【笔记】效率之门——Python中的函数式编程技巧
文章目录
- Python函数式编程
- 1. 数据
- 2. 推导式
- 3. 函数式编程
- 3.1. Lambda函数
- 3.2. python内置函数
- 3.3. 高阶函数
- 4. 函数式编程的应用
Python函数式编程
我的AI Studio项目:【笔记】LearnDL第三课:Python高级编程——抽象与封装 - 飞桨AI Studio (baidu.com)
python中函数式编程的一些技巧,可以提升我们编写代码的效率,写出更加清晰且高效的代码。
开发者关心两个效率,
- 程序运行的效率:程序用更少的计算资源完成更多的任务。
- 编写代码的效率:程序员在更少的时间实现更多的功能。
随着计算机算力的不断增长,如今我们更加关心的往往是后者。
本文主要包含以下内容:
- 函数应当如何使用数据
- 使用列表推导式或字典推导式简化代码
- 使用Lambda函数,简化代码
- python中一些内置函数的使用技巧
- 什么是高阶函数
- 使用函数式编程的技巧完成一个小任务
最后一节“函数式编程的应用”中用到了一个图像数据集,在AI Studio中可以使用它。
1. 数据
- 数据的状态——尤为重要
- 不修改外部环境,不影响输入,多次调用函数不会产生不同的结果
- 避免可变的状态
- 尽量使用不可变的数据结构。例如list可变,而tuple不可变。
# 返回斐波那契数列的某一项
def fib(i):if i > 1:return fib(i - 1) + fib(i - 2)else:return 1
print(fib(5))
8
使用可变的数据结构,例如list
,有时会踩坑,例如作为函数的默认参数时,下面是一个例子。
你惊讶地发现,put(10)
和put(5)
竟然输出了一样的结果,而你单独运行put(5)
时又能得到正确的结果。
def put(n, x=[1, 1]):while len(x) < n:x.append(x[-1] + x[-2])return xprint(put(10))
print(put(5))
[1, 1, 2, 3, 5, 8, 13, 21, 34, 55]
[1, 1, 2, 3, 5, 8, 13, 21, 34, 55]
原因就是,两次调用put
函数时,默认参数[1, 1]
都是同一个对象,例如可以打印出对象的id(相当于在内存中的地址)看一下,发现两次调用put函数都在直接操作同一个list对象。
def put(n, x=[1, 1]):print(id(x))while len(x) < n:x.append(x[-1] + x[-2])return x
put(10), put(5)
140357520173648
140357520173648
([1, 1, 2, 3, 5, 8, 13, 21, 34, 55], [1, 1, 2, 3, 5, 8, 13, 21, 34, 55])
而使用不可变的数据对象作为函数的默认参数,就不会出现该问题。之前因为默认参数list本身也是一个对象实例,“可变”就是可以被篡改;而元组对象本身是无法修改的,后面代码怎么写都不会影响默认参数的值。
下面的例子中,你发现两次调用时元组x的id还是相同的,但是转换成的list的id就不同了,put(5)
也得到了正确的结果。
def put(n, x=(1, 1)):print("tuple:", id(x))x = list(x)print("list: ", id(x))while len(x) < n:x.append(x[-1] + x[-2])return x
put(10), put(5)
tuple: 140357563320112
list: 140357519739872
tuple: 140357563320112
list: 140357519346416
([1, 1, 2, 3, 5, 8, 13, 21, 34, 55], [1, 1, 2, 3, 5])
2. 推导式
列表推导式与字典推导式,可以提升我们编码的效率,以及程序运行的效率。
例如前面的put
函数,使用推导式,函数体仅需一行代码,而且代码逻辑也清晰易懂。
# 列表推导式
def put(n):return [fib(i) for i in range(n)]
print(put(5))# 字典推导式
def put(n):return {i:fib(i) for i in range(n)}
print(put(5))
[1, 1, 2, 3, 5]
{0: 1, 1: 1, 2: 2, 3: 3, 4: 5}
3. 函数式编程
善用一些函数式编程技巧,提高编程效率。
- Lambda函数
- python内置函数
- sum:求和
- all:有一个None就返回False
- any:有一个不是None,就返回True
- reduce:自定义求和方式
- 高阶函数
3.1. Lambda函数
Lambda定义的函数,与def
定义的函数是等价的,但是在形式上更简单。
f = lambda a, b : a + b
print(f(1, 2))def f(a, b):return a + b
print(f(1, 2))
3
3
Lambda与其它迭代器对象结合使用,有时会十分方便。例如,生成一个完全平方数列:
# 生成一个完全平方数列
x = list(map(lambda x : x * x, range(11)))
