南宁网站seo优化公司/自己做一个网站
官方文档
If you have a lot of HTML to port to JSX, you can use an online converter.
You’ll get two things from useState: the current state (count), and the function that lets you update it (setCount).
- To “remember” things, components use state.
- To make the UI interactive, you need to let users change your underlying data model. You will use state for this.
Which of these are state? Identify the ones that are not:
- Does it remain unchanged over time? If so, it isn’t state.
- Is it passed in from a parent via props? If so, it isn’t state.
- Can you compute it based on existing state or props in your component? If so, it definitely isn’t state!
After identifying your app’s minimal state data, you need to identify which component is responsible for changing this state, or owns the state.
For each piece of state in your application:
- Identify every component that renders something based on that state.
- Find their closest common parent component—a component above them all in the hierarchy.
- Decide where the state should live:
- Often, you can put the state directly into their common parent.
- You can also put the state into some component above their common parent.
- If you can’t find a component where it makes sense to own the state, create a new component solely for holding the state and add it somewhere in the hierarchy above the common parent component.
You can find other built-in Hooks in the API reference.
Hooks are more restrictive than other functions. You can only call Hooks at the top of your components (or other Hooks). If you want to use useState in a condition or a loop, extract a new component and put it there.
A JSX element is a combination of JavaScript code and HTML tags that describes what you’d like to display.
To “escape into JavaScript” from JSX, you need curly braces. Add curly braces around value in JSX like so:
function Square({ value }) {return <button className="square">{value}</button>;
}
React DevTools let you check the props and the state of your React components.
When you were passing onSquareClick={handleClick}
, you were passing the handleClick function down as a prop. You were not calling it! But now you are calling that function right away—notice the parentheses in handleClick(0)
— and that’s why it runs too early. You don’t want to call handleClick until the user clicks! Instead, let’s do this: <Square value={squares[0]} onSquareClick={() => handleClick(0)} />
. Notice the new () => syntax
. Here, () => handleClick(0)
is an arrow function, which is a shorter way to define functions.
In React, it’s conventional to use onSomething names for props which represent events and handleSomething for the function definitions which handle those events.
**Why immutability is important?**Note how in handleClick, you call .slice()
to create a copy of the squares array instead of modifying the existing array?
https://react.dev/learn/tutorial-tic-tac-toe#why-immutability-is-important
You can learn more about how React chooses when to re-render a component in the memo API reference.
https://react.dev/reference/react/memo
When you render a list, React stores some information about each rendered list item. When you update a list, React needs to determine what has changed. You could have added, removed, re-arranged, or updated the list’s items. React is a computer program and does not know what you intended, so you need to specify a key property for each list item to differentiate each list item from its siblings. When a list is re-rendered, React takes each list item’s key and searches the previous list’s items for a matching key. If the current list has a key that didn’t exist before, React creates a component. If the current list is missing a key that existed in the previous list, React destroys the previous component. If two keys match, the corresponding component is moved.
https://react.dev/learn/tutorial-tic-tac-toe#picking-a-key
Using the array index as a key is problematic when trying to re-order a list’s items or inserting/removing list items?
To implement a UI in React, you will usually follow the same five steps.
Step 1: Break the UI into a component hierarchy
Step 2: Build a static version in React
Step 3: Find the minimal but complete representation of UI state
Step 4: Identify where your state should live
Step 5: Add inverse data flow
https://react.dev/learn/thinking-in-react
In simpler examples, it’s usually easier to go top-down, and on larger projects, it’s easier to go bottom-up.
You can learn all about handling events and updating state in the Adding Interactivity section.
https://react.dev/learn/adding-interactivity
- Responding to events
- State: a component’s memory
- Render and commit
- State as a snapshot
- Queueing a series of state updates
- Updating objects in state
- Updating arrays in state
state as a snapshot:
console.log(count); // 0
setCount(count + 1); // Request a re-render with 1
console.log(count); // Still 0!
Read State as a Snapshot to learn why state appears “fixed” and unchanging inside the event handlers.
https://react.dev/learn/state-as-a-snapshot
updater function:
console.log(score); // 0
setScore(score + 1); // setScore(0 + 1);
console.log(score); // 0
setScore(score + 1); // setScore(0 + 1);
console.log(score); // 0
setScore(score + 1); // setScore(0 + 1);
console.log(score); // 0
can fix this by passing an updater function when setting state.
function increment() {setScore(s => s + 1);
}
update objects:
Usually, you will use the ...
spread syntax to copy objects and arrays that you want to change.
const [person, setPerson] = useState({name: 'Niki de Saint Phalle',artwork: {title: 'Blue Nana',city: 'Hamburg',image: 'https://i.imgur.com/Sd1AgUOm.jpg',}});function handleNameChange(e) {setPerson({...person,name: e.target.value});}function handleTitleChange(e) {setPerson({...person,artwork: {...person.artwork,title: e.target.value}});}
If copying objects in code gets tedious, you can use a library like Immer to reduce repetitive code:
GitHub - immerjs/use-immer: Use immer to drive state with a React hooks
import { useImmer } from 'use-immer';const [person, updatePerson] = useImmer({name: 'Niki de Saint Phalle',artwork: {title: 'Blue Nana',city: 'Hamburg',image: 'https://i.imgur.com/Sd1AgUOm.jpg',}});function handleNameChange(e) {updatePerson(draft => {draft.name = e.target.value;});}function handleTitleChange(e) {updatePerson(draft => {draft.artwork.title = e.target.value;});}
update arrays:
Arrays are another type of mutable JavaScript objects you can store in state and should treat as read-only.
const [list, setList] = useState(initialList);function handleToggle(artworkId, nextSeen) {setList(list.map(artwork => {if (artwork.id === artworkId) {return { ...artwork, seen: nextSeen };} else {return artwork;}}));}
If copying arrays in code gets tedious, you can use a library like Immer to reduce repetitive code:
import { useImmer } from 'use-immer';
const [list, updateList] = useImmer(initialList);function handleToggle(artworkId, nextSeen) {updateList(draft => {const artwork = draft.find(a =>a.id === artworkId);artwork.seen = nextSeen;});}
相关文章:

WHAT - React 学习系列(一)
官方文档 If you have a lot of HTML to port to JSX, you can use an online converter. You’ll get two things from useState: the current state (count), and the function that lets you update it (setCount). To “remember” things, components use state.To mak…...
