LIN通讯
目录
1 PLinApi.h
2 TLINFrameEntry 结构体
3 自定义函数getTLINFrameEntry
4 TLINScheduleSlot 结构体
5 自定义函数 getTLINScheduleSlot
6 自定义LIN_SetScheduleInit函数
7 自定义 LIN_StartSchedule
8 发送函数
9 线程接收函数
1 PLinApi.h
这是官方头文件
///
// PLinApi.h
//
// Definition of the PLIN-API.
//
//
// Principle:
// ~~~~~~~~~~
// The driver supports multiple clients (= Windows or DOS programs
// that communicate with LIN-busses), and multiple LIN-Devices.
// A LIN-Device represents two LIN-Hardware (each channel is a Hardware)
// Multiple 'Clients' can be connected to one or more LIN-Hardware, which
// itself have an interface to a physical LIN-channel of a device.
//
// Features:
// ~~~~~~~~~
// - 1 Client can be connected to multiple Hardware
// - 1 Hardware supports multiple clients
// - When a Client sends a message to a Hardware, the message will not be routed
// to other clients. The response of the Hardware is routed to the connected
// clients depending on the registered Hardware message filter.
// - each Client only receives the messages that pass its acceptance filter
// - each Client has a Receive Queue to buffer received messages
// - hClient: 'Client handle'. This number is used by the driver to
// identify and manage a Client
// - hHw: 'Hardware handle'. This number is used by the driver to
// identify and manage a Hardware
// - all handles are 1-based. 0 = illegal handle
//
// All functions return a value of type TLINError
//
// Authors: K.Wagner / P.Steil
//
// -----------------------------------------------------------------------
// Copyright (C) 2008-2019 by PEAK-System Technik GmbH, Darmstadt/Germany
// -----------------------------------------------------------------------
//
// Last Change: 2019.02.08
///#ifndef __LINAPIH__
#define __LINAPIH__#include <windows.h>#ifdef __cplusplus
extern "C" {
#endif// Handle types
//
typedef BYTE HLINCLIENT; // Client Handle
typedef WORD HLINHW; // Hardware Handle// Invalid Handle values
//
#define INVALID_LIN_HANDLE 0 // Invalid value for all LIN handles (Client, Hardware)// Hardware Types
//
#define LIN_HW_TYPE_USB 1 // LIN USB type // DEPRECATED
#define LIN_HW_TYPE_USB_PRO 1 // PCAN-USB Pro LIN type
#define LIN_HW_TYPE_USB_PRO_FD 2 // PCAN-USB Pro FD LIN type
#define LIN_HW_TYPE_PLIN_USB 3 // PLIN-USB type// Minimum and Maximum values
//
#define LIN_MAX_FRAME_ID 63 // Maximum allowed Frame ID (0x3F)
#define LIN_MAX_SCHEDULES 8 // Maximum allowed Schedules per Hardware
#define LIN_MIN_SCHEDULE_NUMBER 0 // Minimum Schedule number
#define LIN_MAX_SCHEDULE_NUMBER 7 // Maximum Schedule number
#define LIN_MAX_SCHEDULE_SLOTS 256 // Maximum allowed Schedule slots per Hardware
#define LIN_MIN_BAUDRATE 1000 // Minimum LIN Baudrate
#define LIN_MAX_BAUDRATE 20000 // Maximum LIN Baudrate
#define LIN_MAX_NAME_LENGTH 48 // Maximum number of bytes for Name / ID of a Hardware or Client
#define LIN_MAX_USER_DATA 24 // Maximum number of bytes that a user can read/write on a Hardware
#define LIN_MIN_BREAK_LENGTH 13 // Minimum number of bits that can be used as break field in a LIN frame
#define LIN_MAX_BREAK_LENGTH 32 // Maximum number of bits that can be used as break field in a LIN frame
#define LIN_MAX_RCV_QUEUE_COUNT 65535 // Maximum number of LIN frames that can be stored in the reception queue of a client// Frame flags for LIN Frame Entries
//
#define FRAME_FLAG_RESPONSE_ENABLE 0x1 // Slave Enable Publisher Response
#define FRAME_FLAG_SINGLE_SHOT 0x2 // Slave Publisher Single shot
#define FRAME_FLAG_IGNORE_INIT_DATA 0x4 // Ignore InitialData on set frame entry// Error flags for LIN Rcv Msgs
//
#define MSG_ERR_INCONSISTENT_SYNC 0x1 // Error on Synchronization field
#define MSG_ERR_ID_PARITY_BIT0 0x2 // Wrong parity Bit 0
#define MSG_ERR_ID_PARITY_BIT1 0x4 // Wrong parity Bit 1
#define MSG_ERR_SLAVE_NOT_RESPONDING 0x8 // Slave not responding error
#define MSG_ERR_TIMEOUT 0x10 // A timeout was reached
#define MSG_ERR_CHECKSUM 0x20 // Wrong checksum
#define MSG_ERR_GND_SHORT 0x40 // Bus shorted to ground
#define MSG_ERR_VBAT_SHORT 0x80 // Bus shorted to Vbat
#define MSG_ERR_SLOT_DELAY 0x100 // A slot time (delay) was too small
#define MSG_ERR_OTHER_RESPONSE 0x200 // Response was received from other station
#define TLINMsgErrors INT32// Client Parameters (GetClientParam Function)
//
#define clpName 1 // Client Name
#define clpMessagesOnQueue 2 // Unread messages in the Receive Queue
#define clpWindowHandle 3 // Registered windows handle (information purpose)
#define clpConnectedHardware 4 // Handles of the connected Hardware
#define clpTransmittedMessages 5 // Number of transmitted messages
#define clpReceivedMessages 6 // Number of received messages
#define clpReceiveStatusFrames 7 // Status of the property "Status Frames"
#define clpOnReceiveEventHandle 8 // Handle of the Receive event
#define clpOnPluginEventHandle 9 // Handle of the Hardware plug-in event
#define TLINClientParam WORD// Hardware Parameters (GetHardwareParam function)
//
#define hwpName 1 // Hardware / Device Name
#define hwpDeviceNumber 2 // Index of the owner Device
#define hwpChannelNumber 3 // Channel Index on the owner device (0 or 1)
#define hwpConnectedClients 4 // Handles of the connected clients
#define hwpMessageFilter 5 // Message filter
#define hwpBaudrate 6 // Baudrate
#define hwpMode 7 // Master status
#define hwpFirmwareVersion 8 // LIN hardware firmware version (text with the form xx.yy where:// xx = major version. yy = minor version)
#define hwpBufferOverrunCount 9 // Receive Buffer Overrun Counter
#define hwpBossClient 10 // Registered master Client
#define hwpSerialNumber 11 // Serial number of a Hardware
#define hwpVersion 12 // Version of a Hardware
#define hwpType 13 // Type of a Hardware
#define hwpQueueOverrunCount 14 // Receive Queue Buffer Overrun Counter
#define hwpIdNumber 15 // Hardware identification number
#define hwpUserData 16 // User data on a hardware
#define hwpBreakLength 17 // Number of bits used as break field in a LIN frame
#define hwpLinTermination 18 // LIN Termination status
#define hwpFlashMode 19 // Device flash mode for firmware update
#define TLINHardwareParam WORD// Received Message Types
//
#define mstStandard 0 // Standard LIN Message
#define mstBusSleep 1 // Bus Sleep status message
#define mstBusWakeUp 2 // Bus WakeUp status message
#define mstAutobaudrateTimeOut 3 // Auto-baudrate Timeout status message
#define mstAutobaudrateReply 4 // Auto-baudrate Reply status message
#define mstOverrun 5 // Bus overrun status message
#define mstQueueOverrun 6 // Queue overrun status message
#define mstClientQueueOverrun 7 // Client's receive queue overrun status message
#define TLINMsgType BYTE// Schedule Slot Types
//
#define sltUnconditional 0 // Unconditional frame
#define sltEvent 1 // Event frame
#define sltSporadic 2 // Sporadic frame
#define sltMasterRequest 3 // Diagnostic Master Request frame
#define sltSlaveResponse 4 // Diagnostic Slave Response frame
#define TLINSlotType BYTE// Message Direction Types
//
#define dirDisabled 0 // Direction disabled
#define dirPublisher 1 // Direction is Publisher
#define dirSubscriber 2 // Direction is Subscriber
#define dirSubscriberAutoLength 3 // Direction is Subscriber (detect Length)
#define TLINDirection BYTE// Message Checksum Types
//
#define cstCustom 0 // Custom checksum
#define cstClassic 1 // Classic checksum (ver 1.x)
#define cstEnhanced 2 // Enhanced checksum
#define cstAuto 3 // Detect checksum
#define TLINChecksumType BYTE// Hardware Operation Modes
//
#define modNone 0 // Hardware is not initialized
#define modSlave 1 // Hardware working as Slave
#define modMaster 2 // Hardware working as Master
#define TLINHardwareMode BYTE// Hardware Status
//
#define hwsNotInitialized 0 // Hardware is not initialized
#define hwsAutobaudrate 1 // Hardware is detecting the baudrate
#define hwsActive 2 // Hardware (bus) is active
#define hwsSleep 3 // Hardware (bus) is in sleep mode
#define hwsShortGround 6 // Hardware (bus-line) shorted to ground
#define hwsVBatMissing 7 // Hardware (USB adapter) external voltage supply missing
#define TLINHardwareState BYTE// Error Codes
//
#define errOK 0 // No error. Success.
#define errXmtQueueFull 1 // Transmit Queue is full.
#define errIllegalPeriod 2 // Period of time is invalid.
#define errRcvQueueEmpty 3 // Client Receive Queue is empty.
#define errIllegalChecksumType 4 // Checksum type is invalid.
#define errIllegalHardware 5 // Hardware handle is invalid.
#define errIllegalClient 6 // Client handle is invalid.
#define errWrongParameterType 7 // Parameter type is invalid.
#define errWrongParameterValue 8 // Parameter value is invalid.
#define errIllegalDirection 9 // Direction is invalid.
#define errIllegalLength 10 // Length is outside of the valid range.
#define errIllegalBaudrate 11 // Baudrate is outside of the valid range.
#define errIllegalFrameID 12 // ID is outside of the valid range.
#define errBufferInsufficient 13 // Buffer parameter is too small.
#define errIllegalScheduleNo 14 // Scheduler Number is outside of the valid range.
#define errIllegalSlotCount 15 // Slots count is bigger than the actual number of available slots.
#define errIllegalIndex 16 // Array index is outside of the valid range.
#define errIllegalRange 17 // Range of bytes to be updated is invalid.
#define errOutOfResource 1001 // LIN Manager does not have enough resources for the current task.
#define errManagerNotLoaded 1002 // The LIN Device Manager is not running.
#define errManagerNotResponding 1003 // The communication to the LIN Device Manager was interrupted.
#define errMemoryAccess 1004 // A "MemoryAccessViolation" exception occurred within an API method.
#define errNotImplemented 0xFFFE // An API method is not implemented.
#define errUnknown 0xFFFF // An internal error occurred within the LIN Device Manager.
