江门外贸网站建设/日本域名注册
Gin学习笔记
Gin文档:https://pkg.go.dev/github.com/gin-gonic/gin
1、快速入门
1.1、安装Gin
go get -u github.com/gin-gonic/gin
1.2、main.go
package mainimport ("github.com/gin-gonic/gin""net/http"
)func main() {// 创建路由引擎r := gin.Default()// 添加路由监听r.GET("/", func(c *gin.Context) {c.String(http.StatusOK, "Hello Gin!")})//启用 web 服务err := r.Run()if err != nil {return} // listen and serve on 0.0.0.0:8080 (for windows "localhost:8080")
}
2、配置热更新
Air文档:https://github.com/cosmtrek/air
2.1、下载
# 添加air包
go get -u github.com/cosmtrek/air
# 编译并安装air到 $GOPATH/bin 目录(重启一下Goland)
go install github.com/cosmtrek/air@latest
2.2、使用
# 直接使用air即可热加载
air
3、响应数据
3.1、String
package mainimport ("github.com/gin-gonic/gin""net/http"
)func main() {r := gin.Default()r.LoadHTMLGlob("./template/*.html")r.GET("/", func(c *gin.Context) {c.String(http.StatusOK, "Hello Gin!")})r.GET("/hello", func(c *gin.Context) {//c.JSON(http.StatusOK, map[string]interface{}{// "name": "xumeng03",// "age": "24",//})c.JSON(http.StatusOK, gin.H{"name": "xumeng03","age": "24",})})r.GET("/gin", func(c *gin.Context) {c.HTML(http.StatusOK, "gin.html", gin.H{"path": c.FullPath(),})})err := r.Run()if err != nil {return} // listen and serve on 0.0.0.0:8080 (for windows "localhost:8080")
}
3.2、JSON
package mainimport ("github.com/gin-gonic/gin""net/http"
)func main() {r := gin.Default()r.GET("/hello", func(c *gin.Context) {//c.JSON(http.StatusOK, map[string]interface{}{// "name": "xumeng03",// "age": "24",//})c.JSON(http.StatusOK, gin.H{"name": "xumeng03","age": "24",})})err := r.Run()if err != nil {return} // listen and serve on 0.0.0.0:8080 (for windows "localhost:8080")
}
3.3、HTML
package mainimport ("github.com/gin-gonic/gin""net/http"
)func main() {r := gin.Default()r.LoadHTMLGlob("./template/*.html")r.GET("/gin", func(c *gin.Context) {c.HTML(http.StatusOK, "gin.html", gin.H{"path": c.FullPath(),})})err := r.Run()if err != nil {return} // listen and serve on 0.0.0.0:8080 (for windows "localhost:8080")
}
<!DOCTYPE html>
<html lang="en">
<head><meta charset="UTF-8"><title>Gin Study</title>
</head>
<body>
<h1>Gin Study!</h1>
<p>请求路径:{{.path}}</p>
</body>
</html>
4、请求数据
4.1、Get
1、直接查询
package mainimport ("github.com/gin-gonic/gin""net/http"
)func main() {r := gin.Default()r.GET("/", func(c *gin.Context) {// Query 查询 request 的参数,DefaultQuery 同样查询 request 的参数但若未查询到则赋一个默认值//var name = c.Query("name")var name = c.DefaultQuery("name", "Gin")c.String(http.StatusOK, "Hello %s!", name)})err := r.Run()if err != nil {return} // listen and serve on 0.0.0.0:8080 (for windows "localhost:8080")
}
2、绑定到结构体
package mainimport ("github.com/gin-gonic/gin""net/http"
)func main() {r := gin.Default()r.GET("/", func(c *gin.Context) {var person = Person{}err := c.ShouldBind(&person)if err != nil {c.JSON(http.StatusOK, gin.H{"status": 500,"message": "Params Error!",})return}c.JSON(http.StatusOK, gin.H{"status": 200,"data": person,})})err := r.Run()if err != nil {return} // listen and serve on 0.0.0.0:8080 (for windows "localhost:8080")
}type Person struct {Name string `json:"name" form:"name"`Age string `json:"age" form:"age"`
}
4.2、Post
1、直接查询
package mainimport ("github.com/gin-gonic/gin""net/http"
)func main() {r := gin.Default()r.POST("/", func(c *gin.Context) {// PostForm 查询 request 的参数,DefaultPostForm 同样查询 request 的参数但若未查询到则赋一个默认值var name = c.PostForm("name")var age = c.DefaultPostForm("age", "20")c.String(http.StatusOK, "Hello %s %s!", name, age)})err := r.Run()if err != nil {return} // listen and serve on 0.0.0.0:8080 (for windows "localhost:8080")
}
2、绑定到结构体(form-data)
package mainimport ("github.com/gin-gonic/gin""net/http"
)func main() {r := gin.Default()r.POST("/", func(c *gin.Context) {var person = Person{}err := c.ShouldBind(&person)if err != nil {c.JSON(http.StatusOK, gin.H{"status": 500,})return}c.JSON(http.StatusOK, gin.H{"status": 500,"data": person,})})err := r.Run()if err != nil {return} // listen and serve on 0.0.0.0:8080 (for windows "localhost:8080")
}type Person struct {Name string `json:"name" form:"name"`Age string `json:"age" form:"age"`
}
3、绑定到结构体(json)
package mainimport ("github.com/gin-gonic/gin""net/http"
)func main() {r := gin.Default()r.POST("/json", func(c *gin.Context) {var person = Person{}err := c.ShouldBindJSON(&person)if err != nil {c.JSON(http.StatusOK, gin.H{"status": 500,})return}c.JSON(http.StatusOK, gin.H{"status": 200,"data": person,})})err := r.Run()if err != nil {return} // listen and serve on 0.0.0.0:8080 (for windows "localhost:8080")
}type Person struct {Name string `json:"name" form:"name"`Age string `json:"age" form:"age"`
}
5、Restful
http://127.0.0.1/item/1 查询,GET
http://127.0.0.1/item 新增,POST
http://127.0.0.1/item 更新,PUT
http://127.0.0.1/item/1 删除,DELETE
package mainimport ("github.com/gin-gonic/gin""net/http"
)func main() {r := gin.Default()r.GET("/get/:name", func(c *gin.Context) {param := c.Param("name")c.String(http.StatusOK, "Hello get %s!", param)})r.POST("/post/:name", func(c *gin.Context) {param := c.Param("name")c.String(http.StatusOK, "Hello post %s!", param)})r.PUT("/put/:name", func(c *gin.Context) {param := c.Param("name")c.String(http.StatusOK, "Hello put %s!", param)})r.DELETE("/delete/:name", func(c *gin.Context) {param := c.Param("name")c.String(http.StatusOK, "Hello delete %s!", param)})err := r.Run()if err != nil {return} // listen and serve on 0.0.0.0:8080 (for windows "localhost:8080")
}
6、路由分组
6.1、基本使用
package mainimport ("github.com/gin-gonic/gin""net/http"
)func main() {r := gin.Default()group := r.Group("/restful0"){group.GET("/get/:name", func(c *gin.Context) {param := c.Param("name")c.String(http.StatusOK, "Hello get %s!", param)})group.POST("/post/:name", func(c *gin.Context) {param := c.Param("name")c.String(http.StatusOK, "Hello post %s!", param)})}group1 := r.Group("/restful1"){group1.PUT("/put/:name", func(c *gin.Context) {param := c.Param("name")c.String(http.StatusOK, "Hello put %s!", param)})group1.DELETE("/delete/:name", func(c *gin.Context) {param := c.Param("name")c.String(http.StatusOK, "Hello delete %s!", param)})}err := r.Run()if err != nil {return} // listen and serve on 0.0.0.0:8080 (for windows "localhost:8080")
}
6.2、拆分文件
package mainimport ("ginstudy/router""github.com/gin-gonic/gin"
)func main() {r := gin.Default()router.Restful0(r)router.Restful1(r)err := r.Run()if err != nil {return} // listen and serve on 0.0.0.0:8080 (for windows "localhost:8080")
