C++中的priority_queue模拟实现
目录
priority_queue模拟实现
priority_queue类定义
priority_queue构造函数
priority_queue类push()函数
priority_queue类pop()函数
priority_queue类size()函数
priority_queue类empty()函数
priority_queue类top()函数
仿函数与priority_queue类模拟实现
仿函数
priority_queue模拟实现
实现priority_queue的方式和stack和queue基本类似,因为priority_queue也是一种容器适配器,但是只能使用vector和deque,这里默认使用vector容器作为适配器,因为priority_queue本质是堆结构,所以实现时主要按照堆的实现思路进行
priority_queue类定义
//vector作为默认的容器适配器
template<class T, class Container = vector<T>>
class priority_queue
{
private:Container _con:
}
priority_queue构造函数
priority_queue主要有两种构造函数:
- 无参构造函数
- 迭代器构造函数
📌
需要注意的是,如果不显式实现两个构造函数,则默认是无参构造,该默认构造将会调用容器适配器的构造函数
//有迭代器构造就必须写显式写空参构造
priority_queue():_con()
{}
//迭代器构造函数
template<class Iterator>
priority_queue(Iterator begin, Iterator end):_con(begin, end)
{//向下调整算法建堆for (int i = (size()-2)/2; i >=0 ; i--){adjustDown(i);}
}
priority_queue类push()
函数
因为按照堆实现思路进行,所以push()
函数实现思路如下:
- 向容器适配器中插入数据
- 通过向上/向下调整算法构建堆
//向上调整建堆(以大堆为例)
void adjustUp(int child)
{//通过孩子获取父亲int parent = (child - 1) / 2;while (child > 0){if (_con[child] > _con[parent]){swap(_con[child], _con[parent]);child = parent;parent = (child - 1) / 2;}else{break;}}
}
//向下调整算法
void adjustDown(int parent)
{//获取孩子int child = parent * 2 + 1;while (child < size()){//如果右孩子大于左孩子,更新当前的孩子为右孩子if (child + 1 < size() && _con[child] < _con[child + 1]){++child;}//调整if (_con[child] > _con[parent]){swap(_con[child], _con[parent]);parent = child;child = parent * 2 + 1;}else{break;}}
}
//push()函数
void push(const T& val)
{//调用指定容器的push_back()函数_con.push_back(val);//找到当前孩子的位置int child = _con.size() - 1;//向上调整建堆//adjustUp(child);//向下调整建堆//获取到最后一个孩子对应的父亲int parent = (child - 1) / 2;for (int i = parent; i >= 0; i--){adjustDown(i);}
}
priority_queue类pop()
函数
因为按照堆实现思路进行,所以pop()
函数实现思路如下:
- 交换根节点数据和最后一个叶子节点的数据
- 调用容器适配器的删除,除去最后一个数据
- 向下调整重新为堆
//pop()函数
void pop()
{//先交换堆顶数据和最后一个叶子节点数据swap(_con[0], _con[size() - 1]);_con.pop_back();//向下调整算法调整堆adjustDown(0);
}
priority_queue类size()
函数
//size()函数
const size_t size()
{return _con.size();
}
priority_queue类empty()
函数
//empty()函数
bool empty()
{return _con.empty();
}
priority_queue类top()
函数
//top()函数_const版本
const T& top() const
{return _con[0];
}//top()函数_非const版本
T& top()
{return _con[0];
}
仿函数与priority_queue类模拟实现
在前面的模拟实现中,priority_queue默认是小堆的实现,但是如果此时需要实现大堆,就需要改变向上/向下调整算法,但是这种实现方式不能在一个文件里面同时创建出小堆和大堆,所以此时需要一个函数来控制比较大小,此时就可以用到仿函数
仿函数简单介绍
所谓仿函数就是使用类并且重载()
运算符,例如对于比较两个数值,小于返回true
的仿函数
//仿函数
template<class T>
class less
{
public:bool operator()(const T& val1, const T& val2){return val1 < val2;}
};
同样地,可以实现一个比较两个数值,大于返回true
的仿函数
template<class T>
class greater
{
public:bool operator()(const T& val1, const T& val2){return val1 > val2;}
};
所以此时可以使用仿函数修改向上/向下调整算法
//向上调整建堆(以大堆为例)
void adjustUp(int child)
