CountDownLatch 批量更改使用,
代码
import com.baomidou.mybatisplus.core.conditions.query.QueryWrapper;
import com.baomidou.mybatisplus.extension.service.impl.ServiceImpl;
import com.first.pet.platform.entity.PlatformAddress;
import com.first.pet.platform.mapper.PlatformAddressMapper;
import com.first.pet.platform.service.IPlatformAddressServiceTest;
import com.first.pet.threadPool.ThreadPoolUtils;
import net.sourceforge.pinyin4j.PinyinHelper;
import net.sourceforge.pinyin4j.format.HanyuPinyinCaseType;
import net.sourceforge.pinyin4j.format.HanyuPinyinOutputFormat;
import net.sourceforge.pinyin4j.format.HanyuPinyinToneType;
import net.sourceforge.pinyin4j.format.exception.BadHanyuPinyinOutputFormatCombination;
import org.springframework.stereotype.Service;
import org.springframework.transaction.annotation.Transactional;import javax.annotation.Resource;
import java.util.List;
import java.util.concurrent.CountDownLatch;/*** <p>* 服务类* </p>** @author yangquan* @since 2023-09-26*/
@Service
public class IPlatformAddressServiceTestImpl extends ServiceImpl<PlatformAddressMapper, PlatformAddress> implements IPlatformAddressServiceTest {@Resourceprivate PlatformAddressMapper platformAddressMapper;//每次查询500条数据操作/*** 组装数据*/@Override@Transactional(rollbackFor = Exception.class)public void assembleAddressData() throws InterruptedException {// 1.创建任务计数器 参数为设置任务数量//开三个线程,一个线程执行500条CountDownLatch countDownLatch = new CountDownLatch(4);// 2.开启三个线程 分别执行三个查询// 查询 性别信息ThreadPoolUtils.sqlThreadPool.submit(new Runnable() {@Overridepublic void run() {QueryWrapper<PlatformAddress> addressWrapper = new QueryWrapper<>();addressWrapper.last("limit 1500,500");List<PlatformAddress> platformAddresses = platformAddressMapper.selectList(addressWrapper);List<PlatformAddress> platformAddress = getPlatformAddress(platformAddresses);platformAddressMapper.updateDataById(platformAddress);// 得到查询结果// 计数器减一countDownLatch.countDown();}});// 查询 地区分布ThreadPoolUtils.sqlThreadPool.submit(new Runnable() {@Overridepublic void run() {QueryWrapper<PlatformAddress> addressWrapper = new QueryWrapper<>();addressWrapper.last("limit 2000,500");List<PlatformAddress> platformAddresses = platformAddressMapper.selectList(addressWrapper);List<PlatformAddress> platformAddress = getPlatformAddress(platformAddresses);platformAddressMapper.updateDataById(platformAddress);// 得到查询结果// 计数器减一countDownLatch.countDown();}});// 查询 注册量ThreadPoolUtils.sqlThreadPool.submit(new Runnable() {@Overridepublic void run() {QueryWrapper<PlatformAddress> addressWrapper = new QueryWrapper<>();addressWrapper.last("limit 2500,500");List<PlatformAddress> platformAddresses = platformAddressMapper.selectList(addressWrapper);List<PlatformAddress> platformAddress = getPlatformAddress(platformAddresses);platformAddressMapper.updateDataById(platformAddress);// 得到查询结果// 计数器减一countDownLatch.countDown();}});// 查询 注册量ThreadPoolUtils.sqlThreadPool.submit(new Runnable() {@Overridepublic void run() {QueryWrapper<PlatformAddress> addressWrapper = new QueryWrapper<>();addressWrapper.last("limit 3000,500");List<PlatformAddress> platformAddresses = platformAddressMapper.selectList(addressWrapper);List<PlatformAddress> platformAddress = getPlatformAddress(platformAddresses);platformAddressMapper.updateDataById(platformAddress);// 得到查询结果// 计数器减一countDownLatch.countDown();}});// await() 当计数器为0的时候 主线程向下执行 没有这一步的话,如果一旦主线程向下执行// return map map中可能有的开启的线程还没有执行完毕,即返回的不是线程执行后的结果countDownLatch.await();}private List<PlatformAddress> getPlatformAddress(List<PlatformAddress> platformAddresses) {platformAddresses.stream().forEach(e -> {e.setInitials(ToFirstChar(e.getAddressName()).toUpperCase());e.setCompleteSpelling(ToPinyin(e.getAddressName()));});return platformAddresses;}public static void main(String[] args) {String ss = ToFirstChar("安徽省");System.out.println(ss);}/*** 获取字符串拼音的第一个字母** @param chinese* @return*/public static String ToFirstChar(String chinese) {String pinyinStr = "";
// char[] newChar = chinese.toCharArray(); //转为单个字符char[] newChar = new char[]{chinese.toCharArray()[0]}; //转为单个字符HanyuPinyinOutputFormat defaultFormat = new HanyuPinyinOutputFormat();defaultFormat.setCaseType(HanyuPinyinCaseType.LOWERCASE);defaultFormat.setToneType(HanyuPinyinToneType.WITHOUT_TONE);for (int i = 0; i < newChar.length; i++) {if (newChar[i] > 128) {try {pinyinStr += PinyinHelper.toHanyuPinyinStringArray(newChar[i], defaultFormat)[0].charAt(0);} catch (BadHanyuPinyinOutputFormatCombination e) {e.printStackTrace();}} else {pinyinStr += newChar[i];}}return pinyinStr;}/*** 汉字转为拼音** @param chinese* @return*/public static String ToPinyin(String chinese) {String pinyinStr = "";char[] newChar = chinese.toCharArray();HanyuPinyinOutputFormat defaultFormat = new HanyuPinyinOutputFormat();defaultFormat.setCaseType(HanyuPinyinCaseType.LOWERCASE);defaultFormat.setToneType(HanyuPinyinToneType.WITHOUT_TONE);for (int i = 0; i < newChar.length; i++) {if (newChar[i] > 128) {try {pinyinStr += PinyinHelper.toHanyuPinyinStringArray(newChar[i], defaultFormat)[0];} catch (BadHanyuPinyinOutputFormatCombination e) {e.printStackTrace();}} else {pinyinStr += newChar[i];}}return pinyinStr;}}
批量更改数据sql
<update id="updateDataById" parameterType="java.util.List"><foreach collection="list" item="item" separator=";">update platform_address set initials=#{item.initials},complete_Spelling = #{item.completeSpelling}where id =#{item.id}</foreach>
</update>
数据库连接 必须配置,否则不能批量更改,以下是参考链接
https://blog.csdn.net/carbuser_xl/article/details/127045359
url: jdbc:mysql://rm:5888/first_pet_dev?autoReconnect=true&useUnicode=true&characterEncoding=utf8&serverTimezone=GMT%2B8&useSSL=false&allowMultiQueries=true
拼音依赖
<dependency><groupId>com.belerweb</groupId><artifactId>pinyin4j</artifactId><version>2.5.0</version></dependency>
线程池工具类
import org.apache.tomcat.util.threads.ThreadPoolExecutor;import java.util.concurrent.LinkedBlockingQueue;
import java.util.concurrent.TimeUnit;/*** 描述:* 线程池工具类* - 所有线程的创建与使用请调用此类的方法** @author zhaofeng* @date 2023-08-29*/
public class ThreadPoolUtils {/*** http异步请求* 耗时相对较长,取核心数*2* 阻塞时间60秒* 空闲时间超过60秒后销毁线程*/public static final ThreadPoolExecutor httpThreadPool = new ThreadPoolImpl(8, 16, 60, TimeUnit.SECONDS,new LinkedBlockingQueue<>(1024), new ThreadPoolExecutor.AbortPolicy());/*** 数据库操作请求* 相较http请求耗时较短* 取核心线程数*1* 阻塞时间60秒*/public static final ThreadPoolExecutor sqlThreadPool = new ThreadPoolImpl(4, 8, 60, TimeUnit.SECONDS,new LinkedBlockingQueue<>(1024), new ThreadPoolExecutor.AbortPolicy());}
相关文章:
CountDownLatch 批量更改使用,
代码 import com.baomidou.mybatisplus.core.conditions.query.QueryWrapper; import com.baomidou.mybatisplus.extension.service.impl.ServiceImpl; import com.first.pet.platform.entity.PlatformAddress; import com.first.pet.platform.mapper.PlatformAddressMapper; …...
