04 在Vue3中使用setup语法糖
概述
Starting from Vue 3.0, Vue introduces a new syntactic sugar setup attribute for the <script>
tag. This attribute allows you to write code using Composition API (which we will discuss further in Chapter 5, The Composition API) in SFCs and shorten the amount of code needed for writing simple components.
从 Vue 3.0 开始,Vue 为 <script>
标签引入了一个新的语法糖设置属性。该属性允许您在 SFC 中使用 Composition API编写代码,并缩短编写简单组件所需的代码量。
The code block residing within the <script setup>
tag will then be compiled into a render() function before being deployed to the browser, providing better runtime performance.
<script setup>
标签中的代码块在部署到浏览器之前会被编译成 render() 函数,从而提供更好的运行时性能。
To start using this syntax, we take the following example code:
要开始使用这种语法,我们以下面的代码为例:
<script>
import logo from 'components/logo.vue'export default {components: {logo}
}
</script>
Then, we replace <script>
with <script setup>
, and remove all the code blocks of export default…. The example code now becomes as follows:
然后,将 <script>
替换为 <script setup>
,并删除 export default… 的所有代码块。现在的示例代码如下
<script setup>
import logo from 'components/logo.vue'
</script>
In <template>
, we use logo as usual:
在 <template>
中,我们照常使用徽标:
<template><header><a href="mywebsite.com"><logo /></a></header>
</template>
To define and use local data, instead of using data(), we can declare regular variables as local data and functions as local methods for that component directly. For example, to declare and render a local data property of color, we use the following code:
要定义和使用本地数据,我们可以不使用 data(),而是直接将常规变量声明为本地数据,将函数声明为该组件的本地方法。例如,要声明并呈现颜色的本地数据属性,我们可以使用以下代码:
<script setup>
const color = 'red';
</script>
<template><div>{{color}}</div>
</template>
The preceding code outputs the same result as the example in the previous section –red.
前面的代码输出的结果与上一节的示例相同–red。
As mentioned at the beginning of this section, <script setup>
is the most useful when you need to use Composition API within SFCs. Still, we can always take advantage of its simplicity for simple components.
正如本节开头提到的,<script setup>
在需要在 SFC 中使用 Composition API 时最有用。不过,对于简单的组件,我们也可以利用它的简洁性。
NOTE: From this point onward, we will combine both approaches and use <script setup>
whenever possible.
注意:从现在起,我们将把两种方法结合起来,尽可能使用 <script setup>
。
In the following exercise, we will go into more detail about how to use interpolation and data.
在下面的练习中,我们将详细介绍如何使用插值法和数据。
练习:条件插值
When you want to output data into your template or make elements on a page reactive, interpolate data into the template by using curly braces. Vue can understand and replace that placeholder with data.
当您想在模板中输出数据或使页面上的元素具有反应性时,可使用大括号在模板中插入数据。Vue 可以理解并用数据替换占位符。
Create a new Vue component file named Exercise1-02.vue in the src/components directory.
在 src/components 目录中新建一个名为 Exercise1-02.vue 的 Vue 组件文件。
Inside the Exercise1-02.vue component, let’s add data within the <script setup>
tags by adding a function called data(), and return a key called title with your heading string as the value:
在 Exercise1-02.vue 组件中,让我们在 <script setup>
标记中添加数据,添加一个名为 data() 的函数,并返回一个名为 title 的键,其值为标题字符串:
<script>
export default {data() {return {title: 'My first component!',}},
}
</script>
Reference title by replacing your <h1>
text with the interpolated value of {{ title }}:
将 <h1>
文本替换为 {{ title }} 的内插值,从而引用标题:
<template><div><h1>{{ title }}</h1></div>
</template>
修改App.vue,引入组件并渲染:
<script setup>
import Exercise102 from "./components/Exercise1-02.vue";
</script>
<template><Exercise102/>
</template>
When you save this document, the data title will now appear inside your h1 tag.
保存此文档时,数据标题将显示在 h1 标记内。
In Vue, interpolation will resolve any JavaScript that’s inside curly braces. For example, you can transform the text inside your curly braces using the toUpperCase() method:
在 Vue 中,插值将解决大括号内的任何 JavaScript 问题。例如,您可以使用 toUpperCase() 方法转换大括号内的文本:
<template><div><h1>{{ title.toUpperCase() }}</h1></div>
</template>
Interpolation can also handle conditional logic. Inside the data object, add a Boolean key-value pair, isUppercase: false:
插值还可以处理条件逻辑。在数据对象中,添加一个布尔键值对:isUppercase: false:
<script>
export default {data() {return {title: 'My first component!',isUppercase: false,}},
}
</script><template><div><h1>{{ isUppercase ? title.toUpperCase() : title }}</h1></div>
</template>
Now, let’s replace <script>
with <script setup>
and move all the local data declared within the data() function to its own variable names respectively, such as title and isUpperCase, as shown here:
现在,我们将 <script>
替换为 <script setup>
,并将 data() 函数中声明的所有本地数据分别移到自己的变量名中,如 title 和 isUpperCase,如下所示:
<script setup>
const title ='My first component!';
const isUppercase = true;
</script>
In this exercise, we were able to apply inline conditions within the interpolated tags ({{}}) by using a Boolean variable. The feature allows us to modify what data to display without overly complicated situations, which can be helpful in certain use cases. We also learned how to write a more concise version of the component using <script setup>
in the end.
在本练习中,我们可以使用布尔变量在插值标记({{}})中应用内联条件。这一功能让我们可以修改要显示的数据,而不必考虑过于复杂的情况,这在某些用例中很有帮助。最后,我们还学会了如何使用 <script setup>
编写更简洁的组件版本。
Since we are now familiar with using interpolation to bind local data, we will move on to our next topic – how to attach data and methods to HTML element events and attributes using Vue attributes.