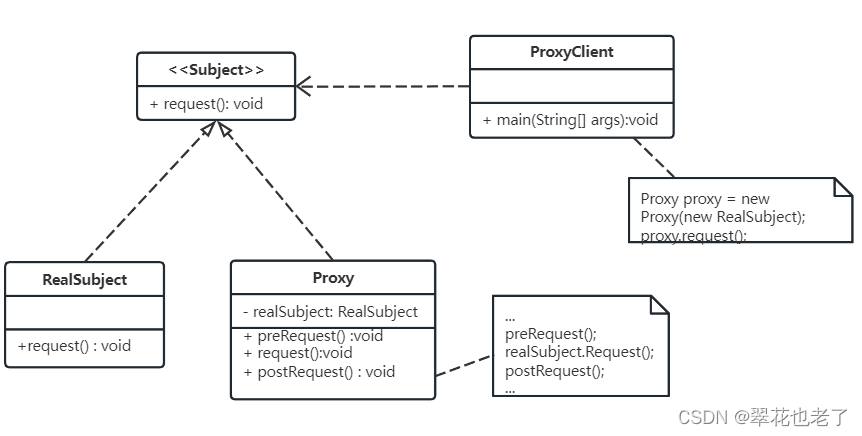
代理模式(静态代理/动态代理)
代理模式(Proxy Pattern) 一 定义 为其他对象提供一种代理,以控制对这个对象的访问。 代理对象在客户端和目标对象之间起到了中介作用,起到保护或增强目标对象的作用。 属于结构型设计模式。 代理模式分为静态代理和动态代理。…...
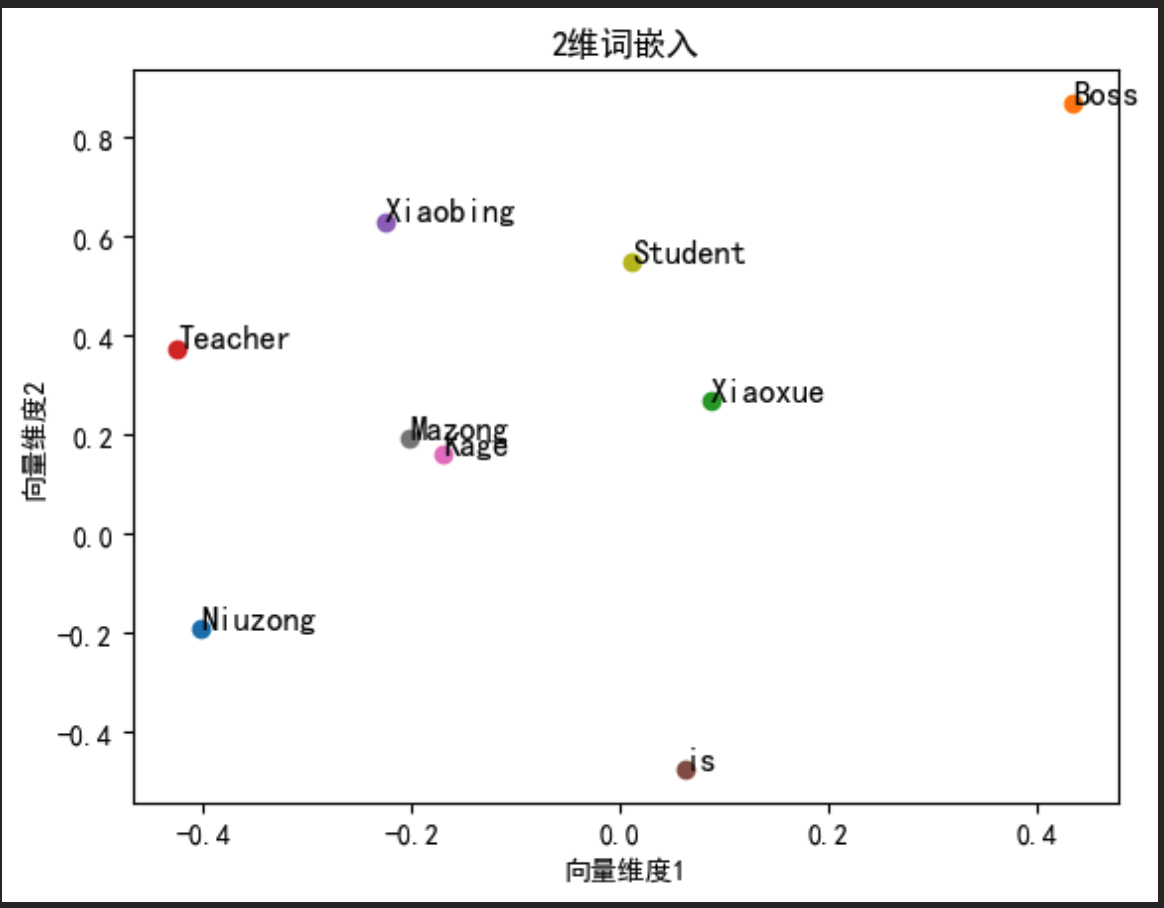
Word2Vec基本实践
系列文章目录 提示:这里可以添加系列文章的所有文章的目录,目录需要自己手动添加 例如:第一章 Python 机器学习入门之pandas的使用 提示:写完文章后,目录可以自动生成,如何生成可参考右边的帮助文档 文章目…...
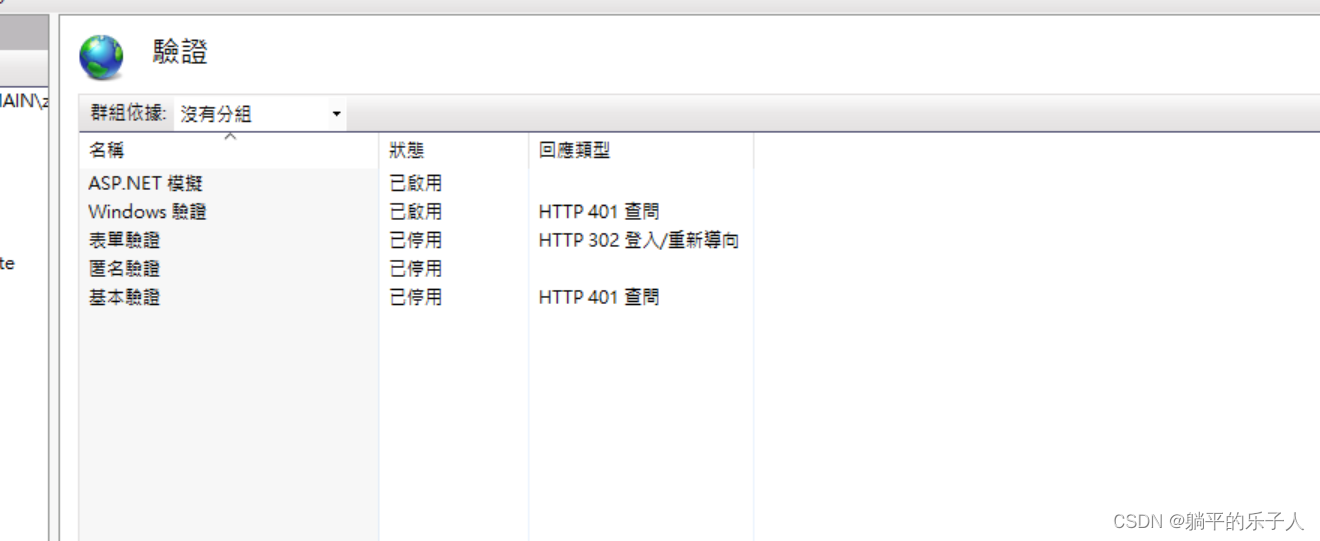
IIS配置網站登錄驗證,禁止匿名登陸
需要維護一個以前的舊系統,這個系統在內網運行,需要抓取電腦的登陸賬號,作為權限管理的一部分因此需要在IIS配置一下...