{//定义仿函数对象,调用对象函数Compare com;//通过孩子获取父亲int parent = (child - 1) / 2;while (child > 0){if (com(_con[parent], _con[child])){swap(_con[child], _con[parent]);child = parent;parent = (child - 1) / 2;}else{break;}}
}
//向下调整算法
void adjustDown(int parent)
{//定义仿函数对象,调用对象函数Compare com;//获取孩子int child = parent * 2 + 1;while (child < size()){//如果右孩子大于左孩子,更新当前的孩子为右孩子if (child + 1 < size() && com(_con[child], _con[child + 1])){++child;}//调整if (com(_con[parent], _con[child])){swap(_con[child], _con[parent]);parent = child;child = parent * 2 + 1;}else{break;}}
}
此时如果需要实现大堆,则只需要改变Compare
的类型即可,下面是小堆和大堆的测试代码
void test()
{//小堆sim_priority_queue::priority_queue<int> pq;//上面的代码等同于//sim_priority_queue::priority_queue<int, vector<int>, sim_priority_queue::less<int>> pq;pq.push(35);pq.push(70);pq.push(56);pq.push(90);pq.push(60);pq.push(25);while (!pq.empty()){cout << pq.top() << " ";pq.pop();}cout << endl;//大堆sim_priority_queue::priority_queue<int, vector<int>, sim_priority_queue::greater<int>> pq2;pq2.push(35);pq2.push(70);pq2.push(56);pq2.push(90);pq2.push(60);pq2.push(25);while (!pq2.empty()){cout << pq2.top() << " ";pq2.pop();}
}int main()
{test();
}
输出结果:
90 70 60 56 35 25
25 35 56 60 70 90
现在考虑前面sort
函数中的仿函数
默认情况下,使用sort
函数会对一段区间的内容进行升序排列,但是如果需要控制降序排列就需要用到仿函数
#include <iostream>
#include <vector>
#include <algorithm>using namespace std;int main()
{vector<int> v{30, 2, 45, 4, 46, 78, 11, 25};// 默认升序排列sort(v.begin(), v.end());for (auto num : v){cout << num << " ";}cout << endl;vector<int> v1{30, 2, 45, 4, 46, 78, 11, 25};// 使用仿函数匿名对象改为降序排列sort(v1.begin(), v1.end(), greater<int>());for (auto num : v1){cout << num << " ";}
}
输出结果:
2 4 11 25 30 45 46 78
78 46 45 30 25 11 4 2
📌
有了仿函数,除了可以使用内置的一些仿函数,也可自定义自己的仿函数来规定比较方式,这种对于自定义类型并且重载了比较运算符非常便捷
相关文章:
C++中的priority_queue模拟实现
目录 priority_queue模拟实现 priority_queue类定义 priority_queue构造函数 priority_queue类push()函数 priority_queue类pop()函数 priority_queue类size()函数 priority_queue类empty()函数 priority_queue类top()函数 仿函数与priority_queue类模拟实现 仿函数 …...
【Kafka】1.Kafka核心概念、应用场景、常见问题及异常
Kafka 是一个分布式流处理平台,最初由 LinkedIn 开发,后成为 Apache 软件基金会的顶级项目。 它主要用于构建实时数据管道和流式应用程序。它能够高效地处理高吞吐量的数据,并支持消息发布和订阅模型。Kafka 的主要用途包括实时分析、事件源、…...
LTE的EARFCN和band之间的对应关系
一、通过EARFCN查询对应band 工作中经常遇到只知道EARFCN而需要计算band的情况,因此查了相关协议,找到了他们之间的对应关系,可以直接查表,非常方便。 具体见: 3GPP TS 36.101 5.7.3 Carrier frequency and EAR…...

解决问题:Docker证书到期(Error grabbing logs: rpc error: code = Unknown)导致无法查看日志
问题描述 Docker查看日志时portainer报错信息如下: Error grabbing logs: rpc error: code Unknown desc warning: incomplete log stream. some logs could not be retrieved for the following reasons: node klf9fdsjjt5tb0w4hxgr4s231 is not available报错…...
【C语言】预处理器
我们在开始编写一份程序的时候,从键盘录入的第一行代码: #include <stdio.h>这里就使用了预处理,引入头文件。 C预处理器不是编译器的组成部分,但是它是编译过程中一个单独的步骤。简言之,C预处理器只不过是一…...
