vue2 + antvx6 实现流程图功能
导入关键包
npm install @antv/x6 --save
npm install @antv/x6-vue-shape
保存插件 (可选)
npm install --save @antv/x6-plugin-clipboard @antv/x6-plugin-history @antv/x6-plugin-keyboard @antv/x6-plugin-selection @antv/x6-plugin-snapline @antv/x6-plugin-stencil @antv/x6-plugin-transform insert-css
写好的组件直接导入即可
<template><div><el-container><el-aside><div id="stencil"><div><div class="dnd-circle dnd-start" @mousedown="startDrag('start',$event)"></div><span>开始</span></div><div><div class="dnd-rect" @mousedown="startDrag('rect',$event)"></div><span>节点1</span></div><div><div class="dnd-polygon" @mousedown="startDrag('polygon',$event)"></div><span>节点2</span></div><div><div class="dnd-circle" @mousedown="startDrag('end',$event)"></div><span>结束</span></div></div></el-aside><el-main><div ref="graphContainer"></div></el-main></el-container><!-- todo drawer 抽屉实现节点内容编辑 --><el-drawertitle="节点属性编辑":visible.sync="drawer":direction="direction":before-close="handleClose"><el-form :data="editNode" :inline="true"><el-form-item label="节点名称" prop="label"><el-input v-model="editNode.label"></el-input></el-form-item><el-form-item label="节点形状" prop="shape"><el-select v-model="editNode.shape"><el-option v-for="(item,index) in shapeList" :key="item.value" :label="item.label":value="item.value"></el-option></el-select></el-form-item><el-button type="primary" @click="saveNode">保存</el-button></el-form></el-drawer></div>
</template><script>
import {Graph} from '@antv/x6'
import '@antv/x6-vue-shape'
// 插件 键盘监听事件
import {Keyboard} from '@antv/x6-plugin-keyboard'
// 拖拽事件
import {Dnd} from '@antv/x6-plugin-dnd'import {Stencil} from '@antv/x6-plugin-stencil'
import {Transform} from '@antv/x6-plugin-transform'
import {Selection} from '@antv/x6-plugin-selection'
import {Snapline} from '@antv/x6-plugin-snapline'
import {Clipboard} from '@antv/x6-plugin-clipboard'
import {History} from '@antv/x6-plugin-history'
import {register} from '@antv/x6-vue-shape'
import insertCss from 'insert-css'export default {name: 'MindMap',data () {return {graphOut: {},drawer: false,direction: 'rtl',currentNode: {},editNode: {},dnd: {},// 节点形状shapeList: [{label: '矩形',value: 'rect'}, {label: '圆形',value: 'circle'}, {label: '椭圆',value: 'ellipse'}, {label: '多边形',value: 'polygon'}, {label: '折线',value: 'polyline'}, {label: '路径',value: 'path'}, {label: '图片',value: 'image'},],// 连接桩ports: {groups: {top: {position: 'top',attrs: {circle: {magnet: true,stroke: 'black',r: 4,},},},bottom: {position: 'bottom',attrs: {circle: {magnet: true,stroke: 'black',r: 4,},},},left: {position: 'left',attrs: {circle: {magnet: true,stroke: 'black',r: 4,},},},right: {position: 'right',attrs: {circle: {magnet: true,stroke: 'black',r: 4,},},},},items: [{id: 'port_1',group: 'bottom',}, {id: 'port_2',group: 'top',}, {id: 'port_3',group: 'left',}, {id: 'port_4',group: 'right',}]}}},mounted () {this.graphOut = this.initGraph()},methods: {initGraph () {const graph = new Graph({container: this.$refs.graphContainer,// autoResize: true, // 大小自适应height: 400,width: '100%',grid: true,magnetThreshold: 'onleave',panning: {enabled: true,modifiers: 'shift',magnetThreshold: 1,// 鼠标画布移动eventTypes: ['leftMouseDown']},// 开启自动吸附connecting: {// 距离节点或者连接桩 50 px 触发自动吸附snap: true,// 是否允许连接到画布空白位置的点allowBlank: false,// 是否允许创建循环连线allowLoop: false,// 拖动边时,是否高亮显示所有可用连接桩或节点highlight: true,},modes: {default: ['drag-node']},background: {color: '#F2F7FA',},mousewheel: {// 是否开启滚轮缩放交互enabled: true,// 滚动缩放因子 默认 1.2factor: 1.2,// 是否将鼠标位置作为中心缩放、默认为truezoomAtMousePosition: true,// 按下什么键 才会缩放modifiers: ['ctrl', 'meta'],// 判断什么情况下 滚轮事件被处理// guard: false,},connector: {name: 'rounded',args: {radius: 8}}})// 支持拖拽this.dnd = new Dnd({target: graph,scaled: false,})Graph.registerNode('custom-node-width-port',{inherit: 'rect',width: 100,height: 40,attrs: {body: {stroke: '#8f8f8f',strokeWidth: 1,fill: '#fff',rx: 6,ry: 6,},},// 上下左右 四条边都有连接桩ports: this.ports},true,)Graph.registerNode('custom-circle-start',{inherit: 'circle',ports: this.ports},true,)Graph.registerNode('custom-polygon',{inherit: 'polygon',points: '0,10 10,0 20,10 10,20',ports: this.ports},true,)Graph.registerNode('custom-rect',{inherit: 'rect',ports: this.ports},true,)graph.addNode({x: 100,y: 40,width: 180,height: 30,label: '中心主题',shape: 'custom-node-width-port', // 节点形状attrs: {body: {fill: '#f5f5f5',stroke: '#333',},type: 'root'},tools: [{name: 'boundary',args: {attrs: {fill: '#16B8AA',stroke: '#2F80EB',strokeWidth: 1,fillOpacity: 0.1,},},}]})// 添加 plugin 插件graph.use(new Keyboard()) // 键盘事件.use(new Selection({enabled: true,multiple: true,rubberband: true,movable: true,showEdgeSelectionBox: true,showNodeSelectionBox: true,pointerEvents: 'none'})) // 绑定框选.use(new Snapline({enabled: true,sharp: true,})) // 对齐线.use(new Clipboard()).use(new History({enabled: true})) // 绑定撤销// 鼠标事件this.mouseEvent(graph)// 键盘时间this.keyboardEvent(graph)// 添加子节点的逻辑...return graph},addChildNode (nodeId, type) {console.log(nodeId, type)},handleClose (done) {this.