【Qt QAxObject】使用 QAxObject 高效任意读写 Excel 表
1. 用什么操作 Excel 表
Qt
的官网库中是不包含 Microsoft Excel
的操作库,关于对 Microsoft Excel
的操作库可选的有很多,包含基于 Windows
系统本身的 ActiveX
、Qt Xlsx
、xlsLib
、LibXL
、qtXLS
、BasicExcel
、Number Duck
。
库 | .xls | .xlsx | 读 | 写 | 平台 |
---|---|---|---|---|---|
Qt Xlsx | ❌ | ✔️ | ✔️ | ✔️ | 跨平台 |
xls | ✔️ | ❌ | ❌ | ✔️ | 跨平台 |
libxls | ✔️ | ❌ | ❌ | ✔️ | 跨平台 |
libXL | ✔️ | ✔️ | ✔️ | ✔️ | 跨平台 |
ActiveX | ✔️ | ✔️ | ✔️ | ✔️ | Windows原生 |
qtXLS | ✔️ | ❌ | ✔️ | ✔️ | Windows |
BasicExcel | ✔️ | ❌ | ✔️ | ✔️ | Windows |
Number Duck | ✔️ | ❌ | ✔️ | ✔️ | Windows,Linux |
本文采用基于 Windows
的 ActiveX
对象,在 Qt
中也就是 QAxObjcet
实现读写 Excel
表。
2. QAxObject 简介
在介绍 QAxObject
之前,首先简单了解一下 Windows
上的 COM
组件对象模型,COM
是一个独立于平台的分布式面向对象的系统,用于创建可以交互的二进制软件组件。 COM
是 Microsoft
的 OLE
(复合文档的基础技术,) 和 ActiveX
(支持 Internet
的组件) 技术。可以使用各种编程语言创建 COM
对象。 面向对象的语言(如 C++
)提供了简化 COM
对象的实现的编程机制。 这些对象可以位于单个进程中、其他进程中,甚至在远程计算机上也是如此。
COM
组件模型对象相信介绍可查看:https://download.csdn.net/download/qq_36393978/88268235
而在 Qt
中的 QAxObject
是对 COM
组件模型对象的封装,QAxObject
派生自QAxBase
,QAxBase
提供了一组 API
通过 IUnknown
指针直接访问 COM
对象,具体结构如下图。
而在 Windows
上的 Excel
也是一个这样的 COM
对象,因此,可以采用在 Qt
中使用 QAxObjcet
实现对 Excel
的操作,其基本的操作流程如下:
如上图描述了 Excel
的层次结构,Excel.Application
对象 → WorkBooks
对象 → WorkBook
对象 → WorkSheet
对象 → Range
对象,1
个 excel
有一个 Application
对象,1
个 Application
对象有多个 Workbook
对象组成,这些 Workbook
对象由 Workbooks
对象统一管理,Workbook
对象下包含若干个 WorkSheet
,这些 WorkSheet
对象由 WorkSheets
对象统一管理,WorkSheet
下面的 Range
对象,对应这 WorkSheet
里面的表格单元了。箭头表示获取其子对象,获取方式需调用 querySubObject()
函数获取子对象实例,各个子对象调用 dynamicCall()
函数执行对各自的具体操作。
3. 基本使用方法
3.1. 包含相关文件
要使用 QAxObject
首先要在 .pro
文件中添加其模块名称 axcontainer
,这样工程文件在编译的时候就会加载该模块。
// project.pro
QT += axcontainer
在头文件中需要包含如下几个头文件
#include <QString>
#include <QFileDialog>
#include <QAxObject>
#include <QVariant>
#include <QVariantList>
3.2. 创建 Excel 进程,获取 Excel 工作簿集
打开文件夹选择 Excel
文件,创建 Excel
进程:
// 打开文件夹
QString strFilePathName = QFileDialog::getOpenFileName(this, QStringLiteral("选择Excel文件"),"", tr("Exel file(*.xls *.xlsx)"));
if(strFilePathName.isNull()) {return ;
}
QAxObject *excel = new QAxObject(this);if (excel->setControl("Excel.Application")) { // 加载 Microsoft Excel 控件
} else {excel->setControl("ket.Application"); // 加载 WPS Excel 控件
}excel->setProperty("Visible", false); // 不显示 Excel 窗体
QAxObject* workBooks = excel->querySubObject("WorkBooks"); //获取工作簿集合
workBooks->dynamicCall("Open(const QString&)", strFilePathName); //打开打开已存在的工作簿
QAxObject* workBook = excel->querySubObject("ActiveWorkBook"); //获取当前工作簿
3.3. 获取电子表格集
每个 Excel
工作簿中都可以包含若干电子表格 Sheet
:
QAxObject* sheets = workBook->querySubObject("Sheets"); //获取工作表集合,Sheets也可换用WorkSheets
获取需要操作的工作表:
QAxObject* sheet = workBook->querySubObject("WorkSheets(int)", 1);//获取工作表集合的工作表1,即sheet1
3.4. 选取需要操作的单元格范围
每页电子表格包含多个单元格,在操作之前需要选取所要操作的单元格范围:
选取当前页面所有已使用单元格:
//获取该sheet的使用范围对象(一般读取 Excel 时会选取全部范围)
QAxObject* usedRange = sheet->querySubObject("UsedRange");
选取指定范围的单元格:
//获取 sheet 的指定范围(一般写入数据时,会选取指定范围)
QAxObject* usedRange = sheet->querySubObject("Range(const QString)", "A1:C12");
这里的 A1:C12
则表示选取,A
到 C
列的 1~12
行单元格;若写成 A1:A12
则表示选取,A
列的 1~12
行单元格;当然你也可以写成 A1:A1
则表示只选取 A1
这一个单元格。
选择指定单元格:
//获取 sheet 的指定范围(一般修改数据时,会选取指定单元格)
QAxObject* usedRange = sheet->querySubObject("Range(QVariant,QVariant)", "A6");
这里的 A6
,则表示选取 A6
这个单元格。
[注]:获取 UsedRange 操作将会得到一个动态创建 (new) 出来的对象。
3.5. 读取单元格范围内容
当选取好要操作的单元各范围后,那么这里介绍如何读取选取范围内所有的单元格内容:
QVariant var = usedRange->dynamicCall("Value");
delete usedRange;
需要注意的是上文代码中除了获取单元格范围 (UsedRange
) 操作之外,其余得到的都是该对象的引用,不会占用内存空间,不需要释放,而获取单元格适用范围 (UsedRange
) 则得到一个 new 出来新分配的对象,因此在读取或写入操作结束后需要手动释放该对象。
QVariant
类是一个数据联合体,用来存放读取到的 Excel
数据。而事实上通过调用 dynamicCall("Value")
读到的数据类型是 QList<QList<QVariant>>
类型的,因此要将读到的 var
转换为该类型的数据。
QList<QList<QVariant>> xls_data;QVariantList varRows = var.toList();
if (varRows.isEmpty()) {return;
}const int rowCount = varRows.size();
this->excel.rowCount = rowCount;QVariantList rowData;for (int i = 0; i < rowCount; i++){rowData = varRows[i].toList();if (rowData.count() > this->excel.columnCount) {this->excel.columnCount = rowData.count();}this->xls_data.push_back(rowData);
}
3.6. 将读取到的数据用 QTableView 展示出来
这里的 TbleView_table1
是在 Forms
中的直接拖拽的 QTableView
控件。
QStandardItemModel *tab_model;
for (int i = 0; i < xls_data.count(); i++) {for (int j = 0; j < xls_data.at(i).count(); j++) {QStandardItem *item = new QStandardItem(QString(xls_data.at(i).at(j).toByteArray()));tab_model->setItem(i, j, item);//delete item;}
}
this->ui->TbleView_table1->setModel(tab_model);
3.7. 向选取的单元格范围写入数据
想必各位也注意到了,在 Excel
中横轴坐标都是用 A~Z
字母表示为了与纵轴的表示方式区别开来,而我们在操作是常用的则是以数字形式,或者说在设置循环中用数字方式更为方便,因此这里需要实现一个数字转 Excel
横坐标的函数,如下。
QString ExcelProcess::to26AlphabetString(int data)
{QChar ch = (QChar)(data + 0x40);//A对应0x41return QString(ch);
}void ExcelProcess::convertToColName(int data, QString &res)
{Q_ASSERT(data > 0 && data < 65535);int tempData = data / 26;if (tempData > 0) {int mode = data % 26;convertToColName(mode, res);convertToColName(tempData, res);} else {res=(to26AlphabetString(data) + res);}
}
按照指定行、列,一行一行的添加要写入的数据。
QList<QList<QVariant>> data_total;int row = 50 - 30, col = 100 - 10; // 表示:选取范围为,30~50 行,10~100 列for (int i = 0; i < row; i++) {QList<QVariant> data_row;for (int j = 0; j < col; j++) {data_row.append("ImagineMiracle");}data_total.append(QVariant(data_row));
}
QString row_s, row_e, col_s, col_e, targetRange;
convertToColName(30, row_s);
convertToColName(50, row_e);
convertToColName(10, col_s);
convertToColName(100, col_e);
targetRange= col_s + row_s + ":" + col_e + row_e ;QAxObject* sheet = workBook->querySubObject("WorkSheets(int)", 1);//获取工作表集合的工作表1,即sheet1QAxObject* usedRange = sheet->querySubObject("Range(const QString)", target);
usedRange->setProperty("NumberFormat", "@"); // 设置所有单元格为文本属性
usedRange->dynamicCall("SetValue(const QVariant&", QVariant(data_total));
workBook->dynamicCall("Save()"); // 保存文件
delete usedRange;
3.8. 退出操作
当执行完所有操作后,需要关闭并退出 Excel
,代码如下:
workBook->dynamicCall("Close()"); //关闭工作簿
excel->dynamicCall("Quit()"); //退出进程delete excel;
4. 示例演示
下面是笔者写的一个简单的 Excel
表操作的代码,其功能包含
1.打开并读取 Excel
表内容;
2.根据指定表格中数据的范围计算范围内数据量并写入表格;
3.根据数据量计算数据的所在范围。
完成工程下载链接:ExcelProcess.zip
文件列表如下:
运行效果演示。
5. 附
这里贴出所有源文件的代码:
5.1. ExcelPrecess.pro
QT += core gui axcontainergreaterThan(QT_MAJOR_VERSION, 4): QT += widgetsCONFIG += c++17
RC_ICONS += pic/ExcelTool.ico# You can make your code fail to compile if it uses deprecated APIs.
# In order to do so, uncomment the following line.
#DEFINES += QT_DISABLE_DEPRECATED_BEFORE=0x060000 # disables all the APIs deprecated before Qt 6.0.0SOURCES += \main.cpp \excelprocess.cppHEADERS += \excelprocess.hFORMS += \excelprocess.ui# Default rules for deployment.