既然我们现在已经熟悉了使用插值绑定本地数据,那么我们将进入下一个主题–如何使用 Vue 属性将数据和方法附加到 HTML 元素事件和属性上。
相关文章:

04 在Vue3中使用setup语法糖
概述 Starting from Vue 3.0, Vue introduces a new syntactic sugar setup attribute for the <script> tag. This attribute allows you to write code using Composition API (which we will discuss further in Chapter 5, The Composition API) in SFCs and shorte…...

vite+ts——user.ts——ts接口定义+axios请求的写法
import axios from axios; import qs from query-string; import {UserState} from /store/modules/user/types;export interface LoginData{username:string;password:string;grant_type?:string;scope?:string;client_id?:string;client_secret?:string;response_type?:…...
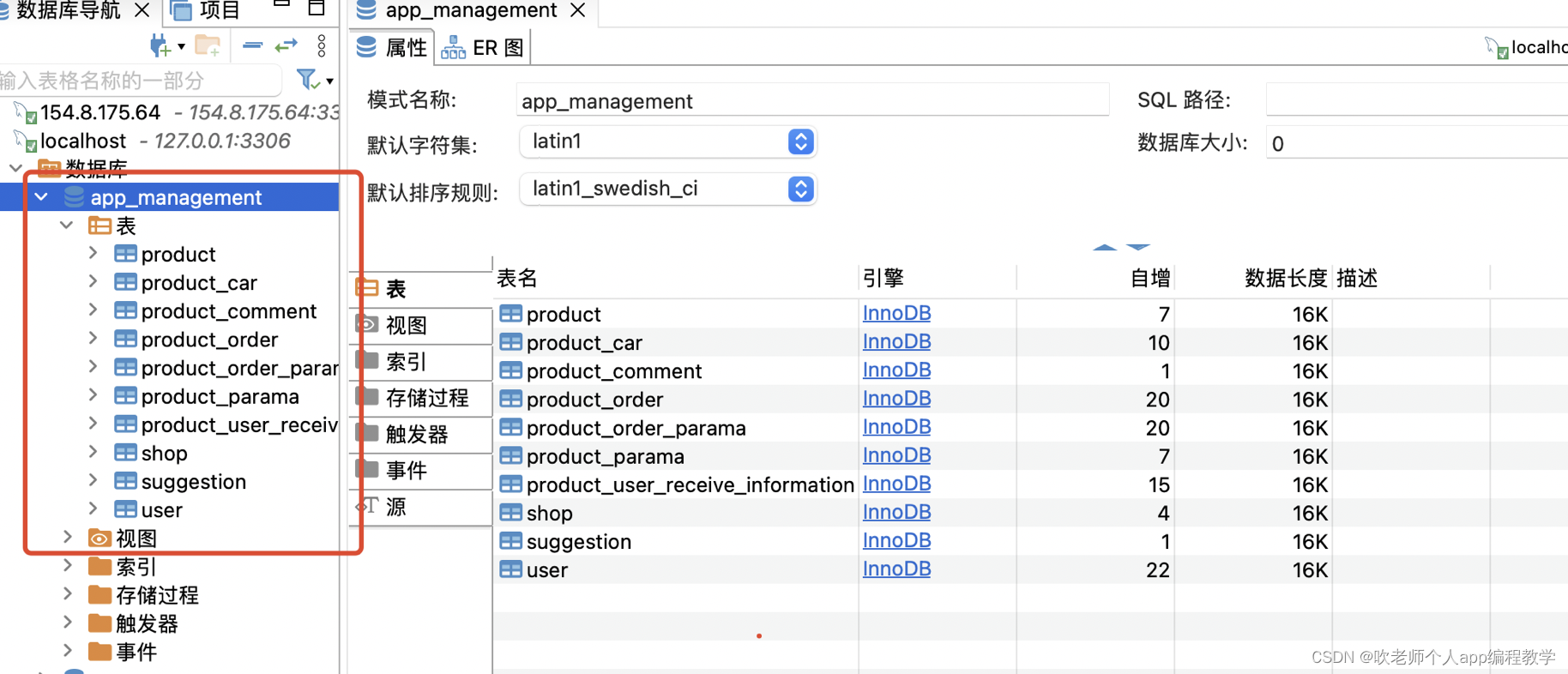
环境搭建及源码运行_java环境搭建_mysql安装
书到用时方恨少、觉知此时要躬行;拥有技术,成就未来,抖音视频教学地址: 1、介绍 MySQL是一个关系型数据库管理系统,由瑞典MySQL AB 公司开发,属于 Oracle旗下产品。MySQL是最…...
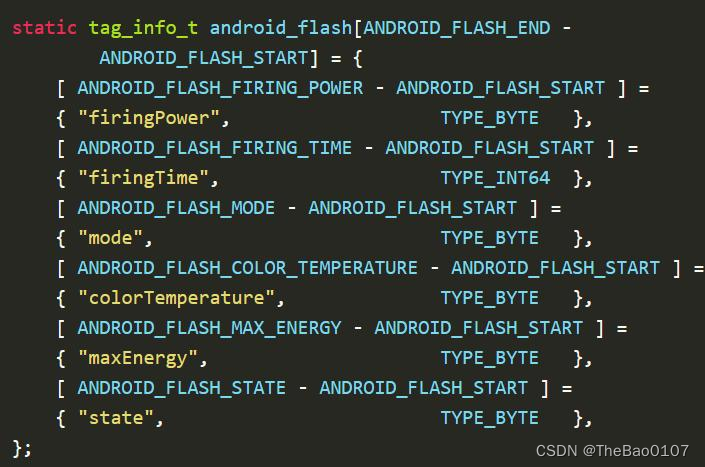
Android camera的metadata
一、实现 先看一下metadata内部是什么样子: 可以看出,metadata 内部是一块连续的内存空间。 其内存分布大致可概括为: 区域一 :存 camera_metadata_t 结构体定义,占用内存 96 Byte 区域二 :保留区&#x…...

ElasticSearch面试题
1.介绍下es的架构? es采用的是分布式的架构,es集群中会有多个结点,而结点的角色主要有下面几种。 协调结点: 请求路由能力,将请求内容将请求转发给对应的结点进行处理。 master结点: 结点管理ÿ…...

