自动优化网站建设/seo优化与sem推广有什么关系
Spring 集成 MyBatis
将 MyBatis 与 Spring 进行整合,主要解决的问题就是将 SqlSessionFactory 对象交由 Spring 来管理。所以该整合,只需要将 SqlSessionFactory 的对象生成器SqlSessionFactoryBean 注册在 Spring 容器中,再将其注入给 Dao 的实现类即可完成整合。
实现 Spring 与 MyBatis 的整合常用的方式:扫描的 Mapper 动态代理
Spring 像插线板一样,mybatis 框架是插头,可以容易的组合到一起。
插线板 spring 插上 mybatis,两个框架就是一个整体。
1、MySQL 创建数据库 db1,新建表 student2
2、maven 依赖 pom.xml
<dependencies><dependency><groupId>junit</groupId><artifactId>junit</artifactId><version>4.12</version><scope>test</scope></dependency><dependency><groupId>org.springframework</groupId><artifactId>spring-context</artifactId><version>5.3.26</version></dependency><dependency><groupId>org.springframework</groupId><artifactId>spring-tx</artifactId><version>5.3.26</version></dependency><dependency><groupId>org.springframework</groupId><artifactId>spring-jdbc</artifactId><version>5.3.26</version></dependency><dependency><groupId>org.mybatis</groupId><artifactId>mybatis</artifactId><version>3.5.11</version></dependency><!-- Spring整合MyBatis的依赖 --><dependency><groupId>org.mybatis</groupId><artifactId>mybatis-spring</artifactId><version>2.1.0</version></dependency><!-- --------------------- --><dependency><groupId>mysql</groupId><artifactId>mysql-connector-java</artifactId><version>8.0.29</version></dependency><dependency><groupId>com.alibaba</groupId><artifactId>druid</artifactId><version>1.2.8</version></dependency>
</dependencies> <!--插件:-->
<build><resources><resource><directory>src/main/java</directory><includes><include>**/*.properties</include><include>**/*.xml</include></includes><filtering>false</filtering></resource></resources><plugins><plugin><groupId>org.apache.maven.plugins</groupId><artifactId>maven-compiler-plugin</artifactId><version>3.11.0</version><configuration><source>1.8</source><target>1.8</target></configuration></plugin></plugins>
</build>
3、定义实体类 Student
代码如下:
package com.ambow.pojo;import lombok.Data;@Data
public class Student {private int id;private String name;private int age;}
4、定义 StudentDao 接口
代码如下:
package com.ambow.dao;import com.ambow.pojo.Student;import java.util.List;public interface StudentDao {int insertStudent(Student student);int updateStudent(Student student);int deleteStudent(int id);Student selectStudentById(int id);List<Student> selectAllStudents();
}
5、定义映射文件 mapper
在 Dao 接口的包中创建 MyBatis 的映射文件 mapper,命名与接口名相同,本例为 StudentDao.xml
mapper 中的 namespace 取值也为 Dao 接口 的全限定性名。
代码如下:
<?xml version="1.0" encoding="UTF-8" ?>
<!DOCTYPE mapperPUBLIC "-//mybatis.org//DTD Mapper 3.0//EN""http://mybatis.org/dtd/mybatis-3-mapper.dtd">
<mapper namespace="com.ambow.dao.StudentDao"><insert id="insertStudent">insert into student2 values(null,#{name},#{age})</insert><update id="updateStudent">update student2 set name = #{name},age = #{age} where id = #{id}</update><delete id="deleteStudent">delete from student2 where id = #{id}</delete><select id="selectStudentById" resultType="com.ambow.pojo.Student">select * from student2 where id = #{id}</select><select id="selectAllStudents" resultType="com.ambow.pojo.Student">select * from student2</select></mapper>
6、定义 Service 接口和实现类
接口定义:
代码如下:
package com.ambow.service;import com.ambow.pojo.Student;import java.util.List;public interface StudentService {int addStudent(Student student);int modifyStudent(Student student);int removeStudent(int id);Student findStudentById(int id);List<Student> findAllStudents();
}
实现类定义:
代码如下:
package com.ambow.service.impl;import com.ambow.dao.StudentDao;
import com.ambow.pojo.Student;
import com.ambow.service.StudentService;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Component;
import org.springframework.stereotype.Service;
import java.util.List;@Service("studentService")
public class StudentServiceImpl implements StudentService {//引入StudentDao的对象@Autowiredprivate StudentDao studentDao;public void setStudentDao(StudentDao studentDao) {this.studentDao = studentDao;}@Overridepublic int addStudent(Student student) {return studentDao.insertStudent(student);}@Overridepublic int modifyStudent(Student student) {return studentDao.updateStudent(student);}@Overridepublic int removeStudent(int id) {return studentDao.deleteStudent(id);}@Overridepublic Student findStudentById(int id) {return studentDao.selectStudentById(id);}@Overridepublic List<Student> findAllStudents() {return studentDao.selectAllStudents();}
}
7、定义 MyBatis 主配置文件
在 src 下定义 MyBatis 的主配置文件,命名为 mybatis.xml
这里有两点需要注意:
(1)主配置文件中不再需要数据源的配置了。因为数据源要交给 Spring 容器 来管理了。
(2)这里对 mapper 映射文件的注册,使用标签,即只需给出 mapper 映射文件所在的包即可。因为 mapper 的名称与 Dao 接口名相同, 可以使用这种简单注册方式。
这种方式的好处是:若有多个映射文件,这里的 配置也是不用改变的。当然,也可使用原来的标签方式。
8 、修改 Spring 配置文件
(1) 数据源的配置(掌握)