qnx: target.path = /tmp/$${TARGET}/bin
else: unix:!android: target.path = /opt/$${TARGET}/bin
!isEmpty(target.path): INSTALLS += targetRESOURCES += \pic.qrc
5.2. excelprocess.h
#ifndef EXCELPROCESS_H
#define EXCELPROCESS_H#include "ui_excelprocess.h"
#include <QMainWindow>
#include <QLineEdit>
#include <QPushButton>
#include <QString>
#include <QLabel>
#include <QFileDialog>
#include <QStandardPaths>
#include <QDebug>
#include <QDragEnterEvent>
#include <QDropEvent>
#include <QMimeData>
#include <QMovie>
#include <QScopedPointer>
#include <QList>
#include <QAxObject>
#include <QVariant>
#include <QVariantList>
#include <QStandardItemModel>
#include <QThread>
#include <QIcon>QT_BEGIN_NAMESPACE
namespace Ui { class ExcelProcess; }
QT_END_NAMESPACE#define APPNAME ("ExcelTool v1.3.0 build 20230828")typedef struct {QAxObject *excel;QAxObject *workBooks;QAxObject *current_workBook;QAxObject *workSheets;QAxObject *current_workSheet;QAxObject *usedRange;QAxObject *rows;QAxObject *columns;int sheetCount, rowCount, columnCount;}ExcelFile;#define MODECOUNT (3)
static QString excel_mode[MODECOUNT] = {"Null", "Calc", "Range"};typedef struct {int sheet;int col_src;//int col;int col_dest;
} CalcMode;class ExcelProcess : public QMainWindow
{Q_OBJECTprivate slots:void showFiles(void);void on_PBtn_View_clicked();void on_ComBox_Mode_currentIndexChanged(int index);void on_Btn_CalcRun_clicked();void on_LEdit_FilePath_textChanged(const QString &arg1);void on_Btn_RangeRun_clicked();protected:void dragEnterEvent(QDragEnterEvent *event); // 拖动进入事件void dropEvent(QDropEvent *event); // 放下事件public:ExcelProcess(QWidget *parent = nullptr);~ExcelProcess();void openExcelFile();void closeExcelFile();void readExcel_OneSheet(int sheet_num);void showOneSheet(int sheet_num);void excelModeDisplay_00(void);void excelModeDisplay_01(void);void excelModeDisplay_02(void);void convertToColName(int data, QString &res);QString to26AlphabetString(int data);void castListListVariant2Variant(const QList<QList<QVariant> > &cells, QVariant &res);private:Ui::ExcelProcess *ui;QString *fileName;QMovie *movie_01;ExcelFile excel;QList<QList<QVariant>> xls_data; // excel 表数据CalcMode calcMode;QStandardItemModel *tab_model;void initUi(void);void initExcel(void);};
#endif // EXCELPROCESS_H
5.3. excelprocess.cpp
#include "excelprocess.h"
#include "ui_excelprocess.h"void ExcelProcess::showFiles()
{QString str = QFileDialog::getOpenFileName(this, "File Explorer", QStandardPaths::writableLocation(QStandardPaths::DocumentsLocation),"Excel 文件(*.xls *.xlsx);;All file(*.*)");this->ui->LEdit_FilePath->setText(str.toUtf8());*this->fileName = this->ui->LEdit_FilePath->text();qDebug() << *this->fileName << "\n";return ;
}void ExcelProcess::dragEnterEvent(QDragEnterEvent *event)
{if ((!event->mimeData()->urls()[0].fileName().right(3).compare("xls")) || (!event->mimeData()->urls()[0].fileName().right(4).compare("xlsx"))) {event->acceptProposedAction();} else {event->ignore();}return ;
}void ExcelProcess::dropEvent(QDropEvent *event)
{const QMimeData *qm = event->mimeData();*this->fileName = qm->urls()[0].toLocalFile(); // 获取拖入的文件名this->ui->LEdit_FilePath->clear();this->ui->LEdit_FilePath->setText(*this->fileName);return ;
}ExcelProcess::ExcelProcess(QWidget *parent): QMainWindow(parent), ui(new Ui::ExcelProcess)
{ui->setupUi(this);this->fileName = new QString;this->tab_model = new QStandardItemModel();this->setAcceptDrops(true); // 设置主界面接收拖动进来的文件this->initUi();this->initExcel();return ;
}ExcelProcess::~ExcelProcess()
{delete ui;delete fileName;this->tab_model->clear();delete this->tab_model;if (this->excel.current_workBook != nullptr) {this->excel.current_workBook->dynamicCall("Save()");this->excel.current_workBook->dynamicCall("Close()"); //关闭文件}if (this->excel.workBooks != nullptr) {this->excel.workBooks->dynamicCall("Close()"); //关闭文件}this->excel.excel->dynamicCall("Quit(void)"); // 退出delete this->excel.workBooks;delete this->excel.excel;return ;
}void ExcelProcess::openExcelFile()
{//this->initExcel();if (this->excel.excel == nullptr) {return ;}this->excel.workBooks->dynamicCall("Open (const QString&)", *this->fileName);this->excel.current_workBook = this->excel.excel->querySubObject("ActiveWorkBook");this->excel.workSheets = this->excel.current_workBook->querySubObject("Sheets");this->excel.rowCount = 0;this->excel.columnCount = 0;this->excel.sheetCount = this->excel.workSheets->property("Count").toInt();qDebug() << "Sheet num: " << this->excel.sheetCount << "\n";}void ExcelProcess::closeExcelFile()
{if (this->excel.current_workBook != nullptr) {this->excel.current_workBook->dynamicCall("Save()");this->excel.current_workBook->dynamicCall("Close()"); //关闭文件}if (this->excel.workBooks != nullptr) {this->excel.workBooks->dynamicCall("Close()"); //关闭文件}this->excel.excel->dynamicCall("Quit(void)"); // 退出delete this->excel.workBooks;delete this->excel.excel;this->initExcel();return ;
}void ExcelProcess::readExcel_OneSheet(int sheet_num)
{if (sheet_num > this->excel.sheetCount) {return;}// 读取一个 sheetthis->excel.current_workSheet = this->excel.current_workBook->querySubObject("Sheets(int)", sheet_num);this->excel.usedRange = this->excel.current_workSheet->querySubObject("UsedRange");if (nullptr == this->excel.usedRange || this->excel.usedRange->isNull()) {return;}QVariant var = this->excel.usedRange->dynamicCall("Value");delete this->excel.usedRange;this->excel.usedRange = nullptr;// 读取一个 sheet 结束for (int i = 0; i < this->xls_data.count(); i++) {this->xls_data.value(i).clear();}this->xls_data.clear();QVariantList varRows = var.toList();if (varRows.isEmpty()) {return;}const int rowCount = varRows.size();this->excel.rowCount = rowCount;QVariantList rowData;for (int i = 0; i < rowCount; i++){rowData = varRows[i].toList();if (rowData.count() > this->excel.columnCount) {this->excel.columnCount = rowData.count();}this->xls_data.push_back(rowData);}//this->excel.current_workBook->dynamicCall("Close()");qDebug() << "Sheet:: row:" << this->excel.rowCount << "colum:" << this->excel.columnCount << "\n";this->ui->ComBox_Sheet->clear();for (int i = 1; i <= this->excel.sheetCount; i++) {this->ui->ComBox_Sheet->addItem(QString::number(i));}this->ui->ComBox_Row->clear();for (int i = 1; i <= this->excel.rowCount; i++) {this->ui->ComBox_Row->addItem(QString::number(i));}this->ui->ComBox_Column->clear();for (int i = 1; i <= this->excel.columnCount; i++) {this->ui->ComBox_Column->addItem(QString::number(i));}}void ExcelProcess::showOneSheet(int sheet_num)
{this->readExcel_OneSheet(sheet_num);this->tab_model->clear();for (int i = 0; i < this->xls_data.count(); i++) {for (int j = 0; j < this->xls_data.at(i).count(); j++) {QStandardItem *item = new QStandardItem(QString(this->xls_data.at(i).at(j).toByteArray()));this->tab_model->setItem(i, j, item);//delete item;}}this->ui->TbleView_table1->setModel(this->tab_model);//this->ui->TbleView_table1->setSectionResizeMode(QHeaderView::Stretch);//delete model;
}void ExcelProcess::excelModeDisplay_00()
{this->ui->Lab_Row->setText("Row:");this->ui->Lab_Column->setText("Row:");this->ui->ComBox_Column->clear();for (int i = 1; i <= this->excel.columnCount; i++) {this->ui->ComBox_Column->addItem(QString::number(i));}this->ui->TbleView_table1->show();this->ui->Lab_Sheet->hide();this->ui->Lab_Row->hide();this->ui->Lab_Column->hide();this->ui->ComBox_Sheet->hide();this->ui->ComBox_Row->hide();this->ui->ComBox_Column->hide();this->ui->Btn_CalcRun->hide();this->ui->Btn_RangeRun->hide();this->ui->ComBox_Mode->setCurrentIndex(0);
}void ExcelProcess::excelModeDisplay_01()
{this->ui->Lab_Row->setText("Col_s:");this->ui->Lab_Column->setText("Col_d:");this->ui->ComBox_Mode->setCurrentIndex(1);this->ui->ComBox_Row->clear();this->ui->ComBox_Column->clear();for (int i = 1; i <= this->excel.columnCount; i++) {this->ui->ComBox_Row->addItem(QString::number(i));}for (int i = 1; i <= this->excel.columnCount + 1; i++) {this->ui->ComBox_Column->addItem(QString::number(i));}this->ui->ComBox_Column->setCurrentIndex(this->ui->ComBox_Column->count() - 1);this->ui->Lab_Sheet->show();this->ui->Lab_Row->show();this->ui->Lab_Column->show();this->ui->TbleView_table1->show();this->ui->ComBox_Sheet->show();this->ui->ComBox_Row->show();this->ui->ComBox_Column->show();this->ui->Btn_CalcRun->show();this->ui->Btn_RangeRun->hide();
}void ExcelProcess::excelModeDisplay_02()
{this->ui->Lab_Row->setText("Col_s:");this->ui->Lab_Column->setText("Col_d:");this->ui->ComBox_Mode->setCurrentIndex(2);this->ui->ComBox_Row->clear();this->ui->ComBox_Column->clear();for (int i = 1; i <= this->excel.columnCount; i++) {this->ui->ComBox_Row->addItem(QString::number(i));}for (int i = 1; i <= this->excel.columnCount + 1; i++) {this->ui->ComBox_Column->addItem(QString::number(i));}this->ui->ComBox_Column->setCurrentIndex(this->ui->ComBox_Column->count() - 1);this->ui->Lab_Sheet->show();this->ui->Lab_Row->show();this->ui->Lab_Column->show();this->ui->TbleView_table1->show();this->ui->ComBox_Sheet->show();this->ui->ComBox_Row->show();this->ui->ComBox_Column->show();this->ui->Btn_CalcRun->hide();this->ui->Btn_RangeRun->show();
}void ExcelProcess::convertToColName(int data, QString &res)
{Q_ASSERT(data>0 && data<65535);int tempData = data / 26;if(tempData > 0) {int mode = data % 26;convertToColName(mode,res);convertToColName(tempData,res);} else {res=(to26AlphabetString(data)+res);}
}QString ExcelProcess::to26AlphabetString(int data)
{QChar ch = (QChar)(data + 0x40);//A对应0x41return QString(ch);
}void ExcelProcess::castListListVariant2Variant(const QList<QList<QVariant> > &cells, QVariant &res)
{QVariantList vars;const int rows = cells.size(); //获取行数for(int i = 0; i < rows; ++i){vars.append(QVariant(cells[i])); //将list(i)添加到QVariantList中 QVariant(cells[i])强制转换}res = QVariant(vars); //强制转换
}void ExcelProcess::initUi()
{this->setWindowTitle(APPNAME);this->setWindowIcon(QIcon(":/bk/pic/ExcelTool.ico"));this->ui->Lab_FilePath->setText("File Path:");this->ui->PBtn_View->setText("Open File");//this->ui->Lab_BottomBar->setText("");this->ui->Lab_Background->setText("");movie_01 = new QMovie(":/bk/pic/bk_01.gif");this->ui->Lab_Background->setGeometry(0, 0, 700, 500);this->ui->Lab_Background->setMovie(movie_01);movie_01->setScaledSize(this->ui->Lab_Background->size());movie_01->start();this->ui->Lab_Sheet->hide();this->ui->Lab_Row->hide();this->ui->Lab_Column->hide();this->ui->ComBox_Sheet->hide();this->ui->ComBox_Row->hide();this->ui->ComBox_Column->hide();this->ui->Lab_Mode->hide();this->ui->ComBox_Mode->hide();this->ui->Btn_CalcRun->hide();this->ui->Btn_RangeRun->hide();for (int i = 0; i < MODECOUNT; i++) {this->ui->ComBox_Mode->addItem(excel_mode[i]);}this->ui->ComBox_Mode->setCurrentIndex(0);this->ui->TbleView_table1->hide();return ;}void ExcelProcess::initExcel()
{this->excel.excel = new QAxObject("Excel.Application"); // 加载 excel 驱动this->excel.excel->setProperty("Visible", false);//不显示Excel界面,如果为true会看到启动的Excel界面//this->excel.excel->setProperty("Visible", true);this->excel.workBooks = this->excel.excel->querySubObject("WorkBooks");
}void ExcelProcess::on_PBtn_View_clicked()
