C++中string容器的字符串操作
目录
1.c_str() 返回C常量字符串
2.date() 返回C常量字符串
3.substr() 构造子串
4.find() 正向查找(查找失败返回npos)
5.rfind() 逆向查找(查找失败返回npos)
6.find_first_of() 正向查找匹配的字符
7.find_last_of() 逆向查找匹配的字符
8.find_first_not_of() 正向查找不匹配的字符
9.find_last_not_of() 逆向查找不匹配的字符
10.compare() 比较字符串
1.c_str() 返回C常量字符串
const char* c_str() const
string s("123");
const char* p = s.c_str();
cout << p << endl;//123
2.date() 返回C常量字符串
const char* data() const
string s("12345");
const char* p = s.data();
cout << p << endl;//12345
3.substr() 构造子串
string substr(size_t pos = 0, size_t len = npos) const
返回pos位置开始的len个字符组成的字符串
string s1 = "12345";
string s2 = s1.substr(2, 3);
cout << s2 << endl;//345
4.find() 正向查找(查找失败返回npos)
string (1) | size_t find (const string& str, size_t pos = 0) const; |
---|---|
c-string (2) | size_t find (const char* s, size_t pos = 0) const; |
buffer (3) | size_t find (const char* s, size_t pos, size_t n) const; |
character (4) | size_t find (char c, size_t pos = 0) const; |
1.size_t find(const string& str, size_t pos = 0) const
从pos位置开始查找匹配的对象str,返回查找到的位置
2.size_t find(const char* s, size_t pos = 0) const
从pos位置开始查找匹配的字符串s,返回查找到的位置
3.size_t find(const char* s, size_t pos, size_t n)
从pos位置查找匹配字符串s的前n个字符,返回查找到的位置
4.size_t find(char c, size_t pos = 0)
从pos位置开始查找字符c,返回查找到的位置
string s1("There are two needles in this haystack with needles.");
string s2("needle");
size_t found = s1.find(s2, 0);
if (found != string::npos)
{cout << "first 'needle' found at " << found << endl;//first 'needle' found at 14
}
else
{cout << "'needle' no found" << endl;
}found = s1.find("needle are small", found + 1, 6);
if (found != string::npos)
{cout << "second 'needle' found at " << found << endl;//second 'needle' found at 44
}
else
{cout << "'needle' no found" << endl;
}found = s1.find("haystack", 0);
if (found != string::npos)
{cout << "'haystack' found at " << found << endl;//'haystack' found at 30
}
else
{cout << "'haystack' no found" << endl;
}found = s1.find('.', 0);
if (found != string::npos)
{cout << "'.' found at " << found << endl;//'.' found at 30
}
else
{cout << "'.' no found" << endl;
}
5.rfind() 逆向查找(查找失败返回npos)
string (1) | size_t rfind (const string& str, size_t pos = npos) const; |
---|---|
c-string (2) | size_t rfind (const char* s, size_t pos = npos) const; |
buffer (3) | size_t rfind (const char* s, size_t pos, size_t n) const; |
character (4) | size_t rfind (char c, size_t pos = npos) const; |
1.size_t rfind(const string& str, size_t pos =npos) const
从pos位置逆向开始查找匹配的对象str,返回查找到的位置
2.size_t rfind(const char* s, size_t pos =npos) const
从pos位置逆向开始查找匹配的字符串s,返回查找到的位置
3.size_t rfind(const char* s, size_t pos, size_t n) const
从pos位置逆向查找匹配字符串s的前n个字符,返回查找到的位置
4.size_t rfind(char c, size_t pos =npos) const
从pos位置逆向开始查找字符c,返回查找到的位置
string str("The sixth sick sheik's sixth sheep's sick.");
string key("sixth");
size_t found = str.rfind(key);
if (found != string::npos)cout << "'sixth' found at " << found << endl;//'sixth' found at 23
6.find_first_of() 正向查找匹配的字符
正向查找首个与指定字符串中任一字符匹配的字符,查找失败返回npos
string (1) | size_t find_first_of (const string& str, size_t pos = 0) const; |
---|---|
c-string (2) | size_t find_first_of (const char* s, size_t pos = 0) const; |
buffer (3) | size_t find_first_of (const char* s, size_t pos, size_t n) const; |
character (4) | size_t find_first_of (char c, size_t pos = 0) const; |
1.size_t find_first_of(const string& str, size_t pos = 0) const
从pos位置开始查找对象str中首次出现的任一字符,查找失败返回npos
2.size_t find_first_of(const char* s, size_t pos = 0) const
从pos位置开始查找字符串s中出现的任一字符,查找失败返回npos
3.size_t find_first_of(const char* s, size_t pos, size_t n) const
从pos位置开始查找字符串s的前n个字符串中出现的任一字符,查找失败返回npos
4.size_t find_first_of(char c, size_t pos =0 ) const
从pos位置开始查找首次出现的字符c,查找失败返回npos
string s("Please, replace the vowels in this sentence by asterisks.");
size_t found = s.find_first_of("aoeiu", 0);
while (found != string::npos)
{s[found] = '*';found = s.find_first_of("aoeiu", found + 1);
}
cout << s << endl;//Pl**s*, r*pl*c* th* v*w*ls *n th*s s*nt*nc* by *st*r*sks.
