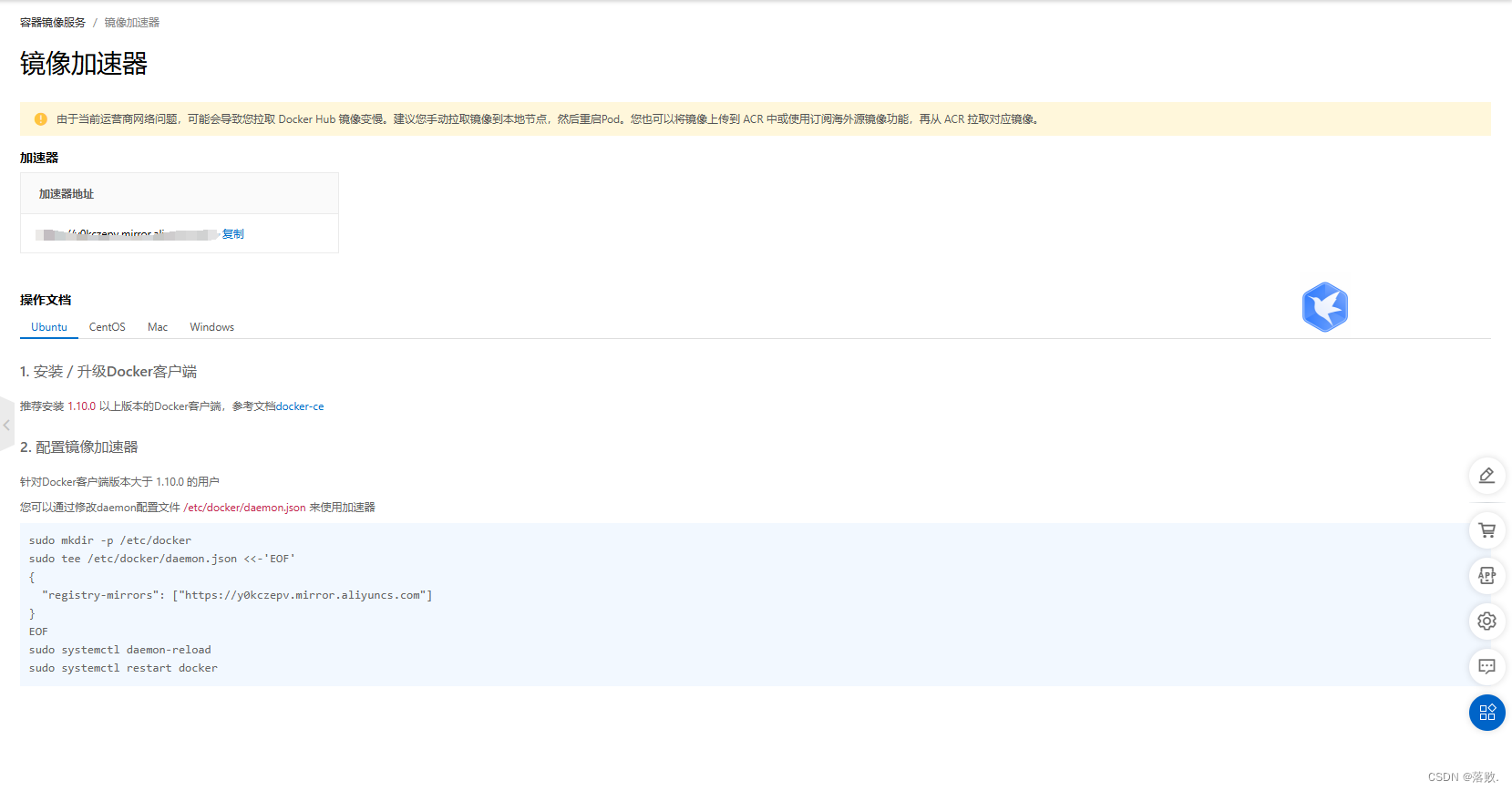
2024.6.13 bailuo-Docker 安装与镜像拉取
2024.6.13 bailuo-Docker 安装与镜像拉取 2024.6.12 bailuo-安装与镜像拉取 卸载 Docker 如果已安装旧版 Docker 则先卸载 yum remove docker \docker-client \docker-client-latest \docker-common \docker-latest \docker-latest-logrotate \docker-logrotate \docker-en…...
