MyBatis3-获取参数值的方式、查询功能及特殊SQL执行
目录
准备工作
获取参数值的方式(重点)
查询功能
查询一个实体类对象
查询一个list集合
查询单个数据
查询一条数据为map集合
查询多条数据为map集合
特殊SQL执行
模糊查询
批量删除
动态设置表名
添加功能获取自增的主键
准备工作
模块Mybatis_demo2
mybatis-config.xml:
<?xml version="1.0" encoding="UTF-8" ?>
<!DOCTYPE configurationPUBLIC "-//mybatis.org//DTD Config 3.0//EN""http://mybatis.org/dtd/mybatis-3-config.dtd">
<configuration><properties resource="jdbc.properties"/><!--类型别名,不区分大小写--><typeAliases><package name="com.qcby.pojo"/></typeAliases><environments default="development"><environment id="development"><transactionManager type="JDBC"/><dataSource type="POOLED"><!--设置连接数据库的驱动--><property name="driver" value="${jdbc.driver}"/><!--设置连接数据库的连接地址--><property name="url" value="${jdbc.url}"/><!--设置连接数据库的用户名--><property name="username" value="${jdbc.username}"/><!--设置连接数据库的密码--><property name="password" value="${jdbc.password}"/></dataSource></environment></environments><!--引入映射文件--><mappers><mapper resource="mapper/ParameterMapper.xml"/></mappers>
</configuration>
jdbc.properties:
jdbc.driver=com.mysql.cj.jdbc.Driver
jdbc.url=jdbc:mysql://localhost:3306/mybatis1028
jdbc.username=root
jdbc.password=123456
实体类:
package com.qcby.pojo;public class User {private Integer id;private String username;private String password;private Integer age;private String sex;private String email;public User() {}public User(Integer id, String username, String password, Integer age, String sex, String email) {this.id = id;this.username = username;this.password = password;this.age = age;this.sex = sex;this.email = email;}public Integer getId() {return id;}public void setId(Integer id) {this.id = id;}public String getUsername() {return username;}public void setUsername(String username) {this.username = username;}public String getPassword() {return password;}public void setPassword(String password) {this.password = password;}public Integer getAge() {return age;}public void setAge(Integer age) {this.age = age;}public String getSex() {return sex;}public void setSex(String sex) {this.sex = sex;}public String getEmail() {return email;}public void setEmail(String email) {this.email = email;}@Overridepublic String toString() {return "User{" +"id=" + id +", username='" + username + '\'' +", password='" + password + '\'' +", age=" + age +", sex='" + sex + '\'' +", email='" + email + '\'' +'}';}
}
封装SqlSession工具类:
package com.qcby.utils;import org.apache.ibatis.io.Resources;
import org.apache.ibatis.session.SqlSession;
import org.apache.ibatis.session.SqlSessionFactory;
import org.apache.ibatis.session.SqlSessionFactoryBuilder;import java.io.IOException;
import java.io.InputStream;public class SqlSessionUtils {public static SqlSession getSqlSession(){SqlSession sqlSession = null;try {InputStream is = Resources.getResourceAsStream("mybatis-config.xml");SqlSessionFactory sqlSessionFactory = new SqlSessionFactoryBuilder().build(is);sqlSession = sqlSessionFactory.openSession(true);} catch (IOException e) {e.printStackTrace();}return sqlSession;}
}
ParameterMapper:
package com.qcby.mapper;import com.qcby.pojo.User;
import org.apache.ibatis.annotations.Mapper;import java.util.List;@Mapper
public interface ParameterMapper {/*** 查询所有的员工*/List<User> getAllUser();
}
ParameterMapperxml:
<?xml version="1.0" encoding="UTF-8"?>
<!DOCTYPE mapperPUBLIC "-//mybatis.org//DTD Mapper 3.0//EN""http://mybatis.org/dtd/mybatis-3-mapper.dtd">
<mapper namespace="com.qcby.mapper.ParameterMapper"><!--查询所有--><select id="getAllUser" resultType="com.qcby.pojo.User">select * from t_user</select>
</mapper>
测试类:
package com.qcby;import com.qcby.mapper.ParameterMapper;
import com.qcby.pojo.User;
import com.qcby.utils.SqlSessionUtils;
import org.apache.ibatis.session.SqlSession;
import org.junit.Test;import java.util.List;public class ParameterMapperTest {@Testpublic void testGetAllUser(){SqlSession sqlSession = SqlSessionUtils.getSqlSession();ParameterMapper mapper = sqlSession.getMapper(ParameterMapper.class);List<User> list = mapper.getAllUser();for (User user : list) {System.out.println(user);}}}
获取参数值的方式(重点)
MyBatis获取参数值的两种方式:${}和#{}
${}的本质就是字符串拼接,#{}的本质就是占位符赋值
${}使用字符串拼接的方式拼接sql,若为字符串类型或日期类型的字段进行赋值时,需要手动加单引号;但是#{}使用占位符赋值的方式拼接sql,此时为字符串类型或日期类型的字段进行赋值时,可以自动添加单引号
1.单个字面量类型的参数
若mapper接口中的方法参数为单个的字面量类型,此时可以使用${}和#{}以任意的名称获取参数的值,可以是aaa,建议与变量名保持一致,注意${}需要手动加单引号
#{}:
/*** 根据用户名查询用户信息*/User getUserByUserName(String username);
<select id="getUserByUserName" resultType="com.qcby.pojo.User">select * from t_user where username = #{username}</select>
@Testpublic void getUserByUserName(){SqlSession sqlSession = SqlSessionUtils.getSqlSession();ParameterMapper mapper = sqlSession.getMapper(ParameterMapper.class);User user = mapper.getUserByUserName("admin");System.out.println(user);}
${}:
<select id="getUserByUserName" resultType="com.qcby.pojo.User">select * from t_user where username = '${username}'</select>
2.多个字面量类型的参数
若mapper接口中的方法参数为多个时,此时MyBatis会自动将这些参数放在一个map集合中,以arg0,arg1...为键,以参数为值;以 param1,param2...为键,以参数为值;因此只需要通过${}和#{}访问map集合的键就可以获取相对应的值,注意${}需要手动加单引号
#{}:
/*** 验证登录*/User checkLogin(String username,String password);
<select id="checkLogin" resultType="com.qcby.pojo.User">select * from t_user where username = #{arg0} and password = #{arg1}</select>
@Testpublic void checkLogin(){SqlSession sqlSession = SqlSessionUtils.getSqlSession();ParameterMapper mapper = sqlSession.getMapper(ParameterMapper.class);User user = mapper.checkLogin("admin","123456");System.out.println(user);}
${}:
<select id="checkLogin" resultType="com.qcby.pojo.User">select * from t_user where username = '${arg0}' and password = '${arg1}'</select>
3.map集合类型的参数
若mapper接口中的方法需要的参数为多个时,此时可以手动创建map集合,将这些数据放在map中,只需要通过${}和#{}访问map集合的键就可以获取相对应的值,注意${}需要手动加单引号
User checkLoginMap(Map<String,Object> map);
<select id="checkLoginMap" resultType="com.qcby.pojo.User">select * from t_user where username = #{username} and password = #{password}</select>