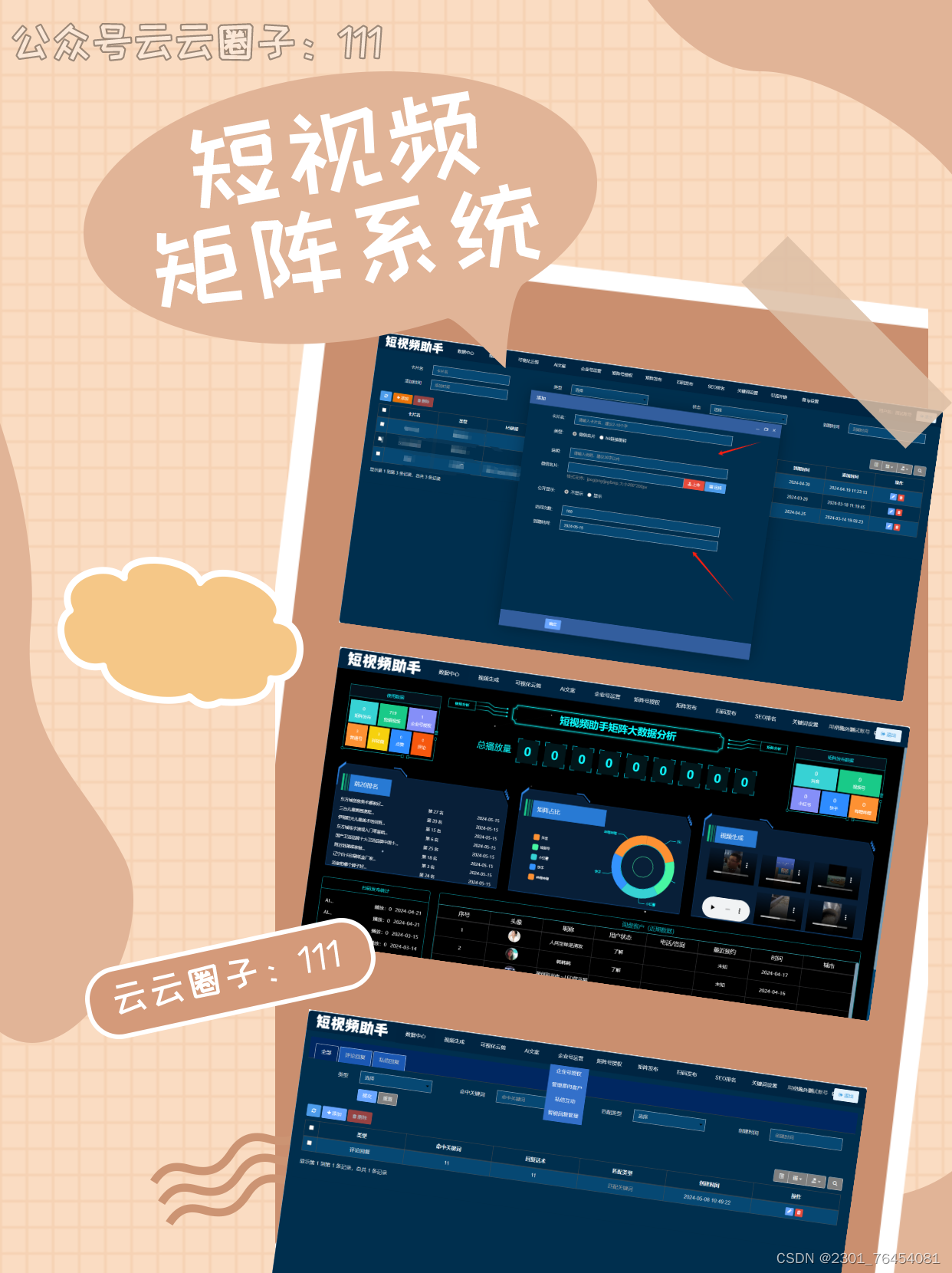
抖音矩阵系统搭建,AI剪辑短视频,一键管理矩阵账号
目录 前言: 一、抖音矩阵系统有哪些功能? 1.AI智能文案 2.多平台账号授权 3.多种剪辑模式 4. 矩阵一键发布,智能发布 5.抖音爆店码功能 6.私信实时互动 7.去水印及外链 二、抖音矩阵系统可以解决哪些问题? 总结ÿ…...