{*this->fileName = this->ui->LEdit_FilePath->text();if ((0 == this->fileName->right(3).compare("xls")) || (0 == this->fileName->right(4).compare("xlsx")) || this->fileName->isEmpty()) {;} else {this->movie_01->stop();this->movie_01->setFileName(":/bk/pic/bk_04.gif");this->ui->Lab_Background->setMovie(movie_01);movie_01->setScaledSize(this->ui->Lab_Background->size());movie_01->start();return ;}if ("Done" == this->ui->PBtn_View->text()) {//QThread::usleep(5);this->ui->LEdit_FilePath->setEnabled(true);this->ui->PBtn_View->setText("Open File");this->tab_model->clear();this->ui->Lab_Sheet->hide();this->ui->Lab_Row->hide();this->ui->Lab_Column->hide();this->ui->ComBox_Sheet->hide();this->ui->ComBox_Row->hide();this->ui->ComBox_Column->hide();this->ui->Lab_Mode->hide();this->ui->ComBox_Mode->hide();this->ui->Btn_CalcRun->hide();this->ui->Btn_RangeRun->hide();this->ui->TbleView_table1->hide();this->movie_01->stop();this->movie_01->setFileName(":/bk/pic/bk_01.gif");this->ui->Lab_Background->setMovie(movie_01);movie_01->setScaledSize(this->ui->Lab_Background->size());movie_01->start();this->closeExcelFile();} else {if (this->ui->LEdit_FilePath->text().isEmpty()) {this->showFiles();} else {//QThread::usleep(5);this->excelModeDisplay_00();this->ui->LEdit_FilePath->setEnabled(false);this->ui->PBtn_View->setText("Done");this->movie_01->stop();this->movie_01->setFileName(":/bk/pic/bk_02.gif");this->ui->Lab_Background->setMovie(movie_01);movie_01->setScaledSize(this->ui->Lab_Background->size());movie_01->start();this->openExcelFile();this->showOneSheet(1);this->ui->TbleView_table1->setStyleSheet("background-color:transparent");this->ui->TbleView_table1->horizontalHeader()->setStyleSheet("QHeaderView::section{background:transparent},QHeaderView::Stretch");this->ui->TbleView_table1->verticalHeader()->setStyleSheet("QHeaderView::section{background:transparent}");this->ui->TbleView_table1->setCornerButtonEnabled(false);this->ui->Lab_Mode->show();this->ui->ComBox_Mode->show();this->ui->TbleView_table1->show();}}}void ExcelProcess::on_ComBox_Mode_currentIndexChanged(int index)
{switch(index) {case 0: {this->excelModeDisplay_00();break;}case 1: {this->excelModeDisplay_01();break;}case 2: {this->excelModeDisplay_02();break;}default: {break;}}return ;
}void ExcelProcess::on_Btn_CalcRun_clicked()
{this->calcMode.sheet = this->ui->ComBox_Sheet->currentText().toInt();this->calcMode.col_src = this->ui->ComBox_Row->currentText().toInt();this->calcMode.col_dest = this->ui->ComBox_Column->currentText().toInt();QString data, num1, num2, result;qDebug() << "Sheet::" << this->calcMode.sheet;this->showOneSheet(this->calcMode.sheet);QList<QVariant> data_total;for (int i = 0; i < this->excel.rowCount; i++) {data = this->xls_data.at(i).at(this->calcMode.col_src - 1).toByteArray();bool flag = true;num1.clear();num2.clear();for (int i_d = 0; i_d < data.length(); i_d++) {if ('-' == data.at(i_d)) {flag = false;continue;}if (flag) {num1 += data.at(i_d);} else {num2 += data.at(i_d);}}QList<QVariant> data_row;result = QString::number(num2.toInt() - num1.toInt());qDebug() << "num1:" << num1 << "num2:" << num2 << "res:" << result << "\n";data_row.append(QString::number(num2.toInt() - num1.toInt() + 1));data_total.append(QVariant(data_row));}QString col_num, target;this->convertToColName(this->calcMode.col_dest, col_num);qDebug() << "Col:" << col_num << "\n";target = col_num + "1:" + col_num + QString::number(this->excel.rowCount);qDebug() << target ;this->excel.current_workSheet = this->excel.current_workBook->querySubObject("Sheets(int)", this->calcMode.sheet);this->excel.usedRange = this->excel.current_workSheet->querySubObject("Range(const QString)", target);this->excel.usedRange->setProperty("NumberFormat", "@"); // 设置所有单元格为文本属性this->excel.usedRange->dynamicCall("SetValue(const QVariant&", QVariant(data_total));this->excel.current_workBook->dynamicCall("Save()");//this->excel.current_workBook->dynamicCall("Close()");this->showOneSheet(this->calcMode.sheet);delete this->excel.usedRange;}void ExcelProcess::on_LEdit_FilePath_textChanged(const QString &arg1)
{if (arg1.isEmpty()) {this->movie_01->stop();this->movie_01->setFileName(":/bk/pic/bk_01.gif");this->ui->Lab_Background->setMovie(movie_01);movie_01->setScaledSize(this->ui->Lab_Background->size());movie_01->start();*this->fileName = "";} else if ((0 == arg1.right(3).compare("xls")) || (0 == arg1.right(4).compare("xlsx"))) {this->movie_01->stop();this->movie_01->setFileName(":/bk/pic/bk_03.gif");this->ui->Lab_Background->setMovie(movie_01);movie_01->setScaledSize(this->ui->Lab_Background->size());movie_01->start();*this->fileName = this->ui->LEdit_FilePath->text();} else {this->movie_01->stop();this->movie_01->setFileName(":/bk/pic/bk_04.gif");this->ui->Lab_Background->setMovie(movie_01);movie_01->setScaledSize(this->ui->Lab_Background->size());movie_01->start();}return ;
}void ExcelProcess::on_Btn_RangeRun_clicked()
{this->calcMode.sheet = this->ui->ComBox_Sheet->currentText().toInt();this->calcMode.col_src = this->ui->ComBox_Row->currentText().toInt();this->calcMode.col_dest = this->ui->ComBox_Column->currentText().toInt();QString data;int num_src = 0, num1 = 0, num2 = 0;qDebug() << "Sheet::" << this->calcMode.sheet;this->showOneSheet(this->calcMode.sheet);QList<QVariant> data_total;for (int i = 0; i < this->excel.rowCount; i++) {data = this->xls_data.at(i).at(this->calcMode.col_src - 1).toByteArray();num_src = data.toInt();num1 = num2 + 1;num2 = num_src + num1 - 1;QList<QVariant> data_row;qDebug() << "src:" << num_src << "num1:" << num1 << "num2:" << num2 << "\n";qDebug() << "range:" << (QString::number(num1) + "-" + QString::number(num2)) << "\n";data_row.append(QString::number(num1) + "-" + QString::number(num2));data_total.append(QVariant(data_row));}QString col_num, target;this->convertToColName(this->calcMode.col_dest, col_num);qDebug() << "Col:" << col_num << "\n";target = col_num + "1:" + col_num + QString::number(this->excel.rowCount);qDebug() << target ;this->excel.current_workSheet = this->excel.current_workBook->querySubObject("Sheets(int)", this->calcMode.sheet);this->excel.usedRange = this->excel.current_workSheet->querySubObject("Range(const QString)", target);this->excel.usedRange->setProperty("NumberFormat", "@"); // 设置所有单元格为文本属性this->excel.usedRange->dynamicCall("SetValue(const QVariant&", QVariant(data_total));this->excel.current_workBook->dynamicCall("Save()");//this->excel.current_workBook->dynamicCall("Close()");this->showOneSheet(this->calcMode.sheet);delete this->excel.usedRange;}
5.4. main.cpp
#include "excelprocess.h"#include <QApplication>int main(int argc, char *argv[])
{QApplication a(argc, argv);ExcelProcess w;w.show();return a.exec();
}
5.5. excelprocess.ui
<?xml version="1.0" encoding="UTF-8"?>
<ui version="4.0"><class>ExcelProcess</class><widget class="QMainWindow" name="ExcelProcess"><property name="geometry"><rect><x>0</x><y>0</y><width>700</width><height>500</height></rect></property><property name="windowTitle"><string>ExcelProcess</string></property><widget class="QWidget" name="centralwidget"><widget class="QLineEdit" name="LEdit_FilePath"><property name="geometry"><rect><x>80</x><y>420</y><width>490</width><height>24</height></rect></property></widget><widget class="QPushButton" name="PBtn_View"><property name="geometry"><rect><x>590</x><y>417</y><width>80</width><height>30</height></rect></property><property name="text"><string>Open File</string></property></widget><widget class="QLabel" name="Lab_FilePath"><property name="geometry"><rect><x>10</x><y>420</y><width>60</width><height>24</height></rect></property><property name="text"><string>File Path:</string></property></widget><widget class="QLabel" name="Lab_Background"><property name="geometry"><rect><x>700</x><y>500</y><width>53</width><height>16</height></rect></property><property name="text"><string>TextLabel</string></property></widget><widget class="QTableView" name="TbleView_table1"><property name="geometry"><rect><x>19</x><y>280</y><width>531</width><height>100</height></rect></property></widget><widget class="QComboBox" name="ComBox_Sheet"><property name="geometry"><rect><x>80</x><y>390</y><width>60</width><height>24</height></rect></property></widget><widget class="QLabel" name="Lab_Sheet"><property name="geometry"><rect><x>30</x><y>390</y><width>50</width><height>24</height></rect></property><property name="text"><string>Sheet:</string></property></widget><widget class="QLabel" name="Lab_Row"><property name="geometry"><rect><x>170</x><y>390</y><width>50</width><height>24</height></rect></property><property name="text"><string>Row_s:</string></property></widget><widget class="QComboBox" name="ComBox_Row"><property name="geometry"><rect><x>220</x><y>390</y><width>50</width><height>24</height></rect></property></widget><widget class="QLabel" name="Lab_Column"><property name="geometry"><rect><x>280</x><y>390</y><width>50</width><height>24</height></rect></property><property name="text"><string>Col_d:</string></property></widget><widget class="QComboBox" name="ComBox_Column"><property name="geometry"><rect><x>330</x><y>390</y><width>50</width><height>24</height></rect></property></widget><widget class="QComboBox" name="ComBox_Mode"><property name="geometry"><rect><x>620</x><y>280</y><width>70</width><height>24</height></rect></property></widget><widget class="QLabel" name="Lab_Mode"><property name="geometry"><rect><x>565</x><y>280</y><width>50</width><height>24</height></rect></property><property name="text"><string>Mode:</string></property></widget><widget class="QPushButton" name="Btn_CalcRun"><property name="geometry"><rect><x>620</x><y>350</y><width>70</width><height>30</height></rect></property><property name="text"><string>Run</string></property></widget><widget class="QPushButton" name="Btn_RangeRun"><property name="geometry"><rect><x>620</x><y>350</y><width>70</width><height>30</height></rect></property><property name="text"><string>Run</string></property></widget><zorder>Lab_Background</zorder><zorder>Lab_FilePath</zorder><zorder>PBtn_View</zorder><zorder>LEdit_FilePath</zorder><zorder>TbleView_table1</zorder><zorder>ComBox_Sheet</zorder><zorder>Lab_Sheet</zorder><zorder>Lab_Row</zorder><zorder>ComBox_Row</zorder><zorder>Lab_Column</zorder><zorder>ComBox_Column</zorder><zorder>ComBox_Mode</zorder><zorder>Lab_Mode</zorder><zorder>Btn_CalcRun</zorder><zorder>Btn_RangeRun</zorder></widget><widget class="QMenuBar" name="menubar"><property name="geometry"><rect><x>0</x><y>0</y><width>700</width><height>26</height></rect></property></widget><widget class="QStatusBar" name="statusbar"/></widget><resources/><connections/>
</ui>
#完
相关文章:
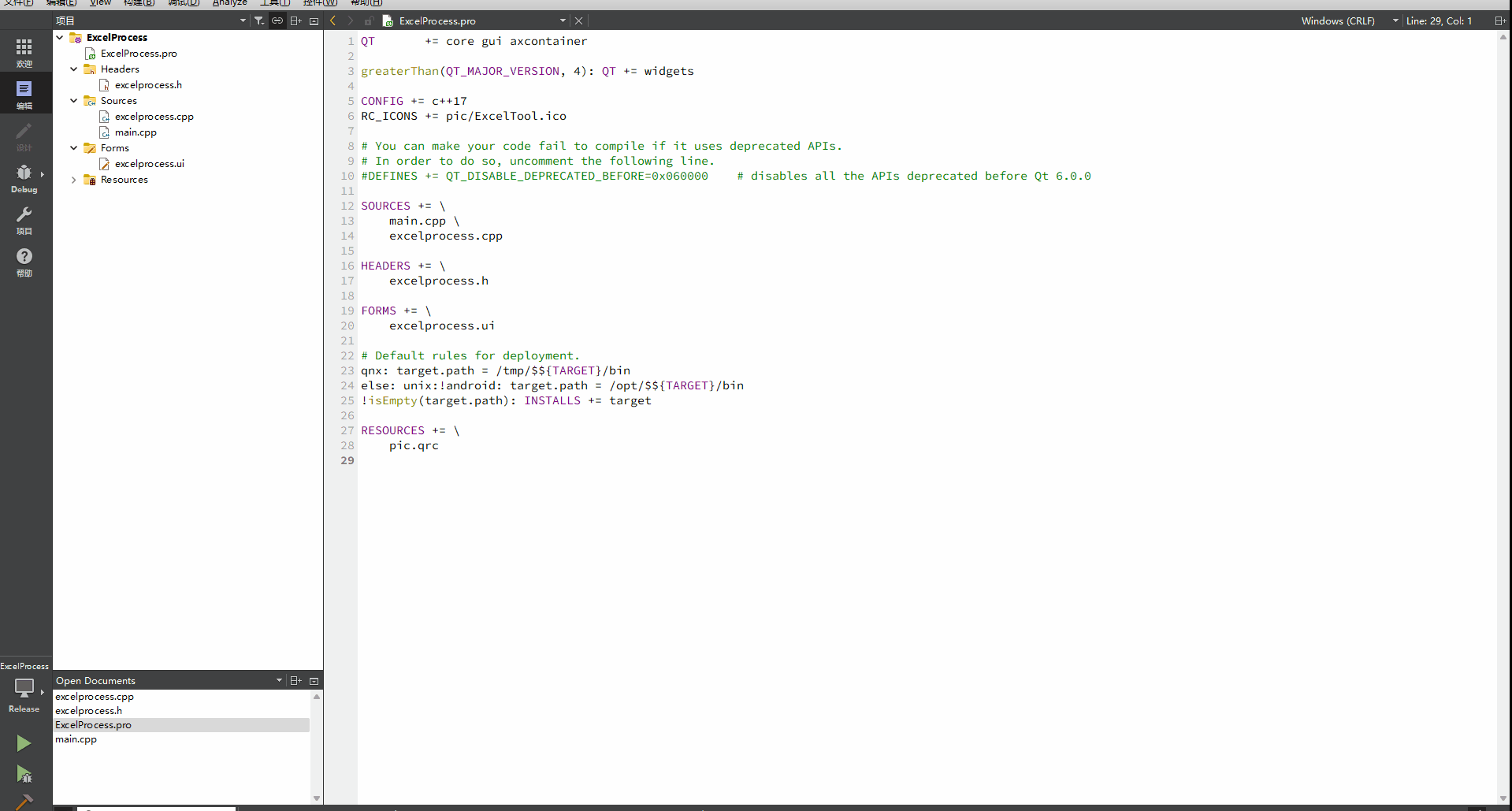
【Qt QAxObject】使用 QAxObject 高效任意读写 Excel 表
1. 用什么操作 Excel 表 Qt 的官网库中是不包含 Microsoft Excel 的操作库,关于对 Microsoft Excel 的操作库可选的有很多,包含基于 Windows 系统本身的 ActiveX、Qt Xlsx、xlsLib、LibXL、qtXLS、BasicExcel、Number Duck。 库.xls.xlsx读写平台Qt Xls…...
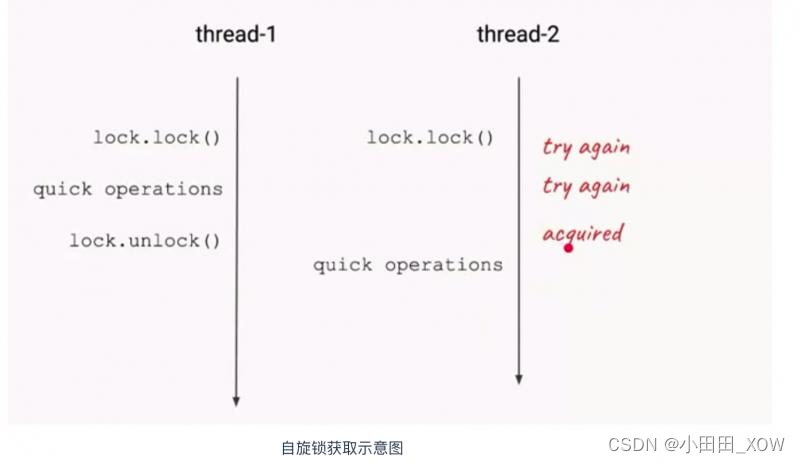
java八股文面试[多线程]——自旋锁
优点: 1. 自旋锁尽可能的减少线程的阻塞,这对于锁的竞争不激烈,且占用锁时间非常短的代码块来说性能能大幅度的提升,因为自旋的消耗会小于线程阻塞挂起再唤醒的操作的消耗 ,这些操作会导致线程发生两次上下文切换&…...
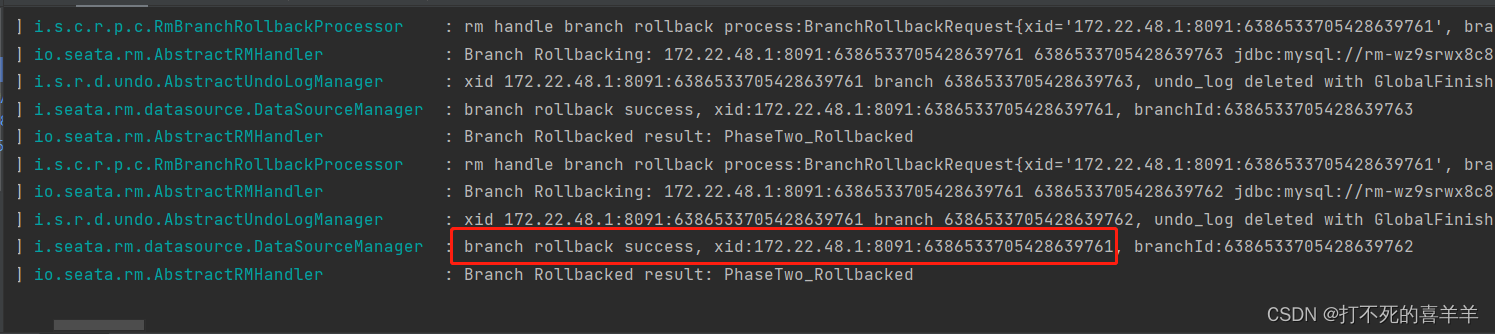
分布式系统的多数据库,实现分布式事务回滚(1.7.0 seata整合2.0.4nacos)
正文 1、解决的应用场景是分布式事务,每个服务有独立的数据库。 2、例如:A服务的数据库是A1,B服务的数据库是B2,A服务通过feign接口调用B服务,B涉及提交数据到B2,业务是在B提交数据之后,在A服…...