使用 JDBC 模板,首先需要配置好数据源,数据源直接以 Bean 的形式配置 在 Spring 配置文件中。根据数据源的不同,其配置方式不同:
Druid 数据源 DruidDataSource Druid 是阿里的开源数据库连接池。是 Java 语言中最好的数据库连接 池。Druid 能够提供强大的监控和扩展功能。Druid 与其他数据库连接池的 最大区别是提供数据库的
官网:GitHub - alibaba/druid: 阿里云计算平台DataWorks(https://help.aliyun.com/document_detail/137663.html) 团队出品,为监控而生的数据库连接池阿里云计算平台DataWorks(https://help.aliyun.com/document_detail/137663.html) 团队出品,为监控而生的数据库连接池 - GitHub - alibaba/druid: 阿里云计算平台DataWorks(https://help.aliyun.com/document_detail/137663.html) 团队出品,为监控而生的数据库连接池https://github.com/alibaba/druid
使用地址:Home · alibaba/druid Wiki · GitHub阿里云计算平台DataWorks(https://help.aliyun.com/document_detail/137663.html) 团队出品,为监控而生的数据库连接池 - Home · alibaba/druid Wikihttps://github.com/alibaba/druid/wiki/
常见问题
配置连接池:
Spring 配置文件:
代码如下:
<!--1.DataSource--><bean id="dataSource" class="com.alibaba.druid.pool.DruidDataSource"><property name="driverClassName" value="${jdbc.driver}" /><property name="url" value="${jdbc.url}" /><property name="username" value="${jdbc.username}" /><property name="password" value="${jdbc.password}" /></bean>
(2) 从属性文件读取数据库连接信息
为了便于维护,可以将数据库连接信息写入到属性文件中,使 Spring 配置 文件从中读取数据。
属性文件名称自定义,但一般都是放在 src 下。
代码如下:
jdbc.driver=com.mysql.jdbc.Driver
jdbc.url=jdbc:mysql://192.168.2.111:3306/db1
jdbc.username=root
jdbc.password=Mysql666!
Spring 配置文件从属性文件中读取数据时,需要在的 value 属性中使用${ },将在属性文件中定义的 key 括起来,以引用指定属性的值。
该属性文件若要被 Spring 配置文件读取,其必须在配置文件中进行注册。 使用<context>标签。
<context:property-placeholder />方式(掌握)
该方式要求在 Spring 配置文件头部加入 spring-context.xsd 约束文件
<context:property-placeholder />标签中有一个属性 location,用于指定属 性文件的位置。
(3) 注册 SqlSessionFactoryBean
代码如下:
<!--2.注册SqlSessionFactoryBean--><bean id="sqlSessionFactory" class="org.mybatis.spring.SqlSessionFactoryBean"><property name="dataSource" ref="dataSource" /><property name="typeAliasesPackage" value="com.ambow.pojo" /><property name="mapperLocations" value="classpath:com/ambow/dao/*.xml" /></bean>
(4) 定义 Mapper 扫描配置器 MapperScannerConfigurer
Mapper 扫描配置器 MapperScannerConfigurer 会自动生成指定的基本 包中 mapper 的代理对象。该 Bean 无需设置 id 属性。basePackage 使用分 号或逗号设置多个包。
代码如下:
<!--3.mapper的扫描配置器 -> 生成mapper的代理对象--><bean class="org.mybatis.spring.mapper.MapperScannerConfigurer"><property name="sqlSessionFactoryBeanName" value="sqlSessionFactory" /><property name="basePackage" value="com.ambow.dao" /></bean>
9、向 Service 注入接口名
向 Service 注入 Mapper 代理对象时需要注意,由于通过 Mapper 扫描配置器 MapperScannerConfigurer 生成的 Mapper 代理对象没有名称,所以在 向 Service 注入 Mapper 代理时,无法通过名称注入。但可通过接口的简单类名注入,因为生成的是这个 Dao 接口的对象。
代码如下:
<!--4.向service层注入Dao-->
<!-- <bean id="studentService" class="com.ambow.service.impl.StudentServiceImpl"><property name="studentDao" ref="studentDao" /></bean>-->
10、Spring 配置文件全部配置
代码如下:
<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns="http://www.springframework.org/schema/beans"xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"xmlns:context="http://www.springframework.org/schema/context"xsi:schemaLocation="http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans.xsd http://www.springframework.org/schema/context https://www.springframework.org/schema/context/spring-context.xsd"><!--整合:把MyBatis中的核心对象,放到Spring容器--><!--引入属性配置文件--><context:property-placeholder location="classpath:jdbc.properties" /><context:component-scan base-package="com.ambow.service" /><!--1.DataSource--><bean id="dataSource" class="com.alibaba.druid.pool.DruidDataSource"><property name="driverClassName" value="${jdbc.driver}" /><property name="url" value="${jdbc.url}" /><property name="username" value="${jdbc.username}" /><property name="password" value="${jdbc.password}" /></bean><!--2.注册SqlSessionFactoryBean--><bean id="sqlSessionFactory" class="org.mybatis.spring.SqlSessionFactoryBean"><property name="dataSource" ref="dataSource" /><property name="typeAliasesPackage" value="com.ambow.pojo" /><property name="mapperLocations" value="classpath:com/ambow/dao/*.xml" /></bean><!--3.mapper的扫描配置器 -> 生成mapper的代理对象--><bean class="org.mybatis.spring.mapper.MapperScannerConfigurer"><property name="sqlSessionFactoryBeanName" value="sqlSessionFactory" /><property name="basePackage" value="com.ambow.dao" /></bean><!--4.向service层注入Dao-->
<!-- <bean id="studentService" class="com.ambow.service.impl.StudentServiceImpl"><property name="studentDao" ref="studentDao" /></bean>--><!--注入的过程--><!--dataSource -> SqlSessionFactoryBean -> MapperScannerConfigurer(生成Dao代理对象) -> studentService--></beans>
项目整体结构如下图:
运行测试类test02:
运行测试类test03:
相关文章:
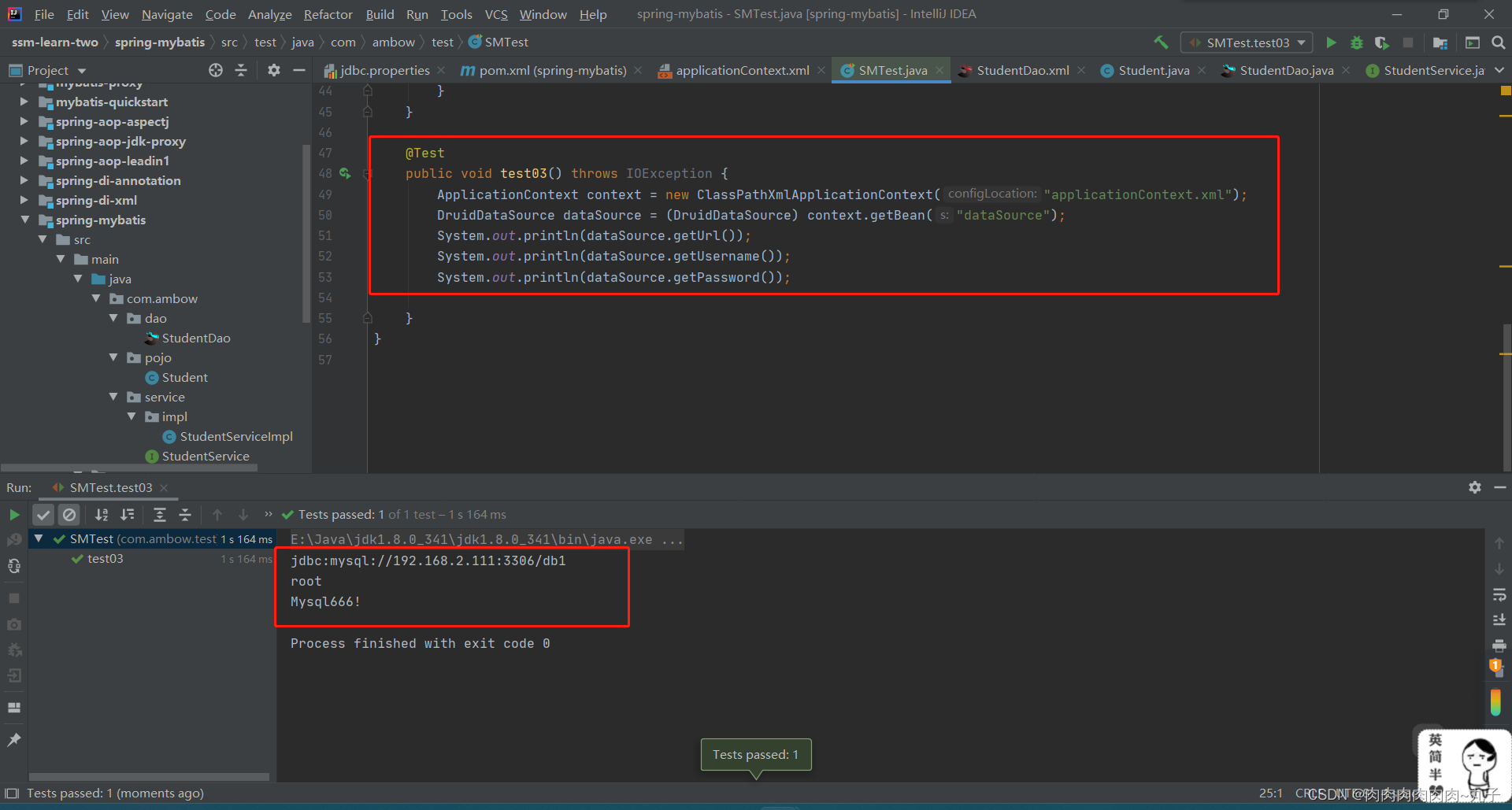
Java课题笔记~ Spring 集成 MyBatis
Spring 集成 MyBatis 将 MyBatis 与 Spring 进行整合,主要解决的问题就是将 SqlSessionFactory 对象交由 Spring 来管理。所以该整合,只需要将 SqlSessionFactory 的对象生成器SqlSessionFactoryBean 注册在 Spring 容器中,再将其注入给 Dao…...