$confirm('确认关闭?').then(_ => {done()}).catch(_ => {})},saveNode () {this.$confirm('确认保存?').then(_ => {console.log(this.editNode)this.currentNode['label'] = this.editNode['label']// this.currentNode['shape'] = this.editNode['shape']}).catch(_ => {})// 关闭当前 抽屉 el-drawerthis.drawer = false},startDrag (type, e) {this.startDragToGraph(this.graphOut, type, e)},startDragToGraph (graph, type, e) {const startNode = this.graphOut.createNode({shape: 'custom-circle-start',width: 38,height: 38,attrs: {body: {strokeWidth: 1,stroke: '#000000',fill: '#ffffff',rx: 10,ry: 10,},},})const polygonNode = this.graphOut.createNode({shape: 'custom-polygon',width: 80,height: 60,attrs: {body: {strokeWidth: 1,stroke: '#000000',fill: '#ffffff',rx: 10,ry: 10,},label: {fontSize: 13,fontWeight: 'bold',},},})const rectNode = this.graphOut.createNode({shape: 'custom-rect',width: 80,height: 60,attrs: {body: {strokeWidth: 1,stroke: '#000000',fill: '#ffffff',rx: 10,ry: 10,},label: {fontSize: 13,fontWeight: 'bold',},},})const endNode = this.graphOut.createNode({shape: 'custom-circle-start',width: 38,height: 38,key: 'end',attrs: {body: {strokeWidth: 4,stroke: '#000000',fill: '#ffffff',rx: 10,ry: 10,},label: {text: '结束',fontSize: 13,fontWeight: 'bold',},},})let dragNodeif (type === 'start') {dragNode = startNode} else if (type === 'end') {dragNode = endNode} else if (type === 'rect') {dragNode = rectNode} else if (type === 'polygon') {dragNode = polygonNode}console.log('dnd', dragNode, e, type)this.dnd.start(dragNode, e)},// 删除事件 节点removeNode (node) {this.graphOut.removeNode(node)},// 鼠标事件mouseEvent (graph) {// 鼠标事件// 鼠标 Hover 时添加按钮graph.on('node:mouseenter', ({node}) => {node.addTools({name: 'button',args: {x: 0,y: 0,offset: {x: 18, y: 18},// onClick({ view }) { ... },},})})// 鼠标移开时删除按钮graph.on('node:mouseleave', ({node}) => {node.removeTools() // 删除所有的工具})graph.on('node:dblclick', ({node}) => {// 添加连接桩node.addPort({group: 'top',attrs: {circle: {magnet: true,stroke: '#8f8f8f',r: 5,},},})// 编辑nodethis.currentNode = nodethis.drawer = true})graph.on('edge:mouseenter', ({cell}) => {cell.addTools([{name: 'vertices'},{name: 'button-remove',args: {distance: 20},},])})graph.on('node:click', ({node}) => {this.currentNode = node})},// 键盘事件keyboardEvent (graph) {// 键盘事件graph.bindKey('tab', (e) => {e.preventDefault()const selectedNodes = graph.getCells().filter((item) => item.isNode())console.log(selectedNodes)if (selectedNodes.length) {const node = selectedNodes[0]const type = node.attrs['type']this.addChildNode(node.id, type)}})graph.bindKey('delete', (e) => {this.removeNode(this.currentNode)})graph.bindKey('backspace', (e) => {this.removeNode(this.currentNode)})},},watch: {// currentNode: {// handler (nwVal, old) {// },// immediate: true,// deep: true// }}
}
</script><style>
/* 样式调整 */
#stencil {width: 100px;height: 100%;position: relative;display: flex;flex-direction: column;align-items: center;border-right: 1px solid #dfe3e8;text-align: center;font-size: 12px;
}.dnd-rect {width: 50px;height: 30px;line-height: 40px;text-align: center;border: 2px solid #000000;border-radius: 6px;cursor: move;font-size: 12px;margin-top: 30px;
}.dnd-polygon {width: 35px;height: 35px;border: 2px solid #000000;transform: rotate(45deg);cursor: move;font-size: 12px;margin-top: 30px;margin-bottom: 10px;
}.dnd-circle {width: 35px;height: 35px;line-height: 45px;text-align: center;border: 5px solid #000000;border-radius: 100%;cursor: move;font-size: 12px;margin-top: 30px;
}.dnd-start {border: 2px solid #000000;
}.x6-widget-stencil {background-color: #f8f9fb;
}.x6-widget-stencil-title {background: #eee;font-size: 1rem;
}.x6-widget-stencil-group-title {font-size: 1rem !important;background-color: #fff !important;height: 40px !important;
}.x6-widget-transform {margin: -1px 0 0 -1px;padding: 0px;border: 1px solid #239edd;
}.x6-widget-transform > div {border: 1px solid #239edd;
}.x6-widget-transform > div:hover {background-color: #3dafe4;
}.x6-widget-transform-active-handle {background-color: #3dafe4;
}.x6-widget-transform-resize {border-radius: 0;
}</style>
相关文章:

vue2 + antvx6 实现流程图功能
导入关键包 npm install antv/x6 --save npm install antv/x6-vue-shape 保存插件 (可选) npm install --save antv/x6-plugin-clipboard antv/x6-plugin-history antv/x6-plugin-keyboard antv/x6-plugin-selection antv/x6-plugin-snapline antv/x6-plugin-stencil antv/…...
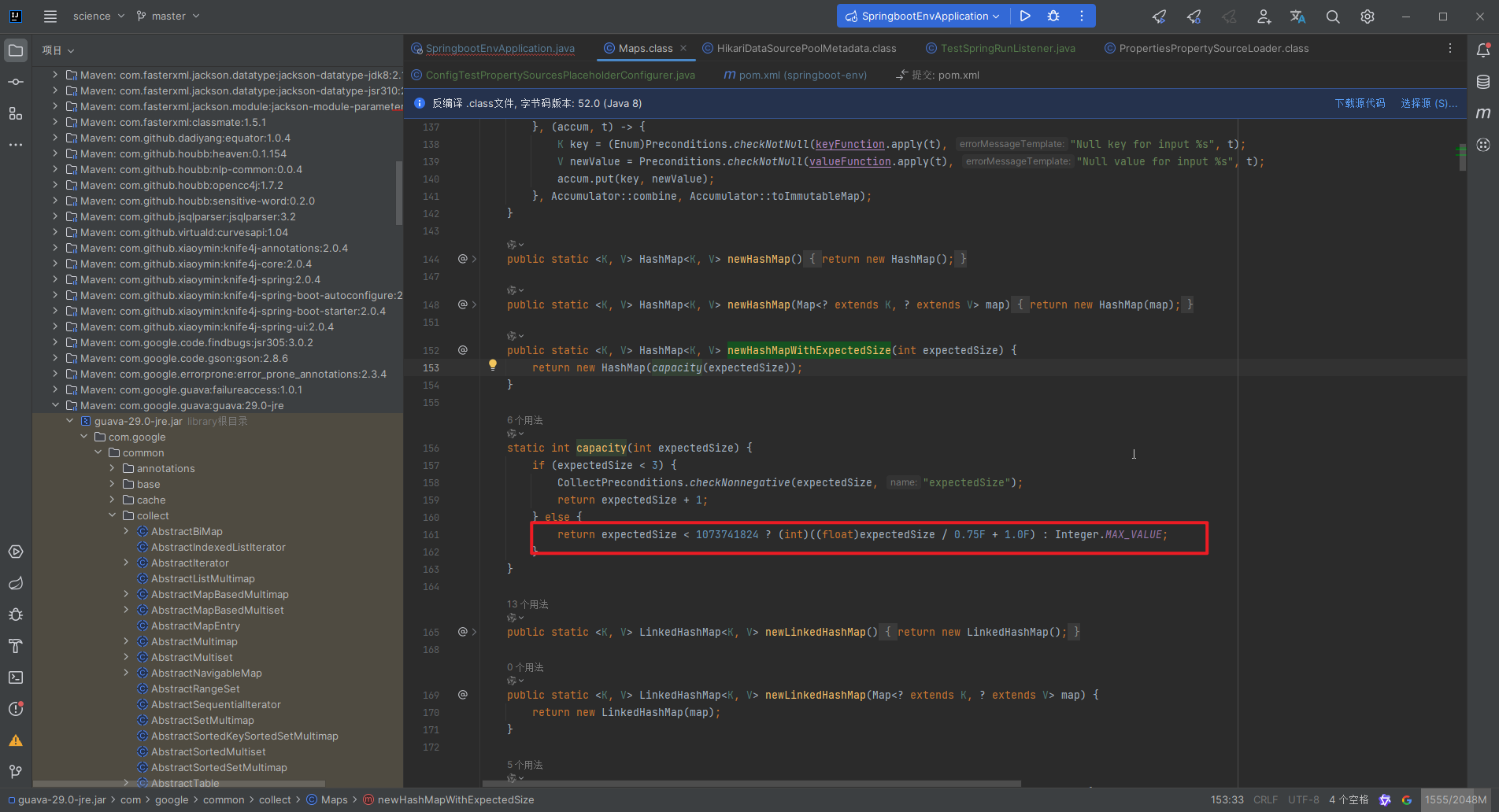
IDEA 中的奇技淫巧
IDEA 中的奇技淫巧 书签 在使用ctrlalt方向键跳转时,或者追踪代码时,经常遇到的情况是层级太多,找不到代码的初始位置,入口。可以通过书签的形式去打上一个标记,后续可以直接跳转到书签位置。 标记书签:c…...
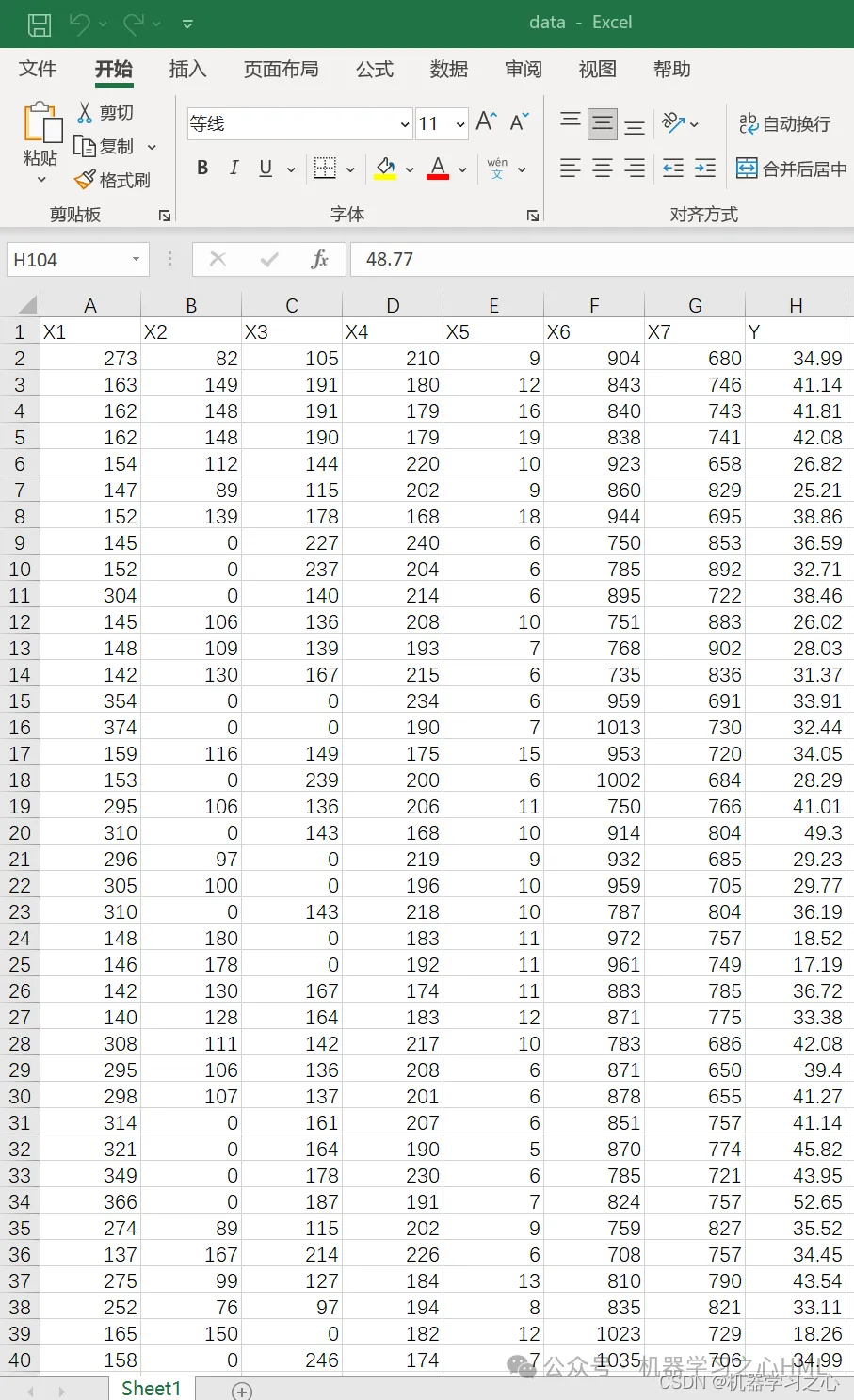
LSTM-KDE的长短期记忆神经网络结合核密度估计多变量回归区间预测(Matlab)
LSTM-KDE的长短期记忆神经网络结合核密度估计多变量回归区间预测(Matlab) 目录 LSTM-KDE的长短期记忆神经网络结合核密度估计多变量回归区间预测(Matlab)效果一览基本介绍程序设计参考资料 效果一览 基本介绍 1.LSTM-KDE的长短期…...