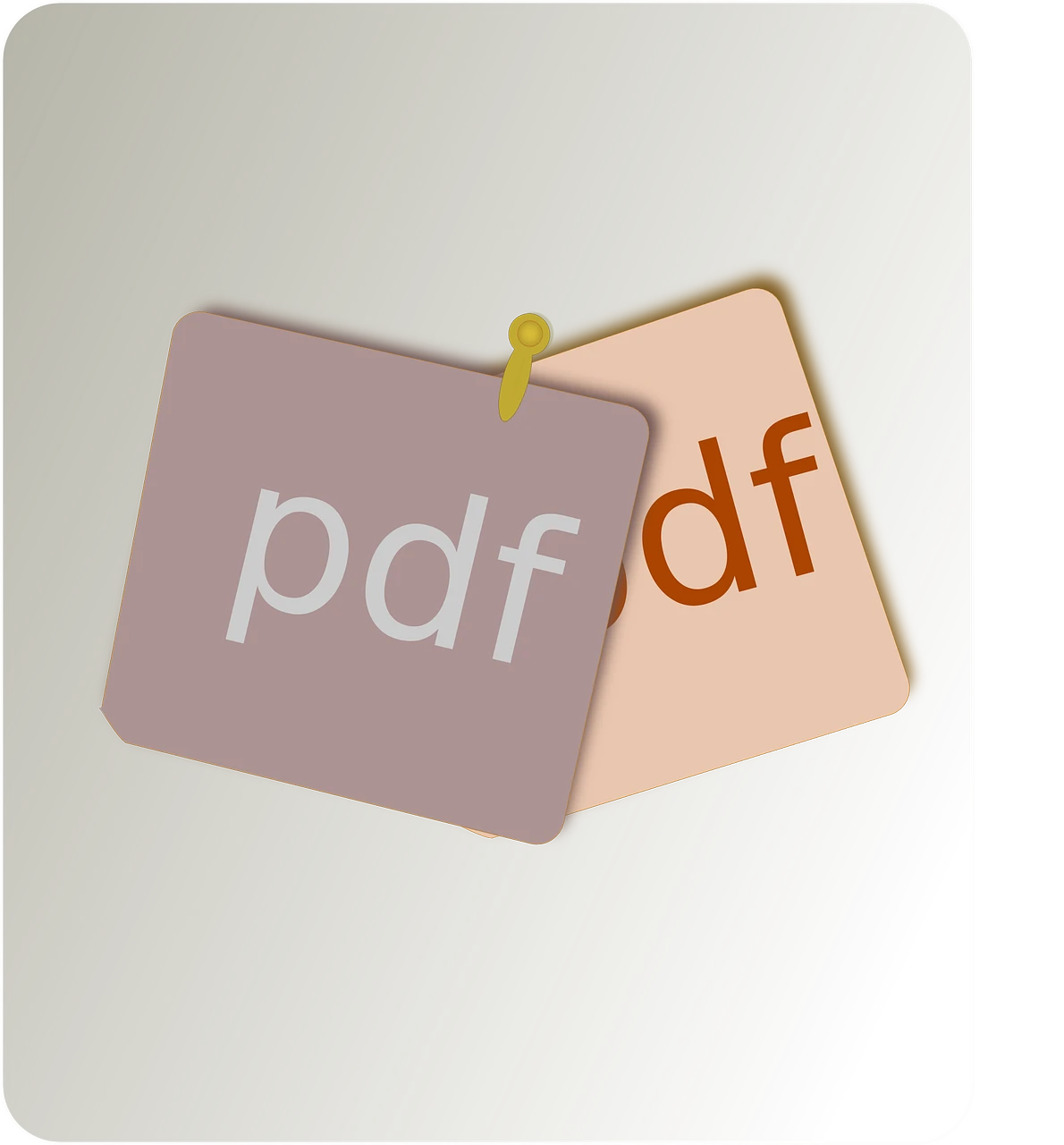
PDF可以修改内容吗?有什么注意的事项?
PDF是一种跨平台的电子文档格式,可以在各种设备上轻松阅读和共享。许多人喜欢将文档转换为PDF格式以确保格式的一致性和易读性。但是,PDF文件一般被认为是“只读”文件,即无法编辑。那么,PDF文件是否可以修改呢? 答案是…...
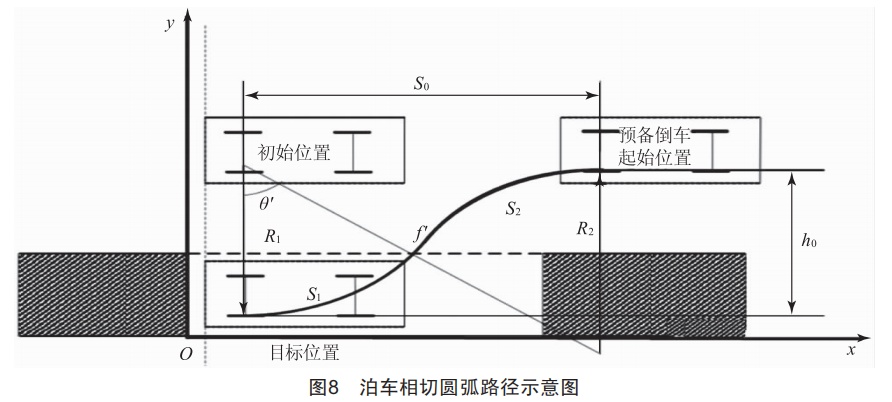
自动泊车的自动驾驶控制算法
1. 自动泊车系统 自动泊车系统(AutomatedParkingASSiSt,APA)利用车辆搭载的传感器感知车辆周边环境,扫描满足当前车辆停放的障碍物空间车位或线车位,并通过人机交互(HumanMachine Interface,HMI)获取驾驶员对目标车位的选择或自动确定目标车位,自动规划泊车路径,通过控制器向车…...

Java doc等文件生成PDF、多个PDF合并
之前写过一遍文章是 图片生成PDF。 今天继续来对 doc等文件进行pdf合并以及多个pdf合并为一个pdf。 兄弟们,还是开箱即用。 1、doc生成pdf 依赖 <!-- doc生成pdf --><dependency><groupId>com.aspose</groupId><artifactId>aspose…...
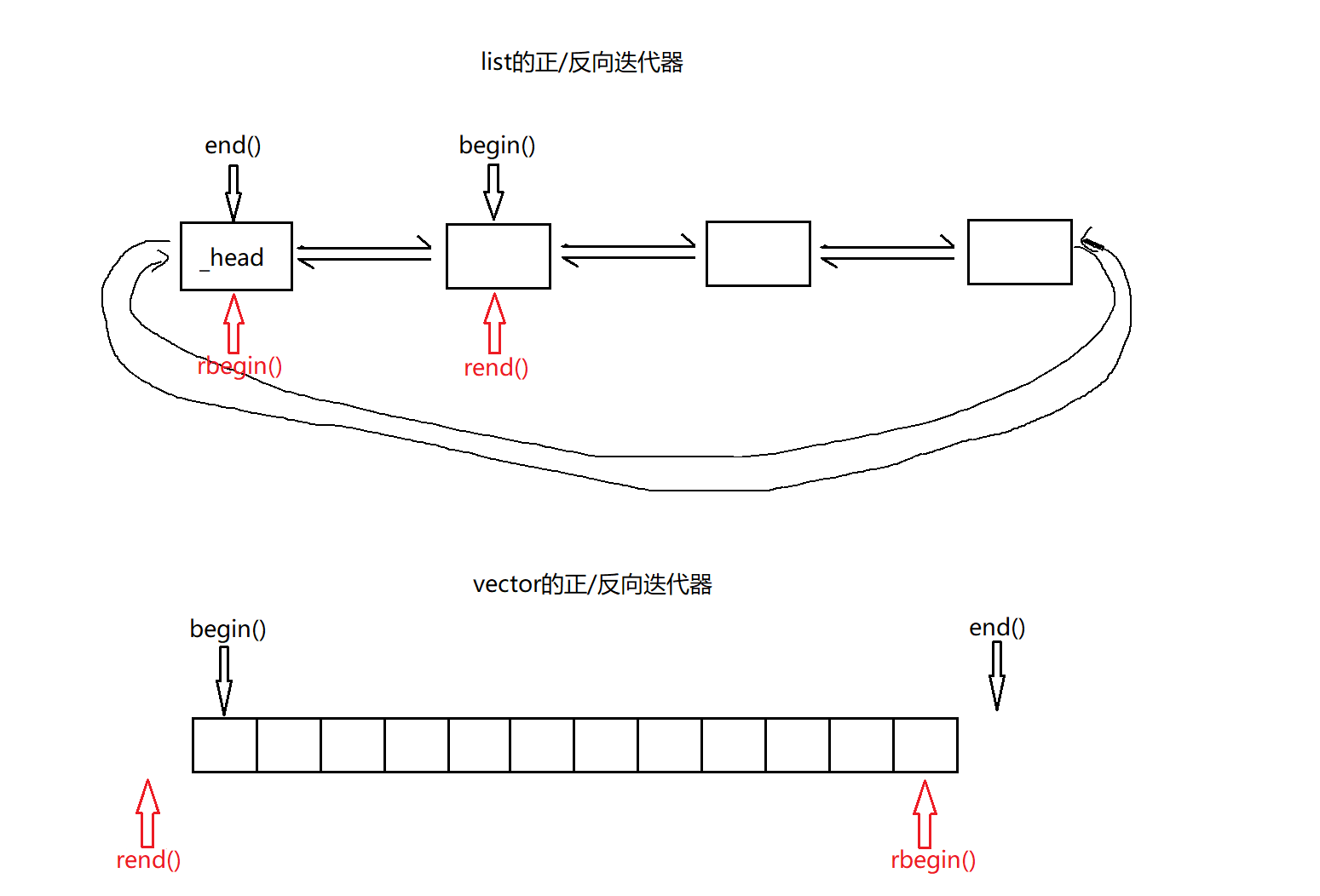
【C++】list类的模拟实现
🏖️作者:malloc不出对象 ⛺专栏:C的学习之路 👦个人简介:一名双非本科院校大二在读的科班编程菜鸟,努力编程只为赶上各位大佬的步伐🙈🙈 目录 前言一、list类的模拟实现1.1 list的…...
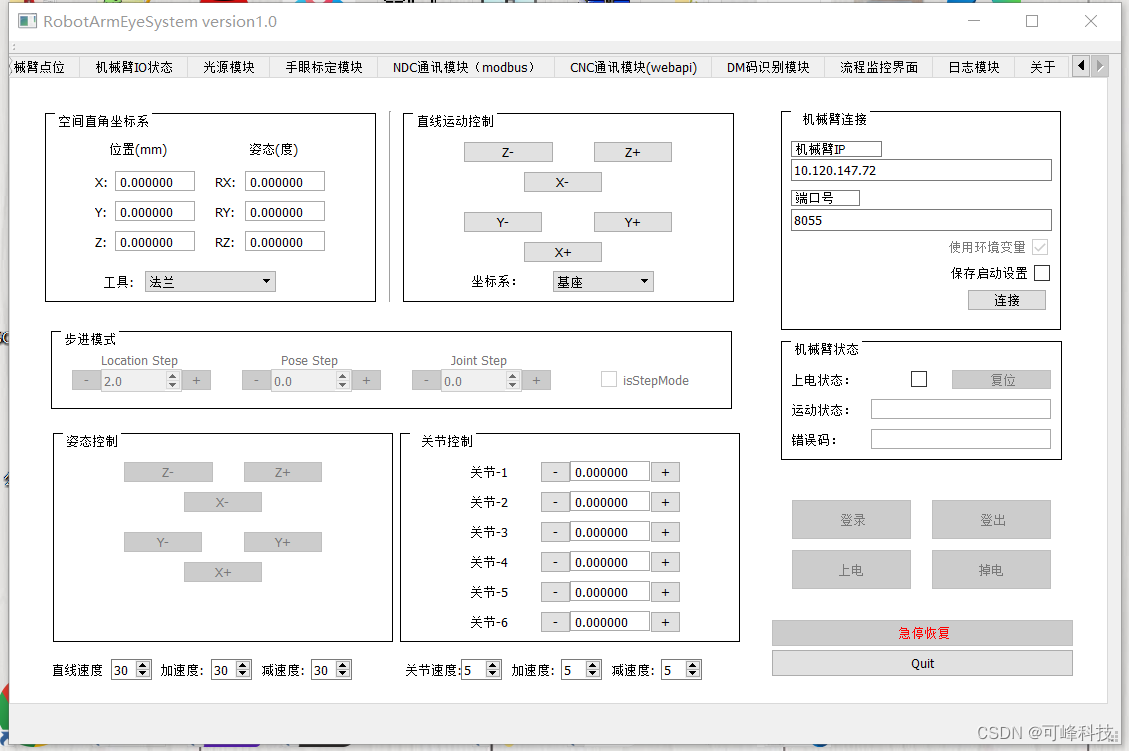
机械臂+2d相机实现复合机器人定位抓取
硬件参数 机械臂:艾利特 相机:海康相机 2d识别库:lindmod,github可以搜到 光源:磐鑫光源 软件参数 系统:windows / Linux 开发平台:Qt 开发语言:C 开发视觉库:OpenCV …...