910数据结构(2019年真题)
算法设计题 问题1 有一种排序算法叫做计数排序。这种排序算法对一个待排序的表(采用顺序存储)进行排序,并将排序结果存放到另一个新的表中。必须注意的是,表中所有待排序的关键字互不相同,计数排序算法针对表中的每个元素,扫描待排序的表一趟,统计表中有多少个元素的关…...
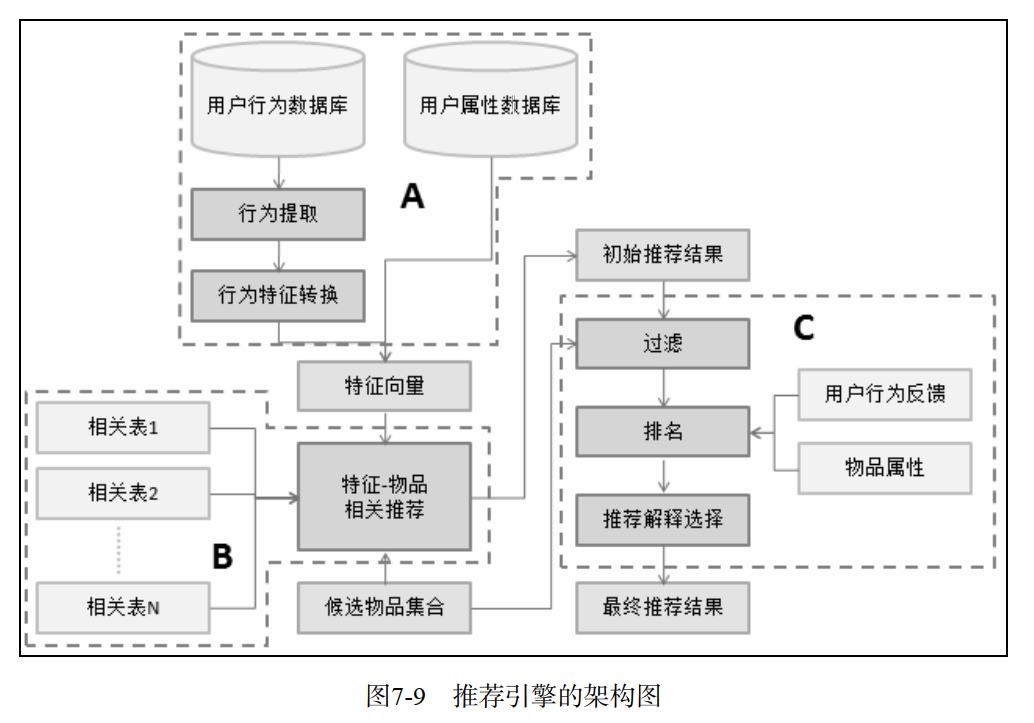
推荐系统实践 笔记
诸神缄默不语-个人CSDN博文目录 这是我2020年写的笔记,我从印象笔记搬过来公开。 如果那年还在读本科的同学也许有印象,那年美赛出了道根据电商评论给商户提建议的题。其实这件事跟推荐系统关系不大,但我们当时病急乱投医,我打开…...
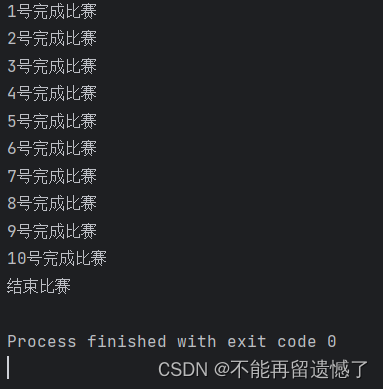
【JavaEE】JUC(Java.util.concurrent)常见类
文章目录 前言ReentrantLock原子类线程池信号量CountDownLatch相关面试题 前言 经过前面文章的学习我们大致了解了如何实现多线程编程和解决多线程编程中遇到的线程不安全问题,java.util.concurrent 是我们多线程编程的一个常用包,那么今天我将为大家分…...