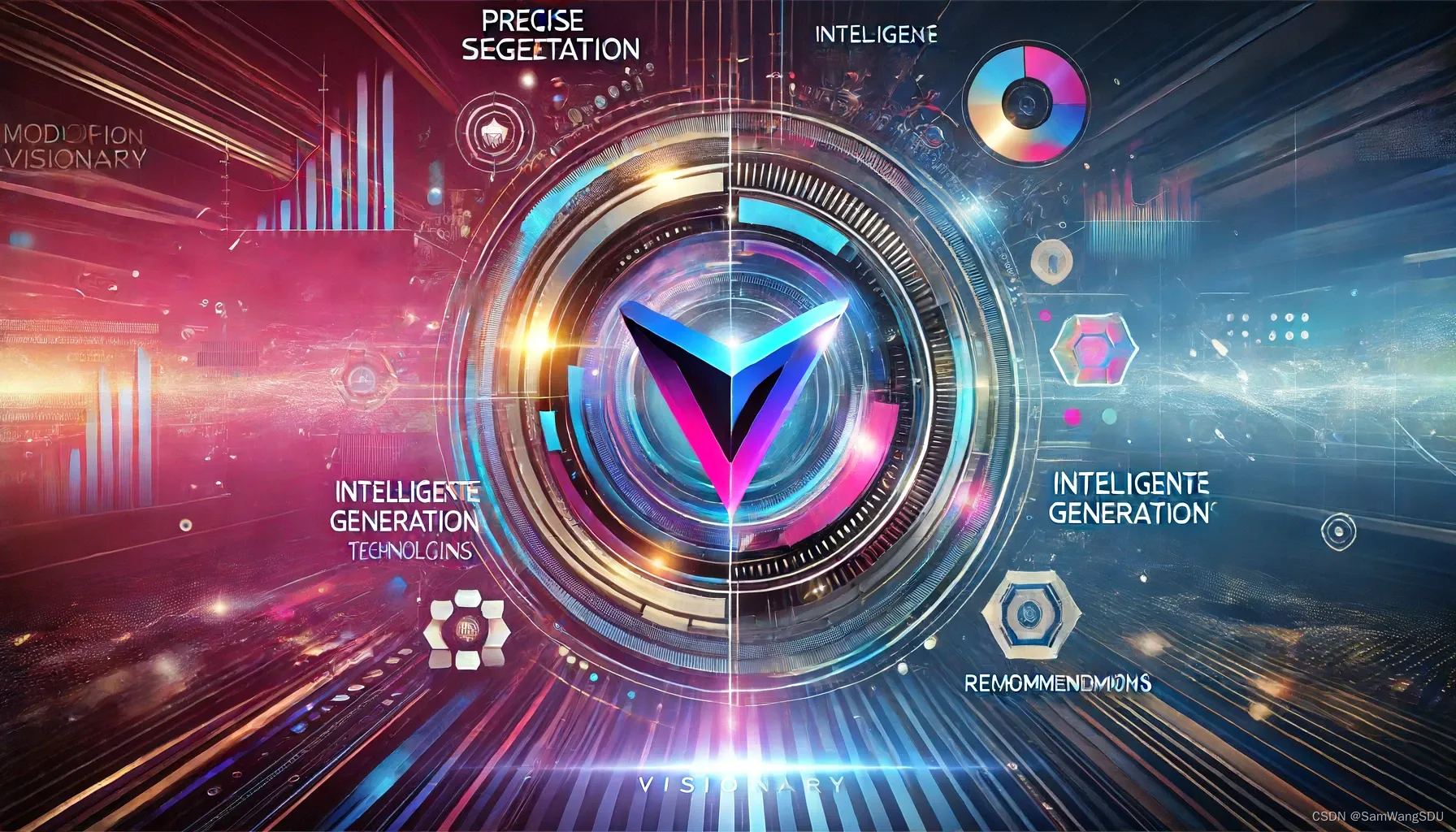
山东大学软件学院创新项目实训开发日志——收尾篇
山东大学软件学院创新项目实训开发日志——收尾篇 项目名称:ModuFusion Visionary:实现跨模态文本与视觉的相关推荐 -------项目目标: 本项目旨在开发一款跨模态交互式应用,用户可以上传图片或视频,并使用文本、点、…...

vue2.7支持组合式API,但是对应的vue-router3并不支持useRoute、useRouter。
最近在做一个项目,因为目标用户浏览器版本并不确定,可能会有较旧版本,于是采用vue2.7而不是vue3,最近一年多使用vue3开发的项目都碰到了很多chrome 63-73版本,而对应UI 库 element plus又问题很多。 为了不碰到这些问…...
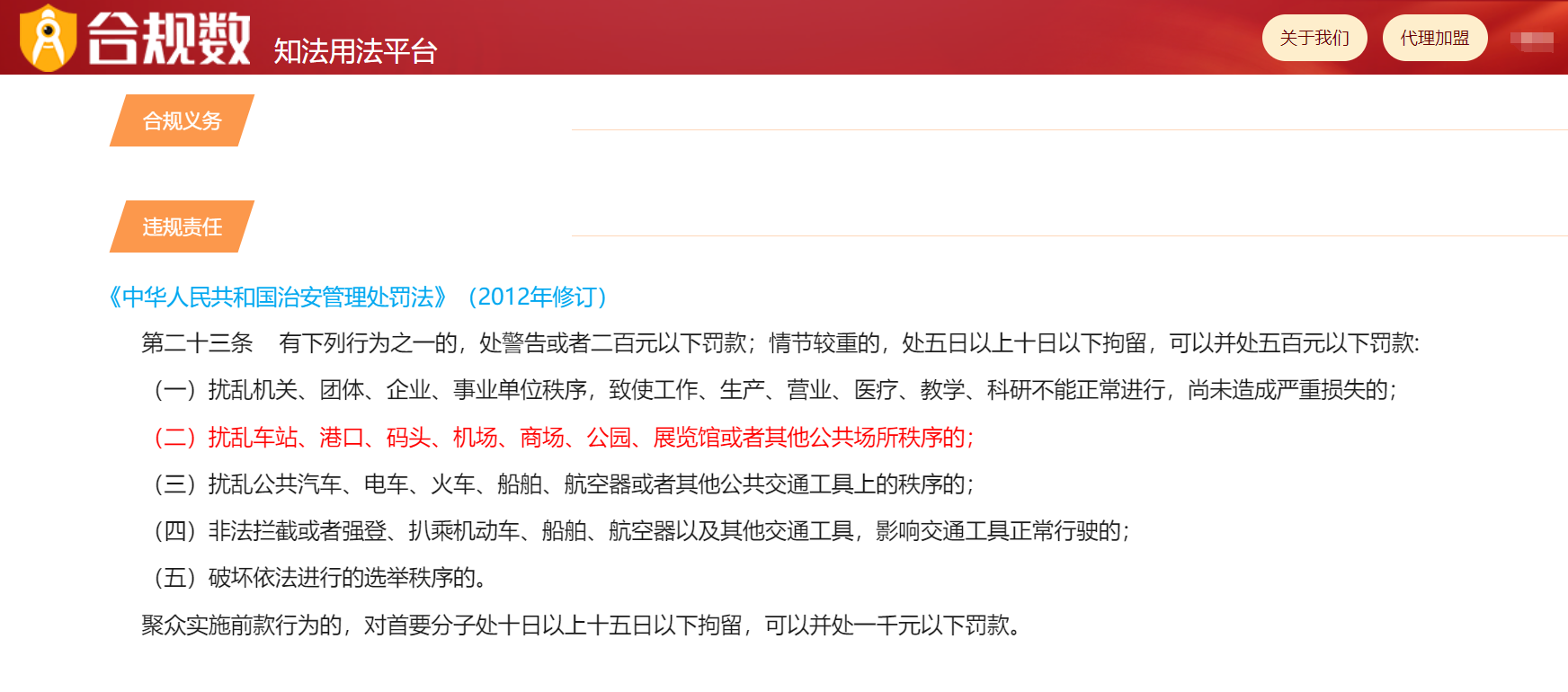
摊位纠纷演变肢体冲突,倒赔了500:残疾夫妇与摊主谁之过?
在一个小商贩密集的街区,一起由摊位纠纷引发的肢体冲突事件在当地社区和网络上引起了热议。涉事双方为一名摊主和一对残疾夫妇,他们的争执源自对一个摊位的使用权。本是口头上的争吵,却由于双方情绪激动,迅速升级为肢体冲突&#…...