7.find_last_of() 逆向查找匹配的字符
逆向查找首个与指定字符串中任一字符匹配的字符,查找失败返回npos
string (1) | size_t find_last_of (const string& str, size_t pos = npos) const; |
---|---|
c-string (2) | size_t find_last_of (const char* s, size_t pos = npos) const; |
buffer (3) | size_t find_last_of (const char* s, size_t pos, size_t n) const; |
character (4) | size_t find_last_of (char c, size_t pos = npos) const; |
1.size_t find_last_of(const string& str, size_t pos = npos) const
从pos位置开始逆向查找首个与对象str中任一字符匹配的字符,查找失败返回npos
2.size_t find_last_of(const char* s, size_t pos = npos) const
ni从pos位置开始逆向查找首个与字符串s中任意字符匹配的字符,查找失败返回npos
3.size_t find_last_of(const char* s,size_t pos, size_t n) const
从pos位置开始逆向查找首个与字符串s的前n个字符中任意字符匹配的字符,查找失败返回npos
4.size_t find_last_of(char c, size_t pos =npos) const
ni从pos位置开始逆向查找首个与字符c匹配的字符,查找失败返回npos
void SplitFilename(const string& str)
{size_t found = str.find_last_of("/\\");cout << "path :" << str.substr(0, found) << endl;cout << "file :" << str.substr(found + 1) << endl;
}
void string_test()
{string s1("/usr/bin/man");string s2("c:\\windows\\winhelp.exe");SplitFilename(s1);SplitFilename(s2);}
int main()
{string_test();//path: / usr / bin//file : man//path : c:\windows//file : winhelp.exereturn 0;
}
8.find_first_not_of() 正向查找不匹配的字符
正向查找首个与指定字符串中任一字符不匹配的字符,查找失败返回npos
string (1) | size_t find_first_not_of (const string& str, size_t pos = 0) const; |
---|---|
c-string (2) | size_t find_first_not_of (const char* s, size_t pos = 0) const; |
buffer (3) | size_t find_first_not_of (const char* s, size_t pos, size_t n) const; |
character (4) | size_t find_first_not_of (char c, size_t pos = 0) const; |
1.size_t find_first_not_of(const string& str, size_t pos = 0) const
从pos位置开始正向查找首个与对象str中字符不匹配的字符,查找失败返回npos
2.size_t find_first_not_of(const char* s, size_t pos = 0) const
从pos位置开始正向查找首个与字符串s中字符不匹配的字符,查找失败返回npos
3.size_t find_first_not_of(const char* s, size_t pos, size_t n) const
从pos位置开始正向查找首个与字符串s前n个字符中字符不匹配的字符,查找失败返回npos
4.size_t find_first_not_of(char c, size_t pos = 0) const
从pos位置开始正向查找首个与字符c不匹配的字符,查找失败返回npos
string str("look for non-alphabetic characters...");
size_t found = str.find_first_not_of("abcdefghijklmnopqrstuvwxyz ");
if (found != string::npos)
{cout << "The first non-alphabetic character is " << str[found] << " at position " << found << endl;//The first non-alphabetic character is - at position 12
}
9.find_last_not_of() 逆向查找不匹配的字符
逆向查找首个与指定字符串中任一字符不匹配的字符,查找失败返回npos
string (1) | size_t find_last_not_of (const string& str, size_t pos = npos) const; |
---|---|
c-string (2) | size_t find_last_not_of (const char* s, size_t pos = npos) const; |
buffer (3) | size_t find_last_not_of (const char* s, size_t pos, size_t n) const; |
character (4) | size_t find_last_not_of (char c, size_t pos = npos) const; |
1.size_t find_last_not_of(const string& str, size_t pos = npos) const
从pos位置开始逆向查找首个与对象str中字符不匹配的字符,查找失败返回npos
2.size_t find_last_not_of(const char* s, size_t pos = npos) const
从pos位置开始逆向查找首个与字符串s中字符不匹配的字符,查找失败返回npos
3.size_t find_last_not_of(const char* s, size_t pos, size_t n) const
从pos位置开始逆向查找首个与字符串s前n个字符中字符不匹配的字符,查找失败返回npos
4.size_t find_last_not_of(char c, size_t pos = npos) const
从pos位置开始逆向查找首个与字符c不匹配的字符,查找失败返回npos
string str("Please, erase trailing white-spaces \n");
string whitespaces(" \t\f\v\n\r");
size_t found = str.find_last_not_of(whitespaces);
if (found != string::npos)str.erase(found + 1); //[Please, erase trailing white-spaces]
elsestr.clear();
cout << "[" << str << "]" << endl;
10.compare() 比较字符串
比较两个字符串的ASCII码值,字符串1大于字符串2返回大于0的数;字符串1等于字符串2返回0;字符串1小于字符串2返回小于0的数
string (1) | int compare (const string& str) const; |
---|---|
substrings (2) | int compare (size_t pos, size_t len, const string& str) const; int compare (size_t pos, size_t len, const string& str,size_t subpos, size_t sublen) const; |
c-string (3) | int compare (const char* s) const; int compare (size_t pos, size_t len, const char* s) const; |
buffer (4) | int compare (size_t pos, size_t len, const char* s, size_t n) const; |
1.int compare(const string& str) const
比较调用对象和str对象的大小
2.int compare(size_t pos, size_t len, const string& str) const
比较调用对象pos位置开始的len个字符与对象str的大小
int compare(size_t pos, size_t len, const string& str, size_t subpos, size_t sublen) const
比较调用对象pos位置开始的len个字符与对象str中subpos位置开始的sublen个字符的大小
3.int compare(const char* s) const
比较调用对象和字符串s的大小
int compare(size_t pos, size_t len, const char* s) const
比较调用对象从pos位置开始的len个字符与字符串s的大小
4.int compare(size_t pos, size_t len, const char* s, size_t n) const
比较调用对象从pos位置开始的len个字符与字符串s前n个字符的大小
//1.
string s1("abcdefg");
string s2("abcdfg");
if (s1.compare(s2) == 0)cout << s1 << " = " << s2 << endl;
else if (s1.compare(s2) > 0)cout << s1 << " > " << s2 << endl;
elsecout << s1 << " < " << s2 << endl; //abcdefg < abcdfg//2.1
string s1("abcdefg");
string s2("abcdfg");
if (s1.compare(1, 6, s2) == 0)cout << s1 << " = " << s2 << endl;
else if (s1.compare(1, 6, s2) > 0)cout << s1 << " > " << s2 << endl;//abcdefg > abcdfg
elsecout << s1 << " < " << s2 << endl; //2.2
string s1("abcdefg");
string s2("abcdfg");
if (s1.compare(1, 6, s2, 1, 5) == 0)cout << s1 << " = " << s2 << endl;
else if (s1.compare(1, 6, s2, 1, 5) > 0)cout << s1 << " > " << s2 << endl;
elsecout << s1 << " < " << s2 << endl;//abcdefg < abcdfg//3.1
string s("abcdefg");
char p[] = "abcdfg";
if (s.compare(p) == 0)cout << s << " = " << p << endl;
else if (s.compare(p) > 0)cout << s << " > " << p << endl;
elsecout << s << " < " << p << endl;//abcdefg < abcdfg//3.2
string s("abcdefg");
char p[] = "abcdfg";
if (s.compare(1, 5, p) == 0)cout << s << " = " << p << endl;
else if (s.compare(1, 5, p) > 0)cout << s << " > " << p << endl;//abcdefg > abcdfg
elsecout << s << " < " << p << endl;//4
string s("abcdefg");
char p[] = "abcdfg";
if (s.compare(1, 5, p, 5) == 0)cout << s << " = " << p << endl;
else if (s.compare(1, 5, p, 5) > 0)cout << s << " > " << p << endl;//abcdefg > abcdfg
elsecout << s << " < " << p << endl;
相关文章:

C++中string容器的字符串操作
目录 1.c_str() 返回C常量字符串 2.date() 返回C常量字符串 3.substr() 构造子串 4.find() 正向查找(查找失败返回npos) 5.rfind() 逆向查找(查找失败返回npos) 6.find_first_of() 正向查找匹配的字符 7.find_last_of() 逆向…...