C++ 数据结构知识点合集-C/C++ 数组允许定义可存储相同类型数据项的变量-供大家学习研究参考
#include <iostream> #include <cstring>using namespace std;// 声明一个结构体类型 Books struct Books {char title[50];char author[50];char subject[100];int book_id; };int main( ) {Books Book1; // 定义结构体类型 Books 的变量 Book1Books …...

【机器学习】5分钟掌握机器学习算法线上部署方法
5分钟掌握机器学习算法线上部署方法 1. 三种情况2. 如何转换PMML,并封装PMML2.1 什么是PMML2.2 PMML的使用方法范例3. 各个算法工具的工程实践4. 只用Linux的Shell来调度模型的实现方法5. 注意事项参考资料本文介绍业务模型的上线流程。首先在训练模型的工具上,一般三个模型训…...
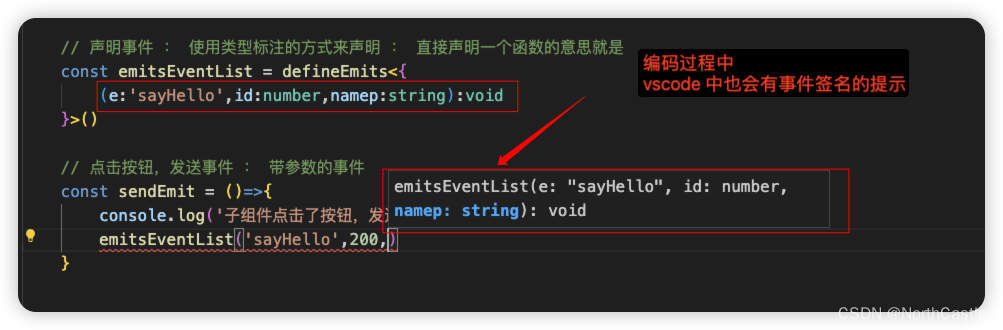
Vue3-21-组件-子组件给父组件发送事件
情景描述 【子组件】中有一个按钮,点击按钮,触发一个事件, 我们希望这个事件的处理逻辑是,给【父组件】发送一条消息过去, 从而实现 【子组件】给【父组件】通信的效果。这个问题的解决就是 “发送事件” 这个操作。 …...
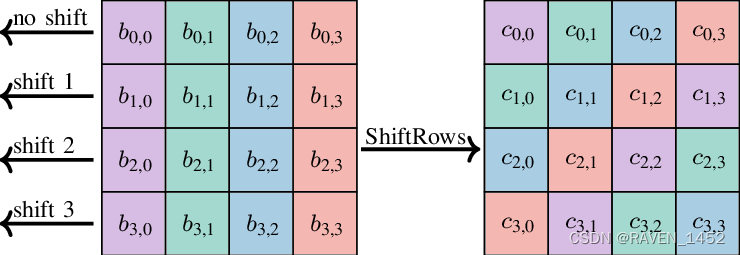
[密码学]AES
advanced encryption standard,又名rijndael密码,为两位比利时数学家的名字组合。 分组为128bit,密钥为128/192/256bit可选,对应加密轮数10/12/14轮。 基本操作为四种: 字节代换(subBytes transformatio…...

CentOS 7 部署pure-ftp
文章目录 (1)简介(2)准备工作(3)更新系统(4)安装依赖环境(5)下载和解压pure-ftp源码包(6)编译和安装pure-ftp(7࿰…...
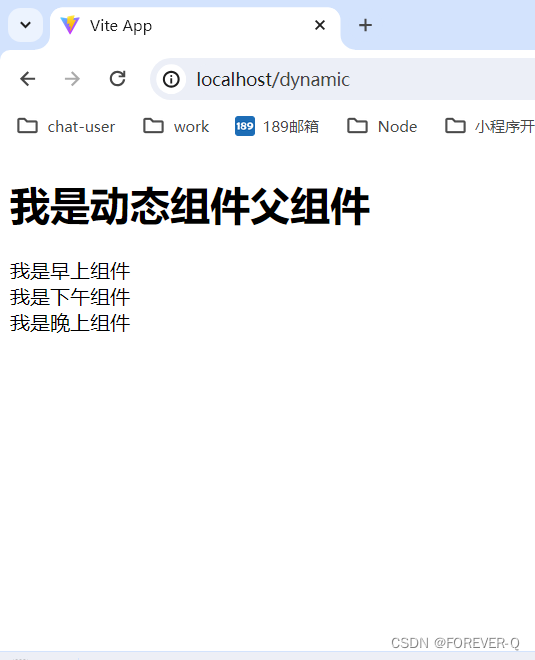
Vue2-动态组件案例
1.component介绍 说明: Type: string | ComponentDefinition | ComponentConstructor Explanation: String: 如果你传递一个字符串给 is,它会被视为组件的名称,用于动态地渲染不同类型的组件。这是一个在运行时动态切换组件类型的常见用例。…...
