php 手机网站cms系统/哪个公司的网站制作
所有继承自QObject的类都有event函数,该函数用来处理自身的事件,函数定义如下:
virtual bool QObject::event(QEvent *e);
Qt帮助文档:
This virtual function receives events to an object and should return true if the event e recognized and processed. The event() function can be reimplemented to customize the behavior of an object. Make sure you call the parent event class implementation for all the events you did not handle.
大致意思:在event
函数中,当事件被识别并处理后,如果返回true
,表示该event
函数执行结束,不再希望执行具体的事件处理函数,例如mousePressEvent
、paintEvent
等;如果返回false
,也表示该event
函数执行结束,不再希望执行具体的事件处理函数,但事件会向上传递,即传递给父组件,进入父组件的event
函数。也可以event
函数中调用父类的event
函数,交给父类来处理,父类仍旧遵循以上规则。
注:当event
函数中返回true
之前,若已经设置了e->ignore()
,仍旧会调用父组件的event
函数;只有当event
函数返回true
,并且e->isAccepted()
也为true
的时候,才不再调用父组件的event
函数。QEvent *e
的默认值是true
,除非在eventFilter
函数中设置为false
。
下面关于文档里说的返回值,进行演示说明
MyButton.h
#ifndef MYBUTTON_H
#define MYBUTTON_H#include <QPushButton>class MyButton : public QPushButton
{Q_OBJECT
public:MyButton(QWidget *parent = nullptr);~MyButton();protected:bool event(QEvent *e) override;
};#endif // MYBUTTON_H
MyButton.cpp
#include "MyButton.h"
#include <QDebug>
#include <QEvent>MyButton::MyButton(QWidget *parent) : QPushButton(parent){}MyButton::~MyButton(){}bool MyButton::event(QEvent *e)
{if (e->type() == QEvent::MouseButtonPress){qDebug() << __FUNCTION__ << event->isAccepted();return true;}return QPushButton::event(e);
}
MainWindow.h
#ifndef MAINWINDOW_H
#define MAINWINDOW_H#include <QMainWindow>namespace Ui {
class MainWindow;
}class MainWindow : public QMainWindow
{Q_OBJECTpublic:explicit MainWindow(QWidget *parent = nullptr);~MainWindow();protected:bool event(QEvent *e) override;private:Ui::MainWindow *ui;
};#endif // MAINWINDOW_H
MainWindow.cpp
#include "MainWindow.h"
#include "ui_MainWindow.h"
#include <QDebug>
#include <QEvent>
#include <QMouseEvent>MainWindow::MainWindow(QWidget *parent) :QMainWindow(parent),ui(new Ui::MainWindow)
{ui->setupUi(this);
}MainWindow::~MainWindow()
{delete ui;
}bool MainWindow::event(QEvent *e)
{if (e->type() == QEvent::MouseButtonPress){qDebug() << __FUNCTION__ << e->isAccepted();}return QMainWindow::event(e);
}
鼠标点击MyButton类按钮,运行结果: 事件向父类传播
// 情形1:直接return false
bool MyButton::event(QEvent *e)
{if (e->type() == QEvent::MouseButtonPress){qDebug() << __FUNCTION__ << event->isAccepted();return false;}return QPushButton::event(e);
}// 情形2:e->ignore()后return true
bool MyButton::event(QEvent *e)
{if (e->type() == QEvent::MouseButtonPress){qDebug() << __FUNCTION__ << event->isAccepted();e->ignore();return true;}return QPushButton::event(e);
}// 运行结果都如下:
MyButton::event true
MainWindow::event true
鼠标点击MyButton类按钮,运行结果: 事件停止向父类传播
// 情形3:直接return true
bool MyButton::event(QEvent *e)
{if (e->type() == QEvent::MouseButtonPress){qDebug() << __FUNCTION__ << event->isAccepted();return true;}return QPushButton::event(e);
}// 情形3:返回父类event函数的处理结果
bool MyButton::event(QEvent *e)
{if (e->type() == QEvent::MouseButtonPress){qDebug() << __FUNCTION__ << event->isAccepted();}return QPushButton::event(e);
}// 运行结果都如下:
MyButton::event true
补充1:QT源码中事件处理过程中调用函数如下:
QApplication::exec()QCoreApplication::exec()QEventLoop::exec(ProcessEventsFlags )QEventLoop::processEvents(ProcessEventsFlags )QEventDispatcherWin32::processEvents(QEventLoop::ProcessEventsFlags)QT_WIN_CALLBACK QtWndProc(HWND hwnd, UINT message, WPARAM wParam, LPARAM lParam)bool QETWidget::translateMouseEvent(const MSG &msg)bool QApplicationPrivate::sendMouseEvent(...)inline bool QCoreApplication::sendSpontaneousEvent(QObject *receiver, QEvent *event)bool QCoreApplication::notifyInternal(QObject *receiver, QEvent *event)bool QApplication::notify(QObject *receiver, QEvent *e)bool QApplicationPrivate::notify_helper(QObject *receiver, QEvent * e)bool QWidget::event(QEvent *event)
补充2:notify函数中处理事件传播
notify
函数中处理事件传播的时候,会调用notify_helper
函数,当(res && eventAccepted)
为真时停止向父类传播,否则w = w->parentWidget()
,进而调用父类的notify_helper
函数,而notify_helper
函数中会调用receiver->event(e)
函数。
bool QApplication::notify(QObject *receiver, QEvent *e)
{QWidget* w = static_cast<QWidget *>(receiver);QMouseEvent* mouse = static_cast<QMouseEvent*>(e);QPoint relpos = mouse->pos();...switch (e->type()) {...case QEvent::MouseButtonPress:case QEvent::MouseButtonRelease:case QEvent::MouseButtonDblClick:case QEvent::MouseMove:{...bool eventAccepted = mouse->isAccepted();QPointer<QWidget> pw = w;while (w) {QMouseEvent me(mouse->type(), relpos, mouse->windowPos(), mouse->globalPos(),mouse->button(), mouse->buttons(), mouse->modifiers(), mouse->source());me.spont = mouse->spontaneous();me.setTimestamp(mouse->timestamp());QGuiApplicationPrivate::setMouseEventFlags(&me, mouse->flags());// throw away any mouse-tracking-only mouse eventsif (!w->hasMouseTracking()&& mouse->type() == QEvent::MouseMove && mouse->buttons() == 0) {// but still send them through all application event filters (normally done by notify_helper)d->sendThroughApplicationEventFilters(w, w == receiver ? mouse : &me);res = true;} else {w->setAttribute(Qt::WA_NoMouseReplay, false);res = d->notify_helper(w, w == receiver ? mouse : &me);e->spont = false;}eventAccepted = (w == receiver ? mouse : &me)->isAccepted();if (res && eventAccepted)break;if (w->isWindow() || w->testAttribute(Qt::WA_NoMousePropagation))break;relpos += w->pos();w = w->parentWidget();}...}...
}
bool QApplicationPrivate::notify_helper(QObject *receiver, QEvent * e)
{// These tracepoints (and the whole function, actually) are very similar// to the ones in QCoreApplicationPrivate::notify_helper; the reason for their// duplication is because tracepoint symbols are not exported by QtCore.// If you adjust the tracepoints here, consider adjusting QCoreApplicationPrivate too.Q_TRACE(QApplication_notify_entry, receiver, e, e->type());bool consumed = false;bool filtered = false;Q_TRACE_EXIT(QApplication_notify_exit, consumed, filtered);// send to all application event filtersif (threadRequiresCoreApplication()&& receiver->d_func()->threadData->thread == mainThread()&& sendThroughApplicationEventFilters(receiver, e)) {filtered = true;return filtered;}if (receiver->isWidgetType()) {QWidget *widget = static_cast<QWidget *>(receiver);#if !defined(QT_NO_CURSOR)// toggle HasMouse widget state on enter and leaveif ((e->type() == QEvent::Enter || e->type() == QEvent::DragEnter) &&(!QApplication::activePopupWidget() || QApplication::activePopupWidget() == widget->window()))widget->setAttribute(Qt::WA_UnderMouse, true);else if (e->type() == QEvent::Leave || e->type() == QEvent::DragLeave)widget->setAttribute(Qt::WA_UnderMouse, false);
#endifif (QLayout *layout=widget->d_func()->layout) {layout->widgetEvent(e);}}// send to all receiver event filtersif (sendThroughObjectEventFilters(receiver, e)) {filtered = true;return filtered;}// deliver the eventconsumed = receiver->event(e);QCoreApplicationPrivate::setEventSpontaneous(e, false);return consumed;
}
注: event
函数中调用e->ignore()
之后,父对象的event
函数中e->isAccepted()
值仍然为true
的原因是每一次调用notify_helper
函数时,传递的事件e
都是临时的。
QMouseEvent* mouse = static_cast<QMouseEvent*>(e);
...
QMouseEvent me(mouse->type(), relpos, mouse->windowPos(), mouse->globalPos(), mouse->button(), mouse->buttons(), mouse->modifiers(), mouse->source());
...
res = d->notify_helper(w, w == receiver ? mouse : &me);
注: 补充1的内容引自QT QEvent 事件调用的来龙去脉,作者:QtC++ 开发从业者
相关文章:

Qt事件处理机制2-事件函数的传播
所有继承自QObject的类都有event函数,该函数用来处理自身的事件,函数定义如下: virtual bool QObject::event(QEvent *e);Qt帮助文档: This virtual function receives events to an object and should return true i…...