分布式系统理论基础
文章目录 介绍目标 正文CAPConsistencyAvailabilityPartition tolerance BASEBasically AvailableSoft StateEventually Consistent ACIDatomicityconsistencyisolationdurability 参考文档 介绍 分布式系统面临的场景往往是众口难调,“这也要,那也要”…...

mfc 编辑框限制
DoDataExchange由框架调用,作用是交互并且验证对话框数据,主要由(DDX) 和 (DDV)宏实现。 永远不要直接调用这个函数,而是通过UpdateData(TRUE/FALSE)实现控件与变量之间值的传递。 当然你也可以不使用DoDataExchange而完成控件与变量之间值…...
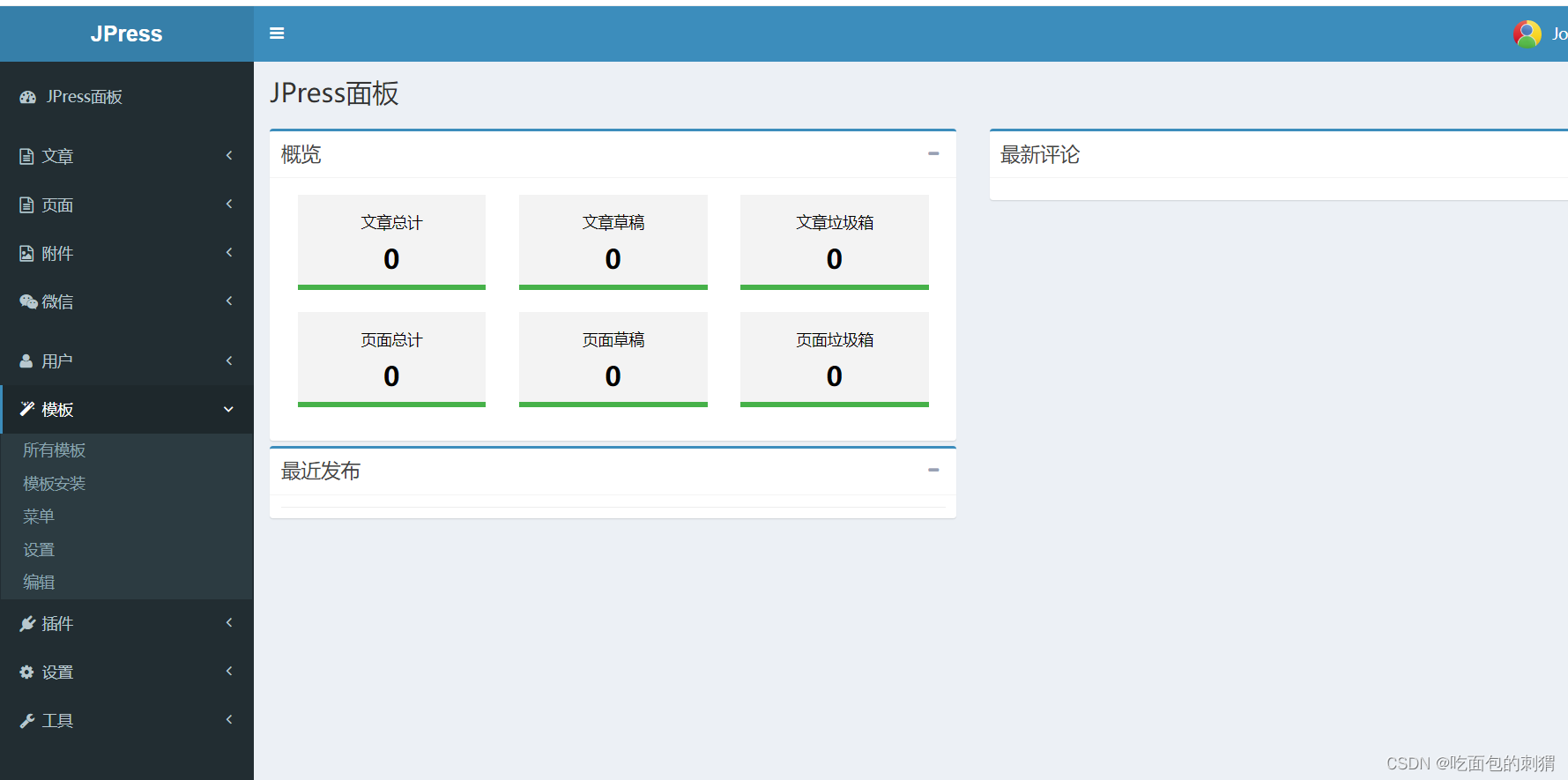
web基础与tomcat环境部署
一. 简述静态网页和动态网页的区别。 请求响应信息,发给客户端进行处理,由浏览器进行解析,显示的页面称为静态页面。处理文件类型如.html、jpg、.gif、.mp4、.swf、.avi、.wmv、.flv等 请求响应信息,发给事务端进行处理࿰…...