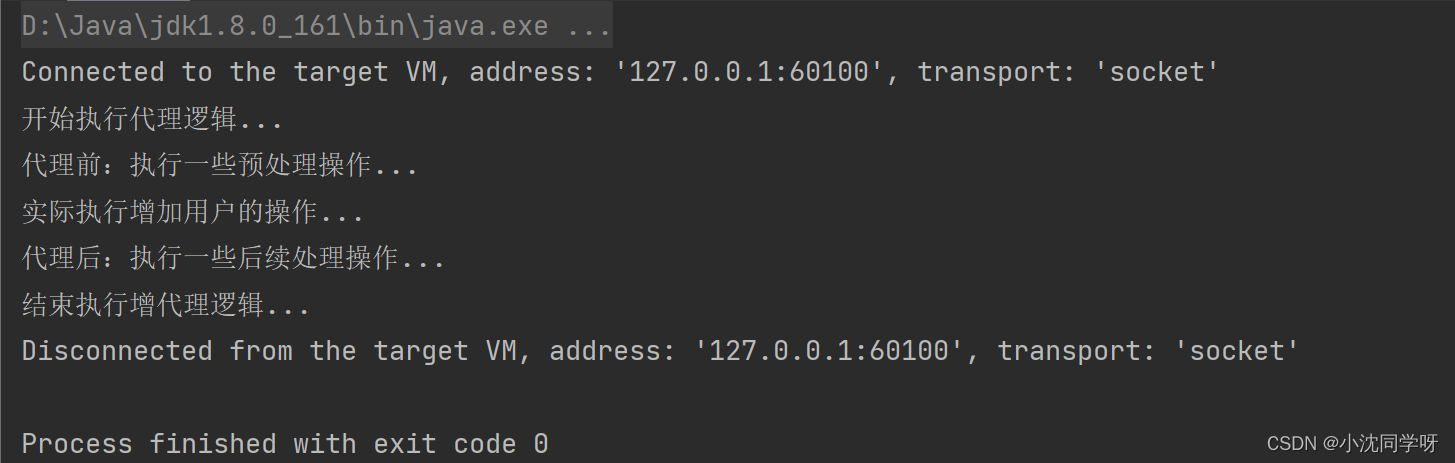
Java编程使用CGLIB动态代理介绍与实战演示
文章目录 前言技术积累核心概念主要功能适用场景与JDK动态代理的对比 实战演示定义待代理的目标类实现MethodInterceptor接口使用代理对象 测试结果写在最后 前言 在Java编程中,CGLIB (Code Generation Library) 是一个强大的高性能代码生成库,它通过生…...
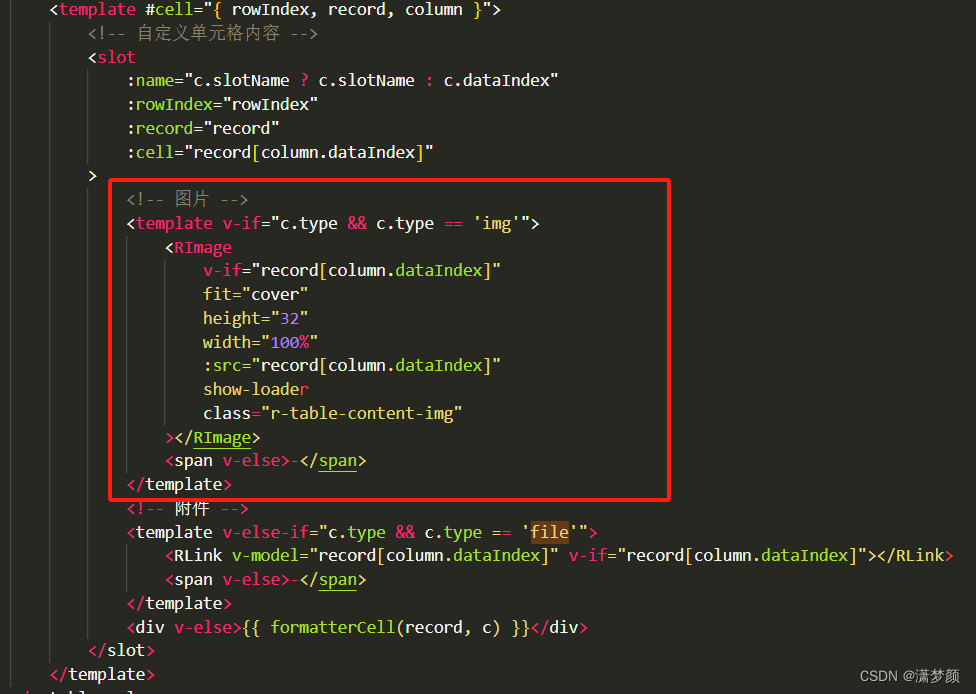
vue3 渲染一个后端返回的图片字段渲染、table表格内放置图片
一、后端直接返回图片url 当图片字段接口直接返回的是图片url,可以直接放到img标签上 <img v-if"thumbLoader" class"r-image-loader-thumb" :src"resUrl" /> 二、当图片字段接口直接返回的是图片Id 那么就需要去拼一下图片…...