}
package routerimport ("github.com/gin-gonic/gin""net/http"
)func Restful0(r *gin.Engine) {group := r.Group("/restful0"){group.GET("/get/:name", func(c *gin.Context) {param := c.Param("name")c.String(http.StatusOK, "Hello get %s!", param)})group.POST("/post/:name", func(c *gin.Context) {param := c.Param("name")c.String(http.StatusOK, "Hello post %s!", param)})}
}
package routerimport ("github.com/gin-gonic/gin""net/http"
)func Restful1(r *gin.Engine) {group1 := r.Group("/restful1"){group1.PUT("/put/:name", func(c *gin.Context) {param := c.Param("name")c.String(http.StatusOK, "Hello put %s!", param)})group1.DELETE("/delete/:name", func(c *gin.Context) {param := c.Param("name")c.String(http.StatusOK, "Hello delete %s!", param)})}
}
7、自定义控制器
7.1、目录结构
7.2、router
app.go
package appimport ("ginstudy/controller/app""github.com/gin-gonic/gin"
)func AppApi(r *gin.Engine) {group := r.Group("/app/api")appController := app.AppController{}group.GET("/", appController.Index)
}
web.go
package webimport ("ginstudy/controller/web""github.com/gin-gonic/gin"
)func WebApi(r *gin.Engine) {group := r.Group("/web/api")webController := web.WebController{}group.GET("/", webController.Index)
}
7.3、controller
app.go
package appimport ("ginstudy/controller""github.com/gin-gonic/gin"
)type AppController struct {controller.StandardController
}func (app AppController) Index(c *gin.Context) {//c.String(http.StatusOK, "Hello App Api!")app.Success(c)
}
web.go
package webimport ("ginstudy/controller""github.com/gin-gonic/gin"
)type WebController struct {controller.StandardController
}func (web WebController) Index(c *gin.Context) {//c.String(http.StatusOK, "Hello Web Api!")web.Success(c)
}
standard.go
package controllerimport ("github.com/gin-gonic/gin""net/http"
)type StandardController struct{}func (standard StandardController) Success(c *gin.Context) {c.JSON(http.StatusOK, gin.H{"status": 200,})
}func (standard StandardController) Error(c *gin.Context) {c.JSON(http.StatusOK, gin.H{"status": 500,})
}
7.4、main
package mainimport ("ginstudy/router/app""ginstudy/router/web""github.com/gin-gonic/gin"
)func main() {r := gin.Default()app.AppApi(r)web.WebApi(r)err := r.Run()if err != nil {return}
}
7.5、go.mod
module ginstudygo 1.20require (dario.cat/mergo v1.0.0 // indirectgithub.com/bep/godartsass v1.2.0 // indirectgithub.com/bep/godartsass/v2 v2.0.0 // indirectgithub.com/bep/golibsass v1.1.1 // indirectgithub.com/bytedance/sonic v1.10.2 // indirectgithub.com/chenzhuoyu/base64x v0.0.0-20230717121745-296ad89f973d // indirectgithub.com/chenzhuoyu/iasm v0.9.1 // indirectgithub.com/cli/safeexec v1.0.1 // indirectgithub.com/cosmtrek/air v1.49.0 // indirectgithub.com/creack/pty v1.1.20 // indirectgithub.com/fatih/color v1.15.0 // indirectgithub.com/fsnotify/fsnotify v1.7.0 // indirectgithub.com/gabriel-vasile/mimetype v1.4.3 // indirectgithub.com/gin-contrib/sse v0.1.0 // indirectgithub.com/gin-gonic/gin v1.9.1 // indirectgithub.com/go-playground/locales v0.14.1 // indirectgithub.com/go-playground/universal-translator v0.18.1 // indirectgithub.com/go-playground/validator/v10 v10.16.0 // indirectgithub.com/goccy/go-json v0.10.2 // indirectgithub.com/gohugoio/hugo v0.120.3 // indirectgithub.com/json-iterator/go v1.1.12 // indirectgithub.com/klauspost/cpuid/v2 v2.2.5 // indirectgithub.com/leodido/go-urn v1.2.4 // indirectgithub.com/mattn/go-colorable v0.1.13 // indirectgithub.com/mattn/go-isatty v0.0.20 // indirectgithub.com/modern-go/concurrent v0.0.0-20180306012644-bacd9c7ef1dd // indirectgithub.com/modern-go/reflect2 v1.0.2 // indirectgithub.com/pelletier/go-toml v1.9.5 // indirectgithub.com/pelletier/go-toml/v2 v2.1.0 // indirectgithub.com/spf13/afero v1.10.0 // indirectgithub.com/tdewolff/parse/v2 v2.7.4 // indirectgithub.com/twitchyliquid64/golang-asm v0.15.1 // indirectgithub.com/ugorji/go/codec v1.2.11 // indirectgolang.org/x/arch v0.6.0 // indirectgolang.org/x/crypto v0.14.0 // indirectgolang.org/x/net v0.17.0 // indirectgolang.org/x/sys v0.14.0 // indirectgolang.org/x/text v0.14.0 // indirectgoogle.golang.org/protobuf v1.31.0 // indirectgopkg.in/yaml.v3 v3.0.1 // indirect
)
8、中间处理程序
8.1、基本使用
standard.go
package controllerimport ("fmt""github.com/gin-gonic/gin""net/http"
)type StandardController struct{}func (standard StandardController) Success(c *gin.Context) {c.JSON(http.StatusOK, gin.H{"status": 200,})
}func (standard StandardController) Error(c *gin.Context) {c.JSON(http.StatusOK, gin.H{"status": 500,})
}func (standard StandardController) Aop(c *gin.Context) {fmt.Println("aop start")// 放行请求c.Next()// 拒绝请求//c.Abort()fmt.Println("aop end")
}
web.go
package webimport ("ginstudy/controller/web""github.com/gin-gonic/gin"
)func WebApi(r *gin.Engine) {group := r.Group("/web/api")webController := web.WebController{}group.GET("/", webController.Aop, webController.Index)
}
app.go
package appimport ("ginstudy/controller/app""github.com/gin-gonic/gin"
)func AppApi(r *gin.Engine) {group := r.Group("/app/api")appController := app.AppController{}group.GET("/", appController.Aop, appController.Index)
}
8.2、多中间处理程序
standard.go
package controllerimport ("fmt""github.com/gin-gonic/gin""net/http"
)type StandardController struct{}func (standard StandardController) Success(c *gin.Context) {c.JSON(http.StatusOK, gin.H{"status": 200,})
}func (standard StandardController) Error(c *gin.Context) {c.JSON(http.StatusOK, gin.H{"status": 500,})
}func (standard StandardController) Aop1(c *gin.Context) {fmt.Println("aop1 start")// 放行请求c.Next()// 拒绝请求//c.Abort()fmt.Println("aop1 end")
}func (standard StandardController) Aop2(c *gin.Context) {fmt.Println("aop2 start")// 放行请求c.Next()// 拒绝请求//c.Abort()fmt.Println("aop2 end")
}
web.go
package webimport ("ginstudy/controller/web""github.com/gin-gonic/gin"
)func WebApi(r *gin.Engine) {group := r.Group("/web/api")webController := web.WebController{}group.GET("/", webController.Aop1, webController.Aop2, webController.Index)
}
app.go
package appimport ("ginstudy/controller/app""github.com/gin-gonic/gin"
)func AppApi(r *gin.Engine) {group := r.Group("/app/api")appController := app.AppController{}group.GET("/", appController.Aop1, appController.Aop2, appController.Index)
}
8.3、全局中间处理程序
路由组也可单独配置中间处理程序!
main.go
package mainimport ("ginstudy/controller""ginstudy/router/app""ginstudy/router/web""github.com/gin-gonic/gin"
