【Leetcode】 17. 电话号码的字母组合
给定一个仅包含数字 2-9
的字符串,返回所有它能表示的字母组合。答案可以按 任意顺序
返回。
给出数字到字母的映射如下(与电话按键相同)。注意 1 不对应任何字母。
示例 1:
输入:digits = "23"
输出:["ad","ae","af","bd","be","bf","cd","ce","cf"]
示例 2:
输入:digits = ""
输出:[]
示例 3:
输入:digits = "2"
输出:["a","b","c"]
提示:
0 <= digits.length <= 4
digits[i]
是范围 ['2', '9']
的一个数字。
AC
:
/** @lc app=leetcode.cn id=17 lang=cpp** [17] 电话号码的字母组合*/// @lc code=start
class Solution {
private:const string letterMap[10] = {"","","abc","def","ghi","jkl","mno","pqrs","tuv","wxyz",};
public:string s;vector<string> result;void backtracking(const string& digits, int index) {if(digits.size() == index) {result.push_back(s);return ;}int digit = digits[index] - '0';string letter = letterMap[digit];for(int i = 0; i < letter.size(); i++){s.push_back(letter[i]);backtracking(digits, index + 1);s.pop_back();}}vector<string> letterCombinations(string digits) {s.clear();result.clear();if(digits.size() == 0)return result;backtracking(digits, 0);return result;}
};
// @lc code=end
The function uses a private member variable letterMap to map each digit to its corresponding set of letters. The letterMap is an array of strings, where each string represents the set of letters corresponding to a digit. For example, letterMap[2] is the string “abc”, which represents the letters ‘a’, ‘b’, and ‘c’ corresponding to the digit 2.
The function uses a recursive backtracking
approach to generate all possible letter combinations. The backtracking function takes two arguments: the input string of digits and an index representing the current position in the input string. The function first checks if the current position is equal to the length of the input string. If so, it adds the current combination of letters to the result vector and returns.
If the current position is less than the length of the input string, the function retrieves the set of letters corresponding to the current digit using the letterMap array. It then iterates over each letter in the set and adds it to the current combination of letters. The function then recursively calls itself with the updated combination of letters and the next index. After the recursive call returns, the function removes the last letter from the combination of letters to backtrack and try the next letter in the set.
The letterCombinations function initializes the result vector and calls the backtracking function with the input string and an initial index of 0. If the input string is empty, the function returns an empty vector.
Overall, this implementation is a simple and efficient way to generate all possible letter combinations of a given string of digits using a backtracking algorithm. One possible way to improve the code would be to add error checking to ensure that the input string only contains digits. Additionally, the variable names could be more descriptive to make the code easier to read and understand.
How does the backtracking algorithm work in generating all possible letter combinations?
The backtracking algorithm used in this code works by recursively generating all possible letter combinations of a given string of digits. The algorithm uses a private member variable letterMap to map each digit to its corresponding set of letters.
The backtracking function takes two arguments
: the input string of digits and an index representing the current position in the input string. The function first checks if the current position is equal to the length of the input string. If so, it adds the current combination of letters to the result vector and returns.
If the current position is less than the length of the input string, the function retrieves the set of letters corresponding to the current digit using the letterMap array. It then iterates over each letter in the set and adds it to the current combination of letters. The function then recursively calls itself with the updated combination of letters and the next index. After the recursive call returns, the function removes the last letter from the combination of letters to backtrack and try the next letter in the set.
The letterCombinations function initializes the result vector and calls the backtracking function with the input string and an initial index of 0 . If the input string is empty, the function returns an empty vector.
Overall, the backtracking algorithm works by generating all possible letter combinations of a given string of digits by recursively iterating over each digit and its corresponding set of letters. The algorithm uses a simple and efficient approach to generate all possible combinations of letters, and is a common technique used in many other combinatorial problems .
相关文章:
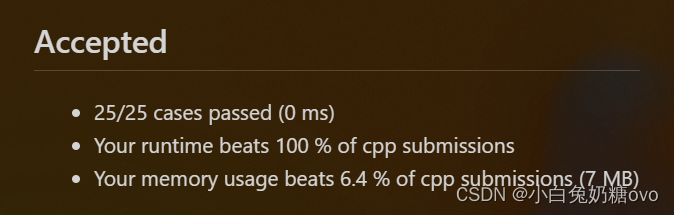
【Leetcode】 17. 电话号码的字母组合
给定一个仅包含数字 2-9 的字符串,返回所有它能表示的字母组合。答案可以按 任意顺序 返回。 给出数字到字母的映射如下(与电话按键相同)。注意 1 不对应任何字母。 示例 1: 输入:digits "23" 输出&…...
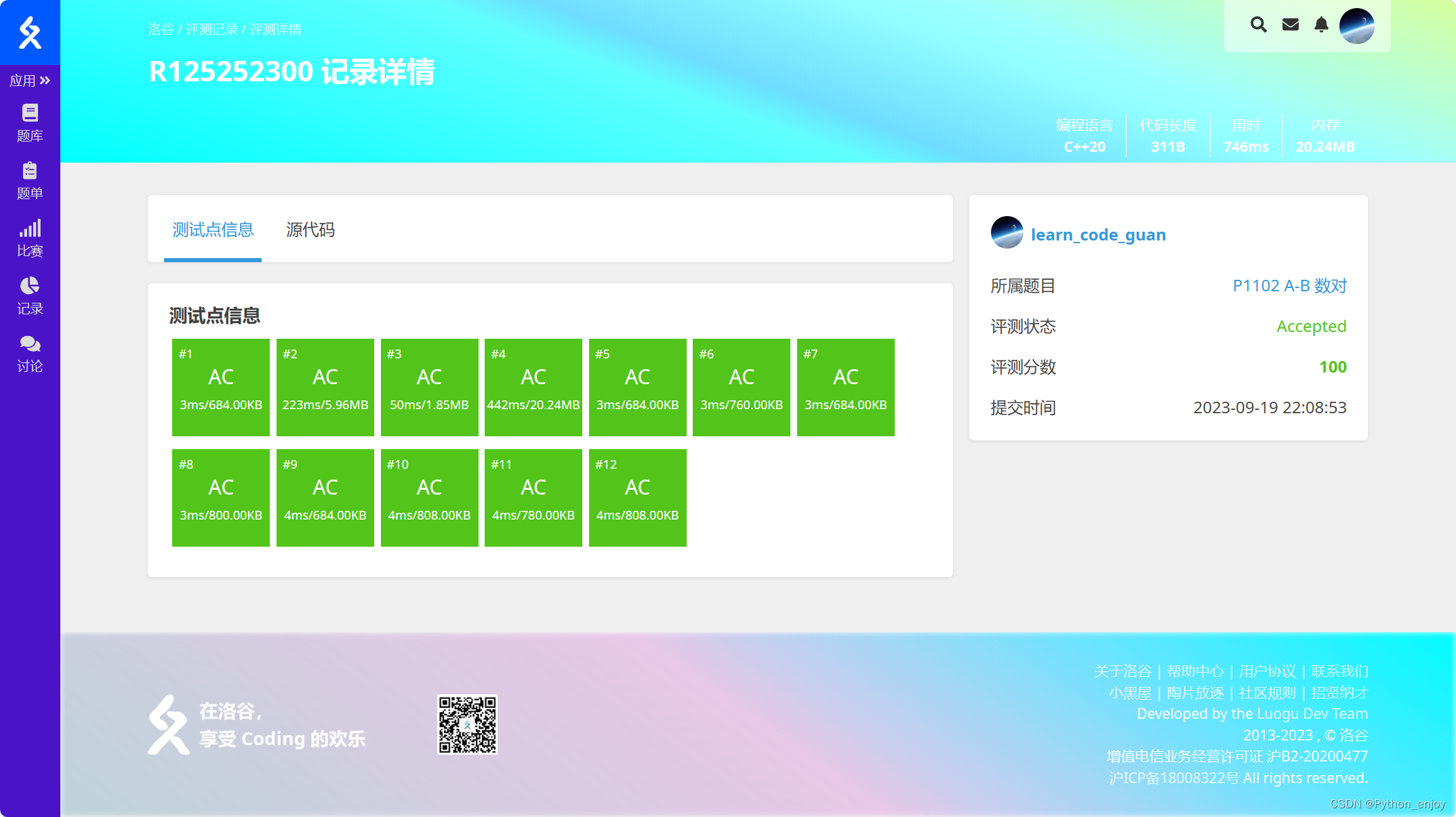
洛谷P1102 A-B 数对题解
目录 题目A-B 数对题目背景题目描述输入格式输出格式样例 #1样例输入 #1样例输出 #1提示传送门 代码解释亲测 题目 A-B 数对 题目背景 出题是一件痛苦的事情! 相同的题目看多了也会有审美疲劳,于是我舍弃了大家所熟悉的 AB Problem,改用 …...