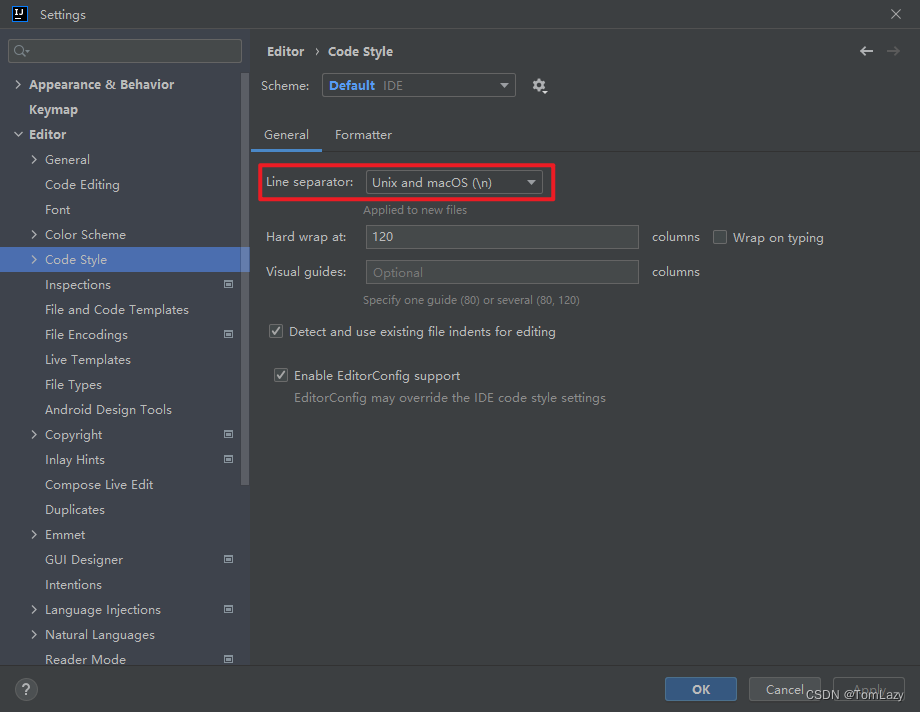
【Java开发规范】IDEA 设置 text file encoding 为 UTF-8,且文件的换行符使用 Unix 格式
1. IDEA 设置 text file encoding 为 UTF-8 file -> settings -> editor -> code style -> file encoding Transparent-native-to-asci conversion 要不要勾选?> 不推荐勾选(它的作用是用来自动转换ASCII编码,防止文件乱码&am…...

使用`LD_PRELOAD`和`jemalloc`实现C/C++信号的内存堆栈信息收集
文章目录 0. 概要1. 编译jemalloc2. 编译钩子共享库liballoc_hook.so3. 使用LD_PRELOAD加载钩子库liballoc_hook.so测试3.1 设置环境变量3.2 使用LD_PRELOAD加载钩子库并运行程序3.3 发送SIGUSR1信号以触发堆栈信息打印3.4 使用jeprof解析heap堆栈信息文件 4. 示例程序example.…...