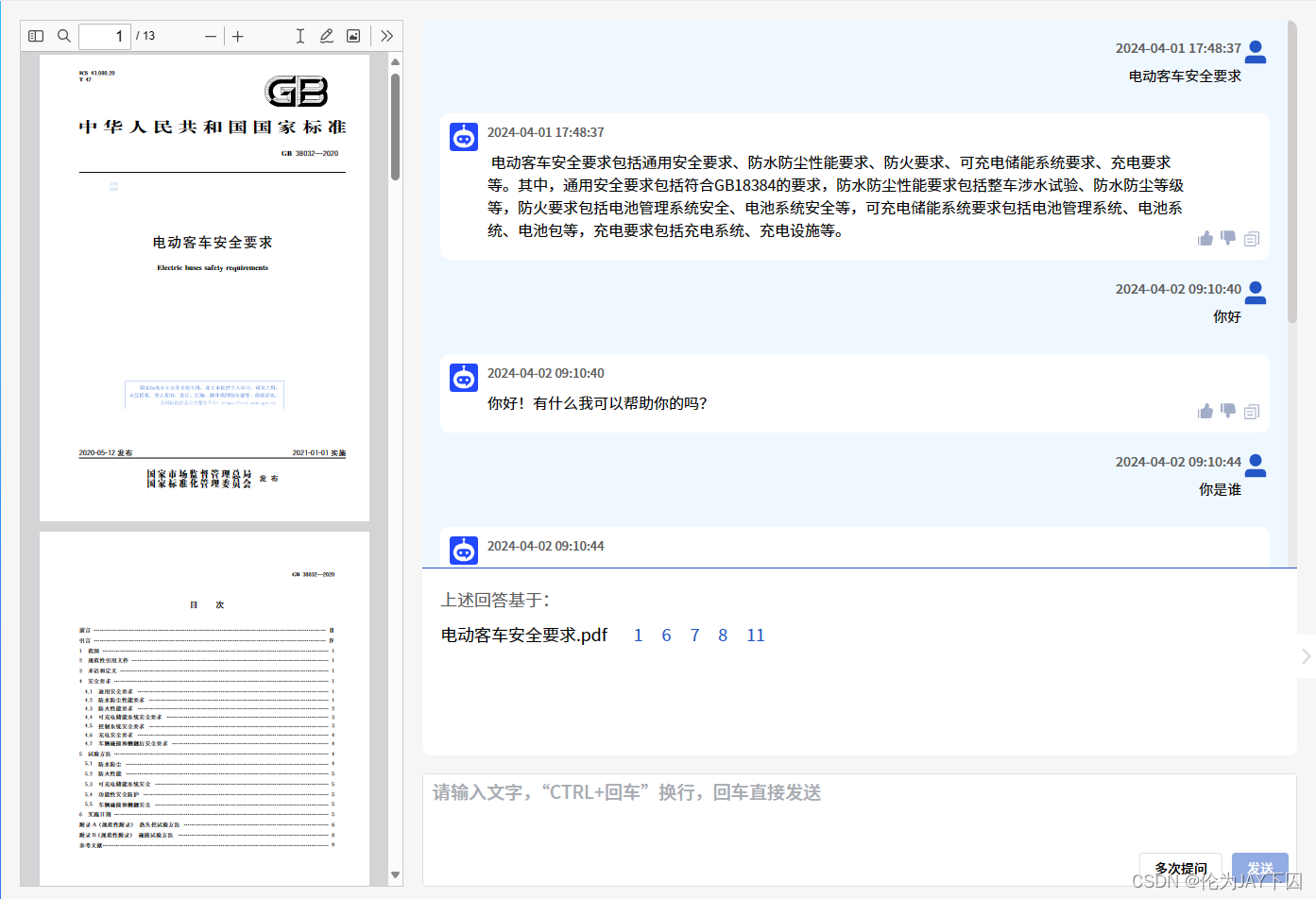
【PDF.js】PDF文件预览
【PDF.js】PDF文件预览 一、PDF.js二、PDF.js 下载1、下载PDF.js2、在项目中引入3、屏蔽跨域错误 三、项目中使用四、说明五、实现效果 使用PDFJS实现pdf文件的预览,支持预览指定页、关键词搜索、缩略图、页面尺寸调整等等。 一、PDF.js 官方地址 文档地址 二、PD…...
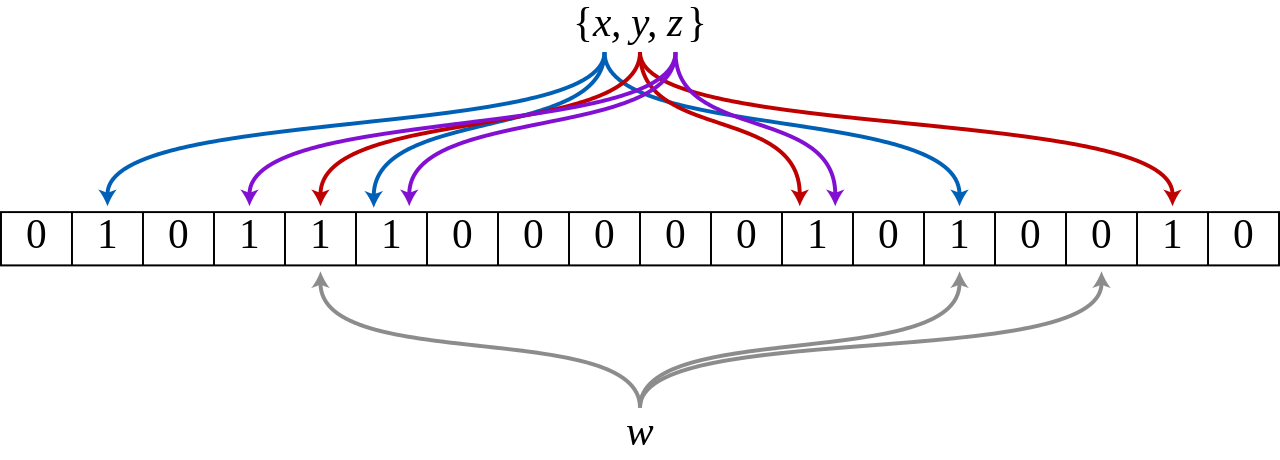
从建表语句带你学习doris_表索引
1、doris建表概述 1.1、doris建表模板 CREATE [EXTERNAL] TABLE [IF NOT EXISTS] [DATABASE.]table_name (column_definition1[,column_deinition2,......][,index_definition1,[,index_definition2,]] ) [ENGINE [olap|mysql|broker|hive]] [key_desc] [COMMENT "tabl…...
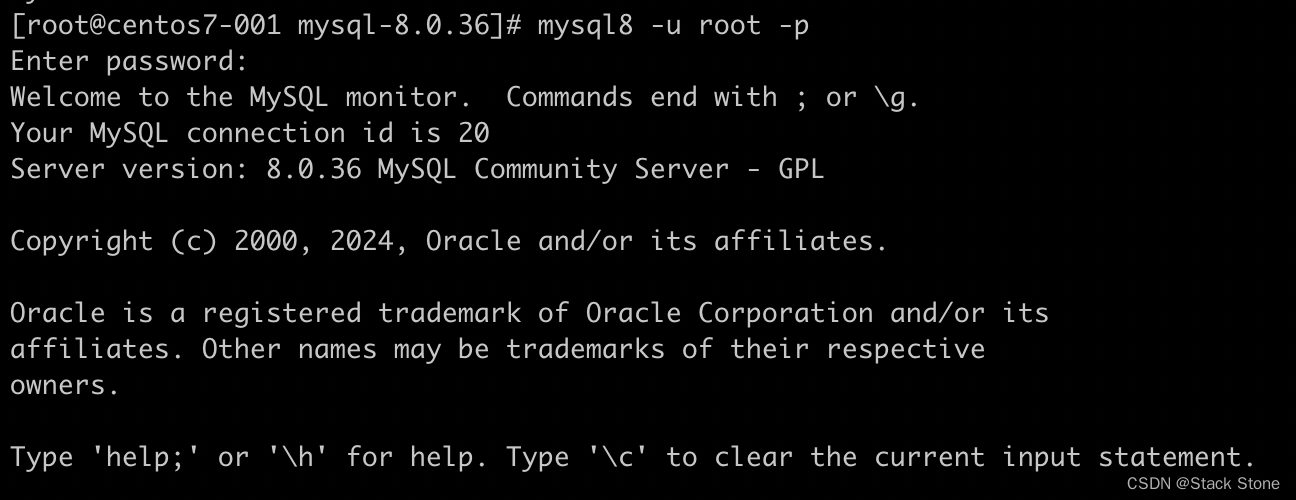
Linux CentOS 安装 MySQL 服务教程
Linux CentOS 安装 MySQL 服务教程 1. 查看系统和GNU C库(glibc)版本信息 1.1 查询机器 glibc 版本信息 glibc,全名GNU C Library,是大多数Linux发行版中使用的C库,为系统和应用程序提供核心的API接口。在Linux系统中,特别是在…...