Go 变量
在Go中,有不同的变量类型,例如: int 存储整数(整数),例如123或-123float32 存储浮点数字,带小数,例如19.99或-19.99string - 存储文本,例如“ Hello World”。字符串值用…...
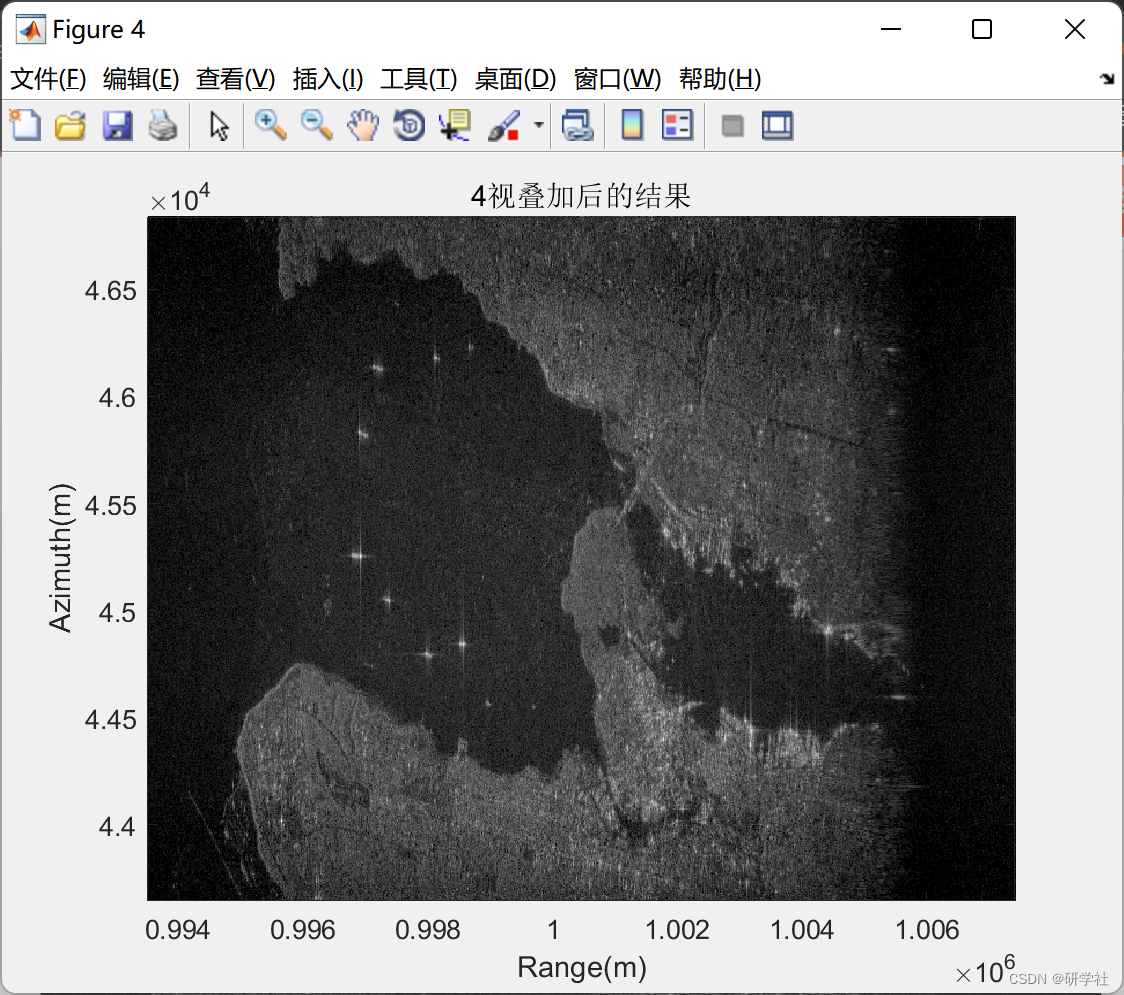
【雷达通信】非相干多视处理(CSA)(Matlab代码实现)
💥💥💞💞欢迎来到本博客❤️❤️💥💥 🏆博主优势:🌞🌞🌞博客内容尽量做到思维缜密,逻辑清晰,为了方便读者。 ⛳️座右铭&a…...
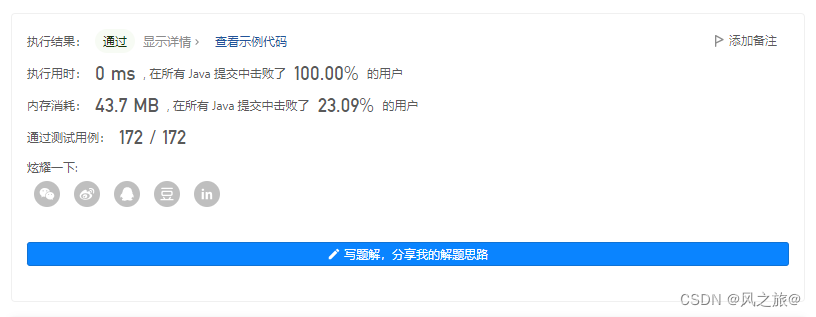
73. 矩阵置零
题目链接:力扣 解题思路: 方法一:比较容易想到的方向,使用两个数组row和col保存有0的行或者列,然后将有0的那一行或那一列的所有元素都设置为0 AC代码 class Solution {public void setZeroes(int[][] matrix) {in…...

‘大数据技术与应用’和‘数据科学与大数据技术’有什么区别
一、侧重点不同 ‘大数据技术与应用’主要侧重于大数据的存储、处理和分析技术、包括数据挖掘、机器学习、数据仓库、分布式计算等方面的研究,旨在开发大数据相关的应用程序和系统,以满足商业和企业的需求。 ‘数据科学与大数据技术’则更加注重数据本…...

没有jsoup,rust怎么解析html呢?
在 Rust 中,你可以使用各种库来解析网页内容。一个常用的库是 reqwest ,它提供了一个简单的方式来发送 HTTP 请求并获取网页内容。另外,你可以使用 scraper 或 select 等库来解析 HTML 或 XML 格式的网页内容。 下面是一个使用 reqwest 和 sc…...

【C高级】Day4 shell脚本 排序
1. 整理思维导图 2. 写一个函数,获取用户的uid和gid并使用变量接收 #!/bin/bash function getid() {uidid -ugidid -g }getid echo "uid$uid" echo "gid$gid"3. 整理冒泡排序、选择排序和快速排序的代码 #include <myhead.h>void Inp…...

大模型开发(十六):从0到1构建一个高度自动化的AI项目开发流程(中)
全文共1w余字,预计阅读时间约40~60分钟 | 满满干货(附代码),建议收藏! 本文目标:通过LtM提示流程实现自动构建符合要求的函数,并通过实验逐步完整测试code_generate函数功能。 代码下载点这里 一、介绍 此篇文章为…...