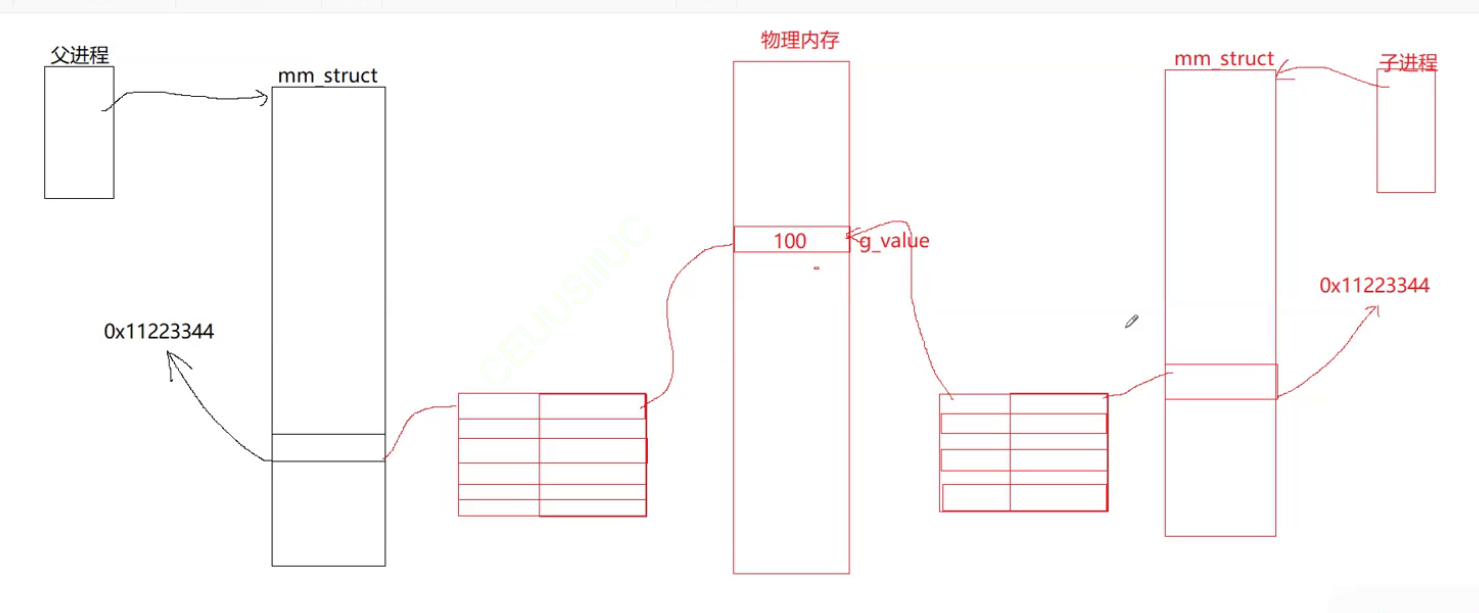
【Linux进行时】进程地址空间
进程地址空间 例子引入: 我们在讲C语言的时候,老师给大家画过这样的空间布局图,但是我们对它不了解 我们写一个代码来验证Linux进程地址空间 #include<stdio.h> #include<assert.h> #include<unistd.h> int g_value100; …...
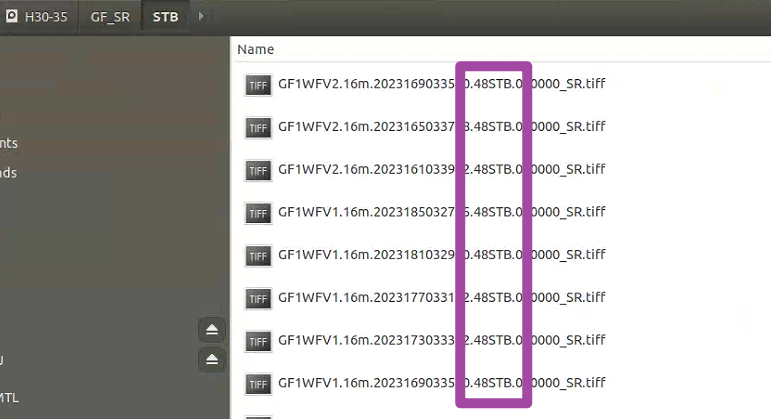
批量将文件名称符合要求的文件自动复制到新文件夹:Python实现
本文介绍基于Python语言,读取一个文件夹,并将其中每一个子文件夹内符合名称要求的文件加以筛选,并将筛选得到的文件复制到另一个目标文件夹中的方法。 本文的需求是:现在有一个大的文件夹,其中含有多个子文件夹&#x…...