CMakeLists.txt语法规则:部分常用命令说明三
一. 简介 前面几篇文章学习了CMakeLists.txt语法中 add_executable命令,add_library命令,aux_source_directory命令,include_directories命令,add_subdirectory 命令的简单使用。文章如下: CMakeLists.txt语法规则&…...
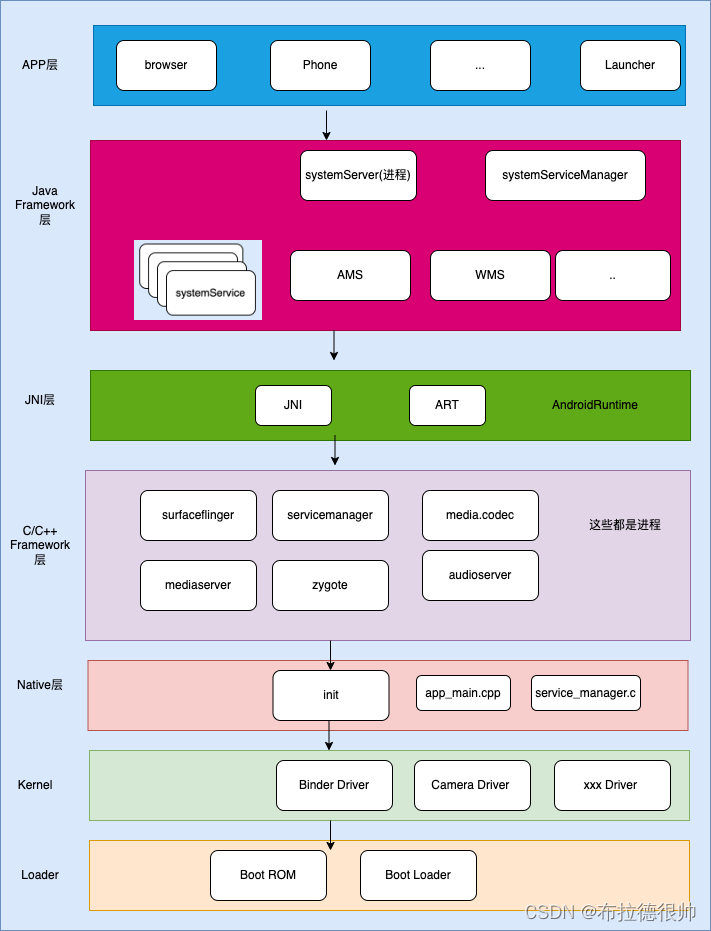
android init进程启动流程
一,Android系统完整的启动流程 二,android 系统架构图 三,init进程的启动流程 四,init进程启动服务的顺序 五,android系统启动架构图 六,Android系统运行时架构图 bool Service::Start() {// Starting a service removes it from the disabled or reset state and// imme…...
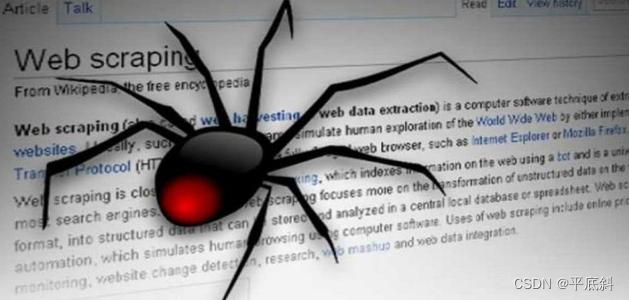
利用爬虫解决数据采集难题
文章目录 安装为什么选择 BeautifulSoup 和 requests?安装 BeautifulSoup 和 requests解决安装问题 示例总结 在现代信息时代,数据是企业决策和发展的关键。然而,许多有用的数据分散在网络上,且以各种格式和结构存在,因…...
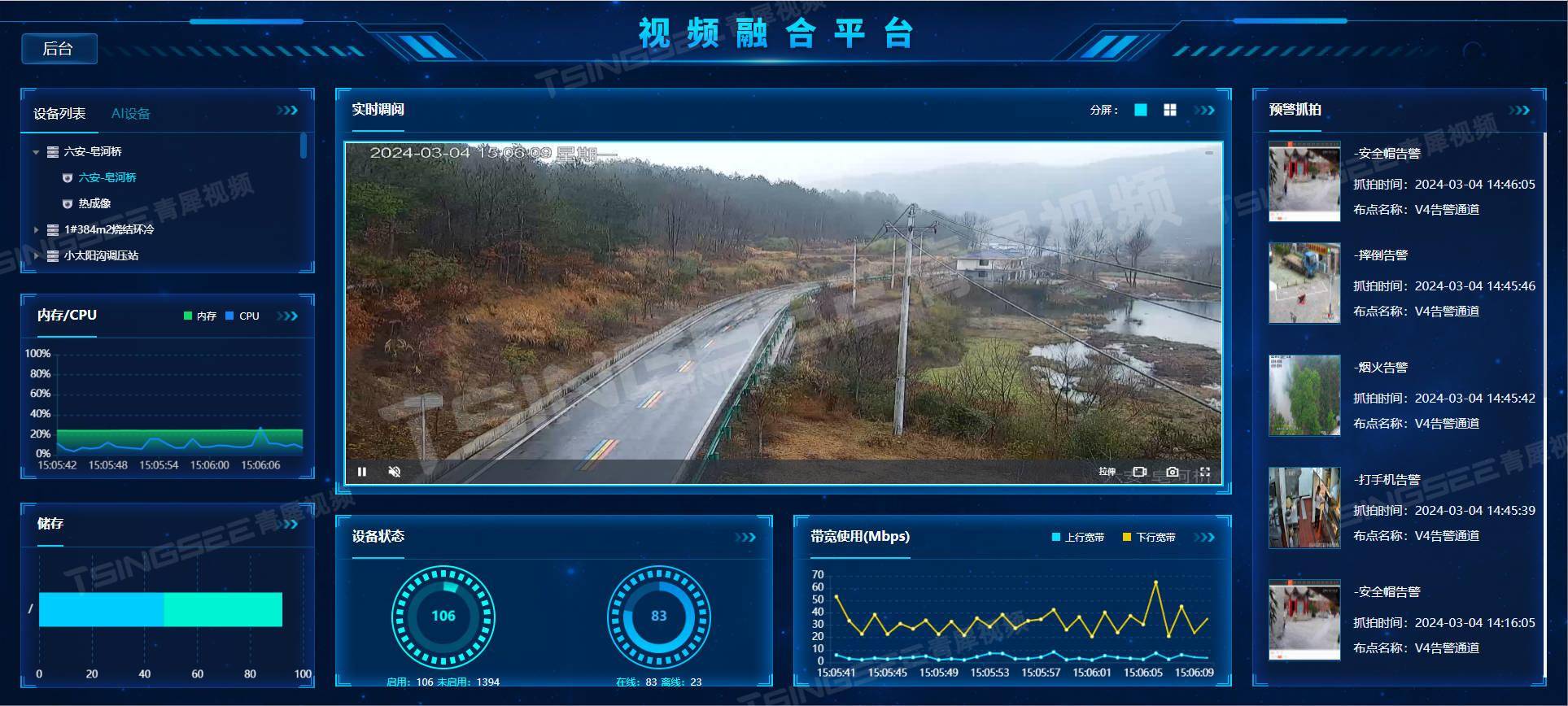
智慧粮库/粮仓视频监管系统:AI视频智能监测保障储粮安全
智慧粮库视频监管系统是一种基于物联网、AI技术和视频监控技术的先进管理系统,主要用于对粮食储存环境进行实时监测、数据分析和预警。TSINGSEE青犀智慧粮库/粮仓视频智能管理系统方案通过部署多区域温、湿度、空气成分等多类传感器以及视频监控等设施,对…...
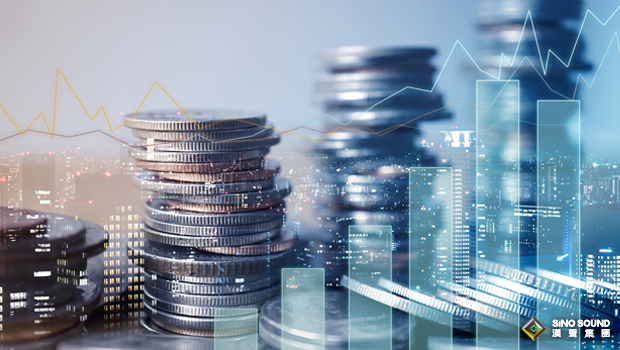
经验浅谈!伦敦银如何交易?
近期,伦敦银价格出现很强的上涨,这促使一些新手投资者进入了市场,但由于缺乏经验,他们不知道该怎么在市场中交易,下面我们就从宏观上介绍一些方法,来讨论一下伦敦银如何交易。 首先我们要知道,要…...

