【Qt之QWizardPage】使用
介绍
QWizardPage
类是向导页面的基类。
QWizard
表示一个向导。每个页面都是一个QWizardPage
。当创建自己的向导时,可以直接使用QWizardPage
,也可以子类化它以获得更多控制。
页面具有以下属性,由QWizard
呈现:a title
,a subTitle
和a set of pixmaps
。有关详细信息,请参见向导页面元素。一旦将页面添加到向导中(使用QWizard :: addPage()
或QWizard :: setPage()
),wizard()
将返回指向相关的QWizard
对象的指针。
页面提供了五个虚函数,可以重新实现以提供自定义行为:
initializePage()
在用户单击向导的“下一步”按钮时调用以初始化页面的内容。如果您想从先前页面输入的内容中派生页面的默认值,则应该重新实现此函数。cleanupPage()
在用户单击向导的“返回”按钮时调用以重置页面的内容。validatePage()
在用户单击Next或Finish时验证页面。如果用户输入了不完整或无效的信息,通常会使用此函数显示错误消息。nextId()
返回下一页的ID。它在创建非线性向导时很有用,这些向导允许基于用户提供的信息进行不同的遍历路径。isComplete(
)用于确定是否应启用或禁用“下一步”和/或“完成”按钮。如果重新实现isComplete(),还必须确保每当完成状态更改时发出completeChanged()。
通常,向导的“下一步”
按钮和“完成”
按钮是互斥的。如果isFinalPage()
返回true
,则可用Finish
;否则,可用下一步
。默认情况下,isFinalPage()
仅在nextId()
返回-1
时为true
。如果要在页面上同时显示“下一步”
和“完成”
(允许用户执行“早期完成”
),请在该页面上调用setFinalPage(true)
。对于支持早期完成的向导,您可能还想在向导上设置HaveNextButtonOnLastPage
和HaveFinishButtonOnEarlyPages
选项。
在许多向导中,页面的内容可能会影响后续页面的字段的默认值。为了方便页面之间的通信,QWizard支持“字段”
机制,允许您在页面上注册字段(例如QLineEdit
)并从任何页面访问其值。字段对整个向导程序是全局的,并使任何单个页面都可以访问存储在另一个页面中的信息,而无需将所有逻辑放入QWizard
或页面明确知道彼此。使用registerField()
注册字段,可以使用field()
和setField()
随时访问它们。
常用方法
virtual void initializePage(int id)
这个虚函数由QWizard::initializePage()调用,以便在页面显示之前对其进行准备,或者由于QWizard::restart()被调用,或者由于用户单击Next而显示页面。(但是,如果设置了QWizard::IndependentPages选项,则仅在第一次显示页面时调用此函数。)
通过重新实现这个函数,您可以确保页面的字段是基于先前页面中的字段正确初始化的。例如:
void OutputFilesPage: initializePage ()
{QString className = field("className").toString();headerLineEdit->setText(className.toLower() + ".h");implementationLineEdit->setText(className.toLower() + ".cpp");outputDirLineEdit - > setText (QDir:: toNativeSeparators (QDir: tempPath ()));
}
默认实现什么都不做。
2. void setButtonText(QWizard::WizardButton which, const QString &text)
将按钮上的文本设置为本页上的文本。
默认情况下,按钮上的文本依赖于QWizard::wizardStyle,但可以使用QWizard::setButtonText()为整个向导重新定义。
setButtonText(QWizard::NextButton, "hei");
3. void setCommitPage(bool commitPage)
如果commitPage为true,则将该页设置为提交页;否则,将其设置为普通页面。
提交页是表示不能通过单击“返回”或“取消”来撤消的操作的页面。
提交按钮取代提交页面上的Next按钮。单击此按钮只调用QWizard::next(),就像单击next一样。
直接从提交页面进入的页面会禁用后退按钮。
setCommitPage(true);
void setFinalPage(bool finalPage)
如果finalPage为true,则显式地将此页面设置为final。
调用setFinalPage(true)后,isFinalPage()返回true, Finish按钮可见(如果isComplete()返回true则启用)。
调用setFinalPage(false)后,如果nextId()返回-1,isFinalPage()返回true;否则,返回false。
示例
.h
#ifndef CLASSWIZARD_H
#define CLASSWIZARD_H#include <QWizard>QT_BEGIN_NAMESPACE
class QCheckBox;
class QGroupBox;
class QLabel;
class QLineEdit;
class QRadioButton;
QT_END_NAMESPACEclass ClassWizard : public QWizard
{Q_OBJECTpublic:ClassWizard(QWidget *parent = 0);void accept() override;
};class IntroPage : public QWizardPage
{Q_OBJECTpublic:IntroPage(QWidget *parent = 0);private:QLabel *label;
};class ClassInfoPage : public QWizardPage
{Q_OBJECTpublic:ClassInfoPage(QWidget *parent = 0);private:QLabel *classNameLabel;QLabel *baseClassLabel;QLineEdit *classNameLineEdit;QLineEdit *baseClassLineEdit;QCheckBox *qobjectMacroCheckBox;QGroupBox *groupBox;QRadioButton *qobjectCtorRadioButton;QRadioButton *qwidgetCtorRadioButton;QRadioButton *defaultCtorRadioButton;QCheckBox *copyCtorCheckBox;
};class CodeStylePage : public QWizardPage
{Q_OBJECTpublic:CodeStylePage(QWidget *parent = 0);protected:void initializePage() override;private:QCheckBox *commentCheckBox;QCheckBox *protectCheckBox;QCheckBox *includeBaseCheckBox;QLabel *macroNameLabel;QLabel *baseIncludeLabel;QLineEdit *macroNameLineEdit;QLineEdit *baseIncludeLineEdit;
};class OutputFilesPage : public QWizardPage
{Q_OBJECTpublic:OutputFilesPage(QWidget *parent = 0);protected:void initializePage() override;private:QLabel *outputDirLabel;QLabel *headerLabel;QLabel *implementationLabel;QLineEdit *outputDirLineEdit;QLineEdit *headerLineEdit;QLineEdit *implementationLineEdit;
};class ConclusionPage : public QWizardPage
{Q_OBJECTpublic:ConclusionPage(QWidget *parent = 0);protected:void initializePage() override;private:QLabel *label;
};#endif
.cpp
#include <QtWidgets>#include "classwizard.h"ClassWizard::ClassWizard(QWidget *parent): QWizard(parent)
{addPage(new IntroPage);addPage(new ClassInfoPage);addPage(new CodeStylePage);addPage(new OutputFilesPage);addPage(new ConclusionPage);setPixmap(QWizard::BannerPixmap, QPixmap(":/images/banner.png"));setPixmap(QWizard::BackgroundPixmap, QPixmap(":/images/background.png"));setWindowTitle(tr("Class Wizard"));
}void ClassWizard::accept()
{QByteArray className = field("className").toByteArray();QByteArray baseClass = field("baseClass").toByteArray();QByteArray macroName = field("macroName").toByteArray();QByteArray baseInclude = field("baseInclude").toByteArray();QString outputDir = field("outputDir").toString();QString header = field("header").toString();QString implementation = field("implementation").toString();QByteArray block;if (field("comment").toBool()) {block += "/*\n";block += " " + header.toLatin1() + '\n';block += "*/\n";block += '\n';}if (field("protect").toBool()) {block += "#ifndef " + macroName + '\n';block += "#define " + macroName + '\n';block += '\n';}if (field("includeBase").toBool()) {block += "#include " + baseInclude + '\n';block += '\n';}block += "class " + className;if (!baseClass.isEmpty())block += " : public " + baseClass;block += '\n';block += "{\n";/* qmake ignore Q_OBJECT */if (field("qobjectMacro").toBool()) {block += " Q_OBJECT\n";block += '\n';}block += "public:\n";if (field("qobjectCtor").toBool()) {block += " " + className + "(QObject *parent = 0);\n";} else if (field("qwidgetCtor").toBool()) {block += " " + className + "(QWidget *parent = 0);\n";} else if (field("defaultCtor").toBool()) {block += " " + className + "();\n";if (field("copyCtor").toBool()) {block += " " + className + "(const " + className + " &other);\n";block += '\n';block += " " + className + " &operator=" + "(const " + className+ " &other);\n";}}block += "};\n";if (field("protect").toBool()) {block += '\n';block += "#endif\n";}QFile headerFile(outputDir + '/' + header);if (!headerFile.open(QFile::WriteOnly | QFile::Text)) {QMessageBox::warning(0, QObject::tr("Simple Wizard"),QObject::tr("Cannot write file %1:\n%2").arg(headerFile.fileName()).arg(headerFile.errorString()));return;}headerFile.write(block);block.clear();if (field("comment").toBool()) {block += "/*\n";block += " " + implementation.toLatin1() + '\n';block += "*/\n";block += '\n';}block += "#include \"" + header.toLatin1() + "\"\n";block += '\n';if (field("qobjectCtor").toBool()) {block += className + "::" + className + "(QObject *parent)\n";block += " : " + baseClass + "(parent)\n";block += "{\n";block += "}\n";} else if (field("qwidgetCtor").toBool()) {block += className + "::" + className + "(QWidget *parent)\n";block += " : " + baseClass + "(parent)\n";block += "{\n";block += "}\n";} else if (field("defaultCtor").toBool()) {block += className + "::" + className + "()\n";block += "{\n";block += " // missing code\n";block += "}\n";if (field("copyCtor").toBool()) {block += "\n";block += className + "::" + className + "(const " + className+ " &other)\n";block += "{\n";block += " *this = other;\n";block += "}\n";block += '\n';block += className + " &" + className + "::operator=(const "+ className + " &other)\n";block += "{\n";if (!baseClass.isEmpty())block += " " + baseClass + "::operator=(other);\n";block += " // missing code\n";block += " return *this;\n";block += "}\n";}}QFile implementationFile(outputDir + '/' + implementation);if (!implementationFile.open(QFile::WriteOnly | QFile::Text)) {QMessageBox::warning(0, QObject::tr("Simple Wizard"),QObject::tr("Cannot write file %1:\n%2").arg(implementationFile.fileName()).arg(implementationFile.errorString()));return;}implementationFile.write(block);QDialog::accept();