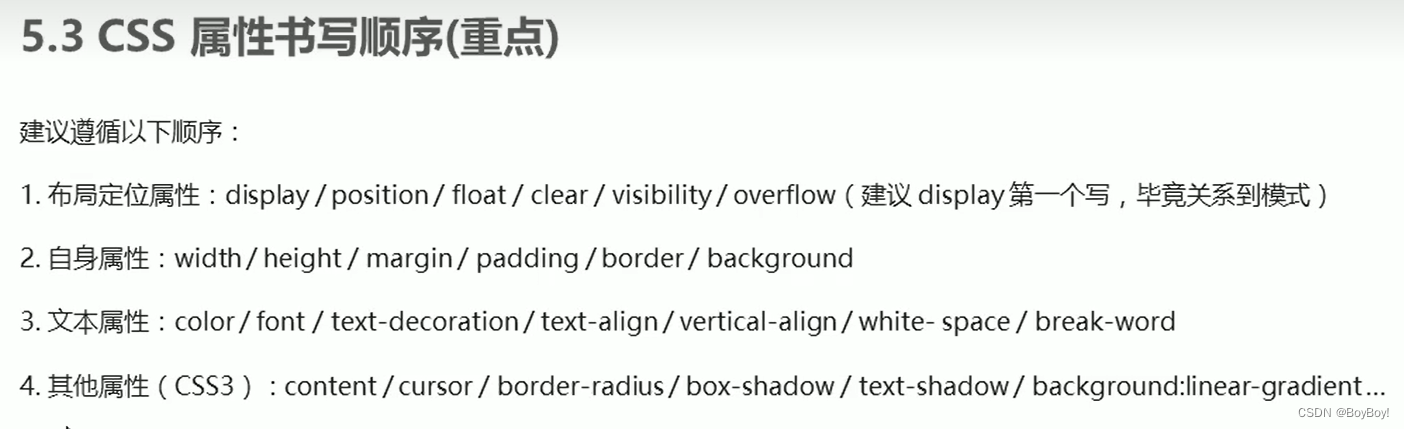
清除浮动的方法
为什么需要清除浮动? 父级的盒子不能把height定死这样,浮动子类就没有了(行内块元素的特点),父类高度为零。故引用清除浮动 1、父级没有高度 2、子盒子浮动了 3、影响下面的布局了,我们就应该清除浮动了…...
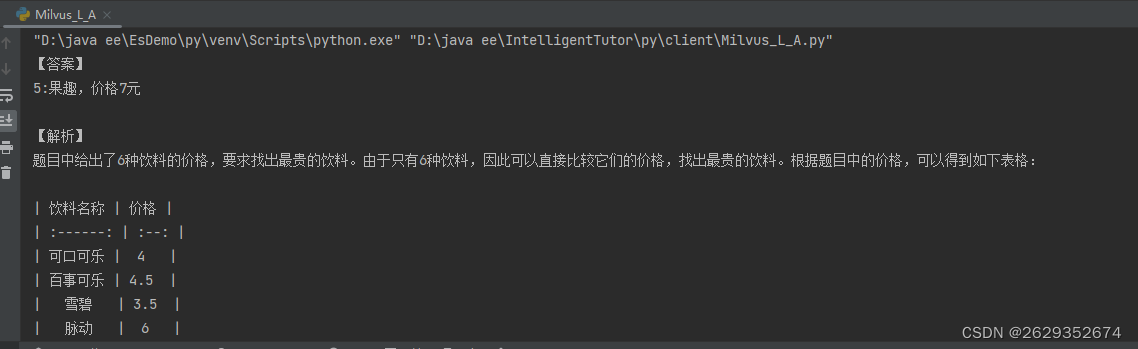
LangChain 摘要 和问答示例
在Azure上的OpenAI端点 注意 OpenAI key 可以用微软 用例【1. 嵌入 ,2. 问答】 1. import os import openai from langchain.embeddings import OpenAIEmbeddings os.environ["OPENAI_API_KEY"] "****" # Azure 的密钥 os.environ["OP…...
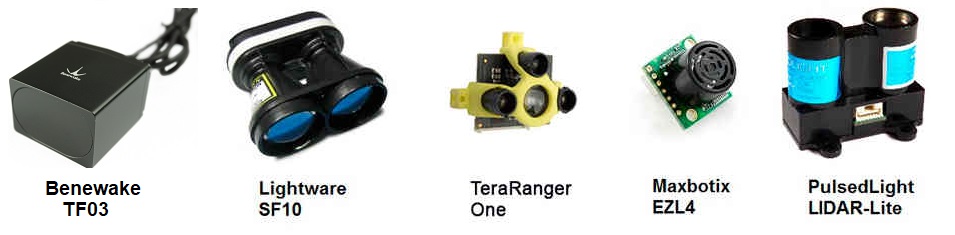
(32)测距仪(声纳、激光雷达、深度摄影机)
文章目录 前言 32.1 单向测距仪 32.2 全向性近距离测距仪 32.3 基于视觉的传感器 前言 旋翼飞机/固定翼/无人车支持多种不同的测距仪,包括激光雷达(使用激光或红外线光束进行距离测量)、360 度激光雷达(可探测多个方向的障碍…...
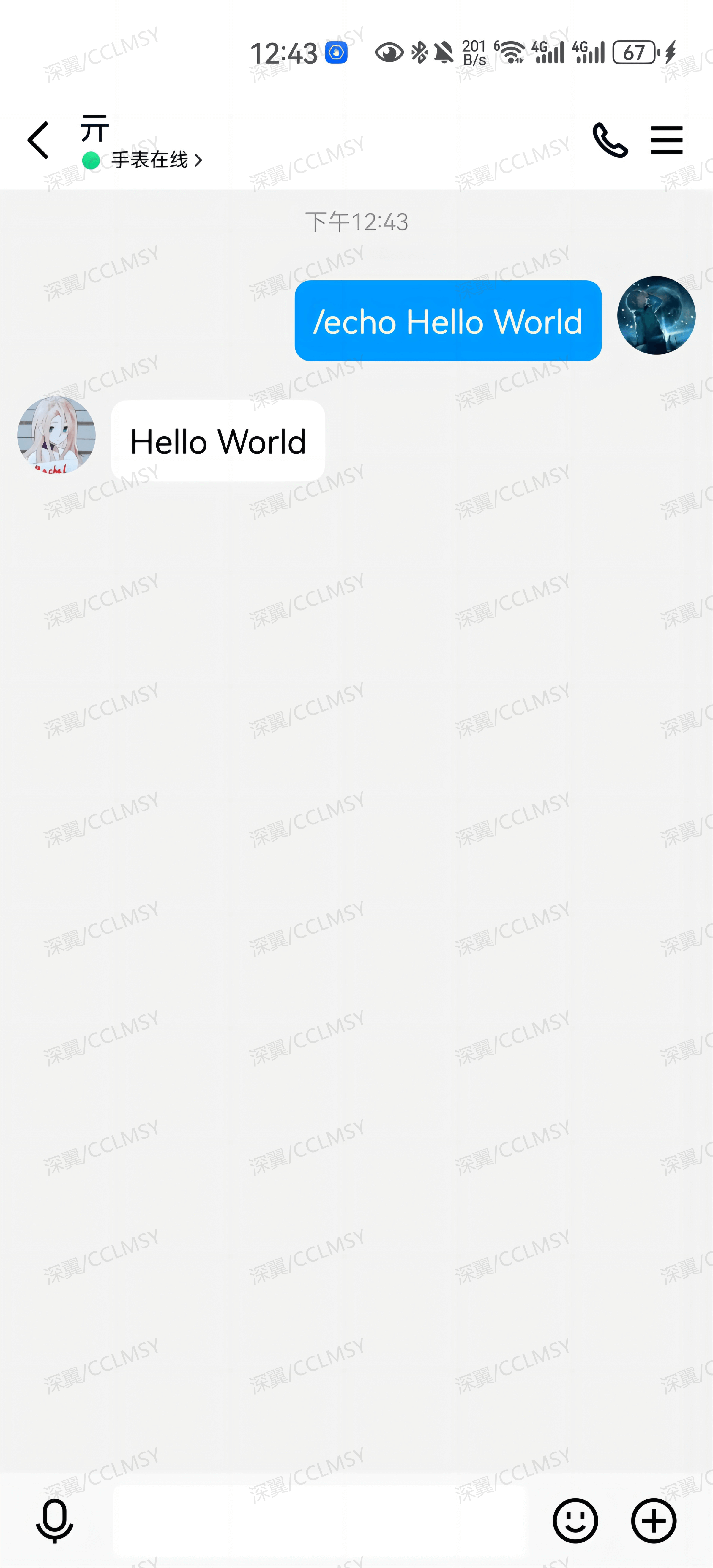
教你拥有一个自己的QQ机器人!0基础超详细保姆级教学!基于NoneBot2 Windows端搭建QQ机器人
0.序言 原文链接:教你本地化部署一个QQ机器人本教程主要面向Windows系统用户教程从0开始全程详细指导,0基础萌新请放心食用🍕如果你遇到了问题,请仔细检查是否哪一步有遗漏。如果你确定自己的操作没问题,可以到原文链…...