QtConcurrent::run操作界面ui的注意事项(2)
前面的“QtConcurrent::run操作界面ui的注意事项(1)”,末尾说了跨线程的问题,引出了Qt千好万好,就是跨线程不好。下面是认为的最简单的解决办法:使用QMetaObject::invokeMethod(相比较信号-槽&a…...
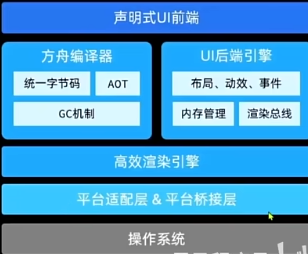
黑马程序员HarmonyOS4+NEXT星河版入门到企业级实战教程笔记
HarmonyOS NEXT是纯血鸿蒙,鸿蒙原生应用,彻底摆脱安卓 本课程是基于harmony os4的,与next仅部分api有区别 套件 语言&框架 harmony os design ArkTs 语言 ArkUI 提供各种组件 ArkCompiler 方舟编译器 开发&测试 DevEco Studio 开发…...

嵌入式全栈开发学习笔记---C语言笔试复习大全13(编程题9~16)
目录 9.查找字符数组中字符位置(输入hello e 输出2); 10、查找字符数组中字符串的位置(输入hello ll 输出3); 11、字符数组中在指定位置插入字符;(输入hello 3 a 输出heallo…...

https网站安全证书的作用与免费申请办法
HTTPS网站安全证书,也称为SSL证书,网站通过申请SSL证书将http协议升级到https协议 HTTPS网站安全证书的作用 1 增强用户信任:未使用https协议的网站,用户访问时浏览器会有“不安全”弹窗提示 2 提升SEO排名:搜索引擎…...
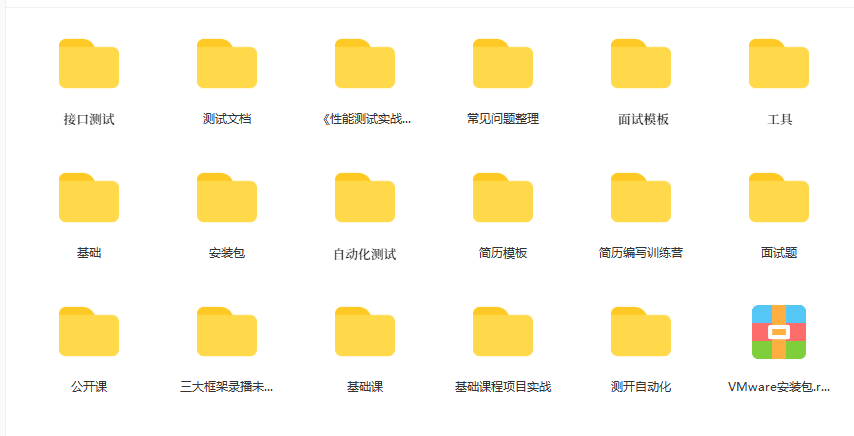
自动化测试再升级,大模型与软件测试相结合
近年来,软件行业一直在迅速发展,为了保证软件质量和提高效率,软件测试领域也在不断演进。如今,大模型技术的崛起为软件测试带来了前所未有的智能化浪潮。 软件测试一直是确保软件质量的关键环节,但传统的手动测试方法存…...
centos7 基础命令
一、基础信息: 查看IP地址: ip add 重启网络服务: service network restart 查看网卡配置: cat /etc/sysconfig/network-scripts/ifcfg-ens33 启动网卡: ifup ens33 查看内存: free -m 查看CPU: cat /proc/cpuin…...
【设计模式】之单例模式
系列文章目录 【设计模式】之责任链模式【设计模式】之策略模式【设计模式】之模板方法模式 文章目录 系列文章目录 前言 一、什么是单例模式 二、如何使用单例模式 1.单线程使用 2.多线程使用(一) 3.多线程使用(二) 4.多线程使用…...