@Testpublic void checkLoginMap(){SqlSession sqlSession = SqlSessionUtils.getSqlSession();ParameterMapper mapper = sqlSession.getMapper(ParameterMapper.class);Map<String,Object> map = new HashMap<>();map.put("username","admin");map.put("password","123456");User user = mapper.checkLoginMap(map);System.out.println(user);}
4.实体类类型的参数
若mapper接口中的方法参数为实体类对象时,此时可以使用${}和#{},通过访问实体类对象中的属性名获取属性值,注意${}需要手动加单引号
/*** 添加用户信息*/int insertUser(User user);
<insert id="insertUser">insert into t_user values (null ,#{username},#{password},#{age},#{sex},#{email})</insert>
@Testpublic void insert(){SqlSession sqlSession = SqlSessionUtils.getSqlSession();ParameterMapper mapper = sqlSession.getMapper(ParameterMapper.class);int result = mapper.insertUser(new User(null, "李四", "123", 23, "男", "123@qq.com"));System.out.println(result);}
5.使用@Param标识参数
可以通过@Param注解标识mapper接口中的方法参数
此时,会将这些参数放在map集合中,以@Param注解的value属性值为键,以参数为值;以param1,param2...为键,以参数为值;只需要通过${}和#{}访问map集合的键就可以获取相对应的值, 注意${}需要手动加单引号
User checkLoginByParam(@Param("username") String username, @Param("password") String password);
<select id="checkLoginByParam" resultType="com.qcby.pojo.User">select * from t_user where username = #{username} and password = #{password}</select>
@Testpublic void checkLoginByParam(){SqlSession sqlSession = SqlSessionUtils.getSqlSession();ParameterMapper mapper = sqlSession.getMapper(ParameterMapper.class);User user = mapper.checkLoginByParam("admin", "123456");System.out.println(user);}
查询功能
若查询出的数据只有一条,可以通过实体类对象或者集合接收
若查询出的数据有多条,可以通过实体类类型的list集合接收;可以通过map类型的list集合接收;可以在mapper接口的方法上添加@MapKey注解,此时就可以将每条数据转换的map集合作为值,以某个字段的值作为键,放在同一个map集合中
查询一个实体类对象
/*** 根据id查询用户信息*/User getUserById(@Param("id") Integer id);
<select id="getUserById" resultType="com.qcby.pojo.User">select * from t_user where id = #{id}</select>
@Testpublic void getUserById() {SqlSession sqlSession = SqlSessionUtils.getSqlSession();SelectMapper mapper = sqlSession.getMapper(SelectMapper.class);System.out.println(mapper.getUserById(3));}
查询一个list集合
/*** 查询所有的用户信息*/List<User> getAllUser();
<select id="getAllUser" resultType="com.qcby.pojo.User">select * from t_user</select>
@Testpublic void getAllUser() {SqlSession sqlSession = SqlSessionUtils.getSqlSession();SelectMapper mapper = sqlSession.getMapper(SelectMapper.class);List<User> users = mapper.getAllUser();for (User user : users) {System.out.println(user);}}
查询单个数据
/*** 查询用户的总记录数*/
Integer getCount();
在MyBatis中,对于Java中常用的类型都设置了类型别名* 例如:java.lang.Integer-->int,integer* 例如:int-->_int,_integer* 例如:Map-->map,List-->list <select id="getCount" resultType="java.lang.Integer">select count(*) from t_user</select>
@Testpublic void getCount() {SqlSession sqlSession = SqlSessionUtils.getSqlSession();SelectMapper mapper = sqlSession.getMapper(SelectMapper.class);System.out.println(mapper.getCount());}
查询一条数据为map集合
/*** 根据id查询用户信息为一个map集合*/Map<String,Object> getUserByIdToMap(@Param("id") Integer id);
<select id="getUserByIdToMap" resultType="java.util.Map">select * from t_user where id = #{id}</select>
@Testpublic void getUserByIdToMap() {SqlSession sqlSession = SqlSessionUtils.getSqlSession();SelectMapper mapper = sqlSession.getMapper(SelectMapper.class);System.out.println(mapper.getUserByIdToMap(3));}
查询多条数据为map集合
方法一:
/*** 查询所有用户信息为map集合* @return* 将表中的数据以map集合的方式查询,一条数据对应一个map;若有多条数据,就会产生多个map集合,此
时可以将这些map放在一个list集合中获取*/
List<Map<String,Object>> getAllUserToMap();
<select id="getUserByIdToMap" resultType="java.util.Map">select * from t_user where id = #{id}</select>
@Testpublic void getAllUserToMap() {SqlSession sqlSession = SqlSessionUtils.getSqlSession();SelectMapper mapper = sqlSession.getMapper(SelectMapper.class);System.out.println(mapper.getAllUserToMap());}
方法二:
/*** 查询所有用户信息为map集合* @return* 将表中的数据以map集合的方式查询,一条数据对应一个map;若有多条数据,就会产生多个map集合,并
且最终要以一个map的方式返回数据,此时需要通过@MapKey注解设置map集合的键,值是每条数据所对应的
map集合*/
@MapKey("id")
Map<String,Object> getAllUserToMap();
特殊SQL执行
模糊查询
/*** 根据用户名模糊查询用户信息*/List<User> getUserByLike(@Param("username") String username);
<select id="getUserByLike" resultType="com.qcby.pojo.User"><!--select * from t_user where username like '%${username}%'--><!--select * from t_user where username like concat('%',#{username},'%')-->select * from t_user where username like "%"#{username}"%"</select>
@Testpublic void getUserByLike(){SqlSession sqlSession = SqlSessionUtils.getSqlSession();SQLMapper mapper = sqlSession.getMapper(SQLMapper.class);List<User> list = mapper.getUserByLike("a");System.out.println(list);}
批量删除
/*** 批量删除*/Integer deleteMore(@Param("ids") String ids);
<delete id="deleteMore">delete from t_user where id in(${ids})</delete>
@Testpublic void deleteMore(){SqlSession sqlSession = SqlSessionUtils.getSqlSession();SQLMapper mapper = sqlSession.getMapper(SQLMapper.class);Integer result = mapper.deleteMore("1,2,3");System.out.println(result);}
动态设置表名
/*** 查询指定表中的数据*/List<User> getUserByTableName(@Param("tableName") String tableName);
<select id="getUserByTableName" resultType="com.qcby.pojo.User">select * from ${tableName}</select>
@Testpublic void getUserByTableName(){SqlSession sqlSession = SqlSessionUtils.getSqlSession();SQLMapper mapper = sqlSession.getMapper(SQLMapper.class);List<User> list = mapper.getUserByTableName("t_user");System.out.println(list);}
添加功能获取自增的主键
t_clazz(clazz_id,clazz_name) t_student(student_id,student_name,clazz_id)
1.添加班级信息
2.获取新添加的班级的id
3.为班级分配学生,即将某学的班级id修改为新添加的班级的id
void insertUser(User user);
<!--useGeneratedKeys:设置使用自增的主键keyProperty:因为增删改有统一的返回值是受影响的行数,因此只能将获取的自增的主键放在传输的参
数user对象的某个属性中--><insert id="insertUser" useGeneratedKeys="true" keyProperty="id">insert into t_user values (null,#{username},#{password},#{age},#{sex},#{email})</insert>
@Testpublic void insertUser(){SqlSession sqlSession = SqlSessionUtils.getSqlSession();SQLMapper mapper = sqlSession.getMapper(SQLMapper.class);User user = new User(null,"王五","123",23,"男","123@qq.com");mapper.insertUser(user);System.out.println(user);}
相关文章:
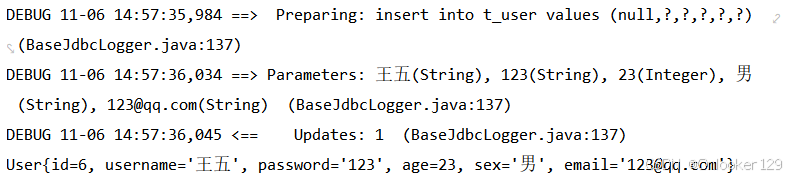
MyBatis3-获取参数值的方式、查询功能及特殊SQL执行
目录 准备工作 获取参数值的方式(重点) 查询功能 查询一个实体类对象 查询一个list集合 查询单个数据 查询一条数据为map集合 查询多条数据为map集合 特殊SQL执行 模糊查询 批量删除 动态设置表名 添加功能获取自增的主键 准备工作 模块My…...