#define TLINError DWORD#pragma pack(push, 8) // These Records are 8-Bytes aligned!// Version Information structure
//
typedef struct { // Size = 8 bytesshort Major; // #0 +0 Major part of a version numbershort Minor; // #1 +2 Minor part of a version numbershort Revision; // #2 +4 Revision part of a version numbershort Build; // #3 +6 Build part of a version number
}TLINVersion;// A LIN Message to be sent
//
typedef struct { // Size = 13 bytesBYTE FrameId; // #0 +0 Frame ID (6 bit) + Parity (2 bit)BYTE Length; // #1 +1 Frame Length (1..8)TLINDirection Direction; // #2 +2 Frame Direction (see Message Direction Types)TLINChecksumType ChecksumType; // #3 +3 Frame Checksum type (see Message Checksum Types)BYTE Data[8]; // #4 +4 Data bytes (0..7)BYTE Checksum; // #5 +12 Frame Checksum
} TLINMsg;// A received LIN Message
//
typedef struct { // Size = 40 bytesTLINMsgType Type; // #0 +0 Frame type (see Received Message Types)BYTE FrameId; // #1 +1 Frame ID (6 bit) + Parity (2 bit)BYTE Length; // #2 +2 Frame Length (1..8)TLINDirection Direction; // #3 +3 Frame Direction (see Message Direction Types)TLINChecksumType ChecksumType; // #4 +4 Frame Checksum type (see Message Checksum Types)BYTE Data[8]; // #5 +5 Data bytes (0..7)BYTE Checksum; // #6 +13 Frame ChecksumTLINMsgErrors ErrorFlags; // #7 +16 Frame error flags (see Error flags for LIN Rcv Msgs)unsigned __int64 TimeStamp; // #8 +24 Timestamp in microsecondsHLINHW hHw; // #9 +32 Handle of the Hardware which received the message
} TLINRcvMsg;// A LIN Frame Entry
//
typedef struct { // Size = 14 bytesBYTE FrameId; // #0 +0 Frame ID (without parity)BYTE Length; // #1 +1 Frame Length (1..8)TLINDirection Direction; // #2 +2 Frame Direction (see Message Direction Types)TLINChecksumType ChecksumType; // #3 +3 Frame Checksum type (see Message Checksum Types)WORD Flags; // #4 +4 Frame flags (see Frame flags for LIN Msgs)BYTE InitialData[8]; // #5 +6 Data bytes (0..7)
} TLINFrameEntry;// A LIN Schedule slot
//
typedef struct { // Size = 20 bytesTLINSlotType Type; // #0 +0 Slot Type (see Schedule Slot Types)WORD Delay; // #1 +2 Slot Delay in MillisecondsBYTE FrameId[8]; // #2 +4 Frame IDs (without parity)BYTE CountResolve; // #3 +12 ID count for sporadic frames// Resolve schedule number for Event framesDWORD Handle; // #4 +16 Slot handle (read-only)
} TLINScheduleSlot;// LIN Status data
//
typedef struct { // Size = 8 bytesTLINHardwareMode Mode; // #0 +0 Node state (see Hardware Operation Modes)TLINHardwareState Status; // #1 +1 Bus state (see Hardware Status)BYTE FreeOnSendQueue; // #2 +2 Count of free places in the Transmit QueueWORD FreeOnSchedulePool; // #3 +4 Free slots in the Schedule pool (see Minimum and Maximum values)WORD ReceiveBufferOverrun; // #4 +6 USB receive buffer overrun counter
} TLINHardwareStatus;#pragma pack(pop)///
// Function prototypes//-----------------------------------------------------------------------------
// LIN_RegisterClient()
// Registers a Client at the LIN Manager. Creates a Client handle and
// allocates the Receive Queue (only one per Client). The hWnd parameter
// can be zero for DOS Box Clients. The Client does not receive any
// messages until LIN_RegisterFrameId() or LIN_SetClientFilter() is called.
//
// Possible DLL interaction errors:
// errManagerNotLoaded, errManagerNotResponding, errMemoryAccess
//
// Possible API errors:
// errWrongParameterValue
//
TLINError __stdcall LIN_RegisterClient (LPSTR strName, // Name of the ClientDWORD hWnd, // Window handle of the Client (only for information purposes)HLINCLIENT *hClient); // Pointer to the Client handle buffer//-----------------------------------------------------------------------------
// LIN_RemoveClient()
// Removes a Client from the Client list of the LIN Manager. Frees all
// resources (receive queues, message counters, etc.). If the Client was
// a Boss-Client for one or more Hardware, the Boss-Client property for
// those Hardware will be set to INVALID_LIN_HANDLE.
//
// Possible DLL interaction errors:
// errManagerNotLoaded, errManagerNotResponding, errMemoryAccess
//
// Possible API errors:
// errWrongParameterValue, errIllegalClient
//
TLINError __stdcall LIN_RemoveClient (HLINCLIENT hClient); // Handle of the Client//-----------------------------------------------------------------------------
// LIN_ConnectClient()
// Connects a Client to a Hardware.
// The Hardware is assigned by its Handle.
//
// Possible DLL interaction errors:
// errManagerNotLoaded, errManagerNotResponding, errMemoryAccess
//
// Possible API errors:
// errWrongParameterValue, errIllegalClient, errIllegalHardware
//
TLINError __stdcall LIN_ConnectClient (HLINCLIENT hClient, // Connect this Client ...HLINHW hHw); // to this Hardware//-----------------------------------------------------------------------------
// LIN_DisconnectClient()
// Disconnects a Client from a Hardware. This means: no more messages
// will be received by this Client from this Hardware.
//
// Possible DLL interaction errors:
// errManagerNotLoaded, errManagerNotResponding, errMemoryAccess
//
// Possible API errors:
// errWrongParameterValue, errIllegalClient, errIllegalHardware
//
TLINError __stdcall LIN_DisconnectClient (HLINCLIENT hClient, // Disconnect this Client ...HLINHW hHw); // from this Hardware.//-----------------------------------------------------------------------------
// LIN_ResetClient()
// Flushes the Receive Queue of the Client and resets its counters.
//
// Possible DLL interaction errors:
// errManagerNotLoaded, errManagerNotResponding, errMemoryAccess
//
// Possible API errors:
// errWrongParameterValue, errIllegalClient
//
TLINError __stdcall LIN_ResetClient (HLINCLIENT hClient); // Handle of the Client//-----------------------------------------------------------------------------
// LIN_SetClientParam()
// Sets a Client parameter to a given value.
//
// Allowed TLINClientParam Parameter
// values in wParam: type: Description:
// ------------------------- ---------- ------------------------------------
// clpReceiveStatusFrames int 0 = Status Frames deactivated,
// otherwise active
//
// Possible DLL interaction errors:
// errManagerNotLoaded, errManagerNotResponding, errMemoryAccess
//
// Possible API errors:
// errWrongParameterType, errWrongParameterValue, errIllegalClient
//
TLINError __stdcall LIN_SetClientParam (HLINCLIENT hClient, // Client HandleTLINClientParam wParam, // TLINClientParam parameter DWORD dwValue); // Parameter value//-----------------------------------------------------------------------------
// LIN_GetClientParam()
// Gets a Client parameter.
//
// Allowed TLINClientParam Parameter
// values in wParam: type: Description:
// ------------------------- ---------- ------------------------------------
// clpName char[] Name of the Client
// clpMessagesOnQueue int Unread messages in the Receive Queue
// clpWindowHandle int Window handle of the Client application
// (can be zero for DOS Box Clients)
// clpConnectedHardware HLINHW[] Array of Hardware Handles connected by a Client
// The first item in the array refers to the
// amount of handles. So [*] = Total handles + 1
// clpTransmittedMessages int Number of transmitted messages
// clpReceivedMessages int Number of received messages
// clpReceiveStatusFrames int 0 = Status Frames deactivated, otherwise active
//
// Possible DLL interaction errors:
// errManagerNotLoaded, errManagerNotResponding, errMemoryAccess
//
// Possible API errors:
// errWrongParameterType, errWrongParameterValue, errIllegalClient,
// errBufferInsufficient
//
TLINError __stdcall LIN_GetClientParam (HLINCLIENT hClient, // Client HandleTLINClientParam wParam, // TLINClientParam parametervoid *pBuff, // Buffer for the parameter valueWORD wBuffSize); // Size of the buffer in bytes//-----------------------------------------------------------------------------
// LIN_SetClientFilter()
// Sets the filter of a Client and modifies the filter of
// the connected Hardware.
//
// Possible DLL interaction errors:
// errManagerNotLoaded, errManagerNotResponding, errMemoryAccess
//
// Possible API errors:
// errWrongParameterValue, errIllegalClient, errIllegalHardware
//
TLINError __stdcall LIN_SetClientFilter (HLINCLIENT hClient, // Set for this ClientHLINHW hHw, // within this Hardwareunsigned __int64 iRcvMask); // this message filter: each bit corresponds // to a Frame ID (0..63). //-----------------------------------------------------------------------------
// LIN_GetClientFilter()
// Gets the filter corresponding to a given Client-Hardware pair.
//
// Possible DLL interaction errors:
// errManagerNotLoaded, errManagerNotResponding, errMemoryAccess
//
// Possible API errors:
// errWrongParameterValue, errIllegalClient, errIllegalHardware
//
TLINError __stdcall LIN_GetClientFilter (HLINCLIENT hClient, // Client HandleHLINHW hHw, // Hardware Handleunsigned __int64 *pRcvMask);// Buffer for the message filter: each bit // corresponds to a Frame ID (0..63) //-----------------------------------------------------------------------------
// LIN_Read()
// Reads the next message/status information from a Client's Receive
// Queue. The message will be written to 'pMsg'.
//
// Possible DLL interaction errors:
// errManagerNotLoaded, errManagerNotResponding, errMemoryAccess
//
// Possible API errors:
// errWrongParameterValue, errIllegalClient, errRcvQueueEmpty
//
TLINError __stdcall LIN_Read (HLINCLIENT hClient, // Client HandleTLINRcvMsg *pMsg); // Buffer for the message//-----------------------------------------------------------------------------
// LIN_ReadMulti()
// Reads several received messages.
// pMsgBuff must be an array of 'iMaxCount' entries (must have at least
// a size of iMaxCount * sizeof(TLINRcvMsg) bytes).
// The size 'iMaxCount' of the array = max. messages that can be received.
// The real number of read messages will be returned in 'pCount'.
//
// Possible DLL interaction errors:
// errManagerNotLoaded, errManagerNotResponding, errMemoryAccess
//
// Possible API errors:
// errWrongParameterValue, errIllegalClient, errRcvQueueEmpty
//
TLINError __stdcall LIN_ReadMulti ( HLINCLIENT hClient, // Client HandleTLINRcvMsg *pMsgBuff, // Buffer for the messagesint iMaxCount, // Maximum number of messages to readint *pCount); // Buffer for the real number of messages read//-----------------------------------------------------------------------------
// LIN_Write()
// The Client 'hClient' transmits a message 'pMsg' to the Hardware 'hHw'.
// The message is written into the Transmit Queue of the Hardware.
//
// Possible DLL interaction errors:
// errManagerNotLoaded, errManagerNotResponding, errMemoryAccess
//
// Possible API errors:
// errWrongParameterValue, errIllegalClient, errIllegalHardware,
// errIllegalDirection, errIllegalLength
//
TLINError __stdcall LIN_Write (HLINCLIENT hClient, // Client HandleHLINHW hHw, // Hardware HandleTLINMsg *pMsg); // Message Buffer to be written//-----------------------------------------------------------------------------
// LIN_InitializeHardware()
// Initializes a Hardware with a given Mode and Baudrate.