print(x)
# 找到数列中以‘1’结尾的数字,组成一个新的数列
x = list(filter(lambda x : str(x).endswith("1"), x))
print(x)
[0, 1, 4, 9, 16, 25, 36, 49, 64, 81, 100]
[1, 81]
3.2. python内置函数
关于python内置函数,下面给出几个示例代码。其中all
和any
常用于有效数据的筛选。
x = [1, 2, 3, 4, 5]
print(sum(x))
x = [1, 2, 3, None, 5]
print(all(x))
x = [None, None, 3, None, None]
print(any(x))
x = ['a', 'b', 'c', 'd']
reduce(lambda x, y : x * 2 + y, x)
15
False
True
'aabaabcaabaabcd'
reduce可能看起来不太好懂,其实这里的“求和”对于字符串而言就是“连接”,x作为之前的和传入,y就是下一项,(其实和sum的求和还是很相似的,可以仔细比较一下)。过程如下:
- a * 2 + b = aab
- aab * 2 + c = aabaabc
- aabaabc * 2 + d = aabaabcaabaabcd
3.3. 高阶函数
在python中,函数其实可以玩得挺花。例如,函数可以作为返回值,也可以作为函数的参数。
# 1. 函数作为返回值
# 这里例子中,函数k()的定义受函数f()的控制
def f(n):def k(x):return n * xreturn kF = f(3)
print(F(2)) # 2. 函数作为参数
def F(x, f):x = f(x)return x * x
print(F(1, lambda x : x + 1))
6
4
4. 函数式编程的应用
对于一个任务,先梳理需求,把共性、重复的地方抽象出来,再统一安排。
例如下面的任务:
- 将两个image文件夹归类至dataset/image文件夹,并将文件按照顺序重新编号
待处理数据集结构:
- PlantSegmentationDatasets- vinecuttings- images- masks- weeds- weed50images- weed50masks
images
文件夹中都是".JPG"后缀的文件,weed50images
中既有".JPG"也有".png"。
首先,我们要得到待复制的所有文件,
dir_1 = "/home/aistudio/PlantSegmentationDatasets/vinecuttings/images/"
dir_2 = "/home/aistudio/PlantSegmentationDatasets/weeds/weed50images/"# 1. glob模糊匹配
paths = glob(dir_1 + "*.JPG")
for path in paths[:5]:print(path)# 2. 列表推导式 --> 匹配多种文件后缀
# 此时得到了一个两层嵌套的列表 [[], [], [], []]
ends = ["*.JPG", "*.jpg", "*.png", "*.jpeg"]
paths = [glob(dir_2 + end) for end in ends]
print(paths)# 3. reduce(求和) --> 拼接嵌套列表为一层
paths = reduce(lambda x, y : x + y, paths)
for path in paths[:5]:print(path)
输出:
输出内容过长, 已自动对输出内容进行截断
/home/aistudio/PlantSegmentationDatasets/vinecuttings/images/DSC00149.JPG
/home/aistudio/PlantSegmentationDatasets/vinecuttings/images/DSC00241.JPG
/home/aistudio/PlantSegmentationDatasets/vinecuttings/images/DSC00248.JPG
/home/aistudio/PlantSegmentationDatasets/vinecuttings/images/DSC00214.JPG
/home/aistudio/PlantSegmentationDatasets/vinecuttings/images/DSC00200.JPG[['/home/aistudio/PlantSegmentationDatasets/weeds/weed50images/08192020_NikonD3300_YL_SpurredAnoda_0026.JPG', '/home/aistudio/PlantSegmentationDatasets/weeds/weed50images/08192020_NikonD3300_YL_SpurredAnoda_035.JPG'], [], ['/home/aistudio/PlantSegmentationDatasets/weeds/weed50images/08192020_NikonD3300_YL_Carpetweed_0035-YuzhenLu(C).png', '/home/aistudio/PlantSegmentationDatasets/weeds/weed50images/08192020_NikonD3300_YL_Carpetweed_0021-YuzhenLu(B).png', '/home/aistudio/PlantSegmentationDatasets/weeds/weed50images/08192020_NikonD3300_YL_Carpetweed_0031-YuzhenLu.png', '/home/aistudio/PlantSegmentationDatasets/weeds/weed50images/08192020_NikonD3300_YL_Carpetweed_0035-YuzhenLu(A).png', '/home/aistudio/PlantSegmentationDatasets/weeds/weed50images/08192020_NikonD3300_YL_Carpetweed_0017-YuzhenLu(B).png', '/home/aistudio/PlantSegmentationDatasets/weeds/weed50images/08192020_NikonD3300_YL_Carpetweed_0042-YuzhenLu.png', '/home/aistudio/PlantSegmentationDatasets/weeds/weed50images/08192020_Nikon/home/aistudio/PlantSegmentationDatasets/weeds/weed50images/08192020_NikonD3300_YL_SpurredAnoda_0026.JPG
/home/aistudio/PlantSegmentationDatasets/weeds/weed50images/08192020_NikonD3300_YL_SpurredAnoda_035.JPG
/home/aistudio/PlantSegmentationDatasets/weeds/weed50images/08192020_NikonD3300_YL_Carpetweed_0035-YuzhenLu(C).png
/home/aistudio/PlantSegmentationDatasets/weeds/weed50images/08192020_NikonD3300_YL_Carpetweed_0021-YuzhenLu(B).png
/home/aistudio/PlantSegmentationDatasets/weeds/weed50images/08192020_NikonD3300_YL_Carpetweed_0031-YuzhenLu.png
下面是完整的代码:
# 将in_dirs里每个目录的图片,分别复制到同一个out_dir中,并为它们重新编号
def copy_dir(in_dirs, out_dir):!mkdir $out_dir # 创建文件夹for j, in_dir in enumerate(in_dirs):ends = ["*.JPG", "*.png", "*.jpg", "*.jpeg"]paths = reduce(lambda x, y : x + y, [glob(in_dir + end) for end in ends])for i, in_filename in enumerate(paths):out_filename = out_dir + str(j) + "_" + str(i) + ".jpg"print(out_filename)!cp $in_filename $out_filename # 复制图片dir_1 = "/home/aistudio/PlantSegmentationDatasets/vinecuttings/images/"
dir_2 = "/home/aistudio/PlantSegmentationDatasets/weeds/weed50images/"
out_dir = "/home/aistudio/data/image/"
in_dirs = [dir_1, dir_2]
copy_dir(in_dirs, out_dir)
更多的应用,就以后在实践中慢慢摸索咯。
完
相关文章:
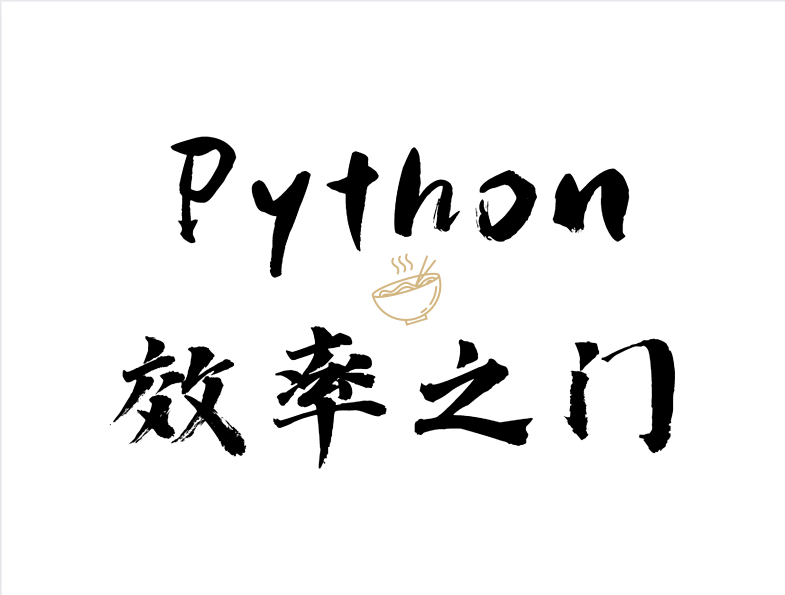
【笔记】效率之门——Python中的函数式编程技巧
文章目录Python函数式编程1. 数据2. 推导式3. 函数式编程3.1. Lambda函数3.2. python内置函数3.3. 高阶函数4. 函数式编程的应用Python函数式编程 我的AI Studio项目:【笔记】LearnDL第三课:Python高级编程——抽象与封装 - 飞桨AI Studio (baidu.com) p…...
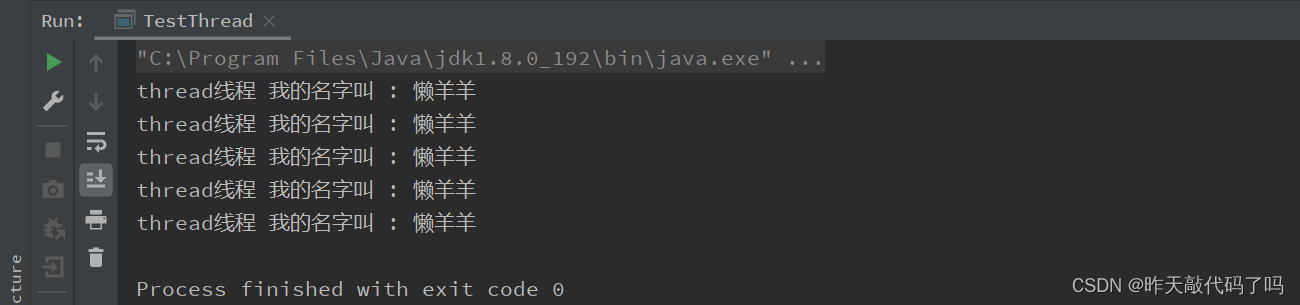
Java【多线程基础2】 Thread类 及其常用方法
文章目录前言一、Thread类1, 构造方法2, 常用成员属性3, 常用成员方法3.1, start 启动线程3.2, interrupt 中断线程 (重点)3.2.1, 手动设置标记位3.2.2, 使用内置标记位3.3.3, interrupt 方法 的作用3.3 sleep 休眠线程3.4, jion 等待线程3.5 获取当前线程的引用总结前言 各位读…...