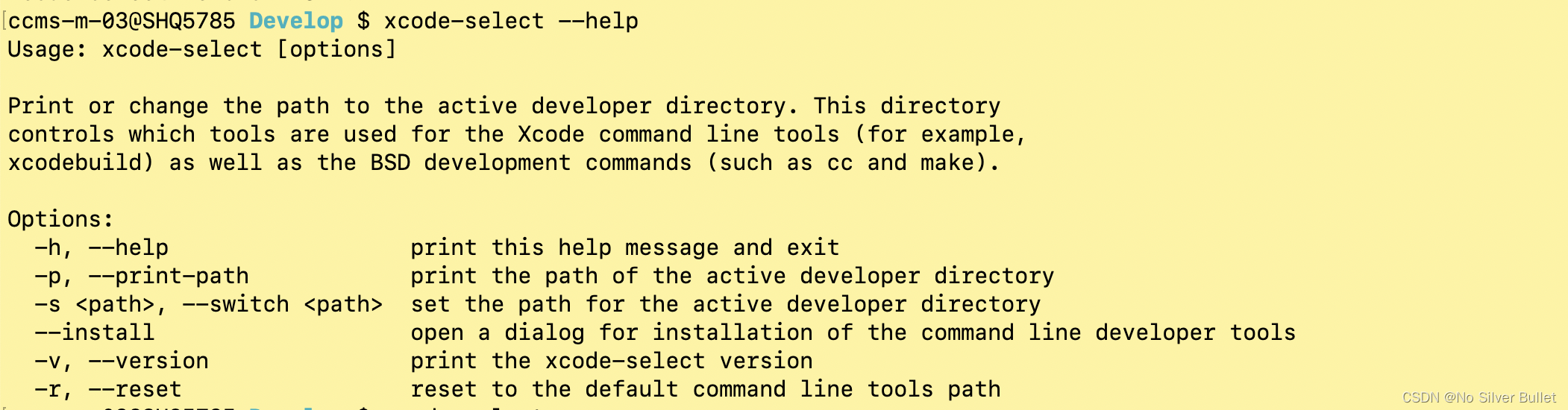
iOS开发进阶(十三):脚手架创建iOS项目
文章目录 一、前言二、xcode-select 命令三、拓展阅读 一、前言 项目初期,需要搭建项目基本框架,为此离不开辅助工具,即脚手架。当然,IDE也可以实现新建空白项目,但是其新建后的项目结构可能不符合预期设计࿰…...
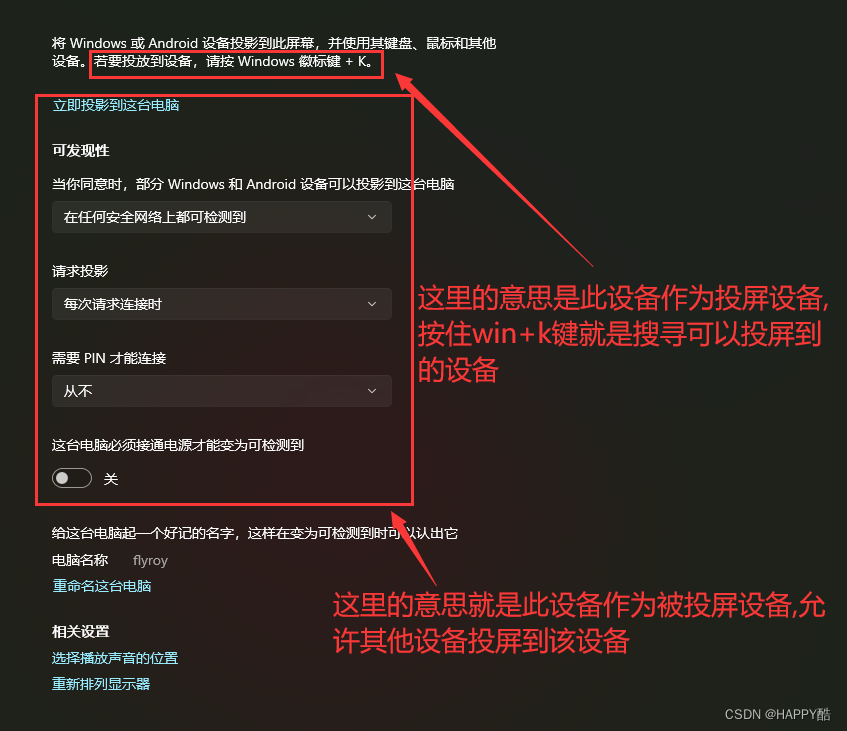
手机无线投屏到windows11电脑
1 安装无线投影组件 2 电脑端打开允许其他设备投影的开关 3 手机找到投屏选项 4 手机搜索可用设备连接即可 这里的官方文档给的不太好,给了一些让人眼花撩乱的信息,以下是经过整合的有效信息...
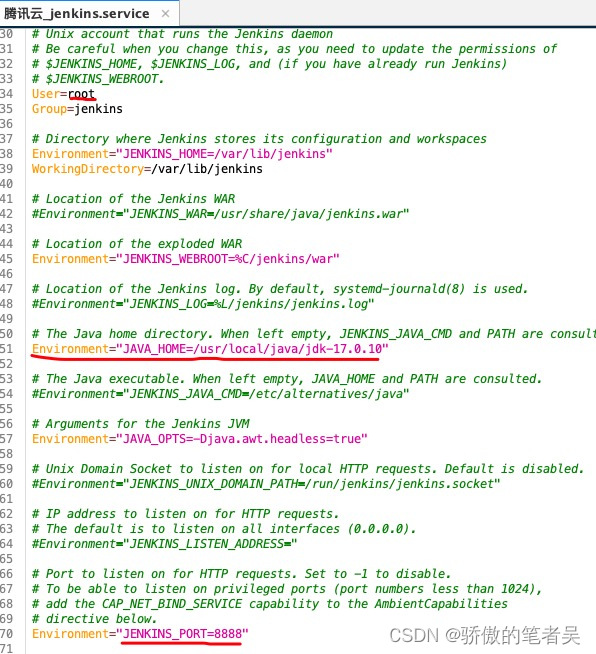
linux 环境安装配置
安装java17 1.下载安装包 wget https://download.oracle.com/java/17/latest/jdk-17_linux-x64_bin.tar.gz 2.解压到自定义目录/usr/local/java mkdir /usr/local/java tar zxvf jdk-17_linux-x64_bin.tar.gz -C /usr/local/java 3.配置环境变量 echo export PATH$PATH:/…...
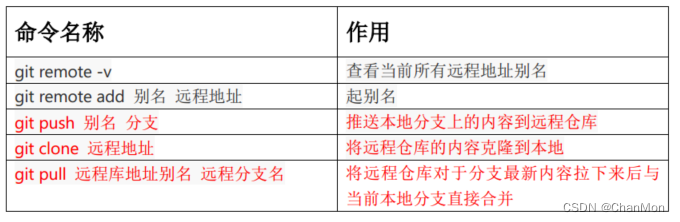
Git常用语句
设置用户名 git config --global user.name "用户名" git config --global user.email "邮箱"查看git用户信息 cat ~/.gitconfig初始化本地库 git initclone指定分支的代码 git clone -b my_branch gitgitlabxxxxxxxxxxxxxxxxxxxxxx.gitpush三件套 gi…...