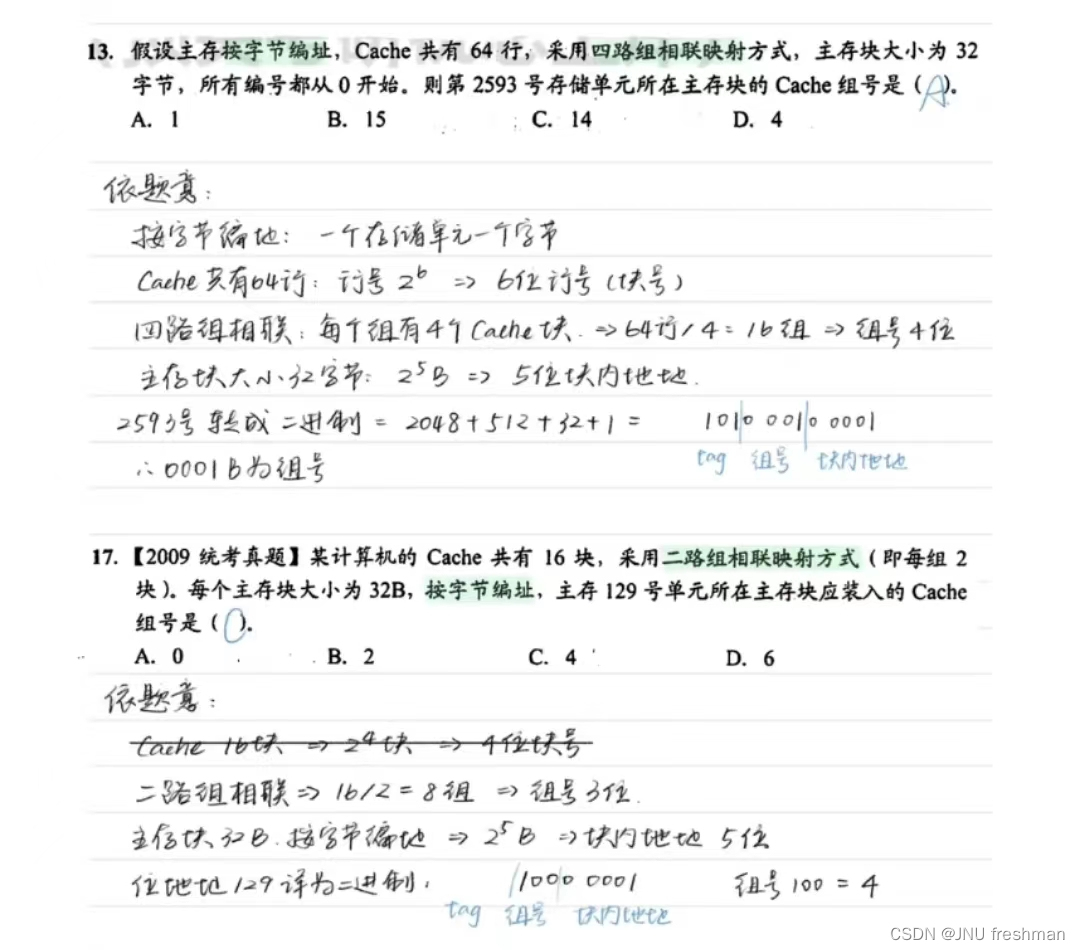
计算机组成原理(四)Cache存储器
文章目录 Cache存储器的基本原理cache命中率、平均访问时间、效率地址映射全相联映射直接映射组相联映射 查找算法cache 存储器替换策略cache 存储器-写操作策略习题 Cache存储器的基本原理 Cache是一种高速缓冲寄存器,是为了解决CPU和主存之间速度不匹配而采用的一…...
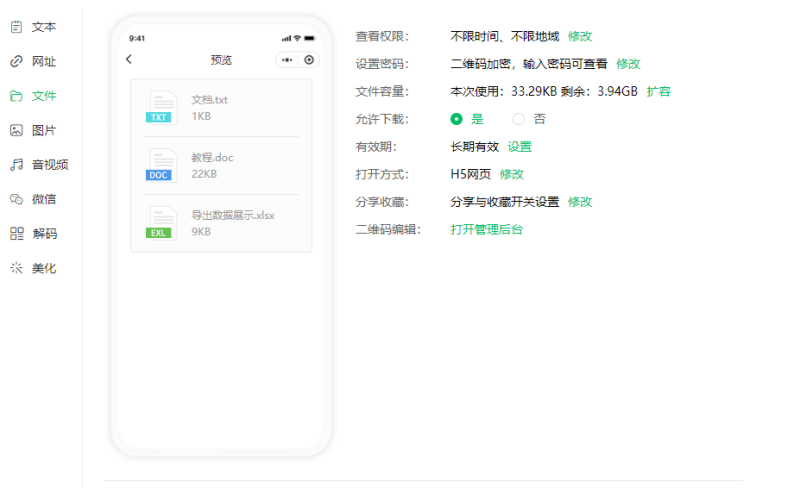
怎么做成的文件二维码?扫阅览文件的制作方法
现在用二维码来分享或者查看文件是一种很常用的方式,比如常见的文件内容有简历、资料、作品、压缩包等等。通过将文件生成二维码能够在提升文件传输速度的同时还有利于用户体验的提升,那么如何制作可以长期提供文件预览或者下载的二维码呢? …...

js 前端 Function.prototype.call.call(0[‘toString‘], *, 16)
这个函数将 数组转任意进制 Function.prototype.call.call(0[toString], *, 16)...