信息系统项目管理师(高项)_习题杂记
1.GB/T16260-2006《软件工程产品质量》系列标准: 1)GB/T16260.1-2006《软件工程产品质量第1部分:质量模型》,提出了软件生存周期中的质量模型; 2)GB/T16260.2-2006《软件工程产品质量第2部分:…...

CMakeLists.txt 简单的语法介绍
一. 简介 前面通过几个简单地示例向大家演示了 cmake 的使用方法,由此可知,cmake 的使用方法其实还是非常简单的,重点在于编写 CMakeLists.txt,CMakeLists.txt 的语法规则也简单,并没有 Makefile 的语法规则那么复杂难…...
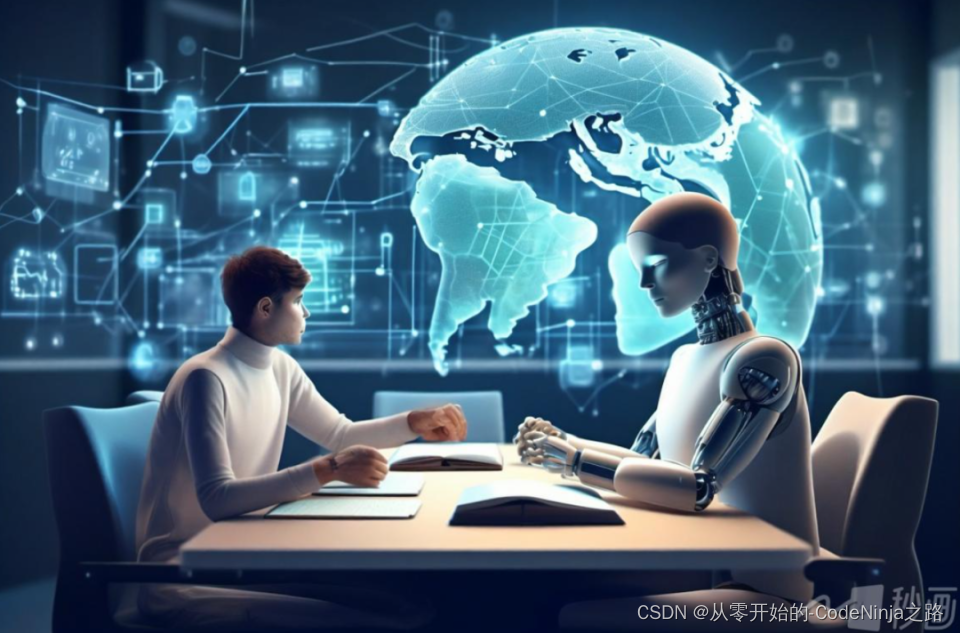
AI时代:人工智能大模型引领科技创造新时代
目录 前言一. AI在国家战略中有着举足轻重的地位1.1 战略1.2 能源1.3 教育 二. AI在日常生活中扮演着重要角色2.1 医疗保健2.2 智能客服2.3 自动驾驶2.4 娱乐和媒体2.5 智能家居 三. AI的未来发展趋势 总结 前言 随着AI技术的进步,新一代的AI技术已经开始尝试摆脱依…...
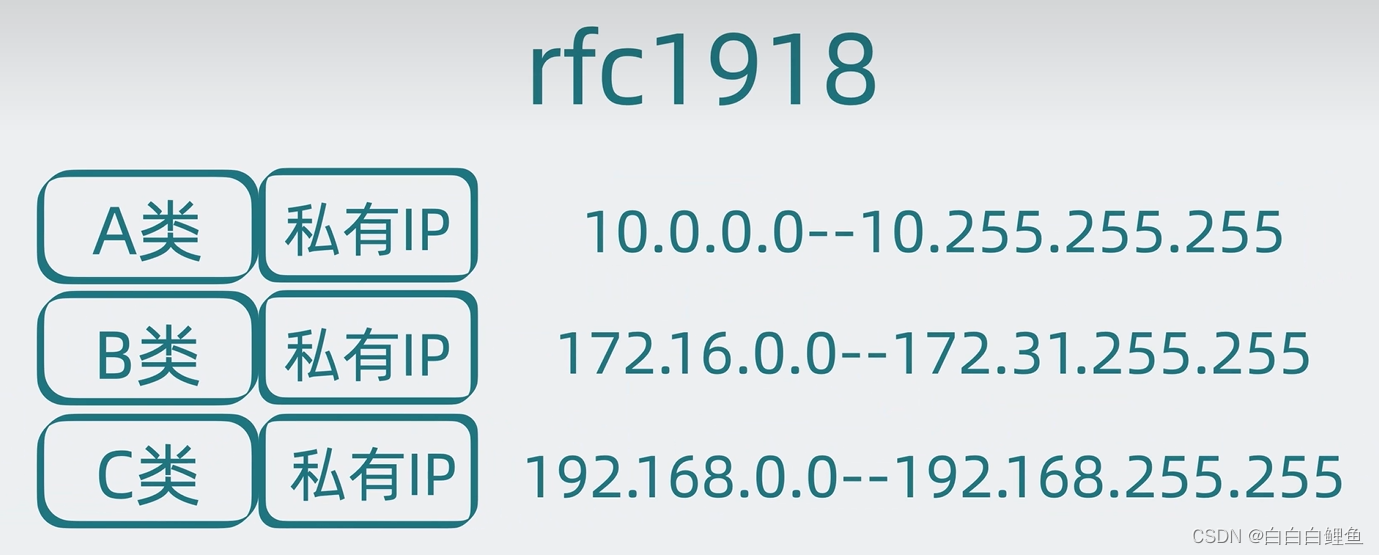
为什么 IP 地址通常以 192.168 开头?(精简版)
网络通讯的本质就是收发数据包。如果说收发数据包就跟收发快递一样。IP地址就类似于快递上填的收件地址和发件地址一样,路由器就充当快递员的角色,在这个纷繁复杂的网络世界里找到该由谁来接收这个数据包,所以说:IP地址就像快递里…...