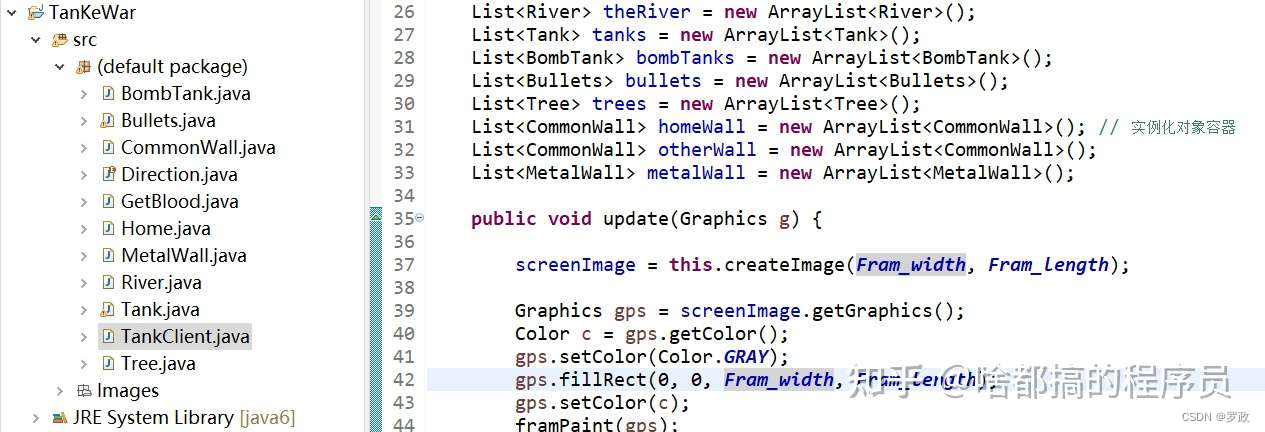
坦克大战_java源码_swing界面_带毕业论文
一. 演示视频 坦克大战_java源码_swing界面_带毕业论文 二. 实现步骤 完整项目获取 https://githubs.xyz/y22.html 部分截图 启动类是 TankClinet.java,内置碰撞检测算法,线程,安全集合,一切皆对象思想等,是java进阶…...
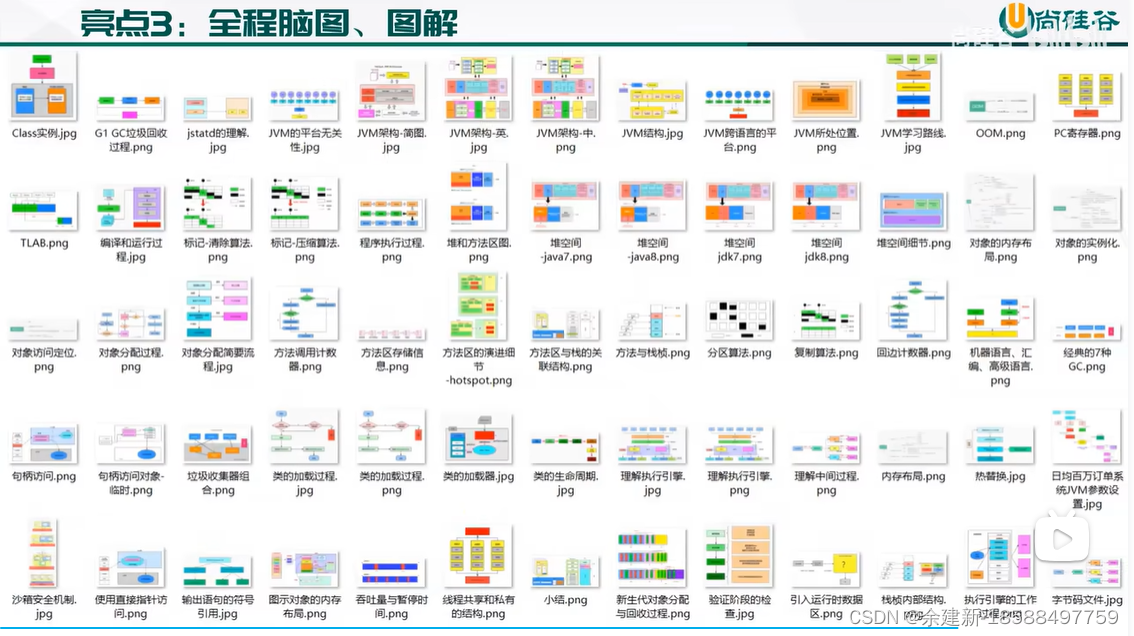
JVM 记录
记录 工具 https://gceasy.io 资料 尚硅谷宋红康JVM全套教程(详解java虚拟机) https://www.bilibili.com/video/BV1PJ411n7xZ?p361 全套课程分为《内存与垃圾回收篇》《字节码与类的加载篇》《性能监控与调优篇》三个篇章。 上篇《内存与垃圾回收篇…...

Linux学习笔记————C 语言版 LED 灯实验
这里写目录标题 一、实验程序编写二、 汇编部分实验程序编写三、C 语言部分实验程序编写四、编译下载验证 汇编 LED 灯实验中,我们讲解了如何使用汇编来编写 LED 灯驱动,实际工作中是很少用到汇编去写嵌入式驱动的,毕竟汇编太难,而…...
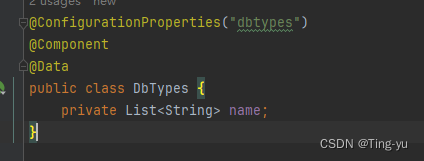
Spring Boot 配置文件
1. 配置文件的作用 配置文件主要是为了解决硬件编码带来的问题,把可能会发生改变的信息,放在一个集中的地方,当我们启动某个程序时,程序从配置文件中读取一些数据,并加载运行。 硬编码是将数据直接放在源代码中&…...

IPKISS ------ 查看器件默认端口名称
IPKISS ------ 查看器件默认端口名称 正文正文 我们这里以 Grating Coupler 举例。 import si_fab.all as pdk import ipkiss3.all as i3class MyGratingCoupler(i3.circuit):gc = i3.childcellProperty(<...

uni-app踩坑记录
uni-app踩坑记录 Failed to load local image resource xxx the server responded with a status of 500 (HTTP/1.1 500 Internal Server Error) Failed to load local image resource xxx the server responded with a status of 500 (HTTP/1.1 500 Internal Server Error) 文…...