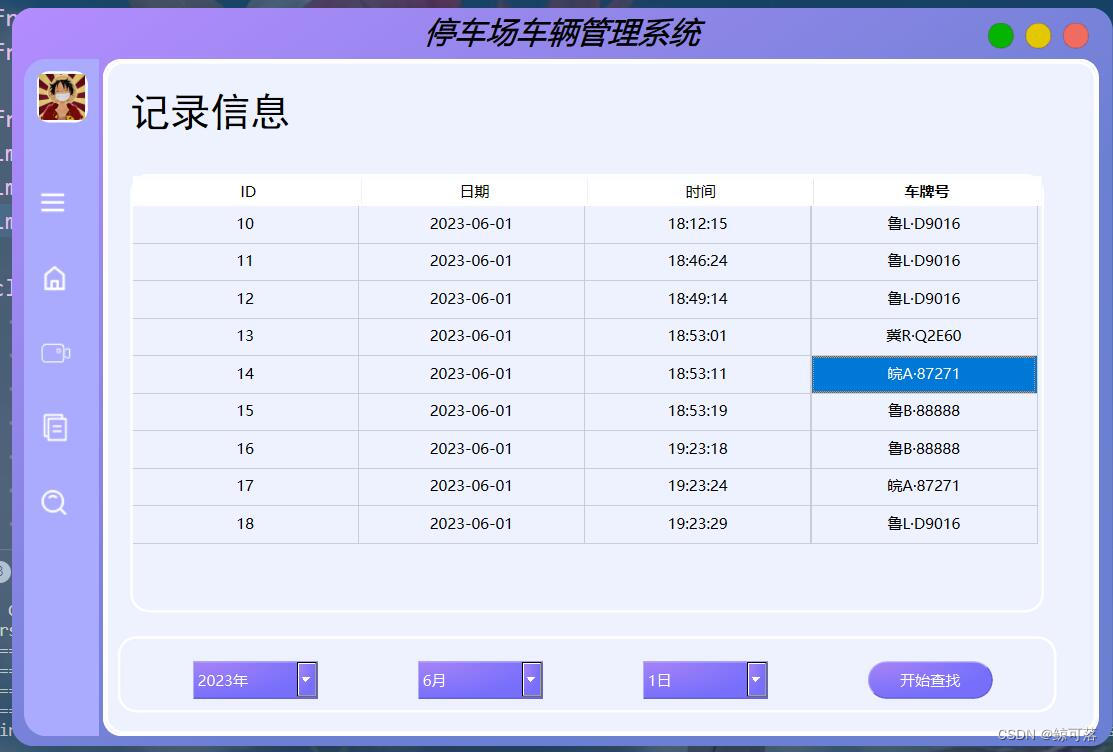
【源码】车牌检测+QT界面+附带数据库
目录 1、基本介绍2、基本环境3、核心代码3.1、车牌识别3.2、车牌定位3.3、车牌坐标矫正 4、界面展示4.1、主界面4.2、车牌检测4.3、查询功能 5、演示6、链接 1、基本介绍 本项目采用tensorflow,opencv,pyside6和pymql编写,pyside6用来编写UI界…...
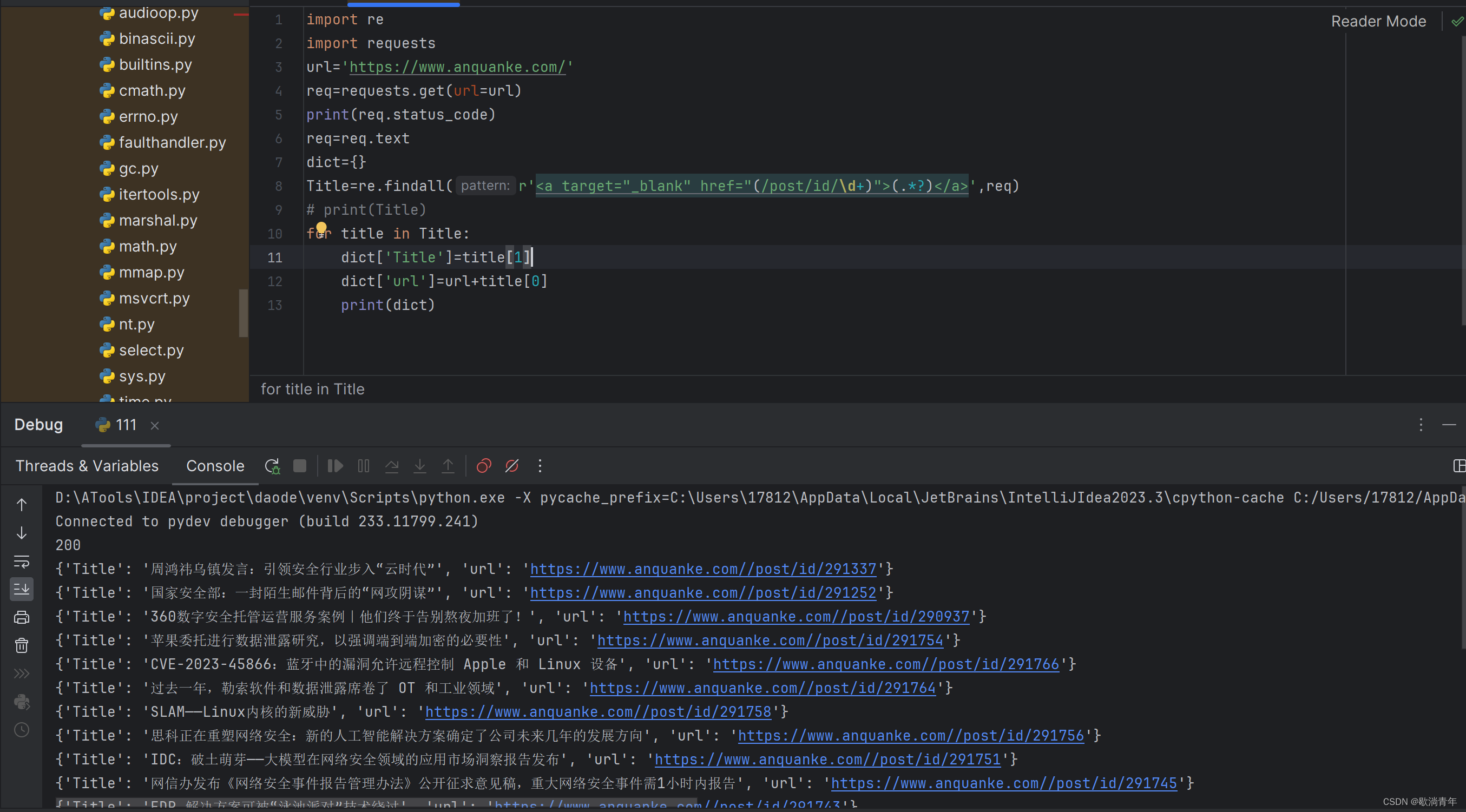
实战1-python爬取安全客新闻
一般步骤:确定网站--搭建关系--发送请求--接受响应--筛选数据--保存本地 1.拿到网站首先要查看我们要爬取的目录是否被允许 一般网站都会议/robots.txt目录,告诉你哪些地址可爬,哪些不可爬,以安全客为例子 2. 首先测试在不登录的…...