}IntroPage::IntroPage(QWidget *parent): QWizardPage(parent)
{setTitle(tr("Introduction"));setPixmap(QWizard::WatermarkPixmap, QPixmap(":/images/watermark1.png"));label = new QLabel(tr("This wizard will generate a skeleton C++ class ""definition, including a few functions. You simply ""need to specify the class name and set a few ""options to produce a header file and an ""implementation file for your new C++ class."));label->setWordWrap(true);QVBoxLayout *layout = new QVBoxLayout;layout->addWidget(label);setLayout(layout);
}ClassInfoPage::ClassInfoPage(QWidget *parent): QWizardPage(parent)
{setTitle(tr("Class Information"));setSubTitle(tr("Specify basic information about the class for which you ""want to generate skeleton source code files."));setPixmap(QWizard::LogoPixmap, QPixmap(":/images/logo1.png"));classNameLabel = new QLabel(tr("&Class name:"));classNameLineEdit = new QLineEdit;classNameLabel->setBuddy(classNameLineEdit);baseClassLabel = new QLabel(tr("B&ase class:"));baseClassLineEdit = new QLineEdit;baseClassLabel->setBuddy(baseClassLineEdit);qobjectMacroCheckBox = new QCheckBox(tr("Generate Q_OBJECT ¯o"));groupBox = new QGroupBox(tr("C&onstructor"));qobjectCtorRadioButton = new QRadioButton(tr("&QObject-style constructor"));qwidgetCtorRadioButton = new QRadioButton(tr("Q&Widget-style constructor"));defaultCtorRadioButton = new QRadioButton(tr("&Default constructor"));copyCtorCheckBox = new QCheckBox(tr("&Generate copy constructor and ""operator="));defaultCtorRadioButton->setChecked(true);connect(defaultCtorRadioButton, &QAbstractButton::toggled,copyCtorCheckBox, &QWidget::setEnabled);registerField("className*", classNameLineEdit);registerField("baseClass", baseClassLineEdit);registerField("qobjectMacro", qobjectMacroCheckBox);registerField("qobjectCtor", qobjectCtorRadioButton);registerField("qwidgetCtor", qwidgetCtorRadioButton);registerField("defaultCtor", defaultCtorRadioButton);registerField("copyCtor", copyCtorCheckBox);QVBoxLayout *groupBoxLayout = new QVBoxLayout;groupBoxLayout->addWidget(qobjectCtorRadioButton);groupBoxLayout->addWidget(qwidgetCtorRadioButton);groupBoxLayout->addWidget(defaultCtorRadioButton);groupBoxLayout->addWidget(copyCtorCheckBox);groupBox->setLayout(groupBoxLayout);QGridLayout *layout = new QGridLayout;layout->addWidget(classNameLabel, 0, 0);layout->addWidget(classNameLineEdit, 0, 1);layout->addWidget(baseClassLabel, 1, 0);layout->addWidget(baseClassLineEdit, 1, 1);layout->addWidget(qobjectMacroCheckBox, 2, 0, 1, 2);layout->addWidget(groupBox, 3, 0, 1, 2);setLayout(layout);
}CodeStylePage::CodeStylePage(QWidget *parent): QWizardPage(parent)
{setTitle(tr("Code Style Options"));setSubTitle(tr("Choose the formatting of the generated code."));setPixmap(QWizard::LogoPixmap, QPixmap(":/images/logo2.png"));commentCheckBox = new QCheckBox(tr("&Start generated files with a ""comment"));commentCheckBox->setChecked(true);protectCheckBox = new QCheckBox(tr("&Protect header file against multiple ""inclusions"));protectCheckBox->setChecked(true);macroNameLabel = new QLabel(tr("&Macro name:"));macroNameLineEdit = new QLineEdit;macroNameLabel->setBuddy(macroNameLineEdit);includeBaseCheckBox = new QCheckBox(tr("&Include base class definition"));baseIncludeLabel = new QLabel(tr("Base class include:"));baseIncludeLineEdit = new QLineEdit;baseIncludeLabel->setBuddy(baseIncludeLineEdit);connect(protectCheckBox, &QAbstractButton::toggled,macroNameLabel, &QWidget::setEnabled);connect(protectCheckBox, &QAbstractButton::toggled,macroNameLineEdit, &QWidget::setEnabled);connect(includeBaseCheckBox, &QAbstractButton::toggled,baseIncludeLabel, &QWidget::setEnabled);connect(includeBaseCheckBox, &QAbstractButton::toggled,baseIncludeLineEdit, &QWidget::setEnabled);registerField("comment", commentCheckBox);registerField("protect", protectCheckBox);registerField("macroName", macroNameLineEdit);registerField("includeBase", includeBaseCheckBox);registerField("baseInclude", baseIncludeLineEdit);QGridLayout *layout = new QGridLayout;layout->setColumnMinimumWidth(0, 20);layout->addWidget(commentCheckBox, 0, 0, 1, 3);layout->addWidget(protectCheckBox, 1, 0, 1, 3);layout->addWidget(macroNameLabel, 2, 1);layout->addWidget(macroNameLineEdit, 2, 2);layout->addWidget(includeBaseCheckBox, 3, 0, 1, 3);layout->addWidget(baseIncludeLabel, 4, 1);layout->addWidget(baseIncludeLineEdit, 4, 2);setLayout(layout);
}void CodeStylePage::initializePage()
{QString className = field("className").toString();macroNameLineEdit->setText(className.toUpper() + "_H");QString baseClass = field("baseClass").toString();includeBaseCheckBox->setChecked(!baseClass.isEmpty());includeBaseCheckBox->setEnabled(!baseClass.isEmpty());baseIncludeLabel->setEnabled(!baseClass.isEmpty());baseIncludeLineEdit->setEnabled(!baseClass.isEmpty());QRegularExpression rx("Q[A-Z].*");if (baseClass.isEmpty()) {baseIncludeLineEdit->clear();} else if (rx.match(baseClass).hasMatch()) {baseIncludeLineEdit->setText('<' + baseClass + '>');} else {baseIncludeLineEdit->setText('"' + baseClass.toLower() + ".h\"");}
}OutputFilesPage::OutputFilesPage(QWidget *parent): QWizardPage(parent)
{setTitle(tr("Output Files"));setSubTitle(tr("Specify where you want the wizard to put the generated ""skeleton code."));setPixmap(QWizard::LogoPixmap, QPixmap(":/images/logo3.png"));outputDirLabel = new QLabel(tr("&Output directory:"));outputDirLineEdit = new QLineEdit;outputDirLabel->setBuddy(outputDirLineEdit);headerLabel = new QLabel(tr("&Header file name:"));headerLineEdit = new QLineEdit;headerLabel->setBuddy(headerLineEdit);implementationLabel = new QLabel(tr("&Implementation file name:"));implementationLineEdit = new QLineEdit;implementationLabel->setBuddy(implementationLineEdit);registerField("outputDir*", outputDirLineEdit);registerField("header*", headerLineEdit);registerField("implementation*", implementationLineEdit);QGridLayout *layout = new QGridLayout;layout->addWidget(outputDirLabel, 0, 0);layout->addWidget(outputDirLineEdit, 0, 1);layout->addWidget(headerLabel, 1, 0);layout->addWidget(headerLineEdit, 1, 1);layout->addWidget(implementationLabel, 2, 0);layout->addWidget(implementationLineEdit, 2, 1);setLayout(layout);
}void OutputFilesPage::initializePage()
{QString className = field("className").toString();headerLineEdit->setText(className.toLower() + ".h");implementationLineEdit->setText(className.toLower() + ".cpp");outputDirLineEdit->setText(QDir::toNativeSeparators(QDir::tempPath()));
}ConclusionPage::ConclusionPage(QWidget *parent): QWizardPage(parent)
{setTitle(tr("Conclusion"));setPixmap(QWizard::WatermarkPixmap, QPixmap(":/images/watermark2.png"));label = new QLabel;label->setWordWrap(true);QVBoxLayout *layout = new QVBoxLayout;layout->addWidget(label);setLayout(layout);
}void ConclusionPage::initializePage()
{QString finishText = wizard()->buttonText(QWizard::FinishButton);finishText.remove('&');label->setText(tr("Click %1 to generate the class skeleton.")