// REMARK: If the Hardware was initialized by another Client, the function
// will re-initialize the Hardware. All connected clients will be affected.
// It is the job of the user to manage the setting and/or configuration of
// Hardware, e.g. by using the Boss-Client parameter of the Hardware.
//
// Possible DLL interaction errors:
// errManagerNotLoaded, errManagerNotResponding, errMemoryAccess
//
// Possible API errors:
// errWrongParameterValue, errIllegalClient, errIllegalHardware,
// errIllegalBaudrate
//
TLINError __stdcall LIN_InitializeHardware (HLINCLIENT hClient, // Client Handle HLINHW hHw, // Hardware HandleTLINHardwareMode bMode, // Hardware Mode (see Hardware Operation Modes)WORD wBaudrate); // LIN Baudrate (see LIN_MIN_BAUDRATE and LIN_MAX_BAUDRATE)//-----------------------------------------------------------------------------
// LIN_GetAvailableHardware()
// Gets an array containing the handles of the current Hardware
// available in the system.
// The count of Hardware handles returned in the array is written in
// 'pCount'.
//
// REMARK: To ONLY get the count of available Hardware, call this
// function using 'pBuff' = NULL and wBuffSize = 0.
//
// Possible DLL interaction errors:
// errManagerNotLoaded, errManagerNotResponding, errMemoryAccess
//
// Possible API errors:
// errWrongParameterValue, errBufferInsufficient
//
TLINError __stdcall LIN_GetAvailableHardware(HLINHW *pBuff, // Buffer for the handlesWORD wBuffSize, // Size of the buffer in bytes int *pCount); // Number of Hardware available//-----------------------------------------------------------------------------
// LIN_SetHardwareParam()
// Sets a Hardware parameter to a given value.
//
// Allowed TLINHardwareParam Parameter
// values in wParam: type: Description:
// ------------------------- ---------- -----------------------------------
// hwpMessageFilter unsigned Hardware message filter. Each bit
// _int64 corresponds to a Frame ID (0..63)
// hwpBossClient HLINCLIENT Handle of the new Boss-Client
// hwpIdNumber int Identification number for a hardware
// hwpUserData BYTE[] User data to write on a hardware. See LIN_MAX_USER_DATA
//
// Possible DLL interaction errors:
// errManagerNotLoaded, errManagerNotResponding, errMemoryAccess
//
// Possible API errors:
// errWrongParameterType, errWrongParameterValue, errIllegalClient,
// errIllegalHardware
//
TLINError __stdcall LIN_SetHardwareParam (HLINCLIENT hClient, // Client HandleHLINHW hHw, // Hardware HandleTLINHardwareParam wParam, // TLINHardwareParam parametervoid *pBuff, // Buffer for the parameter valueWORD wBuffSize); // Size of the buffer//-----------------------------------------------------------------------------
// LIN_GetHardwareParam()
// Gets a Hardware parameter.
//
// Allowed TLINHardwareParam Parameter
// values in wParam: type: Description:
// ------------------------- ---------- -----------------------------------
// hwpName char[] Name of the Hardware. See LIN_MAX_NAME_LENGTH
// hwpDeviceNumber int Index of the Device owner of the Hardware
// hwpChannelNumber int Channel Index of the Hardware on the owner device
// hwpConnectedClients BYTE[*] Array of Client Handles conencted to a Hardware
// The first item in the array refers to the
// amount of handles. So [*] = Total handles + 1
// hwpMessageFilter unsigned Configured message filter. Each bit corresponds
// _int64 to a Frame ID (0..63)
// hwpBaudrate int Configured baudrate
// hwpMode int 0 = Slave, otehrwise Master
// hwpFirmwareVersion TLINVersion A TLINVersion structure containing the Firmware Version
// hwpBufferOverrunCount int Receive Buffer Overrun Counter
// hwpBossClient HLINCLIENT Handle of the current Boss-Client
// hwpSerialNumber int Serial number of the Hardware
// hwpVersion int Version of the Hardware
// hwpType int Type of the Hardware
// hwpQueueOverrunCount int Receive Queue Buffer Overrun Counter
// hwpIdNumber int Identification number for a hardware
// hwpUserData BYTE[] User data saved on the hardware. See LIN_MAX_USER_DATA
//
// Possible DLL interaction errors:
// errManagerNotLoaded, errManagerNotResponding, errMemoryAccess
//
// Possible API errors:
// errWrongParameterType, errWrongParameterValue, errIllegalHardware,
// errBufferInsufficient
//
TLINError __stdcall LIN_GetHardwareParam (HLINHW hHw, // Hardware HandleTLINHardwareParam wParam, // TLINHardwareParam parametervoid *pBuff, // Buffer for the parameter valueWORD wBuffSize); // Size of the buffer//-----------------------------------------------------------------------------
// LIN_ResetHardware()
// Flushes the queues of the Hardware and resets its counters.
//
// Possible DLL interaction errors:
// errManagerNotLoaded, errManagerNotResponding, errMemoryAccess
//
// Possible API errors:
// errWrongParameterValue, errIllegalClient, errIllegalHardware
//
TLINError __stdcall LIN_ResetHardware (HLINCLIENT hClient, // Client HandleHLINHW hHw); // Hardware Handle//-----------------------------------------------------------------------------
// LIN_ResetHardwareConfig()
// Deletes the current configuration of the Hardware and sets its defaults.
// The Client 'hClient' must be registered and connected to the Hardware to
// be accessed.
//
// Possible DLL interaction errors:
// errManagerNotLoaded, errManagerNotResponding, errMemoryAccess
//
// Possible API errors:
// errWrongParameterValue, errIllegalClient, errIllegalHardware
//
TLINError __stdcall LIN_ResetHardwareConfig (HLINCLIENT hClient, // Client HandleHLINHW hHw); // Hardware handle//-----------------------------------------------------------------------------
// LIN_IdentifyHardware()
// Phisically identifies a LIN Hardware (a channel on a LIN Device) by
// blinking its associated LED.
//
// Possible DLL interaction errors:
// errManagerNotLoaded, errManagerNotResponding, errMemoryAccess
//
// Possible API errors:
// errWrongParameterValue, errIllegalHardware
//
TLINError __stdcall LIN_IdentifyHardware (HLINHW hHw); // Hardware Handle//-----------------------------------------------------------------------------
// LIN_RegisterFrameId()
// Modifies the filter of a Client and, eventually, the filter of the
// connected Hardware. The messages with FrameID 'bFromFrameId' to
// 'bToFrameId' will be received.
//
// Possible DLL interaction errors:
// errManagerNotLoaded, errManagerNotResponding, errMemoryAccess
//
// Possible API errors:
// errWrongParameterValue, errIllegalClient, errIllegalHardware,
// errIllegalFrameID
//
TLINError __stdcall LIN_RegisterFrameId (HLINCLIENT hClient, // Client HandleHLINHW hHw, // Hardware HandleBYTE bFromFrameId, // First ID of the frame rangeBYTE bToFrameId); // Last ID of the frame range//-----------------------------------------------------------------------------
// LIN_SetFrameEntry()
// Configures a LIN Frame in a given Hardware. The Client 'hClient' must
// be registered and connected to the Hardware to be accessed.
//
// Possible DLL interaction errors:
// errManagerNotLoaded, errManagerNotResponding, errMemoryAccess
//
// Possible API errors:
// errWrongParameterValue, errIllegalClient, errIllegalHardware,
// errIllegalFrameID, errIllegalLength
//
TLINError __stdcall LIN_SetFrameEntry (HLINCLIENT hClient, // Client HandleHLINHW hHw, // Hardware HandleTLINFrameEntry *pFrameEntry); // Frame entry buffer//-----------------------------------------------------------------------------
// LIN_GetFrameEntry()
// Gets the configuration of a LIN Frame from a given Hardware.
// The 'pFrameEntry.FrameId' must be set to the ID of the frame, whose
// configuration should be returned.
//
// Possible DLL interaction errors:
// errManagerNotLoaded, errManagerNotResponding, errMemoryAccess
//
// Possible API errors:
// errWrongParameterValue, errIllegalHardware, errIllegalFrameID
//
TLINError __stdcall LIN_GetFrameEntry (HLINHW hHw, // Hardware HandleTLINFrameEntry *pFrameEntry); // Frame Entry buffer//-----------------------------------------------------------------------------
// LIN_UpdateByteArray()
// Updates the data of a LIN Frame for a given Hardware. The Client
// 'hClient' must be registered and connected to the Hardware to be
// accessed. 'pData' must have at least a size of 'bLen'.
//
// Possible DLL interaction errors:
// errManagerNotLoaded, errManagerNotResponding, errMemoryAccess
//
// Possible API errors:
// errWrongParameterValue, errIllegalClient, errIllegalHardware,
// errIllegalFrameID, errIllegalLength, errIllegalIndex,
// errIllegalRange
//
TLINError __stdcall LIN_UpdateByteArray (HLINCLIENT hClient, // Client handleHLINHW hHw, // Hardware HandleBYTE bFrameId, // Frame IDBYTE bIndex, // Index where the update data Starts (0..7)BYTE bLen, // Count of Data bytes to be updated.BYTE *pData); // Data buffer//-----------------------------------------------------------------------------
// LIN_StartKeepAlive()
// Sets the Frame 'bFrameId' as Keep-Alive frame for the given Hardware and
// starts to send it every 'wPeriod' Milliseconds. The Client 'hClient' must
// be registered and connected to the Hardware to be accessed.
//
// Possible DLL interaction errors:
// errManagerNotLoaded, errManagerNotResponding, errMemoryAccess
//
// Possible API errors:
// errWrongParameterValue, errIllegalClient, errIllegalHardware,
// errIllegalFrameID
//
TLINError __stdcall LIN_StartKeepAlive ( HLINCLIENT hClient, // Client HandleHLINHW hHw, // Hardware HandleBYTE bFrameId, // ID of the Keep-Alive FrameWORD wPeriod); // Keep-Alive Interval in Milliseconds//-----------------------------------------------------------------------------
// LIN_SuspendKeepAlive()
// Suspends the sending of a Keep-Alive frame in the given Hardware.
// The Client 'hClient' must be registered and connected to the Hardware
// to be accessed.
//
// Possible DLL interaction errors:
// errManagerNotLoaded, errManagerNotResponding, errMemoryAccess
//
// Possible API errors:
// errWrongParameterValue, errIllegalClient, errIllegalHardware
//
TLINError __stdcall LIN_SuspendKeepAlive (HLINCLIENT hClient, // Client HandleHLINHW hHw); // Hardware Handle//-----------------------------------------------------------------------------
// LIN_ResumeKeepAlive()
// Resumes the sending of a KeepAlive frame in the given Hardware.
// The Client 'hClient' must be registered and connected to the Hardware
// to be accessed.
//
// Possible DLL interaction errors:
// errManagerNotLoaded, errManagerNotResponding, errMemoryAccess
//
// Possible API errors:
// errWrongParameterValue, errIllegalClient, errIllegalHardware
//
TLINError __stdcall LIN_ResumeKeepAlive (HLINCLIENT hClient, // Client HandleHLINHW hHw); // Hardware Handle//-----------------------------------------------------------------------------
// LIN_SetSchedule()
// Configures the slots of a Schedule in a given Hardware. The Client
// 'hClient' must be registered and connected to the Hardware to be
// accessed. The Slot handles will be returned in the parameter
// "pSchedule" (Slots buffer), when this function successfully completes.