JVM调优实战及常量池详解
目录 阿里巴巴Arthas详解 Arthas使用场景 Arthas使用 GC日志详解 如何分析GC日志 CMS G1...
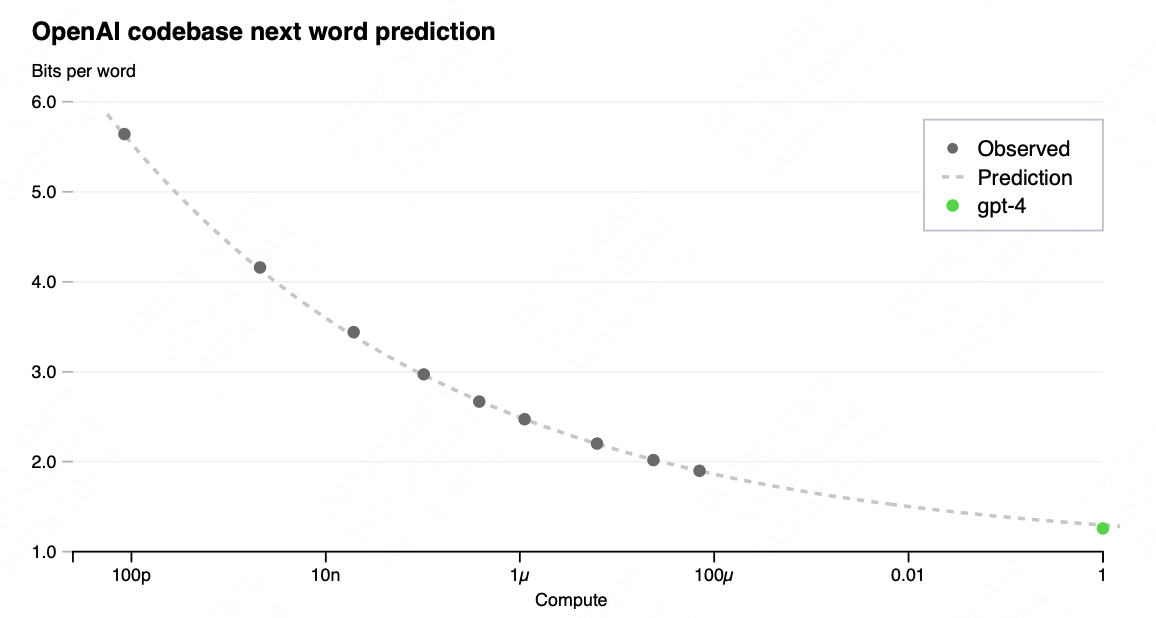
ChatGPT研究分析:GPT-4做了什么
前脚刚研究了一轮GPT3.5,OpenAI很快就升级了GPT-4,整体表现有进一步提升。追赶一下潮流,研究研究GPT-4干了啥。本文内容全部源于对OpenAI公开的技术报告的解读,通篇以PR效果为主,实际内容不多。主要强调的工作…...
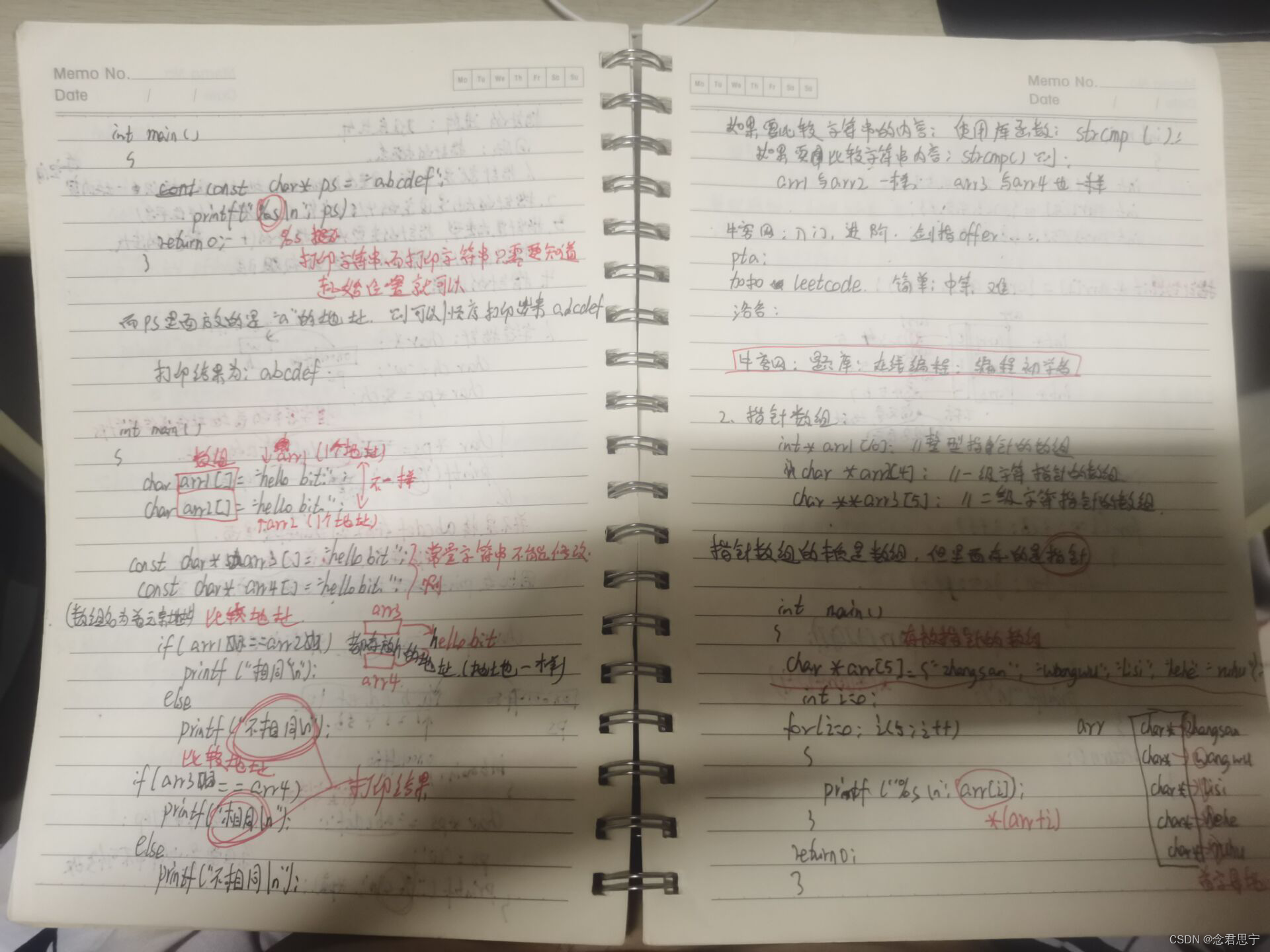
我为什么要写博客,写博客的意义是什么??
曾经何时我也不知道,怎样才能变成我自己所羡慕的大佬!!在一次次的CSDN阅读的过程中,结实了许多志同道合的人!!包过凉哥,擦姐……大佬,但是,很遗憾,与这些人只…...
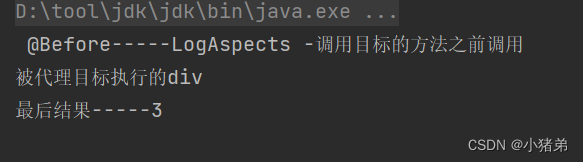
ssm框架之spring:浅聊AOP
AOP(Aspect Oriented Programming),是一种设计思想。先看一下百度百科的解释: 在软件业,AOP为Aspect Oriented Programming的缩写,意为:面向切面编程,通过预编译方式和运行期间动态…...