.main
#include <QApplication>
#include <QTranslator>
#include <QLocale>
#include <QLibraryInfo>#include "classwizard.h"int main(int argc, char *argv[])
{Q_INIT_RESOURCE(classwizard);QApplication app(argc, argv);#ifndef QT_NO_TRANSLATIONQString translatorFileName = QLatin1String("qt_");translatorFileName += QLocale::system().name();QTranslator *translator = new QTranslator(&app);if (translator->load(translatorFileName, QLibraryInfo::location(QLibraryInfo::TranslationsPath)))app.installTranslator(translator);
#endifClassWizard wizard;wizard.show();return app.exec();
}
相关文章:
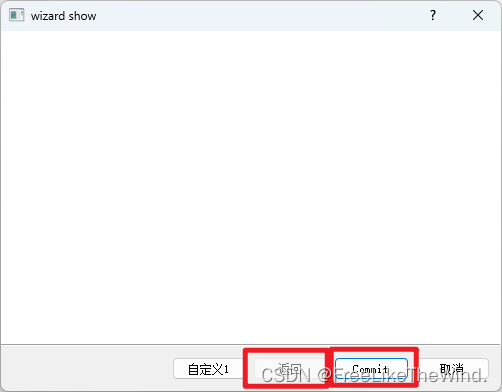
【Qt之QWizardPage】使用
介绍 QWizardPage类是向导页面的基类。 QWizard表示一个向导。每个页面都是一个QWizardPage。当创建自己的向导时,可以直接使用QWizardPage,也可以子类化它以获得更多控制。 页面具有以下属性,由QWizard呈现:a title,…...
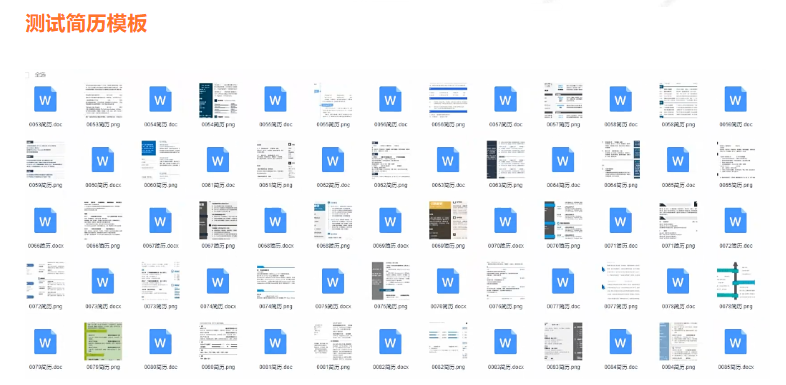
自动化测试,5个技巧轻松搞定
想要在质量保证团队中赢得核心?当你组建你的网络应用时要记住这些技巧,可以变得更容易分析并快速创建更多准确可重复的自动化测试。 1.歧义是敌人 尽可能使你的代码具体化。当然,你已经遵循了W3C标准,对吗?以下有三件…...
EasyWeChat调用企业微信接口获取客户群数据
use EasyWeChat\Factory; use fast\Http;$config [corp_id > Config::get(site.corp_id),agent_id > Config::get(site.agend_id), // 如果有 agend_id 则填写secret > Config::get(site.agent_secret),// 指定 API 调用返回结果的类型:array(default)…...
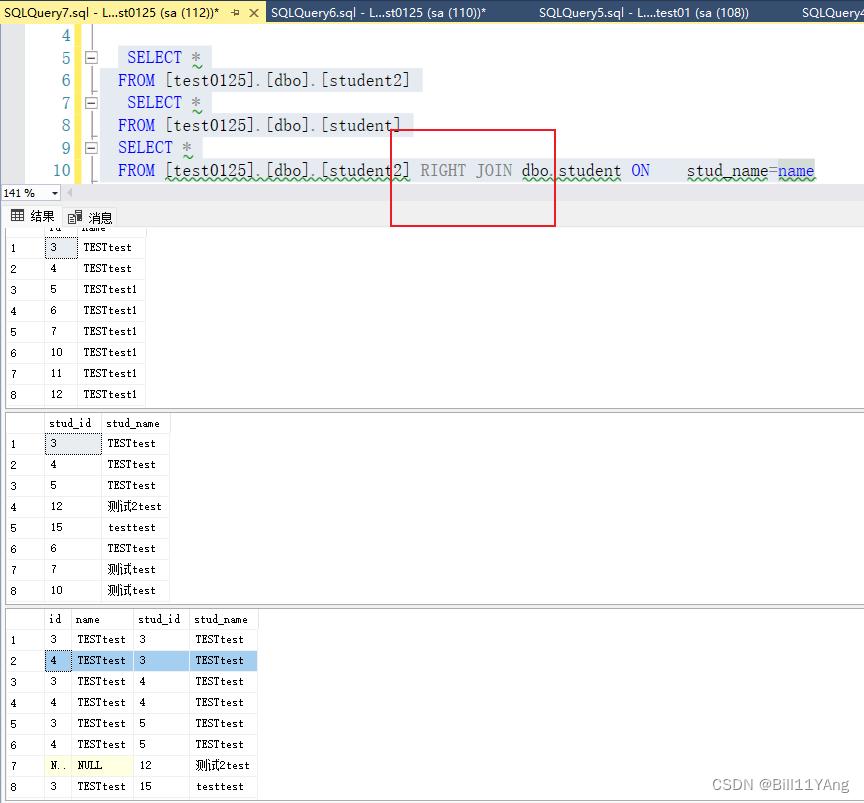
sql 左联 右联
...
k8s中的端口hostPort、port、nodePort、targetPort
hostPort:apiVersion: v1 kind: Pod metadata:name: tomcat spec:containers:- name: tomcatimage: tomcat:8.5ports:- hostPort: 8081containerPort: 8080protocol: TCPhostPort 类似docker -p 参数做的端口映射,将容器内端口映射到宿主机上(hostPort), 在k8s中&am…...
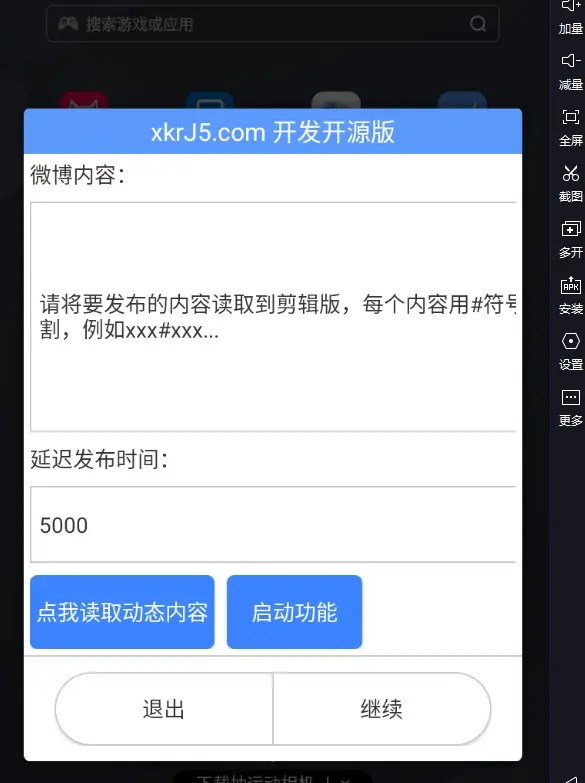
自动发微博脚本工具,可批量定时发送,按键精灵完全开源版
就跟标题上面讲的,软件是我之前开发好的,所有功能都能用,是按键精灵的脚本,只是单设备操作,也可以在模拟器下面操作,UI代码方面都设计的很完整,我这边就干脆分享出来给大家用,不用繁…...