【深入了解pytorch】PyTorch强化学习:强化学习的基本概念、马尔可夫决策过程(MDP)和常见的强化学习算法
【深入了解pytorch】PyTorch强化学习:强化学习的基本概念、马尔可夫决策过程(MDP)和常见的强化学习算法 PyTorch强化学习:介绍强化学习的基本概念、马尔可夫决策过程(MDP)和常见的强化学习算法引言强化学习的基本概念状态(State)动作(Action)奖励(Reward)策略(Pol…...
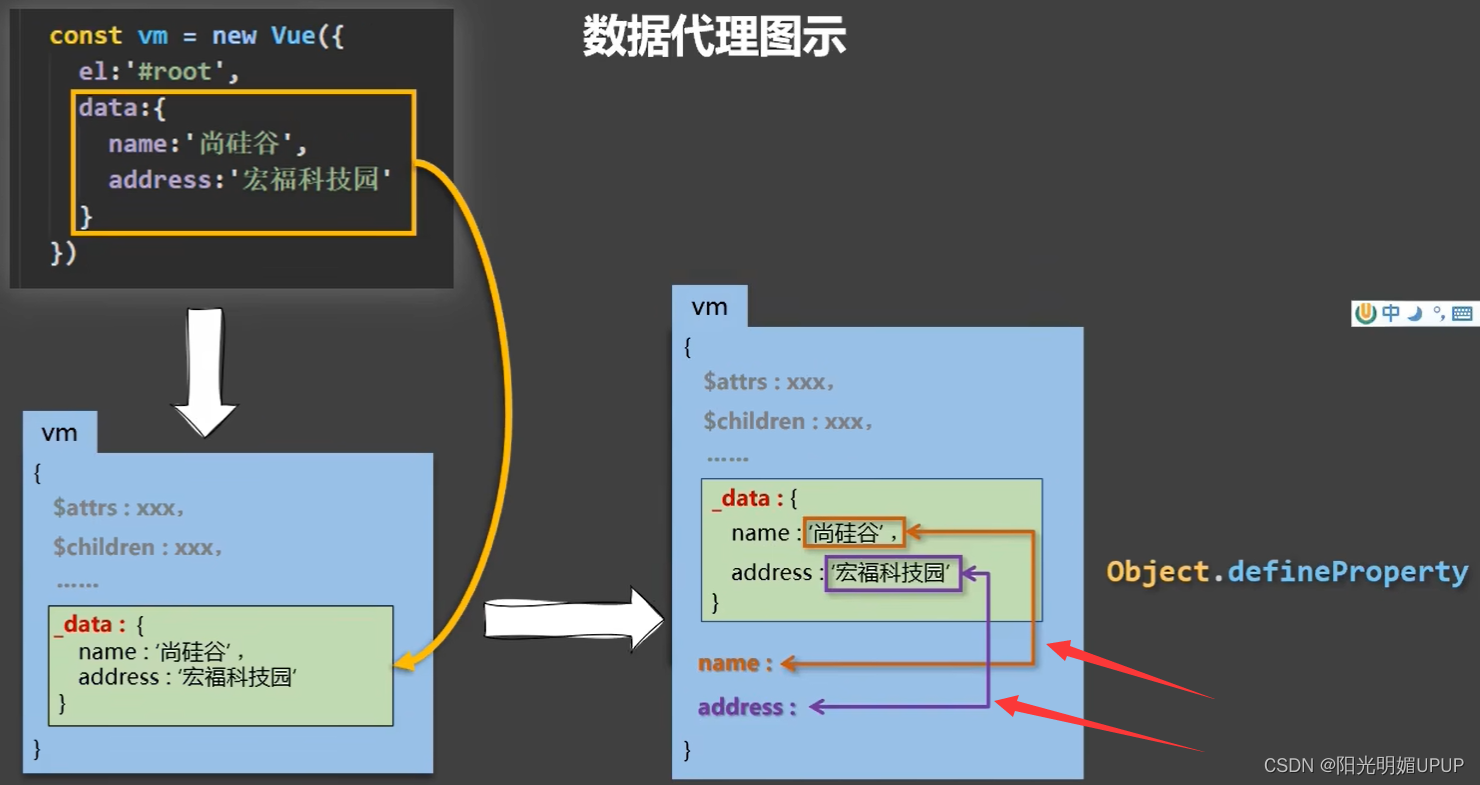
尚硅谷张天禹Vue2+Vue3笔记(待续)
简介 什么是Vue? 一套用于构建用户界面的渐进式JavaScript框架。将数据转变成用户可看到的界面。 什么是渐进式? Vue可以自底向上逐层的应用 简单应用:只需一个轻量小巧的核心库 复杂应用:可以引入各式各样的Vue插件 Vue的特点是什么? 1.采…...
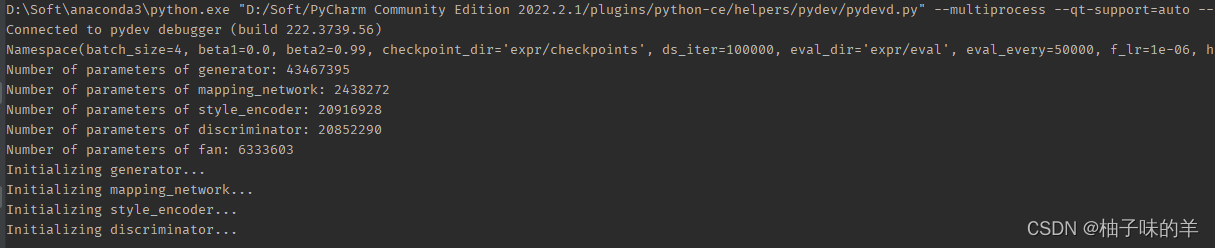
深度学习(35)—— StarGAN(2)
深度学习(34)—— StarGAN(2) 完整项目在这里:欢迎造访 文章目录 深度学习(34)—— StarGAN(2)1. build model(1)generator(2&#…...