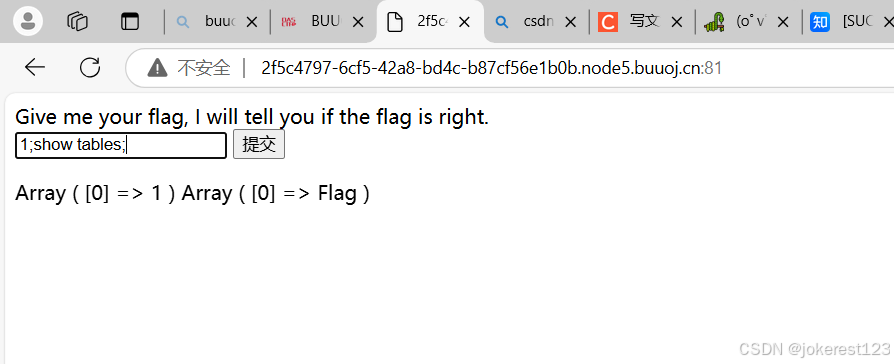
web——[SUCTF 2019]EasySQL1——堆叠注入
这个题主要是讲述了堆叠注入的用法,来复现一下 什么是堆叠注入 堆叠注入:将多条SQL语句放在一起,并用分号;隔开。 1.查看数据库的名称 查看数据库名称 1;show databases; 发现有名称为ctftraining的数据库 2.对表进行查询 1;show tabl…...
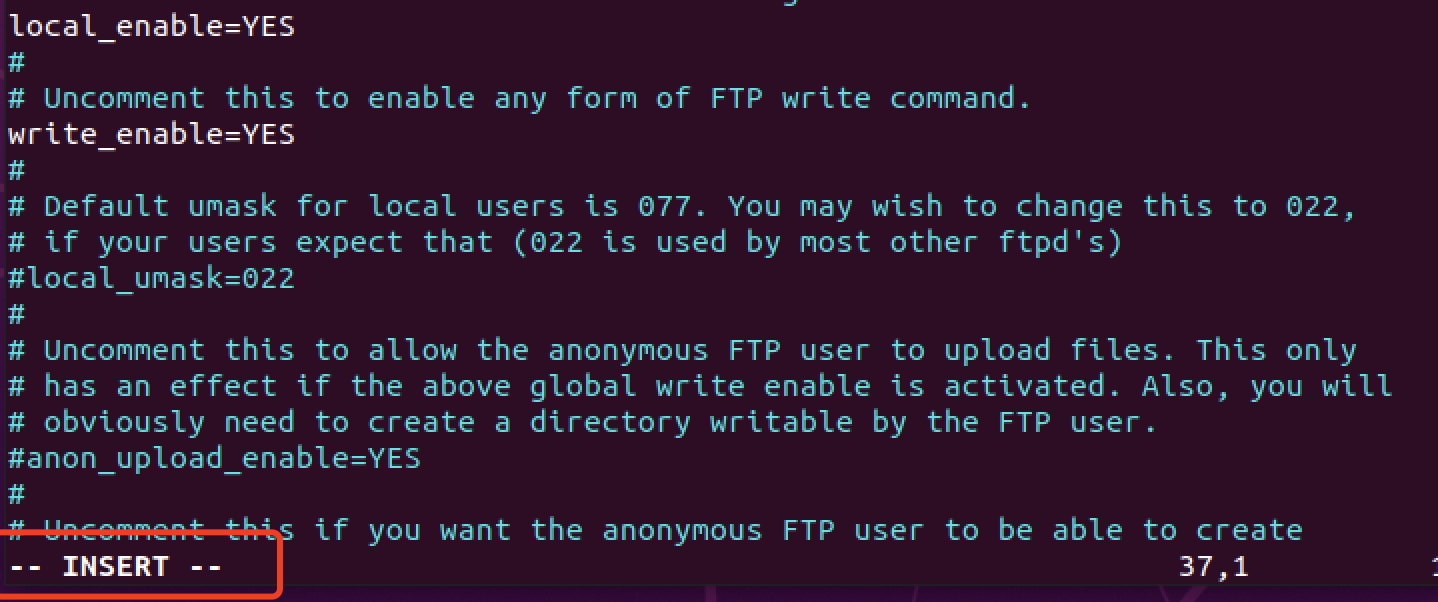
【Ubuntu学习】Ubuntu无法使用vim命令编辑
问题 在VMware首次安装Ubuntu,使用vi指令对文件进行编辑,按i键后无法更改文件内容。 原因 由于Ubuntu中预装的是vim-tiny,平时开发中需要使用vim-full。 解决方案 卸载预装vim sudo apt-get remove vim-common安装vim-full sudo apt-get …...
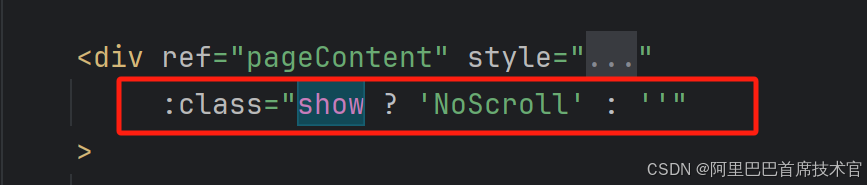
UniAPP u-popup 禁止背景滑动
增加class .NoScroll {overflow: hidden;position: fixed; }在外层div上增加该class判断条件...

F5全新报告揭示AI时代API安全面临严峻挑战
F5 《2024年应用策略现状报告:API安全》揭示了 API 保护中的漏洞以及对全面安全措施的迫切需求 西雅图,2024年11月11日 – F5(NASDAQ: FFIV)日前发布《2024年应用策略现状报告:API 安全》(以下简称为“报告”),揭示了跨行业API安全面临的严峻现状。该报告强调了企业API保护方面…...
