做网站推广的流程/百度站长平台链接提交
需要引用NPOI库 https://github.com/dotnetcore/NPOI
调用Read 函数将excel读取到dataexcel控件
调用Save 函数将dataexcel控件文件保存为excel文件
using NPOI.HSSF.UserModel;
using NPOI.HSSF.Util;
using NPOI.SS.UserModel;
using NPOI.SS.Util;
using System;
using System.Collections.Generic;
using System.Drawing;
using System.IO;
using System.Text;
using System.Windows.Forms;namespace Feng.DataTool
{public class ExcelTools{public static void Save(Feng.Excel.DataExcel grid, string file){HSSFWorkbook hssfworkbook = new HSSFWorkbook();ISheet sheet1 = hssfworkbook.CreateSheet("Sheet1");List<CellStyleTemp> cellstyletemplist = new List<CellStyleTemp>();foreach (Feng.Excel.Interfaces.IRow gridrow in grid.Rows){if (gridrow.Index < 1)continue;IRow row = sheet1.CreateRow(gridrow.Index-1);row.Height = (short)(gridrow.Height * 20);foreach (Feng.Excel.Interfaces.IColumn column in grid.Columns){if (column.Index < 1)continue;Feng.Excel.Interfaces.ICell gridcell = gridrow[column];if (gridcell == null)continue;ushort width = (ushort)((column.Width) / 7 * 256);sheet1.SetColumnWidth(column.Index-1, width);ICell cell = row.CreateCell(column.Index-1);string text = gridcell.Text;cell.SetCellValue(text);bool bottomlinestylevisible = false;bool leftlinestylevisible = false;bool rightlinestylevisible = false;bool toplinestylevisible = false;bool isstrikeout = false;bool isbold = false;double fontheightinpoints = 29;string fontname = string.Empty;if (gridcell.BorderStyle != null){if (gridcell.BorderStyle.BottomLineStyle != null){if (gridcell.BorderStyle.BottomLineStyle.Visible){bottomlinestylevisible = true;}}if (gridcell.BorderStyle.LeftLineStyle != null){if (gridcell.BorderStyle.LeftLineStyle.Visible){leftlinestylevisible = true;}}if (gridcell.BorderStyle.RightLineStyle != null){if (gridcell.BorderStyle.RightLineStyle.Visible){rightlinestylevisible = true;}}if (gridcell.BorderStyle.TopLineStyle != null){if (gridcell.BorderStyle.TopLineStyle.Visible){toplinestylevisible = true;}}}isstrikeout = gridcell.Font.Strikeout; isbold = gridcell.Font.Bold;fontheightinpoints = gridcell.Font.Size;fontname = gridcell.Font.Name;int alignment = GetHAlignment(gridcell.HorizontalAlignment);int verticalalignment = GetVAlignment(gridcell.VerticalAlignment);ICellStyle style = GetCellStyle(hssfworkbook, cellstyletemplist, bottomlinestylevisible, leftlinestylevisible, rightlinestylevisible, toplinestylevisible, isstrikeout, isbold, fontheightinpoints, fontname, alignment, verticalalignment);cell.CellStyle = style;}}foreach (Feng.Excel.Interfaces.IMergeCell item in grid.MergeCells){sheet1.AddMergedRegion(new CellRangeAddress(item.MinCell.Row.Index-1,item.MaxRowIndex - 1, item.MinCell.Column.Index - 1, item.MaxColumnIndex - 1));//sheet1.AddMergedRegion(new CellRangeAddress(2, 7, 2, 7));}FileStream stream = new FileStream(file, FileMode.Create);hssfworkbook.Write(stream);stream.Close();}public static int GetHAlignment(StringAlignment alignment){switch (alignment){case StringAlignment.Near:return 1; case StringAlignment.Center:return 2;case StringAlignment.Far:return 3;default:break;}return 0;}public static int GetVAlignment(StringAlignment alignment){switch (alignment){case StringAlignment.Near:return 0;case StringAlignment.Center:return 1;case StringAlignment.Far:return 2;default:break;}return 0;}public class CellStyleTemp{public CellStyleTemp(){}public bool BottomLineStyleVisible { get; set; }public bool LeftLineStyleVisible { get; set; }public bool RightLineStyleVisible { get; set; }public bool TopLineStyleVisible { get; set; }public bool IsStrikeout { get; set; }public bool IsBold { get; set; }public double FontHeightInPoints { get; set; }public string FontName { get; set; }public int Alignment { get; set; }public int VerticalAlignment { get; set; }public ICellStyle style { get; set; }}public static ICellStyle GetCellStyle(HSSFWorkbook hssfworkbook, List<CellStyleTemp> styles, bool bottomlinestylevisible, bool leftlinestylevisible, bool rightlinestylevisible, bool toplinestylevisible, bool isstrikeout, bool isbold, double fontheightinpoints, string fontname, int alignment, int verticalalignment){ foreach (CellStyleTemp item in styles){ if (item.BottomLineStyleVisible != bottomlinestylevisible){continue;}double upfontheightinpoints = fontheightinpoints + 0.1;double downfontheightinpoints = fontheightinpoints - 0.1;if (!