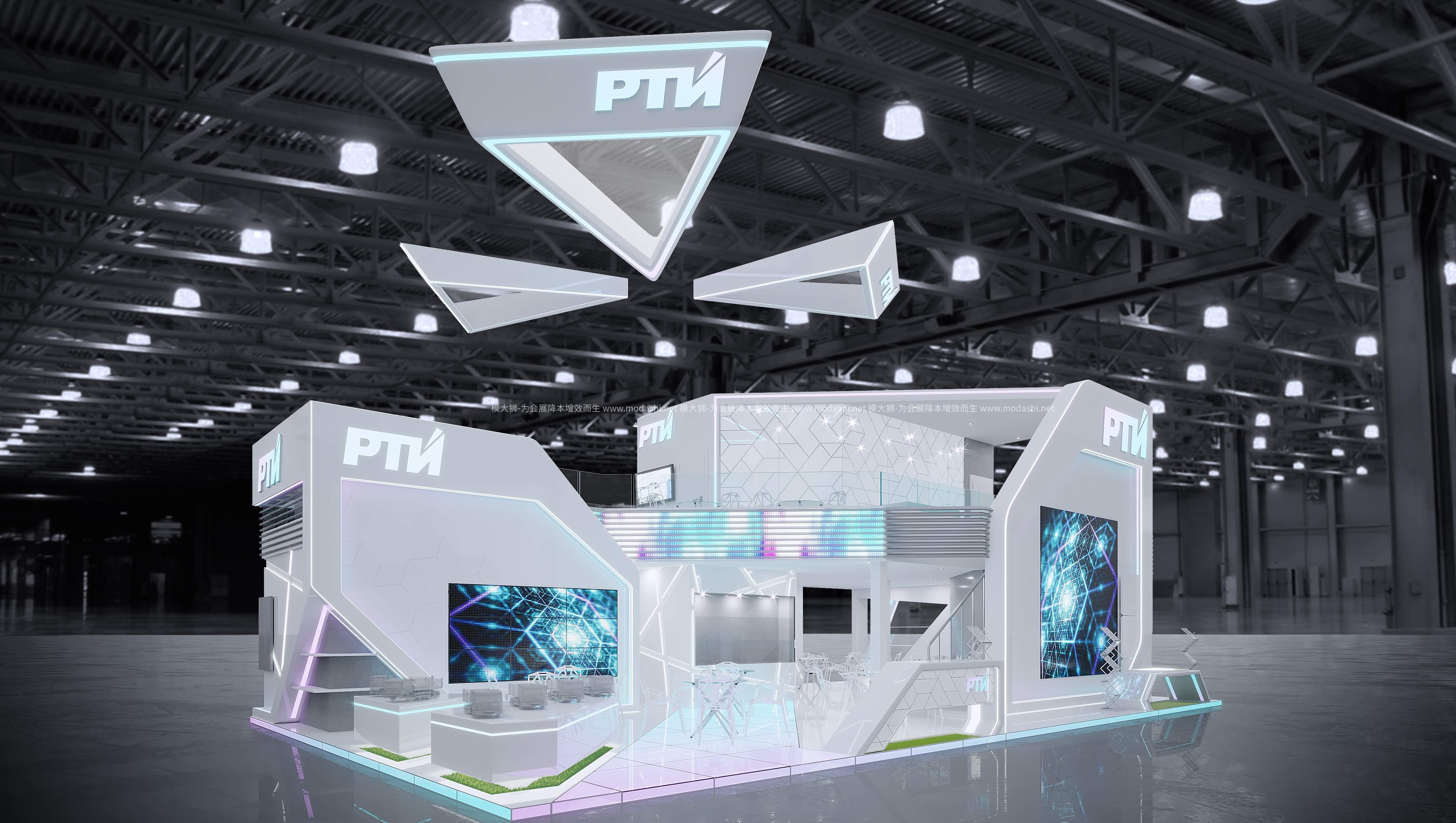
3d模型实体显示有隐藏黑线?---模大狮模型网
在3D建模和设计领域,细节决定成败。然而,在处理3D模型时,可能会遇到模型实体上出现隐藏黑线的问题。这些黑线可能影响模型的视觉质量和呈现效果。因此,了解并解决这些隐藏黑线的问题至关重要。本文将探讨隐藏黑线出现的原因&#…...