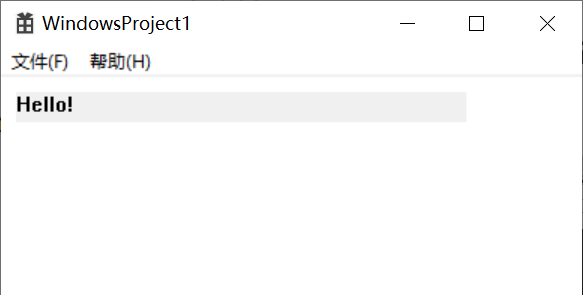
深入理解和实现Windows进程间通信(消息队列)
常见的进程间通信方法 常见的进程间通信方法有: 管道(Pipe)消息队列共享内存信号量套接字 下面,我们将详细介绍消息队列的原理以及具体实现。 什么是消息队列? Windows操作系统使用消息机制来促进应用程序与操作系…...

Web网页前端教程免费:引领您踏入编程的奇幻世界
Web网页前端教程免费:引领您踏入编程的奇幻世界 在当今数字化时代,Web前端技术已成为互联网发展的重要驱动力。想要踏入这一领域,掌握相关技能,却苦于找不到合适的教程?别担心,本文将为您带来一份免费的We…...
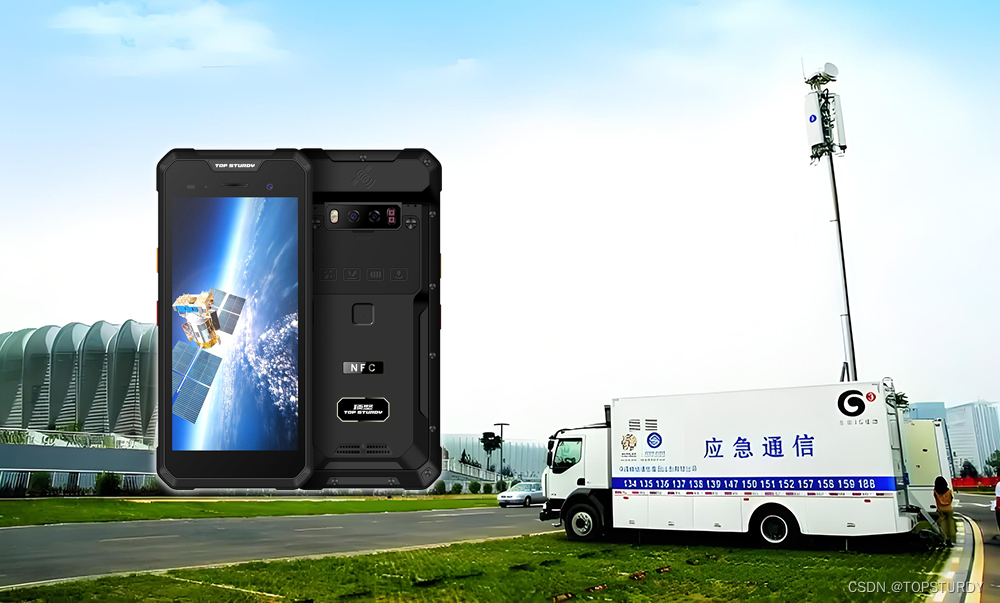
北斗短报文终端在应急消防通信场景中的应用
在应对自然灾害和紧急情况时,北斗三号短报文终端以其全球覆盖、实时通信和精准定位的能力,成为应急消防通信的得力助手。它不仅能够在地面通信中断的极端条件下保障信息传递的畅通,还能提供精准的位置信息,为救援行动提供有力支持…...

Java跳动爱心代码
1.计算爱心曲线上的点的公式 计算爱心曲线上的点的公式通常基于参数方程。以下是两种常见的参数方程表示方法,用于绘制爱心曲线: 1.1基于 (x, y) 坐标的参数方程 x a * (2 * cos(θ) - sin(θ))^3 y a * (2 * sin(θ) - cos(θ))^3 其中ÿ…...

Swift Combine — Operators(常用Filtering类操作符介绍)
目录 filter(_: )tryFilter(_: )compactMap(_: )tryCompactMap(_: )removeDuplicates()first(where:)last(where:) Combine中对 Publisher的值进行操作的方法称为 Operator(操作符)。 Combine中的 Operator通常会生成一个 Publisher,该 …...

Windows11+CUDA12.0+RTX4090如何配置安装Tensorflow2-GPU环境?
1 引言 电脑配置 Windows 11 cuda 12.0 RTX4090 由于tensorflow2官网已经不支持cuda11以上的版本了,配置cuda和tensorflow可以通过以下步骤配置实现。 2 步骤 (1)创建conda环境并安装cuda和cudnn,以及安装tensorflow2.10 con…...

韩顺平0基础学Java——第27天
p548-568 明天开始坦克大战 Entry 昨天没搞明白的Map、Entry、EntrySet://GPT教的 Map 和 Entry 的关系 1.Map 接口:它定义了一些方法来操作键值对集合。常用的实现类有 HashMap、TreeMap 等。 2. Entry接口:Entry 是 Map 接口的一个嵌…...