((item.FontHeightInPoints > downfontheightinpoints) && (item.FontHeightInPoints < upfontheightinpoints))){continue;}if (item.FontName != fontname){continue;}if (item.IsBold != isbold){continue;}if (item.IsStrikeout != isstrikeout){continue;}if (item.LeftLineStyleVisible != leftlinestylevisible){continue;}if (item.RightLineStyleVisible != rightlinestylevisible){continue;}if (item.TopLineStyleVisible != toplinestylevisible){continue;}if (item.Alignment != alignment){continue;}if (item.VerticalAlignment != verticalalignment){continue;}return item.style;}CellStyleTemp cellStyleTemp = new CellStyleTemp();cellStyleTemp.BottomLineStyleVisible = bottomlinestylevisible;cellStyleTemp.FontHeightInPoints = fontheightinpoints;cellStyleTemp.FontName = fontname;cellStyleTemp.IsBold = isbold;cellStyleTemp.IsStrikeout = isstrikeout;cellStyleTemp.LeftLineStyleVisible = leftlinestylevisible;cellStyleTemp.RightLineStyleVisible = rightlinestylevisible;cellStyleTemp.TopLineStyleVisible = toplinestylevisible;cellStyleTemp.Alignment = alignment;cellStyleTemp.VerticalAlignment = verticalalignment;ICellStyle style = CreateCellStyle(hssfworkbook, bottomlinestylevisible, leftlinestylevisible, rightlinestylevisible, toplinestylevisible, isstrikeout, isbold, fontheightinpoints, fontname, alignment, verticalalignment);cellStyleTemp.style = style;styles.Add(cellStyleTemp);return cellStyleTemp.style;}public static ICellStyle CreateCellStyle(HSSFWorkbook hssfworkbook, bool BottomLineStyleVisible, bool LeftLineStyleVisible, bool RightLineStyleVisible, bool TopLineStyleVisible, bool IsStrikeout, bool IsBold, double FontHeightInPoints, string FontName, int alignment, int verticalalignment){ICellStyle style = hssfworkbook.CreateCellStyle();if (BottomLineStyleVisible){style.BorderBottom = NPOI.SS.UserModel.BorderStyle.Thin;}if (LeftLineStyleVisible){style.BorderLeft = NPOI.SS.UserModel.BorderStyle.Thin;}if (RightLineStyleVisible){style.BorderRight = NPOI.SS.UserModel.BorderStyle.Thin;}if (TopLineStyleVisible){style.BorderTop = NPOI.SS.UserModel.BorderStyle.Thin;}style.Alignment = (NPOI.SS.UserModel.HorizontalAlignment)alignment;style.VerticalAlignment = (NPOI.SS.UserModel.VerticalAlignment)verticalalignment;IFont font2 = hssfworkbook.CreateFont();font2.Color = HSSFColor.Black.Index;if (IsStrikeout){font2.IsStrikeout = true;}if (IsBold){font2.IsBold = true;}font2.FontHeightInPoints = FontHeightInPoints;font2.FontName = FontName;style.SetFont(font2);return style;}public static void Read(Feng.Excel.DataExcel grid, string file){FileStream stream = new FileStream(file, FileMode.Open);HSSFWorkbook hssfworkbook = new HSSFWorkbook(stream);stream.Close();ISheet sheet = hssfworkbook.GetSheetAt(0);int rowcount = sheet.LastRowNum;bool hassetcolumnwidth = false;for (int rowindex = 0; rowindex < rowcount; rowindex++){IRow row = sheet.GetRow(rowindex);if (row == null)continue;Feng.Excel.Interfaces.IRow gridrow = grid.GetRow(rowindex + 1);gridrow.Height = row.Height / 20;short columncount = row.LastCellNum;for (short columnindex = 0; columnindex < columncount; columnindex++){Feng.Excel.Interfaces.IColumn gridcolumn = grid.GetColumn(columnindex + 1);int width = sheet.GetColumnWidth(columnindex); gridcolumn.Width = (width / 256) * 7;ICell cell = row.GetCell(columnindex);if (cell == null)continue;Feng.Excel.Interfaces.ICell gridcell = grid.GetCell(gridrow.Index, gridcolumn.Index);gridcell.BorderStyle = new Excel.Styles.CellBorderStyle();try{if (cell.CellType == CellType.String){gridcell.Value = cell.StringCellValue;}if (cell.CellType == CellType.Numeric){gridcell.Value = cell.NumericCellValue;}if (cell.CellType == CellType.Boolean){gridcell.Value = cell.BooleanCellValue;}if (cell.CellType == CellType.Unknown){gridcell.Value = cell.StringCellValue;}if (cell.CellStyle.BorderBottom == NPOI.SS.UserModel.BorderStyle.Thin){gridcell.BorderStyle.BottomLineStyle = new Excel.Styles.LineStyle();gridcell.BorderStyle.BottomLineStyle.Visible = true;}if (cell.CellStyle.BorderLeft == NPOI.SS.UserModel.BorderStyle.Thin){gridcell.BorderStyle.LeftLineStyle = new Excel.Styles.LineStyle();gridcell.BorderStyle.LeftLineStyle.Visible = true;}if (cell.CellStyle.BorderRight == NPOI.SS.UserModel.BorderStyle.Thin){gridcell.BorderStyle.RightLineStyle = new Excel.Styles.LineStyle();gridcell.BorderStyle.RightLineStyle.Visible = true;}if (cell.CellStyle.BorderTop == NPOI.SS.UserModel.BorderStyle.Thin){gridcell.BorderStyle.TopLineStyle = new Excel.Styles.LineStyle();gridcell.BorderStyle.TopLineStyle.Visible = true;}}catch (Exception ex){Feng.Utils.TraceHelper.WriteTrace("SysTools", "ExcelTools", "Read", ex);}try{IFont font2 = cell.CellStyle.GetFont(hssfworkbook);System.Drawing.FontStyle fontstyle = System.Drawing.FontStyle.Regular;if (font2.IsStrikeout){fontstyle = fontstyle | System.Drawing.FontStyle.Strikeout;}if (font2.IsBold){fontstyle = fontstyle | System.Drawing.FontStyle.Bold;}font2.FontHeightInPoints = gridcell.Font.Size;font2.FontName = gridcell.Font.Name;gridcell.Font = new System.Drawing.Font(font2.FontName, (float)font2.FontHeightInPoints, fontstyle);}catch (Exception ex){Feng.Utils.TraceHelper.WriteTrace("SysTools", "ExcelTools", "Read", ex);}try{if (cell.CellStyle.Alignment == NPOI.SS.UserModel.HorizontalAlignment.Center){gridcell.HorizontalAlignment = StringAlignment.Center;}if (cell.CellStyle.Alignment == NPOI.SS.UserModel.HorizontalAlignment.Left){gridcell.HorizontalAlignment = StringAlignment.Near;}if (cell.CellStyle.Alignment == NPOI.SS.UserModel.HorizontalAlignment.Right){gridcell.HorizontalAlignment = StringAlignment.Far;}}catch (Exception ex){ Feng.Utils.TraceHelper.WriteTrace("SysTools", "ExcelTools", "Read", ex);}try{if (cell.CellStyle.VerticalAlignment == NPOI.SS.UserModel.VerticalAlignment.Center){gridcell.VerticalAlignment = StringAlignment.Center;}if (cell.CellStyle.VerticalAlignment == NPOI.SS.UserModel.VerticalAlignment.Top){gridcell.VerticalAlignment = StringAlignment.Near;}if (cell.CellStyle.VerticalAlignment == NPOI.SS.UserModel.VerticalAlignment.Bottom){gridcell.VerticalAlignment = StringAlignment.Far;}}catch (Exception ex){Feng.Utils.TraceHelper.WriteTrace("SysTools", "ExcelTools", "Read", ex);}}}List<CellRangeAddress> listmer = sheet.MergedRegions;if (listmer != null){foreach (CellRangeAddress item in listmer){grid.MergeCell(item.FirstRow + 1, item.FirstColumn + 1, item.LastRow + 1, item.LastColumn + 1);}}}}
}
相关文章:
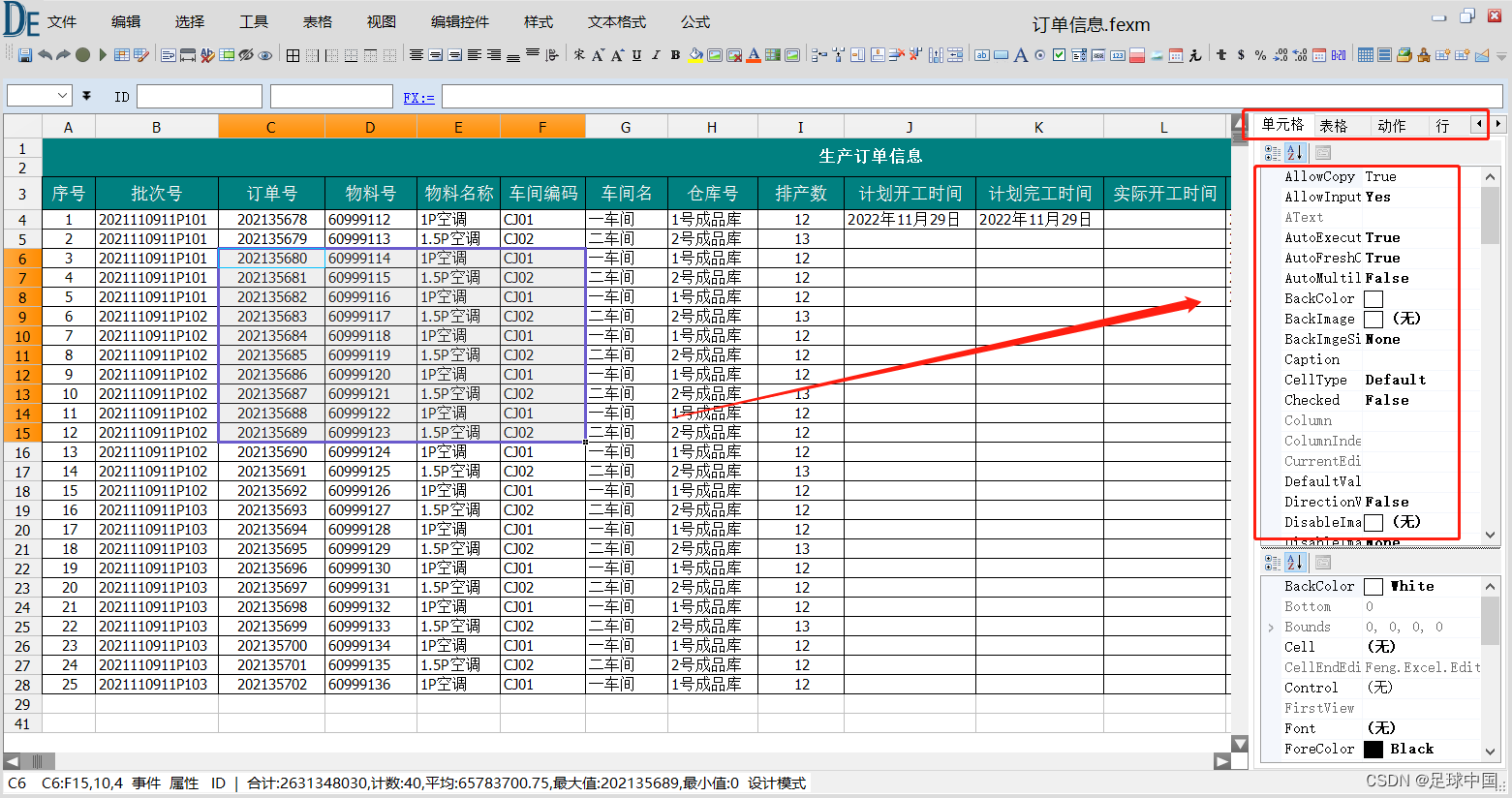
DataExcel控件读取和保存excel xlsx 格式文件
需要引用NPOI库 https://github.com/dotnetcore/NPOI 调用Read 函数将excel读取到dataexcel控件 调用Save 函数将dataexcel控件文件保存为excel文件 using NPOI.HSSF.UserModel; using NPOI.HSSF.Util; using NPOI.SS.UserModel; using NPOI.SS.Util; using System; using …...

【JavaEE】CAS(Compare And Swap)操作
文章目录 什么是 CASCAS 的应用如何使用 CAS 操作实现自旋锁CAS 的 ABA 问题CAS 相关面试题 什么是 CAS CAS(Compare and Swap)是一种原子操作,用于在无锁情况下保证数据一致性的问题。它包含三个操作数——内存位置、预期原值及更新值。在执…...

第三章:最新版零基础学习 PYTHON 教程(第三节 - Python 运算符—Python 中的关系运算符)
关系运算符用于比较值。它根据条件返回 True 或 False。这些运算符也称为比较运算符。 操作员描述 句法> 大于:如果左操作数大于右操作数,则为 Truex > y...
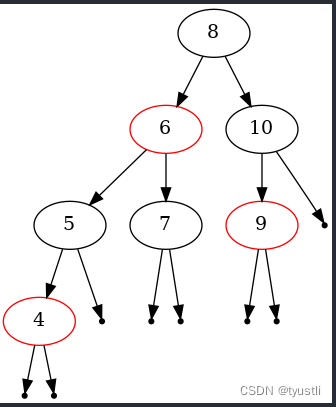
【GDB】使用 GDB 自动画红黑树
阅读本文前需要的基础知识 用 python 扩展 gdb python 绘制 graphviz 使用 GDB 画红黑树 前面几节中介绍了 gdb 的 python 扩展,参考 用 python 扩展 gdb 并且 python 有 graphviz 模块,那么可以用 gdb 调用 python,在 python 中使用 grap…...
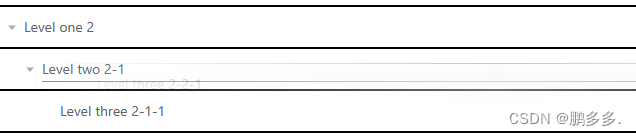
使用Vue3+elementPlus的Tree组件实现一个拖拽文件夹管理
文章目录 1、前言2、分析3、实现4、踩坑4.1、拖拽辅助线的坑4.2、数据的坑4.3、限制拖拽4.4、样式调整 1、前言 最近在做一个文件夹管理的功能,要实现一个树状的文件夹面板。里面包含两种元素,文件夹以及文件。交互要求如下: 创建、删除&am…...