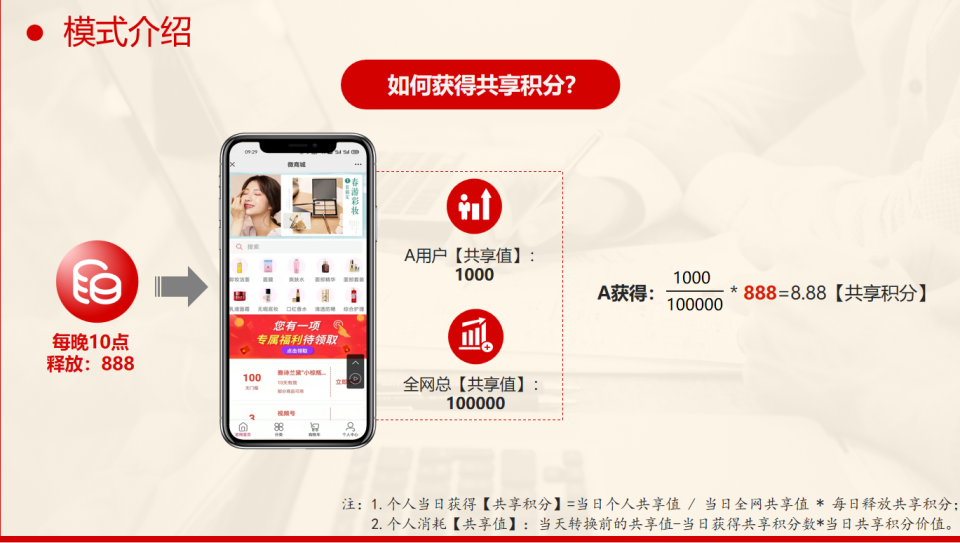
共享购:全新消费模式的探索与实践
在消费模式日益创新的今天,共享购模式以其独特的消费与收益双重机制,吸引了众多消费者的目光。这一模式不仅为消费者带来了全新的购物体验,也为商家和平台带来了可观的收益。 一、会员体系:共享购的基石 在共享购模式下ÿ…...
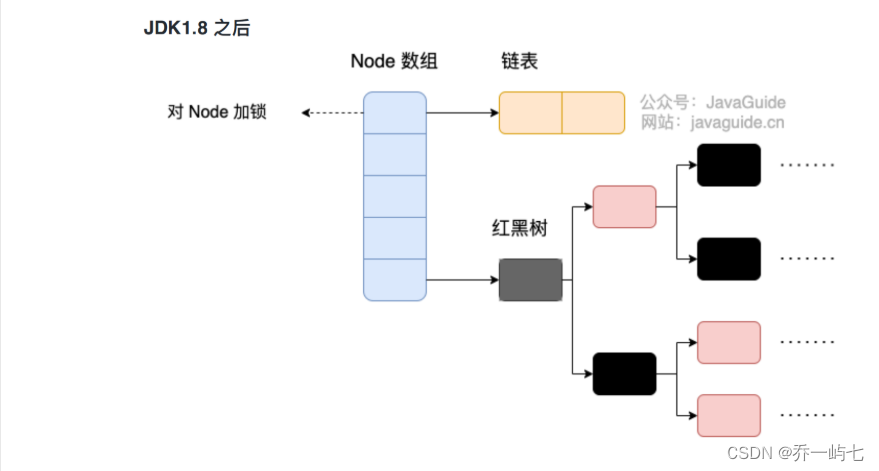
Java集合 总结篇(全)
Java集合 集合底层框架总结 List 代表的有序,可重复的集合。 ArrayList -- 数组 -- 把他想象成C中的Vector就可以,当数组空间不够的时候,会自动扩容。 -- 线程不安全 LinkedList -- 双向链表 -- 可以将他理解成一个链表,不支持…...
Dubbo分层架构深度解析
引言 Dubbo作为一款备受欢迎的高性能、轻量级的Java RPC框架,在现代分布式系统中扮演着至关重要的角色。随着互联网行业的快速发展,服务间的通信变得越来越频繁,这也使得对于高效、可靠的远程通信方案的需求变得愈发迫切。在这样的背景下&am…...
LocalDate 数据库不兼容问题,因为LocalDate 是 long 类型的
我今天遇到一报错: SqlSession [org.apache.ibatis.session.defaults.DefaultSqlSession316f9272] was not registered for synchronization because synchronization is not active JDBC Connection [HikariProxyConnection2127597288 wrapping com.mysql.cj.jdbc…...