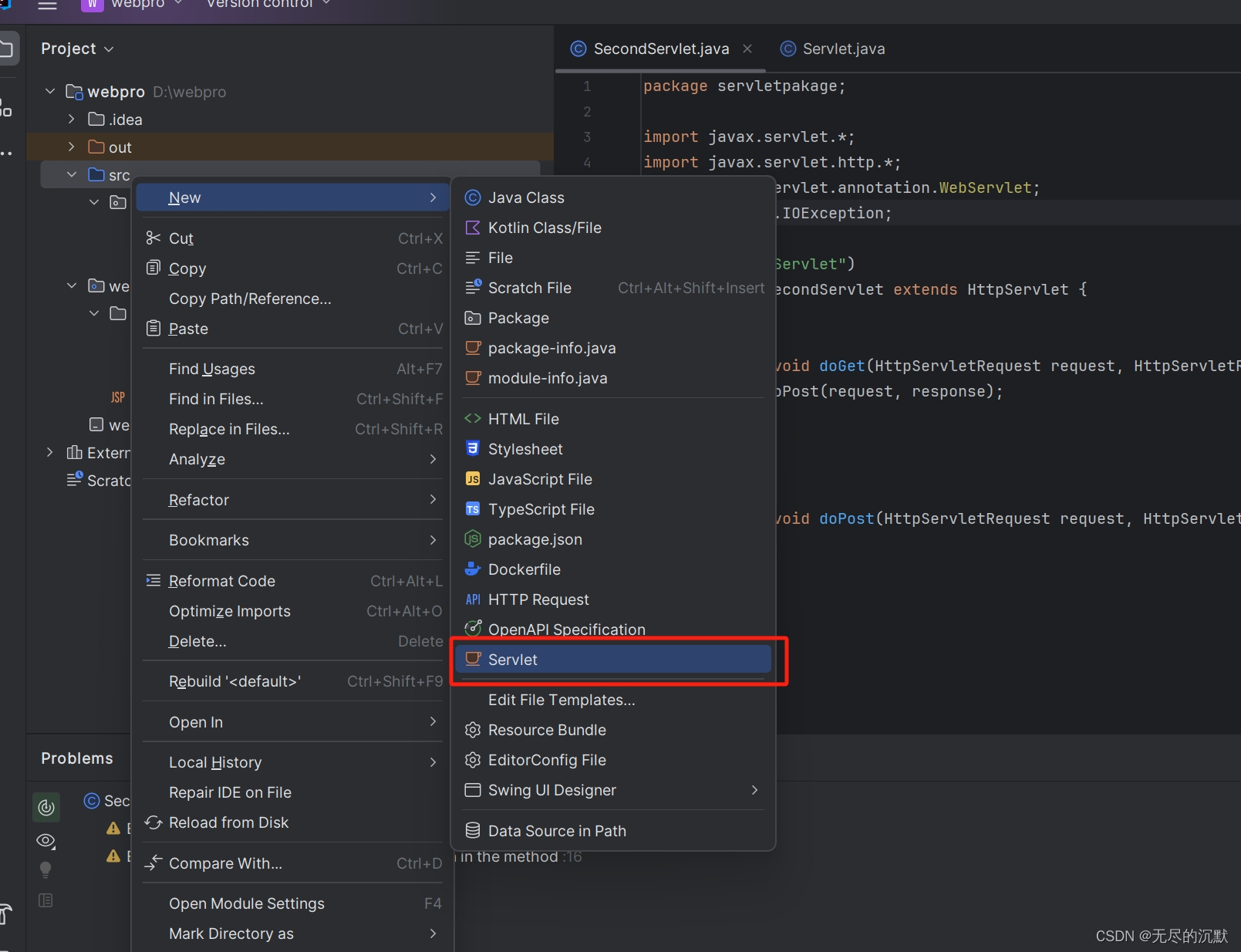
2023版Idea创建JavaWeb时,右键new没有Servlet快捷键选项
问题:右键时,没有创建servlet的快捷键,如下图: 解决方法: 1.打开idea,点击File>settings(设置),进入settings页面,如下 从上图中的Files选项中没看到有servlet选项,…...
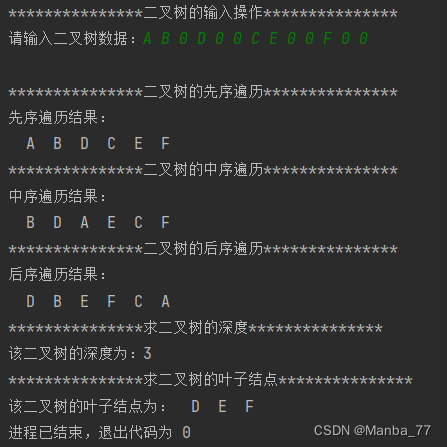
数据结构(c语言版本) 二叉树的遍历
要求 实现二叉树的创建,并输入二叉树数据 然后先序遍历输出二叉树、中序遍历输出二叉树、后序输出二叉树 输出二叉树的深度、二叉树的叶子结点 例如二叉树为: 该二叉树的先序遍历结果为: A B D C E F 该二叉树的中序遍历结果为:…...
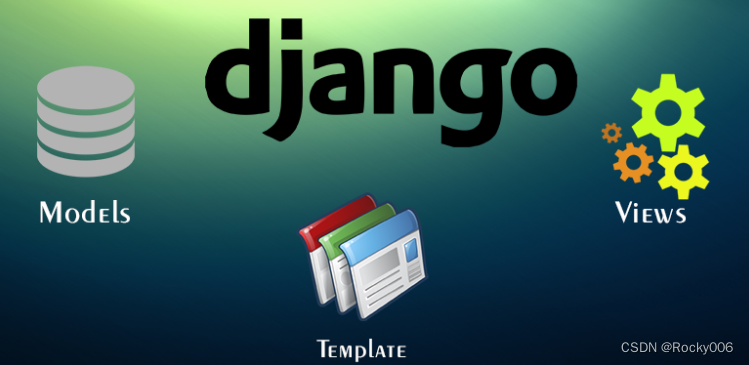
Django 配置 Email Admin 详细指南
概要 Django 是一个高级的 Python Web 框架,它鼓励快速开发和清洁、实用的设计。当你正在开发一个 Django 项目时,监控网站的运行情况是非常必要的。Django 提供了一个功能强大的 admin 界面,但同时也可以通过配置 email admin 来获取网站的…...
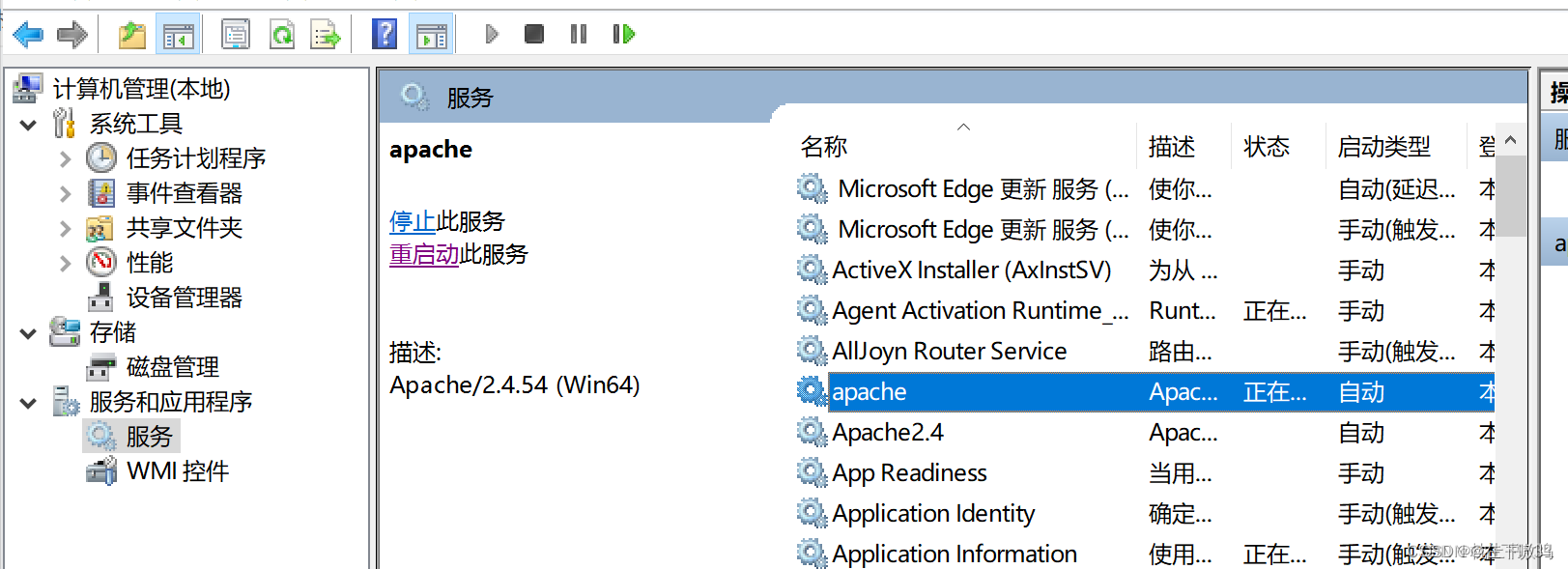
Apache阿帕奇安装配置
目录 一、下载程序 1. 点击Download 2. 点击Files for Microsoft Windows 3. 点击Apache Lounge 4. 点击httpd-2.4.54-win64-VSI6.zip 5. 下载压缩包 6.解压到文件夹里 二、配置环境变量 1. 右键我的电脑 - 属性 2. 高级系统设置 3. 点击环境变量 4. 点击系统变…...