智能银行卡明细筛选与统计,轻松掌握账户总花销!
作为现代生活的重要组成部分,银行卡成为了我们日常消费和收入的主要途径。但是,当我们需要了解自己的银行卡账户的总花销时,繁琐的明细筛选和统计工作常常让人头疼。现在,让我们向您推荐一款智能银行卡明细筛选与统计工具…...

SRT服务器SLS
目前互联网上的视频直播有两种,一种是基于RTMP协议的直播,这种直播方式上行推流使用RTMP协议,下行播放使用RTMP,HTTPFLV或者HLS,直播延时一般大于3秒,广泛应用秀场、游戏、赛事和事件直播,满足了…...
Linux 安装 Android SDK
先安装jdk RUN apt-get install default-jdk 参考:http://t.zoukankan.com/braveym-p-6143356.html mkdir -p $HOME/install/android-sdk wget https://dl.google.com/android/repository/commandlinetools-linux-9123335_latest.zip unzip commandlinetools-linu…...
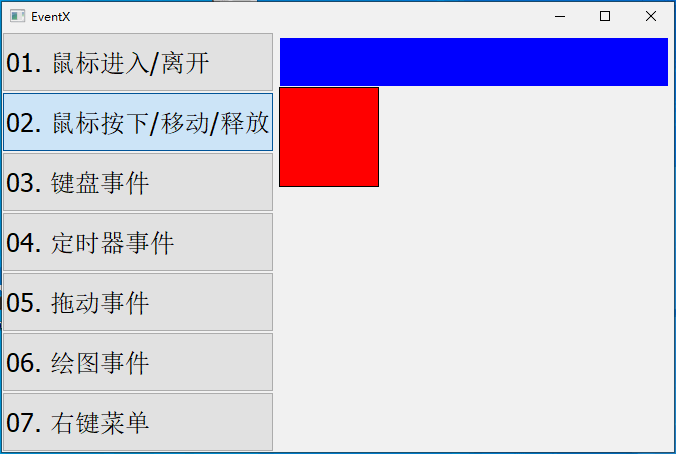
【QT开发笔记-基础篇】| 第四章 事件QEvent | 4.4 鼠标按下、移动、释放事件
本章要实现的整体效果如下: QEvent::MouseButtonPress 鼠标按下时,触发该事件,它对应的子类是 QMouseEvent QEvent::MouseMove 鼠标移动时,触发该事件,它对应的子类是 QMouseEvent QEvent::MouseButtonRel…...
vue3父子通信+ref,toRef,toRefs使用实例
ref是什么? 生成值类型的响应式数据可用于模板和reactive通过.value修改值可以获取DOM元素 <p ref”elemRef”>{{nameRef}} -- {{state.name}}</p> // 获取dom元素 onMounted(()>{ console.log(elemRef.value); }); toRef是什么? 针对一个响应式对象(rea…...
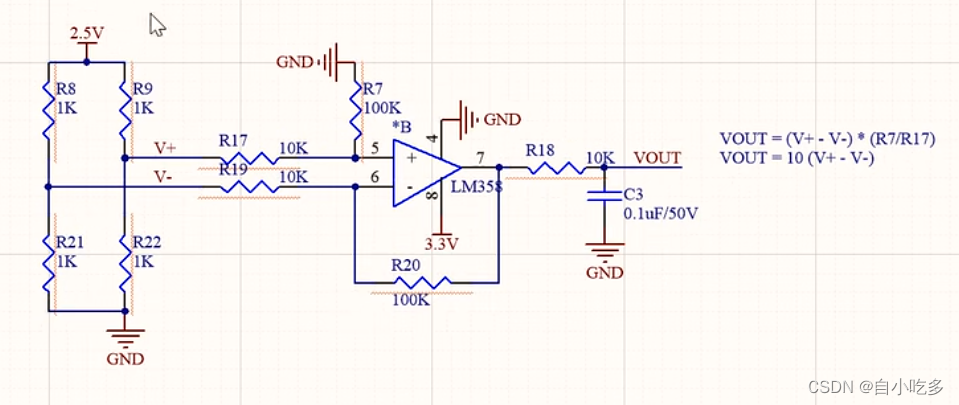
输入电压转化为电流性 5~20mA方案
输入电压转化为电流性 5~20mA方案 方案一方案二方案三 方案一 XTR111是一款精密的电压-电流转换器是最广泛应用之一。原因有二:一是线性度非常好、二是价格便宜。总结成一点,就是性价比高。 典型电路 最终电路 Z1二极管处输出电流表达式:…...