连续四年入选!三项荣耀!博云科技强势上榜Gartner ICT技术成熟度曲线
日,全球知名咨询公司Gartner发布了2023年度的《中国ICT技术成熟度曲线》(《Hype Cycle for ICT in China, 2023》,以下简称“报告”)。令人瞩目的是,博云科技在报告中荣获三项殊荣,入选云原生计算ÿ…...
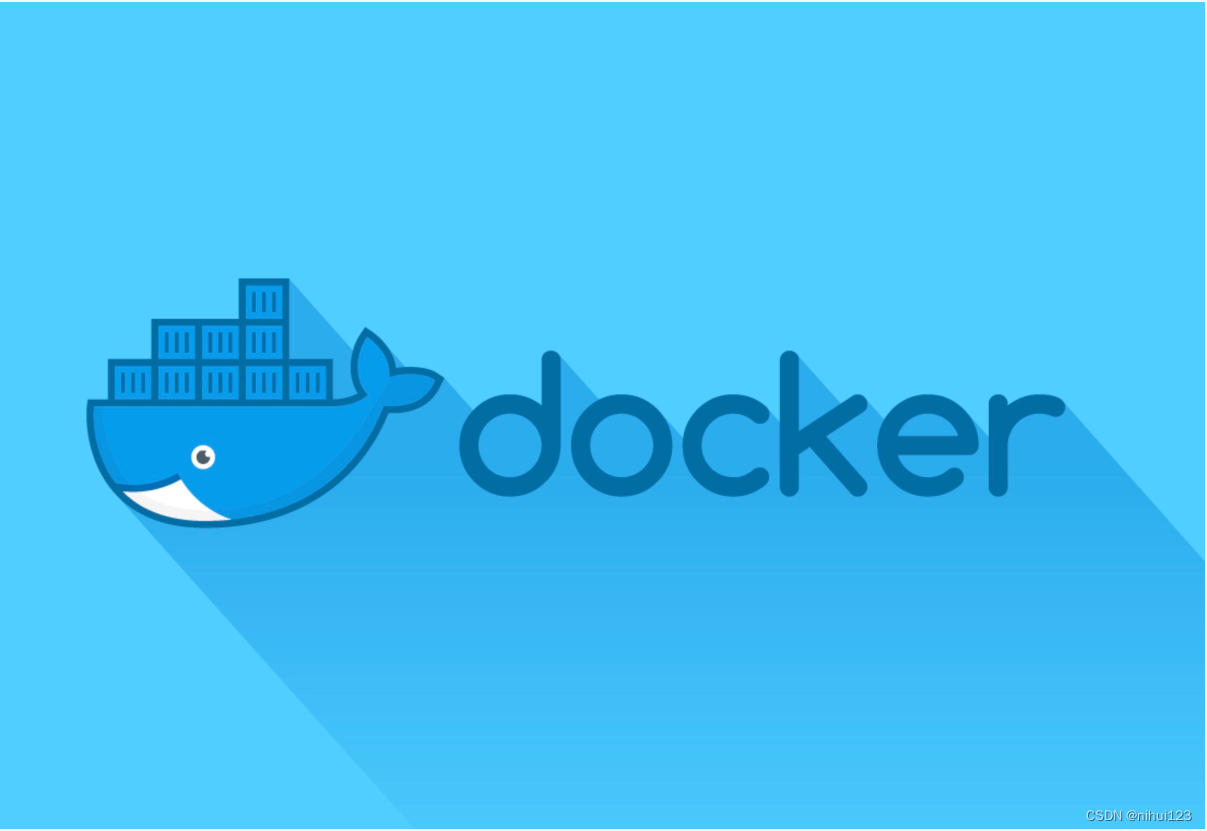
Docker实战-操作Docker容器实战(一)
导语 在之前的分享中,我们介绍了关于如何去操作Docker镜像,下面我们来看看如何去操作容器。 简单来讲,容器是镜像运行的一个实例,与镜像不同的是镜像只能作为一个静态文件进行读取,而容器是可以在运行时进行写入操…...
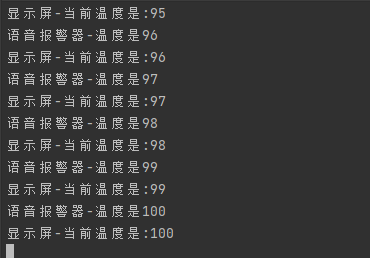
c#设计模式-行为型模式 之 观察者模式
定义: 又被称为发布-订阅(Publish/Subscribe)模式,它定义了一种一对多的依赖关系,让多个观察者 对象同时监听某一个主题对象。这个主题对象在状态变化时,会通知所有的观察者对象,使他们能够自 …...
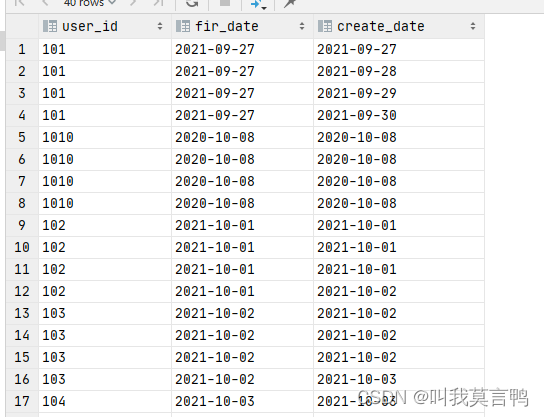
开窗积累之学习更新版
1. 开窗使用1之 count range between current row and current row 将相同排序字段的值进行函数计算 selectsku_id,substr(create_date,1,7) date_month,order_id,create_date,sku_num*price,sum(sku_num*price) over (partition by sku_id order by substr(create_date,1,7)…...
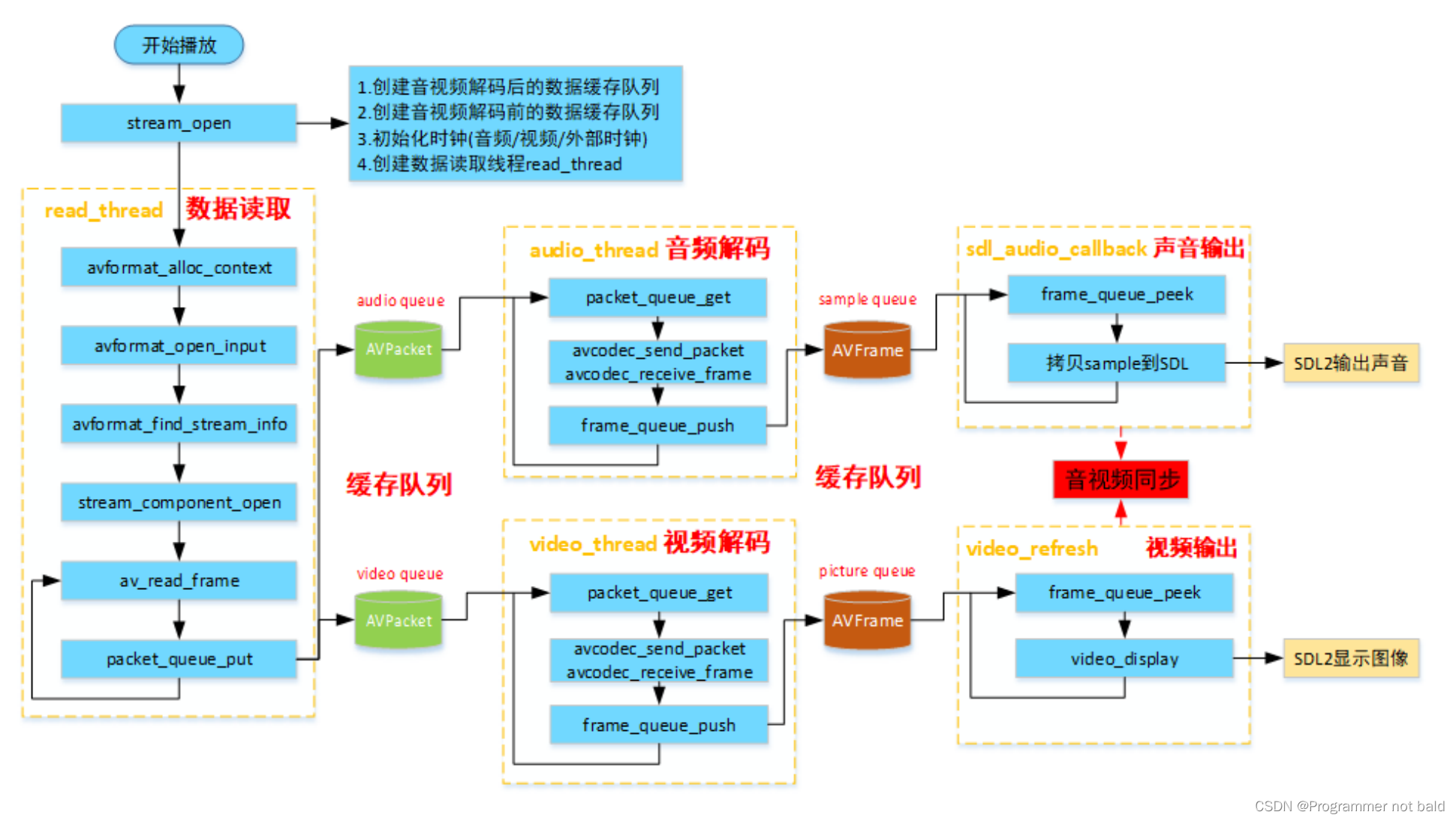
ffplay简介
本文为相关课程的学习记录,相关分析均来源于课程的讲解,主要学习音视频相关的操作,对字幕的处理不做分析 ffplay播放器的意义 ffplay.c是FFmpeg源码⾃带的播放器,调⽤FFmpeg和SDL API实现⼀个⾮常有⽤的播放器。 ffplay实现了播…...

mysql之limit语句详解
一、介绍 LIMIT是MySQL内置函数,其作用是用于限制查询结果的条数。 二、使用 1. 语法格式 LIMIT [位置偏移量,] 行数 其中,中括号里面的参数是可选参数,位置偏移量是指MySQL查询分析器要从哪一行开始显示,索引值从0开始ÿ…...