//
// Possible DLL interaction errors:
// errManagerNotLoaded, errManagerNotResponding, errMemoryAccess
//
// Possible API errors:
// errWrongParameterValue, errIllegalClient, errIllegalHardware,
// errIllegalScheduleNo, errIllegalSlotCount
//
TLINError __stdcall LIN_SetSchedule (HLINCLIENT hClient, // Client HandleHLINHW hHw, // Hardware Handleint iScheduleNumber, // Schedule number (see LIN_MIN_SCHEDULE_NUMBER // and LIN_MAX_SCHEDULE_NUMBER)TLINScheduleSlot *pSchedule, // Slots bufferint iSlotCount); // Count of Slots in the slots buffer//-----------------------------------------------------------------------------
// LIN_GetSchedule()
// Gets the slots of a Schedule from a given Hardware. The count of slots
// returned in the array is written in 'pSlotCount'.
//
// REMARK: To ONLY get the count of slots contained in the given Schedule,
// call this function using 'pScheduleBuff' = NULL and iMaxSlotCount = 0.
//
// Possible DLL interaction errors:
// errManagerNotLoaded, errManagerNotResponding, errMemoryAccess
//
// Possible API errors:
// errWrongParameterValue, errIllegalHardware, errIllegalScheduleNo,
// errIllegalSlotCount
//
TLINError __stdcall LIN_GetSchedule (HLINHW hHw, // Hardware Handleint iScheduleNumber, // Schedule Number (see LIN_MIN_SCHEDULE_NUMBER // and LIN_MAX_SCHEDULE_NUMBER)TLINScheduleSlot *pScheduleBuff, // Slots Buffer.int iMaxSlotCount, // Maximum number of slots to read.int *pSlotCount); // Real number of slots read.//-----------------------------------------------------------------------------
// LIN_DeleteSchedule()
// Removes all slots contained by a Schedule of a given Hardware. The
// Client 'hClient' must be registered and connected to the Hardware to
// be accessed.
//
// Possible DLL interaction errors:
// errManagerNotLoaded, errManagerNotResponding, errMemoryAccess
//
// Possible API errors:
// errWrongParameterValue, errIllegalClient, errIllegalHardware,
// errIllegalScheduleNo
//
TLINError __stdcall LIN_DeleteSchedule (HLINCLIENT hClient, // Client HandleHLINHW hHw, // Hardware Handleint iScheduleNumber); // Schedule Number (see LIN_MIN_SCHEDULE_NUMBER// and LIN_MAX_SCHEDULE_NUMBER)//-----------------------------------------------------------------------------
// LIN_SetScheduleBreakPoint()
// Sets a 'breakpoint' on a slot from a Schedule in a given Hardware. The
// Client 'hClient' must be registered and connected to the Hardware to
// be accessed.
//
// REMARK: Giving 'dwHandle' a value of 0 ('NULL'), causes the deletion of
// the breakpoint.
//
// Possible DLL interaction errors:
// errManagerNotLoaded, errManagerNotResponding, errMemoryAccess
//
// Possible API errors:
// errWrongParameterValue, errIllegalClient, errIllegalHardware
//
TLINError __stdcall LIN_SetScheduleBreakPoint (HLINCLIENT hClient, // Client HandleHLINHW hHw, // Hardware Handleint iBreakPointNumber, // Breakpoint Number (0 or 1)DWORD dwHandle); // Slot Handle//-----------------------------------------------------------------------------
// LIN_StartSchedule()
// Activates a Schedule in a given Hardware. The Client 'hClient' must
// be registered and connected to the Hardware to be accessed.
//
// Possible DLL interaction errors:
// errManagerNotLoaded, errManagerNotResponding, errMemoryAccess
//
// Possible API errors:
// errWrongParameterValue, errIllegalClient, errIllegalHardware,
// errIllegalScheduleNo
//
TLINError __stdcall LIN_StartSchedule (HLINCLIENT hClient, // Client HandleHLINHW hHw, // Hardware Handleint iScheduleNumber); // Schedule Number (see LIN_MIN_SCHEDULE_NUMBER // and LIN_MAX_SCHEDULE_NUMBER)//-----------------------------------------------------------------------------
// LIN_SuspendSchedule()
// Suspends an active Schedule in a given Hardware. The Client 'hClient'
// must be registered and connected to the Hardware to be accessed.
//
// Possible DLL interaction errors:
// errManagerNotLoaded, errManagerNotResponding, errMemoryAccess
//
// Possible API errors:
// errWrongParameterValue, errIllegalClient, errIllegalHardware
//
TLINError __stdcall LIN_SuspendSchedule (HLINCLIENT hClient, // Client HandleHLINHW hHw); // Hardware Handle//-----------------------------------------------------------------------------
// LIN_ResumeSchedule()
// Restarts a configured Schedule in a given Hardware. The Client 'hClient'
// must be registered and connected to the Hardware to be accessed.
//
// Possible DLL interaction errors:
// errManagerNotLoaded, errManagerNotResponding, errMemoryAccess
//
// Possible API errors:
// errWrongParameterValue, errIllegalClient, errIllegalHardware
//
TLINError __stdcall LIN_ResumeSchedule (HLINCLIENT hClient, // Client HandleHLINHW hHw); // Hardware Handle//-----------------------------------------------------------------------------
// LIN_XmtWakeUp()
// Sends a wake-up impulse (single data byte 0xF0). The Client 'hClient'
// must be registered and connected to the Hardware to be accessed.
//
// Remark: Only in Slave-mode. After sending a wake-up impulse a time
// of 150 milliseconds is used as timeout.
//
// Possible DLL interaction errors:
// errManagerNotLoaded, errManagerNotResponding, errMemoryAccess
//
// Possible API errors:
// errWrongParameterValue, errIllegalClient, errIllegalHardware
//
TLINError __stdcall LIN_XmtWakeUp (HLINCLIENT hClient, // Client HandleHLINHW hHw); // Hardware Handle//-----------------------------------------------------------------------------
// LIN_XmtDynamicWakeUp()
// Sends a wake-up impulse (single data byte 0xF0) and specify a custom
// bus-sleep timeout, in milliseconds. The Client 'hClient'
// must be registered and connected to the Hardware to be accessed.
//
// Remark: Only in Slave-mode. The bus-sleep timeout is set to its default,
// 150 milliseconds, after the custom timeout is exhausted.
//
// Possible DLL interaction errors:
// errManagerNotLoaded, errManagerNotResponse, errMemoryAccess
//
// Possible API errors:
// errWrongParameterValue, errIllegalClient, errIllegalHardware
//
TLINError __stdcall LIN_XmtDynamicWakeUp (HLINCLIENT hClient, // Client HandleHLINHW hHw, // Hardware HandleWORD wTimeOut); // Bus-sleep timeout//-----------------------------------------------------------------------------
// LIN_StartAutoBaud()
// Starts a process to detect the Baud rate of the LIN bus that is
// connected to the indicated Hardware.
// The Client 'hClient' must be registered and connected to the Hardware
// to be accessed. The Hardware must be not initialized in order
// to do an Auto-baudrate procedure.
//
// Possible DLL interaction errors:
// errManagerNotLoaded, errManagerNotResponding, errMemoryAccess
//
// Possible API errors:
// errWrongParameterValue, errIllegalClient, errIllegalHardware
//
TLINError __stdcall LIN_StartAutoBaud (HLINCLIENT hClient, // Client HandleHLINHW hHw, // Hardware HandleWORD wTimeOut); // Auto-baudrate Timeout in Milliseconds//-----------------------------------------------------------------------------
// LIN_GetStatus()
// Retrieves current status information from the given Hardware.
//
// Possible DLL interaction errors:
// errManagerNotLoaded, errManagerNotResponding, errMemoryAccess
//
// Possible API errors:
// errWrongParameterValue, errIllegalHardware
//
TLINError __stdcall LIN_GetStatus (HLINHW hHw, // Hardware handleTLINHardwareStatus *pStatusBuff); // Status data buffer//-----------------------------------------------------------------------------
// LIN_CalculateChecksum()
// Calculates the checksum of a LIN Message and writes it into the
// 'Checksum' field of 'pMsg'.
//
// Possible DLL interaction errors:
// errManagerNotLoaded, errManagerNotResponding, errMemoryAccess
//
// Possible API errors:
// errWrongParameterValue, errIllegalLength
//
TLINError __stdcall LIN_CalculateChecksum (TLINMsg *pMsg); // Message buffer//-----------------------------------------------------------------------------
// LIN_GetVersion()
// Returns a TLINVersion structure containing the PLIN-API DLL version.
//
// Possible DLL interaction errors:
// errManagerNotLoaded, errManagerNotResponding, errMemoryAccess
//
// Possible API errors:
// errWrongParameterValue
//
TLINError __stdcall LIN_GetVersion ( TLINVersion *pVerBuff // Version buffer
);//-----------------------------------------------------------------------------
// LIN_GetVersionInfo()
// Returns a string containing Copyright information.
//
// Possible DLL interaction errors:
// errManagerNotLoaded, errManagerNotResponding, errMemoryAccess
//
// Possible API errors:
// errWrongParameterValue
//
TLINError __stdcall LIN_GetVersionInfo (LPSTR strTextBuff, // String bufferWORD wBuffSize); // Size in bytes of the buffer//-----------------------------------------------------------------------------
// LIN_GetErrorText()
// Converts the error code 'dwError' to a text containing an error
// description in the language given as parameter (when available).
//
// Possible DLL interaction errors:
// errManagerNotLoaded, errManagerNotResponding, errMemoryAccess
//
// Possible API errors:
// errWrongParameterValue, errBufferInsufficient
//
TLINError __stdcall LIN_GetErrorText (TLINError dwError, // A TLINError code BYTE bLanguage, // Indicates a "Primary language ID"LPSTR strTextBuff, // Error string bufferWORD wBuffSize); // Buffer size in bytes//-----------------------------------------------------------------------------
// LIN_GetPID()
// Gets the 'FrameId with Parity' corresponding to the given
// 'pFrameId' and writes the result on it.
//
// Possible DLL interaction errors:
// errManagerNotLoaded, errManagerNotResponding, errMemoryAccess
//
// Possible API errors:
// errWrongParameterValue, errIllegalFrameID
//
TLINError __stdcall LIN_GetPID(BYTE *pFrameId); // Frame ID (0..LIN_MAX_FRAME_ID)//-----------------------------------------------------------------------------
// LIN_GetTargetTime()
// Gets the system time used by the LIN-USB adapter.
//
// Possible DLL interaction errors:
// errManagerNotLoaded, errManagerNotResponding, errMemoryAccess
//
// Possible API errors:
// errWrongParameterValue, errIllegalHardware
//
TLINError __stdcall LIN_GetTargetTime (HLINHW hHw, // Hardware Handleunsigned __int64 *pTargetTime); // TargetTime buffer//-----------------------------------------------------------------------------
// LIN_SetResponseRemap()
// Sets the Response Remap of a LIN Slave
//
// Possible DLL interaction errors:
// errManagerNotLoaded, errManagerNotResponding, errMemoryAccess
//
// Possible API errors:
// errWrongParameterValue, errIllegalFrameID, errIllegalClient, errIllegalHardware,
// errMemoryAccess
//
TLINError __stdcall LIN_SetResponseRemap (HLINCLIENT hClient, // Client HandleHLINHW hHw, // Hardware HandleBYTE *pRemapTab); // Remap Response buffer//-----------------------------------------------------------------------------
// LIN_GetResponseRemap()
// Gets the Response Remap of a LIN Slave
//
// REMARK: The Buffer must be at least 64 bytes length
// the breakpoint.