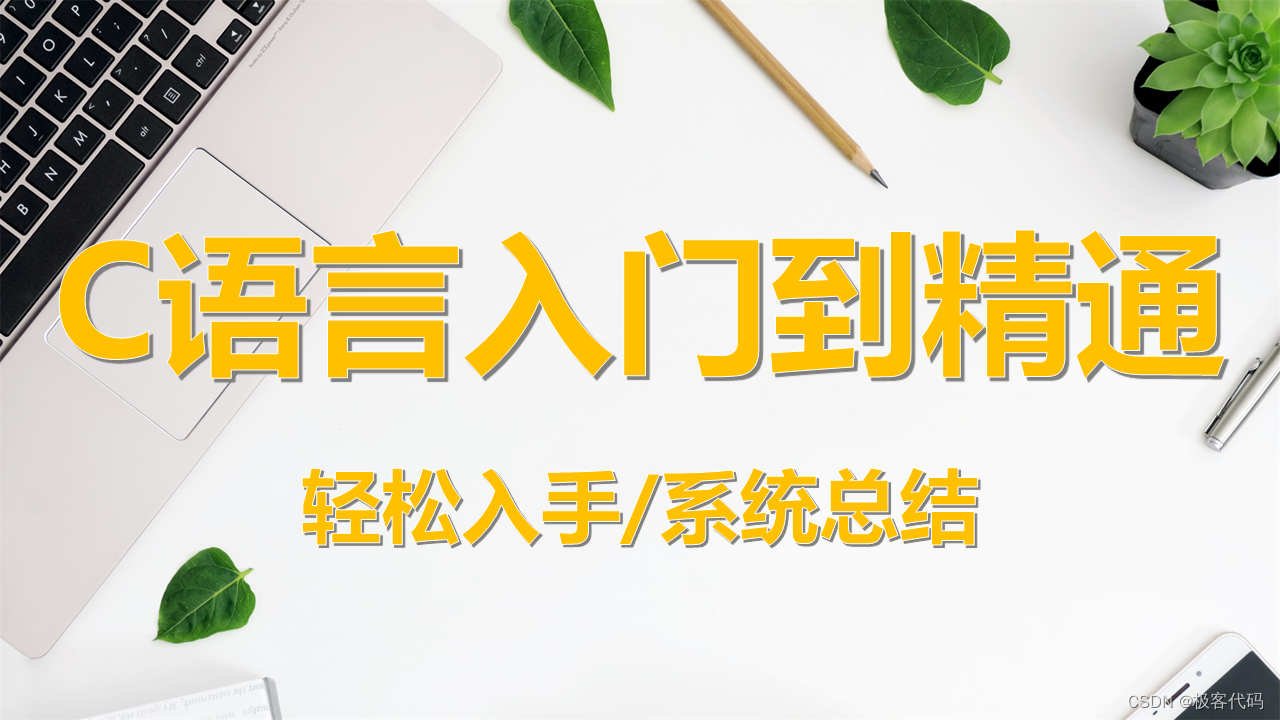
使用C语言进行信号处理:从理论到实践的全面指南
1. 引言 在现代操作系统中,信号是一种进程间通信机制,它允许操作系统或其他进程向一个进程发送消息。信号可以用来通知进程发生了一些重要事件,如用户请求终止进程、硬件异常、定时器超时等。掌握信号处理技术对于开发健壮、高效的系统程序至…...
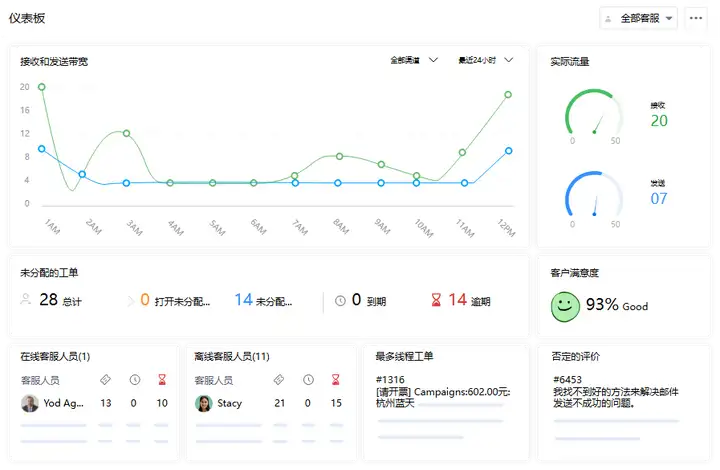
什么是工单管理系统?全面认识指南
在现代企业中,客户服务和支持是业务成功的关键因素之一。为了有效地管理客户请求和问题,许多公司采用了工单管理系统。本文将深入探讨工单管理系统的定义、功能、优势。 一、工单管理系统的定义 工单管理系统是一种软件工具,旨在帮助企业管…...

集群化消息服务解决方案
目录 集群化消息服务解决方案项目概述架构图使用说明服务端通过API接口推送消息给客户端调用方式 请求参数返回参数 客户端推送消息连接websocket或发送消息 接收消息项目地址作者信息 集群化消息服务解决方案 项目概述 集群化消息服务解决方案是一种用于处理大量消息的高可用…...
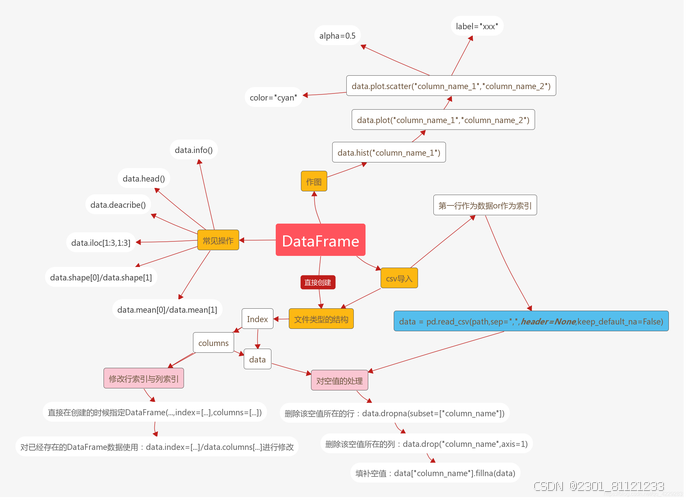
python数据结构操作与可视化的应用
Python具有丰富的数据结构操作和可视化库,可以进行各种数据结构的创建、编辑和分析,并将结果可视化。以下是几个常见的Python数据结构操作和可视化的应用示例: 1. 列表(List)操作和可视化: - 创建列表&a…...

【基于轻量型架构的WEB开发】课程 作业4 AOP
一. 单选题(共7题,38.5分) 1 (单选题)下列选项中,用于通知/增强处理的是( )。 A. Joinpoint B. Pointcut C. Aspect D. Advice 正确答案:D 答案解析:在面向切面编程ÿ…...