k8s详解
一、k8s中的yaml文件 JSON格式:主要用于api接口之间信息的传递YAML格式:主要用于配置和管理,YAML是一种简洁的非标记性语言,内容格式人性化 YAML格式: 大小写敏感使用缩进代表层级关系,不支持TAB制表符缩…...

计算机操作系统(第四版)第一章操作系统引论 1.1操作系统的目标和作用
第一章操作系统引论 1.1操作系统的目标和作用 什么是操作系统OS? 配置在计算机硬件上的第一层软件是对硬件的首次扩充。 是最重要的系统软件,其他系统软件应用软件都依赖于操作系统的支持。 操作系统主要作用? 管理计算机系统所有硬件设…...

git push解决办法: ! [remote rejected] master -> master (pre-receive hook declined)
项目经理远程创建了一个空项目,无任何内容,给我赋予的developer账号权限,本地改为后提交代码试了很多次都上传不上去,报错如下: ! [remote rejected] master -> master (pre-receive hook declined)先说结果&#x…...
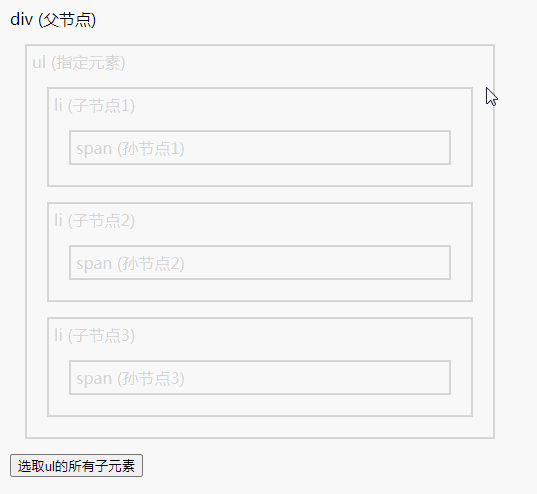
jQuery 遍历方法总结
遍历方法有:1、add(),用于把元素添加到匹配元素的集合中;2、children(),用于返回被选元素的所有直接子元素;3、closest(),用于返回被选元素的第一个祖先元素;4、contents(),用于返回…...

OKHttp 源码解析(二)拦截器
游戏SDK架构设计之代码实现——网络框架 OKHttp 源码解析(一) OKHttp 源码解析(二)拦截器 前言 上一篇解读了OKHttp 的基本框架源码,其中 OKHttp 发送请求的核心是调用 getResponseWithInterceptorChain 构建拦截器链…...
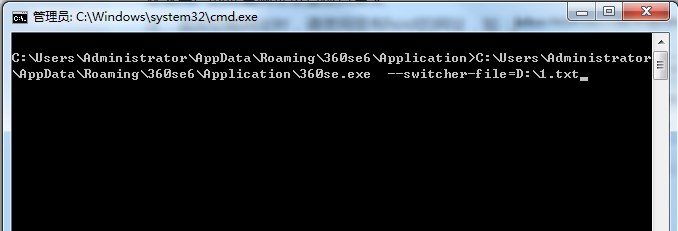
如何修改设置浏览器内核模式
优先级: 强制锁定极速模式 >手动切换(用户)>meta指定(开发者)>浏览器兼容列表(浏览器) 需要用360安全浏览器14,chromium108内核,下载地址https://bbs.360.cn/t…...

30个Python常用小技巧
1、原地交换两个数字 1 2 3 4 x, y 10, 20 print(x, y) y, x x, y print(x, y) 10 20 20 10 2、链状比较操作符 1 2 3 n 10 print(1 < n < 20) print(1 > n < 9) True False 3、使用三元操作符来实现条件赋值 [表达式为真的返回值] if [表达式] else [表达式…...

ubuntu解决中文乱码
1、查看当前系统使用的字符编码 ~$ locale LANGen_US LANGUAGEen_US: LC_CTYPE"en_US" LC_NUMERIC"en_US" LC_TIME"en_US" LC_COLLATE"en_US" LC_MONETARY"en_US" LC_MESSAGES"en_US" LC_PAPER"en_US" …...