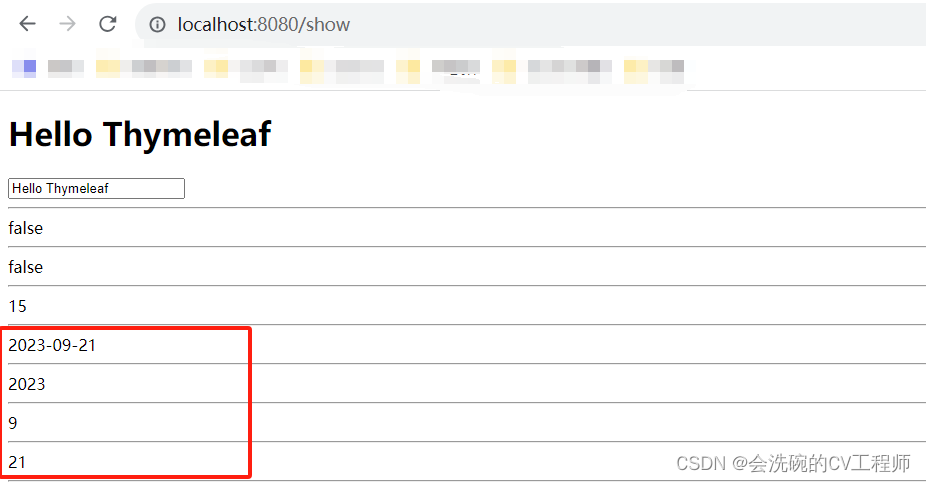
SpringBoot自带模板引擎Thymeleaf使用详解①
目录 前言 一、SpringBoot静态资源相关目录 二、变量输出 2.1 在templates目录下创建视图index.html 2.2 创建对应的Controller 2.3 在视图展示model中的值 三、操作字符串和时间 3.1 操作字符串 3.2 操作时间 前言 Thymeleaf是一款用于渲染XML/HTML5内容的模板引擎&am…...
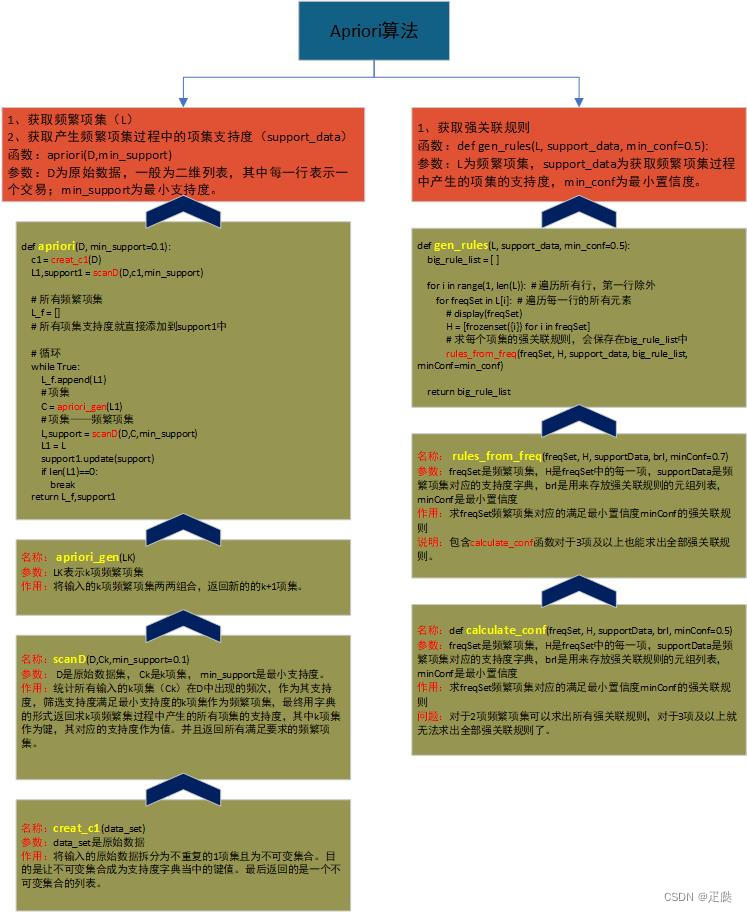
推荐算法——Apriori算法原理
0、前言: 首先名字别读错:an pu ruo ao rui 【拼音发音】Apriori是一种推荐算法推荐系统:从海量数据中,帮助用户进行信息的过滤和选择。主要推荐方法有:基于内容的推荐、协同过滤推荐、基于关联规则的推荐、基于知识的…...
vue ant 隐藏 列
vue ant 隐藏 列 如果你使用的是Vue和Ant Design Vue组件库,你可以使用v-if指令来实现条件渲染来隐藏列。以下是一个示例代码: <template><a-table :columns"columns" :data-source"data"><template v-slot:custom…...
java基础之初始化顺序
初始化顺序 在类中变量定义的顺序决定了它们初始化的顺序。在创建任何java对象时,都是依次调用父类非静态初始化块、父类构造器执行初始化、本类的非静态初始化块、本类构造器执行初始化 public class House { // 构造器之前 Window w1 new Window(1); Ho…...
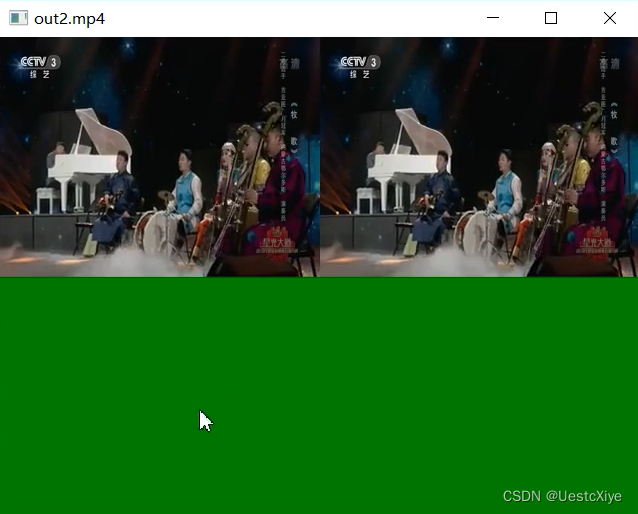
FFmpeg 命令:从入门到精通 | ffmpeg filter(过滤器 / 滤镜)
FFmpeg 命令:从入门到精通 | ffmpeg filter(过滤器 / 滤镜) FFmpeg 命令:从入门到精通 | ffmpeg filter(过滤器 / 滤镜)ffmpeg fliter 基本内置变量视频裁剪文字水印图片水印画中画视频多宫格处理 FFmpeg 命…...