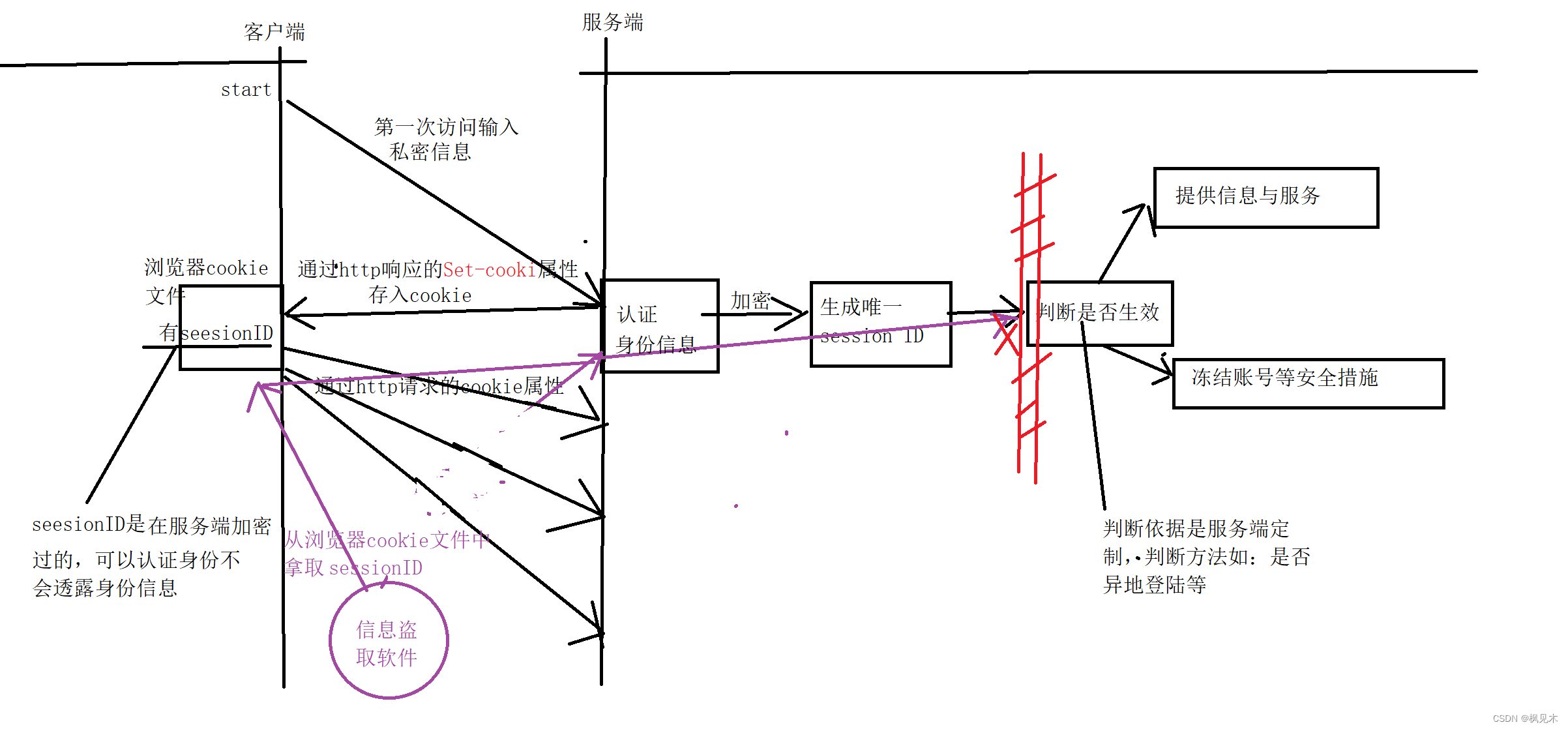
网络编程 http 相关基础概念
文章目录 表单是什么http请求是什么http请求的结构和说明关于http方法 GET和POST区别http常见状态码http响应http 请求是无状态的含义html是什么 (前端内容,了解即可)html 常见标签 (前端内容,了解即可)关于…...

LatexEasy公式渲染教程
LatexEasy使用简单的URL渲染公式为图片 https://r.latexeasy.com/image.svg?1-sin^2(x) 使用单个HTML图像标签将公式添加到任何现有网站 <img src"https://r.latexeasy.com/image.svg?1-sin^2(x)" />...
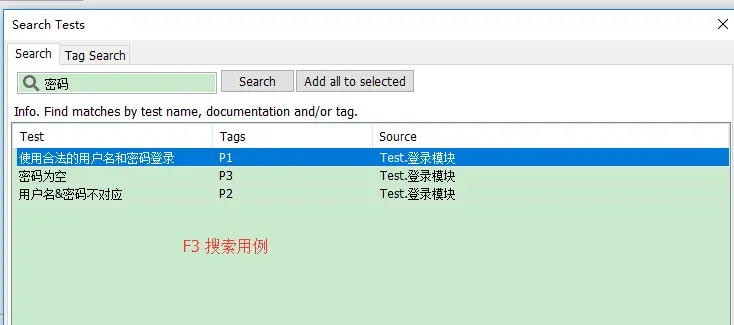
十年测试工程师叙述自动化测试学习思路
自动化测试介绍 自动化测试(Automated Testing),是指把以人为驱动的测试行为转化为机器执行的过程。实际上自动化测试往往通过一些测试工具或框架,编写自动化测试用例,来模拟手工测试过程。比如说,在项目迭代过程中,持…...
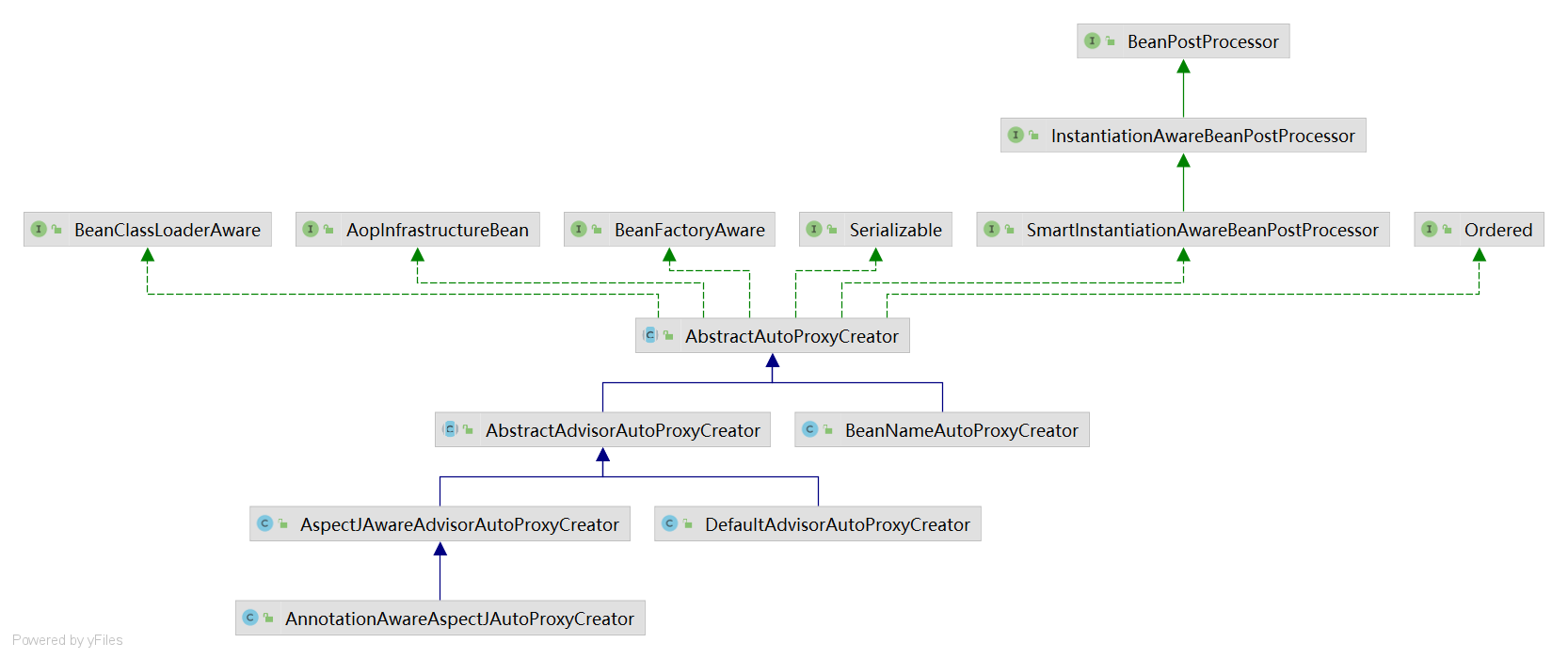
SpringAOP详解(下)
proxyFactory代理对象创建方式和代理对象调用方法过程: springaop创建动态代理对象和代理对象调用方法过程: 一、TargetSource的使用 Lazy注解,当加在属性上时,会产生一个代理对象赋值给这个属性,产生代理对象的代码为…...

主流软件漏洞跟踪 Apache RocketMQ NameServer 远程代码执行漏洞(CVE-2023-37582)
主流软件漏洞跟踪 Apache RocketMQ NameServer 远程代码执行漏洞(CVE-2023-37582) 漏洞描述影响版本安全版本如何修复可供参考的资料主流软件漏洞跟踪 Apache RocketMQ NameServer 远程代码执行漏洞(CVE-2023-37582) CVE编号 : CVE-2023-37582 利用情况 : EXP 已公开 …...
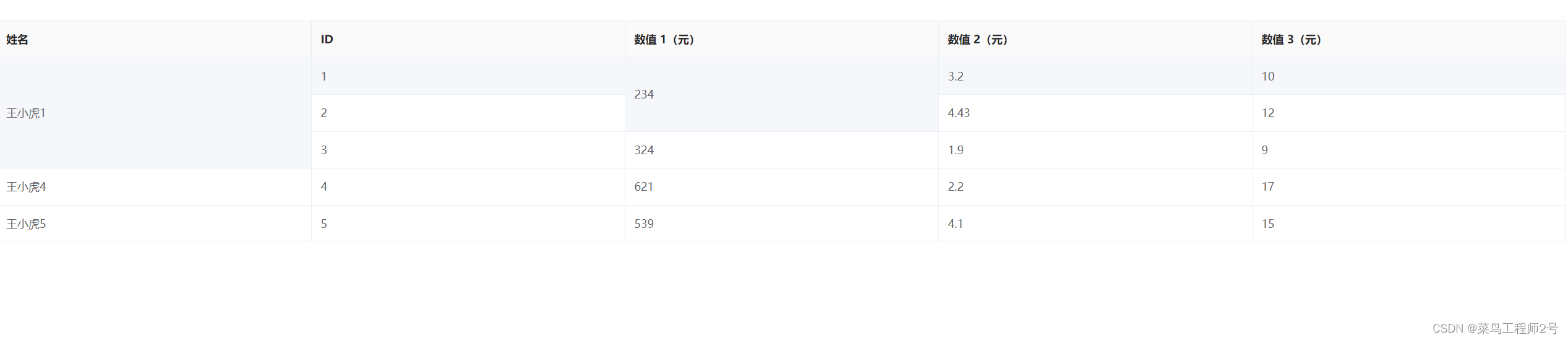
Element table根据字段合并表格(可多字段合并),附带拖拽列动态合并
效果如图,姓名 数值1 字段进行自动合并 封装合并列js - tableMerge.js // 获取列合并的行数 // params // tableData: 表格数据 // mergeId: 合并的列的字段名 export const tagRowSpan (tableData, mergeId) >{const tagArr [];let pos 0;tableData.map((i…...
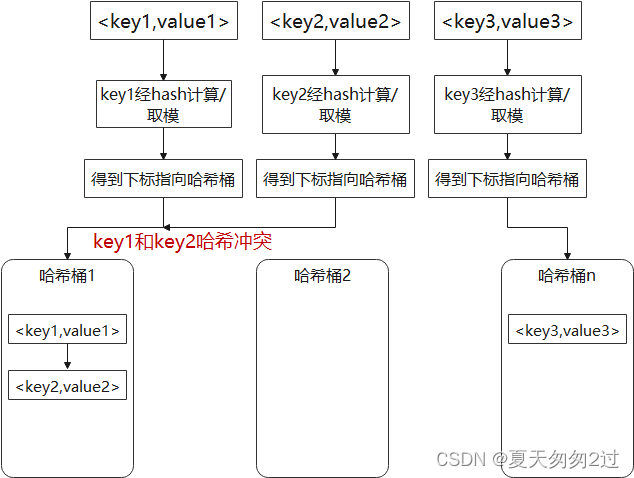
C++标准库STL容器详解
目录 C标准模板库STL容器容器分类容器通用接口 顺序容器vectorlistdeque 容器适配器queuestackpriority_queue 关联容器:红黑树setmultisetmapmultimap 关联容器:哈希表unordered_set和unordered_multisetunordered_map和unordered_multimap 附1…...

ParNew垃圾收集器(Serial+多线程)是干什么用的?
在Java中,ParNew垃圾收集器是一种垃圾收集算法,它是Serial垃圾收集器的多线程版本。它主要用于新生代(Young Generation)的垃圾收集。新生代是Java堆内存的一部分,主要用于存放新创建的对象。 ParNew垃圾收集器的设计目标是在多核CPU上并行地…...
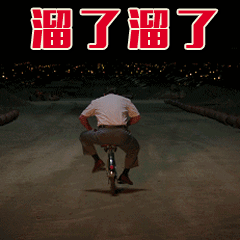
【Android】AES解密抛出异常Cipher functions:OPENSSL_internal:WRONG_FINAL_BLOCK_LENGTH
Java使用AES加密的时候没得问题,但是在解密的时候就出错了,一起来找找原因吧。 首先,Java运行的代码如下,使用AES加解密 Cipher cipher Cipher.getInstance("AES/CBC/NOPadding"); //...主要问题 可调试运行控制台抛…...

菜鸟教程《Python 3 教程》笔记(2):数据类型转换
菜鸟教程《Python 3 教程》笔记(2) 2 数据类型转换2.1 隐式类型转换2.2 显式类型转换2.2.1 int() 函数2.2.2 repr() 函数2.2.3 frozenset ()函数 2 数据类型转换 出处:菜鸟教程 - Python3 数据类型转换 Python 数据类型转换可以分为2种&…...
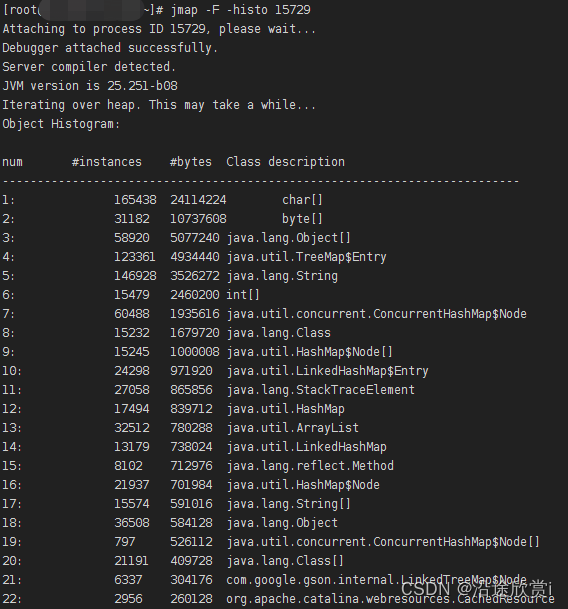
JVM运行时参数查看
常用命令查找文档站点:https://docs.oracle.com/javase/8/docs/technotes/tools/unix/index.html -XX:PrintFlagsInitial 输出所有参数的名称和默认值,默认不包括Diagnostic和Experimental的参数。可以配合 -XX:UnlockDiagnosticVMOptions和-XX:UnlockEx…...