//
// Possible DLL interaction errors:
// errManagerNotLoaded, errManagerNotResponding, errMemoryAccess
//
// Possible API errors:
// errWrongParameterValue, errIllegalHardware, errMemoryAccess
//
TLINError __stdcall LIN_GetResponseRemap (HLINHW hHw, // Hardware HandleBYTE *pRemapTab); // Remap Response buffer//-----------------------------------------------------------------------------
// LIN_GetSystemTime()
// Gets the current system time. The system time is returned by
// Windows as the elapsed number of microseconds since system start.
//
// Possible DLL interaction errors:
// errMemoryAccess
//
// Possible API errors:
// errWrongParameterValue
//
TLINError __stdcall LIN_GetSystemTime(unsigned __int64 *pSystemTime);#ifdef __cplusplus
}
#endif
#endif
2 TLINFrameEntry 结构体
// A LIN Frame Entry
//
typedef struct { // Size = 14 bytesBYTE FrameId; // #0 +0 Frame ID (without parity)BYTE Length; // #1 +1 Frame Length (1..8)TLINDirection Direction; // #2 +2 Frame Direction (see Message Direction Types)TLINChecksumType ChecksumType; // #3 +3 Frame Checksum type (see Message Checksum Types)WORD Flags; // #4 +4 Frame flags (see Frame flags for LIN Msgs)BYTE InitialData[8]; // #5 +6 Data bytes (0..7)
} TLINFrameEntry;
3 自定义函数getTLINFrameEntry
目的:为了获取TLINFrameEntry结构体
TLINFrameEntry PLinApiControl::getTLINFrameEntry(BYTE ChecksumType, BYTE Direction, int FrameId, int Length, int Flags, BYTE *InitialData)
{//关于Flags的解释见下图,一般请将这个数值设置成1。//另外如果将这个值设置成3=1+2,表示同时设置FRAME_FLAG_RESPONSE_ENABLE和FRAME_FLAG_SINGLE_SHOT的flags。TLINFrameEntry pFrameEntry;pFrameEntry.ChecksumType = ChecksumType;pFrameEntry.Direction = Direction;pFrameEntry.FrameId = FrameId;pFrameEntry.Length = 8;pFrameEntry.Flags = Flags;memset(pFrameEntry.InitialData, DEFAULT_DATA, 8);if(Length > 8)Length = 8;for(int i = 0; i < Length; i++){pFrameEntry.InitialData[i] = InitialData[i];}return pFrameEntry;
}
4 TLINScheduleSlot 结构体
// A LIN Schedule slot
//
typedef struct { // Size = 20 bytesTLINSlotType Type; // #0 +0 Slot Type (see Schedule Slot Types)WORD Delay; // #1 +2 Slot Delay in MillisecondsBYTE FrameId[8]; // #2 +4 Frame IDs (without parity)BYTE CountResolve; // #3 +12 ID count for sporadic frames// Resolve schedule number for Event framesDWORD Handle; // #4 +16 Slot handle (read-only)
} TLINScheduleSlot;
5 自定义函数 getTLINScheduleSlot
目的:为了获取TLINScheduleSlot 结构体
void PLinApiControl::getTLINScheduleSlot(TLINScheduleSlot *pSchedule, int delay, int ID)
{//TLINScheduleSlot Delay 0 一直发送 -1 只发送一次pSchedule->Delay = delay;pSchedule->CountResolve = 1;pSchedule->FrameId[0] = ID;pSchedule->FrameId[1] = 0x00;pSchedule->FrameId[2] = 0x00;pSchedule->FrameId[3] = 0x00;pSchedule->FrameId[4] = 0x00;pSchedule->FrameId[5] = 0x00;pSchedule->FrameId[6] = 0x00;pSchedule->FrameId[7] = 0x00;pSchedule->Type = sltUnconditional;}
6 自定义LIN_SetScheduleInit函数
目的:调度表初始化
bool PLinApiControl::LIN_SetScheduleInit()
{int ret;BYTE CtrCmd[8] = {0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00};TLINFrameEntry pFrameEntry = getTLINFrameEntry(cstEnhanced, dirPublisher, ID_SEND, 8, 1, CtrCmd);ret = pSet->m_objPLinApi->SetFrameEntry(pSet->m_hClient, pSet->m_hHw, &pFrameEntry);qDebug()<<"SetFrameEntry ret" << ret;if(ret != errOK)return false;int iSlotCount = 2;TLINScheduleSlot pSchedule[2];//pSchedule_readVal//TLINScheduleSlot Delay 0 一直发送 -1 只发送一次getTLINScheduleSlot(&pSchedule[0], 100, ID_RECV);getTLINScheduleSlot(&pSchedule[1], 50, ID_SEND);ret = pSet->m_objPLinApi->SetSchedule(pSet->m_hClient, pSet->m_hHw, iScheduleNumber, pSchedule, iSlotCount);qDebug()<<"SetSchedule0 ret" << ret;if(ret != errOK)return false;return true;
}
7 自定义 LIN_StartSchedule
目的:开启调度表
void PLinApiControl::LIN_StartSchedule()
{int ret;ret = pSet->m_objPLinApi->StartSchedule(pSet->m_hClient, pSet->m_hHw, iScheduleNumber);qDebug()<<"LIN_StartSchedule ret" << ret;
}
8 发送函数
没有使用调度表的发送函数
/*** 上位机做主机时* 主机写 检验模式增强 帧方向为发布* 主机读 检验模式增强 帧方向为订阅* 上位机做从机时* 回应主机读 帧方向为发布**/
struct LinFrame
{LinFrame(){}LinFrame(int _fid, int len, TLINChecksumType check_type, TLINDirection dir, BYTE *data){m_nID = _fid;m_nLength = len ;m_nChecksumType = check_type;m_bDirection = dir;memset(Data, DEFAULT_DATA, 8);if(len > 8)len = 8;if(data != nullptr)memcpy_s(Data, len, data, len);}// The CGlobalFrameTable holding this framevoid * m_pParent;// LIN-Frame Identifierint m_nID;// Datalength of the LIN-Frameint m_nLength;// Type of the Checksum CalculationTLINChecksumType m_nChecksumType;// Direction of the LIN-FrameTLINDirection m_bDirection;// Protected LIN-Frame m_nIDint m_nProtectedID;BYTE Data[8]; // #4 +4 Data bytes (0..7)BYTE Checksum; // #5 +12 Frame Checksum};
bool PLinApiControl::LIN_Write(LinFrame sLinFrame)
{if(!pSet->m_objPLinApi->bLoadPLinApi())return false;if(!pSet->bConnectHard())return false;TLINMsg pMsg;BYTE pid = sLinFrame.m_nID;pSet->m_objPLinApi->GetPID(&pid);// Create a new LIN frame message and copy the data.pMsg.FrameId = (BYTE) pid;pMsg.Direction = (TLINDirection) sLinFrame.m_bDirection;pMsg.ChecksumType = (TLINChecksumType)sLinFrame.m_nChecksumType;pMsg.Length = 8;//(BYTE) sLinFrame.m_nLength;// Fill data arraypMsg.Data[0] = sLinFrame.Data[0];pMsg.Data[1] = sLinFrame.Data[1];pMsg.Data[2] = sLinFrame.Data[2];pMsg.Data[3] = sLinFrame.Data[3];pMsg.Data[4] = sLinFrame.Data[4];pMsg.Data[5] = sLinFrame.Data[5];pMsg.Data[6] = sLinFrame.Data[6];pMsg.Data[7] = sLinFrame.Data[7];int err = 1;// Check if the hardware is initialize as masterif (pSet->m_HwMode == modMaster){// Calculate the checksum contained with the// checksum type that set some line before.pSet->m_objPLinApi->CalculateChecksum(&pMsg);// Try to send the LIN frame message with LIN_Write.err = pSet->m_objPLinApi->Write(pSet->m_hClient, pSet->m_hHw, &pMsg);/*qDebug()<<"Write :"<<QString::number(pMsg.Data[0], 16)<<" "\<<QString::number(pMsg.Data[1], 16)<<" "\<<QString::number(pMsg.Data[2], 16)<<" "\<<QString::number(pMsg.Data[3], 16)<<" "\<<QString::number(pMsg.Data[4], 16)<<" "\<<QString::number(pMsg.Data[5], 16)<<" "\<<QString::number(pMsg.Data[6], 16)<<" "\<<QString::number(pMsg.Data[7], 16)<<" m_LastLINErr:"\<<err;*/}else{// If the hardare is initialize as slave// only update the data in the LIN frame.pSet->m_LastLINErr = pSet->m_objPLinApi->UpdateByteArray(pSet->m_hClient, pSet->m_hHw, sLinFrame.m_nID, (BYTE)0, pMsg.Length, pMsg.Data);}// Show error if anyif (err != errOK){qDebug()<<pSet->GetFormatedError(pSet->m_LastLINErr);return false;}return true;
}
9 线程接收函数
void PLinApiControl::LIN_Read()
{if(!pSet->m_objPLinApi->bLoadPLinApi())return ;if(!pSet->bConnectHard())return ;qDebug()<<"LIN_Read thread id:"<<QThread::currentThreadId();// We read at least one time the queue looking for messages.// If a message is found, we look again trying to find more.// If the queue is empty or an error occurs, we get out from// the dowhile statement.//do{TLINRcvMsg lpMsg;lpMsg.Type = mstBusSleep;if(!bread_start)break;unsigned char data[8] = {0xff};
#if TEST_ONLYLinFrame sLinFrame(ID_TEST, 8, cstEnhanced, dirSubscriber, data);
#elseLinFrame sLinFrame(ID_RECV, 8, cstEnhanced, dirSubscriber, data);
#endif//qDebug()<<"bwrite ===="<<bwrite;if(bwrite){LIN_Write(sLinFrame);}QThread::msleep(read_delay_time);pSet->m_LastLINErr = pSet->m_objPLinApi->Read(pSet->m_hClient, &lpMsg);// If at least one Frame is received by the LinApi.// Check if the received frame is a standard type.// If it is not a standard type than ignore it.if (lpMsg.Type != mstStandard)continue;if (pSet->m_LastLINErr == errOK && lpMsg.ErrorFlags == 0){// ProcessMessage(lpMsg);/**qDebug()<<"lpMsg read:"<<QString::number(lpMsg.Data[0],16)<<" "\<<QString::number(lpMsg.Data[1],16)<<" "\<<QString::number(lpMsg.Data[2],16)<<" "\<<QString::number(lpMsg.Data[3],16)<<" "\<<QString::number(lpMsg.Data[4],16)<<" "\<<QString::number(lpMsg.Data[5],16)<<" "\<<QString::number(lpMsg.Data[6],16)<<" "\<<QString::number(lpMsg.Data[7],16)<<" ";*/if(lpMsg.Data[0] == RECV_READVAL)emit signal_read_data(lpMsg);
#if TEST_ONLYelse if(lpMsg.Data[0] == 2){// 锁定互斥量QMutexLocker locker(&m_mutex);mSEND_CTRPRM.clear();mSEND_CTRPRM.enqueue(lpMsg);// 唤醒等待的线程m_condition.wakeOne();}
#elseelse if(lpMsg.Data[0] == SEND_CTRPRM){// 锁定互斥量QMutexLocker locker(&m_mutex);//qDebug()<<"aaaaa ";mSEND_CTRPRM.clear();mSEND_CTRPRM.enqueue(lpMsg);// 唤醒等待的线程m_condition.wakeOne();}
#endifelse if(lpMsg.Data[0] == SEND_CALIBPRM){// 锁定互斥量//qDebug()<<"LIN_Read "<<QThread::currentThreadId();QMutexLocker locker(&m_mutex);mSEND_CALIBPRM.clear();mSEND_CALIBPRM.enqueue(lpMsg);// 唤醒等待的线程m_condition.wakeOne();}else{QMutexLocker locker(&m_mutex);mSEND_Other.clear();mSEND_Other.enqueue(lpMsg);// 唤醒等待的线程m_condition.wakeOne();}}} while (pSet->bConnectHard());//while (pSet->bConnectHard() && (!(pSet->m_LastLINErr & errRcvQueueEmpty)));
}
UDS发送时将 bwrite 设置为false, 并等待接收上一帧报文完成后再发送
相关文章:

LIN通讯
目录 1 PLinApi.h 2 TLINFrameEntry 结构体 3 自定义函数getTLINFrameEntry 4 TLINScheduleSlot 结构体 5 自定义函数 getTLINScheduleSlot 6 自定义LIN_SetScheduleInit函数 7 自定义 LIN_StartSchedule 8 发送函数 9 线程接收函数 1 PLinApi.h 这是官方头文件 ///…...