光栅化渲染:可见性问题和深度缓冲区算法
在前面第二章中,我们了解到,在投影点(屏幕空间中的点)的第三个坐标中,我们存储原始顶点 z 坐标(相机空间中点的 z 坐标): 当一个像素与多个三角形重叠时,查找三角形表面上…...
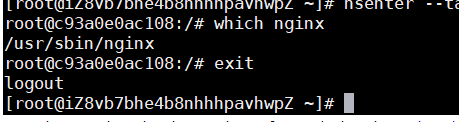
docker入门小结
docker是什么?它有什么优势? 快速获取开箱即用的程序 docker使得所有的应用传输就像我们日常通过聊天工具文件传输一样,发送方将程序传输到超级码头而接收方也只需通过超级码头进行获取即可,就像一只鲸鱼拖着货物来回运输一样。…...
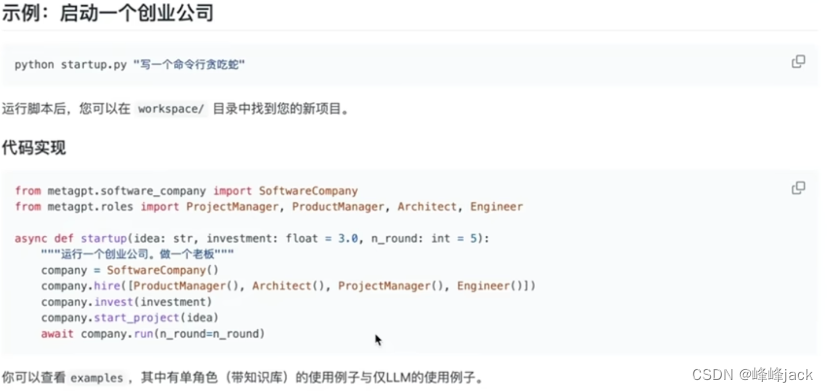
LLM Agent发展演进历史(观看metagpt视频笔记)
LLM相关的6篇重要的论文,其中4篇来自谷歌,2篇来自openai。技术路径演进大致是:SSL (Self-Supervised Learning) -> SFT (Supervised FineTune) IT (Instruction Tuning) -> RLHF。 word embedding的问题:新词如何处理&…...

Linux(操作系统)面经——part2
1、请你说说进程和线程的区别 1.进程是操作系统资源分配和调度的最小单位,实现操作系统内部的并发;线程是进程的子任务,cpu可以识别、执行的最小单位,实现程序内部的并发。 2.一个进程最少有一个线程或有多个,一个线程…...

Flink系列之:WITH clause
Flink系列之:WITH clause 适用流、批提供了一种编写辅助语句以在较大查询中使用的方法。这些语句通常称为公共表表达式 (CTE),可以被视为定义仅针对一个查询而存在的临时视图。 WITH 语句的语法为: WITH <with_item_definition> [ , …...
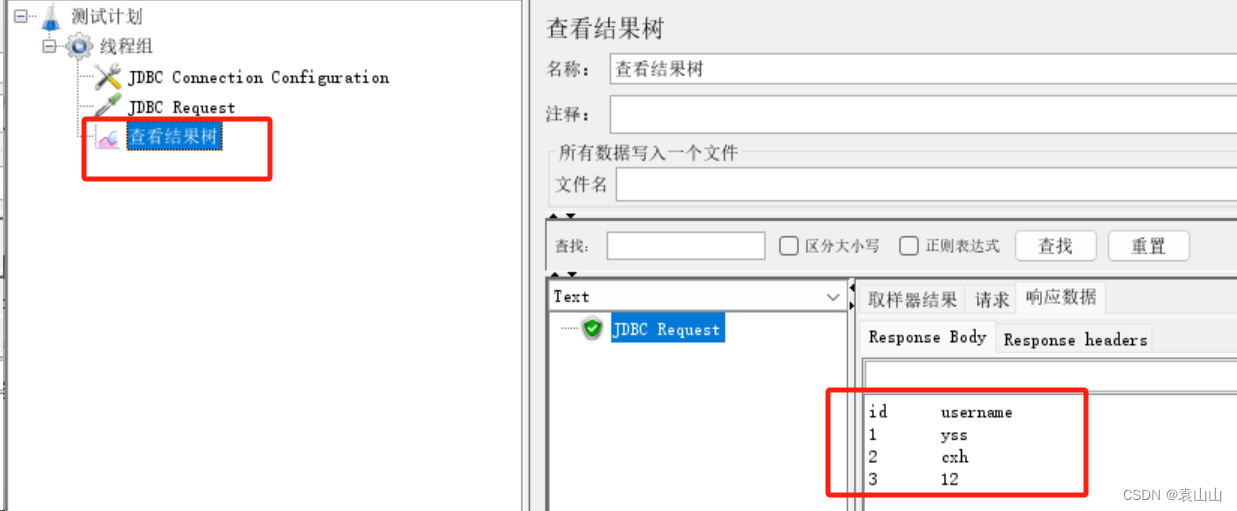
JMeter直连数据库
JMeter直连数据库 使用场景操作步骤 使用场景 用作请求的参数化 登录时需要的用户名,密码可以从数据库中查询获取 用作结果的断言 添加购物车下订单,检查接口返回的订单号,是否与数据库中生成的订单号一致 清理垃圾数据 添加商品后ÿ…...