)func main() {standardController := controller.StandardController{}r := gin.Default()r.Use(standardController.Aop1, standardController.Aop2)app.AppApi(r)web.WebApi(r)err := r.Run()if err != nil {return}
}
web.go
package webimport ("ginstudy/controller/web""github.com/gin-gonic/gin"
)func WebApi(r *gin.Engine) {group := r.Group("/web/api")webController := web.WebController{}group.GET("/", webController.Index)
}
app.go
package appimport ("ginstudy/controller/app""github.com/gin-gonic/gin"
)func AppApi(r *gin.Engine) {group := r.Group("/app/api")appController := app.AppController{}group.GET("/", appController.Index)
}
8.4、中间处理程序通信
standard.go
package controllerimport ("fmt""github.com/gin-gonic/gin""net/http"
)type StandardController struct{}func (standard StandardController) Success(c *gin.Context) {c.JSON(http.StatusOK, gin.H{"status": 200,})
}func (standard StandardController) Error(c *gin.Context) {c.JSON(http.StatusOK, gin.H{"status": 500,})
}func (standard StandardController) Aop1(c *gin.Context) {fmt.Println("aop1 start")c.Set("name", "xumeng03")// 放行请求c.Next()// 拒绝请求//c.Abort()fmt.Println("aop1 end")
}func (standard StandardController) Aop2(c *gin.Context) {fmt.Println("aop2 start")fmt.Println(c.Get("name"))// 放行请求c.Next()// 拒绝请求//c.Abort()fmt.Println("aop2 end")
}
8.5、协程中使用中间处理程序
standard.go
package controllerimport ("fmt""github.com/gin-gonic/gin""net/http""time"
)type StandardController struct{}func (standard StandardController) Success(c *gin.Context) {c.JSON(http.StatusOK, gin.H{"status": 200,})
}func (standard StandardController) Error(c *gin.Context) {c.JSON(http.StatusOK, gin.H{"status": 500,})
}func (standard StandardController) Aop1(c *gin.Context) {fmt.Println("aop1 start")c.Set("name", "xumeng03")// 放行请求c.Next()// 拒绝请求//c.Abort()fmt.Println("aop1 end")
}func (standard StandardController) Aop2(c *gin.Context) {fmt.Println("aop2 start")fmt.Println(c.Get("name"))// 放行请求c.Next()// 拒绝请求//c.Abort()// 不能直接使用gin.Context,需要使用c.Copy()获取gin.Context的副本// c.Copy()只会复制gin.Context结构体中的字段值,并不会复制底层的请求和响应对象cc := c.Copy()go func() {time.Sleep(5 * time.Second)fmt.Println(cc.Request.URL.Path, "被访问了")}()fmt.Println("aop2 end")
}
9、自定义模块
package utilsimport "time"func UnixToDatetime(unix int64) string {return time.Unix(unix, 0).Format("2006-01-02 15:04:05")
}
10、文件上传
10.1、单文件上传
router/webgo
package webimport ("ginstudy/controller/web""github.com/gin-gonic/gin"
)func WebApi(r *gin.Engine) {group := r.Group("/web/api")webController := web.WebController{}group.GET("/", webController.Index)group.POST("/upload", webController.Upload)
}
controller/web.go
package webimport ("fmt""ginstudy/controller""github.com/gin-gonic/gin""path"
)type WebController struct {controller.StandardController
}func (web WebController) Index(c *gin.Context) {//c.String(http.StatusOK, "Hello Web Api!")fmt.Println("Hello Web Api!")web.Success(c)
}func (web WebController) Upload(c *gin.Context) {file, err := c.FormFile("file")if err != nil {fmt.Println("upload error!")}fmt.Println(file.Filename)// 路径为main.go所在目录p := path.Join("static", file.Filename)err = c.SaveUploadedFile(file, p)if err != nil {fmt.Println(err)return}web.Success(c)
}
10.2、多文件上传
router/webgo
package webimport ("ginstudy/controller/web""github.com/gin-gonic/gin"
)func WebApi(r *gin.Engine) {group := r.Group("/web/api")webController := web.WebController{}group.GET("/", webController.Index)group.POST("/uploads", webController.Uploads)
}
controller/web.go
package webimport ("fmt""ginstudy/controller""github.com/gin-gonic/gin""path"
)type WebController struct {controller.StandardController
}func (web WebController) Index(c *gin.Context) {//c.String(http.StatusOK, "Hello Web Api!")fmt.Println("Hello Web Api!")web.Success(c)
}func (web WebController) Uploads(c *gin.Context) {form, _ := c.MultipartForm()fmt.Println(form)files := form.File["files"]for k, file := range files {fmt.Println(k, file.Filename)p := path.Join("static", file.Filename)err := c.SaveUploadedFile(file, p)if err != nil {return}}web.Success(c)
}
11、Cookie&Session
11.1、cookie
package webimport ("fmt""ginstudy/controller""github.com/gin-gonic/gin""path"
)type WebController struct {controller.StandardController
}func (web WebController) Index(c *gin.Context) {//c.String(http.StatusOK, "Hello Web Api!")fmt.Println("Hello Web Api!")web.Success(c)
}func (web WebController) Cookie(c *gin.Context) {// name: cookie 名称// value: cookie 值// maxAge: 存活时间,单位秒(等于0则一直存活,小于0则删除cookie)// path: cookie 可用路径// domain: cookie 可用域名,如需在多个二级域名下共享,设置时需设置为“.bilibili.com”格式// secure: 是否只在 https 启用// httponly: cookie仅后端可操作cookie, err := c.Cookie("GinStudy")if err != nil {fmt.Println(err)}if cookie == "" {c.SetCookie("GinStudy", "xumeng03", 3600, "/", "localhost", false, false)}web.Success(c)
}
11.2、session
https://github.com/gin-contrib/sessions#redis
1、安装
go get github.com/gin-contrib/sessions
2、设置存储引擎
main.go
package mainimport ("ginstudy/router/app""ginstudy/router/web""github.com/gin-contrib/sessions""github.com/gin-contrib/sessions/cookie""github.com/gin-gonic/gin"
)func main() {r := gin.Default()// 创建基于cookie的存储引擎store := cookie.NewStore([]byte("Ginstudy"))r.Use(sessions.Sessions("session", store))app.AppApi(r)web.WebApi(r)err := r.Run()if err != nil {return}
}
3、使用session
router/web.go
package webimport ("ginstudy/controller/web""github.com/gin-gonic/gin"
)func WebApi(r *gin.Engine) {group := r.Group("/web/api")webController := web.WebController{}group.GET("/", webController.Index)group.GET("/session", webController.Session)
}
controller/web.go
package webimport ("fmt""ginstudy/controller""github.com/gin-contrib/sessions""github.com/gin-gonic/gin""path"
)type WebController struct {controller.StandardController
}func (web WebController) Index(c *gin.Context) {//c.String(http.StatusOK, "Hello Web Api!")fmt.Println("Hello Web Api!")web.Success(c)
}func (web WebController) Session(c *gin.Context) {// 获取sessionsession := sessions.Default(c)name := session.Get("name")if name == nil {session.Set("name", "xumeng03")// 设置值(需要手动保存)session.Save()} else {fmt.Println(name)}web.Success(c)
}
11.3、分布式session
待补。。。
相关文章:
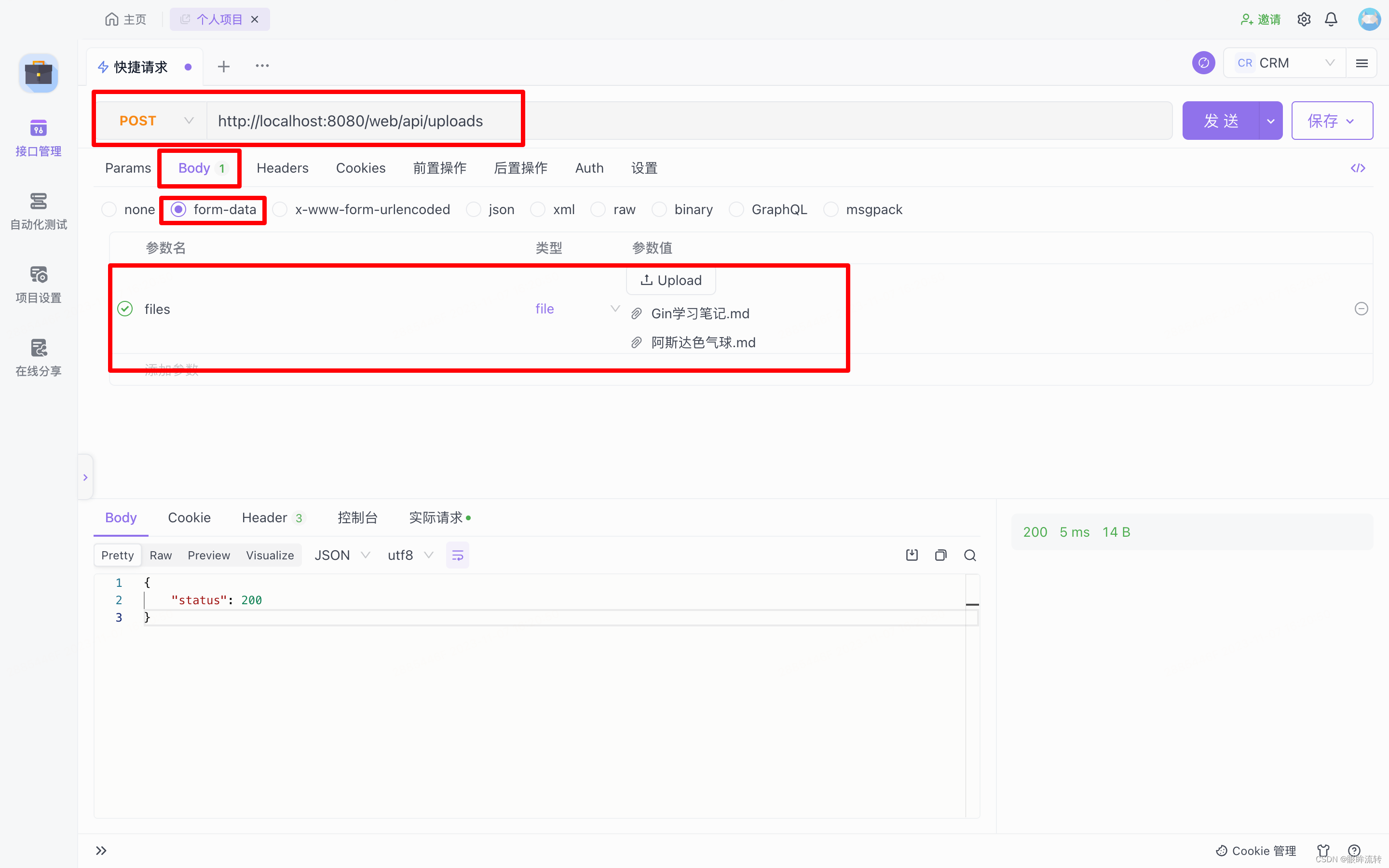
Gin学习笔记
Gin学习笔记 Gin文档:https://pkg.go.dev/github.com/gin-gonic/gin 1、快速入门 1.1、安装Gin go get -u github.com/gin-gonic/gin1.2、main.go package mainimport ("github.com/gin-gonic/gin""net/http" )func main() {// 创建路由引…...