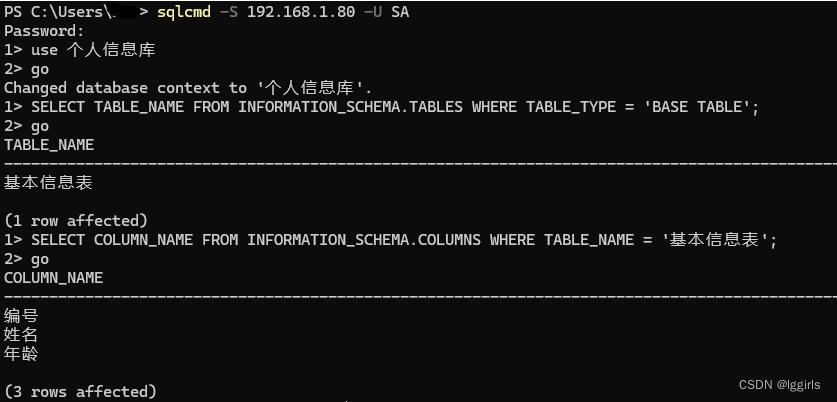
MSSQL 命令行操作说明 sql server 2022 命令行下进行配置管理
说明:本文的内容是因为我在导入Access2019的 *.accdb 格式的数据时,总是出错的背景下,不得已搜索和整理了一下,如何用命令行进行sql server 数据库和用户管理的方法,作为从Access2019 直接导出数据到sql server 数据库…...
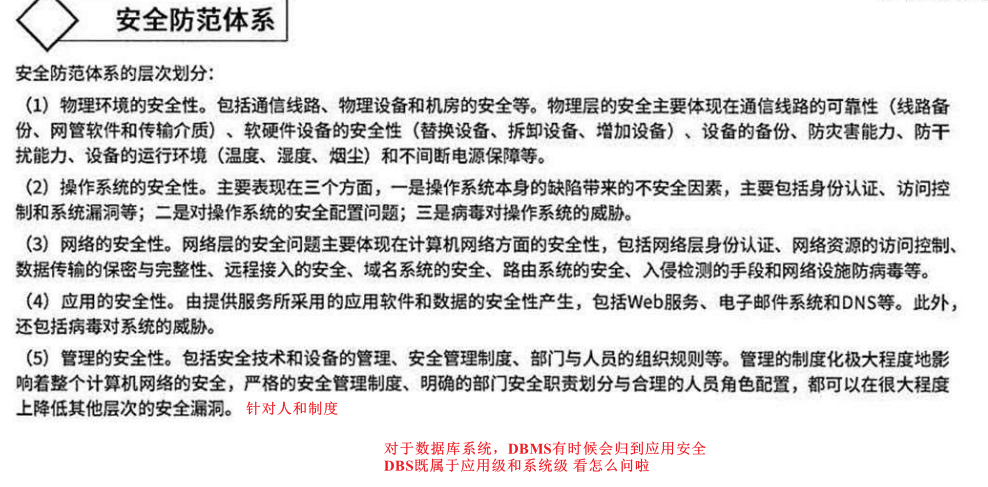
【系统分析师】系统安全分析与设计
文章目录 1、安全基础技术1.1 密码相关1.1.1对称加密1.1.2非对称加密1.1.3信息摘要1.1.4数字签名1.1.5数字信封 1.2 PKI公钥体系 2、信息系统安全2.1 保障层次2.2 网络安全2.2.1WIFI2.2.2 网络威胁与攻击2.2.3 安全保护等级 2.3计算机病毒与木马2.4安全防范体系 1、安全基础技术…...
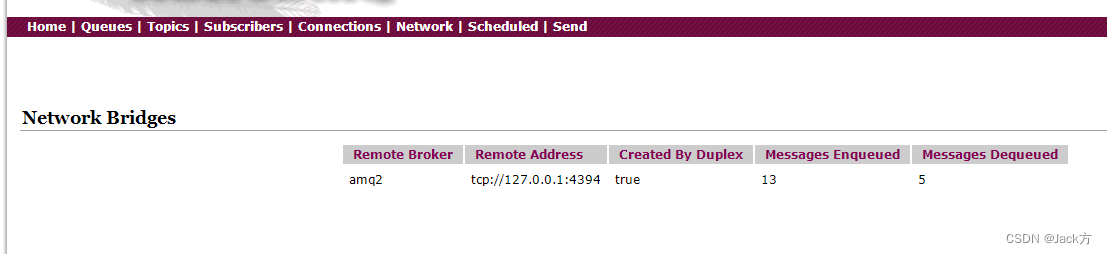
ActiveMQ 07 集群配置
Active MQ 07 集群配置 官方文档 http://activemq.apache.org/clustering 主备集群 http://activemq.apache.org/masterslave.html Master Slave TypeRequirementsProsConsShared File System Master SlaveA shared file system such as a SANRun as many slaves as requ…...
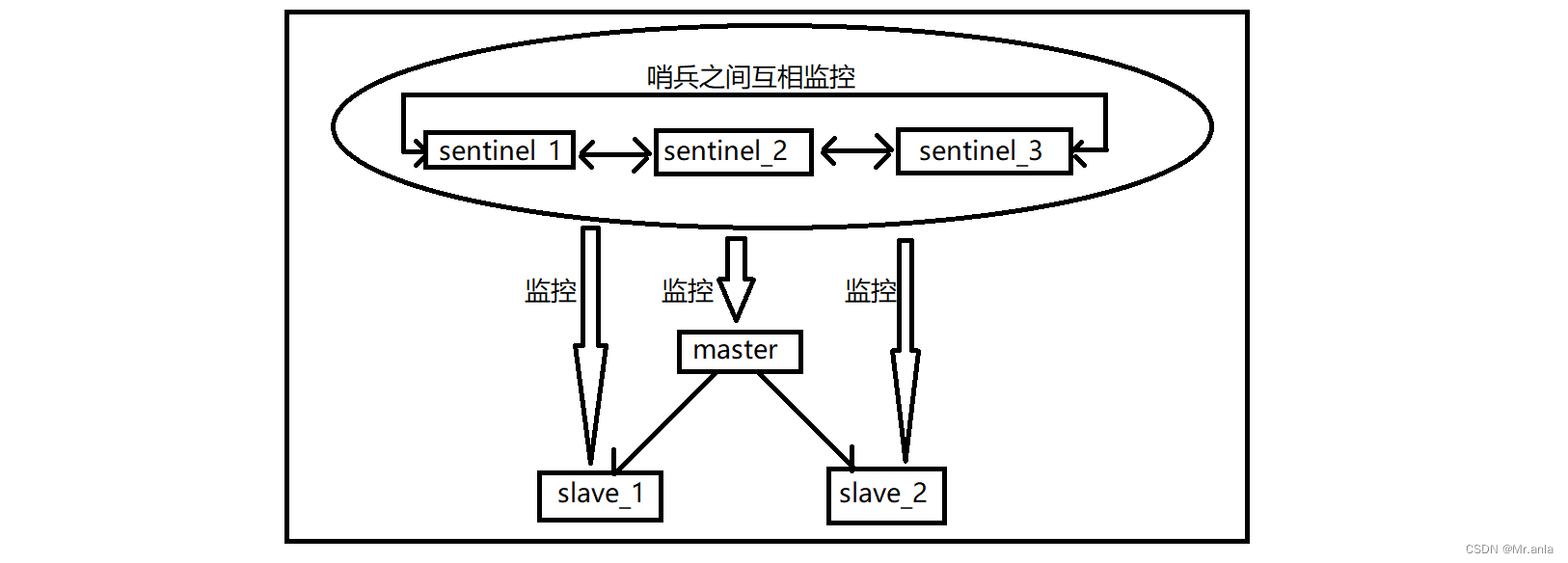
Redis(哨兵模式)
什么是哨兵机制 问题: redis 主从复制模式下, 一旦主节点由于故障不能提供服务, 需要人工进行主从切换, 同时大量客户端需要被通知切换到新的主节点上, 对于有一定规模的应用来说, 对于人力的资源消耗会很大.解决: 通过哨兵对主从结构进行监控, 一旦出现主节点挂了的情况, 自动…...

一种基于镜像指示位办法的RingBuffer实现,解决Mirror和2的幂个数限制
简介 在嵌入式开发中,经常有需要用到RingBuffer的概念,在RingBuffer中经常遇到一个Buffer满和Buffer空的判断的问题,一般的做法是留一个单位的buffer不用,这样做最省事,但是当RingBuffer单位是一个结构体时࿰…...