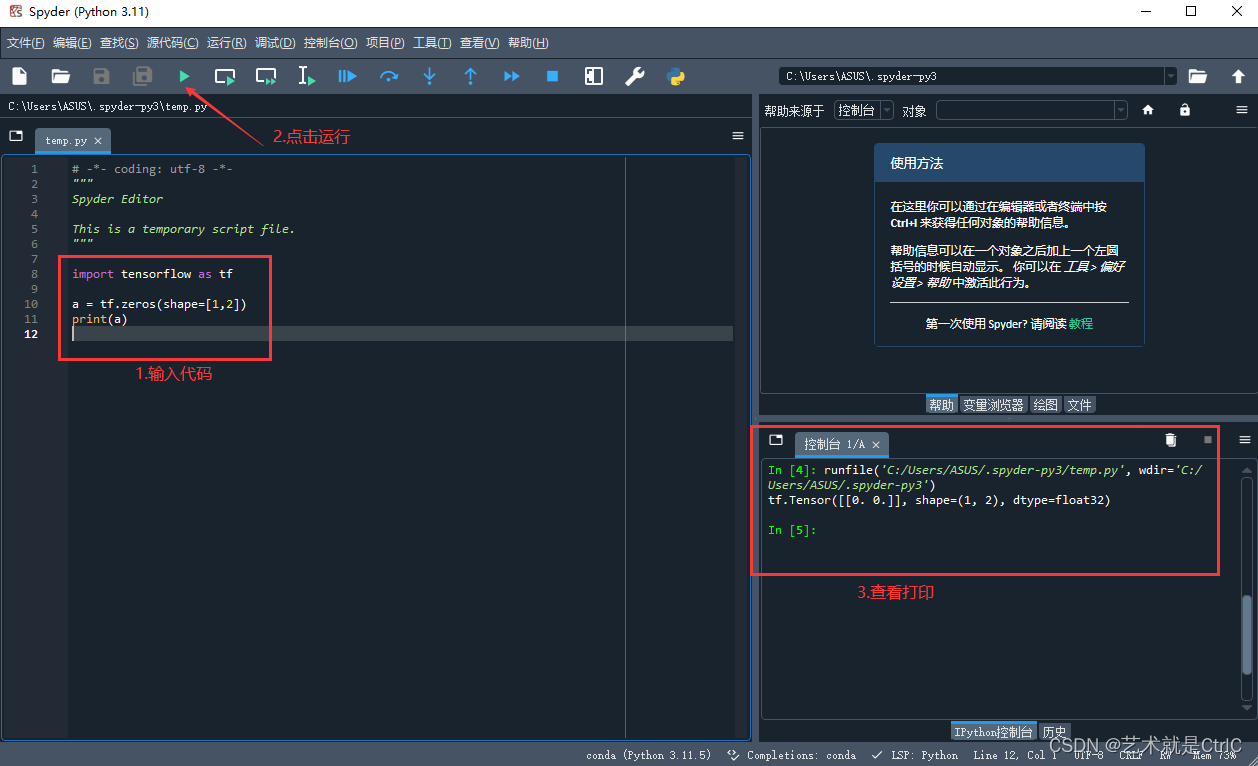
TensorFlow入门(一、环境搭建)
一、下载安装Anaconda 下载地址:http://www.anaconda.comhttp://www.anaconda.com 下载完成后运行exe进行安装 二、下载cuda 下载地址:http://developer.nvidia.com/cuda-downloadshttp://developer.nvidia.com/cuda-downloads 下载完成后运行exe进行安装 安装后winR cmd进…...
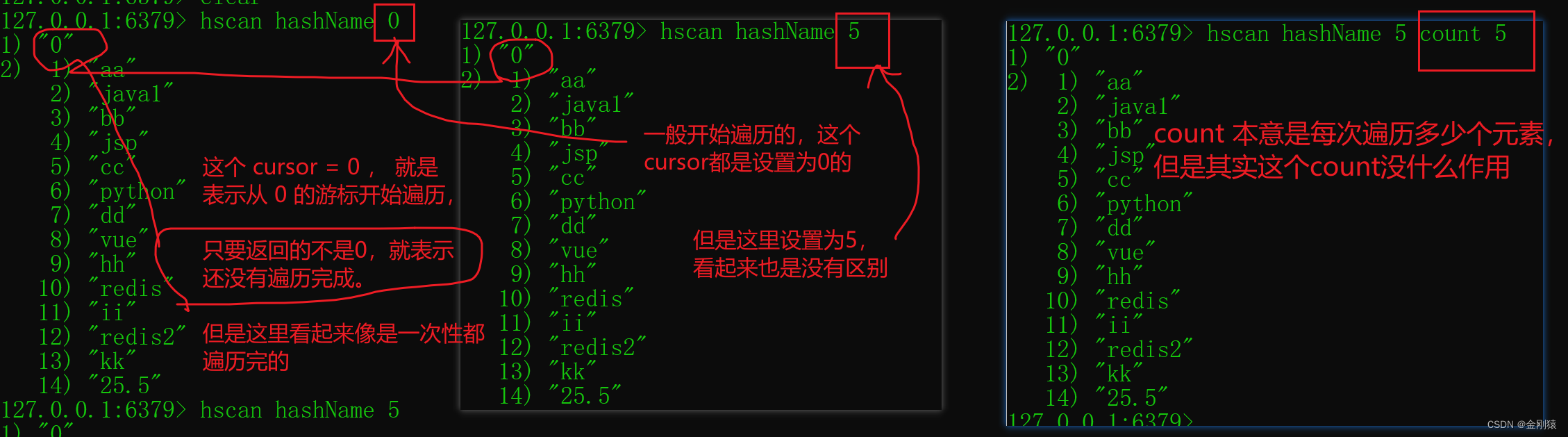
90、Redis 的 value 所支持的数据类型(String、List、Set、Zset、Hash)---->Hash 相关命令
本次讲解要点: Hash 相关命令:是指value中的数据类型 启动redis服务器: 打开小黑窗: C:\Users\JH>e: E:>cd E:\install\Redis6.0\Redis-x64-6.0.14\bin E:\install\Redis6.0\Redis-x64-6.0.14\bin>redis-server.exe red…...

我开源了一个加密算法仓库,支持18种算法!登录注册业务可用!
文章目录 仓库地址介绍安装用法SHA512HMACBcryptScryptAESRSAECC 仓库地址 仓库地址:https://github.com/palp1tate/go-crypto-guard 欢迎star和fork! 介绍 此存储库包含用 Go 编写的全面的密码哈希库。该库支持多种哈希算法,它允许可定制…...
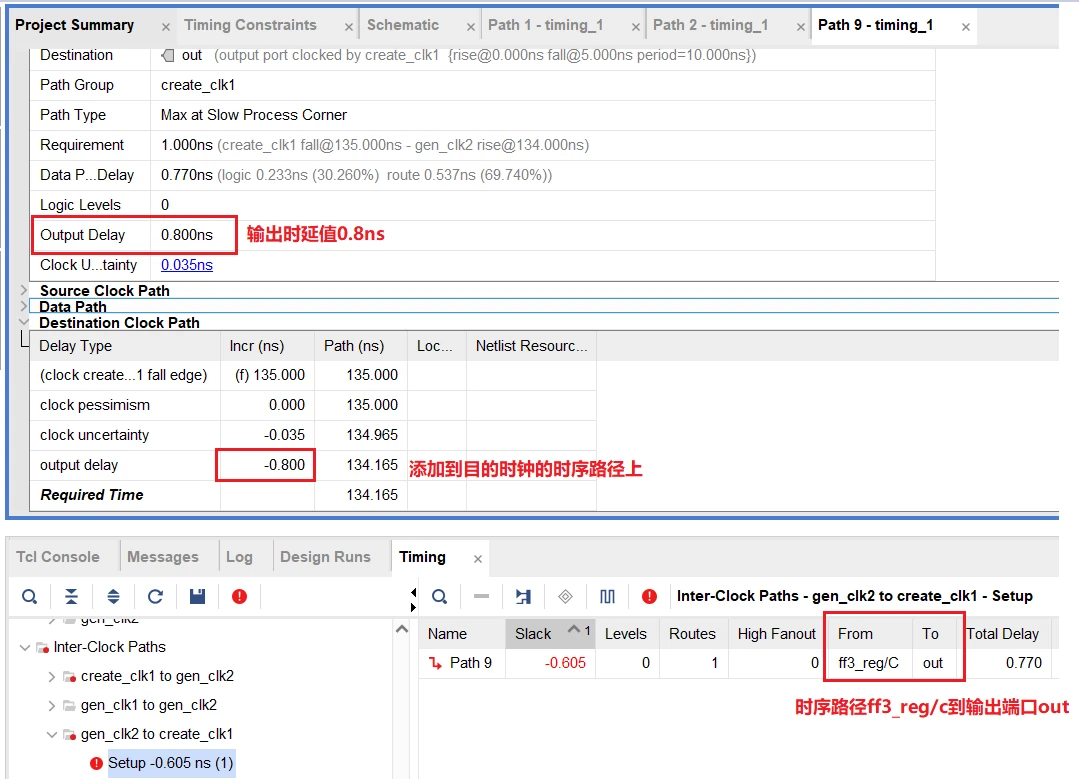
FPGA设计时序约束二、输入延时与输出延时
目录 一、背景 二、set_input_delay 2.1 set_input_delay含义 2.2 set_input_delay参数说明 2.3 使用样例 三、set_output_delay 3.1 set_output_delay含义 3.2 set_output_delay参数说明 3.3 使用样例 四、样例工程 4.1 工程代码 4.2 时序报告 五、参考资料 一、…...