使用 OpenTracing 和 LightStep 监控无服务器功能
无服务器功能的采用在企业组织内达到了创纪录的水平。有趣的是,鉴于越来越多的采用和兴趣,许多监控解决方案孤立了在这些环境中执行的代码的性能,或者仅提供有关执行的基本指标。为了了解应用程序的性能,我想知道存在哪些瓶颈、时…...

Sleep(0)、Sleep(1)、SwitchToThread()
当 timeout 参数为 0 时(如 Sleep(0)),操作系统会检查可运行队列中是否有高于或等于当前线程优先级的其他就绪线程。如果有,当前线程将被移除并放弃处理器时间,让其他线程执行。如果没有高优先级的线程,当前…...
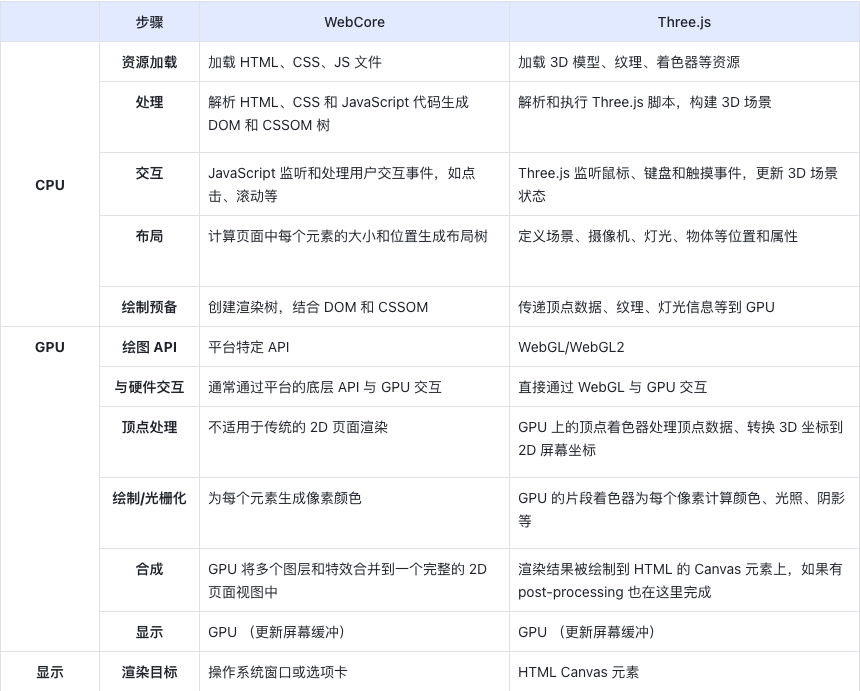
前端食堂技术周刊第 103 期:10 月登陆 Web 平台的新功能、TS 5.3 RC、React 2023 状态、高并发的哲学原理、Web 资源加载优先级
美味值:🌟🌟🌟🌟🌟 口味:夏梦玫珑 食堂技术周刊仓库地址:https://github.com/Geekhyt/weekly 大家好,我是童欧巴。欢迎来到前端食堂技术周刊,我们先来看下…...