2022年全国职业院校技能大赛(中职组)网络安全竞赛试题——MYSQL安全测试解析(详细)
B-3任务三:MYSQL安全测试 *任务说明:仅能获取Server3的IP地址 1.利用渗透机场景kali中的工具确定MySQL的端口,将MySQL端口作为Flag值提交; 2.管理者曾在web界面登陆数据库,并执行了select <?php echo \<pre>\;system($_GET[\cmd\]); echo \</pre>\; ?…...

C++ map和unordered_map的区别
unordered_map 类模板和 map 类模板都是描述了这么一个对象:它是由 std::pair<const Key, value> 组成的可变长容器; 这个容器中每个元素存储两个对象,也就是 key - value 对。 1. unordered_map 在头文件上,引入 <unor…...

BCSP-玄子JAVA开发之JAVA数据库编程CH-04_SQL高级(二)
BCSP-玄子JAVA开发之JAVA数据库编程CH-04_SQL高级(二) 4.1 IN 4.1.1 IN 子查询 如果子查询的结果为多个值,就会导致代码报错解决方案就是使用 IN 关键字,将 替换成 IN SELECT …… FROM 表名 WHERE 字段名 IN (子查询);4.1.…...
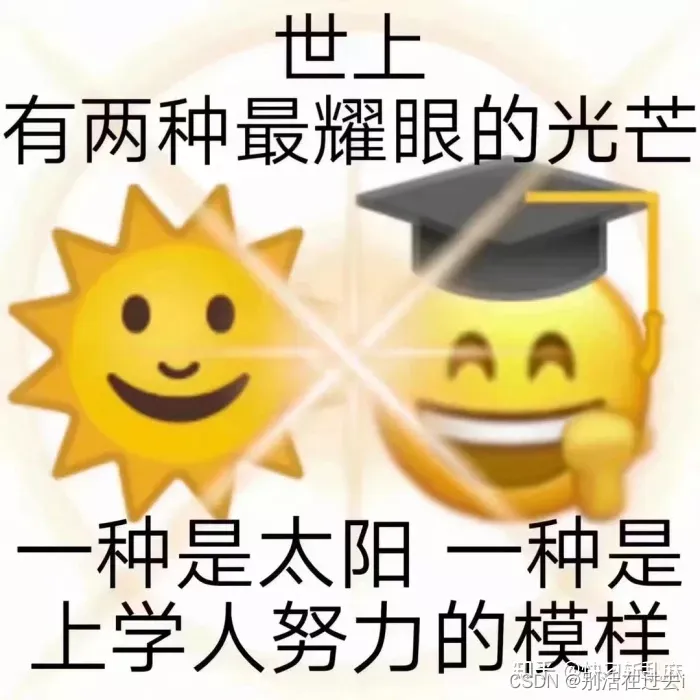
学习java——②面向对象的三大特征
目录 面向对象的三大基本特征 封装 封装demo 继承 继承demo 多态 面向对象的三大基本特征 我们说面向对象的开发范式,其实是对现实世界的理解和抽象的方法,那么,具体如何将现实世界抽象成代码呢?这就需要运用到面向对象的三大…...
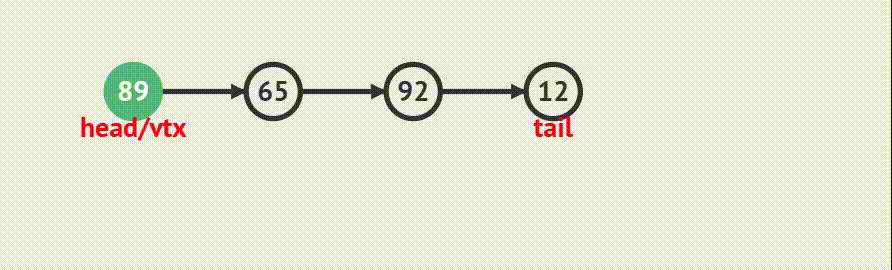
初阶数据结构 - 【单链表】
目录 前言: 1.概念 链表定义 结点结构体定义 结点的创建 2.链表的头插法 动画演示 代码实现 3.链表的尾插 动画演示 代码实现 4.链表的头删 动画演示 代码实现 5.链表的尾删 动画演示 代码实现 6.链表从中间插入结点 动画演示 代码实现 7.从单…...
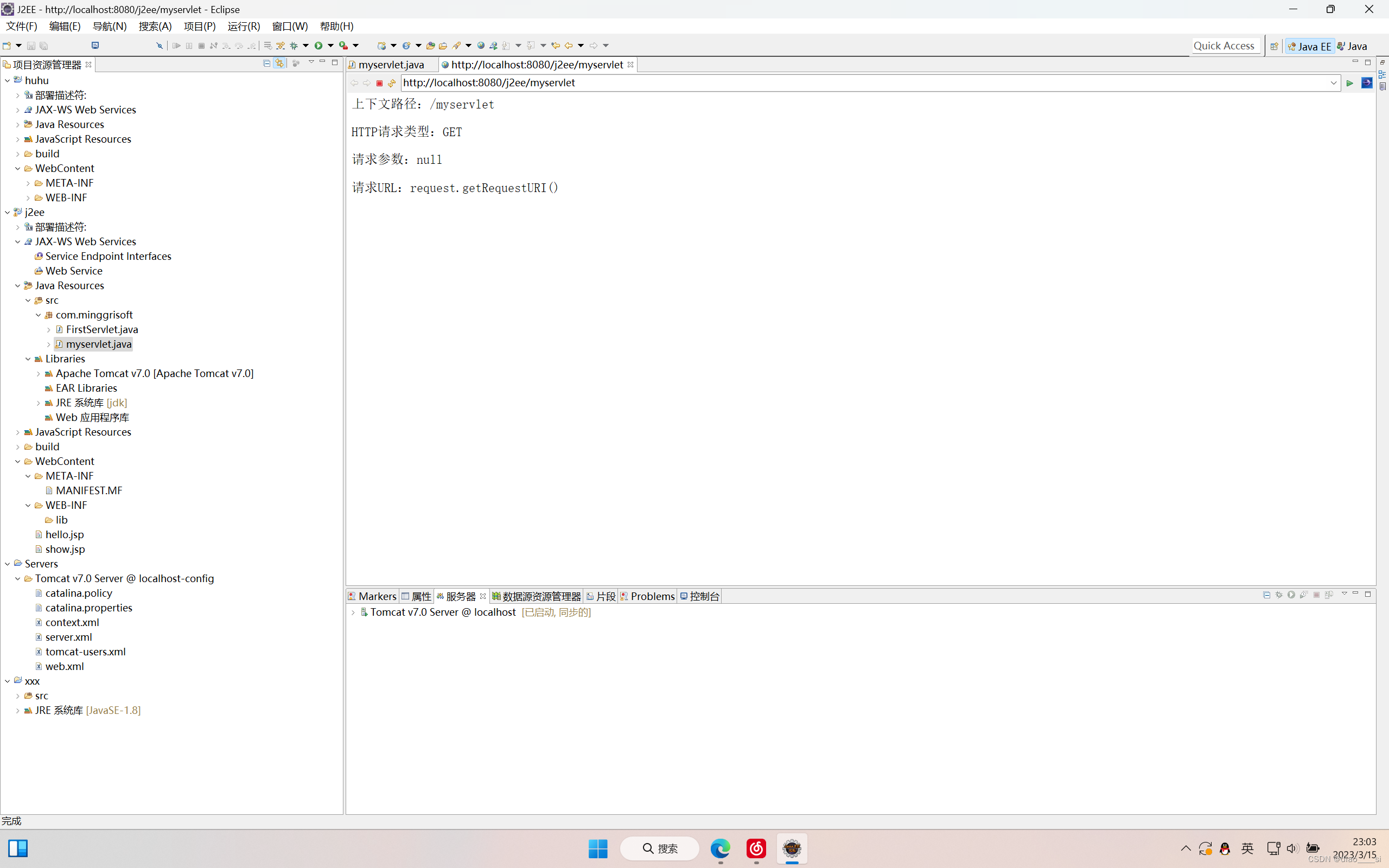
第五周作业、第一次作业(1.5个小时)、练习一
一、创建servlet的过程没有太多好说的,唯一需要注意的就是:旧版本的servlet确实需要手动配置web.xml文件,但是servlet2.5以后,servlet的配置直接在Java代码中进行注解配置。我用的版本就不再需要手动去配置web.xml文件了,所以我只…...
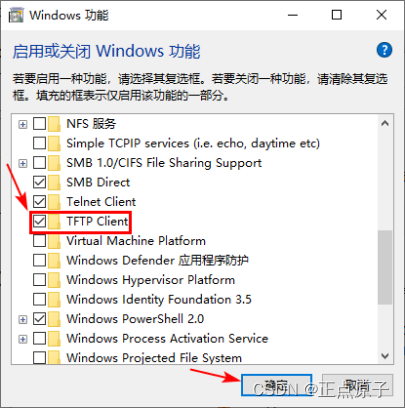
【正点原子FPGA连载】 第三十三章基于lwip的tftp server实验 摘自【正点原子】DFZU2EG_4EV MPSoC之嵌入式Vitis开发指南
第三十三章基于lwip的tftp server实验 文件传输是网络环境中的一项基本应用,其作用是将一台电子设备中的文件传输到另一台可能相距很远的电子设备中。TFTP作为TCP/IP协议族中的一个用来在客户机与服务器之间进行文件传输的协议,常用于无盘工作站、路由器…...