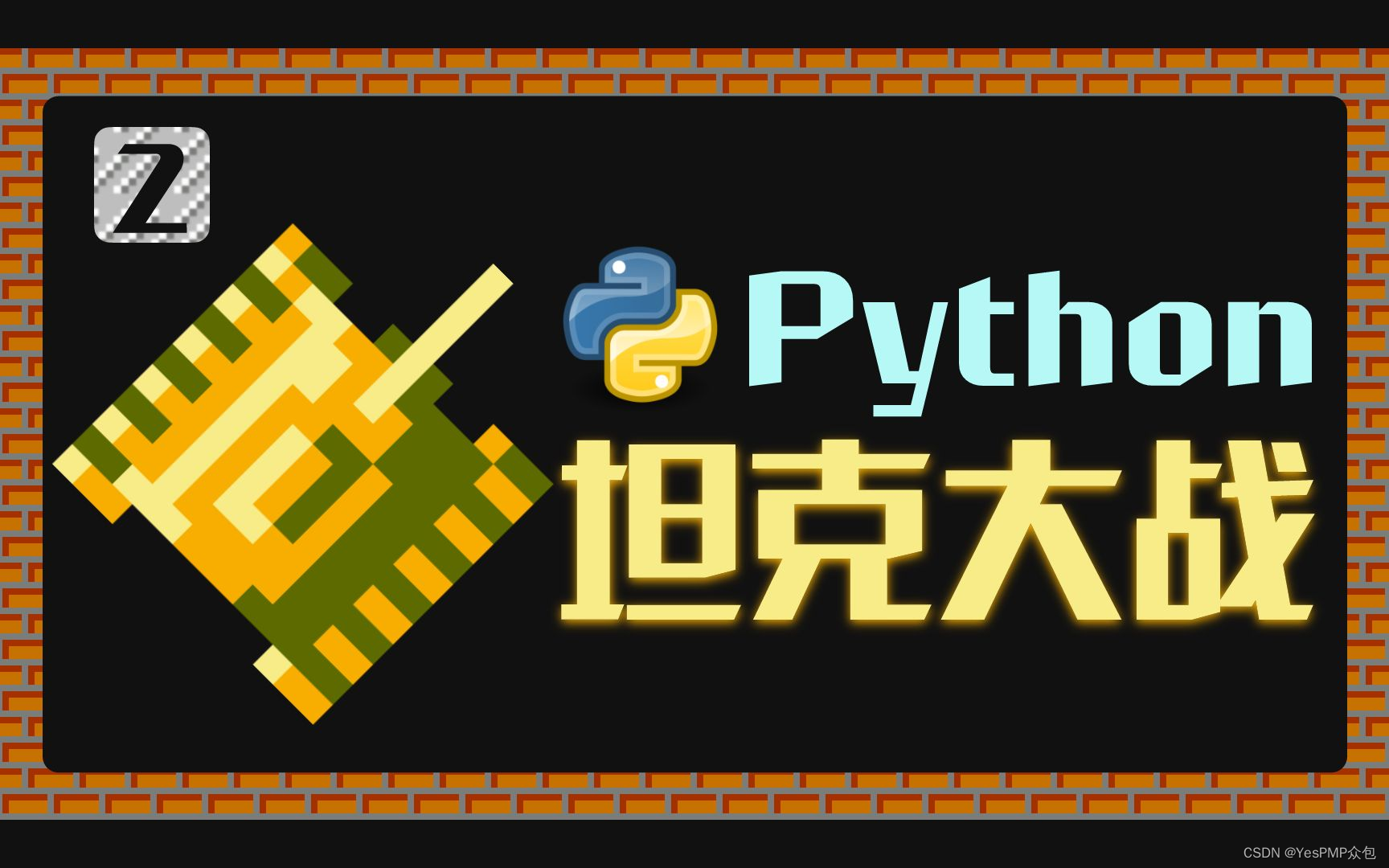
YesPMP探索Python在生活中的应用,助力提升开发效率
Python是一种简单易学、高效强大的编程语言,正变成越来越多人选择的热门技能。学习Python不仅可以提供更多就业机会,还能让自己在职场更加有竞争力,那可以去哪里拓展自己的技能呢? YesPMP平台 为熟练掌握Python语言的程序员提供了…...
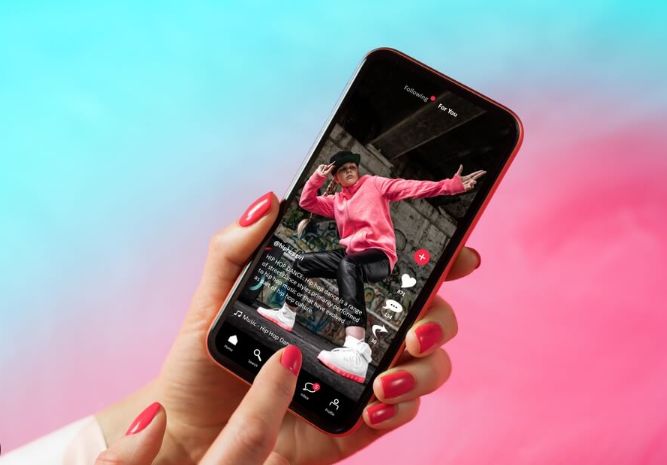
TikTok账号运营:静态住宅IP为什么可以防封?
静态住宅IP代理服务是一种提供稳定、静态IP地址并可隐藏用户真实IP地址的网络代理服务。此类代理服务通常使用高速光纤网络来提供稳定、高速的互联网体验。与动态IP代理相比,静态住宅IP代理的IP地址更稳定,被封的可能性更小,因此更受用户欢迎…...
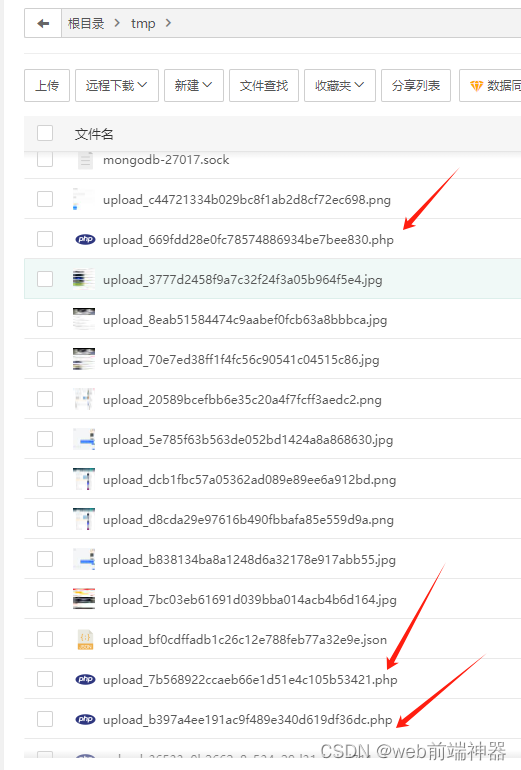
linux系统宝塔服务器temp文件夹里总是被上传病毒php脚本
目录 简介 上传过程 修复上传漏洞 tmp文件夹总是被上传病毒文件如下图: 简介 服务器时不时的会发送短信说你服务器有病毒, 找到了这个tmp文件, 删除了之后又有了。 确实是有很多人就这么无聊, 每天都攻击你的服务器。 找了很久的原因, 网上也提供了一大堆方法,…...
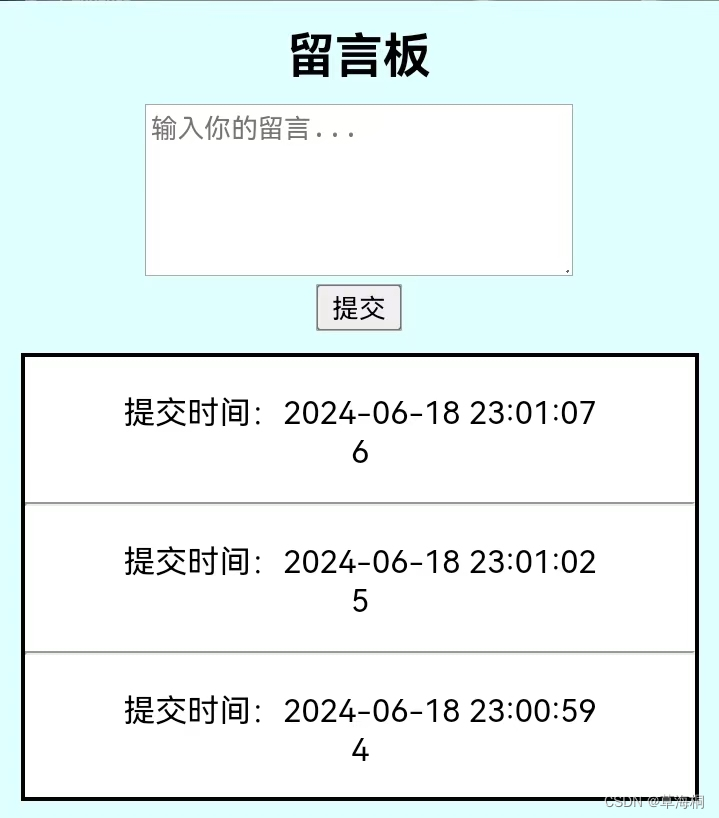
HTML+CSS+PHP实现网页留言板功能(需要创建数据库)
话说前头,我这方面很菜滴。这是我网页作业的一部分。 1.body部分效果展示(不包括footer) 2、代码 2.1 leaving.php(看到的网页) <!DOCTYPE html> <html lang"en"> <head> <met…...