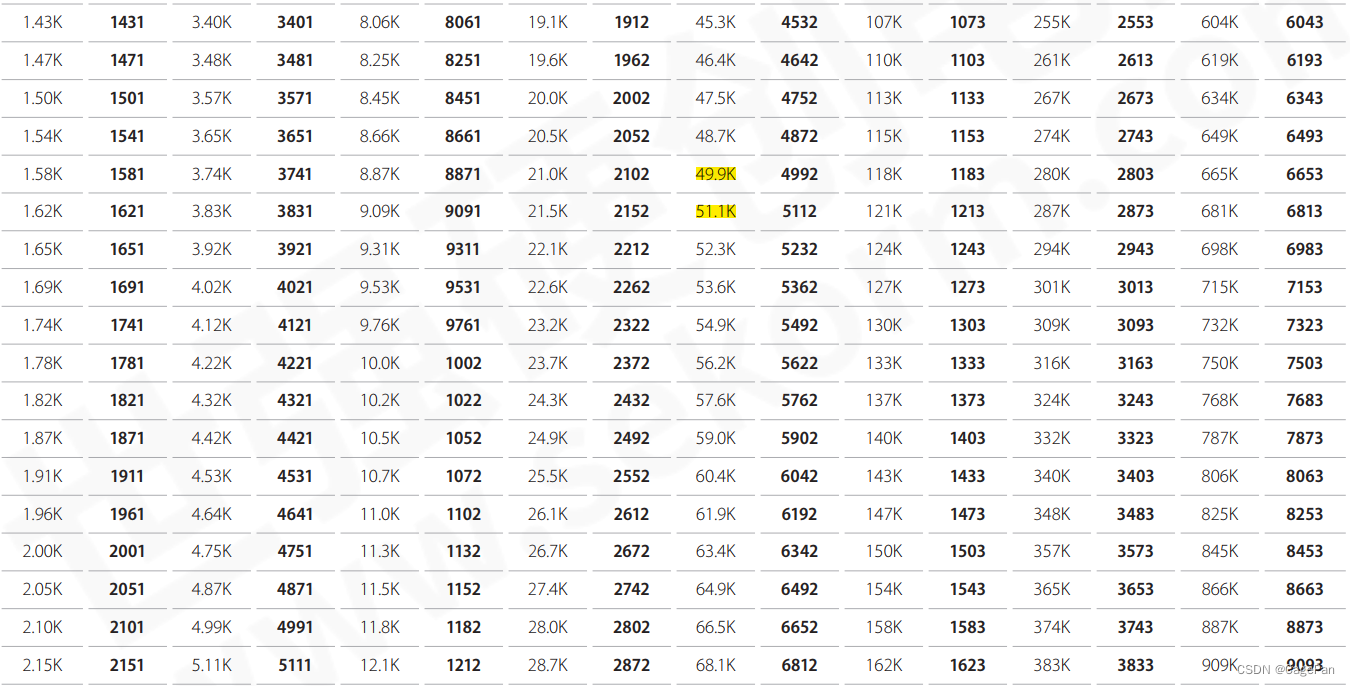
电阻的基础与应用
文章目录 电阻的基础与应用电阻的介绍与分类电阻介绍电阻的分类碳膜/金属膜电阻厚膜/薄膜电阻功能性电阻(光敏/热敏/压敏)特殊电阻(绕线电阻/水泥电阻/铝壳电阻) 电阻的主要厂家与介绍国外厂家VISHAY(威世)KOA(兴亚)Kyocera(京瓷)…...

5.html表格
<table><tr><th>列1标题</th><th>列2标题</th><th>列3标题</th></tr><tr><td>行1列1</td><td>行1列2</td><td>行1列3</td></tr><tr><td>行2列1</td>…...
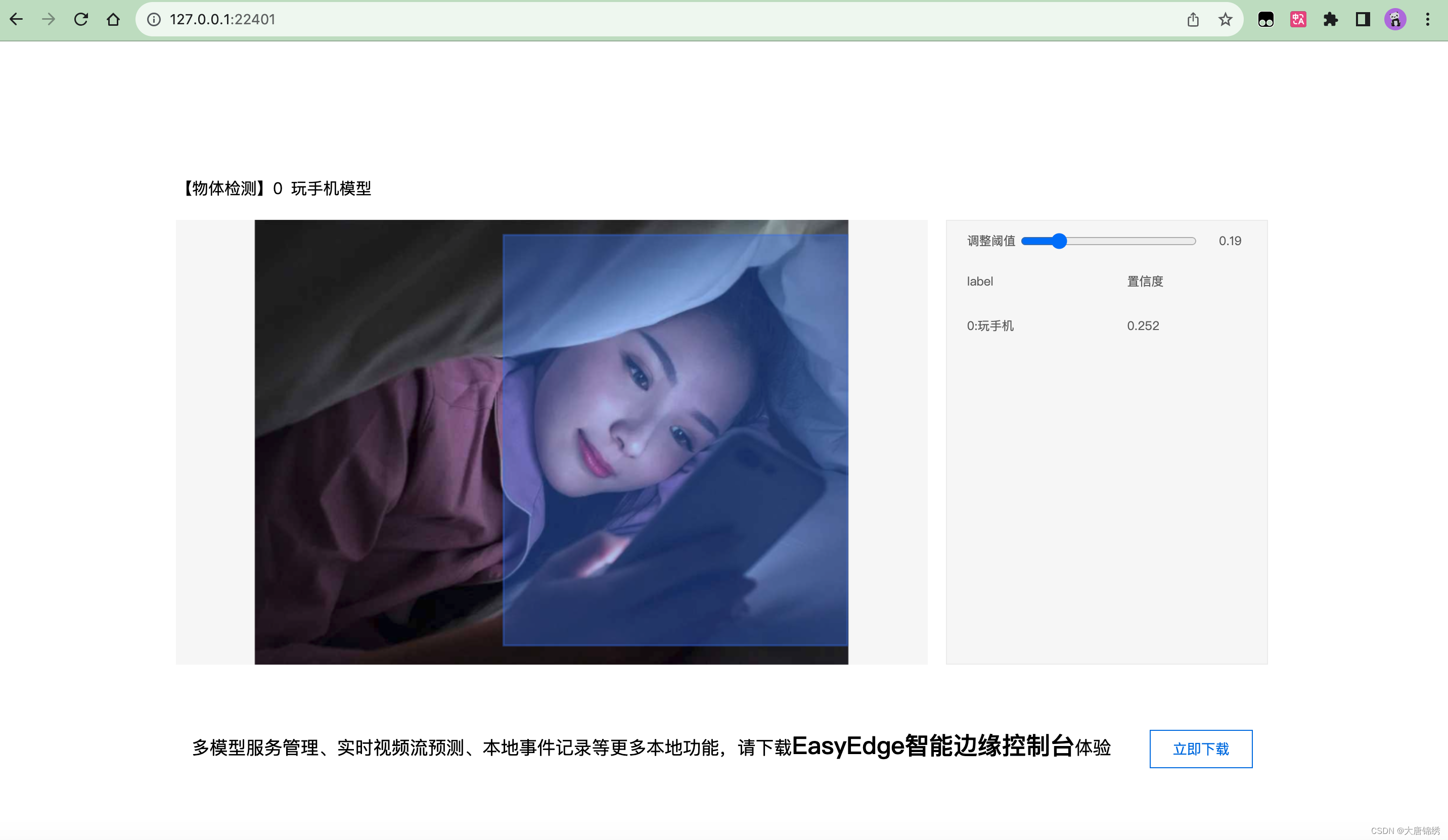
飞桨EasyDL-Mac本地部署离线SDK-Linux集成Python
前言:本文对使用飞桨EasyDL桌面版实现本地部署物体检测做一下说明 一、训练模型 如何使用飞桨EasyDL桌面版这里就不再赘述,直接参照官方文档进行物体检测模型训练。 飞桨EasyDL桌面版-用零代码开发实现物体检测https://ai.baidu.com/ai-doc/EASYDL/Tl2…...