【C语言】23-结构体类型
目录 1. 如何建立结构体类型2. 如何使用结构体2.1 定义结构体变量2.2 结构体变量的初始化和引用2.3 结构体数组2.4 结构体指针2.4.1 指向结构体变量的指针2.4.2 指向结构体数组的指针C 语言提供了一些由系统已定义好的数据类型,如: int、 float、 char 等,用户可以在程序…...
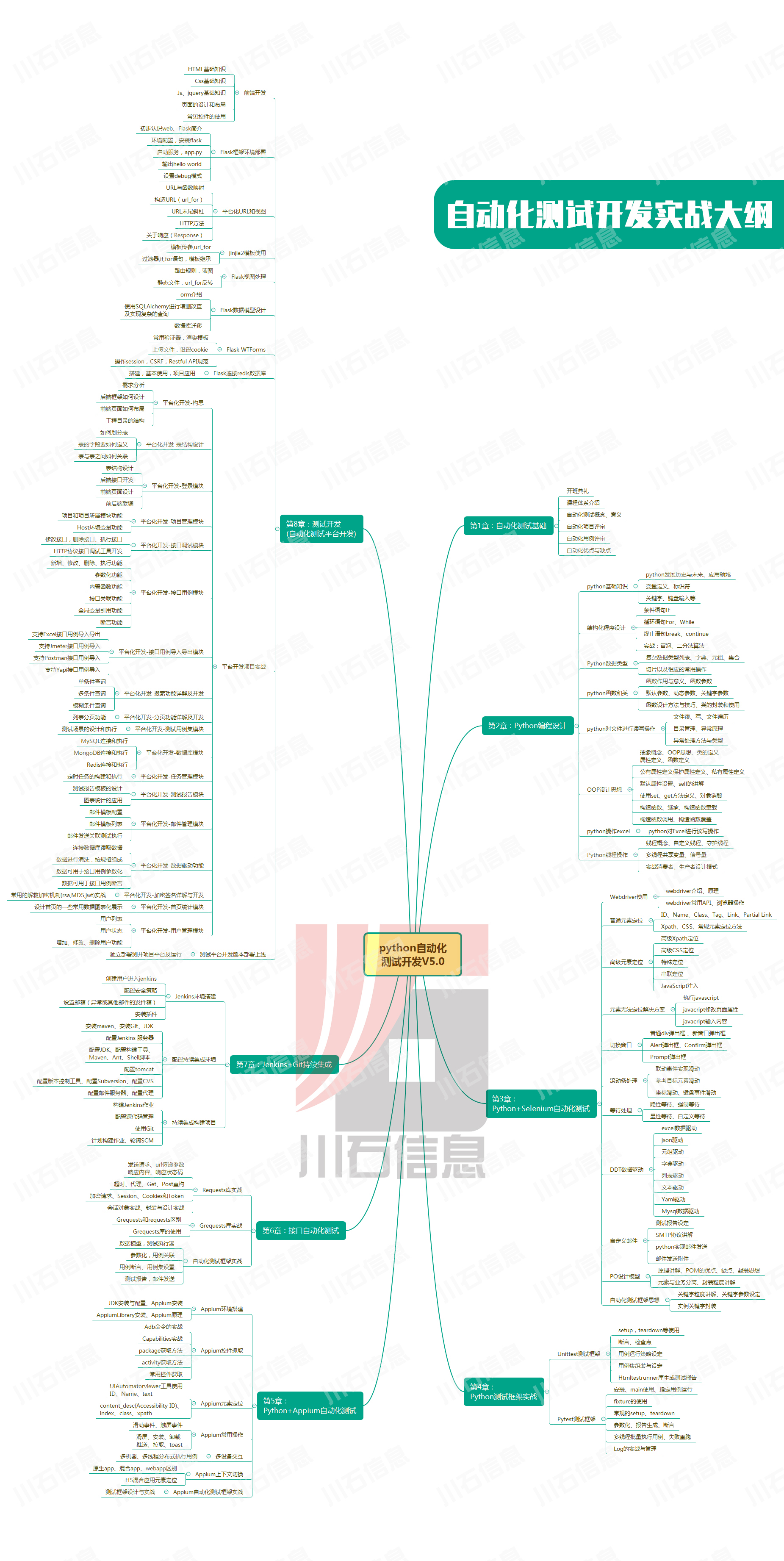
接口测试中缓存处理策略
在接口测试中,缓存处理策略是一个关键环节,直接影响测试结果的准确性和可靠性。合理的缓存处理策略能够确保测试环境的一致性,避免因缓存数据导致的测试偏差。以下是接口测试中常见的缓存处理策略及其详细说明: 一、缓存处理的核…...
生成xcframework
打包 XCFramework 的方法 XCFramework 是苹果推出的一种多平台二进制分发格式,可以包含多个架构和平台的代码。打包 XCFramework 通常用于分发库或框架。 使用 Xcode 命令行工具打包 通过 xcodebuild 命令可以打包 XCFramework。确保项目已经配置好需要支持的平台…...