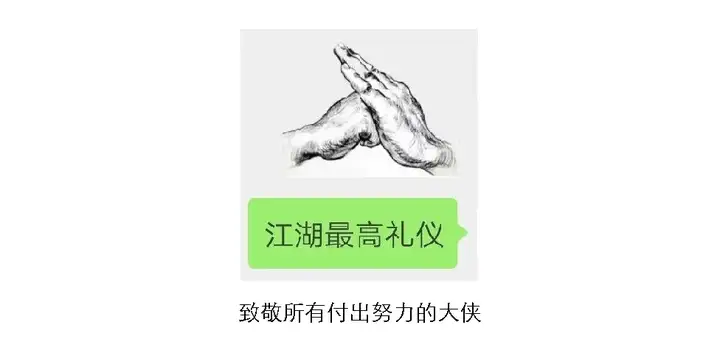
李沐:用随机梯度下降来优化人生!
大侠幸会,在下全网同名「算法金」 0 基础转 AI 上岸,多个算法赛 Top 「日更万日,让更多人享受智能乐趣」 今天我们来聊聊达叔 6 大核心算法之 —— 优化 算法。吴恩达:机器学习的六个核心算法! 梯度下降优化算法是机器…...

Linux 环境.Net程序堆栈查询
# 安装 dotnet tool install --global dotnet-dump# 收集信息, 产生 core_XXX 文件 dotnet-dump collect -p pid# 分析 core_XXX 文件 dotnet dump analyze core_XXX# 列出 大于 XXX 字节的 对象 dumpheap -stat -min XXX# 查看对象具体信息 dumpobj address_XXX# 查看对应引用…...
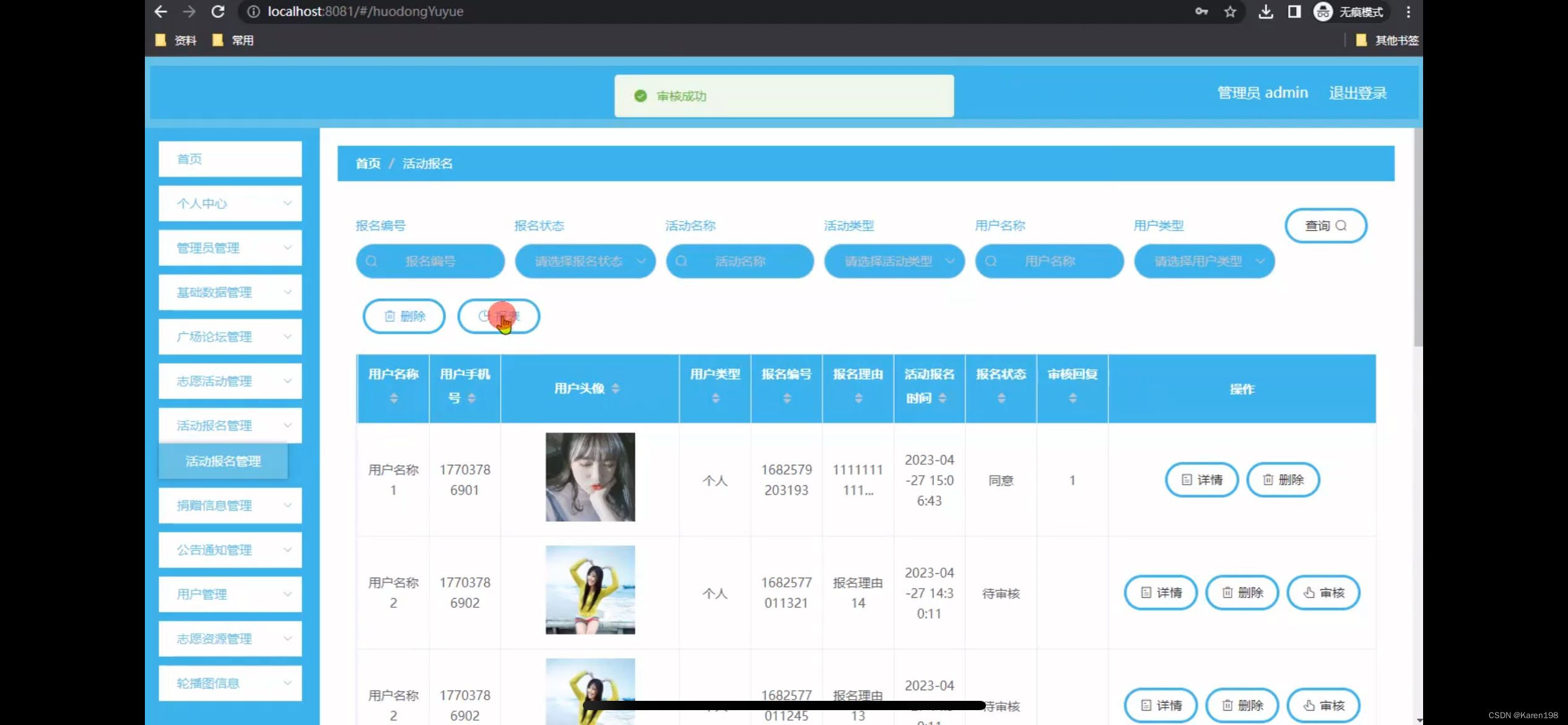
志愿服务管理系统的设计
管理员账户功能包括:系统首页,个人中心,管理员管理,基础数据管理,广场论坛管理,志愿活动管理,活动报名管理 前台账户功能包括:系统首页,个人中心,志愿活动&a…...