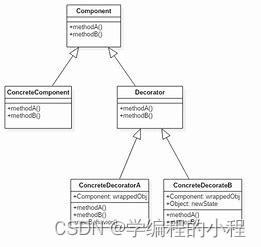
小谈设计模式(7)—装饰模式
小谈设计模式(7)—装饰模式 专栏介绍专栏地址专栏介绍 装饰模式装饰模式角色Component(抽象组件)ConcreteComponent(具体组件)Decorator(抽象装饰器)ConcreteDecorator(具…...

nginx 多层代理 + k8s ingress 后端服务获取客户真实ip 配置
1.nginx http 七层代理 修改命令空间: namespace: nginx-ingress : configmap:nginx-configuration kubectl get cm nginx-configuration -n ingress-nginx -o yaml添加如上配置 compute-full-forwarded-for: “true” forwarded-for-header: X-Forwa…...

6种最常用的3D点云语义分割AI模型对比
由于增强现实/虚拟现实的发展及其在计算机视觉、自动驾驶和机器人领域的广泛应用,点云学习最近引起了人们的关注。 深度学习已成功用于解决 2D 视觉问题,然而,由于其处理面临独特的挑战,深度学习技术在点云上的使用仍处于起步阶段…...

UG NX二次开发(C#)-获取UI中选择对象的handle值
提示:文章写完后,目录可以自动生成,如何生成可参考右边的帮助文档 文章目录 1、前言2、设计一个简单的UI界面3、创建工程项目4、测试结果1、前言 我在哔哩哔哩的视频中看到有人问我如何获取UI选择对象的Handle,本来想把Tag、Taggedobject、Handle三者的关系讲一下,然后看…...
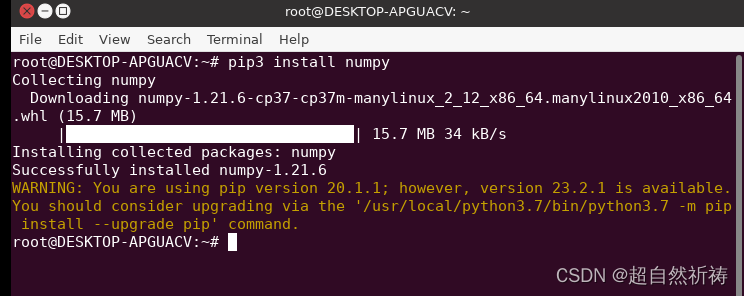
win10,WSL的Ubuntu配python3.7手记
1.装linux 先在windows上安装WSL版本的Ubuntu Windows10系统安装Ubuntu子系统_哔哩哔哩_bilibili (WSL2什么的一直没搞清楚) 图形界面会出一些问题,注意勾选ccsm出的界面设置 win10安装Ubuntu16.04子系统,并开启桌面环境_win…...
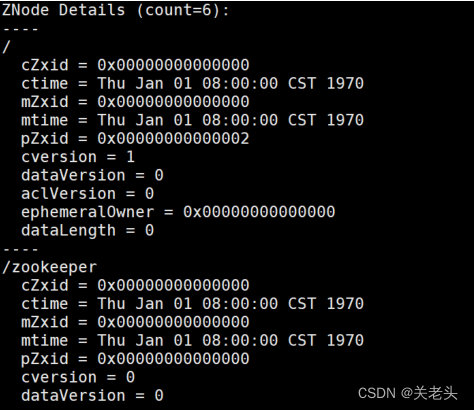
02-Zookeeper实战
上一篇:01-Zookeeper特性与节点数据类型详解 1. zookeeper安装 Step1: 配置JAVA环境,检验环境: java -versionStep2: 下载解压 zookeeper wget https://mirror.bit.edu.cn/apache/zookeeper/zookeeper-3.5.8/apache-zookeepe…...
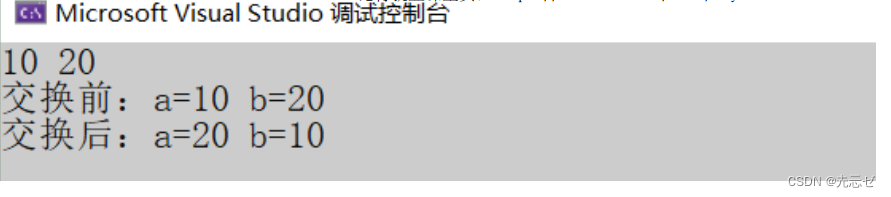
【C语言深入理解指针(1)】
1.内存和地址 1.1内存 在讲内存和地址之前,我们想有个⽣活中的案例: 假设有⼀栋宿舍楼,把你放在楼⾥,楼上有100个房间,但是房间没有编号,你的⼀个朋友来找你玩,如果想找到你,就得挨…...
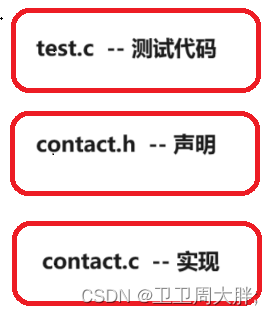
模拟实现简单的通讯录
前言:生活中处处都会看到或是用到通讯录,今天我们就通过C语言来简单的模拟实现一下通讯录。 鸡汤:跨越山海,终见曙光! 链接:gitee仓库:代码链接 目录 主函数声明部分初始化通讯录实现扩容的函数增加通讯录所…...
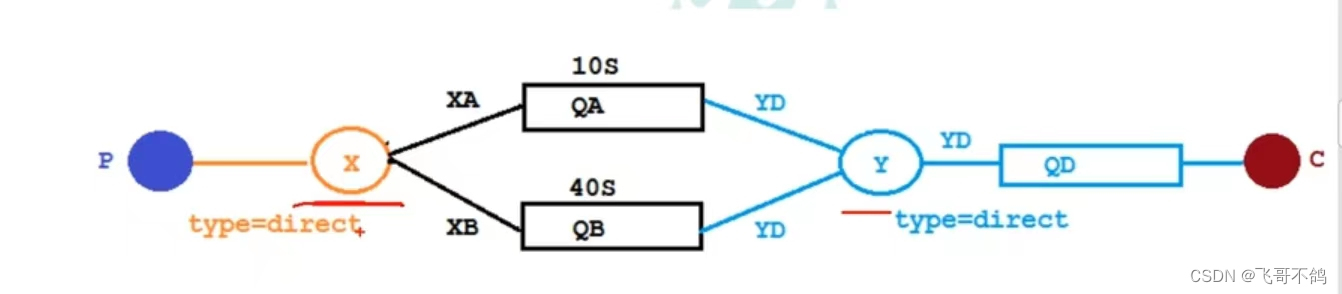
rabbitMQ死信队列快速编写记录
文章目录 1.介绍1.1 什么是死信队列1.2 死信队列有什么用 2. 如何编码2.1 架构分析2.2 maven坐标2.3 工具类编写2.4 consumer1编写2.5 consumer2编写2.6 producer编写 3.整合springboot3.1 架构图3.2 maven坐标3.3 构建配置类,创建exchange,queue&#x…...