【kubernetes】Kubernetes中的DaemonSet使用
目录 1 为什么需要DaemonSet2 DaemonSet的Yaml的关键字段3 DaemonSet的使用4 一种自行控制Pod更新的方式5 总结 1 为什么需要DaemonSet Deployment可以用于部署无状态的应用,例如系统的接口层或者逻辑层,而多个Pod可以用于负载均衡和容灾。如果有这样一…...
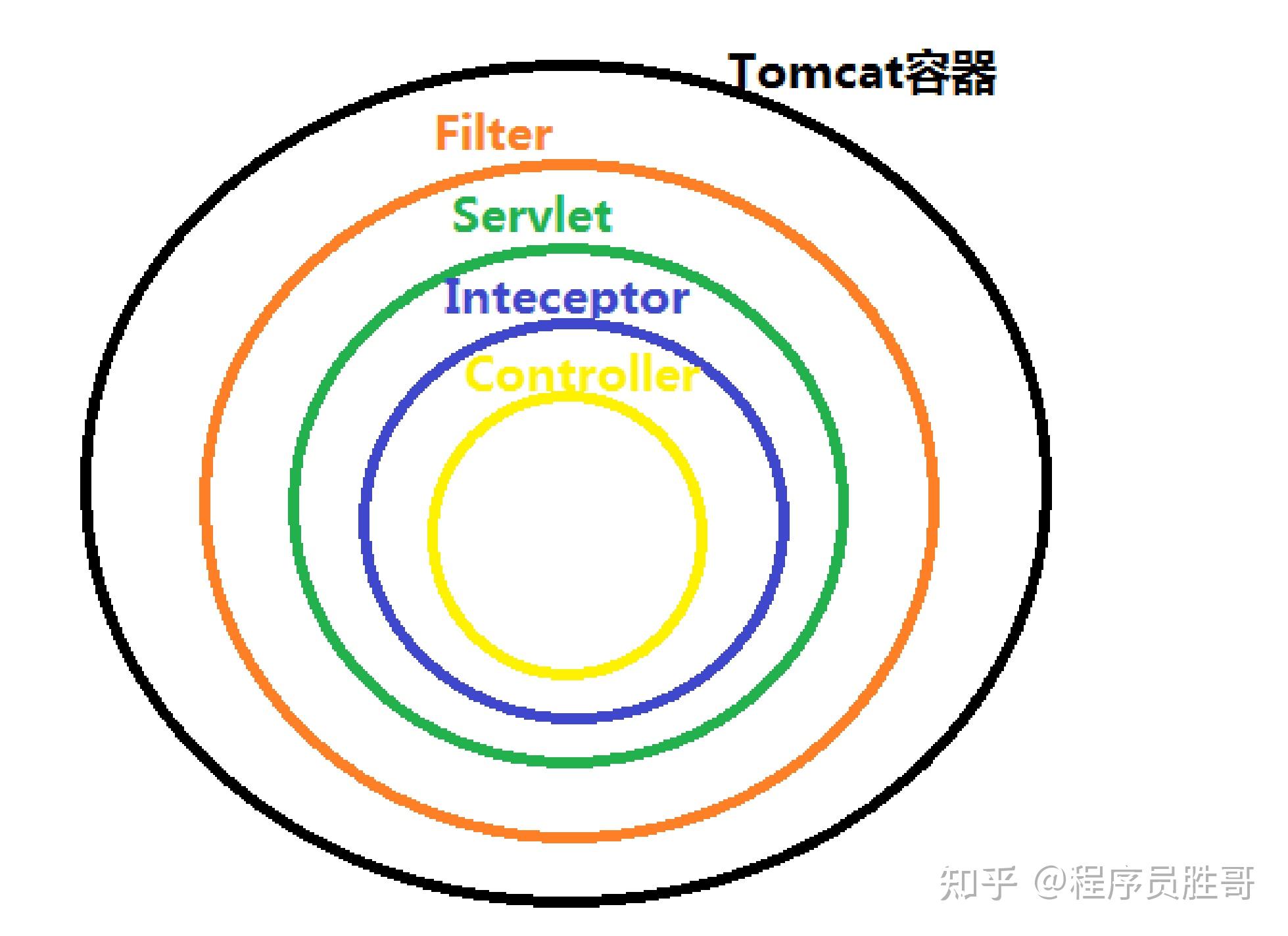
《 新手》web前端(axios)后端(java-springboot)对接简解
文章目录 <font color red>1.何为前后端对接?2.对接中关于http的关键点2.1. 请求方法2.2. 请求参数设置简解: 3.对接中的跨域(CROS)问题**为什么后端处理跨域尽量在业务之前进行?**3.总结 1.何为前后端对接? “前后端对接” 是指前端和后端两个…...