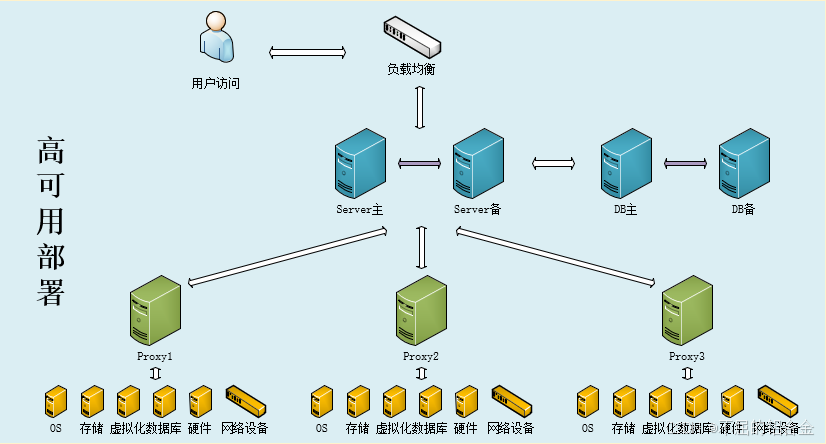
zabbix常见架构及组件
Zabbix作为一个开源的、功能全面的监控解决方案,广泛应用于各类组织中,以实现对网络、服务器、云服务及应用程序性能的全方位监控。部署架构灵活性高,可支持从小型单一服务器环境到大型分布式系统的多种场景。基本架构通常包括监控端…...

plsql表格怎么显示中文 plsql如何导入表格数据
在Oracle数据库开发中,PL/SQL Developer是一款广泛使用的集成开发环境(IDE),它提供了丰富的功能来帮助开发人员高效地进行数据库开发和管理。在使用PL/SQL Developer时,许多用户会遇到表格显示中文的问题,以…...

chromedriver下载地址大全(包括124.*后)以及替换exe后仍显示版本不匹配的问题
Chrome for Testing availability CNPM Binaries Mirror 若已经更新了系统环境变量里的chromdriver路径下的exe,仍显示版本不匹配: 则在cmd界面输入 chromedriver 会跳出version verison与刚刚下载好的exe不匹配,则再输入: w…...
拦截器实现 Mybatis Plus 打印含参数的 SQL 语句
1.实现拦截器 package com.sample.common.interceptor;import com.baomidou.mybatisplus.extension.plugins.inner.InnerInterceptor; import lombok.extern.slf4j.Slf4j; import org.apache.ibatis.executor.Executor; import org.apache.ibatis.mapping.BoundSql; import or…...
Oracle Subprogram即Oracle子程序
Oracle Subprogram,即Oracle子程序,是Oracle数据库中存储的过程(Procedures)和函数(Functions)的统称。这些子程序是存储在数据库中的PL/SQL代码块,用于执行特定的任务或操作。下面详细介绍Orac…...
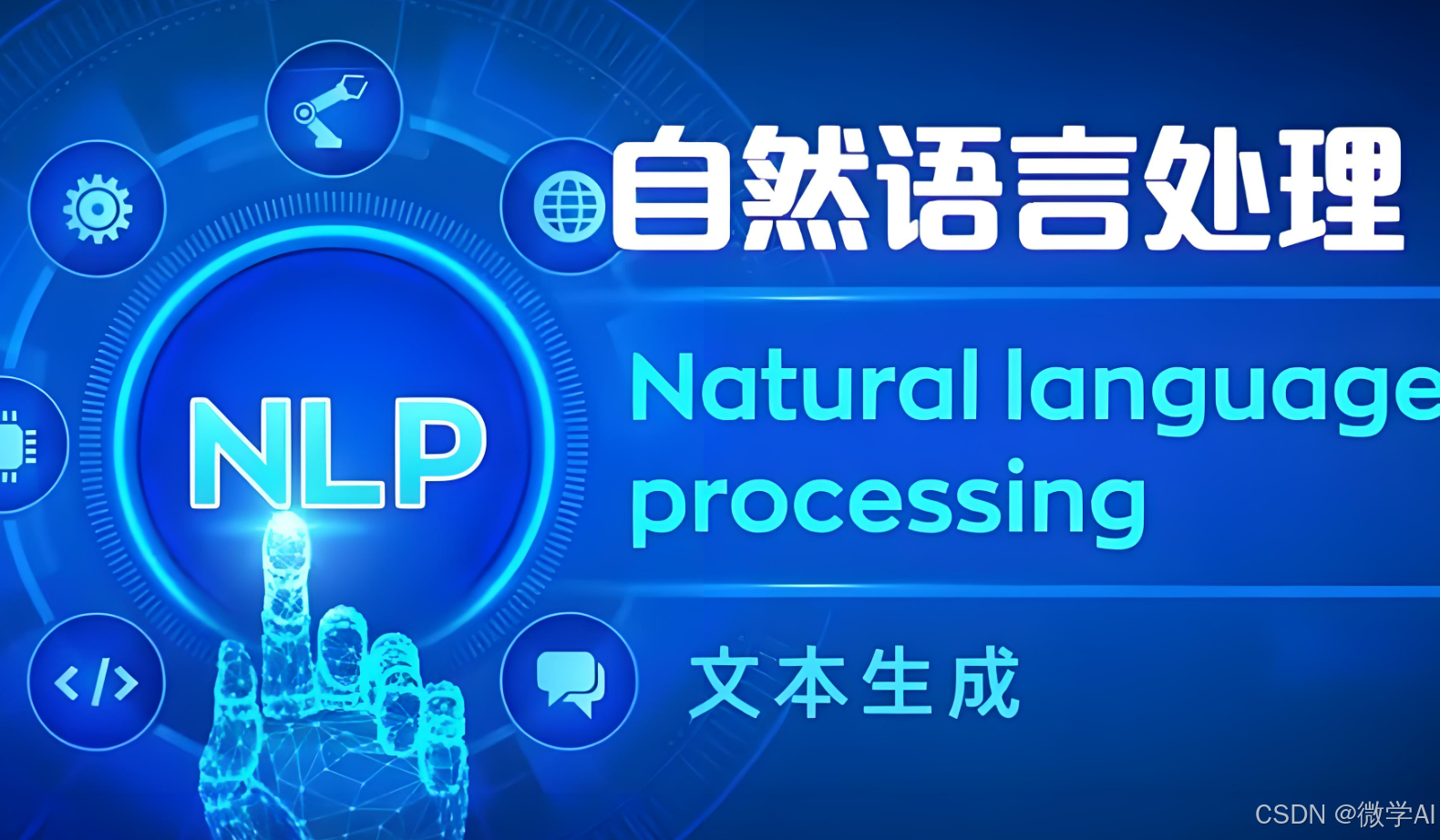
自然语言处理实战项目30-基于RoBERTa模型的高精度的评论文本分类实战,详细代码复现可直接运行
大家好,我是微学AI,今天给大家介绍一下自然语言处理实战项目30-基于RoBERTa模型的高精度的评论文本分类实战,详细代码复现可直接运行。RoBERTa模型是由 Facebook AI Research 和 FAIR 的研究人员提出的一种改进版的 BERT 模型。RoBERTa 通过采用更大的训练数据集、动态掩码机…...
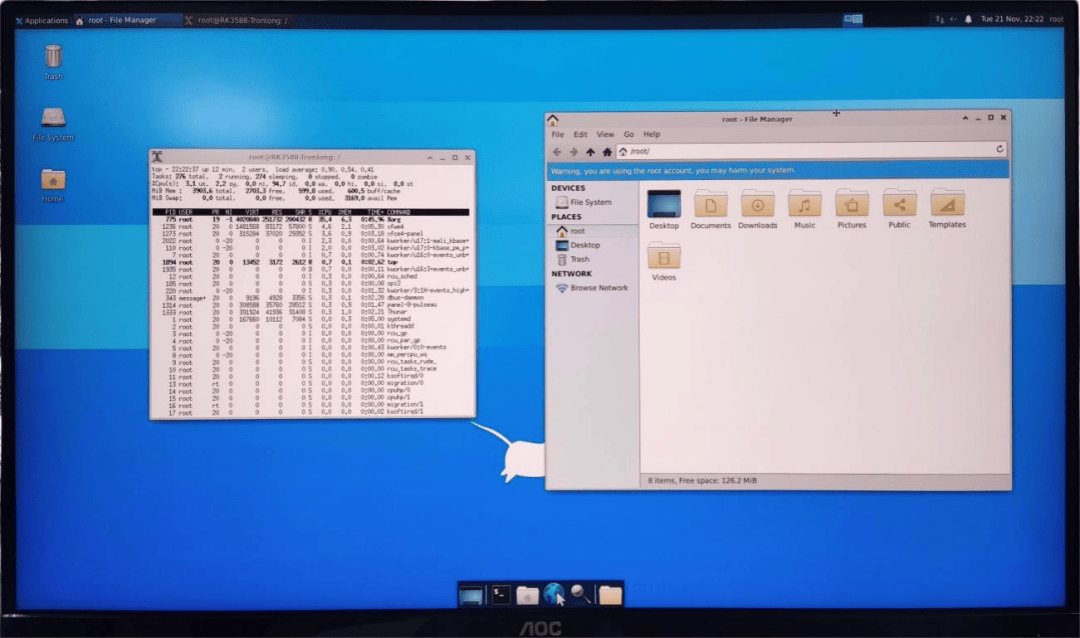
RK3588J正式发布Ubuntu桌面系统,丝滑又便捷!
本文主要介绍瑞芯微RK3588J的Ubuntu系统桌面演示,开发环境如下: U-Boot:U-Boot-2017.09 Kernel:Linux-5.10.160 Ubuntu:Ubuntu20.04.6 LinuxSDK: rk3588-linux5.10-sdk-[版本号] (基于rk3…...