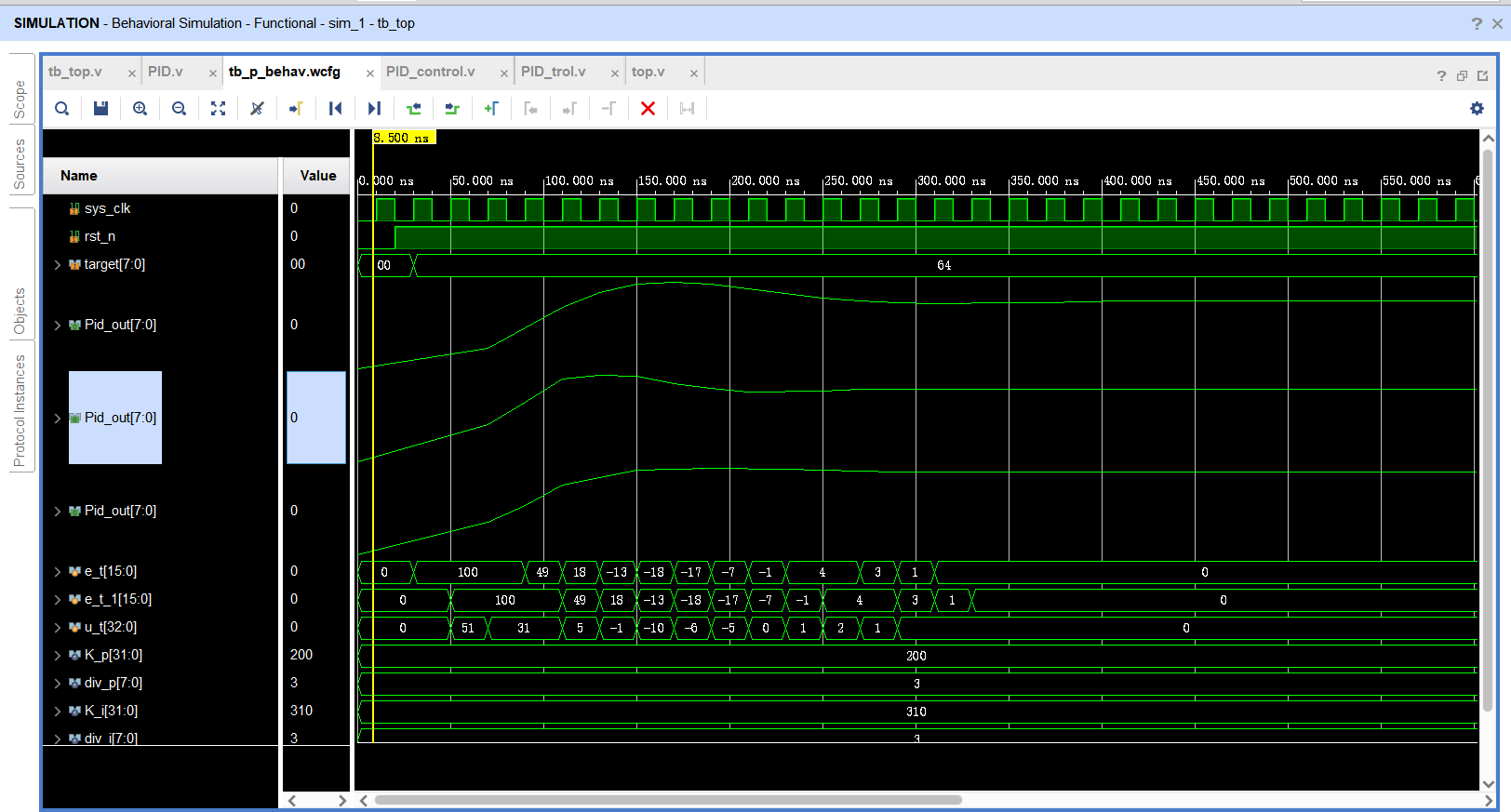
基于FPGA的PID算法学习———实现PID比例控制算法
基于FPGA的PID算法学习 前言一、PID算法分析二、PID仿真分析1. PID代码2.PI代码3.P代码4.顶层5.测试文件6.仿真波形 总结 前言 学习内容:参考网站: PID算法控制 PID即:Proportional(比例)、Integral(积分&…...
进程地址空间(比特课总结)
一、进程地址空间 1. 环境变量 1 )⽤户级环境变量与系统级环境变量 全局属性:环境变量具有全局属性,会被⼦进程继承。例如当bash启动⼦进程时,环 境变量会⾃动传递给⼦进程。 本地变量限制:本地变量只在当前进程(ba…...
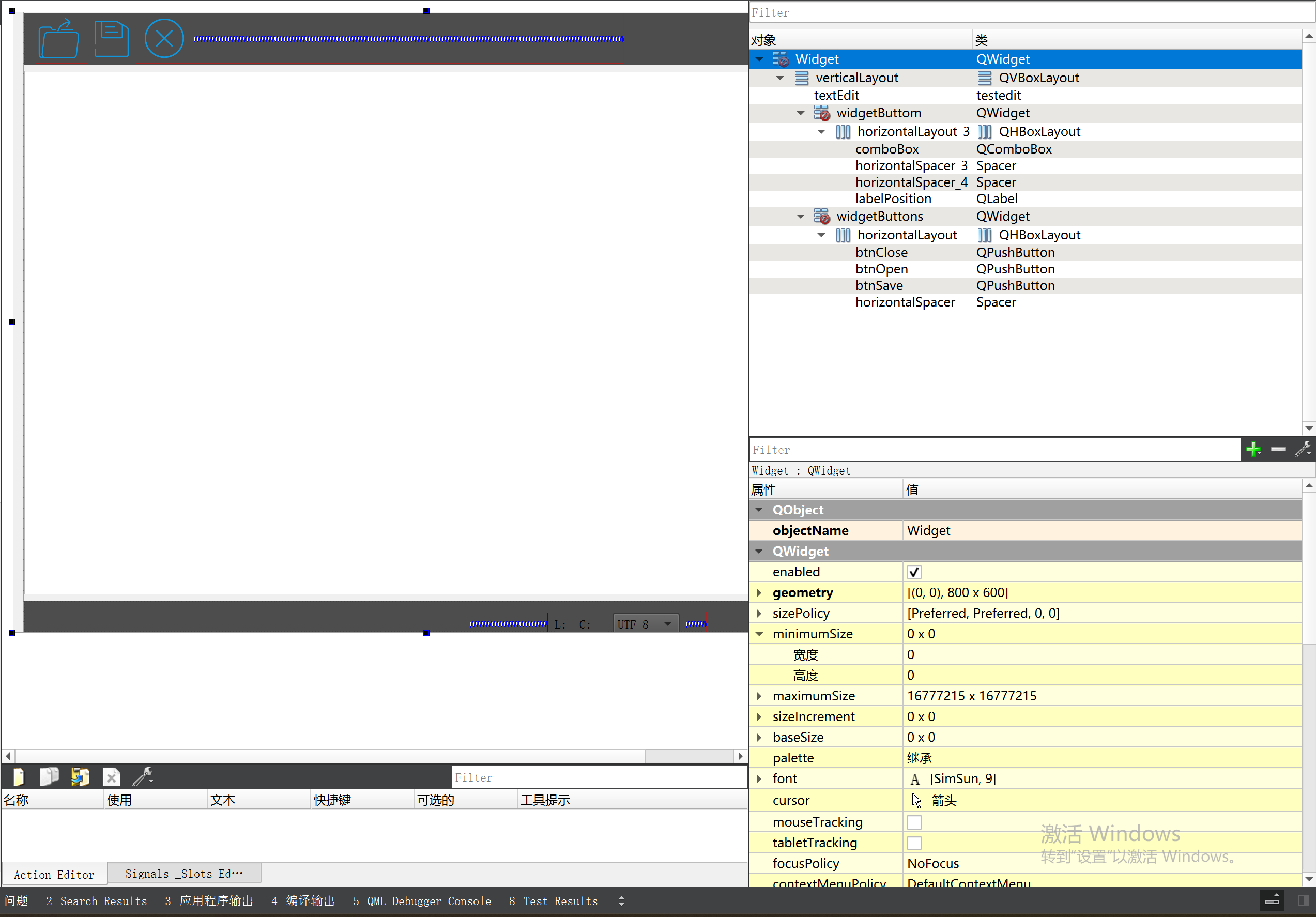
P3 QT项目----记事本(3.8)
3.8 记事本项目总结 项目源码 1.main.cpp #include "widget.h" #include <QApplication> int main(int argc, char *argv[]) {QApplication a(argc, argv);Widget w;w.show();return a.exec(); } 2.widget.cpp #include "widget.h" #include &q…...