【谷歌】实用的搜索技巧
1、使用正确的谷歌网址 我们知道https://www.google.com是谷歌的网址。但根据国家,用户可能会被重定向到 google.fr(法国)或google.co.in(印度)。 最主要的URL——google.com是为美国用户准备的(或是针对全世界所有用户的唯一URL))。当你在谷歌上搜索时,了解这一点是相…...
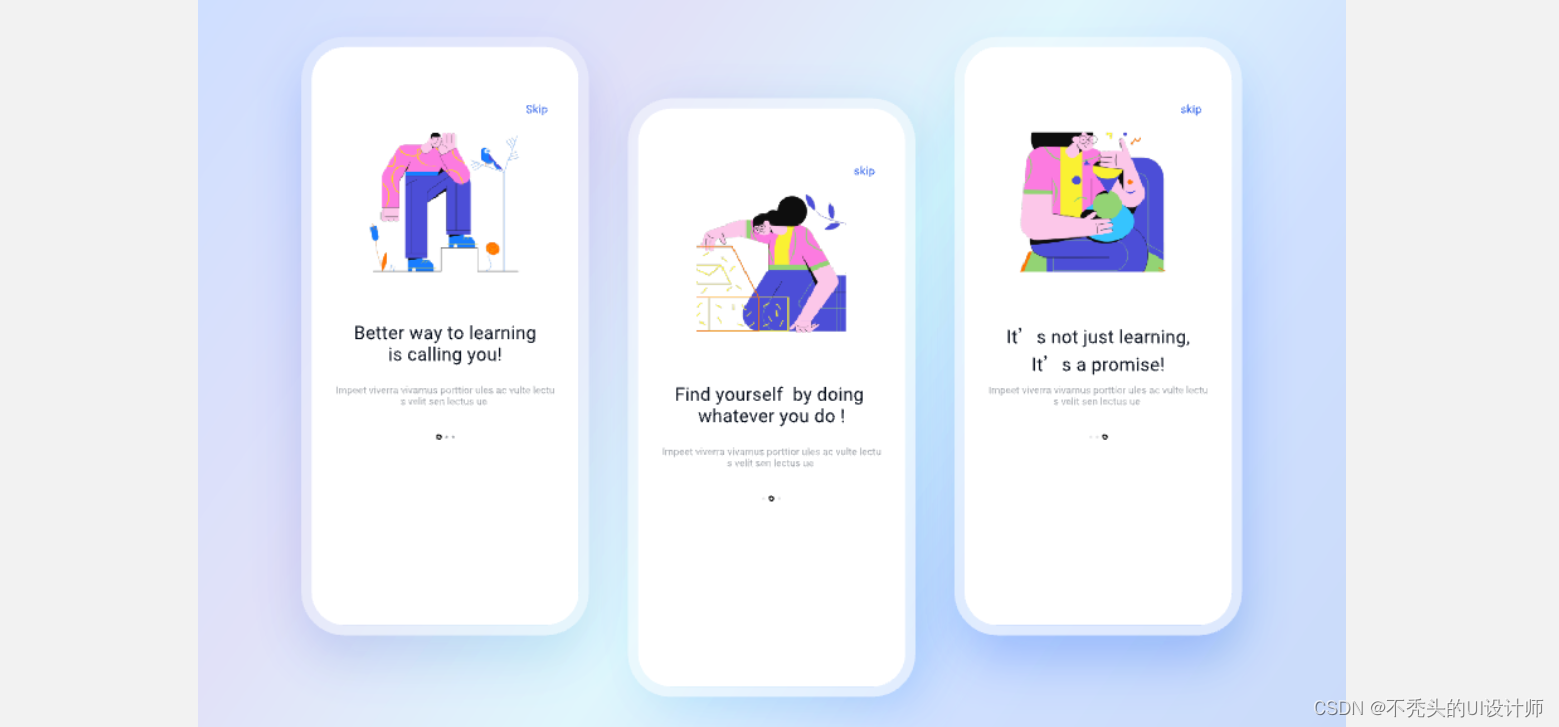
打造完美启动页:关键策略与设计技巧
启动页(Splash Screen)设计是指在应用程序启动时,首先展示给用户的界面设计。这个界面通常在应用加载或初始化期间显示,其主要目的是为用户提供一个视觉缓冲,展示品牌标识,并减少用户在等待过程中的焦虑感。…...

电子书(chm)-加载JS--CS上线
免责声明: 本文仅做技术交流与学习... 目录 cs--web投递 html(js)代码 html生成chm工具--EasyCHM 1-选择powershell 模式 生成 2-选择bitsadmin模式生成 chm反编译成html cs--web投递 cs配置监听器--->攻击---->web投递---> 端口选择没占用的, URL路径到时候会在…...
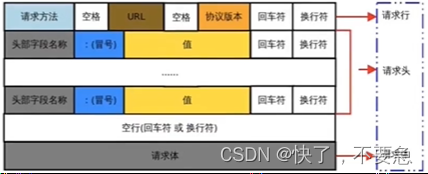
理解HTTP请求格式
HTTP概念 HTTP全称HyperTextTransfer Protocol(超文本传输协议)是一种用于分布式、协作式和超媒体信息系统的应用层协议;HTTP是一个客户端(用户)和服务端(网站)之间请求和响应的标准。 HTTP 协议是以 ASCII 码传输&…...

差分数组汇总
本文涉及知识点 算法与数据结构汇总 差分数组 令 a[i] ∑ j : 0 i v D i f f [ i ] \sum_{j:0}^{i}vDiff[i] ∑j:0ivDiff[i] 如果 vDiff[i1],则a[i1…]全部 如果vDiff[i2]–,则a[i2…]全部–。 令11 < i2 ,则: { a [ i ] 不变&…...