数位dp,338. 计数问题
338. 计数问题 - AcWing题库 给定两个整数 a 和 b,求 a 和 b 之间的所有数字中 0∼90∼9 的出现次数。 例如,a1024,b1032,则 a 和 b 之间共有 9 个数如下: 1024 1025 1026 1027 1028 1029 1030 1031 1032 其中 0 出…...

如何解决git clone http/https仓库失败(403错误)
本来不打算写这篇文章,但是后来又遇到这个问题忘了之前是怎么解决的了。 一般情况下,个人使用 GitHub 等平台时是使用 SSH 协议的,这样不光方便管理可访问用户,也保证了安全性。但是 GitHub 上仓库的 SSH 地址是要登陆才能看到&a…...
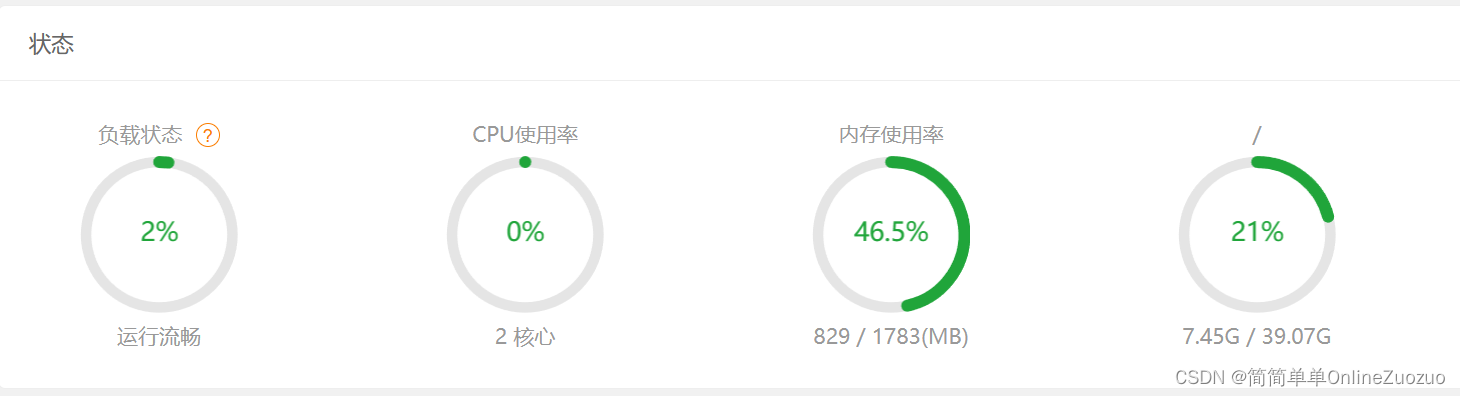
华为云云耀云服务器L实例评测 | 实例评测使用之硬件性能评测:华为云云耀云服务器下的硬件运行评测
华为云云耀云服务器L实例评测 | 实例评测使用之硬件性能评测:华为云云耀云服务器下的硬件运行评测 介绍华为云云耀云服务器 华为云云耀云服务器 (目前已经全新升级为 华为云云耀云服务器L实例) 华为云云耀云服务器是什么华为云云耀…...
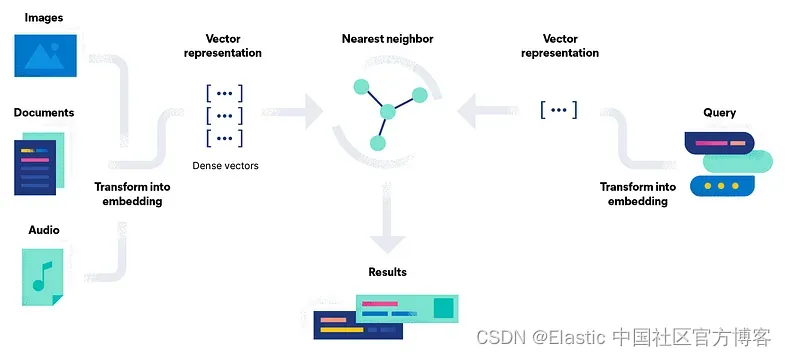
Elasticsearch:使用 Elasticsearch 进行语义搜索
在数字时代,搜索引擎在通过浏览互联网上的大量可用信息来检索数据方面发挥着重要作用。 此方法涉及用户在搜索栏中输入特定术语或短语,期望搜索引擎返回与这些确切关键字匹配的结果。 虽然关键字搜索对于简化信息检索非常有价值,但它也有其局…...

JVM的主要组成及其作用
jvm主要组成部分有: 类加载器、运行时数据区 (内存结构)、执行引擎、本地接口库、垃圾回收机制 Java程序运行的时候,首先会通过类加载器把Java 代码转换成字节码。然后运行时数据区再将字节码加载到内存中,但字节码文件只是JVM 的一套指令集规范…...
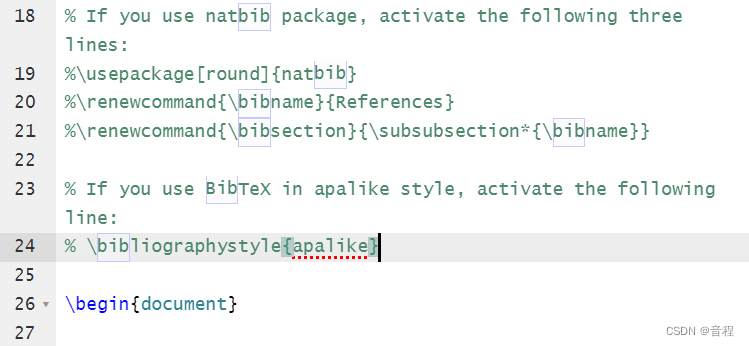
会议AISTATS(Artificial Intelligence and Statistics) Latex模板参考文献引用问题
前言 在看AISTATS2024模板的时候,发现模板里面根本没有教怎么引用,要被气死了。 如下,引用(Cheesman, 1985)的时候,模板是自己手打上去的?而且模板提供的那三个引用,根本也没有Cheesman这个人,…...