微信小游戏5月畅销榜,新老产品更替显著,亿级爆款频出
小游戏由于微信的平台扶持,被视为可以大力发掘的蓝海,成为国内游戏最大的增长机会之一,随着越来越多的大厂和中小厂转向了小游戏赛道,每个月的小游戏畅销榜单都有不同变化。 5月的小游戏畅销榜显示,小游戏市场正经历显…...

自己想要公开自己的学习方法,但是自己很害怕自己的学习方法是一个错误的,因为对于自己而言,专升本的机会只有一次
分享自己的学习方法可能需要一定的勇气,特别是当你担心可能会受到批评或是不被理解时。以下是一些建议,可以帮助你克服这种恐惧:(kimi编辑器自己对于这些内容的基础批注) 自我肯定:首先,认识到你…...

linux centos consul1.15.2一键安装部署
consul原理、作用、安装相关内容 一、理论部分二、安装下载版本地址三、安装consul服务 一、理论部分 1、consul的原理 Consul的原理及作用可以归纳为以下几点: ①、基于Gossip协议的通信:Consul使用了基于Gossip协议的Serf实现来进行通信。 Gossip协议…...

速盾:dns和cdn区别?
DNS(Domain Name System)和CDN(Content Delivery Network)是互联网中两个不同但相互关联的服务。下面将详细解释DNS和CDN的区别。 功能和作用: DNS:DNS是将域名转换为IP地址的服务,它充当着互联…...

多目标跟踪中用到的求解线性分配问题(Linear Assignment Problem,LAP)C++
多目标跟踪中用到的求解线性分配问题(Linear Assignment Problem,LAP)C flyfish python实现,说的比这里详细 lapjv.h和lapjv.cpp代码在https://github.com/shaoshengsong/DeepSORT C代码调用 #include <iostream> #include <ve…...
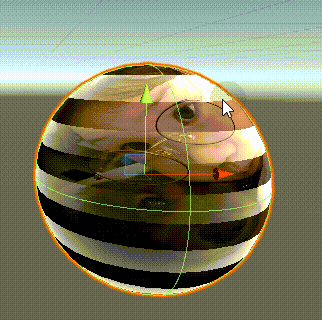
Unity | Shader基础知识(第十四集:简单效果练习)
目录 前言 一、效果预览 1.弧形边缘光 二、效果制作 1. 制作弧形边缘光 2.弧形边缘光进阶 3.弧形边缘光调节渐变范围 4.边缘光突变 5.同心圆 三、加入世界坐标做效果 1.绘制结界 2.斑马球 3.效果合并 四、作者的碎碎念 前言 有粉丝建议说,让我继续更新…...
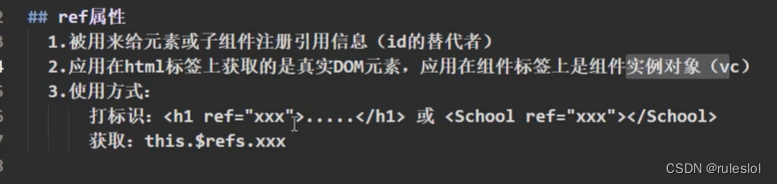
Vue48-ref属性
一、需求:操作DOM元素 1-1、使用原生的id属性 不太好! 1-2、使用 ref属性 原生HTML中,用id属性给元素打标识,vue里面用ref属性。 给哪个元素加了ref属性,vc实例对象就收集哪个元素!!࿰…...