Python(三)数据类型转换
程序员的公众号:源1024,获取更多资料,无加密无套路! 最近整理了一份大厂面试资料《史上最全大厂面试题》,Springboot、微服务、算法、数据结构、Zookeeper、Mybatis、Dubbo、linux、Kafka、Elasticsearch、数据库等等 …...

linq to sql性能优化技巧
linq to sql 是一个代码生成器和ORM工具,他自动为我们做了很多事情,这很容易让我们对他的性能产生怀疑 linq to sql 是一个代码生成器和ORM工具,他自动为我们做了很多事情,这很容易让我们对他的性能产生怀疑。但是也有几个测试证明显示在做好优化的情况下,linq to sql的…...
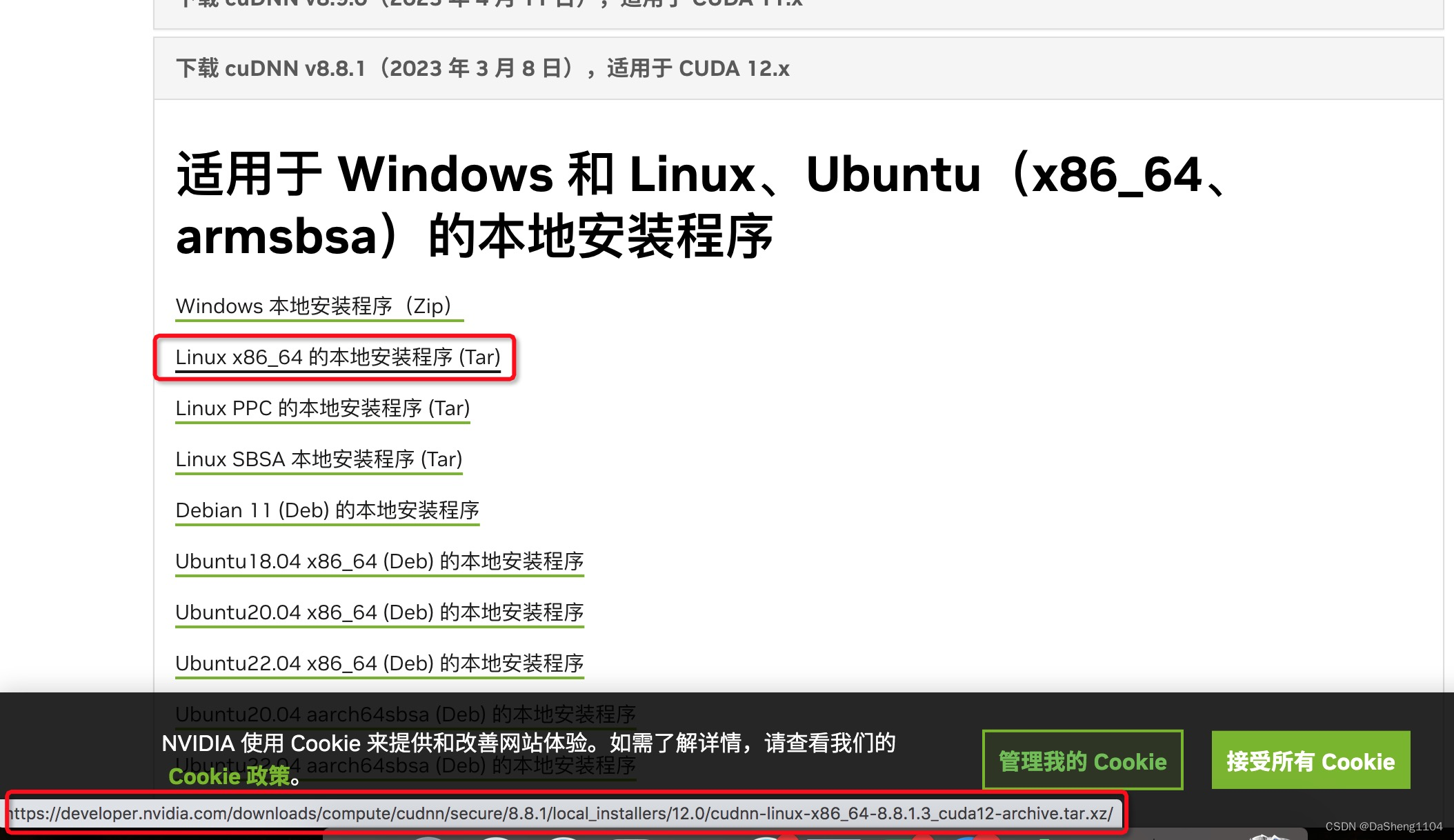
ubuntu20.04 安装cudnn
中文地址是.cn:cuDNN 历史版本 | NVIDIA 开发者 英文地址是.com:cuDNN 历史版本 | NVIDIA 开发者 1、下载cudnn:cudnn-local-repo-ubuntu2004-8.8.1.3_1.0-1_amd64.deb 解压并安装:sudo dpkg -i cudnn-local-repo-ubuntu2004-8.8…...
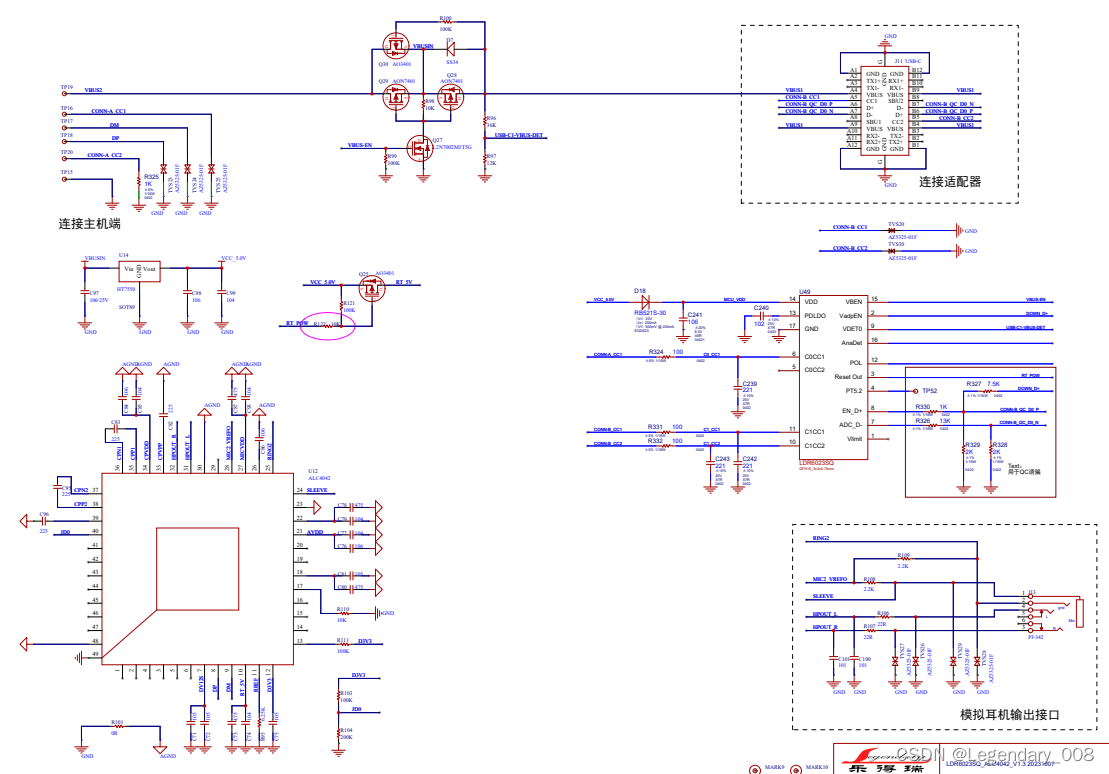
手机转接器实现原理,低成本方案讲解
USB-C PD协议里,SRC和SNK双方之间通过CC通信来协商请求确定充电功率及数据传输速率。当个设备需要充电时,它会发送消息去给适配器请求充电,此时充电器会回应设备的请求,并告知其可提供的档位功率,设备端会根据适配器端…...