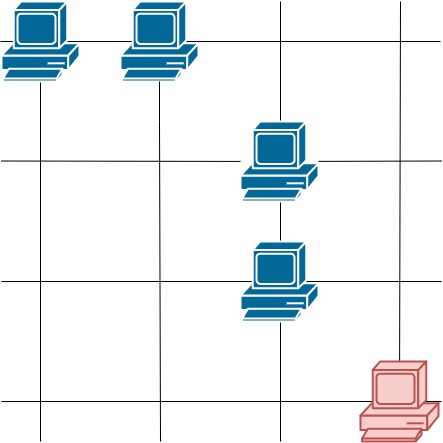
每日一题:leetcode 1267 统计参与通信的服务器
这里有一幅服务器分布图,服务器的位置标识在 m * n 的整数矩阵网格 grid 中,1 表示单元格上有服务器,0 表示没有。 如果两台服务器位于同一行或者同一列,我们就认为它们之间可以进行通信。 请你统计并返回能够与至少一台其他服务…...
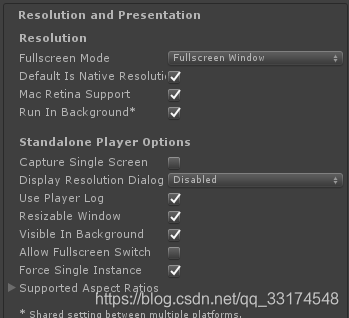
Unity打包Windows程序,概率性出现无法全屏或分辨率不匹配
排除代码和Resolution and Presentation面板设置问题 如果程序还是不能按照预期的分辨率运行,应该是系统注册表记录了对应的设置。 解决方案: 打开注册表,使用快捷键“Win” "R"组合快捷键。在打开后面键入命令:Rege…...
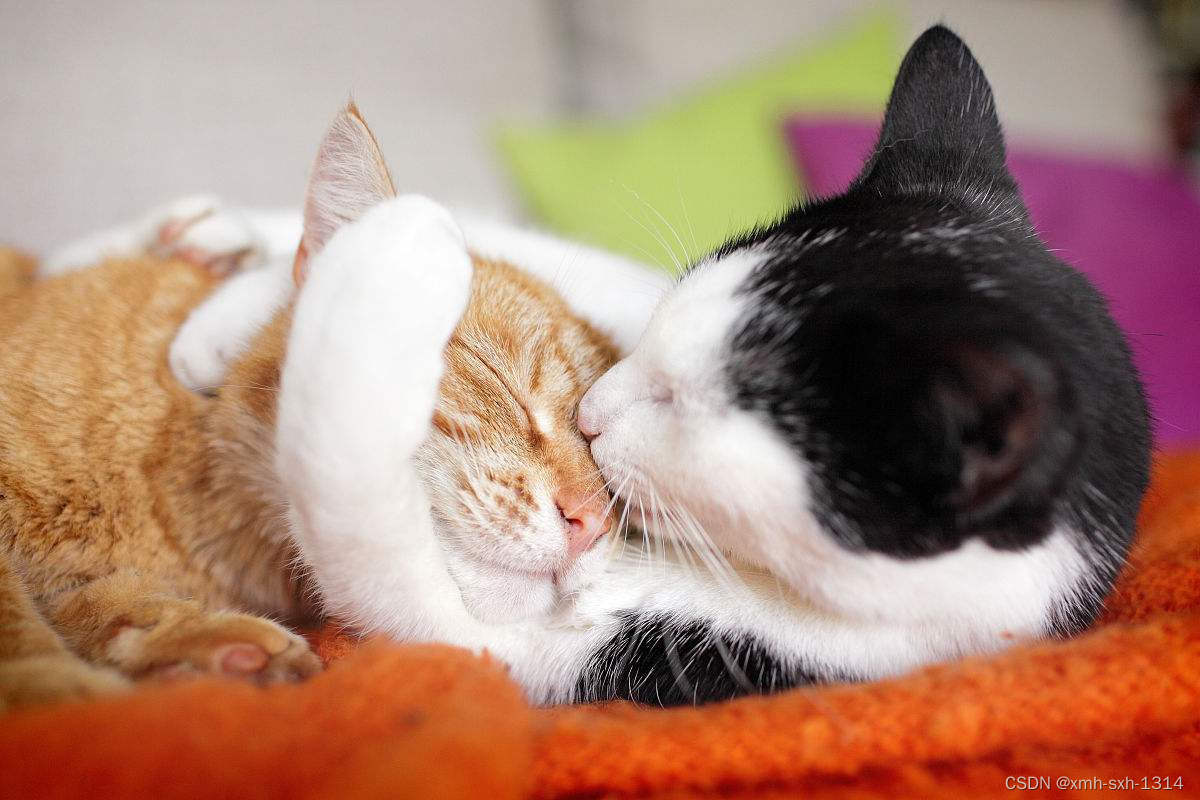
消息中间件 介绍
MQ简介 MQ,Message queue,消息队列,就是指保存消息的一个容器。具体的定义这里就不类似于数据库、缓存等,用来保存数据的。当然,与数据库、缓存等产品比较,也有自己一些特点,具体的特点后文会做详细的介绍。 现在常用…...

JAVA-字符串长度
给定一行长度不超过 100 的非空字符串,请你求出它的具体长度。 输入格式 输入一行,表示一个字符串。注意字符串中可能包含空格。 输出格式 输出一个整数,表示它的长度。 数据范围 1≤字符串长度≤100 字符串末尾无回车 输入样例: …...
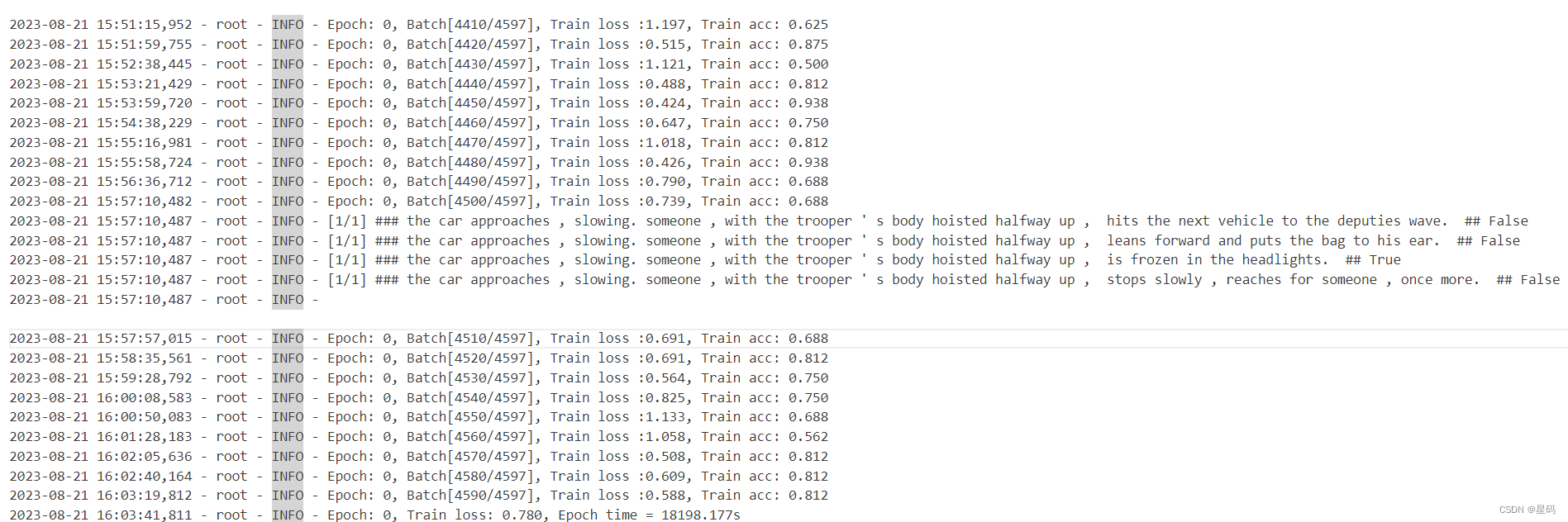
[oneAPI] 基于BERT预训练模型的SWAG问答任务
[oneAPI] 基于BERT预训练模型的SWAG问答任务 基于Intel DevCloud for oneAPI下的Intel Optimization for PyTorch基于BERT预训练模型的SWAG问答任务数据集下载和描述数据集构建问答选择模型训练 结果参考资料 比赛:https://marketing.csdn.net/p/f3e44fbfe46c465f4d…...
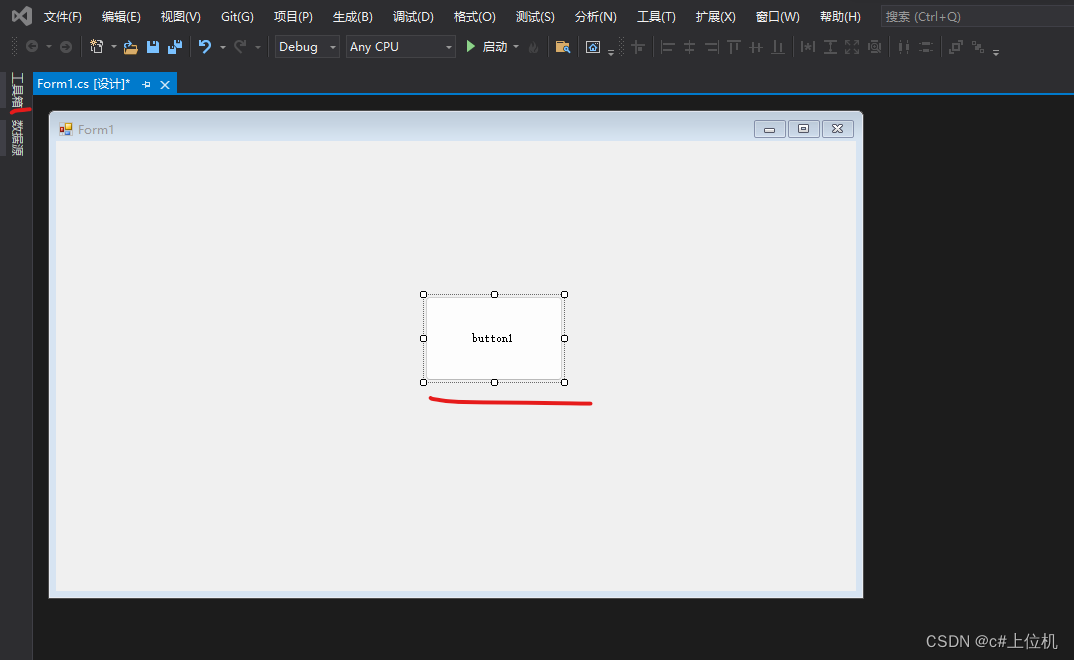
如何为winform控件注册事件
有很多winform的初学者不知道如何为winform注册的事件代码,本篇博文就是以button控件为例子,为winform注册单击事件,如下: 1、新建一个winform 以visual studio 2019 社区版为例子,新建一个winform程序,如下: 关于visual studio 2019 社区版下载方式点击这里:手把手教…...
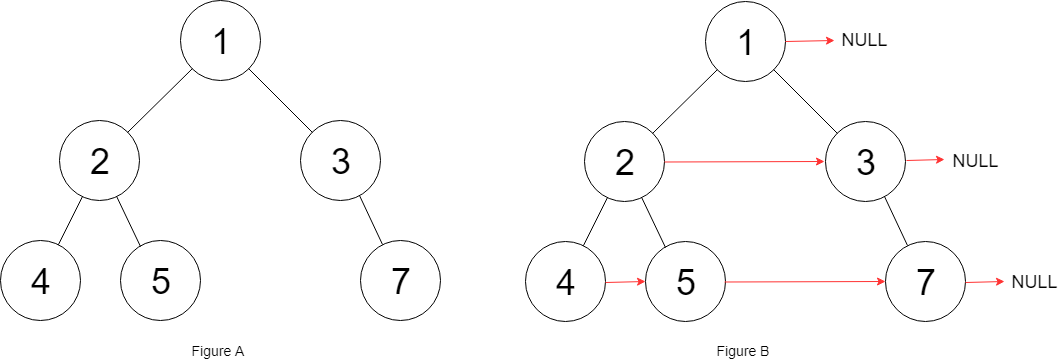
【LeetCode-面试经典150题-day15】
目录 104.二叉树的最大深度 100.相同的树 226.翻转二叉树 101.对称二叉树 105.从前序与中序遍历序列构造二叉树 106.从中序与后序遍历序列构造二叉树 117.填充每个节点的下一个右侧节点指针Ⅱ 104.二叉树的最大深度 题意: 给定一个二叉树 root ,返回其…...

git查看和修改项目远程仓库地址
git查看和修改项目远程仓库地址 一、背景 项目代码仓库迁移,需要本地更新远程仓库地址,进行代码同步与提交。 二、查看项目的远程仓库地址 # 查看远程地址 git remote -v # 查看远程仓库信息(分支、地址等) git remote show origin三、修…...