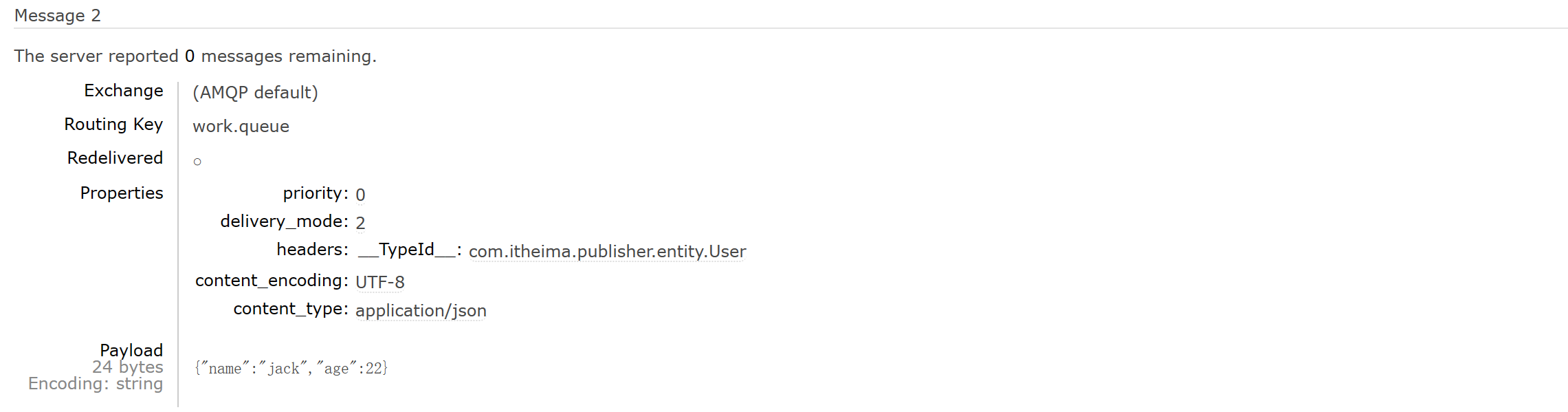
【SpringCloud学习笔记】RabbitMQ(中)
1. 交换机概述 前面《RabbitMQ上篇》我们使用SpringAMQP来演示如何用Java代码操作RabbitMQ,当时采用的是生产者直接将消息发布给队列,但是实际开发中不建议这么做,更加推荐生产者将消息发布到交换机(exchange),然后由exchange路由…...
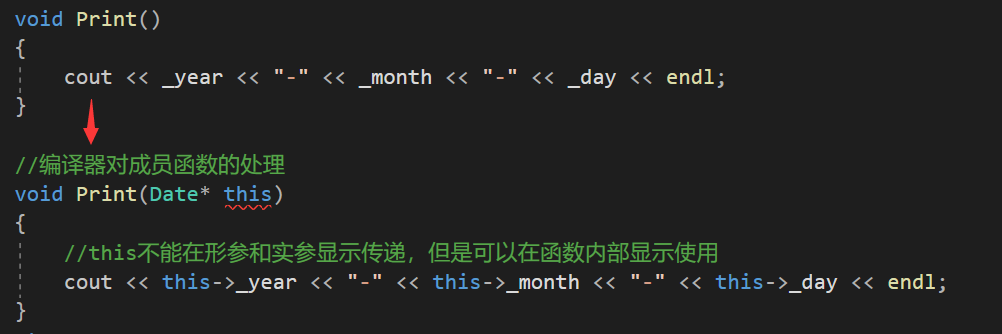
【C++】类和对象的引入
文章目录 前言一、类的定义二、类的访问控制与封装三、类的作用域四、类的实例化五、类的存储方式及大小计算六、隐藏的this指针 前言 C语言是面向过程的,关注的是过程,分析出求解问题的步骤,通过函数调用逐步解决问题。 C是基于面向对象的&…...

11.5.k8s中pod的调度-cordon,drain,delete
目录 一、概念 二、使用 1.cordon 停止调度 1.1.停止调度 1.2.解除恢复 2.drain 驱逐节点 2.1.驱逐节点 2.2.参数介绍 2.3.解除恢复 3.delete 删除节点 一、概念 cordon节点,drain驱逐节点,delete 节点,在对k8s集群节点执行维护&am…...

Java中线程的创建方式
一、继承Thread类,重写run方法 public class MyThread{public static void main(String[] args) {Thread threadDome new ThreadDome();threadDome.start();} }class ThreadDome extends Thread{Overridepublic void run() {for (int i 0; i < 5; i) {try {Th…...