蓝桥冲刺31天之316
如果生活突然向你发难 躲不过那就迎面而战 所谓无坚不摧 是能享受最好的,也能承受最坏的 大不了逢山开路,遇水搭桥 若你决定灿烂,山无遮,海无拦 A:特殊日期 问题描述 对于一个日期,我们可以计算出年份的各个…...
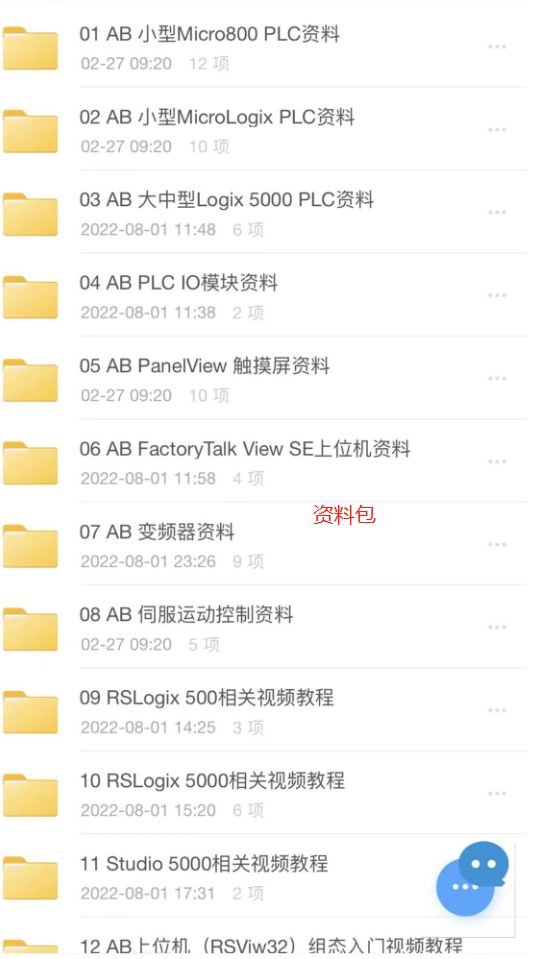
说一个通俗易懂的PLC工程师岗位要求
你到了一家新的单位,人家接了一套新的设备,在了解设备工艺流程之后,你就能决定用什么电气元件,至少95%以上电气原件不论你用过没用过都有把握拍板使用,剩下5%,3%你可以先买来做实验,这次不能用&…...