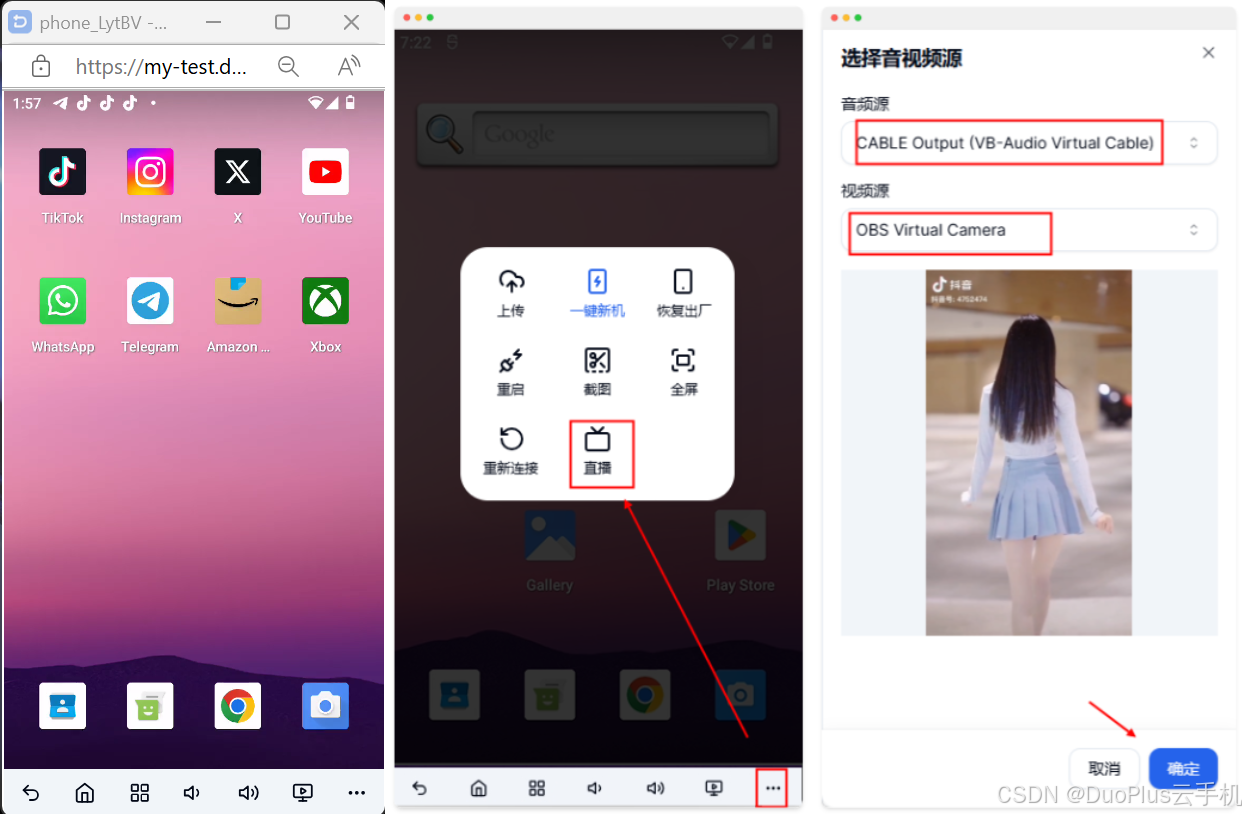
跨境独立站新手,如何用DuoPlus云手机破局海外社媒引流?
独立站作为电商领域的一个重要组成部分,其发展在最近几年里确实令人瞩目,对于想要进入跨境赛道的新手卖家来说,手上握着有优势的货源,建立小型的DTC独立站确实会比入驻第三方平台具有更大的灵活性。本文将给跨境卖家们总结独立站和…...
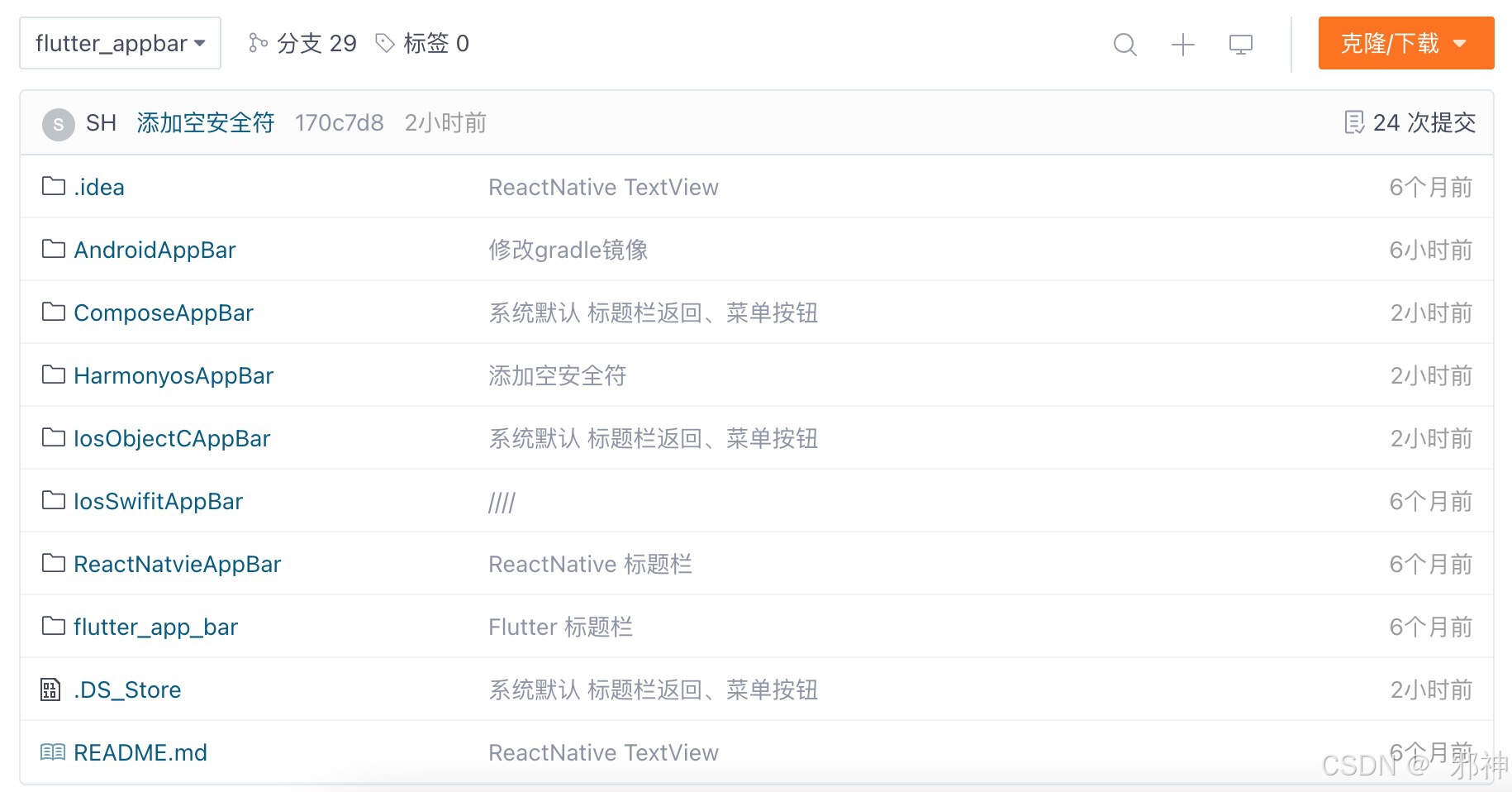
【Android、IOS、Flutter、鸿蒙、ReactNative 】标题栏
Android 标题栏 参考 Android Studio版本 配置gradle镜像 阿里云 Android使用 android:theme 显示标题栏 添加依赖 dependencies {implementation("androidx.appcompat:appcompat:1.6.1")implementation("com.google.android.material:material:1.9.0")…...
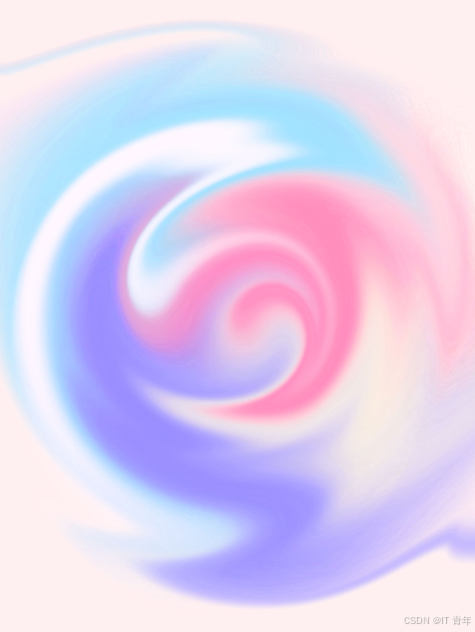
信息安全工程师(83)Windows操作系统安全分析与防护
一、Windows操作系统安全分析 系统漏洞: Windows操作系统由于其复杂性和广泛使用,可能存在一些已知或未知的漏洞。这些漏洞可能会被黑客利用,进行恶意攻击。微软会定期发布系统更新和补丁,以修复这些漏洞,提高系统的安…...