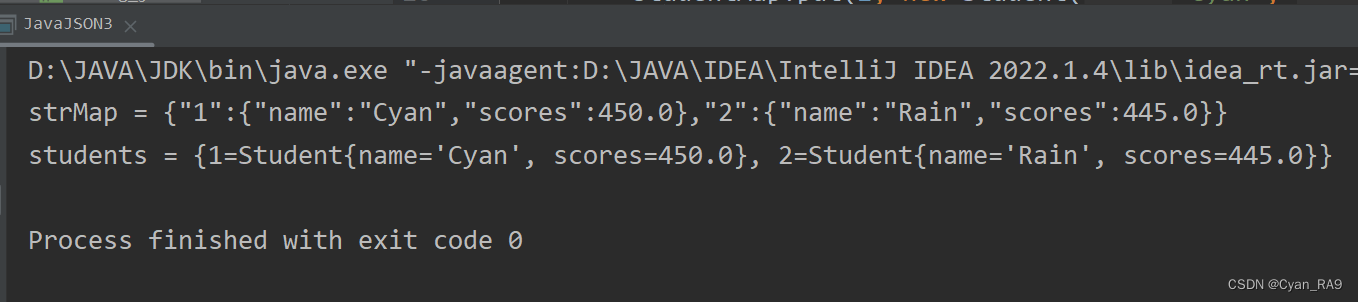
JavaWeb 速通JSON
目录 一、JSON快速入门 1.基本介绍 : 2.定义格式 : 3.入门案例 : 二、JSON对象和字符串的相互转换 1.常用方法 : 2.应用实例 : 3.使用细节 : 三、JSON在Java中的使用 1.基本说明 : 2.应用场景 : 2.1 JSON <---> JavaBean 2.2 JSON <---> List 2.3 JSON …...
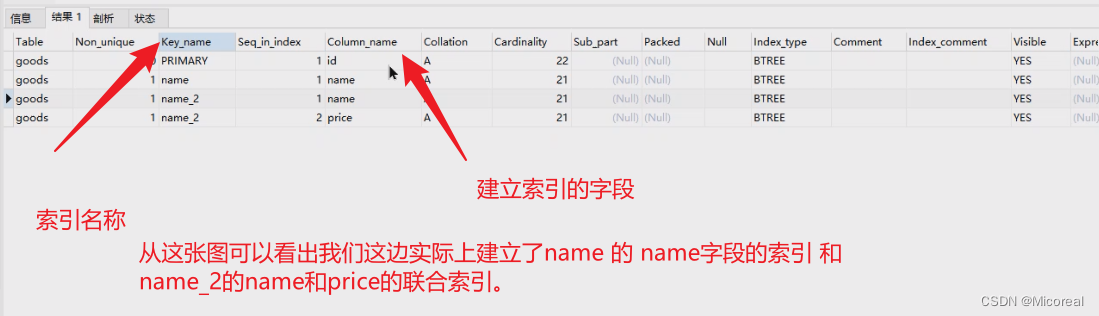
20 MySQL(下)
文章目录 视图视图是什么定义视图查看视图删除视图视图的作用 事务事务的使用 索引查询索引创建索引删除索引聚集索引和非聚集索引影响 账户管理(了解非DBA)授予权限 与 账户的相关操作 MySQL的主从配置 视图 视图是什么 通俗的讲,视图就是…...
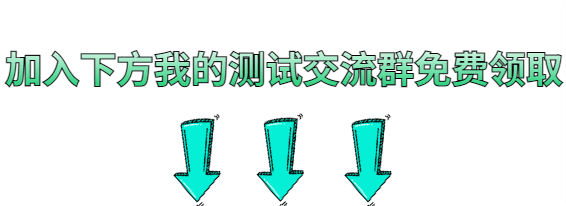
测试圈的网红工具:Jmeter到底难在哪里?!
雨果的公司最近推出了一款在线购物应用,吸引了大量用户。然而随着用户数量的增加,应用的性能开始出现问题。用户抱怨说购物过程中页面加载缓慢,甚至有时候无法完成订单,小欧作为负责人员迫切需要找到解决方案。 在学习JMeter之前…...

深度学习10:Attention 机制
目录 Attention 的本质是什么 Attention 的3大优点 Attention 的原理 Attention 的 N 种类型 Attention 的本质是什么 Attention(注意力)机制如果浅层的理解,跟他的名字非常匹配。他的核心逻辑就是「从关注全部到关注重点」。 Attention…...

简单着色器编写(中下)
这篇我们来介绍另一部分函数。 static unsigned int CreateShader(const std::string& vertexShader, const std::string& fragmentShader) {unsigned int program glCreateProgram();unsigned int vs CompileShader(GL_VERTEX_SHADER,vertexShader);unsigned int f…...
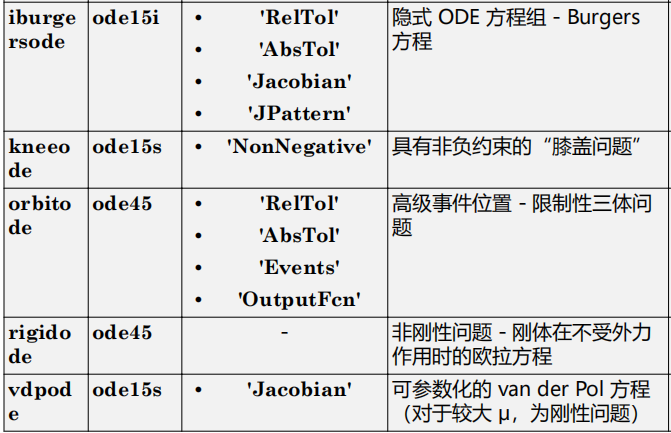
matlab使用教程(24)—常微分方程(ODE)求解器
1.常微分方程 常微分方程 (ODE) 包含与一个自变量 t(通常称为时间)相关的因变量 y 的一个或多个导数。此处用于表示 y 关于 t 的导数的表示法对于一阶导数为 y ′ ,对于二阶导数为 y ′′,依此类推。ODE 的阶数等于 y 在方程中…...
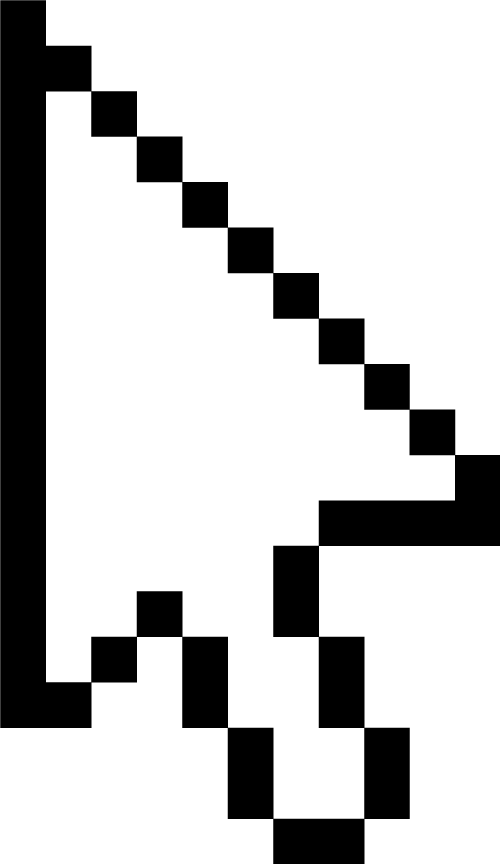
企业级数据共享规模化模式
数据共享正在成为企业数据战略的重要元素。对于公司而言,Amazon Data Exchange 这样的亚马逊云科技服务提供了与其他公司共享增值数据或从这些数据获利的途径。一些企业希望有一个数据共享平台,他们可以在该平台上建立协作和战略方法,在封闭、…...
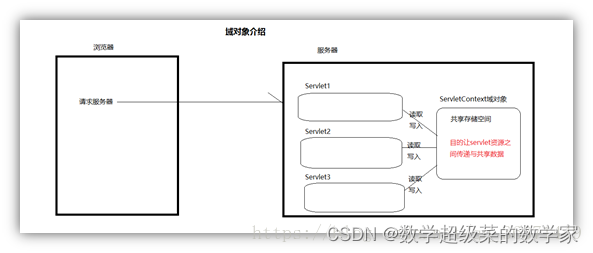
Web服务器-Tomcat详细原理与实现
Tomcat 安装与使用 :MAC 安装配置使用Tomcat - 掘金 安装后本计算机就相当于一台服务器了!!! 方式一:使用本地安装的Tomcat 1、将项目文件移动到Tomcat的webapps目录下。 2、启动Tomcat 3、在浏览器输入想要加载的…...
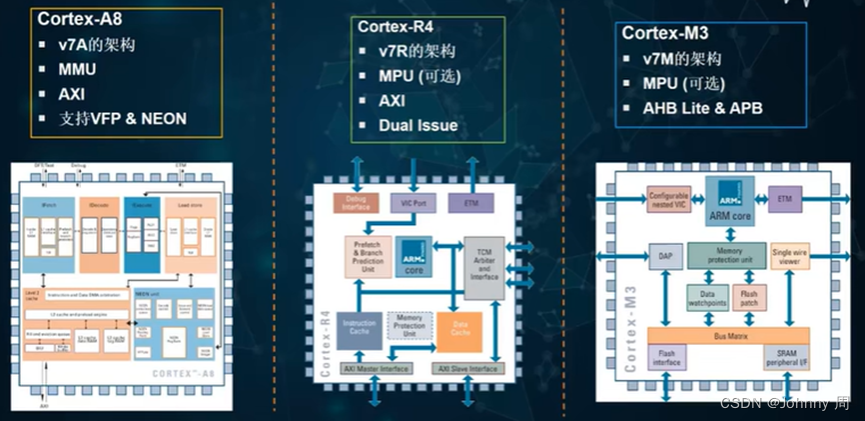
ARM处理器核心概述
一、基于ARM处理器的嵌入式系统 ARM核深度嵌入SOC中,通过JTAG口进行外部调试。计通常既有外部内存又有内部内存,从而支持不通的内存宽度、速度和大小。一般会包含一个中断控制器。可能包含一些Primece外设,需要从ARM公司取得授权。总线使用A…...
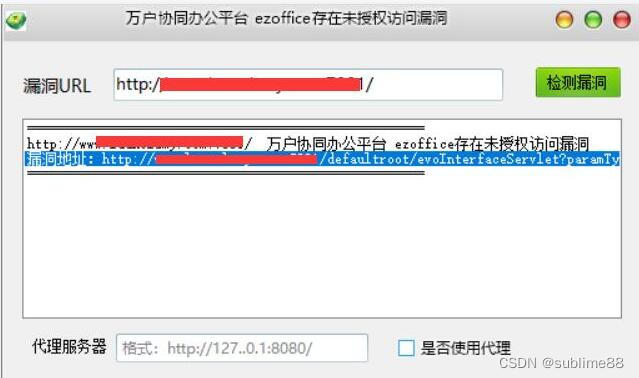
万户协同办公平台 ezoffice存在未授权访问漏洞 附POC
文章目录 万户协同办公平台 ezoffice存在未授权访问漏洞 附POC1. 万户协同办公平台 ezoffice简介2.漏洞描述3.影响版本4.fofa查询语句5.漏洞复现6.POC&EXP7.整改意见8.往期回顾 万户协同办公平台 ezoffice存在未授权访问漏洞 附POC 免责声明:请勿利用文章内的相…...
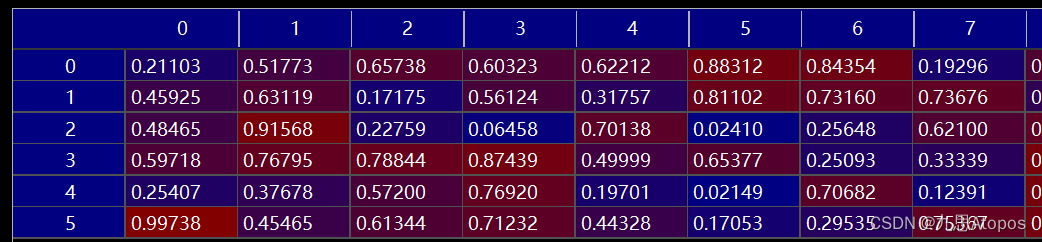
使用ctcloss训练矩阵生成目标字符串
首先我们需要明确 c t c l o s s ctcloss ctcloss是用来做什么的。比如说要生成的目标字符串长度为 l l l,而这个字符串包含 s s s个字符,字符串允许的最大长度为 L L L,这里认为一个位置是一个时间步,就是一拍,记为 T…...
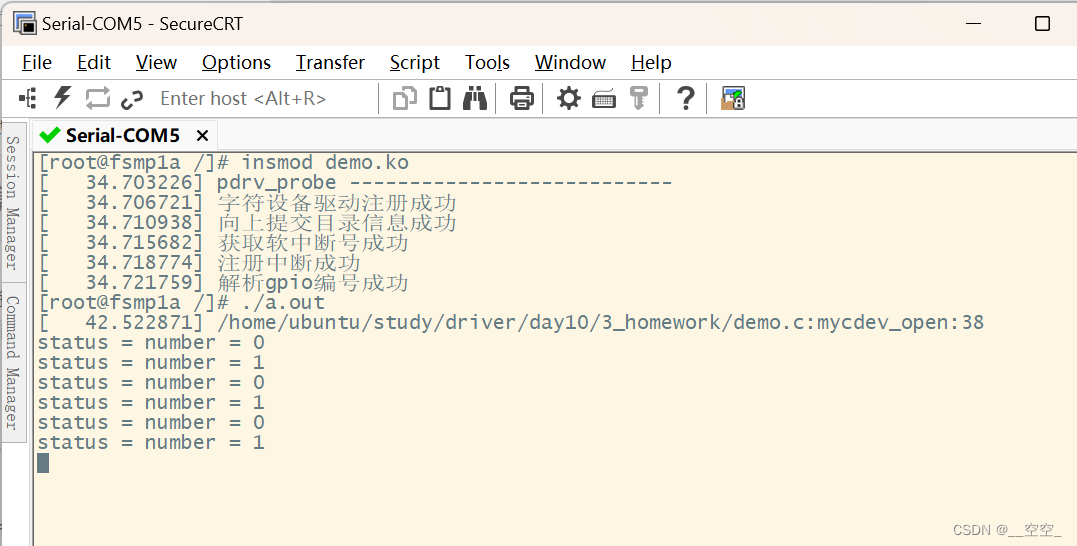
驱动 - 20230829
练习 基于platform实现 在根节点下,增加设备树 myplatform {compatible"hqyj,myplatform";interrupts-extended<&gpiof 9 0>, <&gpiof 7 0>, <&gpiof 8 0>;led1-gpio<&gpioe 10 0>;reg<0x12345678 59>;}…...
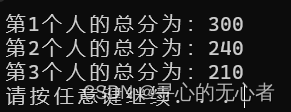
数组(个人学习笔记黑马学习)
一维数组 1、定义方式 #include <iostream> using namespace std;int main() {//三种定义方式//1.int arr[5];arr[0] 10;arr[1] 20;arr[2] 30;arr[3] 40;arr[4] 50;//访问数据元素/*cout << arr[0] << endl;cout << arr[1] << endl;cout &l…...