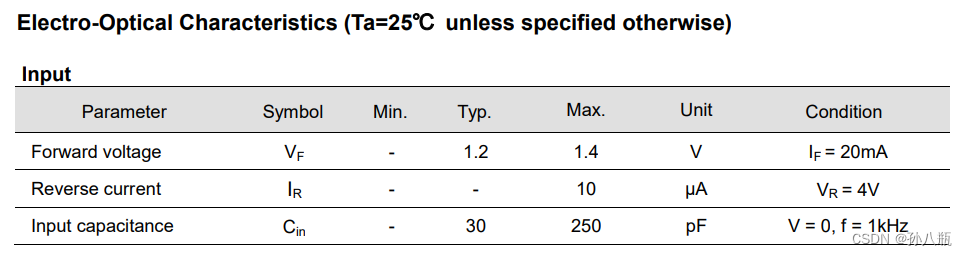
【嵌入式硬件】光耦
1.光耦作用 光耦一般用于信号的隔离。当两个电路的电源参考点不相关时,使用光耦可以保证在两边不共地的情况下,完成信号的传输。 2.光耦原理 光耦的原理图如下所示,其内部可以看做一个特殊的“三极管”; 一般的三极管是通过基极B和发射极E间的电流,去控制集电极C和发射极…...
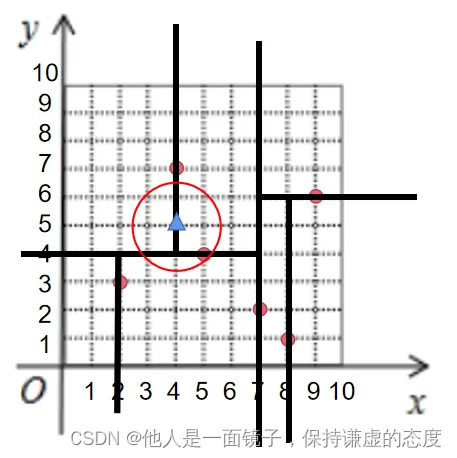
学习Fast-LIO系列代码中相关概念理解
目录 一、流形和流形空间(姿态) 1.1 定义 1.2 为什么要有流形? 1.3 流形要满足什么性质? (1) 拓扑同胚 (2) 可微结构 1.4 欧式空间和流形空间的区别和联系? (1) 区别: (2) 联系: 1.5 将姿态定义在流形上比…...

React 掌握及对比常用的8个Hooks,优化及使用场景
1、useState 在函数组件中,可以使用useState来定义函数组件的状态。使用useState来创建状态。 1.引入2.接收一个参数作为初始值3.返回一个数组,第一个值为状态,第二个值为改变状态的函数 2、 useEffect useEffect又称副作用hooks。作用&…...
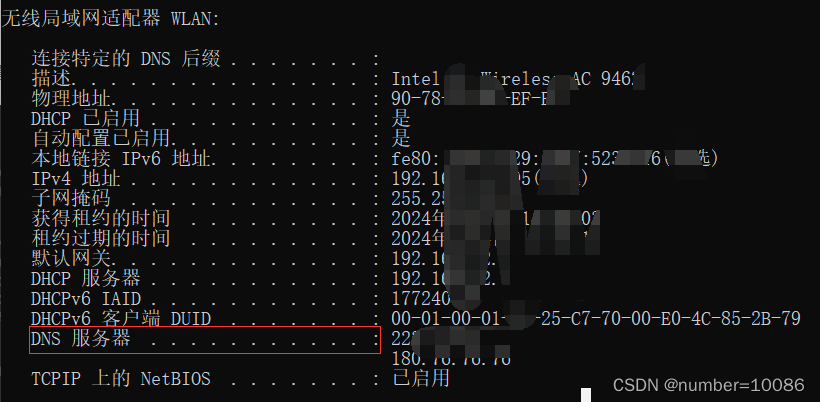
DNS域名解析过程
在互联网中我们通信目标是对方的IP,但是由于IP不便于记忆所以引入了域名 域名和IP是一一对应的关系,需要注意的是域名和网址是不同的概念 比如:www.csdn.net是域名,https://www.csdn.net/?spm1001.2101.3001.4476是网址 首先了解…...
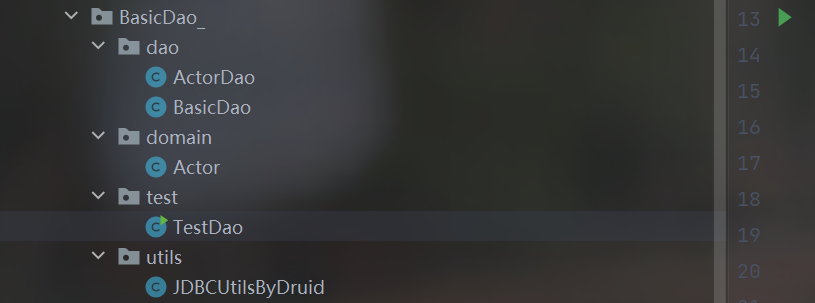
MySQL数据库(数据库连接池)
文章目录 1.批处理应用1.基本介绍2.批处理演示1.创建测试表2.修改url3.编写java代码 3.批处理源码分析 2.数据库连接池1.传统连接弊端分析2.数据库连接池基本介绍1.概念介绍2.数据库连接池示意图3.数据库连接池种类 3.C3P0连接池1.环境配置1.导入jar包2.将整个lib添加到项目中3…...
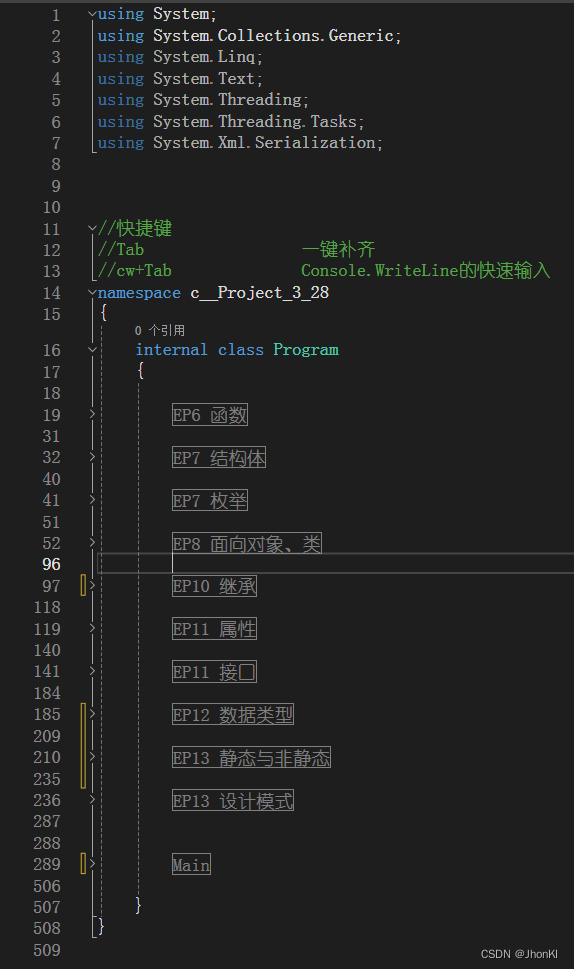
【C#】知识点速通
前言: 笔者是跟着哔站课程(Trigger)学习unity才去学习的C#,并且C语言功底尚存,所以只是简单地跟着课程将unity所用的C#语言的关键部分进行了了解,然后在后期unity学习过程中加以深度学习。如需完善的C#知识…...