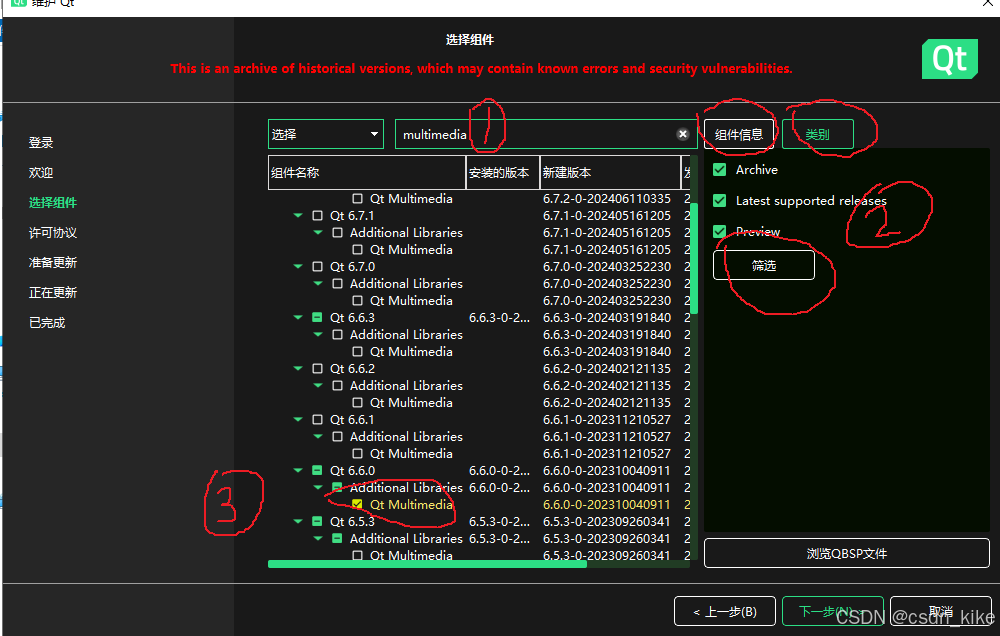
QT Unknown module(s) in QT 以及maintenance tool的更详细用法(qt6.6.0)
不小心接了同事的委托,帮改一个qt的工程代码。然后出事了,那个proj是qt5.9版本的吧,搞到6.6版本的环境中各种问题。至少有3个是这样的: :-1: error: Unknown module(s) in QT: multimedia 直接百度,好像很简单&#x…...
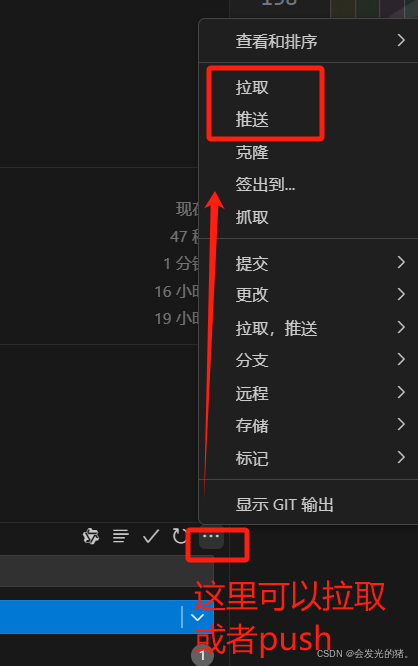
如何在vscode中安装git详细新手教程
一、安装git后点击vscode中的设置 今天教大家如何在VScode中编写代码后提交到git仓库,如果我们不想切换到git的命令行窗口,可以在VScode中配置git,然后就可以很方便快捷的把代码提交到仓库中。 二、在输入框中输入 git.path ,再点…...
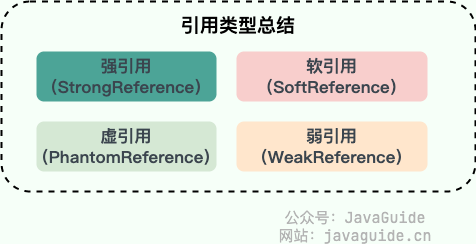
JVM垃圾回收详解二(重点)
死亡对象判断方法 堆中几乎放着所有的对象实例,对堆垃圾回收前的第一步就是要判断哪些对象已经死亡(即不能再被任何途径使用的对象)。 引用计数法 给对象中添加一个引用计数器: 每当有一个地方引用它,计数器就加 1…...
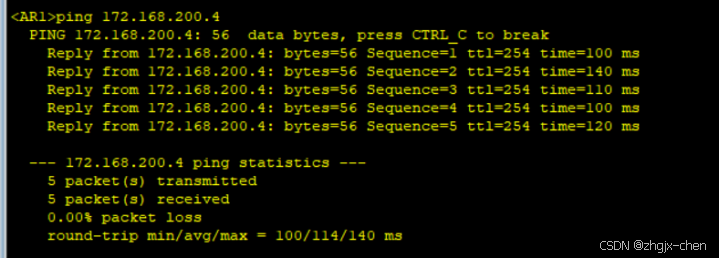
VLAN 高级技术实验
目录 一、实验背景 二、实验任务 三、实验步骤 四、实验总结 一、实验背景 假如你是公司的网络管理员,为了节省内网的IP地址空间,你决定在内网部署VLAN聚合,同时为了限制不同业务之间的访问,决定同时部署MUX VLAN。 二、实验…...

windowsC#-创建和引发异常
异常用于指示在运行程序时发生了错误。 此时将创建一个描述错误的异常对象,然后使用 throw 语句或表达式引发。 然后,运行时搜索最兼容的异常处理程序。 当存在下列一种或多种情况时,程序员应引发异常: 1. 方法无法完成其定义的…...