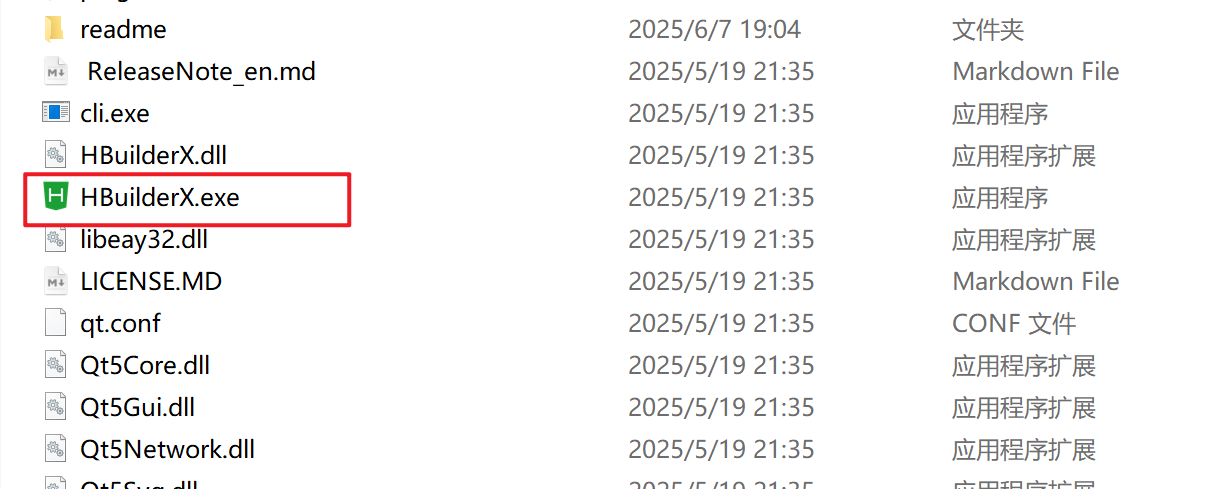
HBuilderX安装(uni-app和小程序开发)
下载HBuilderX 访问官方网站:https://www.dcloud.io/hbuilderx.html 根据您的操作系统选择合适版本: Windows版(推荐下载标准版) Windows系统安装步骤 运行安装程序: 双击下载的.exe安装文件 如果出现安全提示&…...
Python 高效图像帧提取与视频编码:实战指南
Python 高效图像帧提取与视频编码:实战指南 在音视频处理领域,图像帧提取与视频编码是基础但极具挑战性的任务。Python 结合强大的第三方库(如 OpenCV、FFmpeg、PyAV),可以高效处理视频流,实现快速帧提取、压缩编码等关键功能。本文将深入介绍如何优化这些流程,提高处理…...
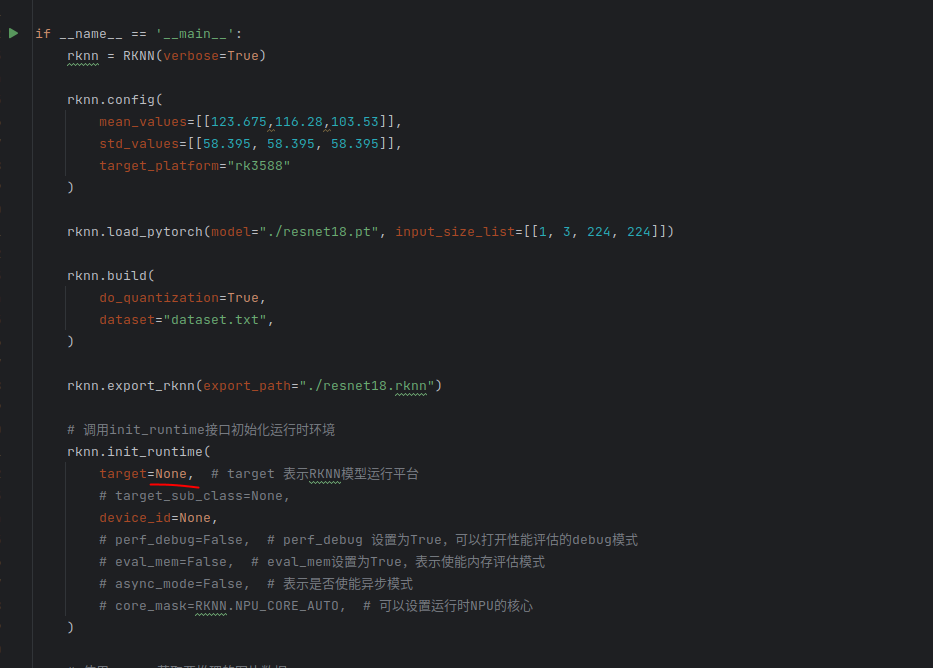
rknn toolkit2搭建和推理
安装Miniconda Miniconda - Anaconda Miniconda 选择一个 新的 版本 ,不用和RKNN的python版本保持一致 使用 ./xxx.sh进行安装 下面配置一下载源 # 清华大学源(最常用) conda config --add channels https://mirrors.tuna.tsinghua.edu.cn…...
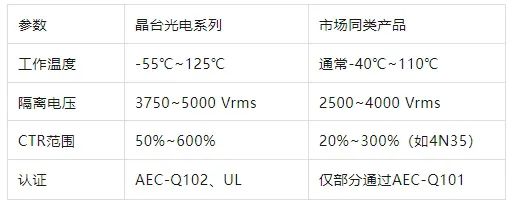
高抗扰度汽车光耦合器的特性
晶台光电推出的125℃光耦合器系列产品(包括KL357NU、KL3H7U和KL817U),专为高温环境下的汽车应用设计,具备以下核心优势和技术特点: 一、技术特性分析 高温稳定性 采用先进的LED技术和优化的IC设计,确保在…...
Docker环境下安装 Elasticsearch + IK 分词器 + Pinyin插件 + Kibana(适配7.10.1)
做RAG自己打算使用esmilvus自己开发一个,安装时好像网上没有比较新的安装方法,然后找了个旧的方法对应试试: 🚀 本文将手把手教你在 Docker 环境中部署 Elasticsearch 7.10.1 IK分词器 拼音插件 Kibana,适配中文搜索…...