【HEC】HECRAS中的降雨边界
目录 说明HEC-RAS网格降雨模型与HEC-HMS的比较HECRAS 降雨边界2D Area降雨边界添加降水边界条件调整2D Flow Area特性添加入渗网格数据创建土地覆盖层创建土壤层创建入渗层指定几何图形关联具有空间变化的网格降水数据Point点数据Gridded网格化数据Constant恒定值蒸散和风数据...

搜索算法系列之三(插值查找)
前言 插值查找仅适用于有序数据、有序数组,和二分查找类似,更讲究数据有序均匀分布。 算法原理 插值查找(interpolation search)是一种查找算法,它与二分查找类似,但在寻找元素时更加智能化。这种算法假设数据集是等距的或者有…...
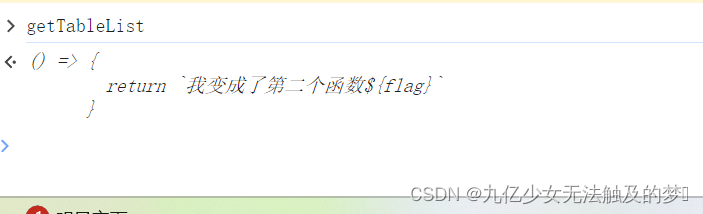
前端奇怪面试题总结
面试题总结 不修改下面的代码进行正常解构 这道题考的是迭代器和生成器的概念 let [a,b] {a:1,b:2}答案 对象缺少迭代器,需要手动加上 Object.prototype[Symbol.iterator] function* (){// return Object.values(this)[Symbol.iterator]()return yeild* Object.v…...

NPM--最新淘宝镜像源地址
最新淘宝镜像源地址: 原来的 https://registry.npm.taobao.org 已替换为 https://registry.npmmirror.com 查看镜像源 npm config get registry 更换为淘宝最新镜像源 npm config set registry https://registry.npmmirror.com...

vue3中实现地区下拉选择组件封装
1组件文件 新建一个文件夹内,包含inde.vue,index.ts,pac.json这三个文件 index.vue文件 <template><el-cascaderv-model"data":options"pcaData":style"{ width: props.width }":placeholder"props.placeholder&quo…...

责任链模式案例
需求背景: 请你设计一个员工休假审批流程,当员工的休假天数<1时,由直接领导审批,休假天数<2时,分别由直接领导、一级部门领导审批,休假天数>3时,分别由直接领导、一级部门领导、分管领…...
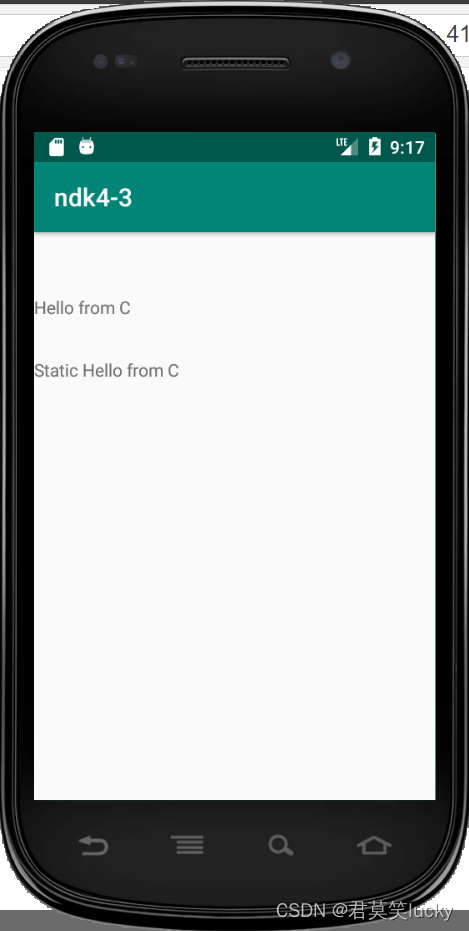
Android NDK开发(二)——JNIEnv、jobject与jclass关系
本文主要讲解Android NDK开发中JNIEnv、jobject与jclass的相关知识,并用c和c两种语言实现了jobject和jclass。 本专栏知识点是通过<零声教育>的音视频流媒体高级开发课程进行系统学习,梳理总结后写下文章,对音视频相关内容感兴趣的读者…...

机器学习入门:sklearn基础教程
Scikit-learn(简称sklearn)是Python中最受欢迎的机器学习库之一,它提供了丰富的机器学习算法和工具,适用于各种任务和场景。本文将为您介绍sklearn的基础知识和常用功能,带您踏入机器学习的世界。 1. 安装与导入 首先…...
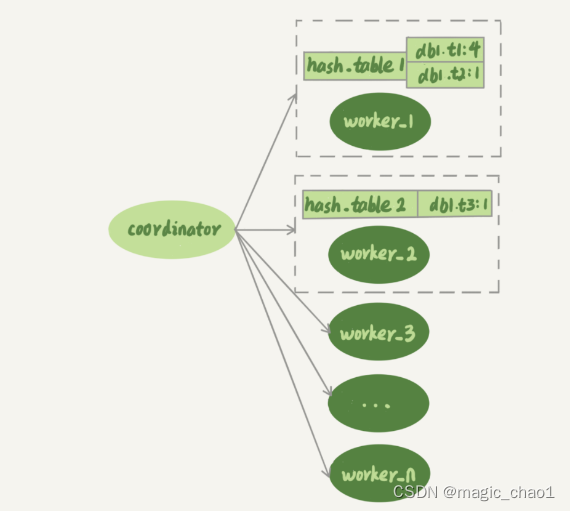
26 | 备库为什么会延迟好几个小时?
在官方的 5.6 版本之前,MySQL 只支持单线程复制,由此在主库并发高、TPS 高时就会出现严重的主备延迟问题。 coordinator 就是原来的 sql_thread, 不过现在它不再直接更新数据了,只负责读取中转日志和分发事务。真正更新日志的,变成了 worker 线程。而 work 线程的个数,就是…...