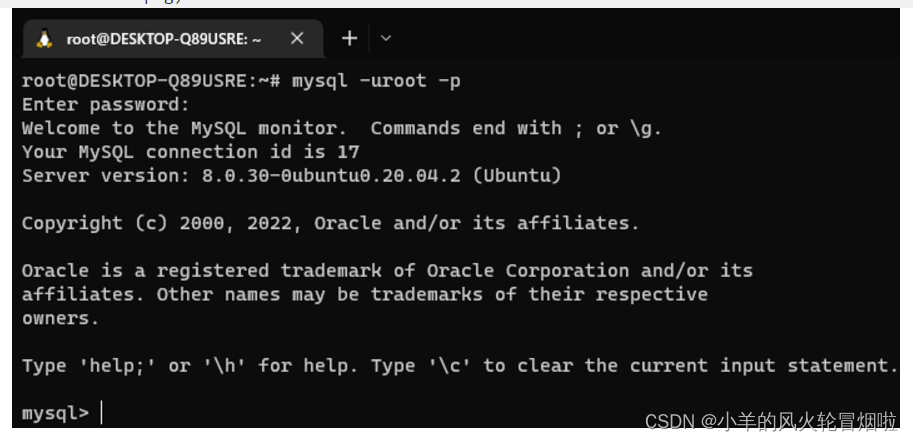
Linux部署MySQL5.7和8.0版本 | CentOS和Ubuntu系统详细步骤安装
一、MySQL数据库管理系统安装部署【简单】 简介 MySQL数据库管理系统(后续简称MySQL),是一款知名的数据库系统,其特点是:轻量、简单、功能丰富。 MySQL数据库可谓是软件行业的明星产品,无论是后端开发、…...

STL中set和multiset容器的用法(轻松易懂~)
目录 1. 基本概念 2. 构造和赋值 3. 大小和交换 4. 插入 和 删除 5. 统计 和 查找 6. set容器的排序 1. 基本概念 set和multiset属于关联式容器,底层结构式二叉树,所有元素都会在插入时自动排序。 如果你对容器的概念,或是二叉树不太了…...
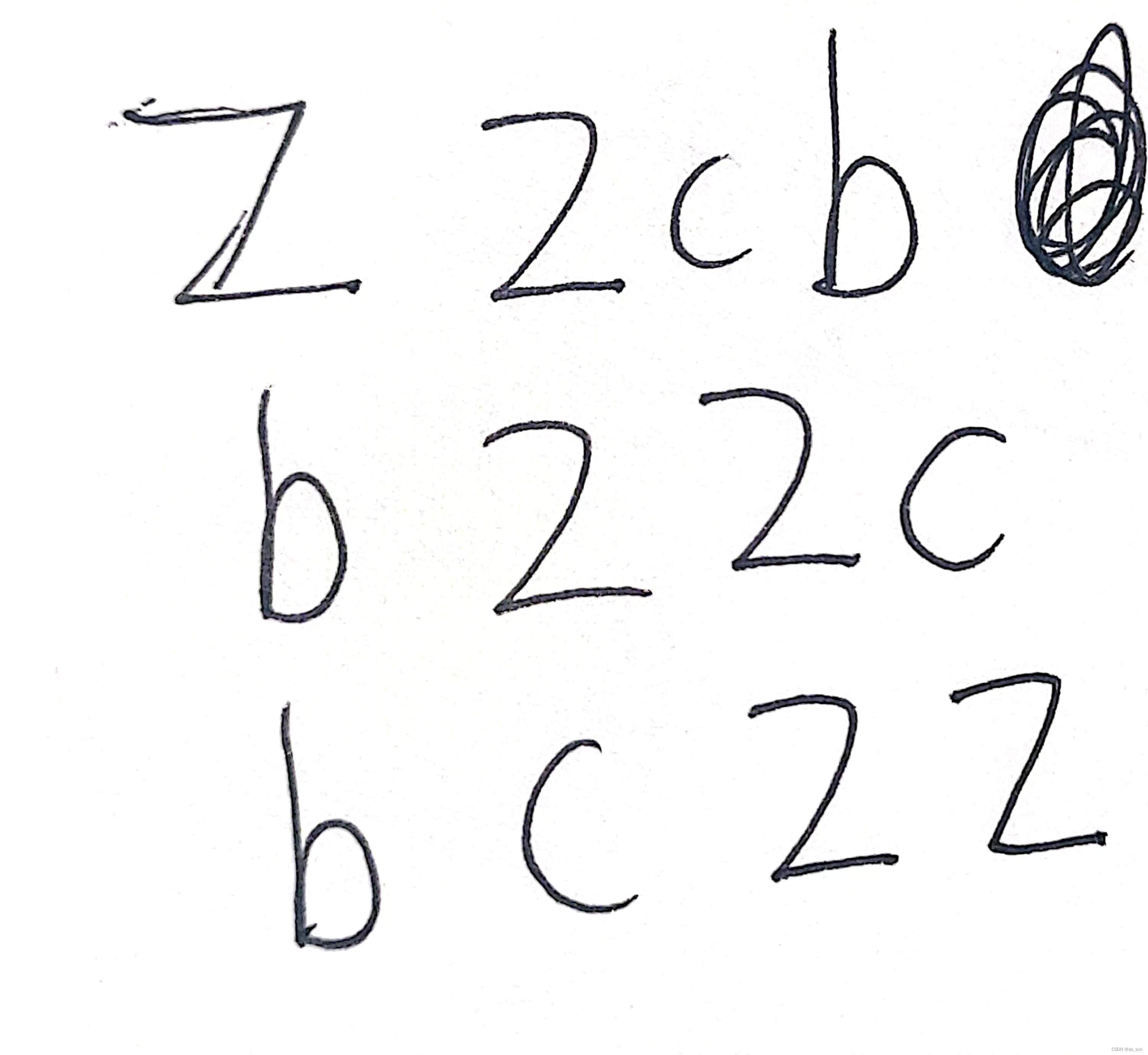
Codeforces Round 915 (Div. 2)
Constructive Problems(Problem - A - Codeforces) 题目大意:现在有一片城市被摧毁了,需要进行重建,当一个城市水平相邻和竖直相邻的位置都至少有一个城市的时候,该城市可以被重建。所有城市排成n行m列的矩…...