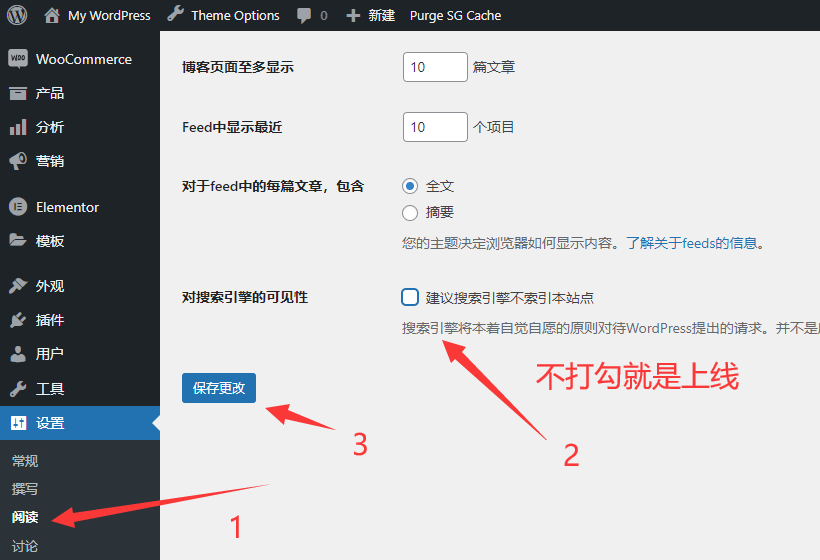
2023最新外贸建站:WordPress搭建外贸独立站零基础小白保姆级教程
想从零开始建立一个外贸自建站,那么你来对地方了。 如果你还在找外贸建站或者是WordPress建站教程,不妨看看这篇文章,本教程涵盖了2023最新的外贸建站教程,你将学会使用WordPress自建外贸独立站,步骤包括购买域名主机…...

HTTP请求交互基础(基于GPT3.5,持续更新)
HTTP交互基础 目的HTTP定义详解HTTP协议(规范)1. 主要组成部分1.1 请求行(Request Line):包含请求方法、请求URI(Uniform Resource Identifier)和HTTP协议版本。1.2 请求头部(Reques…...
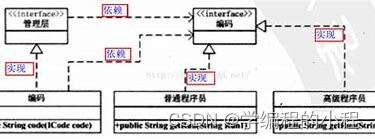
小谈设计模式(6)—依赖倒转原则
小谈设计模式(6)—依赖倒转原则 专栏介绍专栏地址专栏介绍 依赖倒转原则核心思想关键点分析abc 优缺点分析优点降低模块间的耦合度提高代码的可扩展性便于进行单元测试 缺点增加代码的复杂性需要额外的设计和开发工作 Java代码实现示例分析 总结 专栏介绍…...
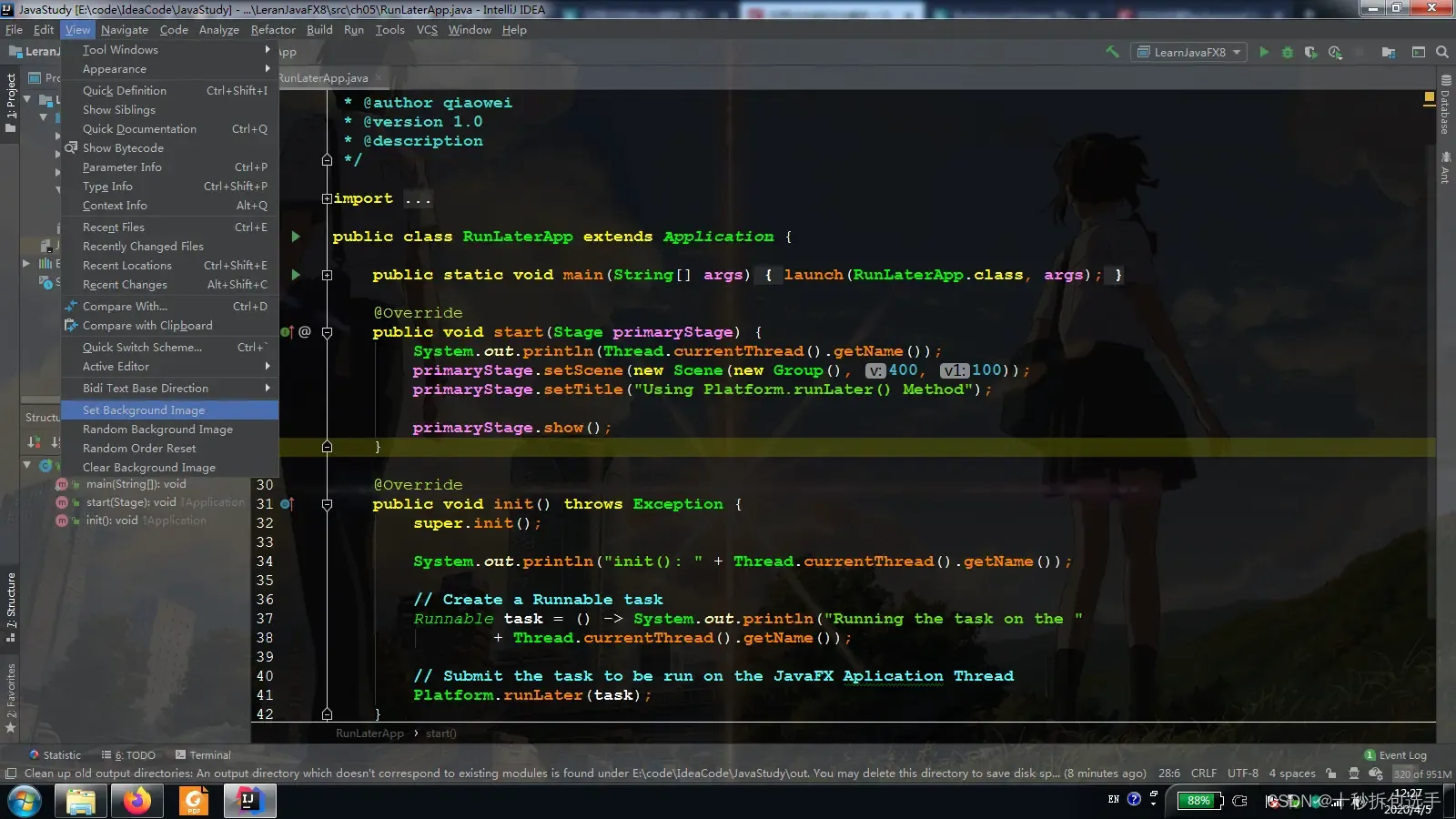
JetBrains常用插件
Codota AI Autocomplete Java and JavaScript:自动补全插件 Background Image plus:背景图片设置 rainbow brackets:彩虹括号,便于识别 CodeGlance2: 类似于 Sublime 中的代码缩略图(代码小地图ÿ…...