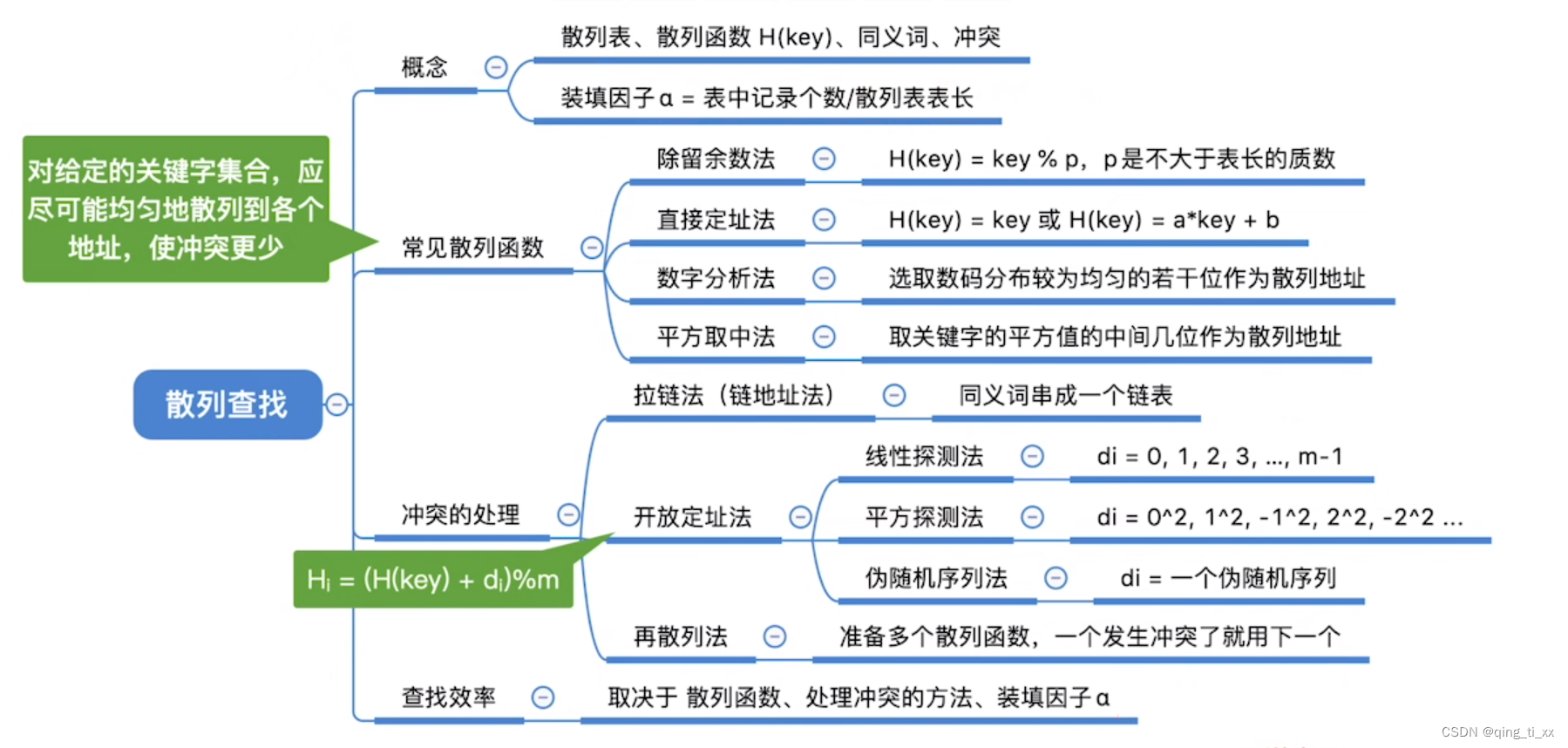
第七章 查找 十、散列查找
一、哈希表(散列表) 哈希表的数据元素的关键字与其存储地址直接相关。 二、解决冲突的方法 三、散列表中元素的查找 总共对比了3个关键字,所以查找长度为3. 四、查找效率计算 (1)成功的概率 需要对比一次的关键字为…...

第一章 C语言知识补充
求字节数运算符:sizeof 强制类型转换运算符:(类型) 下标运算符:[ ] 函数调用运算符:( ) 算术移位指令 算术移位指令有:算术左移SAL和算术右移SAR。算术移位指令的功能描述如下:…...

【Book And Paper 】
【paper Interactive Segmentation of Radiance Fields 算法设计: 电子版...
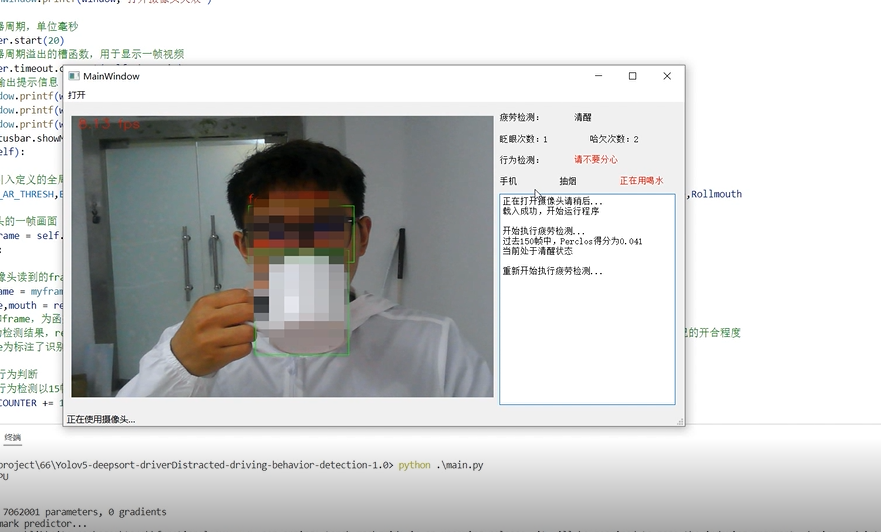
计算机竞赛 深度学习疲劳检测 驾驶行为检测 - python opencv cnn
文章目录 0 前言1 课题背景2 相关技术2.1 Dlib人脸识别库2.2 疲劳检测算法2.3 YOLOV5算法 3 效果展示3.1 眨眼3.2 打哈欠3.3 使用手机检测3.4 抽烟检测3.5 喝水检测 4 最后 0 前言 🔥 优质竞赛项目系列,今天要分享的是 🚩 **基于深度学习加…...

代码随想录 动态规划 13
300. 最长递增子序列 给你一个整数数组 nums ,找到其中最长严格递增子序列的长度。 子序列 是由数组派生而来的序列,删除(或不删除)数组中的元素而不改变其余元素的顺序。例如,[3,6,2,7] 是数组 [0,3,1,6,2,2,7] 的子…...
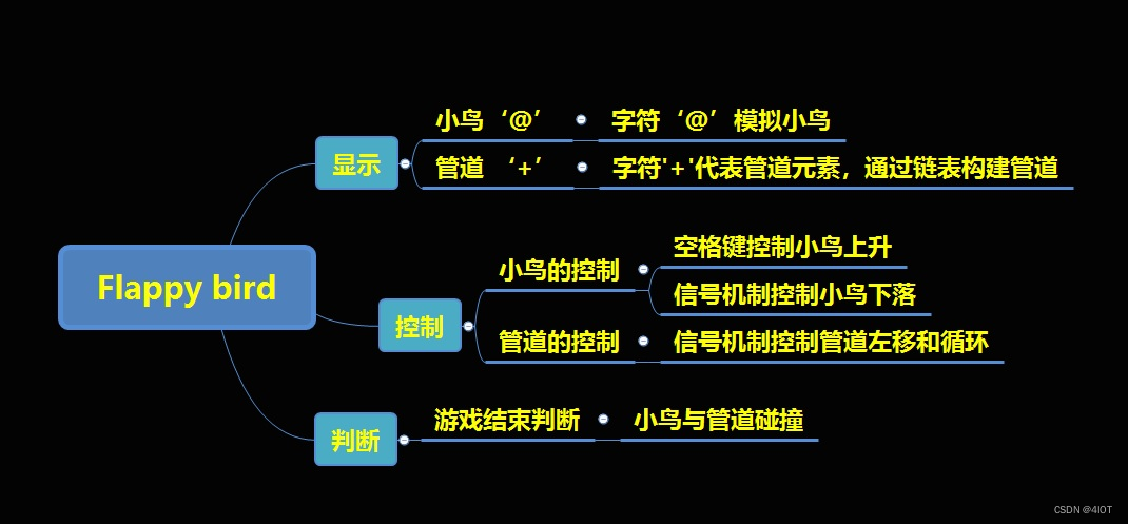
lv6 嵌入式开发-Flappy bird项目
目录 1 项目功能总结 2 知识储备: 3 项目框图 4 Ncurses库介绍 做Flappy bird项目有什么用? 1. 复习、巩固c语言知识 2. 培养做项目的逻辑思维能力 3. 具备开发简单小游戏的能力 学会了Flappy bird项目,贪吃蛇和推房子两款小游戏也可…...