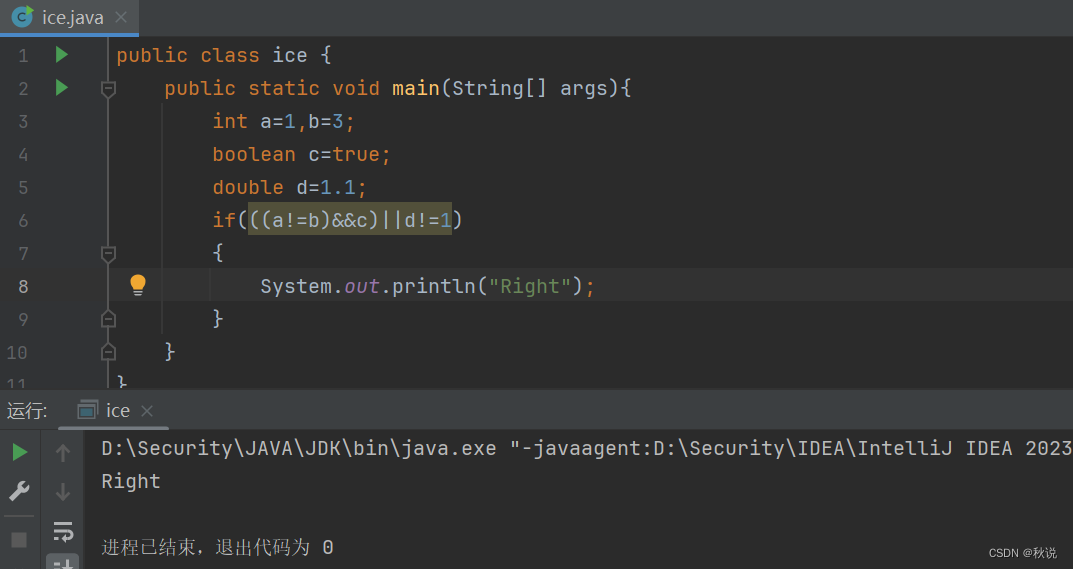
【Java开发指南 | 第十一篇】Java运算符
读者可订阅专栏:Java开发指南 |【CSDN秋说】 文章目录 算术运算符关系运算符位运算符逻辑运算符赋值运算符条件运算符(?:)instanceof 运算符Java运算符优先级 Java运算符包括:算术运算符、关系运算符、位运算符、逻辑运算符、赋值…...
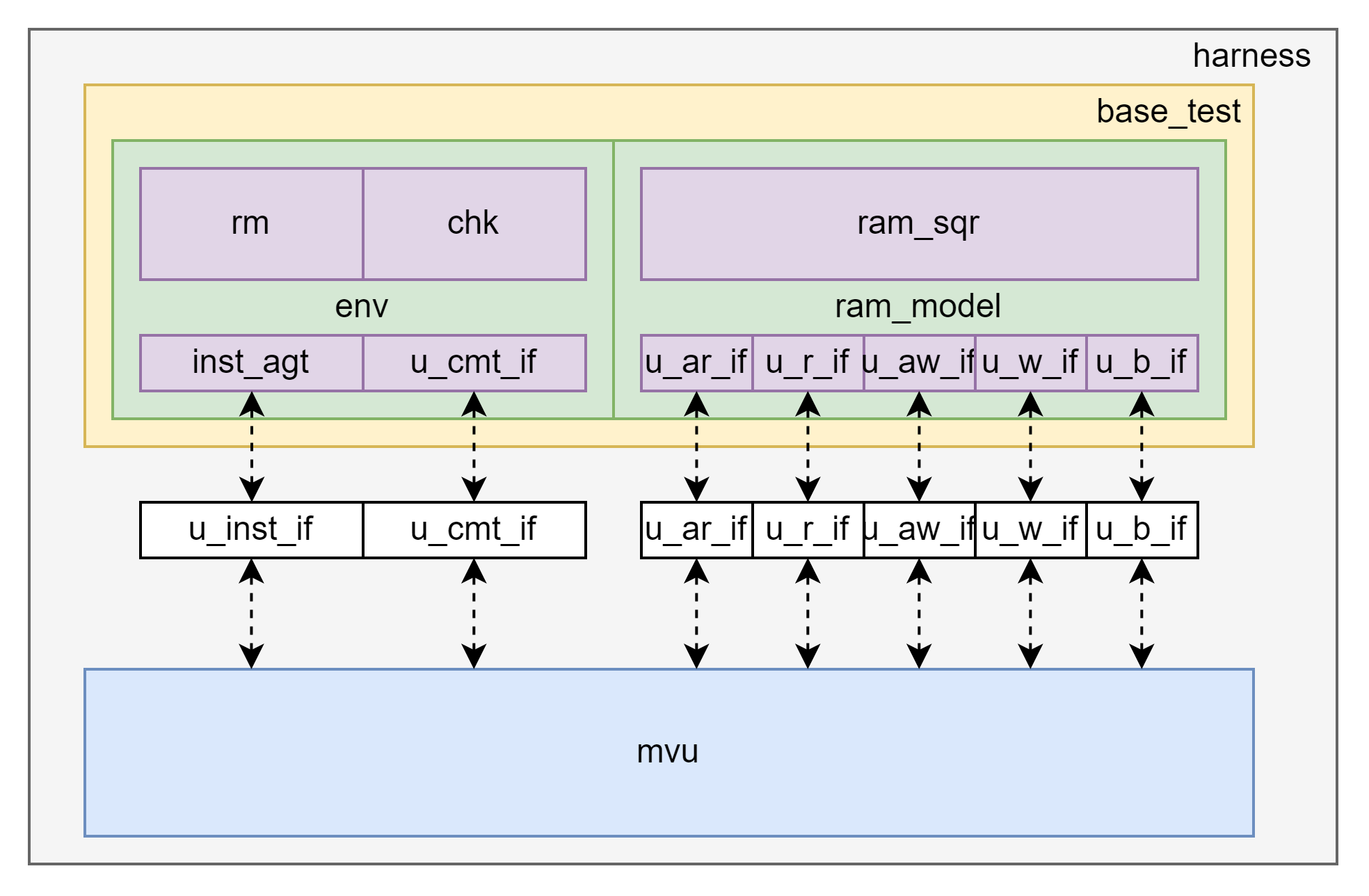
【IC前端虚拟项目】验证环境方案思路和文档组织
【IC前端虚拟项目】数据搬运指令处理模块前端实现虚拟项目说明-CSDN博客 对于mvu的验证环境,从功能角度就可以分析出需要搭建哪些部分,再看一下mvu的周围环境哈: 很明显验证环境必然要包括几个部分: 1.模拟idu发送指令; 2.模拟ram/ddr读写数据; 3.rm模拟mvu的行为; …...
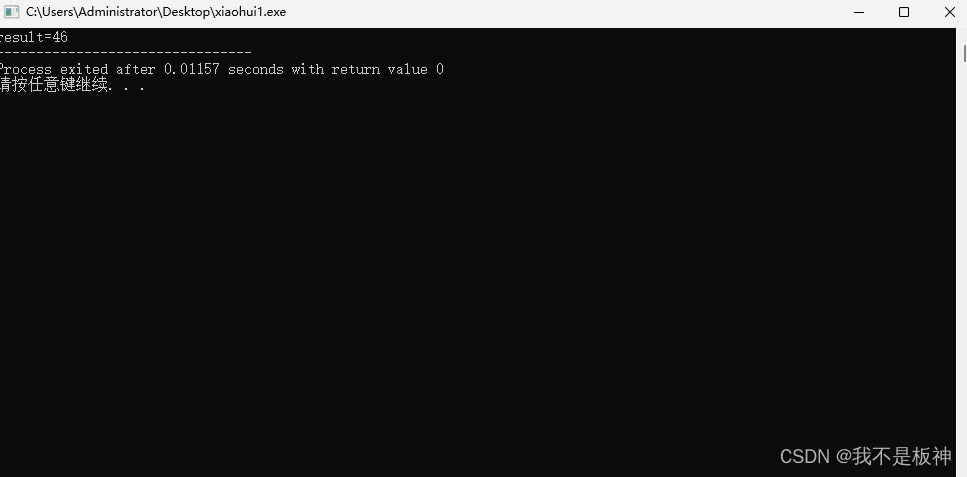
程序设计|C语言教学——C语言基础1:C语言的引入和入门
一、程序的执行 1.定义 解释:借助一个程序,那个程序能够试图理解你的程序,然后按照你的要求执行。下次执行的时候还需要从零开始解释。 编译:借助一个程序,能够像翻译官一样,把你的程序翻译成机器语言&a…...
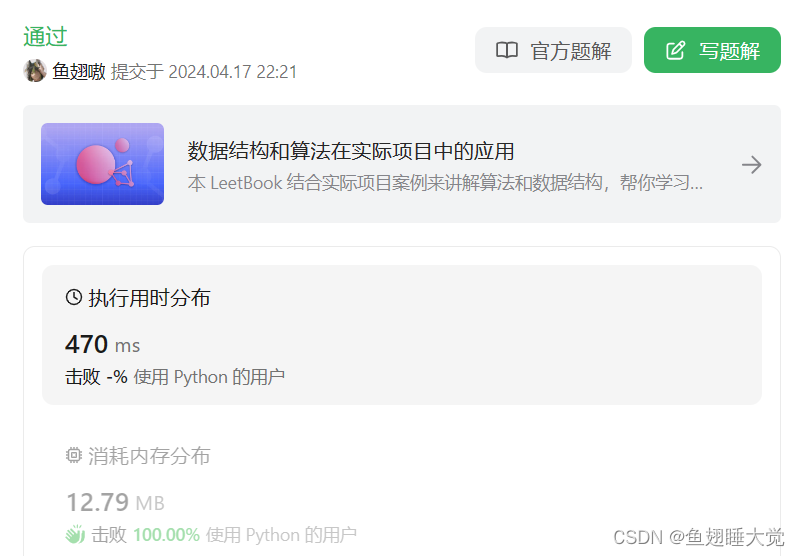
初学python记录:力扣928. 尽量减少恶意软件的传播 II
题目: 给定一个由 n 个节点组成的网络,用 n x n 个邻接矩阵 graph 表示。在节点网络中,只有当 graph[i][j] 1 时,节点 i 能够直接连接到另一个节点 j。 一些节点 initial 最初被恶意软件感染。只要两个节点直接连接,…...