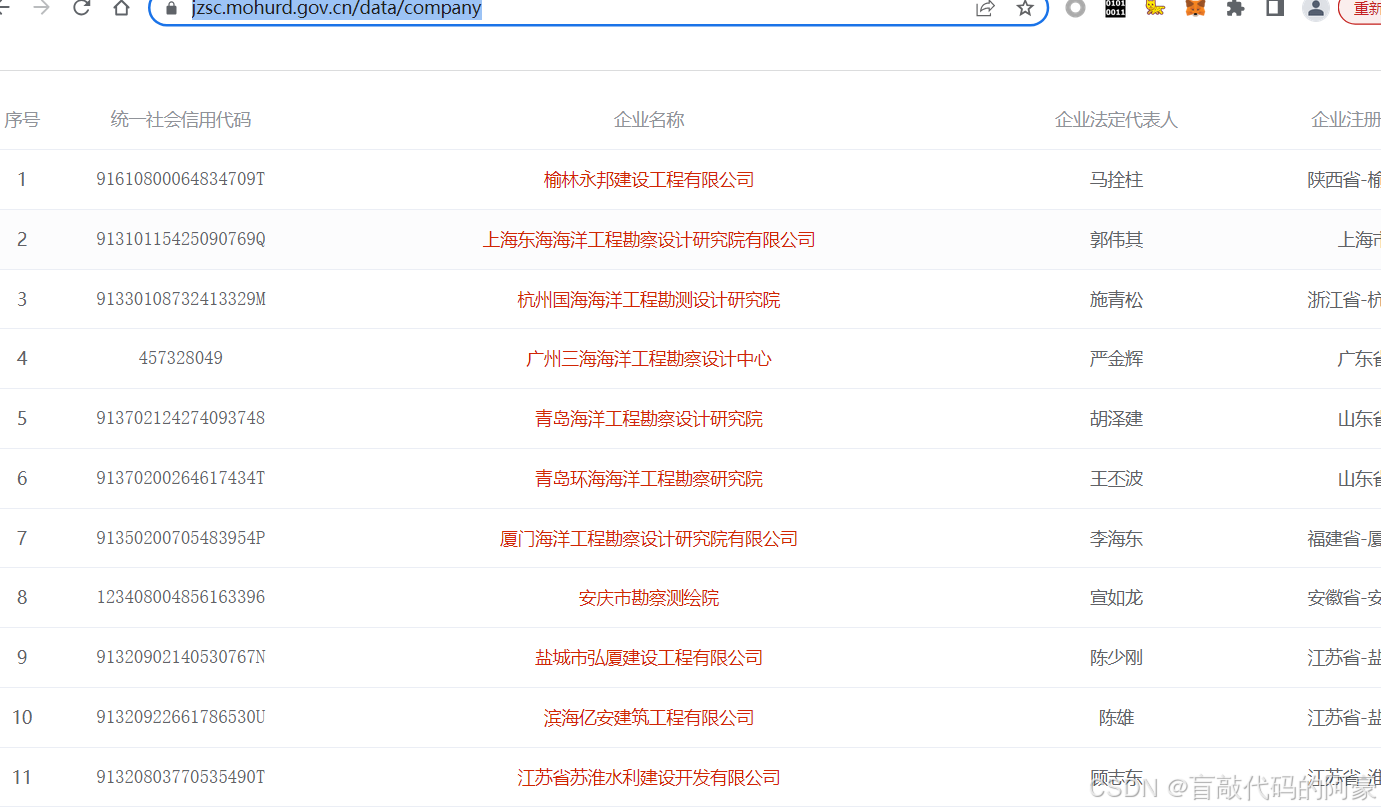
python爬虫案例——请求的网页源码被加密,解密方法全过程(19)
文章目录 1、任务目标2、网页分析3、代码编写1、任务目标 目标网站:https://jzsc.mohurd.gov.cn/data/company,该网站的网页源码被加密了,用于本文测验 要求:解密该网站的网页源码,请求网站并返回解密后的明文数据,网页内容如下: 2、网页分析 进入网站,打开开发者模式,…...

详解广告联盟
某种程度上,动荡的程度甚于以往。产业链中快速挤进了众多不曾有过的角色,产业逻辑被完全颠覆。巨大的变化在几年间迅速产生,源头是快速发展的互联网和科技。 这个行业走到了十字路口,身处其中的大多数人感到乐观,但同…...

Getting accurate time estimates from your tea(从您的团队获得准确的时间估计)
Hi again. 嗨了。 Ready to get back into it? 准备好重新开始了吗? Let’s go. Time estimation, 我们走吧。时间估计, effort estimation, 努力估计, and capacity planning are all helpful techniques for creating your project schedule. 容量规划都是创建项…...

攻防世界35-easyupload-CTFWeb
攻防世界35-easyupload-CTFWeb 通过各种上传发现,过滤了php后缀和内容中有php的文件 有这几种方式上传一句话木马 <script language"php">eval($_POST[1]);</script> <?php eval($_POST[cmd]);?> <? eval($_POST[cmd]);?>…...

在Mysql中,如何定位慢查询
参考回答:之前我们有个项目做压测的时候有的接口非常的慢,接口的响应时间超过了2秒以上,因为在MySOL中也提供了慢日志查询的功能,可以在MySOL的系统配置文件中开启这个慢日志的功能,并且也可以设置SOL执行超过多少时间…...
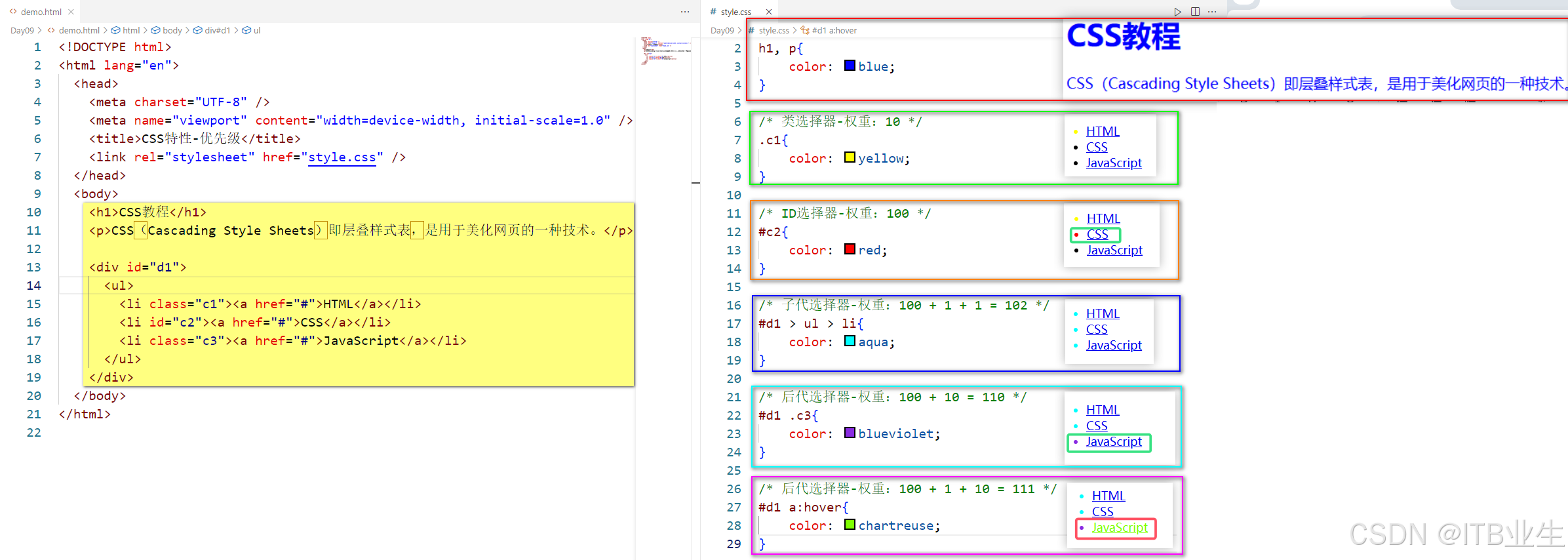
CSS教程(三)- CSS 三大特性
1. 层叠性 介绍 多组CSS样式共同作用于一个元素,就会出现 覆盖(层叠) 另一个冲突的样式。 层叠原则 样式冲突:遵循就近原则(哪个样式离结构近,就执行哪个样式) 样式不冲突,就不会重…...
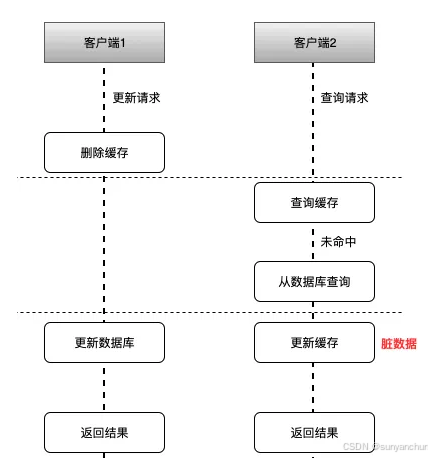
如何保证Redis与MySQL双写一致性
什么是双写一致性问题? 双写一致性主要指在一个数据同时存在于缓存(如Redis)和持久化存储(如MySQL)的情况下,任何一方的数据更新都必须确保另一方数据的同步更新,以保持双方数据的一致状态。这一…...