4.while循环
1、while语句的语法结构如下: while语句可以在条件表达式为真的前提下,循环执行指定的一段代码,直到表达式不为真时结束循环。 1.1while语法结构 while(条件表达式){// 循环体} 执行思路: 1、执行思路 当条件表达式结果为tru…...
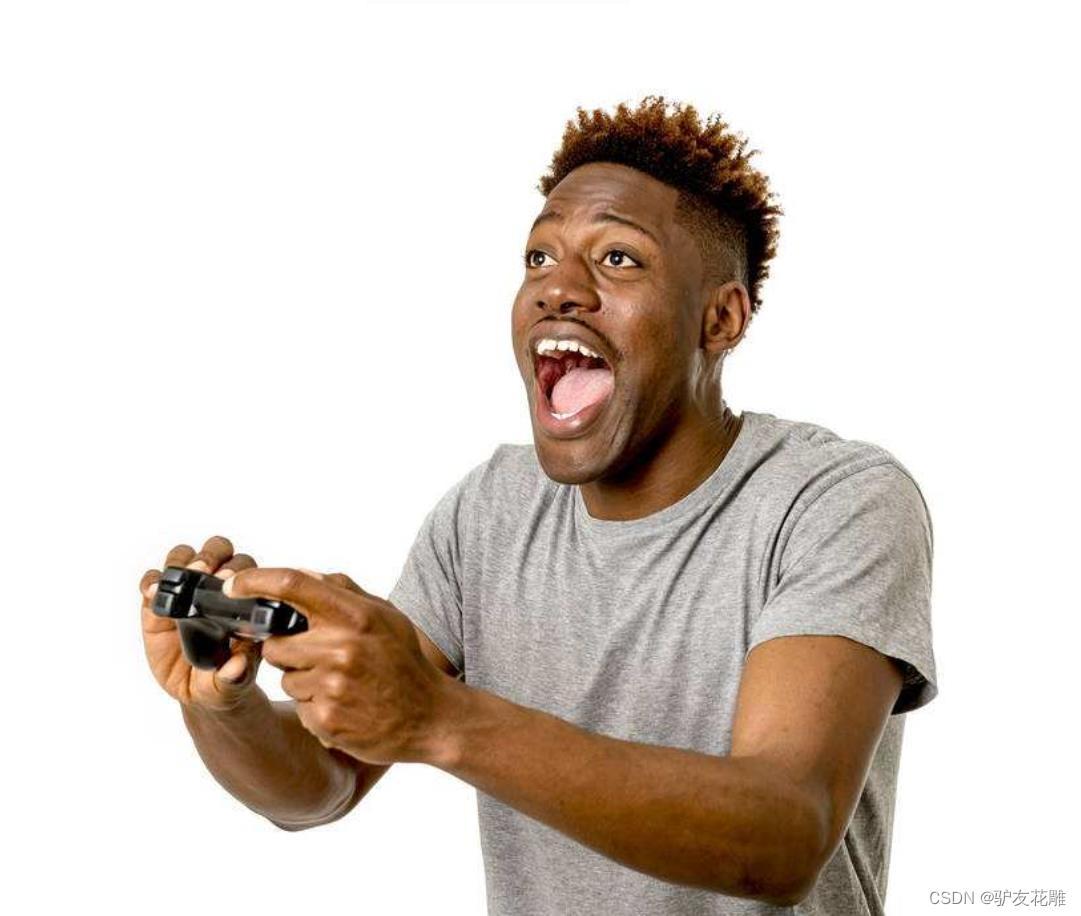
【雕爷学编程】 MicroPython动手做(35)——体验小游戏2
知识点:什么是掌控板? 掌控板是一块普及STEAM创客教育、人工智能教育、机器人编程教育的开源智能硬件。它集成ESP-32高性能双核芯片,支持WiFi和蓝牙双模通信,可作为物联网节点,实现物联网应用。同时掌控板上集成了OLED…...

mouseover 和 mouseenter
mouseover 和 mouseenter 事件是 JavaScript 中常用的两个鼠标事件,它们有一些区别: 触发条件: mouseover 事件在鼠标指针从元素外部进入元素内部时触发,包括子元素。换句话说,只要鼠标进入元素或其子元素,就会触发 mo…...
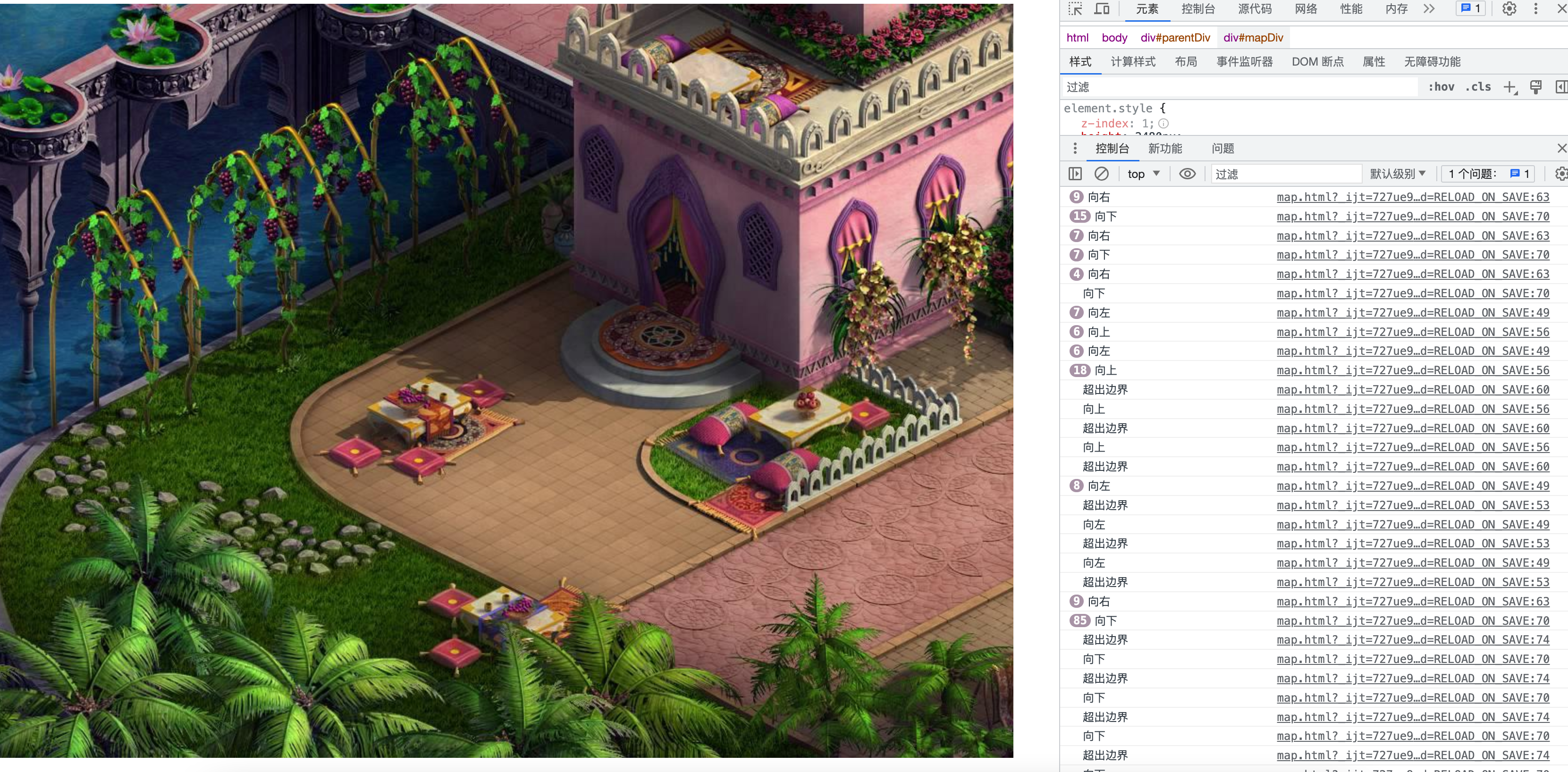
[JavaScript游戏开发] 绘制Q版地图、键盘上下左右地图场景切换
系列文章目录 第一章 2D二维地图绘制、人物移动、障碍检测 第二章 跟随人物二维动态地图绘制、自动寻径、小地图显示(人物红点显示) 第三章 绘制冰宫宝藏地图、人物鼠标点击移动、障碍检测 第四章 绘制Q版地图、键盘上下左右地图场景切换 文章目录 系列文章目录前言一、本章节…...
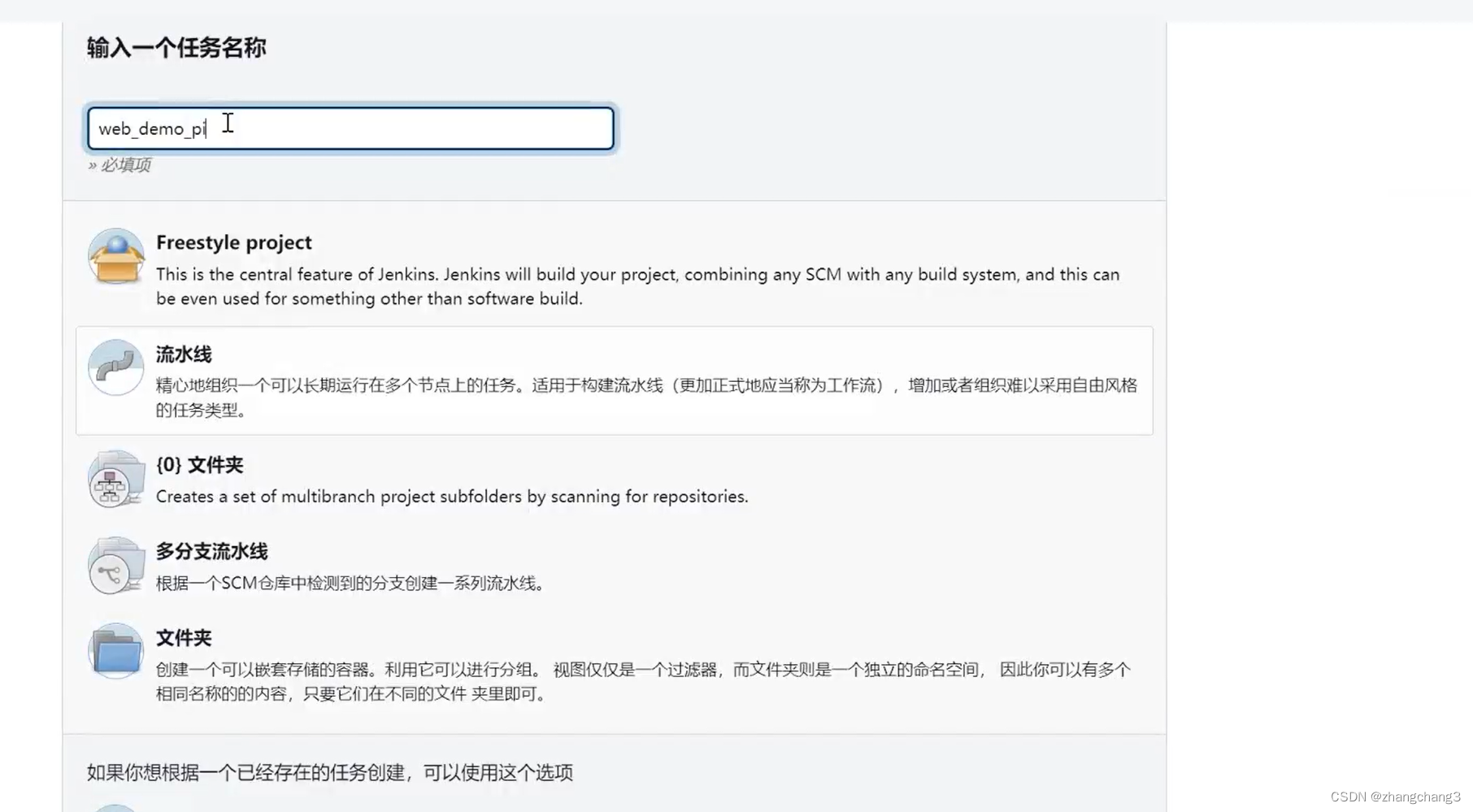
CI/CD持续集成持续发布(jenkins)
1.背景 在实际开发中,我们经常要一边开发一边测试,当然这里说的测试并不是程序员对自己代码的单元测试,而是同组程序员将代码提交后,由测试人员测试; 或者前后端分离后,经常会修改接口,然后重新…...
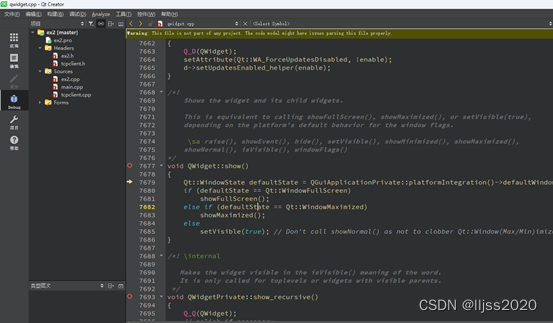
Qt5.14.2+QtCreator+PDB 查看源码
1. 在Creator添加源码 2. 安装PDB文件 Qt下载时没有整合最新的PDB文件下载,如果没有安装PDB文件,即使安装了src也无法调试。 双击MaintenanceTool.exe->设置->资料档案库->临时资料档案库->添加按钮,添加如下下载源:…...