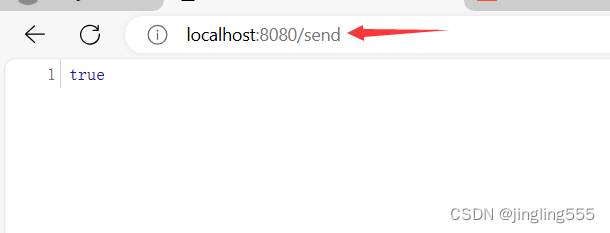
SpringBoot | 实现邮件发送
运行环境: IntelliJ IDEA 2022.2.5 (Ultimate Edition) (注意:idea必须在2021版本以上)JDK17 项目目录: 该项目分为pojo,service,controller,utils四个部分, 在pojo层里面写实体内容(发邮件需要的发件人邮…...
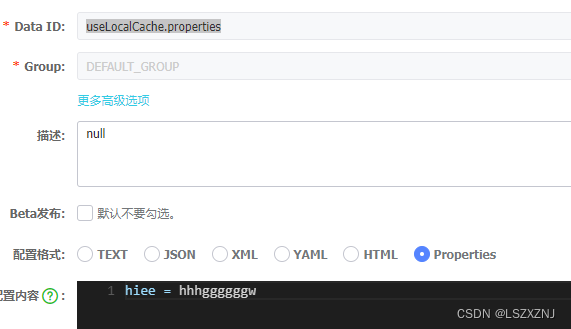
spring boot接入nacos 配置中心
再接入nacos配置中心时,需要确认几点: 1. spring boot 版本 (spring boot 2.x ) 2. nacos 配置中心 服务端 版本 (1.1.4) 3. nacos client 客户端版本 (1.1.4) 方式一 1. 启动 nacos 服务端,这里不做解释 在配置中心中加入几个配置 2. 在…...
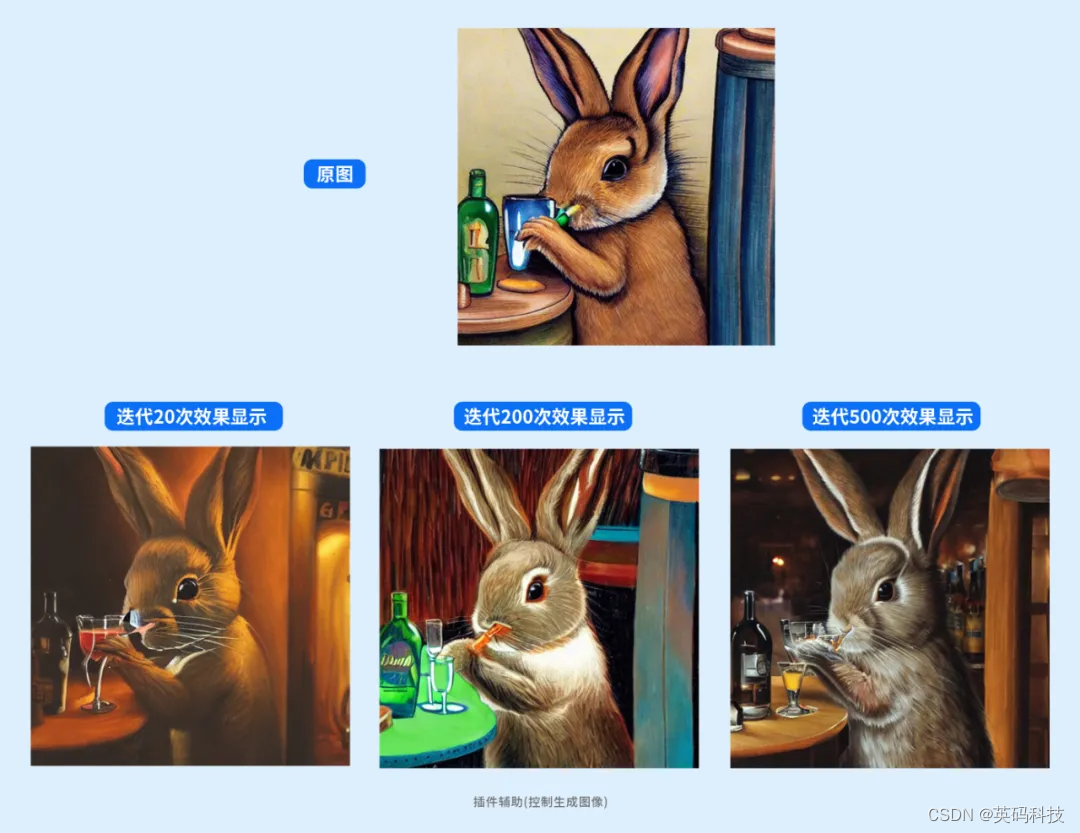
产品应用 | 小盒子跑大模型!英码科技基于算能BM1684X平台实现大模型私有化部署
当前,在人工智能领域,大模型在丰富人工智能应用场景中扮演着重要的角色,经过不断的探索,大模型进入到落地的阶段。而大模型在落地过程中面临两大关键难题:对庞大计算资源的需求和对数据隐私与安全的考量。为应对这些挑…...
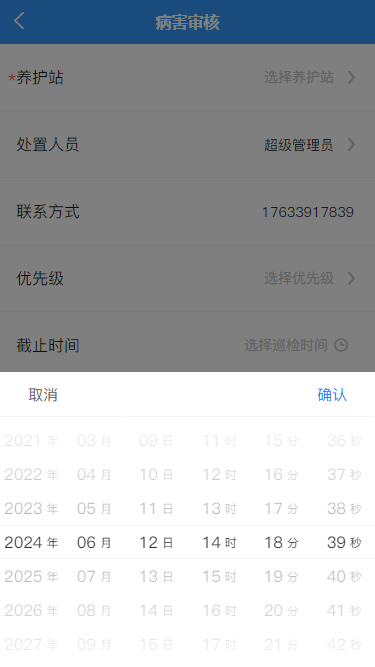
uniapp中u-input点击事件失效
当给u-input设置了disabled/readonly属性后,pc浏览器中点击事件失效,但是app/移动端h5中却仍有效 解决办法 给外边包上一个盒子设置点击事件,给input加上css属性:pointer-events:none pointer-events CSS 属性指定在什…...

[机器学习] 监督学习和无监督学习
监督学习和无监督学习是机器学习的两种主要方法,它们之间有几个关键区别: 1. 定义 监督学习(Supervised Learning): 使用带标签的数据进行训练。数据集包括输入特征和对应的输出标签。目标是学习从输入特征到输出标签…...

使用Python进行自然语言处理:从基础到实战
使用Python进行自然语言处理:从基础到实战 自然语言处理(Natural Language Processing, NLP)是人工智能的重要领域,旨在处理和分析自然语言数据。Python凭借其丰富的库和社区支持,成为NLP的首选编程语言。本文将介绍自然语言处理的基础概念、常用的Python库以及一个实战项…...