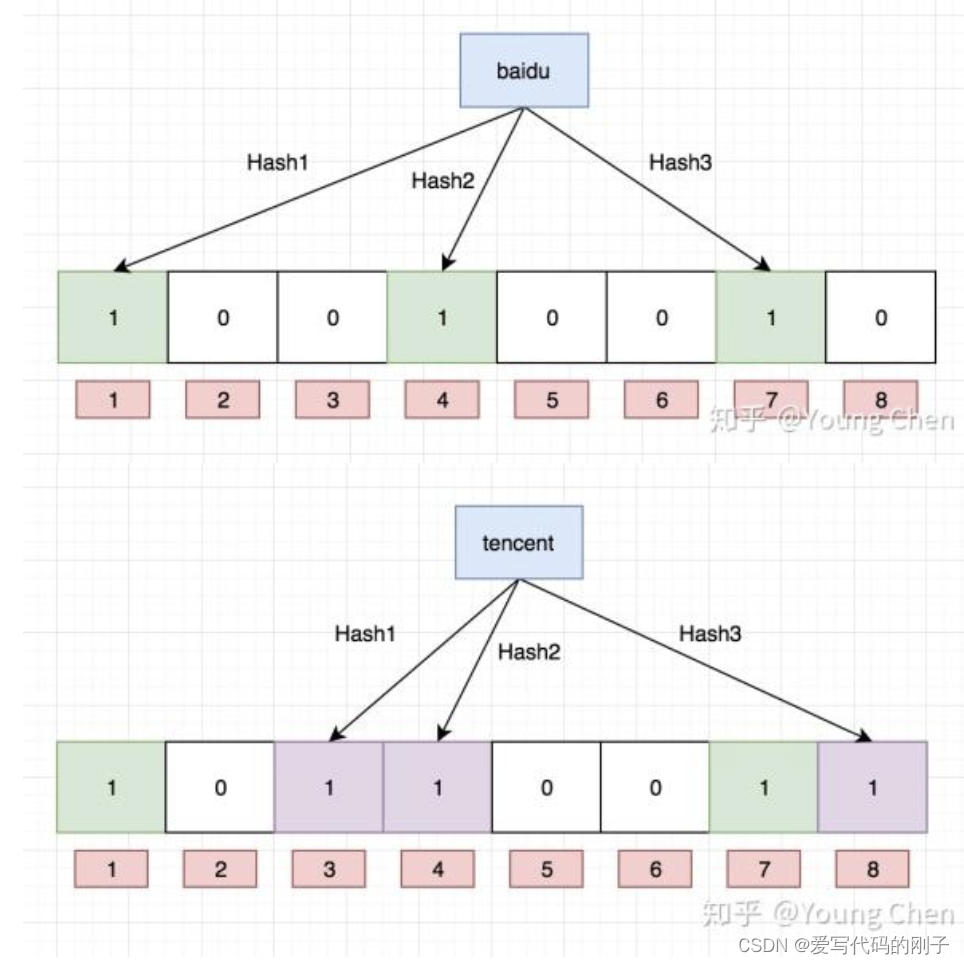
【C++哈希应用】位图、布隆过滤器
【C哈希应用】位图、布隆过滤器 目录 【C哈希应用】位图、布隆过滤器位图概念位图的实现位图改造位图应用总结布隆过滤器布隆过滤器的提出布隆过滤器的概念布隆过滤器的查找布隆过滤器删除布隆过滤器优点布隆过滤器缺陷 作者:爱写代码的刚子 时间:2023.9…...

Qt 编译纯c的C99的项目, error: undefined reference to `f()‘
把Cpp的后缀该为C是什么样的 尝试引用一个奇门排盘的c程序,在git上找到的叫cqm, 然后总是报错 error: undefined reference to f() 很是郁闷 于是新建了个项目试验一下,终于摸清了需要命名空间。 后来这么写就可以了 a.h namespace XX …...