layui表格事件分析实例
在 layui 的表格组件中,区分表头事件和行内事件是通过事件类型(toolbar 和 tool)以及 lay-filter 值来实现的。 我们有一个表格,其中有一个工具栏按钮和操作按钮。我们将使用 layui 的 table 组件来处理这些事件。 HTML 结构&…...

Android NDK JNI与Java的相互调用
一、Jni调用Java代码 jni可以调用java中的方法和java中的成员变量,因此JNIEnv定义了一系列的方法来帮助我们调用java的方法和成员变量。 以上就是jni调用java类的大部分方法,如果是静态的成员变量和静态方法,可以使用***GetStaticMethodID、CallStaticObjectMethod等***。就…...
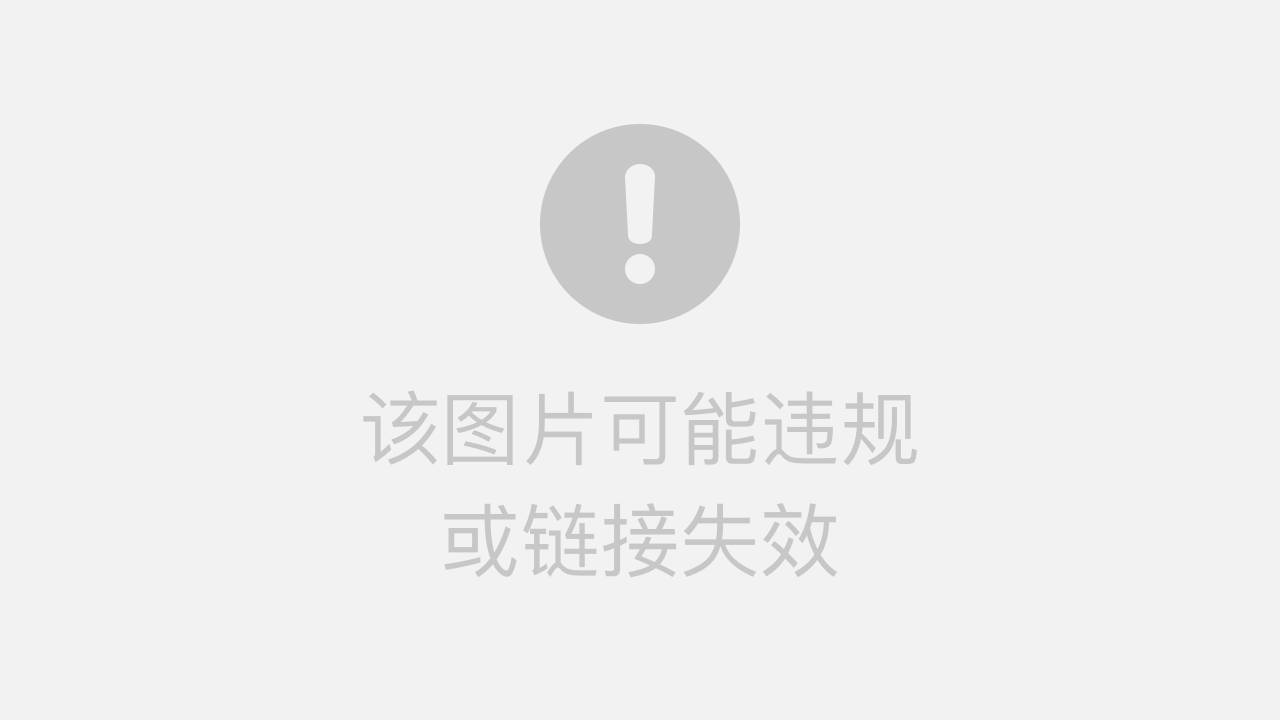
装备制造企业如何执行精益管理?
导 读 ( 文/ 2358 ) 精益管理是一种以提高效率、降低成本和优化流程为目标的管理方法。装备制造行业具备人工参与度高,产成品价值高,质量要求高的特点。 在装备制造企业中实施精益管理可以帮助企业提高竞争力、提升生产效率并提供高质量的产品。本文将…...
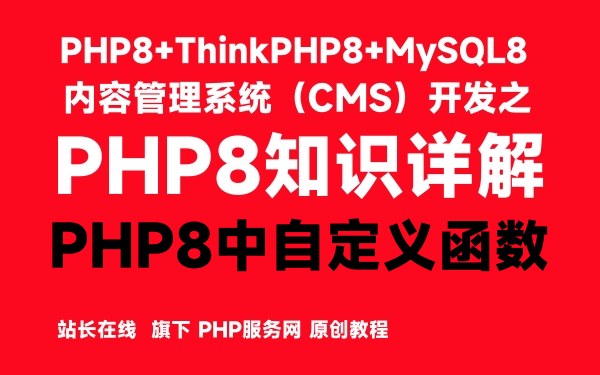
PHP8中自定义函数-PHP8知识详解
1、什么是函数? 函数,在英文中的单词是function,这个词语有功能的意思,也就是说,使用函数就是在编程的过程中,实现一定的功能。即函数就是实现一定功能的一段特定代码。 在前面的教学中,我们已…...
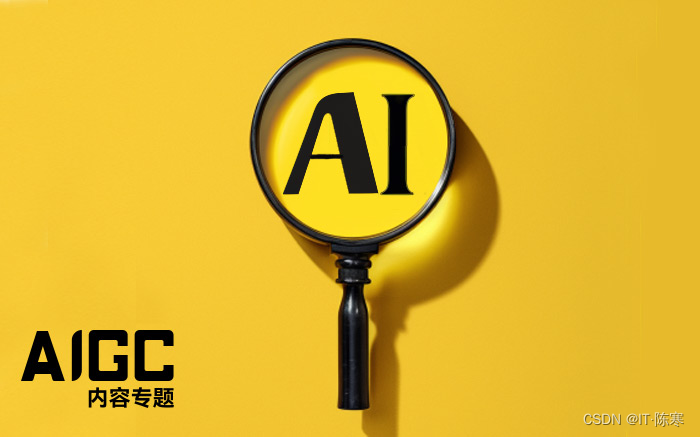
虚拟化技术:云计算发展的核心驱动力
文章目录 虚拟化技术的概念和作用虚拟化技术的优势虚拟化技术对未来发展的影响结论 🎉欢迎来到AIGC人工智能专栏~虚拟化技术:云计算发展的核心驱动力 ☆* o(≧▽≦)o *☆嗨~我是IT陈寒🍹✨博客主页:IT陈寒的博客🎈该系…...
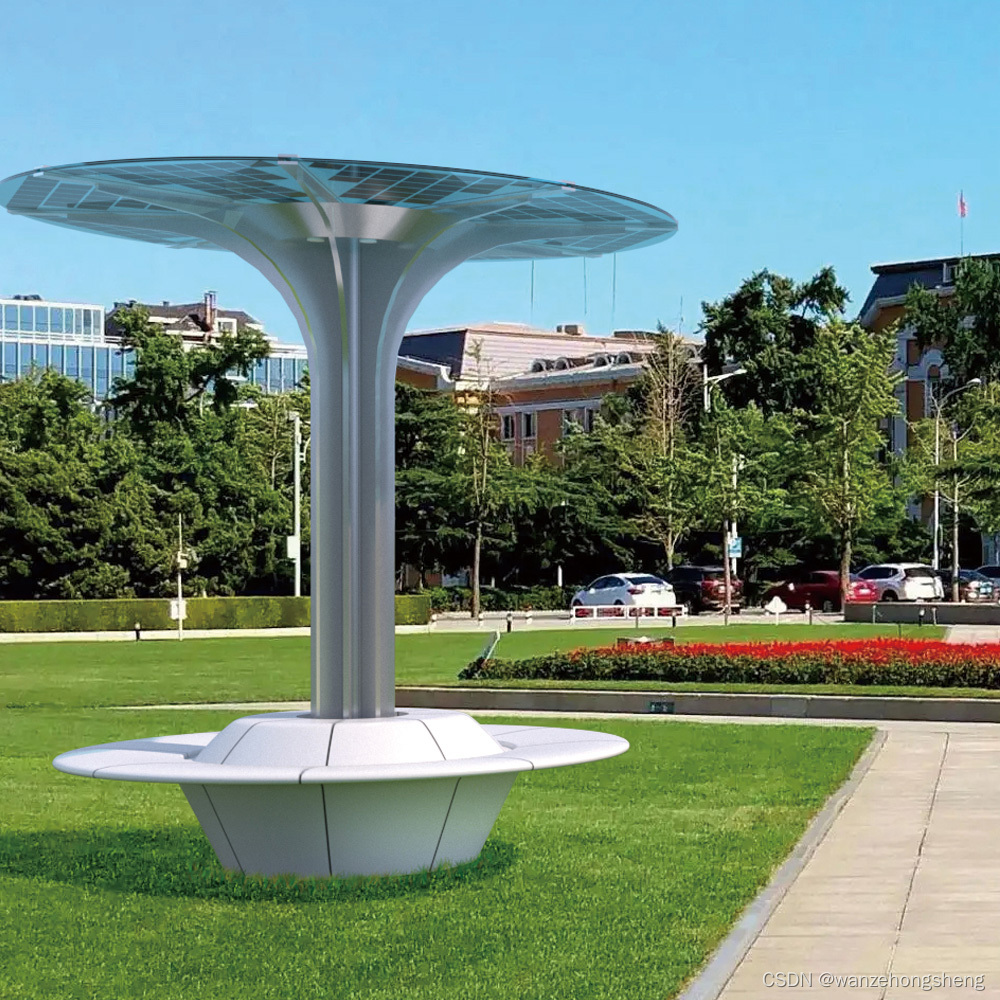
光伏+旅游景区
传统化石燃料可开发量逐渐减少,并且对环境造成的危害日益突出。全世界都把目光投向了可再生能源,希望可再生能源能够改变人类的能源结构。丰富的太阳能取之不尽、用之不竭,同时对环境没有影响,光伏发电是近些年来发展最快…...

手搓文本向量数据库(自然语言搜索生成模型)
import paddle import jieba import pandas as pd import numpy as np import os from glob import glob from multiprocessing import Process, Manager, freeze_supportfrom tqdm import tqdm# 首先 确定的是输出的时候一定要使用pd.to_pickle() pd.read_pickle() # 计算的时…...
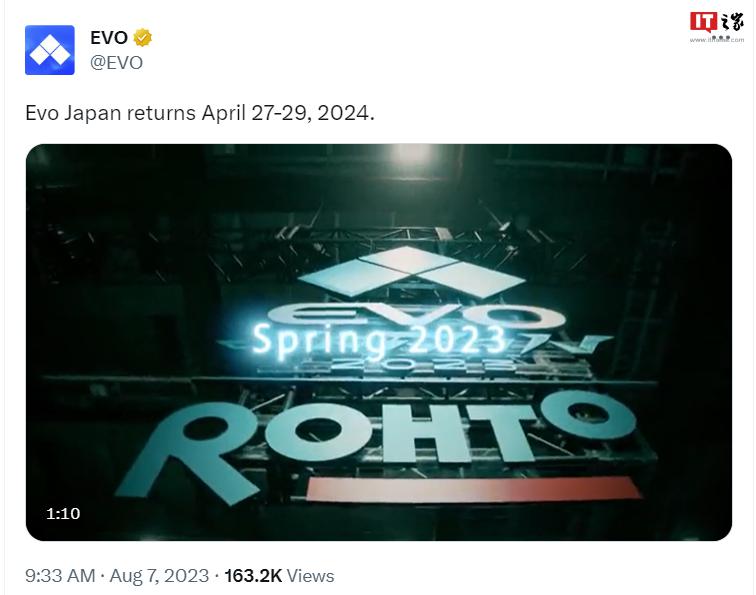
EVO大赛是什么
价格是你所付出的东西,而价值是你得到的东西 EVO大赛是什么? “EVO”大赛全称“Evolution Championship Series”,是北美最高规格格斗游戏比赛,大赛正式更名后已经连续举办12年,是全世界最大规模的格斗游戏赛事。常见…...

linux中使用clash代理
本机环境:ubuntu16 安装代理工具(这里使用clash) 可以手动下载解压,下载地址:https://github.com/Dreamacro/clash 也可以直接使用命令行,演示如下: userlocalhost:~$ curl https://glados.r…...
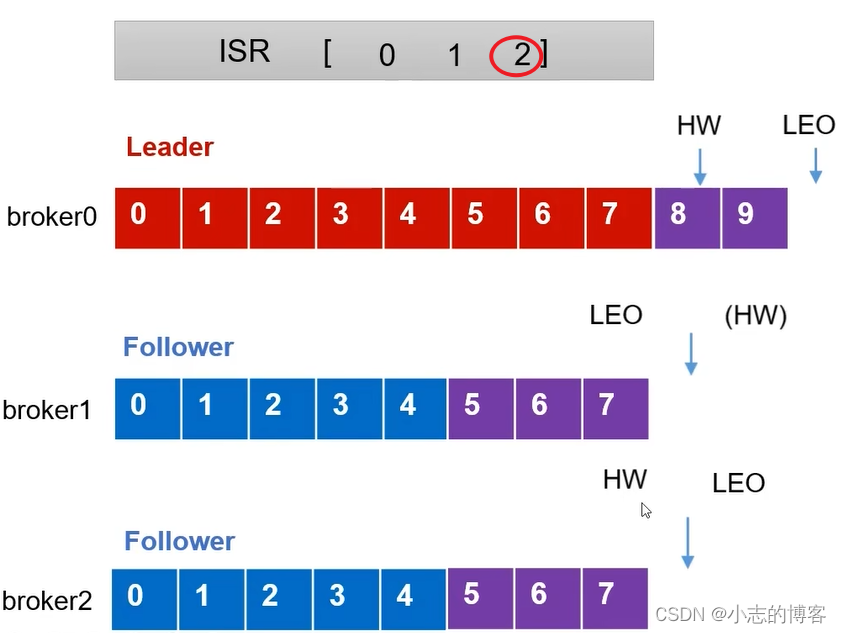
Kafka3.0.0版本——Follower故障处理细节原理
目录 一、服务器信息二、服务器基本信息及相关概念2.1、服务器基本信息2.2、LEO的概念2.3、HW的概念 三、Follower故障处理细节 一、服务器信息 三台服务器 原始服务器名称原始服务器ip节点centos7虚拟机1192.168.136.27broker0centos7虚拟机2192.168.136.28broker1centos7虚拟…...