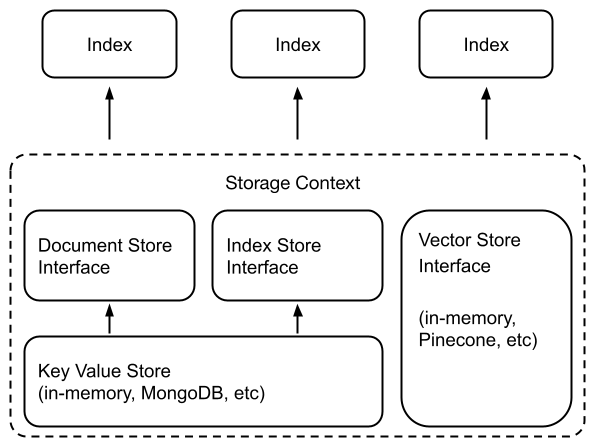
LlamaIndex 组件 - Storing
文章目录 一、储存概览1、概念2、使用模式3、模块 二、Vector Stores1、简单向量存储2、矢量存储选项和功能支持3、Example Notebooks 三、文件存储1、简单文档存储2、MongoDB 文档存储3、Redis 文档存储4、Firestore 文档存储 四、索引存储1、简单索引存储2、MongoDB 索引存储…...

在Linux系统中设定延迟任务
一、在系统中设定延迟任务要求如下: 要求: 在系统中建立easylee用户,设定其密码为easylee 延迟任务由root用户建立 要求在5小时后备份系统中的用户信息文件到/backup中 确保延迟任务是使用非交互模式建立 确保系统中只有root用户和easylee用户…...
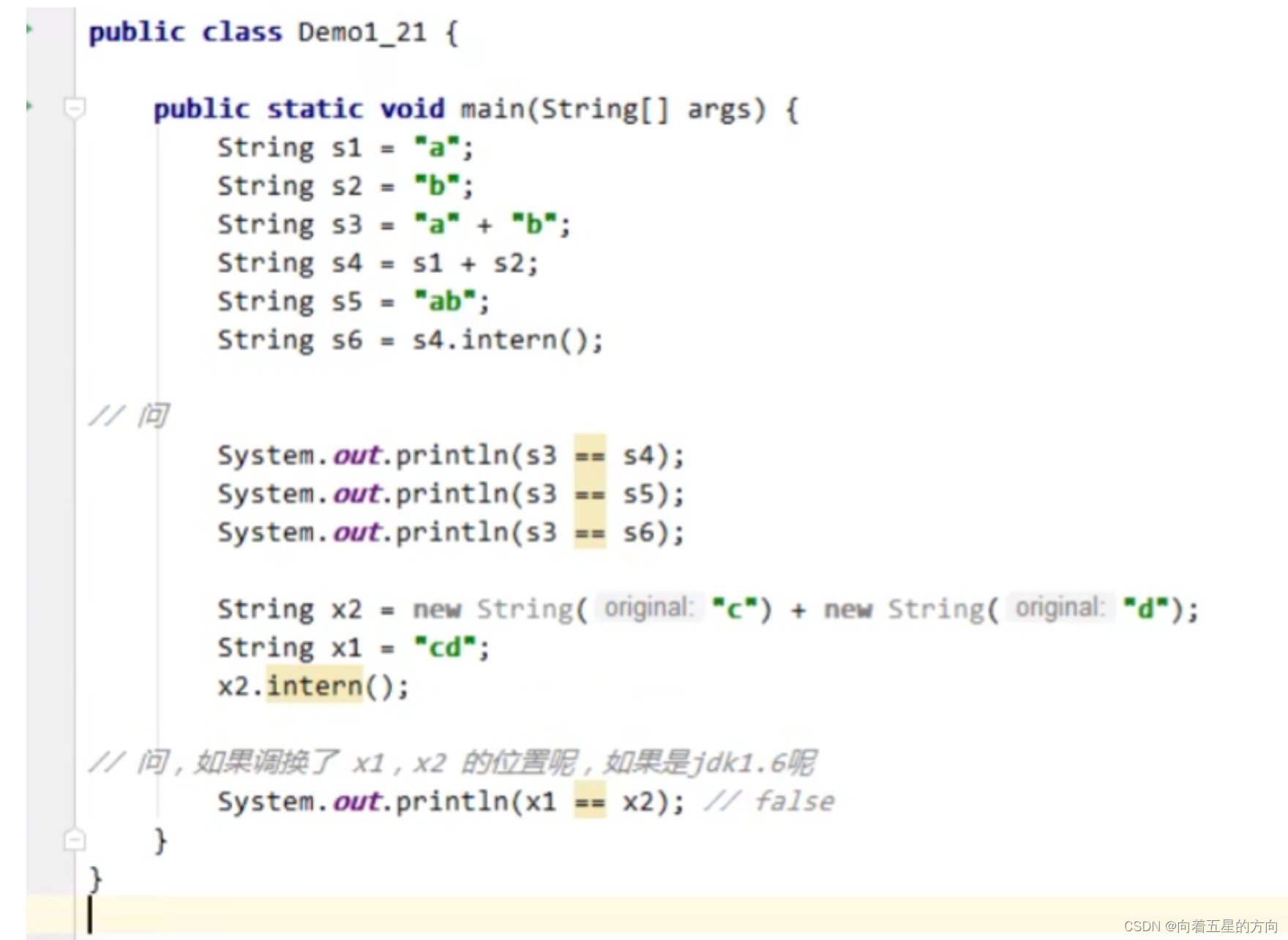
JVM之方法区的详细解析
方法区 方法区:是各个线程共享的内存区域,用于存储已被虚拟机加载的类信息、常量、即时编译器编译后的代码等数据,虽然 Java 虚拟机规范把方法区描述为堆的一个逻辑部分,但是也叫 Non-Heap(非堆) 设置方法…...

Go 使用ObjectID
ObjectID介绍 MongoDB中的ObjectId是一种特殊的12字节 BSON 类型数据,用于为主文档提供唯一的标识符,默认情况下作为 _id 字段的默认值出现在每一个MongoDB集合中的文档中。以下是ObjectId的具体组成: 1. 时间戳(Timestamp&…...
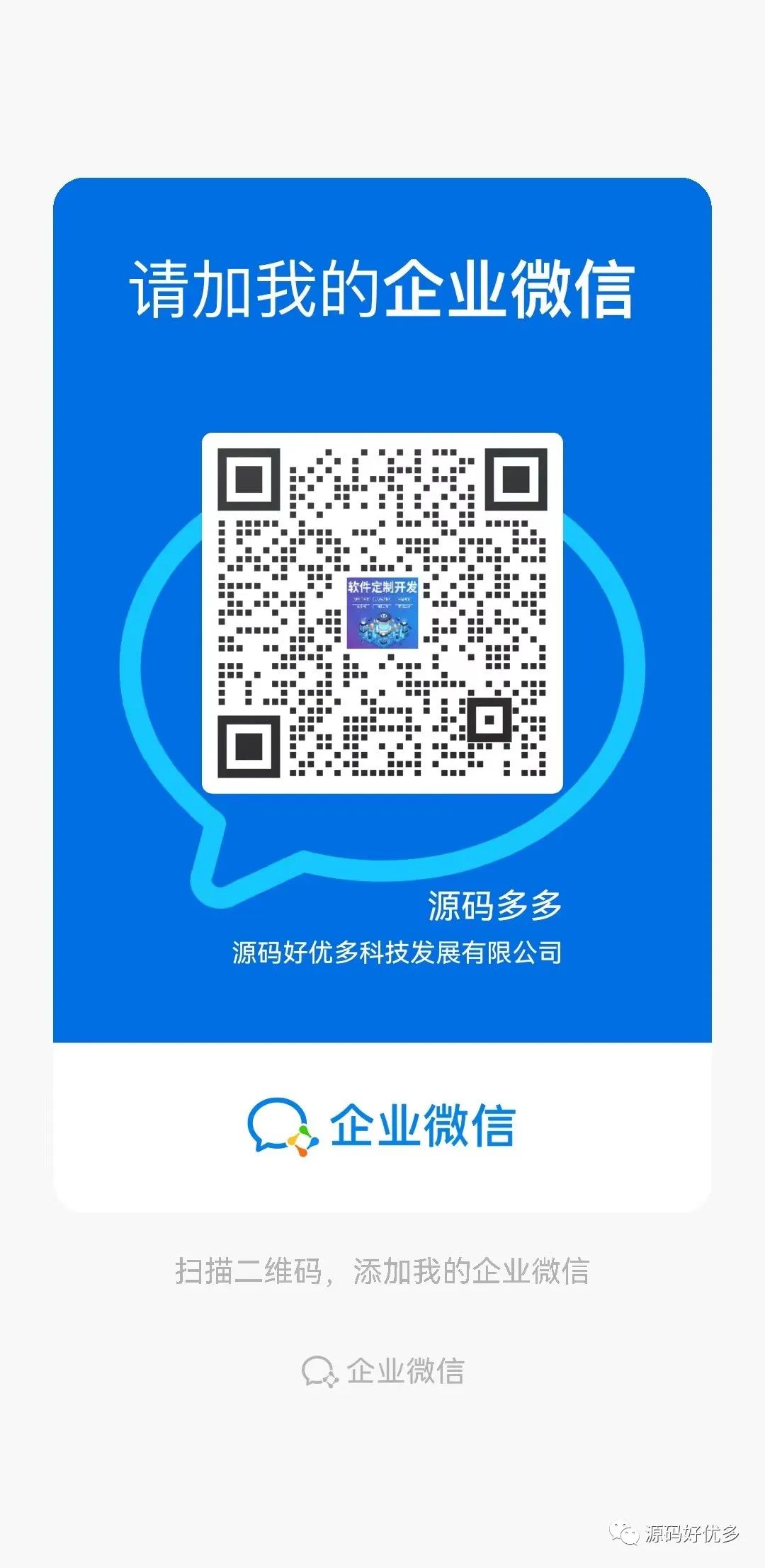
基于SpringBoot+Vue的疾病防控系统设计与实现(源码+文档+包运行)
一.系统概述 在如今社会上,关于信息上面的处理,没有任何一个企业或者个人会忽视,如何让信息急速传递,并且归档储存查询,采用之前的纸张记录模式已经不符合当前使用要求了。所以,对疾病防控信息管理的提升&a…...