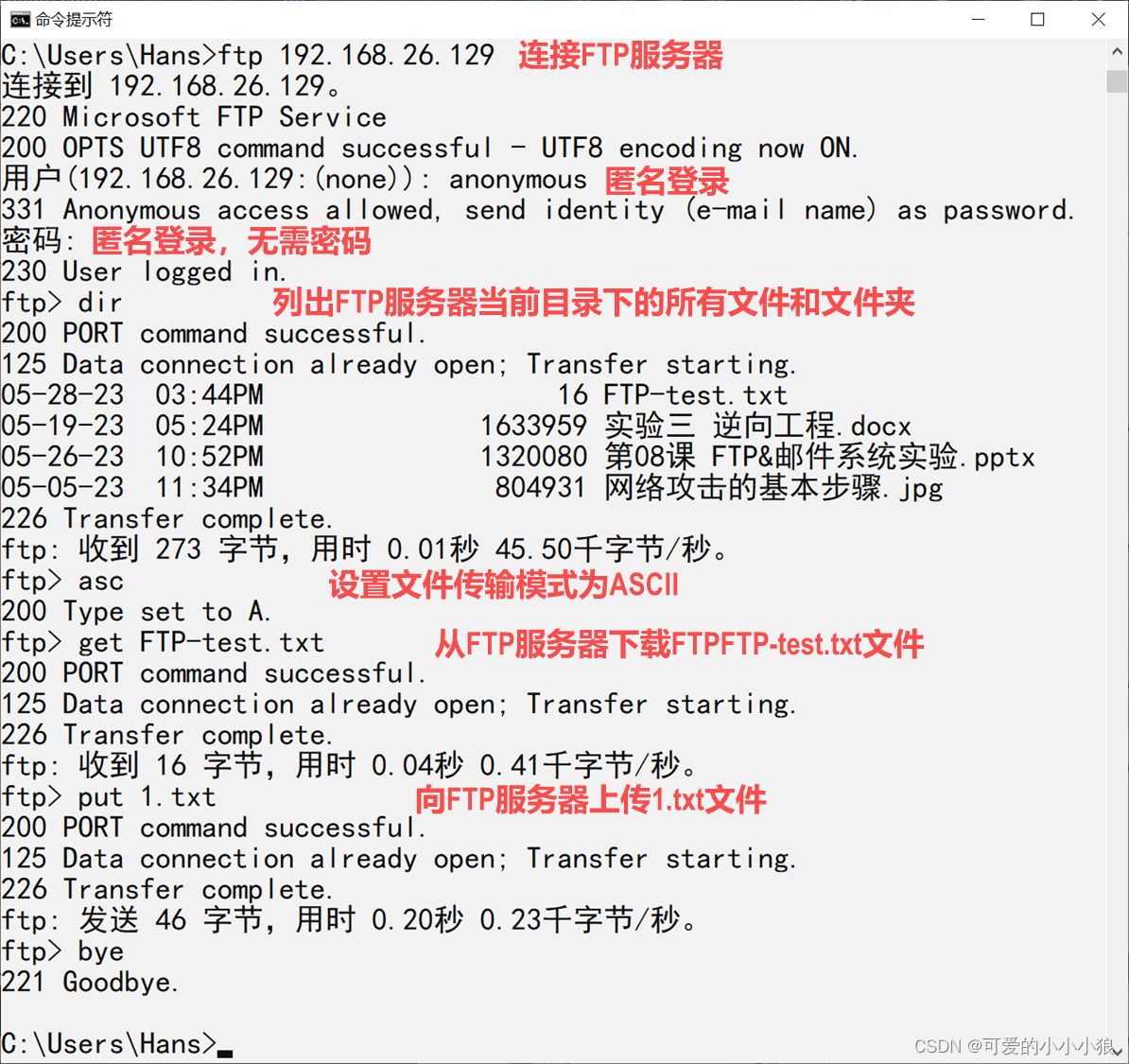
FTP协议
FTP协议 客户端向服务器发送文件。 C/S架构。 运行在TCP/IP协议上面。 FTP客户端要和FTP服务端建立两个TCP连接。 控制连接:运行在整个连接过程,传输控制信息。 数据连接:在每次文件传输时才会建立,文件传输完就关闭。 主动模式…...
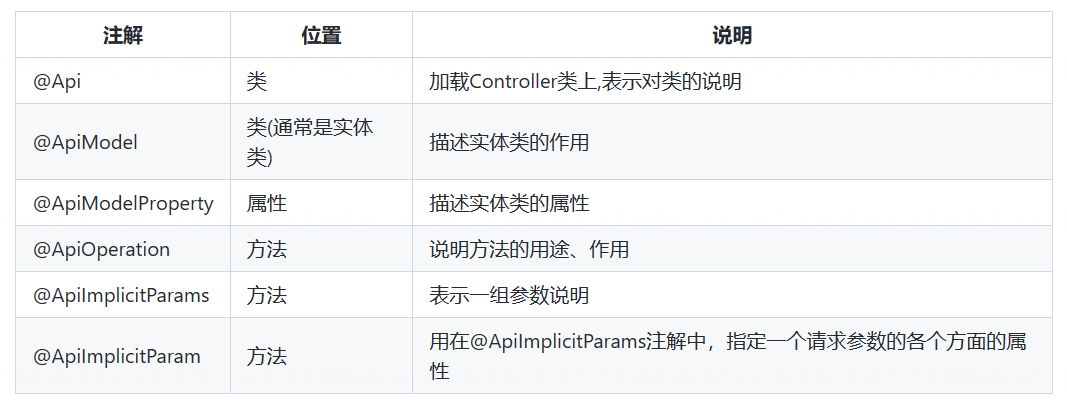
前后端分离开发【Yapi平台】【Swagger注解自动生成接口文档平台】
前后端分离开发 介绍开发流程Yapi(api接口文档编写平台)介绍 Swagger使用方式1). 导入knife4j的maven坐标2). 导入knife4j相关配置类3). 设置静态资源映射4). 在LoginCheckFilter中设置不需要处理的请求路径 查看接口文档常用注解注解介绍 当前项目中&am…...