【IC每日一题:IC验证面试--UVM验证-2】
IC每日一题:IC验证面试--UVM验证-2 2.9 get_next_iterm()和try_next_item()的区别?2.10 一个典型的UVM验证平台,谈一下UVM验证环境结构,各个组件之间的关系?2.11 uvm组件之间通信的方式? analysis_port和其…...
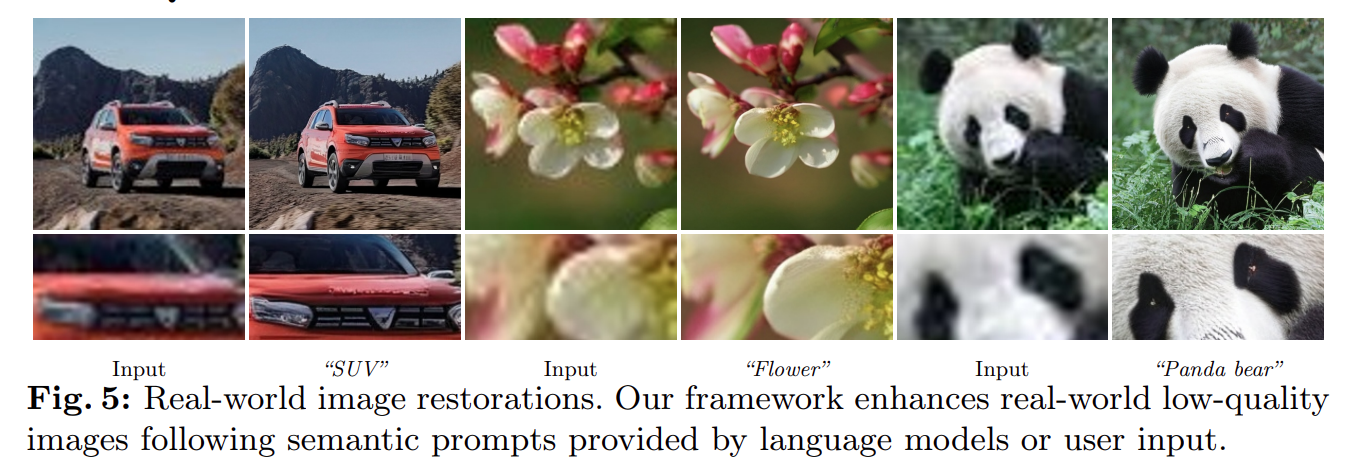
SPIRE: Semantic Prompt-Driven Image Restoration 论文阅读笔记
这是一篇港科大学生在google research 实习期间发在ECCV2024的语义引导生成式修复的文章,港科大陈启峰也挂了名字。从首页图看效果确实很惊艳,尤其是第三行能用文本调控修复结果牌上的字。不过看起来更倾向于生成,对原图内容并不是很复原&…...

#揭秘万维网:从静态页面到智能互联网
揭秘万维网:从静态页面到智能互联网 今天刚上了学校开设的课程,于是便有了下文的思考内容。 在当今数字化时代,Web(万维网)扮演着重要的角色,成为人们获取信息、沟通交流和进行商业活动的主要平台。 1. …...
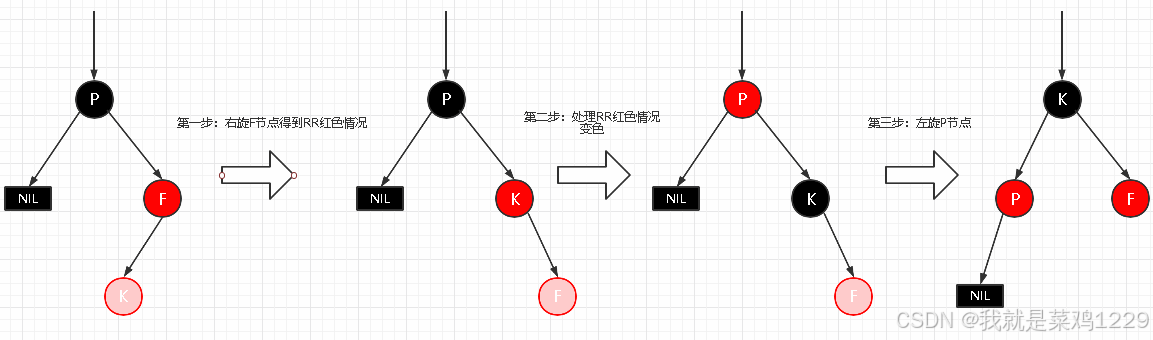
【计算机基础——数据结构——红黑树】
1. 红黑树(RBTree) 为什么HashMap不直接使用AVL树,而是选择了红黑树呢? 由于AVL树必须保证左右子树平衡,Max(最大树高-最小树高) < 1,所以在插入的时候很容易出现不平衡的情况,一旦这样&…...
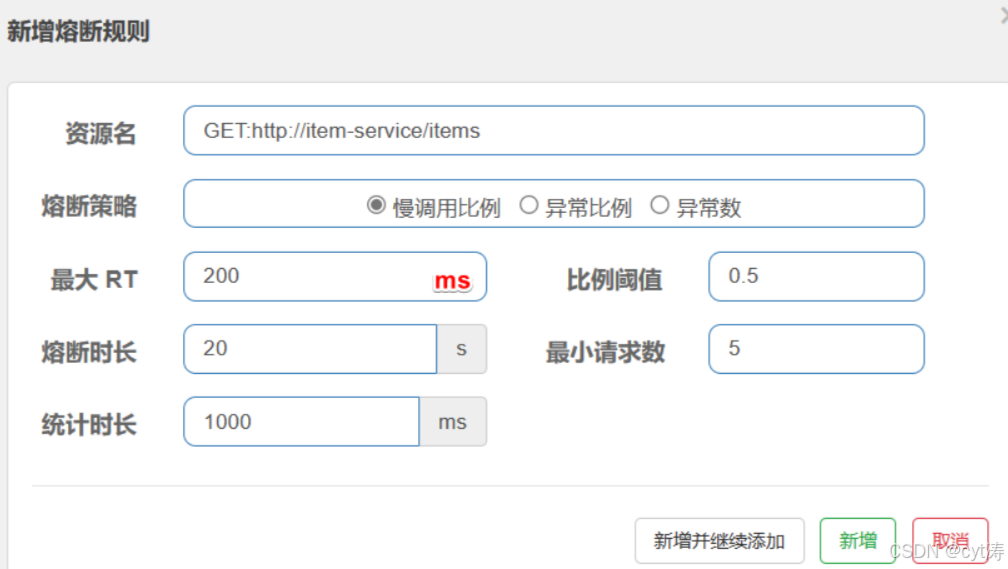
Sentinel — 微服务保护
微服务架构将大型应用程序拆分为多个小而独立的服务,每个服务可以独立部署和扩展。然而,微服务系统需要面对的挑战也随之增加,例如服务之间的依赖、分布式环境下的故障传播和安全问题。因此,微服务保护措施是确保系统在高并发、资…...