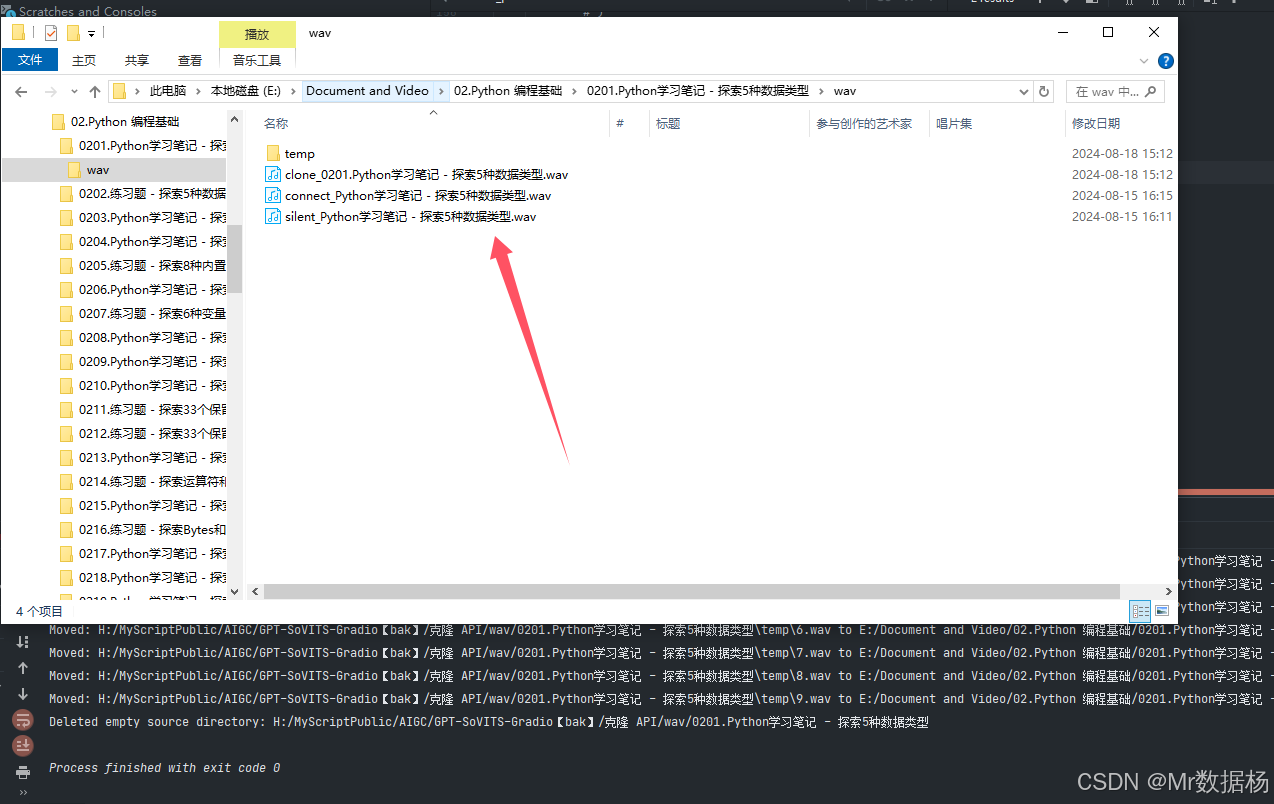
基于GPT-SoVITS的API实现批量克隆声音
目标是将每一段声音通过GPT-SoVITS的API的API进行克隆,因为拼在一起的整个片段处理会造成内存或者缓存溢出。 将目录下的音频文件生成到指定目录下,然后再进行拼接。 通过AI工具箱生成的数据文件是这样的结构,temp目录下是没个片段生成的部分,connect_是正常拼接的音频文件…...
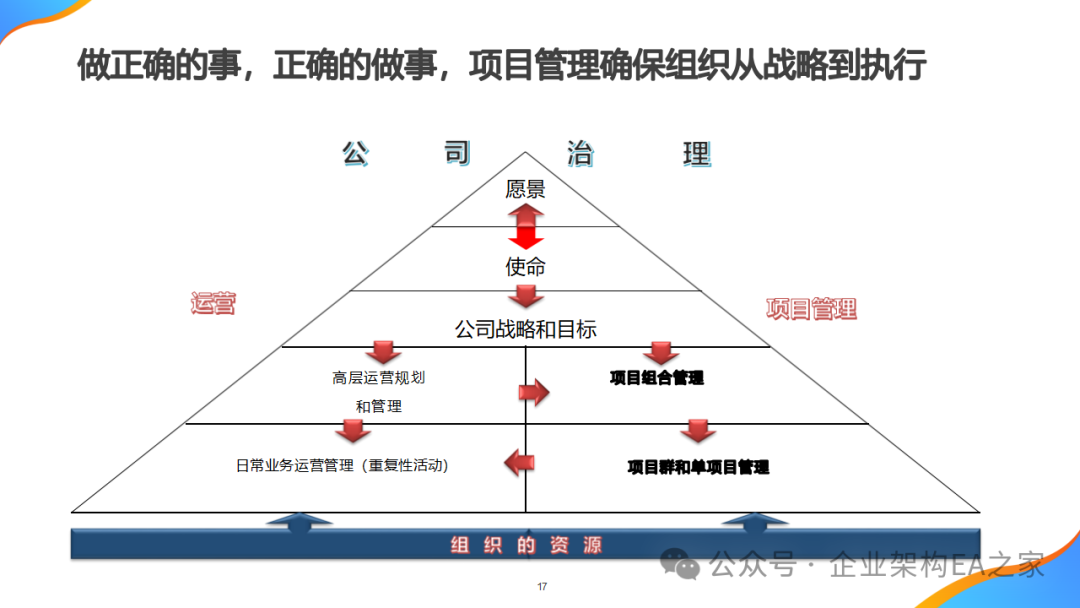
详解华为项目管理,附华为高级项目管理内训材料
(一)华为在项目管理中通过有效的沟通、灵活的组织结构、坚持不懈的努力、细致的管理和科学的考核体系,实现了持续的创新和发展。通过引进先进的管理模式,强调以客户需求为导向,华为不仅优化了技术管理和项目研发流程&a…...
Perl(Practical Extraction and Reporting Language)脚本
Perl(Practical Extraction and Reporting Language)是一种非常灵活的脚本语言,主要用于文本处理、系统管理以及快速原型开发等领域。Perl 脚本可以用来执行一系列任务,包括文件操作、网络通信、数据处理等。 下面是一些关于编写…...
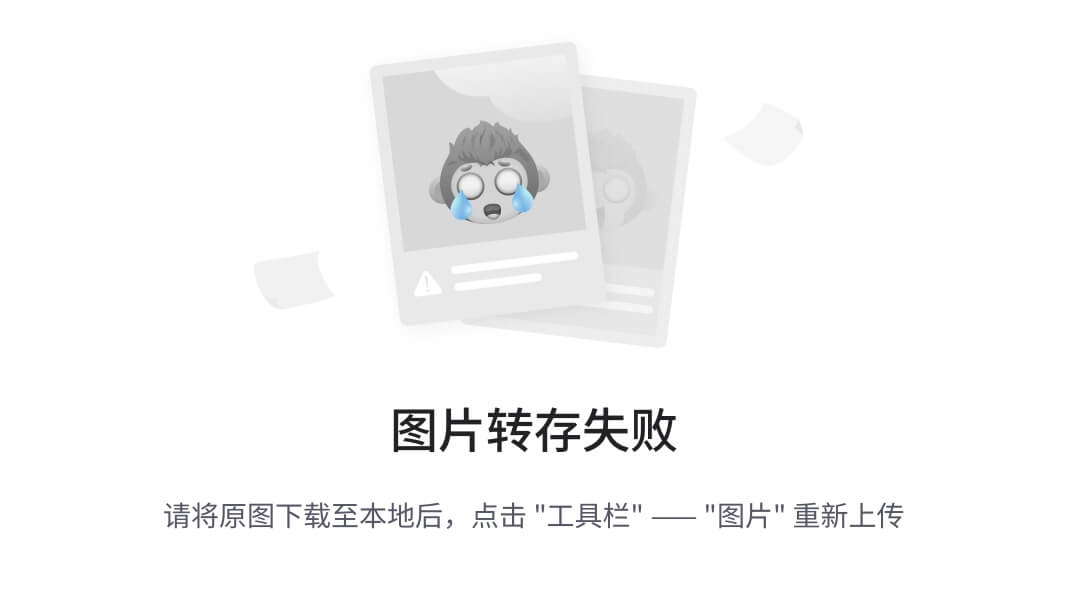
单例模式详细
文章目录 单例模式介绍八种方式1、饿汉式(静态常量)2、饿汉式(静态代码块)3、懒汉式(线程不安全)4、懒汉式(线程安全,同步方法)5、懒汉式(线程不安全…...
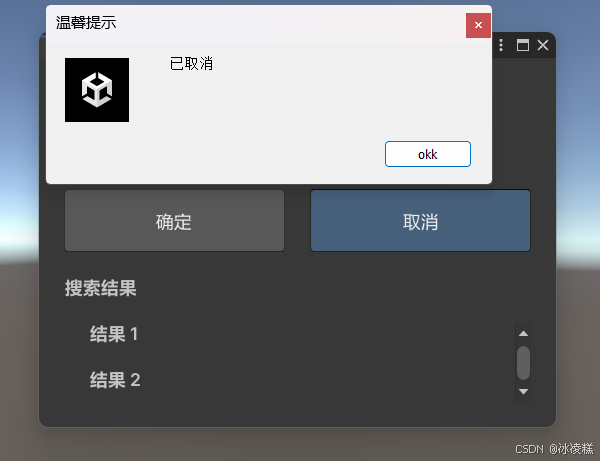
Unity3D 自定义窗口
Unity3D 自定义窗口的实现。 自定义窗口 Unity3D 可以通过编写代码,扩展编辑器的菜单栏和窗口。 简单的功能可以直接一个菜单按钮实现,复杂的功能就需要绘制一个窗口展示更多的信息。 编辑器扩展的脚本,需要放在 Editor 文件夹中。 菜单栏…...
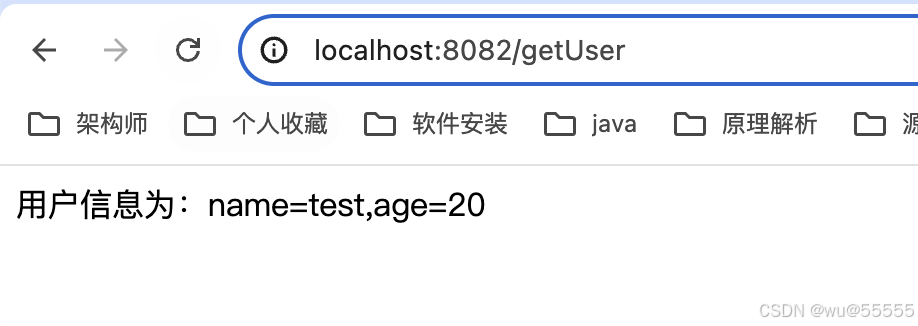
dubbo:dubbo整合nacos实现服务注册中心、配置中心(二)
文章目录 0. 引言1. nacos简介及安装2. 注册中心实现3. 配置中心实现4. 源码5. 总结 0. 引言 之前我们讲解的是dubbozookeeper体系来实现微服务框架,但相对zookeeper很多企业在使用nacos, 并且nacos和dubbo都是阿里出品,所以具备一些天生的契合性&#…...