Android的硬件接口HAL-2 HIDL
没写完哈。 不说废话,直接上干活。 1 创建HAL mkdir -p vendor/fanged/hidltest/1.0/defaultvi vendor/fanged/hidltest/1.0/Ilovefanged.hal package vendor.fanged.hardware.hidltest1.0;interface Ilovefanged {add(int32_t a, int32_t b) generates (int32_t…...

pygame--坦克大战(二)
加载敌方坦克 敌方坦克的方向是随机的,使用随机数生成。 初始化敌方坦克。 class EnemyTank(Tank):def __init__(self,left,top,speed):self.images = {U: pygame.image.load(img/enemy1U.gif),D: pygame.image.load(img/enemy1D.gif),L: pygame.image.load(img/enemy1L.gi…...

【C语言】标准输入/输出(printf, scanf, gets, puts, getchar, putchar)
标准文件文件指针设备标准输入stdin键盘标准输出stdout屏幕标准错误stderr您的屏幕 标准输入/输出的函数在标准库stdio.h。 #include <stdio.h> 1、printf 输出 printf :格式化输出,输出到标准输出stdout中。 printf: int print…...

C、C++、C#中.vscode下json文件记录
C launch.json {// 使用 IntelliSense 了解相关属性。 // 悬停以查看现有属性的描述。// 欲了解更多信息,请访问: https://go.microsoft.com/fwlink/?linkid830387"version": "0.2.0","configurations": [{"name": &quo…...

2013年认证杯SPSSPRO杯数学建模B题(第二阶段)流行音乐发展简史全过程文档及程序
2013年认证杯SPSSPRO杯数学建模 B题 流行音乐发展简史 原题再现: 随着互联网的发展,流行音乐的主要传播媒介从传统的电台和唱片逐渐过渡到网络下载和网络电台等。网络电台需要根据收听者的已知喜好,自动推荐并播放其它音乐。由于每个人喜好…...
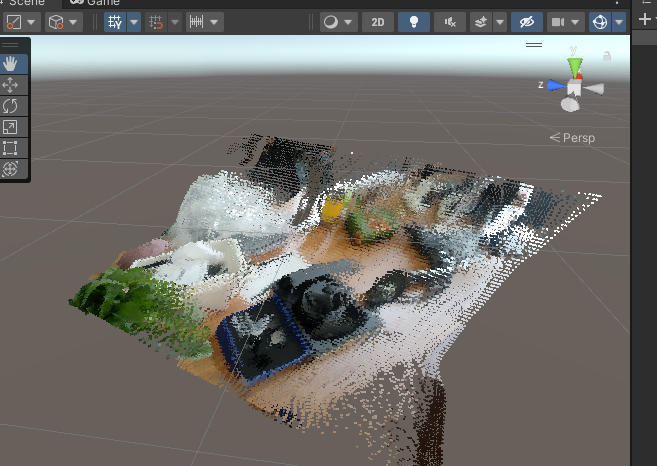
使用ARCore深度API实现点云采集
一、深度API 本小节内容摘自ARCore官方文档。 ARCore 深度API Depth API 可助力实现对象遮挡、提升沉浸感和新颖的互动体验,从而增强 AR 体验的真实感。 在下图中,右侧画面是采用深度API进行遮挡后的效果,与左侧图相比更加真实。 深度值 给…...
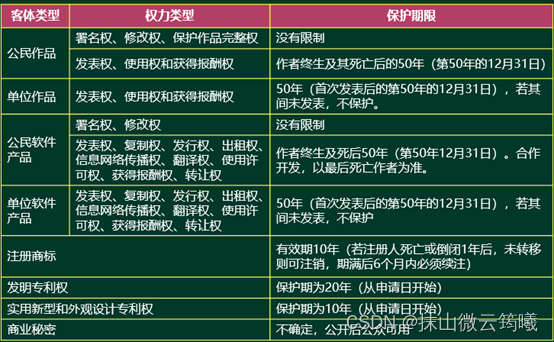
软考数据库
目录 分值分布1. 事务管理1.1 事物的基本概念1.2 数据库的并发控制1.2.1 事务调度概念1.2.2 并发操作带来的问题1.2.3 并发控制技术1.2.4 隔离级别: 1.3 数据库的备份和恢复1.3.1 故障种类1.3.2 备份方法1.3.3 日志文件1.3.4 恢复 2. SQL语言发权限收权限视图触发器…...
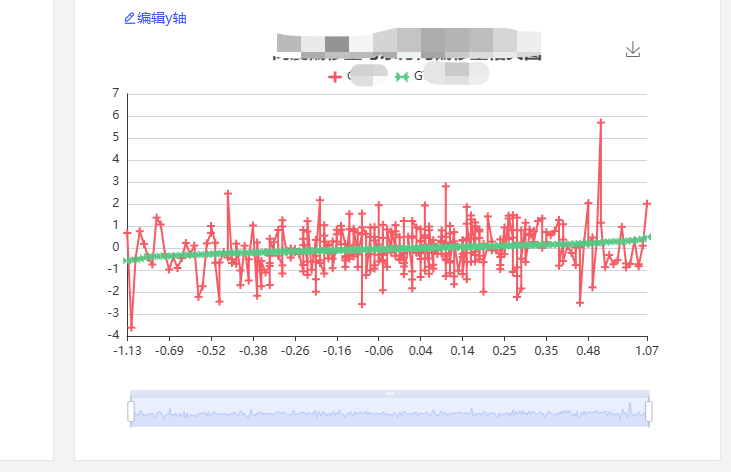
Echarts 自适应宽高,或指定宽高进行自适应
文章目录 需求分析 需求 有一个按钮实现对Echarts的指定缩放与拉长,形成自适应效果 拉长后效果图 该块元素缩短后效果图 分析 因为我习惯使用 ref 来获取组件的 DOM 元素,然后进行挂载 <div ref"echartsRef" id"myDiv" :sty…...

体验报告:为什么Claude-3是码农和学者的新宠?
在这个充斥着海量信息的新时代,人工智能的飞速发展带来了翻天覆地的变化。特别是在编程、学术探索以及专业文案创作等领域,AI的助力显得格外关键。最近,我有机会尝试了一种革命性的人工智能工具——Claude-3,其表现令我震惊&#…...