RDS for MySQL 是什么
RDS for MySQL 是一种托管型数据库服务,RDS代表“关系数据库服务”(Relational Database Service)。这是云服务提供商提供的一种服务,用于简化关系数据库的设置、操作和扩展。对于MySQL版本的RDS,意味着它是专门为运行MySQL数据库管理系统的实…...
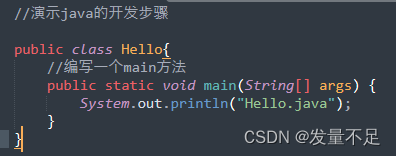
Java开发注意事项和细节说明
👨🎓👨🎓博主:发量不足 个人简介:耐心,自信来源于你强大的思想和知识基础!! 📑📑本期更新内容:Java开发注意事项和细节说明&…...
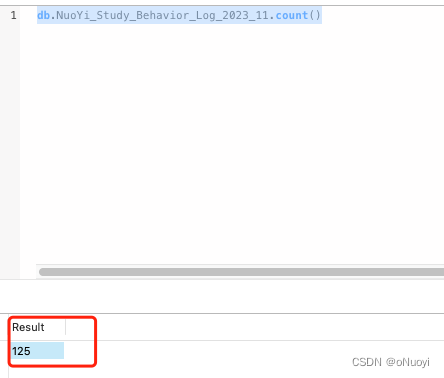
springboot中使用Java代码进行MongoDB集合数据备份
有时候mongo的集合中数据量太大,查询或翻页时可能会超过最大数量报错,可以给mongo的集合进行备份并保留最近一段时间的数据即可 下面是通过Java代码进行mongo的集合备份单元测试 import cn.hutool.core.date.DateUtil; import com.nuoyi.study.dao.mongo…...
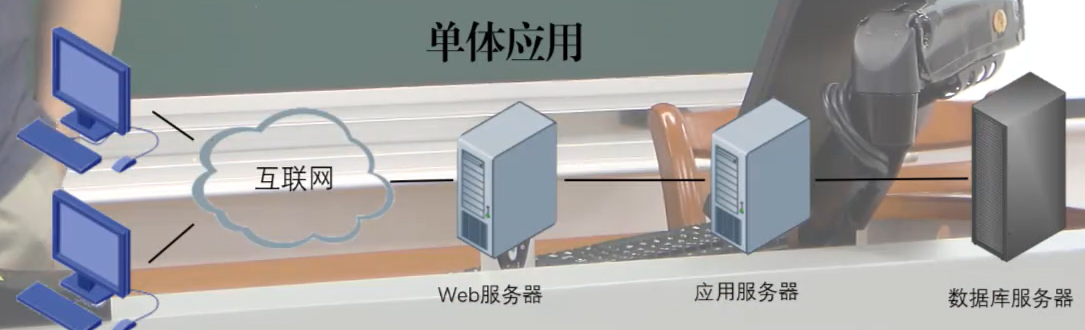
JavaEE的渊源
JavaEE的渊源 1. JavaEE的起源2. JavaEE与Spring的诞生3. JavaEE发展历程(2003-2007)4. JavaEE发展历程(2009-至今)5. Java的Spec数目与网络结构 1. JavaEE的起源 我们首先来讲一下JavaEE的起源 ,为什么要来讲起源 ? …...