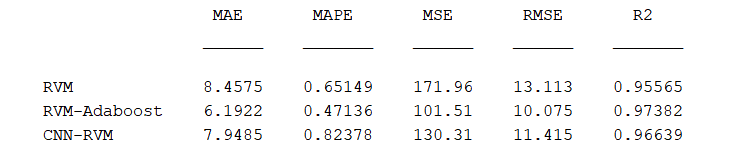
RVM(相关向量机)、CNN_RVM(卷积神经网络结合相关向量机)、RVM-Adaboost(相关向量机结合Adaboost)
当我们谈到RVM(Relevance Vector Machine,相关向量机)、CNN_RVM(卷积神经网络结合相关向量机)以及RVM-Adaboost(相关向量机结合AdaBoost算法)时,每种模型都有其独特的原理和结构。以…...
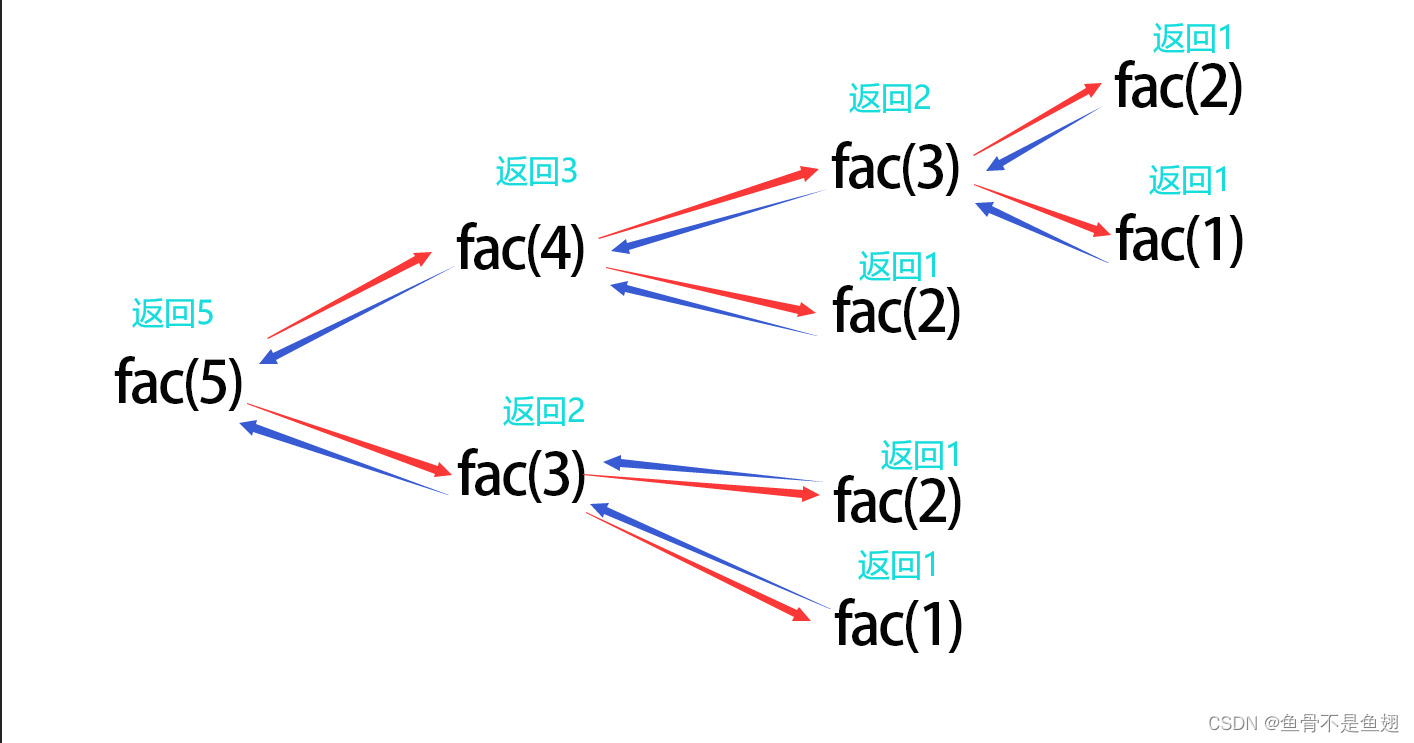
Java--方法的使用
1.1什么是方法 方法顾名思义就是解决问题的办法,在程序员写代码的时候,会遇到很多逻辑结构一样,解决相同问题时,每次都写一样的代码,这会使代码看起来比较绒余,代码量也比较多,为了解决这个问题…...