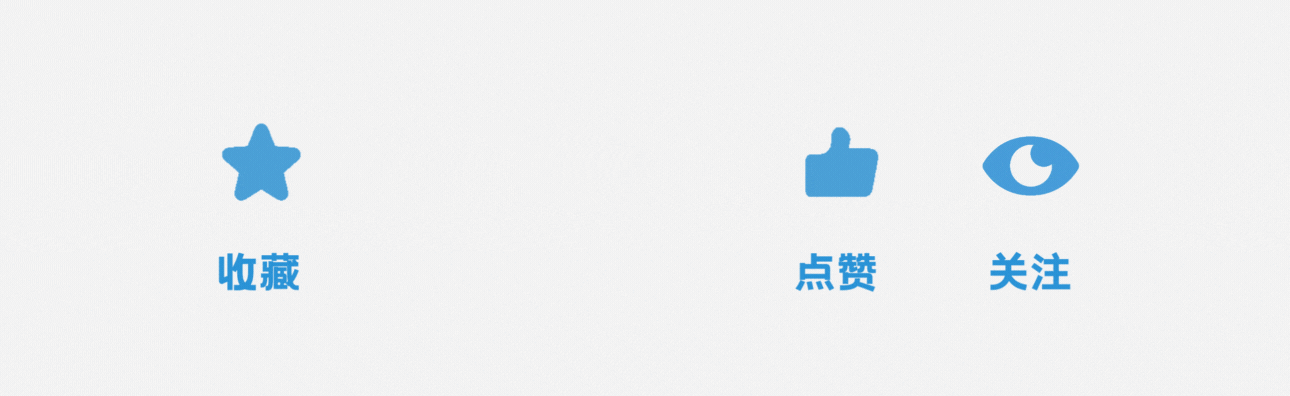
时间序列预测实战(十六)PyTorch实现GRU-FCN模型长期预测并可视化结果
往期回顾:时间序列预测专栏——包含上百种时间序列模型带你从入门到精通时间序列预测 一、本文介绍 本文讲解的实战内容是GRU-FCN(门控循环单元-全卷积网络),这是一种结合了GRU(用于处理时间序列数据)和FCN(全卷积网络…...
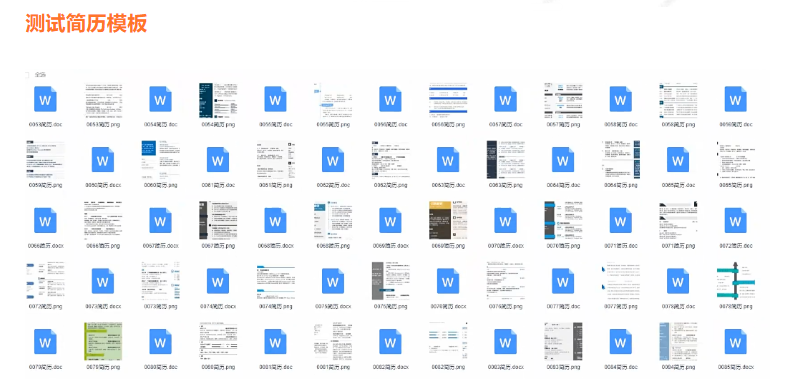
如何提升软件测试效率?本文为你揭示秘密
在软件开发中,测试是至关重要的一个环节。它能帮助我们发现并修复问题,从而确保我们提供的软件具有高质量。然而,测试过程往往费时费力。那么,有没有方法可以提升我们的软件测试效率呢?答案是肯定的。下面,…...
参数估计和非参数估计
一、参数估计 参数估计是统计学中的一个重要概念,它涉及到使用样本数据来估计总体参数的过程。在统计学中,总体是指研究对象的整体集合,而样本是从总体中抽取的部分元素。 参数估计有两种主要方法:点估计和区间估计。 点估计&am…...
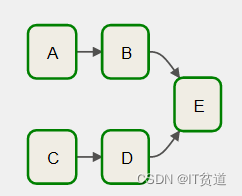
Apache Airflow (八) :DAG任务依赖设置
🏡 个人主页:IT贫道_大数据OLAP体系技术栈,Apache Doris,Clickhouse 技术-CSDN博客 🚩 私聊博主:加入大数据技术讨论群聊,获取更多大数据资料。 🔔 博主个人B栈地址:豹哥教你大数据的个人空间-豹…...
使用 com.jacob.activeX 库实现 Word 到 PDF
使用 com.jacob.activeX 库实现 Word 到 PDF 的转换涉及到使用 Java 和 Microsoft Office 的 COM 自动化。JACOB(Java COM Bridge)库提供了一个桥接器,允许 Java 代码通过 COM(组件对象模型)与 Windows 应用程序&#…...
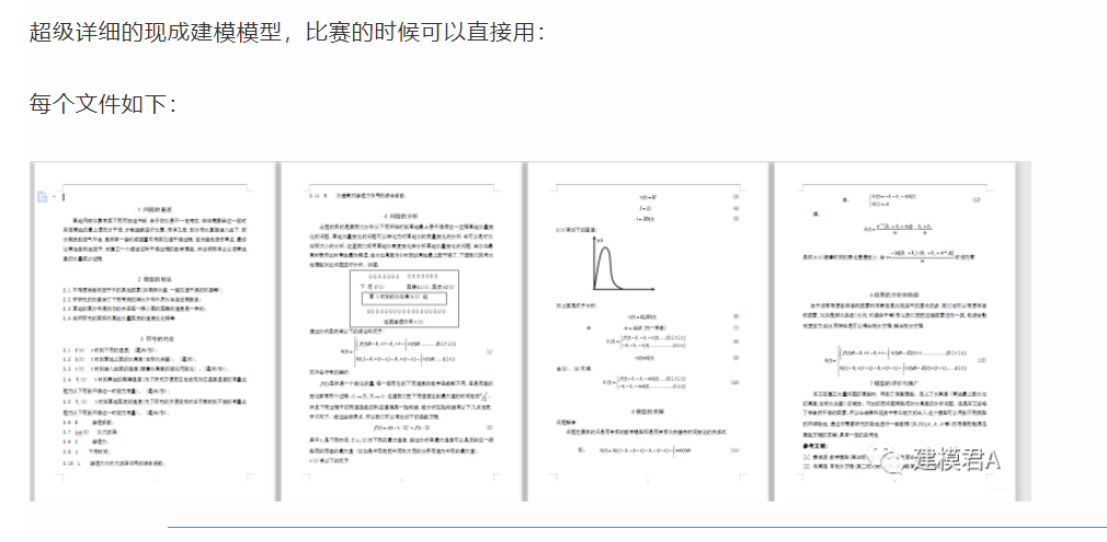
2023亚太杯数学建模思路 - 案例:FPTree-频繁模式树算法
文章目录 算法介绍FP树表示法构建FP树实现代码 建模资料 ## 赛题思路 (赛题出来以后第一时间在CSDN分享) https://blog.csdn.net/dc_sinor?typeblog 算法介绍 FP-Tree算法全称是FrequentPattern Tree算法,就是频繁模式树算法,…...