个人博客指路
Pudding 个人博客 比较懒,直接 github page 了,没国内代理加速。 欢迎大佬们,踩一踩 没做留言,觉得很鸡肋。有问题可以在本文底下评论、或者直接邮件...
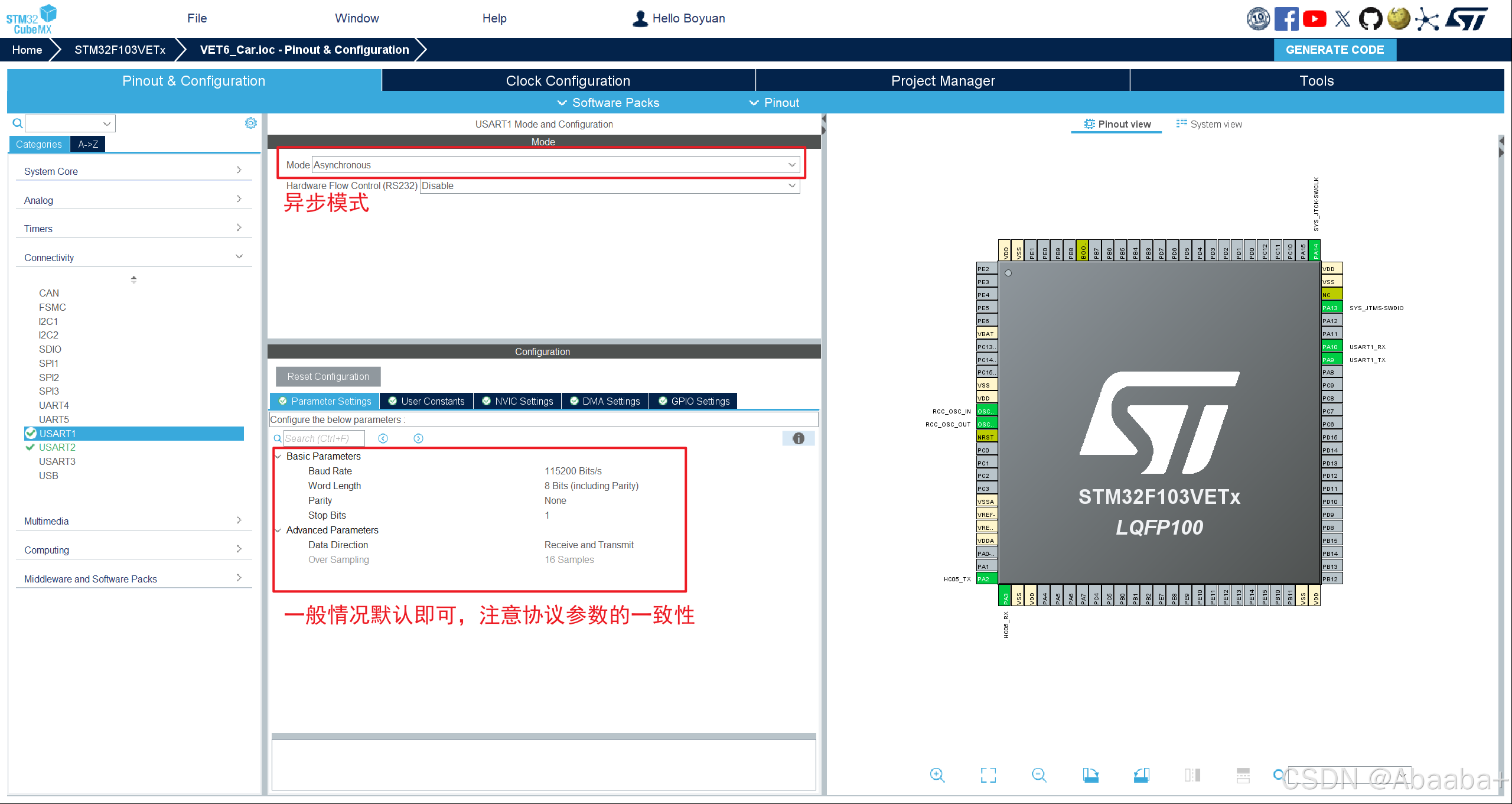
【STM32 HAL】多串口printf重定向
【STM32 HAL】多串口printf重定向 前言单串口printf重定向原理实现CubeMX配置Keil5配置 多串口printf重定向 前言 在近期项目中,作者需要 STM32 同时向上位机和手机发送数据,传统的 printf 重定向只能输出到一个串口。本文介绍如何实现 printf 同时输出…...

帆软报表,达梦数据库驱动上传失败
1、按照正常操作新建数据库连接,上传准备好的达梦驱动时,提示如图一需要修改SystemConfig.driverUpload为true才可以。 2、FineDB存储了数据决策系统中除平台属性配置以外的所有信息。详情请参见: FineDB 数据库简介。 3、因此管理员可通过…...
CSS选择器的优先级是如何确定的?有哪些方法可以提高选择器的效率?
CSS选择器的优先级是如何确定的? CSS选择器的优先级决定了当多个选择器同时应用于一个元素时,哪个选择器将最终生效。CSS选择器的优先级由多个因素决定,主要包括以下几个方面: 特殊性(Specificity) 特殊性…...
【MySQL】基础入门(第二篇)
1.MySQL基本数据类型 数值类型 MySQL 支持所有标准 SQL 数值数据类型。 这些类型包括严格数值数据类型(INTEGER、SMALLINT、DECIMAL 和 NUMERIC),以及近似数值数据类型(FLOAT、REAL 和 DOUBLE PRECISION)。 关键字INT是INTEGER的同义词,关键字DEC是D…...
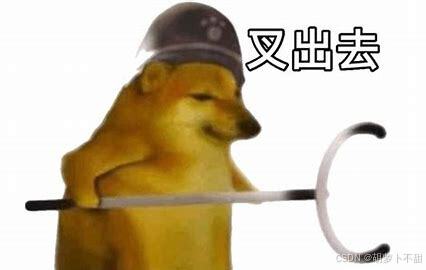
勇闯机器学习(第二关-数据集使用)
以下内容,皆为原创,重在无私分享高质量知识,制作实属不易,请点点关注。 好戏开场了~~~(这关涉及到了加载数据集的代码,下一关,教你们安装机器学习库) 一.数据集 这一关的目标 知道数据集被分为训练集和测…...
【杂谈】-递归进化:人工智能的自我改进与监管挑战
递归进化:人工智能的自我改进与监管挑战 文章目录 递归进化:人工智能的自我改进与监管挑战1、自我改进型人工智能的崛起2、人工智能如何挑战人类监管?3、确保人工智能受控的策略4、人类在人工智能发展中的角色5、平衡自主性与控制力6、总结与…...
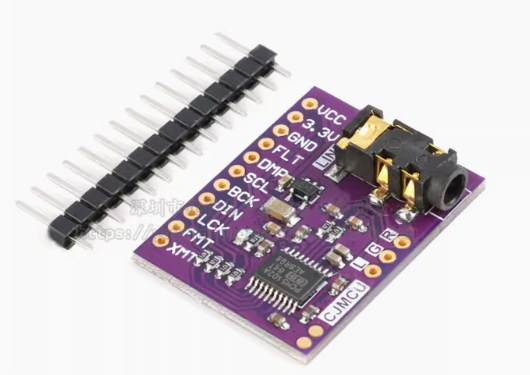
ESP32 I2S音频总线学习笔记(四): INMP441采集音频并实时播放
简介 前面两期文章我们介绍了I2S的读取和写入,一个是通过INMP441麦克风模块采集音频,一个是通过PCM5102A模块播放音频,那如果我们将两者结合起来,将麦克风采集到的音频通过PCM5102A播放,是不是就可以做一个扩音器了呢…...
【算法训练营Day07】字符串part1
文章目录 反转字符串反转字符串II替换数字 反转字符串 题目链接:344. 反转字符串 双指针法,两个指针的元素直接调转即可 class Solution {public void reverseString(char[] s) {int head 0;int end s.length - 1;while(head < end) {char temp …...
Neo4j 集群管理:原理、技术与最佳实践深度解析
Neo4j 的集群技术是其企业级高可用性、可扩展性和容错能力的核心。通过深入分析官方文档,本文将系统阐述其集群管理的核心原理、关键技术、实用技巧和行业最佳实践。 Neo4j 的 Causal Clustering 架构提供了一个强大而灵活的基石,用于构建高可用、可扩展且一致的图数据库服务…...
土地利用/土地覆盖遥感解译与基于CLUE模型未来变化情景预测;从基础到高级,涵盖ArcGIS数据处理、ENVI遥感解译与CLUE模型情景模拟等
🔍 土地利用/土地覆盖数据是生态、环境和气象等诸多领域模型的关键输入参数。通过遥感影像解译技术,可以精准获取历史或当前任何一个区域的土地利用/土地覆盖情况。这些数据不仅能够用于评估区域生态环境的变化趋势,还能有效评价重大生态工程…...
Axios请求超时重发机制
Axios 超时重新请求实现方案 在 Axios 中实现超时重新请求可以通过以下几种方式: 1. 使用拦截器实现自动重试 import axios from axios;// 创建axios实例 const instance axios.create();// 设置超时时间 instance.defaults.timeout 5000;// 最大重试次数 cons…...
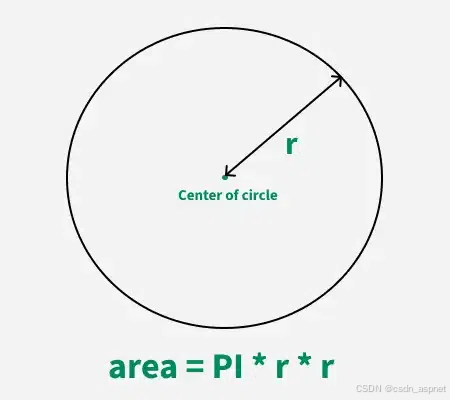
C++ 求圆面积的程序(Program to find area of a circle)
给定半径r,求圆的面积。圆的面积应精确到小数点后5位。 例子: 输入:r 5 输出:78.53982 解释:由于面积 PI * r * r 3.14159265358979323846 * 5 * 5 78.53982,因为我们只保留小数点后 5 位数字。 输…...
C++八股 —— 单例模式
文章目录 1. 基本概念2. 设计要点3. 实现方式4. 详解懒汉模式 1. 基本概念 线程安全(Thread Safety) 线程安全是指在多线程环境下,某个函数、类或代码片段能够被多个线程同时调用时,仍能保证数据的一致性和逻辑的正确性…...
JavaScript 数据类型详解
JavaScript 数据类型详解 JavaScript 数据类型分为 原始类型(Primitive) 和 对象类型(Object) 两大类,共 8 种(ES11): 一、原始类型(7种) 1. undefined 定…...
解决:Android studio 编译后报错\app\src\main\cpp\CMakeLists.txt‘ to exist
现象: android studio报错: [CXX1409] D:\GitLab\xxxxx\app.cxx\Debug\3f3w4y1i\arm64-v8a\android_gradle_build.json : expected buildFiles file ‘D:\GitLab\xxxxx\app\src\main\cpp\CMakeLists.txt’ to exist 解决: 不要动CMakeLists.…...