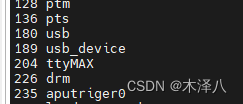
linux - 主次设备号自动申请
alloc_chrdev_region 原型如下,该函数向内核申请一个空闲的主设备号。 alloc_chrdev_region(&g_aputriger_dev, 0, APUTRIGER_MAX_NUM, "aputriger0"); 第四个参数是我们使用cat /proc/devices 看到的名称 /*** alloc_chrdev_region() - register a…...

使用VSCode开发Django指南
使用VSCode开发Django指南 一、概述 Django 是一个高级 Python 框架,专为快速、安全和可扩展的 Web 开发而设计。Django 包含对 URL 路由、页面模板和数据处理的丰富支持。 本文将创建一个简单的 Django 应用,其中包含三个使用通用基本模板的页面。在此…...
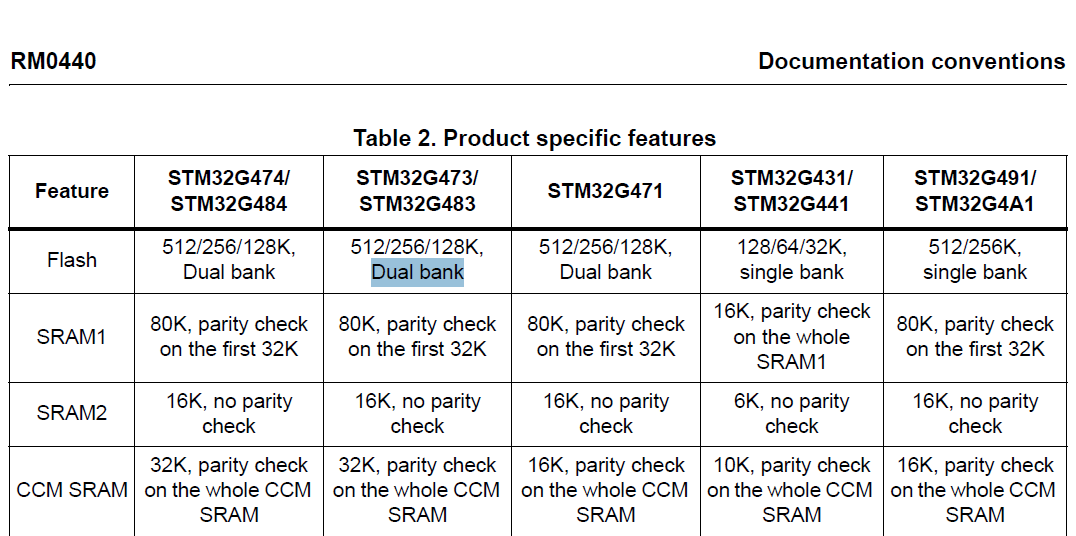
stm32G473的flash模式是单bank还是双bank?
今天突然有人stm32G473的flash模式是单bank还是双bank?由于时间太久,我真忘记了。搜搜发现,还真有人和我一样。见下面的链接:https://shequ.stmicroelectronics.cn/forum.php?modviewthread&tid644563 根据STM32G4系列参考手…...
Leetcode 3577. Count the Number of Computer Unlocking Permutations
Leetcode 3577. Count the Number of Computer Unlocking Permutations 1. 解题思路2. 代码实现 题目链接:3577. Count the Number of Computer Unlocking Permutations 1. 解题思路 这一题其实就是一个脑筋急转弯,要想要能够将所有的电脑解锁&#x…...
React---day11
14.4 react-redux第三方库 提供connect、thunk之类的函数 以获取一个banner数据为例子 store: 我们在使用异步的时候理应是要使用中间件的,但是configureStore 已经自动集成了 redux-thunk,注意action里面要返回函数 import { configureS…...
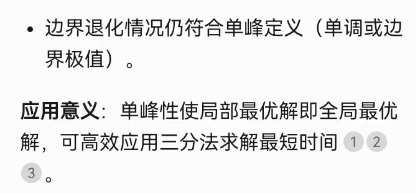
三分算法与DeepSeek辅助证明是单峰函数
前置 单峰函数有唯一的最大值,最大值左侧的数值严格单调递增,最大值右侧的数值严格单调递减。 单谷函数有唯一的最小值,最小值左侧的数值严格单调递减,最小值右侧的数值严格单调递增。 三分的本质 三分和二分一样都是通过不断缩…...