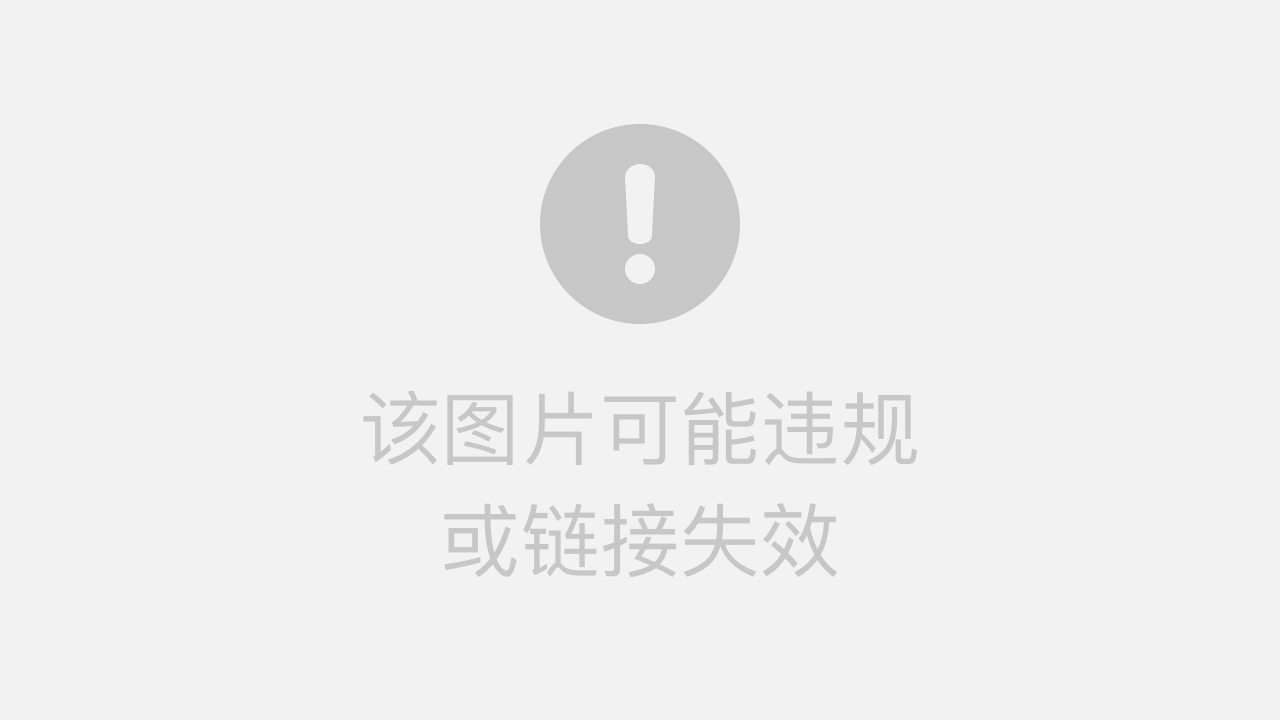
Dart利用私有构造函数_()创建单例模式
文章目录 类的构造函数_()函数dart中构造函数定义 类的构造函数 类的构造函数有两种: 1)默认构造函数: 当实例化对象的时候,会自动调用的函数,构造函数的名称和类的名称相同,在一个类中默认构造函数只能由…...
简述如何使用Androidstudio对文件进行保存和获取文件中的数据
在 Android Studio 中,可以使用以下方法对文件进行保存和获取文件中的数据: 保存文件: 创建一个 File 对象,指定要保存的文件路径和文件名。使用 FileOutputStream 类创建一个文件输出流对象。将需要保存的数据写入文件输出流中…...
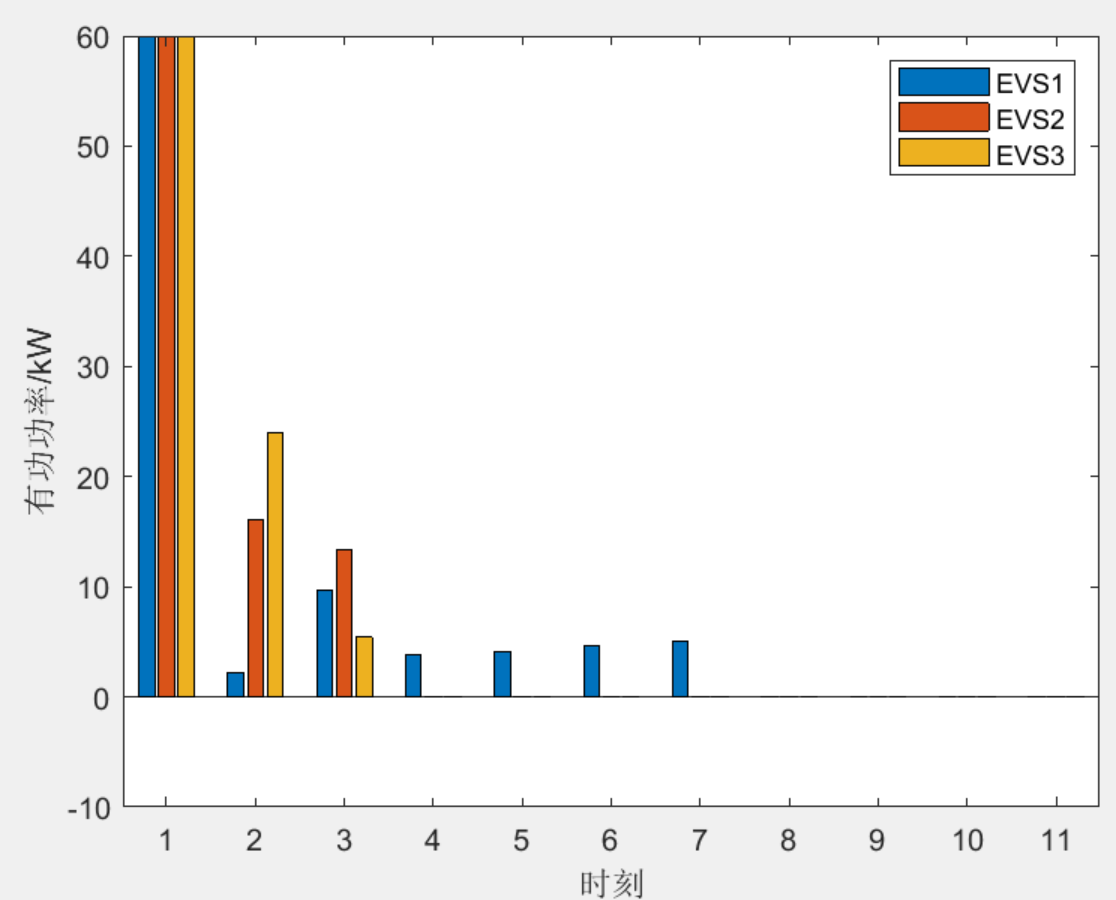
面向配电网韧性提升的移动储能预布局与动态调度策略(matlab代码)
欢迎关注威♥“电击小子程高兴的MATLAB小屋”获取更多资料 该程序复现《面向配电网韧性提升的移动储能预布局与动态调度策略》,具体摘要内容见下图,程序主要分为两大模块,第一部分是灾前预防代码,该部分采用两阶段优化算法&#…...
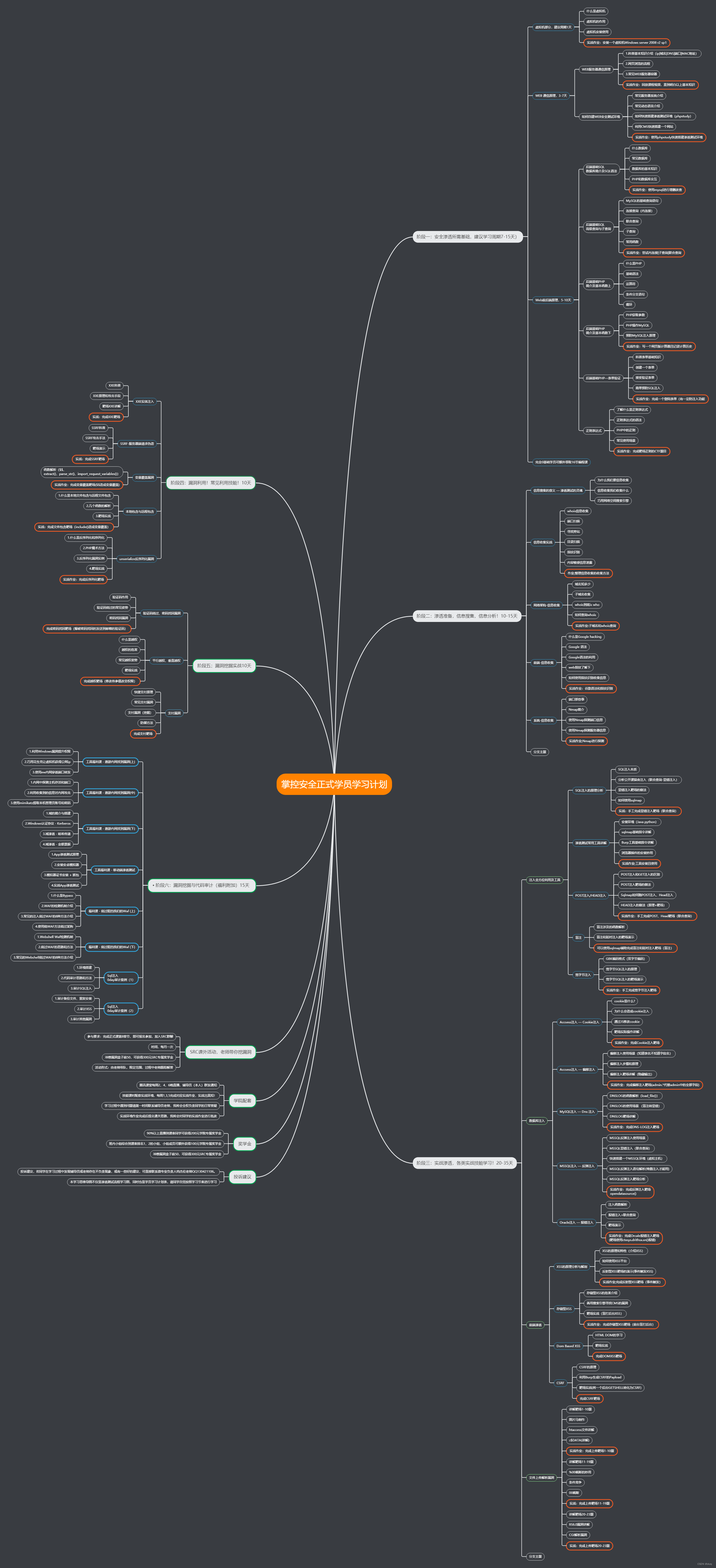
内网信息收集
目录 本机信息收集 查看系统配置信息 查看系统服务信息 查看系统登录信息 自动信息收集 域内信息收集 判断是否存在域 探测域内存主机&端口 powershell arp扫描 小工具 telnet 查看用户&机器&会话相关信息 查看机器相关信息 查看用户相关信息 免费领…...