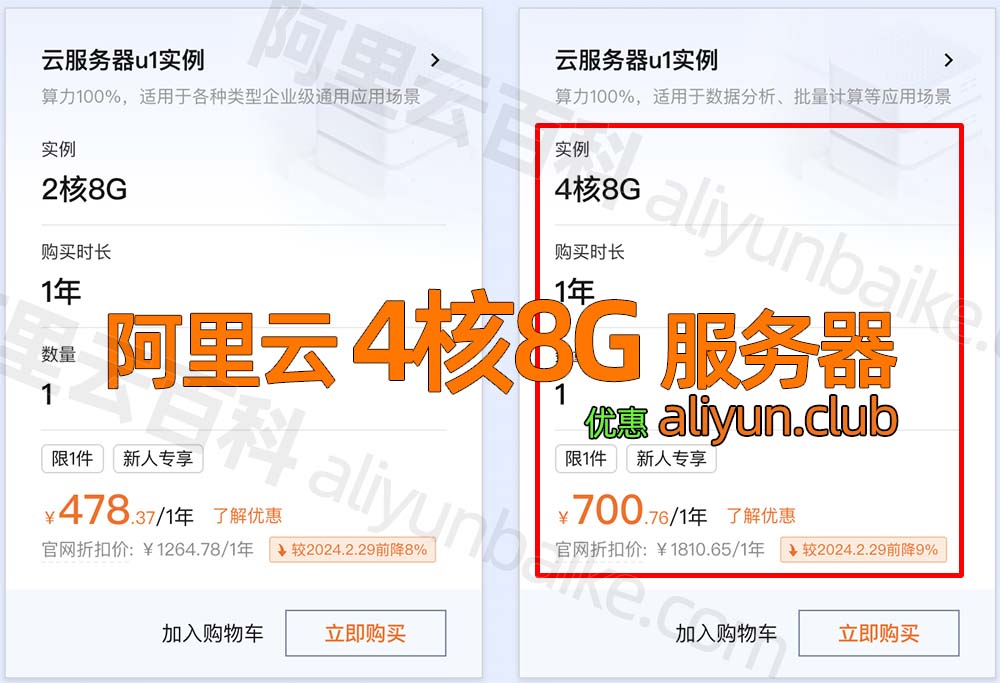
2024年阿里云4核8G配置云服务器价格低性能高!
阿里云4核8G服务器租用优惠价格700元1年,配置为ECS通用算力型u1实例(ecs.u1-c1m2.xlarge)4核8G配置、1M到3M带宽可选、ESSD Entry系统盘20G到40G可选,CPU采用Intel(R) Xeon(R) Platinum处理器,阿里云优惠 aliyunfuwuqi…...

关于ContentProvider这一遍就够了
ContentProvider是什么? ContentProvider是Android四大组件之一,主要用于不同应用程序之间或者同一个应用程序的不同部分之间共享数据。它是Android系统中用于存储和检索数据的抽象层,允许不同的应用程序通过统一的接口访问数据,…...

《1w实盘and大盘基金预测 day23》
这几天预测错麻了,哈哈哈,完全和技术没关系,全是消息面。 昨日预测: 2958-2984-3010 证券继续下跌,昨天诱多把我诱惑进去了(看2-3天的反弹也没了),今天直接出掉昨天买的。 整体操作…...
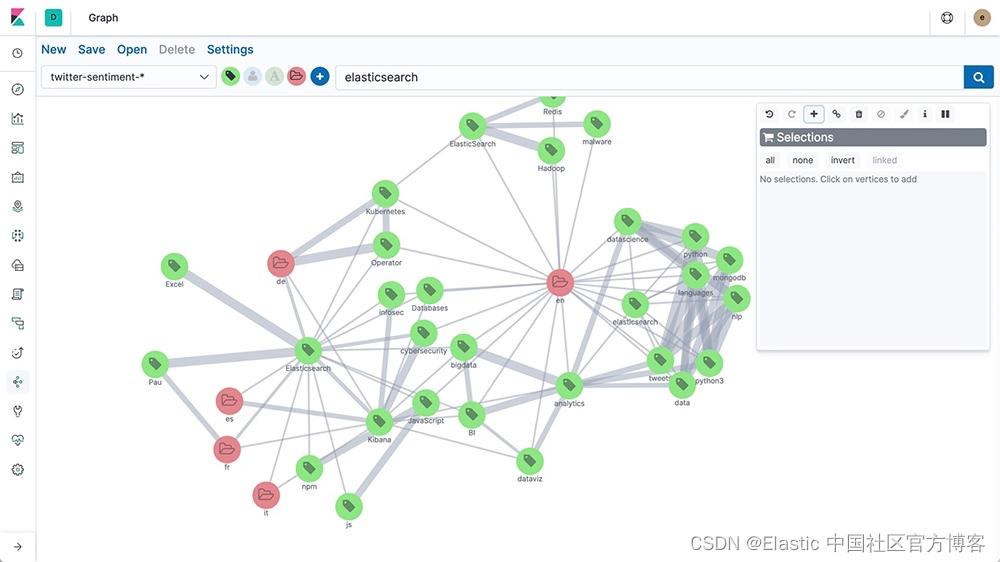
向量数据库与图数据库:理解它们的区别
作者:Elastic Platform Team 大数据管理不仅仅是尽可能存储更多的数据。它关乎能够识别有意义的见解、发现隐藏的模式,并做出明智的决策。这种对高级分析的追求一直是数据建模和存储解决方案创新的驱动力,远远超出了传统关系数据库。 这些创…...