C语言经典错误总结(三)
一.指针与数组理解 我们都知道定义一个数组然后对其进行各种想要的操作,但是你真的能够区分那些是对数组的操作,那些是通过指针实现的吗? 例如;arr[1]10;这个是纯粹对数组操作实现的吗? 答案肯定不是,实际上我们定义…...
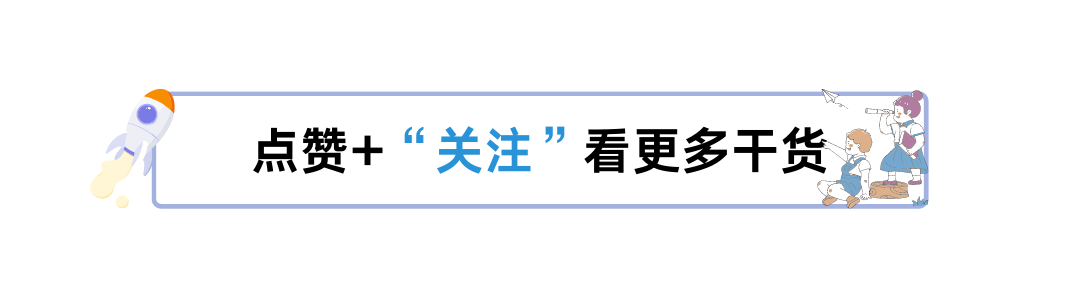
Ubuntu系统入门指南:基础操作和使用
Ubuntu系统的基础操作和使用 一、引言二、安装Ubuntu系统三、Ubuntu系统的基础操作3.1、界面介绍3.2、应用程序的安装和卸载3.3、文件管理3.4、系统设置 四、Ubuntu系统的日常使用4.1、使用软件中心4.2、浏览器的使用和网络连接设置4.3、邮件客户端的配置和使用4.4、文件备份和…...
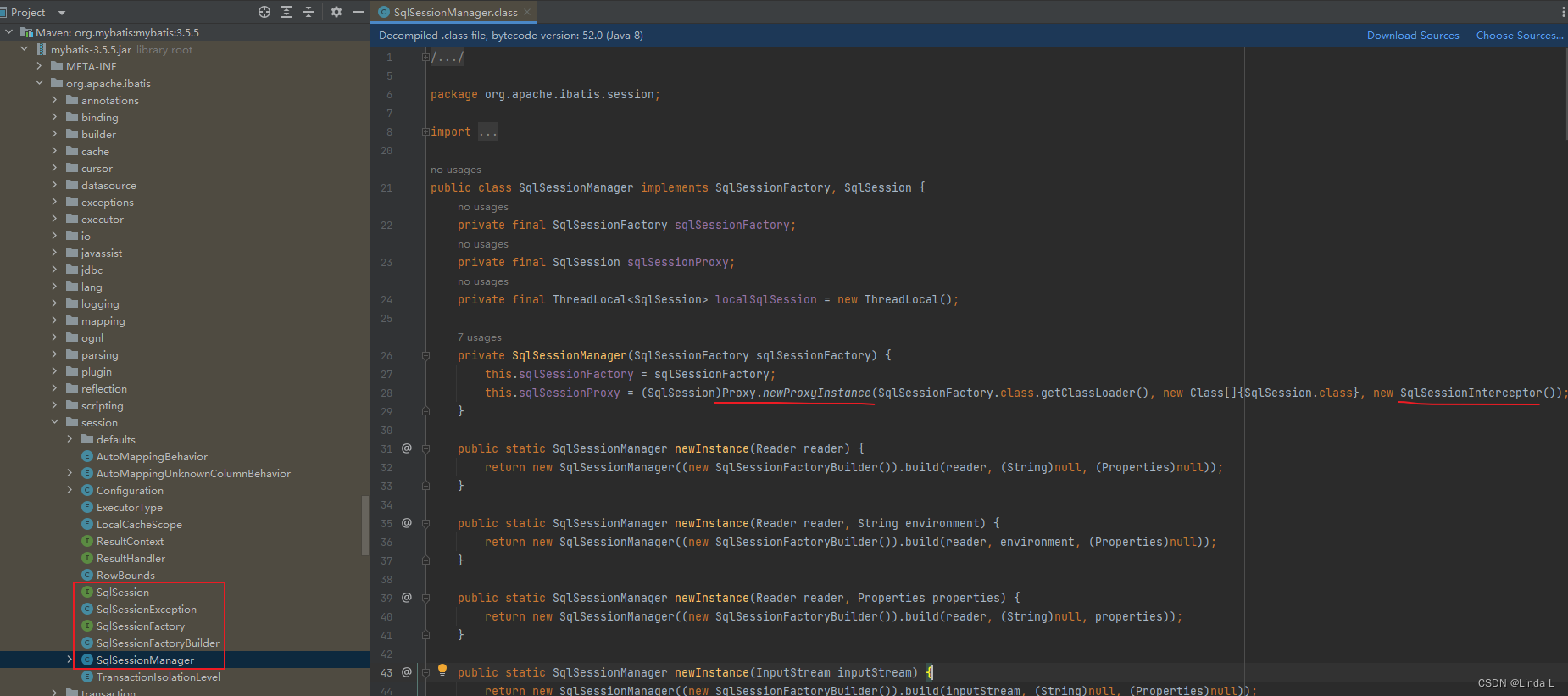
MyBatis原理解读
我们项目中多用MyBatis进行数据库的读写,开源的MyBatis-Plus框架对其进行了增强,使用上更加简单,我们之前的很多项目也是直接用的MyBatis-Plus。 数据库操作的时候,简单的单表读写,我们可以直接在方法里链式组装SQL,复杂的SQL或涉及多表联合join的,需要在xml手写SQL语句…...