linux 如何解压.tar 文件
要在 Linux 中解压 tar 文件,请使用以下命令: tar -xvf yourfile.tar 1 其中,“yourfile.tar”是您要解压的文件名。 这个命令会将文件解压到当前目录中。如果想要将文件解压到不同的目录中,可以使用 -C 选项指定路径。例如&…...
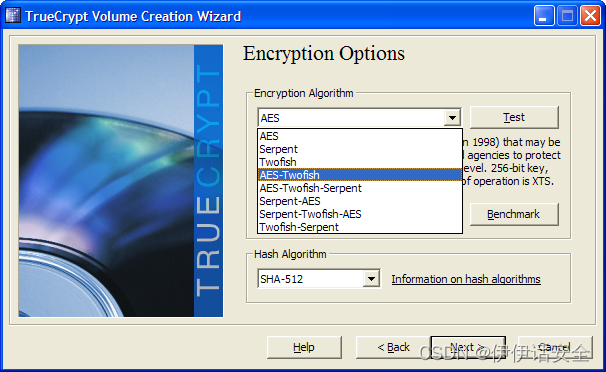
盘点企业信息防泄密软件对比|揭秘企业信息防泄密软件好用榜
在当今信息化社会,企业信息防泄密软件的需求日益凸显。这些软件不仅关乎企业的核心竞争力,更直接关系到企业的生死存亡。本文将对市面上几款主流的企业信息防泄密软件进行深入对比分析,以期为企业提供有益的参考。 一、企业信息防泄密软件好…...
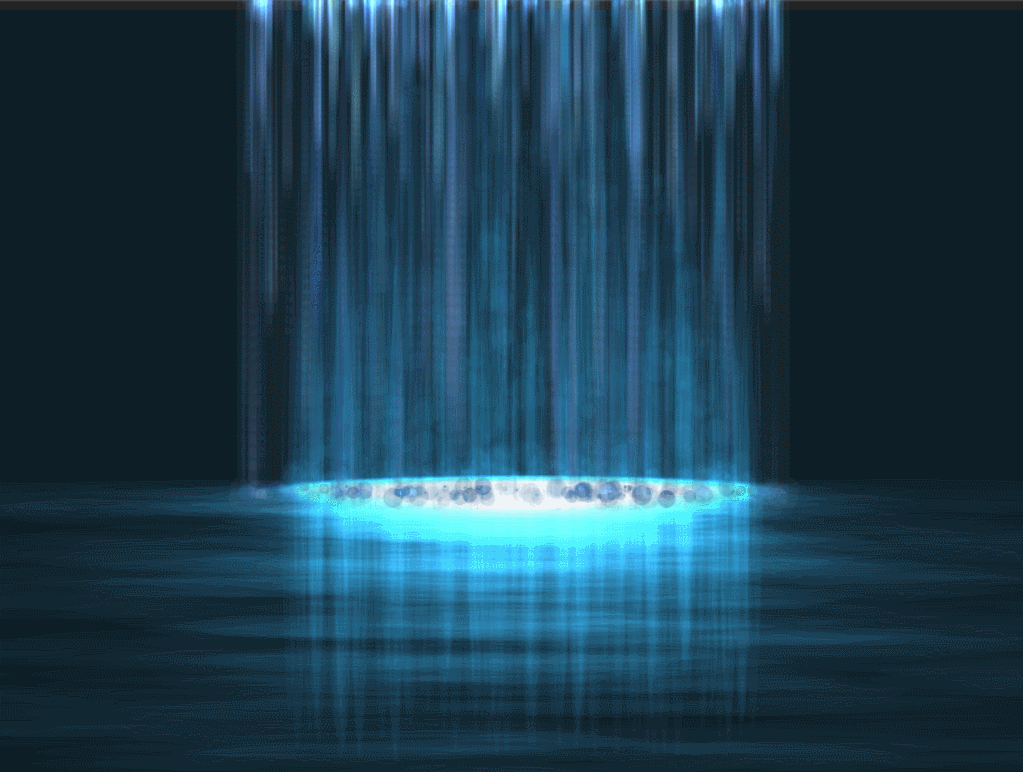
html--瀑布效果
<!doctype html> <html> <head> <meta charset"utf-8"> <title>瀑布效果</title><style> body {background: #222;color: white;overflow:hidden; }#container {box-shadow: inset 0 1px 0 #444, 0 -1px 0 #000;height: 1…...
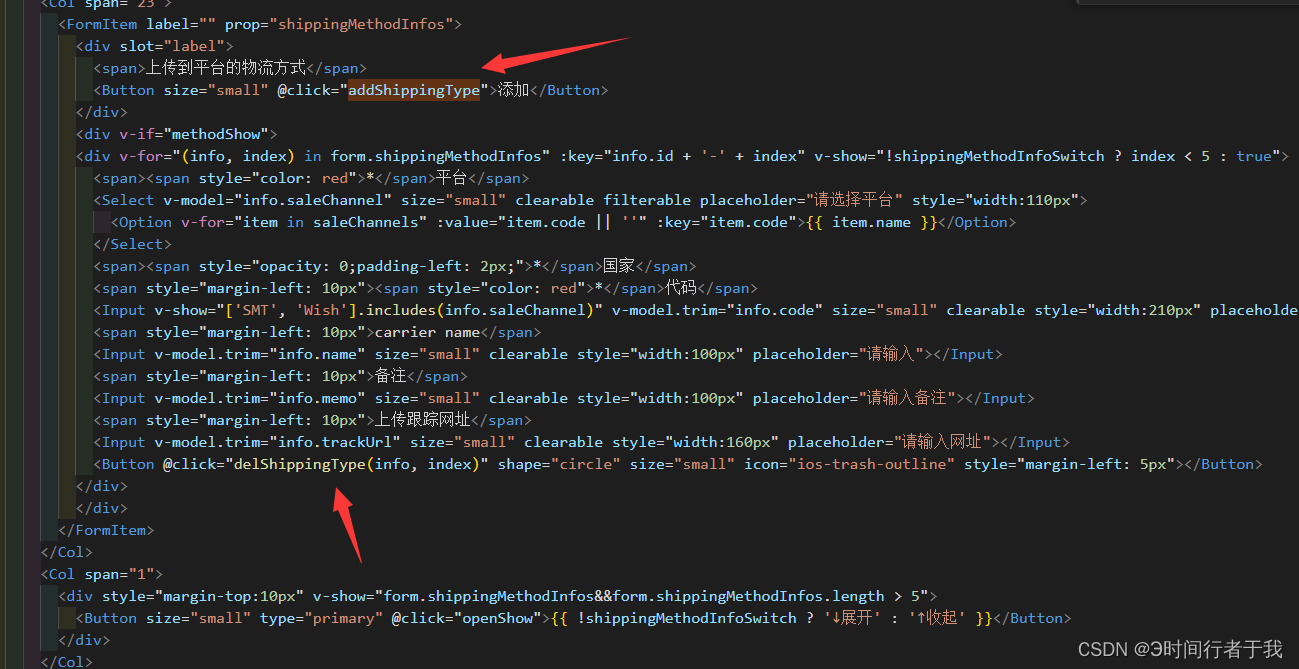
vue视图不刷新强制更新数据this.$forceUpdate()
在vue中,更新视图数据,不刷新页面,需要强制更新数据才可以 前言 在对数据就行添加和删除时,发现页面视图不更新,排除发现需要强制更新才可以 点击添加或删除,新增数据和删除就行,但在不使用fo…...
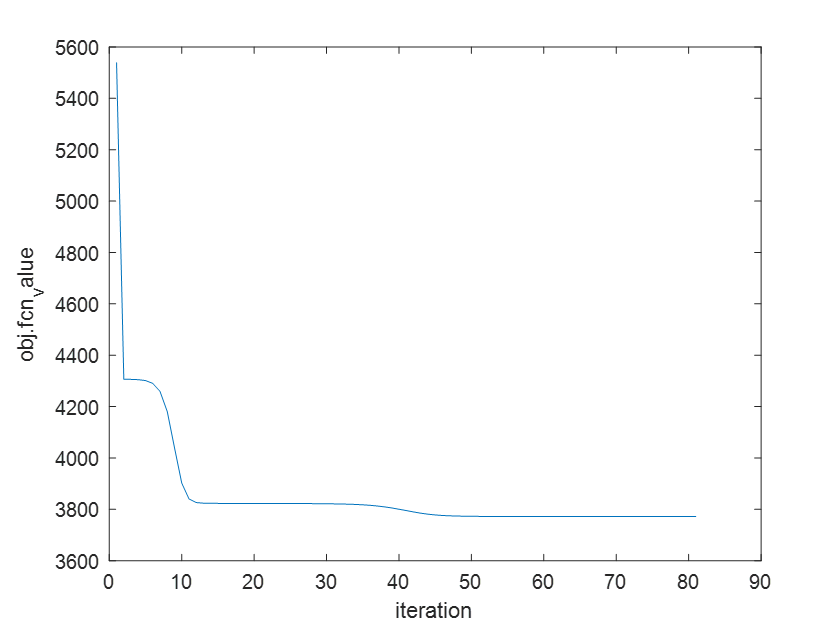
2024年电工杯数学建模竞赛A题B题思路代码分享
您的点赞收藏是我继续更新的最大动力! 一定要点击如下的卡片链接,那是获取资料的入口! 点击链接加入群聊【2024电工杯】:http://qm.qq.com/cgi-bin/qm/qr?_wv1027&kUMFX8lu4qAm0XkZQ6JkW5m5O9F_mxf-L&authKey0hWdf7%2F…...