今年还能学java么?
“Java很卷”、“大家不要再卷Java了”,经常听到同学这样抱怨。但同时,Java的高薪也在吸引越来越多的同学。不少同学开始疑惑:既然Java这么卷,还值得我入行吗? 首先先给你吃一颗定心丸:现在选择Java依然有…...

ajax学习1
不刷新页面的情况下,向服务端发送请求,异步的js和XMLajax不是新的编程语言,只是把现有标准组合到一起使用的新方式...

一题多解-八数码(万字长文)
16 张炜皓 (ζ͡顾念̶) LV 5 1 周前 在做这道题前,先来认识一下deque双端队列 C STL 中的双端队列 题目连接 使用前需要先引入 头文件。 #include; STL 中对 deque 的定义 // clang-format off template< class T, class Allocator std::allocator class d…...
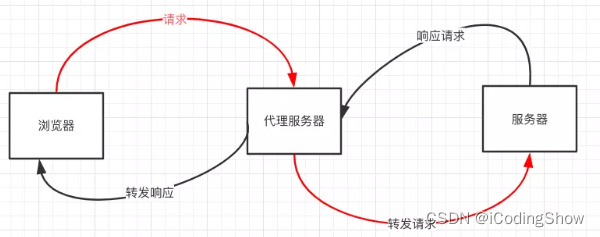
九种跨域方式实现原理(完整版)
前言 前后端数据交互经常会碰到请求跨域,什么是跨域,以及有哪几种跨域方式,这是本文要探讨的内容。 一、什么是跨域? 1.什么是同源策略及其限制内容? 同源策略是一种约定,它是浏览器最核心也最基本的安…...
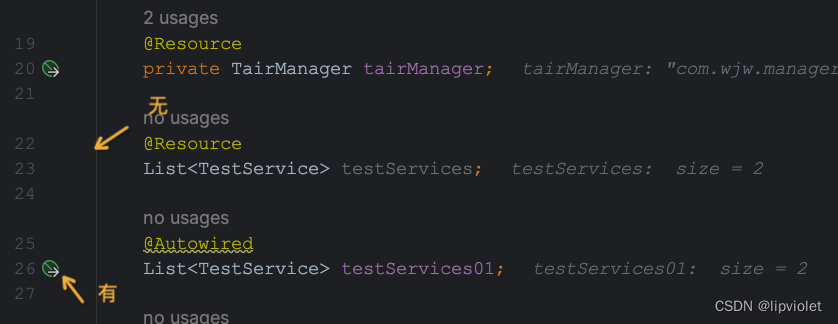
fighting
目录Mysqlgroup by和 distinct哪个性能好java觉得Optional类怎么样isEmpty和isBlank的用法区别使用大对象时需要注意什么内存溢出和内存泄漏的区别及详解SpringResource和Autowired的起源既生“Resource”,何生“Autowired”使用Autowired时为什么Idea会曝出黄色警告…...

网络安全日志监控管理
内部安全的重要性 无论大小,每个拥有IT基础设施的组织都容易发生内部安全攻击。您的损失等同于黑客的收益:访问机密数据、滥用检索到的信息、系统崩溃,以及其他等等。专注于网络外部的入侵是明智的,但同时,内部安全也…...
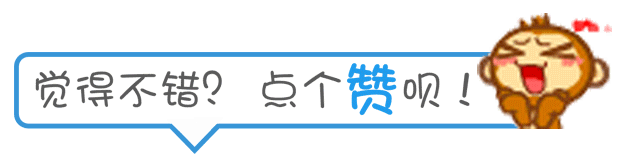
线程池的使用:如何写出高效的多线程程序?
目录1.线程池的使用2.编写高效的多线程程序Java提供了Executor框架来支持线程池的实现,通过Executor框架,可以快速地创建和管理线程池,从而更加方便地编写多线程程序。 1.线程池的使用 在使用线程池时,需要注意以下几点ÿ…...