DOM基础获取元素+事件基础+操作元素
一.DOM简介 DOM,全称“Document Object Model(文档对象模型)”,它是由W3C定义的一个标准。 在实际开发中,我们有时候需要实现鼠标移到某个元素上面时就改变颜色,或者动态添加元素或者删除元素等。其实这些效…...

MATLAB——感知神经网络学习程序
学习目标:从学习第一个最简单的神经网络案例开启学习之路 感知器神经网络 用于点的分类 clear all; close all; P[0 0 1 1;0 1 0 1]; %输入向量 T[0 1 1 1]; %目标向量 netnewp(minmax(P),1,hardlim,lea…...
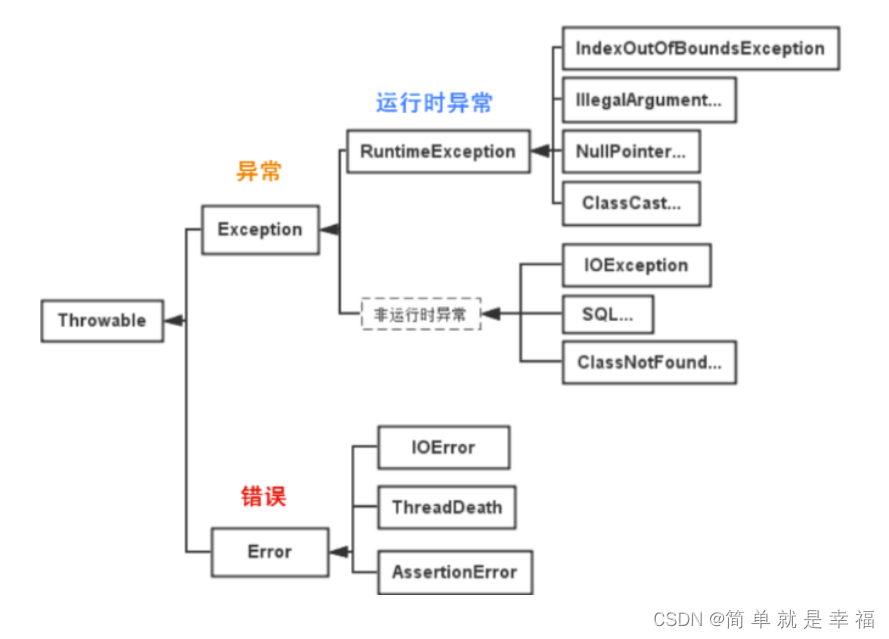
SpringBoot中事务失效的原因
SpringBoot中事务失效的原因 文章目录 SpringBoot中事务失效的原因一、事务方法非public修饰二、非事务方法调用事务方法三、事务方法的异常被捕获四、事务异常类型不对五、事务传播行为不对六、没有被Spring管理6.1、暴漏代理对象6.2、使用代理对象 常见的事务失效原因包括如下…...

Webstorm的一些常用快捷键
下面是Webstorm的一些常用快捷键: ctrl shift n: 打开工程中的文件,目的是打开当前工程下任意目录的文件。ctrl j: 输出模板ctrl b: 跳到变量申明处ctrl alt T: 围绕包裹代码(包括zencoding的Wrap with Abbreviation)ctrl []: 匹配 {}[]ctrl F1…...