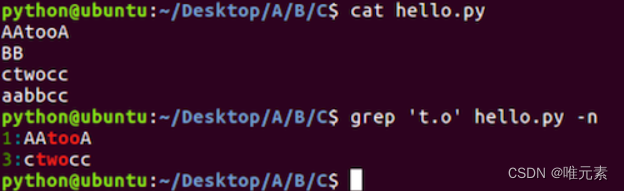
Linux---文本搜索命令
1. grep命令的使用 命令说明grep文本搜索 grep命令效果图: 2. grep命令选项的使用 命令选项说明-i忽略大小写-n显示匹配行号-v显示不包含匹配文本的所有行 -i命令选项效果图: -n命令选项效果图: -v命令选项效果图: 3. grep命令结合正则表达式的使用 正则表达式说明^以指…...

Unity中Shader语义的理解
前言 以下内容主要是个人理解,如有错误,欢迎严厉批评指正。 一、语义的形式在Shader中是必要的吗? 不是必要的。 使用HLSL和CG语言来编写Shader需要语义,使用GLSL编写Shader不需要。 二、语义的意义? 语义是什么&…...

Flink系列之:Top-N
Flink系列之:Top-N 一、TOP-N二、无排名输出优化 一、TOP-N 适用于流、批Top-N 查询可以根据指定列排序后获得前 N 个最小或最大值。最小值和最大值集都被认为是Top-N查询。在需要从批表或流表中仅显示 N 个底部或 N 个顶部记录时,Top-N 查询是非常有用…...
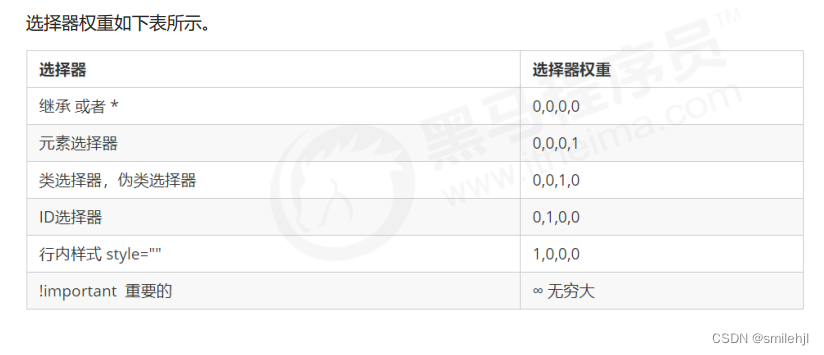
CSS的三大特性(层叠性、继承性、优先级---------很重要)
CSS 有三个非常重要的三个特性:层叠性、继承性、优先级。 层叠性 场景:相同选择器给设置相同的样式,此时一个样式就会覆盖(层叠)另一个冲突的样式。层叠性主要解决样式冲突 的问题 原则: 样式冲突&am…...
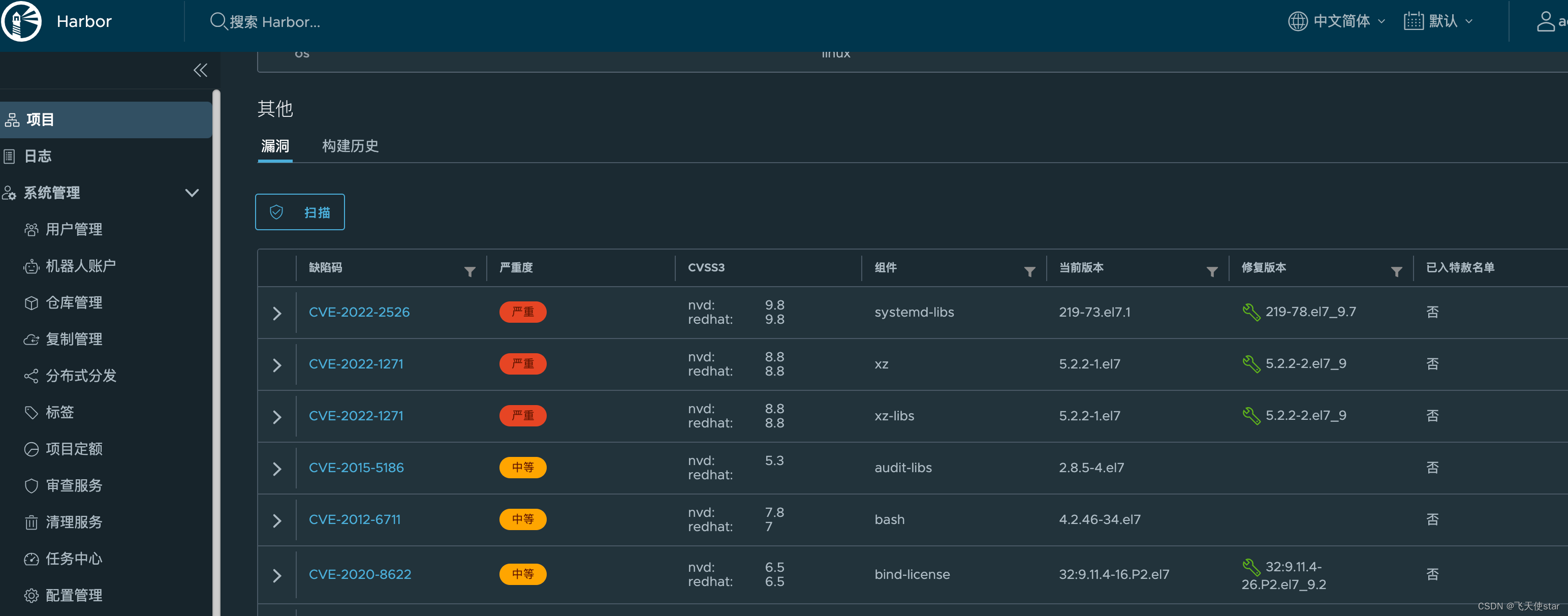
飞天使-docker知识点10-docker总结
文章目录 docker 知识点汇总docker chatgpt解释学习路线cmd和 ENTRYPOINT 的区别harbor安装漏洞扫描 docker 知识点汇总 docker 基础用法 docker 镜像基础用法 docker 容器网络 docker 存储卷 dockerfile docker仓库 harbor docker-compose docker chatgpt解释学习路线 学习…...