Caliper 负载(Workload)详细解析
Caliper 负载(Workload)详细解析 负载(Workload)是 Caliper 性能测试的核心部分,它定义了测试期间要执行的具体合约调用行为和交易模式。下面我将全面深入地讲解负载的各个方面。 一、负载模块基本结构 一个典型的负载模块(如 workload.js)包含以下基本结构: use strict;/…...
掌握 HTTP 请求:理解 cURL GET 语法
cURL 是一个强大的命令行工具,用于发送 HTTP 请求和与 Web 服务器交互。在 Web 开发和测试中,cURL 经常用于发送 GET 请求来获取服务器资源。本文将详细介绍 cURL GET 请求的语法和使用方法。 一、cURL 基本概念 cURL 是 "Client URL" 的缩写…...
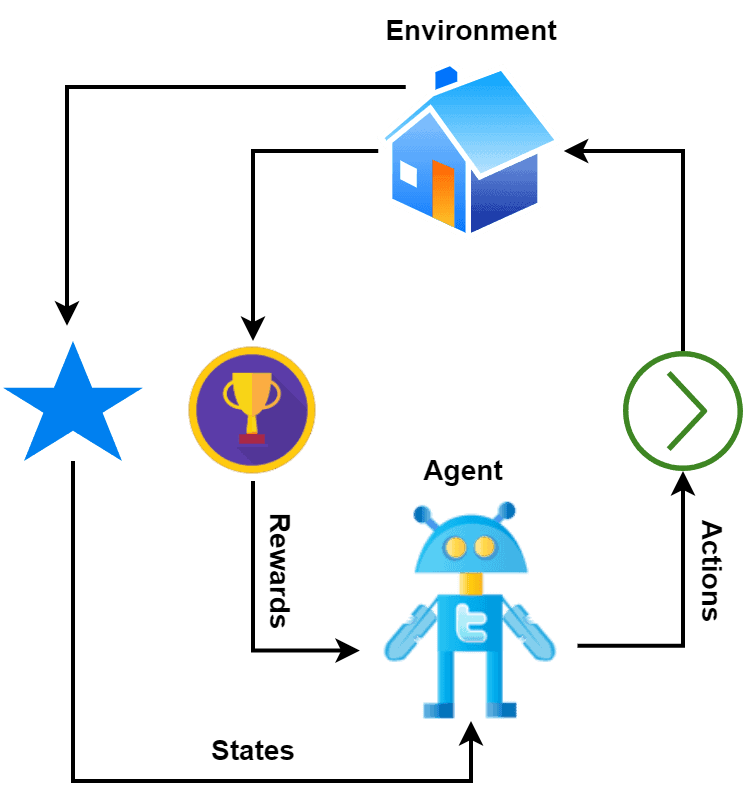
【深度学习新浪潮】什么是credit assignment problem?
Credit Assignment Problem(信用分配问题) 是机器学习,尤其是强化学习(RL)中的核心挑战之一,指的是如何将最终的奖励或惩罚准确地分配给导致该结果的各个中间动作或决策。在序列决策任务中,智能体执行一系列动作后获得一个最终奖励,但每个动作对最终结果的贡献程度往往…...
在golang中如何将已安装的依赖降级处理,比如:将 go-ansible/v2@v2.2.0 更换为 go-ansible/@v1.1.7
在 Go 项目中降级 go-ansible 从 v2.2.0 到 v1.1.7 具体步骤: 第一步: 修改 go.mod 文件 // 原 v2 版本声明 require github.com/apenella/go-ansible/v2 v2.2.0 替换为: // 改为 v…...
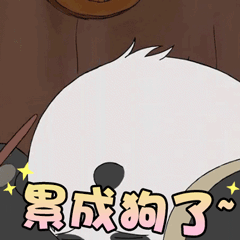
rm视觉学习1-自瞄部分
首先先感谢中南大学的开源,提供了很全面的思路,减少了很多基础性的开发研究 我看的阅读的是中南大学FYT战队开源视觉代码 链接:https://github.com/CSU-FYT-Vision/FYT2024_vision.git 1.框架: 代码框架结构:readme有…...