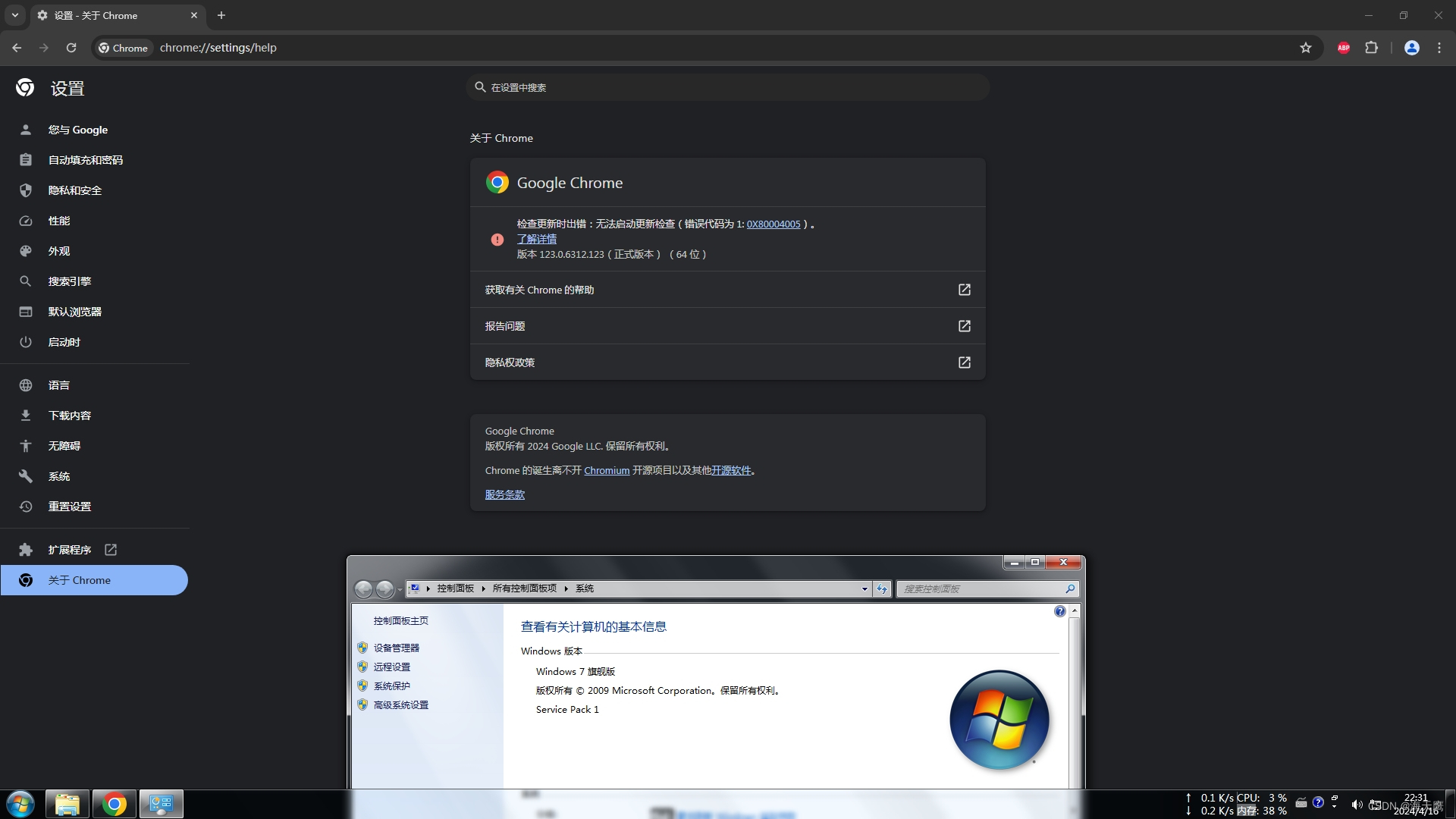
WIN7用上最新版Chrome
1.下载WIN10最新版Chrome的离线安装包 谷歌浏览器 Chrome 最新版离线安装包下载地址 v123.0.6312.123 - 每日自动更新 | 异次元软件 文件名称:123.0.6312.123_chrome_installer.exe。 123.0.6312.123_chrome_installer.exe 文件右键解压缩得到 chrome.7z&#x…...
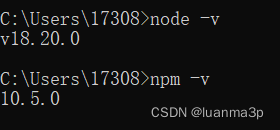
node.jd版本降级/升级
第一步.先清空本地安装的node.js版本 按健winR弹出窗口,键盘输入cmd,然后敲回车(或者鼠标直接点击电脑桌面最左下角的win窗口图标弹出,输入cmd再点击回车键) 进入命令控制行窗口,输入where node,查看本地…...
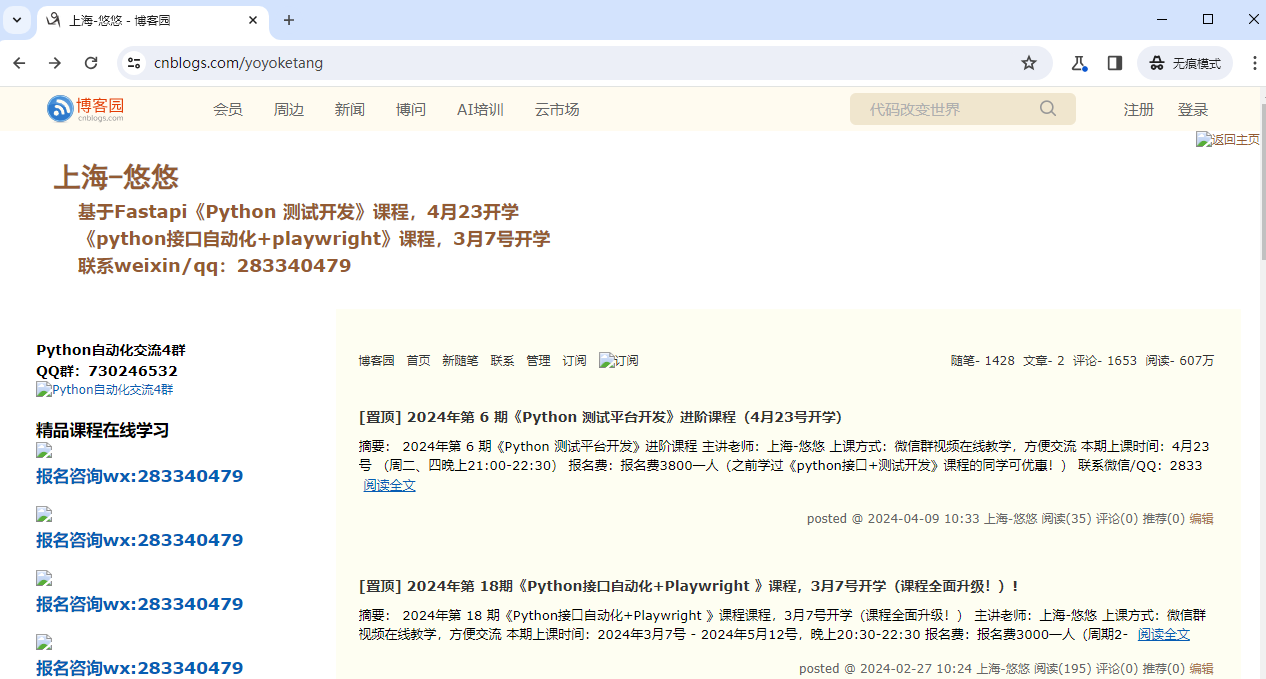
python+playwright 学习-88 禁止加载图片等资源
前言 对于爬虫的小伙伴来说,有时候只需抓取页面的文本,不用加载图片,可以加快操作页面速度,那么我们可以设置禁止加载图片等资源。 禁止图片加载 根据url地址的后缀,图片资源后缀一般是png,jpg,jpeg,gif等格式。 from playwright.sync_api import sync_playwrightwith…...
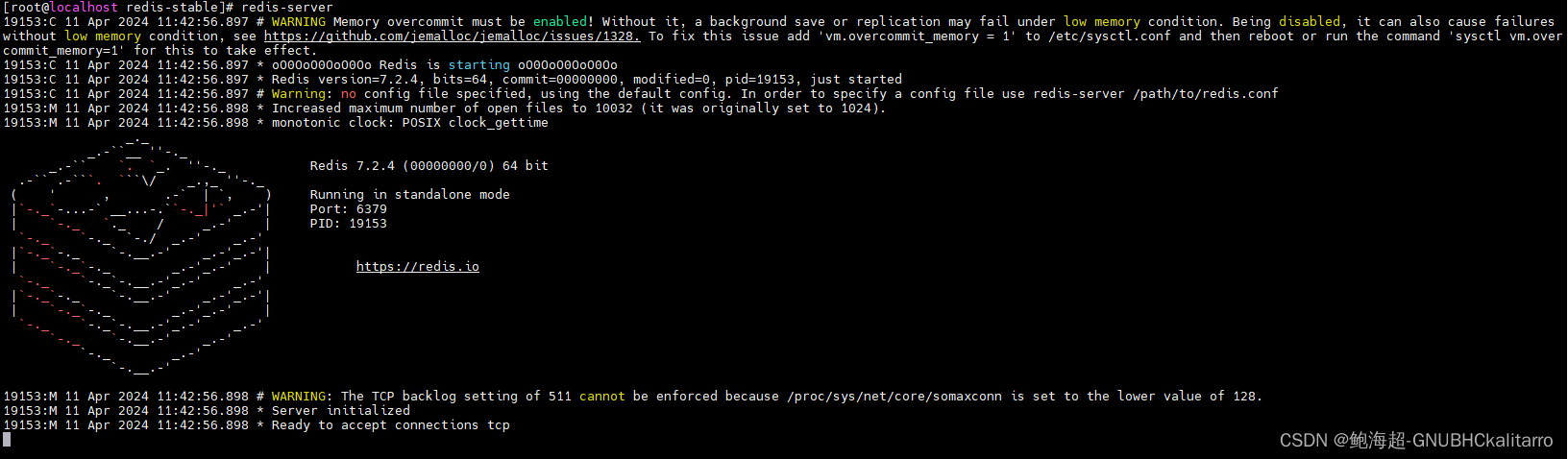
Linux:Redis7.2.4的简单在线部署(1)
注意:我写的这个文章是以最快速的办法去搭建一个redis的基础环境,作用是为了做实验简单的练习,如果你想搭建一个相对稳定的redis去使用,可以看我下面这个文章 Linux:Redis7.2.4的源码包部署(2)-…...