【Java】方法重写
概述 子类中出现了和父类一模一样的方法 当子类需要父类的功能,而功能主体中,子类有自己独特的内容,就可以通过重写父类中的方法,这样即延续了父类的功能,又定义了自己的特有内容 Override 是一个注解,可以…...
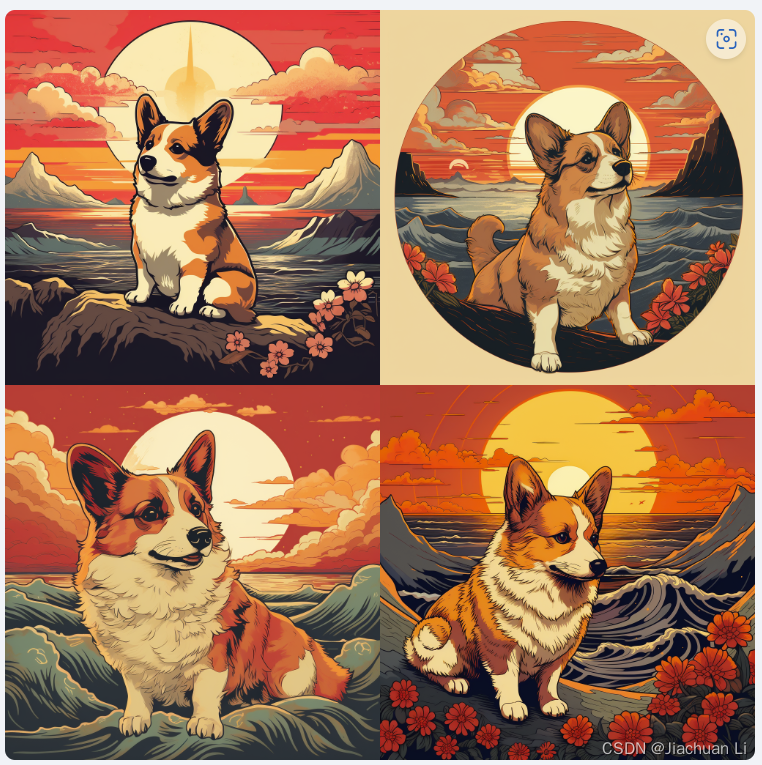
艺术表现形式
abstract expressionism 抽象表现主义 20世纪中期的一种艺术运动,包括多种风格和技巧,特别强调艺术家通过非传统和通常非具象的手段表达态度和情感的自由。 抽象表现主义用有力的笔触和滴落的颜料来表达情感和自发性。 简单地结合“abstract expression…...

PHP 反序列化漏洞:手写序列化文本
文章目录 参考环境序列化文本Scalar Type整数浮点数布尔值字符串 Compound Type数组数据结构序列化文本 对象数据结构序列化文本 Special TypeNULL数据结构序列化文本 手写序列化文本过程中的注意事项个数描述须于现实相符序列化文本前缀的大小写变化符号公共属性 参考 项目描…...
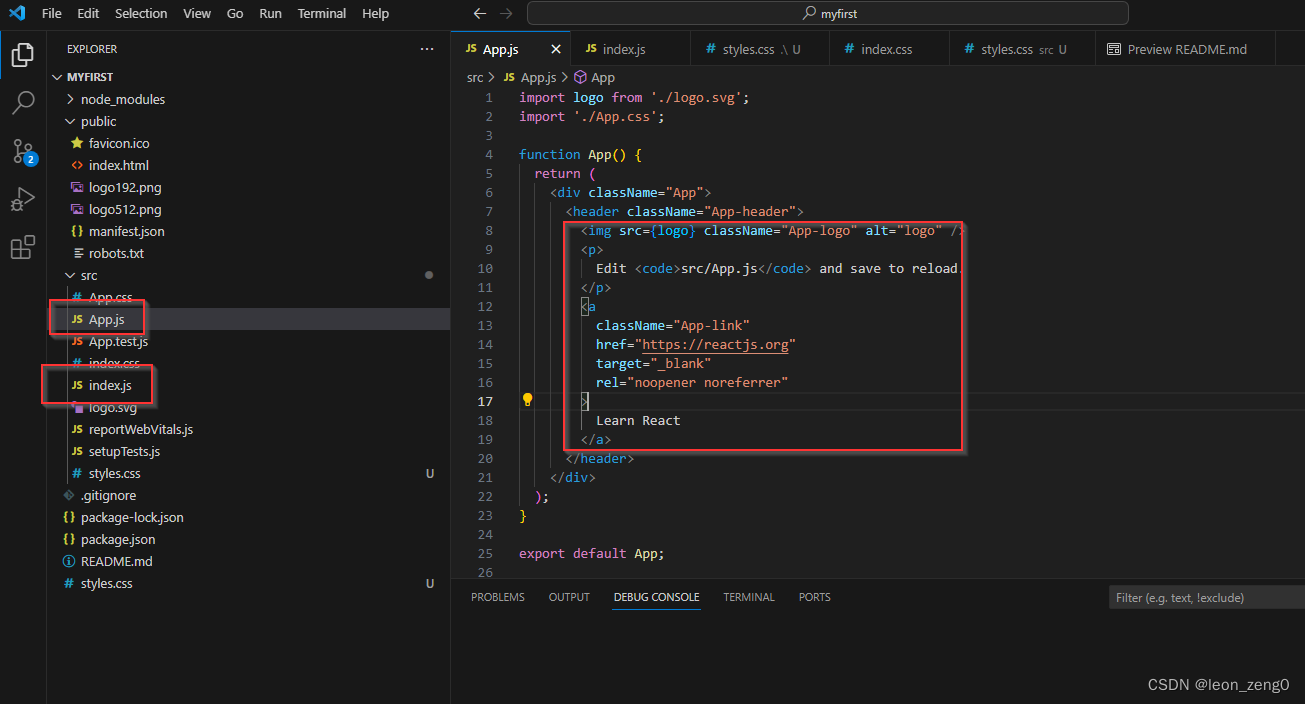
react.js在visual code 下的hello World
想学习reacr.js ,就开始做一个hello world。 我的环境是visual code ,所以我找这个环境下的例子。参照: https://code.visualstudio.com/docs/nodejs/reactjs-tutorial 要学习react.js ,还得先安装node.js,我在visual …...