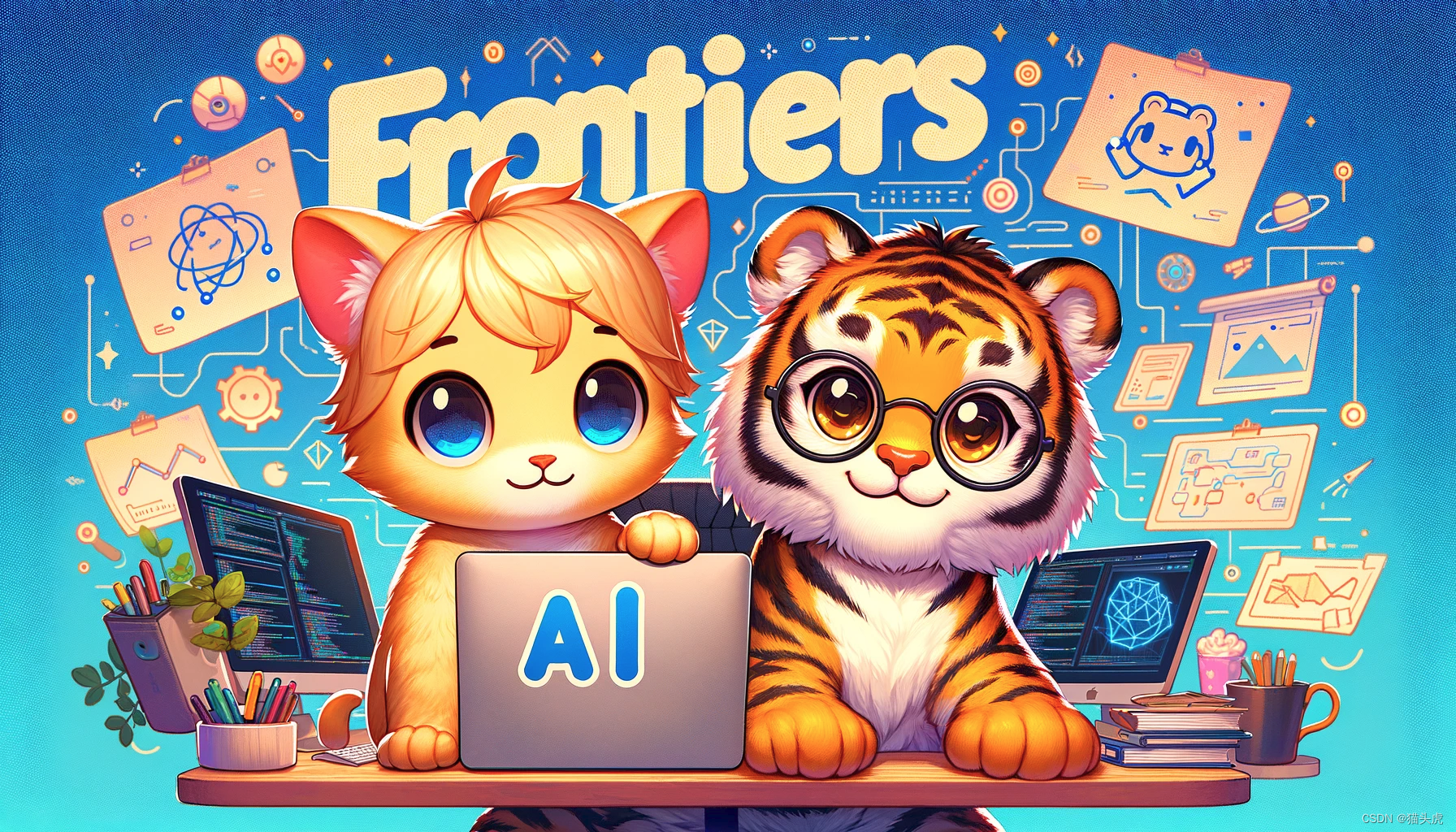
猫头虎推荐20个值得体验的通用大模型
猫头虎推荐20个值得体验的通用大模型 🚀 大家好,我是猫头虎,一名专注于科技领域的自媒体博主。今天是周一,新的开始,我们来深入探讨一下当前最值得体验的通用大模型。这些AI模型不仅功能强大,而且在各自领…...