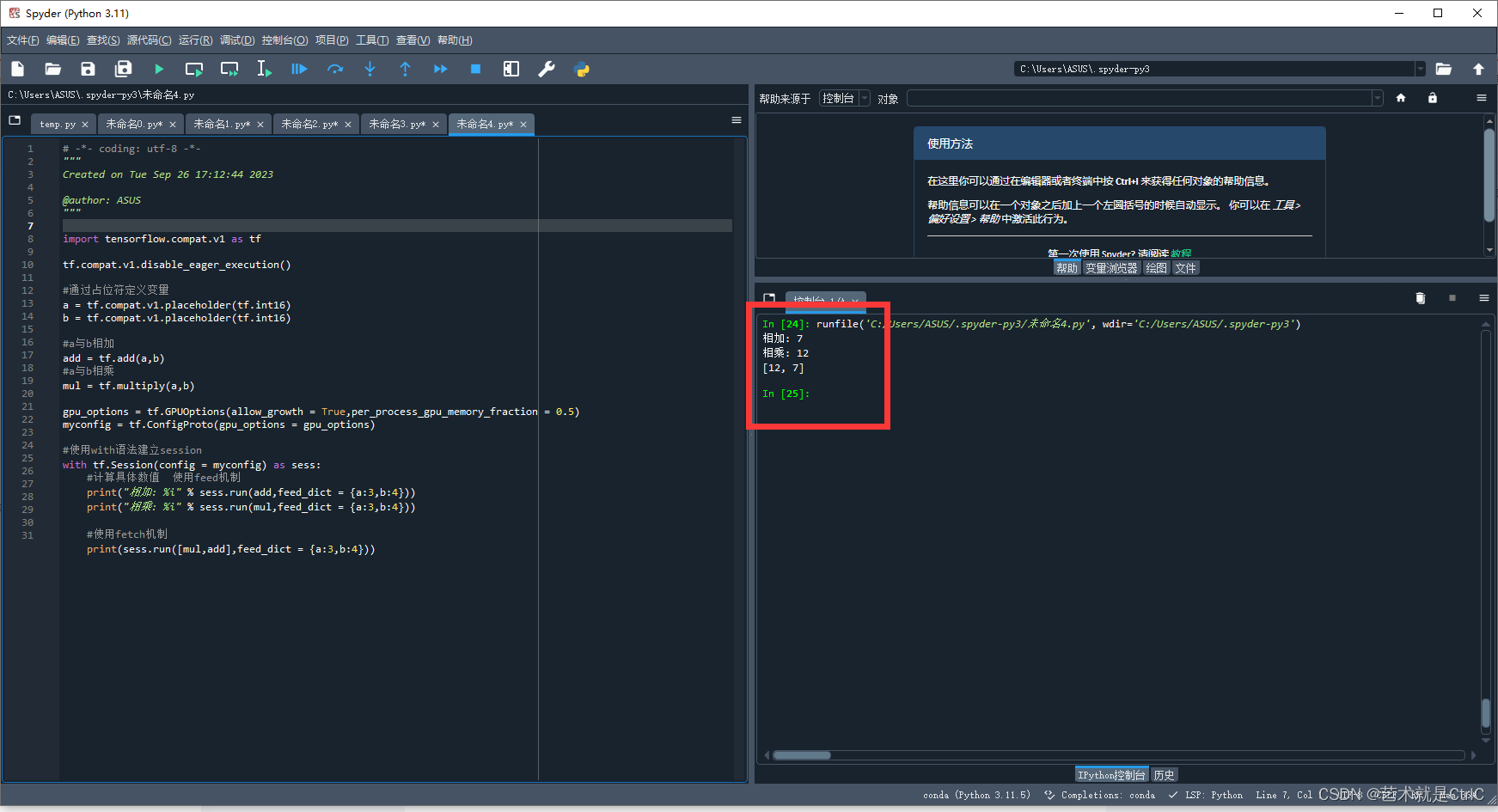
TensorFlow入门(五、指定GPU运算)
一般情况下,下载的TensorFlow版本如果是GPU版本,在运行过程中TensorFlow能自动检测。如果检测到GPU,TensorFlow会默认利用找到的第一个GPU来执行操作。如果机器上有超过一个可用的GPU,除第一个之外的其他GPU默认是不参与计算的。如果想让TensorFlow使用这些GPU执行操作,需要将运…...
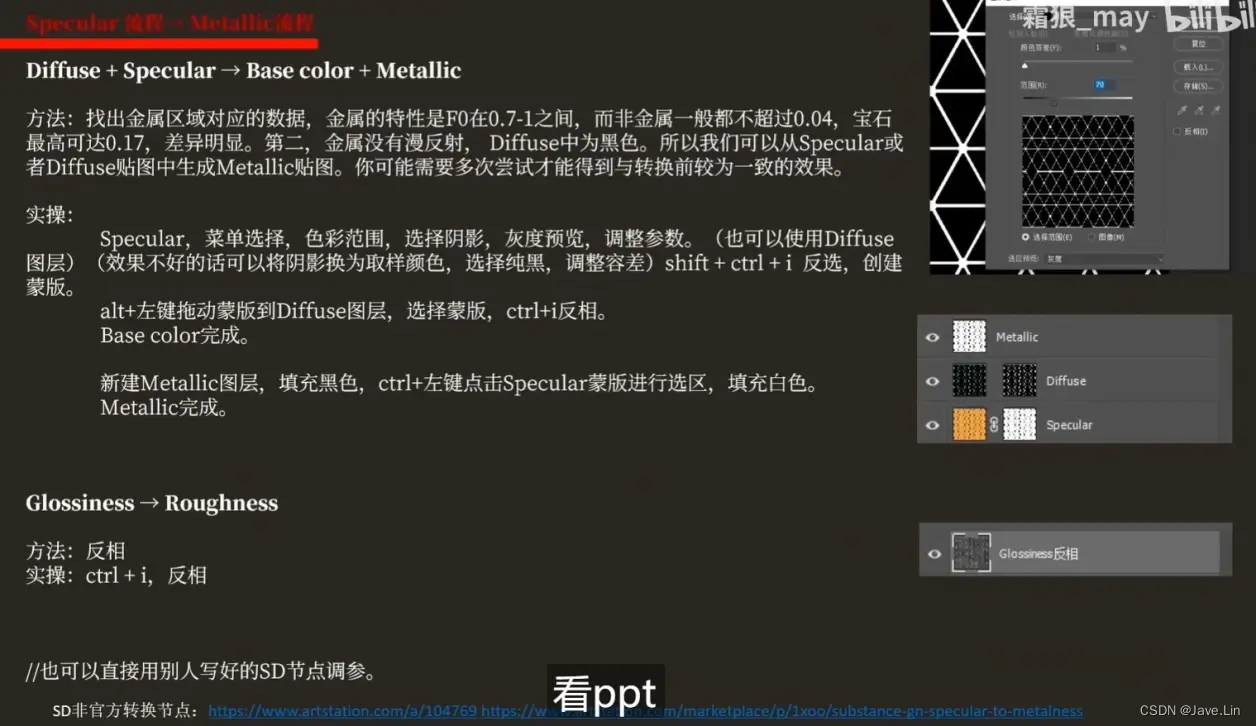
Unity - 实践: Metallic流程贴图 转 Specular流程贴图
文章目录 目的Metallic Flow - SP - 输出输出的 MRA (MGA) 贴图 Metallic->Specular (根据教程一步一步实践)1. Base color Metallic -> Diffuse2. Base color Metallic -> Specular3. Roughness -> Glossiness输出贴图,在 unity 中展示:M…...

第三章:最新版零基础学习 PYTHON 教程(第四节 - Python 运算符—Python 逻辑运算符及示例)
运算符用于对值和变量执行操作。这些是执行算术和逻辑计算的特殊符号。运算符运算的值称为操作数。 表中的内容逻辑运算符 逻辑与运算符 逻辑或运算符 逻辑非运算符 逻辑运算符的求值顺序 逻辑运算符 在 Python 中,逻辑运算符用于条件语句(True 或 False)。它们执行逻辑 AN…...

如何做好测试?(三)功能测试 (Functional Testing, FT)
1. 功能测试的详细介绍: 功能测试 (Functional Testing, FT),是一种软件测试方法,旨在验证系统的功能是否按照需求规格说明书或用户期望的方式正常工作。它关注系统的整体行为,以确保各个功能模块和组件之间的交互和集成正确。 …...