在软件开发中正确使用MySQL日期时间类型的深度解析
在日常软件开发场景中,时间信息的存储是底层且核心的需求。从金融交易的精确记账时间、用户操作的行为日志,到供应链系统的物流节点时间戳,时间数据的准确性直接决定业务逻辑的可靠性。MySQL作为主流关系型数据库,其日期时间类型的…...
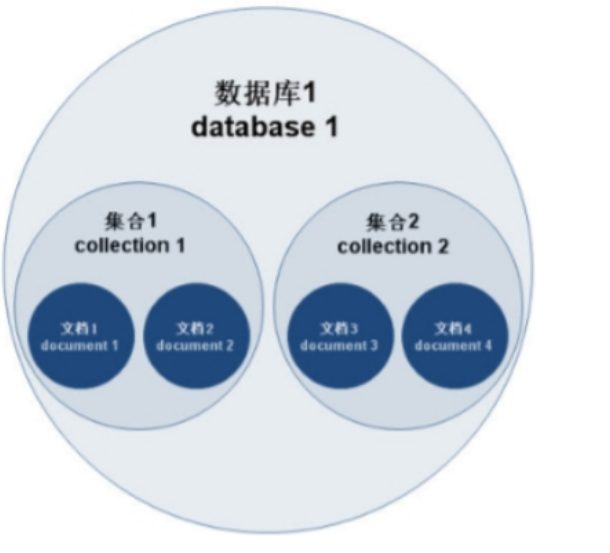
MongoDB学习和应用(高效的非关系型数据库)
一丶 MongoDB简介 对于社交类软件的功能,我们需要对它的功能特点进行分析: 数据量会随着用户数增大而增大读多写少价值较低非好友看不到其动态信息地理位置的查询… 针对以上特点进行分析各大存储工具: mysql:关系型数据库&am…...
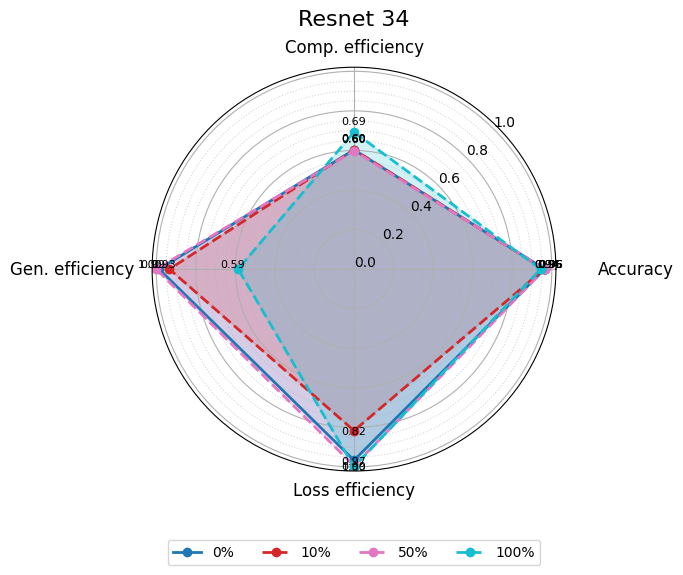
使用分级同态加密防御梯度泄漏
抽象 联邦学习 (FL) 支持跨分布式客户端进行协作模型训练,而无需共享原始数据,这使其成为在互联和自动驾驶汽车 (CAV) 等领域保护隐私的机器学习的一种很有前途的方法。然而,最近的研究表明&…...
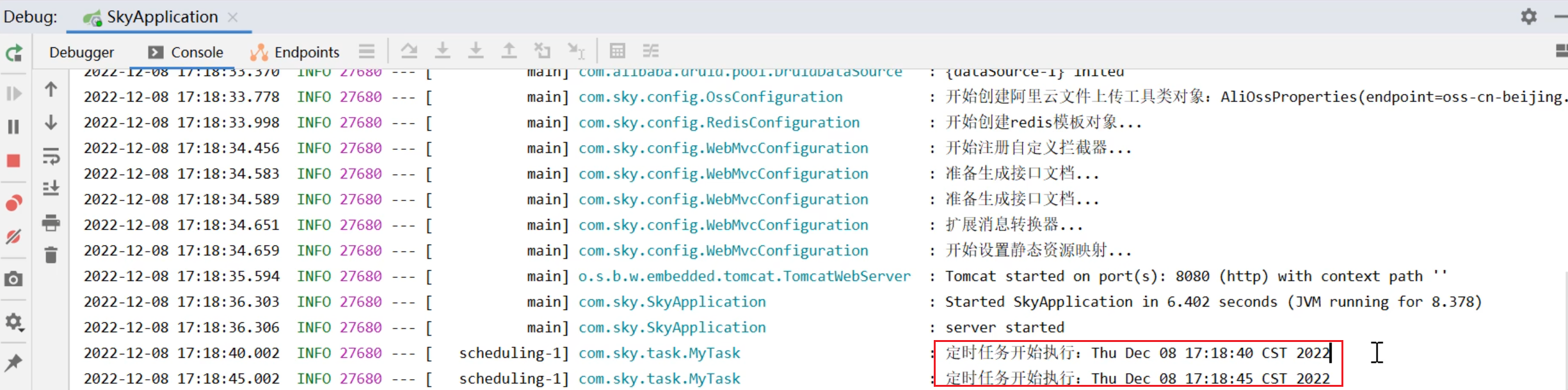
SpringTask-03.入门案例
一.入门案例 启动类: package com.sky;import lombok.extern.slf4j.Slf4j; import org.springframework.boot.SpringApplication; import org.springframework.boot.autoconfigure.SpringBootApplication; import org.springframework.cache.annotation.EnableCach…...
Pinocchio 库详解及其在足式机器人上的应用
Pinocchio 库详解及其在足式机器人上的应用 Pinocchio (Pinocchio is not only a nose) 是一个开源的 C 库,专门用于快速计算机器人模型的正向运动学、逆向运动学、雅可比矩阵、动力学和动力学导数。它主要关注效率和准确性,并提供了一个通用的框架&…...