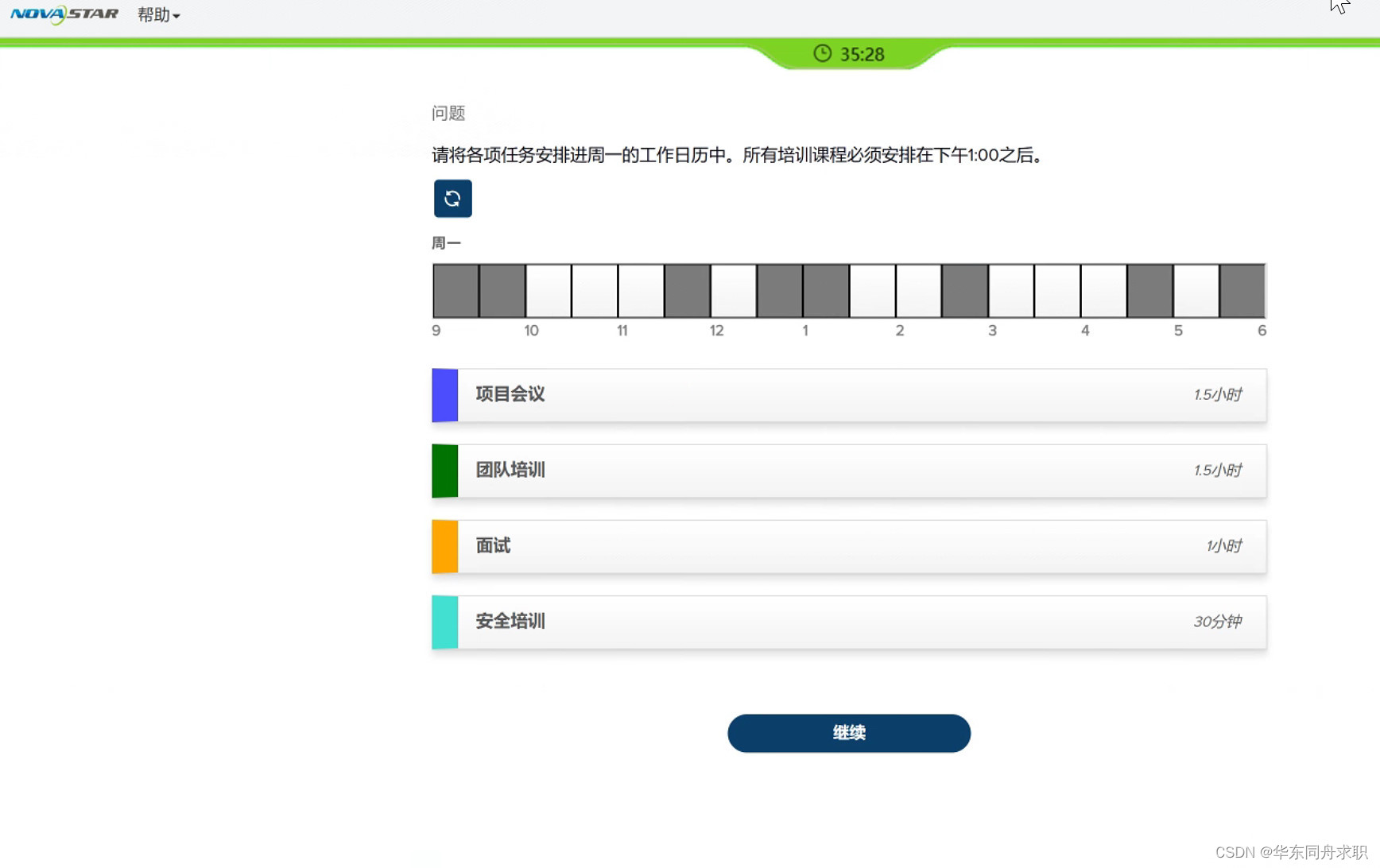
Novartis诺华制药社招综合能力性格动机问卷入职测评笔试题库答案及包过助攻
【华东同舟求职】由资深各行业从业者建立的一站式人才服务网络平台,现阶段目标是“提升全市场各行业岗位信息的流动性和透明度”。我们接受众多行业机构的直接委托发布,并尽力通过各种方法搜寻高价值岗位信息。事实上,我们以发现不为人知的优…...

Adam优化算法
Adam优化算法 Adam(Adaptive Moment Estimation)是一种用于训练深度学习模型的优化算法,由Diederik P. Kingma和Jimmy Ba在2014年提出。Adam结合了动量和自适应学习率的方法,具有高效、稳定和适应性强的特点,被广泛应…...