html中使用JQ自定义锚点偏移量
问题:一般情况下使用href跳转达到效果。如果页面中头部固定住了,点击瞄点的时候自动是最上面,头部会给它覆盖掉一部分,所以要在点击之后额外再加头部高度 <a href"#aa">Technical Documents</a><div id&…...
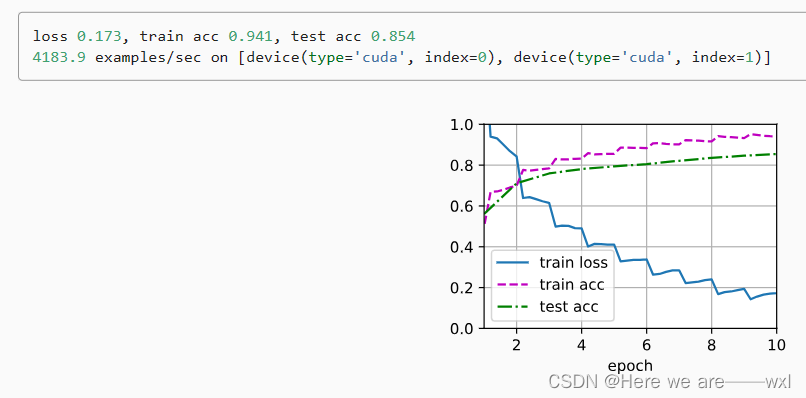
032、数据增广*
之——泛化性提升 杂谈 深度学习的数据增强(Data Augmentation)是一种技术,用于通过对原始数据进行多样性的变换和扩充,以增加训练数据的多样性,提高模型的泛化能力。这有助于减轻过拟合问题,提高深度学习模…...
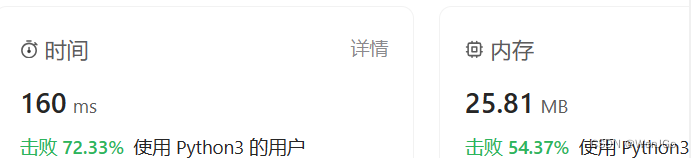
力扣最热一百题——盛水最多的容器
终于又来了。我的算法记录的文章已经很久没有更新了。为什么呢? 这段时间都在更新有关python的文章,有对python感兴趣的朋友可以在主页找到。 但是这也并不是主要的原因 在10月5号我发布了我的第一篇博客,大家也可以看见我的每一篇算法博客…...
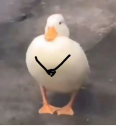
备份扫描工具 god_bak
Part1 前言 不想写东西,或者说换种说法 有些东西还没写完,有些系列也还没整完。就放一个昨天摸鱼写的东西。 如图,每个系列都还是会按照自己的风格来写,代码审计实战等都会结合自己挖掘或审计过的案例进行结合知识点的风格去写&…...

软考 系统架构设计师系列知识点之数字孪生体(2)
接前一篇文章:软考 系统架构设计师系列知识点之数字孪生体(1) 所属章节: 第11章. 未来信息综合技术 第5节. 数字孪生体技术概述 2. 数字孪生体的定义 AFRL(Air Force Research Laboratory,美国空军研究实…...
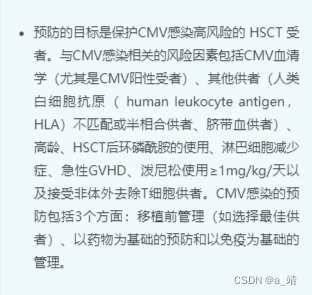
CSS实现文本左右对齐
因为文本里面有中午符号,英文,英文符号等,导致设置宽度以后右侧凌乱,可以通过以下代码设置样式,让文本工整对齐。 让我们看一下设置前和设置后的对比图片: 效果图如下:(左边是设置…...

利用exec命令进入docker容器时的报错问题
进入Docker 容器 docker exec [CONTAINER ID] bin/bash报错问题 一、详细报错信息 执行docker exec -it [containerId] /bin/bash报错: OCI runtime exec failed: exec failed: unable to start container process: exec: "/bin/bash": stat /bin/ba…...

Java 与C++ 语言的一些区别
Java 与C 语言的一些区别 前言不同之外 前言 之前用C、C 的多,目前开始学习和接触 Java ,拿Java和C 做一个对比,帮助快速掌握Java的开发。 不同之外 数据类型的差别: java中 byte 类型类似于c/c 中的char类型 boolean 与C 的bo…...

npm ERR! network ‘proxy‘ config is set properly. See: ‘npm help config解决方法
这个错误提示通常表示在使用 npm 安装包时出现了网络连接问题。具体来说,可能是由于以下原因之一: 你的网络连接不稳定或者被防火墙拦截了。你的计算机设置了代理,但是 npm 没有正确配置代理。npm 的配置文件中的 registry 配置不正确&#…...

An Empirical Study of Instruction-tuning Large Language Models in Chinese
本文是LLM系列文章,针对《An Empirical Study of Instruction-tuning Large Language Models in Chinese》的翻译。 汉语大语言模型指令调整的实证研究 摘要1 引言2 指令调整三元组3 其他重要因素4 迈向更好的中文LLM5 结论局限性 摘要 ChatGPT的成功验证了大型语…...

[MICROSAR Adaptive] --- 开发环境准备
Ubuntu 20.04/22.04版本默认的cmake版本不超过3.19,gcc/g++为9.x版本 而ap开发要求cmake版本大于3.19,gcc/g++版本为gcc-7 1 安装高版本cmake cmake源码下载路径 https://cmake.org/files/tar zxvf cmake-3.19.2.tar.gz cd cmake-3.19.2 ./bootstrap --prefix=/usr/local …...