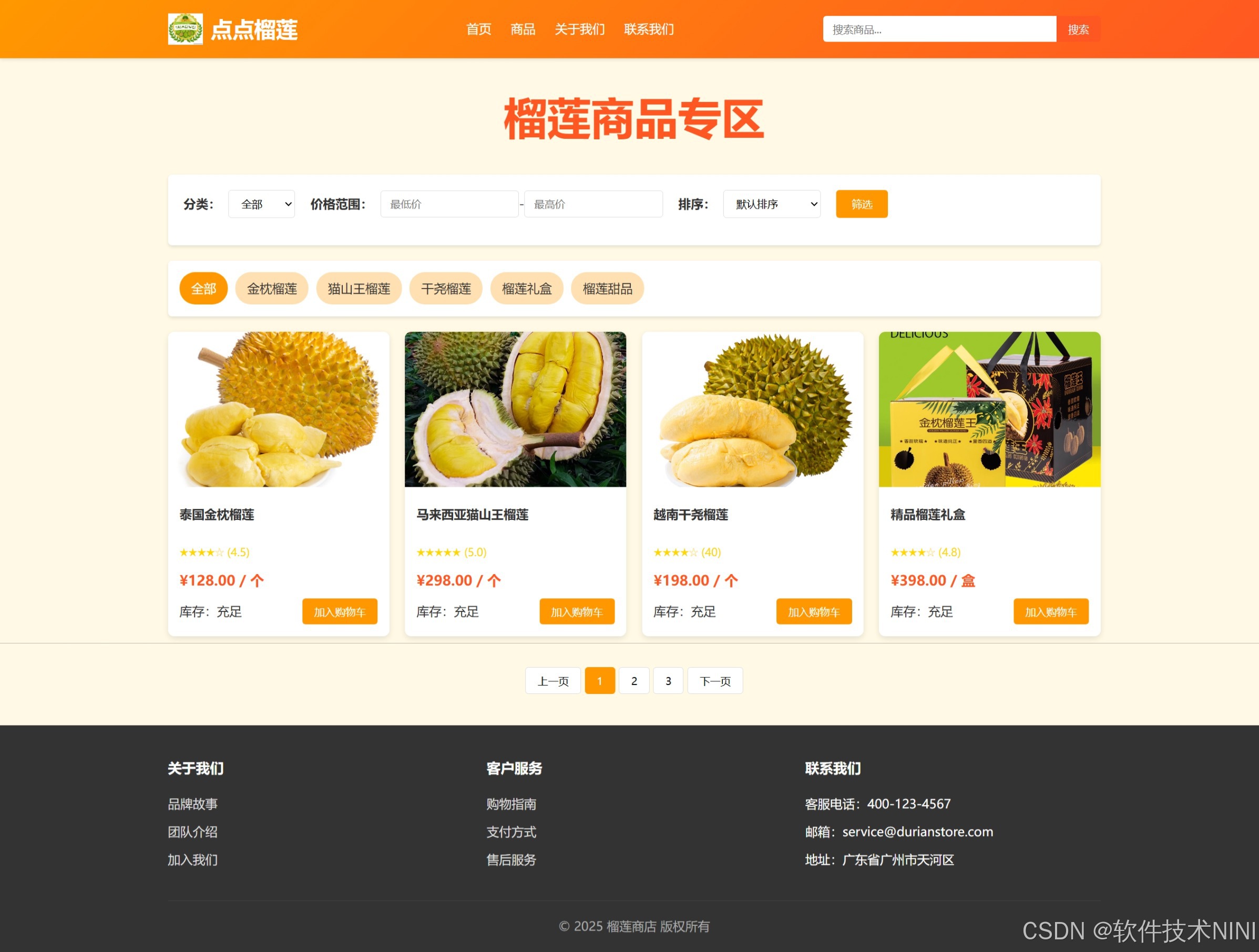
html css js网页制作成品——HTML+CSS榴莲商城网页设计(4页)附源码
目录 一、👨🎓网站题目 二、✍️网站描述 三、📚网站介绍 四、🌐网站效果 五、🪓 代码实现 🧱HTML 六、🥇 如何让学习不再盲目 七、🎁更多干货 一、👨…...
Python ROS2【机器人中间件框架】 简介
销量过万TEEIS德国护膝夏天用薄款 优惠券冠生园 百花蜂蜜428g 挤压瓶纯蜂蜜巨奇严选 鞋子除臭剂360ml 多芬身体磨砂膏280g健70%-75%酒精消毒棉片湿巾1418cm 80片/袋3袋大包清洁食品用消毒 优惠券AIMORNY52朵红玫瑰永生香皂花同城配送非鲜花七夕情人节生日礼物送女友 热卖妙洁棉…...
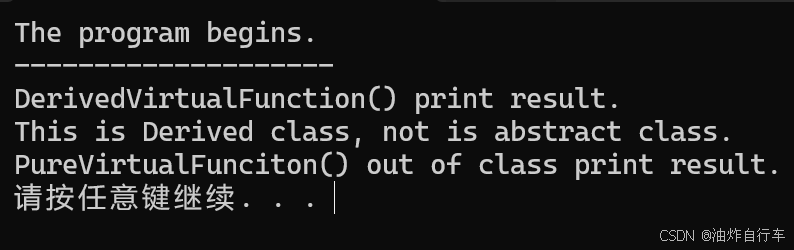
【C++】纯虚函数类外可以写实现吗?
1. 答案 先说答案,可以。 2.代码测试 .h头文件 #include <iostream> #include <string>// 抽象基类 class AbstractBase { public:AbstractBase() default;virtual ~AbstractBase() default; // 默认析构函数public:virtual int PureVirtualFunct…...
6️⃣Go 语言中的哈希、加密与序列化:通往区块链世界的钥匙
Go 语言中的哈希、加密与序列化:通往区块链世界的钥匙 一、前言:离区块链还有多远? 区块链听起来可能遥不可及,似乎是只有密码学专家和资深工程师才能涉足的领域。但事实上,构建一个区块链的核心并不复杂,尤其当你已经掌握了一门系统编程语言,比如 Go。 要真正理解区…...
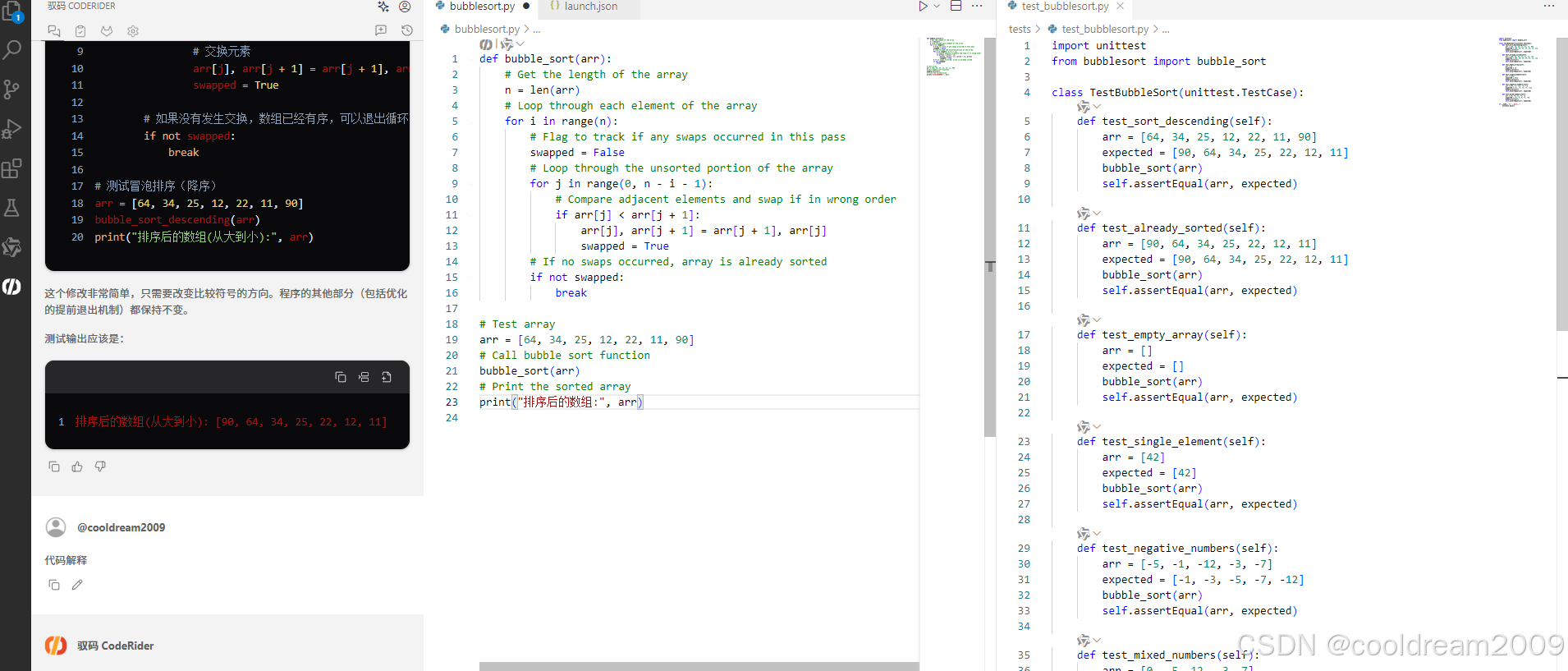
在 Visual Studio Code 中使用驭码 CodeRider 提升开发效率:以冒泡排序为例
目录 前言1 插件安装与配置1.1 安装驭码 CodeRider1.2 初始配置建议 2 示例代码:冒泡排序3 驭码 CodeRider 功能详解3.1 功能概览3.2 代码解释功能3.3 自动注释生成3.4 逻辑修改功能3.5 单元测试自动生成3.6 代码优化建议 4 驭码的实际应用建议5 常见问题与解决建议…...