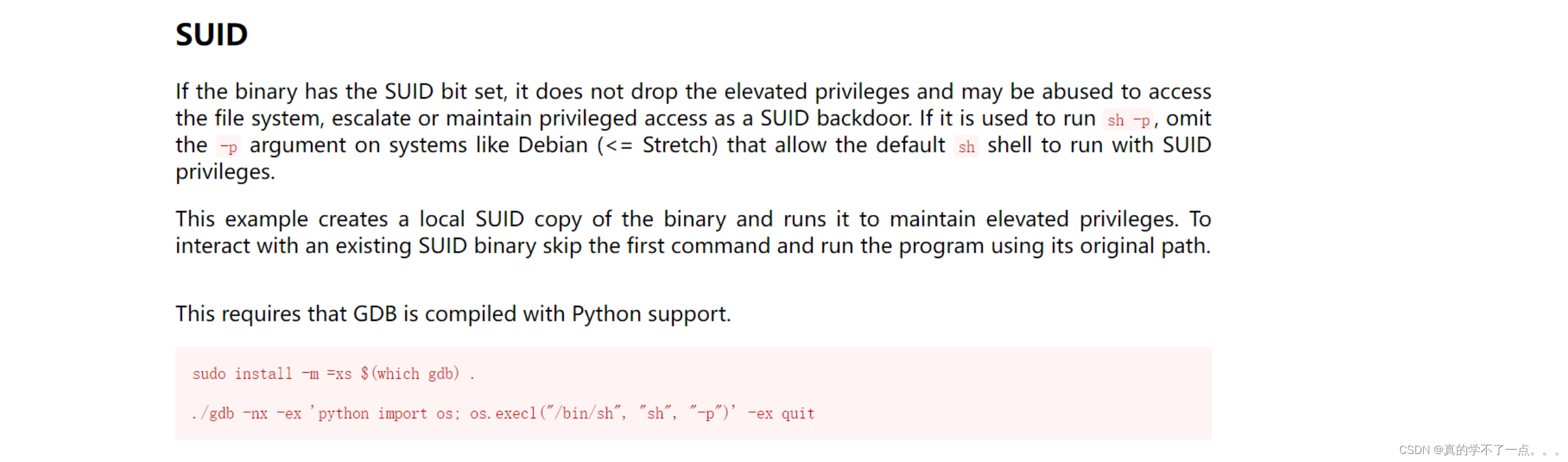
HackMyVM-Connection
目录 信息收集 arp nmap WEB web信息收集 dirsearch smbclient put shell 提权 系统信息收集 suid gdb提权 信息收集 arp ┌─[rootparrot]─[~/HackMyVM] └──╼ #arp-scan -l Interface: enp0s3, type: EN10MB, MAC: 08:00:27:16:3d:f8, IPv4: 192.168.9.115 S…...
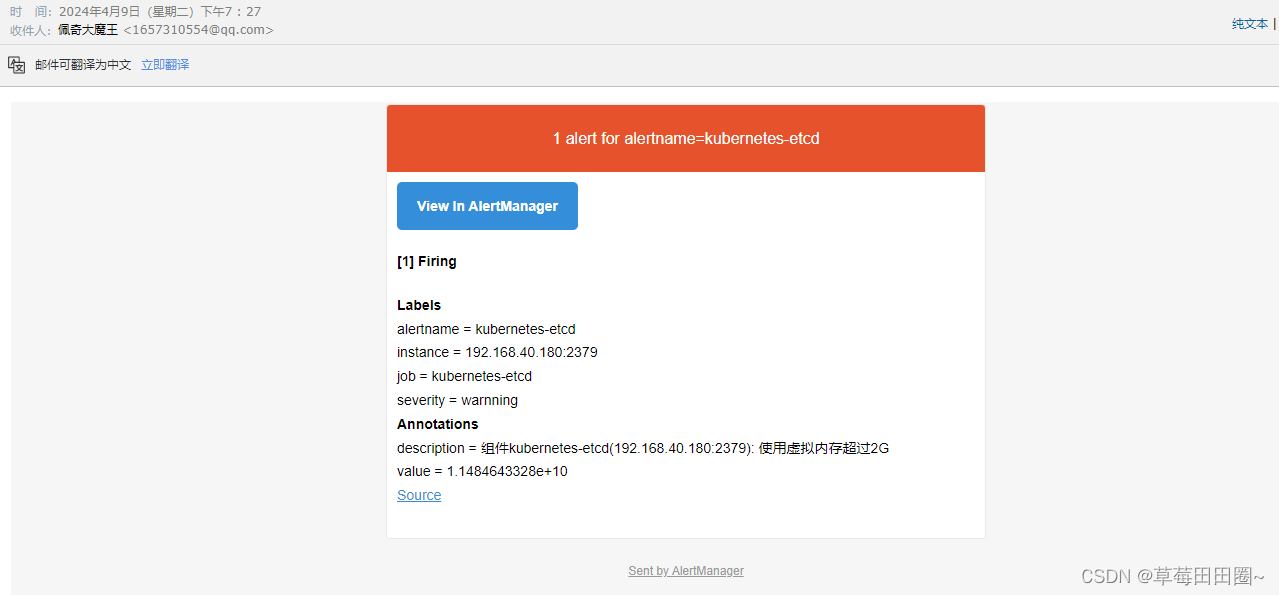
Prometheus接入AlterManager配置邮件告警(基于K8S环境部署)
目录 一.配置Alertmanager告警发送至邮箱二.Prometheus接入AlertManager三.部署PrometheusAlterManager(放到一个Pod中)四. 测试告警 基于 此环境做实验 一.配置Alertmanager告警发送至邮箱 1.创建AlertManager ConfigMap资源清单 vim alertmanager-cm.yaml --- kind: Confi…...
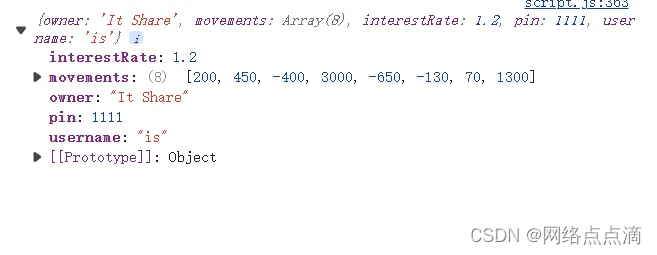
find方法
find() 方法用于在数组中查找符合条件的第一个元素,并返回该元素。如果找到匹配的元素,则返回该元素的值;如果未找到匹配的元素,则返回 undefined。 例如: const firstWithdrawal movements.find(mov > mov < 0); consol…...
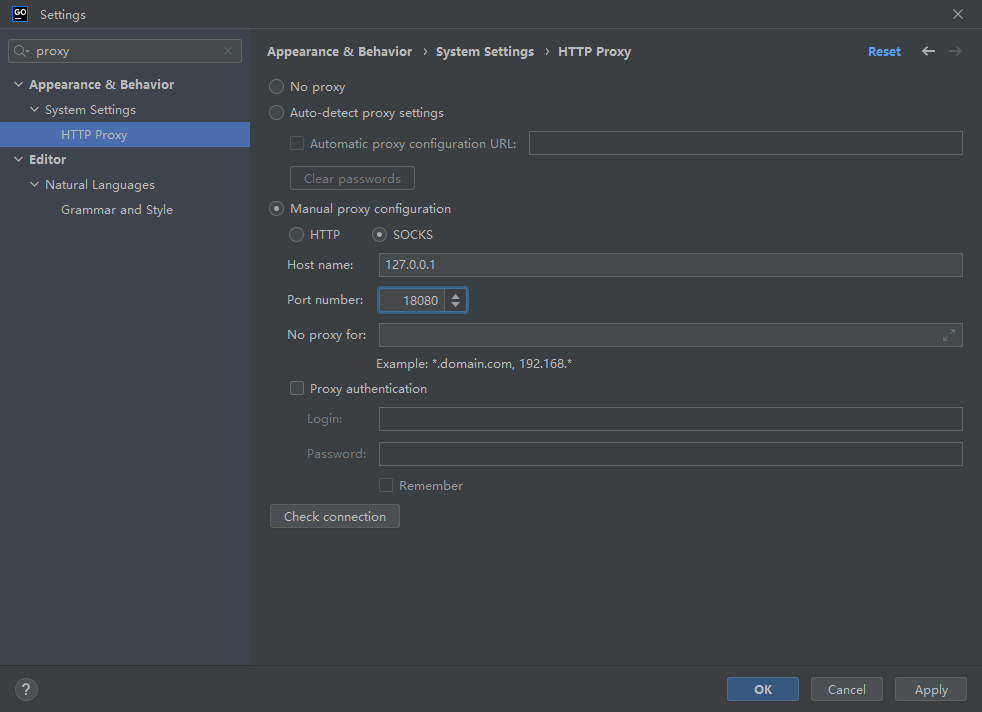
TLS v1.3 导致JetBrains IDE jdk.internal.net.http.common CPU占用高
开发环境 GoLand版本:2022.3.4 问题原因 JDK 中的 TLS v1.3 实现引起 解决办法 使用 SOCKS 代理代替HTTP代理 禁用 Space 和 Code With Me 插件 禁用 TLS v1.3,参考:https://stackoverflow.com/questions/54485755/java-11-httpclient-…...