Yolov5 batch 推理
前言 想要就有了 代码 import shutil import time import traceback import torchimport os import cv2 class PeopleDetect(object):def __init__(self, repo_or_dir, weight_path, confidence) -> None:self.model torch.hub.load(repo_or_dir, "custom", p…...
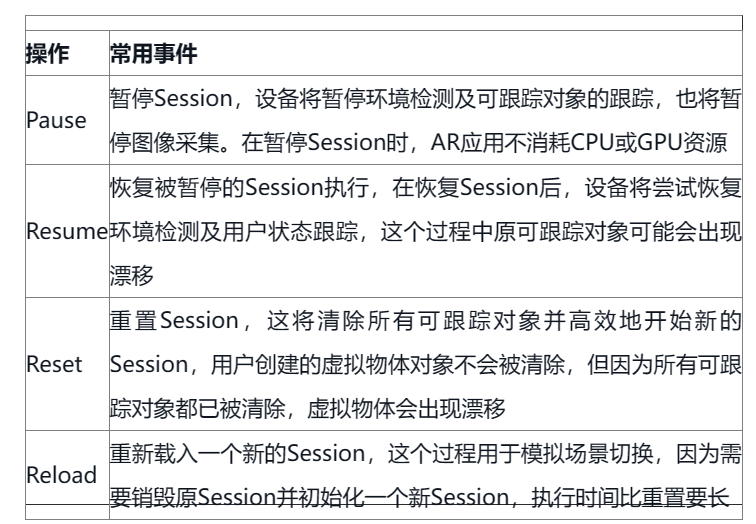
【ARFoundation学习笔记】ARFoundation基础(下)
写在前面的话 本系列笔记旨在记录作者在学习Unity中的AR开发过程中需要记录的问题和知识点。难免出现纰漏,更多详细内容请阅读原文。 文章目录 TrackablesTrackableManager可跟踪对象事件管理可跟踪对象 Session管理 Trackables 在AR Foundation中,平面…...
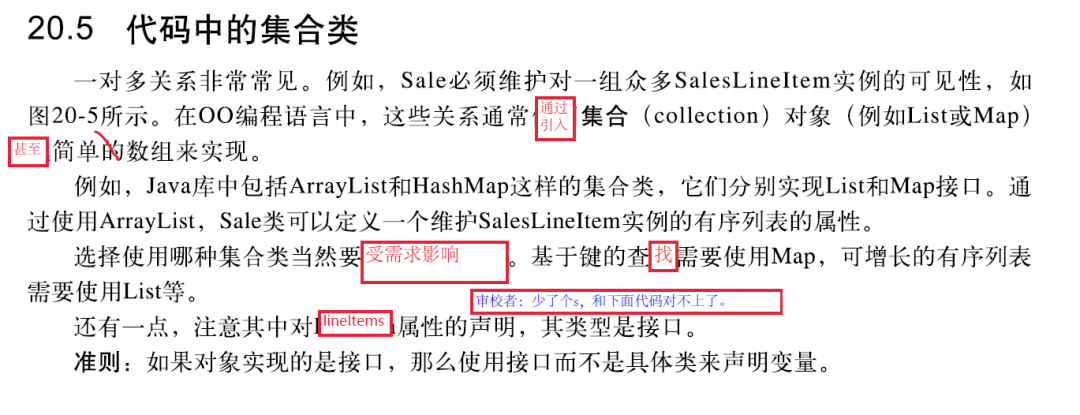
《UML和模式应用(原书第3版)》2024新修订译本部分截图
DDD领域驱动设计批评文集 做强化自测题获得“软件方法建模师”称号 《软件方法》各章合集 机械工业出版社即将在2024春节前后推出《UML和模式应用(原书第3版)》的典藏版。 受出版社委托,UMLChina审校了原中译本并做了一些修订。同比来说&a…...
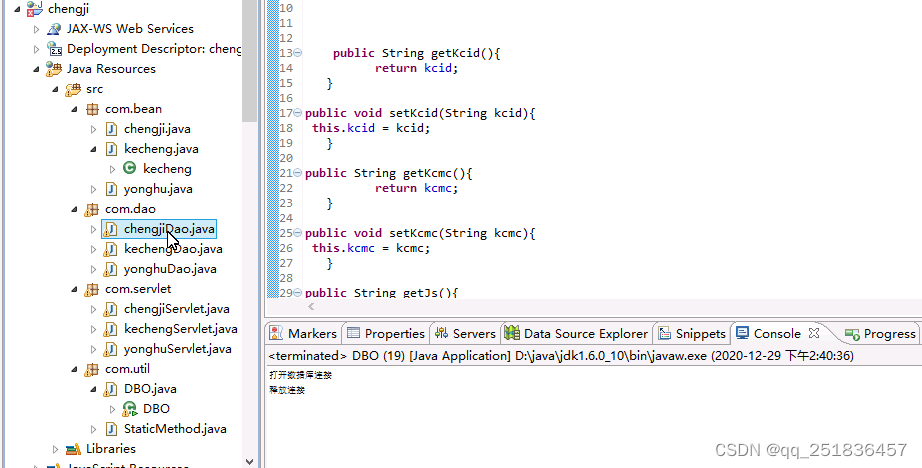
JSP 学生成绩查询管理系统eclipse开发sql数据库serlvet框架bs模式java编程MVC结构
一、源码特点 JSP 学生成绩查询管理系统 是一套完善的web设计系统,对理解JSP java编程开发语言有帮助,比较流行的servlet框架系统具有完整的源代码和数据库,eclipse开发系统主要采用B/S模式 开发。 java 学生成绩查询管理系统 代码下载链接…...

技术分享 | app自动化测试(Android)-- 属性获取与断言
断言是 UI 自动化测试的三要素之一,是 UI 自动化不可或缺的部分。在使用定位器定位到元素后,通过脚本进行业务操作的交互,想要验证交互过程中的正确性就需要用到断言。 常规的UI自动化断言 分析正确的输出结果,常规的断言一般包…...

flutter实现上拉到底部加载更多数据
实现上拉加载数据,效果如下: flutter滚动列表加载数据 使用的库主要是infinite_scroll_pagination , 安装请查看官网 接口用的是https://reqres.in/提供的接口 请求接口用到的库是dio 下面主要是介绍如何使用infinite_scroll_pagination实现上拉加载…...
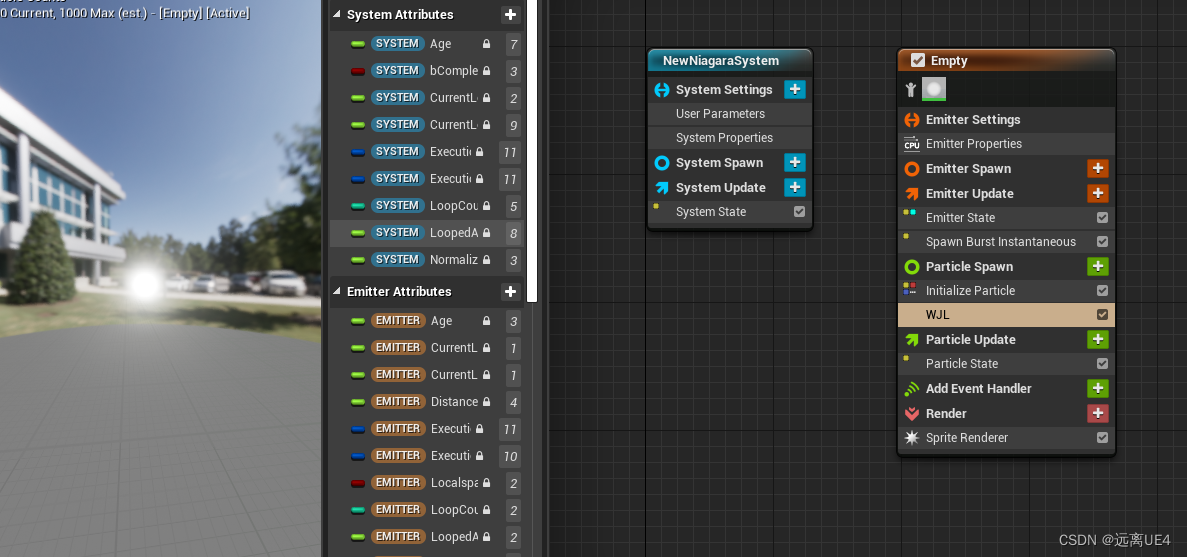
UE4 Niagara Module Script 初次使用笔记
这里可以创建一个Niagara模块脚本 创建出来长这样 点击号,输出staticmesh,点击它 这样就可以拿到对应的一些模型信息 这里的RandomnTriCoord是模型的坐标信息 根据坐标信息拿到位置信息 最后的Position也是通过Map Set的号,选择Particles的P…...