leetcode 797.所有可能的路径
思路:dfs。 其实很简单,我们只需要和昨天做的题一样,直接遍历所给数组中的元素,因为这里的数组意义已经很清楚了,就是当前位置的结点和哪一个顶点有联系。 注意:在存储路径的时候,我们需要按顺…...

NPM 基础
介绍 npm 是 JavaScript 编程语言的一个包管理器,它允许开发者安装、共享和管理依赖项。npm 与 Node.js 紧密集成,是 Node.js 生态系统中不可或缺的一部分。它提供了一个命令行工具,使得开发者能够轻松地安装、配置和管理项目所需的各种包。…...
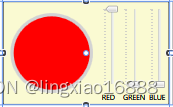
WPF之创建无外观控件
1,定义无外观控件。 定义默认样式,在其静态构造函数中调用DefaultStyleKeyProperty.OverrideMetadata()。 //设置默认样式DefaultStyleKeyProperty.OverrideMetadata(typeof(ColorPicker), new FrameworkPropertyMetadata(typeof(ColorPicker))); 在项目…...
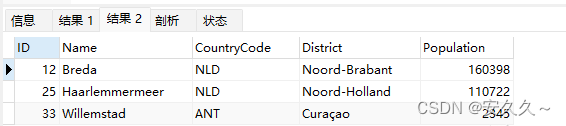
MySQL利用变量进行查询操作
新建连接,自带world数据库,里面自带city表格。 # MySQL利用变量进行查询操作 set cityNameHaarlemmermeer; select * from city where NamecityName;# 多个结果查询 set cityName1Haarlemmermeer; set cityName2Breda; set cityName3Willemstad; selec…...

农场会员营销网站建设/百度站长seo
去中心化和幂律结合在一起,是一个很有意思的topic。 去中心化的动机在于大多数人不希望被第三方约束,只是因为他不信任第三方,然而一旦放开约束,便成就了他们所谓的去中心化,接下来的事情很有意思,他们会很…...

新浪云sae免费wordpress网站/推广手段和渠道有哪些
[LeetCode]1705.吃苹果的最大数目题目示例方法贪心题目 有一棵特殊的苹果树,一连 n 天,每天都可以长出若干个苹果。在第 i 天,树上会长出 apples[i] 个苹果,这些苹果将会在 days[i] 天后(也就是说,第 i d…...

军事的网站应如何建设/外链平台有哪些
(*) 窗口的摆放在一个窗口上点右键,有floating, dockable, tabbed document这三种摆放方式。floating: 浮在最上,任何地方。dockable: 浮在最上,任何地方,且可以锚定在一侧。有了dockable之后其实floating就成鸡肋了。tabbed docu…...

中国品牌网官网查询/百度seo关键词排名查询
今天数据迁移的小组找到我,希望我能够重新构建一些测试环境,其中测试环境中的一些分区表都需要去掉分区,转换成普通表的形式,因为他们在做一些工作的时候碰到了问题,而且希望必要的约束等都保留,这个需求听…...
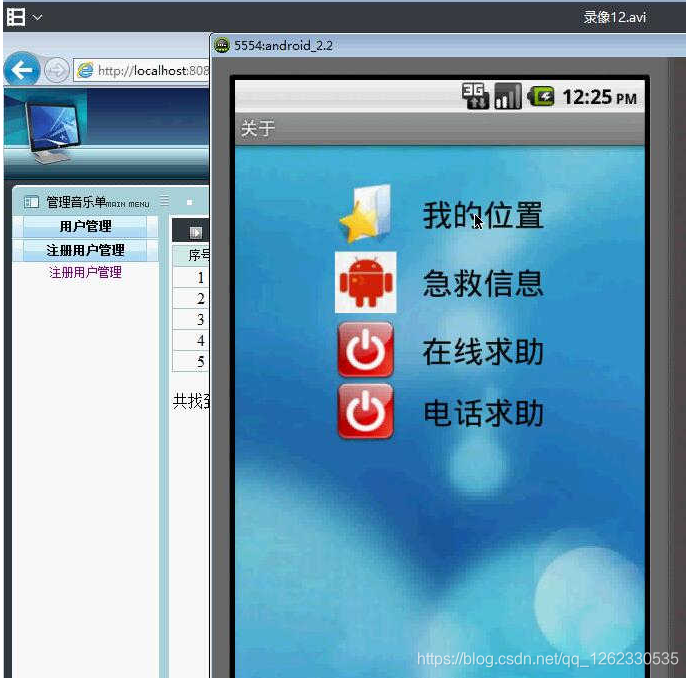
竞拍网站开发/网站推广的方式
项目介绍 旅游自主系统一个Android 客户端JSP Servlet服务端应用程序,启动Android上的应用程序后可以查看信息等。Android客户端上的信息数据是通过后台JSP Servlet服务端应用程序获取的,这个后台服务可以根据客户端发出的请求,返回信息。 图…...

wordpress 5.1.1简体中文版/网络销售公司经营范围
在Linux系统中,虽然有各种各样的图形化接口工具,但是sell仍然是一个非常灵活的工具。Shell不仅仅是命令的收集,而且是一门非常棒的编程语言。您可以通过使用shell使大量的任务自动化,shell特别擅长系统管理任务,尤其适…...