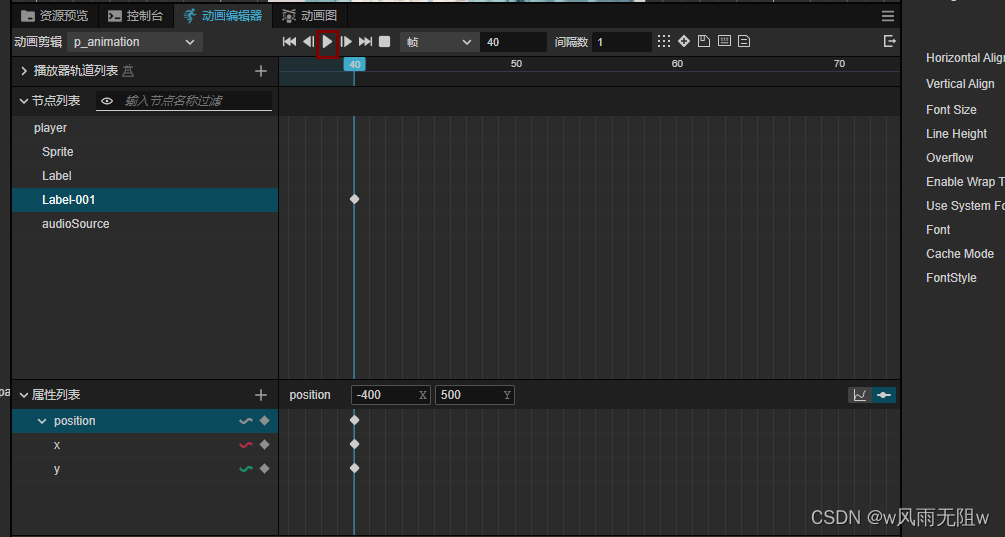
CocosCreator3.8研究笔记(二十四)CocosCreator 动画系统-动画编辑器实操-关键帧实现动态水印动画效果
上一篇,我们介绍了动画编辑器相关功能面板说明,感兴趣的朋友可以前往阅读: CocosCreator3.8研究笔记(二十三)CocosCreator 动画系统-动画编辑器相关功能面板说明。 熟悉了动画编辑器的基础操作,那么再使用动…...
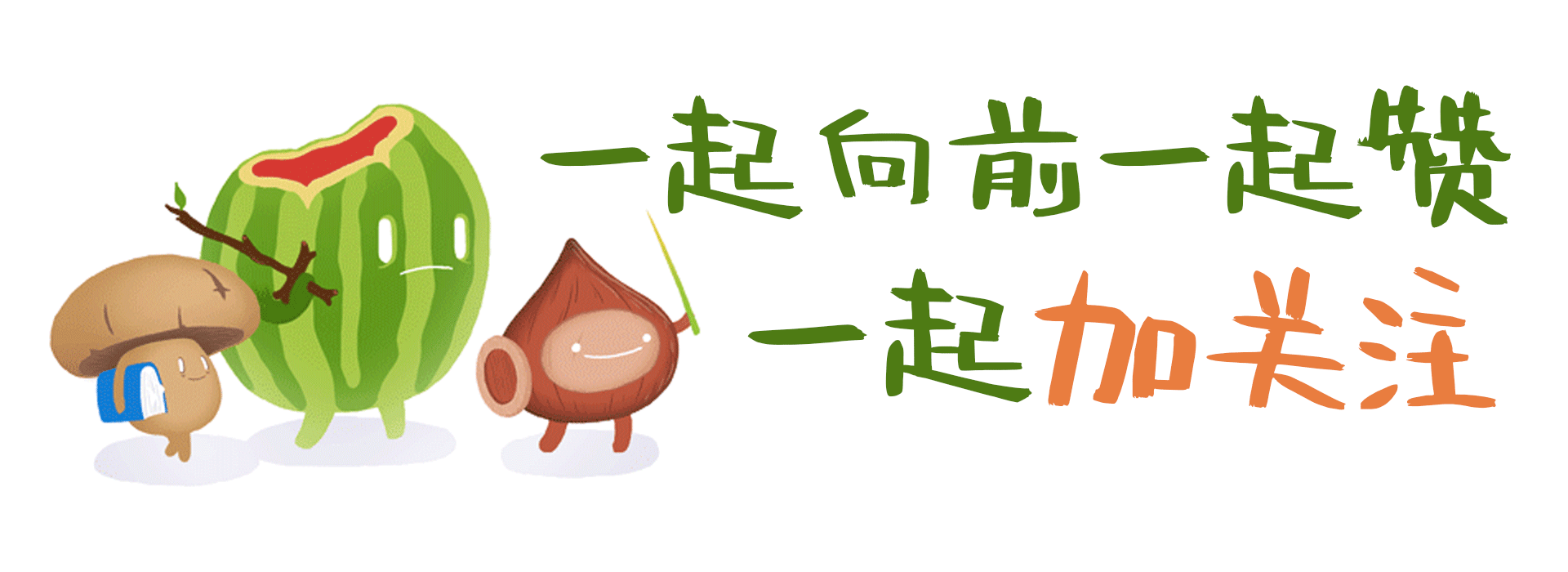
第1篇 目标检测概述 —(3)YOLO系列算法
前言:Hello大家好,我是小哥谈。YOLO(You Only Look Once)系列算法是一种目标检测算法,主要用于实时物体检测。相较于传统的目标检测算法,YOLO具有更快的检测速度和更高的准确率。YOLO系列算法的核心思想是将…...

SpringBoot整合数据库连接
JDBC 1、SQL准备 DROP TABLE IF EXISTS t_book;CREATE TABLE t_book (book_id int(11) NOT NULL,book_name varchar(255) DEFAULT NULL,price int(11) DEFAULT NULL,stock int(11) DEFAULT NULL ) ENGINEInnoDB DEFAULT CHARSETutf8mb4;/*Data for the table t_book */insert…...

uni-app:canvas-绘制图形4(获取画布宽高,根据画布宽高进行图形绘制)
效果 代码 var width ; var height ; const query uni.createSelectorQuery(); //获取宽度 query.select(#firstCanvas).fields({ size: true }, (res) > { width res.width; height res.height; }).exec(); console.log(宽度width); console.log(高…...

EM@坐标@函数@图象的对称和翻折变换
文章目录 abstract翻折变换关于坐标轴翻折 f ( − x ) , f ( x ) f(-x),f(x) f(−x),f(x) − f ( x ) , f ( x ) -f(x),f(x) −f(x),f(x) 偶函数奇函数小结 其他翻折变换关于 y x y\pm x yx对称的直角坐标 关于 x u 对称 关于xu对称 关于xu对称的函数关于 y v yv yv对称的两…...

Python之json模块
JSON (JavaScript Object Notation),由 RFC 7159 (它取代了 RFC 4627) 和 ECMA-404 指定,是一个受 JavaScript 的对象字面值句法启发的轻量级数据交换格式。JSON独立于编程语言的文本格式来存储和表示数据,现在大部分的数据传输基本使用的都是…...

机器学习---BP算法
1. 多级网络 层号确定层的高低:层号较小者,层次较低,层号较大者,层次较高。 输入层:被记作第0层。该层负责接收来自网络外部的信息。 第j层:第j-1层的直接后继层(j>0)ÿ…...